Chapter 8 UserDefined Classes and ADTs Java Programming
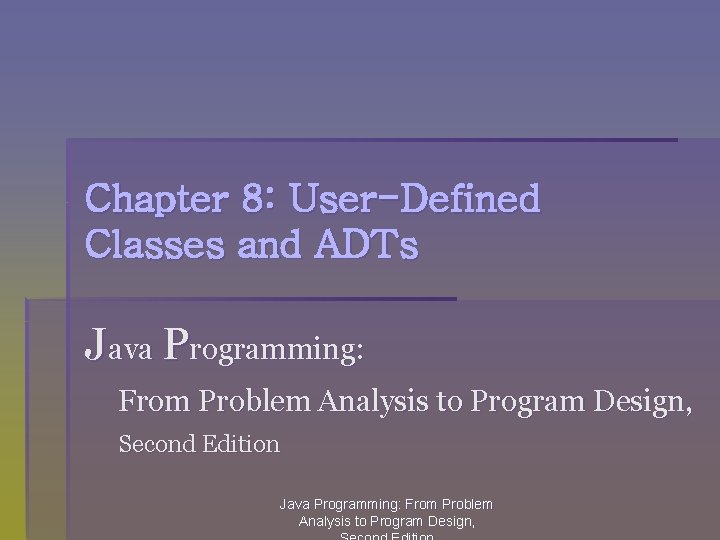
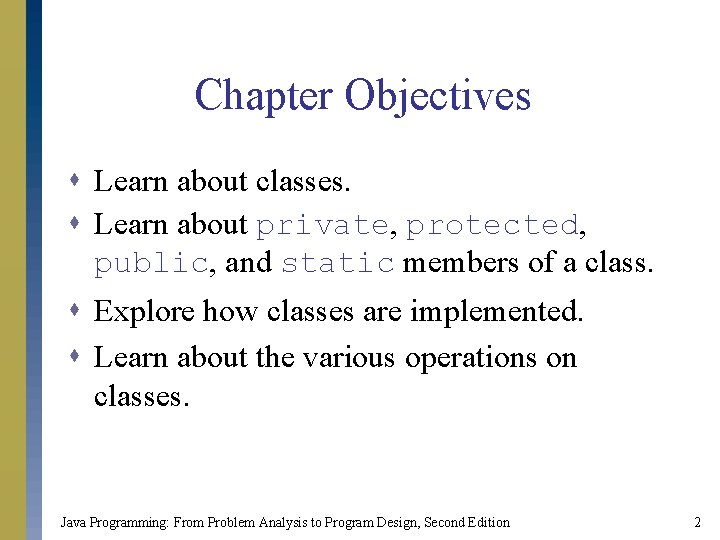
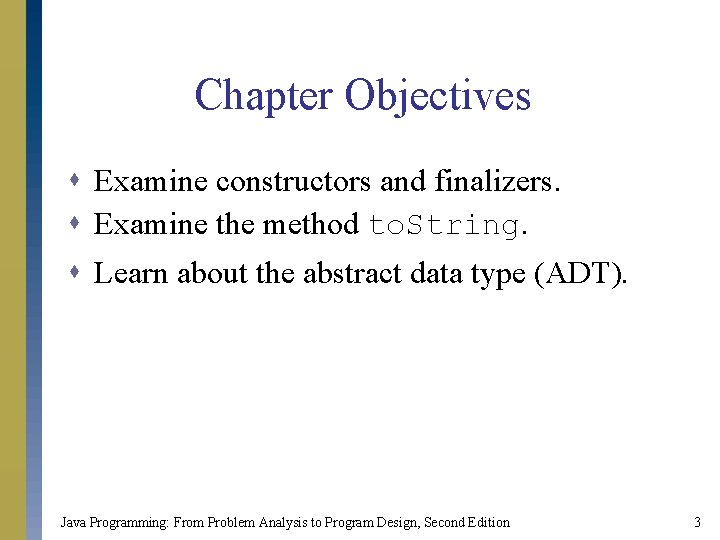
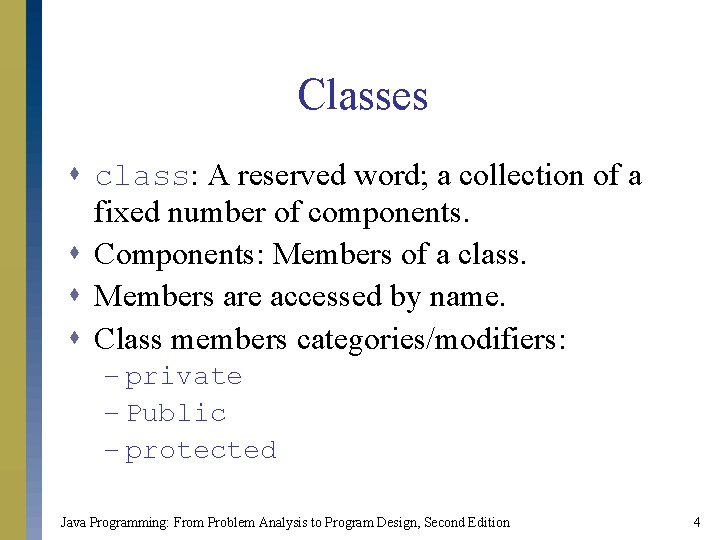
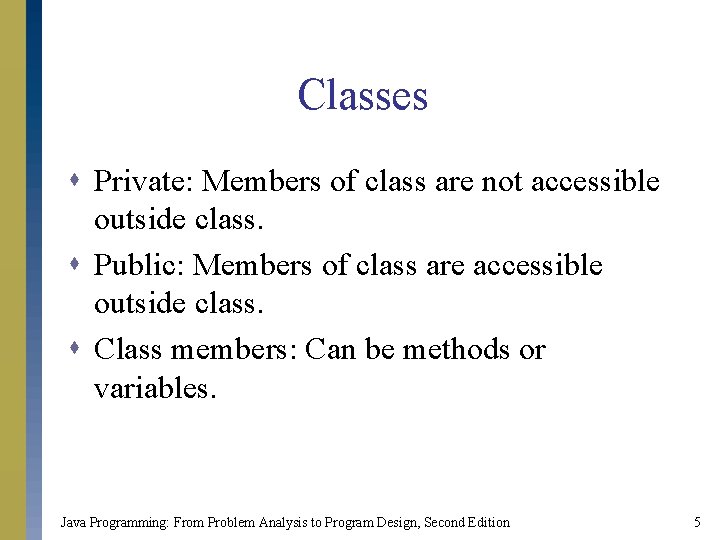
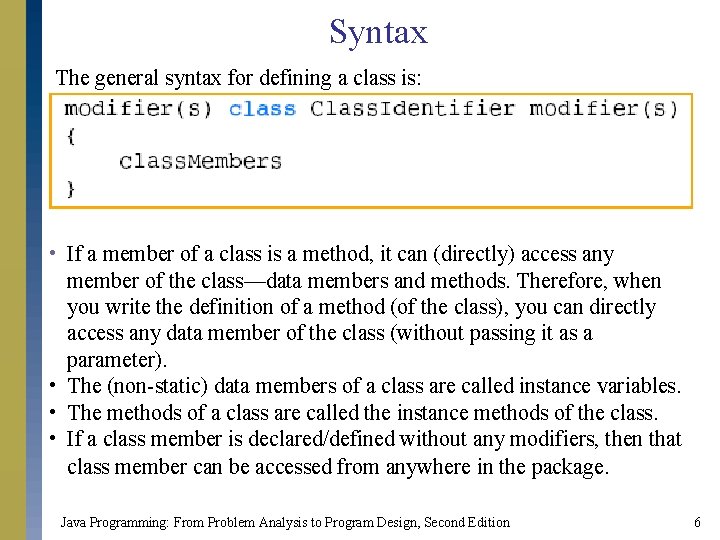
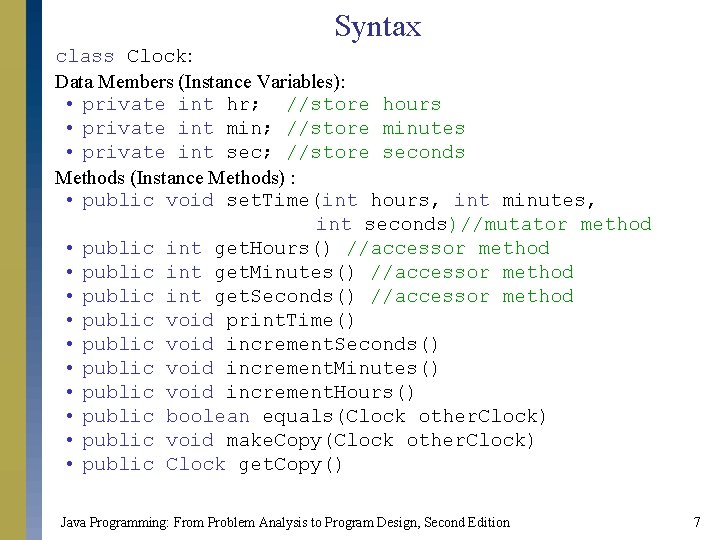
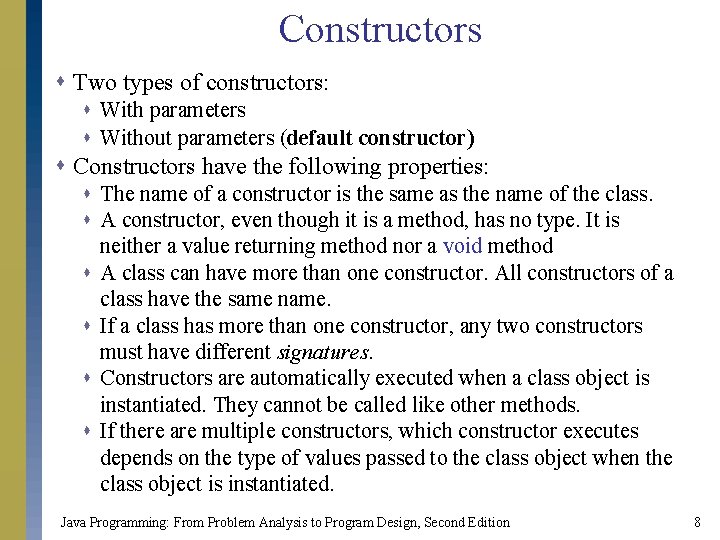
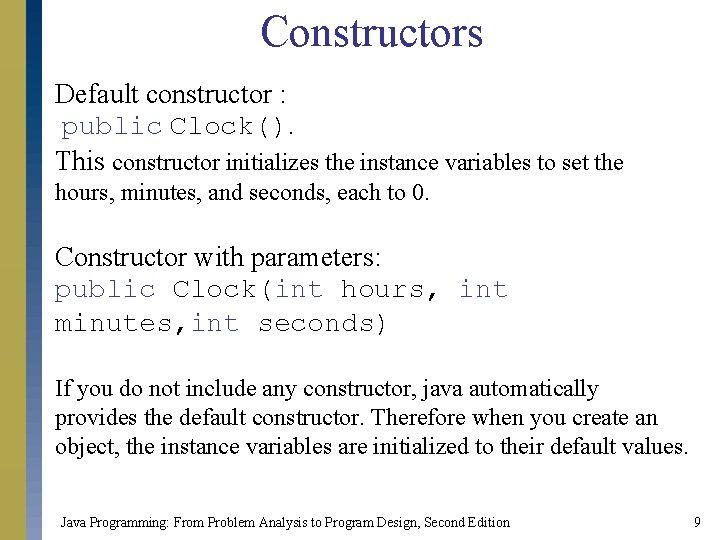
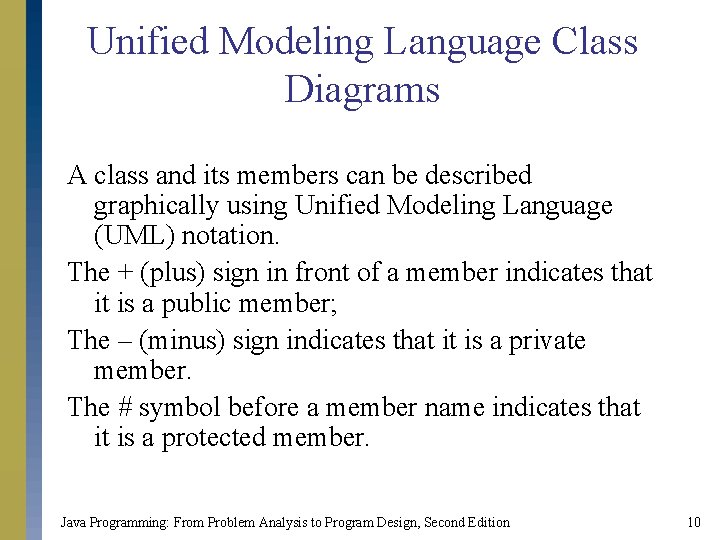
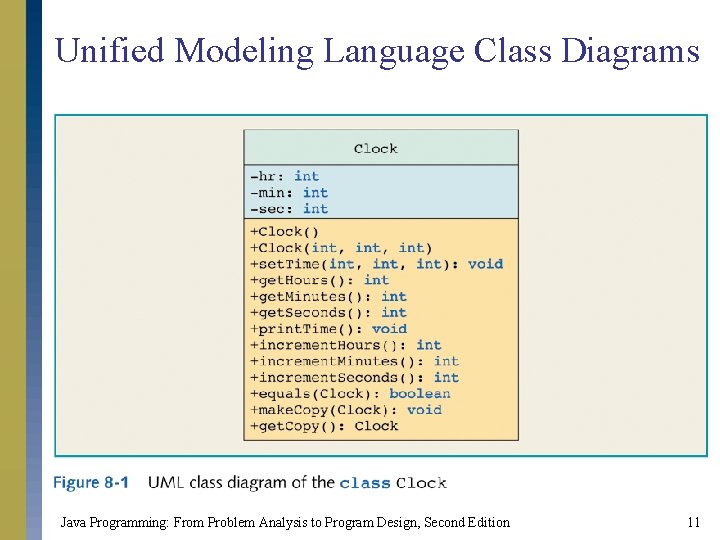
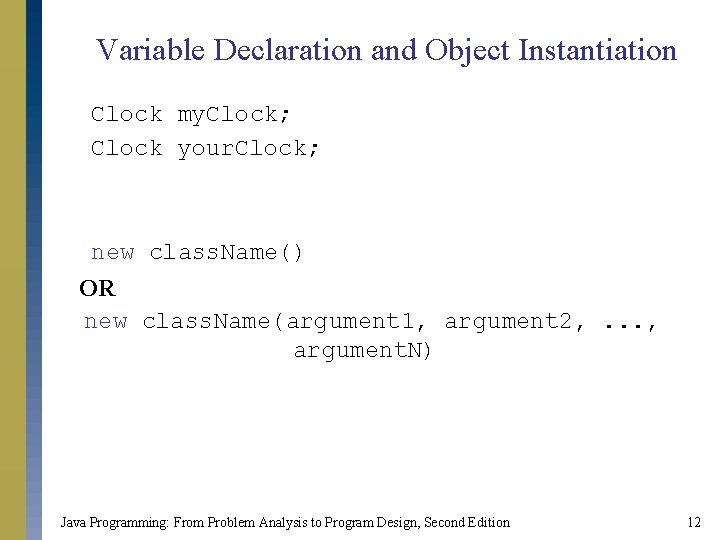
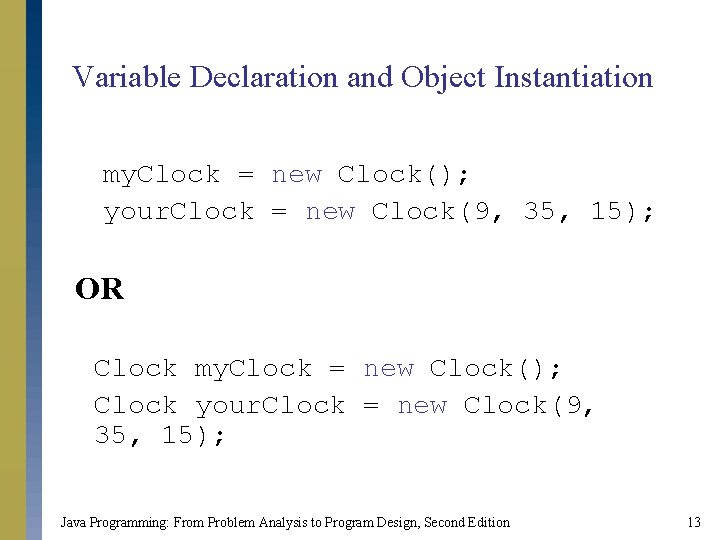
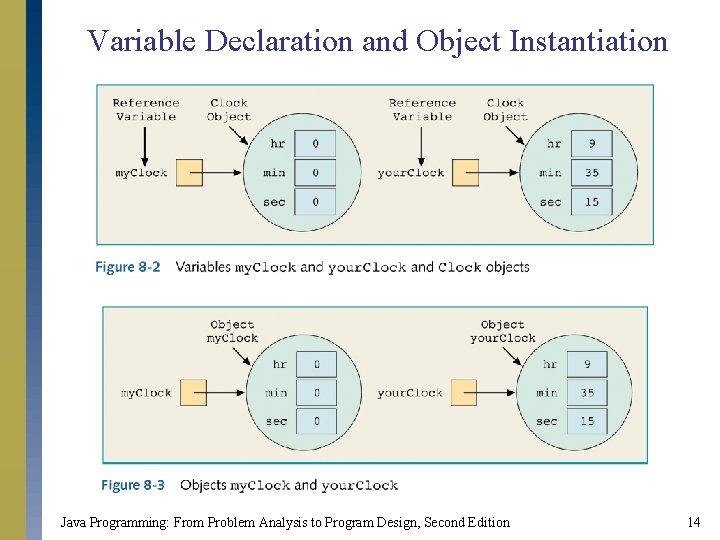
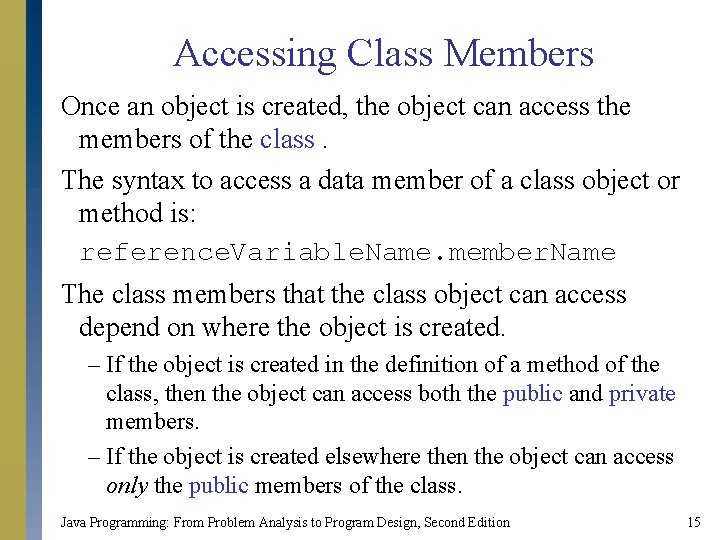
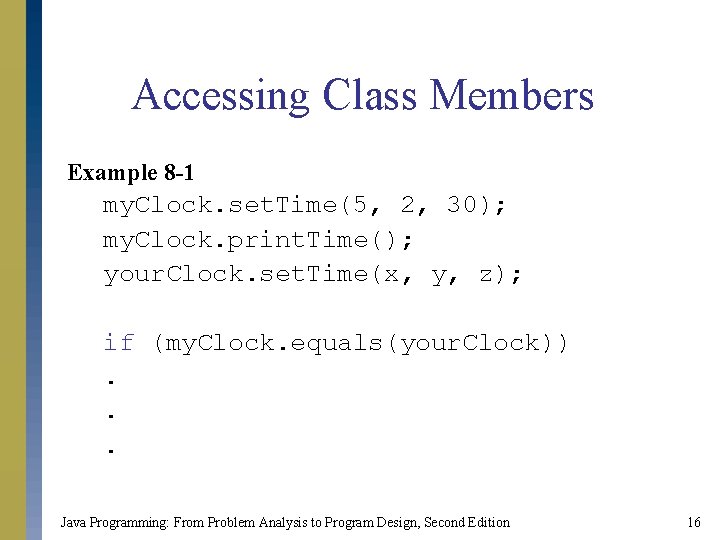
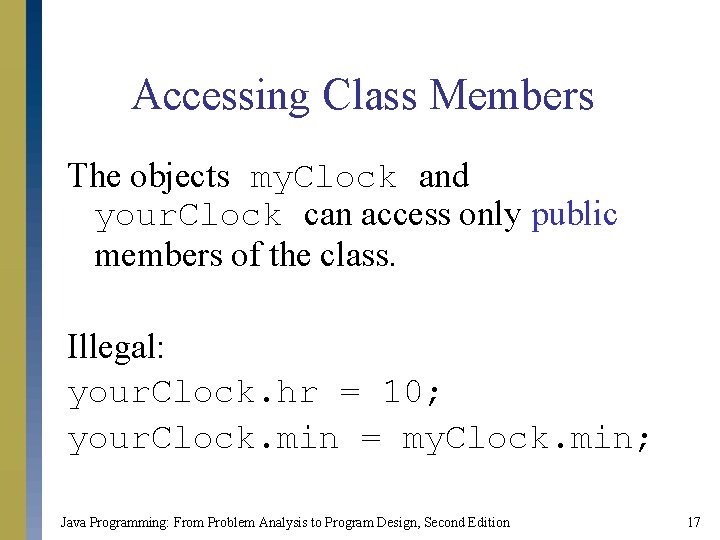
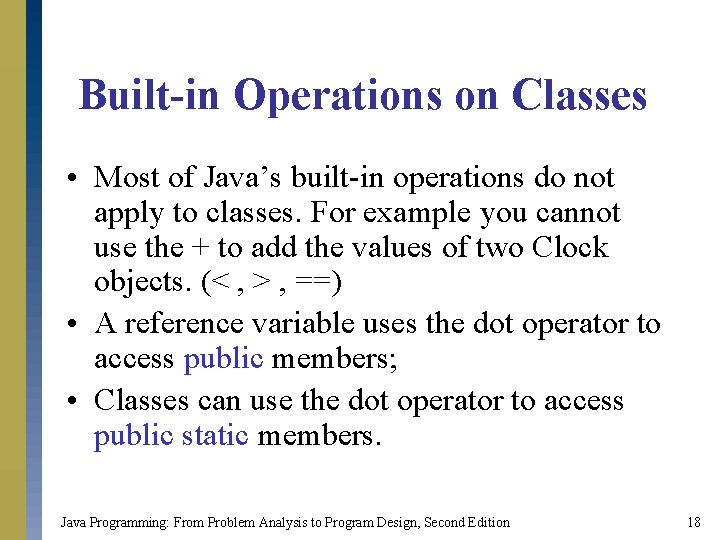
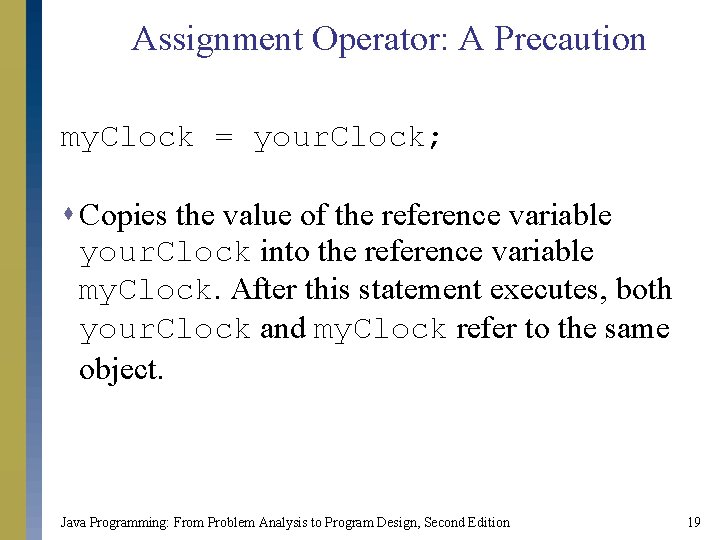
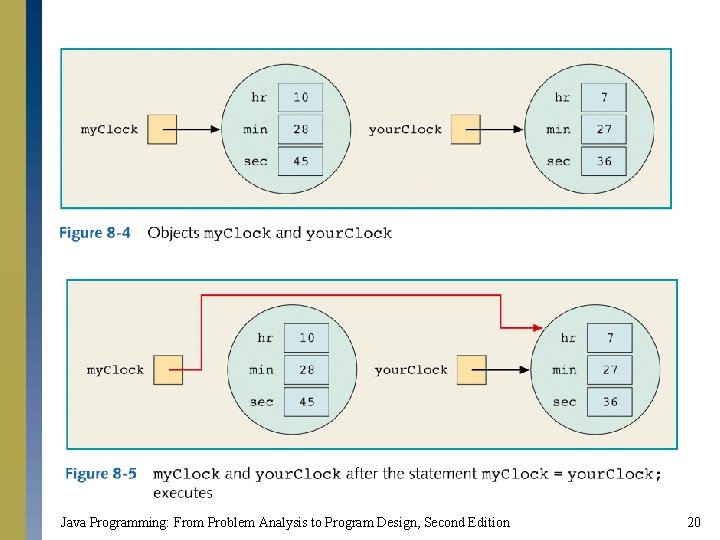
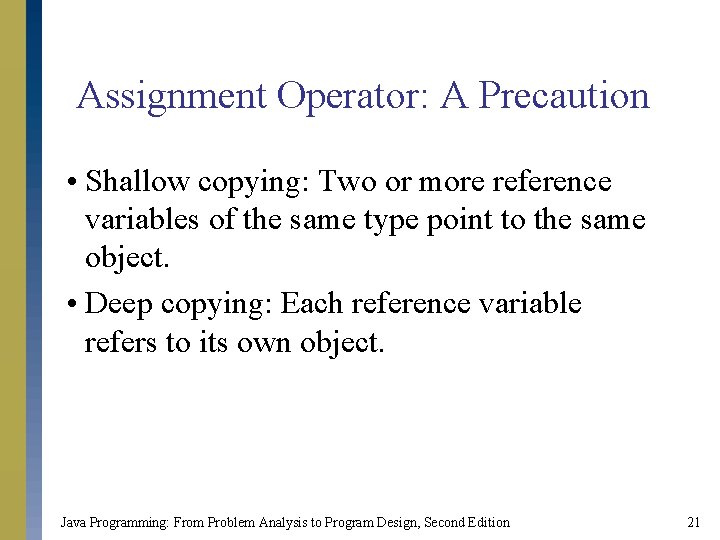
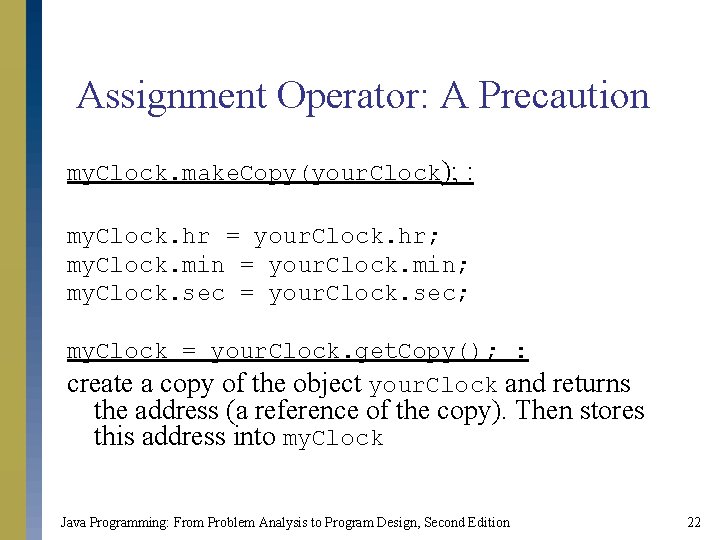
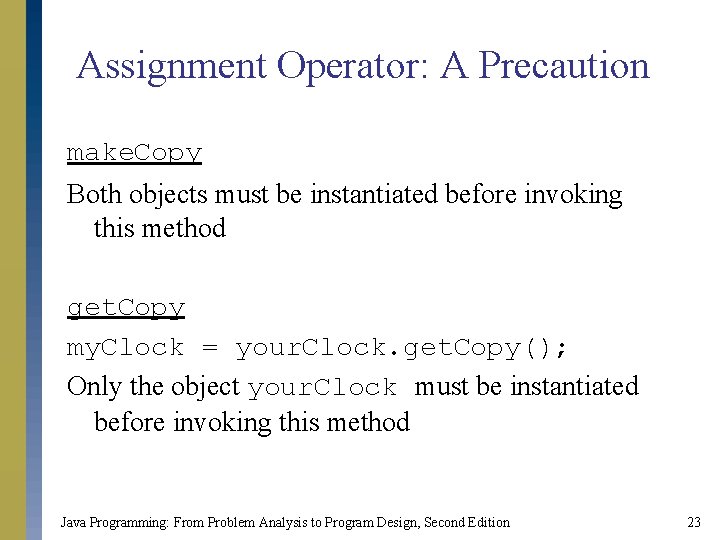
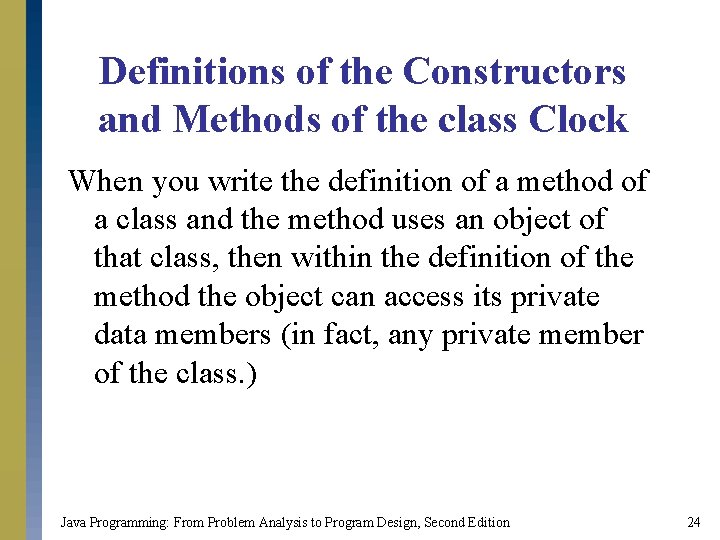
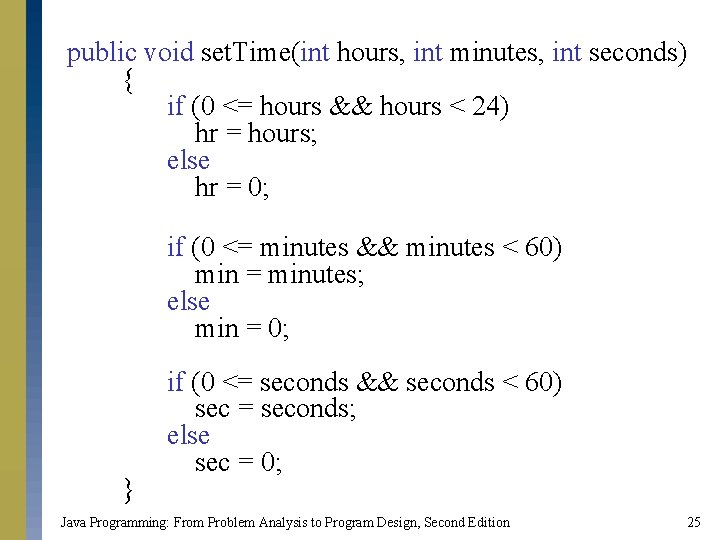
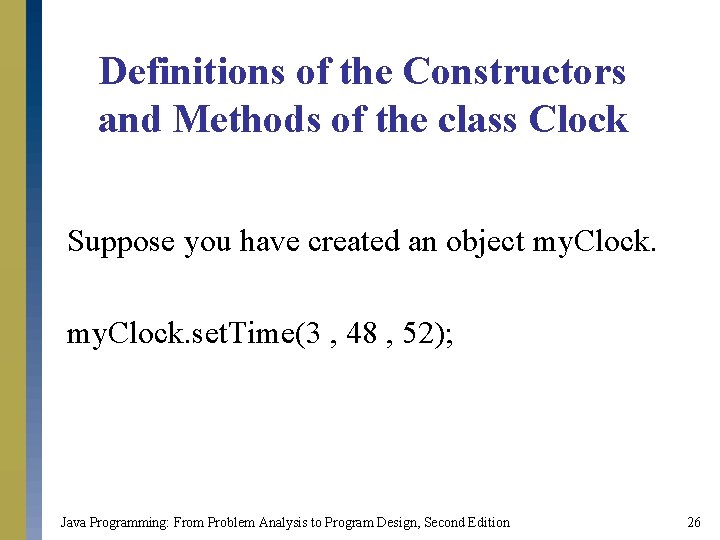
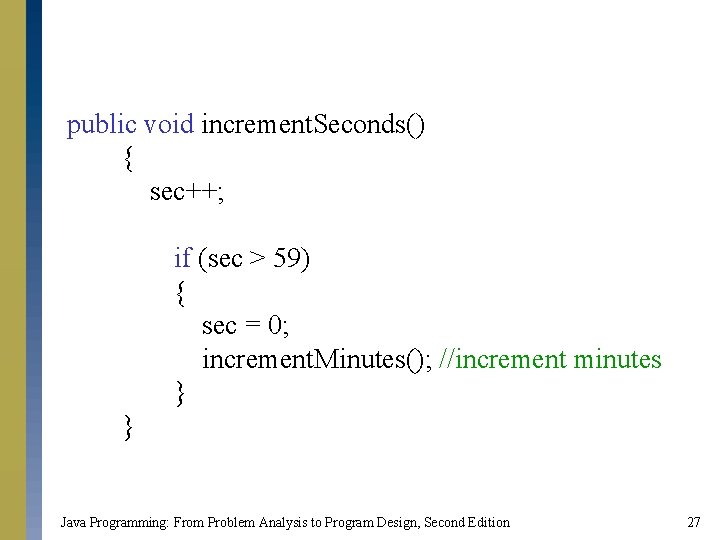
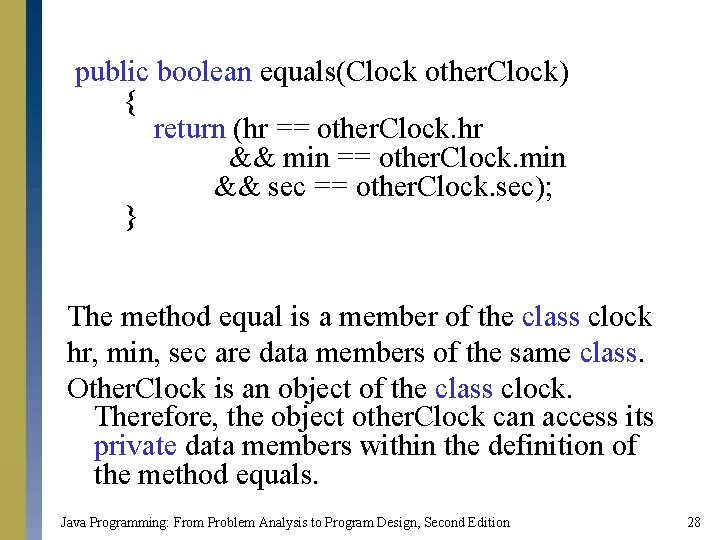
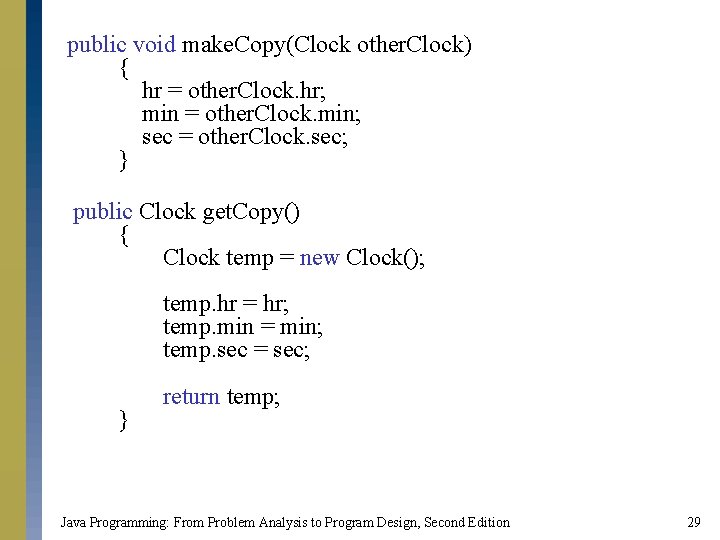
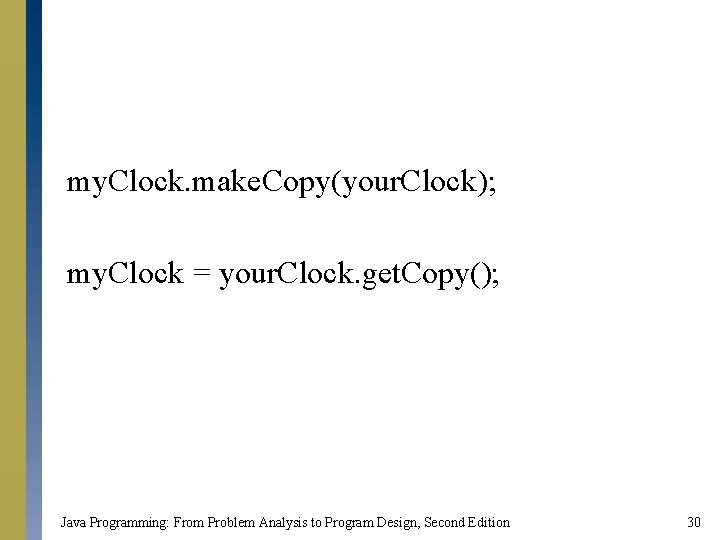
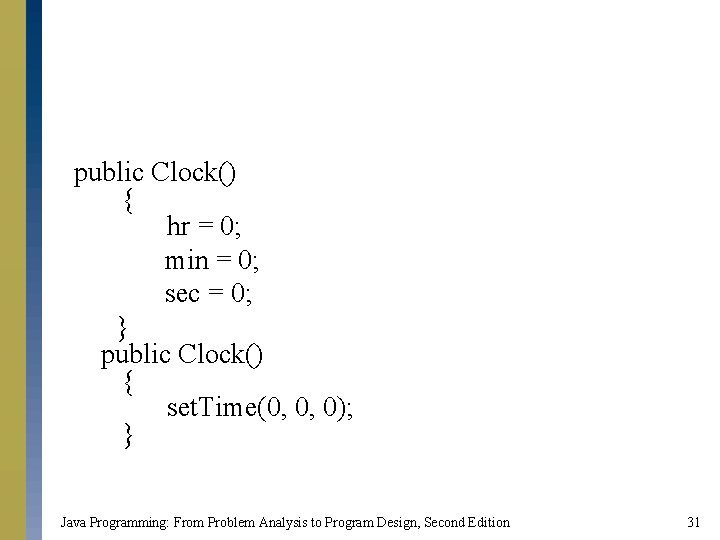
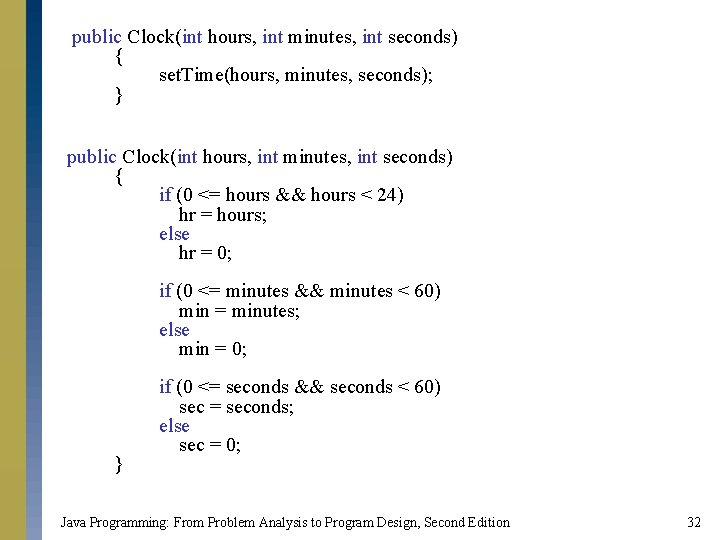
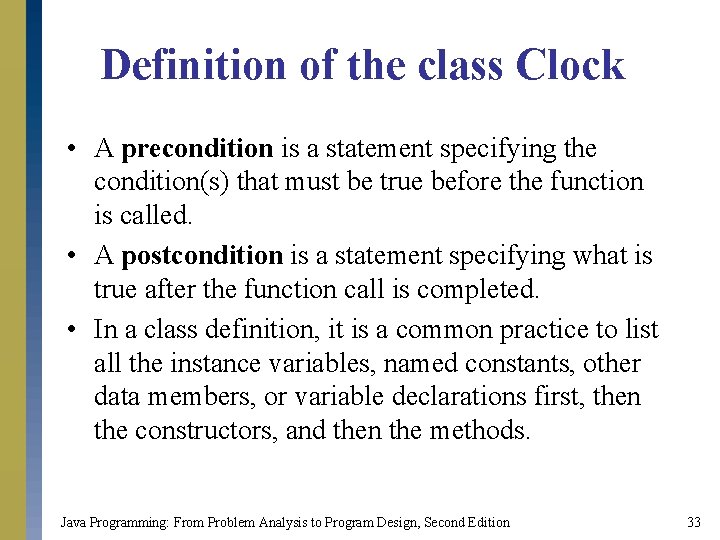
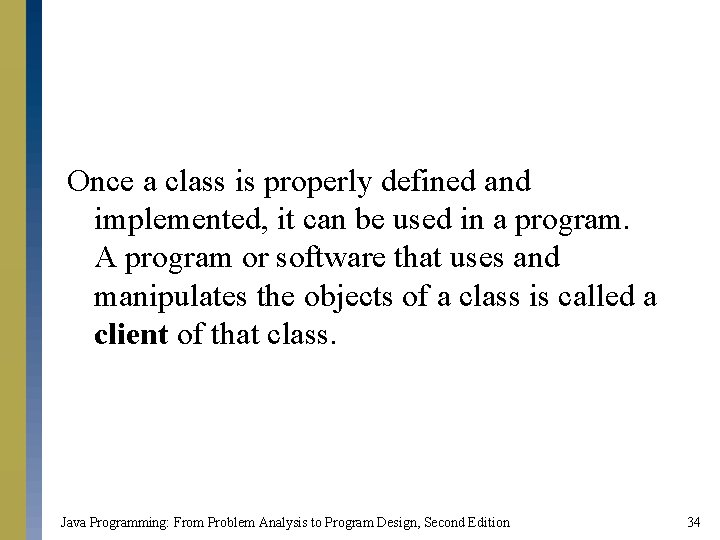
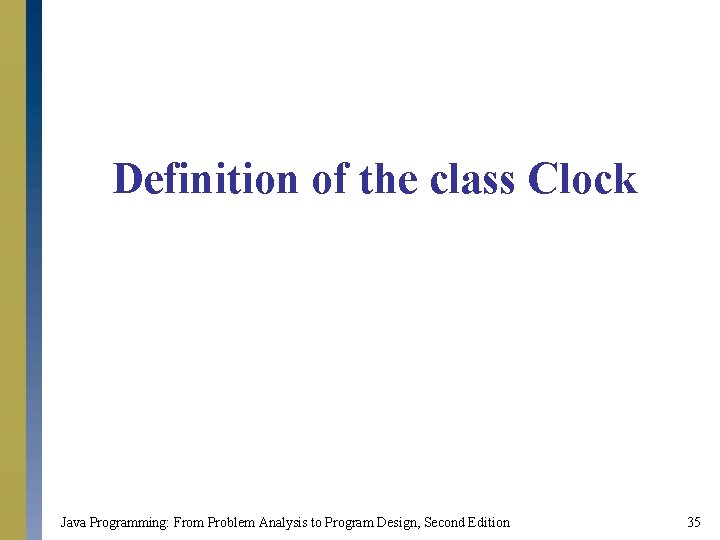
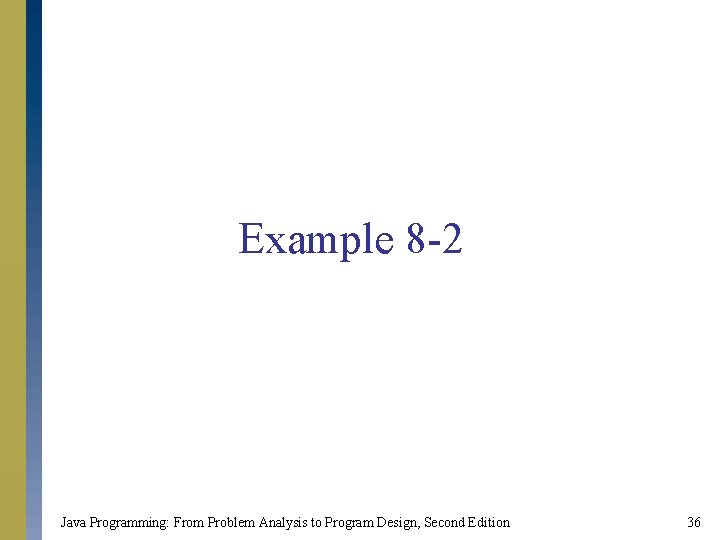
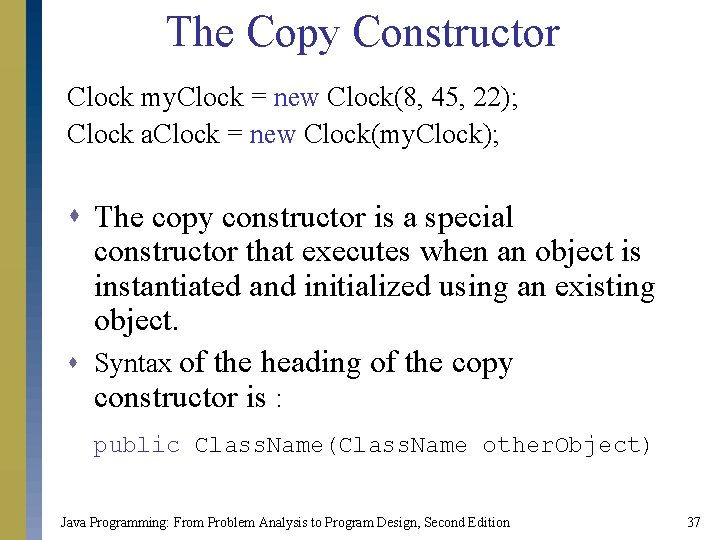
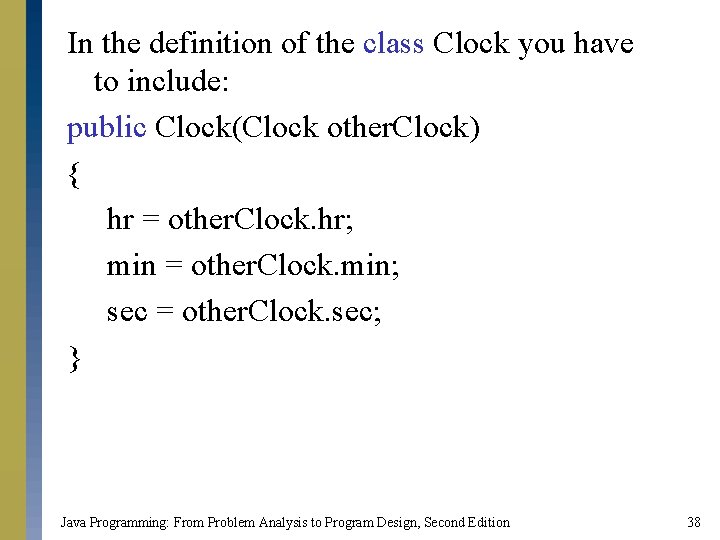
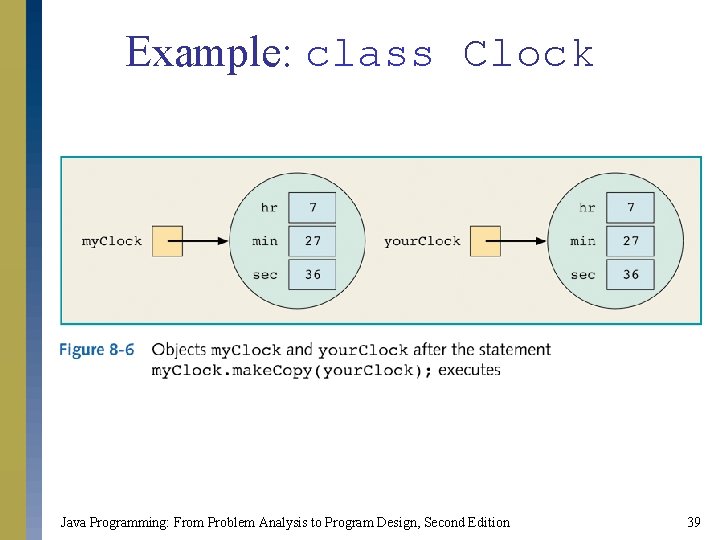
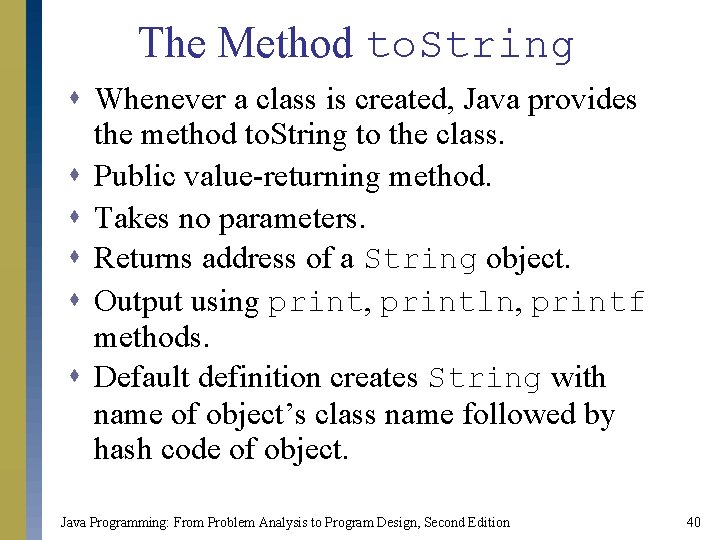
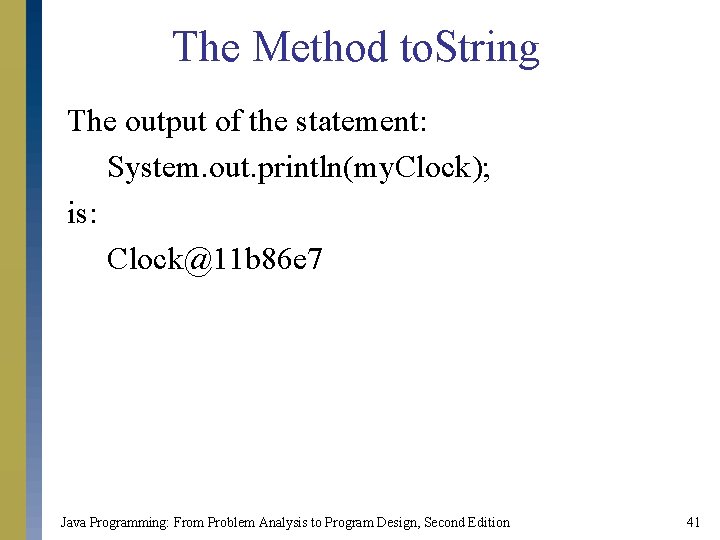
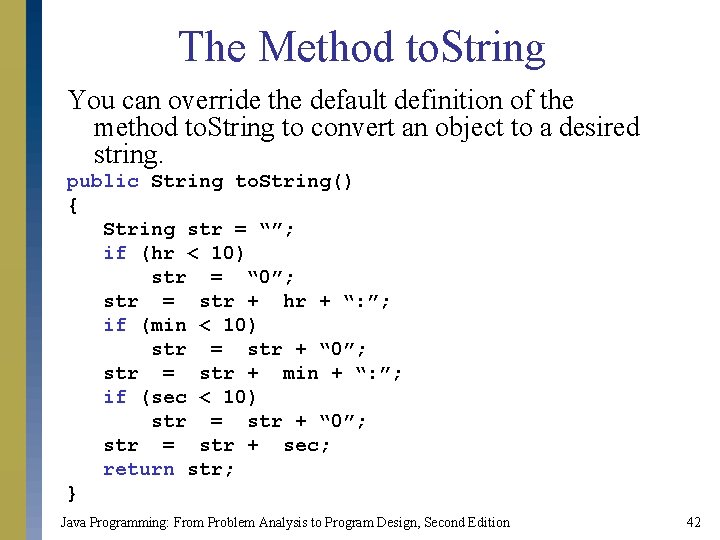
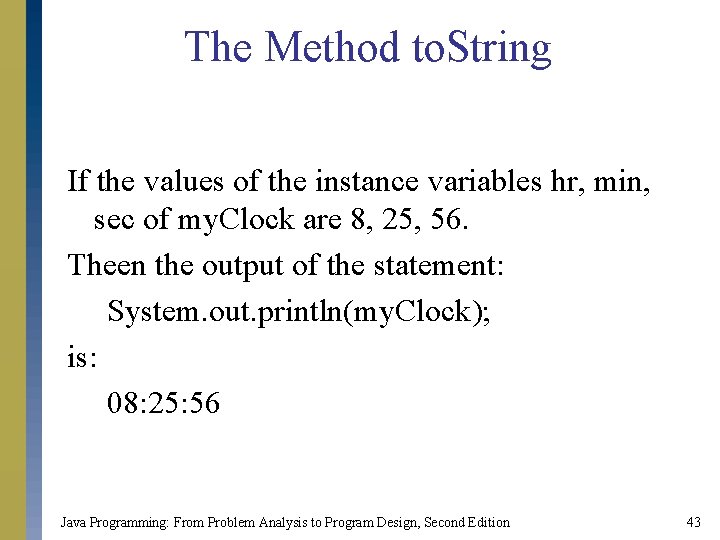
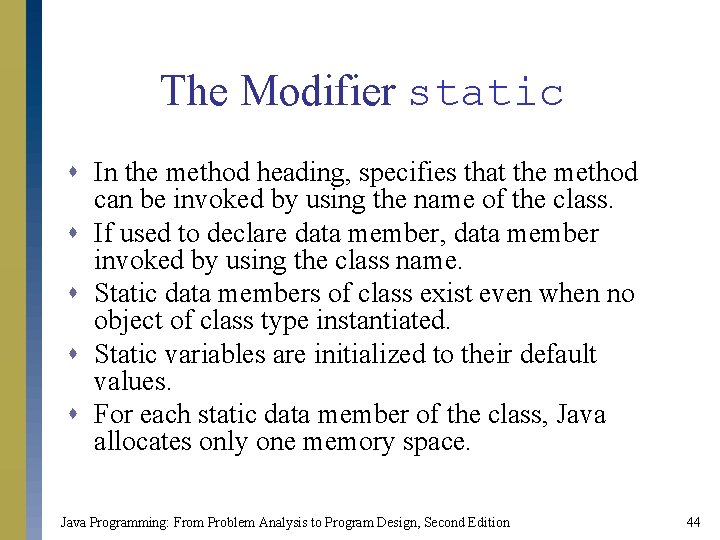
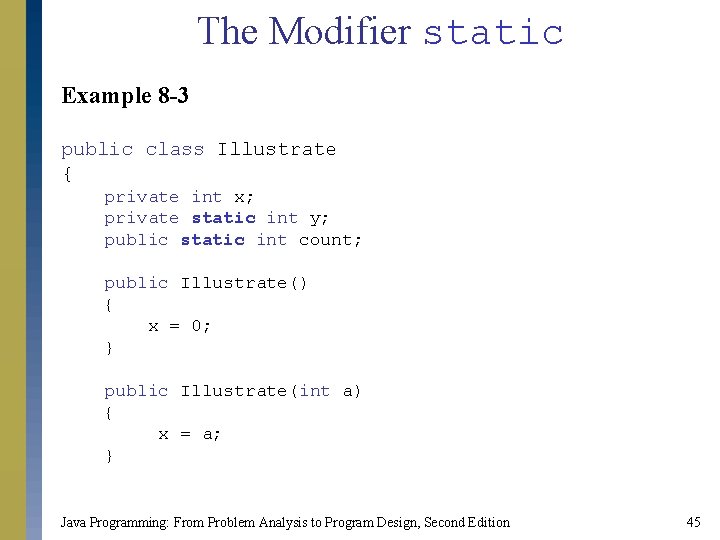
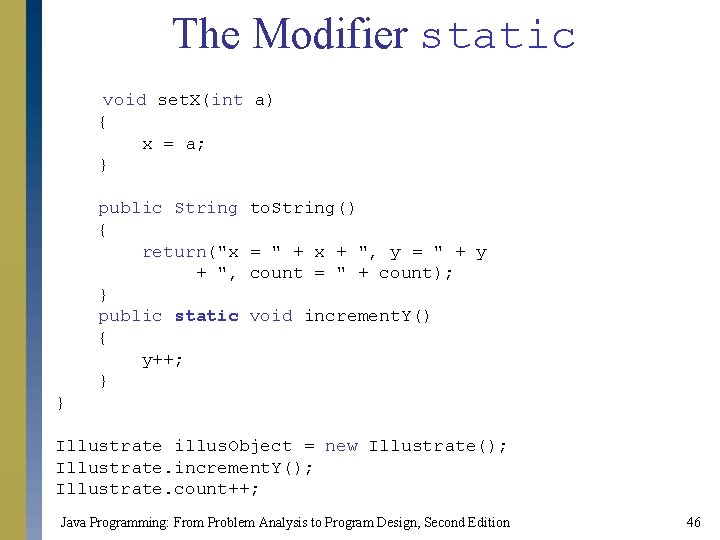
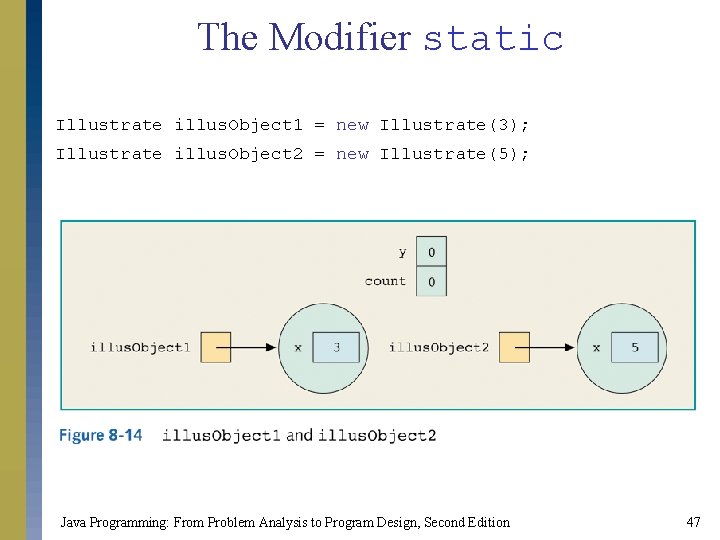
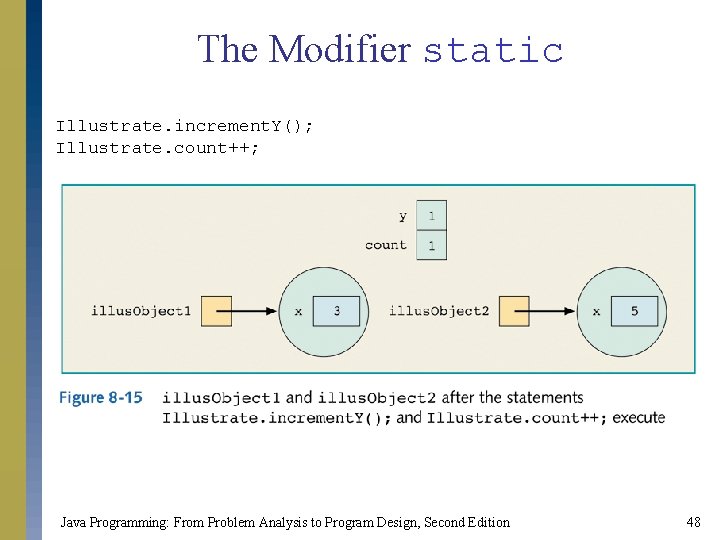
![public class Static. Members { public static void main(String[] args) { Illustrate illus. Object public class Static. Members { public static void main(String[] args) { Illustrate illus. Object](https://slidetodoc.com/presentation_image_h/2bcbdd0ceb58cd46c49e9cc2946eb43c/image-49.jpg)
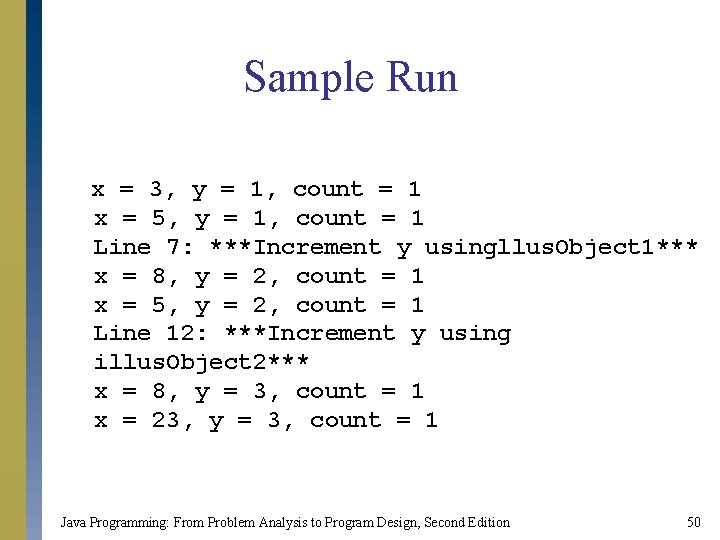
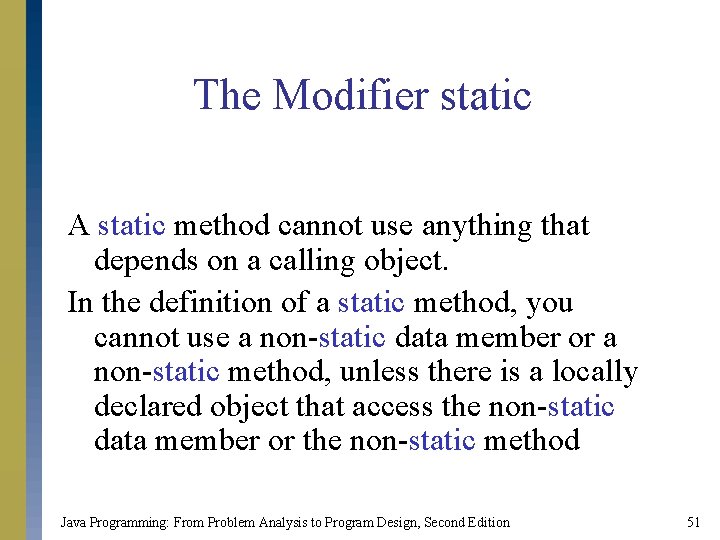
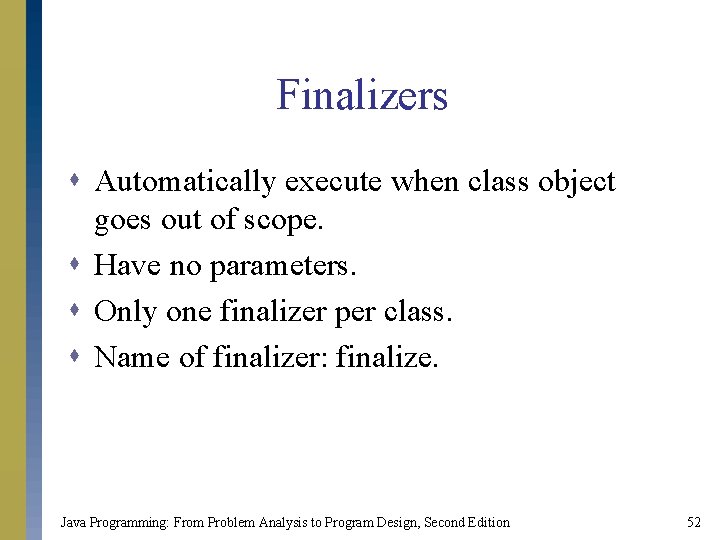
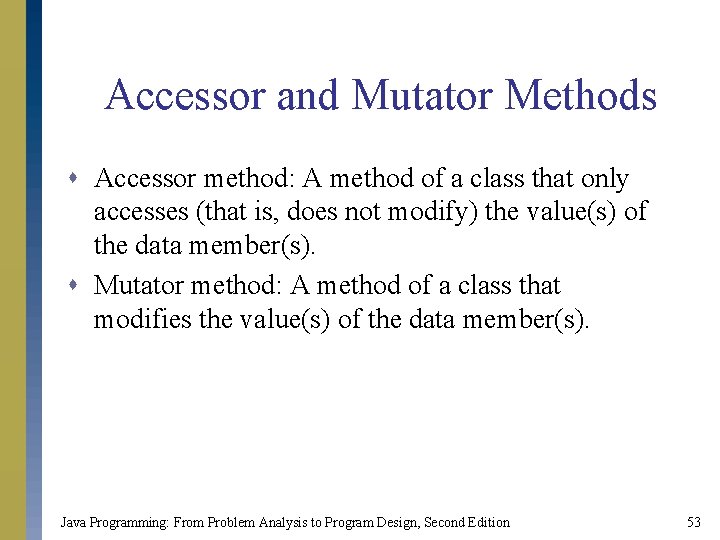
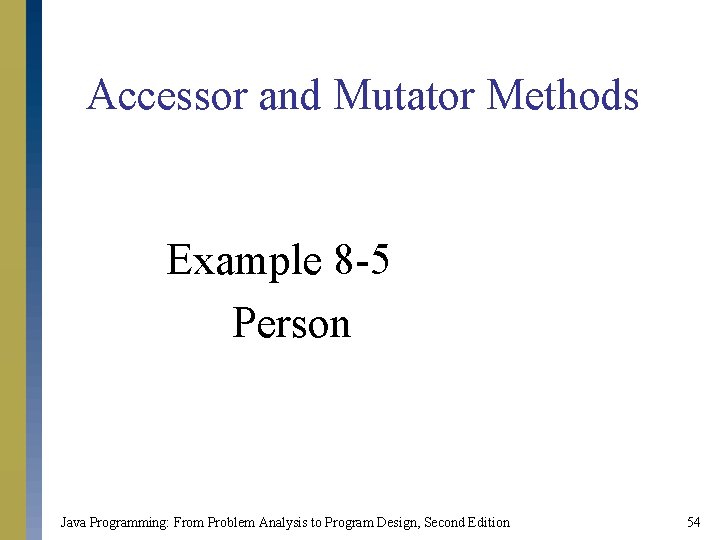
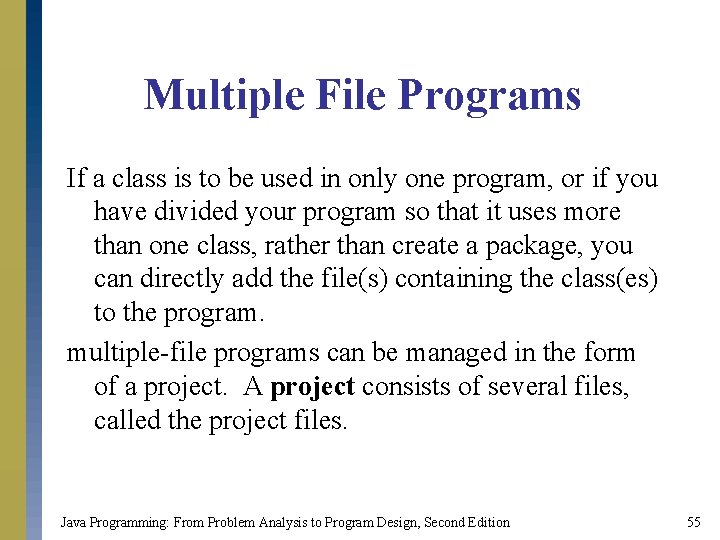
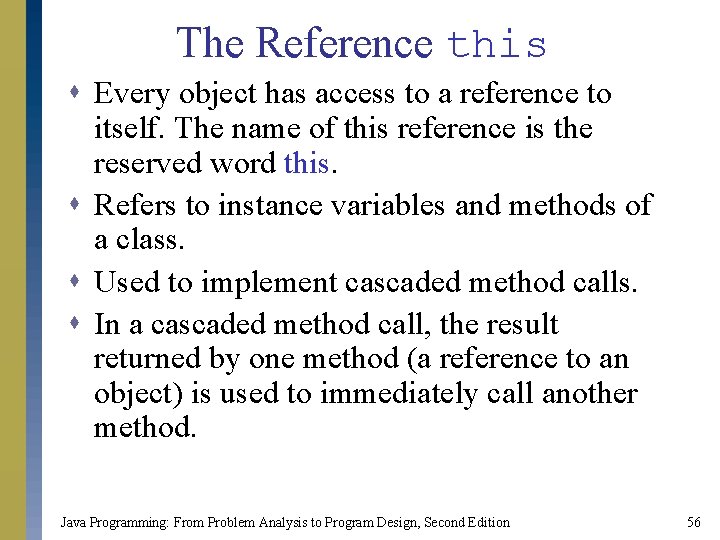
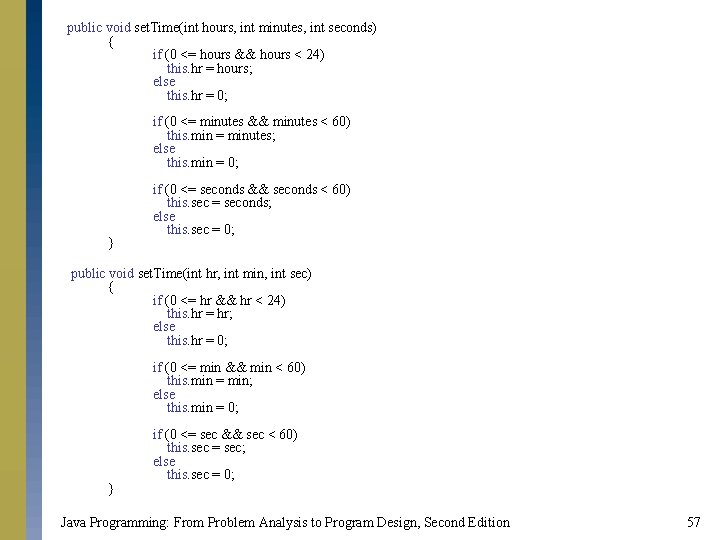
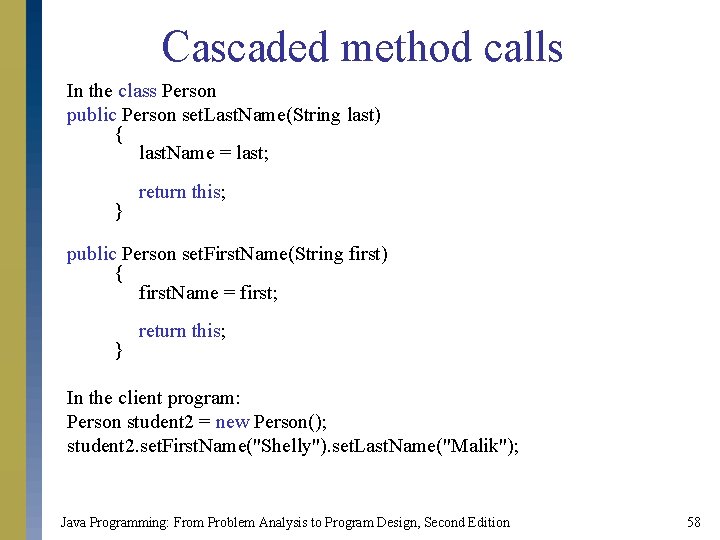
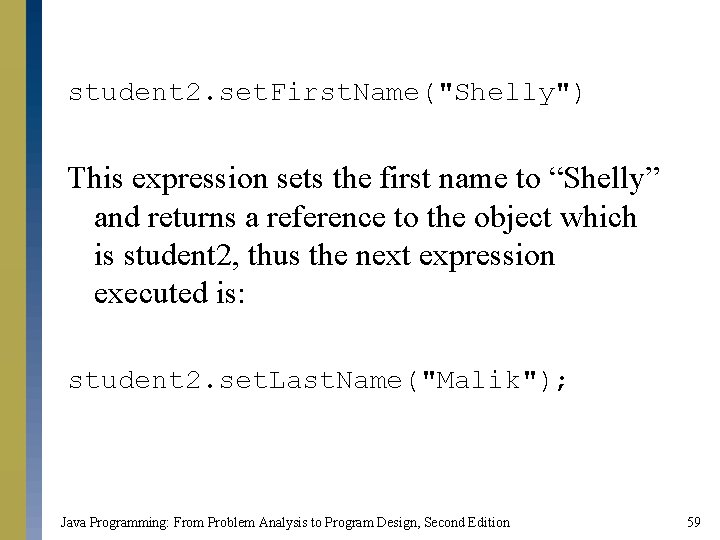
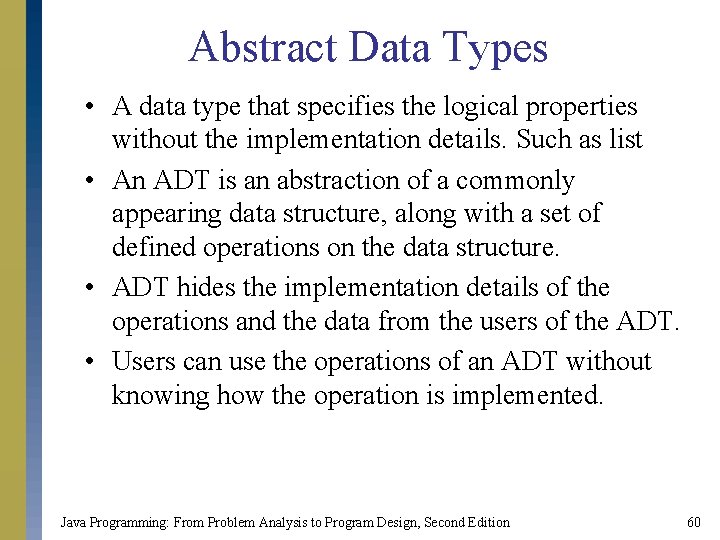
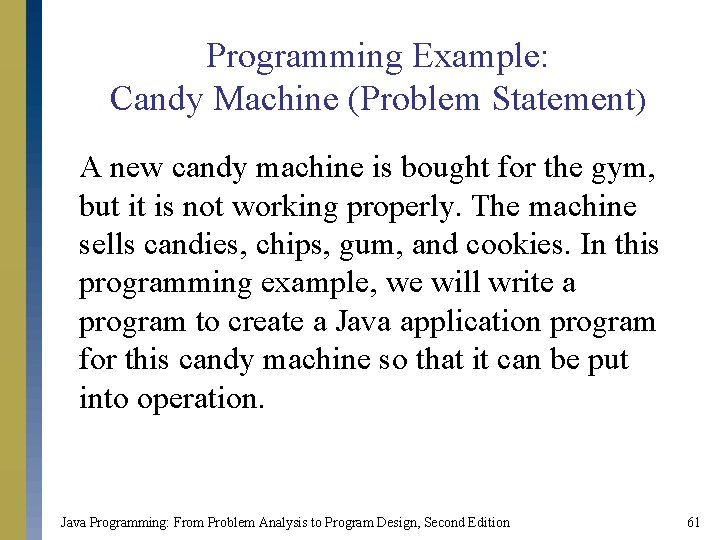
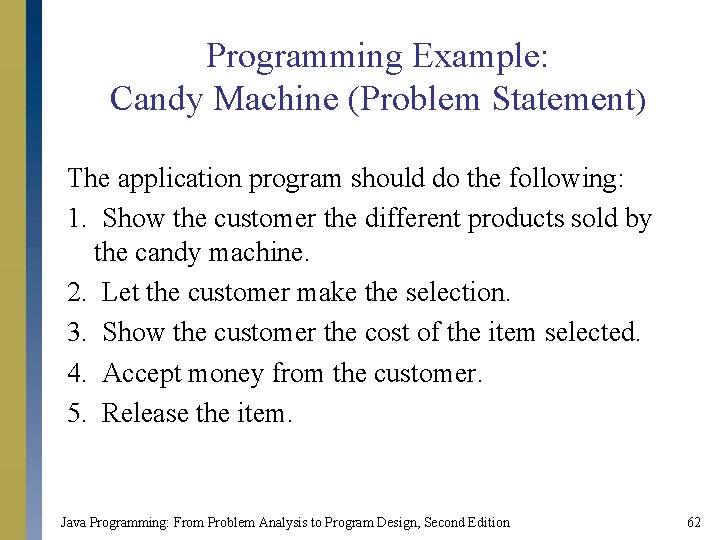
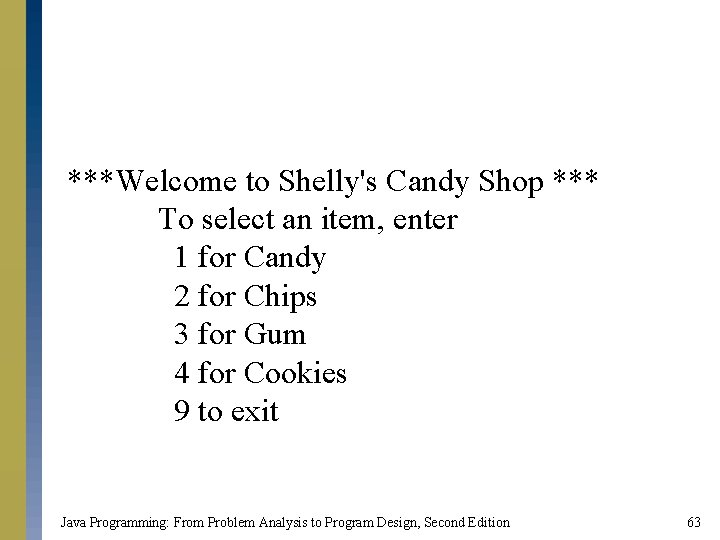
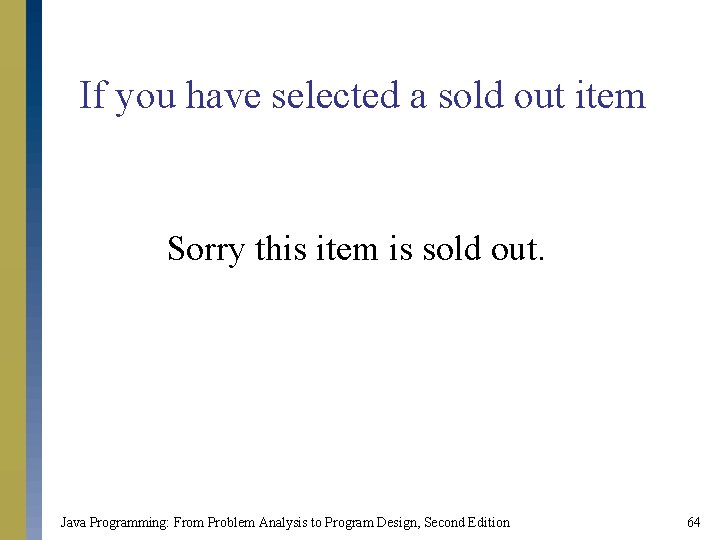
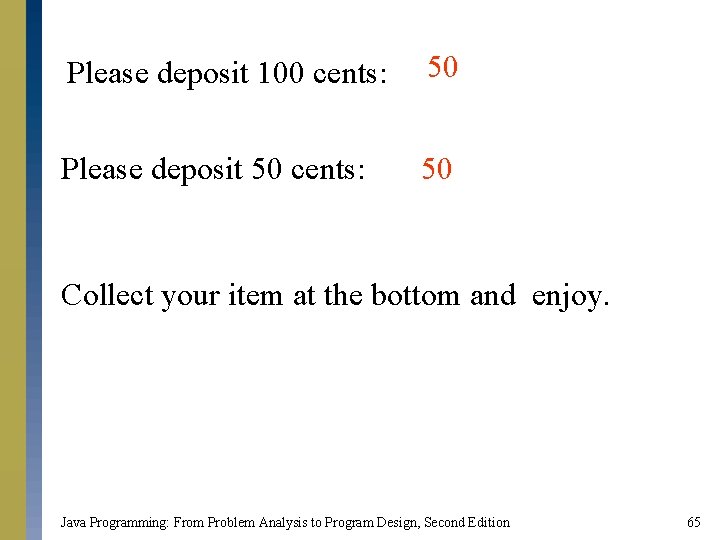
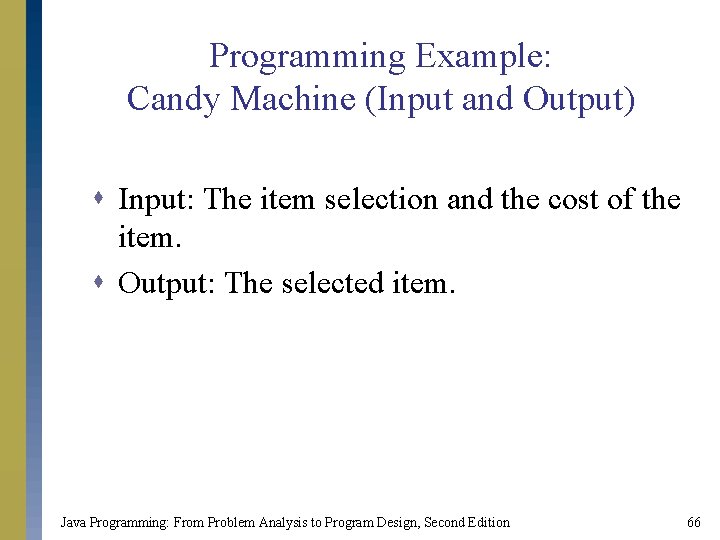
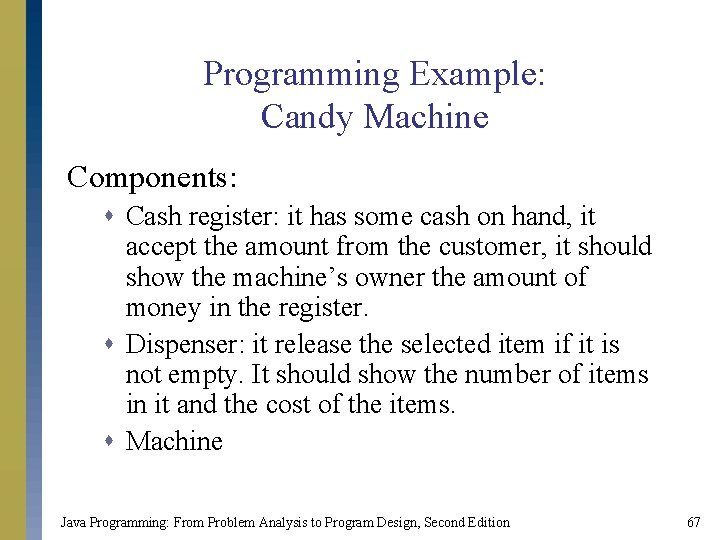
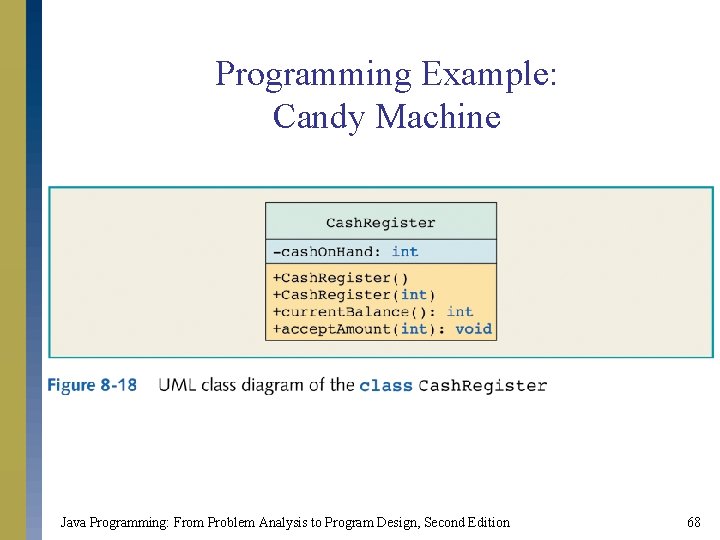
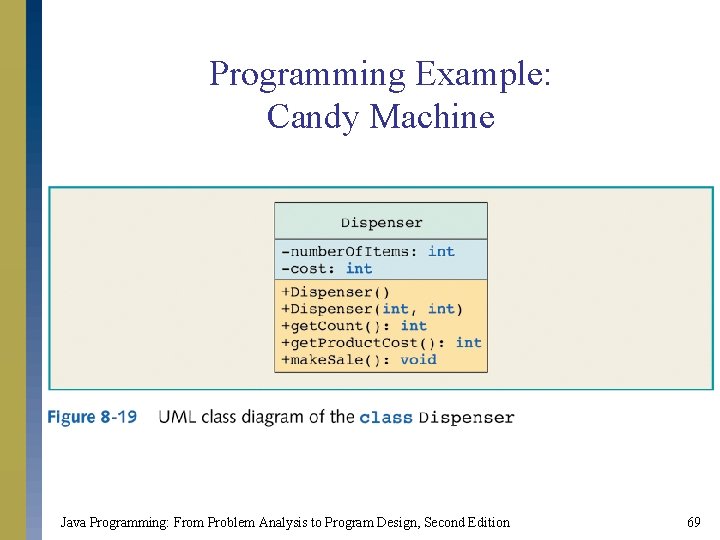
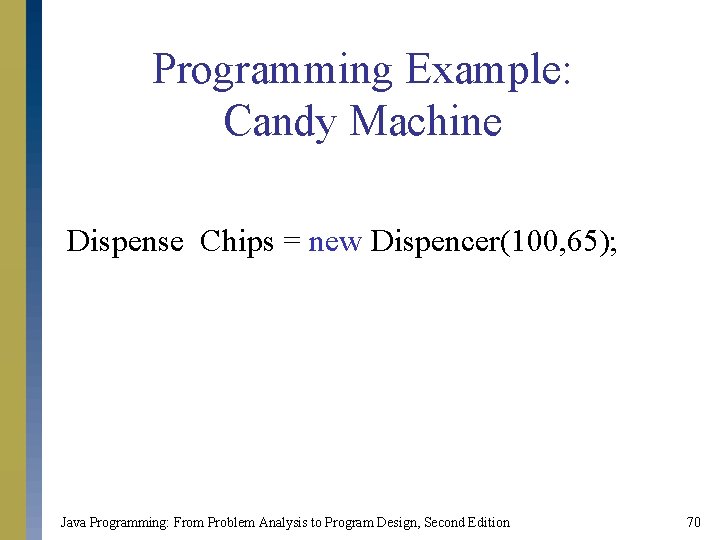
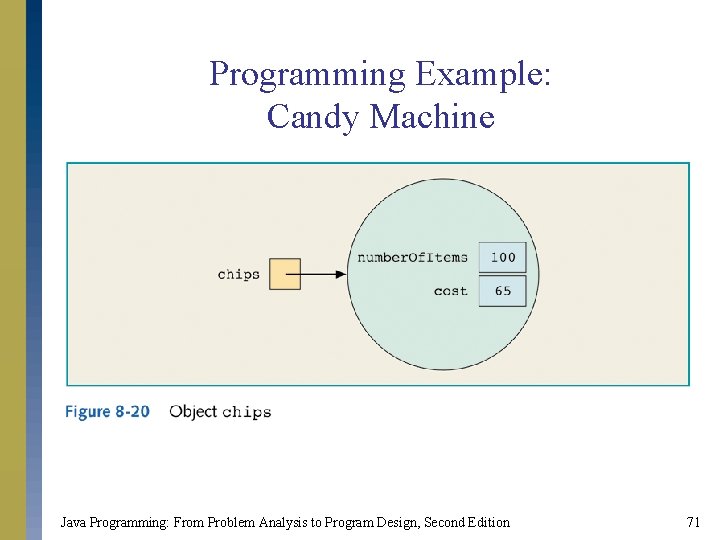
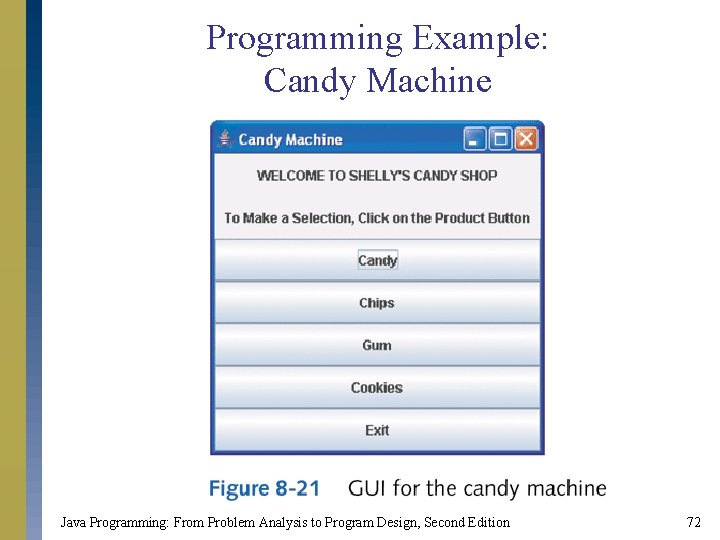
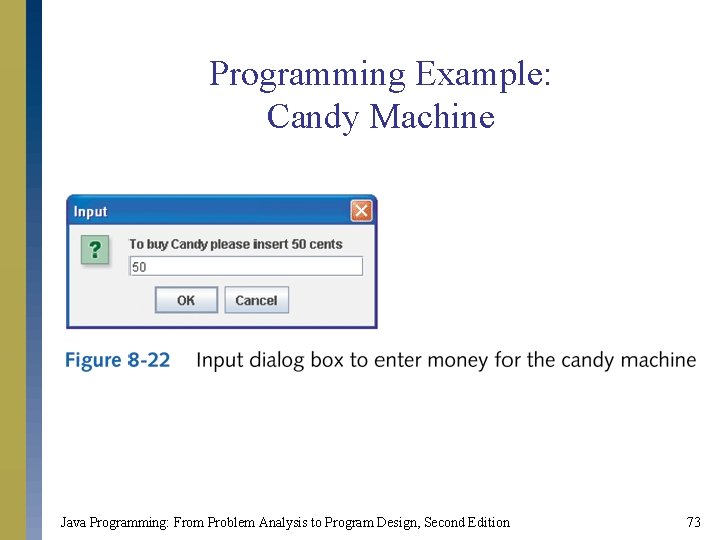
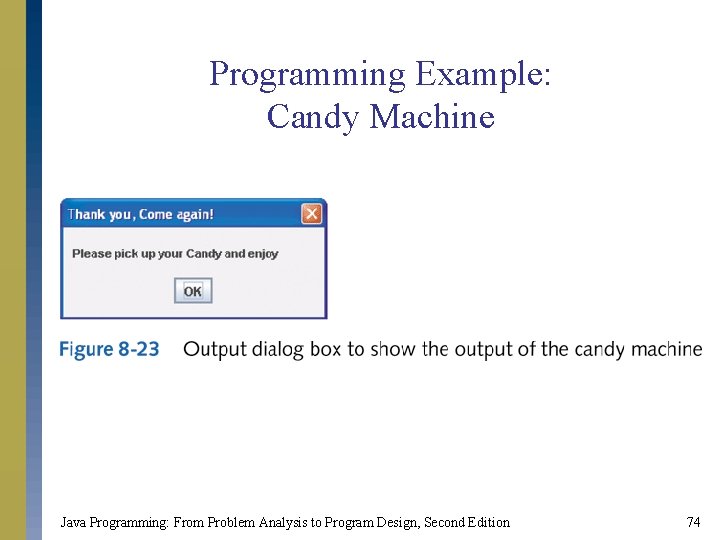
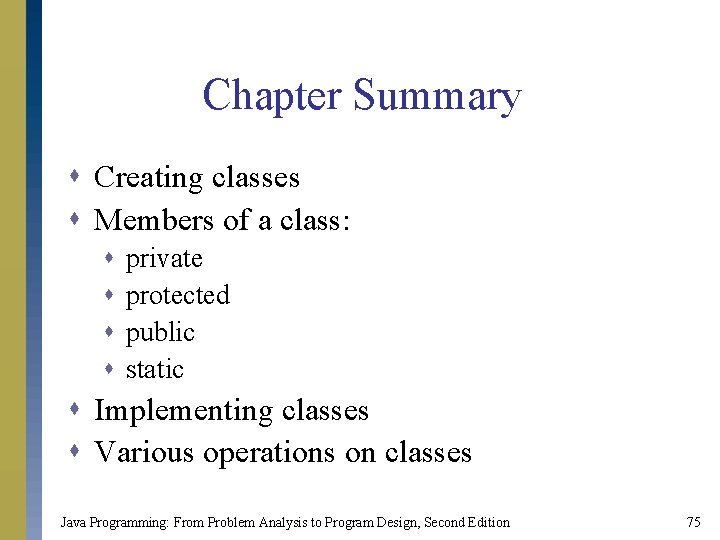
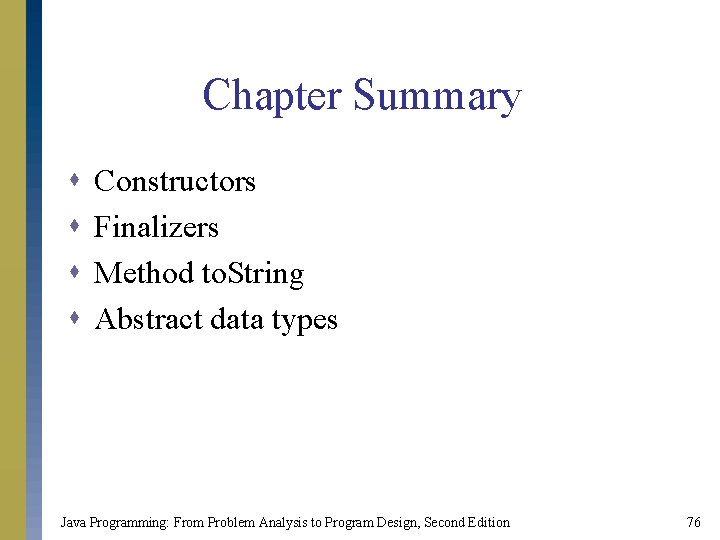
- Slides: 76
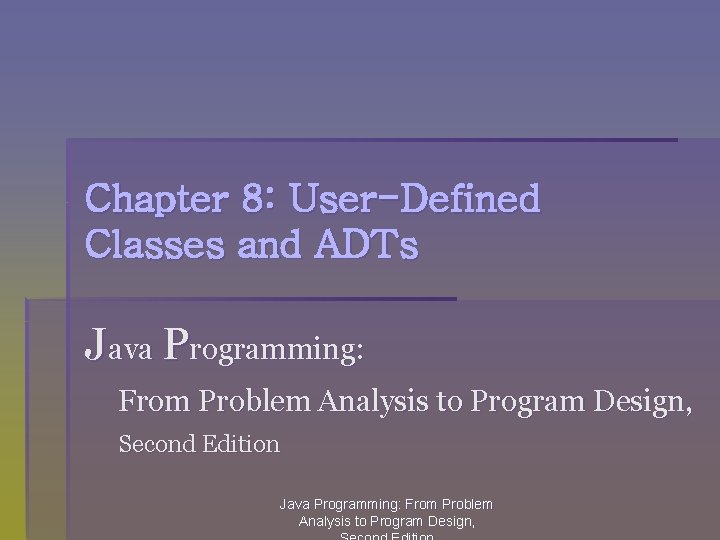
Chapter 8: User-Defined Classes and ADTs Java Programming: From Problem Analysis to Program Design, Second Edition Java Programming: From Problem Analysis to Program Design,
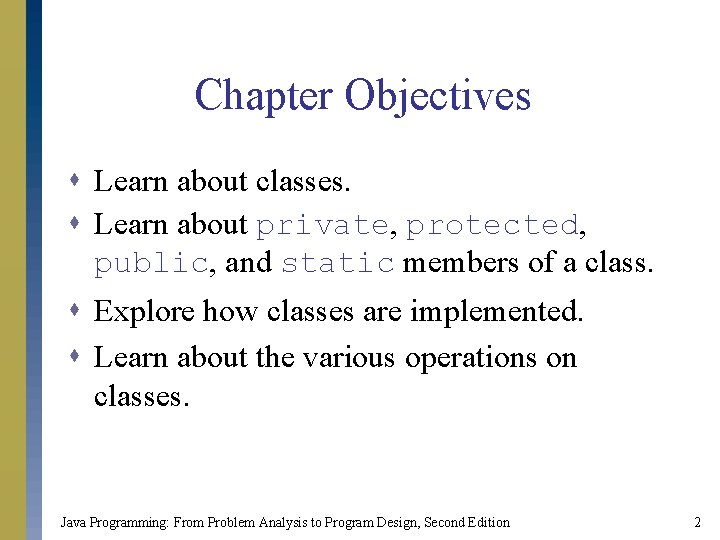
Chapter Objectives s Learn about classes. s Learn about private, protected, public, and static members of a class. s Explore how classes are implemented. s Learn about the various operations on classes. Java Programming: From Problem Analysis to Program Design, Second Edition 2
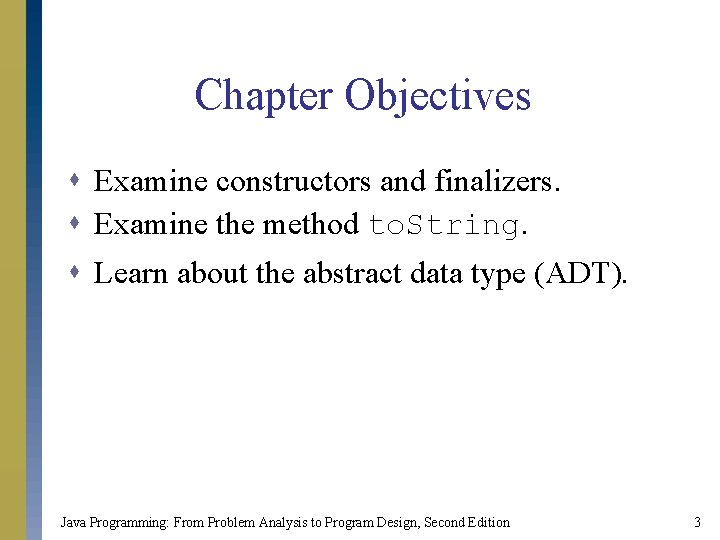
Chapter Objectives s Examine constructors and finalizers. s Examine the method to. String. s Learn about the abstract data type (ADT). Java Programming: From Problem Analysis to Program Design, Second Edition 3
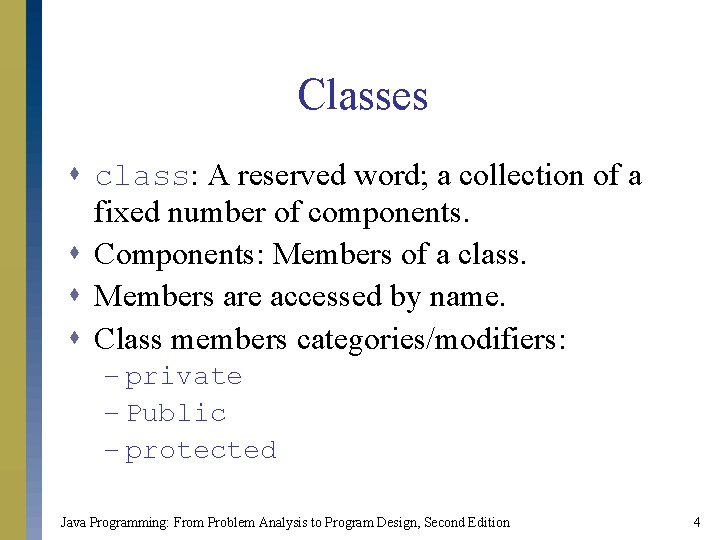
Classes s class: A reserved word; a collection of a fixed number of components. s Components: Members of a class. s Members are accessed by name. s Class members categories/modifiers: – private – Public – protected Java Programming: From Problem Analysis to Program Design, Second Edition 4
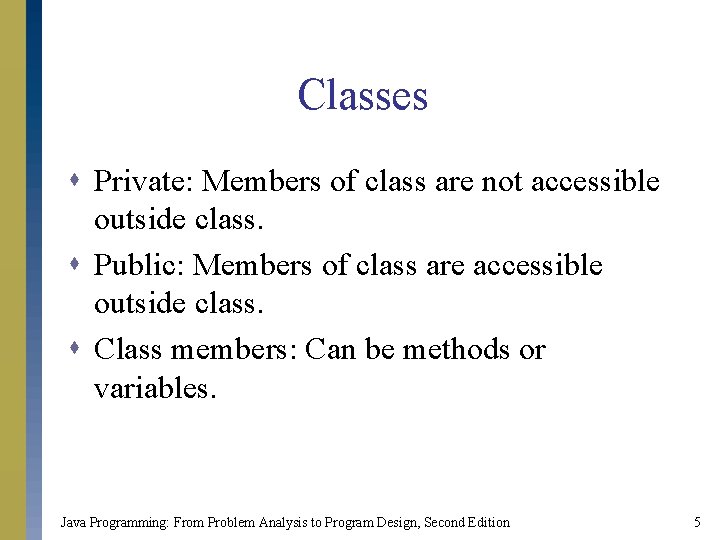
Classes s Private: Members of class are not accessible outside class. s Public: Members of class are accessible outside class. s Class members: Can be methods or variables. Java Programming: From Problem Analysis to Program Design, Second Edition 5
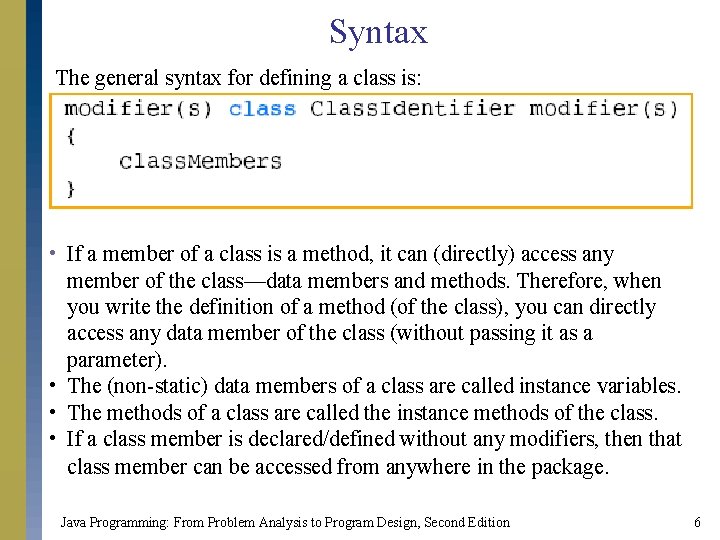
Syntax The general syntax for defining a class is: • If a member of a class is a method, it can (directly) access any member of the class—data members and methods. Therefore, when you write the definition of a method (of the class), you can directly access any data member of the class (without passing it as a parameter). • The (non-static) data members of a class are called instance variables. • The methods of a class are called the instance methods of the class. • If a class member is declared/defined without any modifiers, then that class member can be accessed from anywhere in the package. Java Programming: From Problem Analysis to Program Design, Second Edition 6
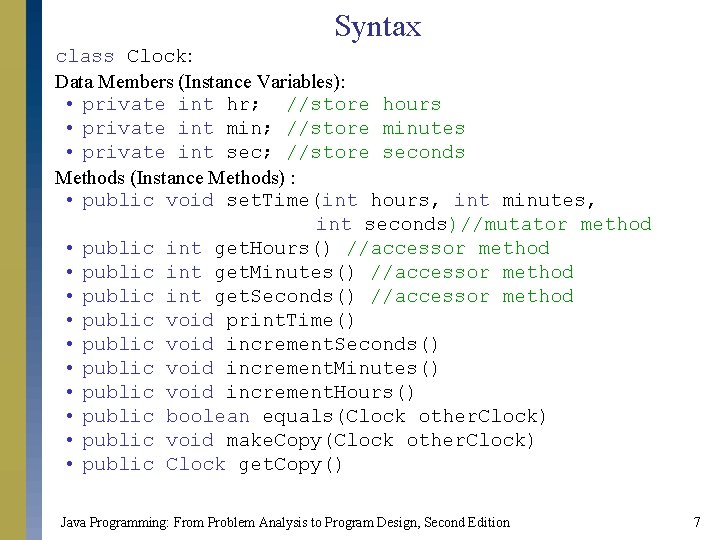
Syntax class Clock: Data Members (Instance Variables): • private int hr; //store hours • private int min; //store minutes • private int sec; //store seconds Methods (Instance Methods) : • public void set. Time(int hours, int minutes, int seconds)//mutator method • public int get. Hours() //accessor method • public int get. Minutes() //accessor method • public int get. Seconds() //accessor method • public void print. Time() • public void increment. Seconds() • public void increment. Minutes() • public void increment. Hours() • public boolean equals(Clock other. Clock) • public void make. Copy(Clock other. Clock) • public Clock get. Copy() Java Programming: From Problem Analysis to Program Design, Second Edition 7
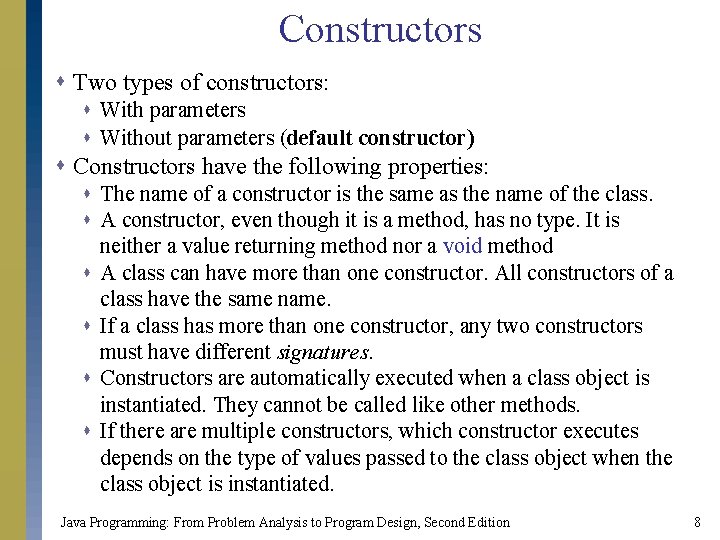
Constructors s Two types of constructors: s With parameters s Without parameters (default constructor) s Constructors have the following properties: s The name of a constructor is the same as the name of the class. s A constructor, even though it is a method, has no type. It is neither a value returning method nor a void method s A class can have more than one constructor. All constructors of a class have the same name. s If a class has more than one constructor, any two constructors must have different signatures. s Constructors are automatically executed when a class object is instantiated. They cannot be called like other methods. s If there are multiple constructors, which constructor executes depends on the type of values passed to the class object when the class object is instantiated. Java Programming: From Problem Analysis to Program Design, Second Edition 8
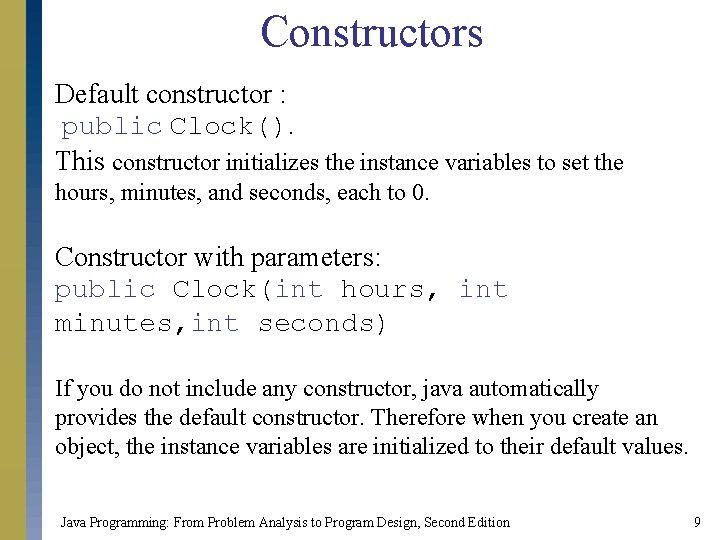
Constructors Default constructor : public Clock(). This constructor initializes the instance variables to set the hours, minutes, and seconds, each to 0. Constructor with parameters: public Clock(int hours, int minutes, int seconds) If you do not include any constructor, java automatically provides the default constructor. Therefore when you create an object, the instance variables are initialized to their default values. Java Programming: From Problem Analysis to Program Design, Second Edition 9
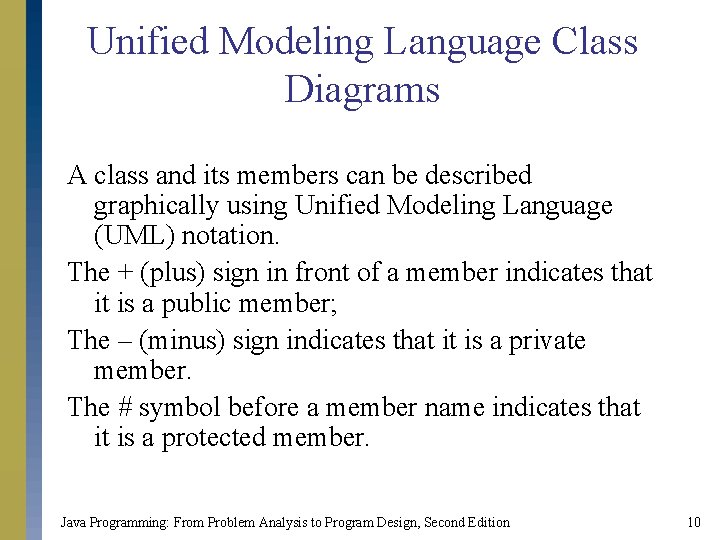
Unified Modeling Language Class Diagrams A class and its members can be described graphically using Unified Modeling Language (UML) notation. The + (plus) sign in front of a member indicates that it is a public member; The – (minus) sign indicates that it is a private member. The # symbol before a member name indicates that it is a protected member. Java Programming: From Problem Analysis to Program Design, Second Edition 10
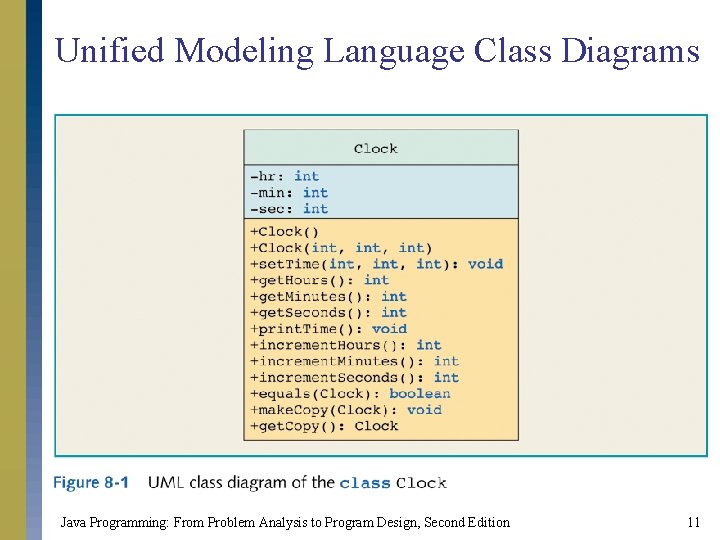
Unified Modeling Language Class Diagrams Java Programming: From Problem Analysis to Program Design, Second Edition 11
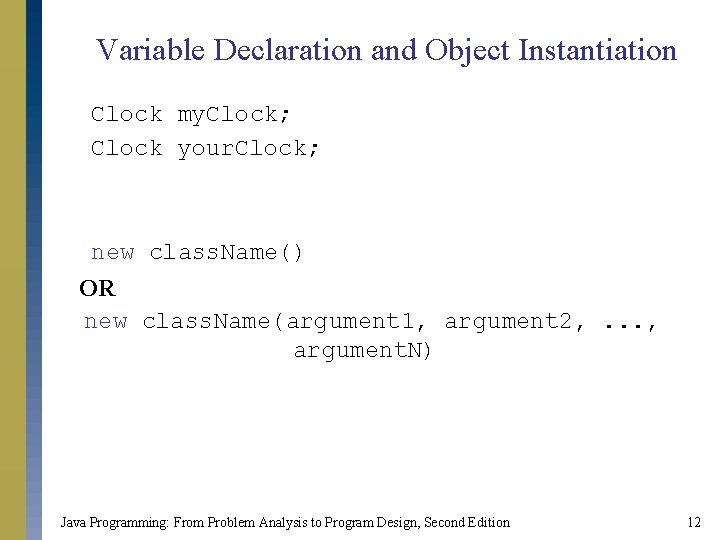
Variable Declaration and Object Instantiation Clock my. Clock; Clock your. Clock; new class. Name() OR new class. Name(argument 1, argument 2, . . . , argument. N) Java Programming: From Problem Analysis to Program Design, Second Edition 12
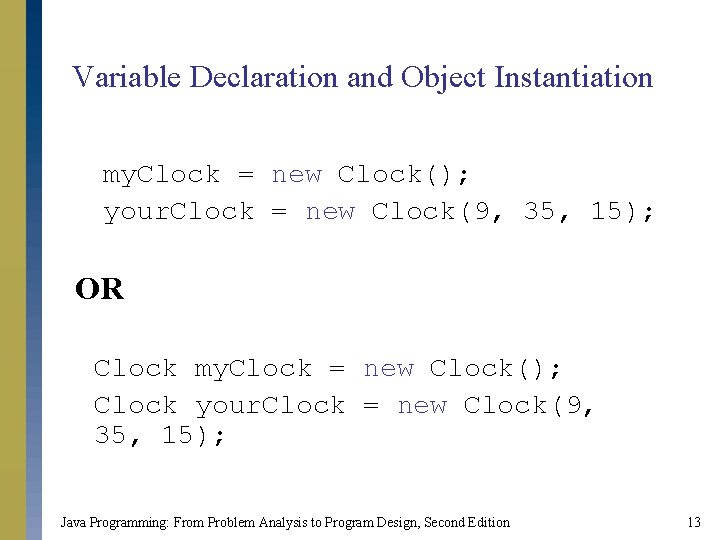
Variable Declaration and Object Instantiation my. Clock = new Clock(); your. Clock = new Clock(9, 35, 15); OR Clock my. Clock = new Clock(); Clock your. Clock = new Clock(9, 35, 15); Java Programming: From Problem Analysis to Program Design, Second Edition 13
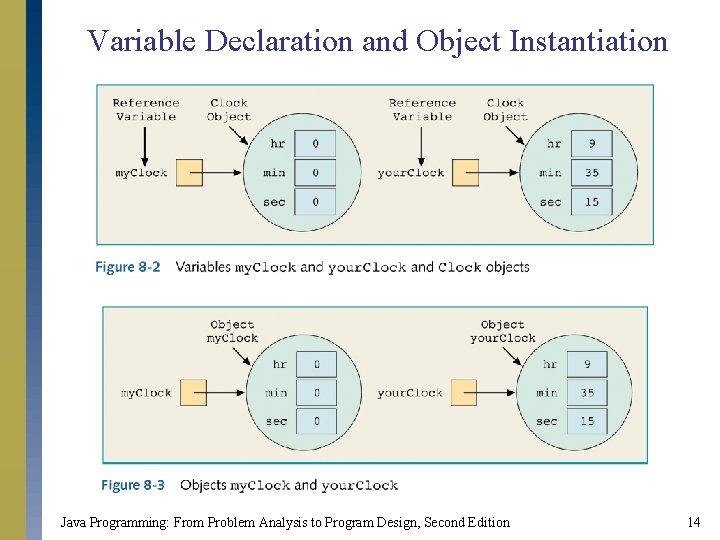
Variable Declaration and Object Instantiation Java Programming: From Problem Analysis to Program Design, Second Edition 14
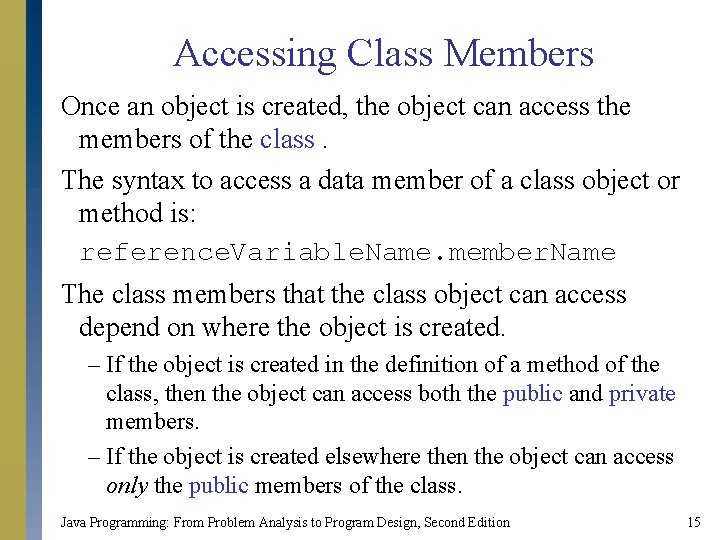
Accessing Class Members Once an object is created, the object can access the members of the class. The syntax to access a data member of a class object or method is: reference. Variable. Name. member. Name The class members that the class object can access depend on where the object is created. – If the object is created in the definition of a method of the class, then the object can access both the public and private members. – If the object is created elsewhere then the object can access only the public members of the class. Java Programming: From Problem Analysis to Program Design, Second Edition 15
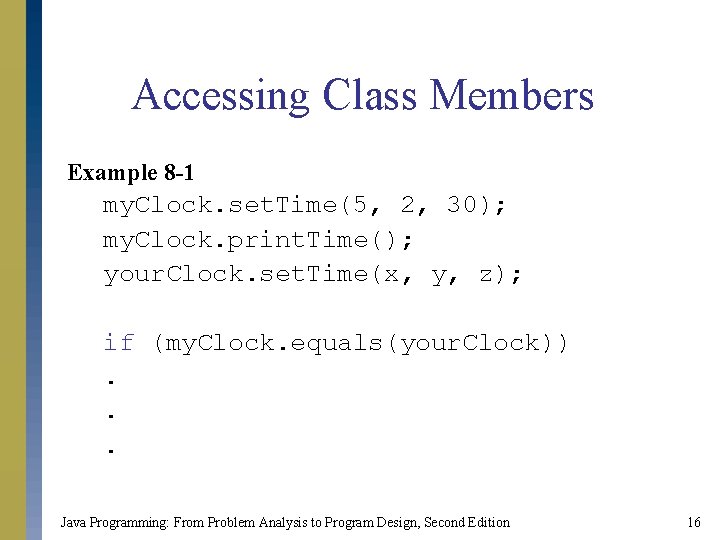
Accessing Class Members Example 8 -1 my. Clock. set. Time(5, 2, 30); my. Clock. print. Time(); your. Clock. set. Time(x, y, z); if (my. Clock. equals(your. Clock)). . . Java Programming: From Problem Analysis to Program Design, Second Edition 16
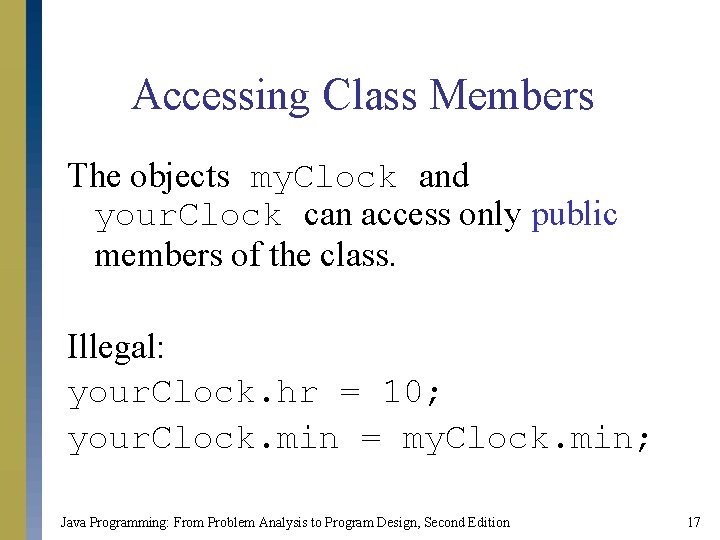
Accessing Class Members The objects my. Clock and your. Clock can access only public members of the class. Illegal: your. Clock. hr = 10; your. Clock. min = my. Clock. min; Java Programming: From Problem Analysis to Program Design, Second Edition 17
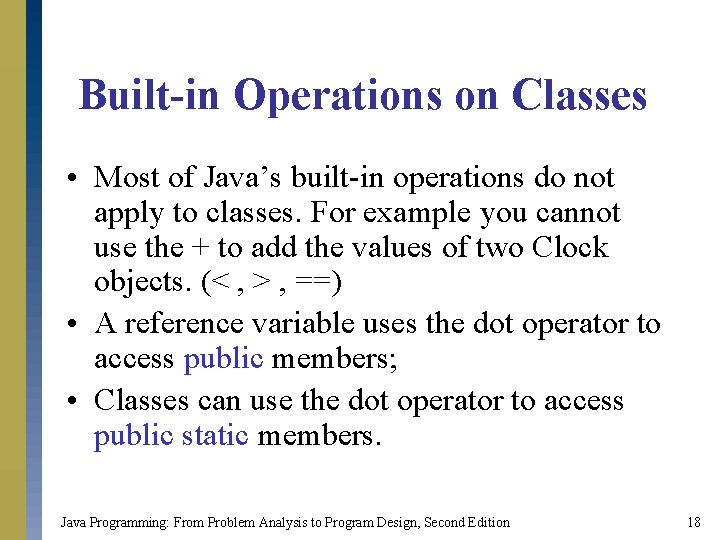
Built-in Operations on Classes • Most of Java’s built-in operations do not apply to classes. For example you cannot use the + to add the values of two Clock objects. (< , > , ==) • A reference variable uses the dot operator to access public members; • Classes can use the dot operator to access public static members. Java Programming: From Problem Analysis to Program Design, Second Edition 18
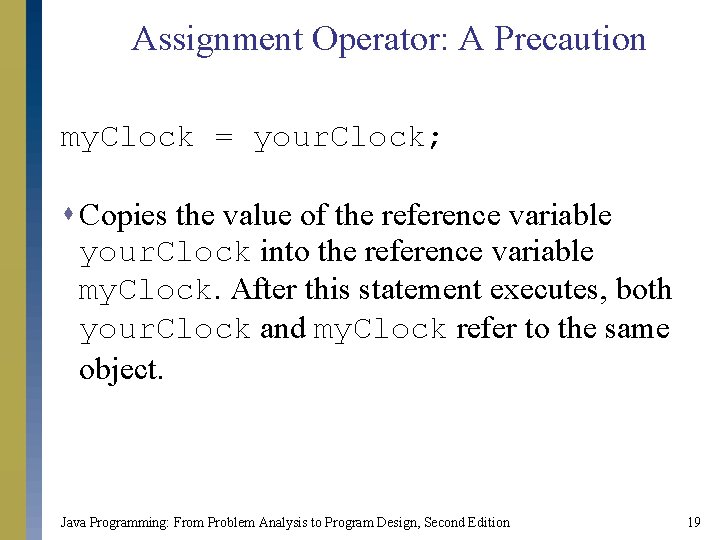
Assignment Operator: A Precaution my. Clock = your. Clock; s Copies the value of the reference variable your. Clock into the reference variable my. Clock. After this statement executes, both your. Clock and my. Clock refer to the same object. Java Programming: From Problem Analysis to Program Design, Second Edition 19
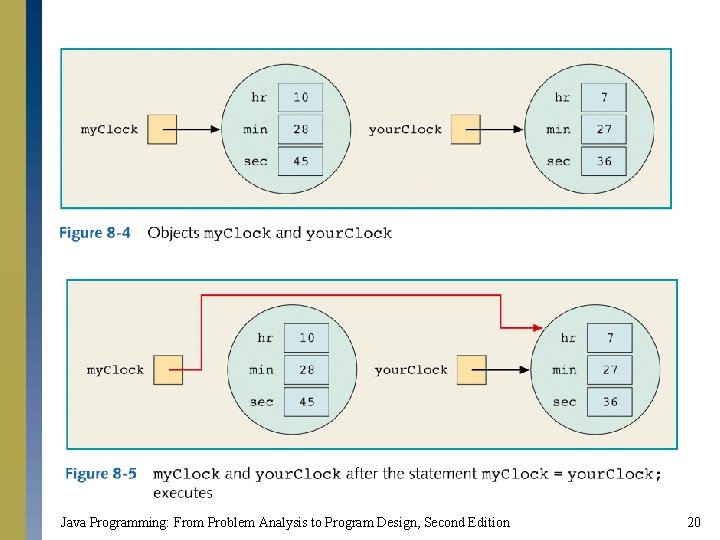
Java Programming: From Problem Analysis to Program Design, Second Edition 20
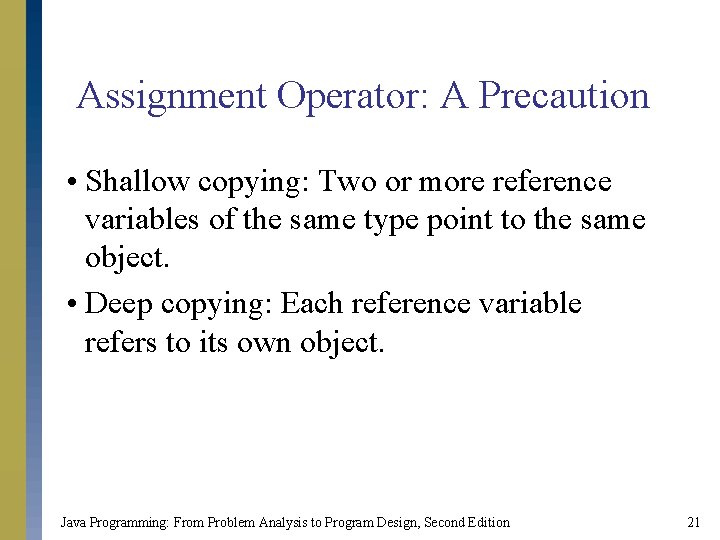
Assignment Operator: A Precaution • Shallow copying: Two or more reference variables of the same type point to the same object. • Deep copying: Each reference variable refers to its own object. Java Programming: From Problem Analysis to Program Design, Second Edition 21
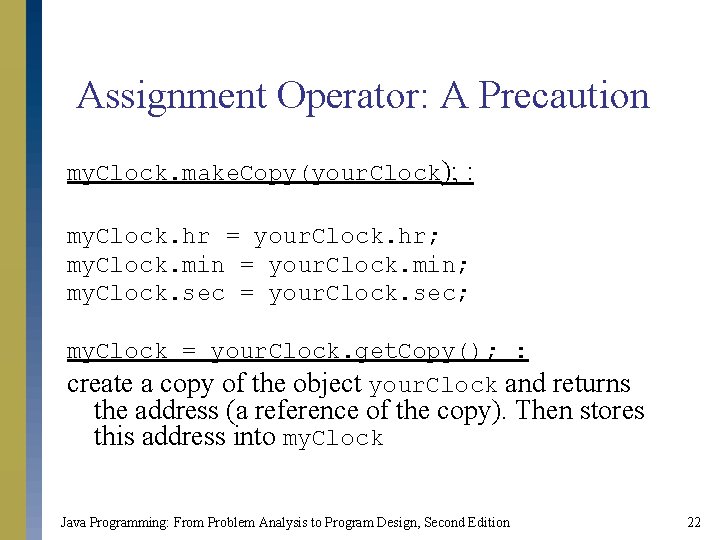
Assignment Operator: A Precaution my. Clock. make. Copy(your. Clock); : my. Clock. hr = your. Clock. hr; my. Clock. min = your. Clock. min; my. Clock. sec = your. Clock. sec; my. Clock = your. Clock. get. Copy(); : create a copy of the object your. Clock and returns the address (a reference of the copy). Then stores this address into my. Clock Java Programming: From Problem Analysis to Program Design, Second Edition 22
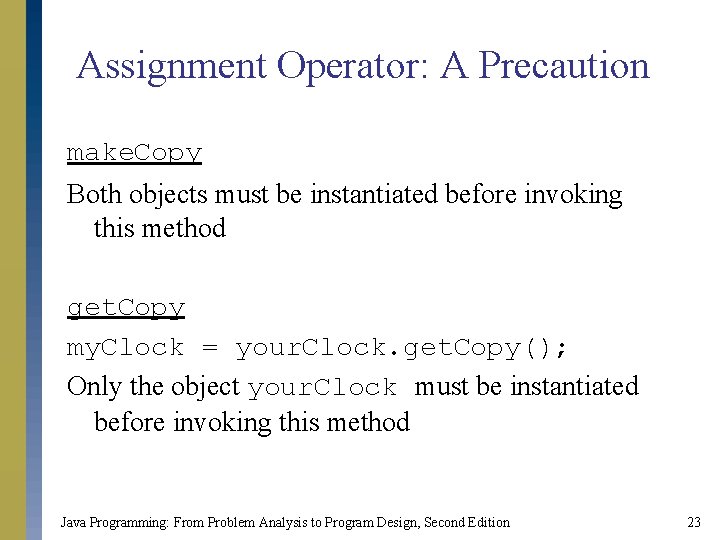
Assignment Operator: A Precaution make. Copy Both objects must be instantiated before invoking this method get. Copy my. Clock = your. Clock. get. Copy(); Only the object your. Clock must be instantiated before invoking this method Java Programming: From Problem Analysis to Program Design, Second Edition 23
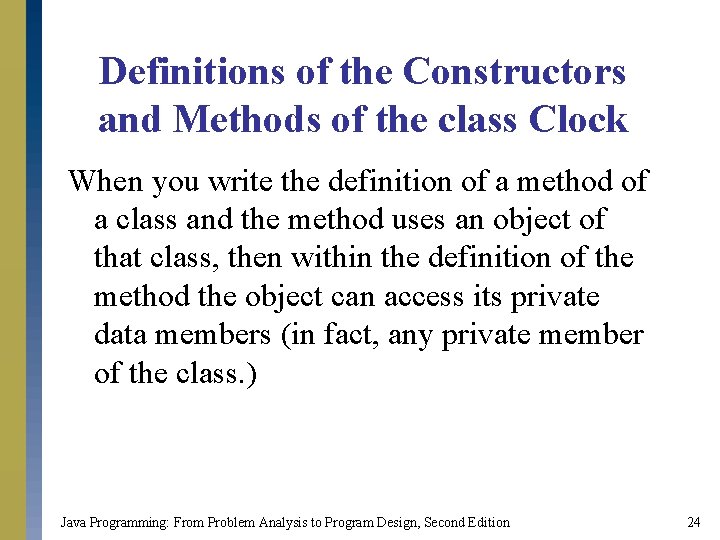
Definitions of the Constructors and Methods of the class Clock When you write the definition of a method of a class and the method uses an object of that class, then within the definition of the method the object can access its private data members (in fact, any private member of the class. ) Java Programming: From Problem Analysis to Program Design, Second Edition 24
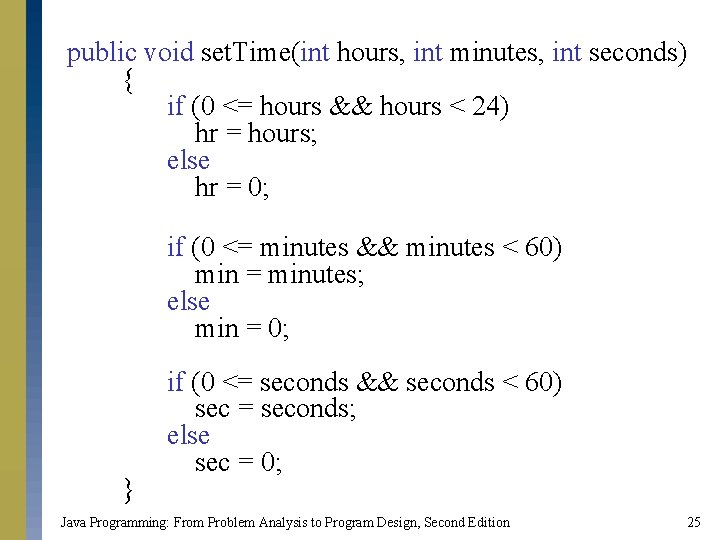
public void set. Time(int hours, int minutes, int seconds) { if (0 <= hours && hours < 24) hr = hours; else hr = 0; if (0 <= minutes && minutes < 60) min = minutes; else min = 0; } if (0 <= seconds && seconds < 60) sec = seconds; else sec = 0; Java Programming: From Problem Analysis to Program Design, Second Edition 25
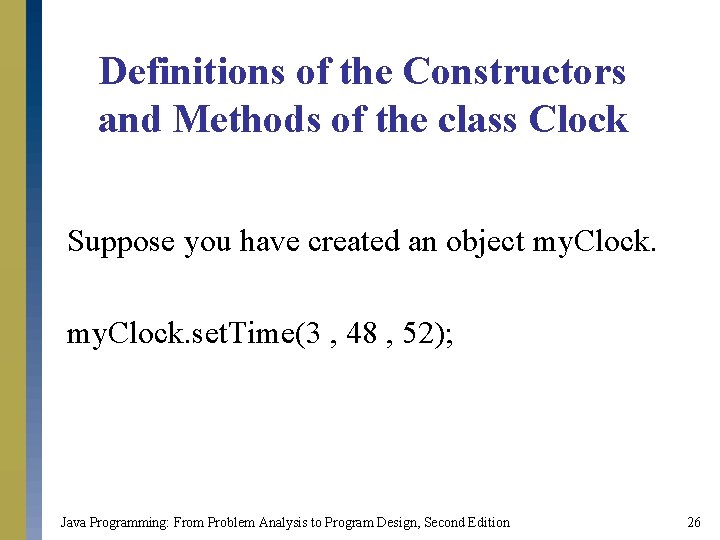
Definitions of the Constructors and Methods of the class Clock Suppose you have created an object my. Clock. set. Time(3 , 48 , 52); Java Programming: From Problem Analysis to Program Design, Second Edition 26
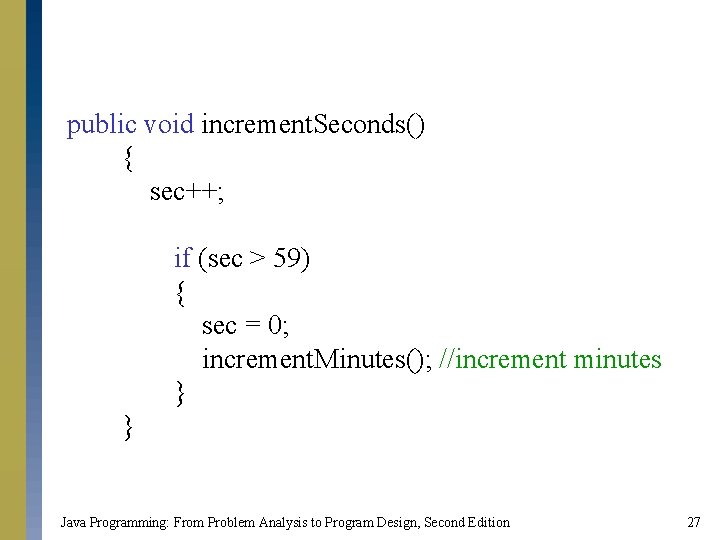
public void increment. Seconds() { sec++; if (sec > 59) { sec = 0; increment. Minutes(); //increment minutes } } Java Programming: From Problem Analysis to Program Design, Second Edition 27
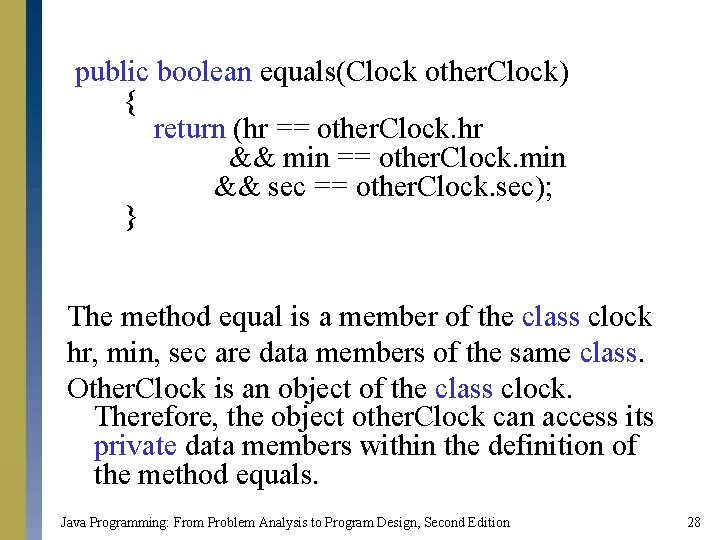
public boolean equals(Clock other. Clock) { return (hr == other. Clock. hr && min == other. Clock. min && sec == other. Clock. sec); } The method equal is a member of the class clock hr, min, sec are data members of the same class. Other. Clock is an object of the class clock. Therefore, the object other. Clock can access its private data members within the definition of the method equals. Java Programming: From Problem Analysis to Program Design, Second Edition 28
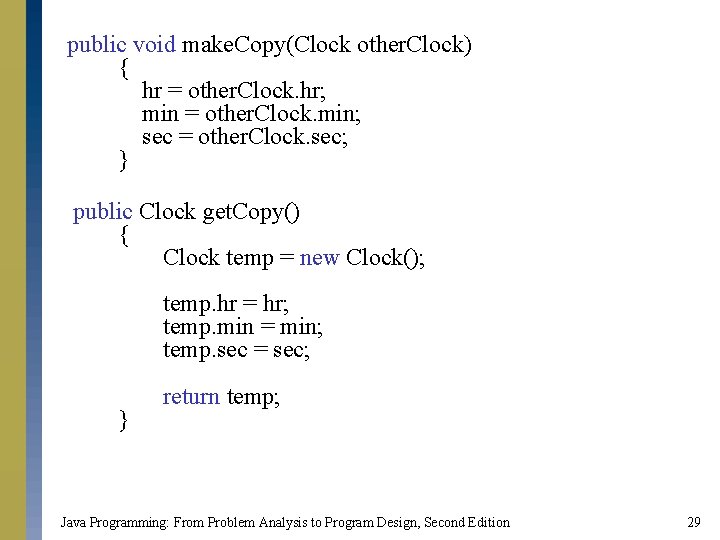
public void make. Copy(Clock other. Clock) { hr = other. Clock. hr; min = other. Clock. min; sec = other. Clock. sec; } public Clock get. Copy() { Clock temp = new Clock(); temp. hr = hr; temp. min = min; temp. sec = sec; } return temp; Java Programming: From Problem Analysis to Program Design, Second Edition 29
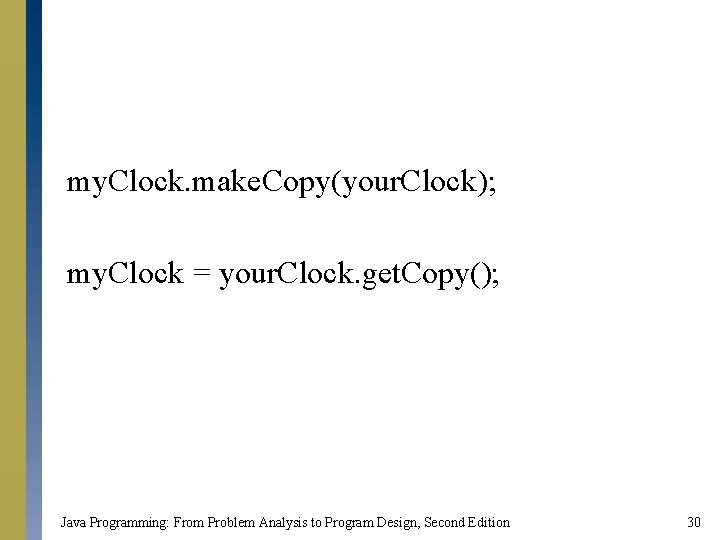
my. Clock. make. Copy(your. Clock); my. Clock = your. Clock. get. Copy(); Java Programming: From Problem Analysis to Program Design, Second Edition 30
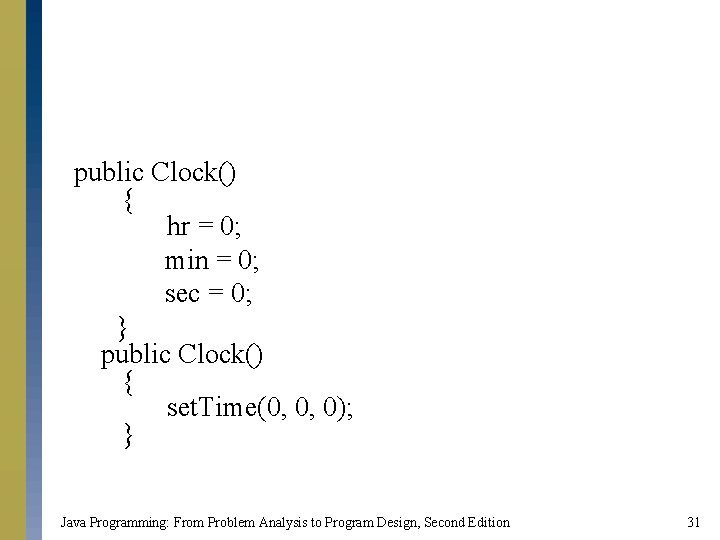
public Clock() { hr = 0; min = 0; sec = 0; } public Clock() { set. Time(0, 0, 0); } Java Programming: From Problem Analysis to Program Design, Second Edition 31
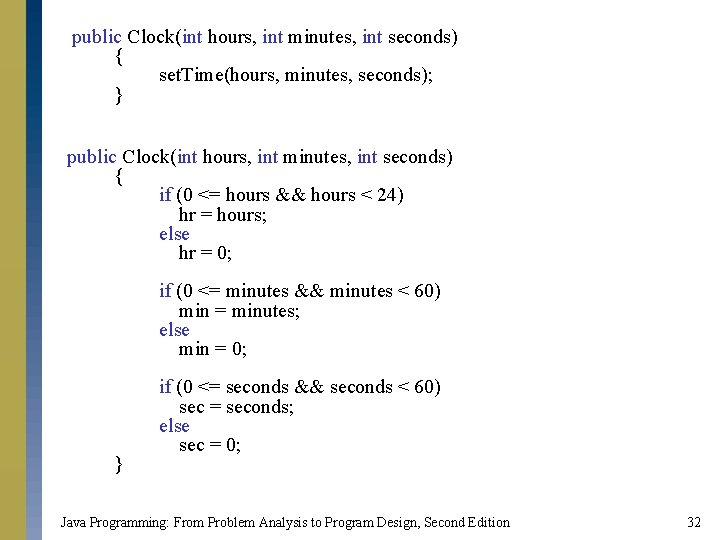
public Clock(int hours, int minutes, int seconds) { set. Time(hours, minutes, seconds); } public Clock(int hours, int minutes, int seconds) { if (0 <= hours && hours < 24) hr = hours; else hr = 0; if (0 <= minutes && minutes < 60) min = minutes; else min = 0; } if (0 <= seconds && seconds < 60) sec = seconds; else sec = 0; Java Programming: From Problem Analysis to Program Design, Second Edition 32
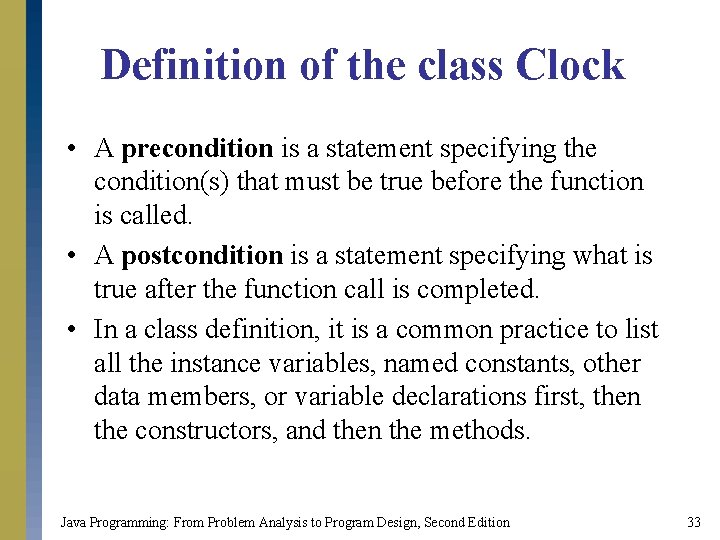
Definition of the class Clock • A precondition is a statement specifying the condition(s) that must be true before the function is called. • A postcondition is a statement specifying what is true after the function call is completed. • In a class definition, it is a common practice to list all the instance variables, named constants, other data members, or variable declarations first, then the constructors, and then the methods. Java Programming: From Problem Analysis to Program Design, Second Edition 33
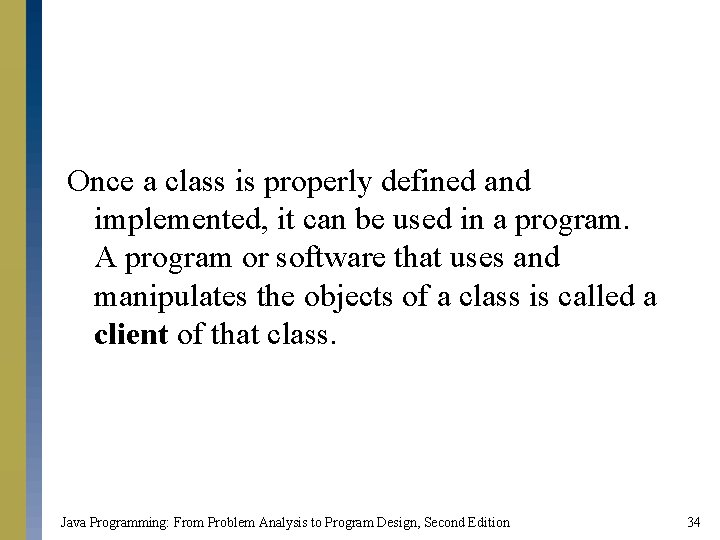
Once a class is properly defined and implemented, it can be used in a program. A program or software that uses and manipulates the objects of a class is called a client of that class. Java Programming: From Problem Analysis to Program Design, Second Edition 34
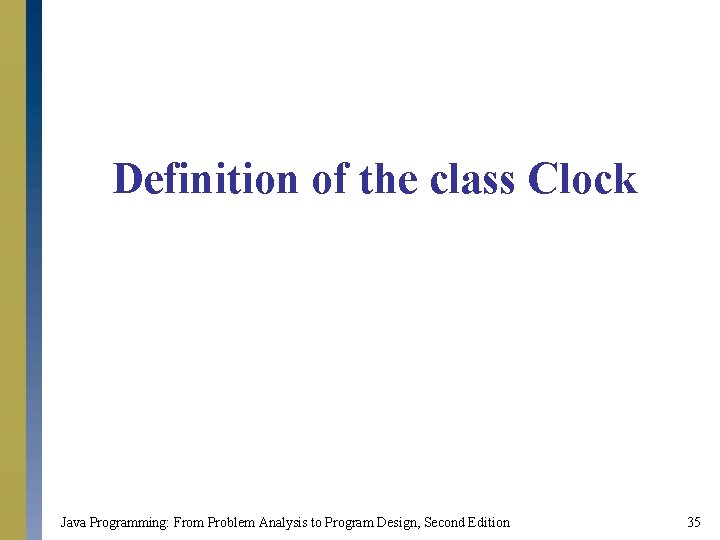
Definition of the class Clock Java Programming: From Problem Analysis to Program Design, Second Edition 35
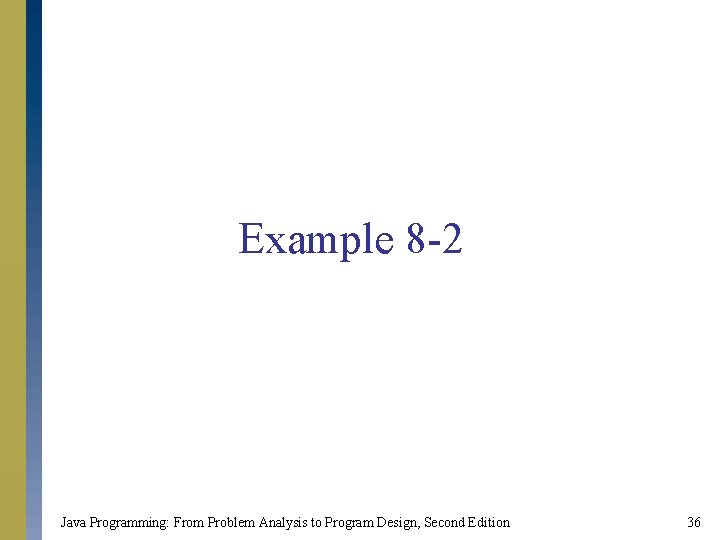
Example 8 -2 Java Programming: From Problem Analysis to Program Design, Second Edition 36
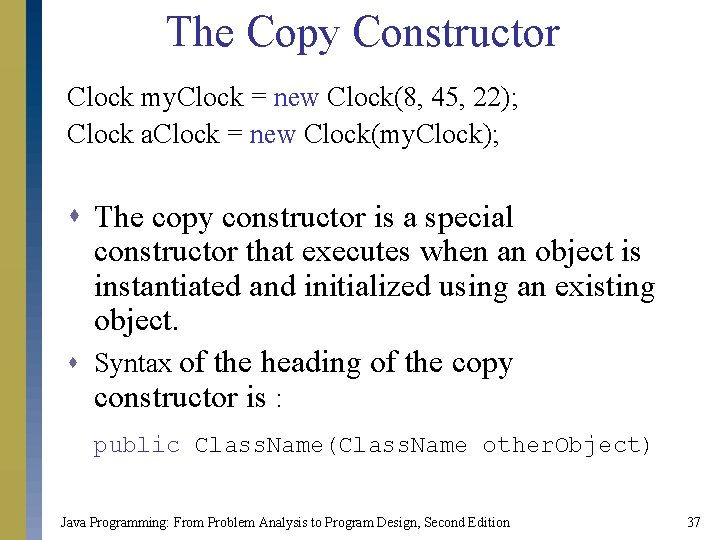
The Copy Constructor Clock my. Clock = new Clock(8, 45, 22); Clock a. Clock = new Clock(my. Clock); s The copy constructor is a special constructor that executes when an object is instantiated and initialized using an existing object. s Syntax of the heading of the copy constructor is : public Class. Name(Class. Name other. Object) Java Programming: From Problem Analysis to Program Design, Second Edition 37
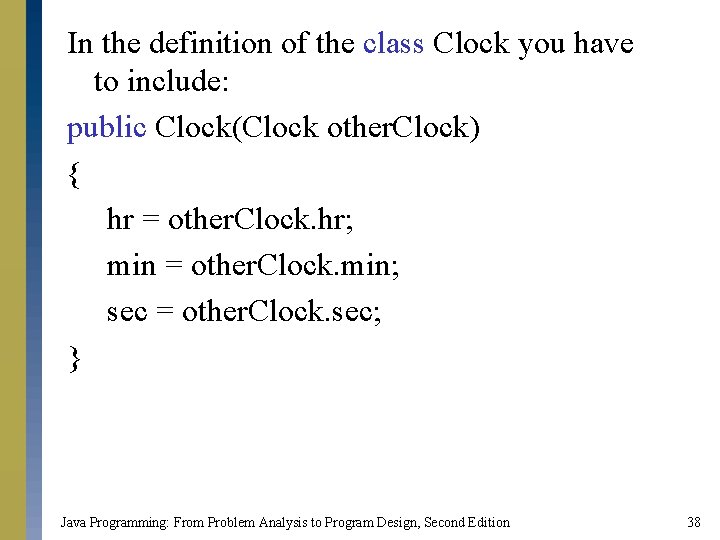
In the definition of the class Clock you have to include: public Clock(Clock other. Clock) { hr = other. Clock. hr; min = other. Clock. min; sec = other. Clock. sec; } Java Programming: From Problem Analysis to Program Design, Second Edition 38
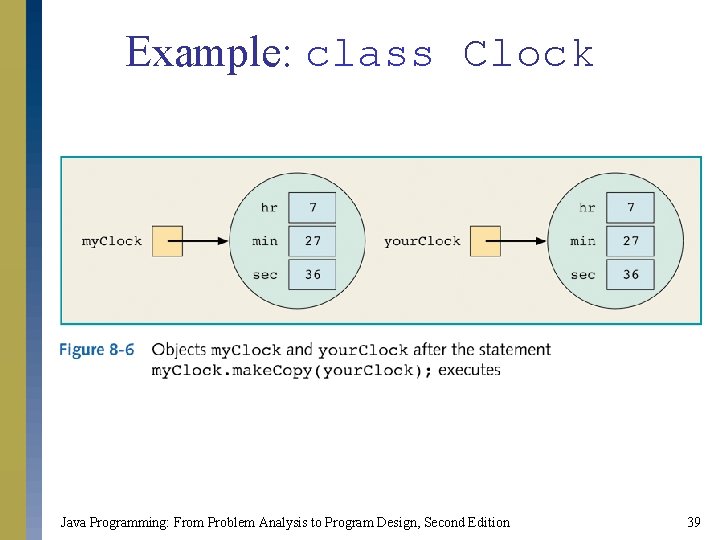
Example: class Clock Java Programming: From Problem Analysis to Program Design, Second Edition 39
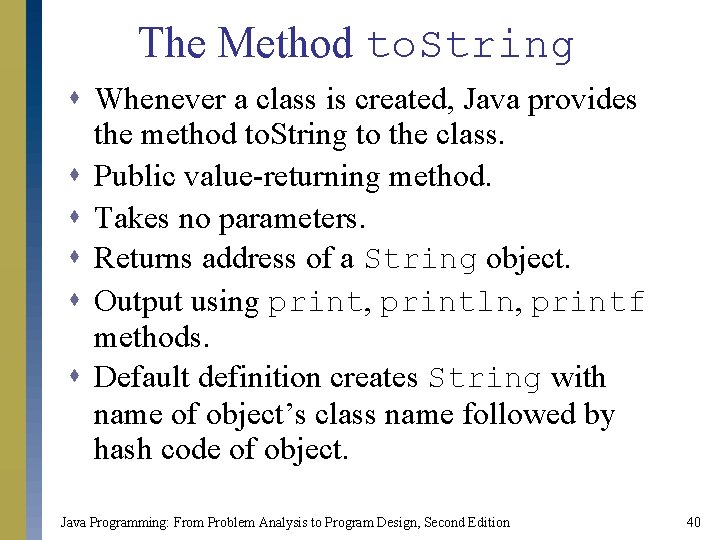
The Method to. String s Whenever a class is created, Java provides the method to. String to the class. s Public value-returning method. s Takes no parameters. s Returns address of a String object. s Output using print, println, printf methods. s Default definition creates String with name of object’s class name followed by hash code of object. Java Programming: From Problem Analysis to Program Design, Second Edition 40
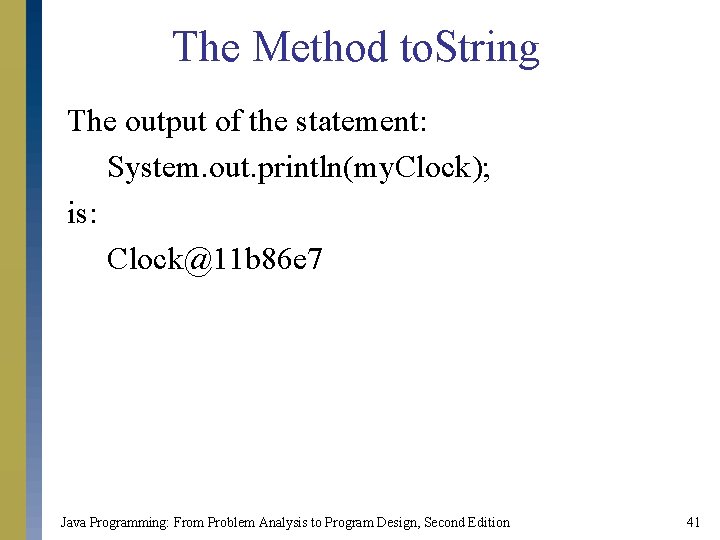
The Method to. String The output of the statement: System. out. println(my. Clock); is: Clock@11 b 86 e 7 Java Programming: From Problem Analysis to Program Design, Second Edition 41
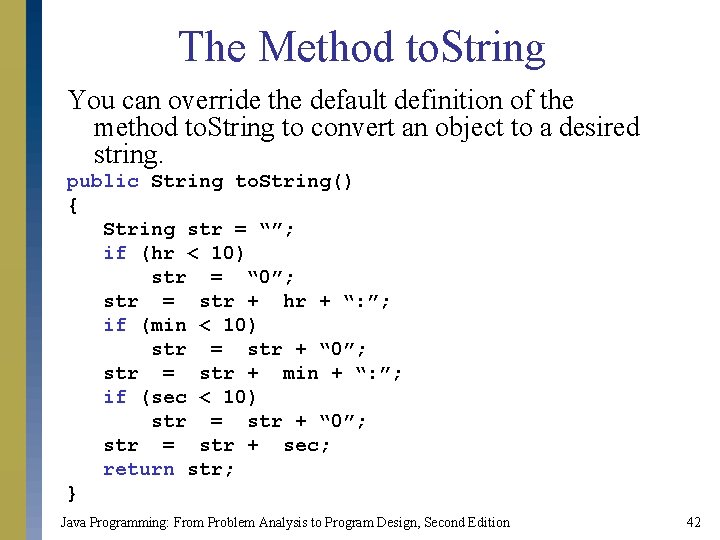
The Method to. String You can override the default definition of the method to. String to convert an object to a desired string. public String to. String() { String str = “”; if (hr < 10) str = “ 0”; str = str + hr + “: ”; if (min < 10) str = str + “ 0”; str = str + min + “: ”; if (sec < 10) str = str + “ 0”; str = str + sec; return str; } Java Programming: From Problem Analysis to Program Design, Second Edition 42
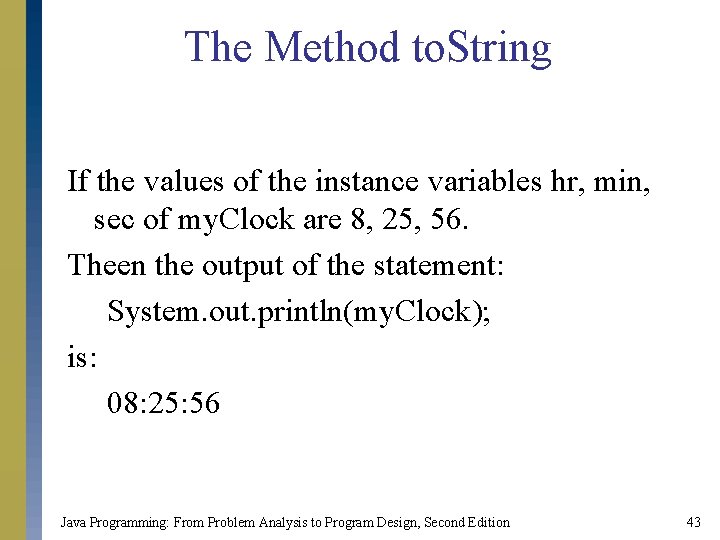
The Method to. String If the values of the instance variables hr, min, sec of my. Clock are 8, 25, 56. Theen the output of the statement: System. out. println(my. Clock); is: 08: 25: 56 Java Programming: From Problem Analysis to Program Design, Second Edition 43
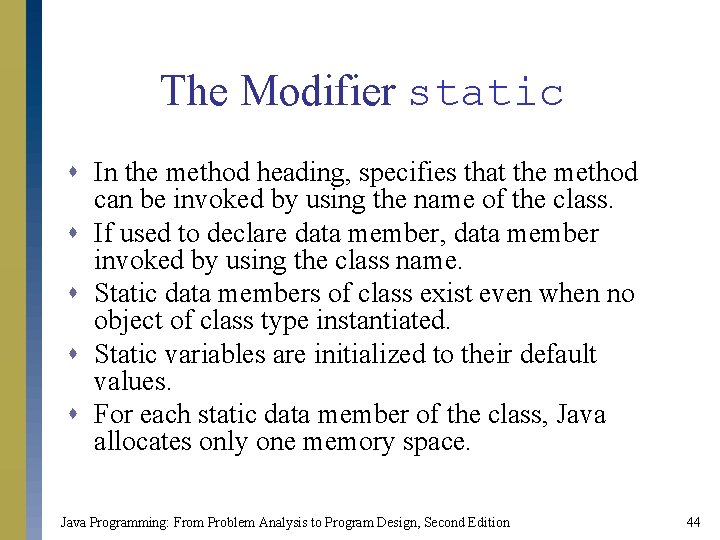
The Modifier static s In the method heading, specifies that the method can be invoked by using the name of the class. s If used to declare data member, data member invoked by using the class name. s Static data members of class exist even when no object of class type instantiated. s Static variables are initialized to their default values. s For each static data member of the class, Java allocates only one memory space. Java Programming: From Problem Analysis to Program Design, Second Edition 44
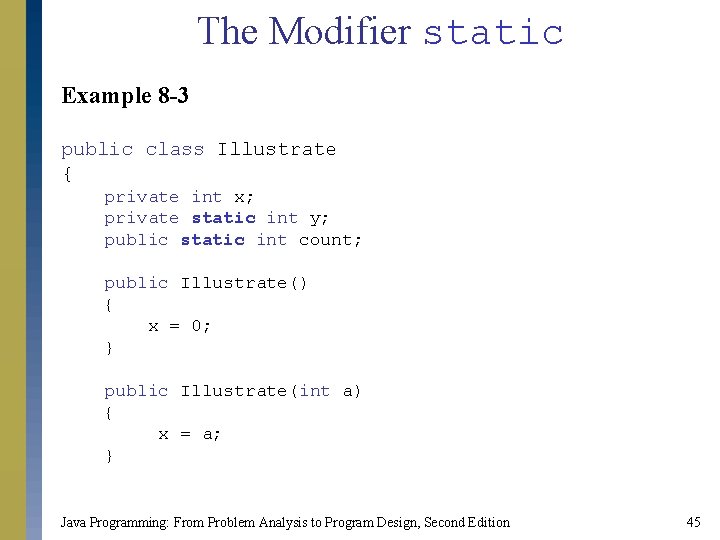
The Modifier static Example 8 -3 public class Illustrate { private int x; private static int y; public static int count; public Illustrate() { x = 0; } public Illustrate(int a) { x = a; } Java Programming: From Problem Analysis to Program Design, Second Edition 45
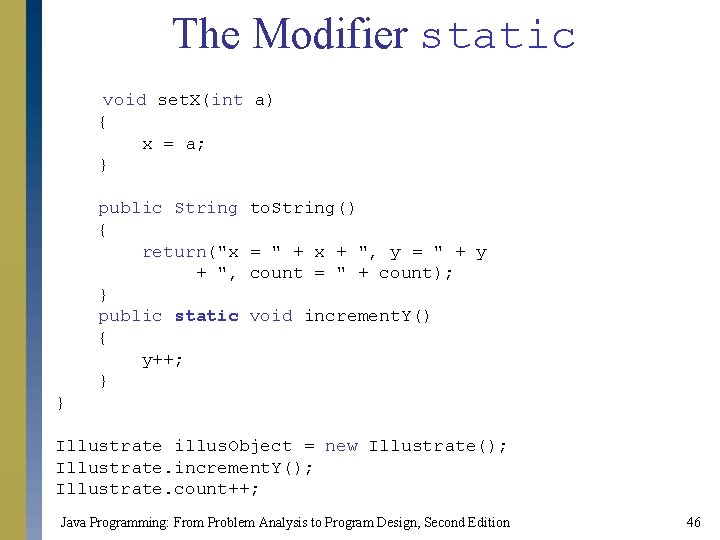
The Modifier static void set. X(int a) { x = a; } public String { return("x + ", } public static { y++; } to. String() = " + x + ", y = " + y count = " + count); void increment. Y() } Illustrate illus. Object = new Illustrate(); Illustrate. increment. Y(); Illustrate. count++; Java Programming: From Problem Analysis to Program Design, Second Edition 46
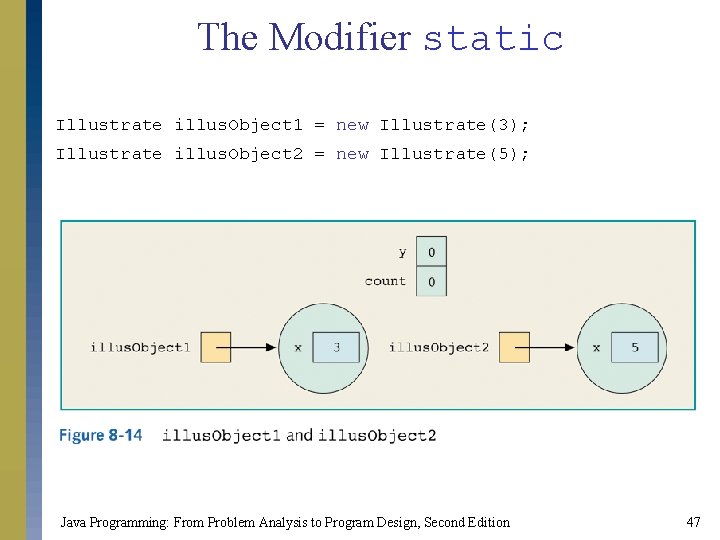
The Modifier static Illustrate illus. Object 1 = new Illustrate(3); Illustrate illus. Object 2 = new Illustrate(5); Java Programming: From Problem Analysis to Program Design, Second Edition 47
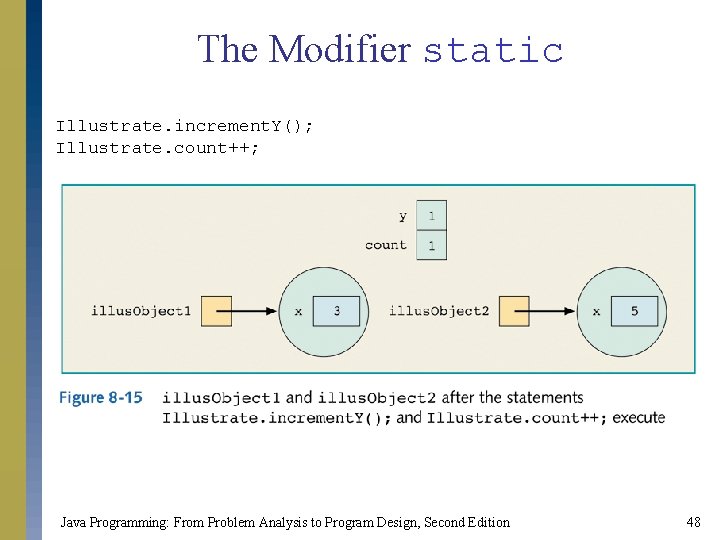
The Modifier static Illustrate. increment. Y(); Illustrate. count++; Java Programming: From Problem Analysis to Program Design, Second Edition 48
![public class Static Members public static void mainString args Illustrate illus Object public class Static. Members { public static void main(String[] args) { Illustrate illus. Object](https://slidetodoc.com/presentation_image_h/2bcbdd0ceb58cd46c49e9cc2946eb43c/image-49.jpg)
public class Static. Members { public static void main(String[] args) { Illustrate illus. Object 1 = new Illustrate(3); Illustrate illus. Object 2 = new Illustrate(5); } } //Line 1 //Line 2 Illustrate. increment. Y(); Illustrate. count++; System. out. println(illus. Object 1); System. out. println(illus. Object 2); //Line 3 4 5 6 System. out. println("Line 7: ***Increment y " + "using illus. Object 1***"); illus. Object 1. increment. Y(); illus. Object 1. set. X(8); System. out. println(illus. Object 1); System. out. println(illus. Object 2); //Line //Line 7 8 9 10 11 System. out. println("Line 12: ***Increment y " + "using illus. Object 2***"); illus. Object 2. increment. Y(); illus. Object 2. set. X(23); System. out. println(illus. Object 1); System. out. println(illus. Object 2); //Line //Line 12 13 14 15 16 Java Programming: From Problem Analysis to Program Design, Second Edition 49
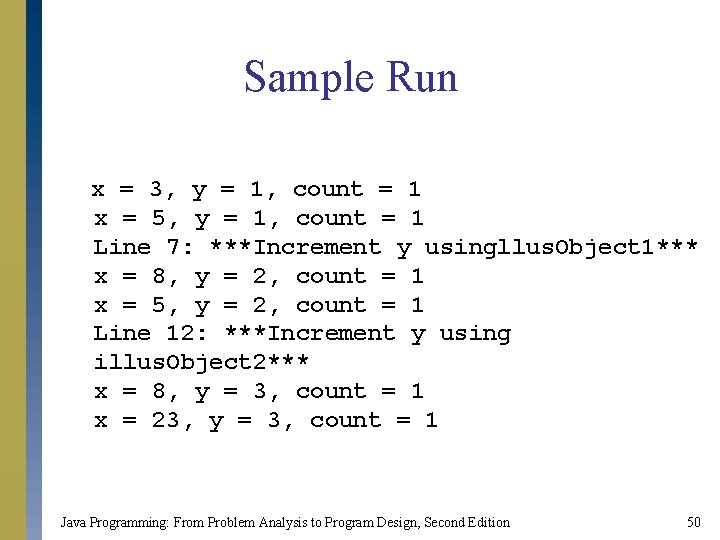
Sample Run x = 3, y = 1, count = 1 x = 5, y = 1, count = 1 Line 7: ***Increment y usingllus. Object 1*** x = 8, y = 2, count = 1 x = 5, y = 2, count = 1 Line 12: ***Increment y using illus. Object 2*** x = 8, y = 3, count = 1 x = 23, y = 3, count = 1 Java Programming: From Problem Analysis to Program Design, Second Edition 50
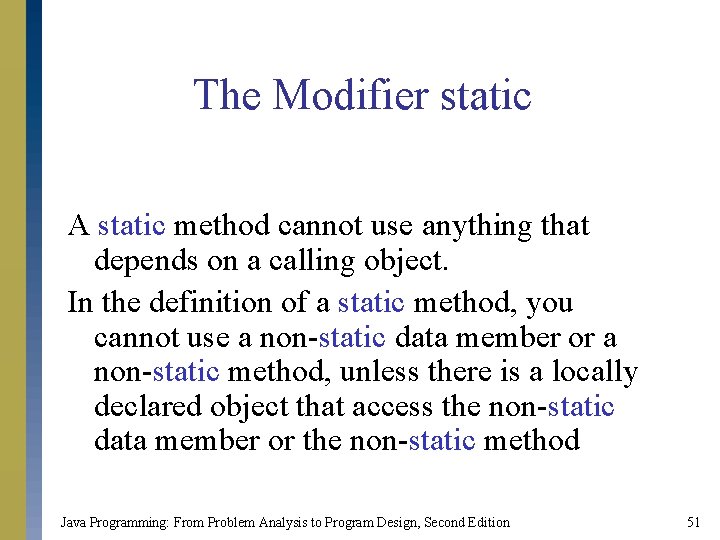
The Modifier static A static method cannot use anything that depends on a calling object. In the definition of a static method, you cannot use a non-static data member or a non-static method, unless there is a locally declared object that access the non-static data member or the non-static method Java Programming: From Problem Analysis to Program Design, Second Edition 51
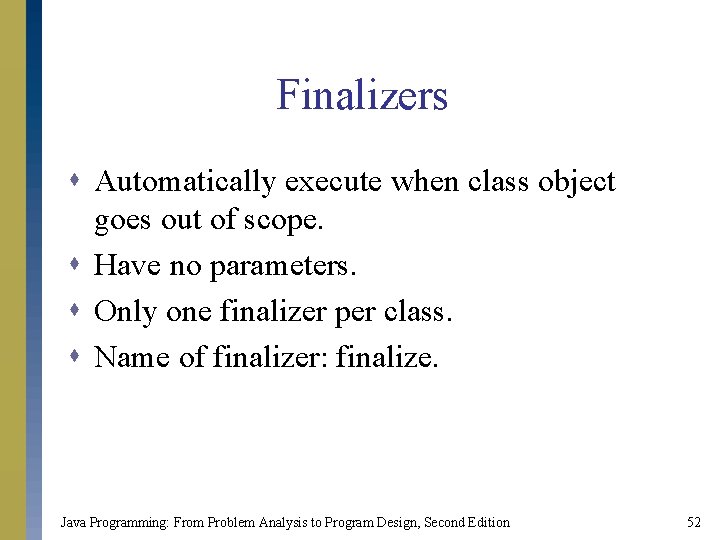
Finalizers s Automatically execute when class object goes out of scope. s Have no parameters. s Only one finalizer per class. s Name of finalizer: finalize. Java Programming: From Problem Analysis to Program Design, Second Edition 52
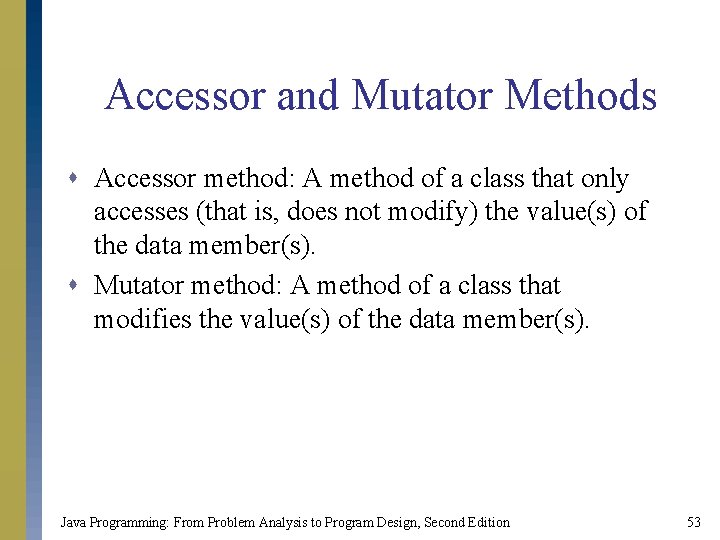
Accessor and Mutator Methods s Accessor method: A method of a class that only accesses (that is, does not modify) the value(s) of the data member(s). s Mutator method: A method of a class that modifies the value(s) of the data member(s). Java Programming: From Problem Analysis to Program Design, Second Edition 53
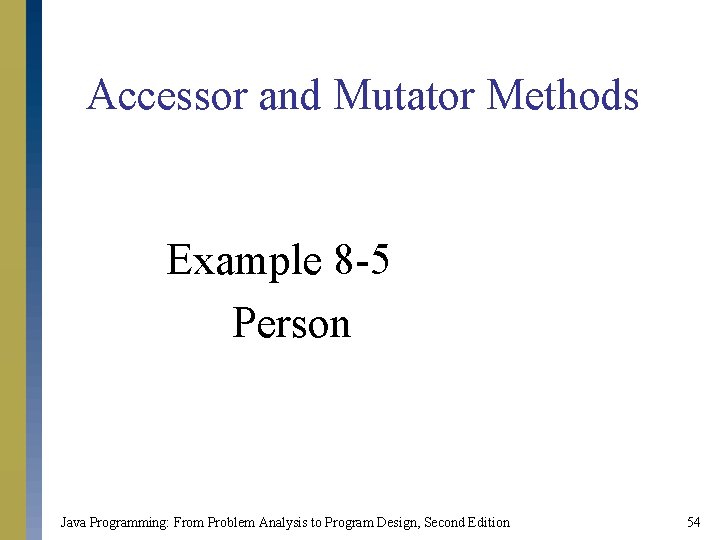
Accessor and Mutator Methods Example 8 -5 Person Java Programming: From Problem Analysis to Program Design, Second Edition 54
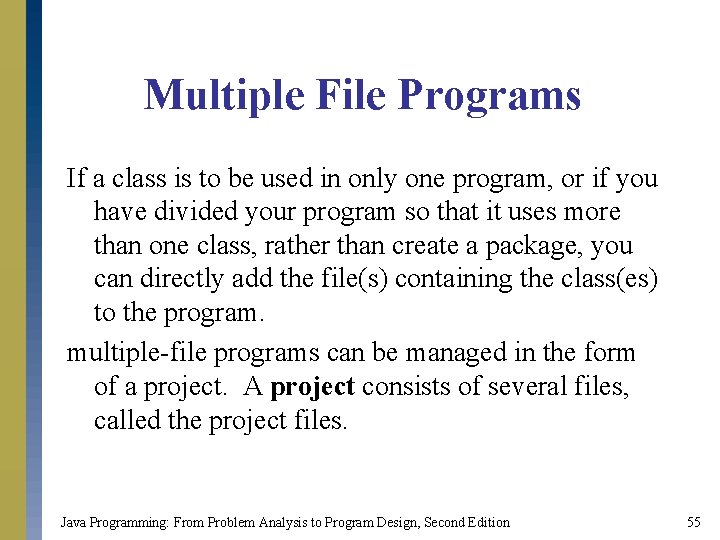
Multiple File Programs If a class is to be used in only one program, or if you have divided your program so that it uses more than one class, rather than create a package, you can directly add the file(s) containing the class(es) to the program. multiple-file programs can be managed in the form of a project. A project consists of several files, called the project files. Java Programming: From Problem Analysis to Program Design, Second Edition 55
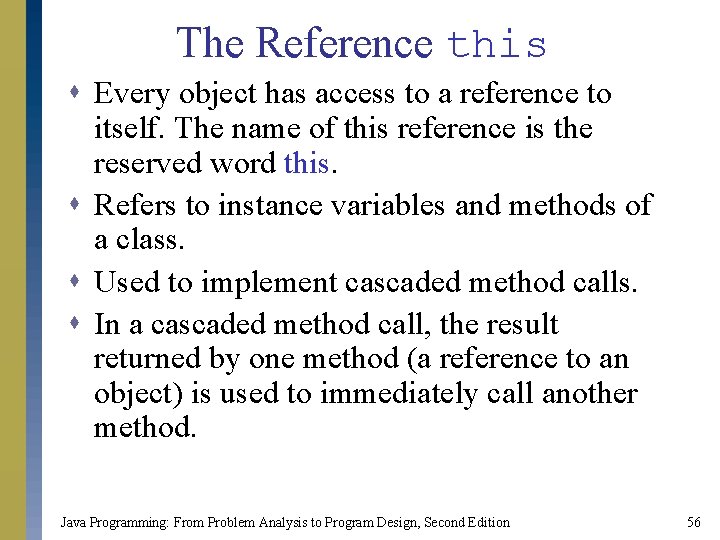
The Reference this s Every object has access to a reference to itself. The name of this reference is the reserved word this. s Refers to instance variables and methods of a class. s Used to implement cascaded method calls. s In a cascaded method call, the result returned by one method (a reference to an object) is used to immediately call another method. Java Programming: From Problem Analysis to Program Design, Second Edition 56
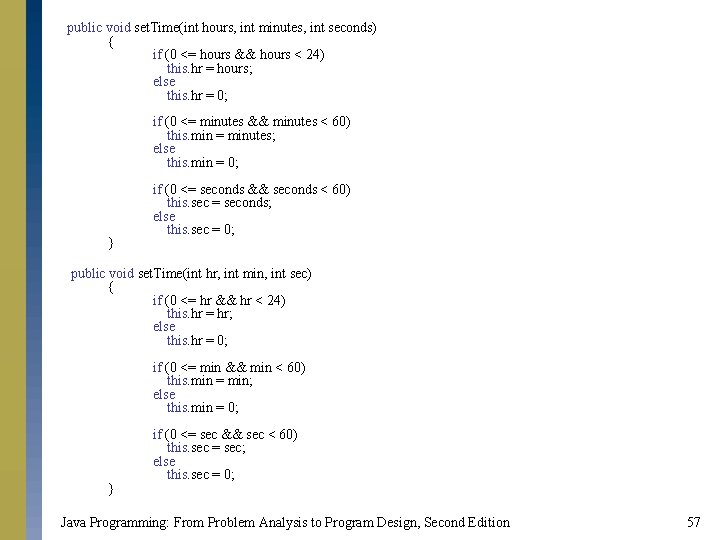
public void set. Time(int hours, int minutes, int seconds) { if (0 <= hours && hours < 24) this. hr = hours; else this. hr = 0; if (0 <= minutes && minutes < 60) this. min = minutes; else this. min = 0; } if (0 <= seconds && seconds < 60) this. sec = seconds; else this. sec = 0; public void set. Time(int hr, int min, int sec) { if (0 <= hr && hr < 24) this. hr = hr; else this. hr = 0; if (0 <= min && min < 60) this. min = min; else this. min = 0; } if (0 <= sec && sec < 60) this. sec = sec; else this. sec = 0; Java Programming: From Problem Analysis to Program Design, Second Edition 57
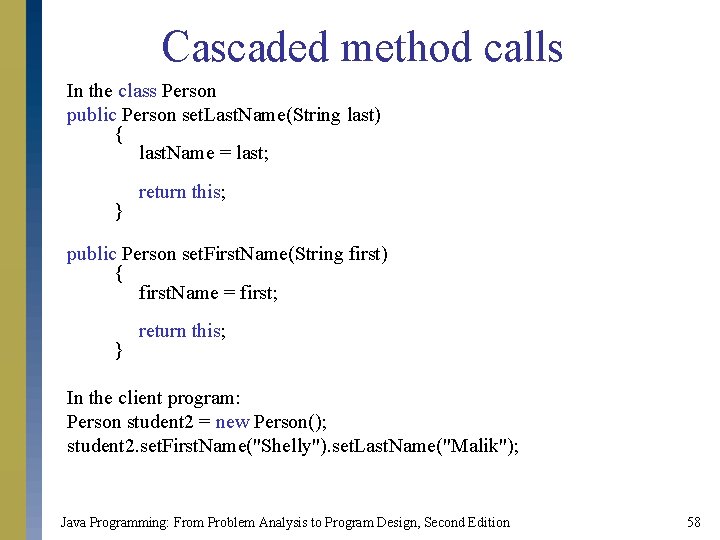
Cascaded method calls In the class Person public Person set. Last. Name(String last) { last. Name = last; } return this; public Person set. First. Name(String first) { first. Name = first; } return this; In the client program: Person student 2 = new Person(); student 2. set. First. Name("Shelly"). set. Last. Name("Malik"); Java Programming: From Problem Analysis to Program Design, Second Edition 58
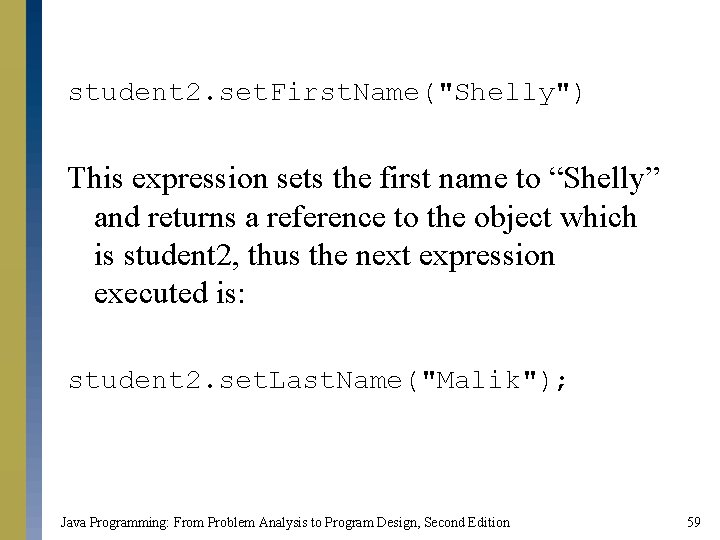
student 2. set. First. Name("Shelly") This expression sets the first name to “Shelly” and returns a reference to the object which is student 2, thus the next expression executed is: student 2. set. Last. Name("Malik"); Java Programming: From Problem Analysis to Program Design, Second Edition 59
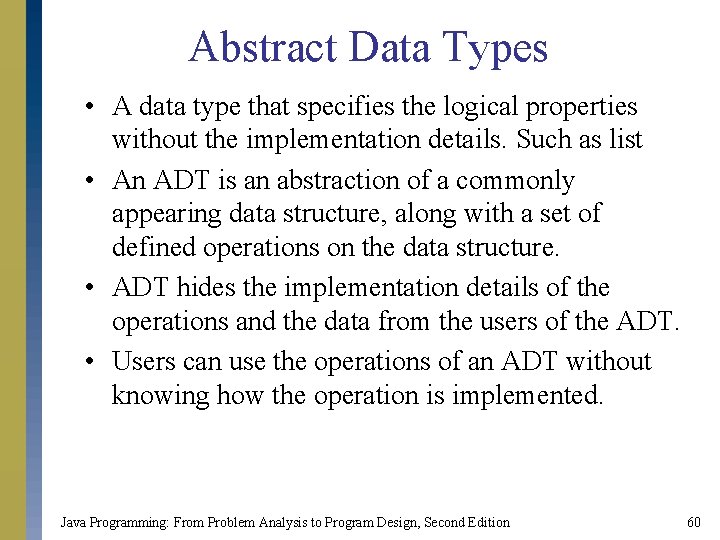
Abstract Data Types • A data type that specifies the logical properties without the implementation details. Such as list • An ADT is an abstraction of a commonly appearing data structure, along with a set of defined operations on the data structure. • ADT hides the implementation details of the operations and the data from the users of the ADT. • Users can use the operations of an ADT without knowing how the operation is implemented. Java Programming: From Problem Analysis to Program Design, Second Edition 60
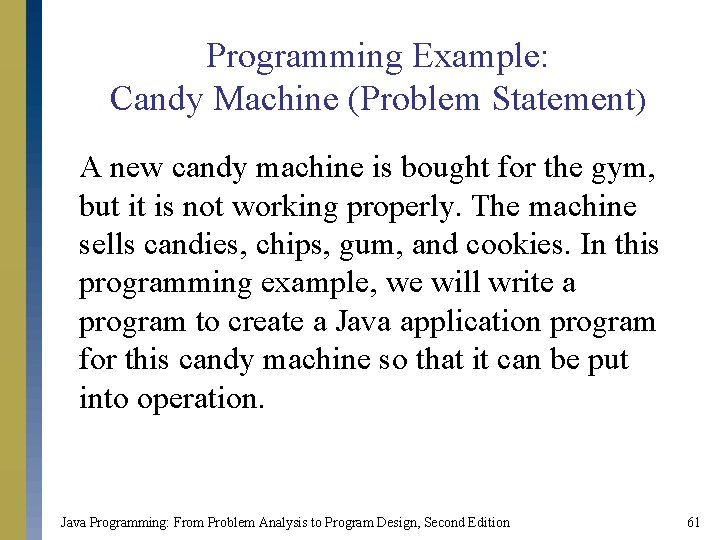
Programming Example: Candy Machine (Problem Statement) A new candy machine is bought for the gym, but it is not working properly. The machine sells candies, chips, gum, and cookies. In this programming example, we will write a program to create a Java application program for this candy machine so that it can be put into operation. Java Programming: From Problem Analysis to Program Design, Second Edition 61
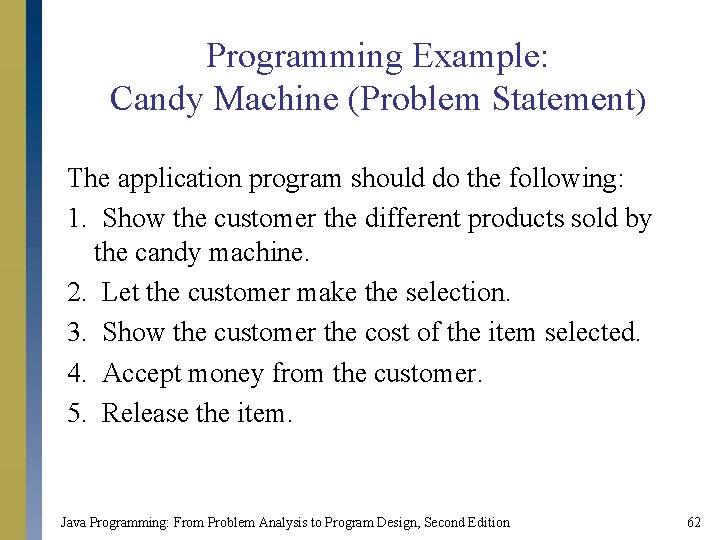
Programming Example: Candy Machine (Problem Statement) The application program should do the following: 1. Show the customer the different products sold by the candy machine. 2. Let the customer make the selection. 3. Show the customer the cost of the item selected. 4. Accept money from the customer. 5. Release the item. Java Programming: From Problem Analysis to Program Design, Second Edition 62
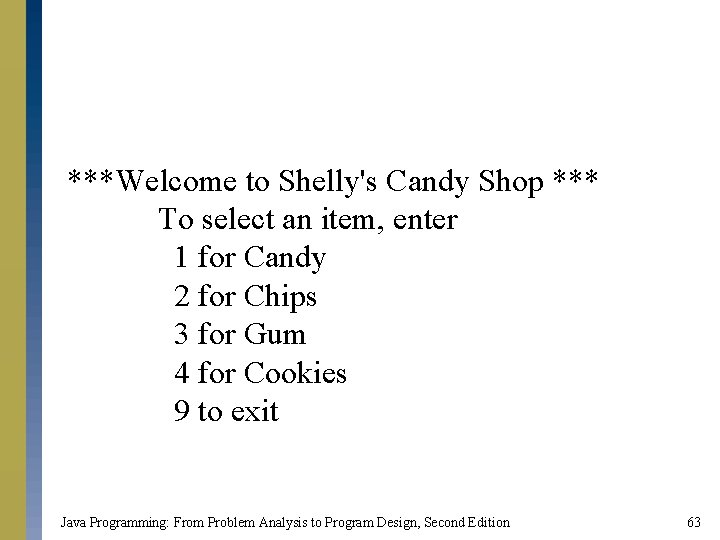
***Welcome to Shelly's Candy Shop *** To select an item, enter 1 for Candy 2 for Chips 3 for Gum 4 for Cookies 9 to exit Java Programming: From Problem Analysis to Program Design, Second Edition 63
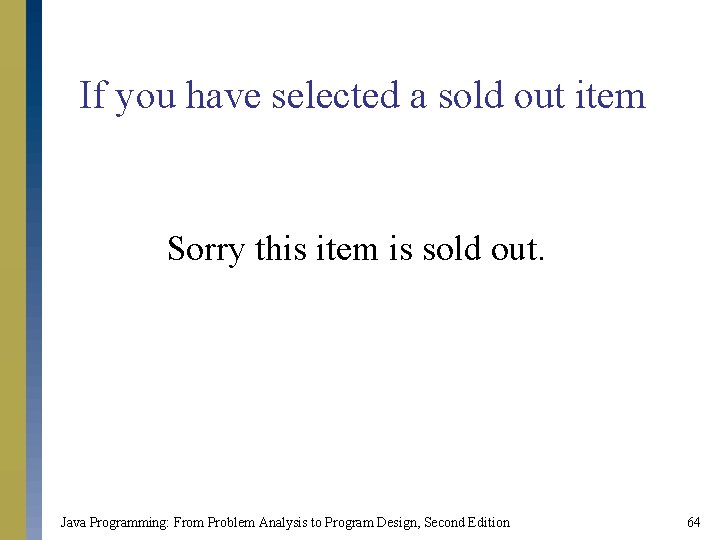
If you have selected a sold out item Sorry this item is sold out. Java Programming: From Problem Analysis to Program Design, Second Edition 64
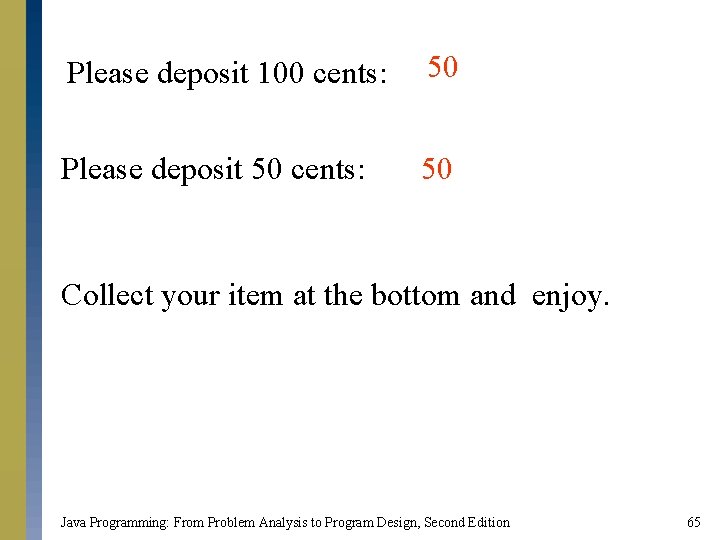
Please deposit 100 cents: 50 Please deposit 50 cents: 50 Collect your item at the bottom and enjoy. Java Programming: From Problem Analysis to Program Design, Second Edition 65
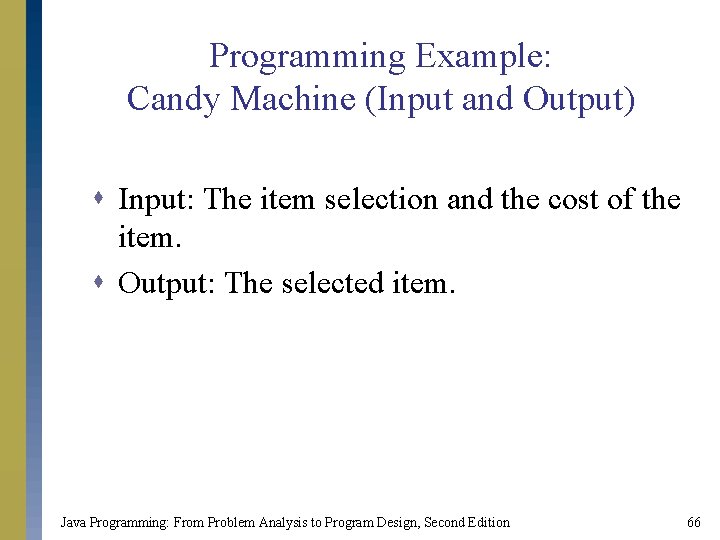
Programming Example: Candy Machine (Input and Output) s Input: The item selection and the cost of the item. s Output: The selected item. Java Programming: From Problem Analysis to Program Design, Second Edition 66
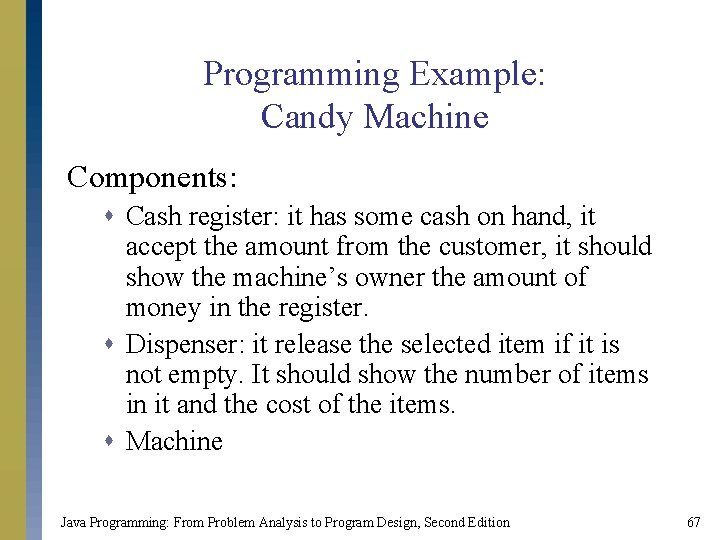
Programming Example: Candy Machine Components: s Cash register: it has some cash on hand, it accept the amount from the customer, it should show the machine’s owner the amount of money in the register. s Dispenser: it release the selected item if it is not empty. It should show the number of items in it and the cost of the items. s Machine Java Programming: From Problem Analysis to Program Design, Second Edition 67
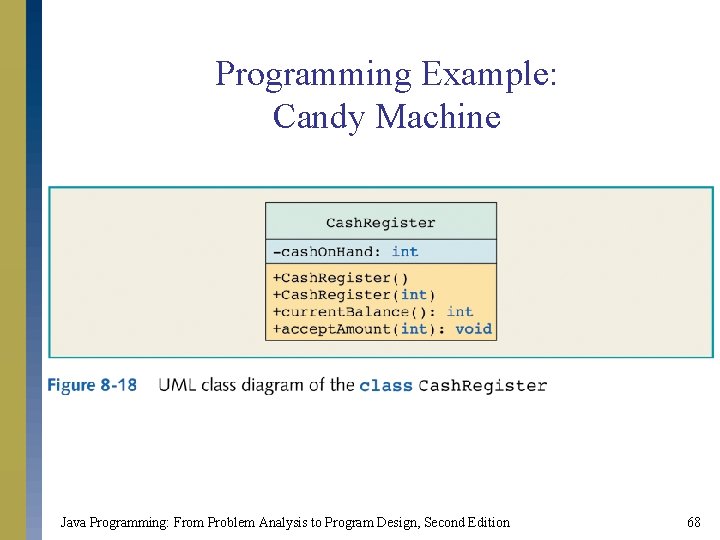
Programming Example: Candy Machine Java Programming: From Problem Analysis to Program Design, Second Edition 68
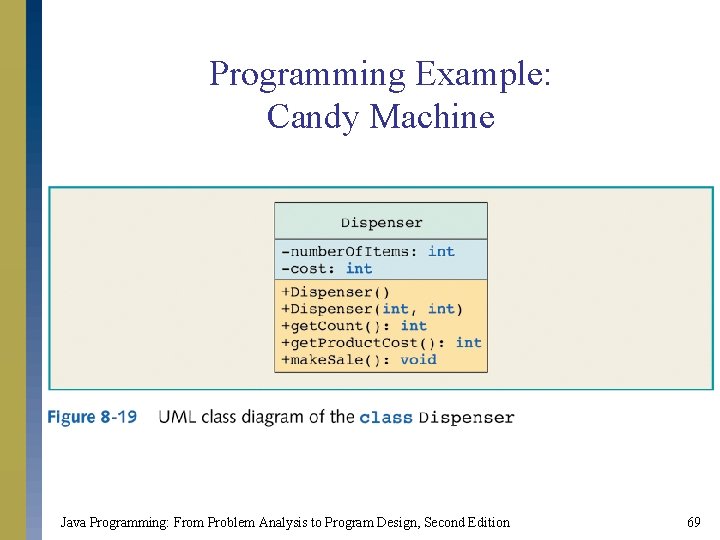
Programming Example: Candy Machine Java Programming: From Problem Analysis to Program Design, Second Edition 69
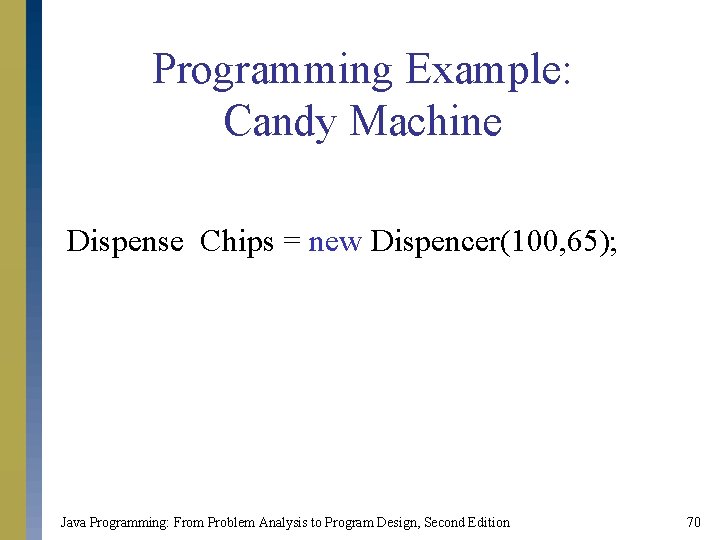
Programming Example: Candy Machine Dispense Chips = new Dispencer(100, 65); Java Programming: From Problem Analysis to Program Design, Second Edition 70
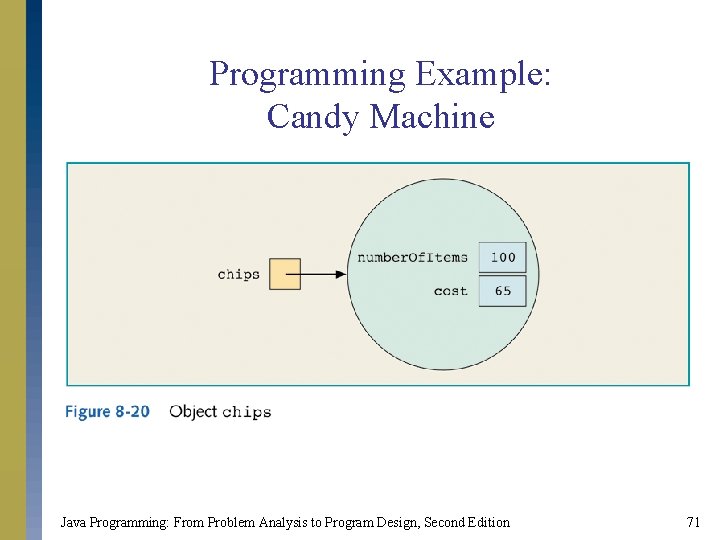
Programming Example: Candy Machine Java Programming: From Problem Analysis to Program Design, Second Edition 71
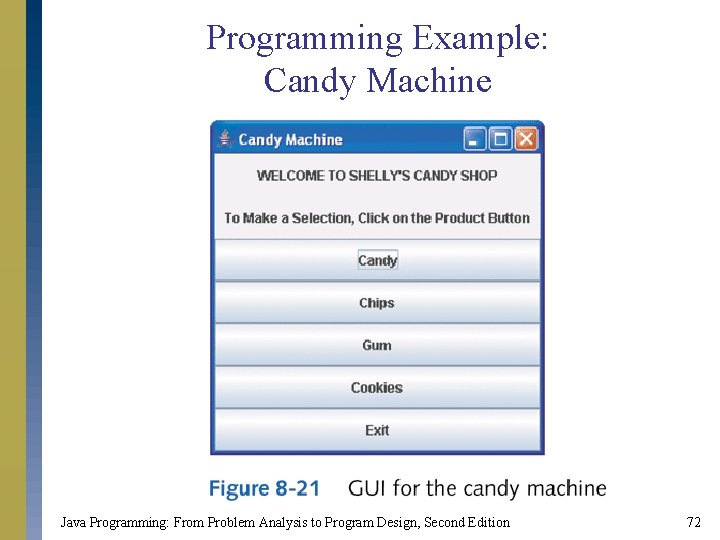
Programming Example: Candy Machine Java Programming: From Problem Analysis to Program Design, Second Edition 72
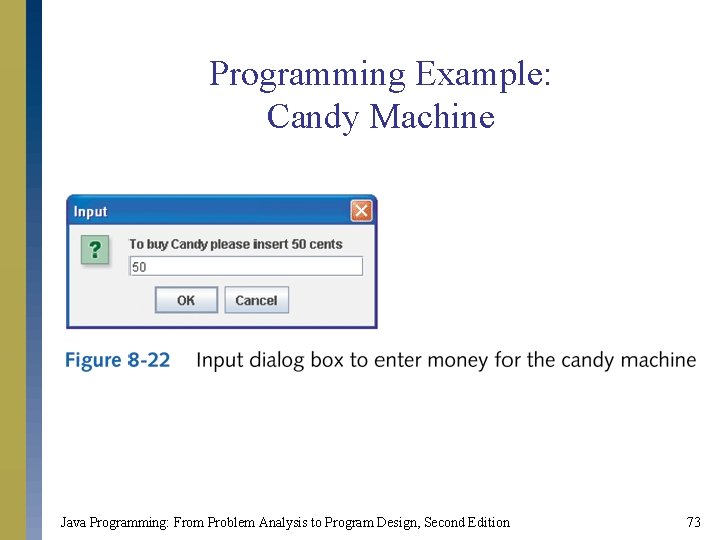
Programming Example: Candy Machine Java Programming: From Problem Analysis to Program Design, Second Edition 73
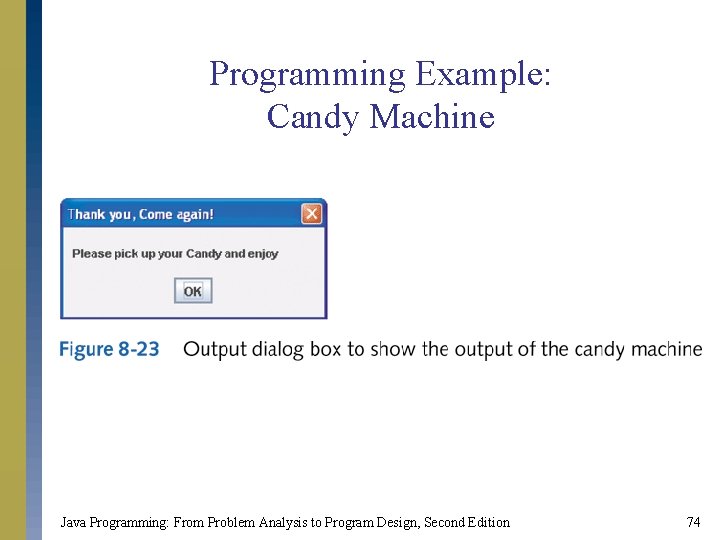
Programming Example: Candy Machine Java Programming: From Problem Analysis to Program Design, Second Edition 74
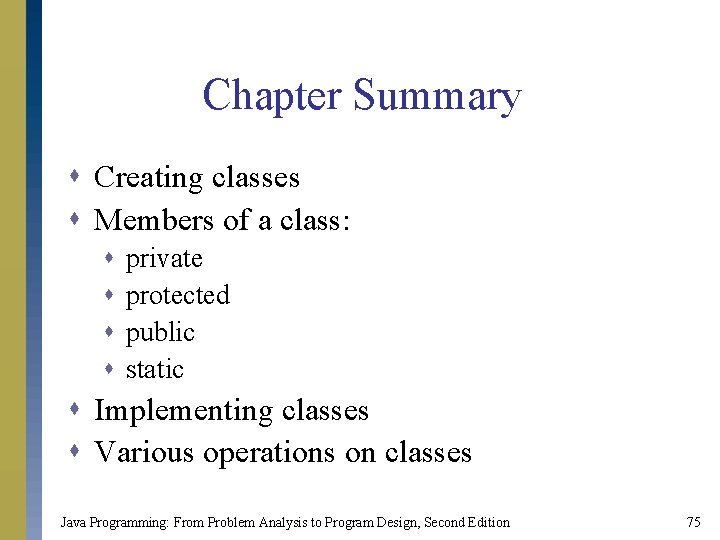
Chapter Summary s Creating classes s Members of a class: s s private protected public static s Implementing classes s Various operations on classes Java Programming: From Problem Analysis to Program Design, Second Edition 75
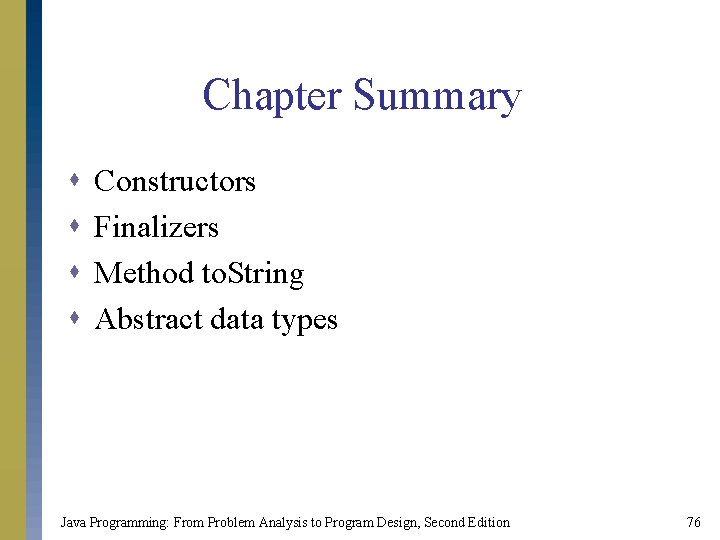
Chapter Summary s s Constructors Finalizers Method to. String Abstract data types Java Programming: From Problem Analysis to Program Design, Second Edition 76