Chapter 7 Expressions and Assignment Statements Chapter 7
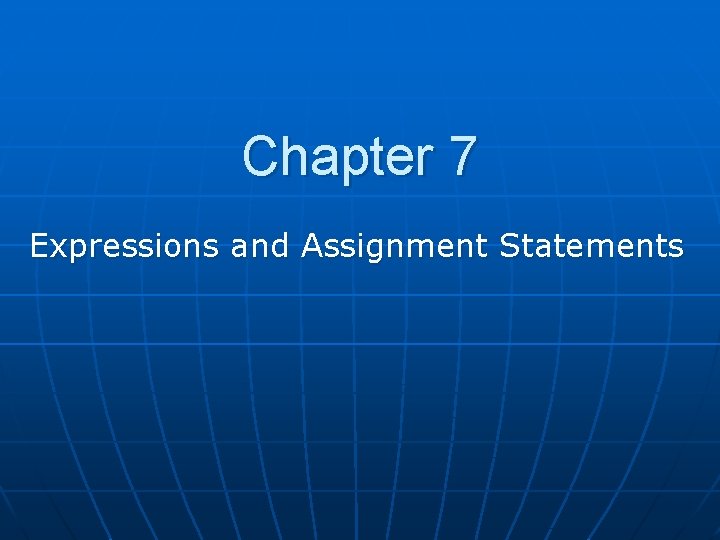
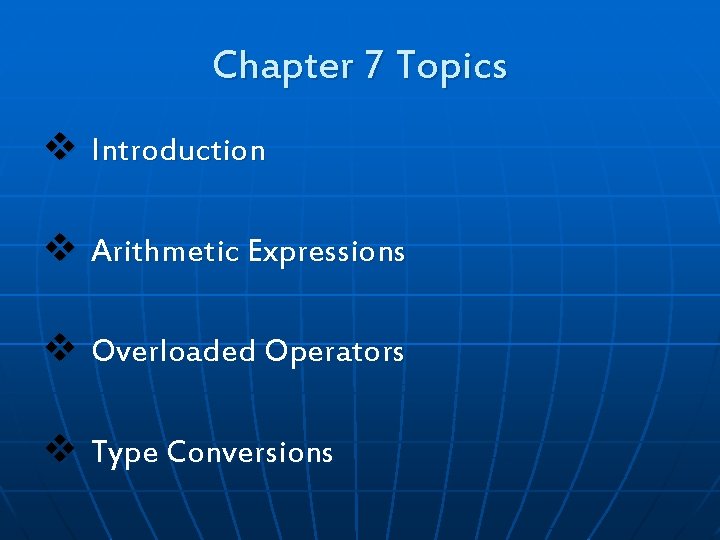
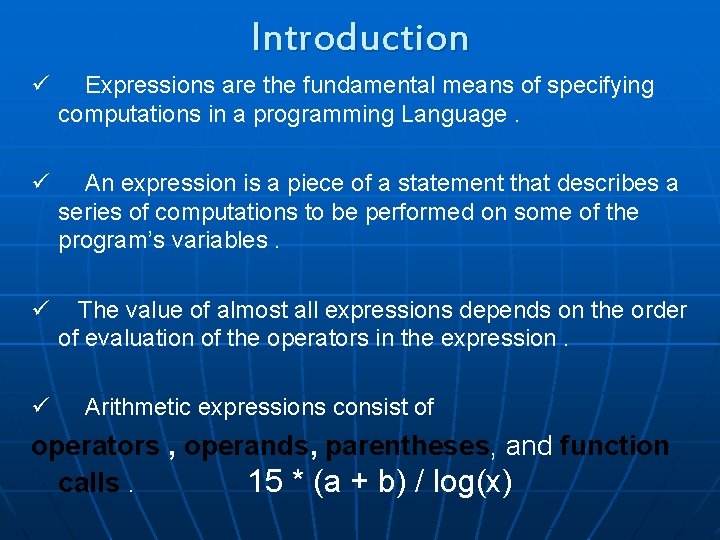
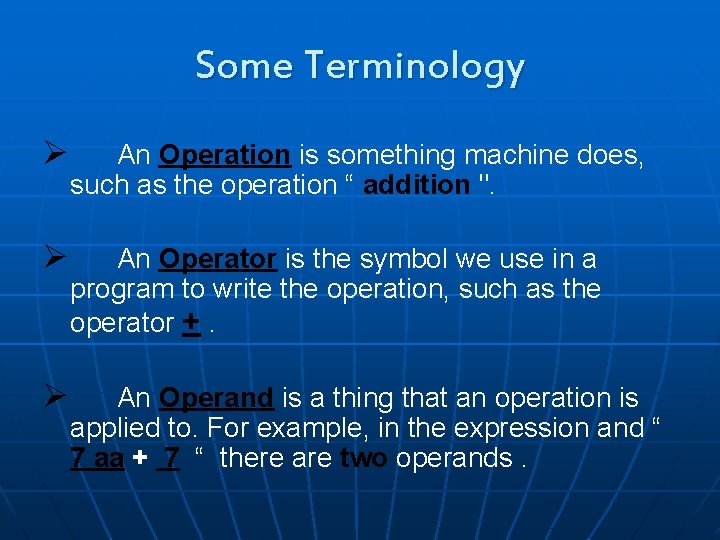
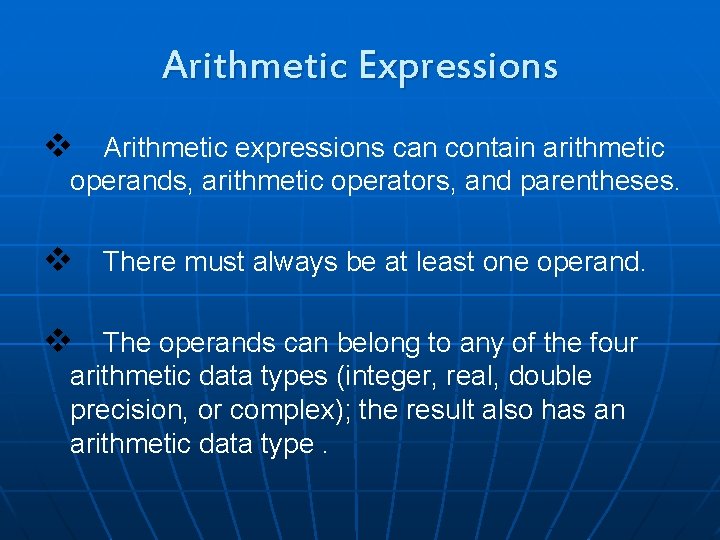
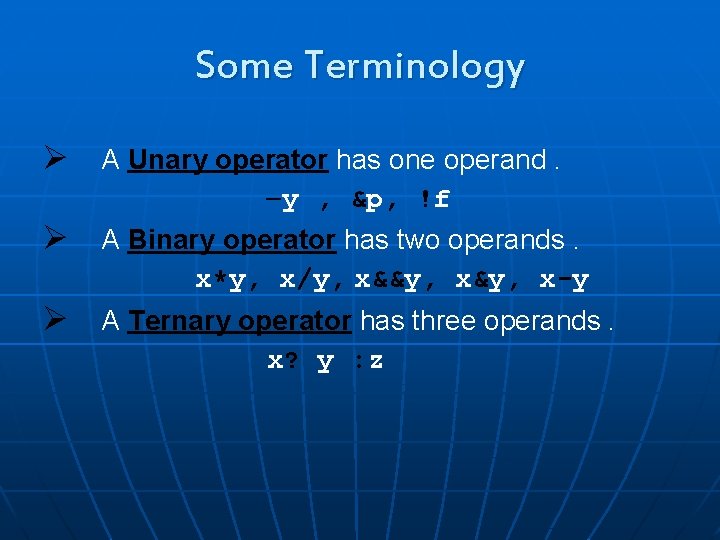
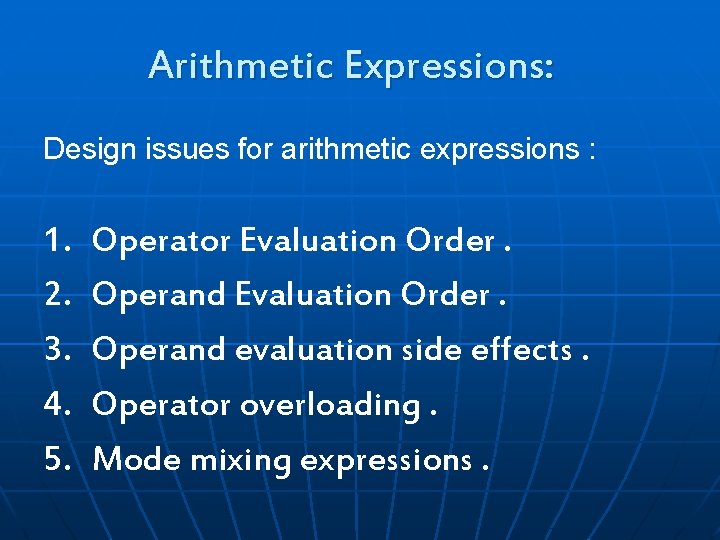
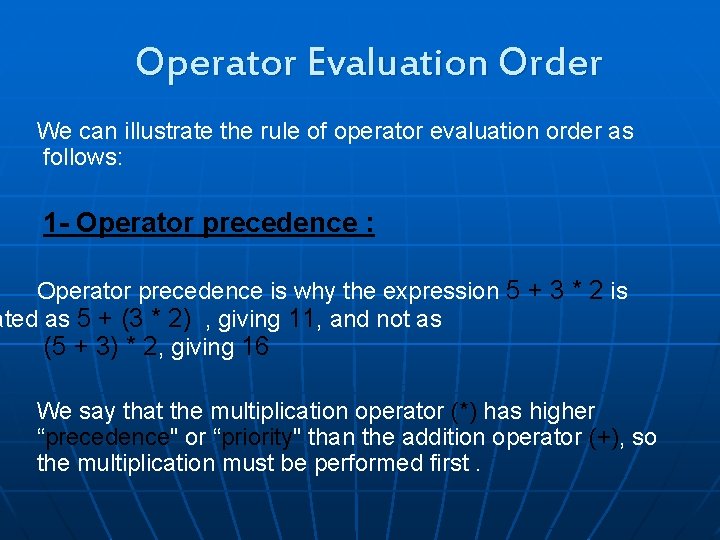
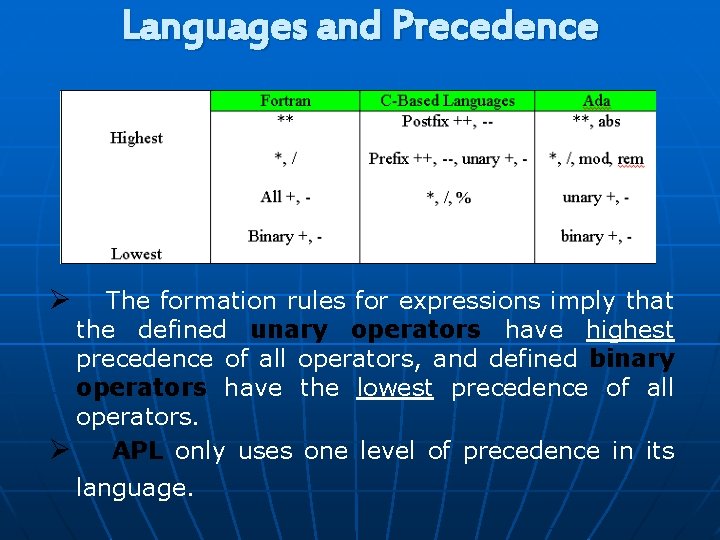
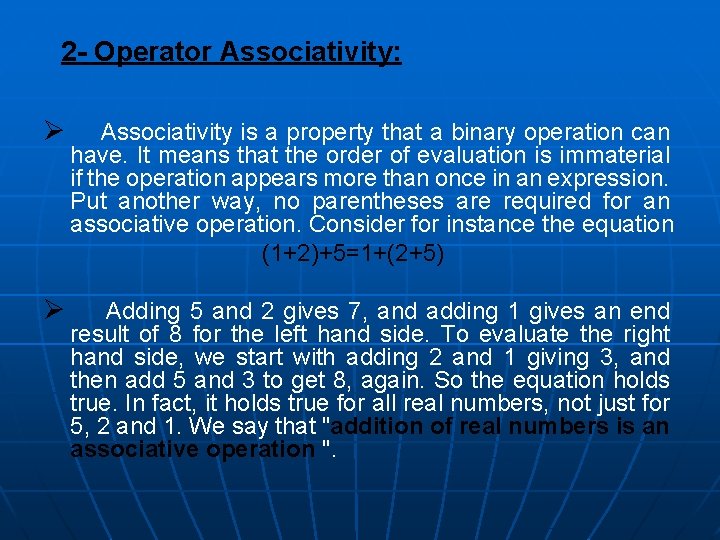
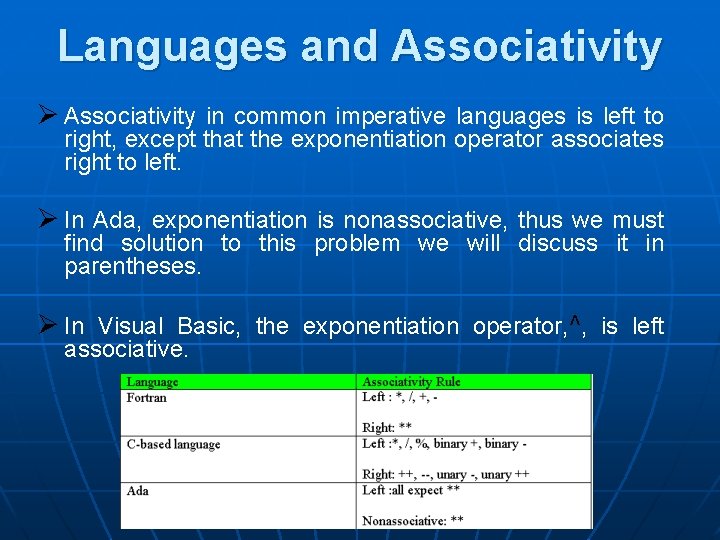
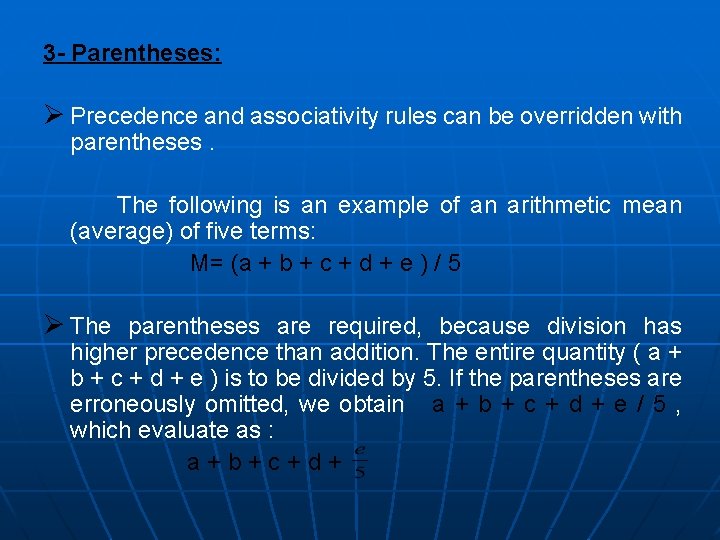
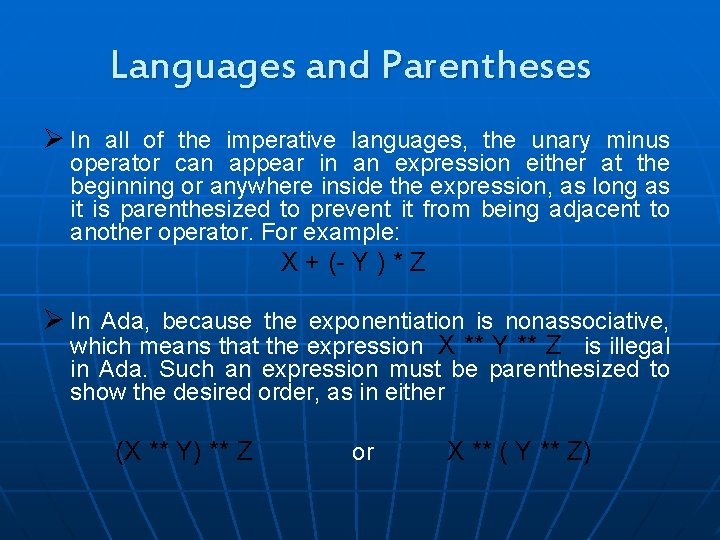
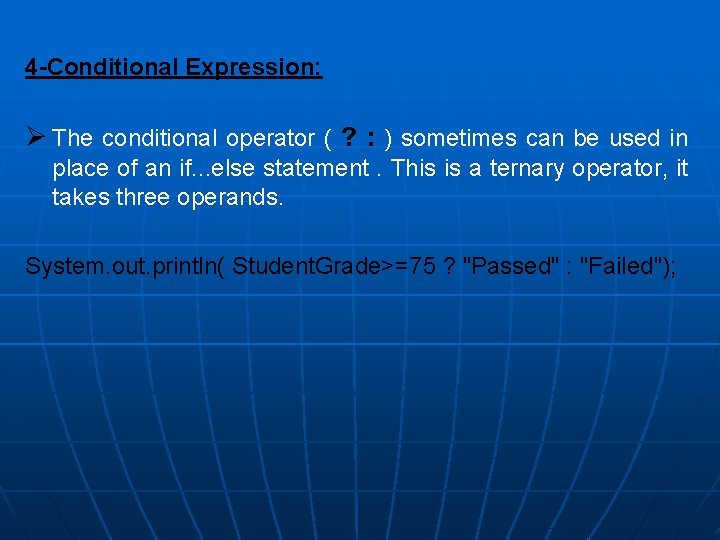
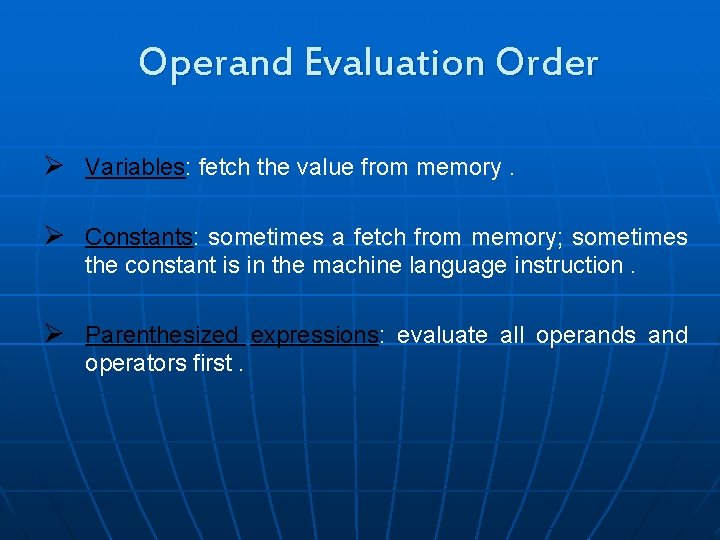
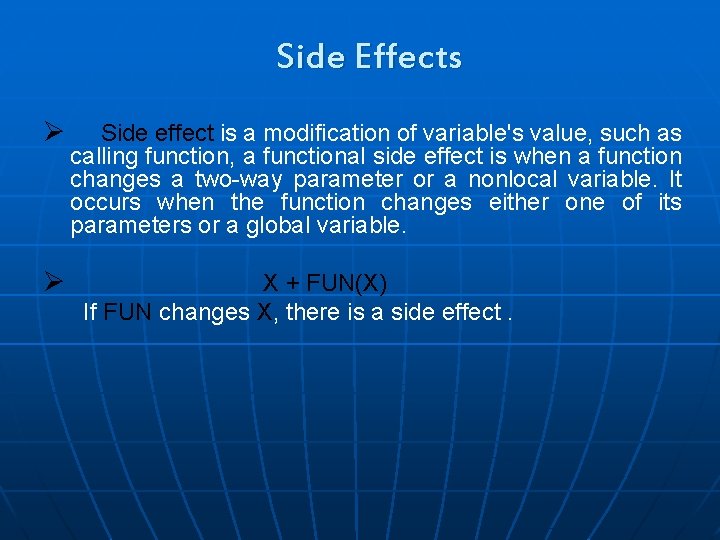
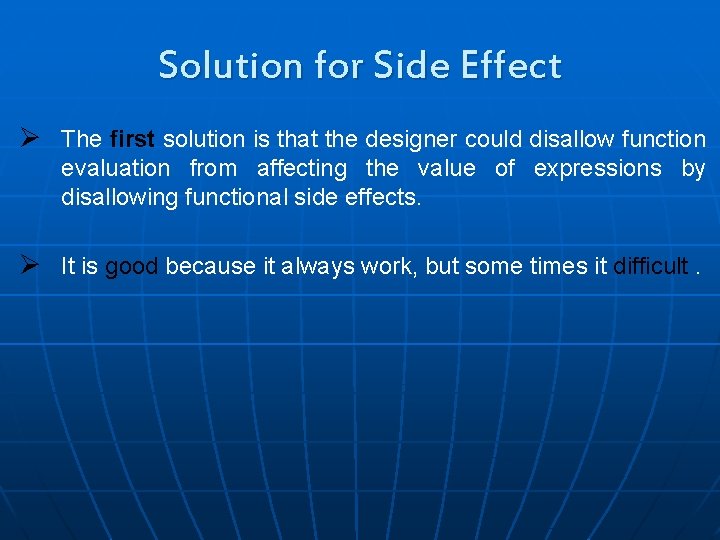
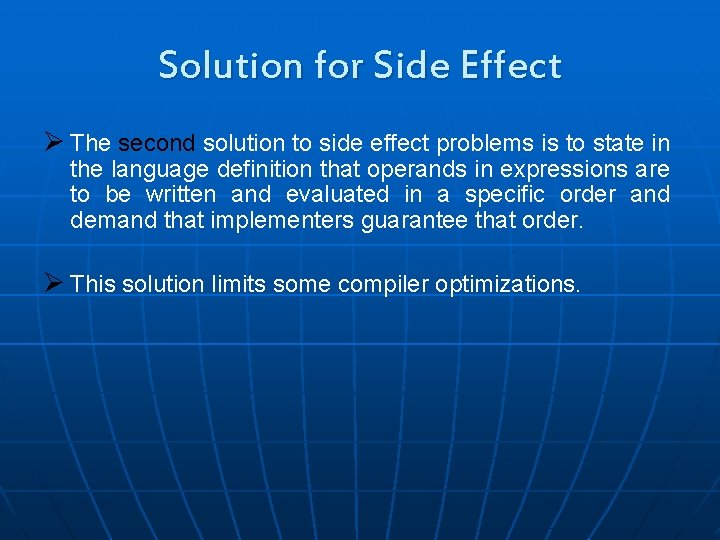
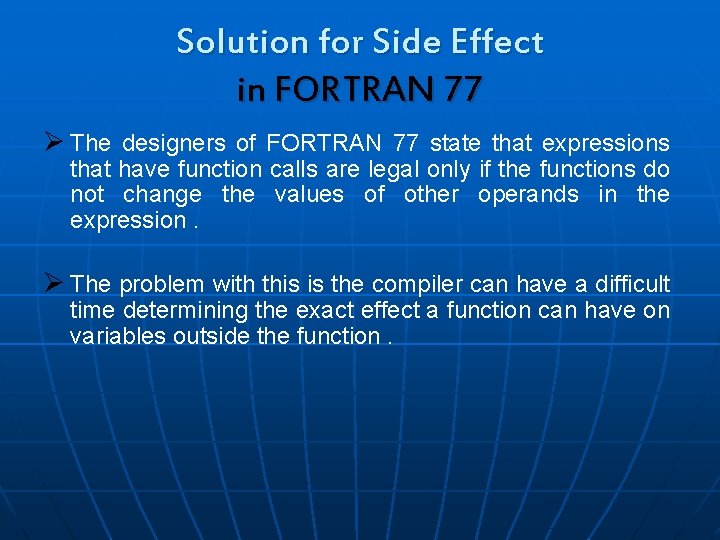
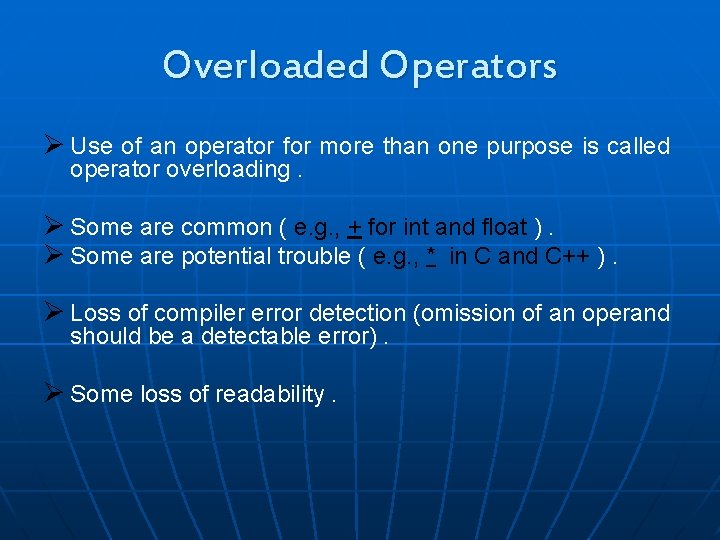
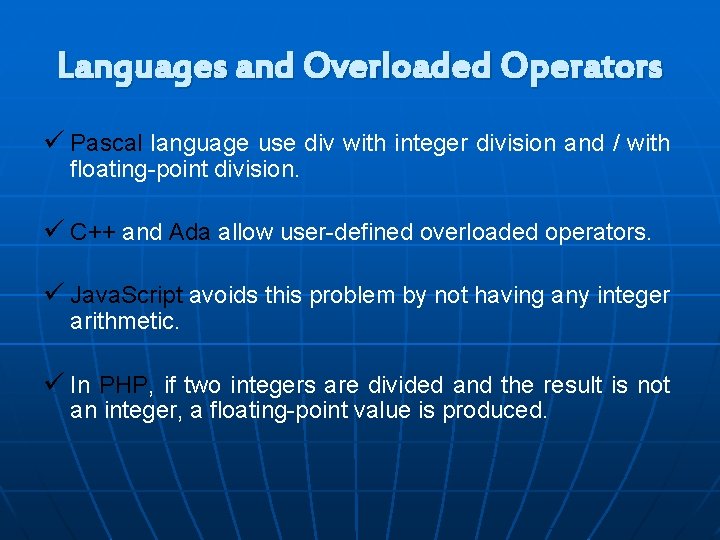
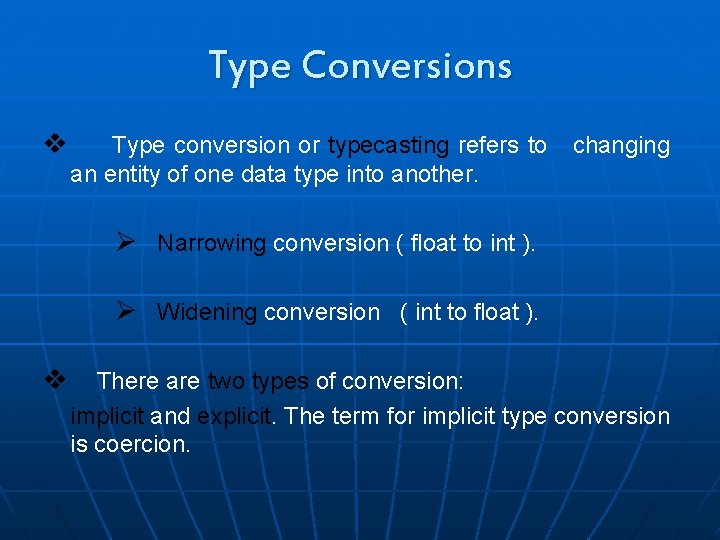
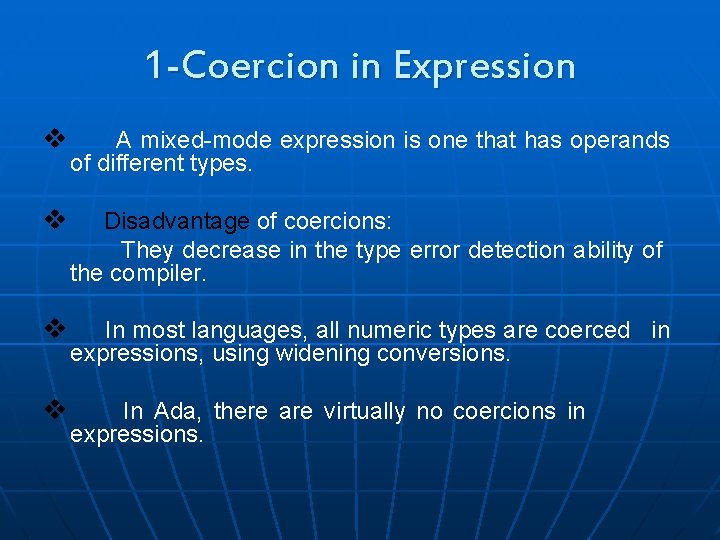
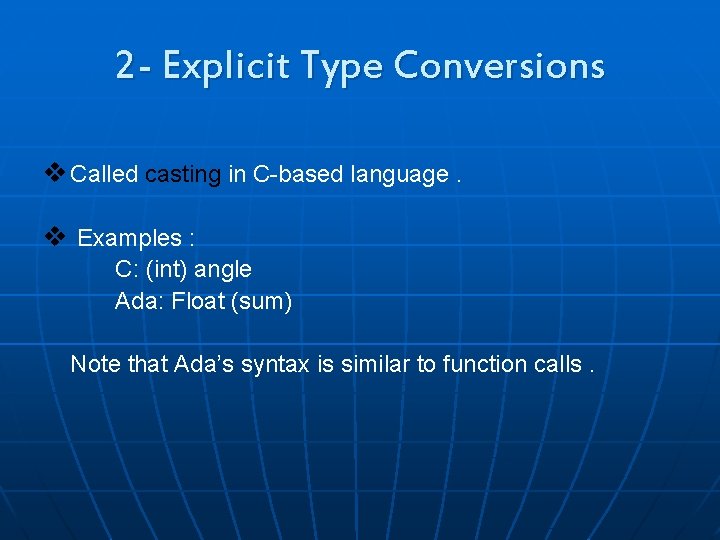
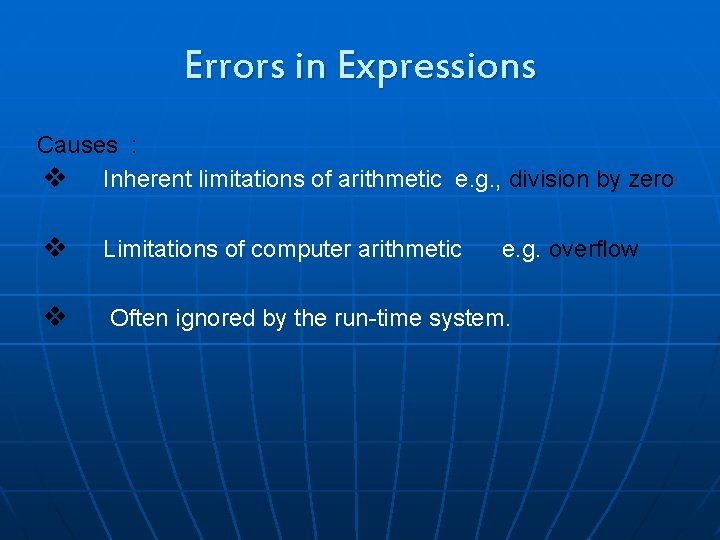
- Slides: 25
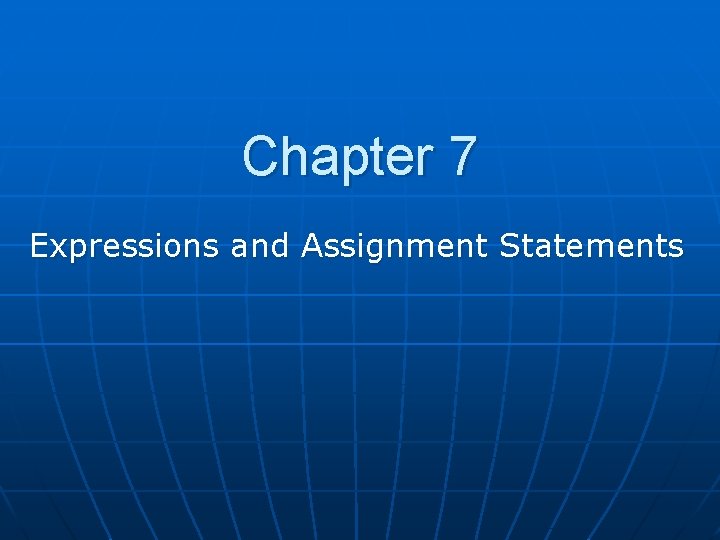
Chapter 7 Expressions and Assignment Statements
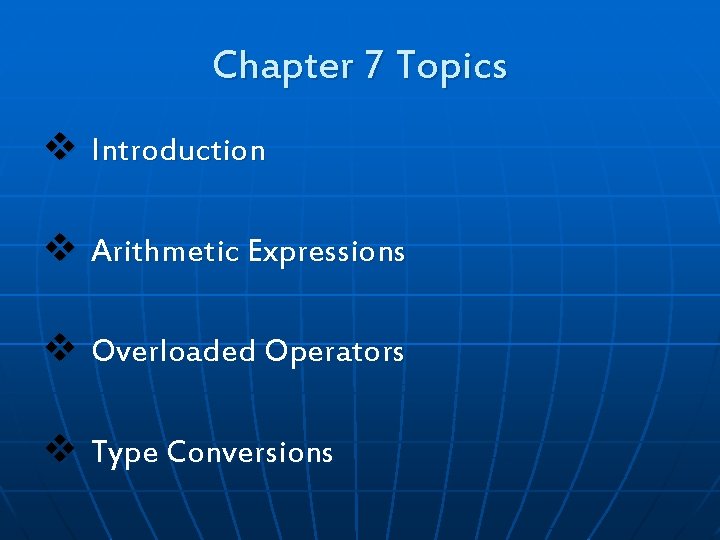
Chapter 7 Topics v Introduction v Arithmetic Expressions v Overloaded Operators v Type Conversions
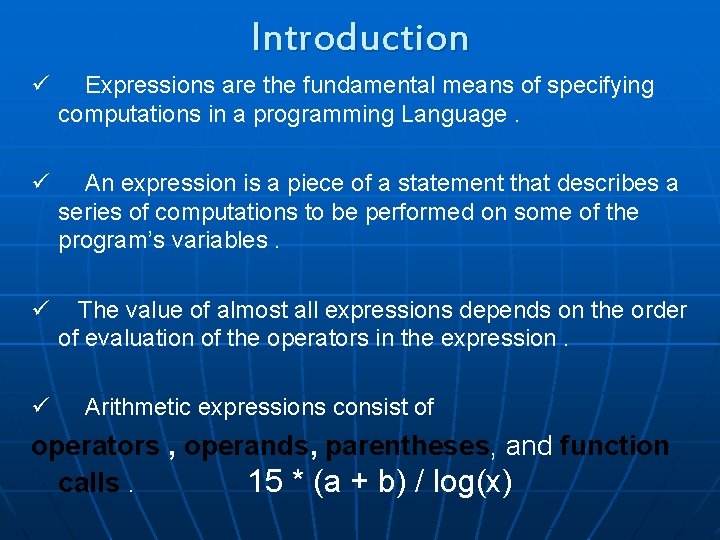
Introduction ü Expressions are the fundamental means of specifying computations in a programming Language. ü An expression is a piece of a statement that describes a series of computations to be performed on some of the program’s variables. ü The value of almost all expressions depends on the order of evaluation of the operators in the expression. ü Arithmetic expressions consist of operators , operands, parentheses, and function calls. 15 * (a + b) / log(x)
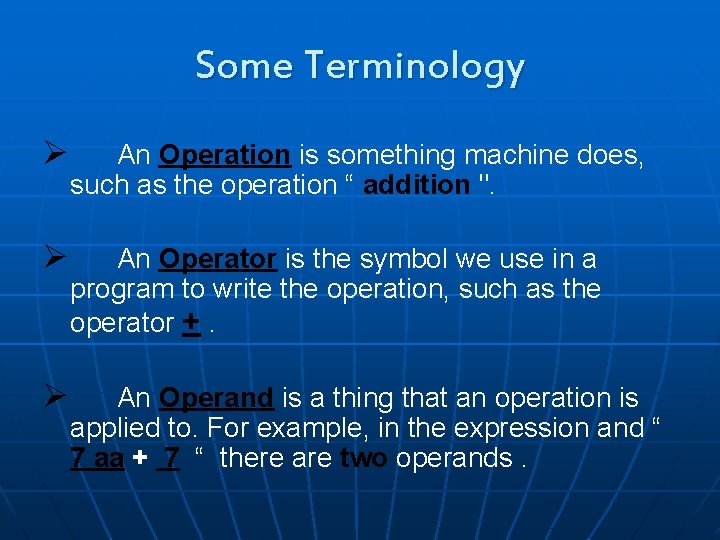
Some Terminology Ø An Operation is something machine does, such as the operation “ addition ". Ø An Operator is the symbol we use in a program to write the operation, such as the operator +. Ø An Operand is a thing that an operation is applied to. For example, in the expression and “ 7 aa + 7 “ there are two operands.
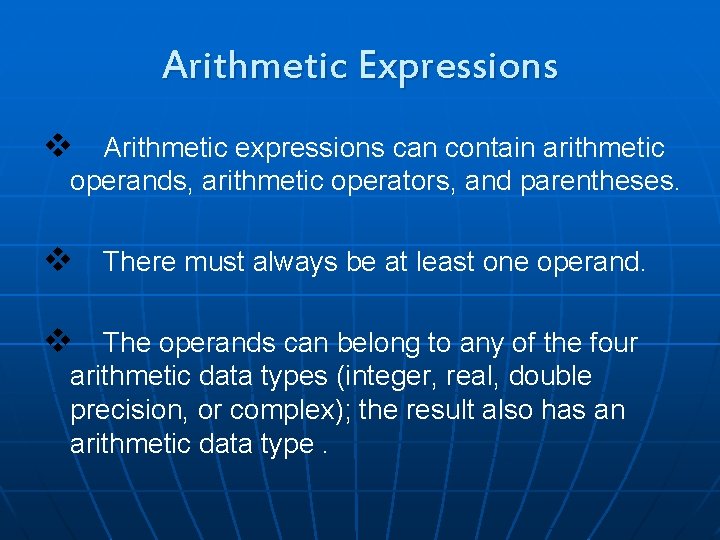
Arithmetic Expressions v Arithmetic expressions can contain arithmetic operands, arithmetic operators, and parentheses. v There must always be at least one operand. v The operands can belong to any of the four arithmetic data types (integer, real, double precision, or complex); the result also has an arithmetic data type.
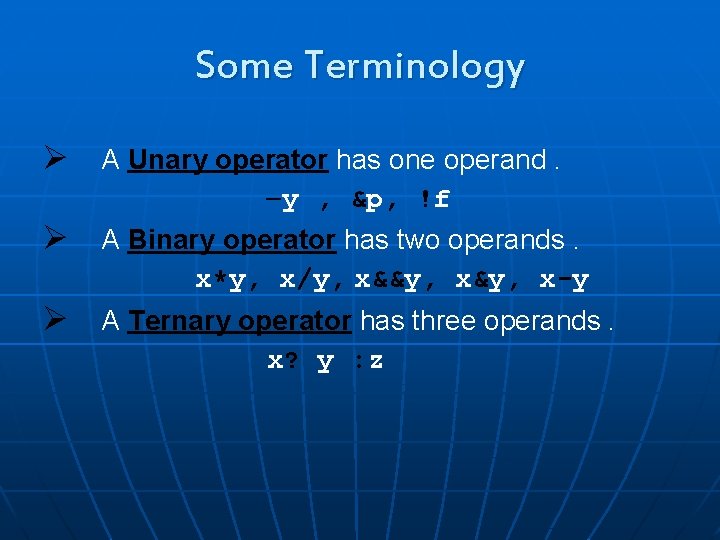
Some Terminology Ø A Unary operator has one operand. y , &p, !f Ø A Binary operator has two operands. x*y, x/y, x&&y, x-y Ø A Ternary operator has three operands. x? y : z
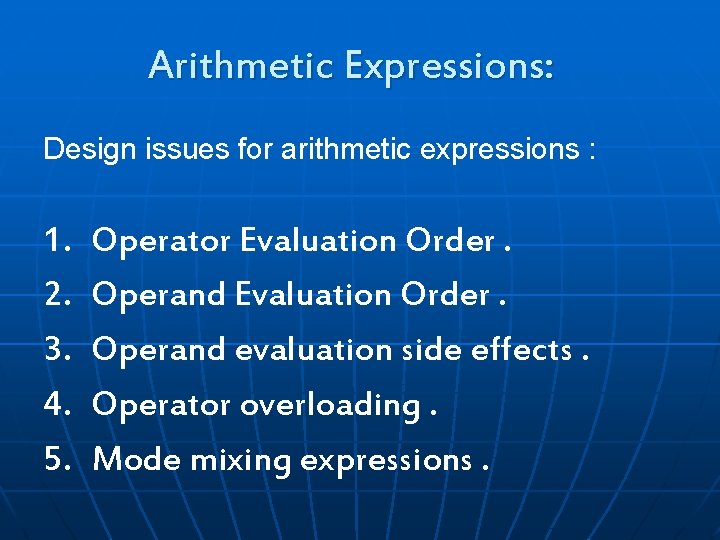
Arithmetic Expressions: Design issues for arithmetic expressions : 1. 2. 3. 4. 5. Operator Evaluation Order. Operand evaluation side effects. Operator overloading. Mode mixing expressions.
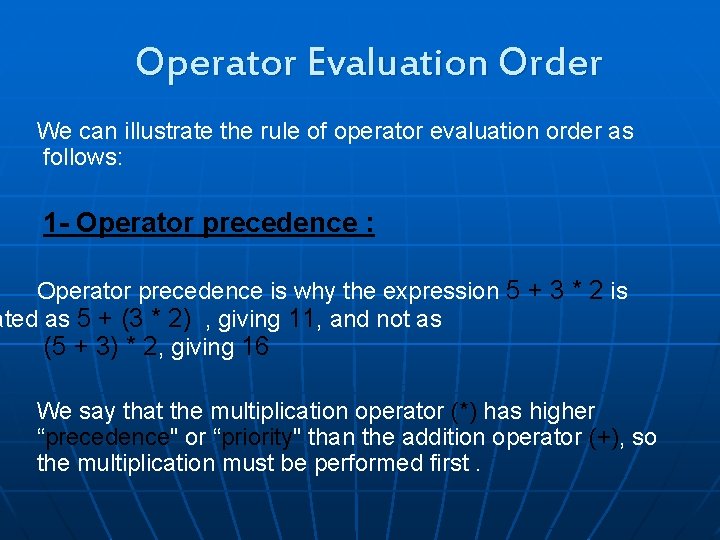
Operator Evaluation Order We can illustrate the rule of operator evaluation order as follows: 1 - Operator precedence : Operator precedence is why the expression 5 + 3 * 2 is ated as 5 + (3 * 2) , giving 11, and not as (5 + 3) * 2, giving 16 We say that the multiplication operator (*) has higher “precedence" or “priority" than the addition operator (+), so the multiplication must be performed first.
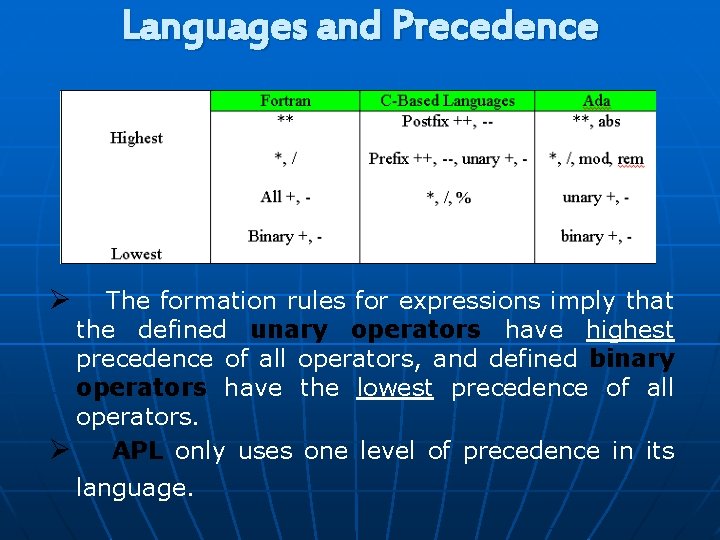
Languages and Precedence Ø The formation rules for expressions imply that the defined unary operators have highest precedence of all operators, and defined binary operators have the lowest precedence of all operators. Ø APL only uses one level of precedence in its language.
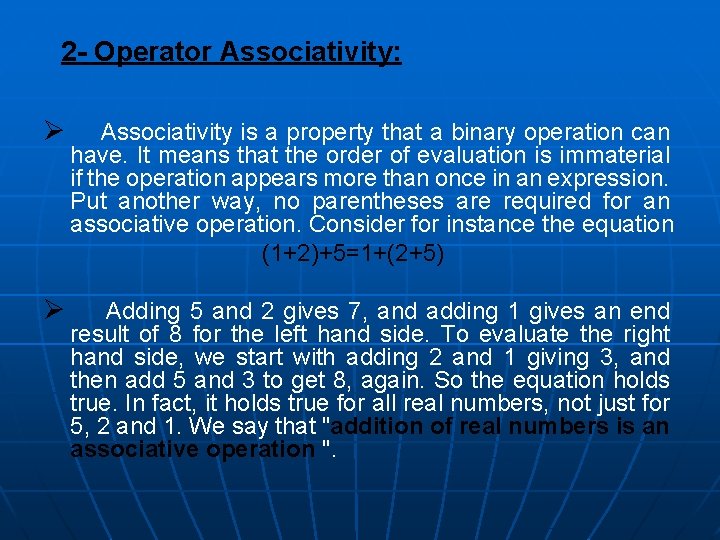
2 - Operator Associativity: Ø Associativity is a property that a binary operation can have. It means that the order of evaluation is immaterial if the operation appears more than once in an expression. Put another way, no parentheses are required for an associative operation. Consider for instance the equation (1+2)+5=1+(2+5) Ø Adding 5 and 2 gives 7, and adding 1 gives an end result of 8 for the left hand side. To evaluate the right hand side, we start with adding 2 and 1 giving 3, and then add 5 and 3 to get 8, again. So the equation holds true. In fact, it holds true for all real numbers, not just for 5, 2 and 1. We say that "addition of real numbers is an associative operation ".
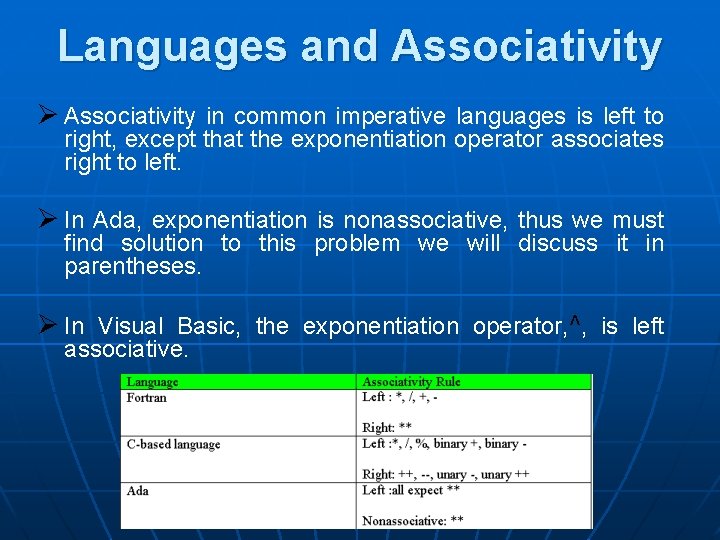
Languages and Associativity Ø Associativity in common imperative languages is left to right, except that the exponentiation operator associates right to left. Ø In Ada, exponentiation is nonassociative, thus we must find solution to this problem we will discuss it in parentheses. Ø In Visual Basic, the exponentiation operator, ^, is left associative.
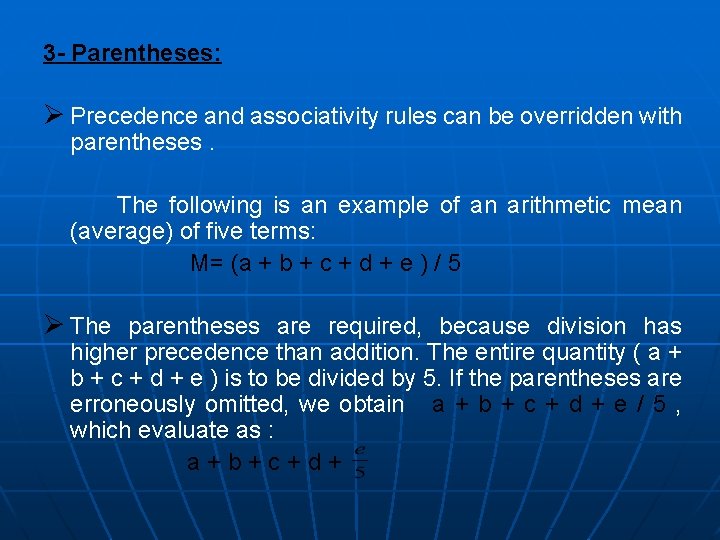
3 - Parentheses: Ø Precedence and associativity rules can be overridden with parentheses. The following is an example of an arithmetic mean (average) of five terms: M= (a + b + c + d + e ) / 5 Ø The parentheses are required, because division has higher precedence than addition. The entire quantity ( a + b + c + d + e ) is to be divided by 5. If the parentheses are erroneously omitted, we obtain a + b + c + d + e / 5 , which evaluate as : a+b+c+d+
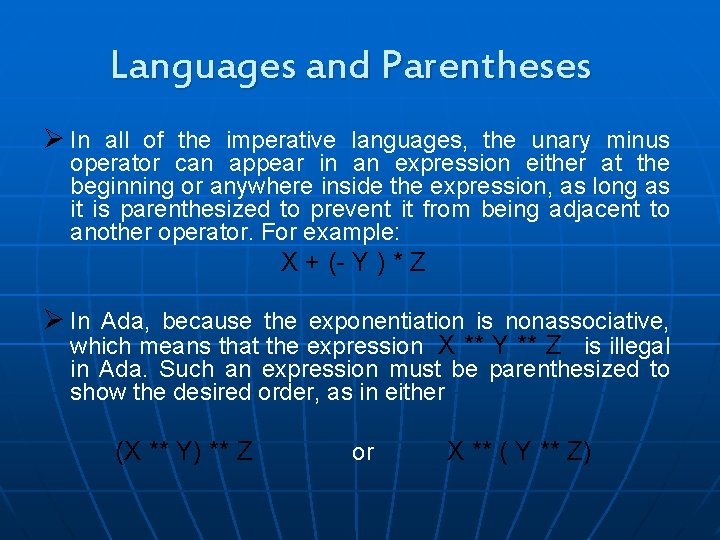
Languages and Parentheses Ø In all of the imperative languages, the unary minus operator can appear in an expression either at the beginning or anywhere inside the expression, as long as it is parenthesized to prevent it from being adjacent to another operator. For example: X + (- Y ) * Z Ø In Ada, because the exponentiation is nonassociative, which means that the expression X ** Y ** Z is illegal in Ada. Such an expression must be parenthesized to show the desired order, as in either (X ** Y) ** Z or X ** ( Y ** Z)
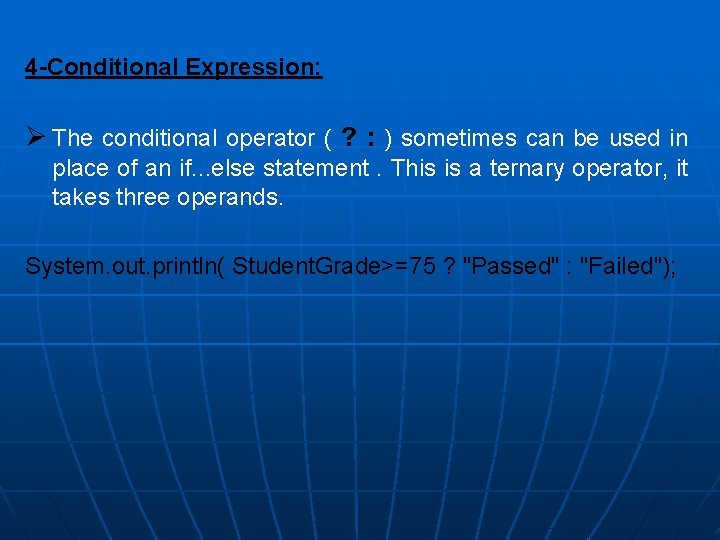
4 -Conditional Expression: Ø The conditional operator ( ? : ) sometimes can be used in place of an if. . . else statement. This is a ternary operator, it takes three operands. System. out. println( Student. Grade>=75 ? "Passed" : "Failed");
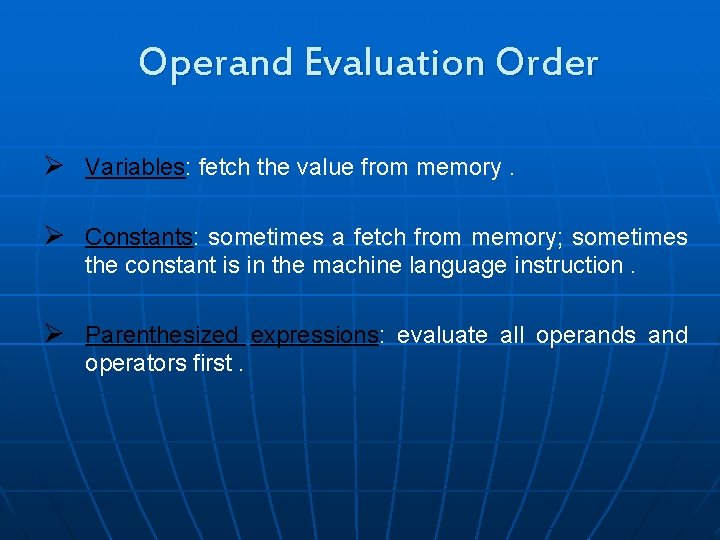
Operand Evaluation Order Ø Variables: fetch the value from memory. Ø Constants: sometimes a fetch from memory; sometimes the constant is in the machine language instruction. Ø Parenthesized expressions: evaluate all operands and operators first.
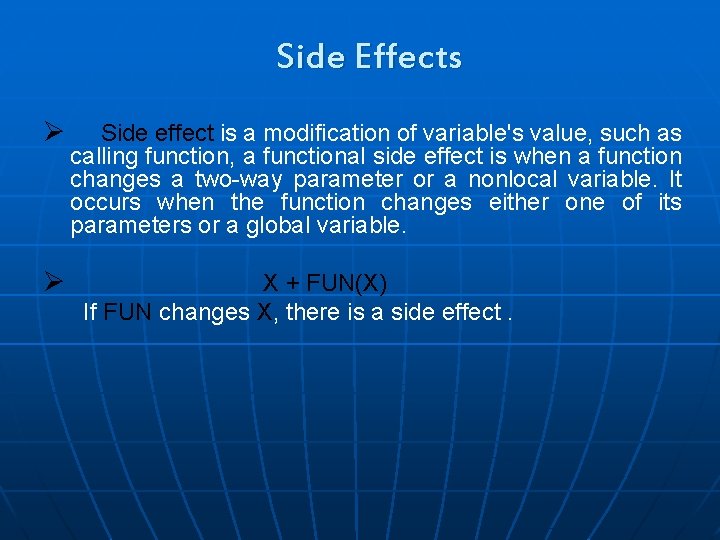
Side Effects Ø Ø Side effect is a modification of variable's value, such as calling function, a functional side effect is when a function changes a two-way parameter or a nonlocal variable. It occurs when the function changes either one of its parameters or a global variable. X + FUN(X) If FUN changes X, there is a side effect.
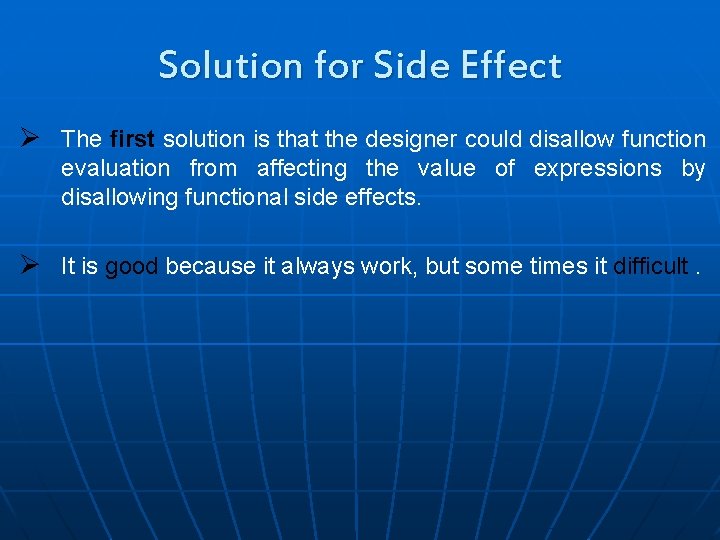
Solution for Side Effect Ø The first solution is that the designer could disallow function evaluation from affecting the value of expressions by disallowing functional side effects. Ø It is good because it always work, but some times it difficult.
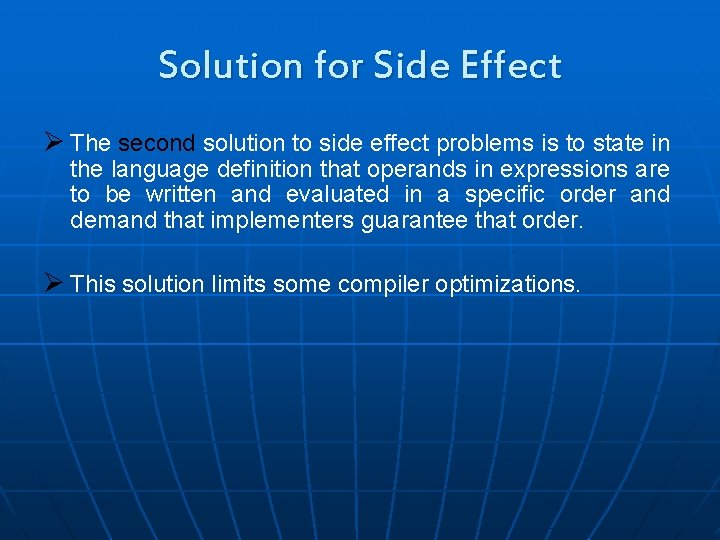
Solution for Side Effect Ø The second solution to side effect problems is to state in the language definition that operands in expressions are to be written and evaluated in a specific order and demand that implementers guarantee that order. Ø This solution limits some compiler optimizations.
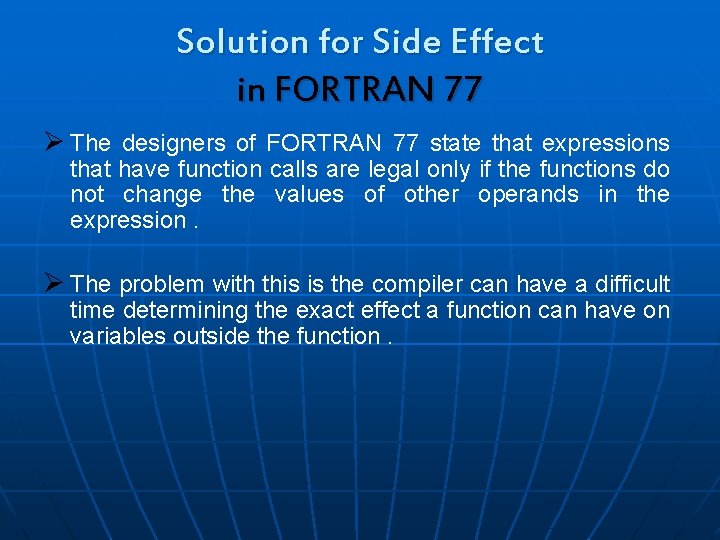
Solution for Side Effect in FORTRAN 77 Ø The designers of FORTRAN 77 state that expressions that have function calls are legal only if the functions do not change the values of other operands in the expression. Ø The problem with this is the compiler can have a difficult time determining the exact effect a function can have on variables outside the function.
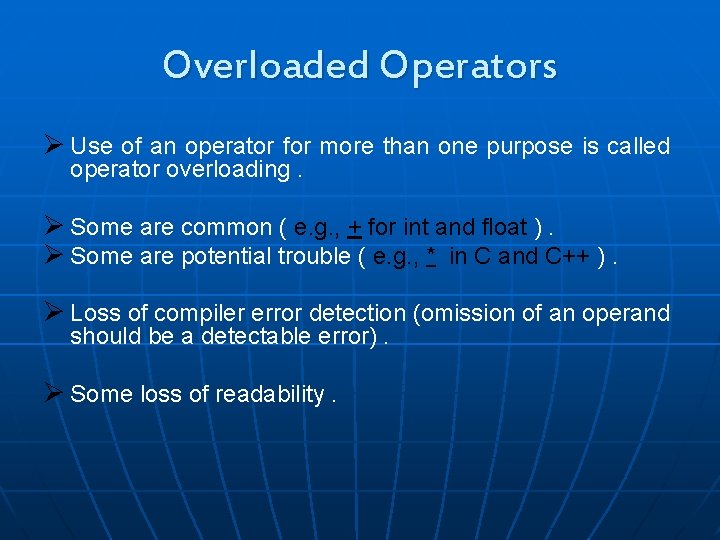
Overloaded Operators Ø Use of an operator for more than one purpose is called operator overloading. Ø Some are common ( e. g. , + for int and float ). Ø Some are potential trouble ( e. g. , * in C and C++ ). Ø Loss of compiler error detection (omission of an operand should be a detectable error). Ø Some loss of readability.
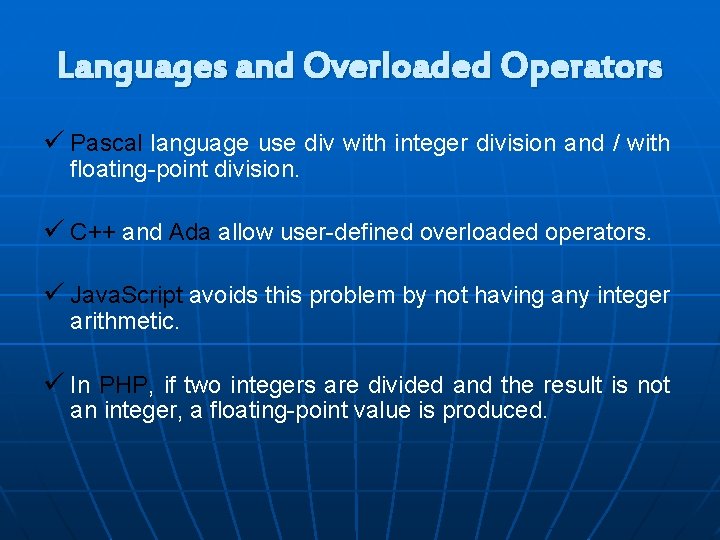
Languages and Overloaded Operators ü Pascal language use div with integer division and / with floating-point division. ü C++ and Ada allow user-defined overloaded operators. ü Java. Script avoids this problem by not having any integer arithmetic. ü In PHP, if two integers are divided and the result is not an integer, a floating-point value is produced.
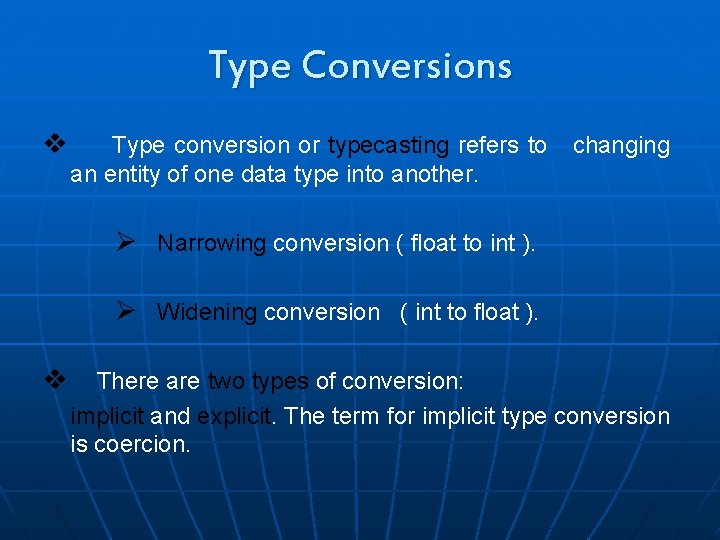
Type Conversions v Type conversion or typecasting refers to an entity of one data type into another. changing Ø Narrowing conversion ( float to int ). Ø Widening conversion ( int to float ). v There are two types of conversion: implicit and explicit. The term for implicit type conversion is coercion.
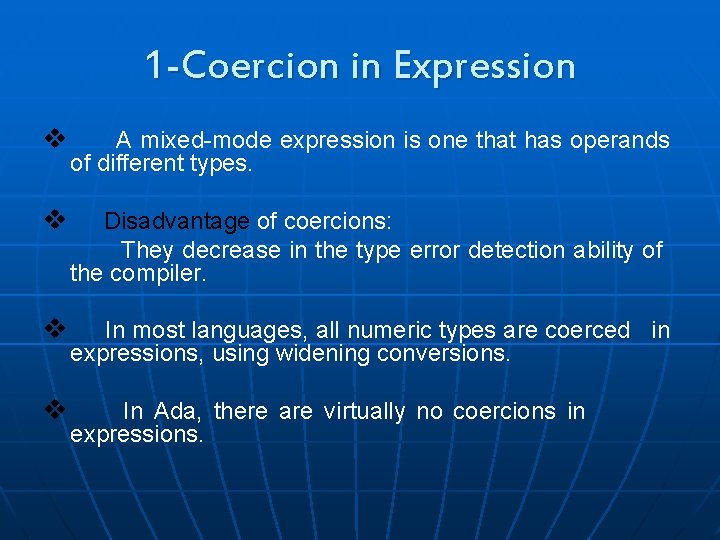
1 -Coercion in Expression v A mixed-mode expression is one that has operands of different types. v Disadvantage of coercions: They decrease in the type error detection ability of the compiler. v In most languages, all numeric types are coerced in expressions, using widening conversions. v In Ada, there are virtually no coercions in expressions.
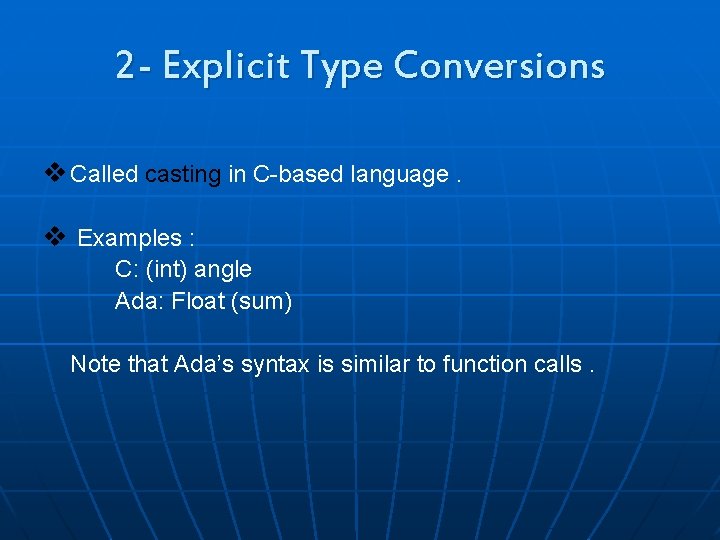
2 - Explicit Type Conversions v Called casting in C-based language. v Examples : C: (int) angle Ada: Float (sum) Note that Ada’s syntax is similar to function calls.
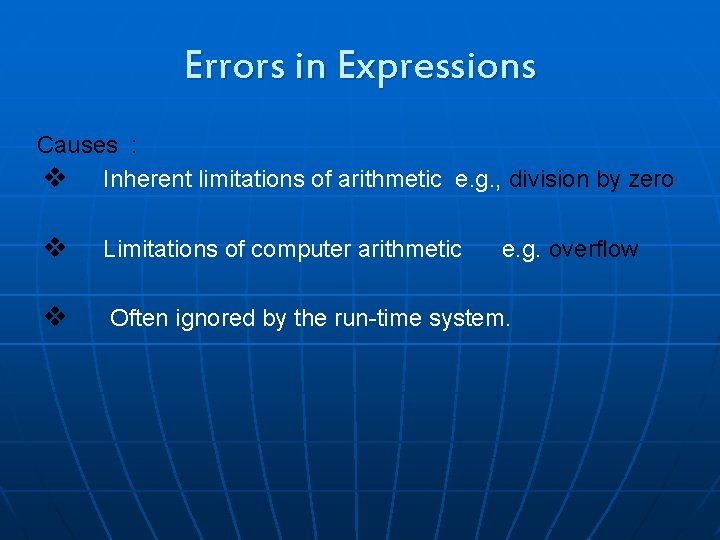
Errors in Expressions Causes : v Inherent limitations of arithmetic e. g. , division by zero v Limitations of computer arithmetic v Often ignored by the run-time system. e. g. overflow