Chapter 8 Advanced Swing MVC Originals of Slides
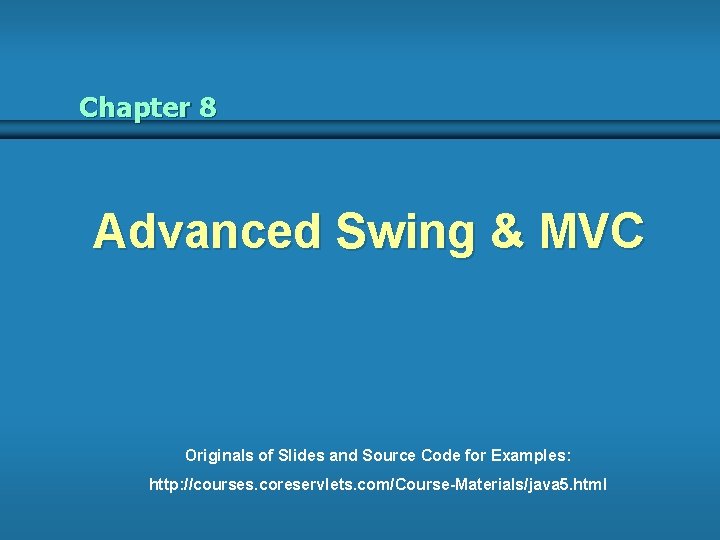
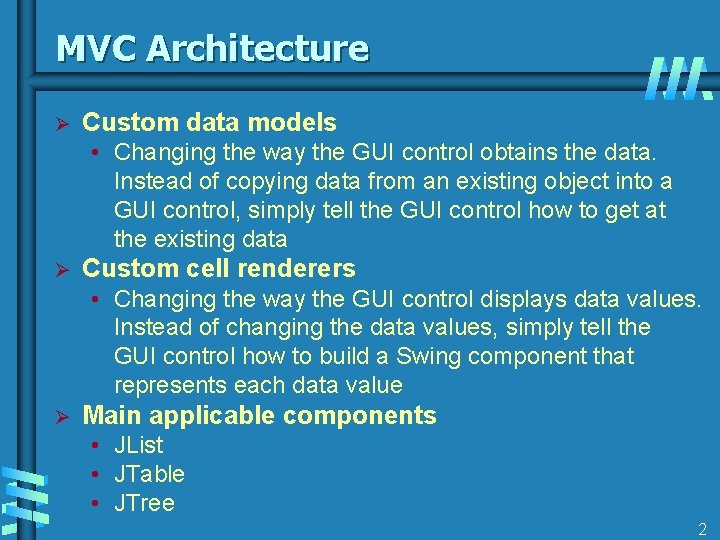
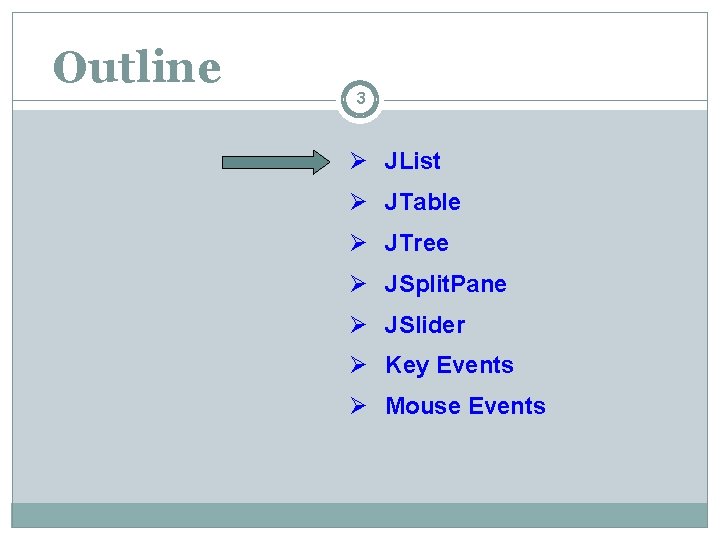
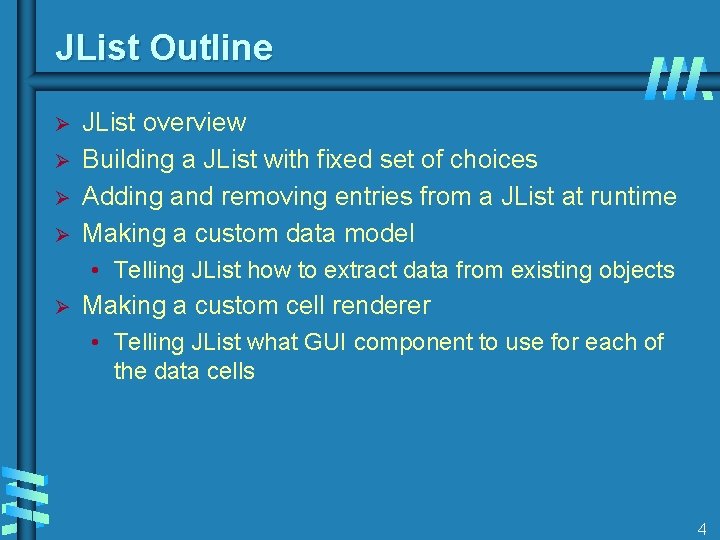
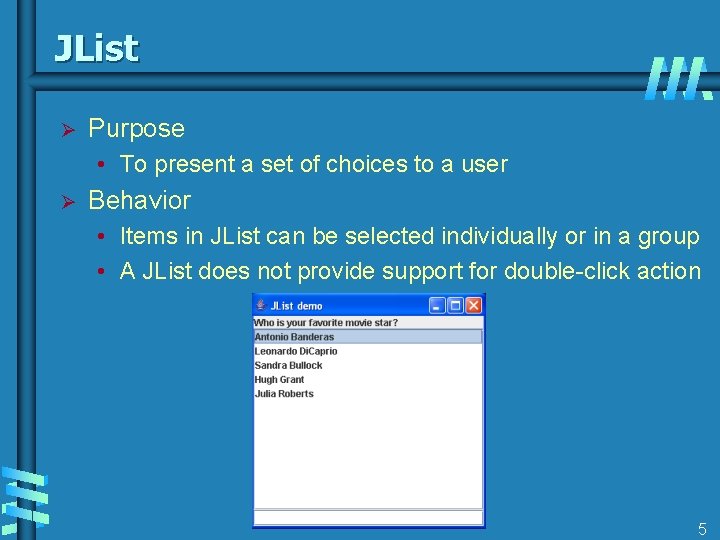
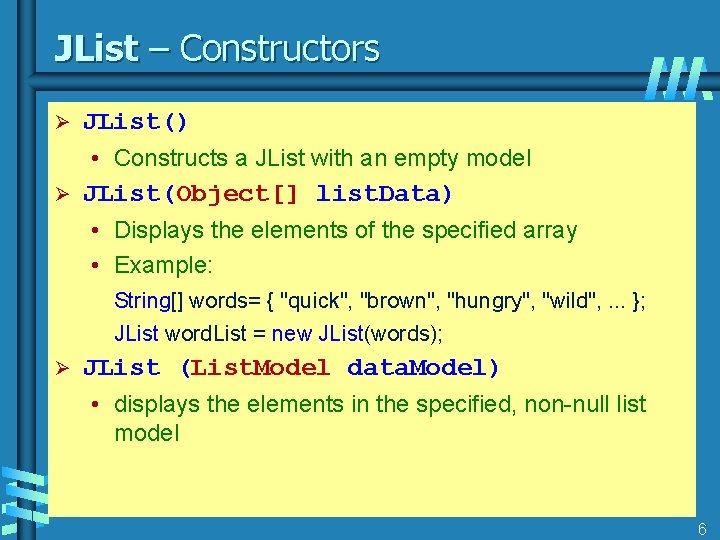
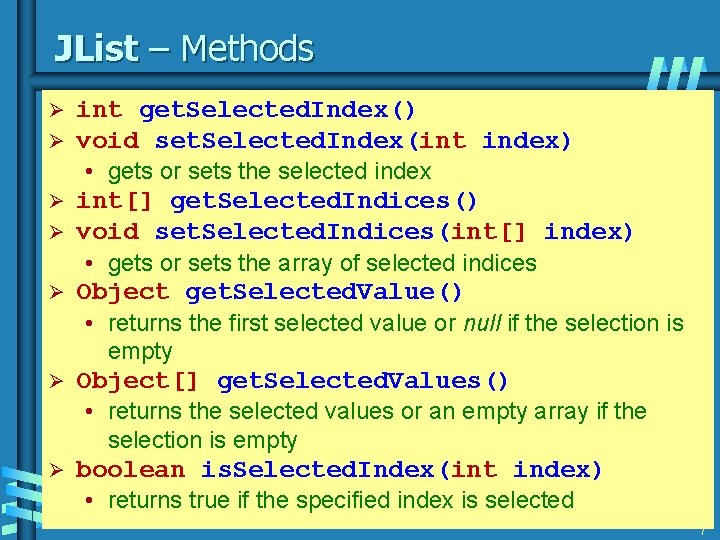
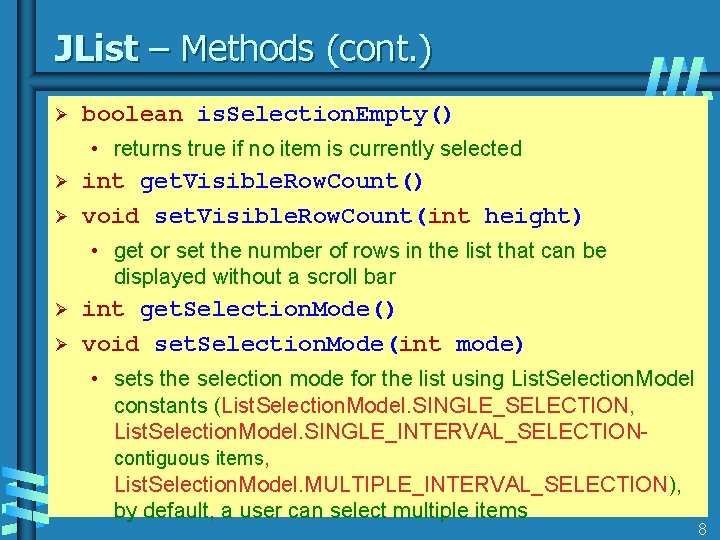
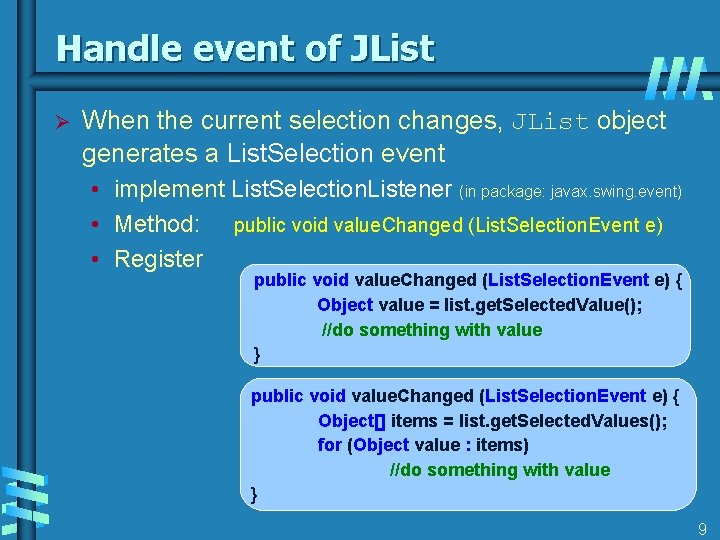
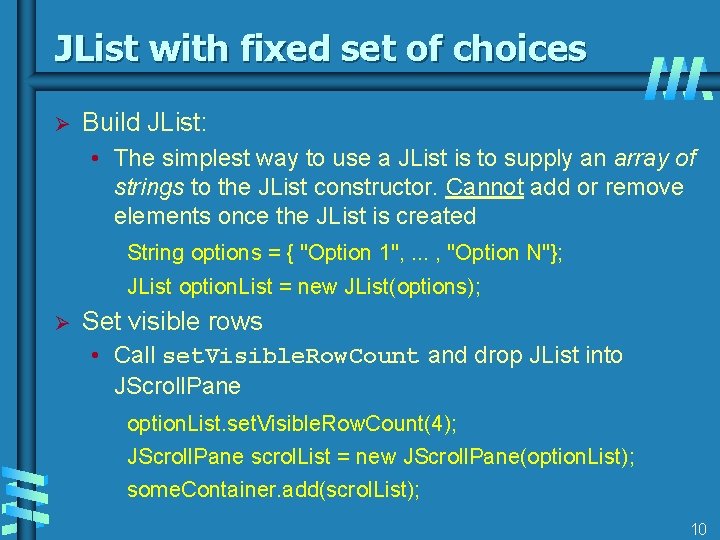
![Example: JList. Simple. Demo. java String[] entries = { "Entry 1", "Entry 2", "Entry Example: JList. Simple. Demo. java String[] entries = { "Entry 1", "Entry 2", "Entry](https://slidetodoc.com/presentation_image_h2/f4808711ed7de67909459c043a9af1c7/image-11.jpg)
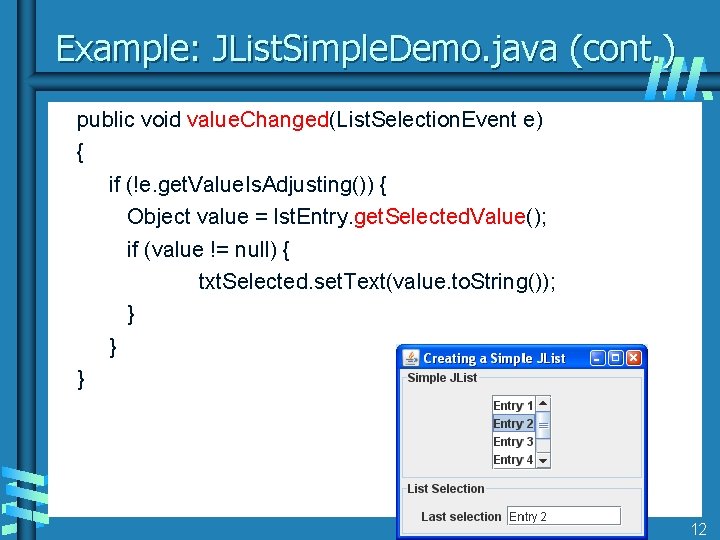
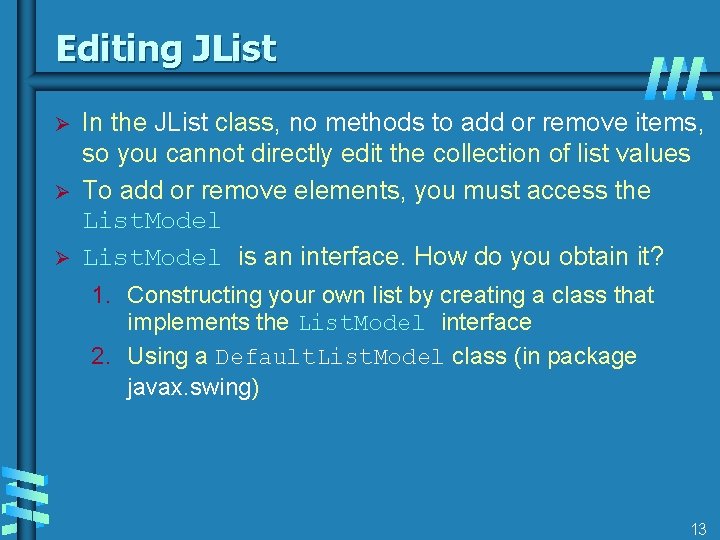
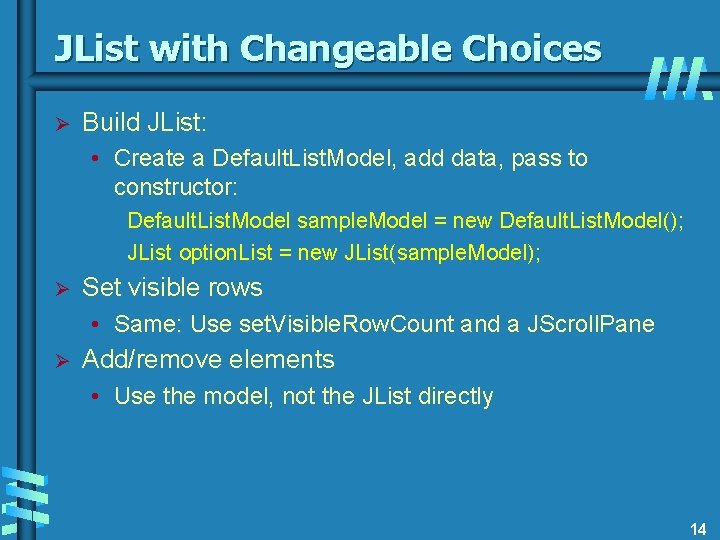
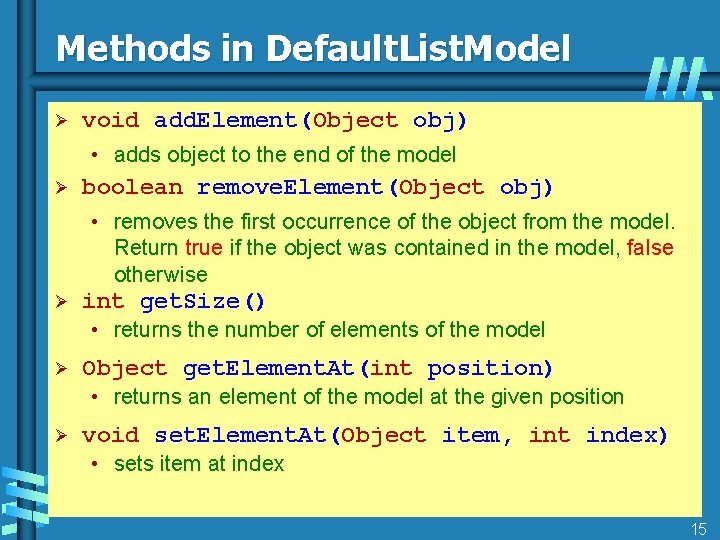
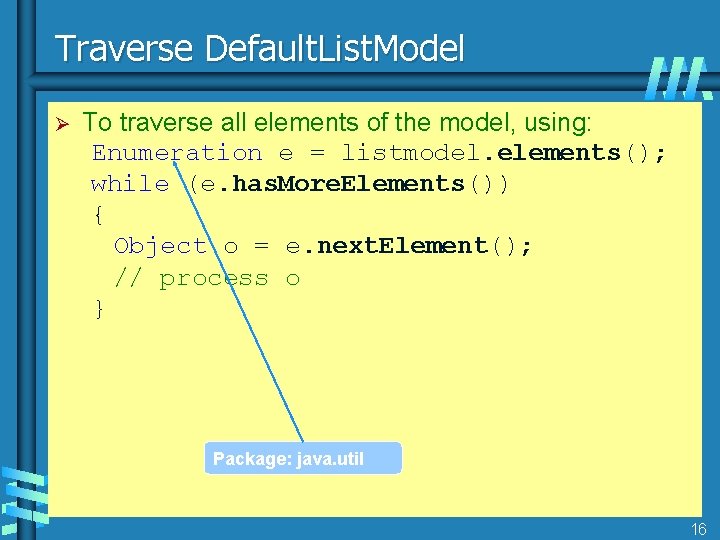
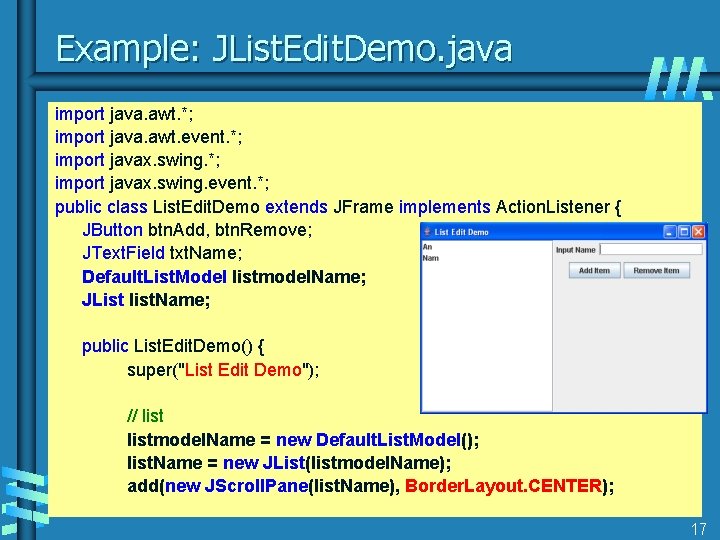
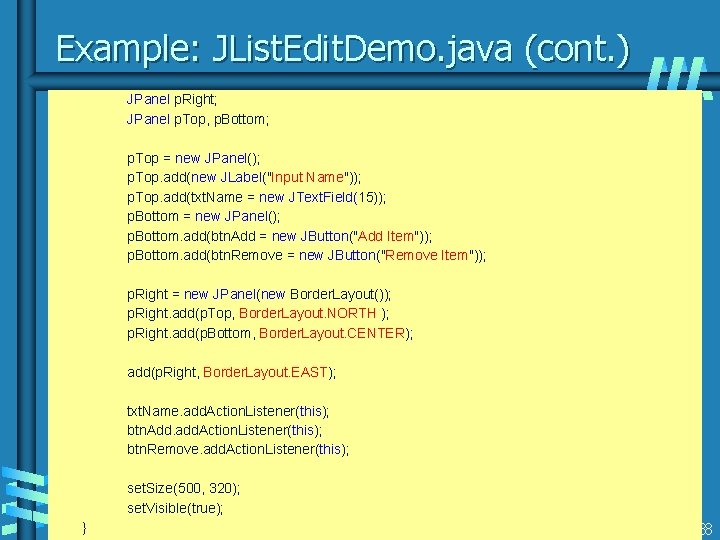
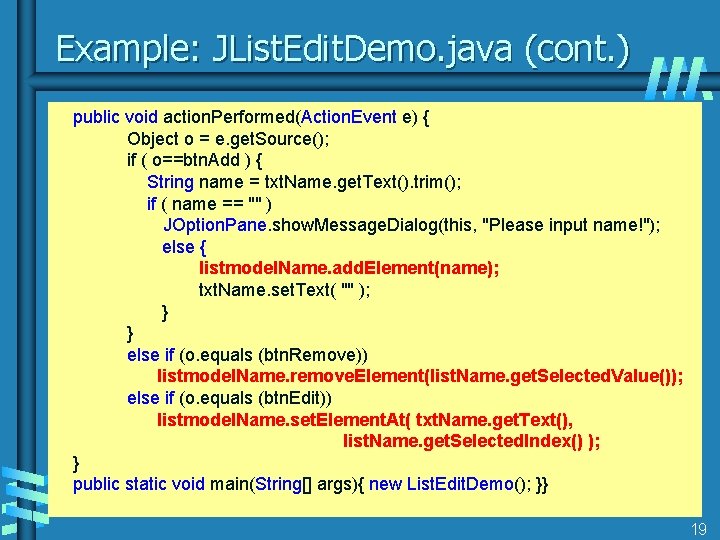
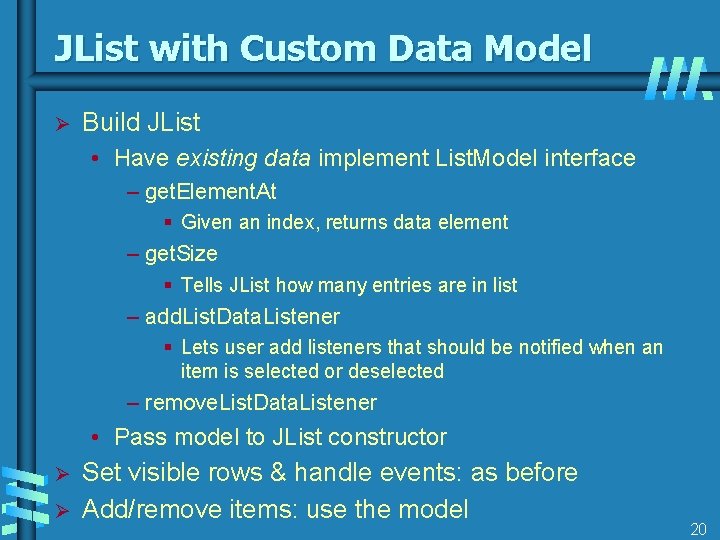
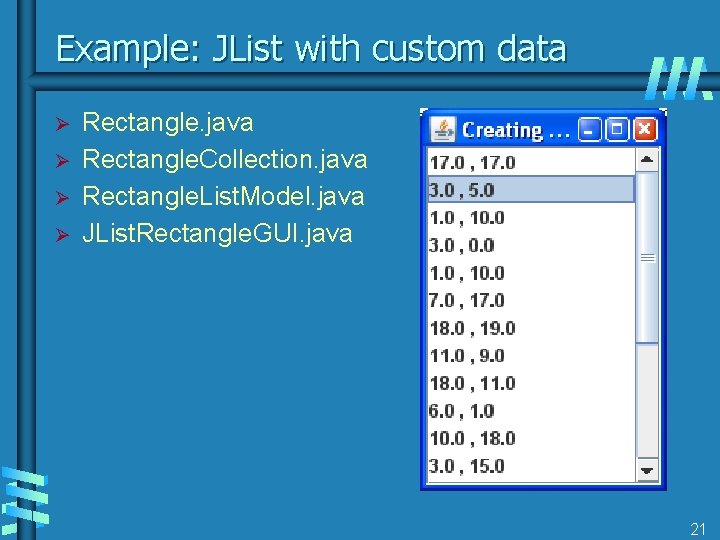
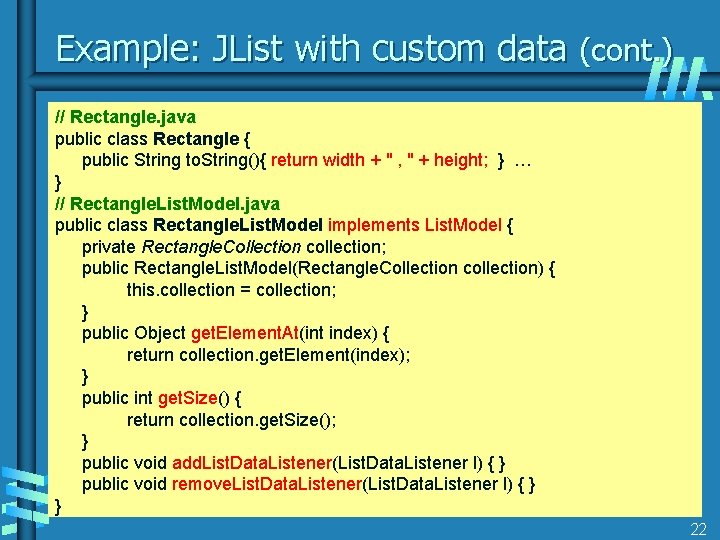
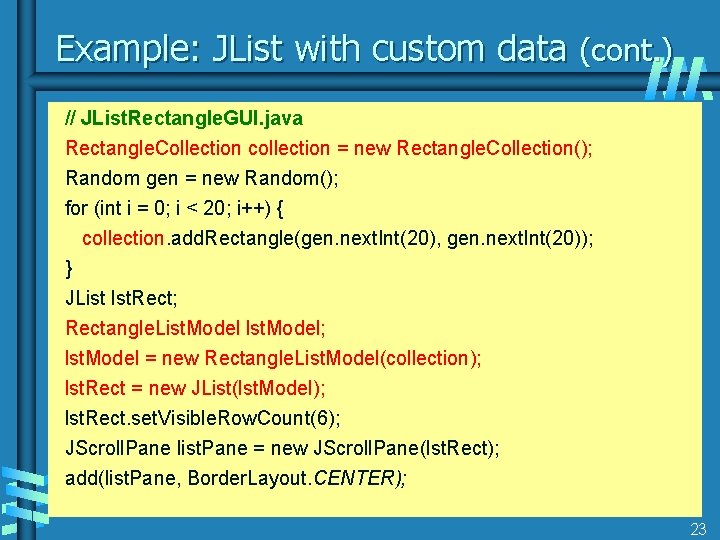
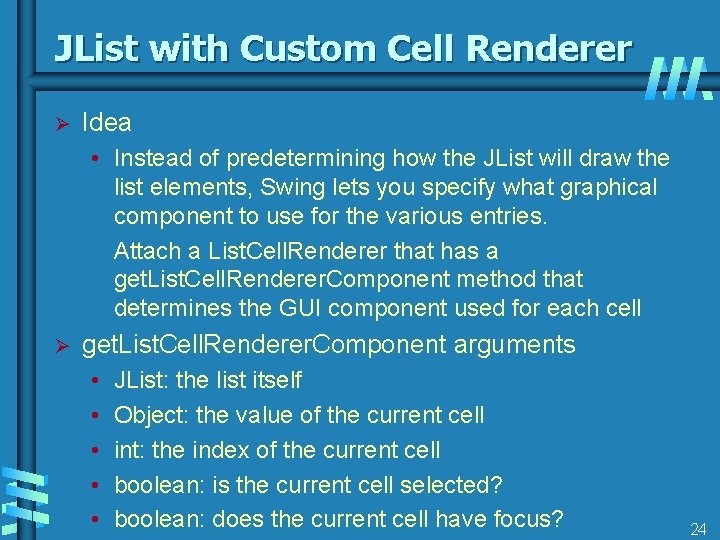
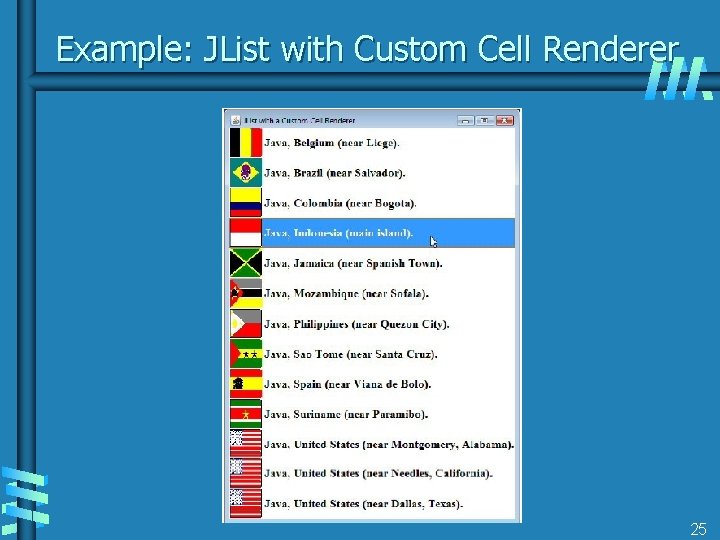
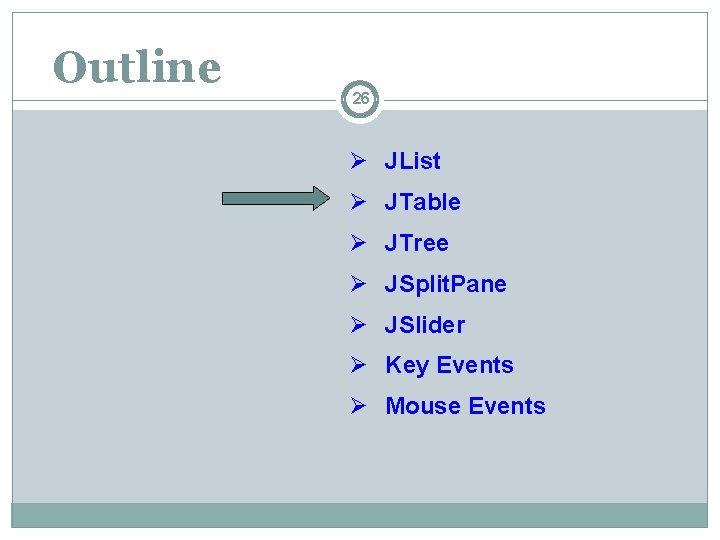
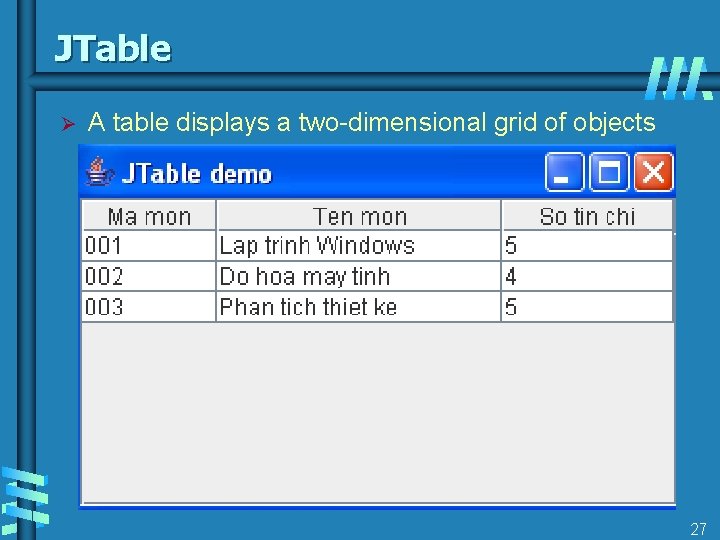
![Constructors - Methods of JTable Ø JTable(Object[][] entries, Object[] column. Names) • constructs a Constructors - Methods of JTable Ø JTable(Object[][] entries, Object[] column. Names) • constructs a](https://slidetodoc.com/presentation_image_h2/f4808711ed7de67909459c043a9af1c7/image-28.jpg)
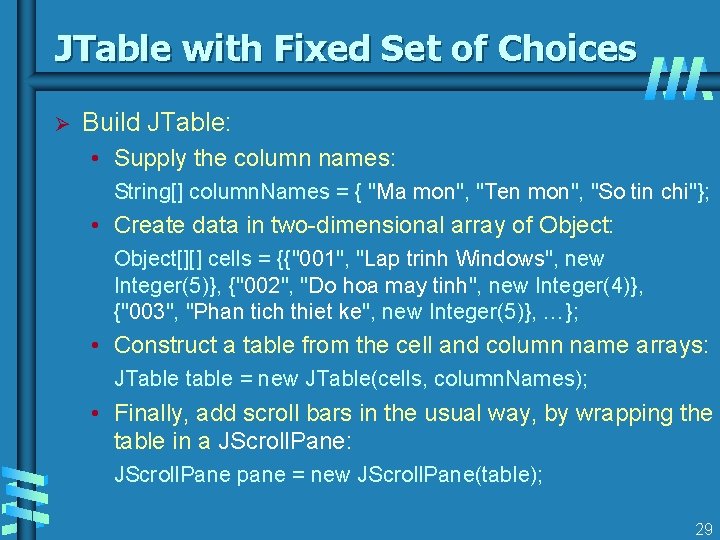
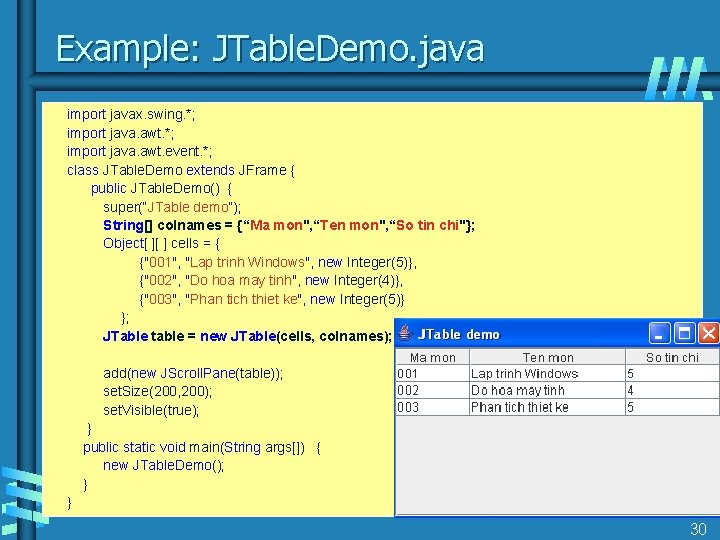
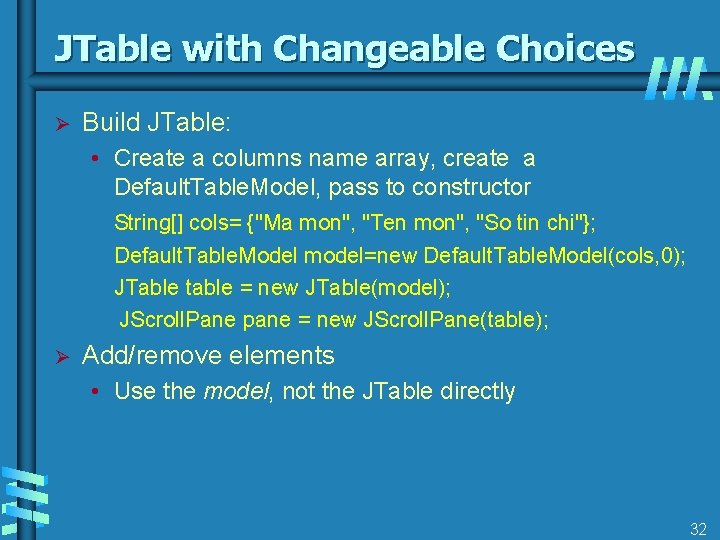
![Methods in Default. Table. Model Ø void add. Row(Object[] row. Data) • add a Methods in Default. Table. Model Ø void add. Row(Object[] row. Data) • add a](https://slidetodoc.com/presentation_image_h2/f4808711ed7de67909459c043a9af1c7/image-32.jpg)
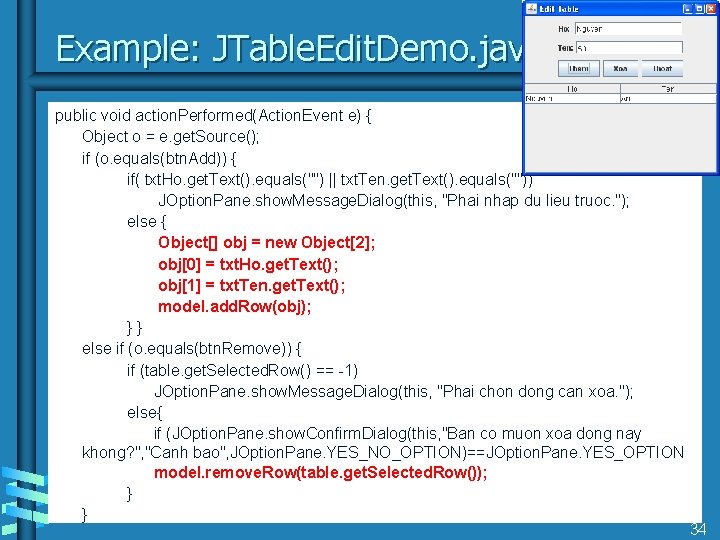
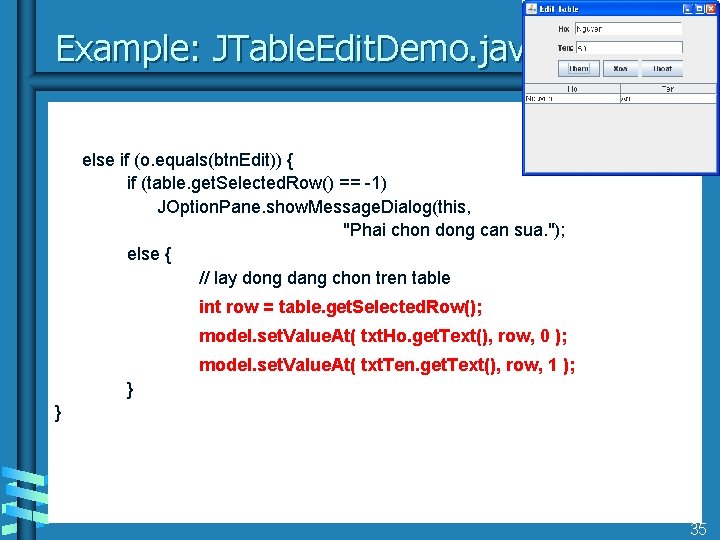
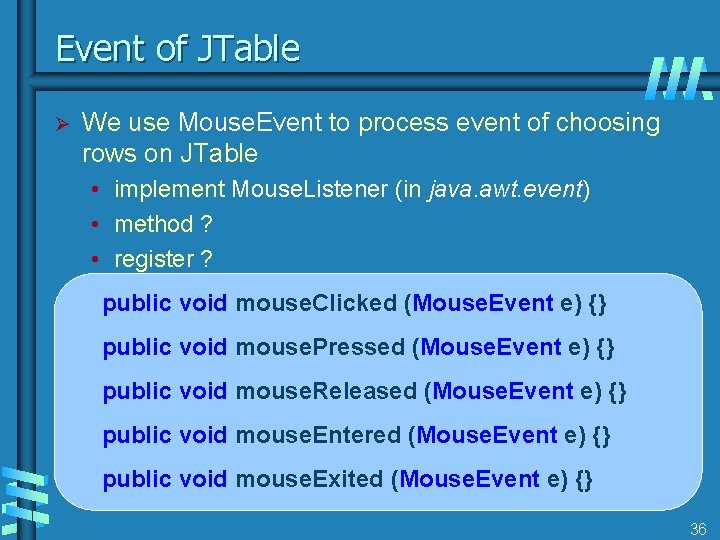
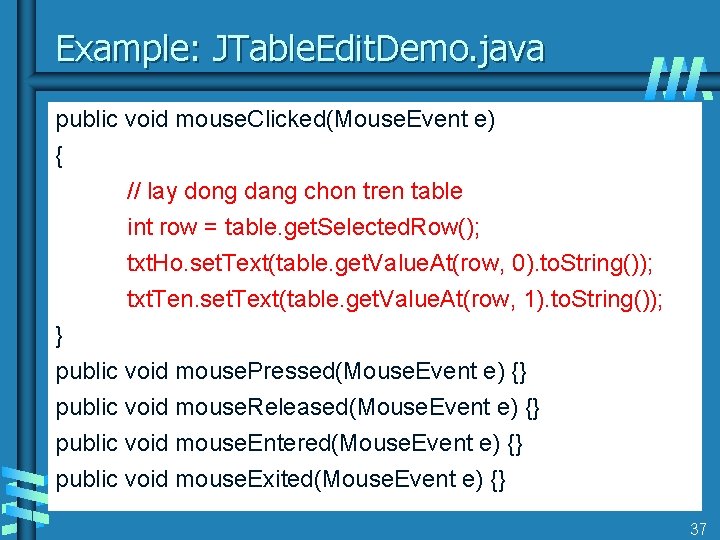
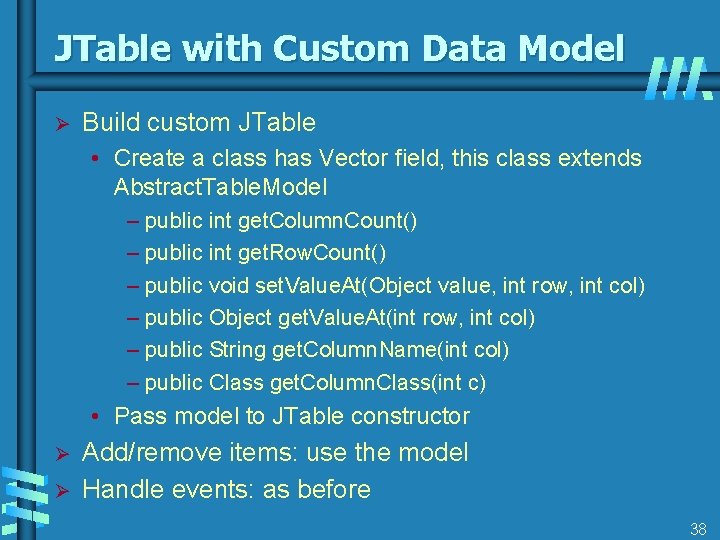
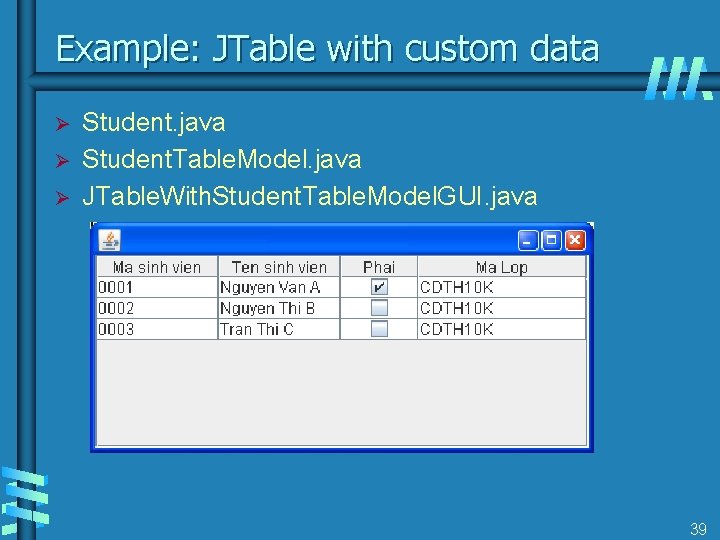
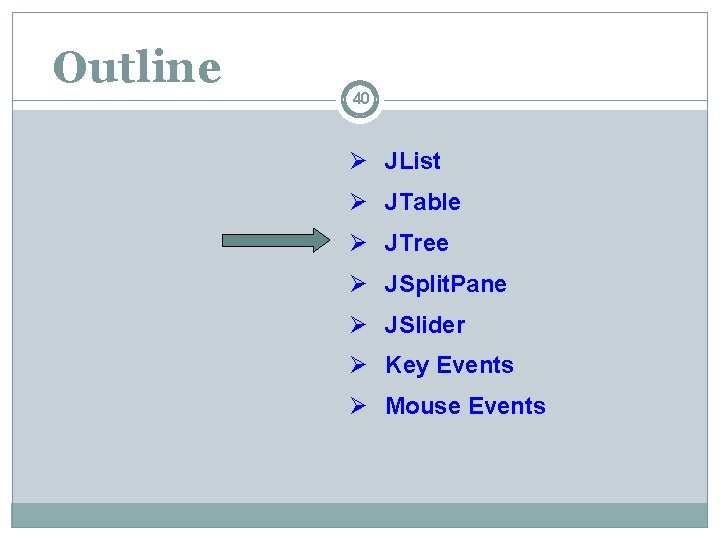
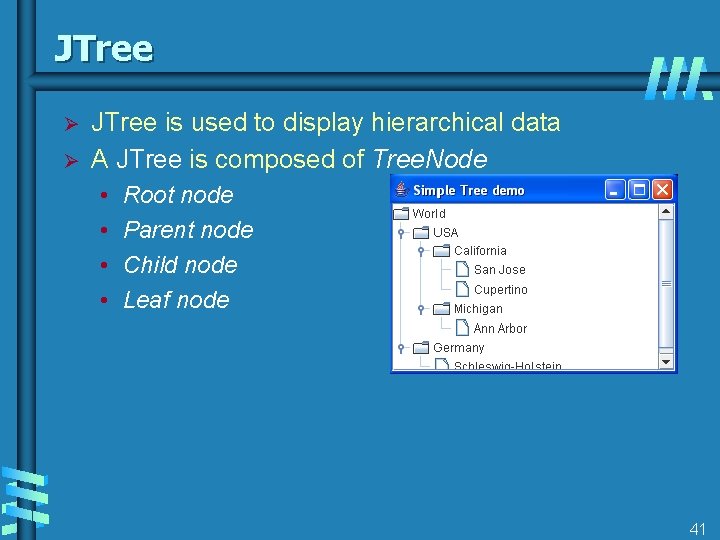
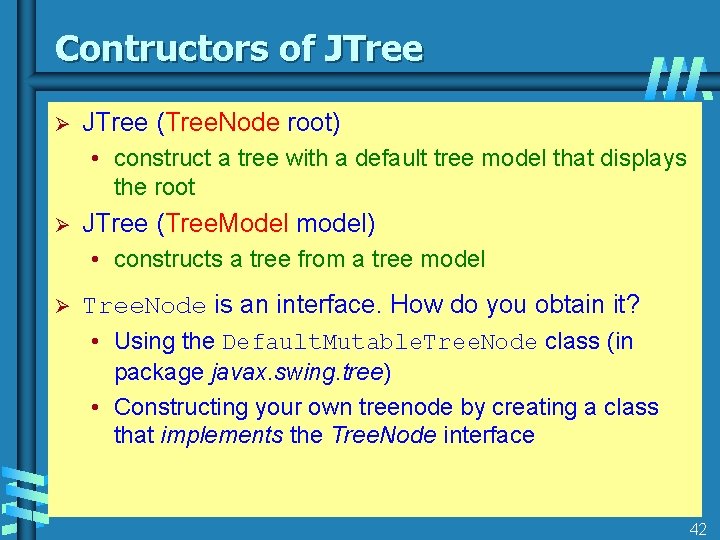
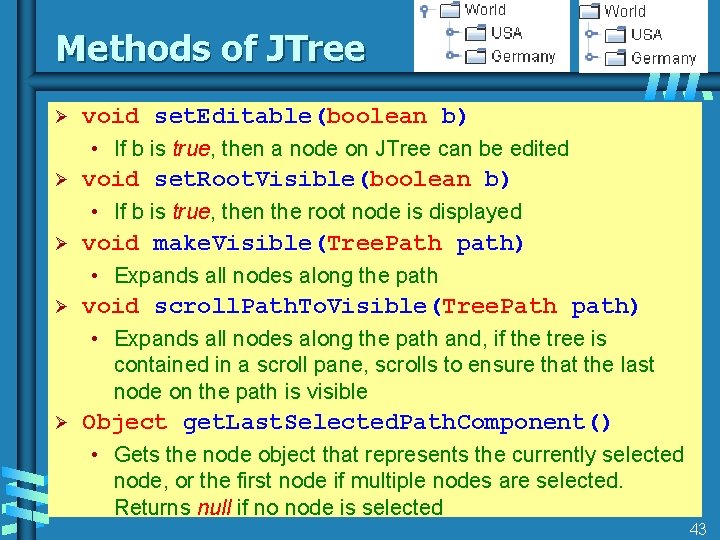
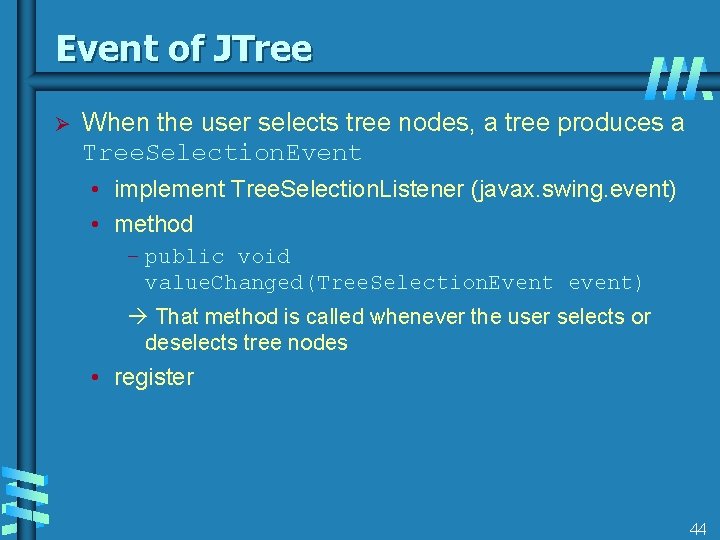
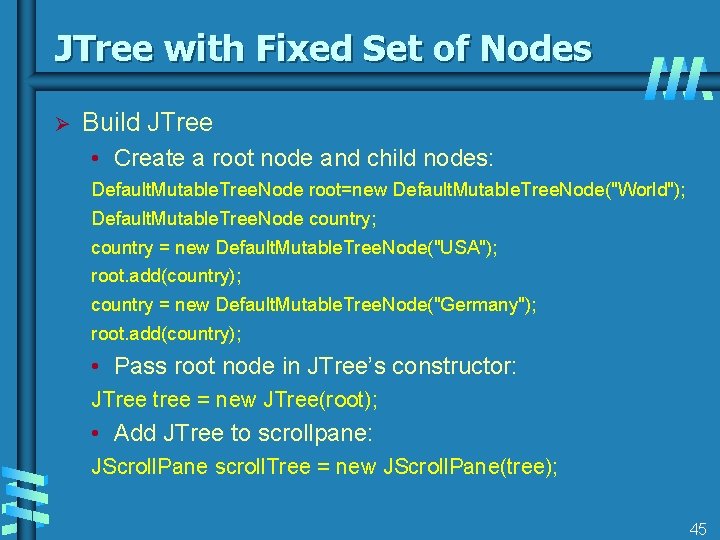
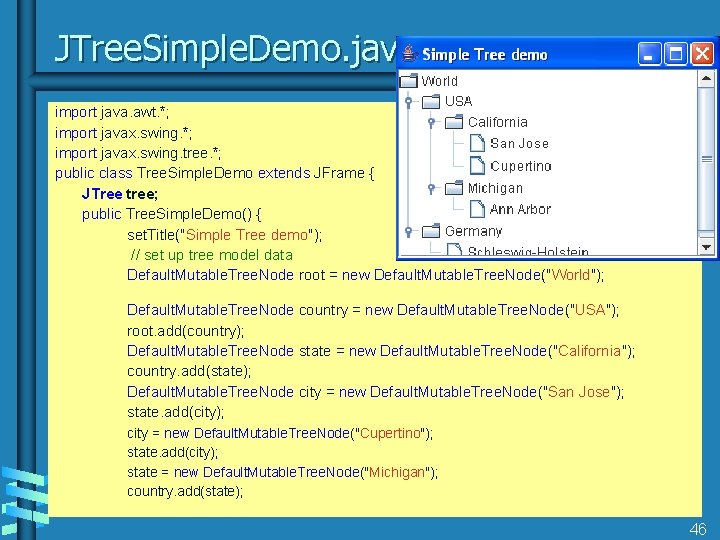
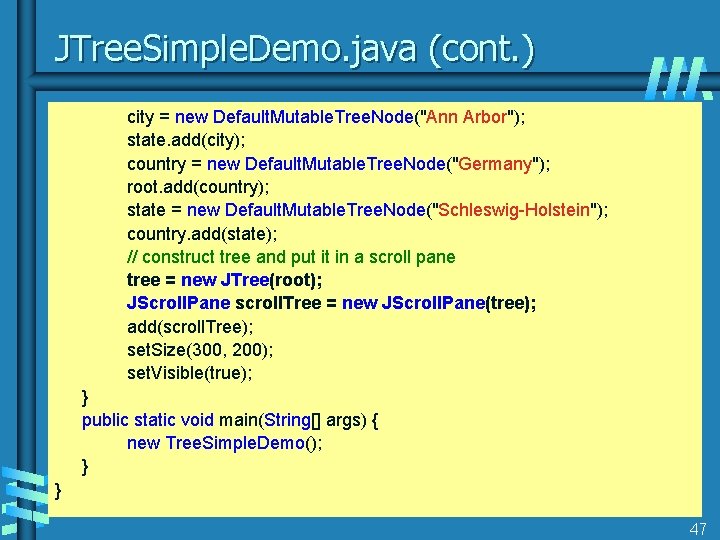
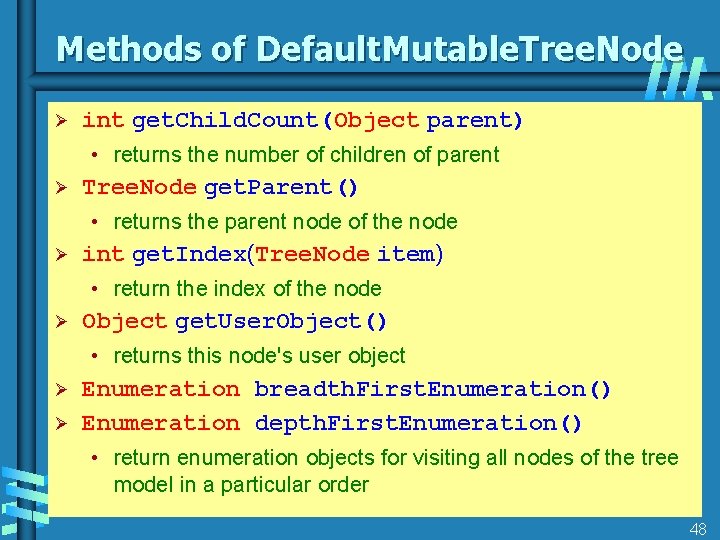
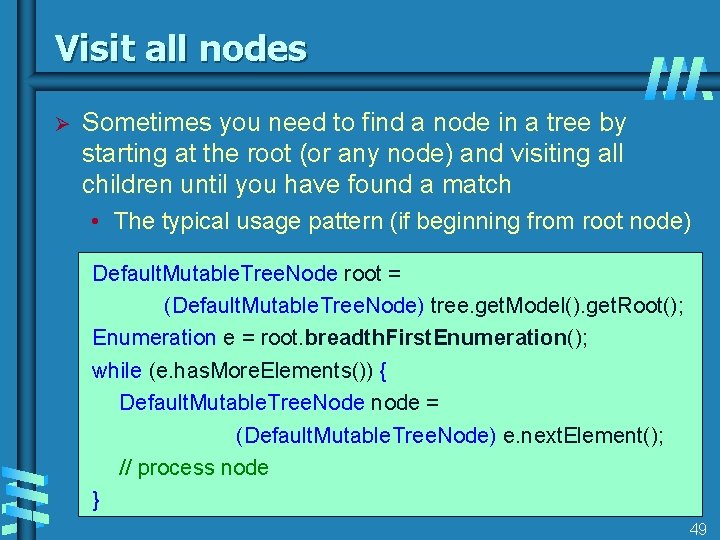
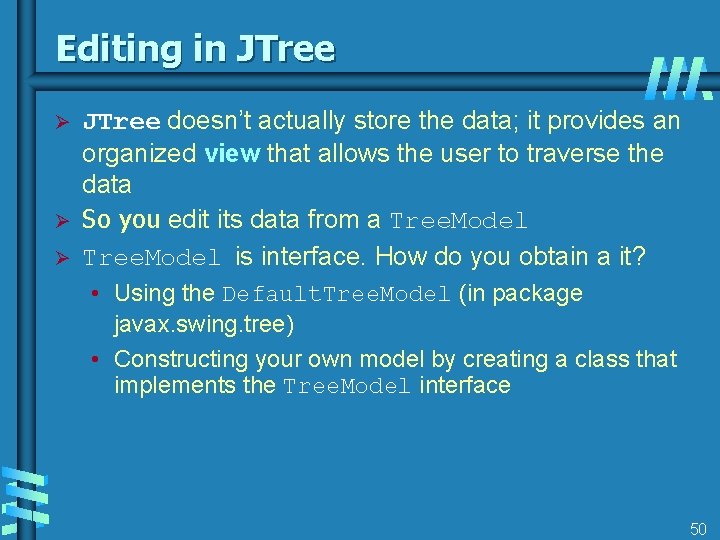
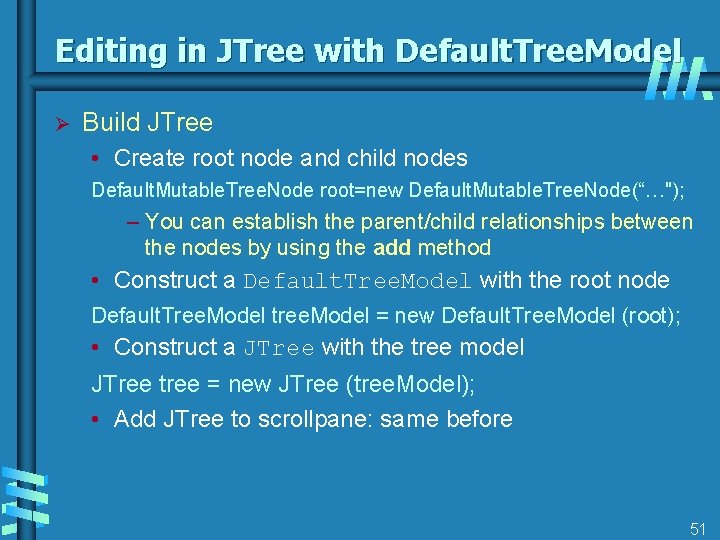
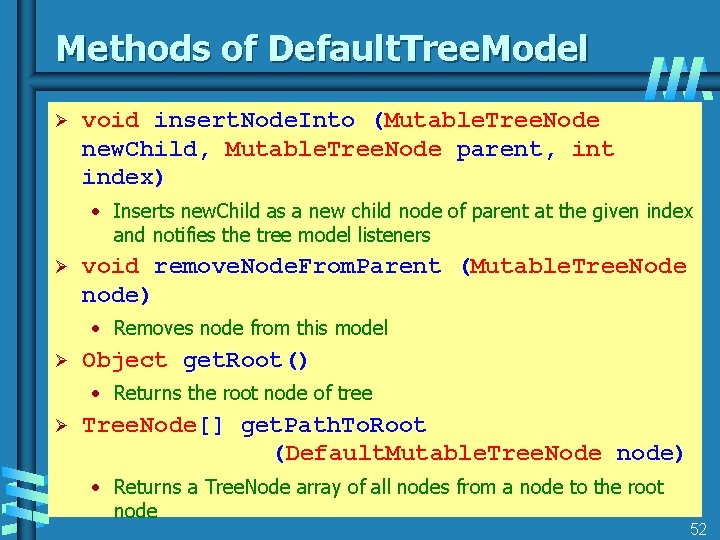
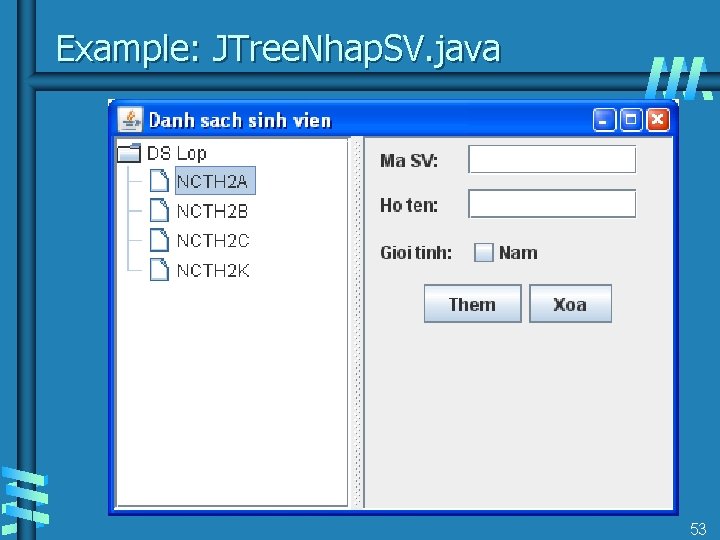
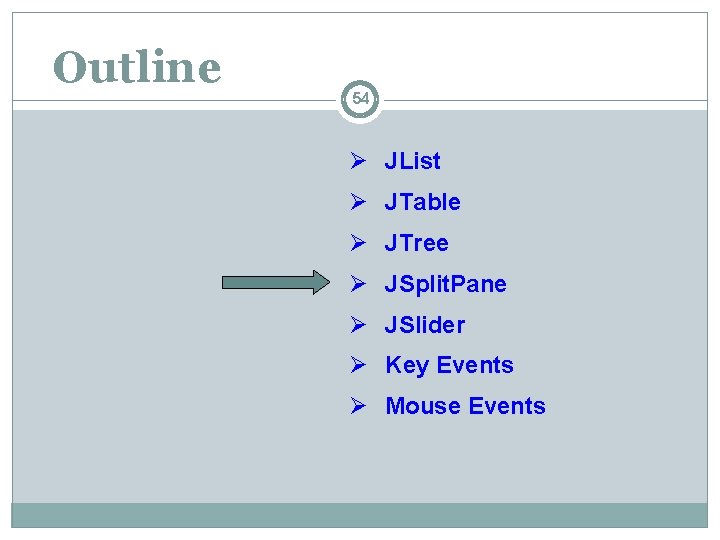
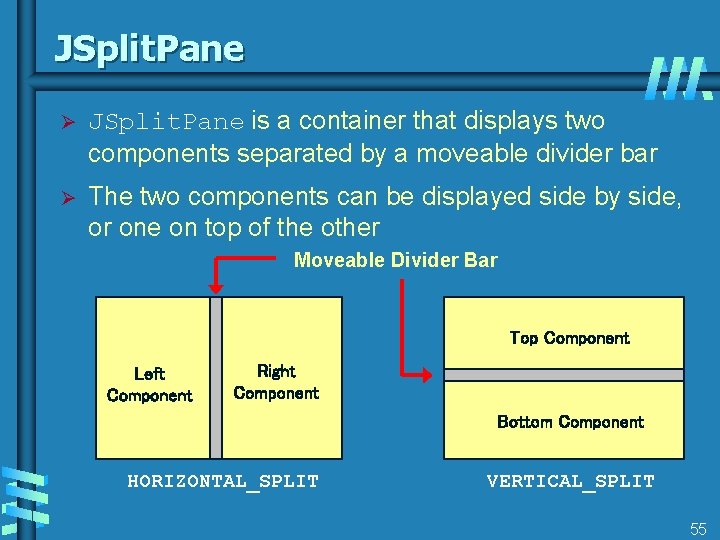
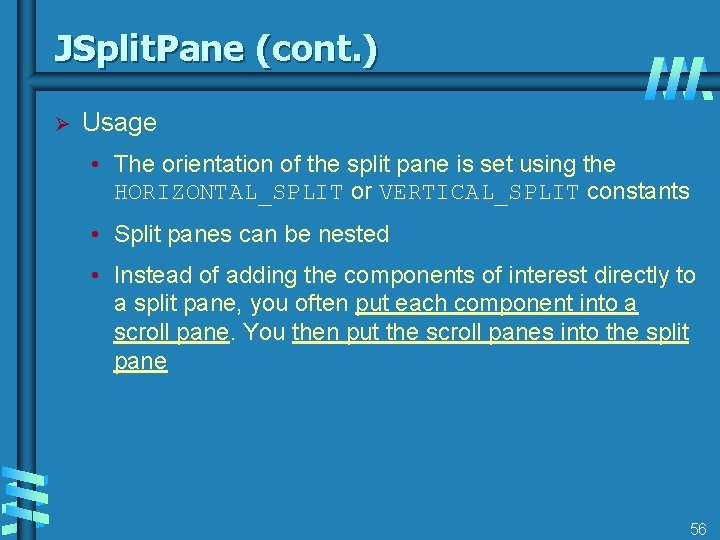
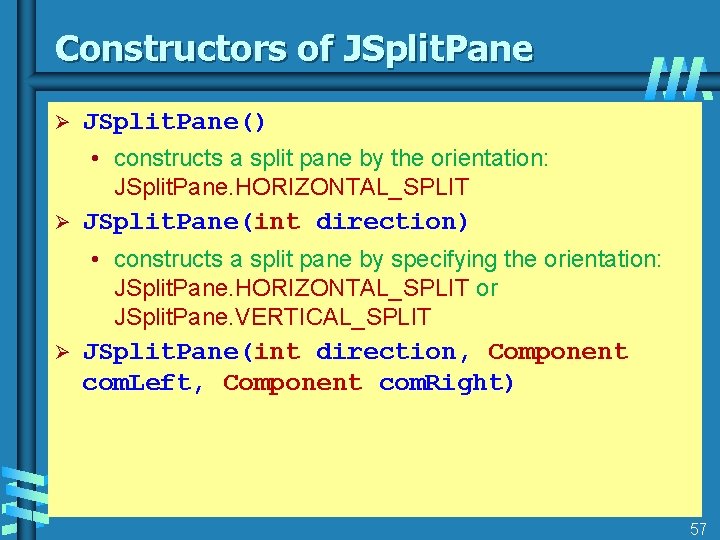
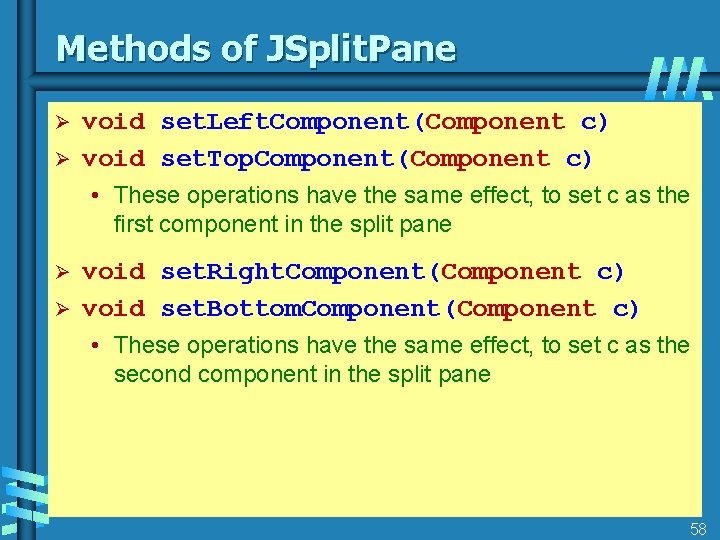
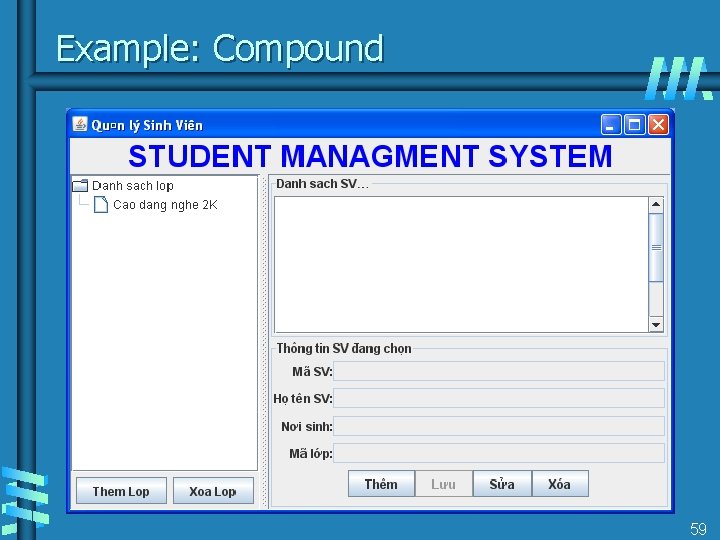
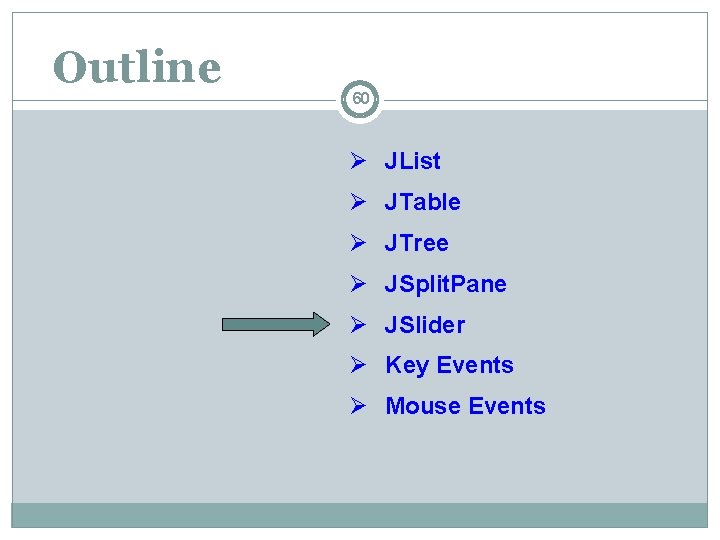
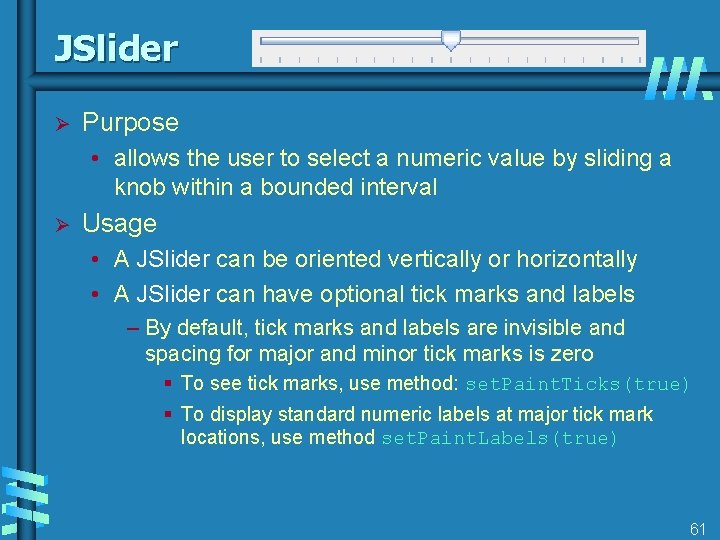
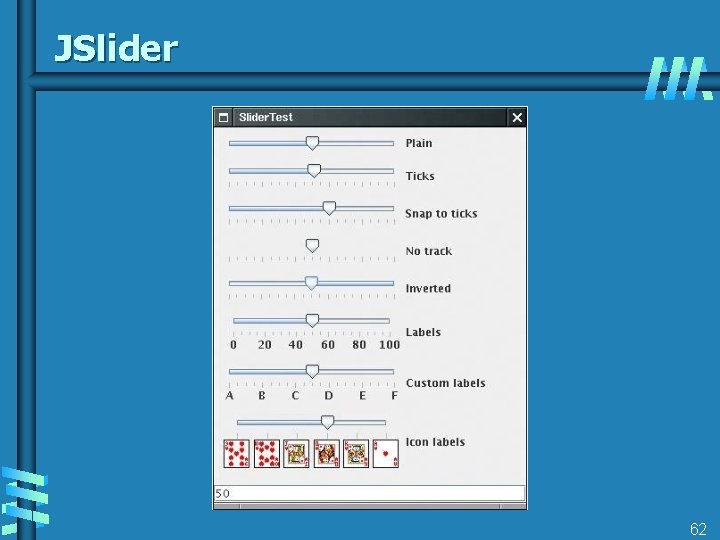
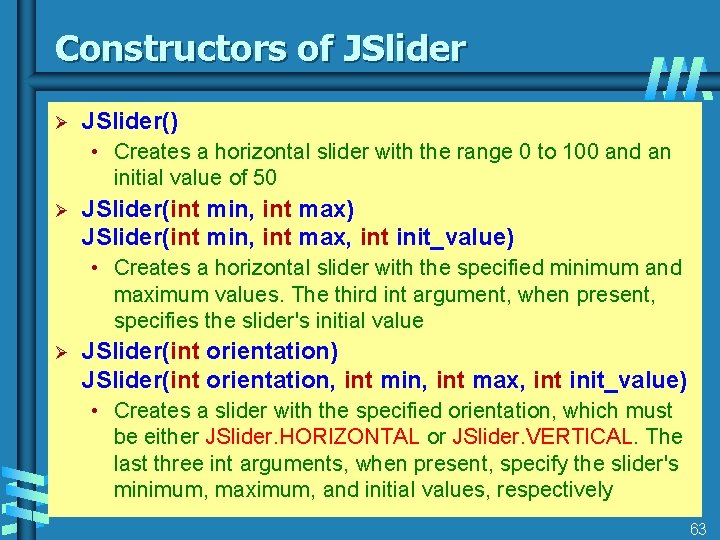
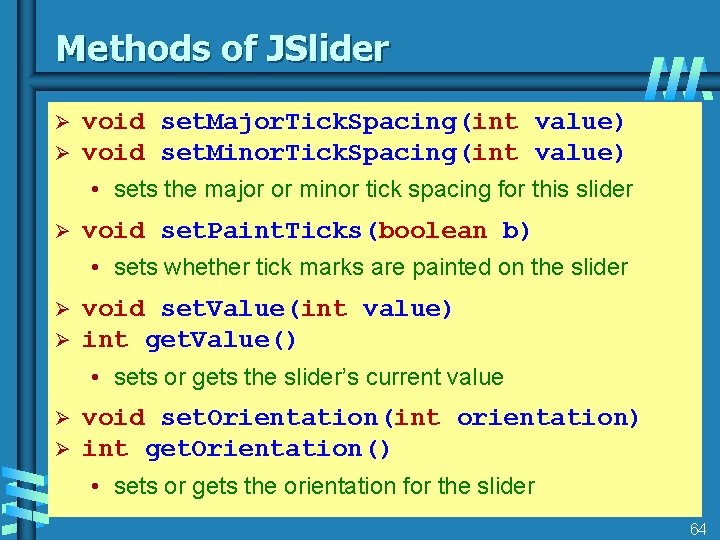
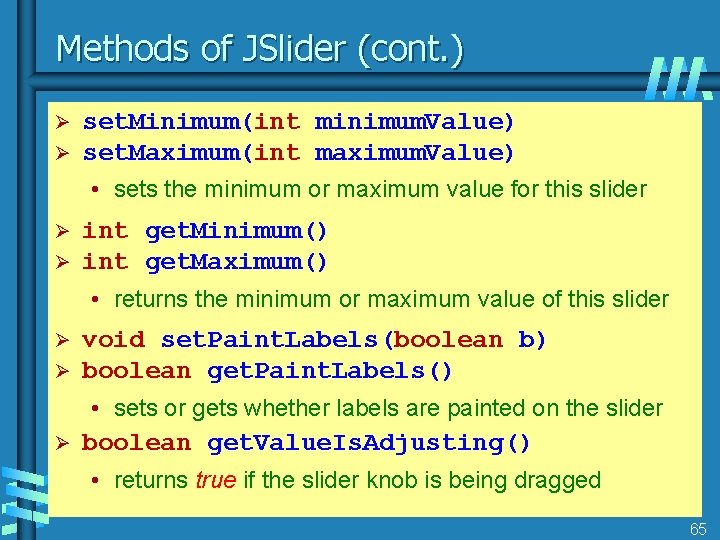
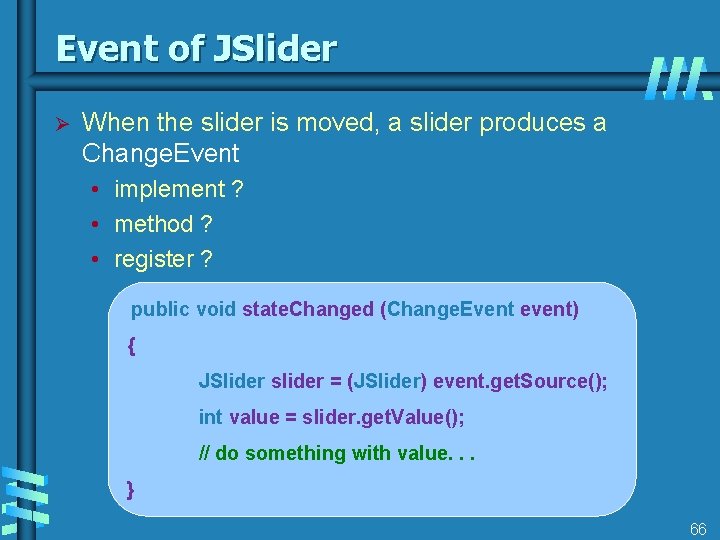
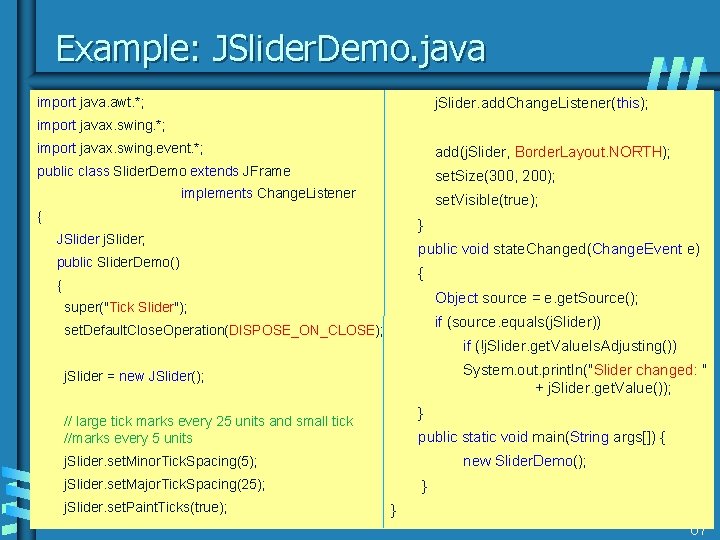
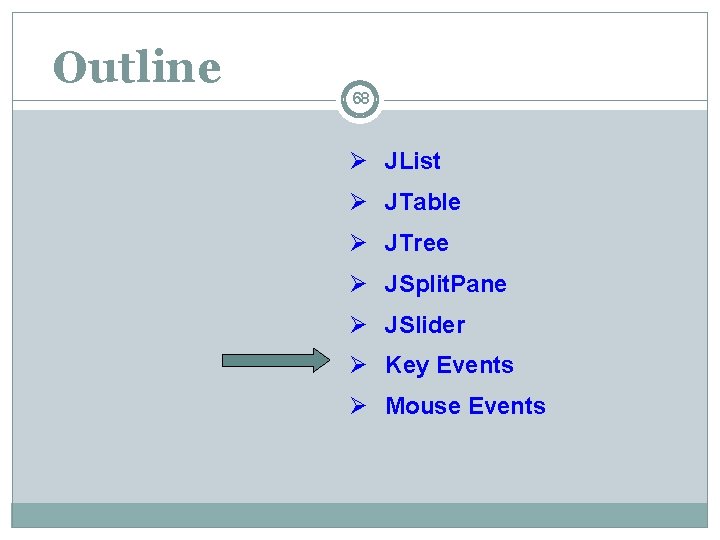
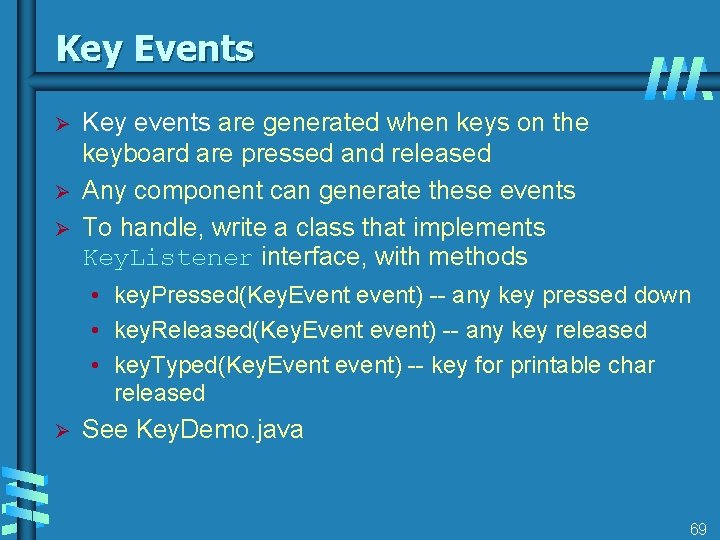
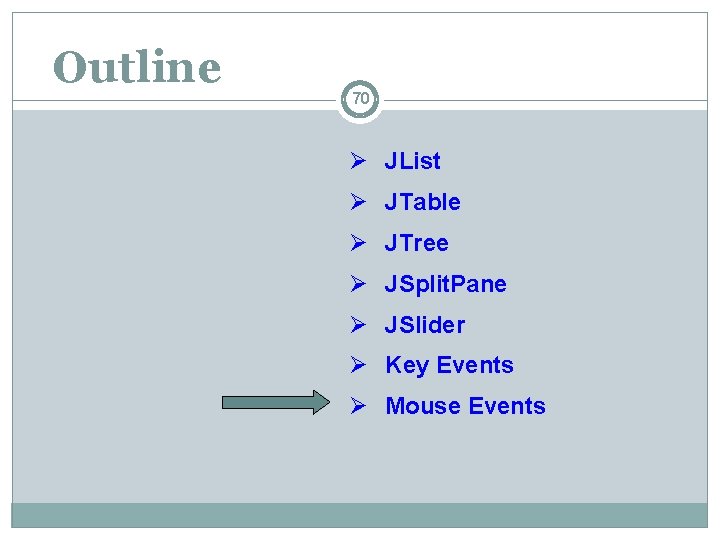
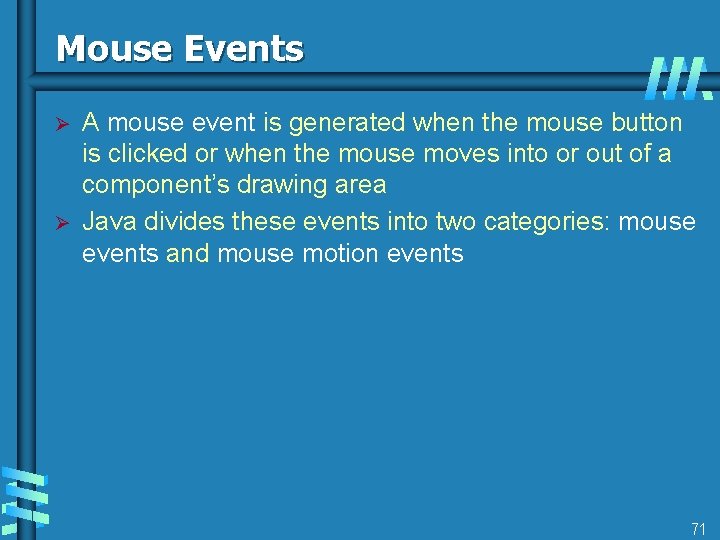
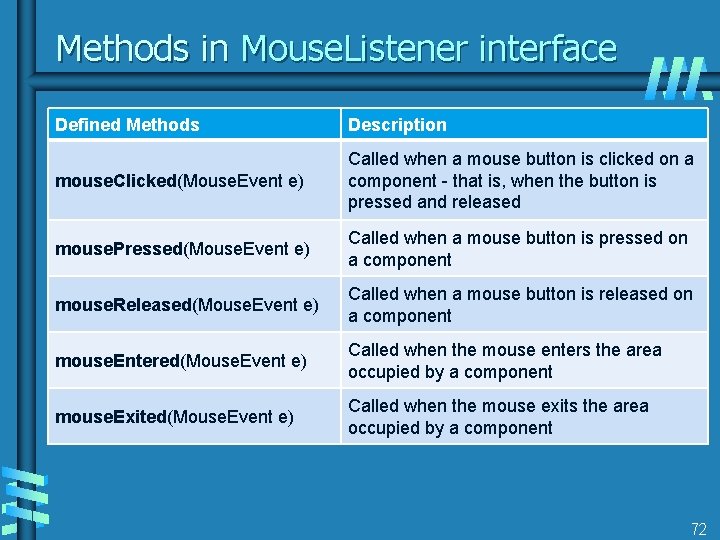
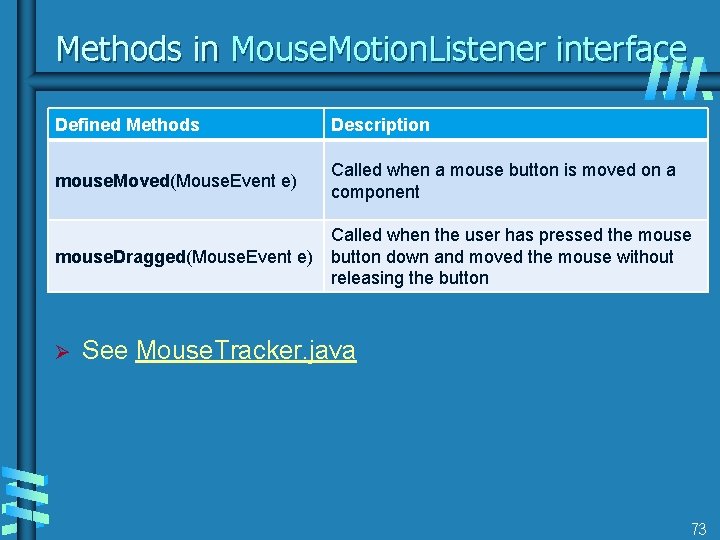
- Slides: 72
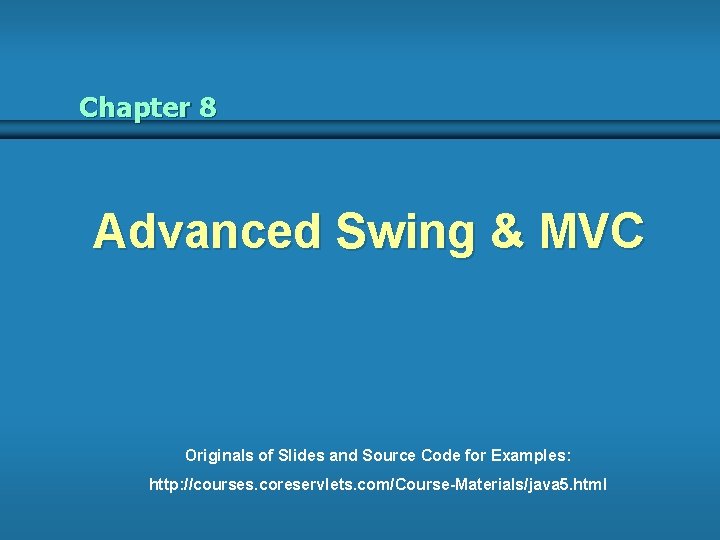
Chapter 8 Advanced Swing & MVC Originals of Slides and Source Code for Examples: http: //courses. coreservlets. com/Course-Materials/java 5. html
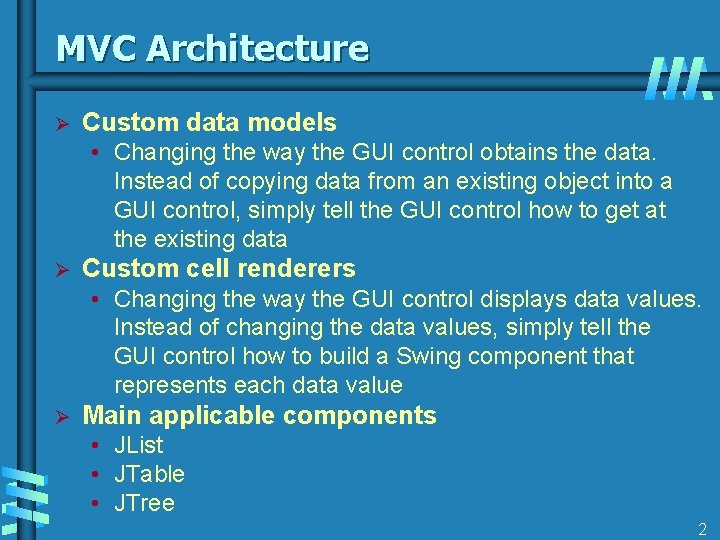
MVC Architecture Ø Custom data models • Changing the way the GUI control obtains the data. Instead of copying data from an existing object into a GUI control, simply tell the GUI control how to get at the existing data Ø Custom cell renderers • Changing the way the GUI control displays data values. Instead of changing the data values, simply tell the GUI control how to build a Swing component that represents each data value Ø Main applicable components • JList • JTable • JTree 2
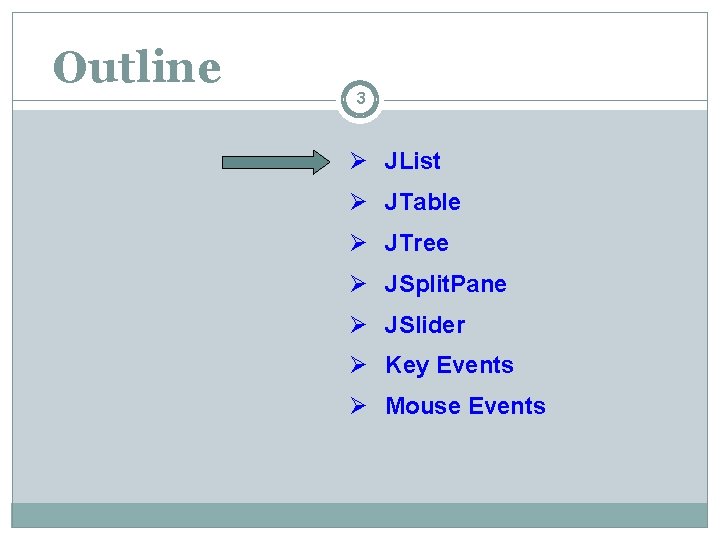
Outline 3 Ø JList Ø JTable Ø JTree Ø JSplit. Pane Ø JSlider Ø Key Events Ø Mouse Events
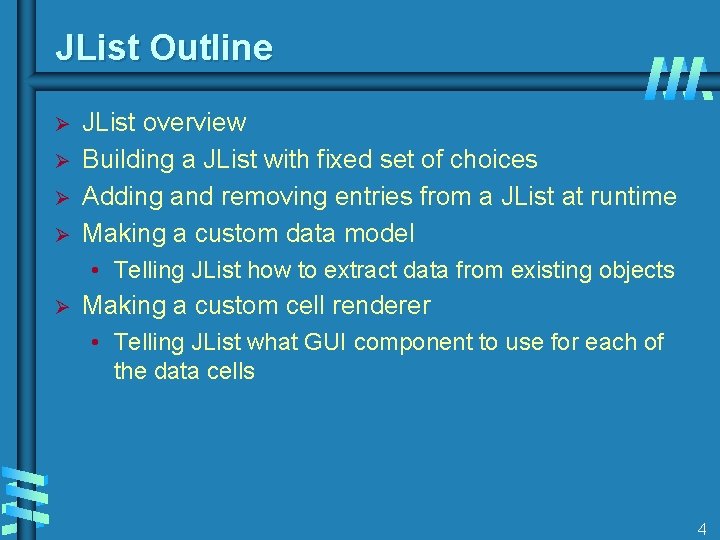
JList Outline Ø Ø JList overview Building a JList with fixed set of choices Adding and removing entries from a JList at runtime Making a custom data model • Telling JList how to extract data from existing objects Ø Making a custom cell renderer • Telling JList what GUI component to use for each of the data cells 4
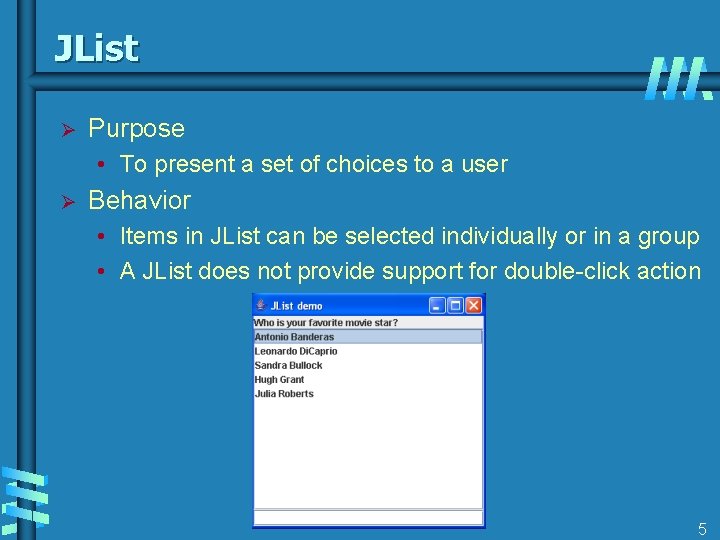
JList Ø Purpose • To present a set of choices to a user Ø Behavior • Items in JList can be selected individually or in a group • A JList does not provide support for double-click action 5
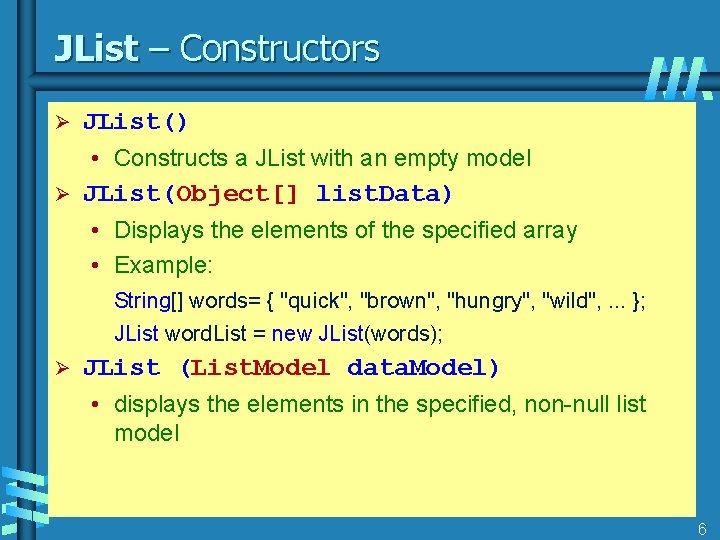
JList – Constructors Ø JList() • Constructs a JList with an empty model Ø JList(Object[] list. Data) • Displays the elements of the specified array • Example: String[] words= { "quick", "brown", "hungry", "wild", . . . }; JList word. List = new JList(words); Ø JList (List. Model data. Model) • displays the elements in the specified, non-null list model 6
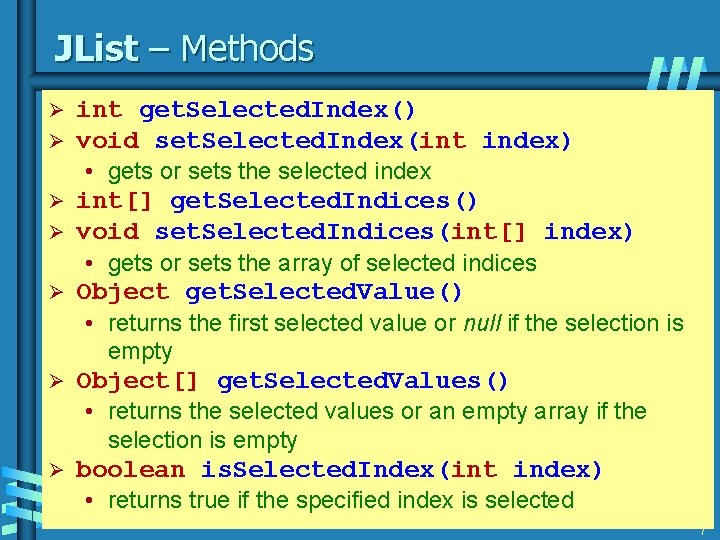
JList – Methods Ø Ø int get. Selected. Index() void set. Selected. Index(int index) • gets or sets the selected index Ø Ø int[] get. Selected. Indices() void set. Selected. Indices(int[] index) • gets or sets the array of selected indices Ø Object get. Selected. Value() • returns the first selected value or null if the selection is empty Ø Object[] get. Selected. Values() • returns the selected values or an empty array if the selection is empty Ø boolean is. Selected. Index(int index) • returns true if the specified index is selected 7
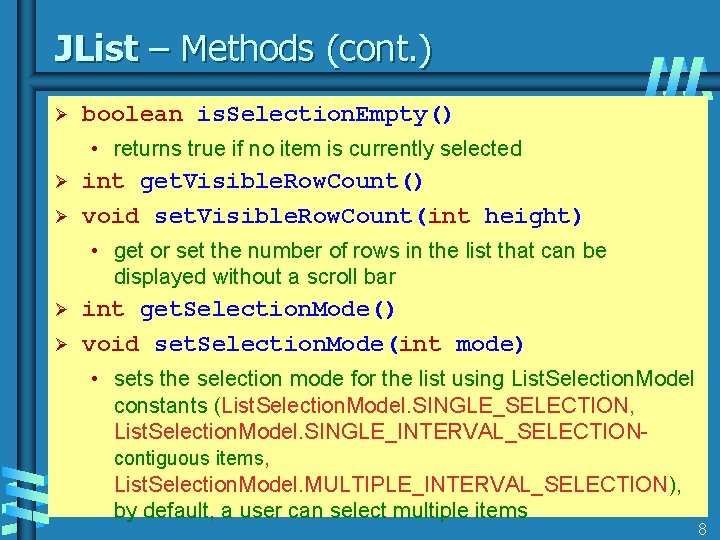
JList – Methods (cont. ) Ø boolean is. Selection. Empty() • returns true if no item is currently selected Ø Ø int get. Visible. Row. Count() void set. Visible. Row. Count(int height) • get or set the number of rows in the list that can be displayed without a scroll bar Ø Ø int get. Selection. Mode() void set. Selection. Mode(int mode) • sets the selection mode for the list using List. Selection. Model constants (List. Selection. Model. SINGLE_SELECTION, List. Selection. Model. SINGLE_INTERVAL_SELECTIONcontiguous items, List. Selection. Model. MULTIPLE_INTERVAL_SELECTION), by default, a user can select multiple items 8
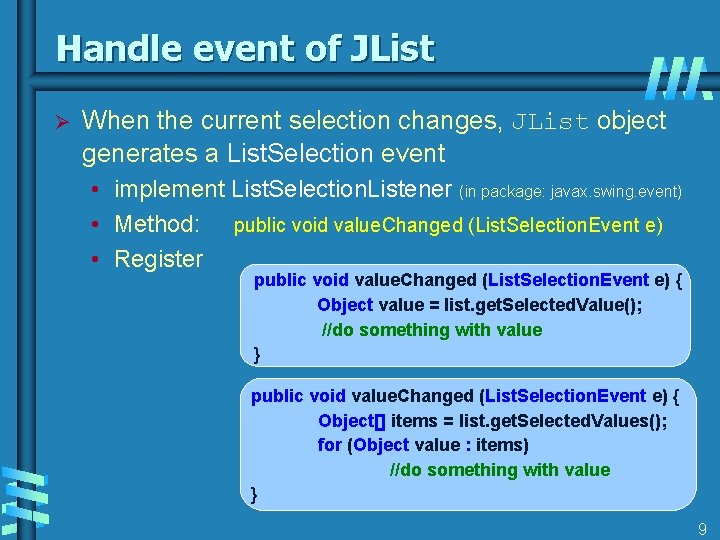
Handle event of JList Ø When the current selection changes, JList object generates a List. Selection event • implement List. Selection. Listener (in package: javax. swing. event) • Method: public void value. Changed (List. Selection. Event e) • Register public void value. Changed (List. Selection. Event e) { Object value = list. get. Selected. Value(); //do something with value } public void value. Changed (List. Selection. Event e) { Object[] items = list. get. Selected. Values(); for (Object value : items) //do something with value } 9
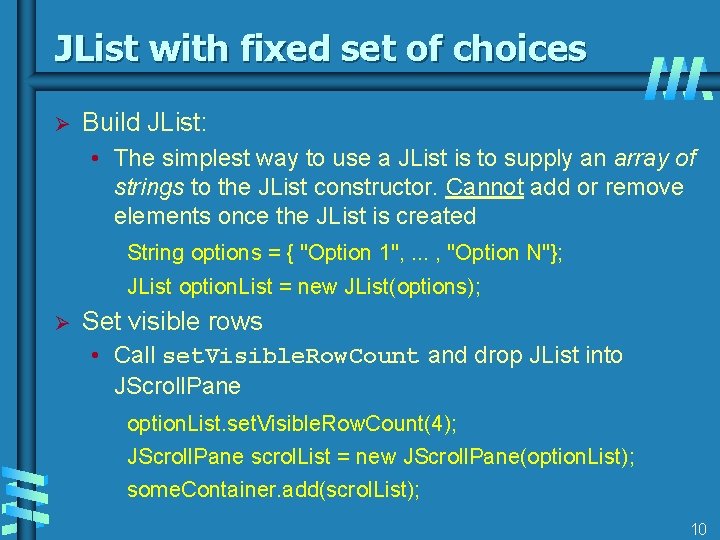
JList with fixed set of choices Ø Build JList: • The simplest way to use a JList is to supply an array of strings to the JList constructor. Cannot add or remove elements once the JList is created String options = { "Option 1", . . . , "Option N"}; JList option. List = new JList(options); Ø Set visible rows • Call set. Visible. Row. Count and drop JList into JScroll. Pane option. List. set. Visible. Row. Count(4); JScroll. Pane scrol. List = new JScroll. Pane(option. List); some. Container. add(scrol. List); 10
![Example JList Simple Demo java String entries Entry 1 Entry 2 Entry Example: JList. Simple. Demo. java String[] entries = { "Entry 1", "Entry 2", "Entry](https://slidetodoc.com/presentation_image_h2/f4808711ed7de67909459c043a9af1c7/image-11.jpg)
Example: JList. Simple. Demo. java String[] entries = { "Entry 1", "Entry 2", "Entry 3", "Entry 4", "Entry 5", "Entry 6" }; JList lst. Entry; lst. Entry = new JList(entries); lst. Entry. set. Visible. Row. Count(4); JScroll. Pane list. Pane = new JScroll. Pane(lst. Entry); JPanel p. Cen = new JPanel(); p. Cen. set. Border(Border. Factory. create. Titled. Border("Simple JList")); p. Cen. add(list. Pane); add(p. Cen, Border. Layout. CENTER); 11
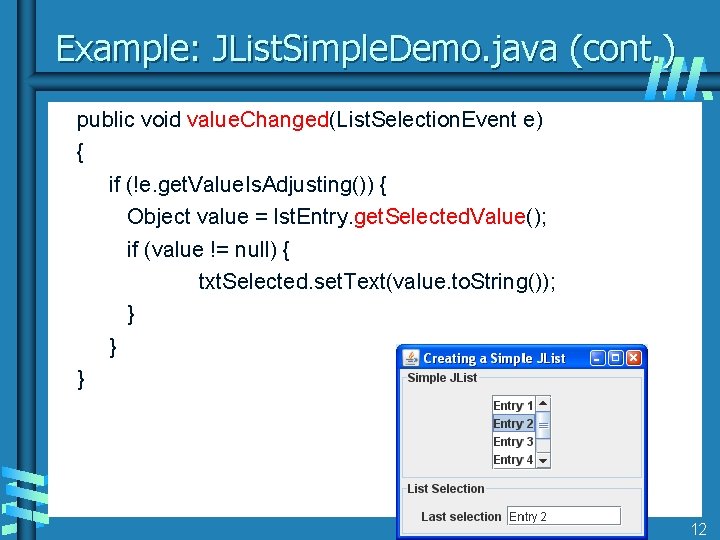
Example: JList. Simple. Demo. java (cont. ) public void value. Changed(List. Selection. Event e) { if (!e. get. Value. Is. Adjusting()) { Object value = lst. Entry. get. Selected. Value(); if (value != null) { txt. Selected. set. Text(value. to. String()); } } } 12
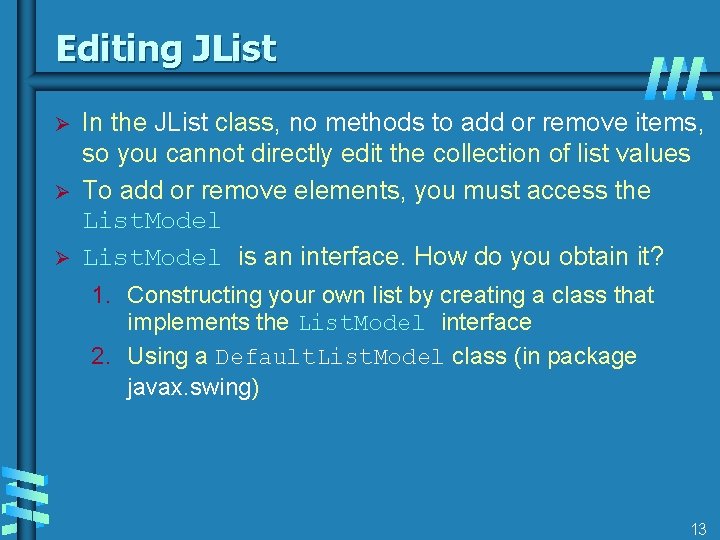
Editing JList Ø Ø Ø In the JList class, no methods to add or remove items, so you cannot directly edit the collection of list values To add or remove elements, you must access the List. Model is an interface. How do you obtain it? 1. Constructing your own list by creating a class that implements the List. Model interface 2. Using a Default. List. Model class (in package javax. swing) 13
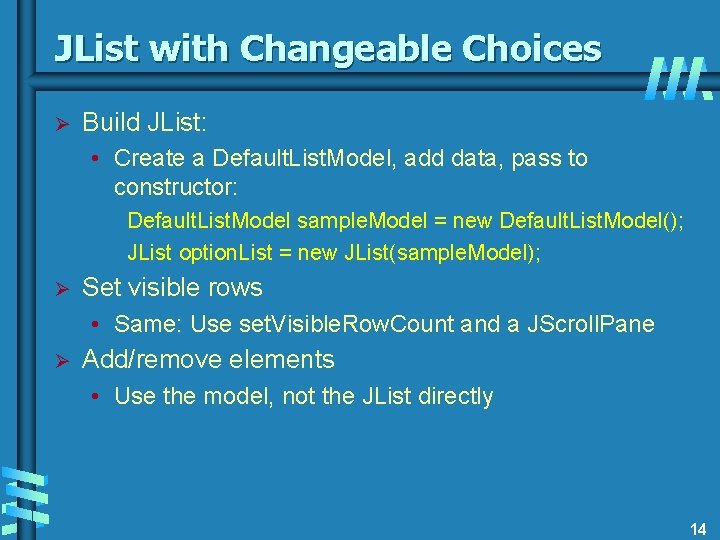
JList with Changeable Choices Ø Build JList: • Create a Default. List. Model, add data, pass to constructor: Default. List. Model sample. Model = new Default. List. Model(); JList option. List = new JList(sample. Model); Ø Set visible rows • Same: Use set. Visible. Row. Count and a JScroll. Pane Ø Add/remove elements • Use the model, not the JList directly 14
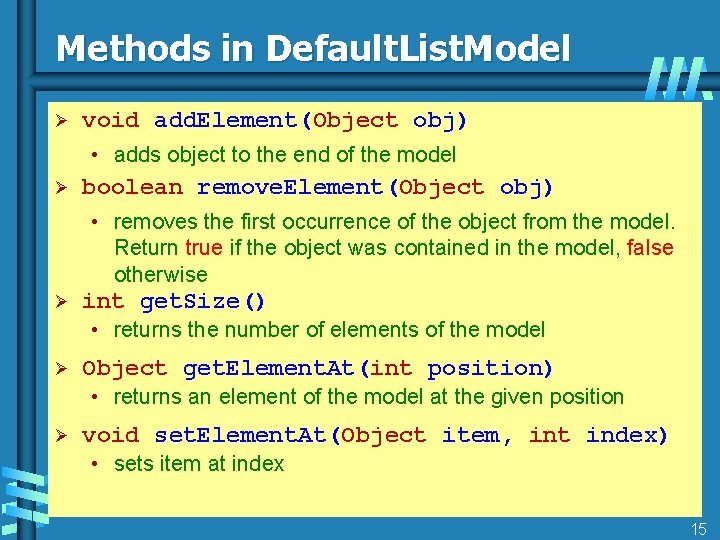
Methods in Default. List. Model Ø void add. Element(Object obj) • adds object to the end of the model Ø boolean remove. Element(Object obj) • removes the first occurrence of the object from the model. Return true if the object was contained in the model, false otherwise Ø int get. Size() • returns the number of elements of the model Ø Object get. Element. At(int position) • returns an element of the model at the given position Ø void set. Element. At(Object item, int index) • sets item at index 15
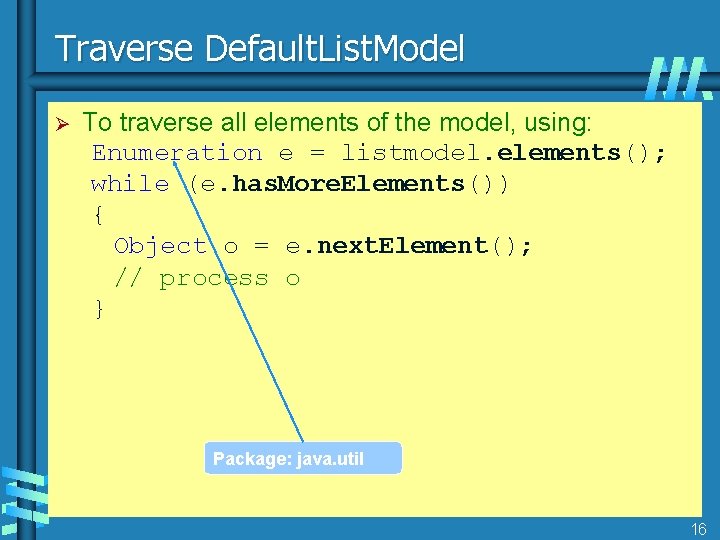
Traverse Default. List. Model Ø To traverse all elements of the model, using: Enumeration e = listmodel. elements(); while (e. has. More. Elements()) { Object o = e. next. Element(); // process o } Package: java. util 16
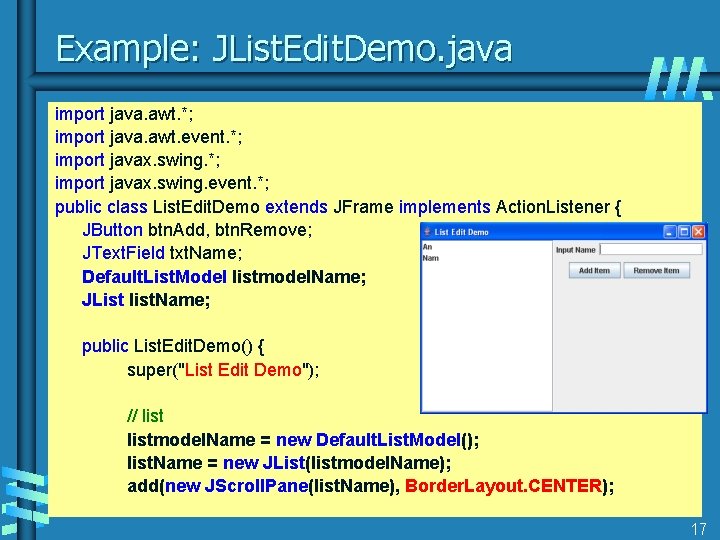
Example: JList. Edit. Demo. java import java. awt. *; import java. awt. event. *; import javax. swing. event. *; public class List. Edit. Demo extends JFrame implements Action. Listener { JButton btn. Add, btn. Remove; JText. Field txt. Name; Default. List. Model listmodel. Name; JList list. Name; public List. Edit. Demo() { super("List Edit Demo"); // listmodel. Name = new Default. List. Model(); list. Name = new JList(listmodel. Name); add(new JScroll. Pane(list. Name), Border. Layout. CENTER); 17
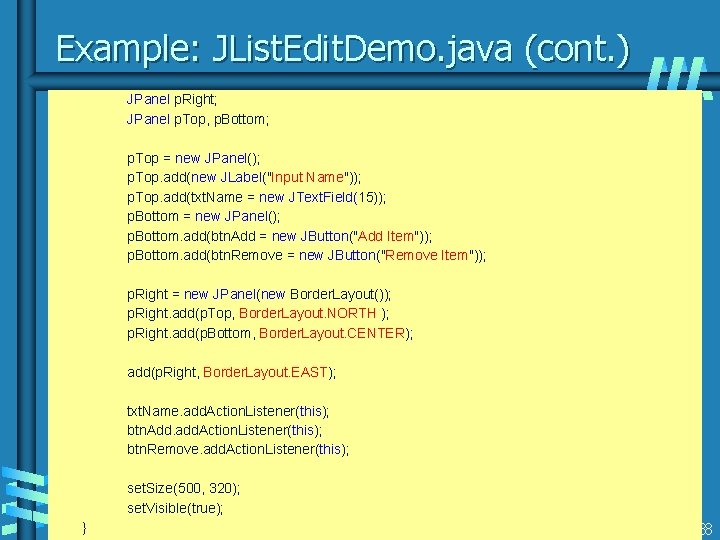
Example: JList. Edit. Demo. java (cont. ) JPanel p. Right; JPanel p. Top, p. Bottom; p. Top = new JPanel(); p. Top. add(new JLabel("Input Name")); p. Top. add(txt. Name = new JText. Field(15)); p. Bottom = new JPanel(); p. Bottom. add(btn. Add = new JButton("Add Item")); p. Bottom. add(btn. Remove = new JButton("Remove Item")); p. Right = new JPanel(new Border. Layout()); p. Right. add(p. Top, Border. Layout. NORTH ); p. Right. add(p. Bottom, Border. Layout. CENTER); add(p. Right, Border. Layout. EAST); txt. Name. add. Action. Listener(this); btn. Add. add. Action. Listener(this); btn. Remove. add. Action. Listener(this); set. Size(500, 320); set. Visible(true); } 18 18
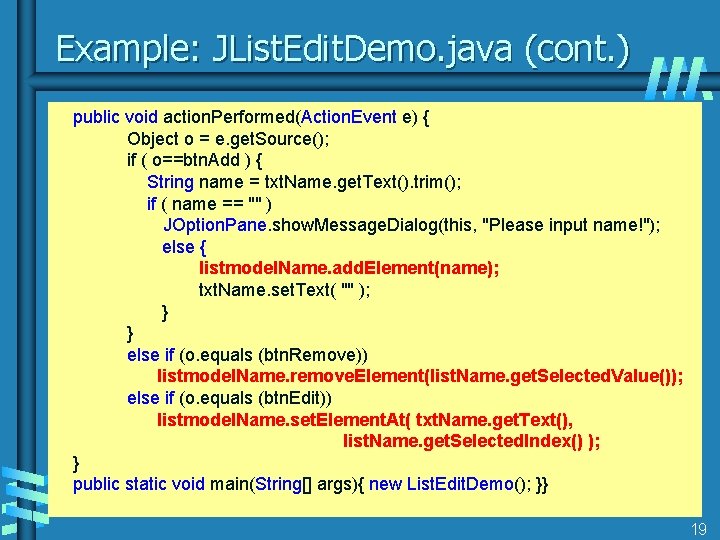
Example: JList. Edit. Demo. java (cont. ) public void action. Performed(Action. Event e) { Object o = e. get. Source(); if ( o==btn. Add ) { String name = txt. Name. get. Text(). trim(); if ( name == "" ) JOption. Pane. show. Message. Dialog(this, "Please input name!"); else { listmodel. Name. add. Element(name); txt. Name. set. Text( "" ); } } else if (o. equals (btn. Remove)) listmodel. Name. remove. Element(list. Name. get. Selected. Value()); else if (o. equals (btn. Edit)) listmodel. Name. set. Element. At( txt. Name. get. Text(), list. Name. get. Selected. Index() ); } public static void main(String[] args){ new List. Edit. Demo(); }} 19
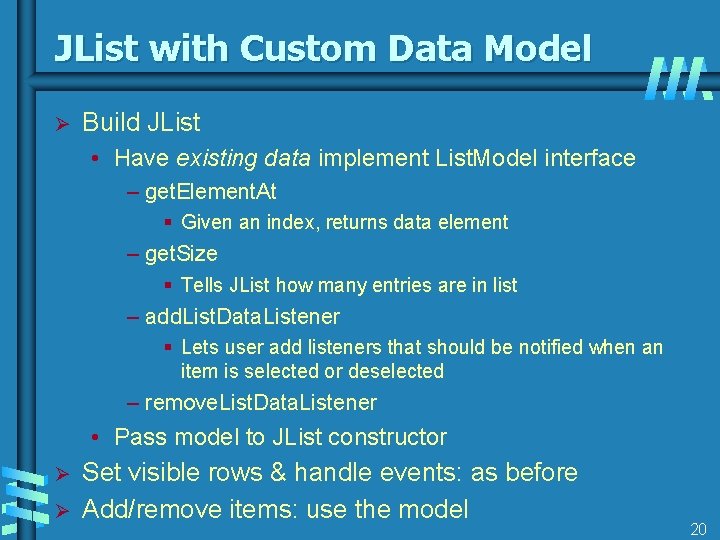
JList with Custom Data Model Ø Build JList • Have existing data implement List. Model interface – get. Element. At § Given an index, returns data element – get. Size § Tells JList how many entries are in list – add. List. Data. Listener § Lets user add listeners that should be notified when an item is selected or deselected – remove. List. Data. Listener • Pass model to JList constructor Ø Ø Set visible rows & handle events: as before Add/remove items: use the model 20
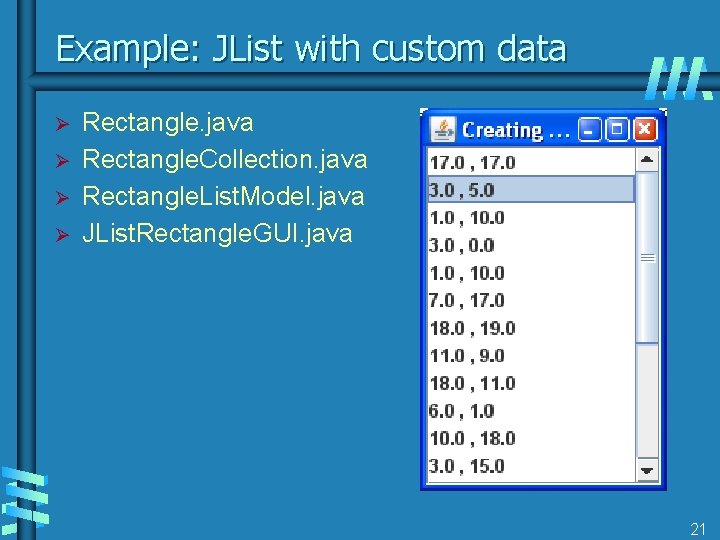
Example: JList with custom data Ø Ø Rectangle. java Rectangle. Collection. java Rectangle. List. Model. java JList. Rectangle. GUI. java 21
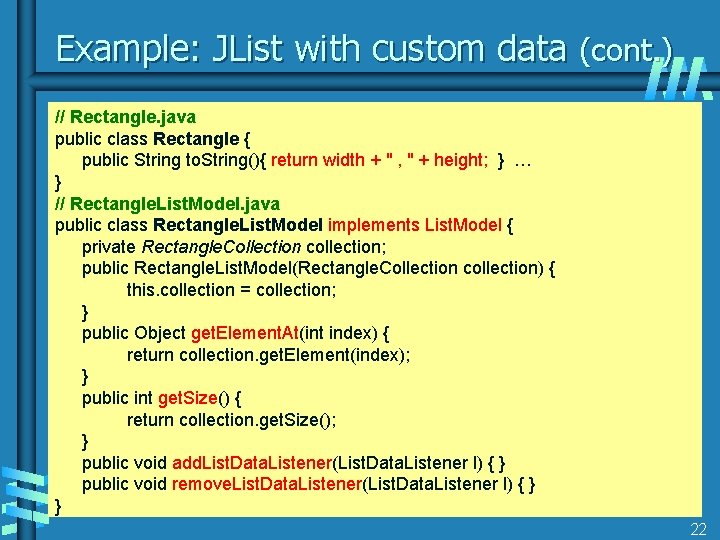
Example: JList with custom data (cont. ) // Rectangle. java public class Rectangle { public String to. String(){ return width + " , " + height; } … } // Rectangle. List. Model. java public class Rectangle. List. Model implements List. Model { private Rectangle. Collection collection; public Rectangle. List. Model(Rectangle. Collection collection) { this. collection = collection; } public Object get. Element. At(int index) { return collection. get. Element(index); } public int get. Size() { return collection. get. Size(); } public void add. List. Data. Listener(List. Data. Listener l) { } public void remove. List. Data. Listener(List. Data. Listener l) { } } 22
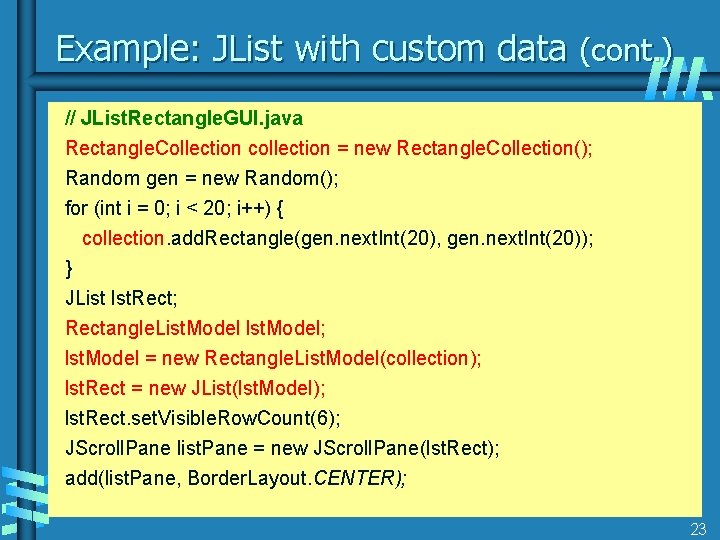
Example: JList with custom data (cont. ) // JList. Rectangle. GUI. java Rectangle. Collection collection = new Rectangle. Collection(); Random gen = new Random(); for (int i = 0; i < 20; i++) { collection. add. Rectangle(gen. next. Int(20), gen. next. Int(20)); } JList lst. Rect; Rectangle. List. Model lst. Model; lst. Model = new Rectangle. List. Model(collection); lst. Rect = new JList(lst. Model); lst. Rect. set. Visible. Row. Count(6); JScroll. Pane list. Pane = new JScroll. Pane(lst. Rect); add(list. Pane, Border. Layout. CENTER); 23
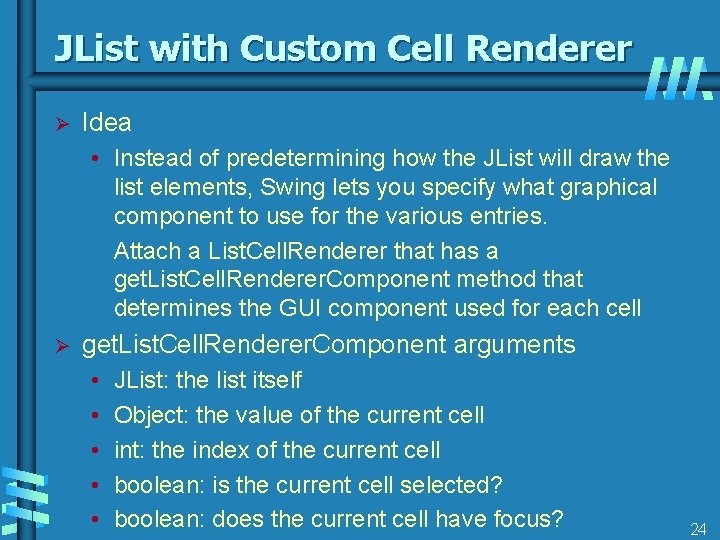
JList with Custom Cell Renderer Ø Idea • Instead of predetermining how the JList will draw the list elements, Swing lets you specify what graphical component to use for the various entries. Attach a List. Cell. Renderer that has a get. List. Cell. Renderer. Component method that determines the GUI component used for each cell Ø get. List. Cell. Renderer. Component arguments • • • JList: the list itself Object: the value of the current cell int: the index of the current cell boolean: is the current cell selected? boolean: does the current cell have focus? 24
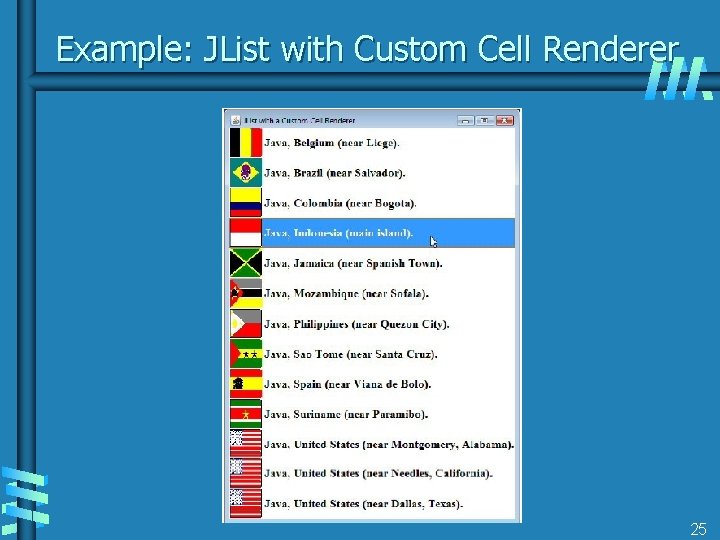
Example: JList with Custom Cell Renderer 25
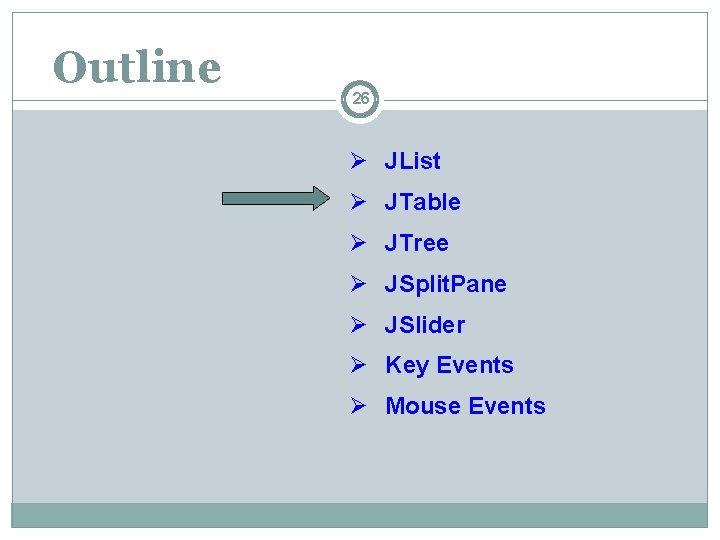
Outline 26 Ø JList Ø JTable Ø JTree Ø JSplit. Pane Ø JSlider Ø Key Events Ø Mouse Events
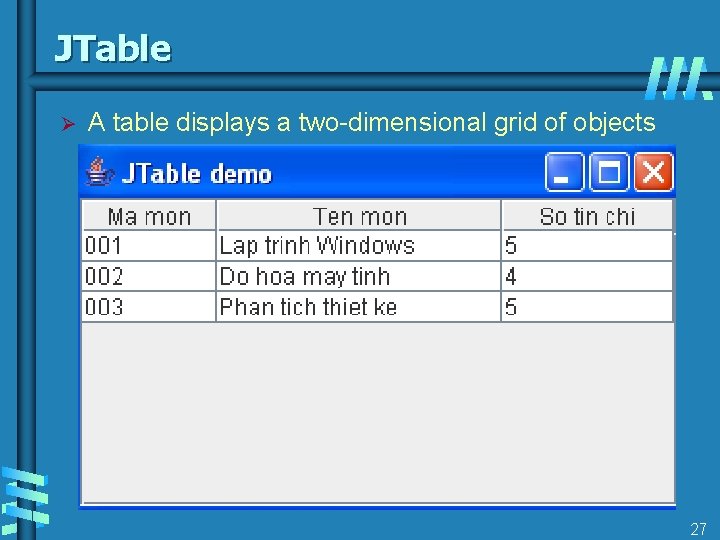
JTable Ø A table displays a two-dimensional grid of objects 27
![Constructors Methods of JTable Ø JTableObject entries Object column Names constructs a Constructors - Methods of JTable Ø JTable(Object[][] entries, Object[] column. Names) • constructs a](https://slidetodoc.com/presentation_image_h2/f4808711ed7de67909459c043a9af1c7/image-28.jpg)
Constructors - Methods of JTable Ø JTable(Object[][] entries, Object[] column. Names) • constructs a table with a default table model Ø JTable(Table. Model model) • displays the elements in the specified, non-null table model Ø int get. Selected. Row() • returns the index of the first selected row, -1 if no row is selected Ø Ø Object get. Value. At(int row, int column) void set. Value. At(Object value, int row, int column) • gets or sets the value at the given row and column Ø int get. Row. Count() • returns the number of row in the table 28
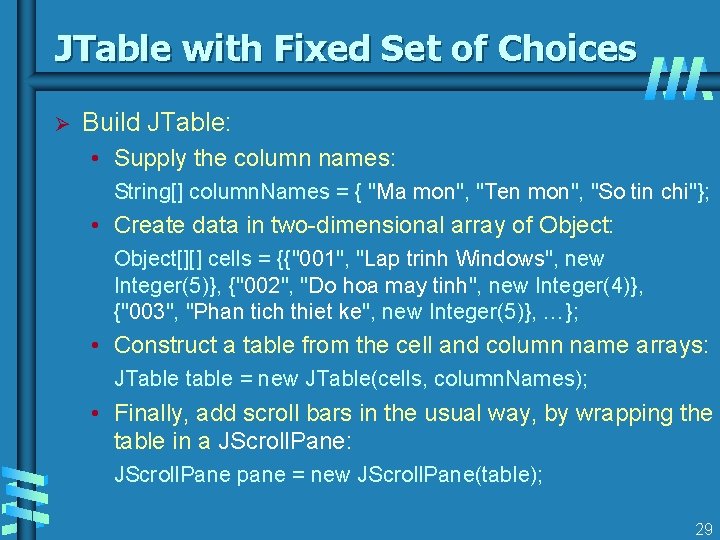
JTable with Fixed Set of Choices Ø Build JTable: • Supply the column names: String[] column. Names = { "Ma mon", "Ten mon", "So tin chi"}; • Create data in two-dimensional array of Object: Object[][] cells = {{"001", "Lap trinh Windows", new Integer(5)}, {"002", "Do hoa may tinh", new Integer(4)}, {"003", "Phan tich thiet ke", new Integer(5)}, …}; • Construct a table from the cell and column name arrays: JTable table = new JTable(cells, column. Names); • Finally, add scroll bars in the usual way, by wrapping the table in a JScroll. Pane: JScroll. Pane pane = new JScroll. Pane(table); 29
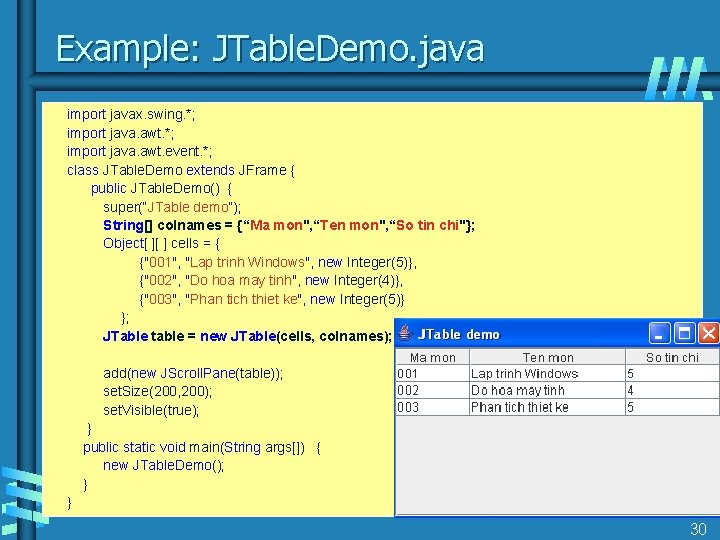
Example: JTable. Demo. java import javax. swing. *; import java. awt. event. *; class JTable. Demo extends JFrame { public JTable. Demo() { super(“JTable demo”); String[] colnames = {“Ma mon", “Ten mon", “So tin chi"}; Object[ ][ ] cells = { {"001", "Lap trinh Windows", new Integer(5)}, {"002", "Do hoa may tinh", new Integer(4)}, {"003", "Phan tich thiet ke", new Integer(5)} }; JTable table = new JTable(cells, colnames); add(new JScroll. Pane(table)); set. Size(200, 200); set. Visible(true); } public static void main(String args[]) { new JTable. Demo(); } } 30
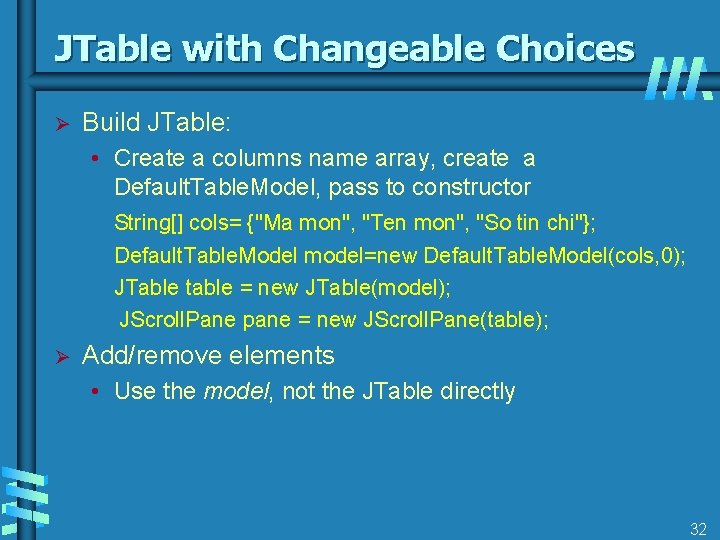
JTable with Changeable Choices Ø Build JTable: • Create a columns name array, create a Default. Table. Model, pass to constructor String[] cols= {"Ma mon", "Ten mon", "So tin chi"}; Default. Table. Model model=new Default. Table. Model(cols, 0); JTable table = new JTable(model); JScroll. Pane pane = new JScroll. Pane(table); Ø Add/remove elements • Use the model, not the JTable directly 32
![Methods in Default Table Model Ø void add RowObject row Data add a Methods in Default. Table. Model Ø void add. Row(Object[] row. Data) • add a](https://slidetodoc.com/presentation_image_h2/f4808711ed7de67909459c043a9af1c7/image-32.jpg)
Methods in Default. Table. Model Ø void add. Row(Object[] row. Data) • add a row of data to the end of the table model Ø void insert. Row(int row, Object[] row. Data) • adds a row of data at index row Ø void remove. Row(int row) • removes the given row from the model 33
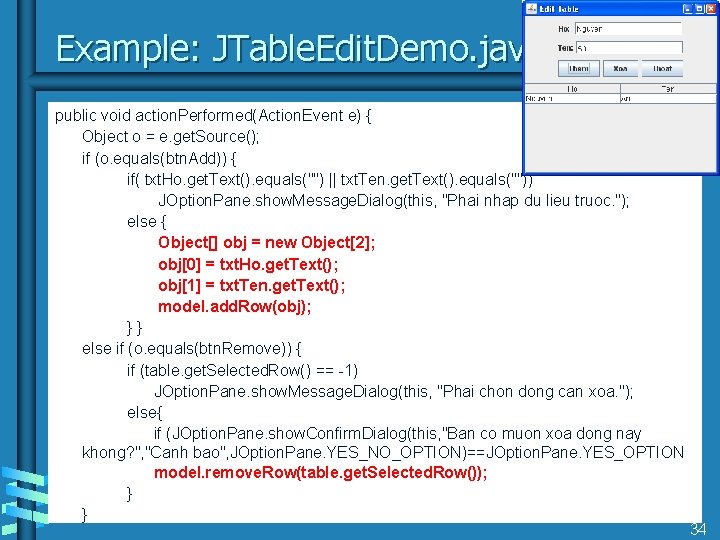
Example: JTable. Edit. Demo. java public void action. Performed(Action. Event e) { Object o = e. get. Source(); if (o. equals(btn. Add)) { if( txt. Ho. get. Text(). equals("") || txt. Ten. get. Text(). equals("")) JOption. Pane. show. Message. Dialog(this, "Phai nhap du lieu truoc. "); else { Object[] obj = new Object[2]; obj[0] = txt. Ho. get. Text(); obj[1] = txt. Ten. get. Text(); model. add. Row(obj); }} else if (o. equals(btn. Remove)) { if (table. get. Selected. Row() == -1) JOption. Pane. show. Message. Dialog(this, "Phai chon dong can xoa. "); else{ if (JOption. Pane. show. Confirm. Dialog(this, "Ban co muon xoa dong nay khong? ", "Canh bao", JOption. Pane. YES_NO_OPTION)==JOption. Pane. YES_OPTION model. remove. Row(table. get. Selected. Row()); } } 34
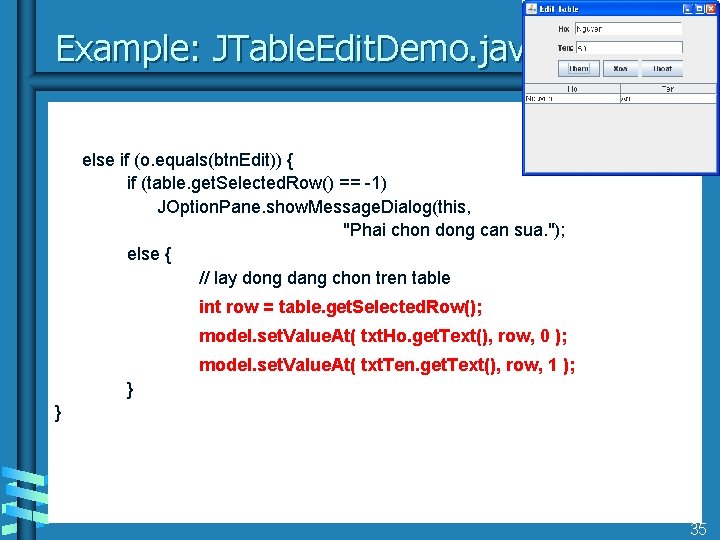
Example: JTable. Edit. Demo. java else if (o. equals(btn. Edit)) { if (table. get. Selected. Row() == -1) JOption. Pane. show. Message. Dialog(this, "Phai chon dong can sua. "); else { // lay dong dang chon tren table int row = table. get. Selected. Row(); model. set. Value. At( txt. Ho. get. Text(), row, 0 ); model. set. Value. At( txt. Ten. get. Text(), row, 1 ); } } 35
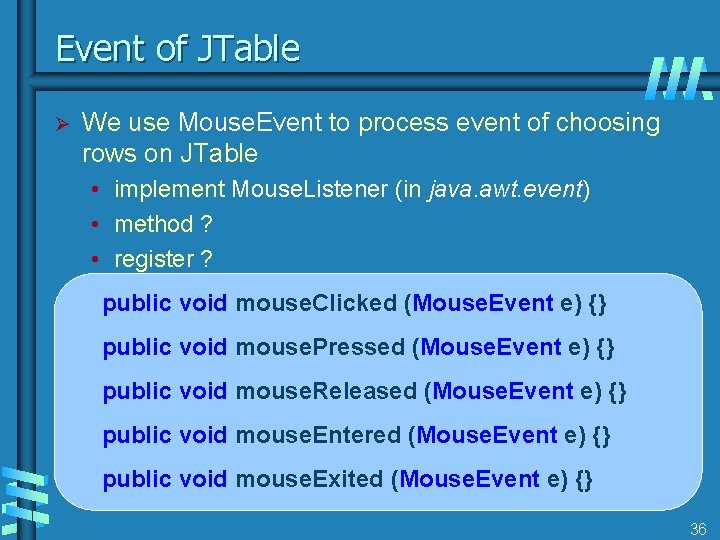
Event of JTable Ø We use Mouse. Event to process event of choosing rows on JTable • implement Mouse. Listener (in java. awt. event) • method ? • register ? public void mouse. Clicked (Mouse. Event e) {} public void mouse. Pressed (Mouse. Event e) {} public void mouse. Released (Mouse. Event e) {} public void mouse. Entered (Mouse. Event e) {} public void mouse. Exited (Mouse. Event e) {} 36
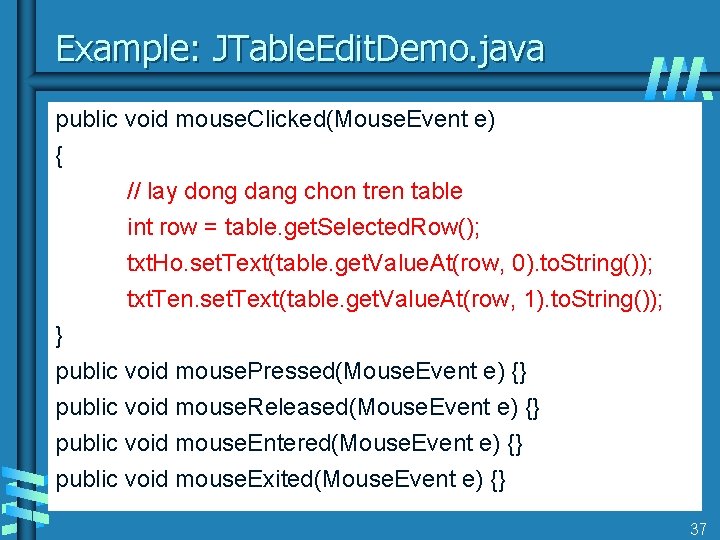
Example: JTable. Edit. Demo. java public void mouse. Clicked(Mouse. Event e) { // lay dong dang chon tren table int row = table. get. Selected. Row(); txt. Ho. set. Text(table. get. Value. At(row, 0). to. String()); txt. Ten. set. Text(table. get. Value. At(row, 1). to. String()); } public void mouse. Pressed(Mouse. Event e) {} public void mouse. Released(Mouse. Event e) {} public void mouse. Entered(Mouse. Event e) {} public void mouse. Exited(Mouse. Event e) {} 37
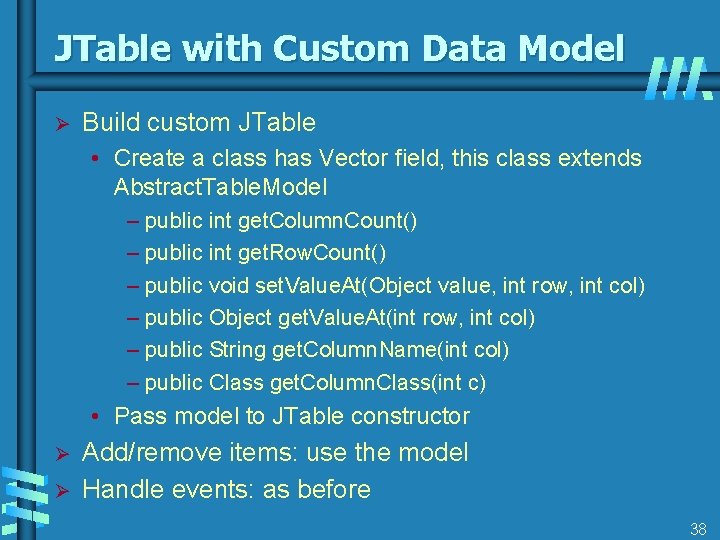
JTable with Custom Data Model Ø Build custom JTable • Create a class has Vector field, this class extends Abstract. Table. Model – public int get. Column. Count() – public int get. Row. Count() – public void set. Value. At(Object value, int row, int col) – public Object get. Value. At(int row, int col) – public String get. Column. Name(int col) – public Class get. Column. Class(int c) • Pass model to JTable constructor Ø Ø Add/remove items: use the model Handle events: as before 38
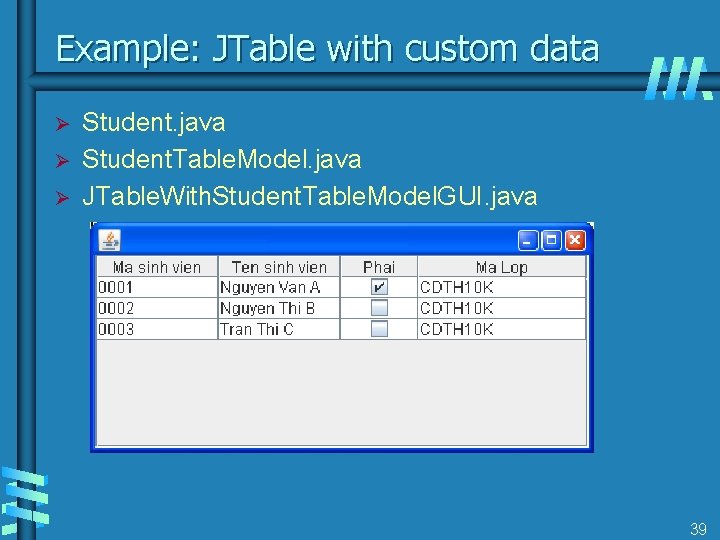
Example: JTable with custom data Ø Ø Ø Student. java Student. Table. Model. java JTable. With. Student. Table. Model. GUI. java 39
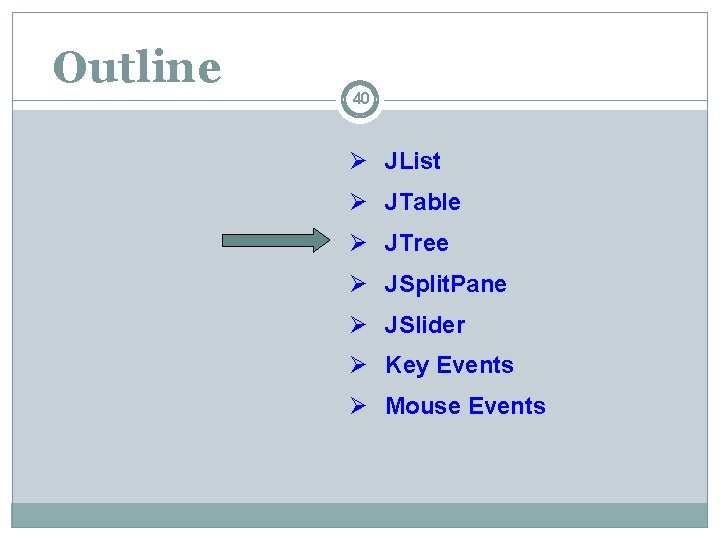
Outline 40 Ø JList Ø JTable Ø JTree Ø JSplit. Pane Ø JSlider Ø Key Events Ø Mouse Events
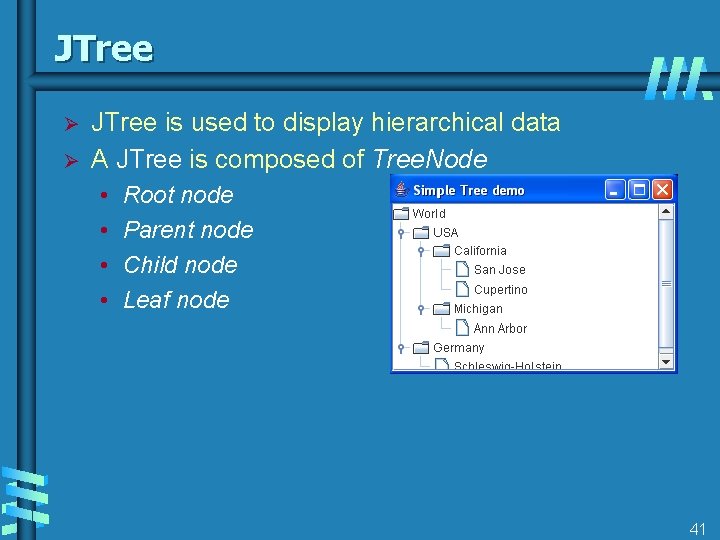
JTree Ø Ø JTree is used to display hierarchical data A JTree is composed of Tree. Node • • Root node Parent node Child node Leaf node 41
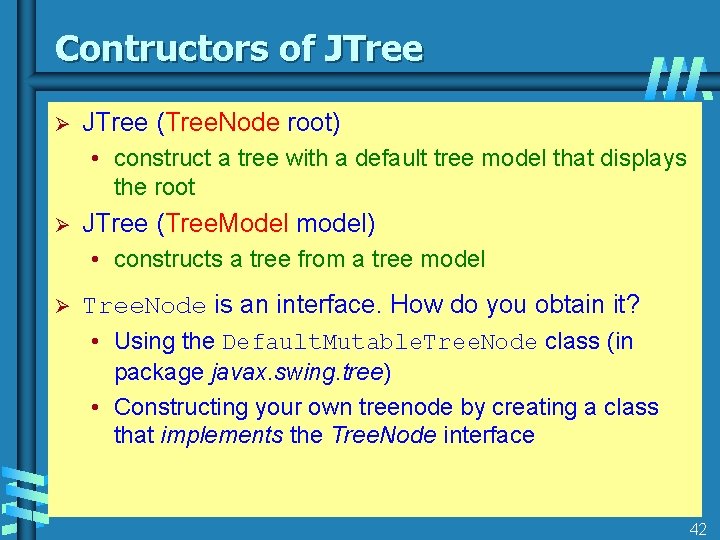
Contructors of JTree Ø JTree (Tree. Node root) • construct a tree with a default tree model that displays the root Ø JTree (Tree. Model model) • constructs a tree from a tree model Ø Tree. Node is an interface. How do you obtain it? • Using the Default. Mutable. Tree. Node class (in package javax. swing. tree) • Constructing your own treenode by creating a class that implements the Tree. Node interface 42
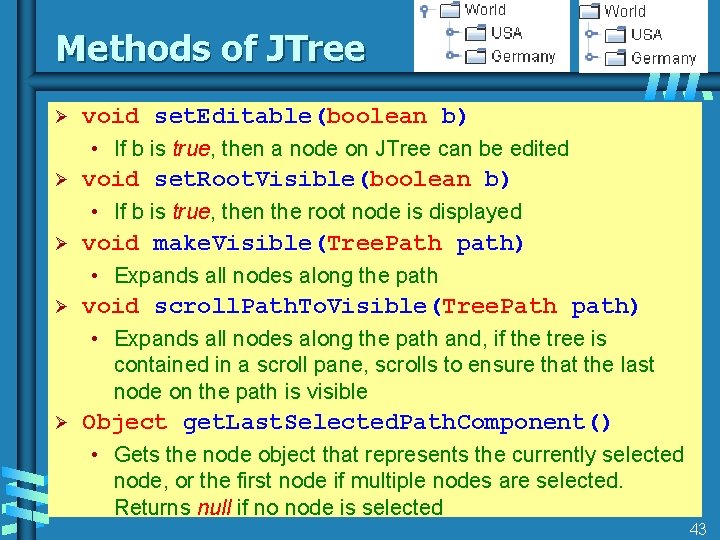
Methods of JTree Ø void set. Editable(boolean b) • If b is true, then a node on JTree can be edited Ø void set. Root. Visible(boolean b) • If b is true, then the root node is displayed Ø void make. Visible(Tree. Path path) • Expands all nodes along the path Ø void scroll. Path. To. Visible(Tree. Path path) • Expands all nodes along the path and, if the tree is contained in a scroll pane, scrolls to ensure that the last node on the path is visible Ø Object get. Last. Selected. Path. Component() • Gets the node object that represents the currently selected node, or the first node if multiple nodes are selected. Returns null if no node is selected 43
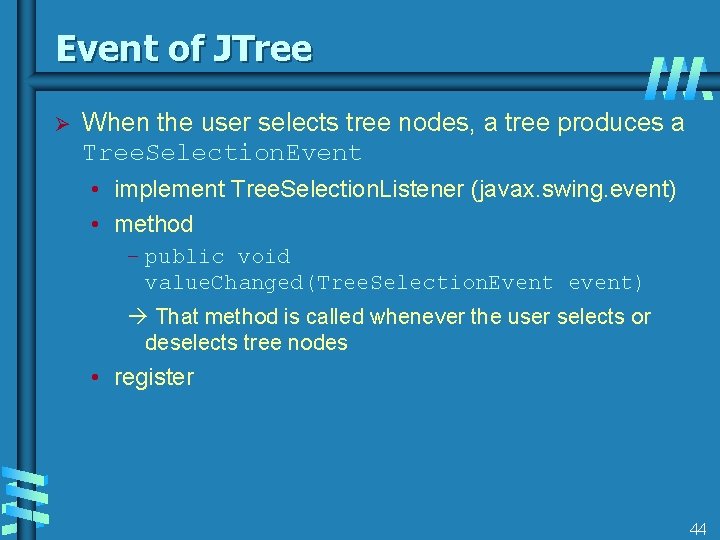
Event of JTree Ø When the user selects tree nodes, a tree produces a Tree. Selection. Event • implement Tree. Selection. Listener (javax. swing. event) • method – public void value. Changed(Tree. Selection. Event event) That method is called whenever the user selects or deselects tree nodes • register 44
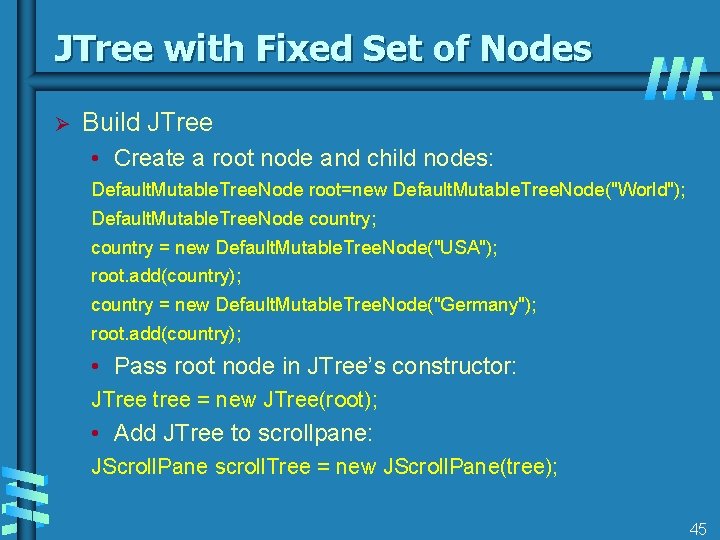
JTree with Fixed Set of Nodes Ø Build JTree • Create a root node and child nodes: Default. Mutable. Tree. Node root=new Default. Mutable. Tree. Node("World"); Default. Mutable. Tree. Node country; country = new Default. Mutable. Tree. Node("USA"); root. add(country); country = new Default. Mutable. Tree. Node("Germany"); root. add(country); • Pass root node in JTree’s constructor: JTree tree = new JTree(root); • Add JTree to scrollpane: JScroll. Pane scroll. Tree = new JScroll. Pane(tree); 45
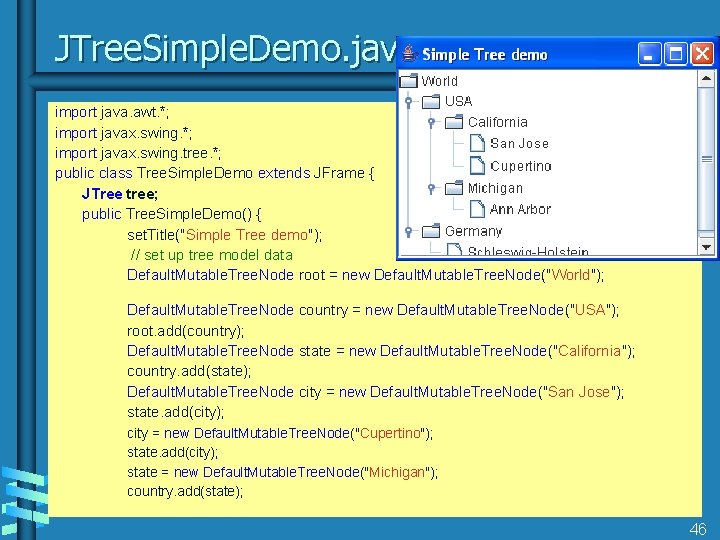
JTree. Simple. Demo. java import java. awt. *; import javax. swing. tree. *; public class Tree. Simple. Demo extends JFrame { JTree tree; public Tree. Simple. Demo() { set. Title("Simple Tree demo"); // set up tree model data Default. Mutable. Tree. Node root = new Default. Mutable. Tree. Node("World"); Default. Mutable. Tree. Node country = new Default. Mutable. Tree. Node("USA"); root. add(country); Default. Mutable. Tree. Node state = new Default. Mutable. Tree. Node("California"); country. add(state); Default. Mutable. Tree. Node city = new Default. Mutable. Tree. Node("San Jose"); state. add(city); city = new Default. Mutable. Tree. Node("Cupertino"); state. add(city); state = new Default. Mutable. Tree. Node("Michigan"); country. add(state); 46
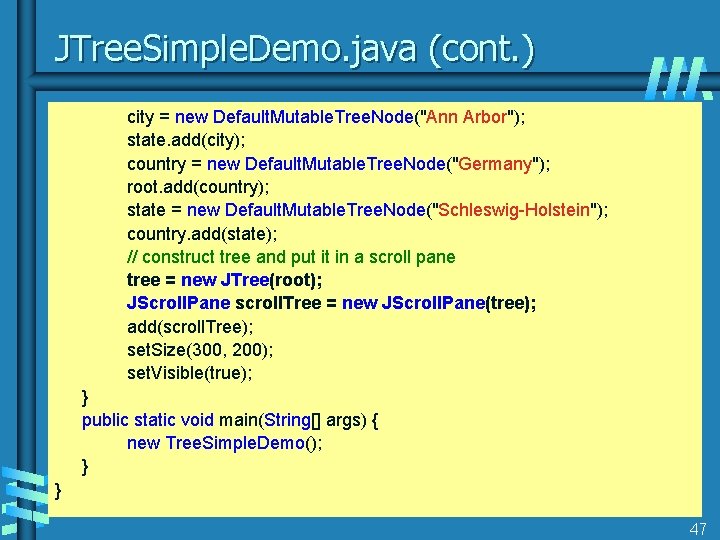
JTree. Simple. Demo. java (cont. ) city = new Default. Mutable. Tree. Node("Ann Arbor"); state. add(city); country = new Default. Mutable. Tree. Node("Germany"); root. add(country); state = new Default. Mutable. Tree. Node("Schleswig-Holstein"); country. add(state); // construct tree and put it in a scroll pane tree = new JTree(root); JScroll. Pane scroll. Tree = new JScroll. Pane(tree); add(scroll. Tree); set. Size(300, 200); set. Visible(true); } public static void main(String[] args) { new Tree. Simple. Demo(); } } 47
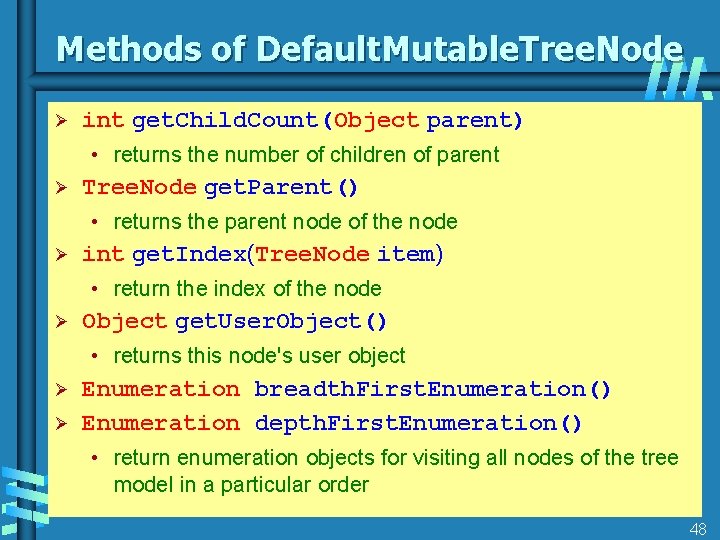
Methods of Default. Mutable. Tree. Node Ø int get. Child. Count(Object parent) • returns the number of children of parent Ø Tree. Node get. Parent() • returns the parent node of the node Ø int get. Index(Tree. Node item) • return the index of the node Ø Object get. User. Object() • returns this node's user object Ø Ø Enumeration breadth. First. Enumeration() Enumeration depth. First. Enumeration() • return enumeration objects for visiting all nodes of the tree model in a particular order 48
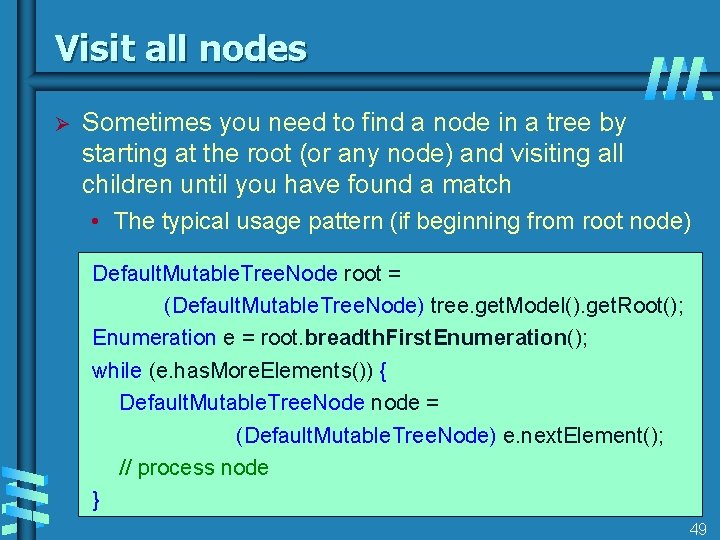
Visit all nodes Ø Sometimes you need to find a node in a tree by starting at the root (or any node) and visiting all children until you have found a match • The typical usage pattern (if beginning from root node) Default. Mutable. Tree. Node root = (Default. Mutable. Tree. Node) tree. get. Model(). get. Root(); Enumeration e = root. breadth. First. Enumeration(); while (e. has. More. Elements()) { Default. Mutable. Tree. Node node = (Default. Mutable. Tree. Node) e. next. Element(); // process node } 49
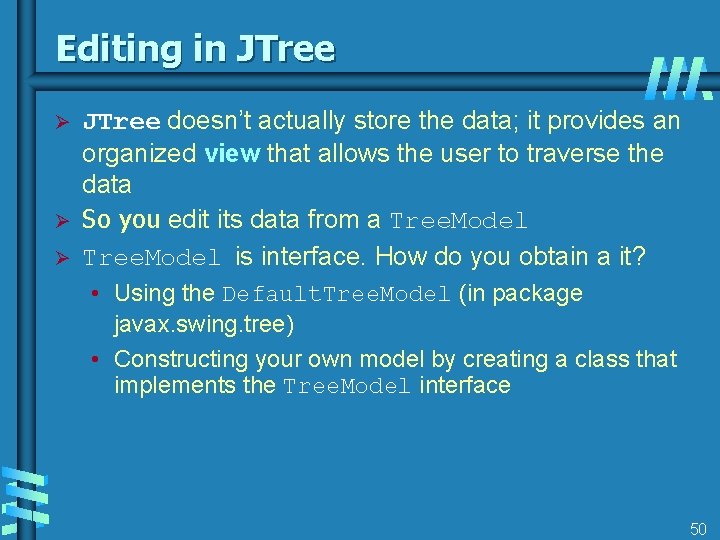
Editing in JTree Ø Ø Ø JTree doesn’t actually store the data; it provides an organized view that allows the user to traverse the data So you edit its data from a Tree. Model is interface. How do you obtain a it? • Using the Default. Tree. Model (in package javax. swing. tree) • Constructing your own model by creating a class that implements the Tree. Model interface 50
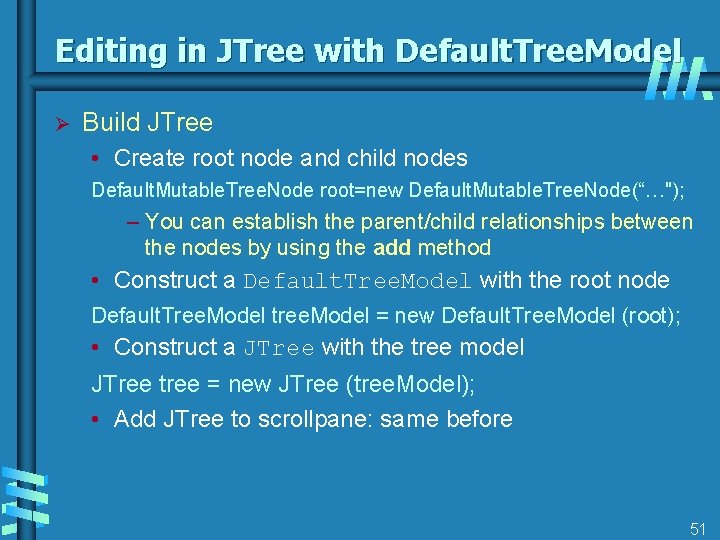
Editing in JTree with Default. Tree. Model Ø Build JTree • Create root node and child nodes Default. Mutable. Tree. Node root=new Default. Mutable. Tree. Node(“…"); – You can establish the parent/child relationships between the nodes by using the add method • Construct a Default. Tree. Model with the root node Default. Tree. Model tree. Model = new Default. Tree. Model (root); • Construct a JTree with the tree model JTree tree = new JTree (tree. Model); • Add JTree to scrollpane: same before 51
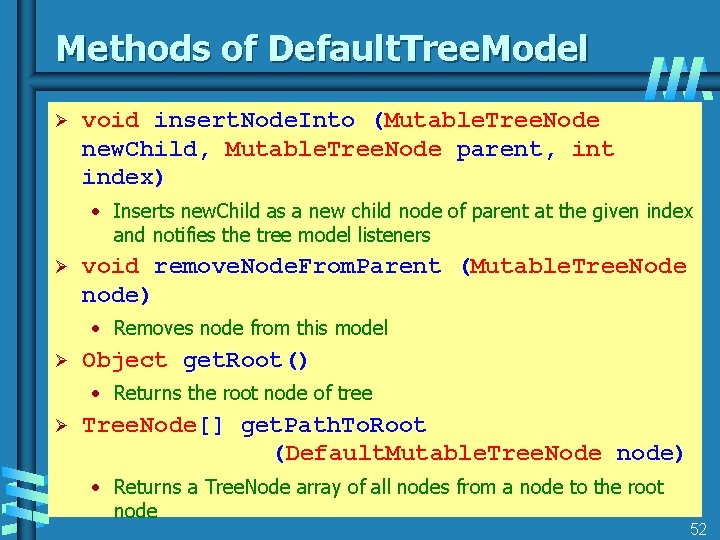
Methods of Default. Tree. Model Ø void insert. Node. Into (Mutable. Tree. Node new. Child, Mutable. Tree. Node parent, int index) • Inserts new. Child as a new child node of parent at the given index and notifies the tree model listeners Ø void remove. Node. From. Parent (Mutable. Tree. Node node) • Removes node from this model Ø Object get. Root() • Returns the root node of tree Ø Tree. Node[] get. Path. To. Root (Default. Mutable. Tree. Node node) • Returns a Tree. Node array of all nodes from a node to the root node 52
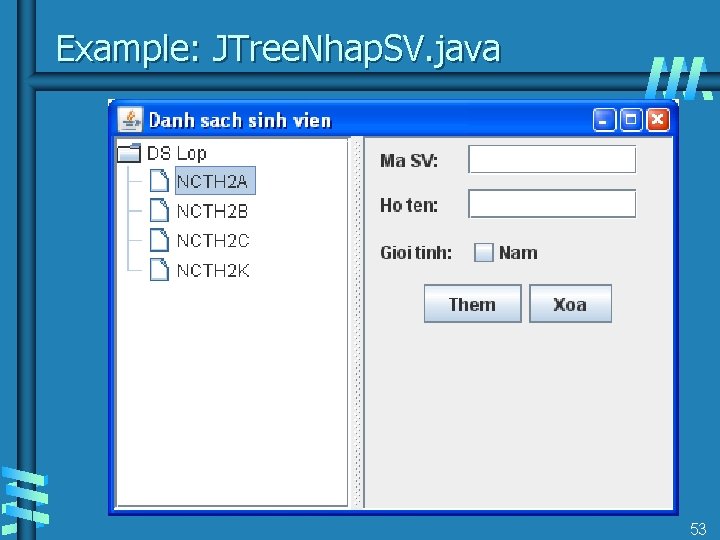
Example: JTree. Nhap. SV. java 53
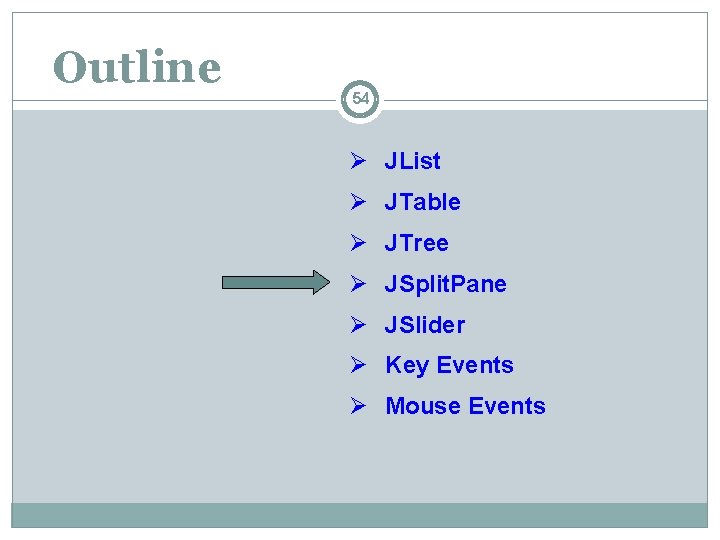
Outline 54 Ø JList Ø JTable Ø JTree Ø JSplit. Pane Ø JSlider Ø Key Events Ø Mouse Events
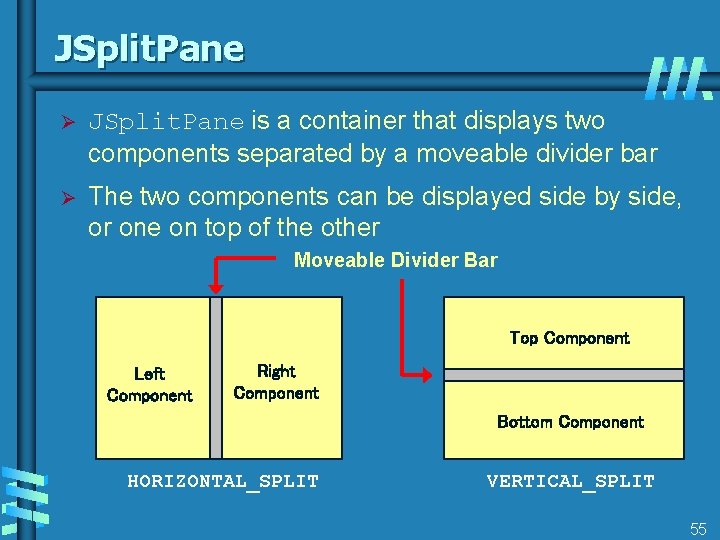
JSplit. Pane Ø JSplit. Pane is a container that displays two components separated by a moveable divider bar Ø The two components can be displayed side by side, or one on top of the other Moveable Divider Bar Top Component Left Component Right Component Bottom Component HORIZONTAL_SPLIT VERTICAL_SPLIT 55
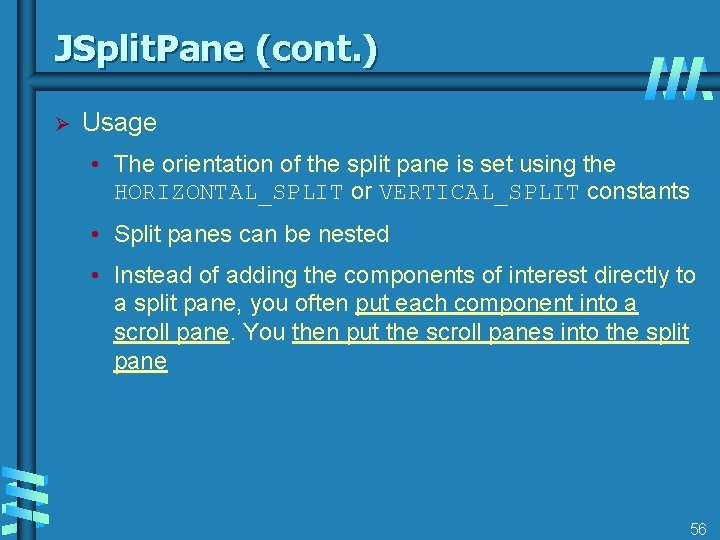
JSplit. Pane (cont. ) Ø Usage • The orientation of the split pane is set using the HORIZONTAL_SPLIT or VERTICAL_SPLIT constants • Split panes can be nested • Instead of adding the components of interest directly to a split pane, you often put each component into a scroll pane. You then put the scroll panes into the split pane 56
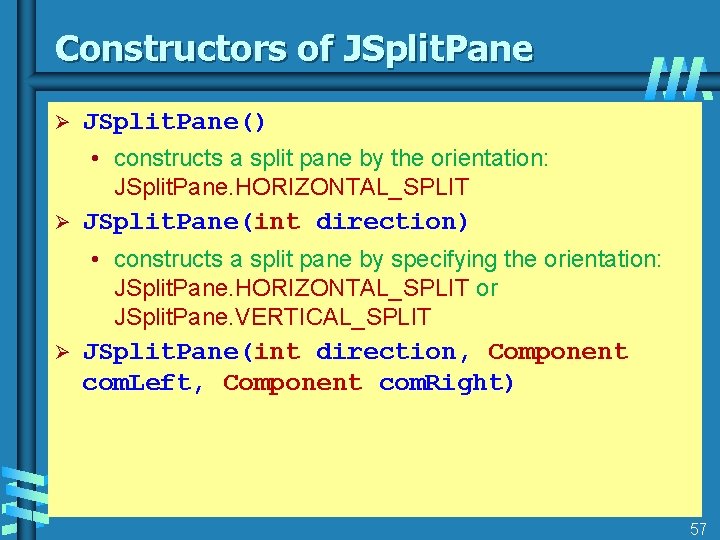
Constructors of JSplit. Pane Ø JSplit. Pane() • constructs a split pane by the orientation: JSplit. Pane. HORIZONTAL_SPLIT Ø JSplit. Pane(int direction) • constructs a split pane by specifying the orientation: JSplit. Pane. HORIZONTAL_SPLIT or JSplit. Pane. VERTICAL_SPLIT Ø JSplit. Pane(int direction, Component com. Left, Component com. Right) 57
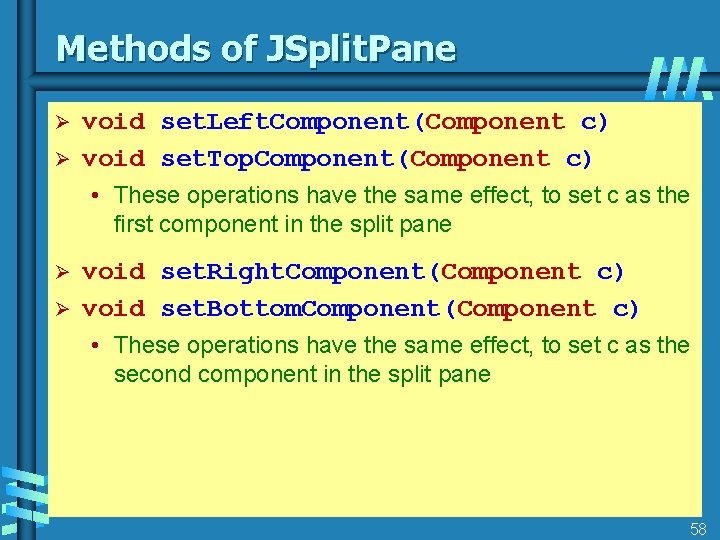
Methods of JSplit. Pane Ø Ø void set. Left. Component(Component c) void set. Top. Component(Component c) • These operations have the same effect, to set c as the first component in the split pane Ø Ø void set. Right. Component(Component c) void set. Bottom. Component(Component c) • These operations have the same effect, to set c as the second component in the split pane 58
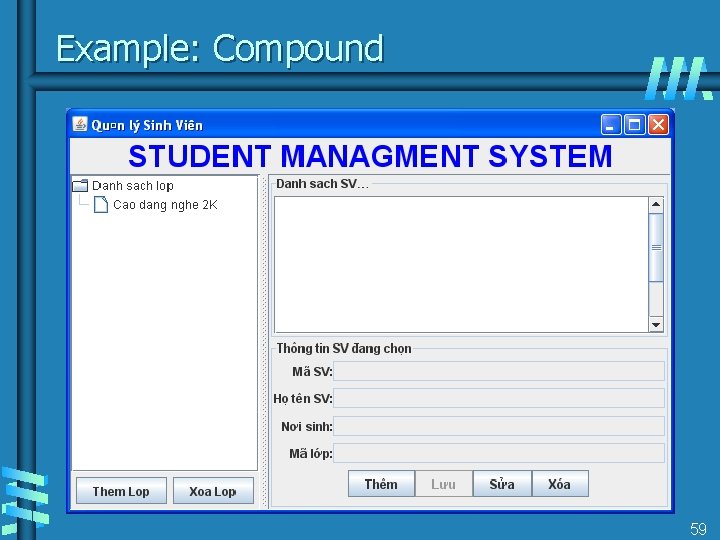
Example: Compound 59
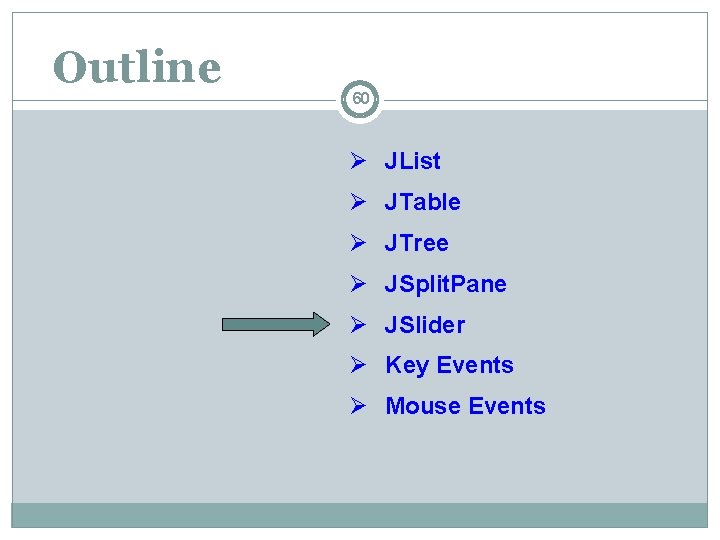
Outline 60 Ø JList Ø JTable Ø JTree Ø JSplit. Pane Ø JSlider Ø Key Events Ø Mouse Events
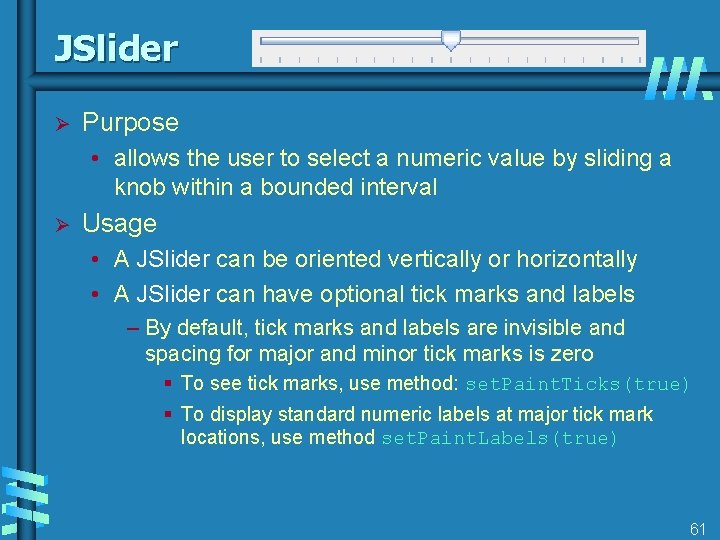
JSlider Ø Purpose • allows the user to select a numeric value by sliding a knob within a bounded interval Ø Usage • A JSlider can be oriented vertically or horizontally • A JSlider can have optional tick marks and labels – By default, tick marks and labels are invisible and spacing for major and minor tick marks is zero § To see tick marks, use method: set. Paint. Ticks(true) § To display standard numeric labels at major tick mark locations, use method set. Paint. Labels(true) 61
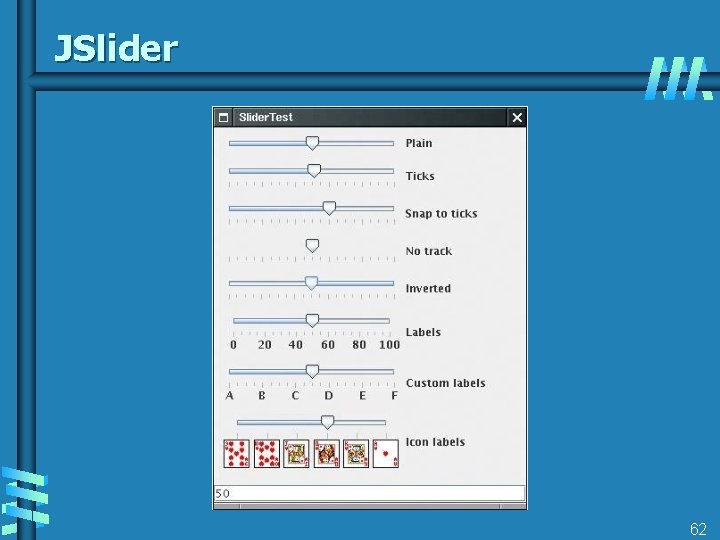
JSlider 62
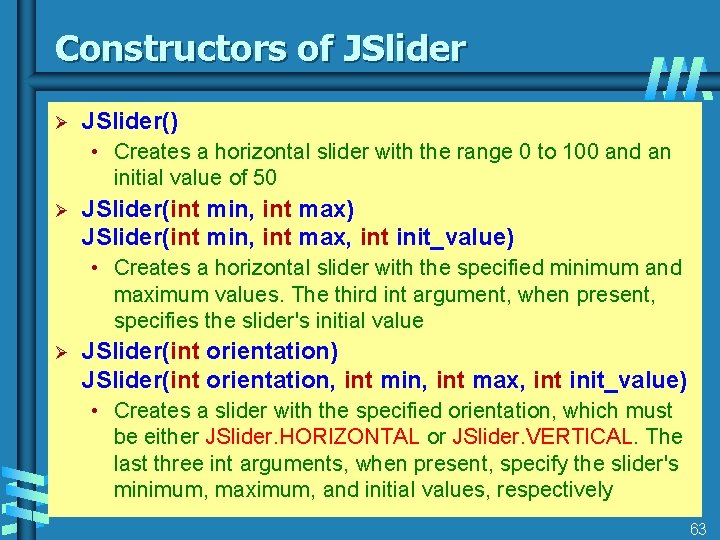
Constructors of JSlider Ø JSlider() • Creates a horizontal slider with the range 0 to 100 and an initial value of 50 Ø JSlider(int min, int max) JSlider(int min, int max, int init_value) • Creates a horizontal slider with the specified minimum and maximum values. The third int argument, when present, specifies the slider's initial value Ø JSlider(int orientation) JSlider(int orientation, int min, int max, int init_value) • Creates a slider with the specified orientation, which must be either JSlider. HORIZONTAL or JSlider. VERTICAL. The last three int arguments, when present, specify the slider's minimum, maximum, and initial values, respectively 63
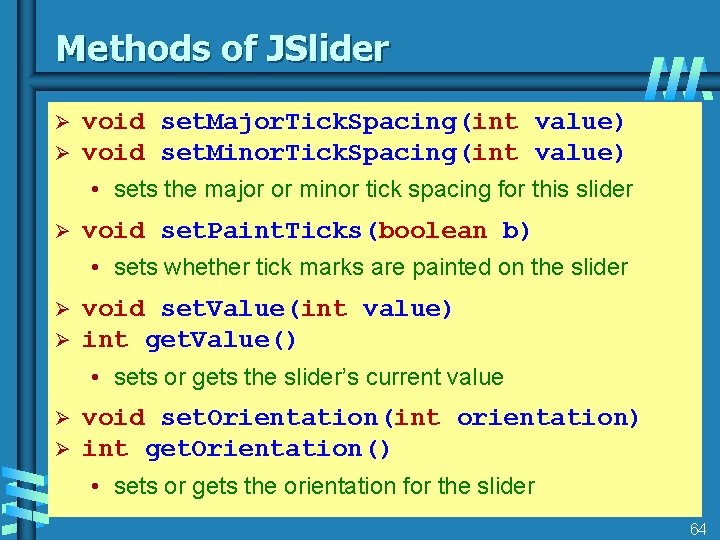
Methods of JSlider Ø Ø void set. Major. Tick. Spacing(int value) void set. Minor. Tick. Spacing(int value) • sets the major or minor tick spacing for this slider Ø void set. Paint. Ticks(boolean b) • sets whether tick marks are painted on the slider Ø Ø void set. Value(int value) int get. Value() • sets or gets the slider’s current value Ø Ø void set. Orientation(int orientation) int get. Orientation() • sets or gets the orientation for the slider 64
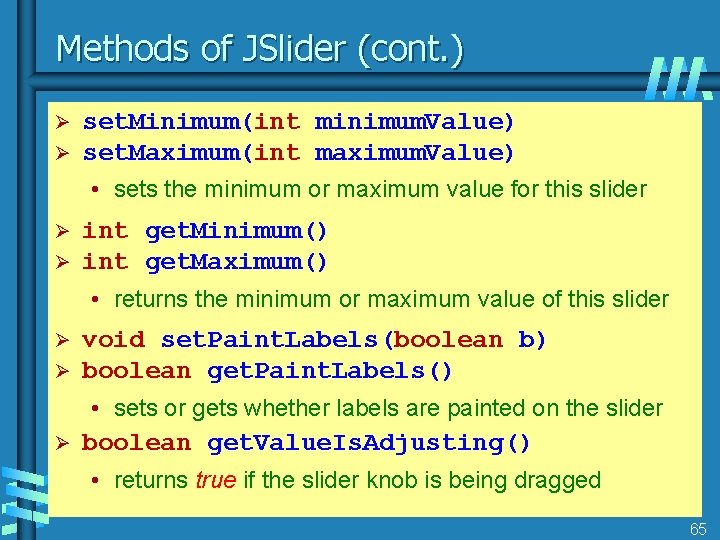
Methods of JSlider (cont. ) Ø Ø set. Minimum(int minimum. Value) set. Maximum(int maximum. Value) • sets the minimum or maximum value for this slider Ø Ø int get. Minimum() int get. Maximum() • returns the minimum or maximum value of this slider Ø Ø void set. Paint. Labels(boolean b) boolean get. Paint. Labels() • sets or gets whether labels are painted on the slider Ø boolean get. Value. Is. Adjusting() • returns true if the slider knob is being dragged 65
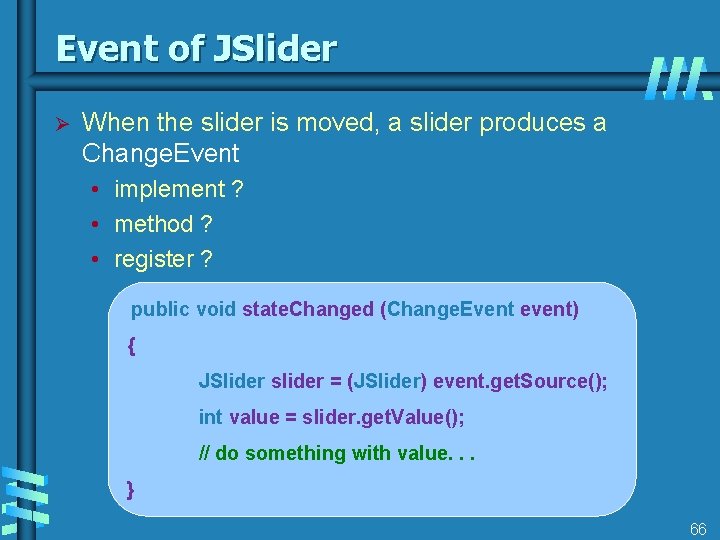
Event of JSlider Ø When the slider is moved, a slider produces a Change. Event • implement ? • method ? • register ? public void state. Changed (Change. Event event) { JSlider slider = (JSlider) event. get. Source(); int value = slider. get. Value(); // do something with value. . . } 66
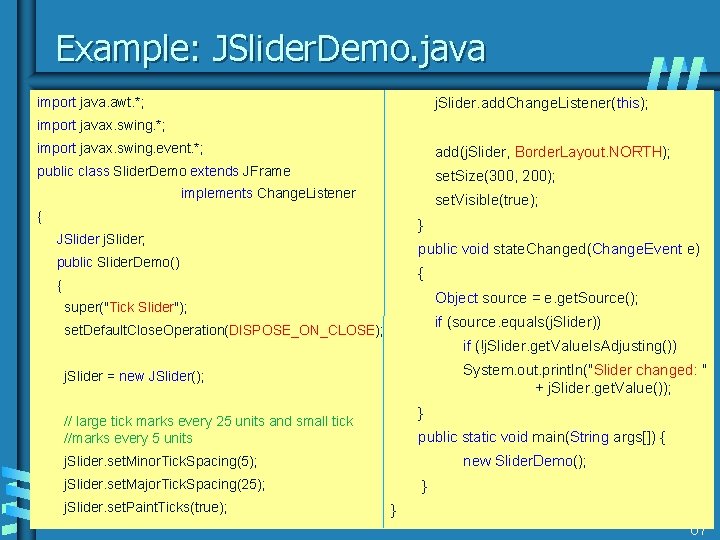
Example: JSlider. Demo. java import java. awt. *; j. Slider. add. Change. Listener(this); import javax. swing. *; import javax. swing. event. *; add(j. Slider, Border. Layout. NORTH); public class Slider. Demo extends JFrame set. Size(300, 200); implements Change. Listener set. Visible(true); { } JSlider j. Slider; public void state. Changed(Change. Event e) public Slider. Demo() { { Object source = e. get. Source(); super("Tick Slider"); if (source. equals(j. Slider)) set. Default. Close. Operation(DISPOSE_ON_CLOSE); if (!j. Slider. get. Value. Is. Adjusting()) System. out. println("Slider changed: " + j. Slider. get. Value()); j. Slider = new JSlider(); } // large tick marks every 25 units and small tick //marks every 5 units public static void main(String args[]) { new Slider. Demo(); j. Slider. set. Minor. Tick. Spacing(5); j. Slider. set. Major. Tick. Spacing(25); j. Slider. set. Paint. Ticks(true); } } 67
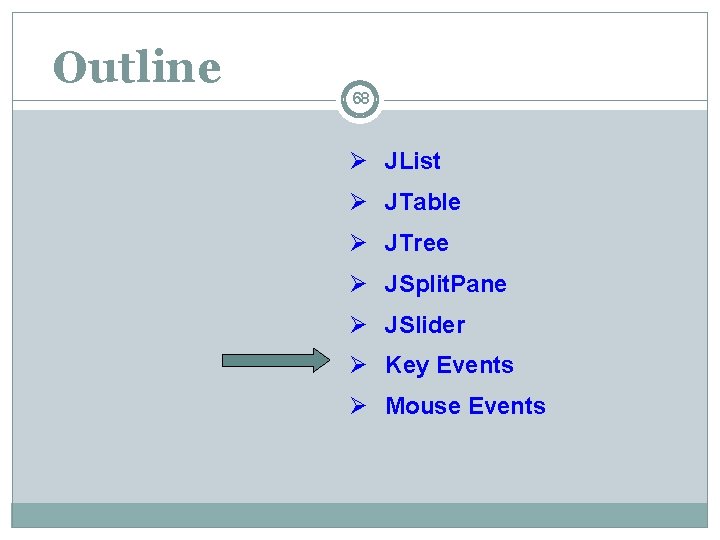
Outline 68 Ø JList Ø JTable Ø JTree Ø JSplit. Pane Ø JSlider Ø Key Events Ø Mouse Events
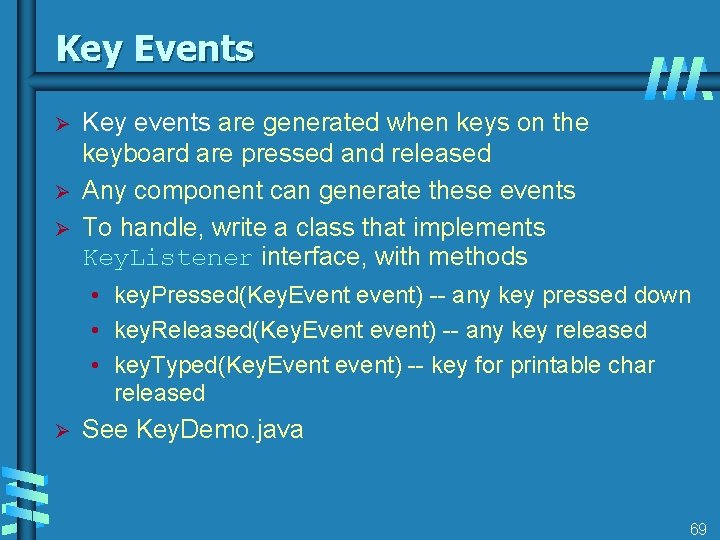
Key Events Ø Ø Ø Key events are generated when keys on the keyboard are pressed and released Any component can generate these events To handle, write a class that implements Key. Listener interface, with methods • key. Pressed(Key. Event event) -- any key pressed down • key. Released(Key. Event event) -- any key released • key. Typed(Key. Event event) -- key for printable char released Ø See Key. Demo. java 69
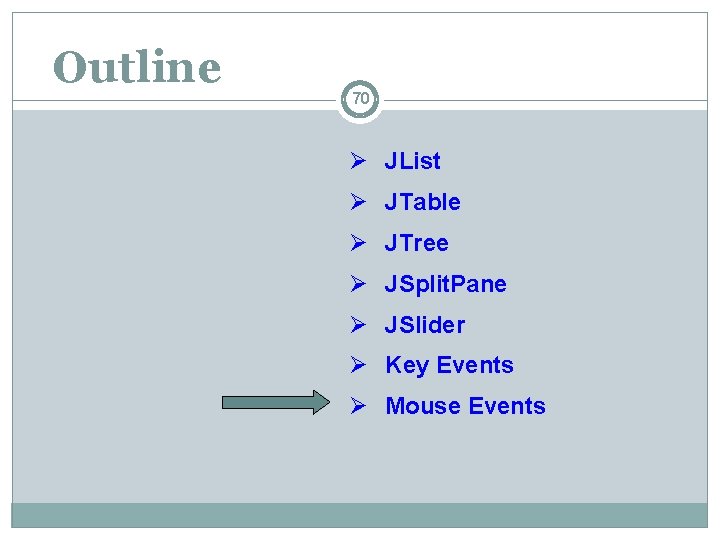
Outline 70 Ø JList Ø JTable Ø JTree Ø JSplit. Pane Ø JSlider Ø Key Events Ø Mouse Events
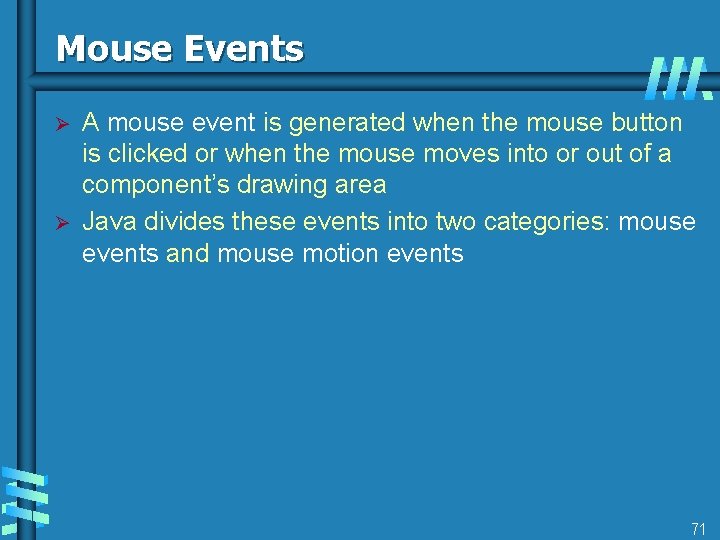
Mouse Events Ø Ø A mouse event is generated when the mouse button is clicked or when the mouse moves into or out of a component’s drawing area Java divides these events into two categories: mouse events and mouse motion events 71
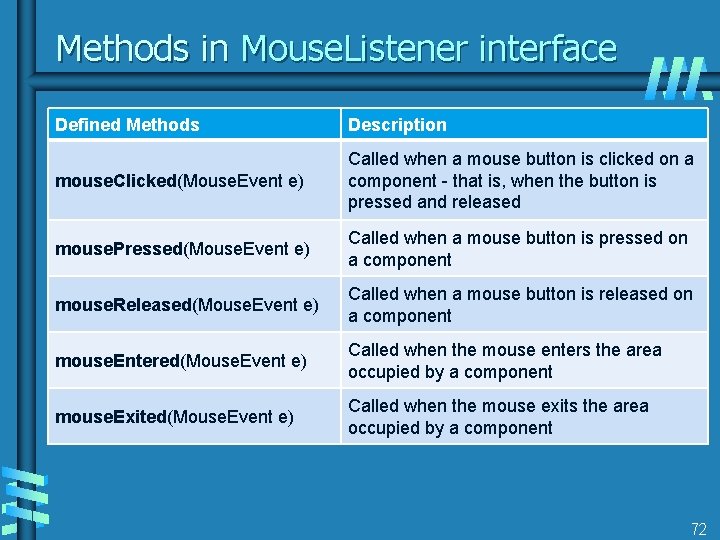
Methods in Mouse. Listener interface Defined Methods Description mouse. Clicked(Mouse. Event e) Called when a mouse button is clicked on a component - that is, when the button is pressed and released mouse. Pressed(Mouse. Event e) Called when a mouse button is pressed on a component mouse. Released(Mouse. Event e) Called when a mouse button is released on a component mouse. Entered(Mouse. Event e) Called when the mouse enters the area occupied by a component mouse. Exited(Mouse. Event e) Called when the mouse exits the area occupied by a component 72
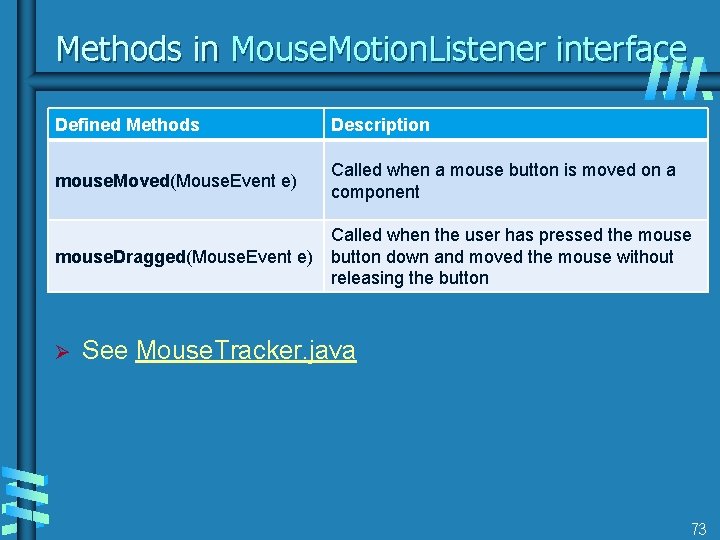
Methods in Mouse. Motion. Listener interface Defined Methods Description mouse. Moved(Mouse. Event e) Called when a mouse button is moved on a component Called when the user has pressed the mouse. Dragged(Mouse. Event e) button down and moved the mouse without releasing the button Ø See Mouse. Tracker. java 73
Swing mvc example
Mvc java swing
Swing mvc example
Orginal markz
Picasa free
Tom clark confections
A small child slides down the four frictionless slides
Energy conservation quick check
Smx advanced 2017 slides
Smx advanced slides
Spring bean lifecycle
Blackboard mvc
Partial view
Mvc muster
Modello mvc
Trygve reenskaug mvc
Jee
What mvc stands for
паттерн mvc java
Mvc-mis
Mvc vs webforms
Mvc architecture in jsp
Mvt vs mvc
Wzorzec projektowy mvc
Mvc paradigm
Struts 2 mvc
Delphi mvc
Asp tutorialspoint
Mvc life cycle in c#
Asp.net mvc 5 identity authentication and authorization
Model layer in mvc
Spring mvc 아이디 찾기
Mvc design pattern java
The mvc will monitor a new driver’s habits for how long?
Obtaining a driver license illegally may result in
Eclipse plugin
V-spring
Spring mvc 로그인
Mvc m
Mvc spencer
Mvc intro
Atc avc afc mc graph
O tero
Trygve reenskaug mvc
Que es el modelo vista controlador
Action selectors in mvc
Asp net core roadmap
Mvc routing
Chapter 6 shielded metal arc welding
Advanced evolution - chapter 4
Advanced evolution chapter 4
Advanced evolution chapter 8
Advanced accounting chapter 1
Merkmale des jazz
Softball ontario rules
Up in the air and over the wall till i can see so wide
Flap valve
Tree swing cartoon construction
Java awt vs swing
Swing scala
Stress equalization in rpd
Event
Awt adalah
Java swing responsive gui
Jazz a swing v české hudbě
Jazz prezentace
Containment in java
Jcheck box
Saját képek
Java gui libraries
Java jlabel wrap text
Barry liked playing board games
Swing lock partial denture