CIS 234 Sorting Dr Ralph D Westfall May
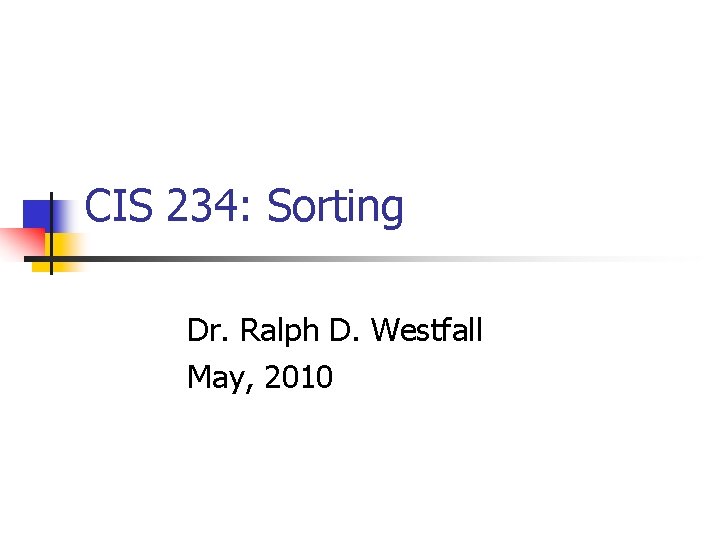
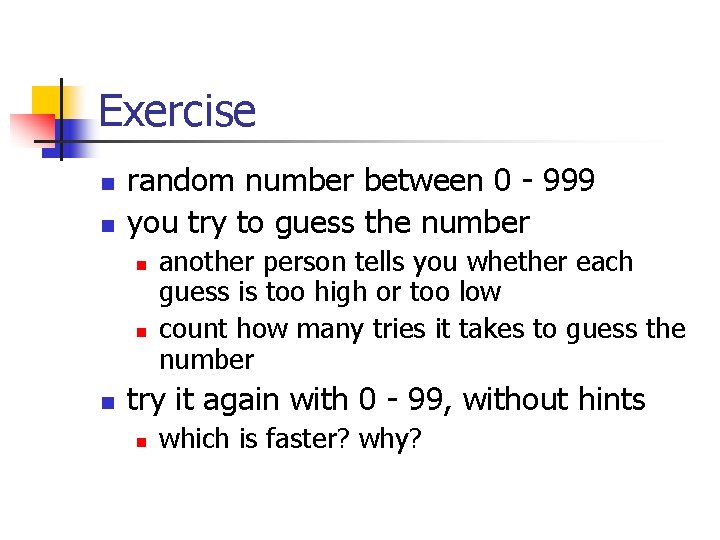
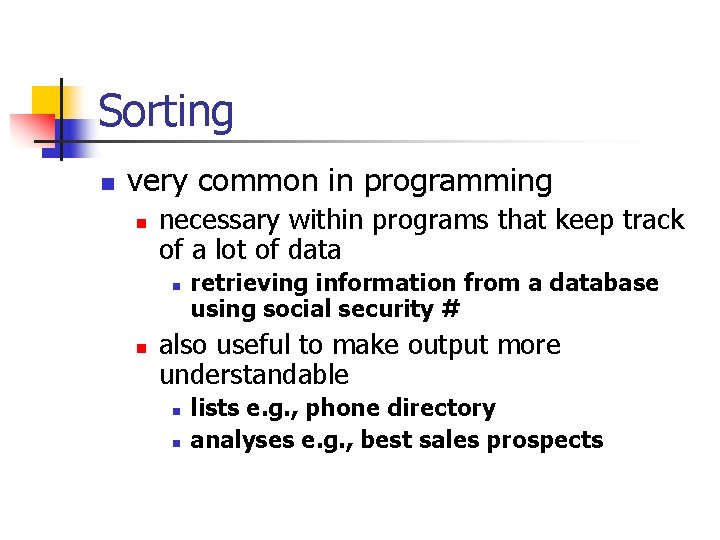
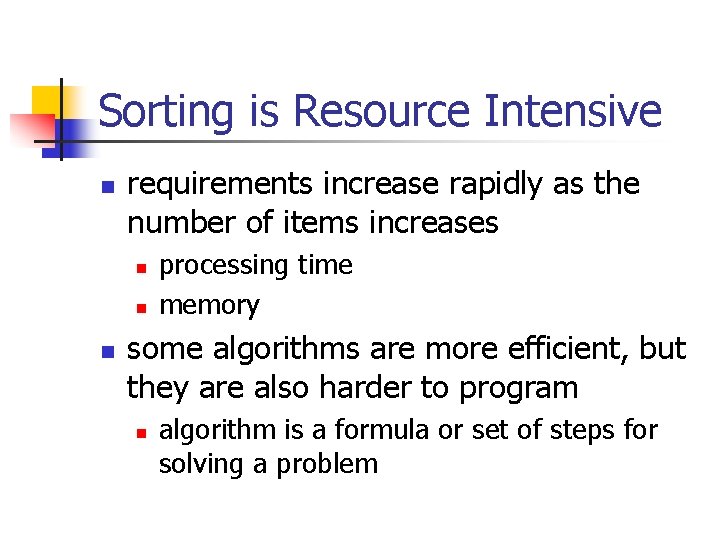
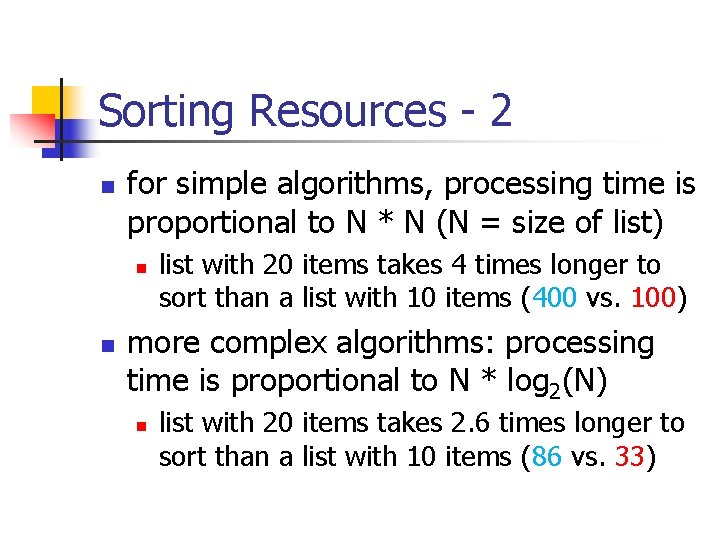
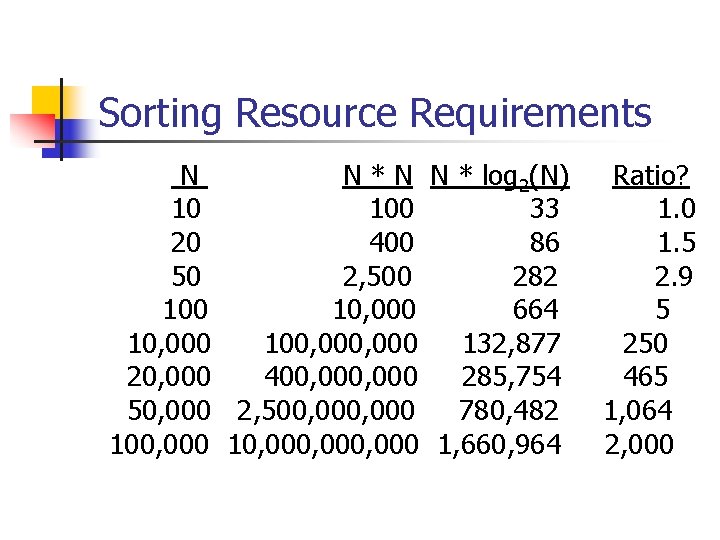
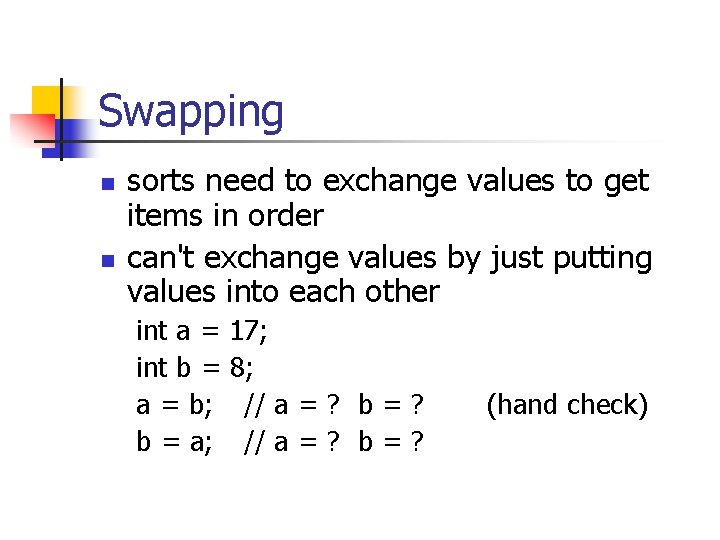
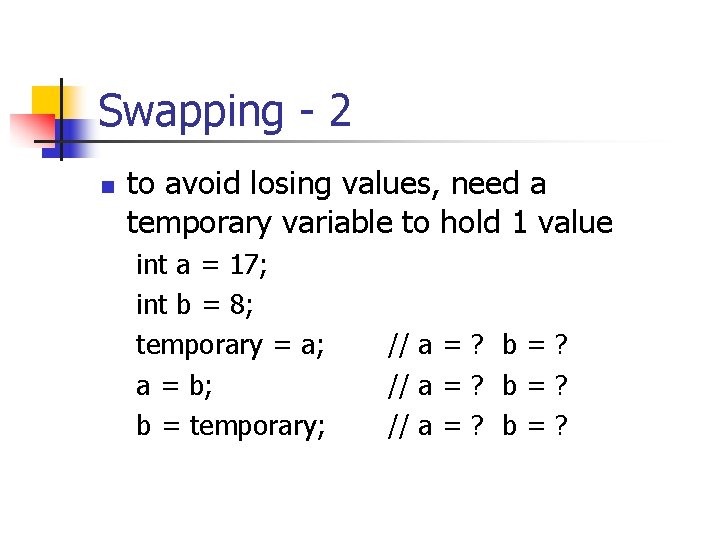
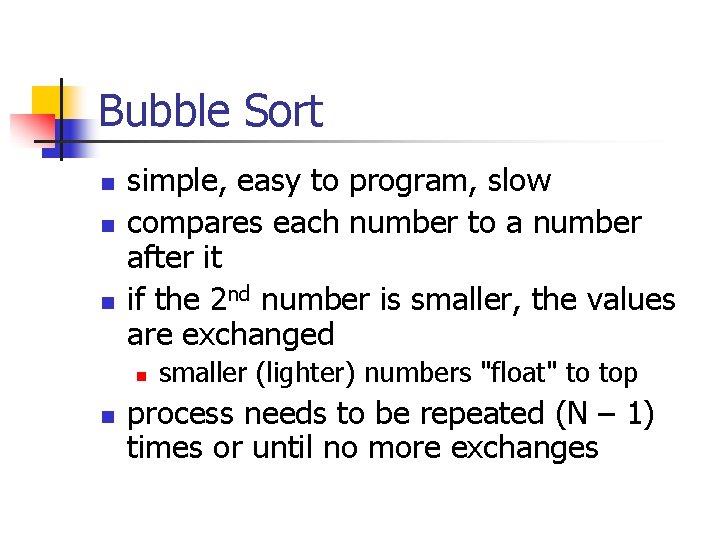
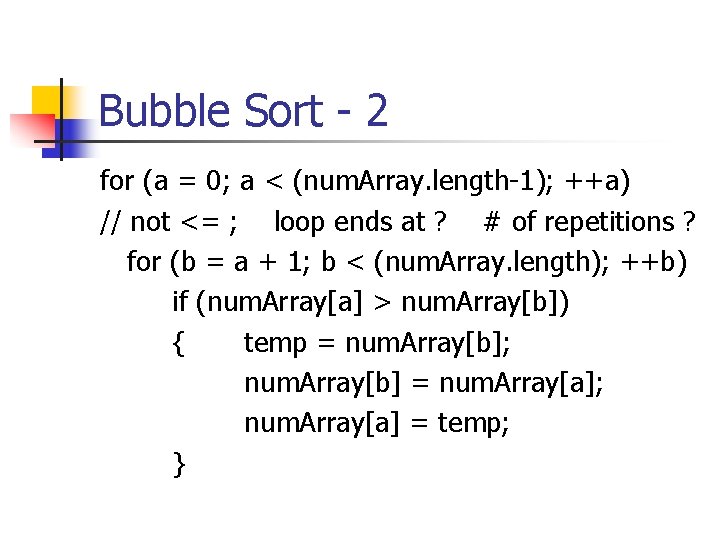
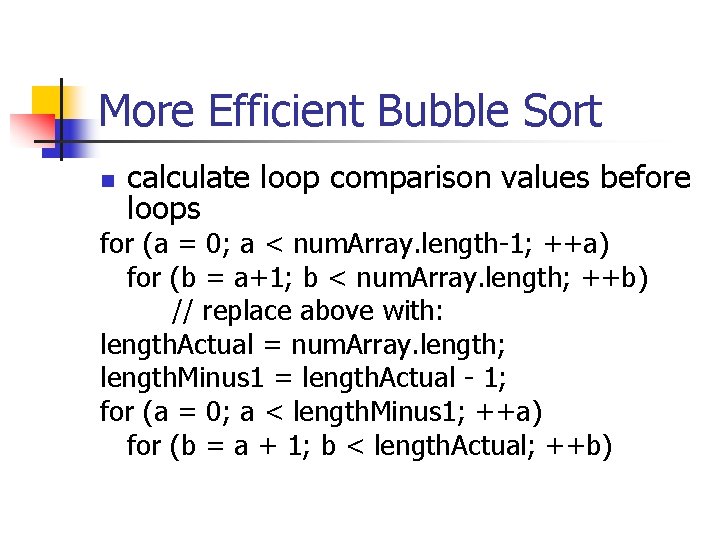
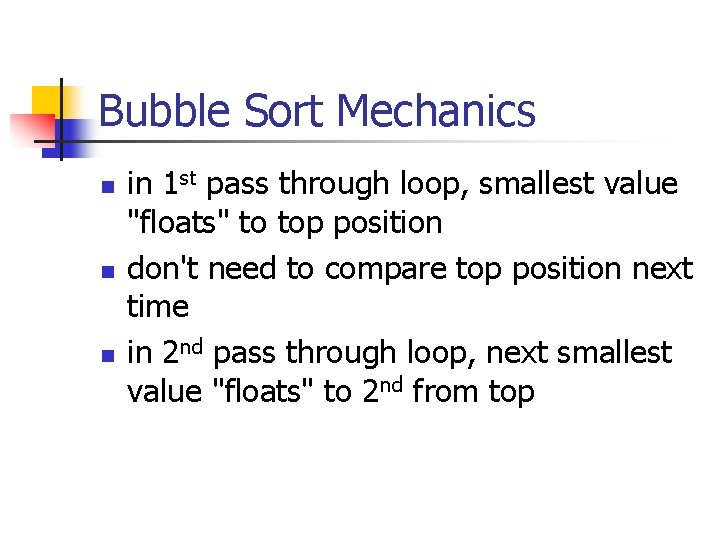
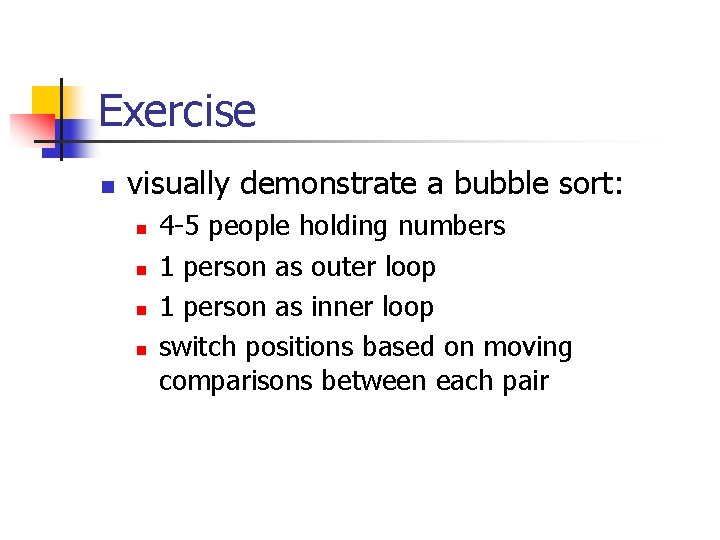
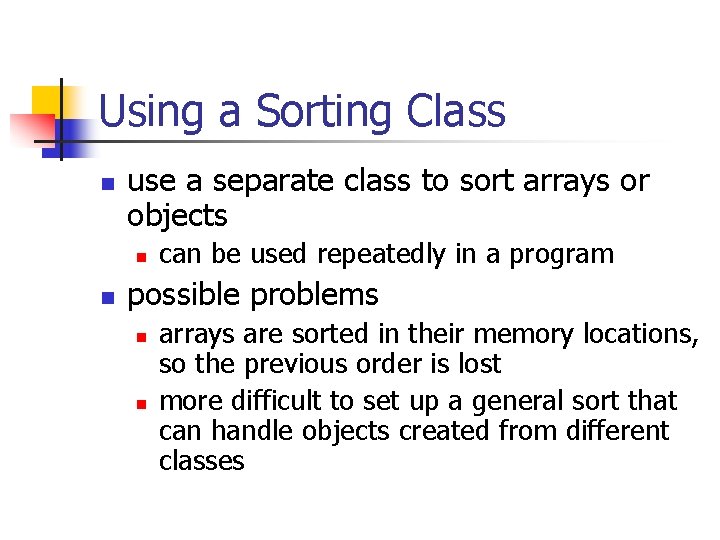
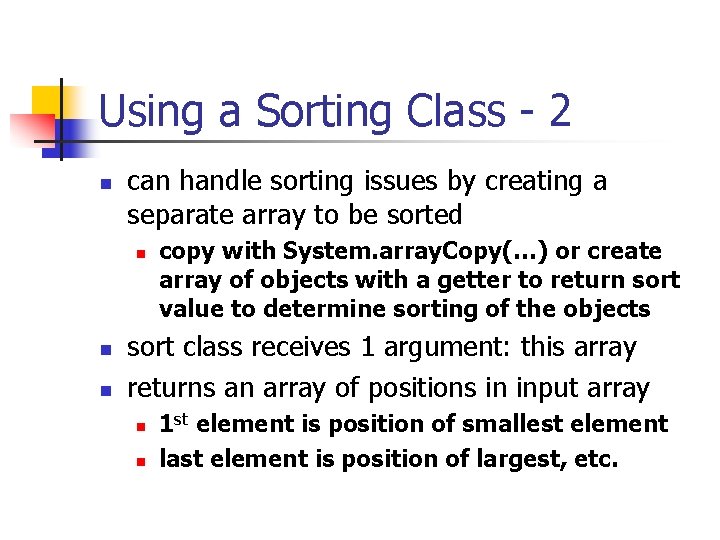
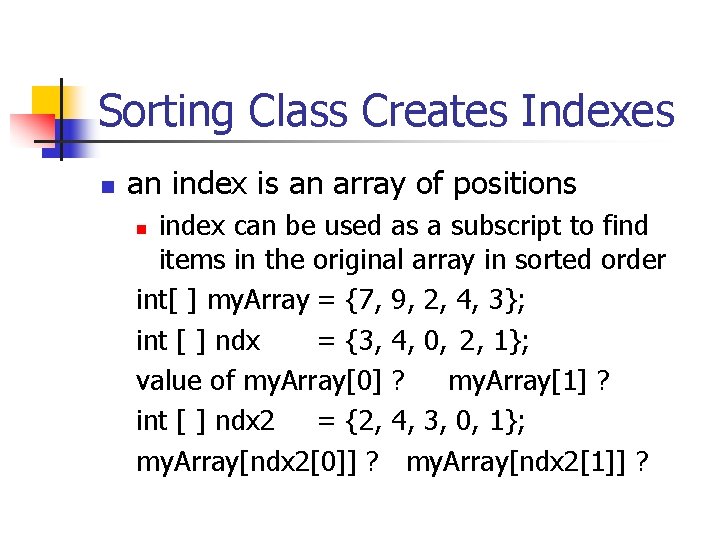
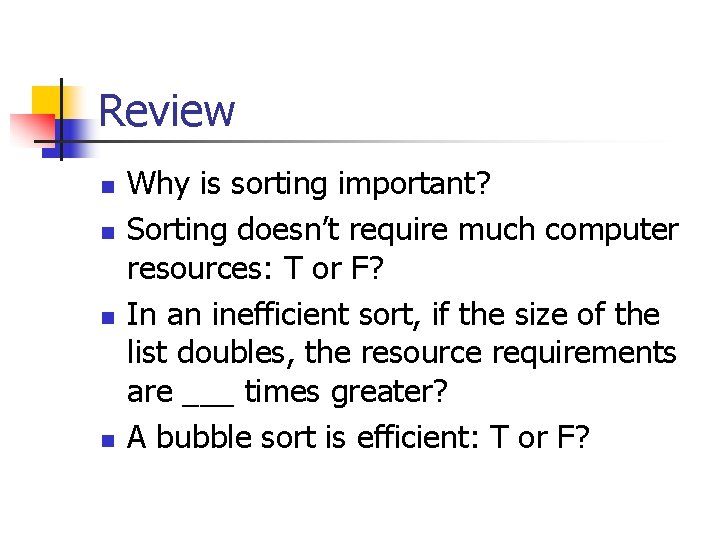
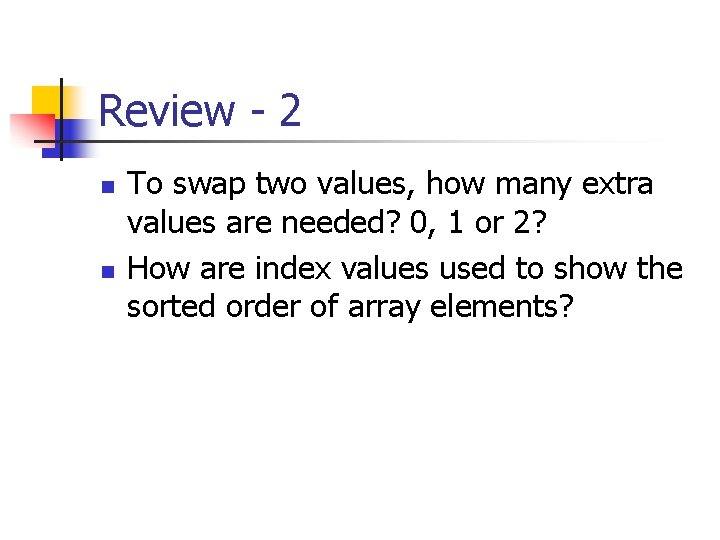
- Slides: 18
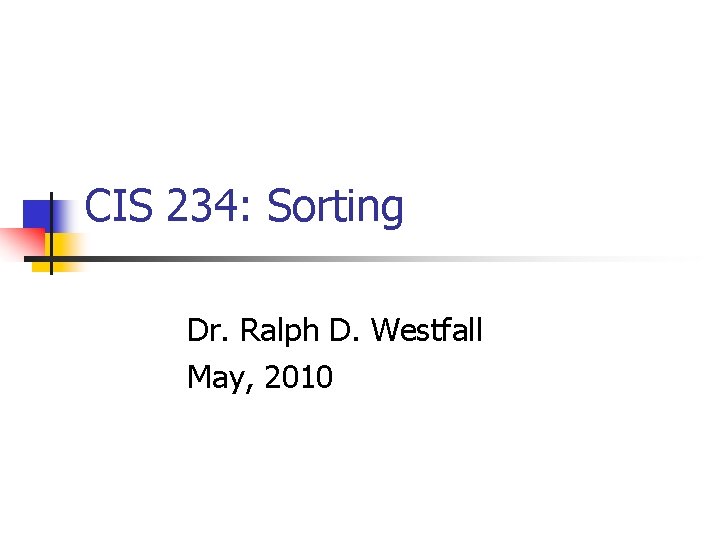
CIS 234: Sorting Dr. Ralph D. Westfall May, 2010
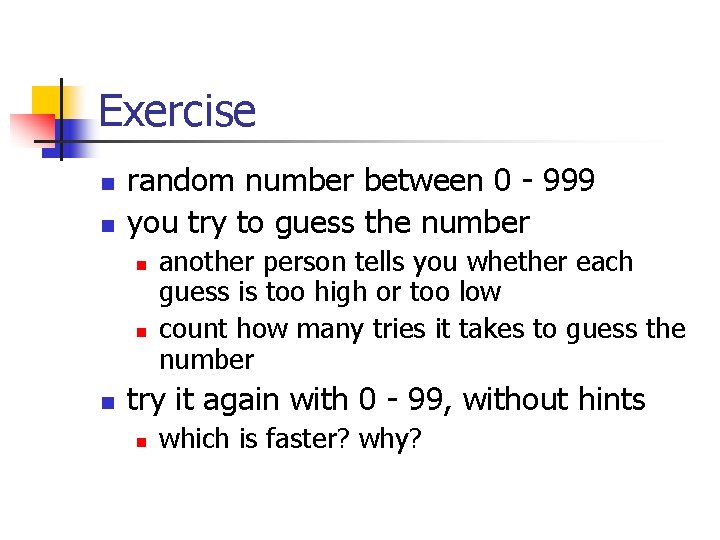
Exercise n n random number between 0 - 999 you try to guess the number n n n another person tells you whether each guess is too high or too low count how many tries it takes to guess the number try it again with 0 - 99, without hints n which is faster? why?
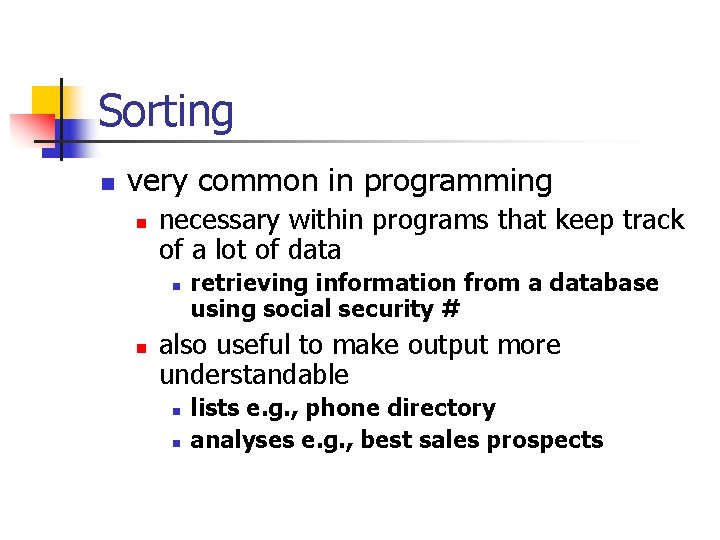
Sorting n very common in programming n necessary within programs that keep track of a lot of data n n retrieving information from a database using social security # also useful to make output more understandable n n lists e. g. , phone directory analyses e. g. , best sales prospects
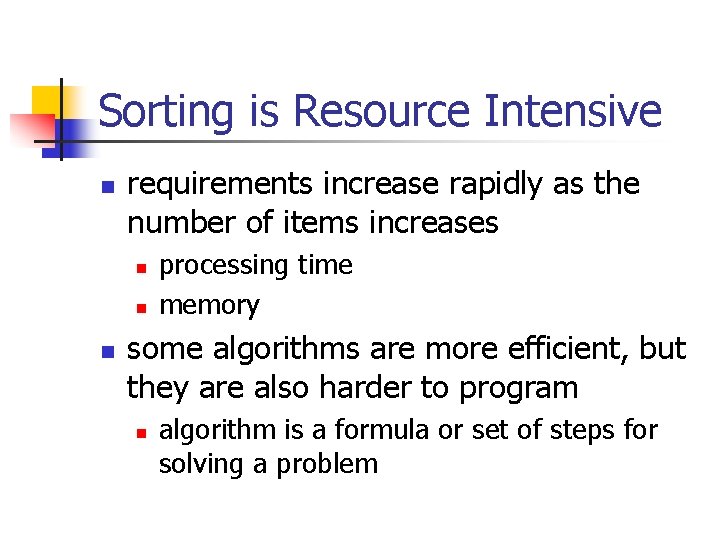
Sorting is Resource Intensive n requirements increase rapidly as the number of items increases n n n processing time memory some algorithms are more efficient, but they are also harder to program n algorithm is a formula or set of steps for solving a problem
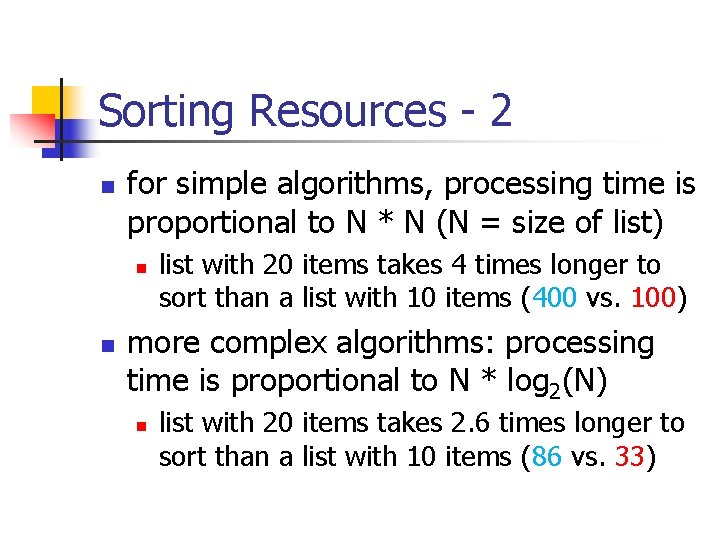
Sorting Resources - 2 n for simple algorithms, processing time is proportional to N * N (N = size of list) n n list with 20 items takes 4 times longer to sort than a list with 10 items (400 vs. 100) more complex algorithms: processing time is proportional to N * log 2(N) n list with 20 items takes 2. 6 times longer to sort than a list with 10 items (86 vs. 33)
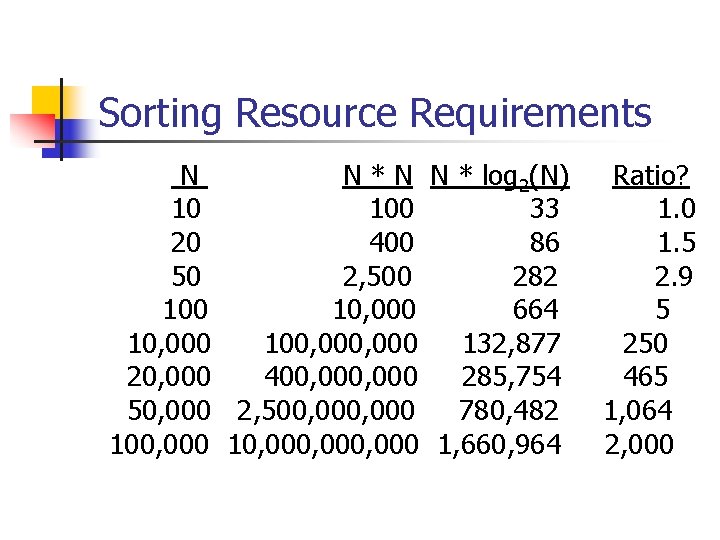
Sorting Resource Requirements N N * log 2(N) 10 100 33 20 400 86 50 2, 500 282 100 10, 000 664 10, 000 100, 000 132, 877 20, 000 400, 000 285, 754 50, 000 2, 500, 000 780, 482 100, 000 10, 000, 000 1, 660, 964 Ratio? 1. 0 1. 5 2. 9 5 250 465 1, 064 2, 000
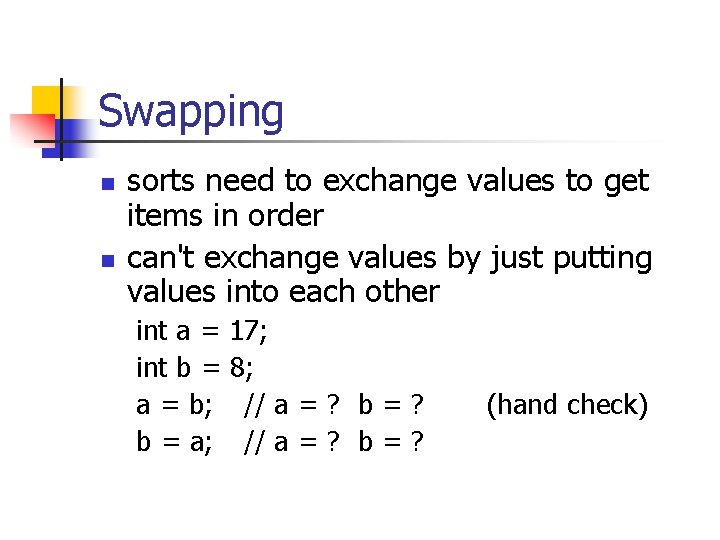
Swapping n n sorts need to exchange values to get items in order can't exchange values by just putting values into each other int a = 17; int b = 8; a = b; // a = ? b = a; // a = ? b = ? (hand check)
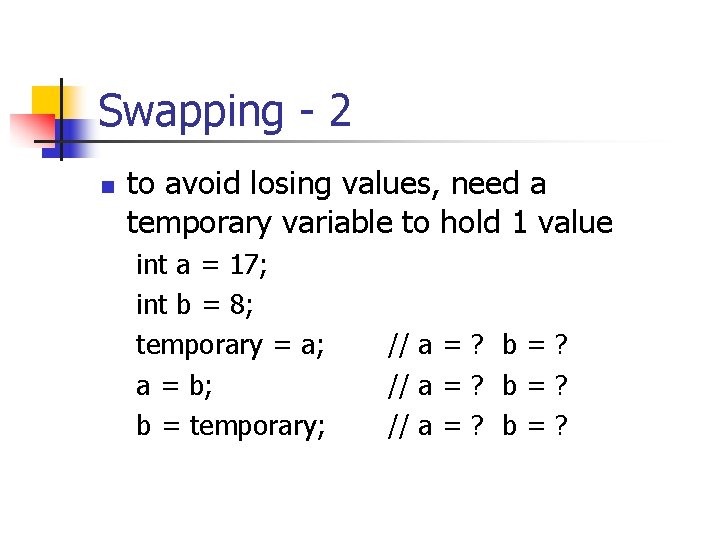
Swapping - 2 n to avoid losing values, need a temporary variable to hold 1 value int a = 17; int b = 8; temporary = a; a = b; b = temporary; // a = ? b = ?
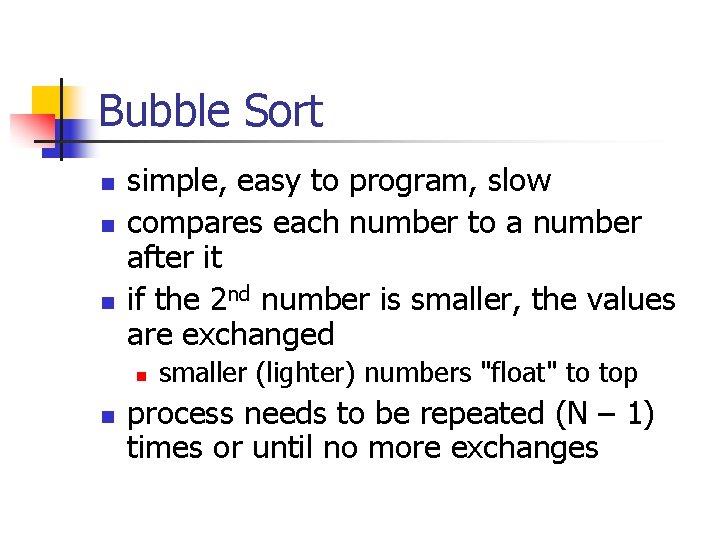
Bubble Sort n n n simple, easy to program, slow compares each number to a number after it if the 2 nd number is smaller, the values are exchanged n n smaller (lighter) numbers "float" to top process needs to be repeated (N – 1) times or until no more exchanges
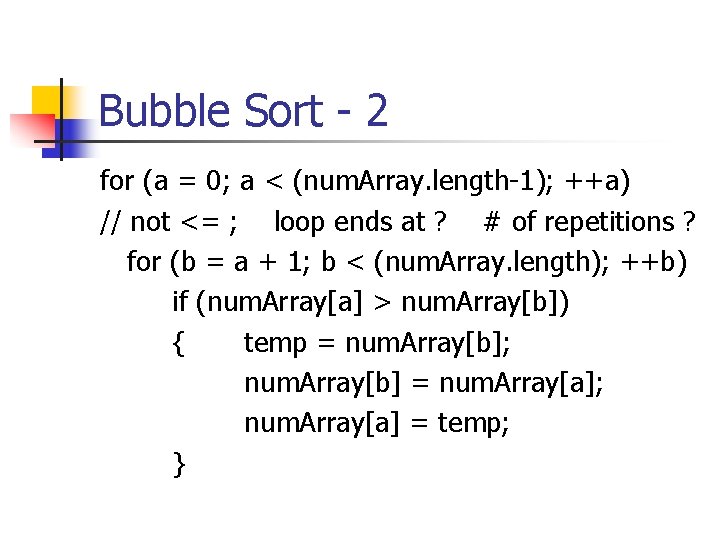
Bubble Sort - 2 for (a = 0; a < (num. Array. length-1); ++a) // not <= ; loop ends at ? # of repetitions ? for (b = a + 1; b < (num. Array. length); ++b) if (num. Array[a] > num. Array[b]) { temp = num. Array[b]; num. Array[b] = num. Array[a]; num. Array[a] = temp; }
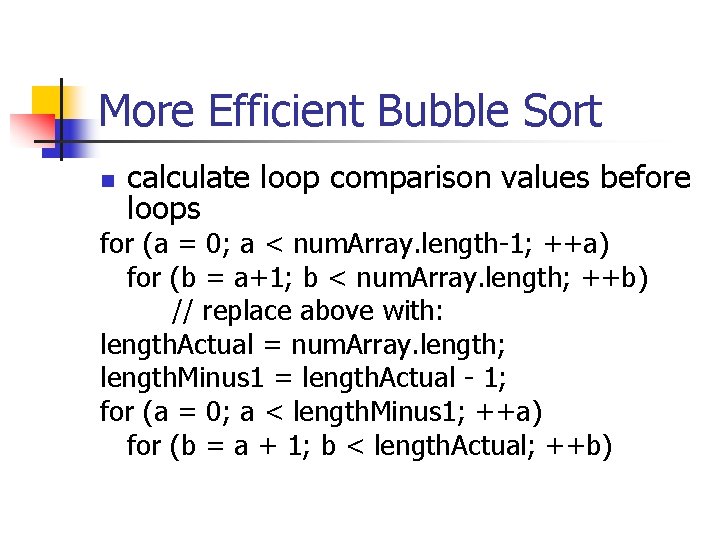
More Efficient Bubble Sort n calculate loop comparison values before loops for (a = 0; a < num. Array. length-1; ++a) for (b = a+1; b < num. Array. length; ++b) // replace above with: length. Actual = num. Array. length; length. Minus 1 = length. Actual - 1; for (a = 0; a < length. Minus 1; ++a) for (b = a + 1; b < length. Actual; ++b)
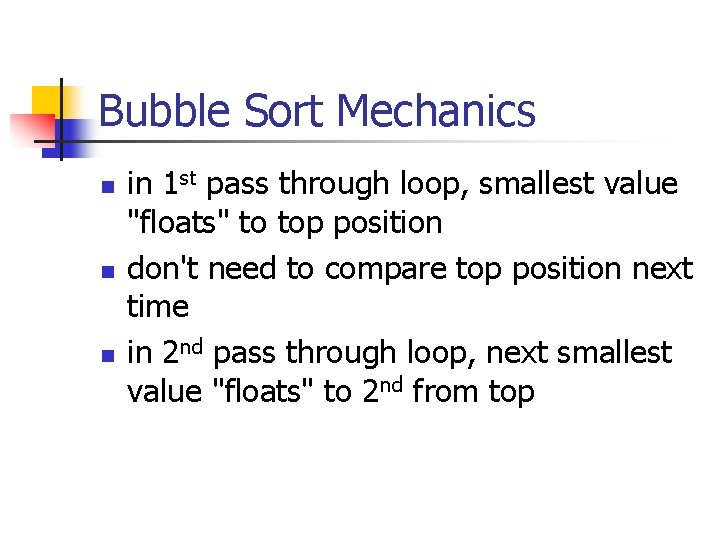
Bubble Sort Mechanics n n n in 1 st pass through loop, smallest value "floats" to top position don't need to compare top position next time in 2 nd pass through loop, next smallest value "floats" to 2 nd from top
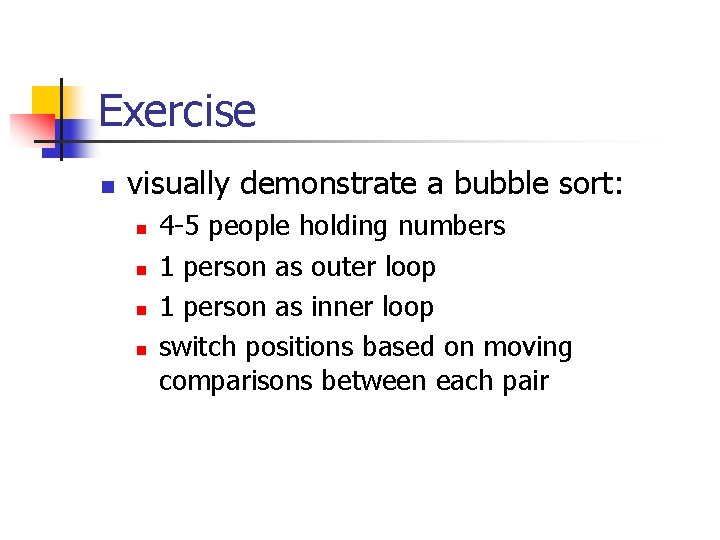
Exercise n visually demonstrate a bubble sort: n n 4 -5 people holding numbers 1 person as outer loop 1 person as inner loop switch positions based on moving comparisons between each pair
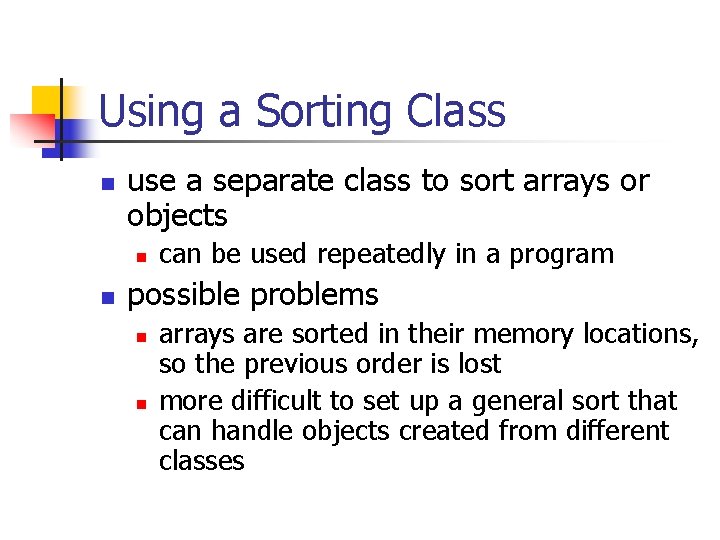
Using a Sorting Class n use a separate class to sort arrays or objects n n can be used repeatedly in a program possible problems n n arrays are sorted in their memory locations, so the previous order is lost more difficult to set up a general sort that can handle objects created from different classes
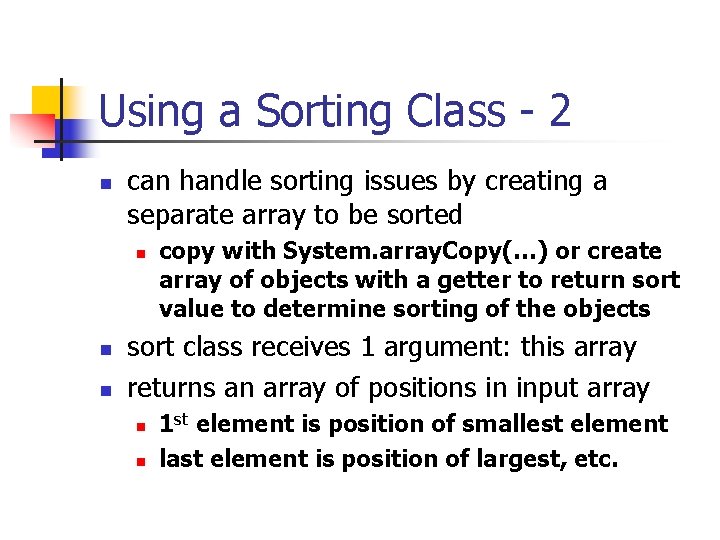
Using a Sorting Class - 2 n can handle sorting issues by creating a separate array to be sorted n n n copy with System. array. Copy(…) or create array of objects with a getter to return sort value to determine sorting of the objects sort class receives 1 argument: this array returns an array of positions in input array n n 1 st element is position of smallest element last element is position of largest, etc.
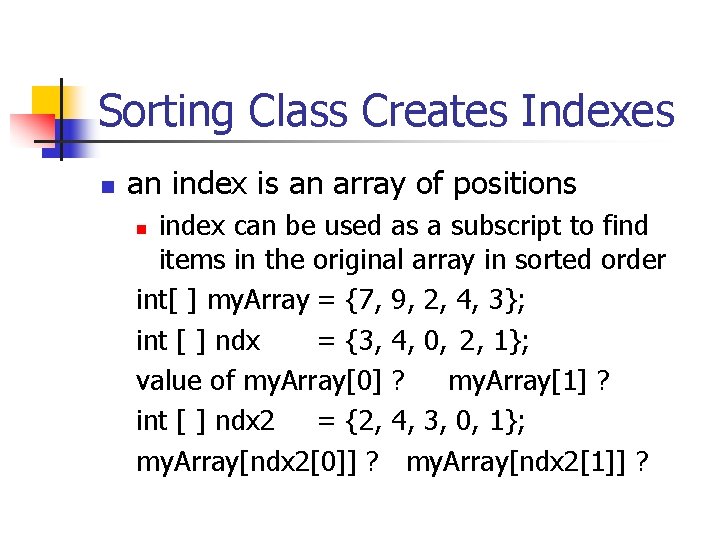
Sorting Class Creates Indexes n an index is an array of positions index can be used as a subscript to find items in the original array in sorted order int[ ] my. Array = {7, 9, 2, 4, 3}; int [ ] ndx = {3, 4, 0, 2, 1}; value of my. Array[0] ? my. Array[1] ? int [ ] ndx 2 = {2, 4, 3, 0, 1}; my. Array[ndx 2[0]] ? my. Array[ndx 2[1]] ? n
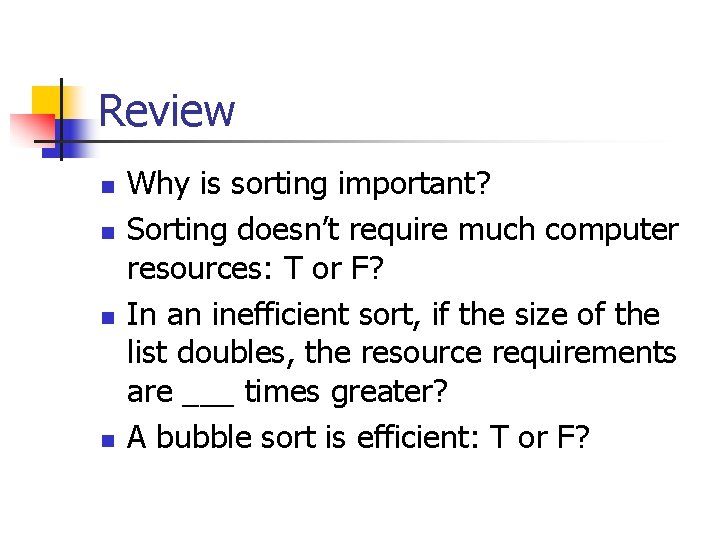
Review n n Why is sorting important? Sorting doesn’t require much computer resources: T or F? In an inefficient sort, if the size of the list doubles, the resource requirements are ___ times greater? A bubble sort is efficient: T or F?
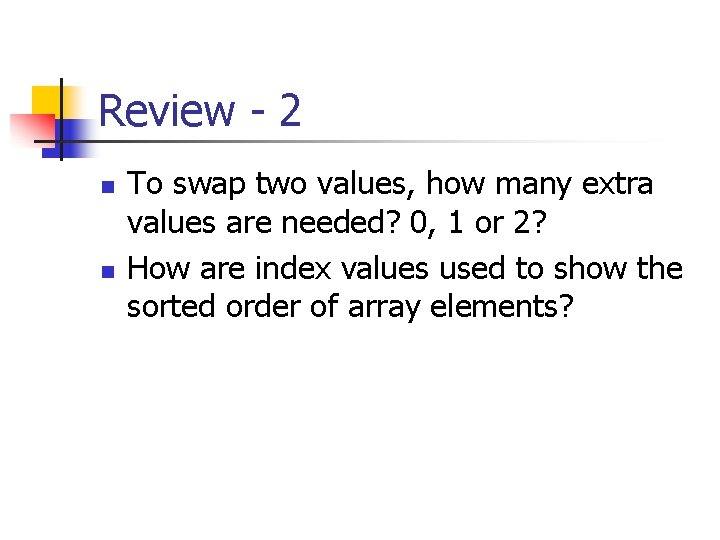
Review - 2 n n To swap two values, how many extra values are needed? 0, 1 or 2? How are index values used to show the sorted order of array elements?