Chapter 8 Sorting Sorting Ordering or Sequencing Since
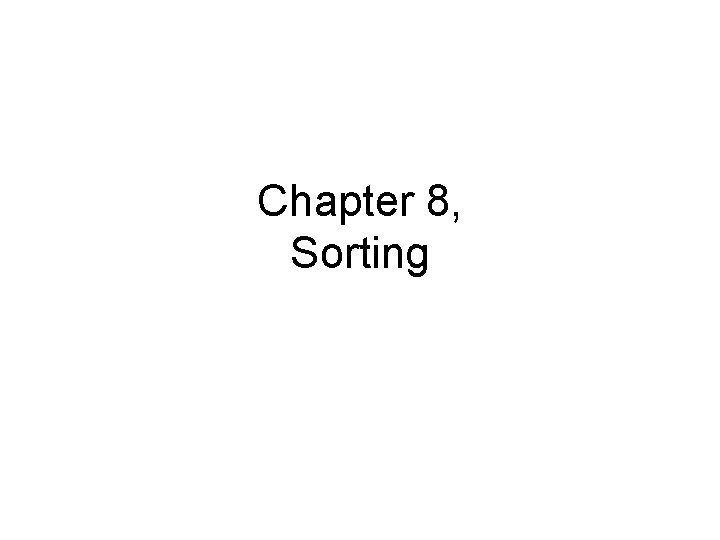
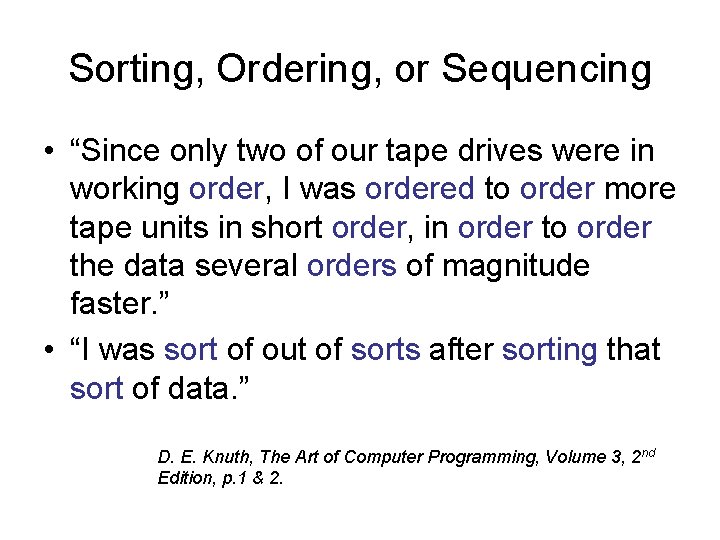
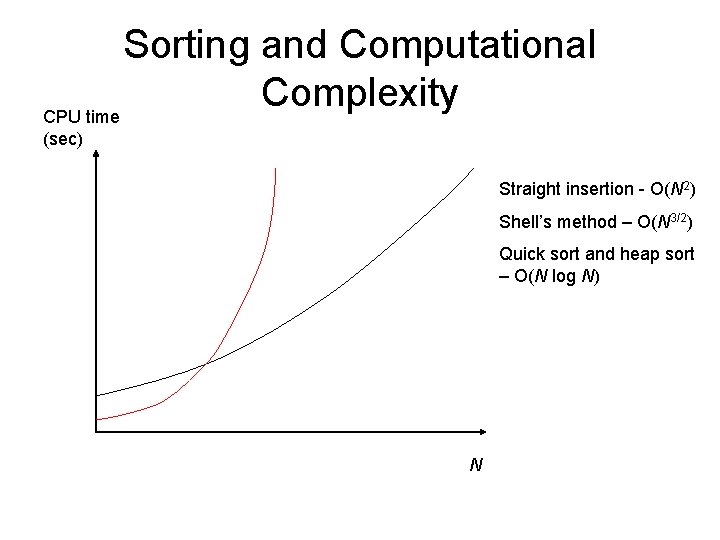
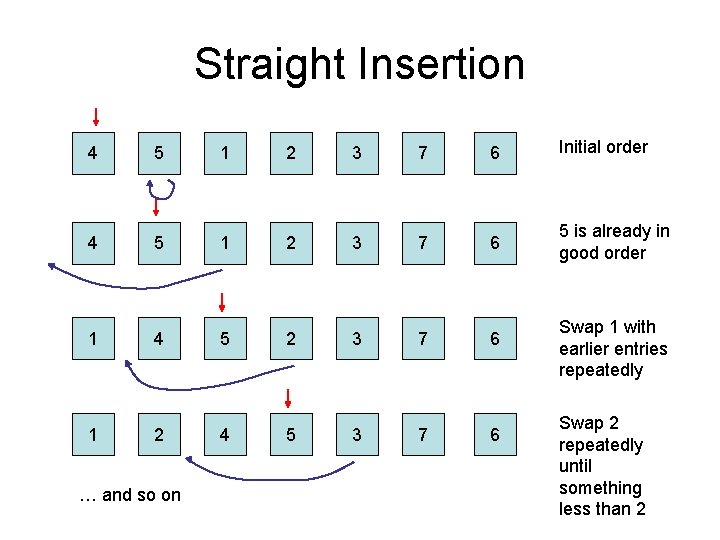
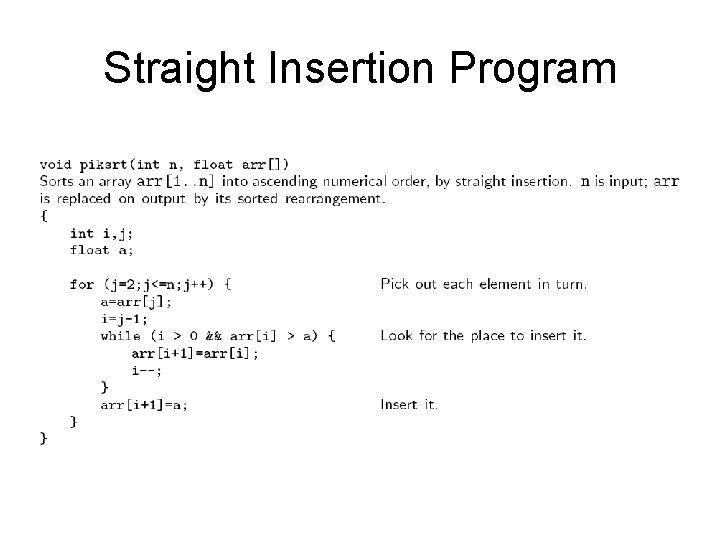
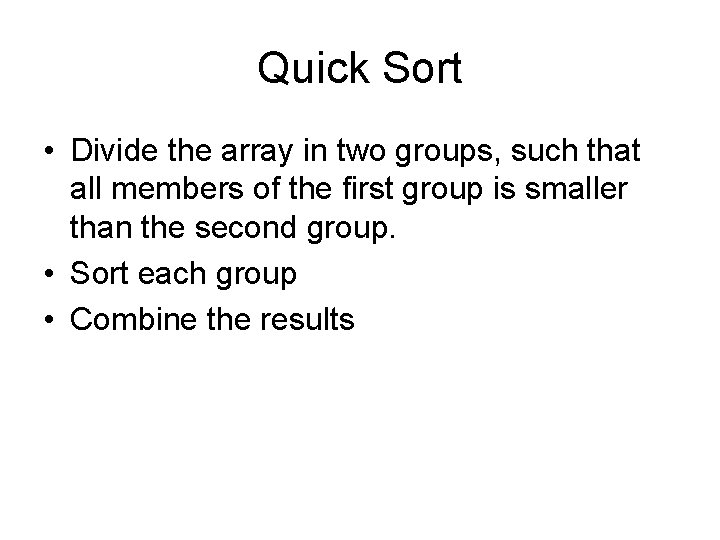
![Quick Sort Pseudo-Code Quicksort(A[ ], p, r) { if (p < r) { then Quick Sort Pseudo-Code Quicksort(A[ ], p, r) { if (p < r) { then](https://slidetodoc.com/presentation_image/5a552859e0eb60f887a08bf1c85a1155/image-7.jpg)
![Pseudo-Code Continued Partition(A[ ], p, r) { x = A[p]; i = p – Pseudo-Code Continued Partition(A[ ], p, r) { x = A[p]; i = p –](https://slidetodoc.com/presentation_image/5a552859e0eb60f887a08bf1c85a1155/image-8.jpg)
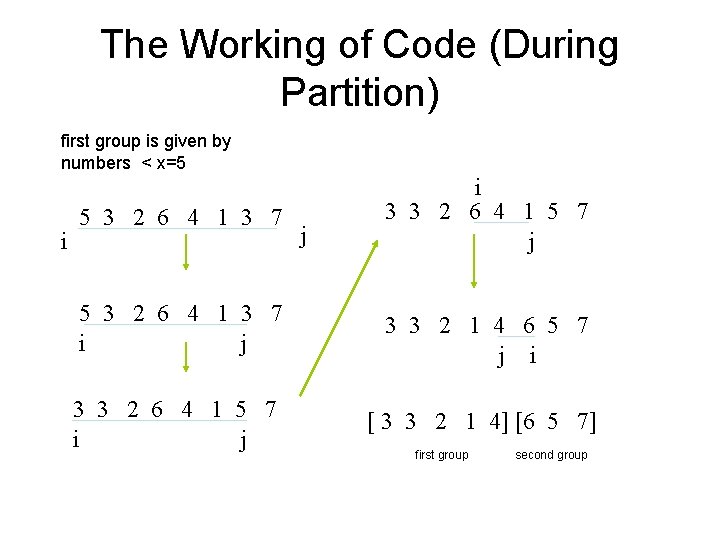
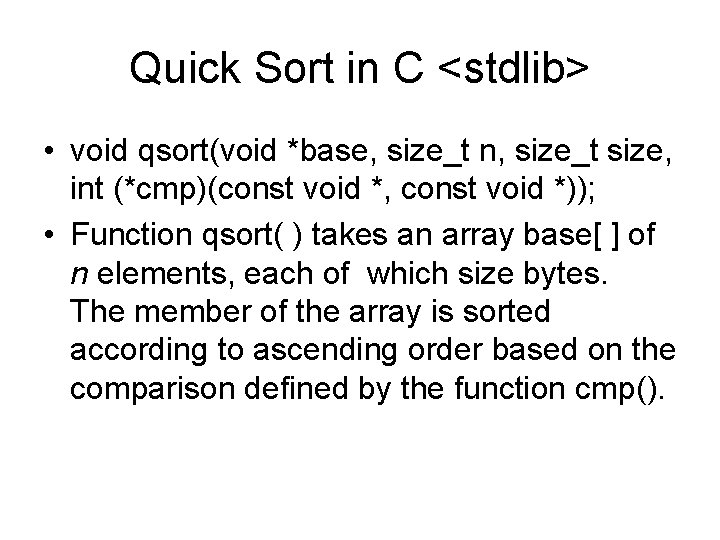
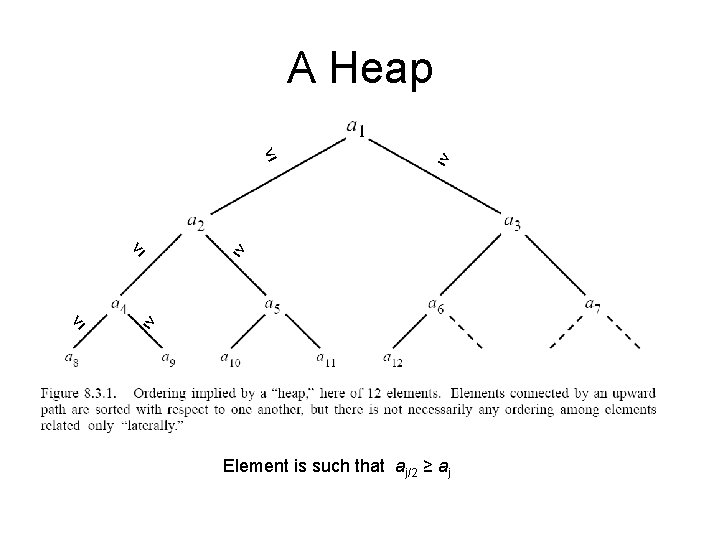
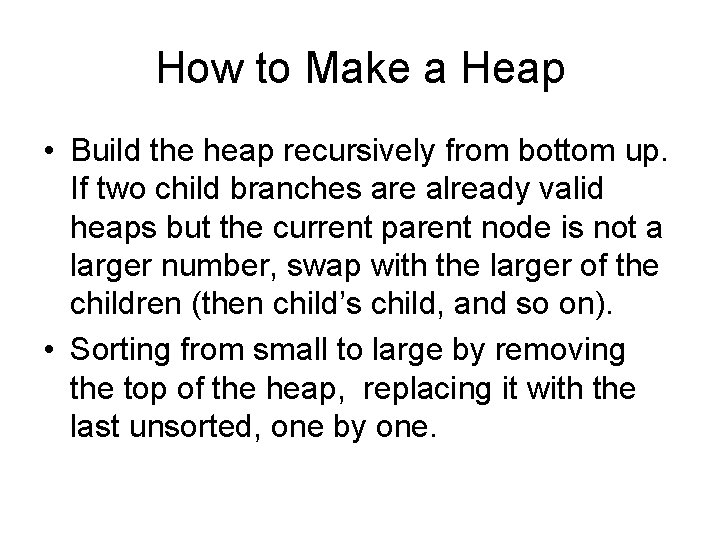
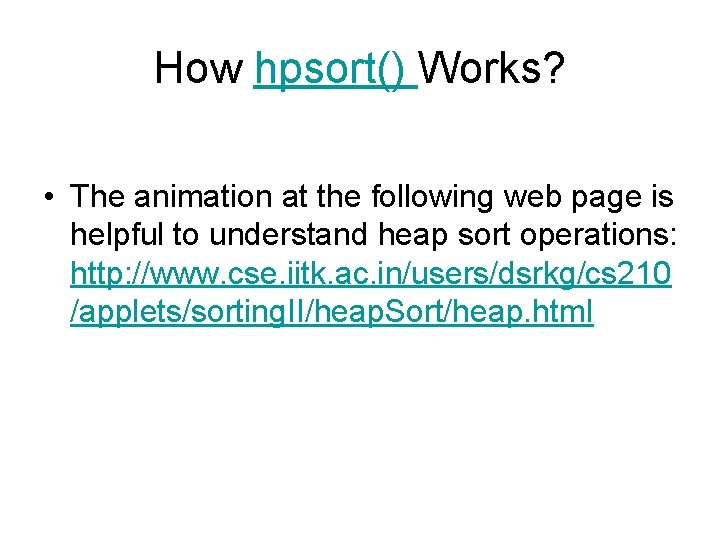
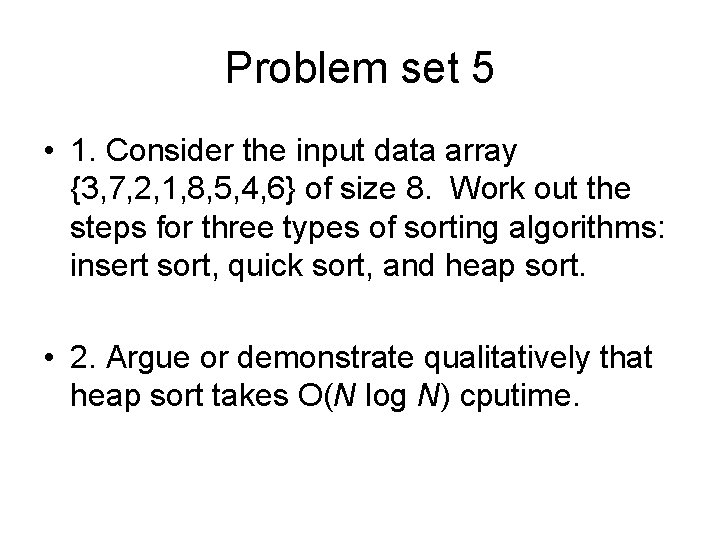
- Slides: 14
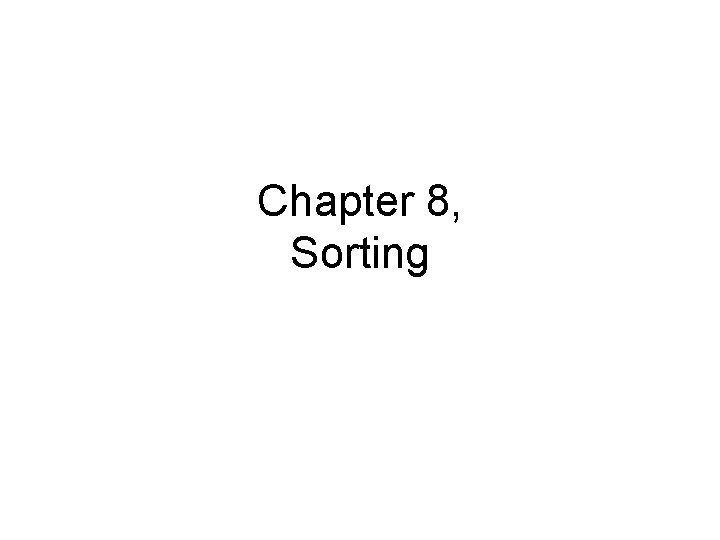
Chapter 8, Sorting
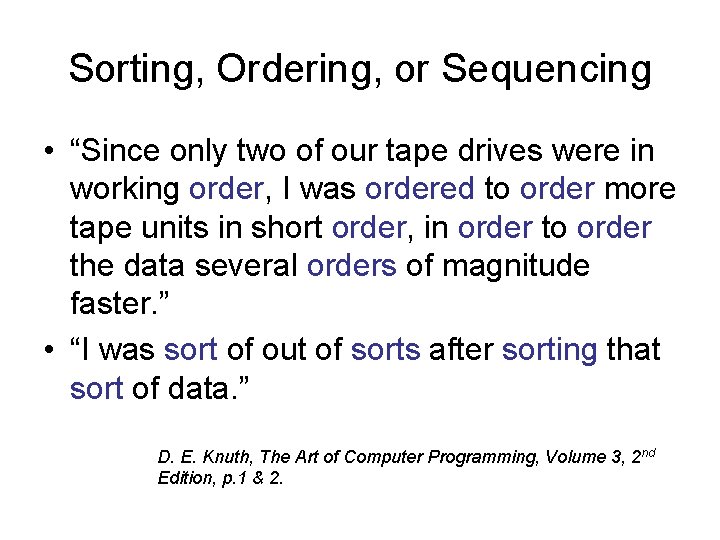
Sorting, Ordering, or Sequencing • “Since only two of our tape drives were in working order, I was ordered to order more tape units in short order, in order to order the data several orders of magnitude faster. ” • “I was sort of out of sorts after sorting that sort of data. ” D. E. Knuth, The Art of Computer Programming, Volume 3, 2 nd Edition, p. 1 & 2.
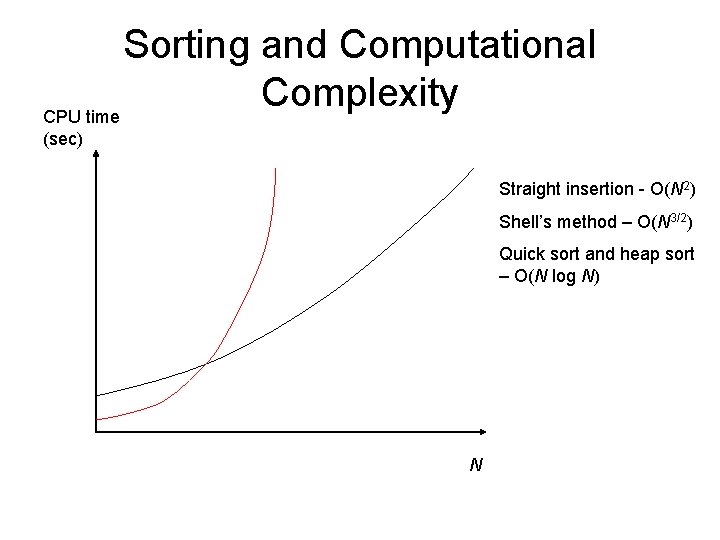
Sorting and Computational Complexity CPU time (sec) Straight insertion - O(N 2) Shell’s method – O(N 3/2) Quick sort and heap sort – O(N log N) N
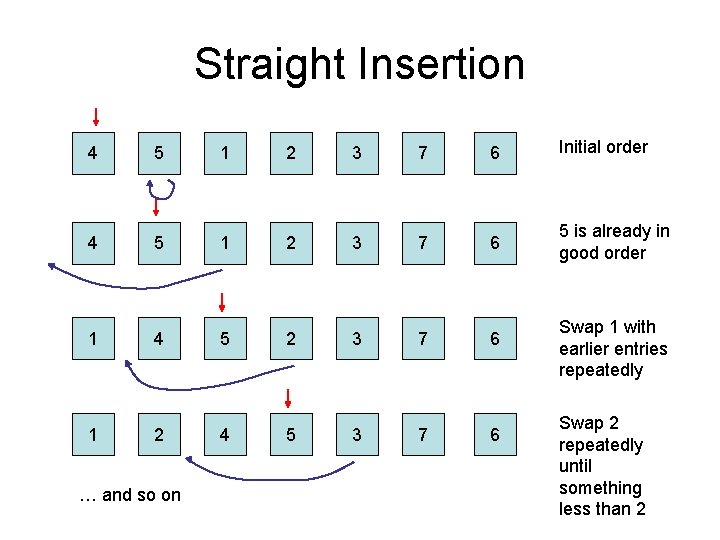
Straight Insertion 4 5 1 2 3 7 6 Initial order 4 5 1 2 3 7 6 5 is already in good order 1 4 5 2 3 7 6 1 2 4 5 3 7 6 … and so on Swap 1 with earlier entries repeatedly Swap 2 repeatedly until something less than 2
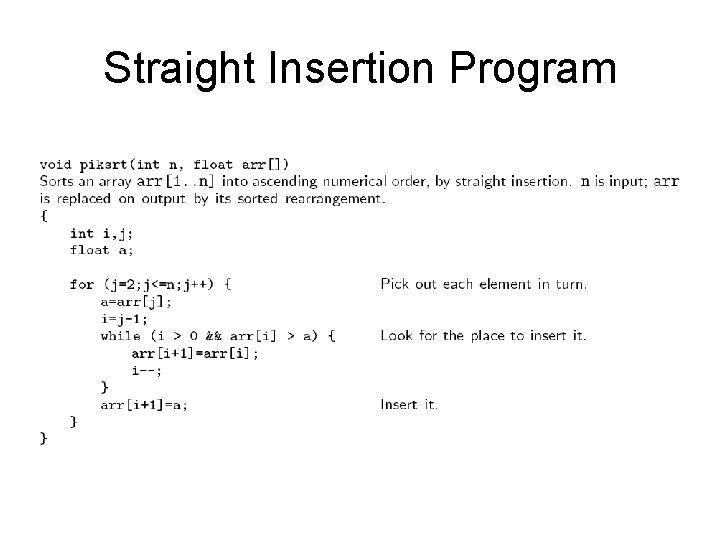
Straight Insertion Program
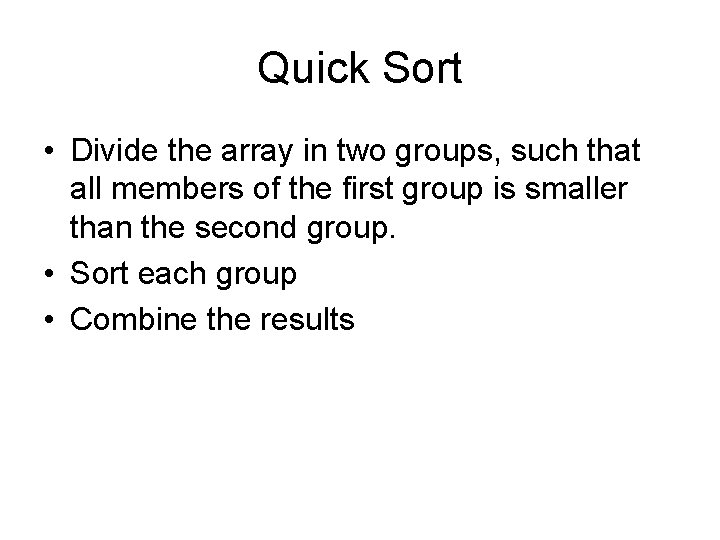
Quick Sort • Divide the array in two groups, such that all members of the first group is smaller than the second group. • Sort each group • Combine the results
![Quick Sort PseudoCode QuicksortA p r if p r then Quick Sort Pseudo-Code Quicksort(A[ ], p, r) { if (p < r) { then](https://slidetodoc.com/presentation_image/5a552859e0eb60f887a08bf1c85a1155/image-7.jpg)
Quick Sort Pseudo-Code Quicksort(A[ ], p, r) { if (p < r) { then q = Partition(A, p, r); Quicksort(A, p, q); Quicksort(A, q+1, r); } } // sorting A[j] for p ≤ j ≤ r // split the data in two groups
![PseudoCode Continued PartitionA p r x Ap i p Pseudo-Code Continued Partition(A[ ], p, r) { x = A[p]; i = p –](https://slidetodoc.com/presentation_image/5a552859e0eb60f887a08bf1c85a1155/image-8.jpg)
Pseudo-Code Continued Partition(A[ ], p, r) { x = A[p]; i = p – 1; j = r + 1; while(1) { do { --j; } while(A[j] > x); do { ++i; } while (A[i] < x); if(i<j) { swap(i, j); } else { return j; } }
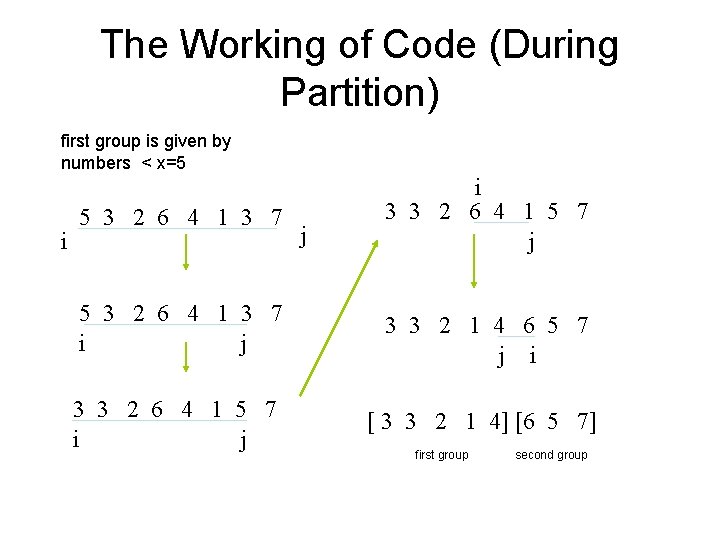
The Working of Code (During Partition) first group is given by numbers < x=5 i 5 3 2 6 4 1 3 7 j i 3 3 2 6 4 1 5 7 j 5 3 2 6 4 1 3 7 i j 3 3 2 1 4 6 5 7 j i 3 3 2 6 4 1 5 7 i j [ 3 3 2 1 4] [6 5 7] first group second group
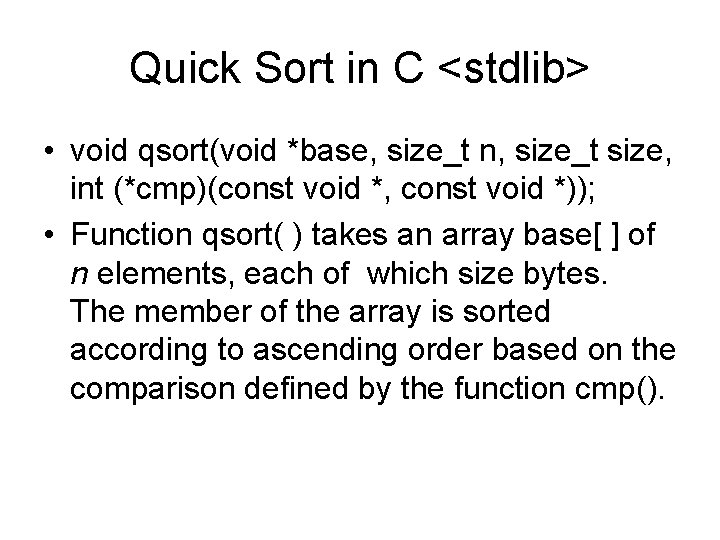
Quick Sort in C <stdlib> • void qsort(void *base, size_t n, size_t size, int (*cmp)(const void *, const void *)); • Function qsort( ) takes an array base[ ] of n elements, each of which size bytes. The member of the array is sorted according to ascending order based on the comparison defined by the function cmp().
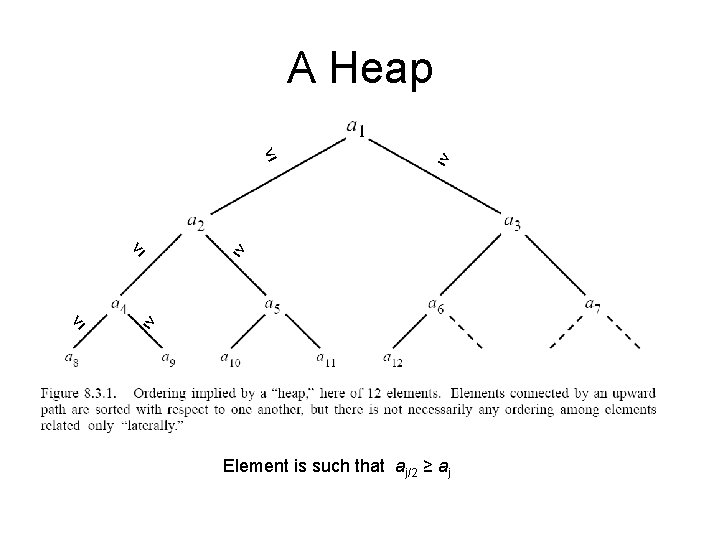
A Heap ≤ ≤ ≤ ≥ ≥ ≥ Element is such that aj/2 ≥ aj
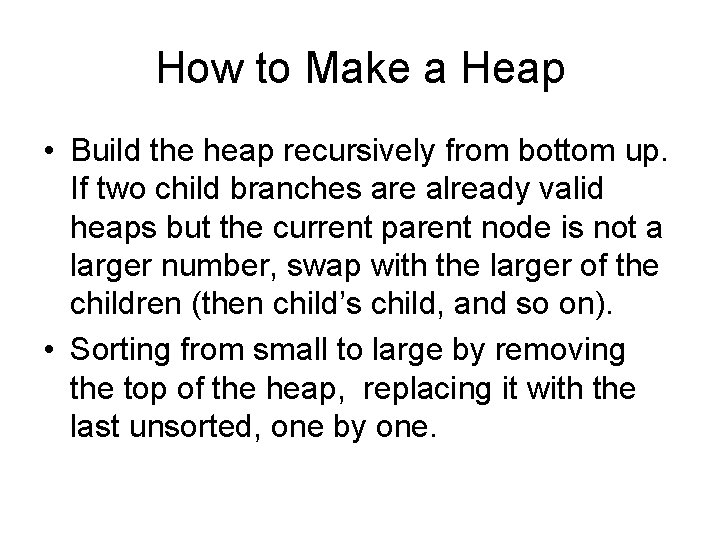
How to Make a Heap • Build the heap recursively from bottom up. If two child branches are already valid heaps but the current parent node is not a larger number, swap with the larger of the children (then child’s child, and so on). • Sorting from small to large by removing the top of the heap, replacing it with the last unsorted, one by one.
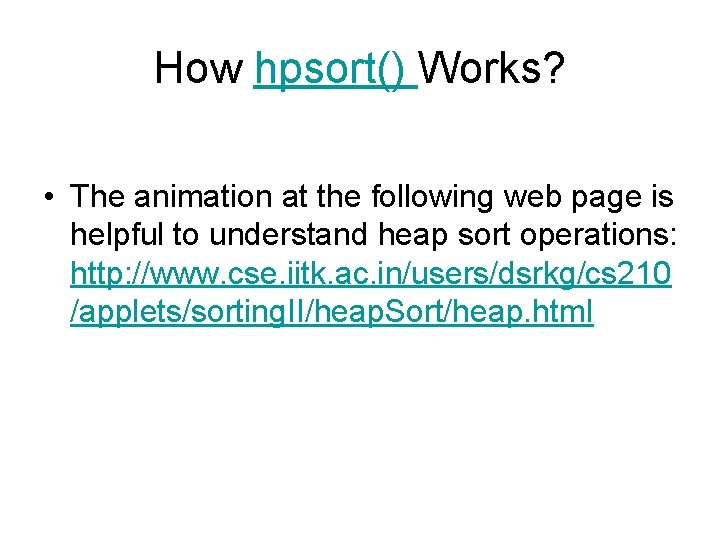
How hpsort() Works? • The animation at the following web page is helpful to understand heap sort operations: http: //www. cse. iitk. ac. in/users/dsrkg/cs 210 /applets/sorting. II/heap. Sort/heap. html
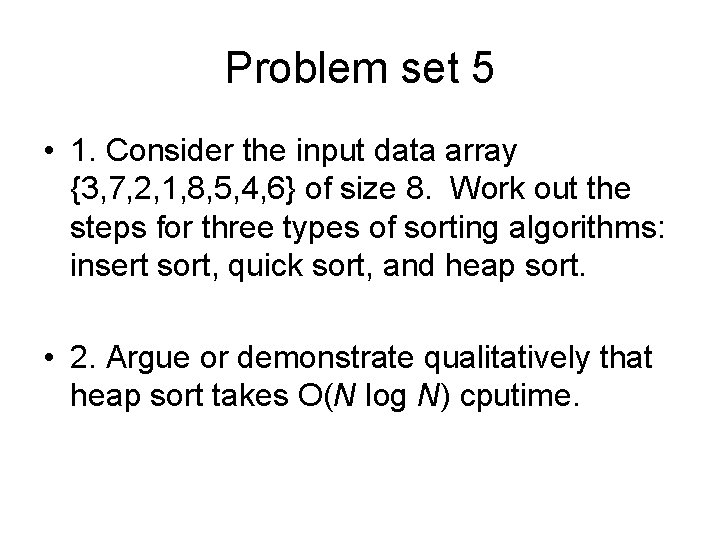
Problem set 5 • 1. Consider the input data array {3, 7, 2, 1, 8, 5, 4, 6} of size 8. Work out the steps for three types of sorting algorithms: insert sort, quick sort, and heap sort. • 2. Argue or demonstrate qualitatively that heap sort takes O(N log N) cputime.