Chapter 8 UserDefined Classes and ADTs Java Programming
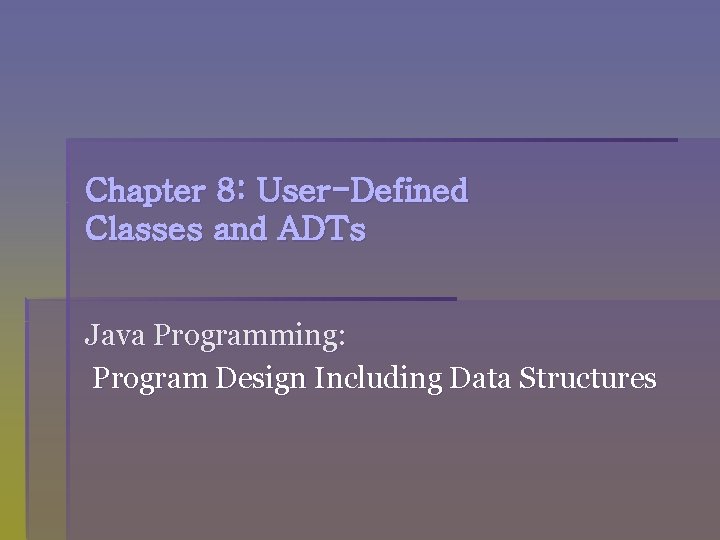
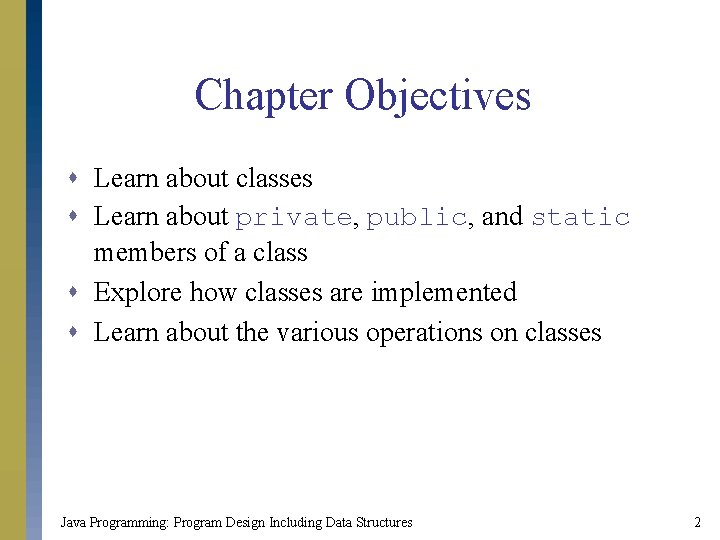
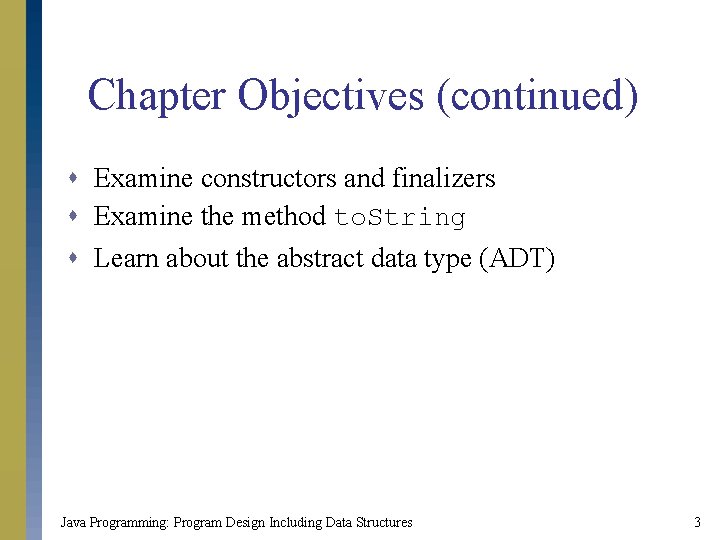
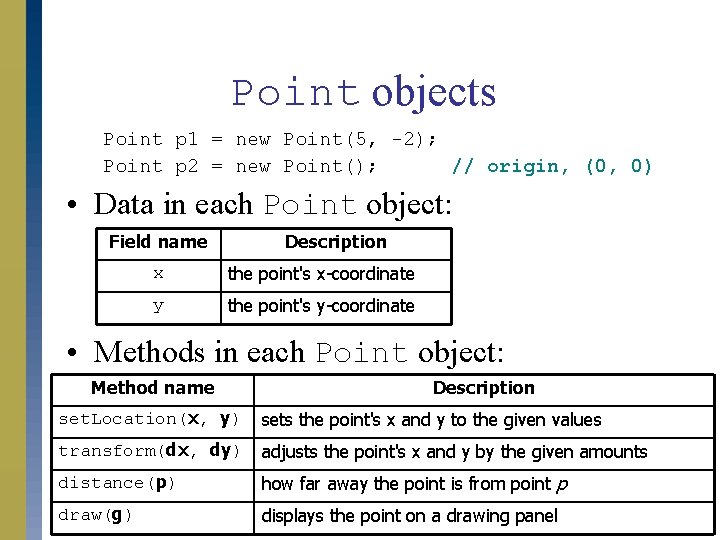
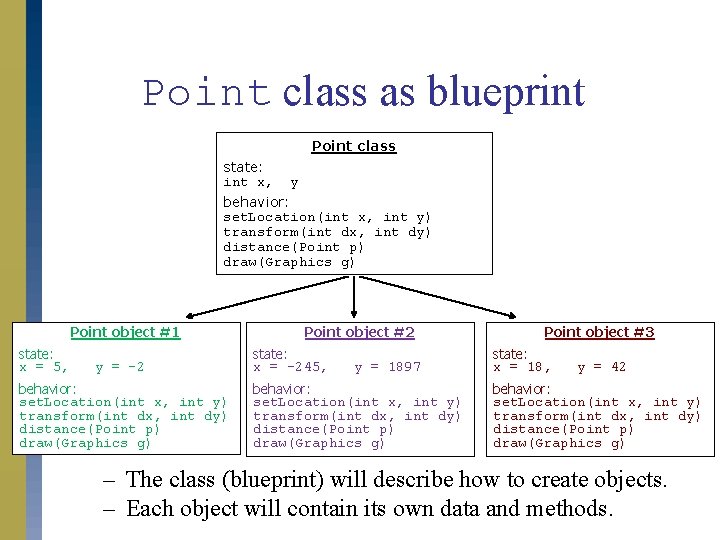
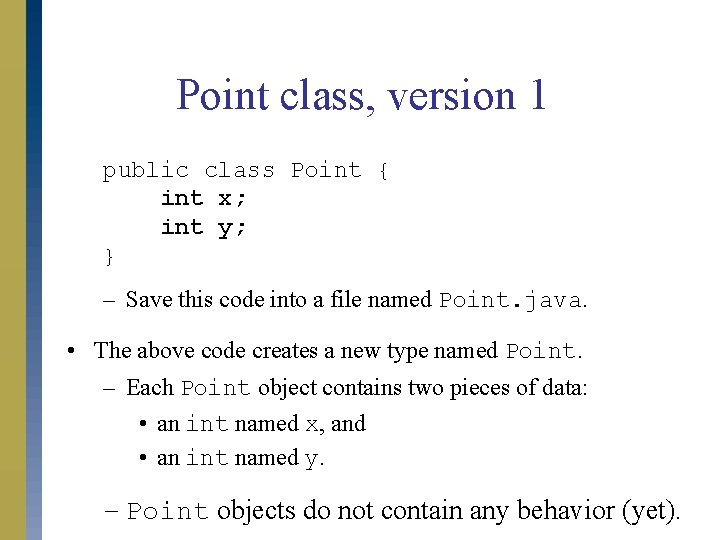
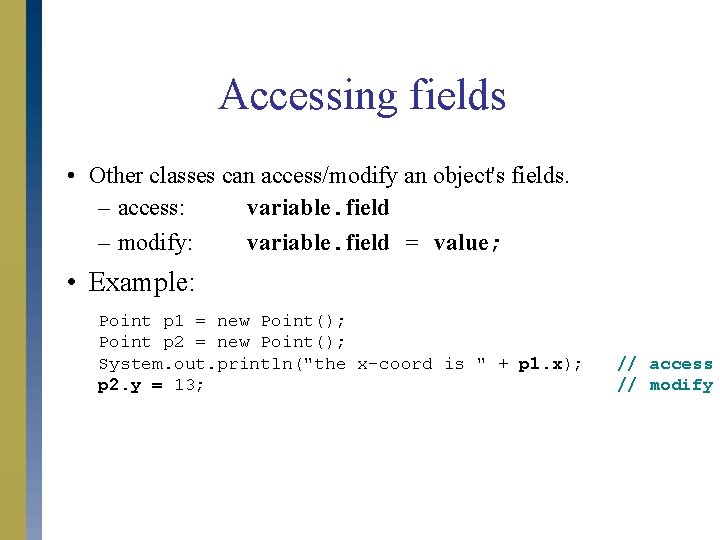
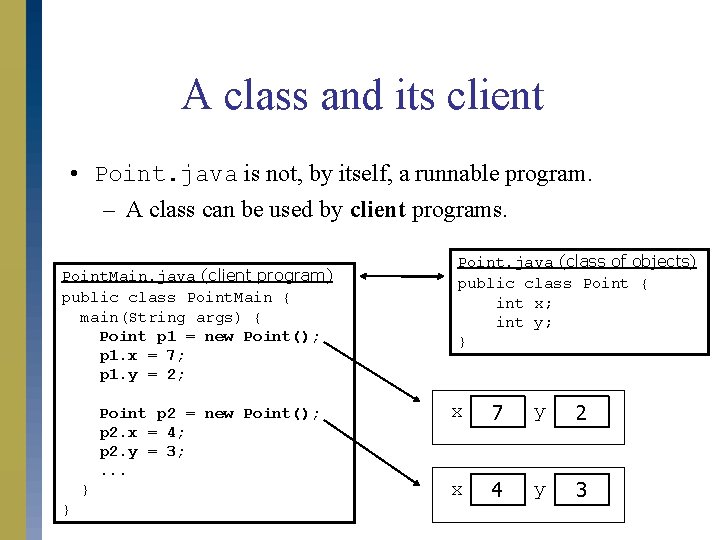
![Point. Main client example public class Point. Main { public static void main(String[] args) Point. Main client example public class Point. Main { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/e9d67f7f2d8dda67dbeb66a51211a0a4/image-9.jpg)
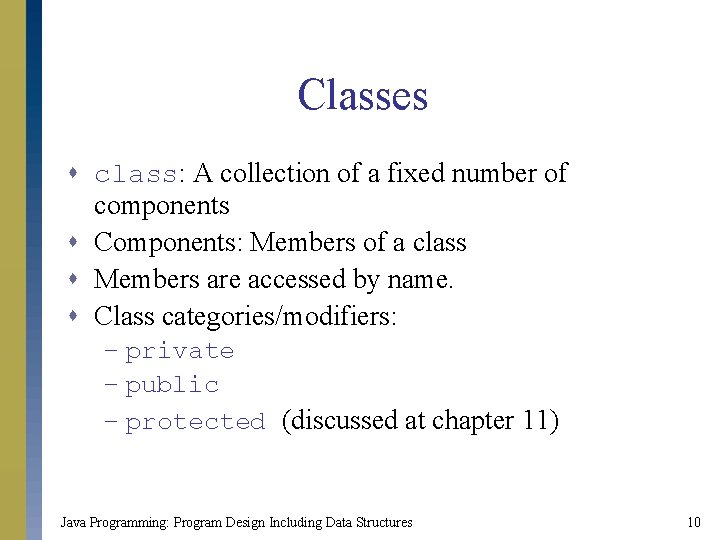
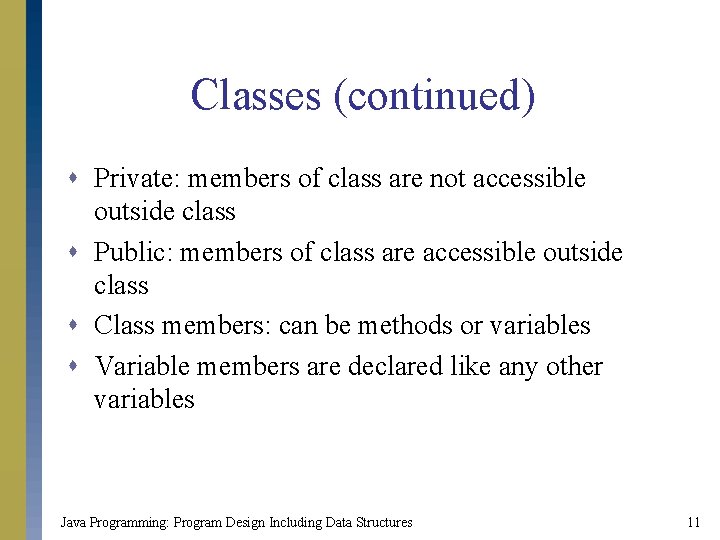
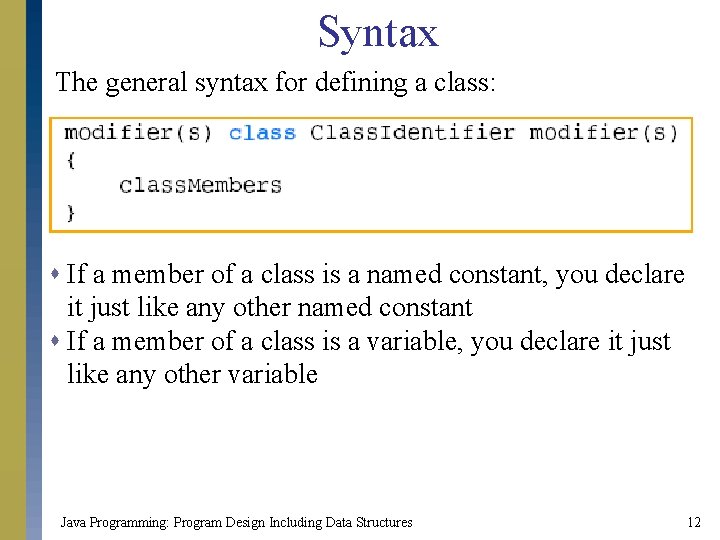
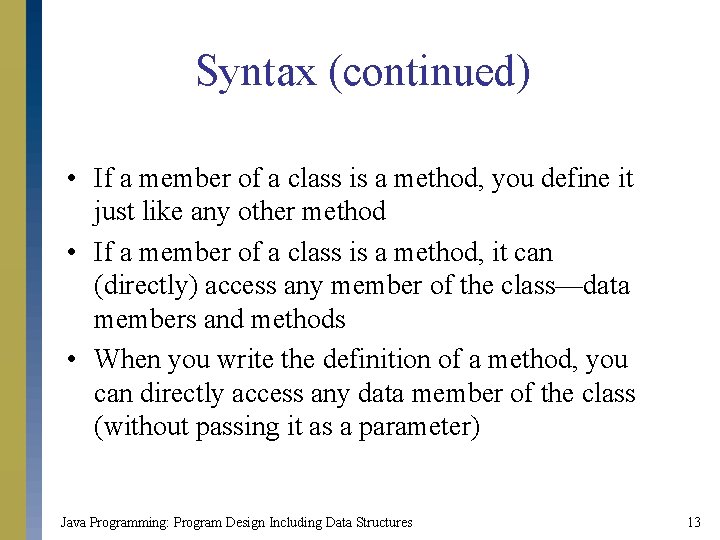
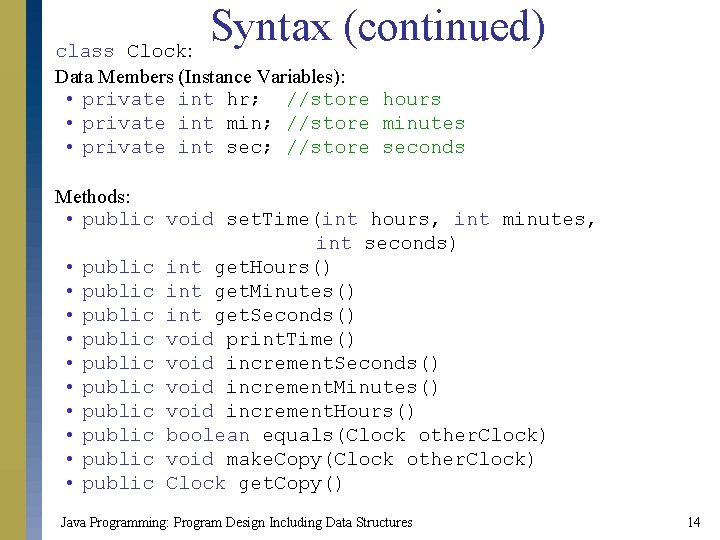
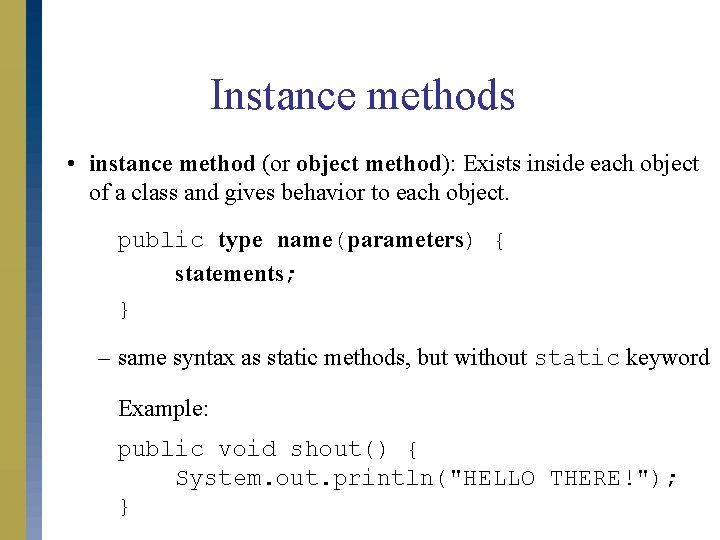
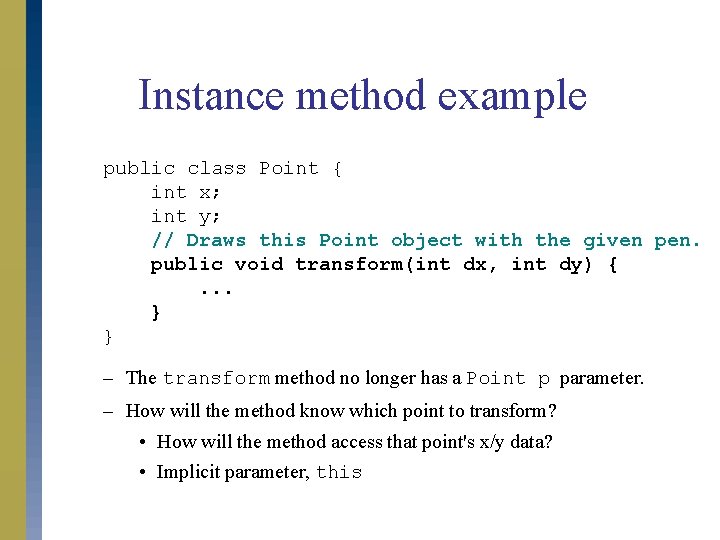
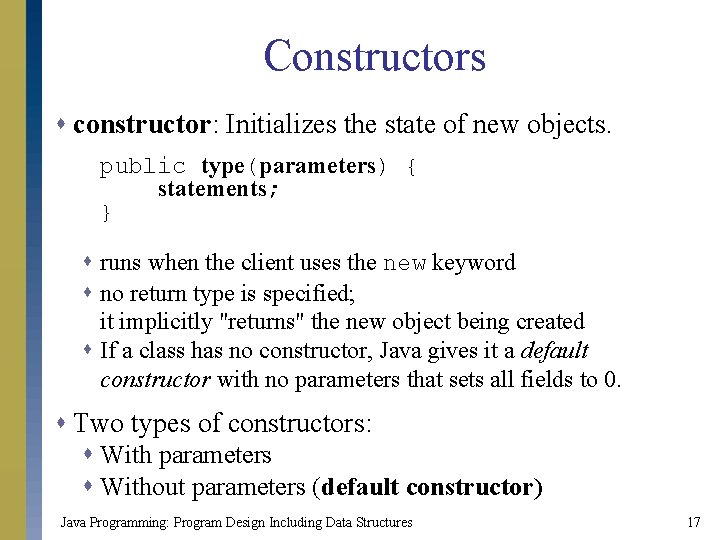
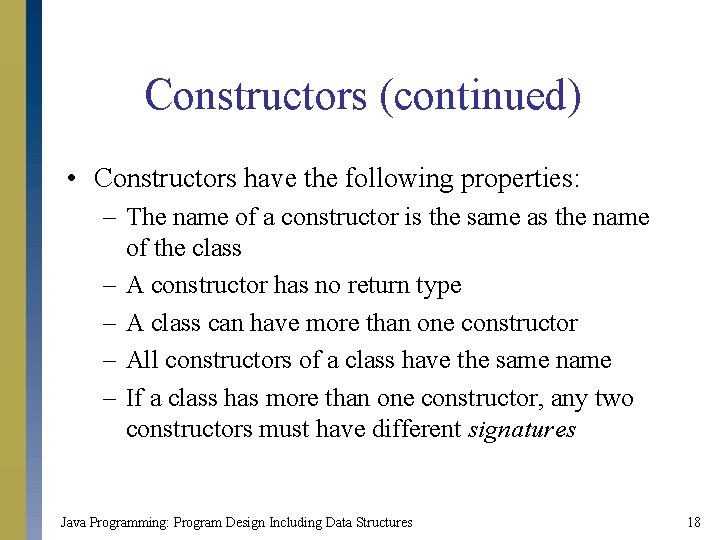
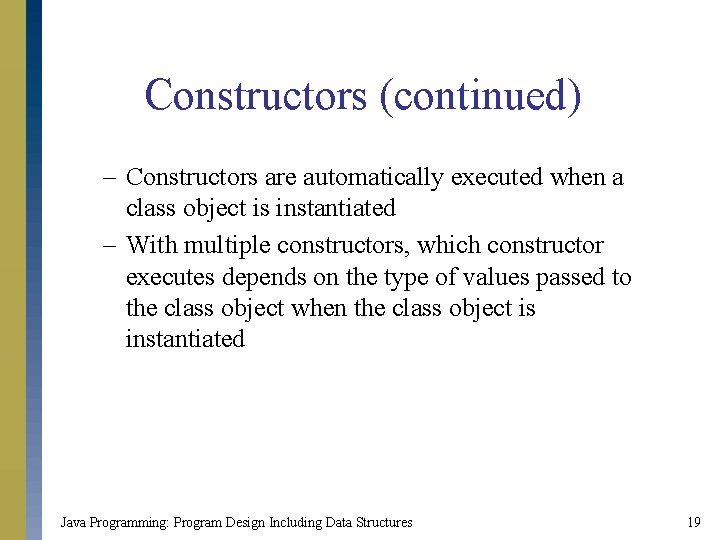
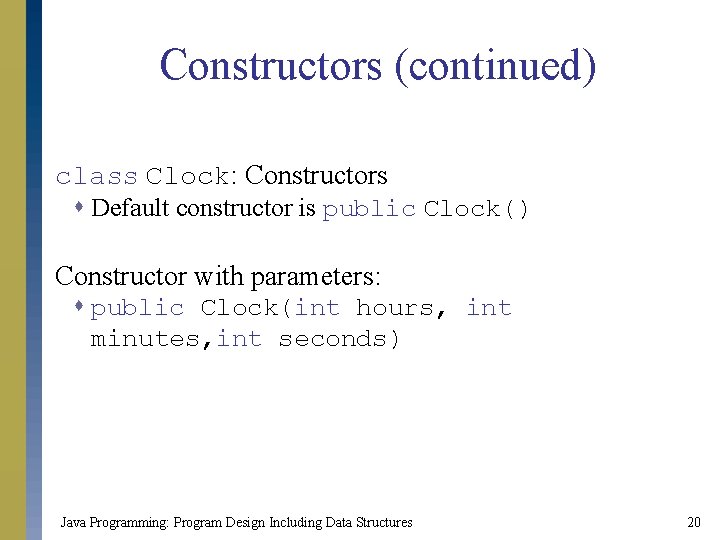
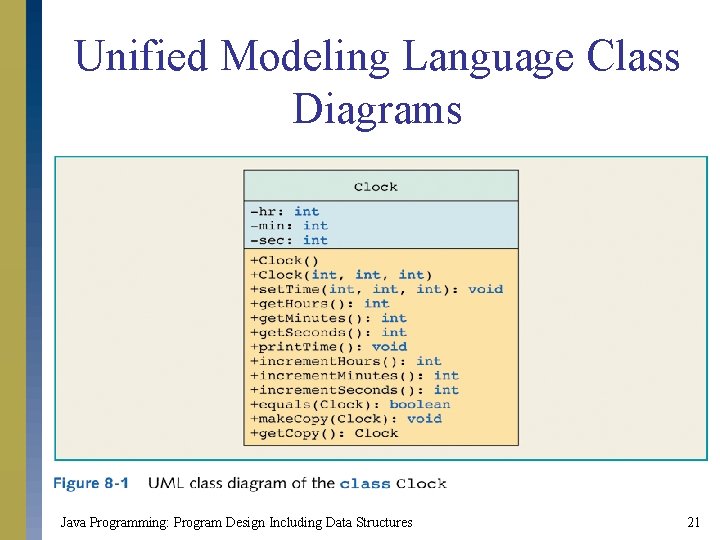
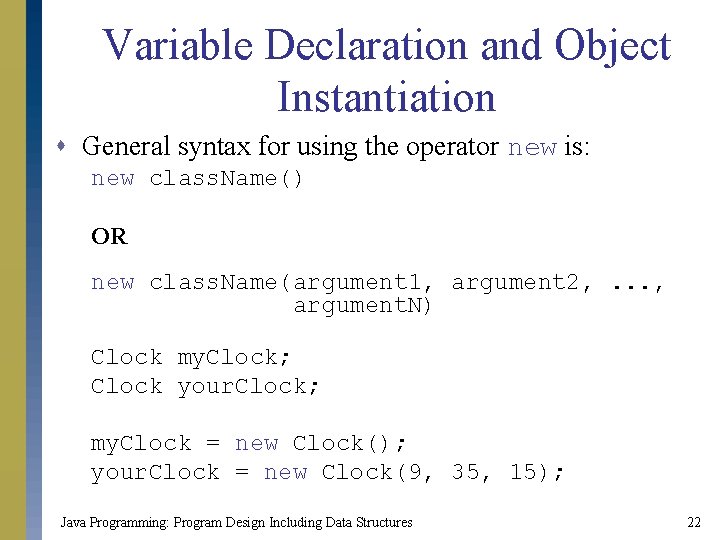
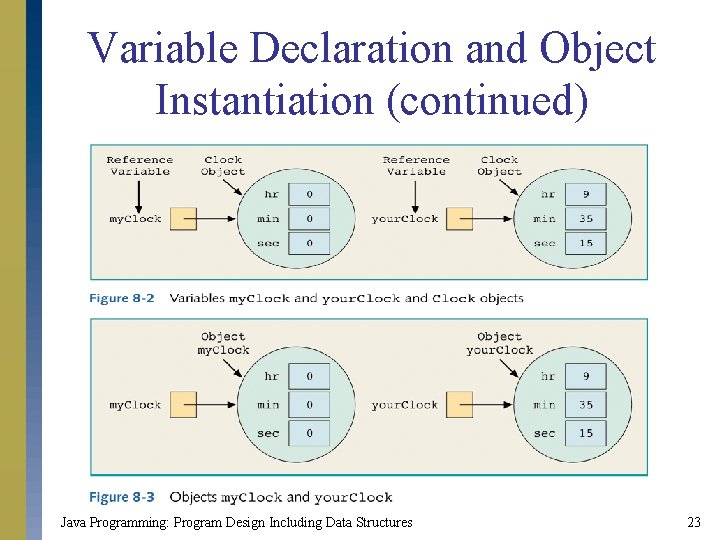
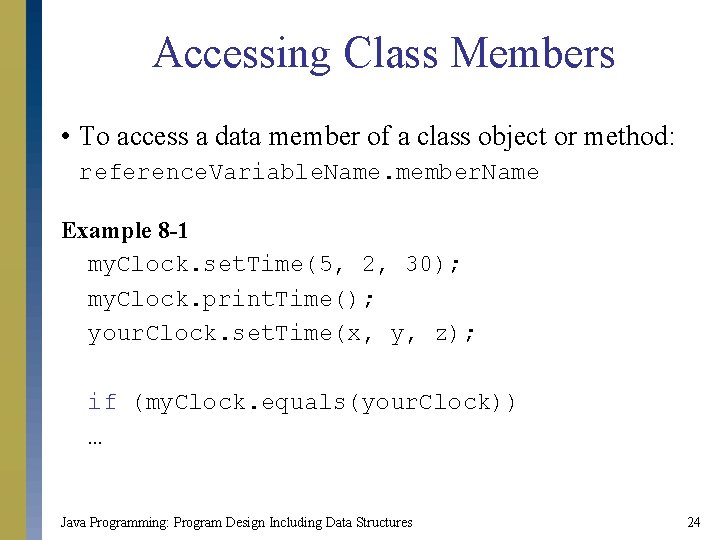
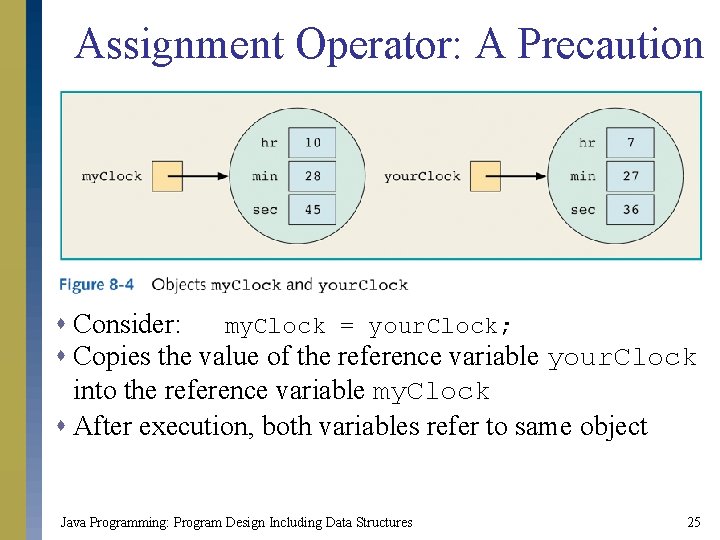
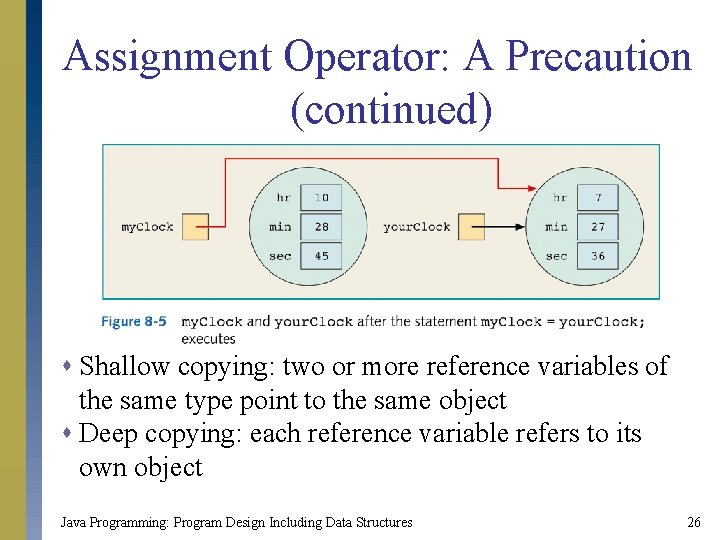
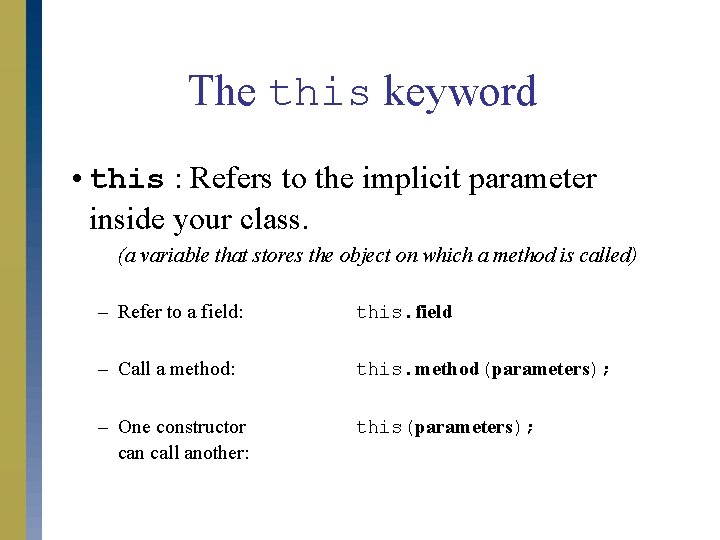
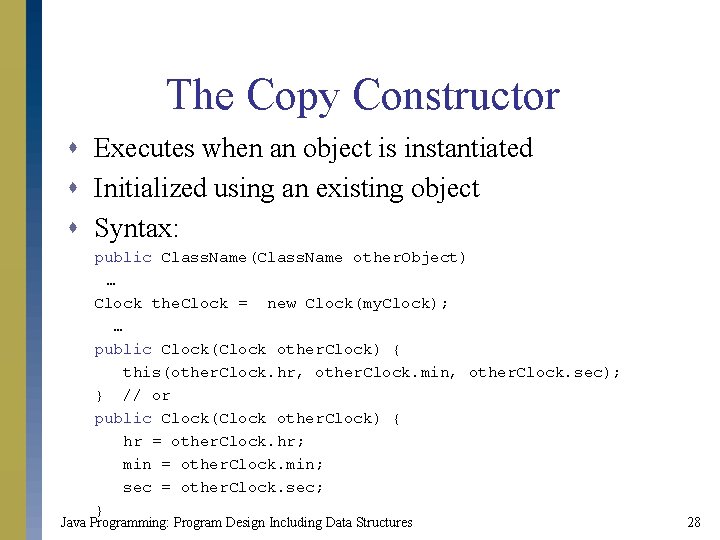
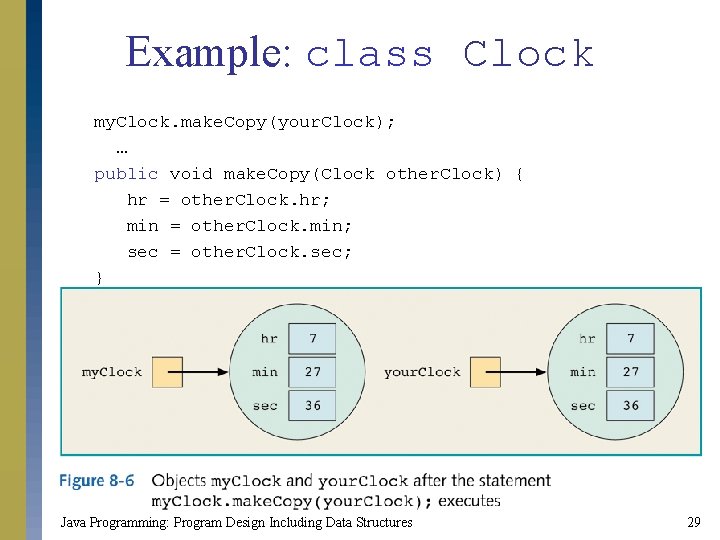
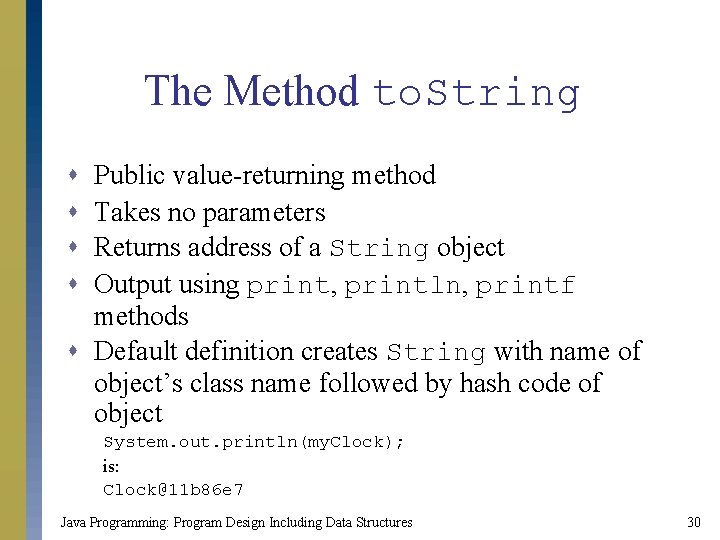
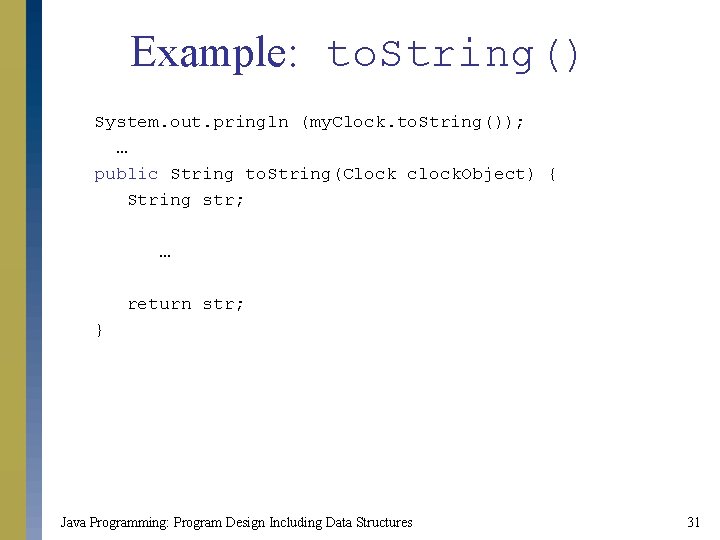
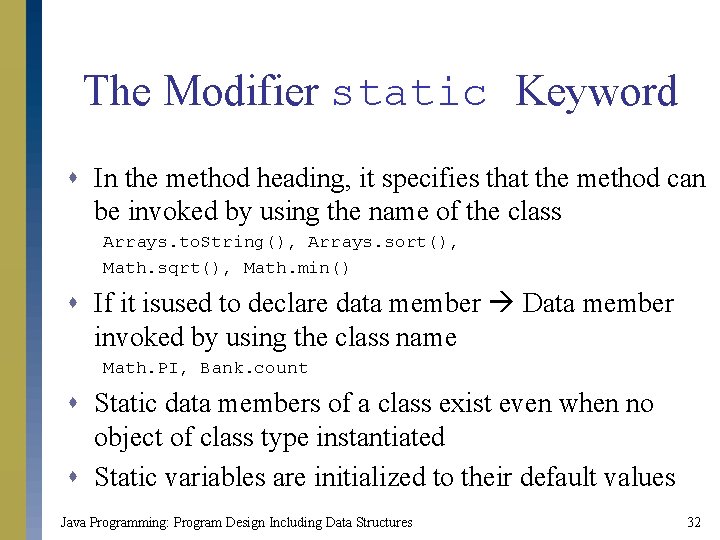
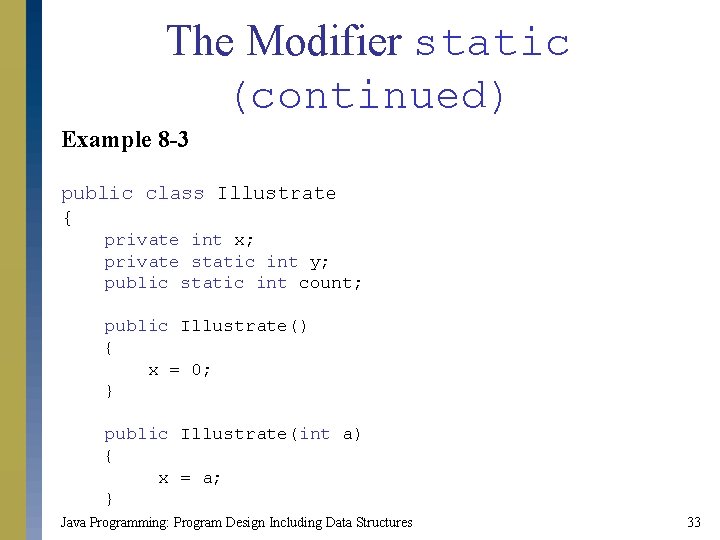
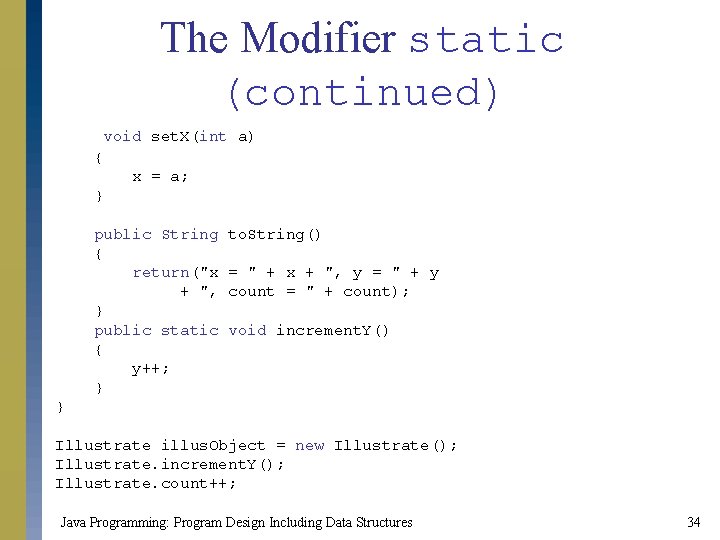
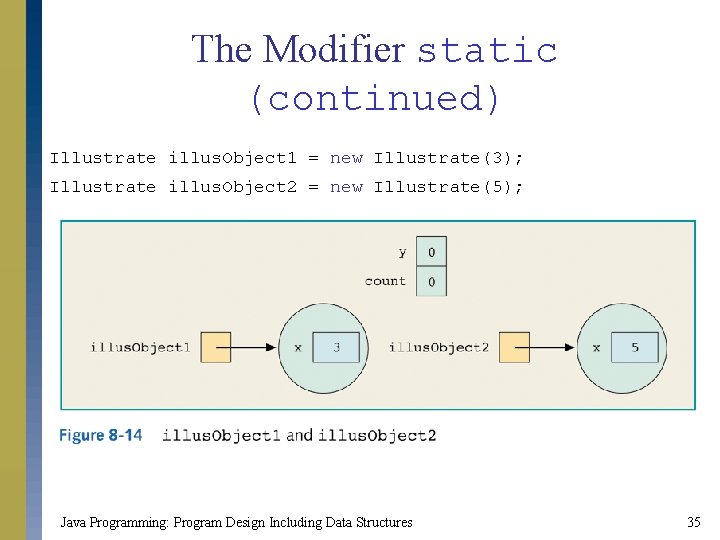
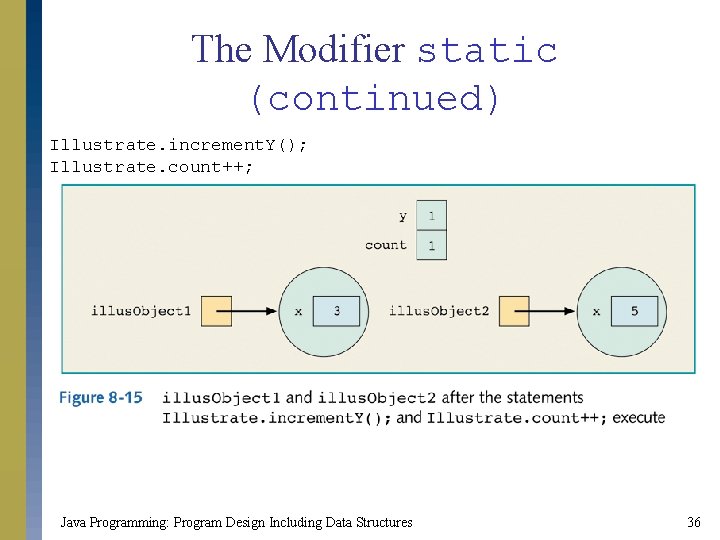
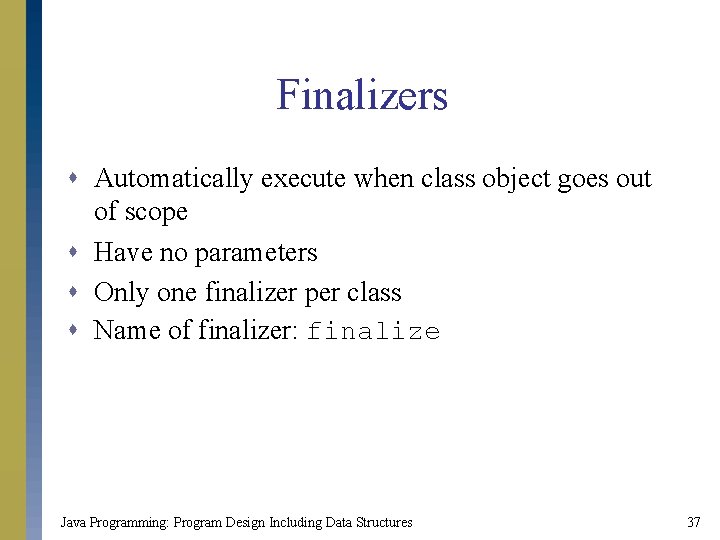
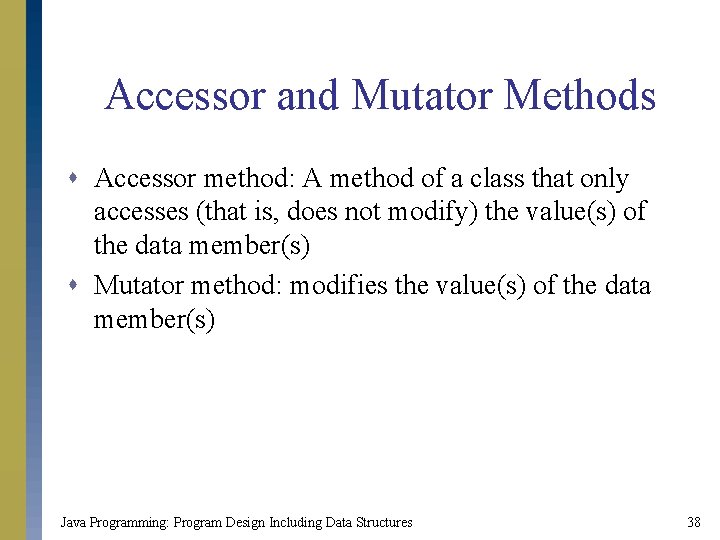
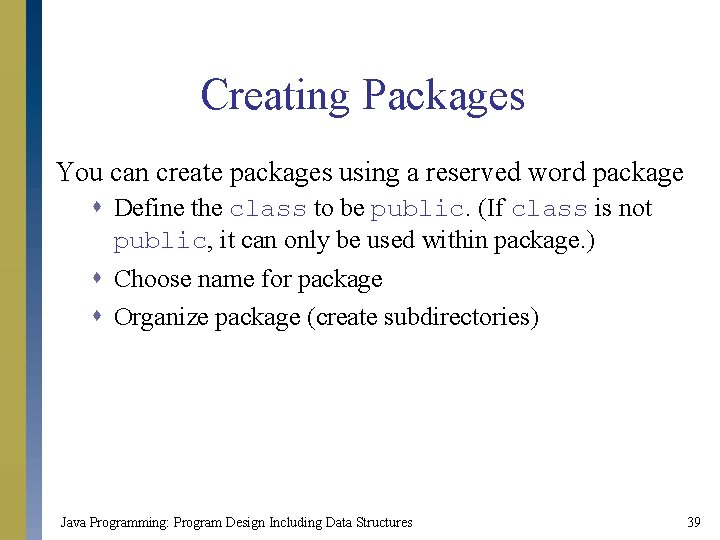
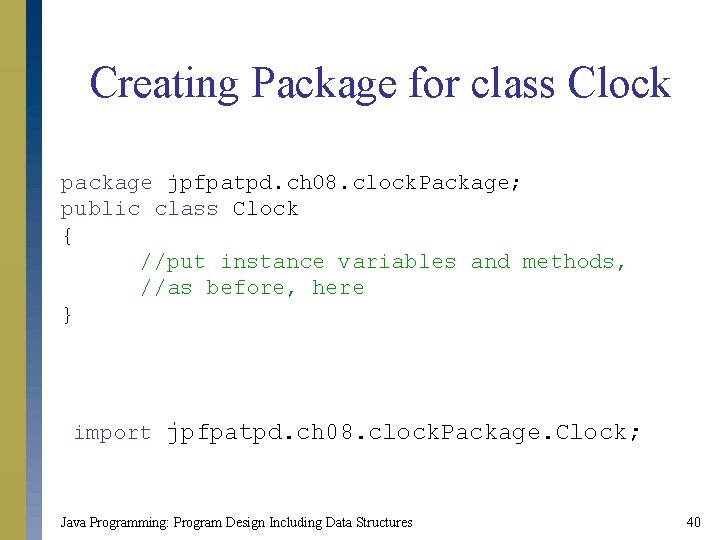
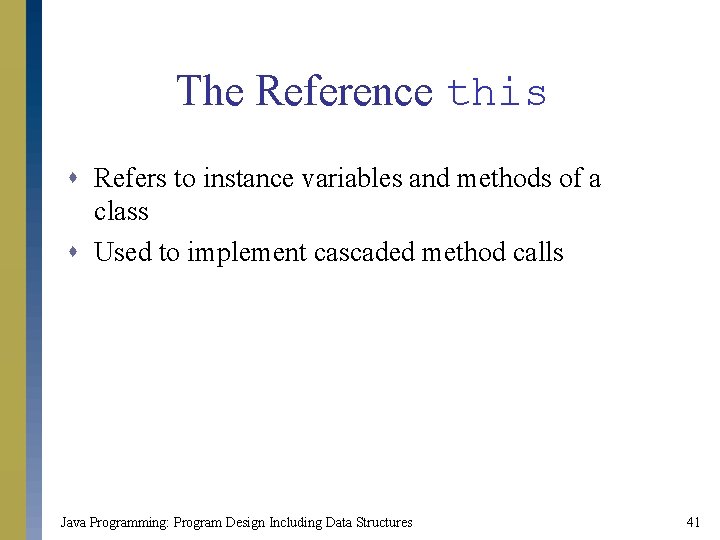
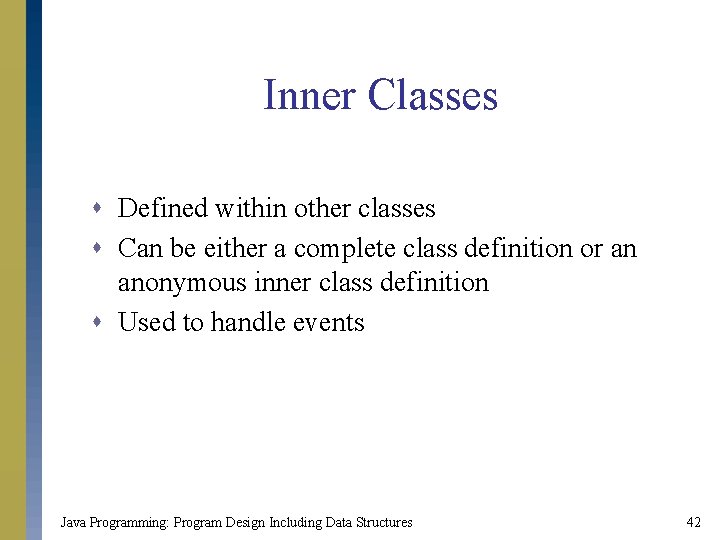
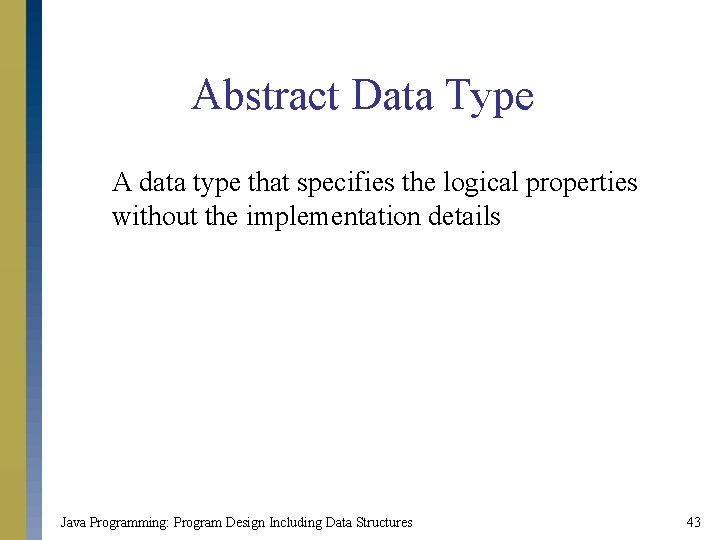
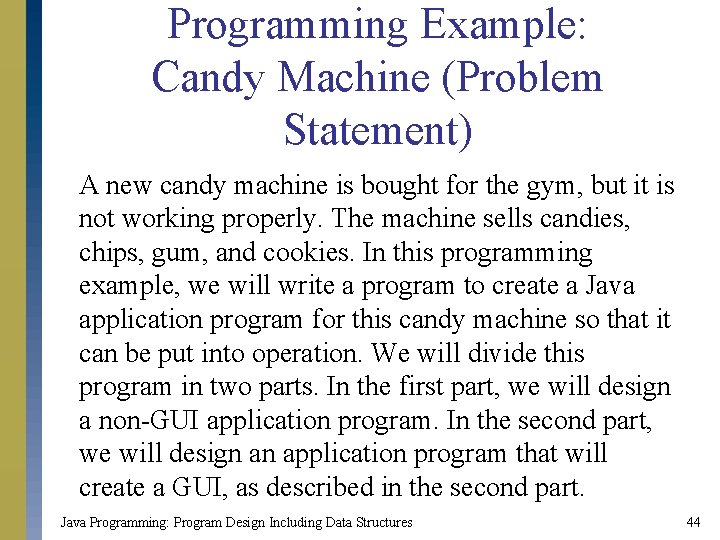
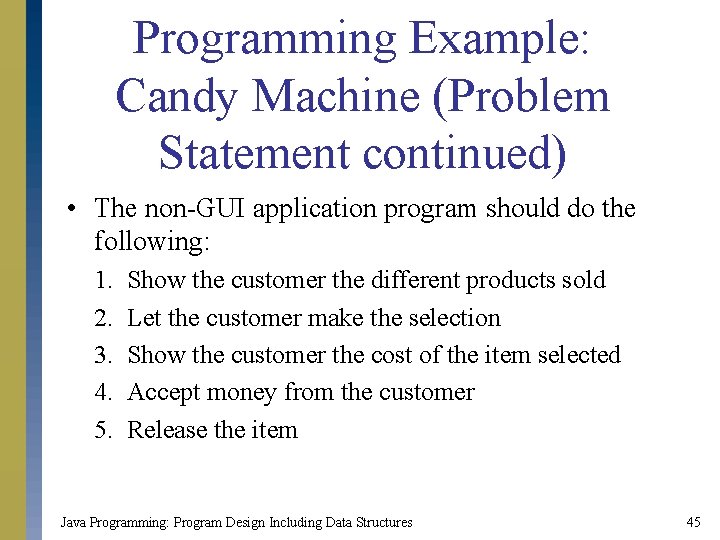
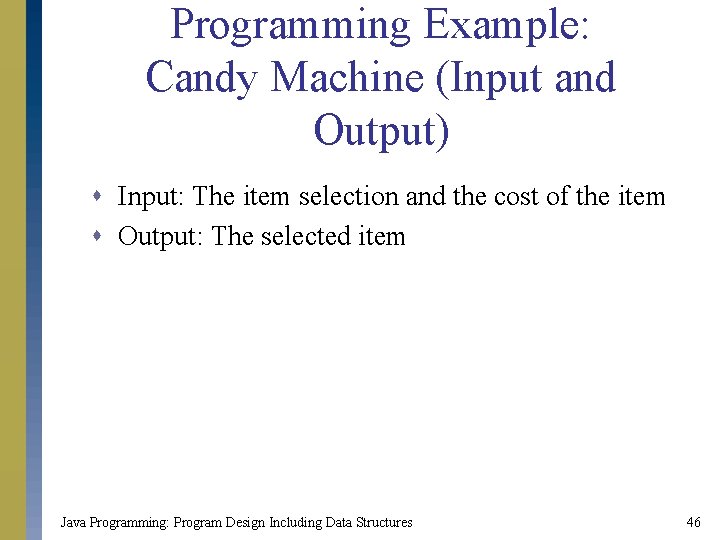
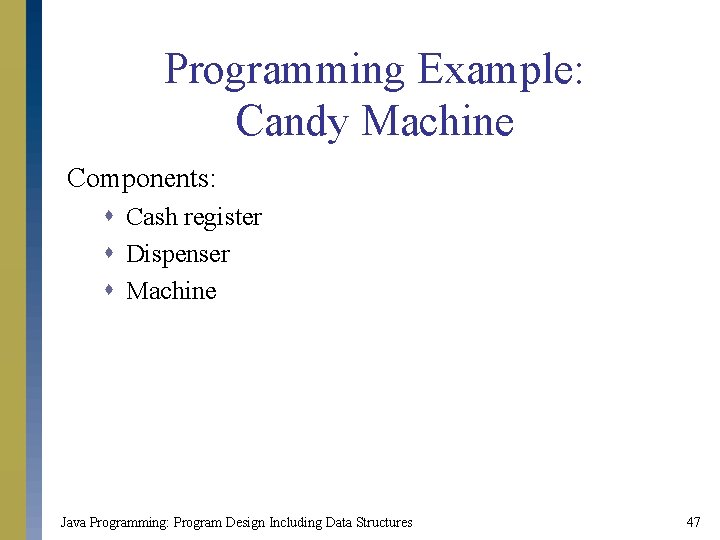
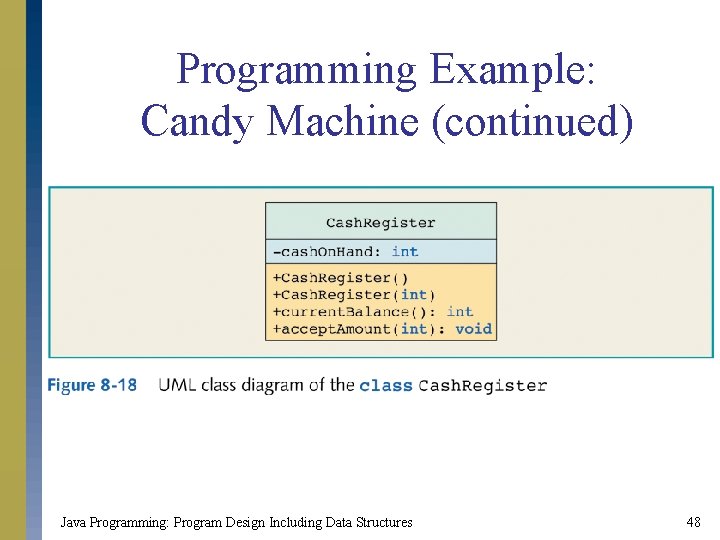
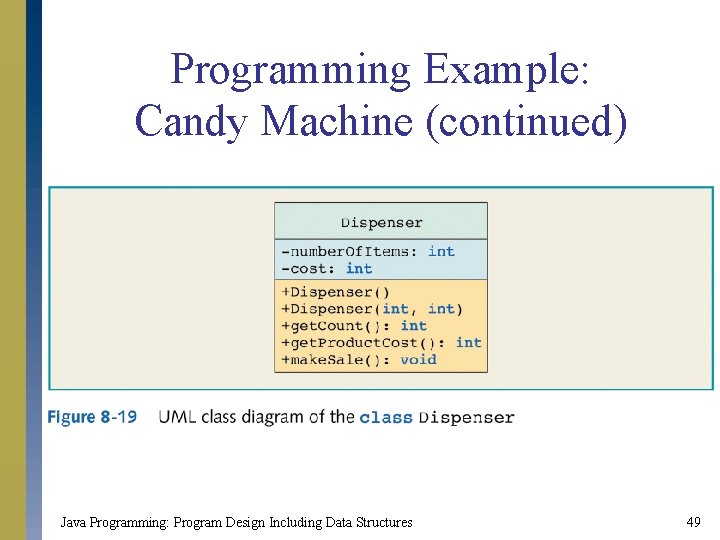
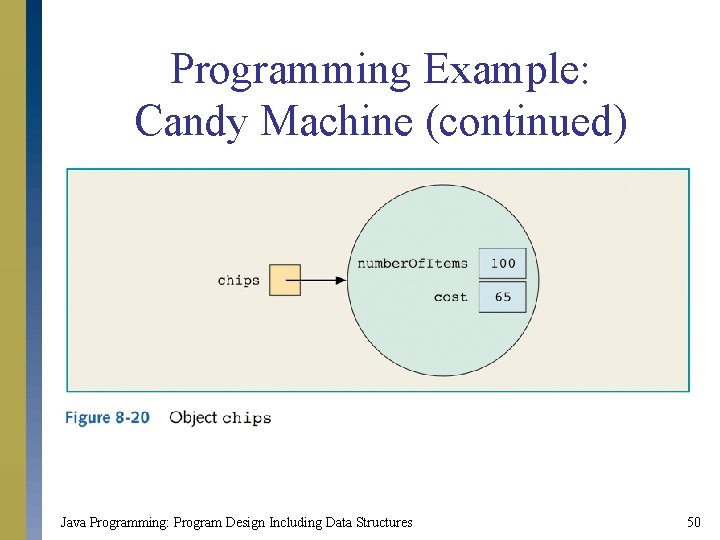
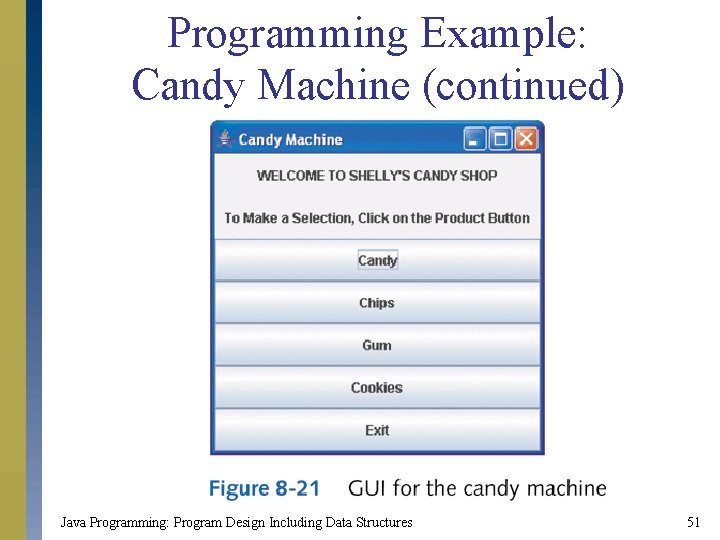
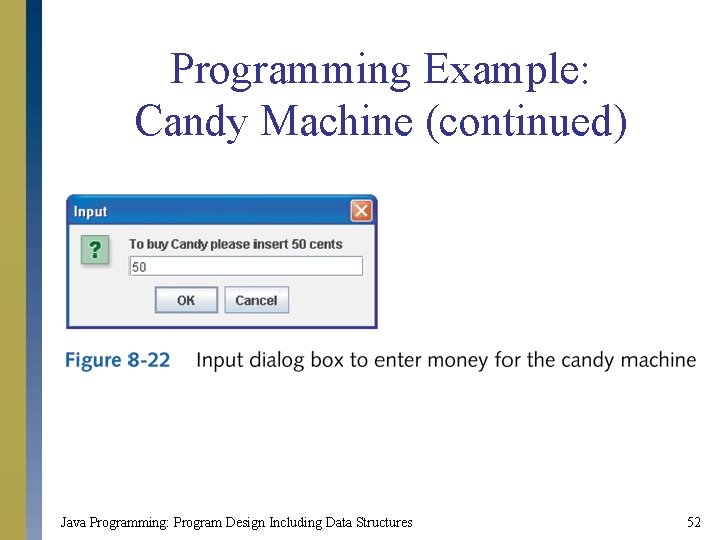
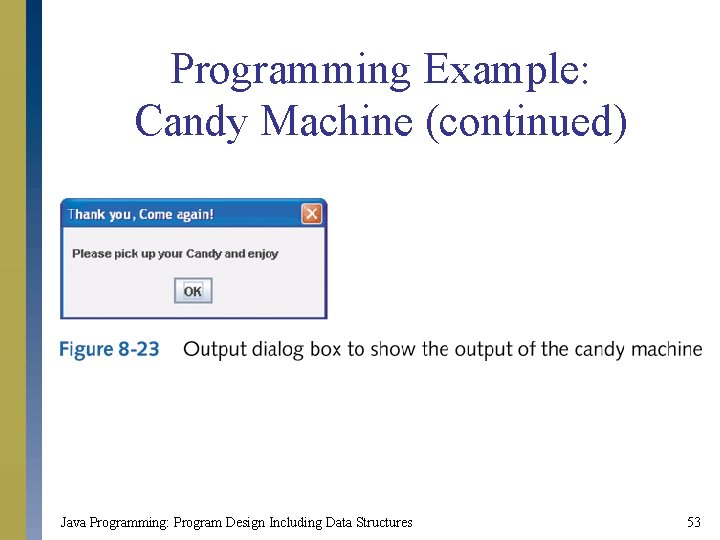
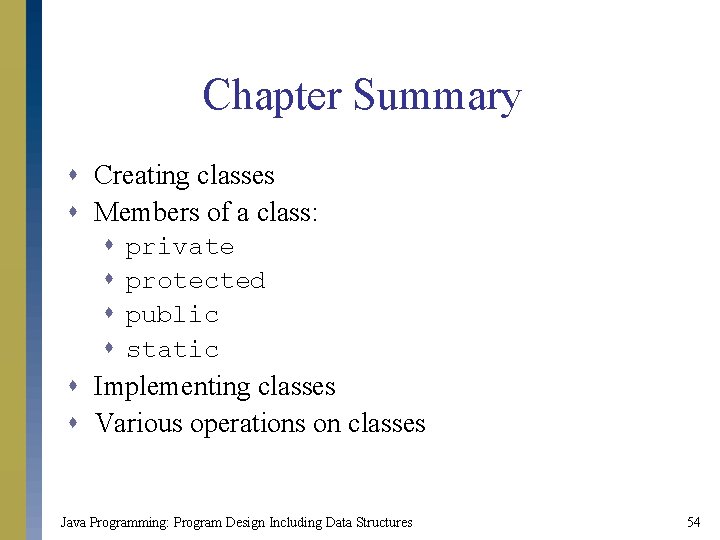
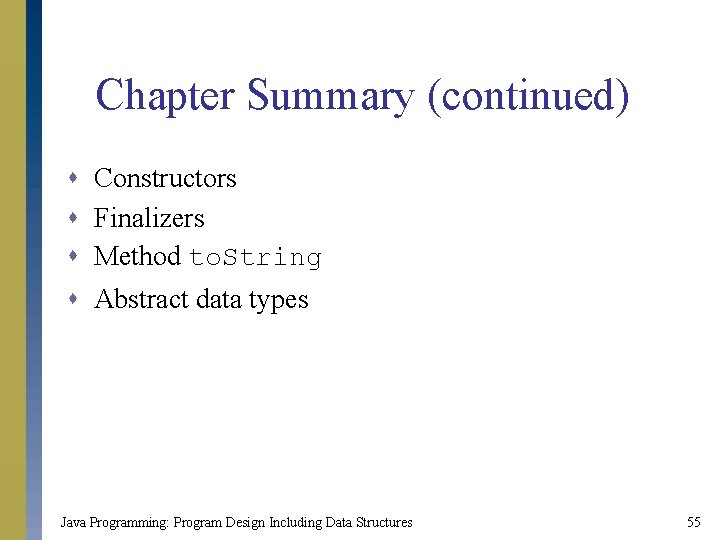
- Slides: 55
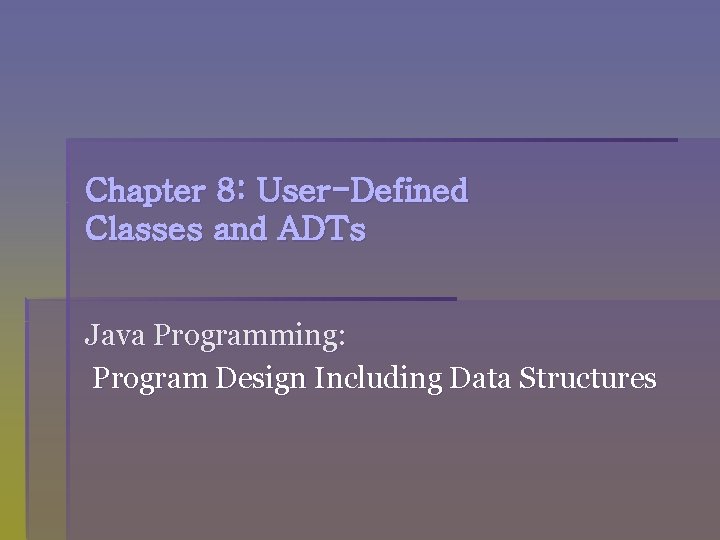
Chapter 8: User-Defined Classes and ADTs Java Programming: Program Design Including Data Structures
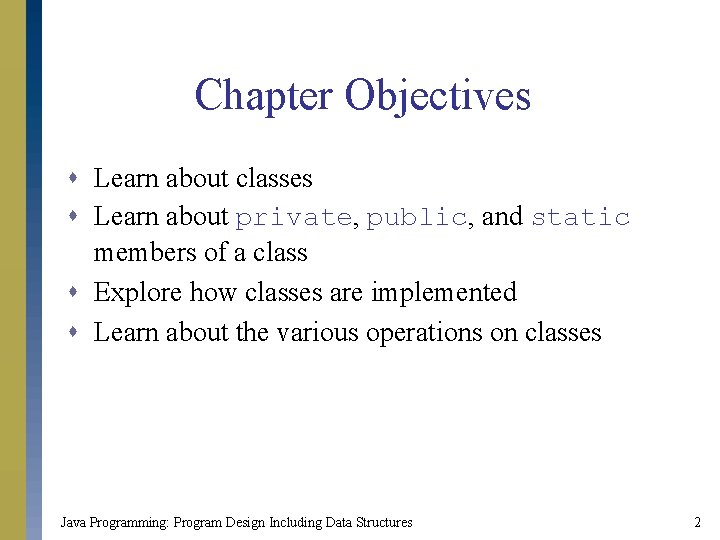
Chapter Objectives s Learn about classes s Learn about private, public, and static members of a class s Explore how classes are implemented s Learn about the various operations on classes Java Programming: Program Design Including Data Structures 2
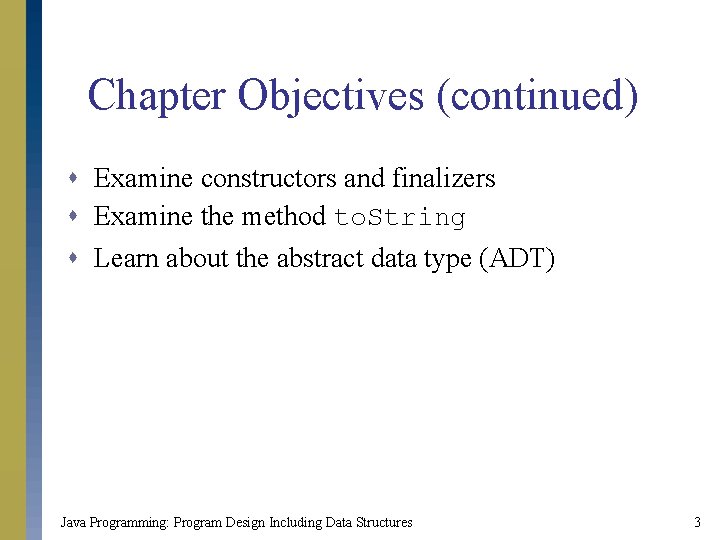
Chapter Objectives (continued) s Examine constructors and finalizers s Examine the method to. String s Learn about the abstract data type (ADT) Java Programming: Program Design Including Data Structures 3
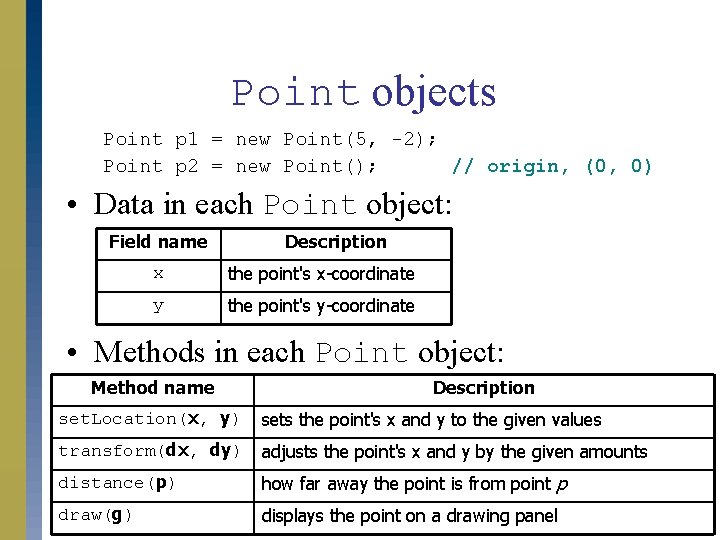
Point objects Point p 1 = new Point(5, -2); Point p 2 = new Point(); // origin, (0, 0) • Data in each Point object: Field name Description x the point's x-coordinate y the point's y-coordinate • Methods in each Point object: Method name Description set. Location(x, y) sets the point's x and y to the given values transform(dx, dy) adjusts the point's x and y by the given amounts distance(p) how far away the point is from point p draw(g) displays the point on a drawing panel
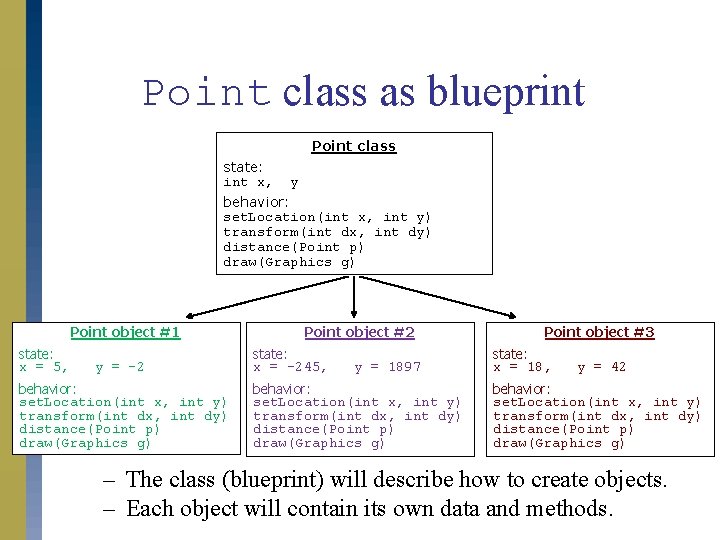
Point class as blueprint Point class state: int x, y behavior: set. Location(int x, int y) transform(int dx, int dy) distance(Point p) draw(Graphics g) Point object #1 state: x = 5, y = -2 behavior: set. Location(int x, int y) transform(int dx, int dy) distance(Point p) draw(Graphics g) Point object #2 state: x = -245, y = 1897 behavior: set. Location(int x, int y) transform(int dx, int dy) distance(Point p) draw(Graphics g) Point object #3 state: x = 18, y = 42 behavior: set. Location(int x, int y) transform(int dx, int dy) distance(Point p) draw(Graphics g) – The class (blueprint) will describe how to create objects. – Each object will contain its own data and methods.
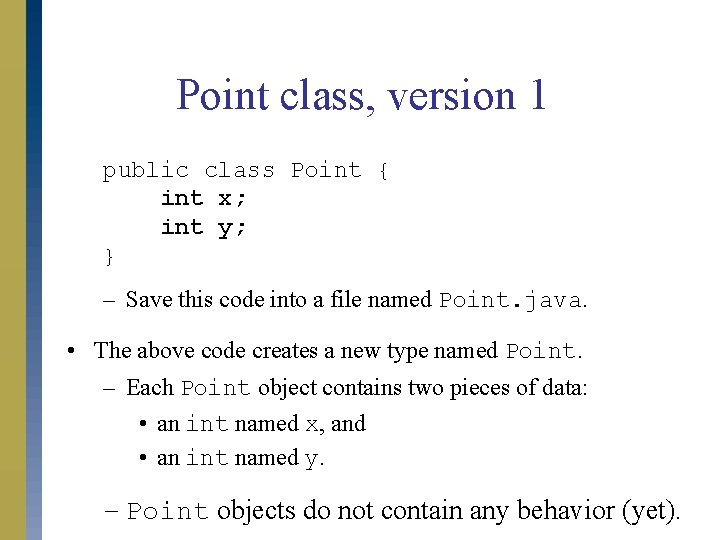
Point class, version 1 public class Point { int x; int y; } – Save this code into a file named Point. java. • The above code creates a new type named Point. – Each Point object contains two pieces of data: • an int named x, and • an int named y. – Point objects do not contain any behavior (yet).
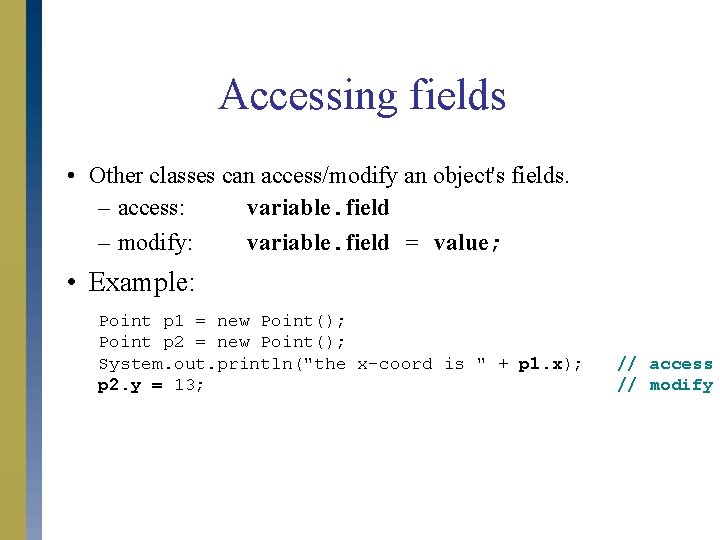
Accessing fields • Other classes can access/modify an object's fields. – access: variable. field – modify: variable. field = value; • Example: Point p 1 = new Point(); Point p 2 = new Point(); System. out. println("the x-coord is " + p 1. x); p 2. y = 13; // access // modify
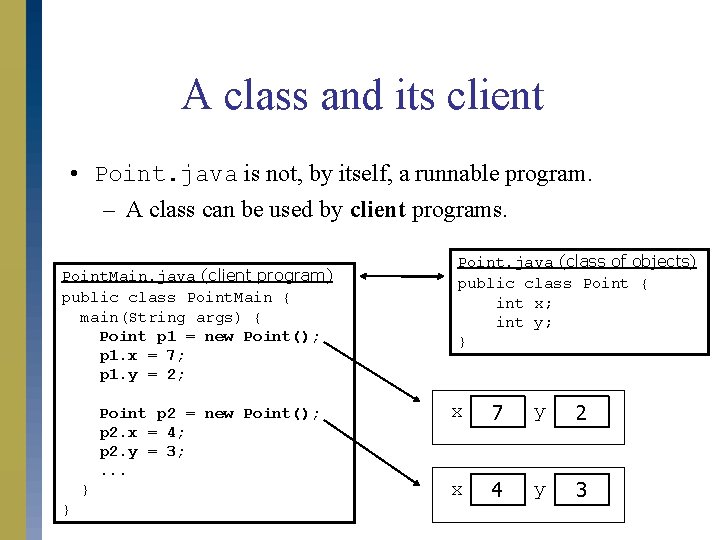
A class and its client • Point. java is not, by itself, a runnable program. – A class can be used by client programs. Point. Main. java (client program) public class Point. Main { main(String args) { Point p 1 = new Point(); p 1. x = 7; p 1. y = 2; Point p 2 = new Point(); p 2. x = 4; p 2. y = 3; . . . } } Point. java (class of objects) public class Point { int x; int y; } x 7 y 2 x 4 y 3
![Point Main client example public class Point Main public static void mainString args Point. Main client example public class Point. Main { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/e9d67f7f2d8dda67dbeb66a51211a0a4/image-9.jpg)
Point. Main client example public class Point. Main { public static void main(String[] args) { // create two Point objects Point p 1 = new Point(); p 1. y = 2; Point p 2 = new Point(); p 2. x = 4; } } System. out. println(p 1. x + ", " + p 1. y); // 0, 2 // move p 2 and then print it p 2. x += 2; p 2. y++; System. out. println(p 2. x + ", " + p 2. y); // 6, 1
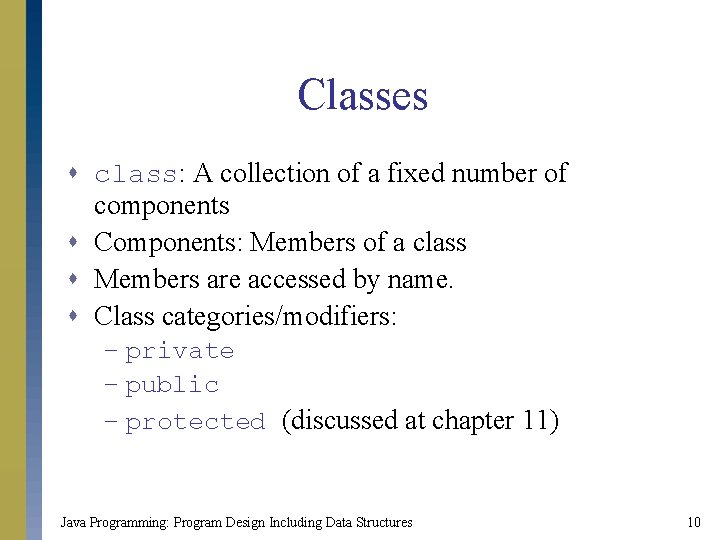
Classes s class: A collection of a fixed number of components s Components: Members of a class s Members are accessed by name. s Class categories/modifiers: – private – public – protected (discussed at chapter 11) Java Programming: Program Design Including Data Structures 10
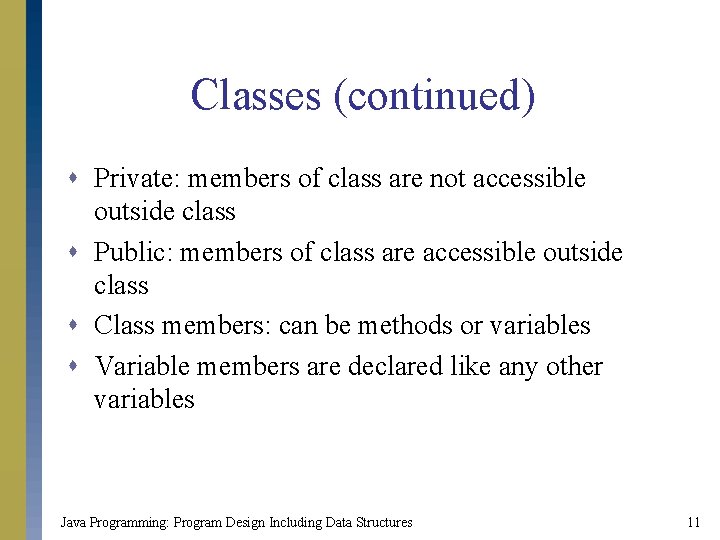
Classes (continued) s Private: members of class are not accessible outside class s Public: members of class are accessible outside class s Class members: can be methods or variables s Variable members are declared like any other variables Java Programming: Program Design Including Data Structures 11
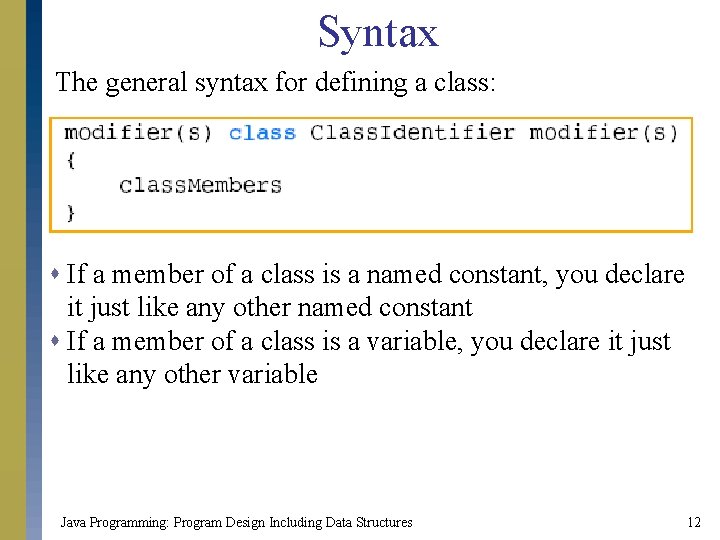
Syntax The general syntax for defining a class: s If a member of a class is a named constant, you declare it just like any other named constant s If a member of a class is a variable, you declare it just like any other variable Java Programming: Program Design Including Data Structures 12
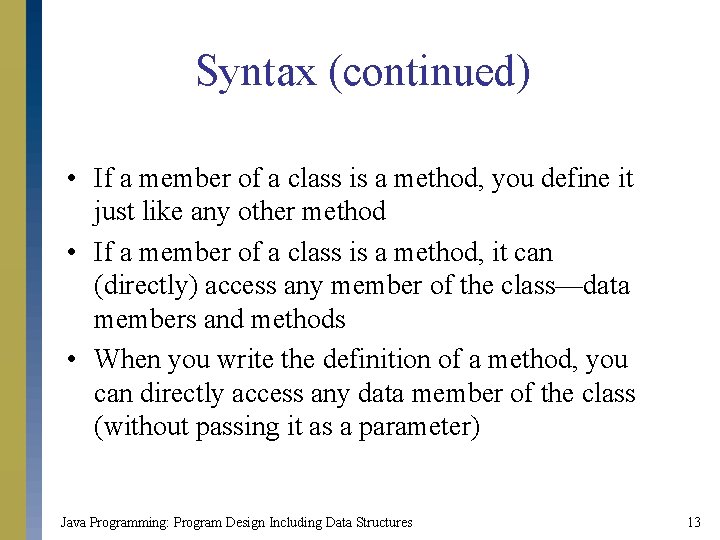
Syntax (continued) • If a member of a class is a method, you define it just like any other method • If a member of a class is a method, it can (directly) access any member of the class—data members and methods • When you write the definition of a method, you can directly access any data member of the class (without passing it as a parameter) Java Programming: Program Design Including Data Structures 13
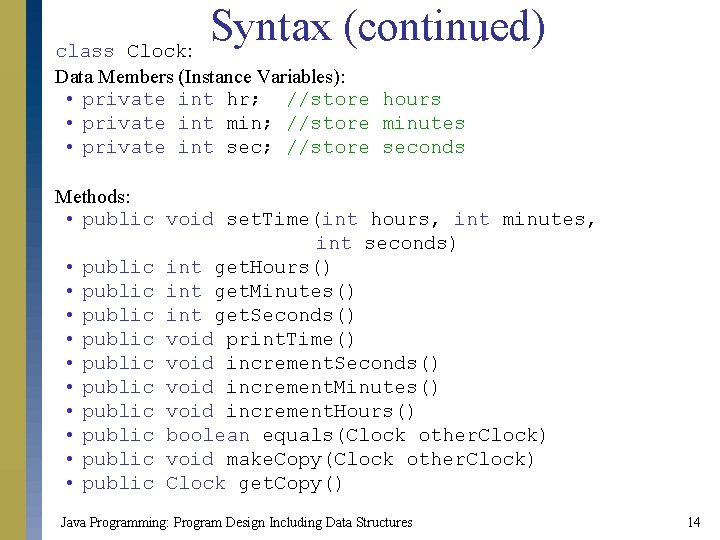
Syntax (continued) class Clock: Data Members (Instance Variables): • private int hr; //store hours • private int min; //store minutes • private int sec; //store seconds Methods: • public void set. Time(int hours, int minutes, int seconds) • public int get. Hours() • public int get. Minutes() • public int get. Seconds() • public void print. Time() • public void increment. Seconds() • public void increment. Minutes() • public void increment. Hours() • public boolean equals(Clock other. Clock) • public void make. Copy(Clock other. Clock) • public Clock get. Copy() Java Programming: Program Design Including Data Structures 14
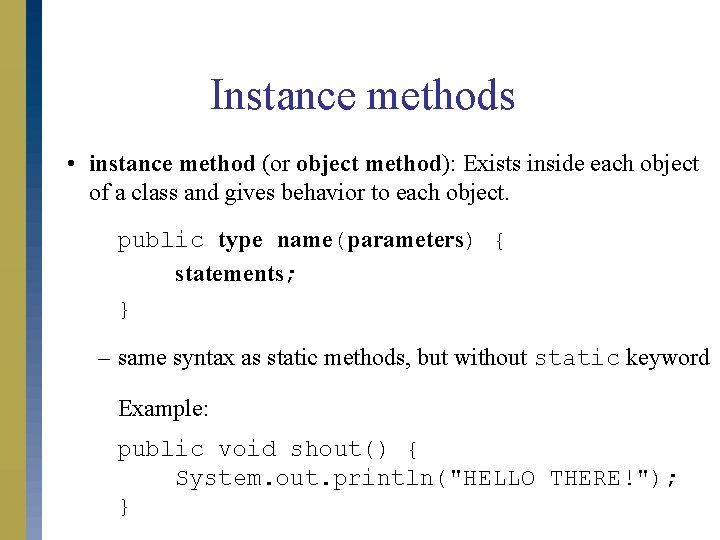
Instance methods • instance method (or object method): Exists inside each object of a class and gives behavior to each object. public type name(parameters) { statements; } – same syntax as static methods, but without static keyword Example: public void shout() { System. out. println("HELLO THERE!"); }
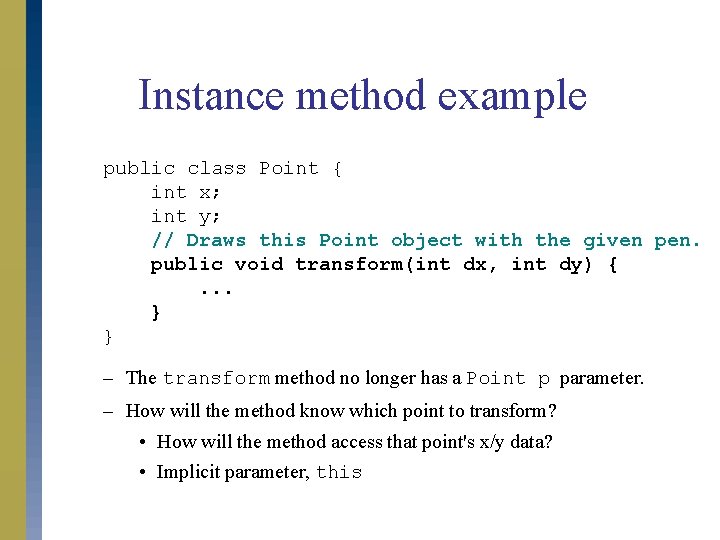
Instance method example public class Point { int x; int y; // Draws this Point object with the given pen. public void transform(int dx, int dy) {. . . } } – The transform method no longer has a Point p parameter. – How will the method know which point to transform? • How will the method access that point's x/y data? • Implicit parameter, this
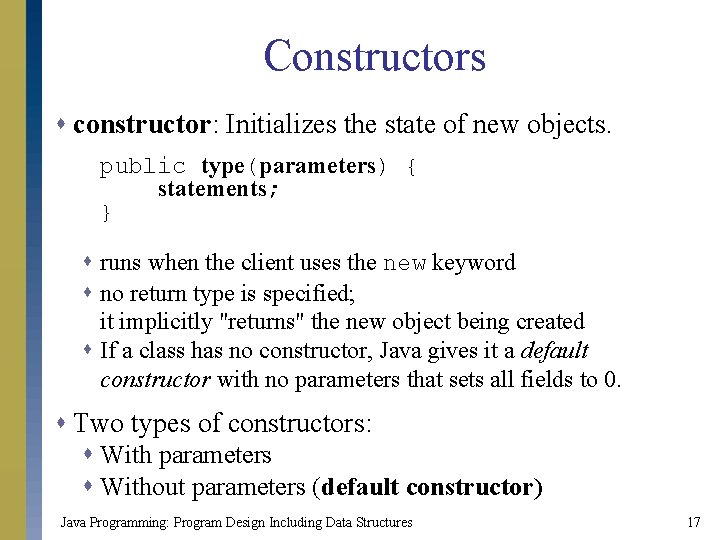
Constructors s constructor: Initializes the state of new objects. public type(parameters) { statements; } s runs when the client uses the new keyword s no return type is specified; it implicitly "returns" the new object being created s If a class has no constructor, Java gives it a default constructor with no parameters that sets all fields to 0. s Two types of constructors: s With parameters s Without parameters (default constructor) Java Programming: Program Design Including Data Structures 17
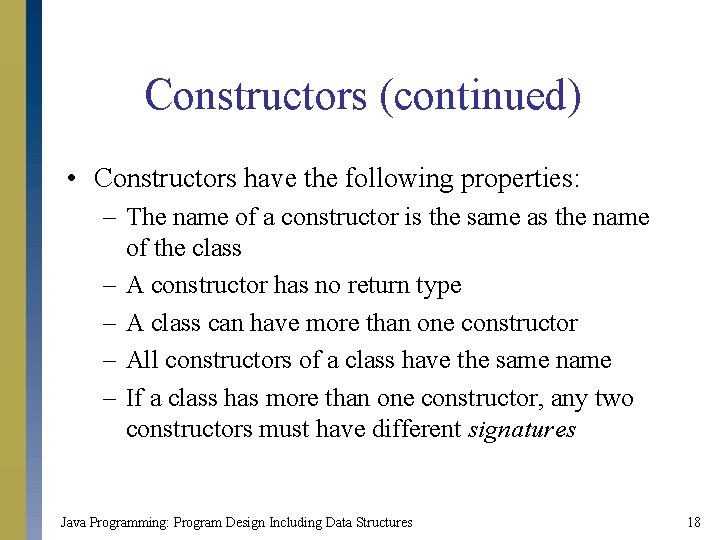
Constructors (continued) • Constructors have the following properties: – The name of a constructor is the same as the name of the class – A constructor has no return type – A class can have more than one constructor – All constructors of a class have the same name – If a class has more than one constructor, any two constructors must have different signatures Java Programming: Program Design Including Data Structures 18
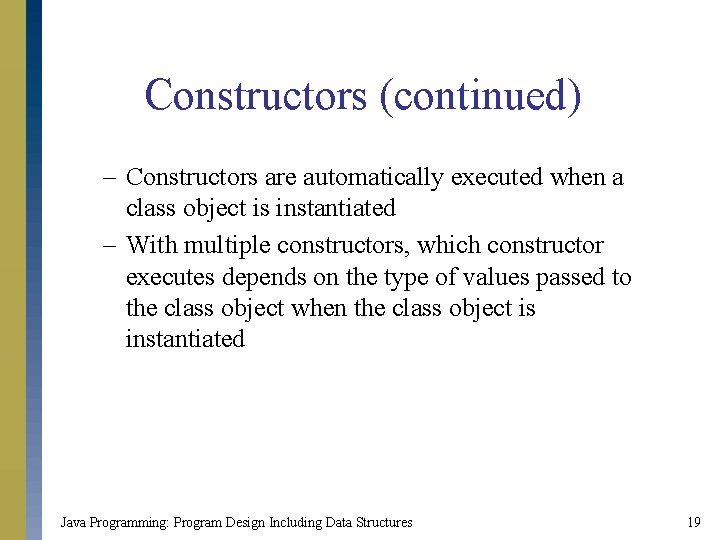
Constructors (continued) – Constructors are automatically executed when a class object is instantiated – With multiple constructors, which constructor executes depends on the type of values passed to the class object when the class object is instantiated Java Programming: Program Design Including Data Structures 19
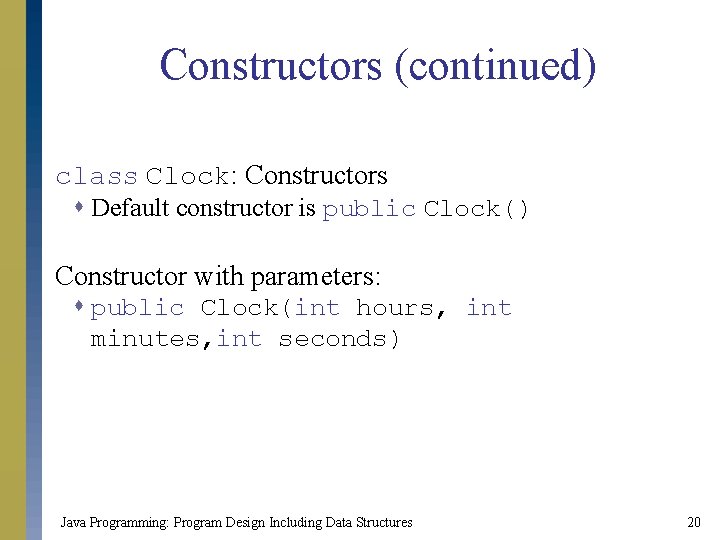
Constructors (continued) class Clock: Constructors s Default constructor is public Clock() Constructor with parameters: s public Clock(int hours, int minutes, int seconds) Java Programming: Program Design Including Data Structures 20
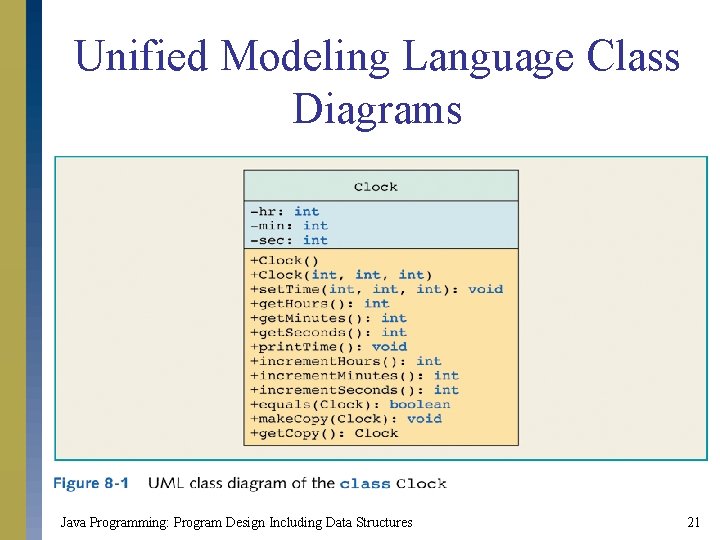
Unified Modeling Language Class Diagrams Java Programming: Program Design Including Data Structures 21
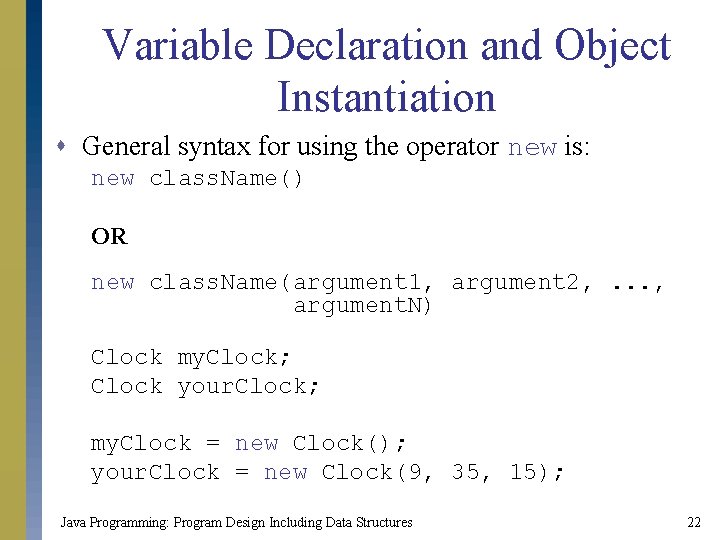
Variable Declaration and Object Instantiation s General syntax for using the operator new is: new class. Name() OR new class. Name(argument 1, argument 2, . . . , argument. N) Clock my. Clock; Clock your. Clock; my. Clock = new Clock(); your. Clock = new Clock(9, 35, 15); Java Programming: Program Design Including Data Structures 22
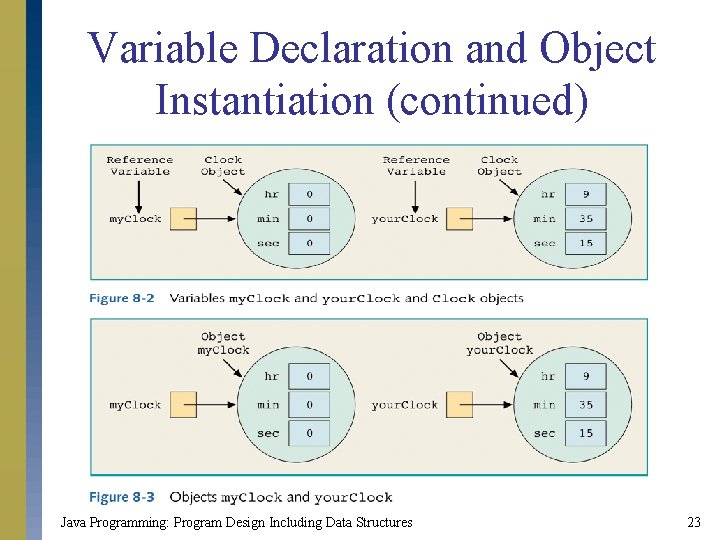
Variable Declaration and Object Instantiation (continued) Java Programming: Program Design Including Data Structures 23
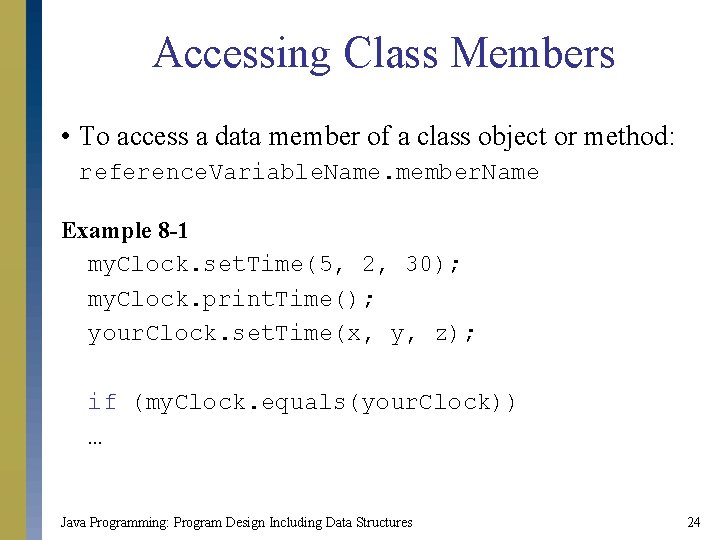
Accessing Class Members • To access a data member of a class object or method: reference. Variable. Name. member. Name Example 8 -1 my. Clock. set. Time(5, 2, 30); my. Clock. print. Time(); your. Clock. set. Time(x, y, z); if (my. Clock. equals(your. Clock)) … Java Programming: Program Design Including Data Structures 24
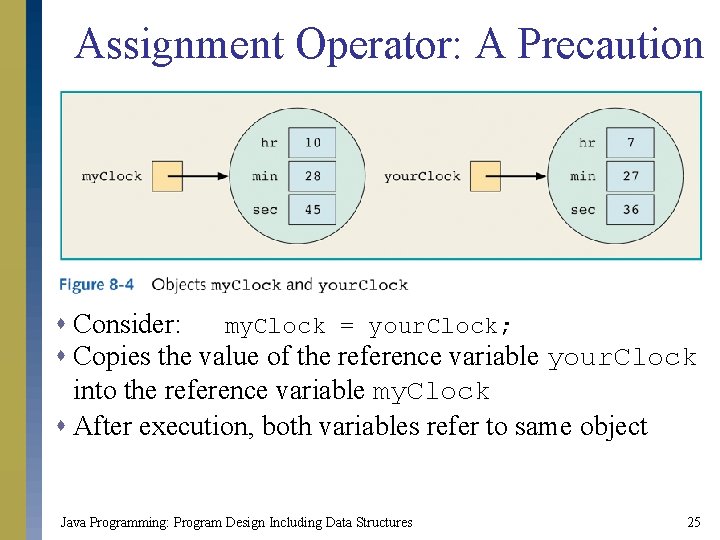
Assignment Operator: A Precaution s Consider: my. Clock = your. Clock; s Copies the value of the reference variable your. Clock into the reference variable my. Clock s After execution, both variables refer to same object Java Programming: Program Design Including Data Structures 25
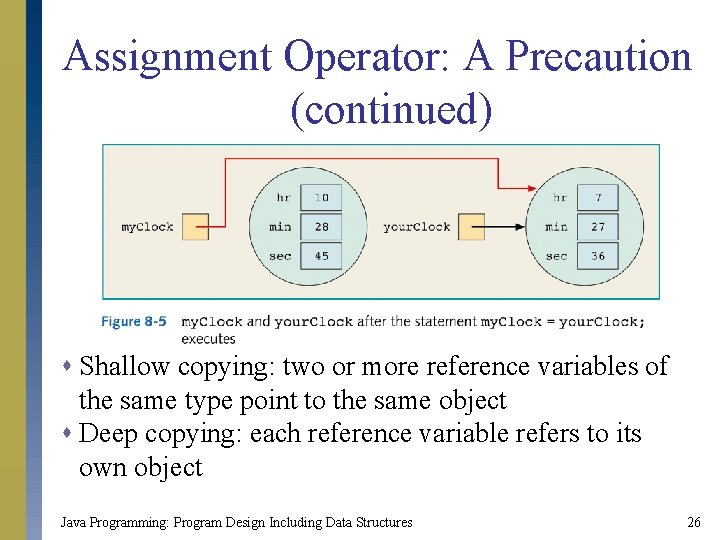
Assignment Operator: A Precaution (continued) s Shallow copying: two or more reference variables of the same type point to the same object s Deep copying: each reference variable refers to its own object Java Programming: Program Design Including Data Structures 26
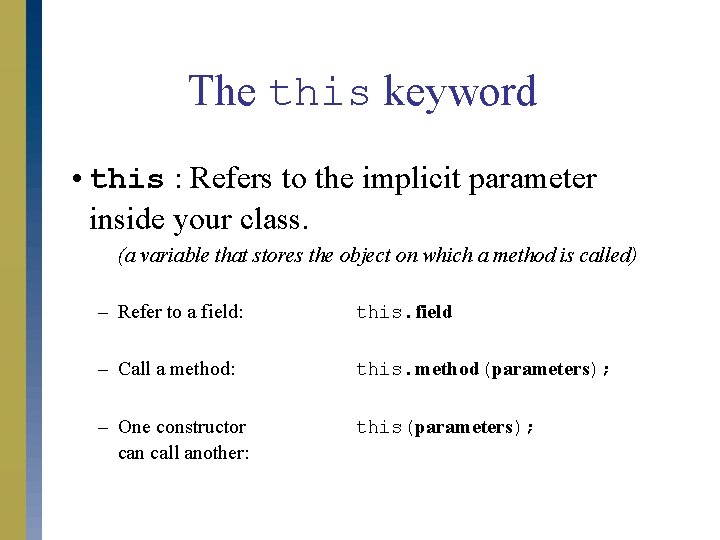
The this keyword • this : Refers to the implicit parameter inside your class. (a variable that stores the object on which a method is called) – Refer to a field: this. field – Call a method: this. method(parameters); – One constructor can call another: this(parameters);
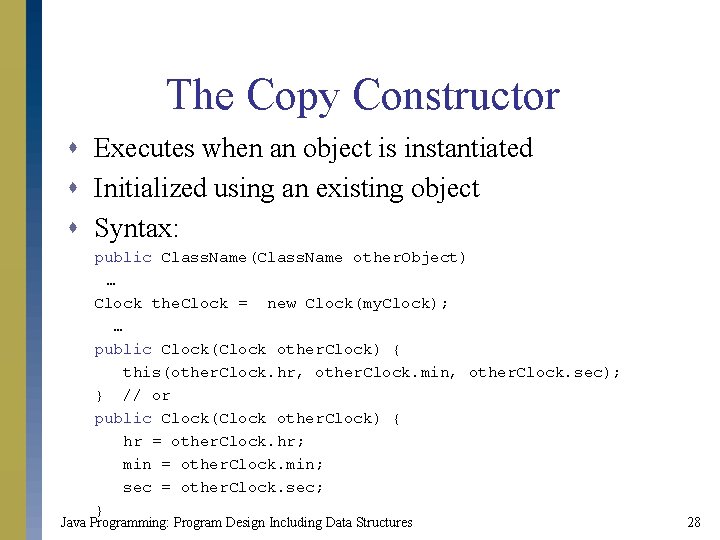
The Copy Constructor s Executes when an object is instantiated s Initialized using an existing object s Syntax: public Class. Name(Class. Name other. Object) … Clock the. Clock = new Clock(my. Clock); … public Clock(Clock other. Clock) { this(other. Clock. hr, other. Clock. min, other. Clock. sec); } // or public Clock(Clock other. Clock) { hr = other. Clock. hr; min = other. Clock. min; sec = other. Clock. sec; } Java Programming: Program Design Including Data Structures 28
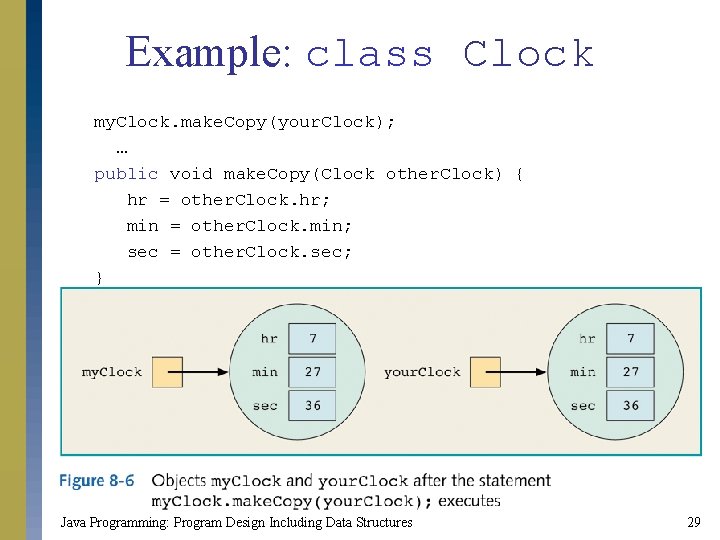
Example: class Clock my. Clock. make. Copy(your. Clock); … public void make. Copy(Clock other. Clock) { hr = other. Clock. hr; min = other. Clock. min; sec = other. Clock. sec; } Java Programming: Program Design Including Data Structures 29
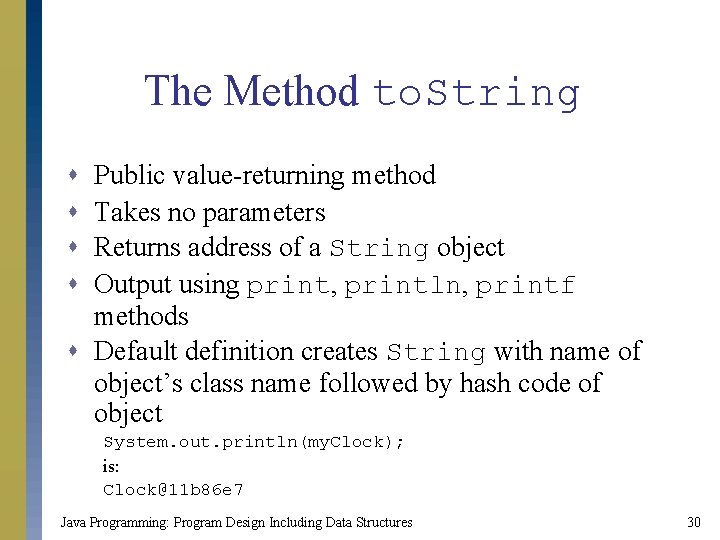
The Method to. String s s Public value-returning method Takes no parameters Returns address of a String object Output using print, println, printf methods s Default definition creates String with name of object’s class name followed by hash code of object System. out. println(my. Clock); is: Clock@11 b 86 e 7 Java Programming: Program Design Including Data Structures 30
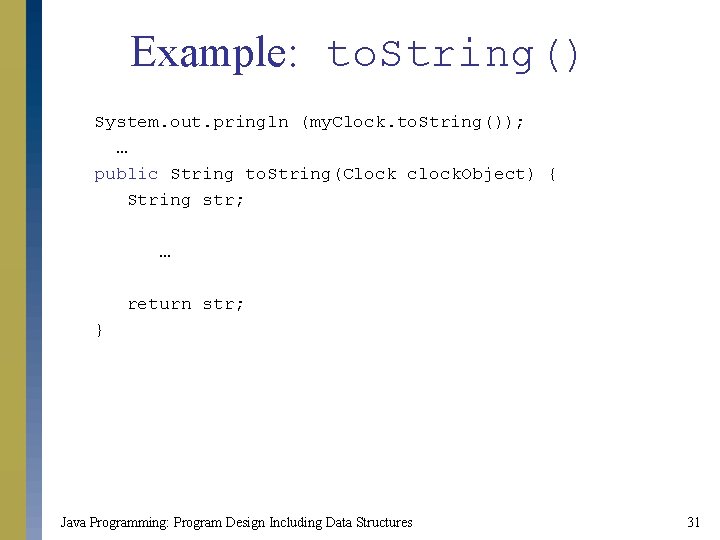
Example: to. String() System. out. pringln (my. Clock. to. String()); … public String to. String(Clock clock. Object) { String str; … return str; } Java Programming: Program Design Including Data Structures 31
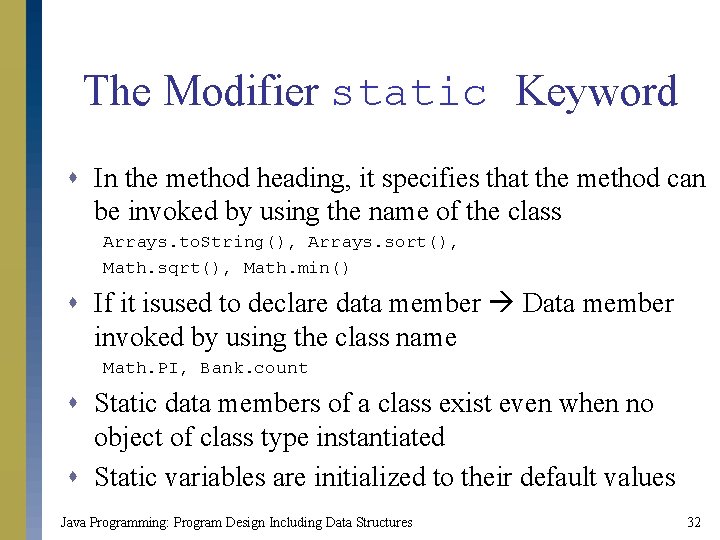
The Modifier static Keyword s In the method heading, it specifies that the method can be invoked by using the name of the class Arrays. to. String(), Arrays. sort(), Math. sqrt(), Math. min() s If it isused to declare data member Data member invoked by using the class name Math. PI, Bank. count s Static data members of a class exist even when no object of class type instantiated s Static variables are initialized to their default values Java Programming: Program Design Including Data Structures 32
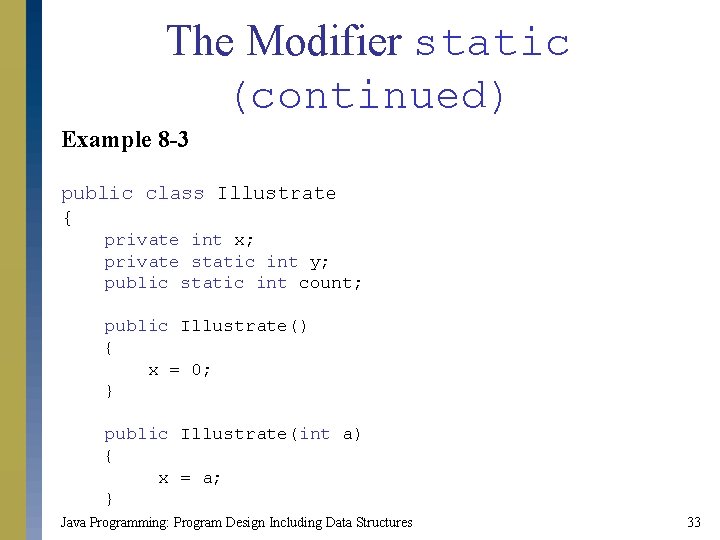
The Modifier static (continued) Example 8 -3 public class Illustrate { private int x; private static int y; public static int count; public Illustrate() { x = 0; } public Illustrate(int a) { x = a; } Java Programming: Program Design Including Data Structures 33
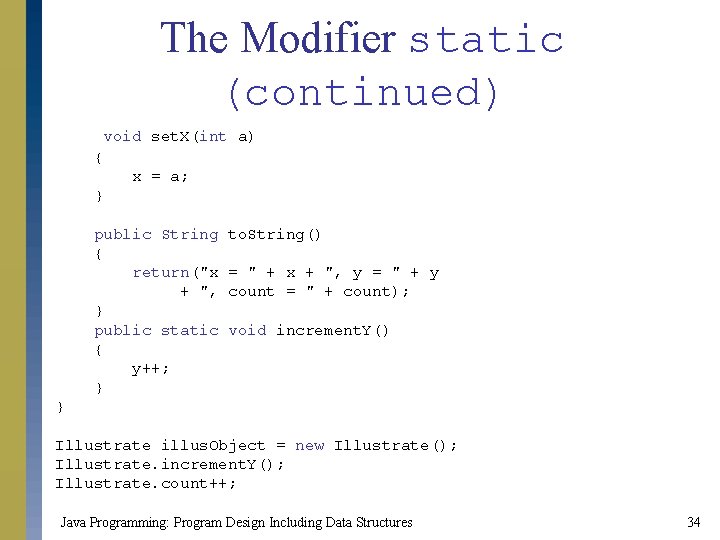
The Modifier static (continued) void set. X(int a) { x = a; } public String { return("x + ", } public static { y++; } to. String() = " + x + ", y = " + y count = " + count); void increment. Y() } Illustrate illus. Object = new Illustrate(); Illustrate. increment. Y(); Illustrate. count++; Java Programming: Program Design Including Data Structures 34
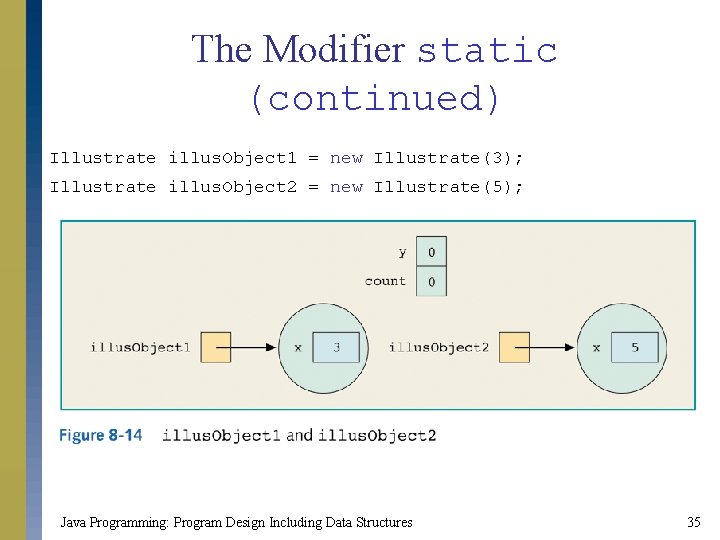
The Modifier static (continued) Illustrate illus. Object 1 = new Illustrate(3); Illustrate illus. Object 2 = new Illustrate(5); Java Programming: Program Design Including Data Structures 35
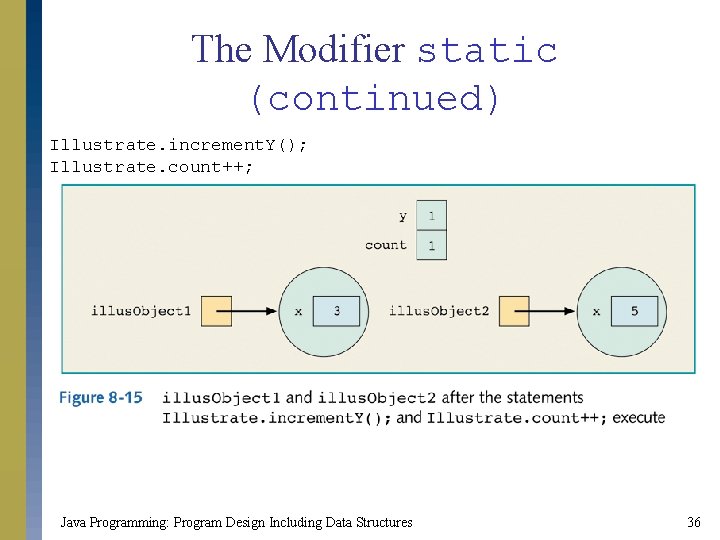
The Modifier static (continued) Illustrate. increment. Y(); Illustrate. count++; Java Programming: Program Design Including Data Structures 36
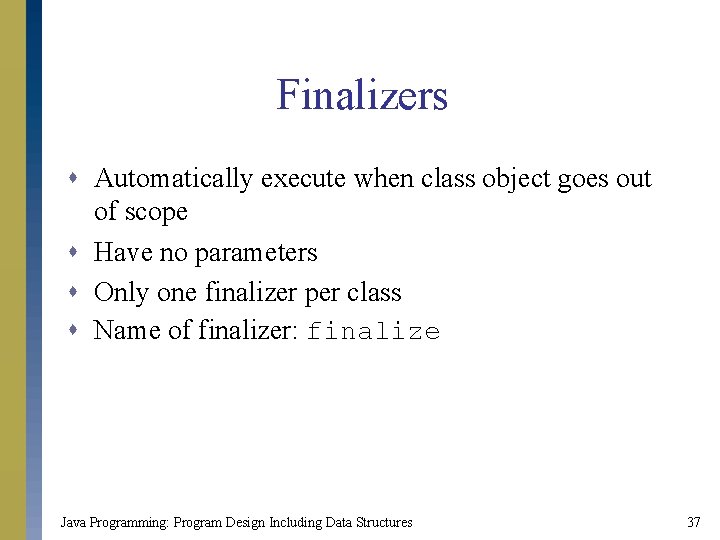
Finalizers s Automatically execute when class object goes out of scope s Have no parameters s Only one finalizer per class s Name of finalizer: finalize Java Programming: Program Design Including Data Structures 37
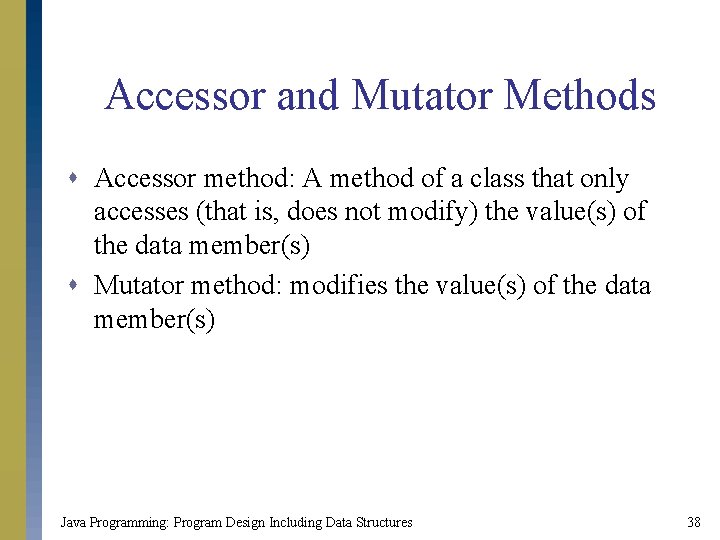
Accessor and Mutator Methods s Accessor method: A method of a class that only accesses (that is, does not modify) the value(s) of the data member(s) s Mutator method: modifies the value(s) of the data member(s) Java Programming: Program Design Including Data Structures 38
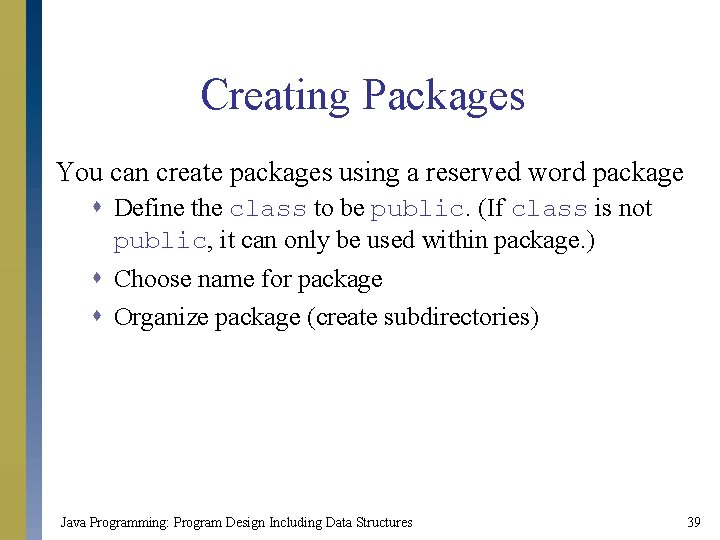
Creating Packages You can create packages using a reserved word package s Define the class to be public. (If class is not public, it can only be used within package. ) s Choose name for package s Organize package (create subdirectories) Java Programming: Program Design Including Data Structures 39
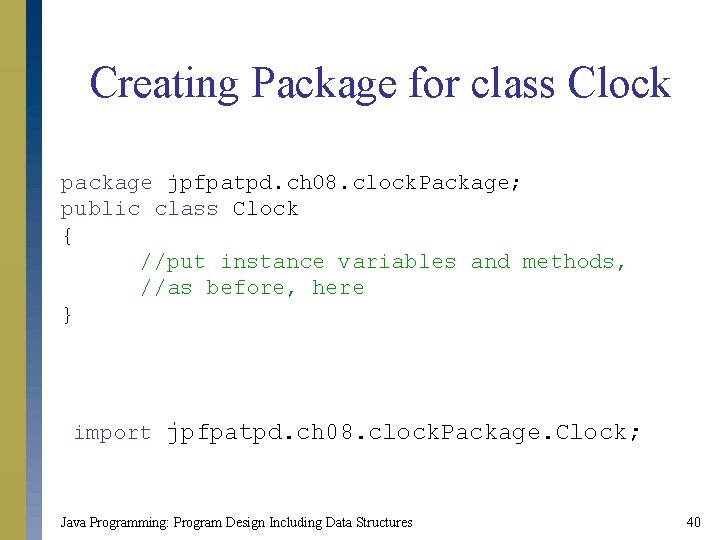
Creating Package for class Clock package jpfpatpd. ch 08. clock. Package; public class Clock { //put instance variables and methods, //as before, here } import jpfpatpd. ch 08. clock. Package. Clock; Java Programming: Program Design Including Data Structures 40
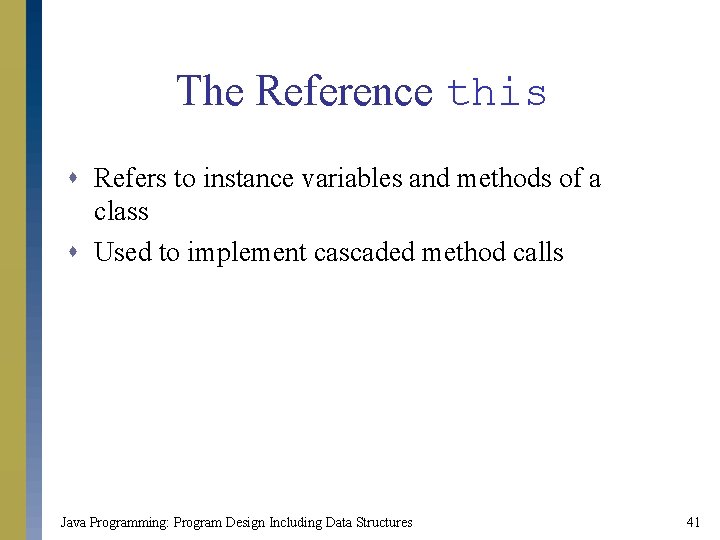
The Reference this s Refers to instance variables and methods of a class s Used to implement cascaded method calls Java Programming: Program Design Including Data Structures 41
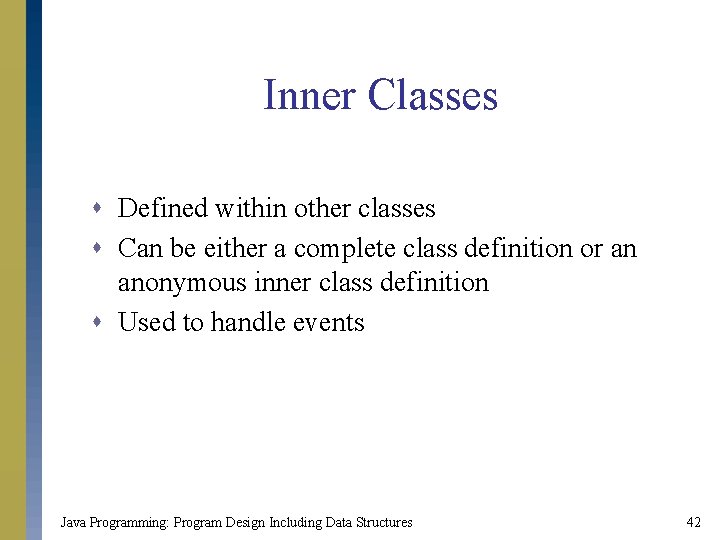
Inner Classes s Defined within other classes s Can be either a complete class definition or an anonymous inner class definition s Used to handle events Java Programming: Program Design Including Data Structures 42
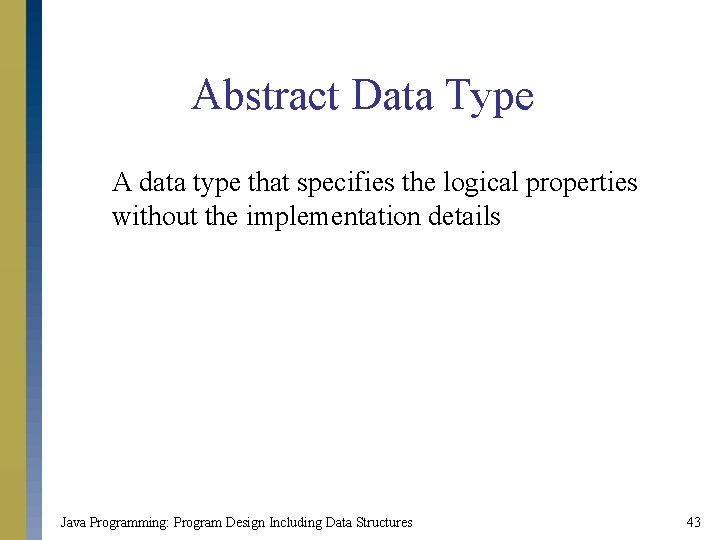
Abstract Data Type A data type that specifies the logical properties without the implementation details Java Programming: Program Design Including Data Structures 43
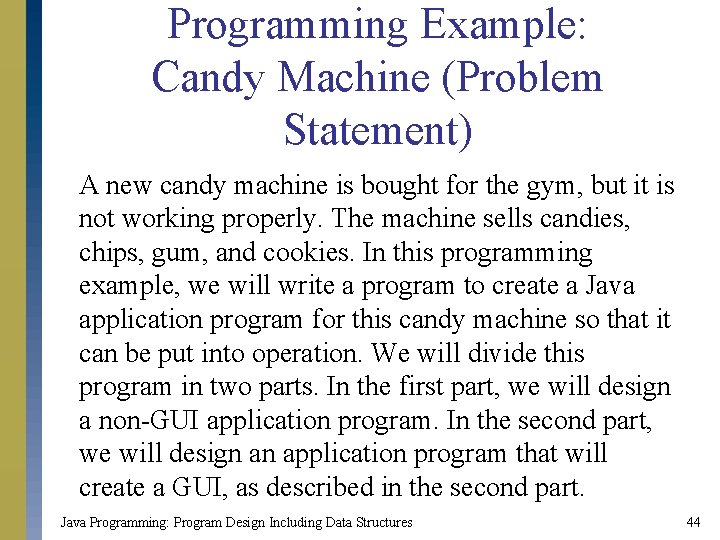
Programming Example: Candy Machine (Problem Statement) A new candy machine is bought for the gym, but it is not working properly. The machine sells candies, chips, gum, and cookies. In this programming example, we will write a program to create a Java application program for this candy machine so that it can be put into operation. We will divide this program in two parts. In the first part, we will design a non-GUI application program. In the second part, we will design an application program that will create a GUI, as described in the second part. Java Programming: Program Design Including Data Structures 44
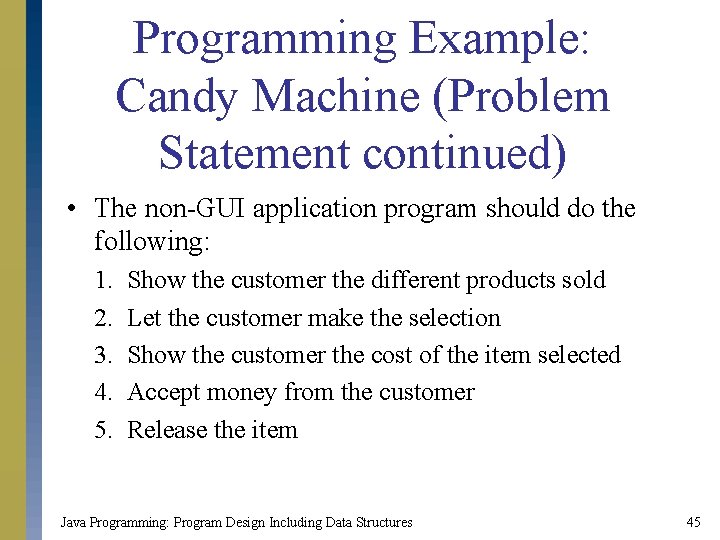
Programming Example: Candy Machine (Problem Statement continued) • The non-GUI application program should do the following: 1. 2. 3. 4. 5. Show the customer the different products sold Let the customer make the selection Show the customer the cost of the item selected Accept money from the customer Release the item Java Programming: Program Design Including Data Structures 45
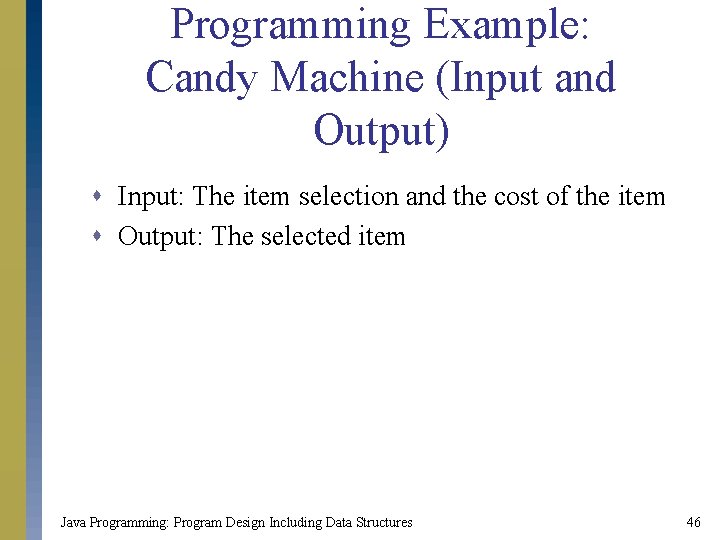
Programming Example: Candy Machine (Input and Output) s Input: The item selection and the cost of the item s Output: The selected item Java Programming: Program Design Including Data Structures 46
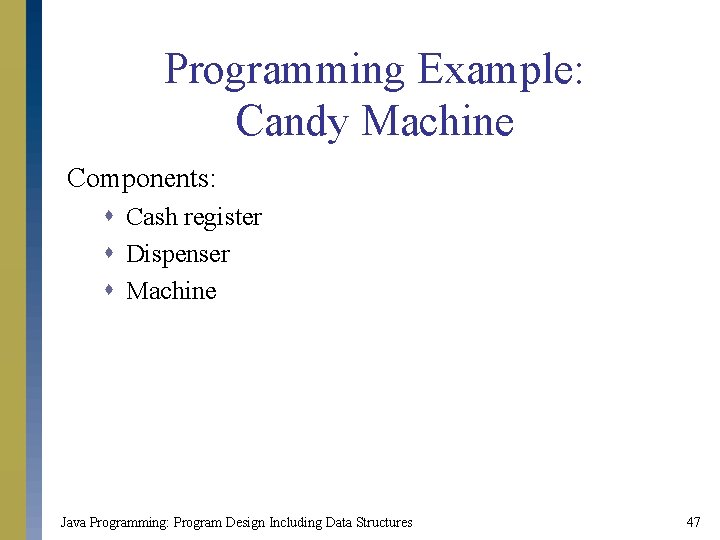
Programming Example: Candy Machine Components: s Cash register s Dispenser s Machine Java Programming: Program Design Including Data Structures 47
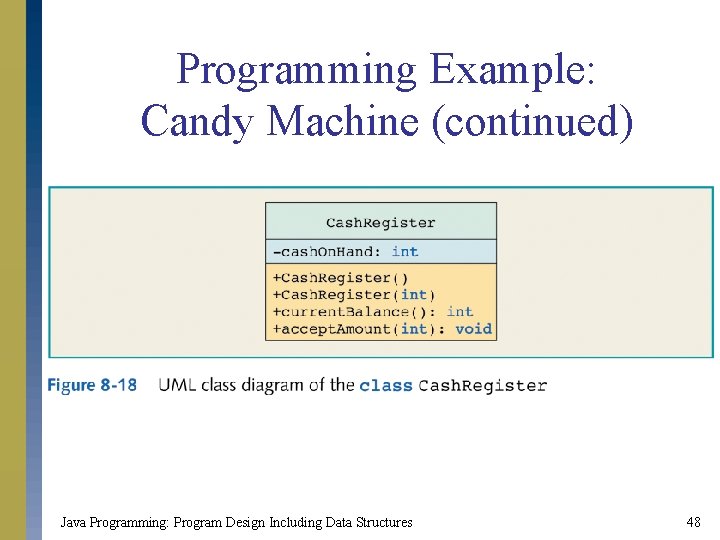
Programming Example: Candy Machine (continued) Java Programming: Program Design Including Data Structures 48
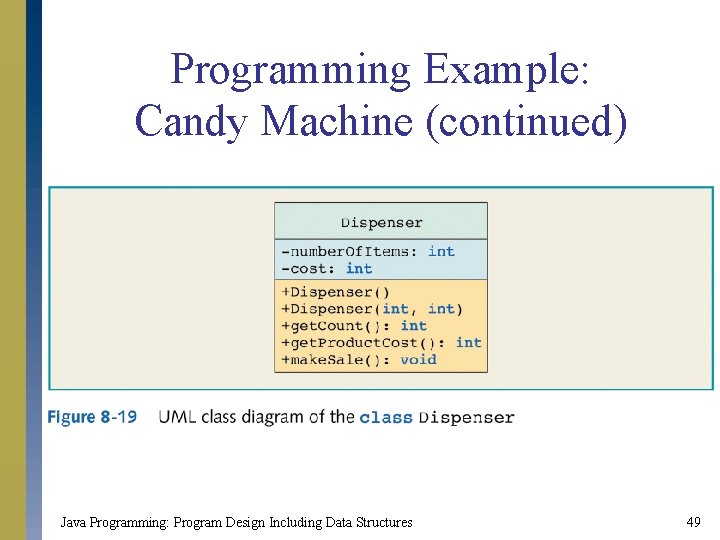
Programming Example: Candy Machine (continued) Java Programming: Program Design Including Data Structures 49
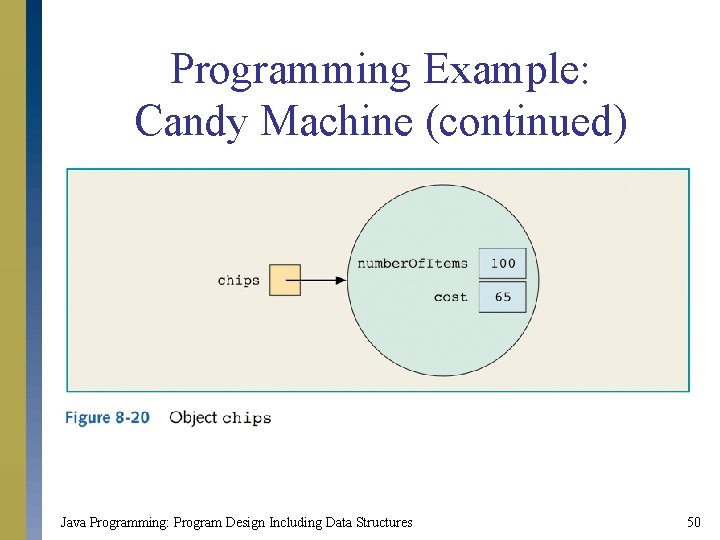
Programming Example: Candy Machine (continued) Java Programming: Program Design Including Data Structures 50
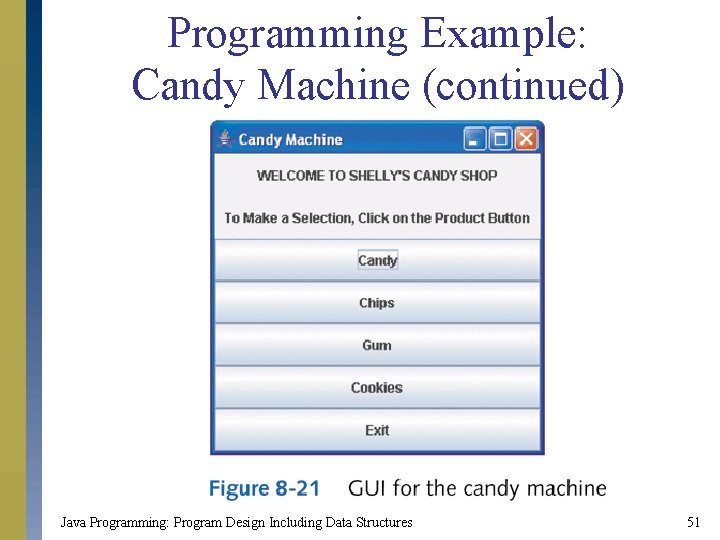
Programming Example: Candy Machine (continued) Java Programming: Program Design Including Data Structures 51
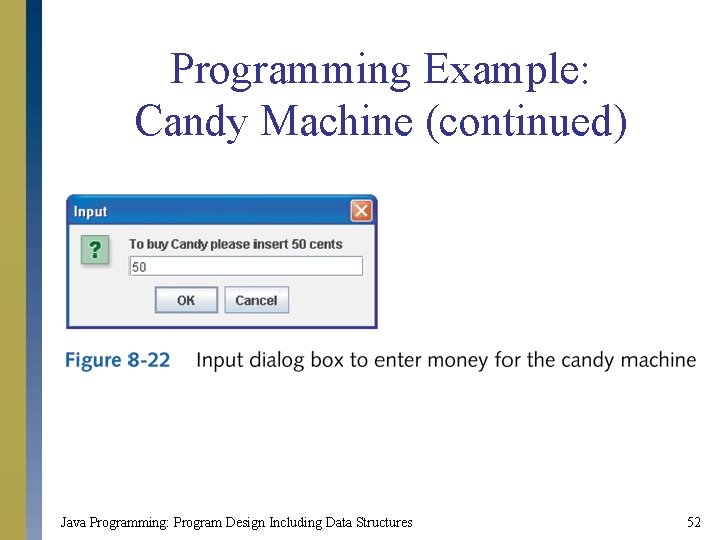
Programming Example: Candy Machine (continued) Java Programming: Program Design Including Data Structures 52
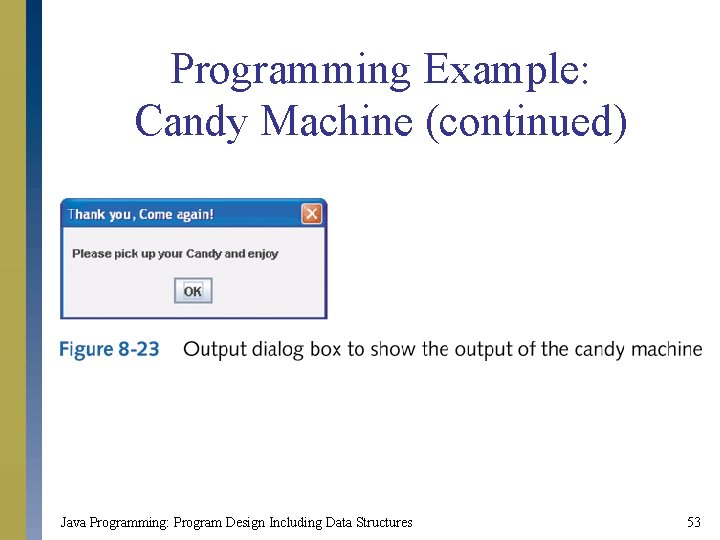
Programming Example: Candy Machine (continued) Java Programming: Program Design Including Data Structures 53
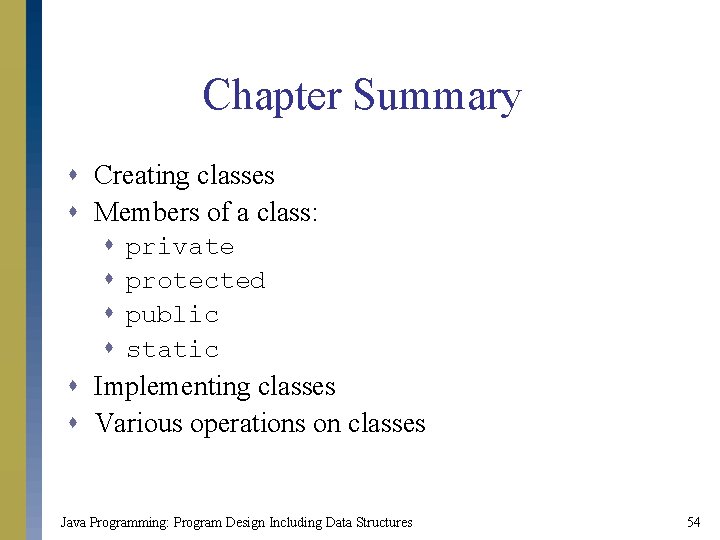
Chapter Summary s Creating classes s Members of a class: s s private protected public static s Implementing classes s Various operations on classes Java Programming: Program Design Including Data Structures 54
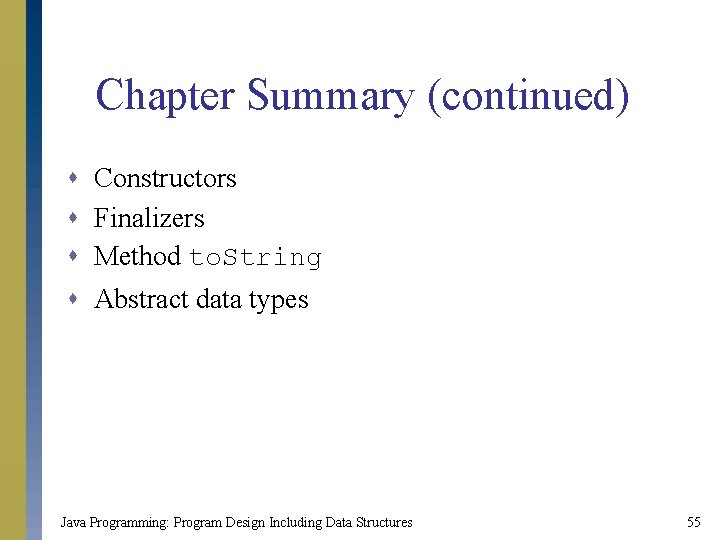
Chapter Summary (continued) s s Constructors Finalizers Method to. String Abstract data types Java Programming: Program Design Including Data Structures 55