Chapter 8 Testing a class This chapter discusses
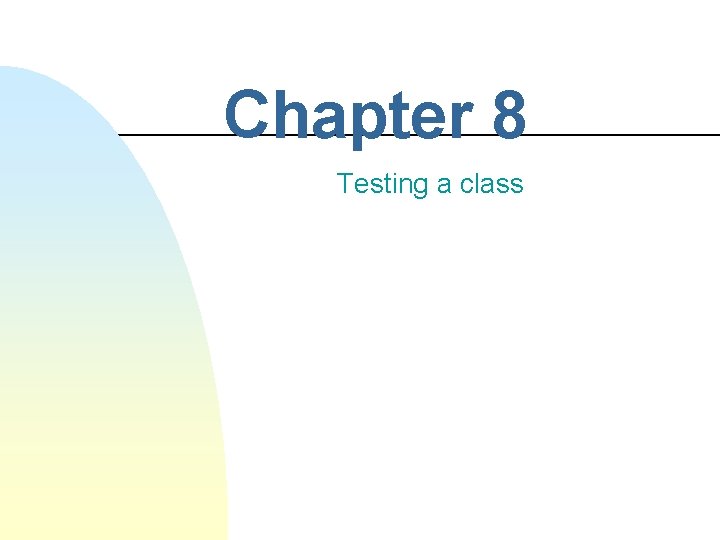
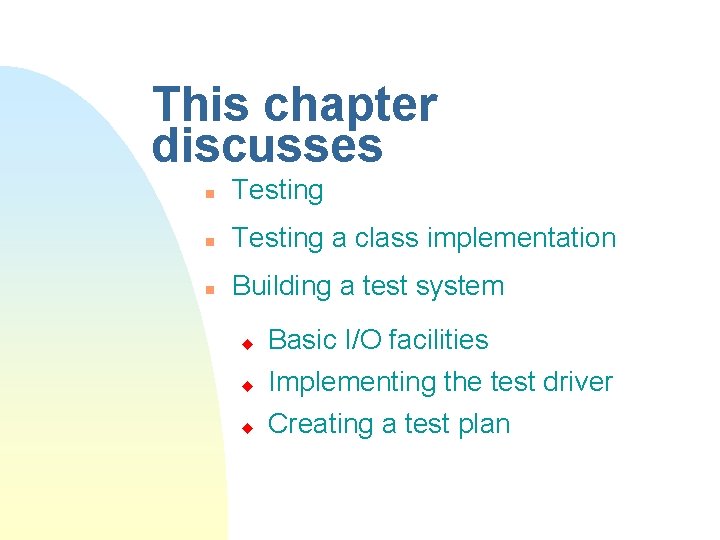
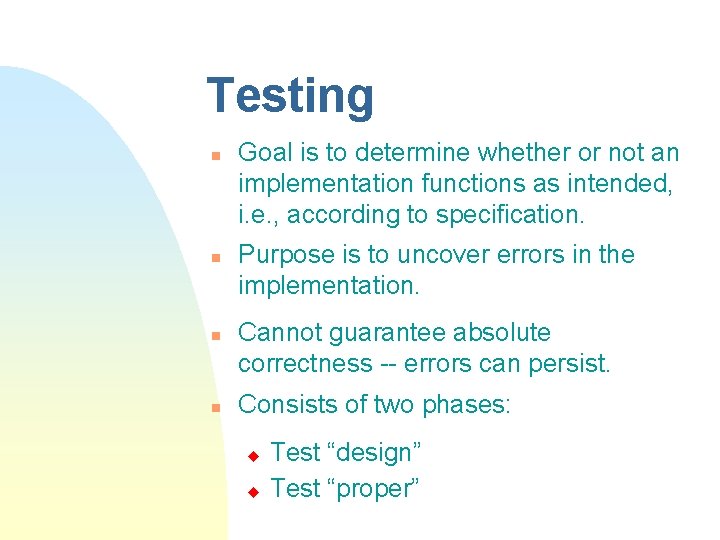
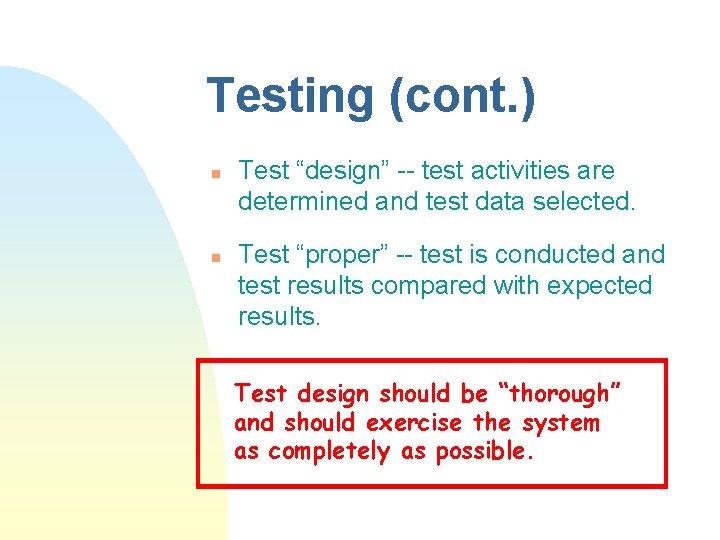
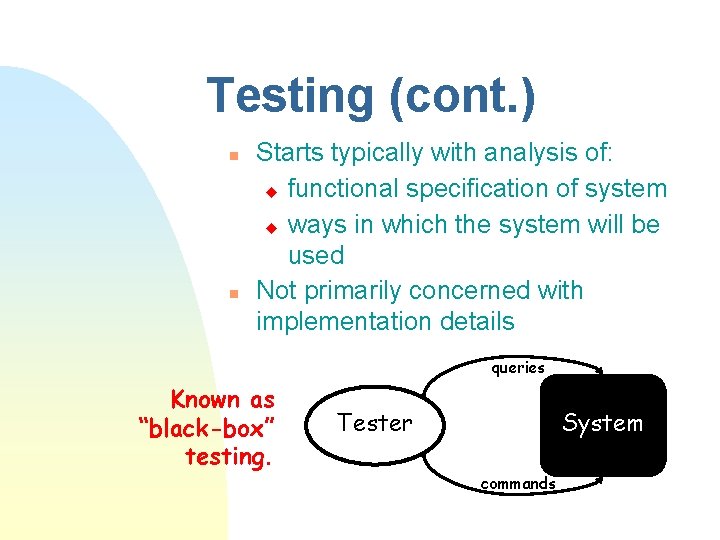
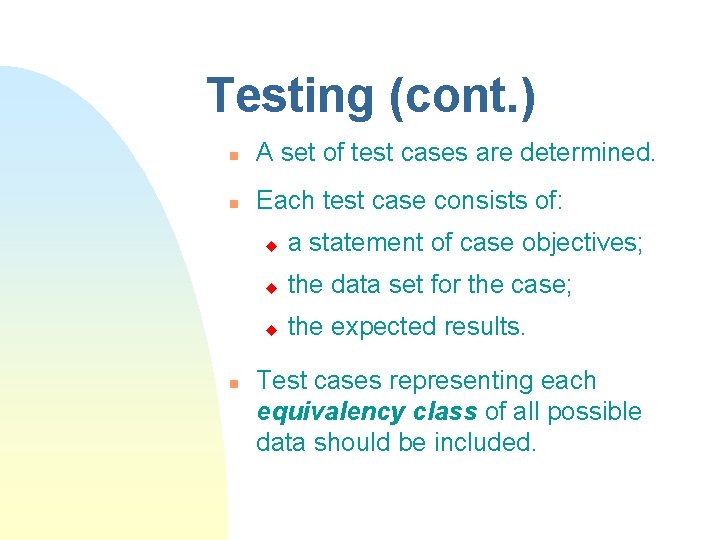
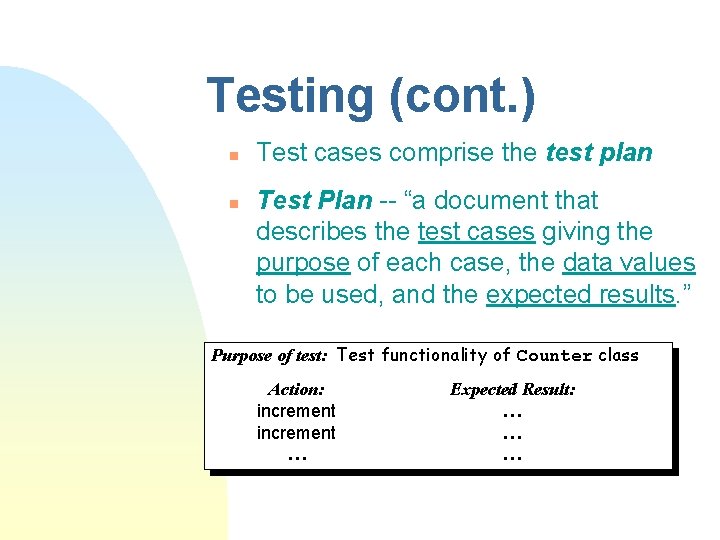
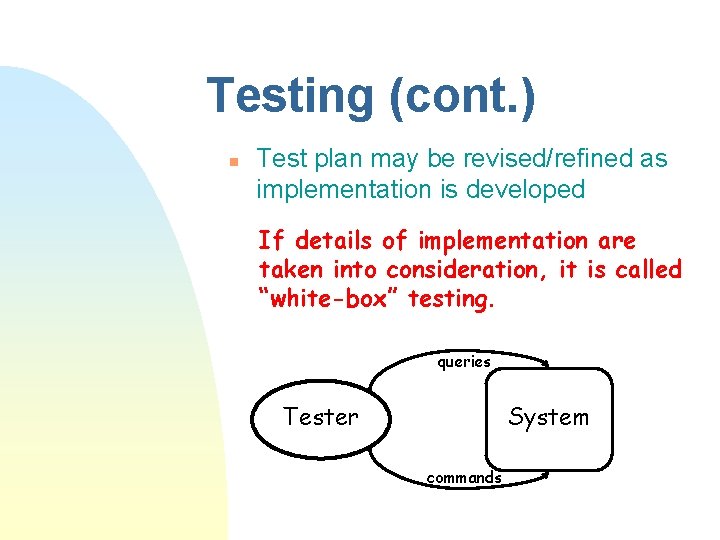
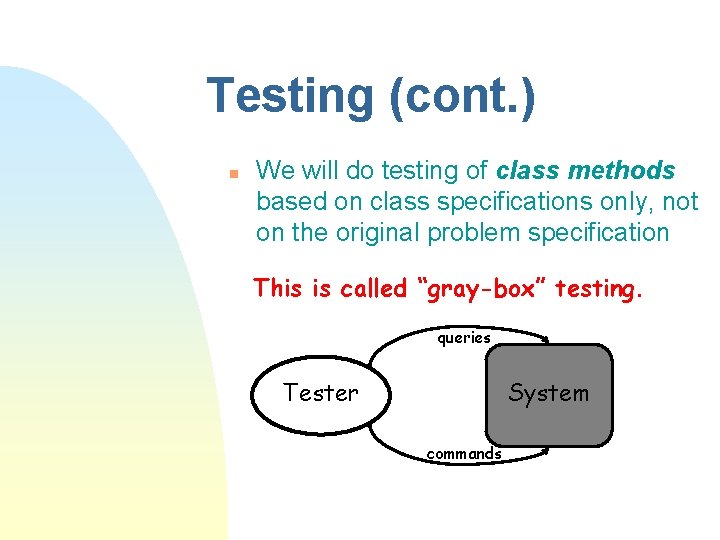
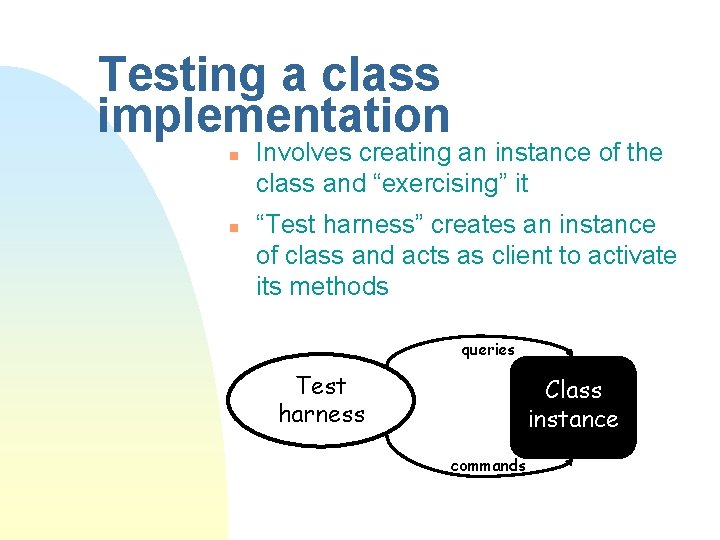
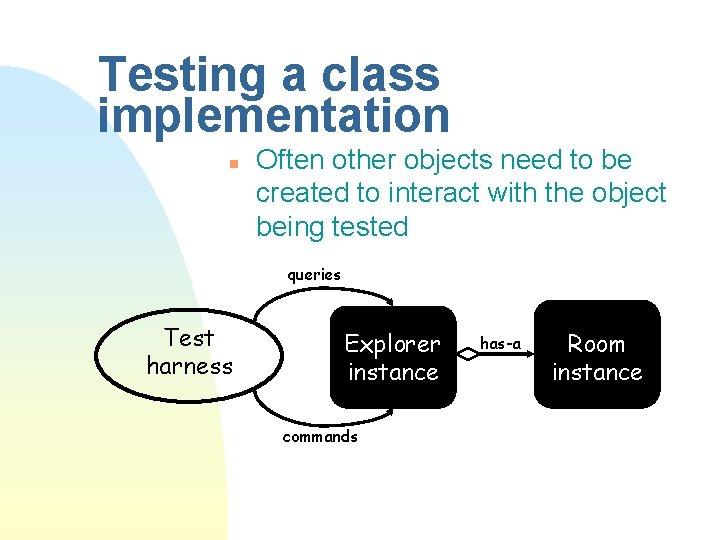
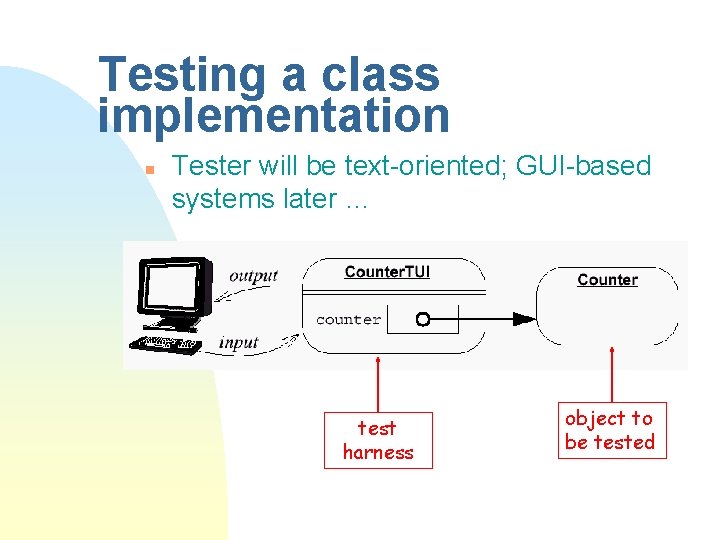
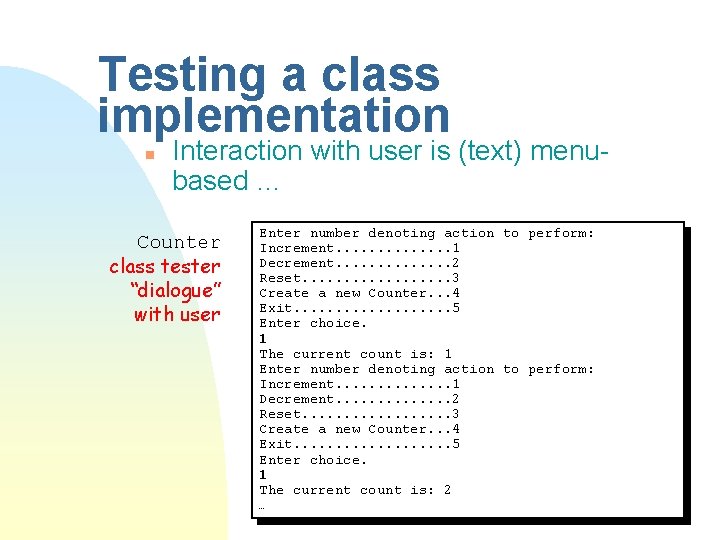
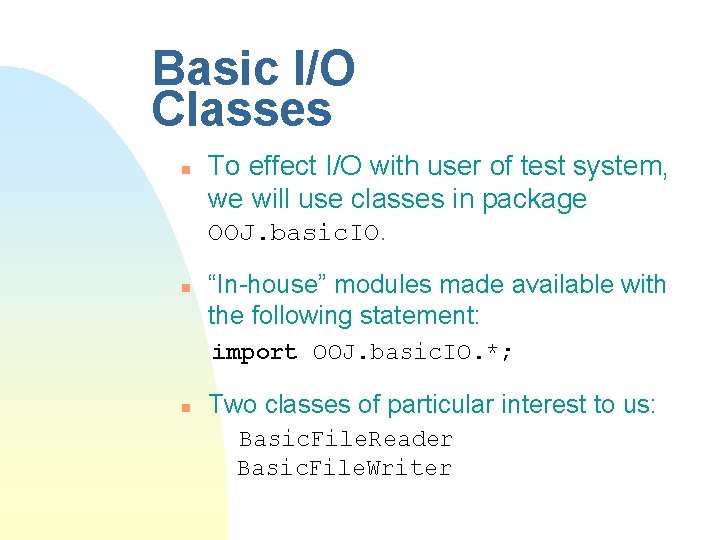
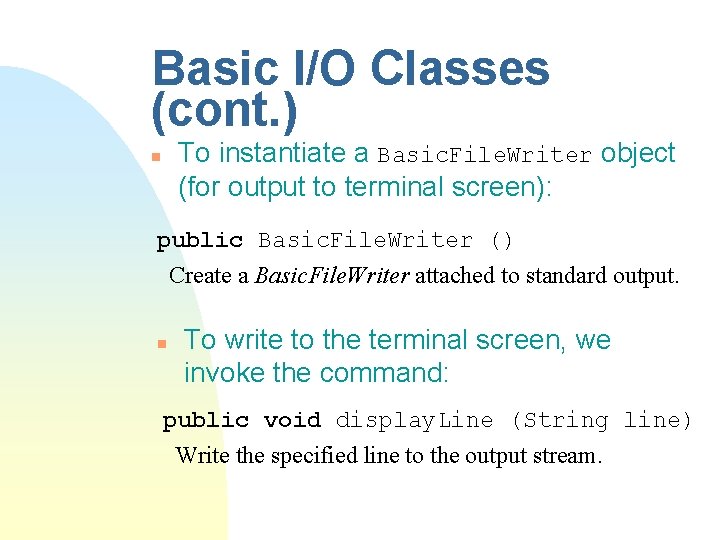
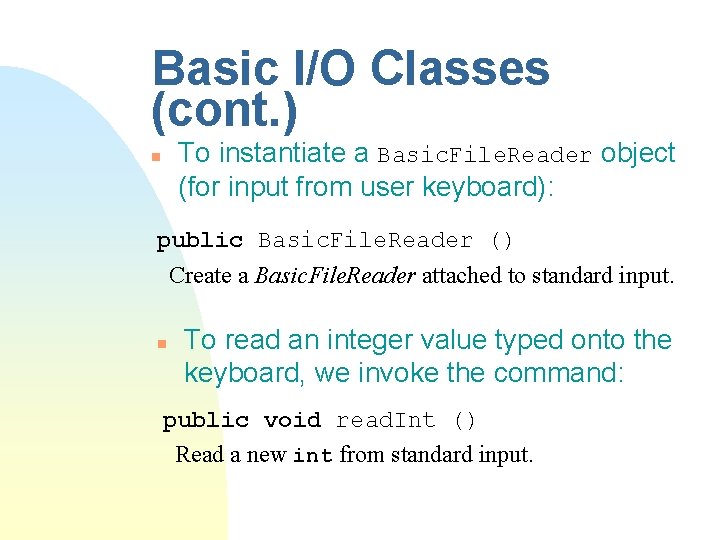
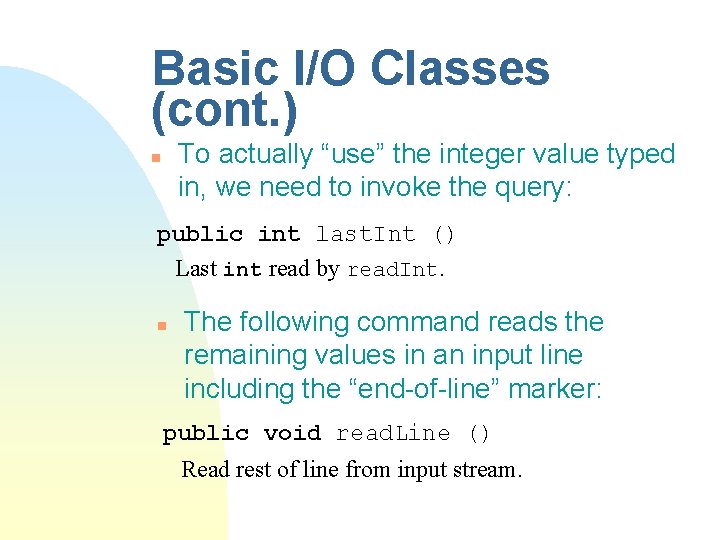
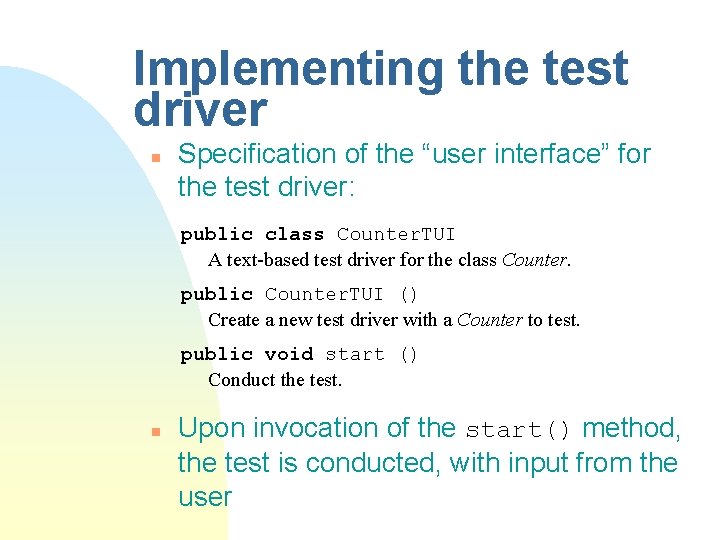
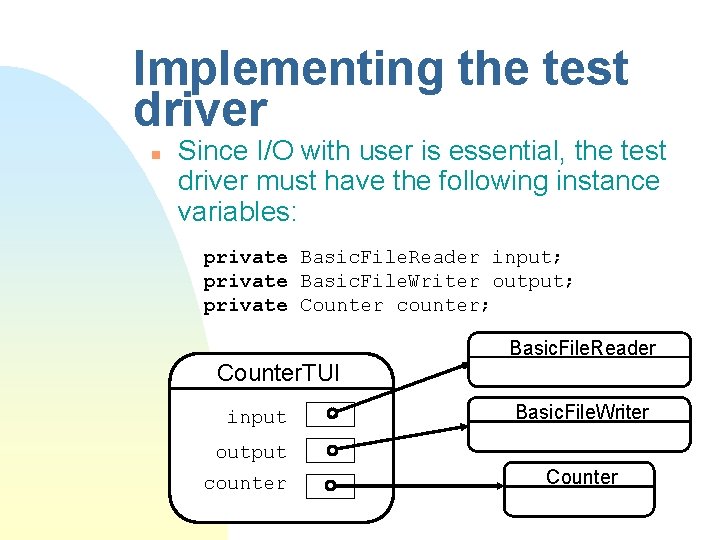
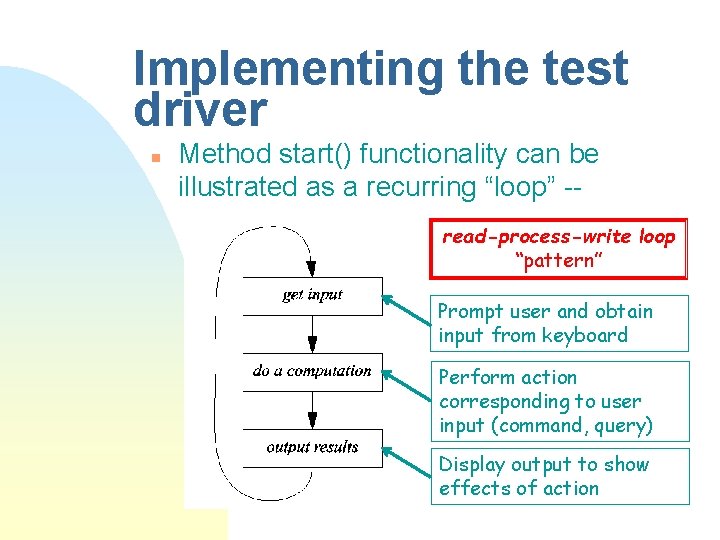
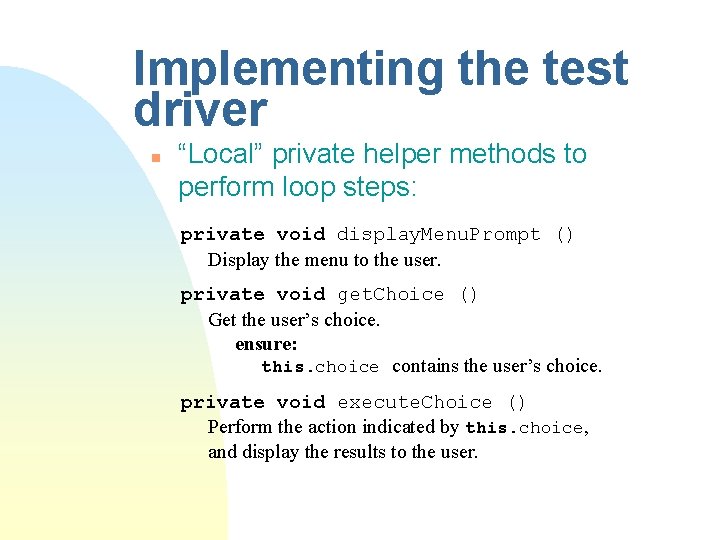
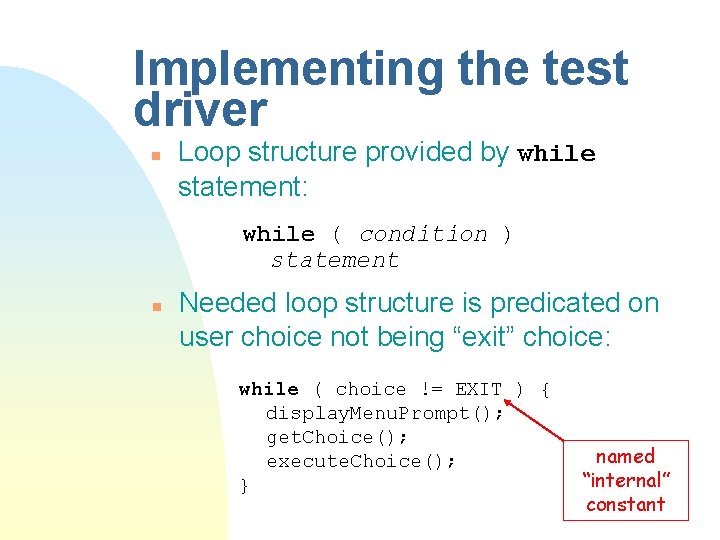
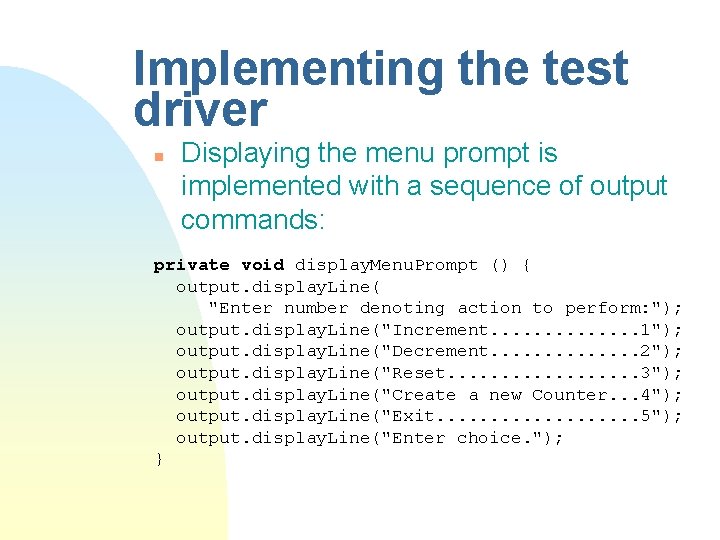
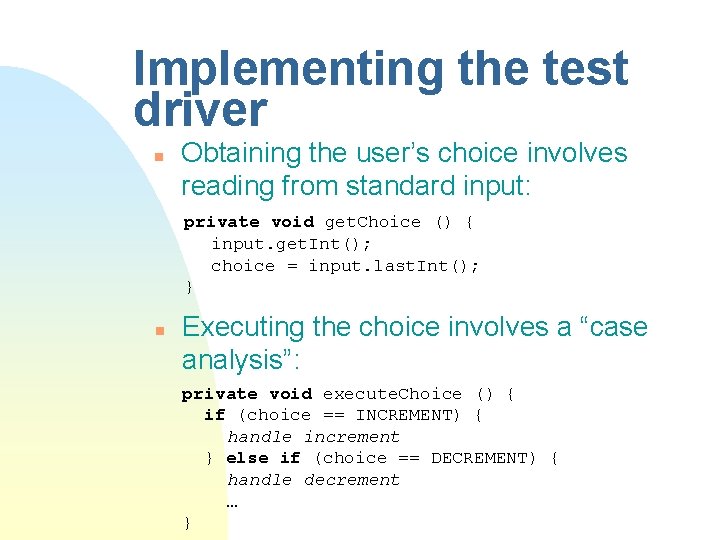
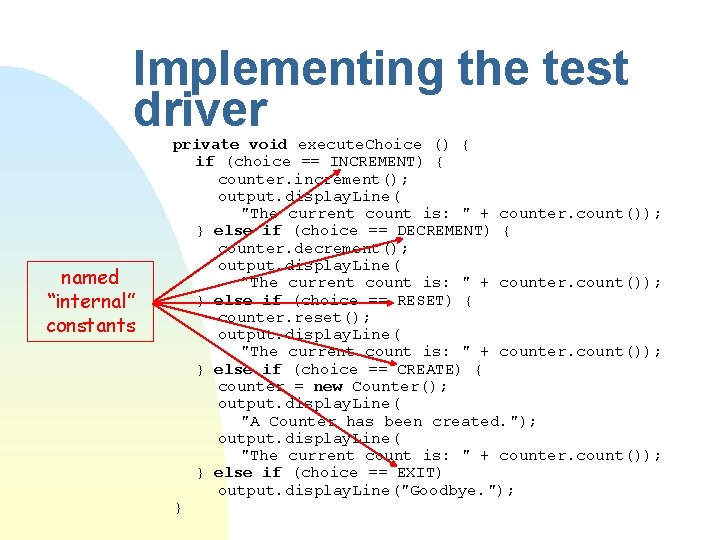
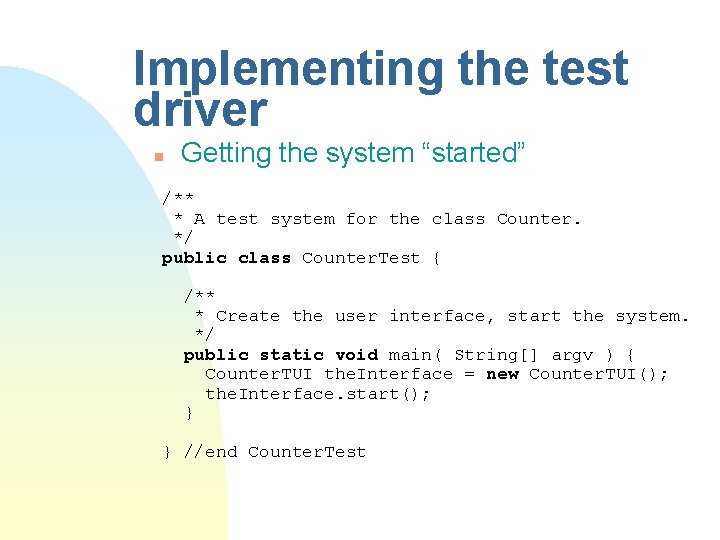
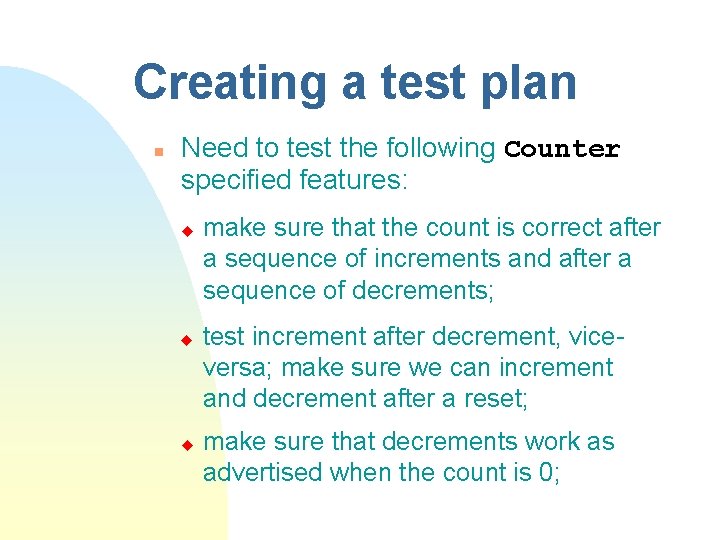
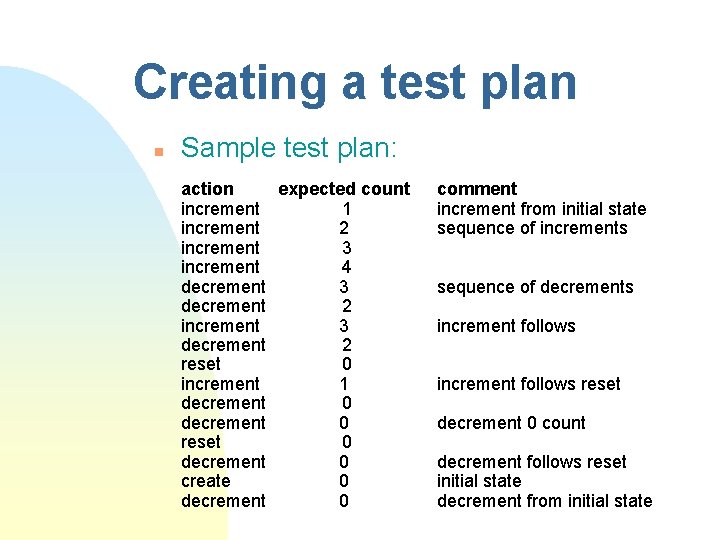
- Slides: 28
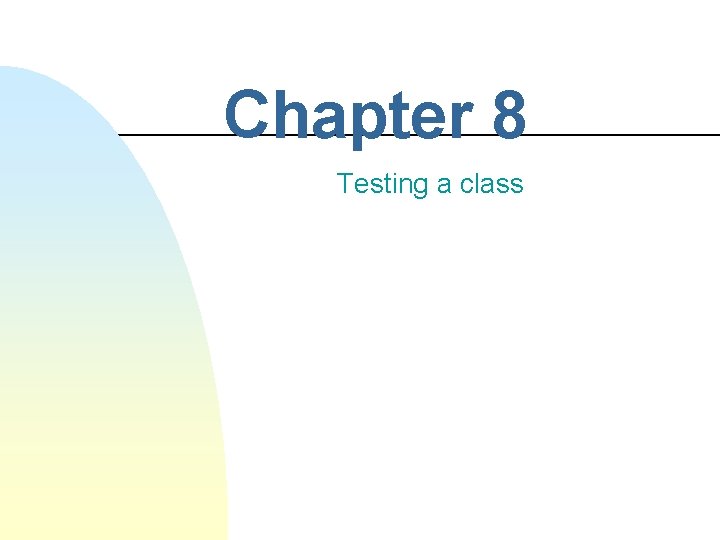
Chapter 8 Testing a class
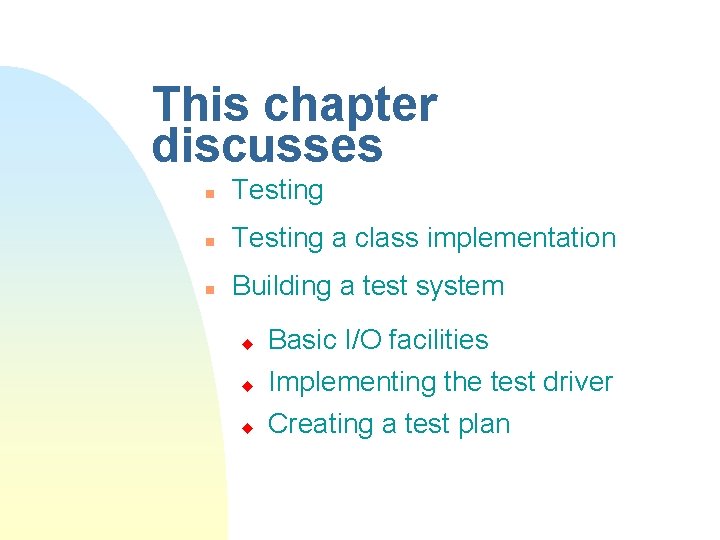
This chapter discusses n Testing a class implementation n Building a test system u u u Basic I/O facilities Implementing the test driver Creating a test plan
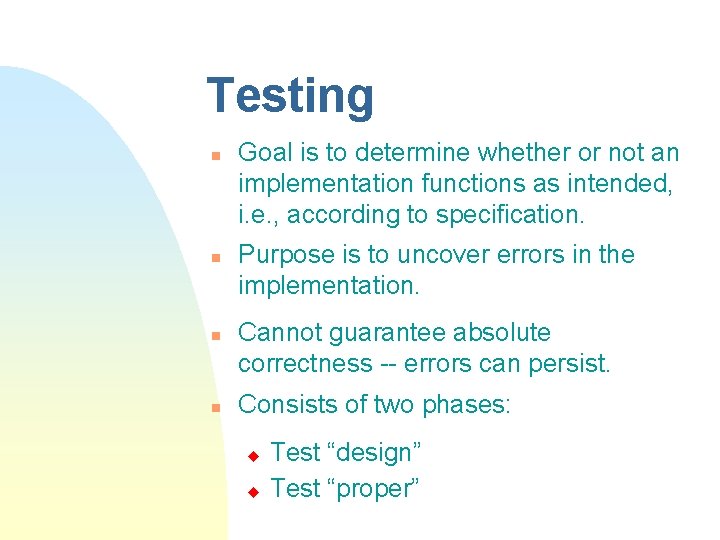
Testing n n Goal is to determine whether or not an implementation functions as intended, i. e. , according to specification. Purpose is to uncover errors in the implementation. Cannot guarantee absolute correctness -- errors can persist. Consists of two phases: u u Test “design” Test “proper”
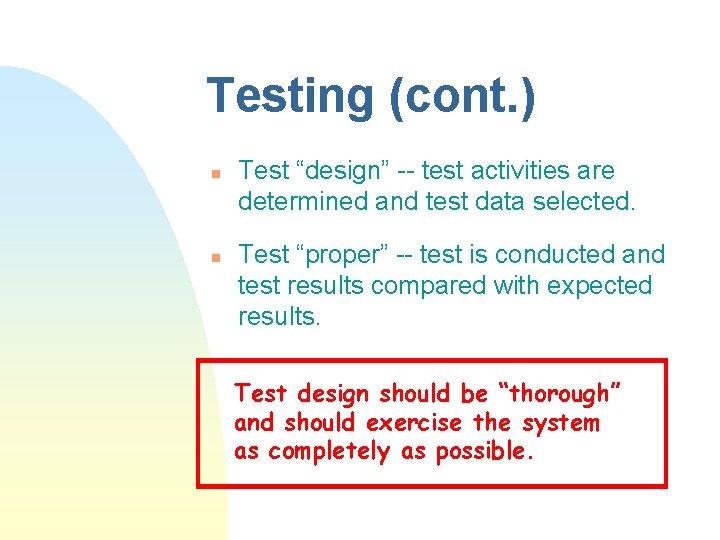
Testing (cont. ) n n Test “design” -- test activities are determined and test data selected. Test “proper” -- test is conducted and test results compared with expected results. Test design should be “thorough” and should exercise the system as completely as possible.
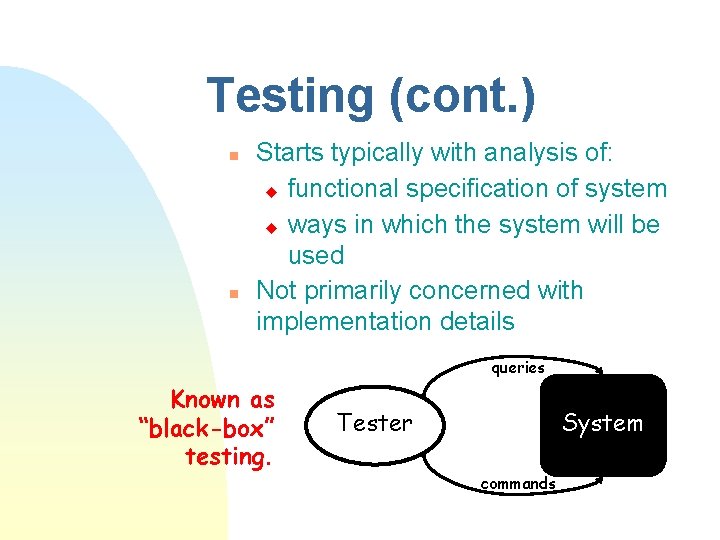
Testing (cont. ) n n Starts typically with analysis of: u functional specification of system u ways in which the system will be used Not primarily concerned with implementation details queries Known as “black-box” testing. Tester System commands
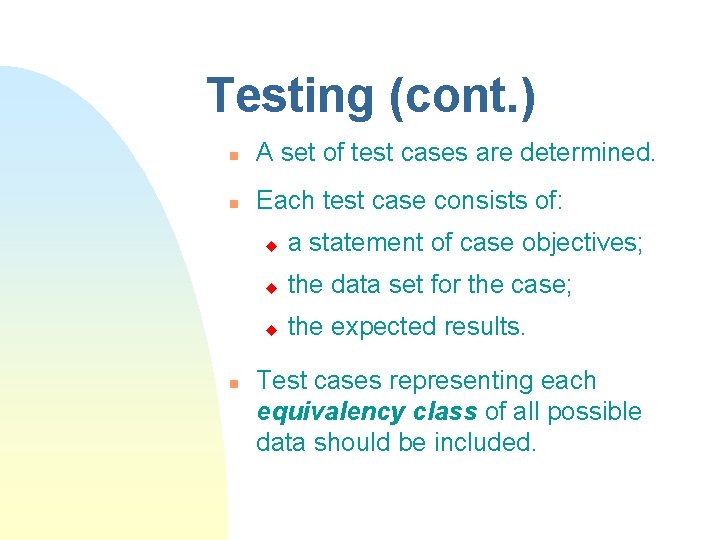
Testing (cont. ) n A set of test cases are determined. n Each test case consists of: n u a statement of case objectives; u the data set for the case; u the expected results. Test cases representing each equivalency class of all possible data should be included.
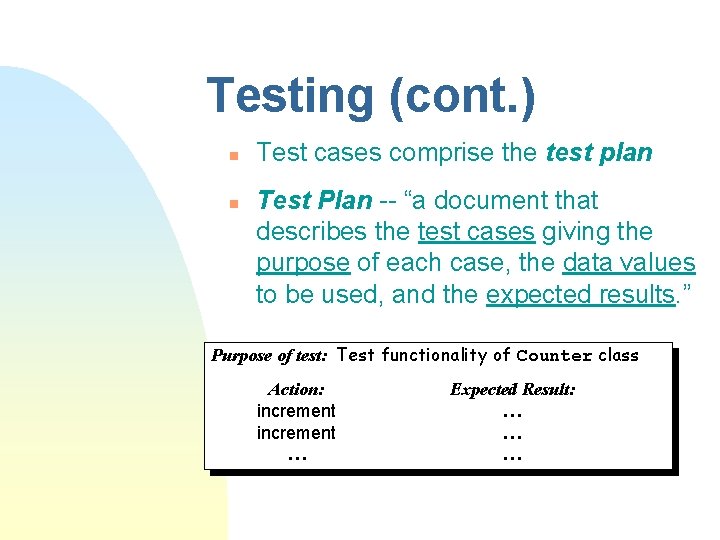
Testing (cont. ) n n Test cases comprise the test plan Test Plan -- “a document that describes the test cases giving the purpose of each case, the data values to be used, and the expected results. ” Purpose of test: Test functionality of Counter class Action: increment … Expected Result: … … …
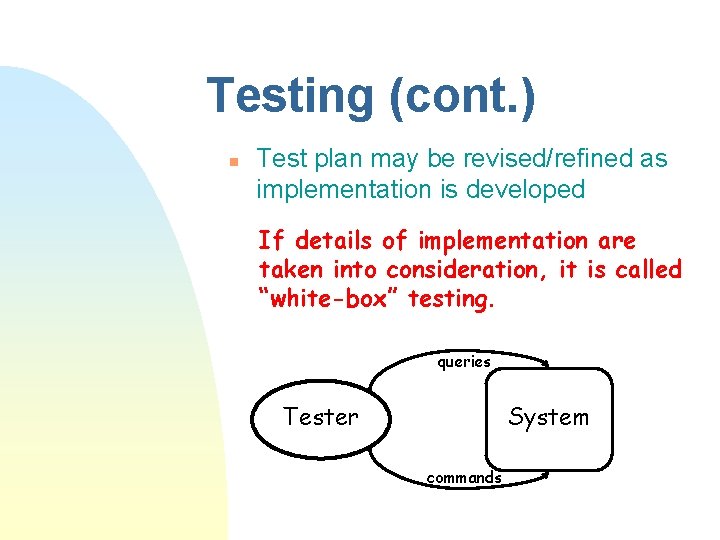
Testing (cont. ) n Test plan may be revised/refined as implementation is developed If details of implementation are taken into consideration, it is called “white-box” testing. queries Tester System commands
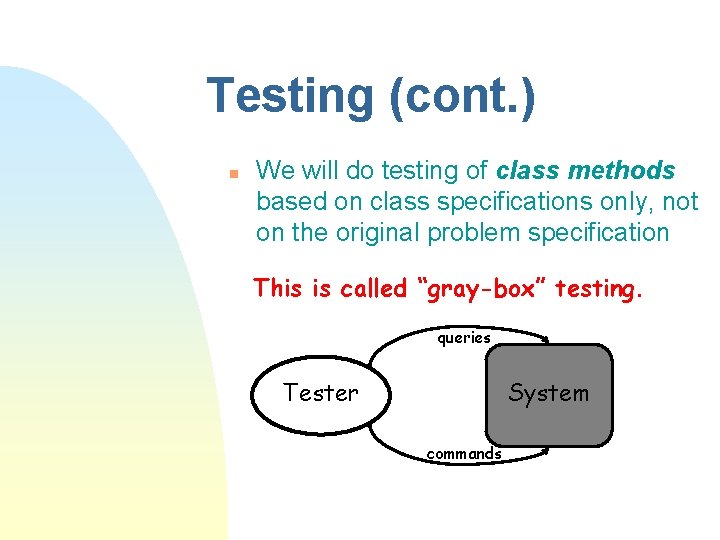
Testing (cont. ) n We will do testing of class methods based on class specifications only, not on the original problem specification This is called “gray-box” testing. queries Tester System commands
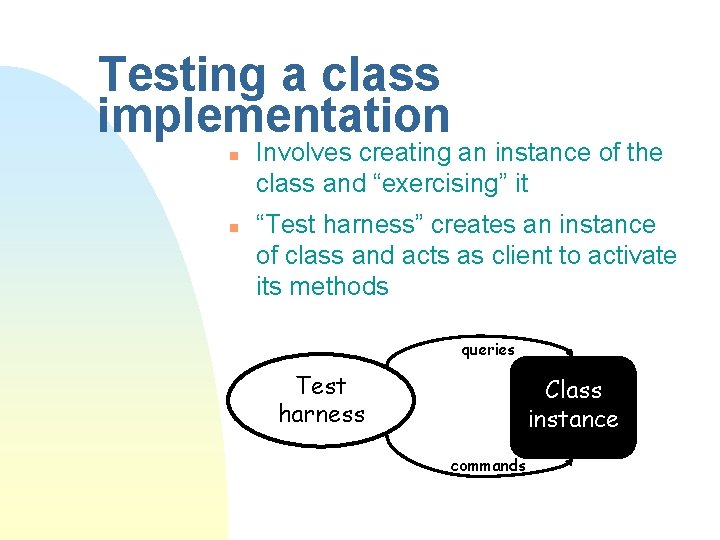
Testing a class implementation n n Involves creating an instance of the class and “exercising” it “Test harness” creates an instance of class and acts as client to activate its methods queries Test harness Class instance commands
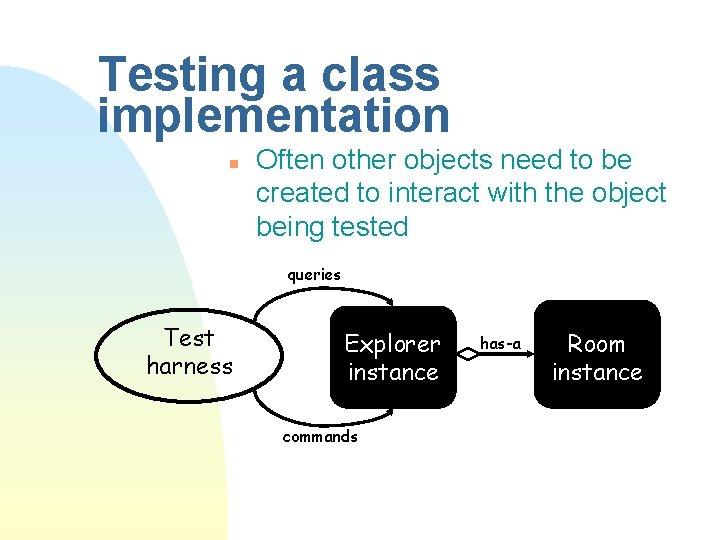
Testing a class implementation n Often other objects need to be created to interact with the object being tested queries Test harness Explorer instance commands has-a Room instance
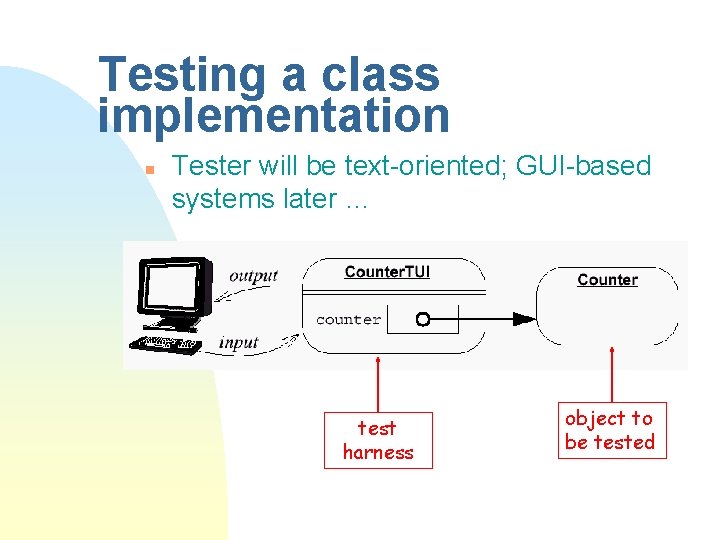
Testing a class implementation n Tester will be text-oriented; GUI-based systems later … test harness object to be tested
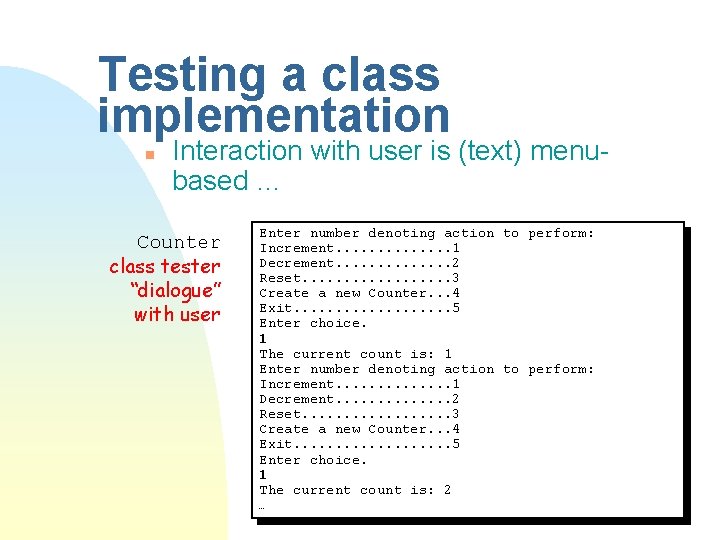
Testing a class implementation n Interaction with user is (text) menubased … Counter class tester “dialogue” with user Enter number denoting action to perform: Increment. . . 1 Decrement. . . 2 Reset. . . . 3 Create a new Counter. . . 4 Exit. . . . . 5 Enter choice. 1 The current count is: 1 Enter number denoting action to perform: Increment. . . 1 Decrement. . . 2 Reset. . . . 3 Create a new Counter. . . 4 Exit. . . . . 5 Enter choice. 1 The current count is: 2 …
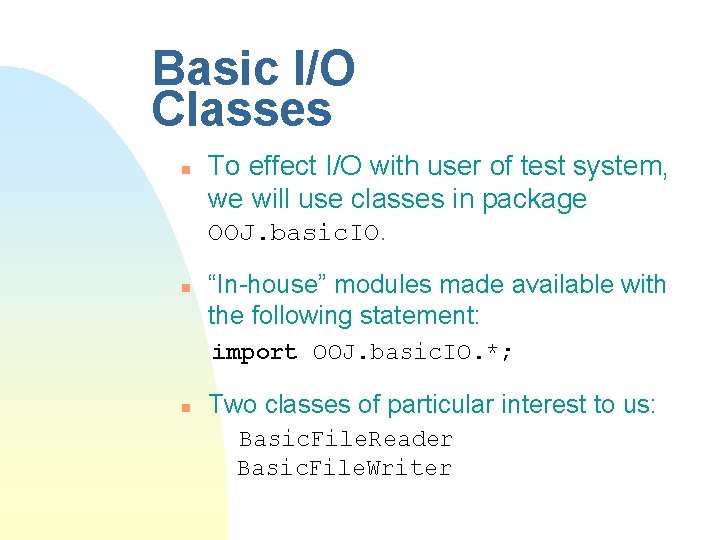
Basic I/O Classes n n To effect I/O with user of test system, we will use classes in package OOJ. basic. IO. “In-house” modules made available with the following statement: import OOJ. basic. IO. *; n Two classes of particular interest to us: Basic. File. Reader Basic. File. Writer
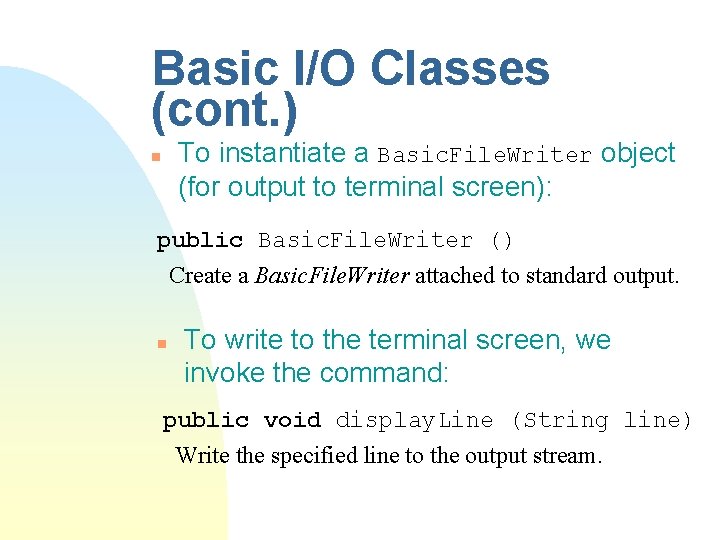
Basic I/O Classes (cont. ) To instantiate a Basic. File. Writer object (for output to terminal screen): n public Basic. File. Writer () Create a Basic. File. Writer attached to standard output. n To write to the terminal screen, we invoke the command: public void display. Line (String line) Write the specified line to the output stream.
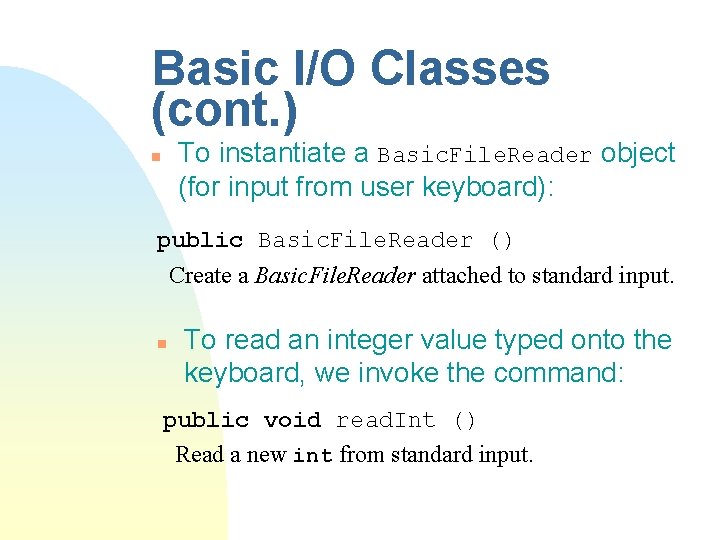
Basic I/O Classes (cont. ) To instantiate a Basic. File. Reader object (for input from user keyboard): n public Basic. File. Reader () Create a Basic. File. Reader attached to standard input. n To read an integer value typed onto the keyboard, we invoke the command: public void read. Int () Read a new int from standard input.
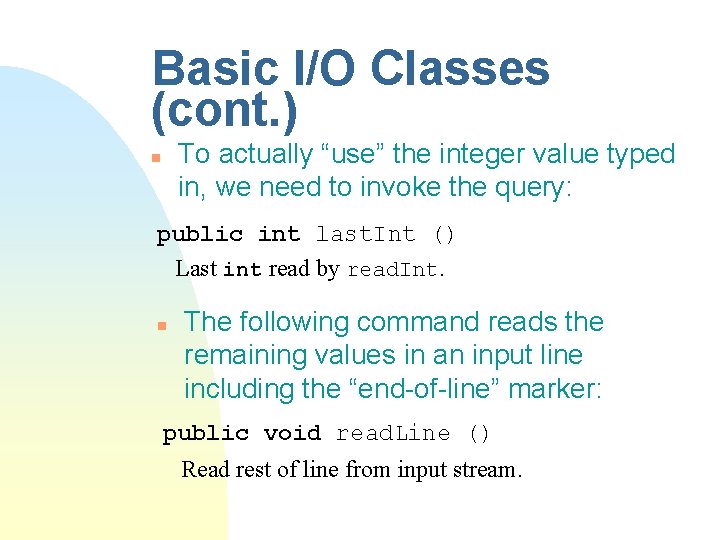
Basic I/O Classes (cont. ) To actually “use” the integer value typed in, we need to invoke the query: n public int last. Int () Last int read by read. Int. n The following command reads the remaining values in an input line including the “end-of-line” marker: public void read. Line () Read rest of line from input stream.
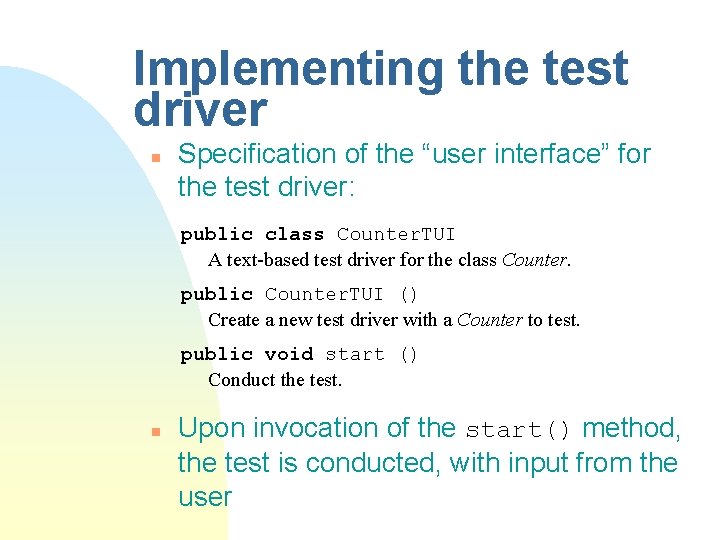
Implementing the test driver n Specification of the “user interface” for the test driver: public class Counter. TUI A text-based test driver for the class Counter. public Counter. TUI () Create a new test driver with a Counter to test. public void start () Conduct the test. n Upon invocation of the start() method, the test is conducted, with input from the user
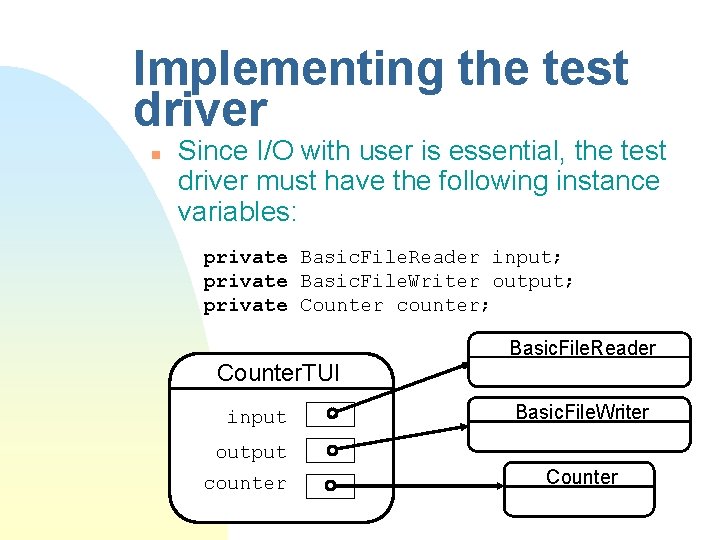
Implementing the test driver n Since I/O with user is essential, the test driver must have the following instance variables: private Basic. File. Reader input; private Basic. File. Writer output; private Counter counter; Basic. File. Reader Counter. TUI input Basic. File. Writer output counter Counter
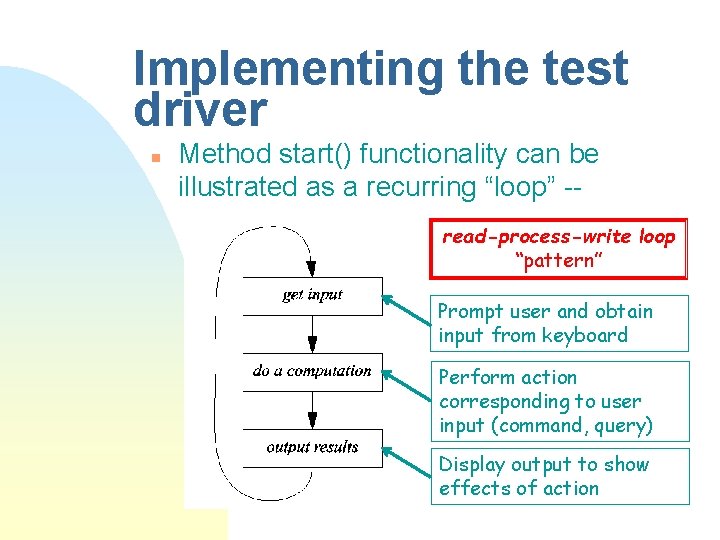
Implementing the test driver n Method start() functionality can be illustrated as a recurring “loop” -read-process-write loop “pattern” Prompt user and obtain input from keyboard Perform action corresponding to user input (command, query) Display output to show effects of action
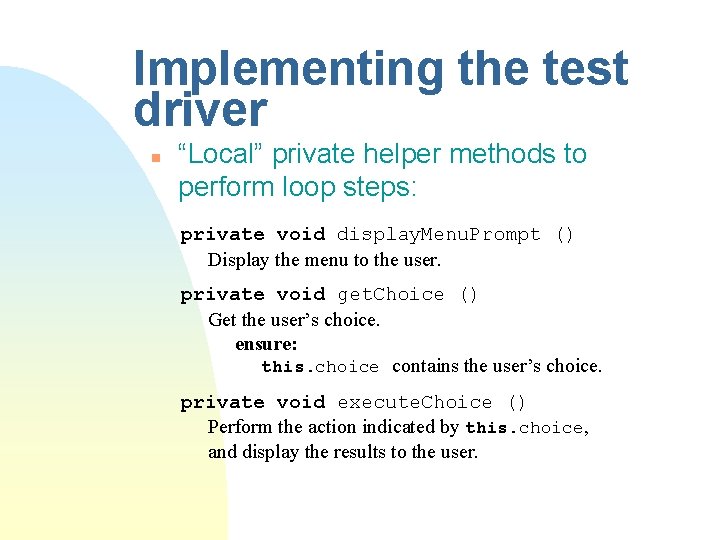
Implementing the test driver n “Local” private helper methods to perform loop steps: private void display. Menu. Prompt () Display the menu to the user. private void get. Choice () Get the user’s choice. ensure: this. choice contains the user’s choice. private void execute. Choice () Perform the action indicated by this. choice, and display the results to the user.
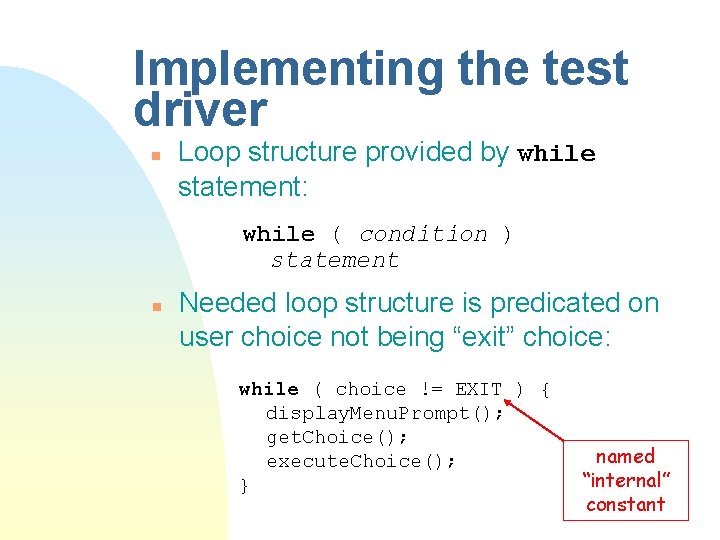
Implementing the test driver n Loop structure provided by while statement: while ( condition ) statement n Needed loop structure is predicated on user choice not being “exit” choice: while ( choice != EXIT ) { display. Menu. Prompt(); get. Choice(); execute. Choice(); } named “internal” constant
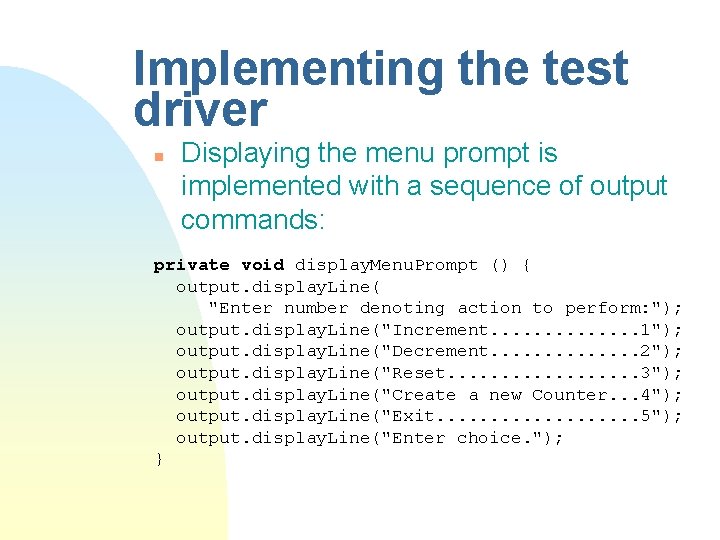
Implementing the test driver n Displaying the menu prompt is implemented with a sequence of output commands: private void display. Menu. Prompt () { output. display. Line( "Enter number denoting action to perform: "); output. display. Line("Increment. . . 1"); output. display. Line("Decrement. . . 2"); output. display. Line("Reset. . . . 3"); output. display. Line("Create a new Counter. . . 4"); output. display. Line("Exit. . . . . 5"); output. display. Line("Enter choice. "); }
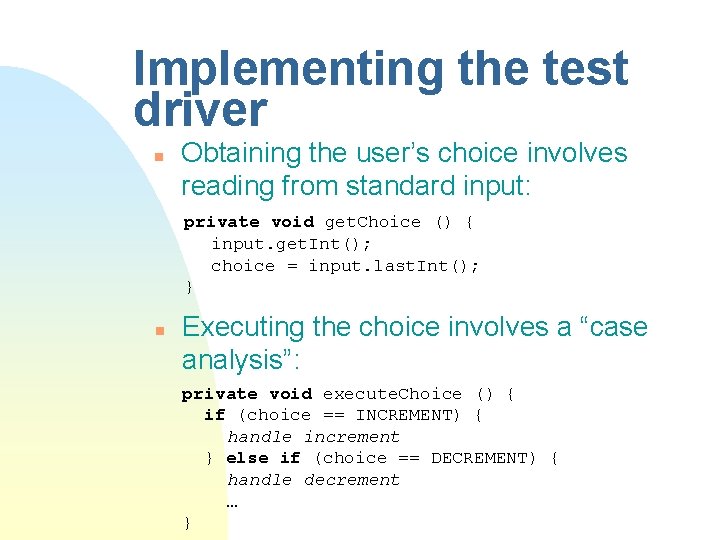
Implementing the test driver n Obtaining the user’s choice involves reading from standard input: private void get. Choice () { input. get. Int(); choice = input. last. Int(); } n Executing the choice involves a “case analysis”: private void execute. Choice () { if (choice == INCREMENT) { handle increment } else if (choice == DECREMENT) { handle decrement … }
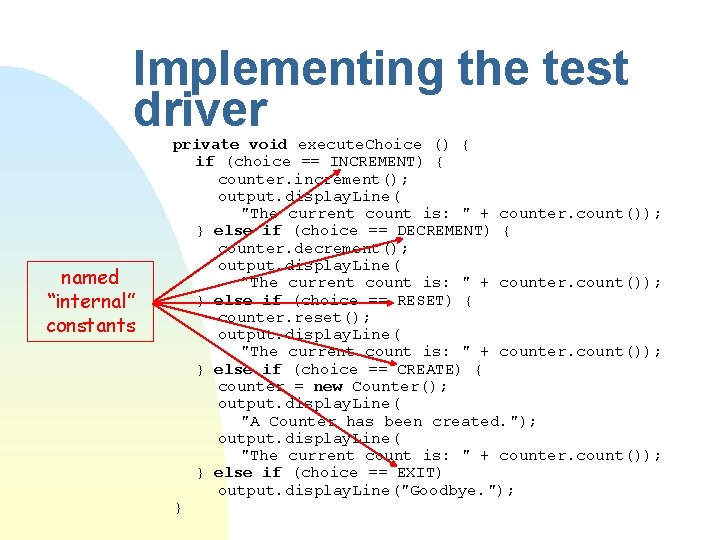
Implementing the test driver named “internal” constants private void execute. Choice () { if (choice == INCREMENT) { counter. increment(); output. display. Line( "The current count is: " + counter. count()); } else if (choice == DECREMENT) { counter. decrement(); output. display. Line( "The current count is: " + counter. count()); } else if (choice == RESET) { counter. reset(); output. display. Line( "The current count is: " + counter. count()); } else if (choice == CREATE) { counter = new Counter(); output. display. Line( "A Counter has been created. "); output. display. Line( "The current count is: " + counter. count()); } else if (choice == EXIT) output. display. Line("Goodbye. "); }
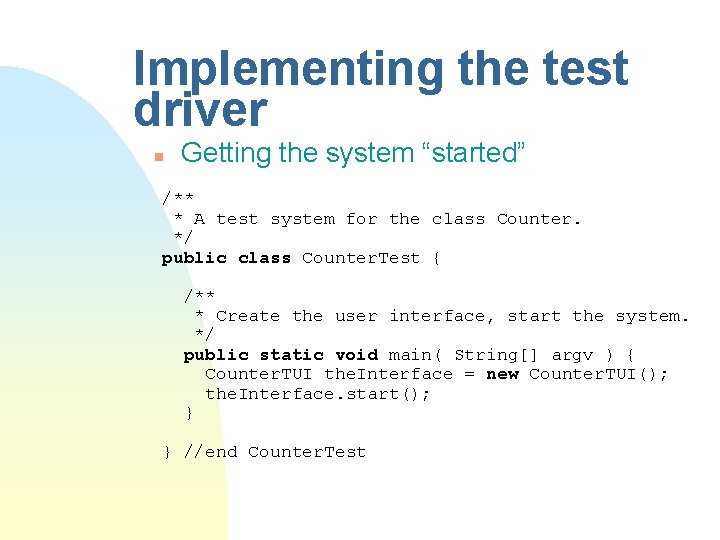
Implementing the test driver n Getting the system “started” /** * A test system for the class Counter. */ public class Counter. Test { /** * Create the user interface, start the system. */ public static void main( String[] argv ) { Counter. TUI the. Interface = new Counter. TUI(); the. Interface. start(); } } //end Counter. Test
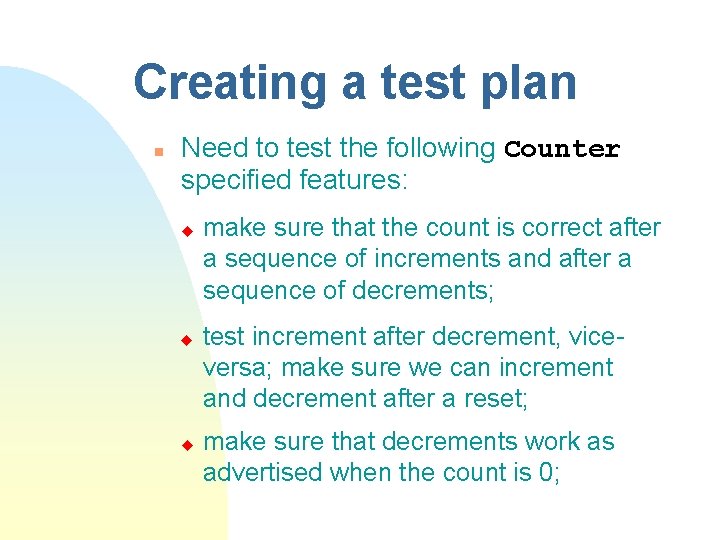
Creating a test plan n Need to test the following Counter specified features: u u u make sure that the count is correct after a sequence of increments and after a sequence of decrements; test increment after decrement, viceversa; make sure we can increment and decrement after a reset; make sure that decrements work as advertised when the count is 0;
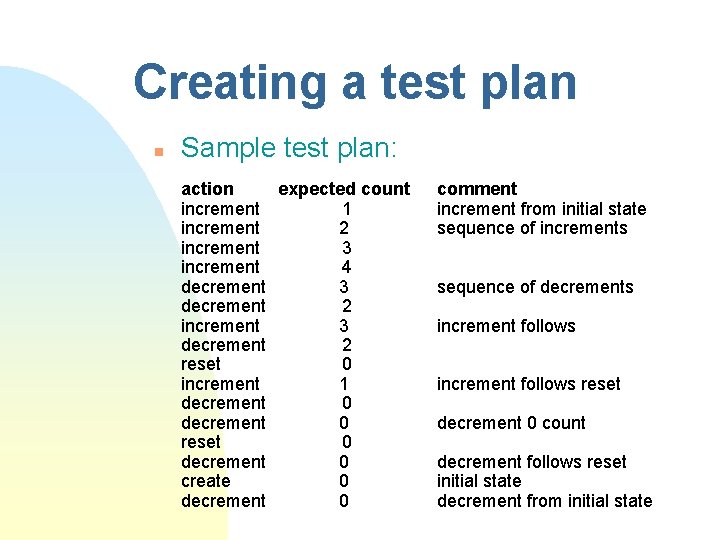
Creating a test plan n Sample test plan: action expected count increment 1 increment 2 increment 3 increment 4 decrement 3 decrement 2 increment 3 decrement 2 reset 0 increment 1 decrement 0 reset 0 decrement 0 create 0 decrement 0 comment increment from initial state sequence of increments sequence of decrements increment follows reset decrement 0 count decrement follows reset initial state decrement from initial state
This chapter discusses
Surface weather
Homosphere
This chapter discusses
The paragraph preceding the passage most probably discusses
The paragraph preceding the passage most probably discusses
Essay discussing advantages and disadvantages
Receiving equipment
Domain testing in software testing
Motivational overview of logic based testing
Data flow testing strategies in software testing
Positive testing and negative testing
Cs3250
Globalization testing example
Functional testing vs unit testing
Language testing
Control structure testing in software testing
Decision table testing in software testing
Decision table testing example
Pengertian blackbox testing
Behavior testing adalah
Decision table testing is white box
Rigorous testing in software testing
Testing blindness in software testing
Component testing is a black box testing
Software domain examples
Special value testing
Weak robust equivalence class testing example
Equivalence partitioning