Chapter 7 Decision Making Class 7 Decision Making
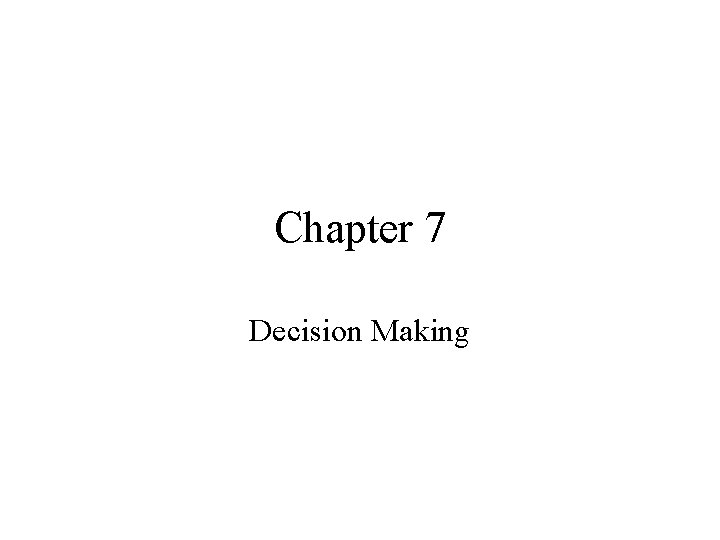
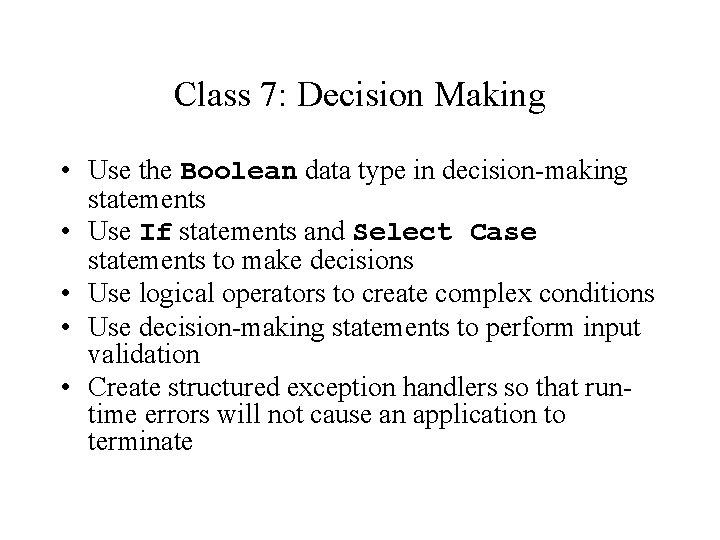
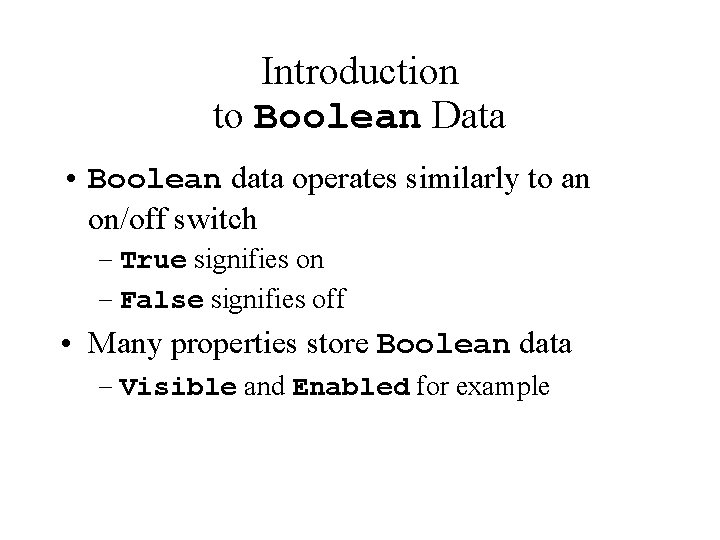
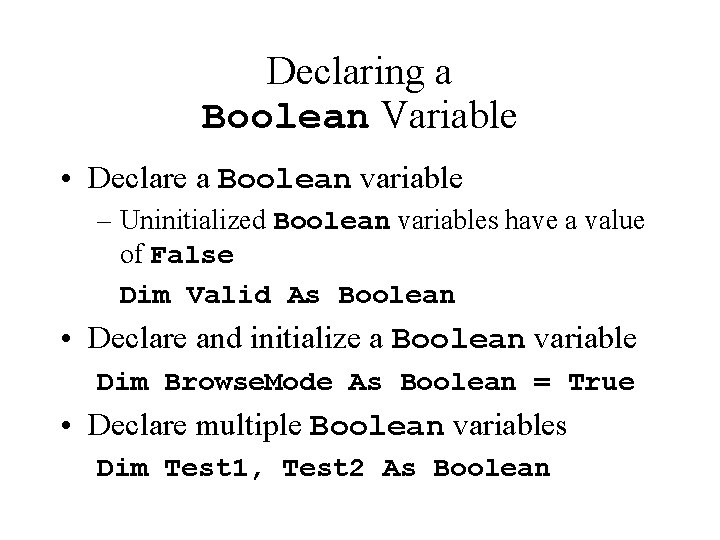
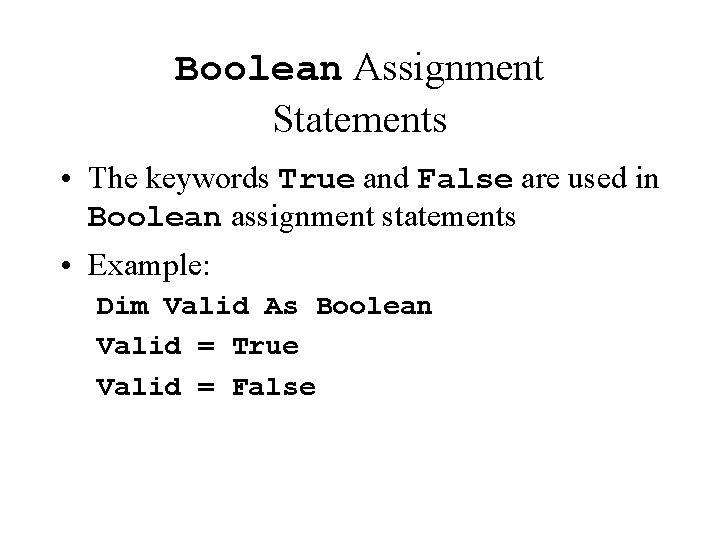
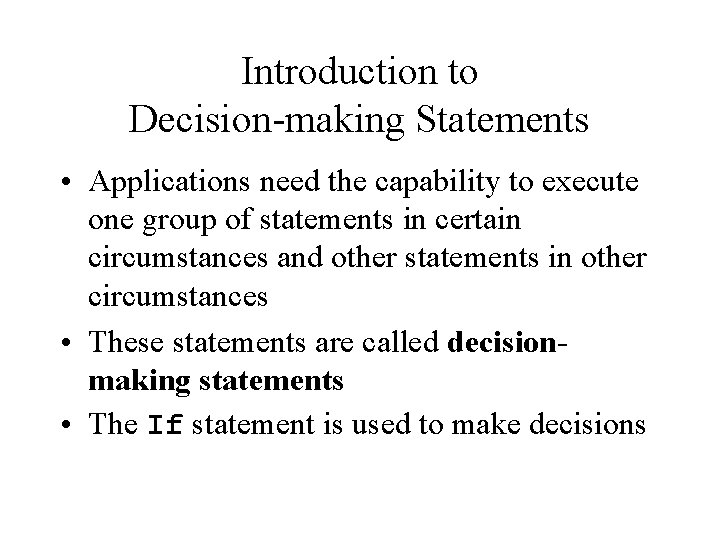
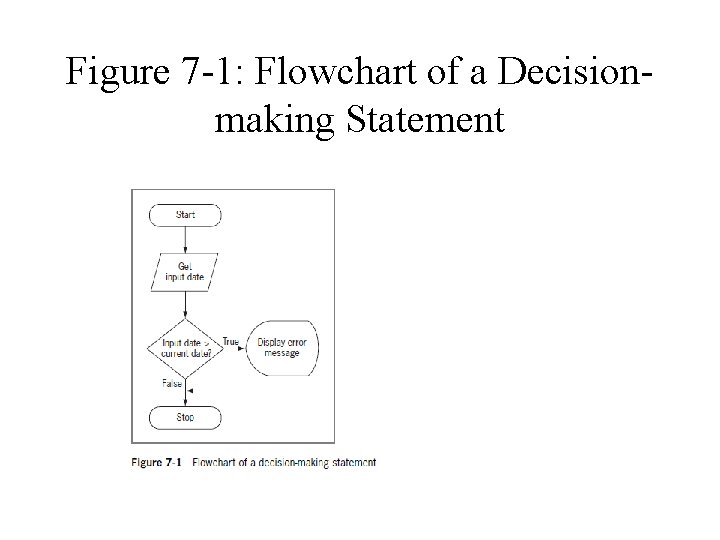
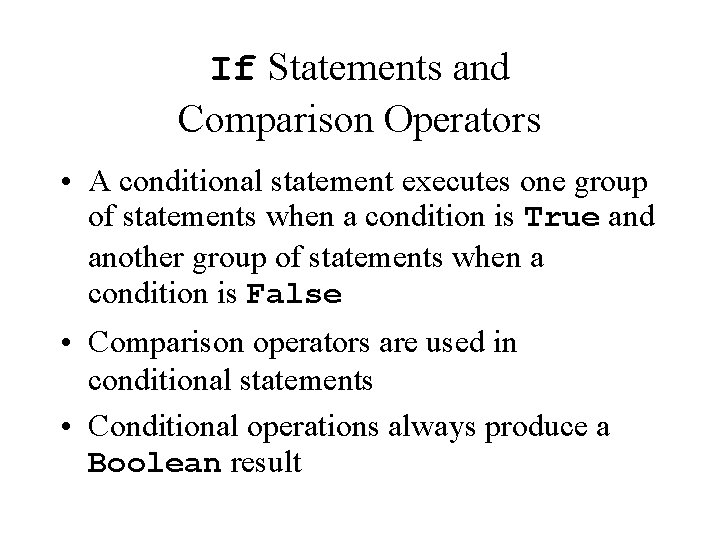
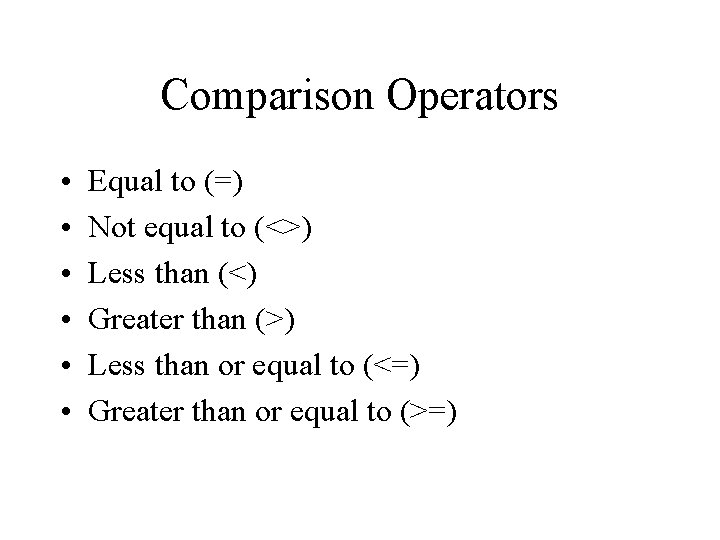
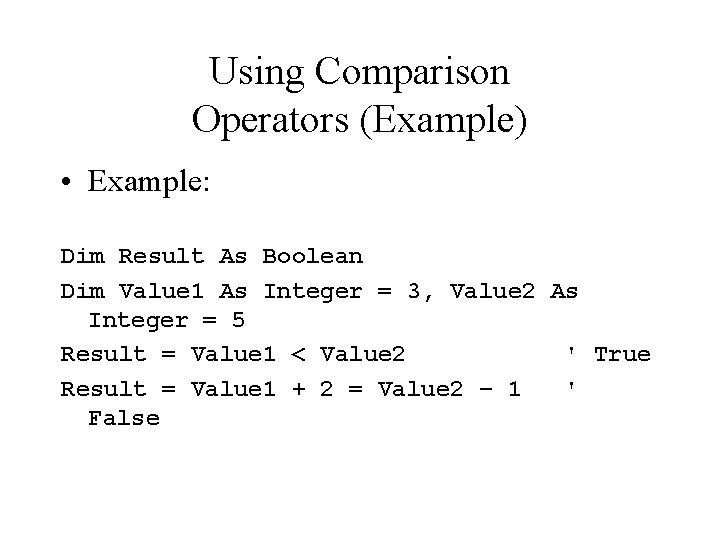
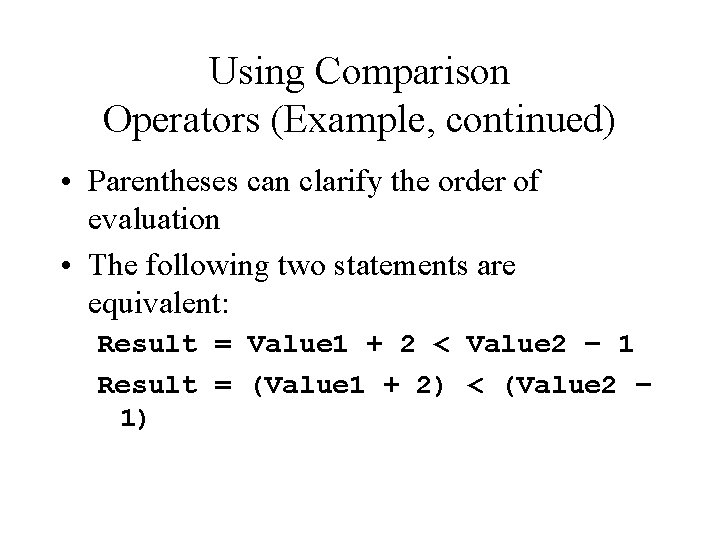
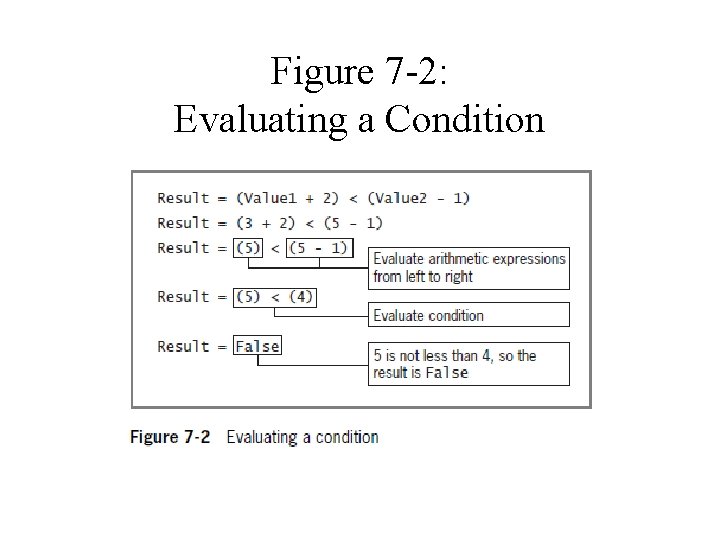
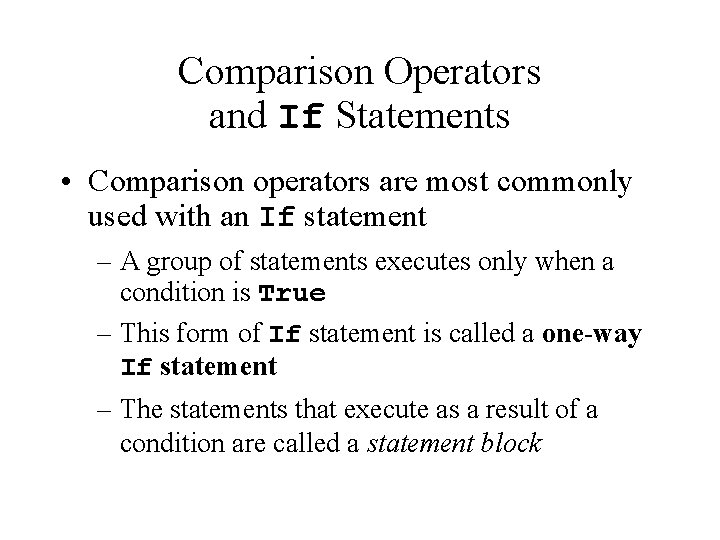
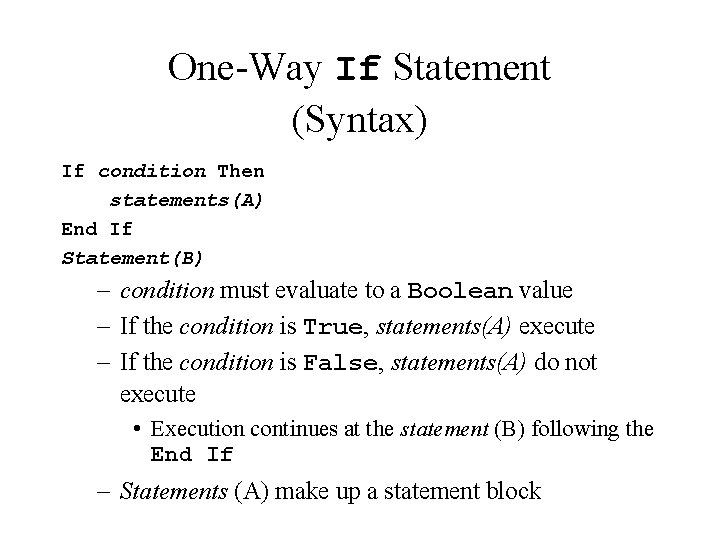
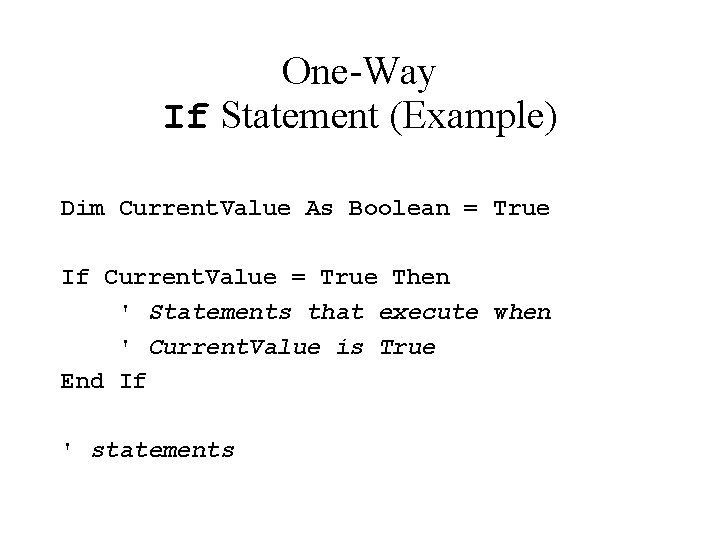
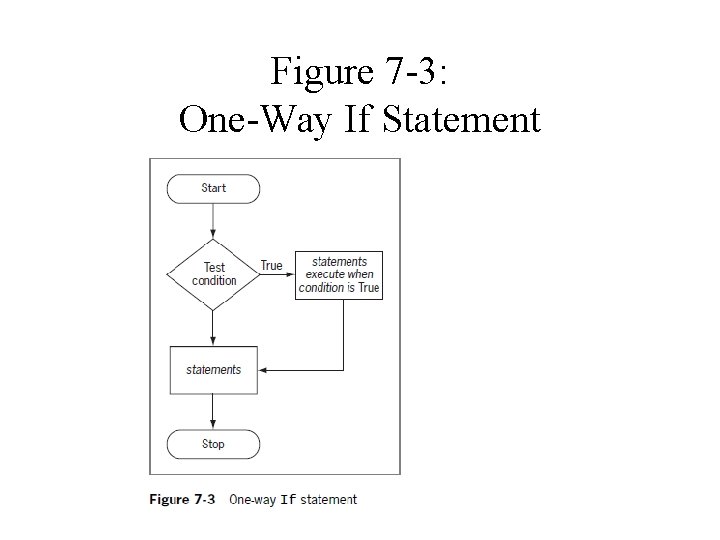
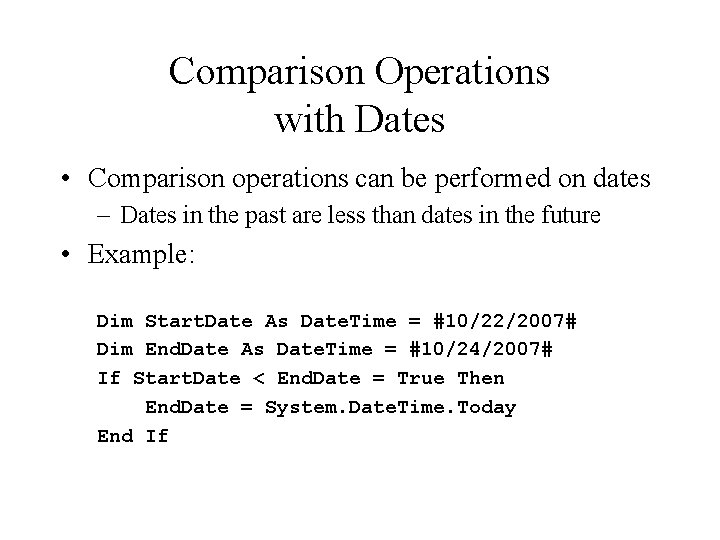
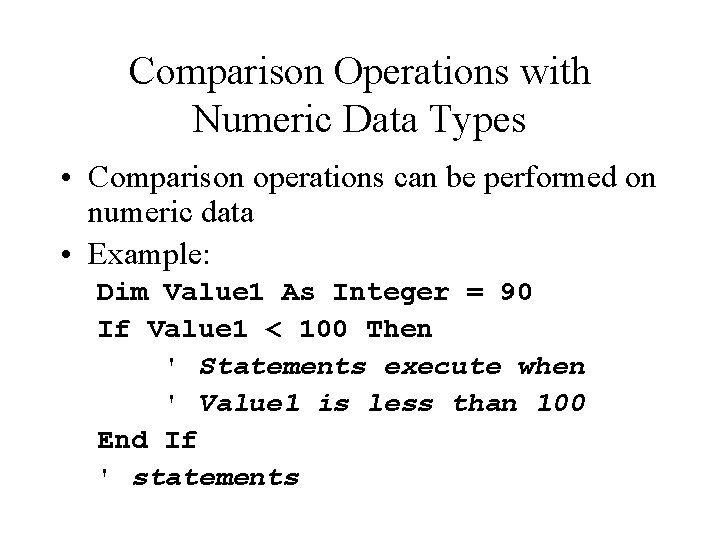
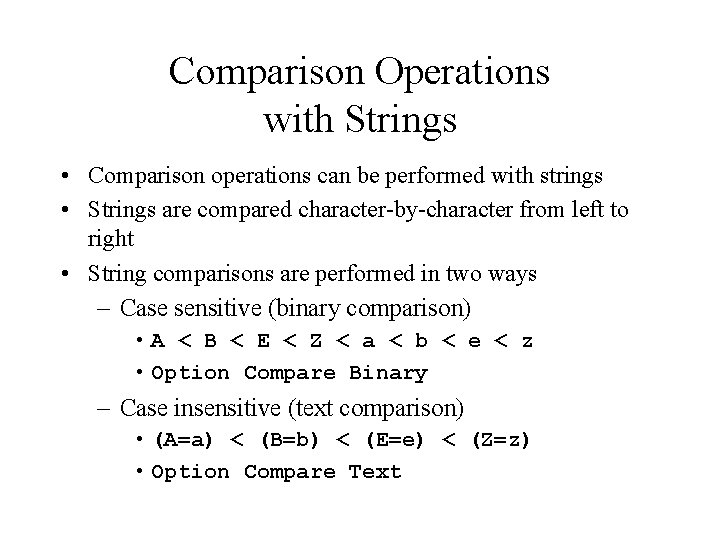
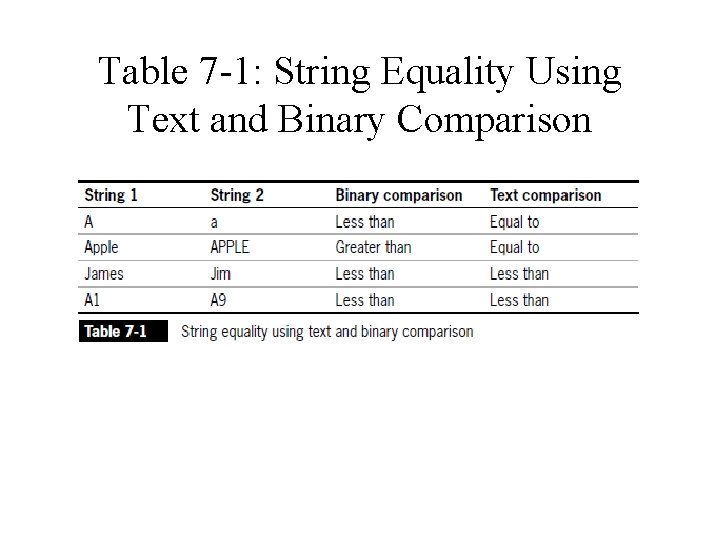
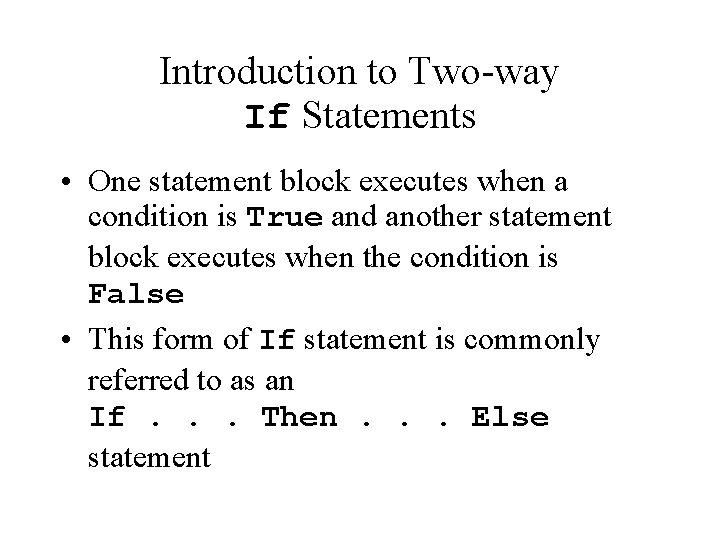
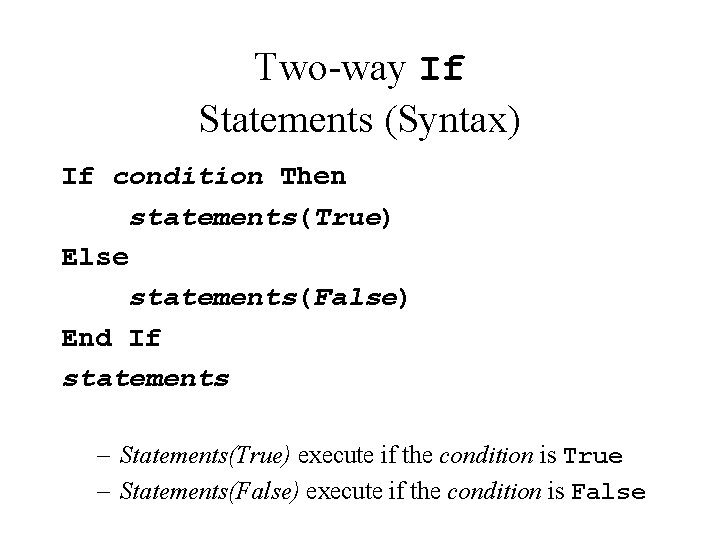
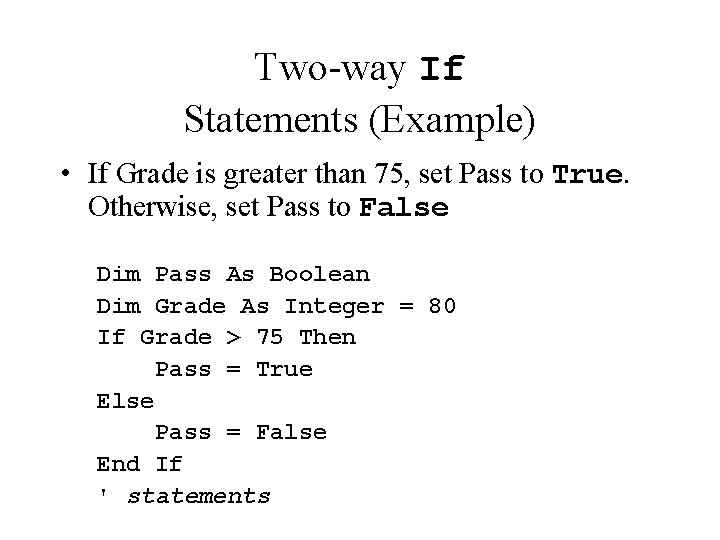
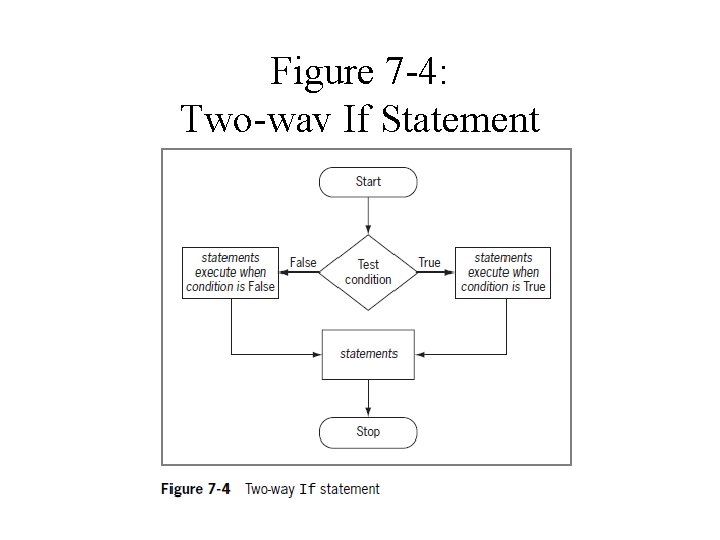
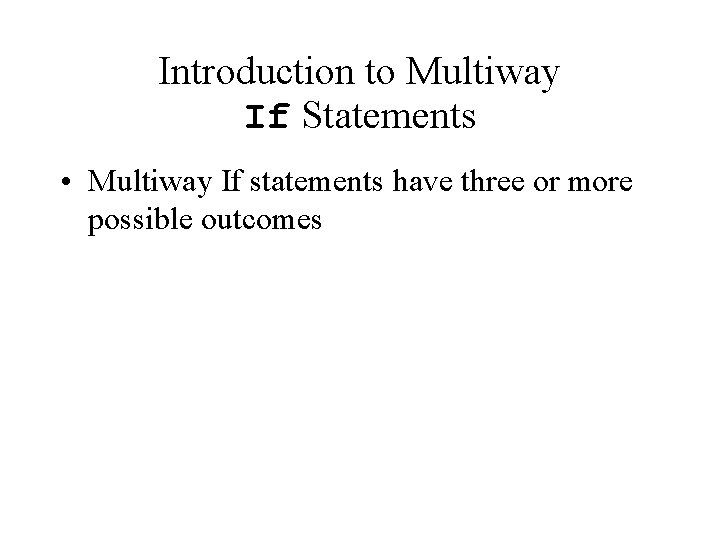
![Multiway If Statements (Syntax) If condition 1 Then [statements] [Else. If condition 2 Then Multiway If Statements (Syntax) If condition 1 Then [statements] [Else. If condition 2 Then](https://slidetodoc.com/presentation_image_h2/3a5d678195b084fa4240981954bf9a8b/image-26.jpg)
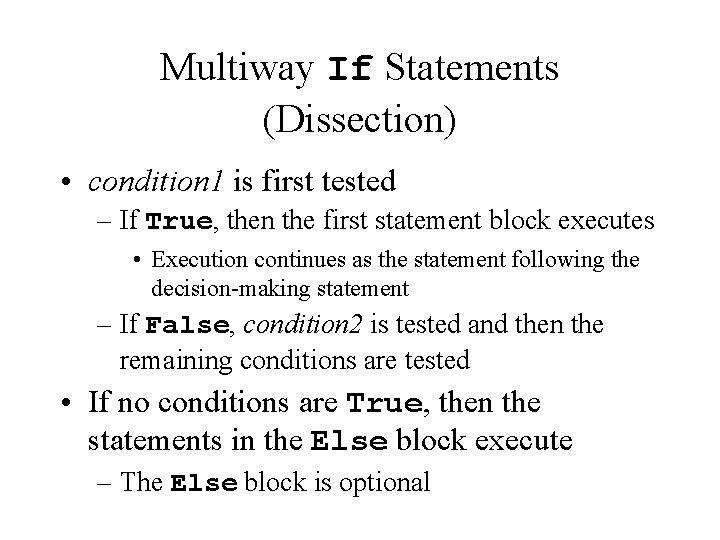
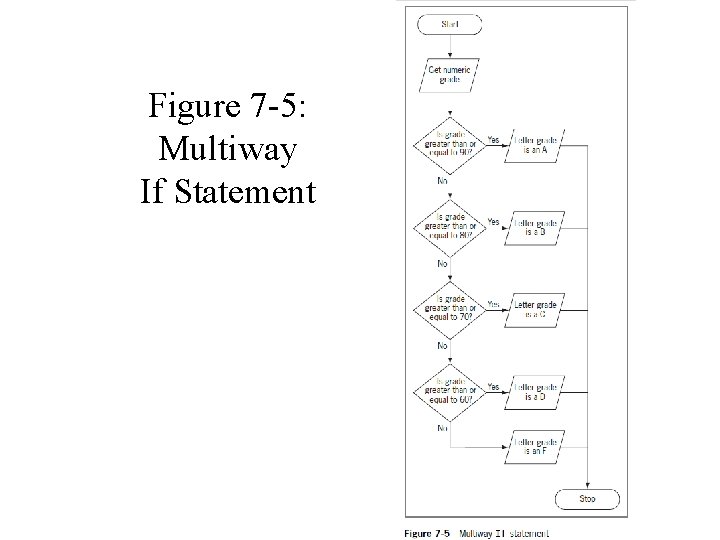
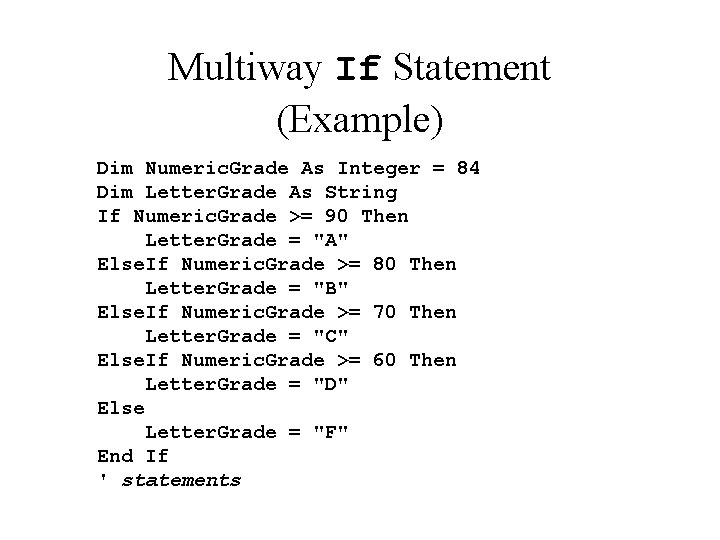
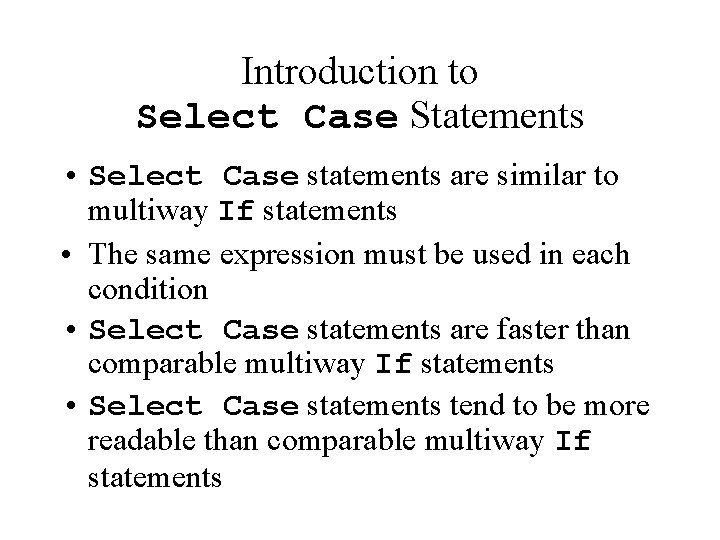
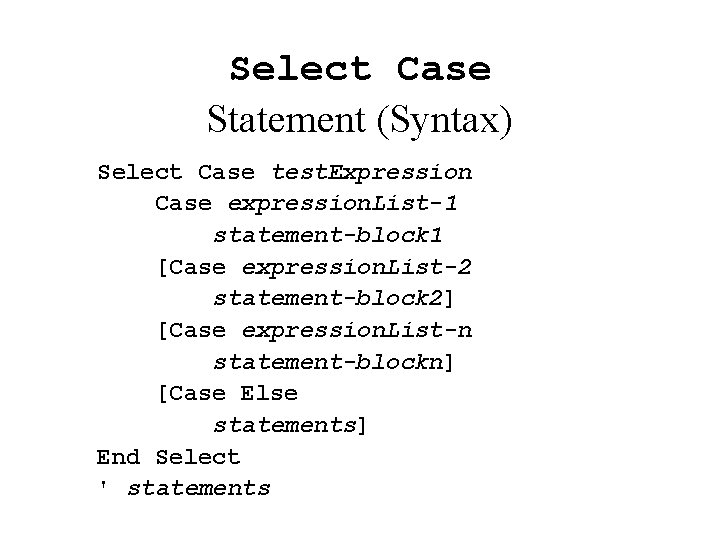
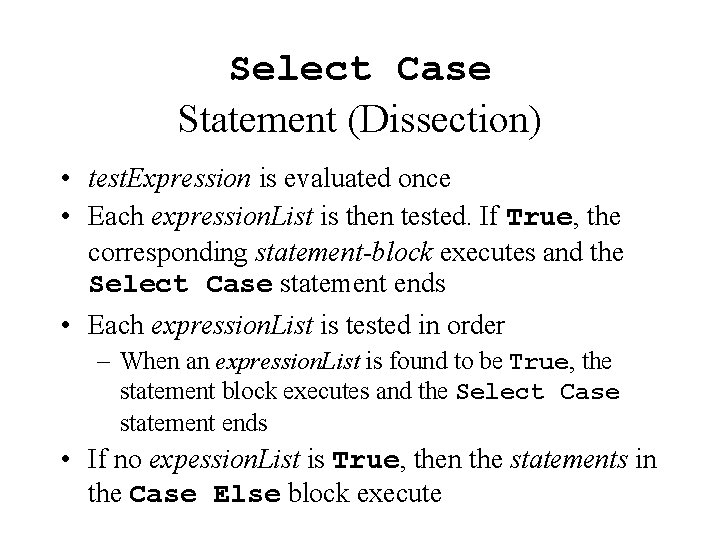
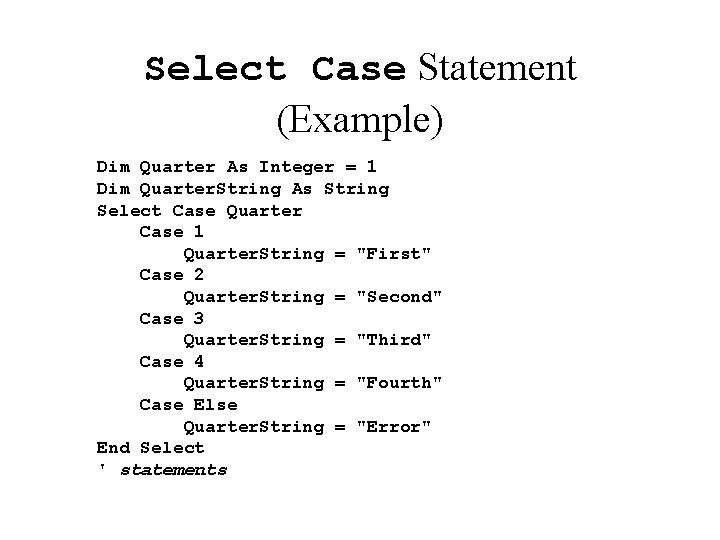
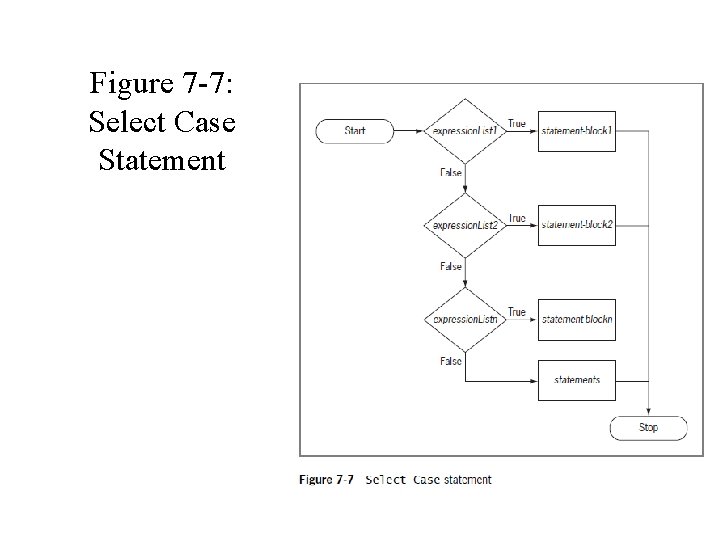
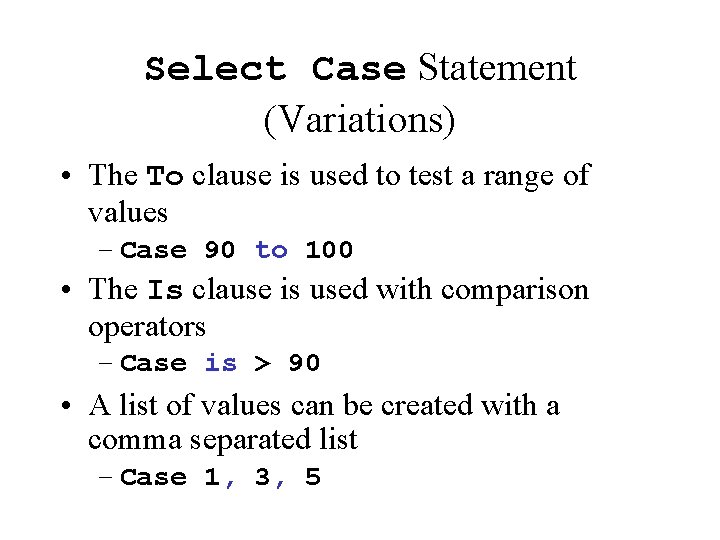
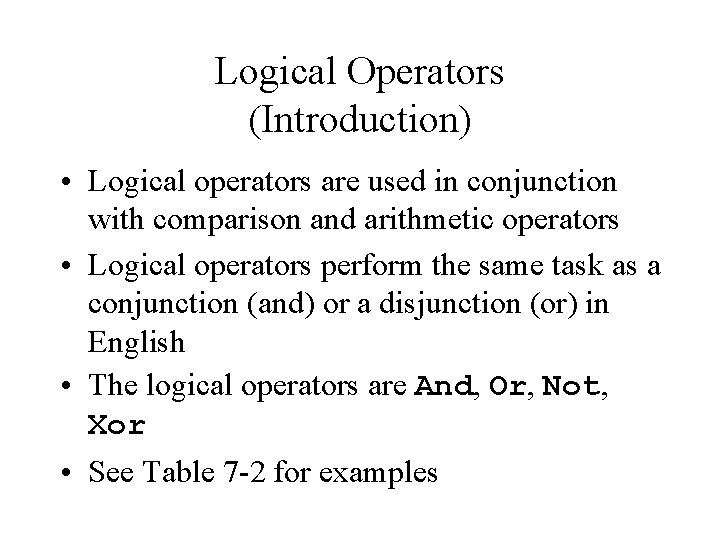
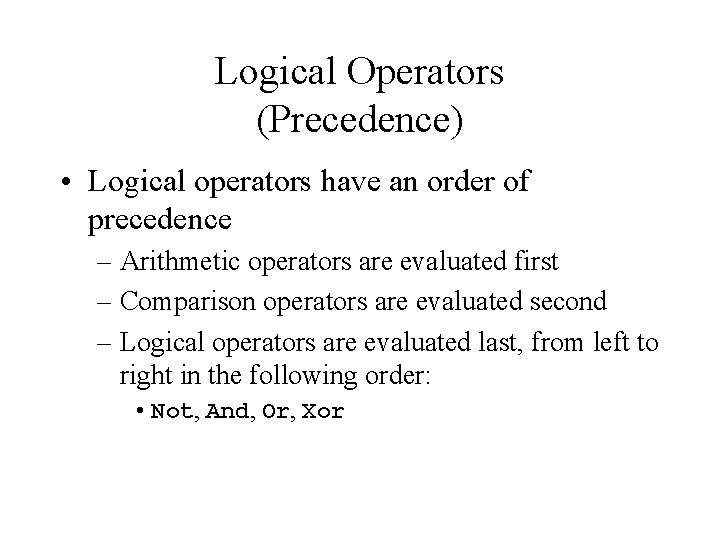
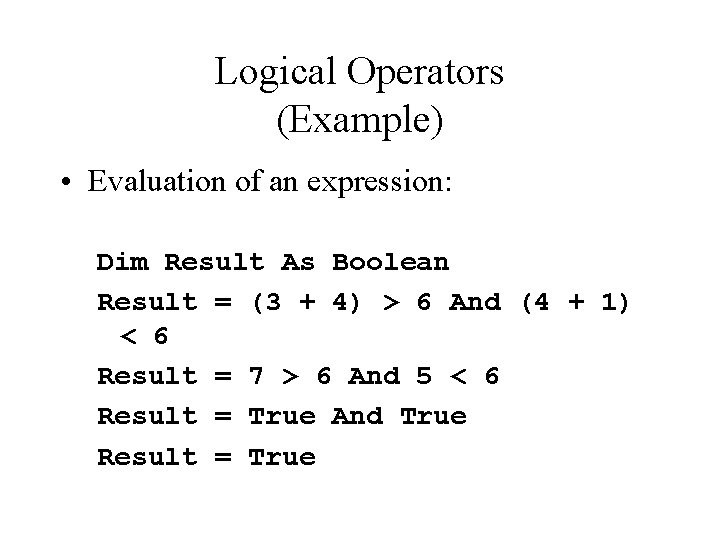
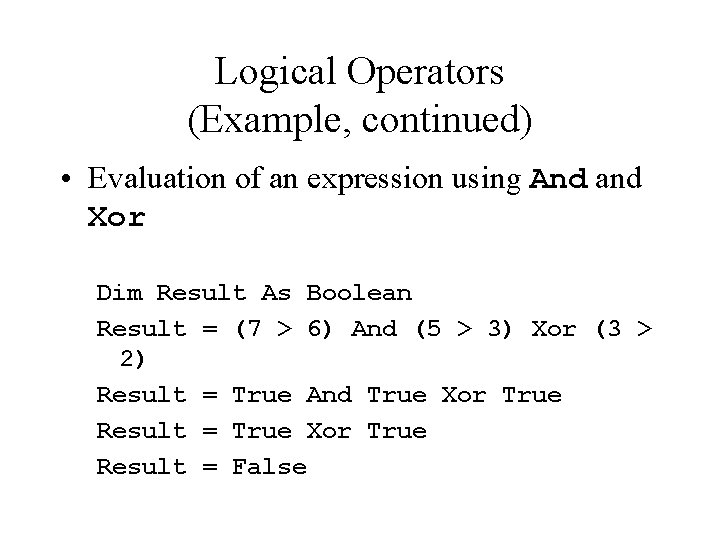
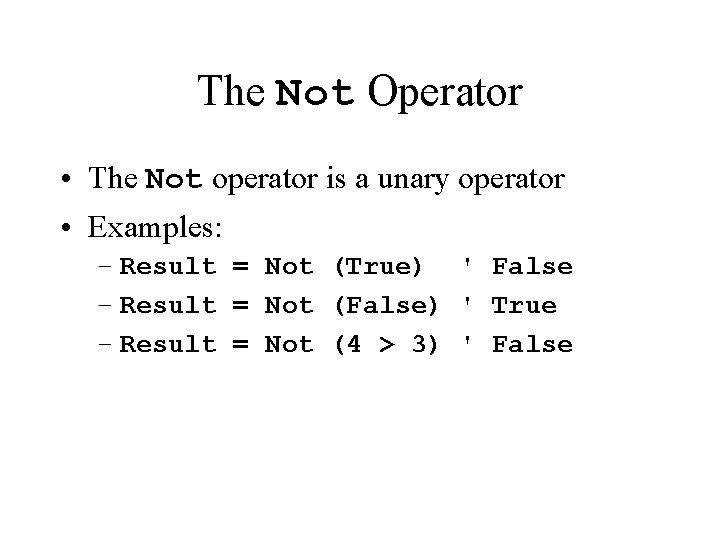
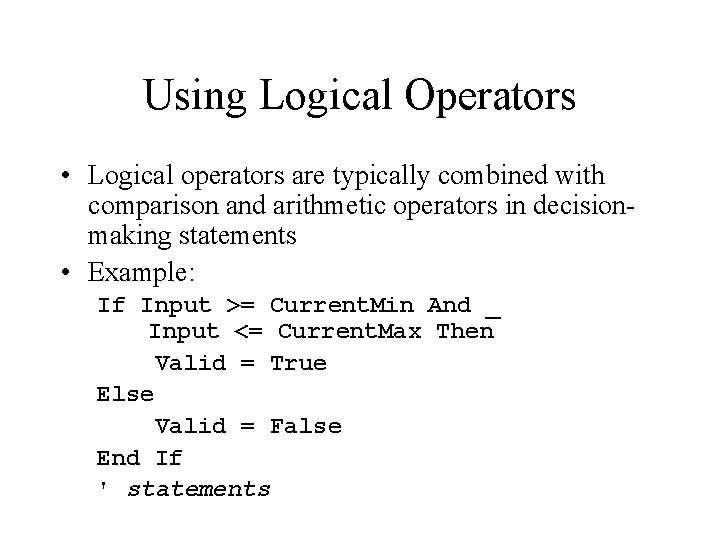
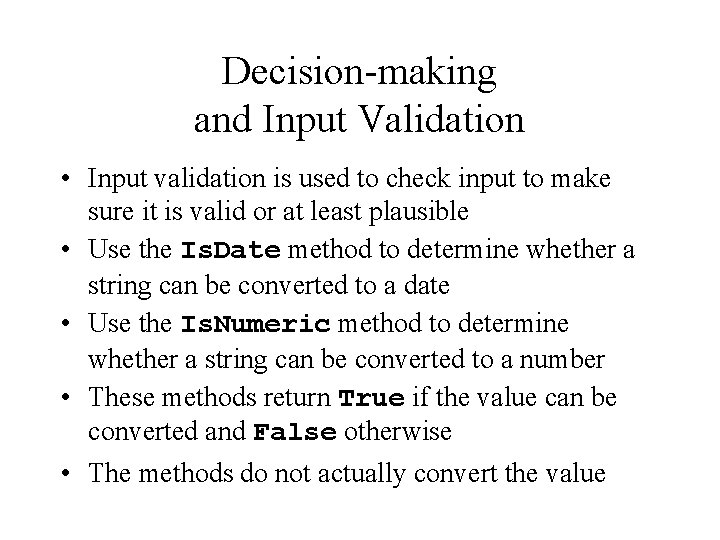
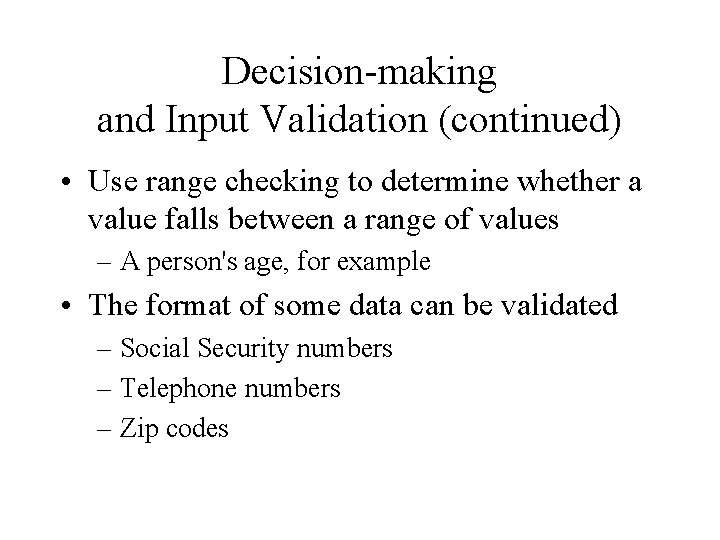
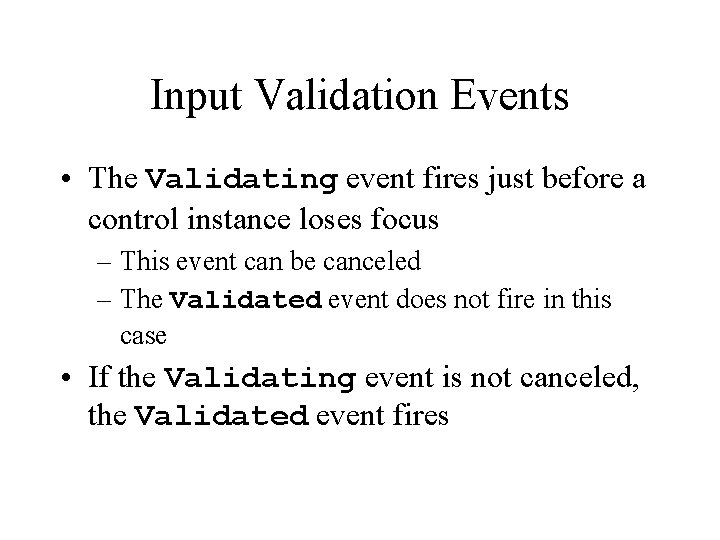
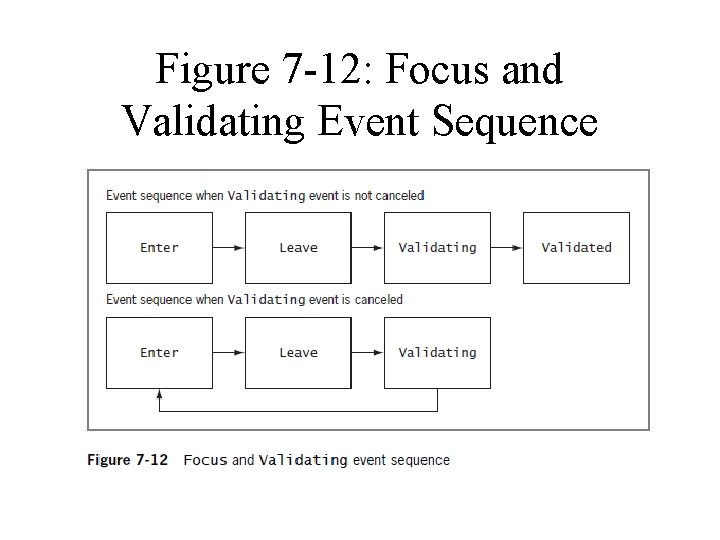
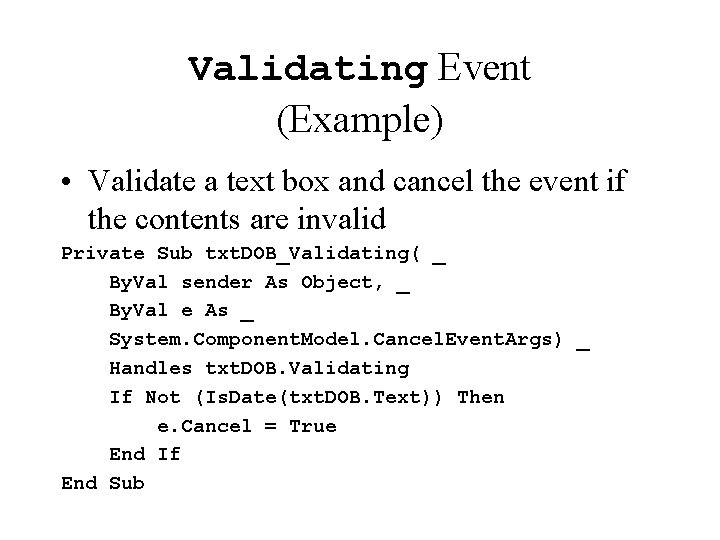
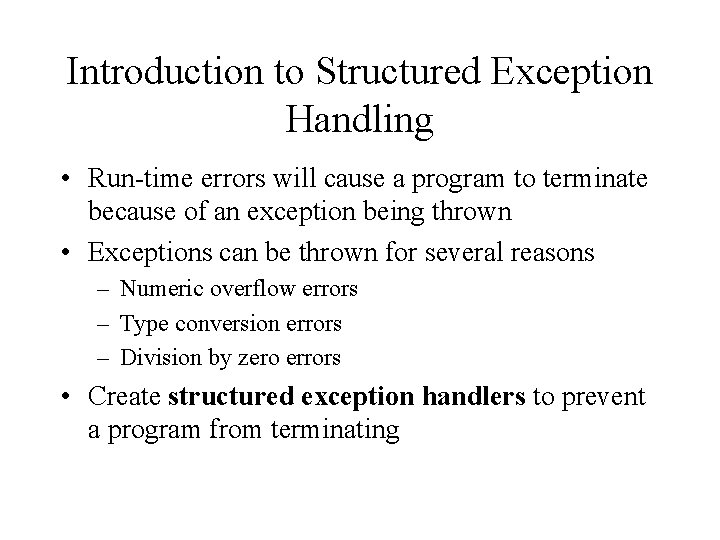
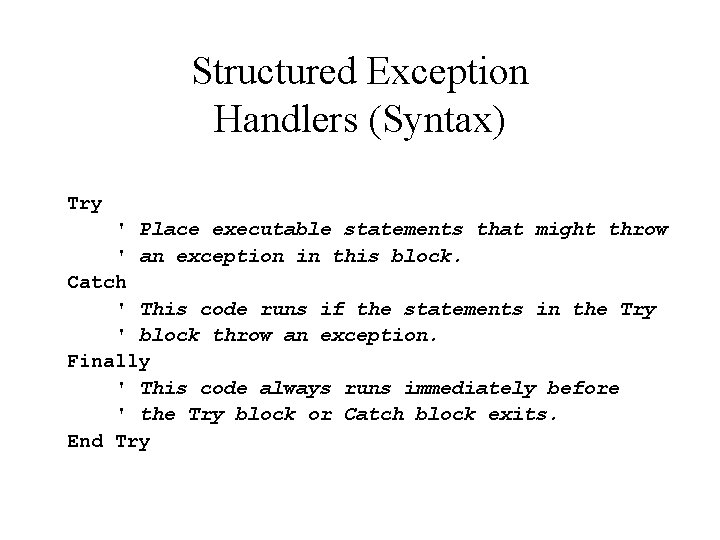
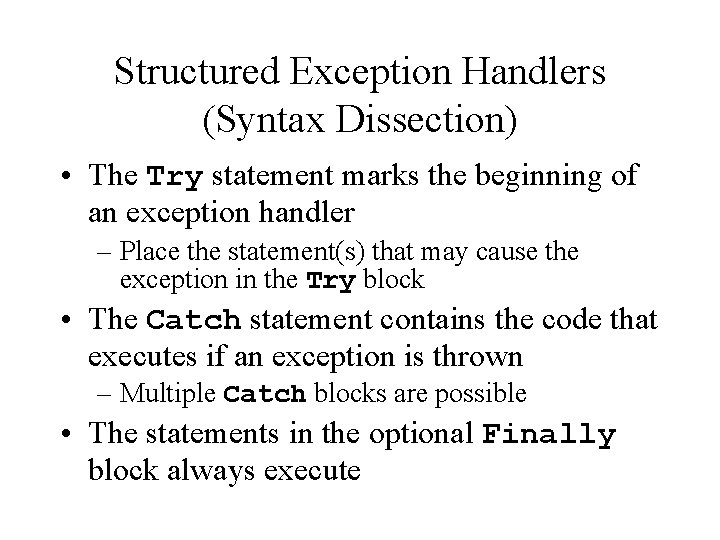
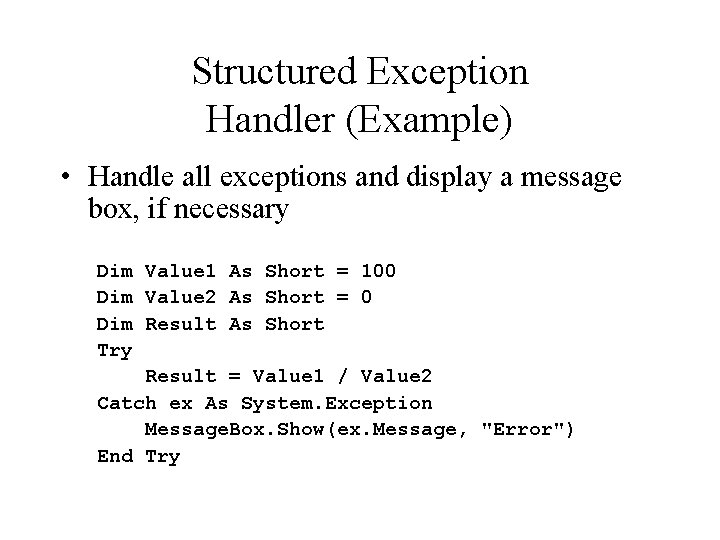
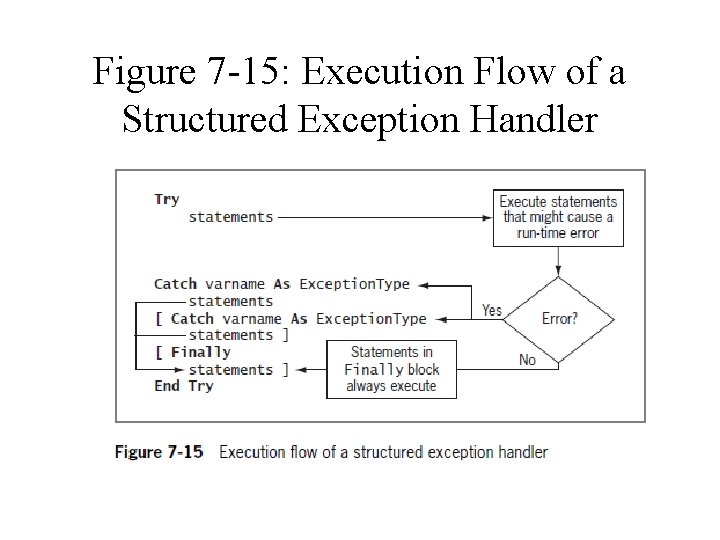
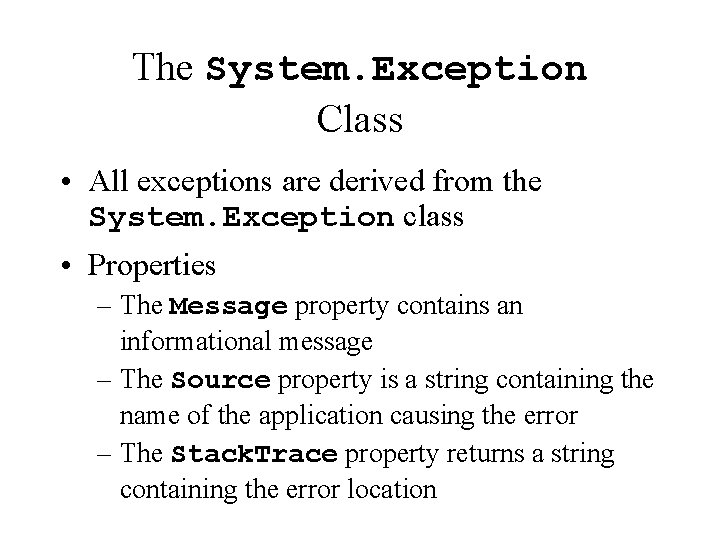
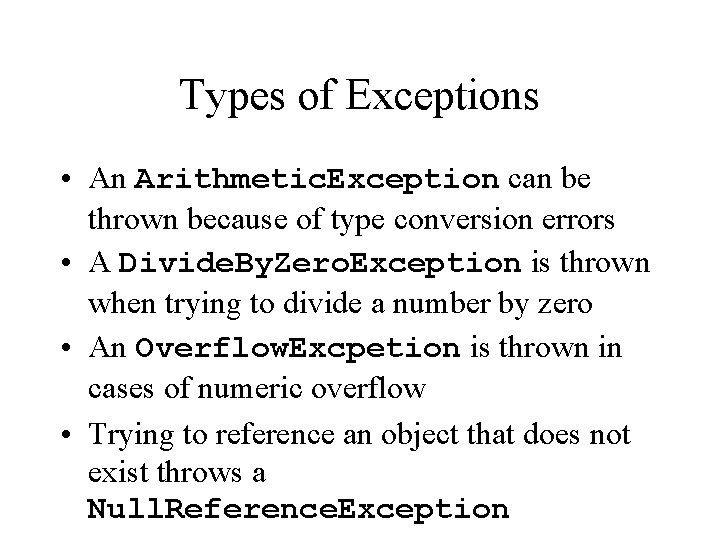
- Slides: 53
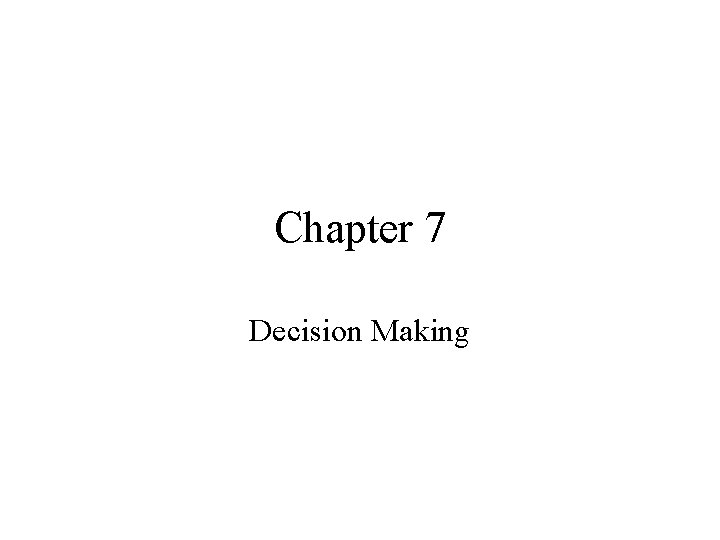
Chapter 7 Decision Making
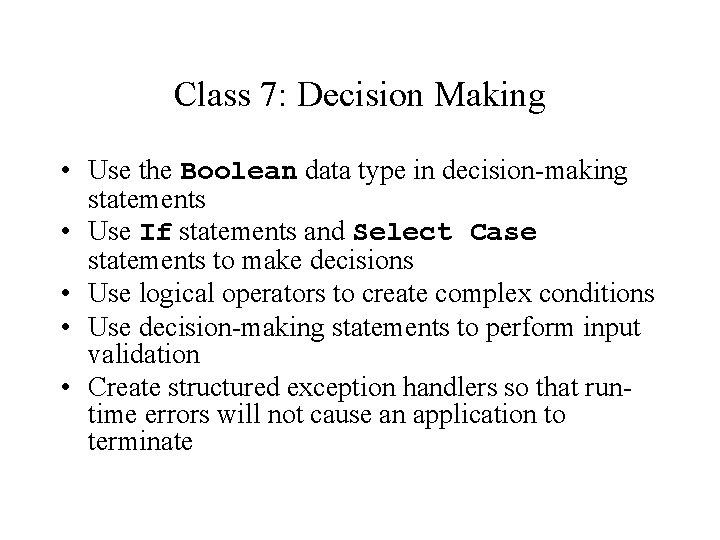
Class 7: Decision Making • Use the Boolean data type in decision-making statements • Use If statements and Select Case statements to make decisions • Use logical operators to create complex conditions • Use decision-making statements to perform input validation • Create structured exception handlers so that runtime errors will not cause an application to terminate
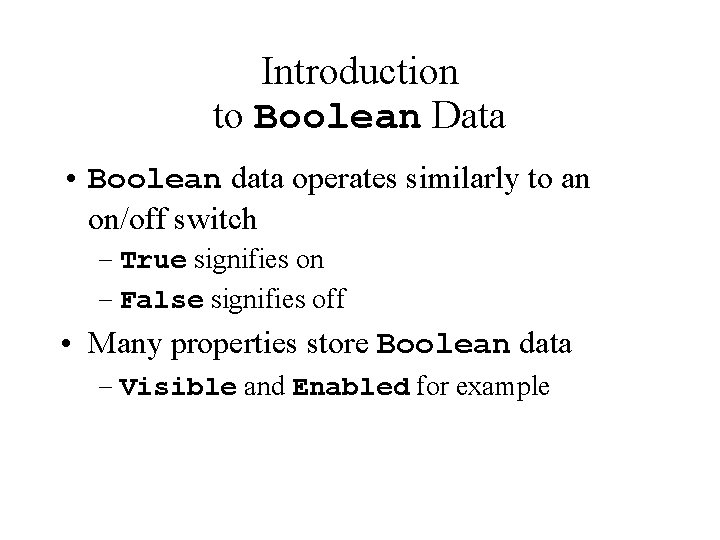
Introduction to Boolean Data • Boolean data operates similarly to an on/off switch – True signifies on – False signifies off • Many properties store Boolean data – Visible and Enabled for example
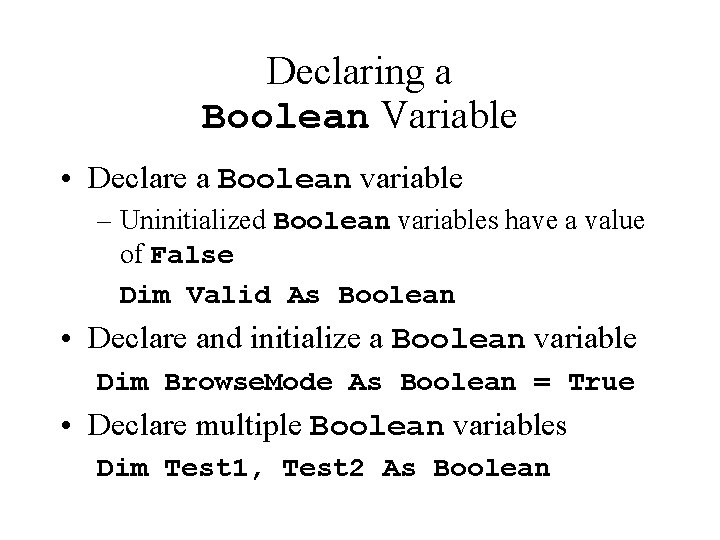
Declaring a Boolean Variable • Declare a Boolean variable – Uninitialized Boolean variables have a value of False Dim Valid As Boolean • Declare and initialize a Boolean variable Dim Browse. Mode As Boolean = True • Declare multiple Boolean variables Dim Test 1, Test 2 As Boolean
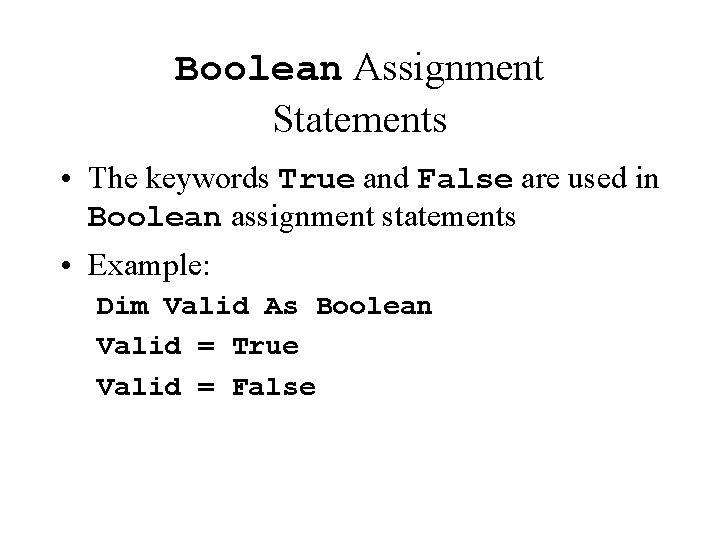
Boolean Assignment Statements • The keywords True and False are used in Boolean assignment statements • Example: Dim Valid As Boolean Valid = True Valid = False
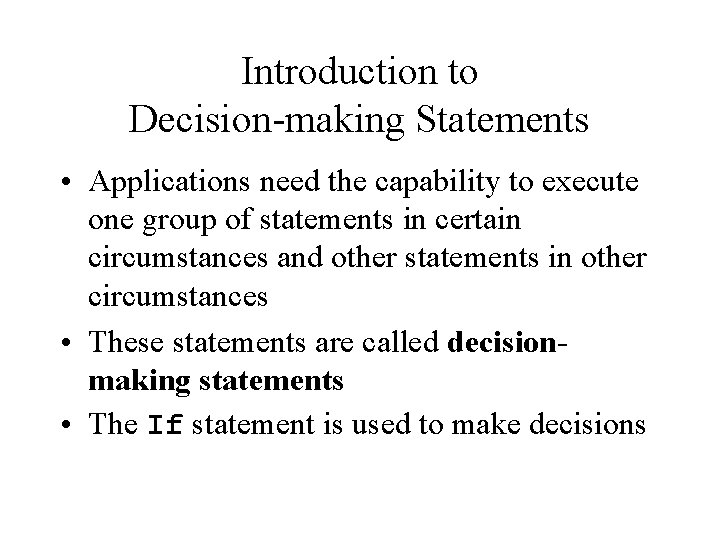
Introduction to Decision-making Statements • Applications need the capability to execute one group of statements in certain circumstances and other statements in other circumstances • These statements are called decisionmaking statements • The If statement is used to make decisions
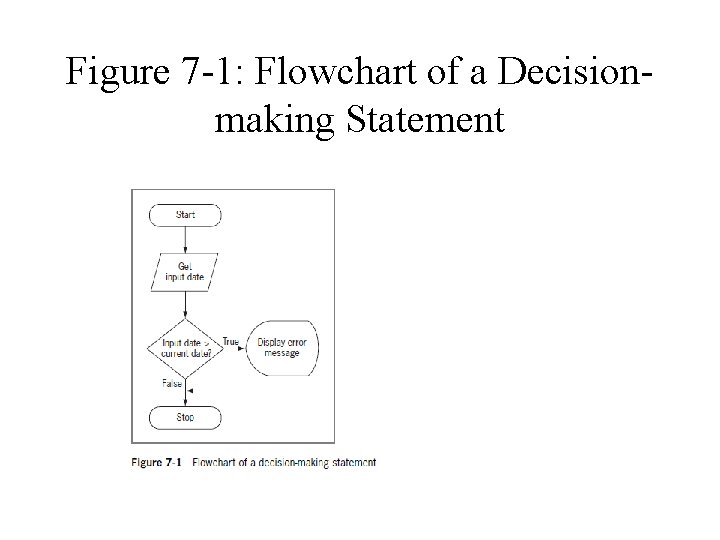
Figure 7 -1: Flowchart of a Decisionmaking Statement
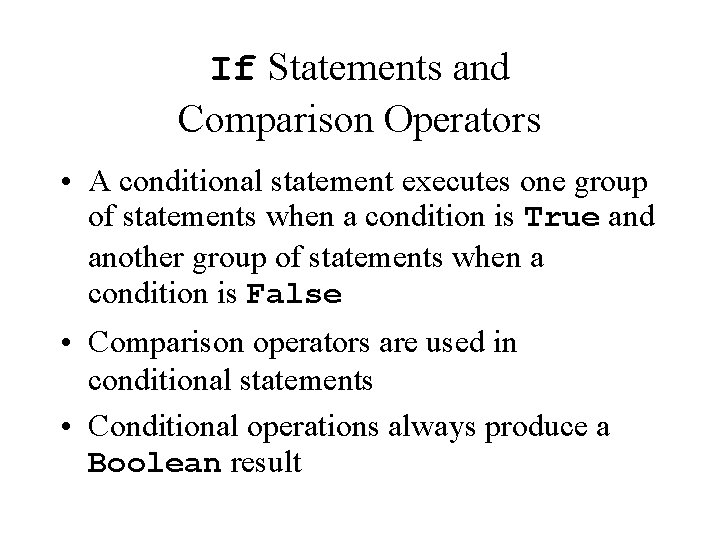
If Statements and Comparison Operators • A conditional statement executes one group of statements when a condition is True and another group of statements when a condition is False • Comparison operators are used in conditional statements • Conditional operations always produce a Boolean result
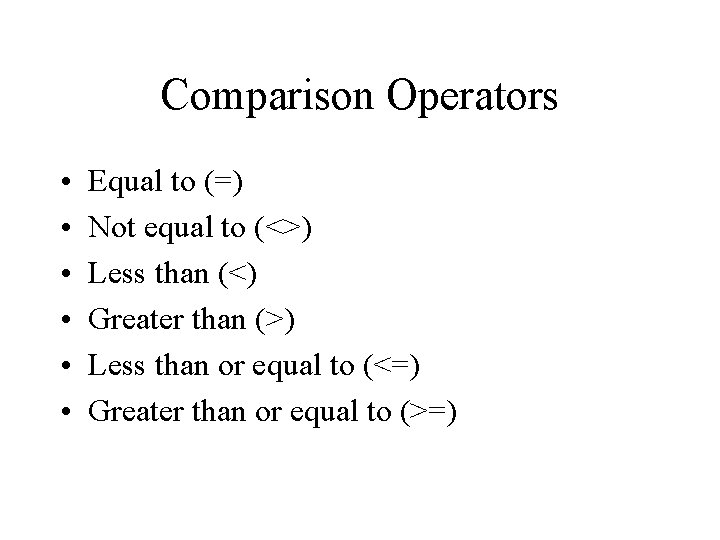
Comparison Operators • • • Equal to (=) Not equal to (<>) Less than (<) Greater than (>) Less than or equal to (<=) Greater than or equal to (>=)
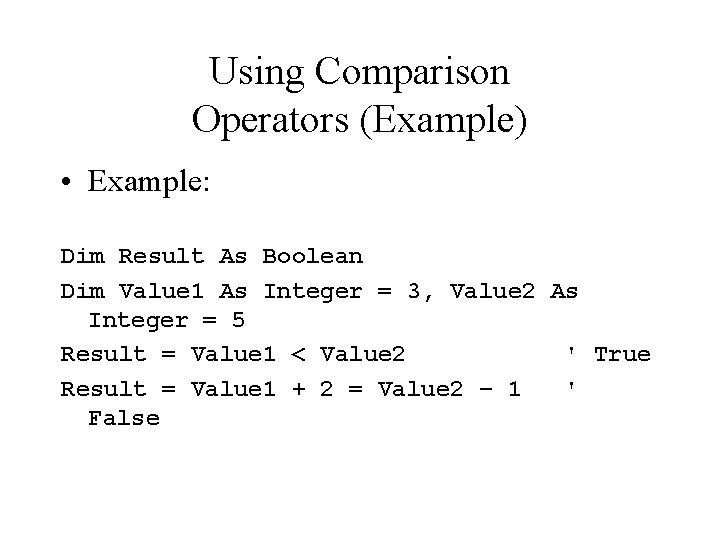
Using Comparison Operators (Example) • Example: Dim Result As Boolean Dim Value 1 As Integer = 3, Value 2 As Integer = 5 Result = Value 1 < Value 2 ' True Result = Value 1 + 2 = Value 2 – 1 ' False
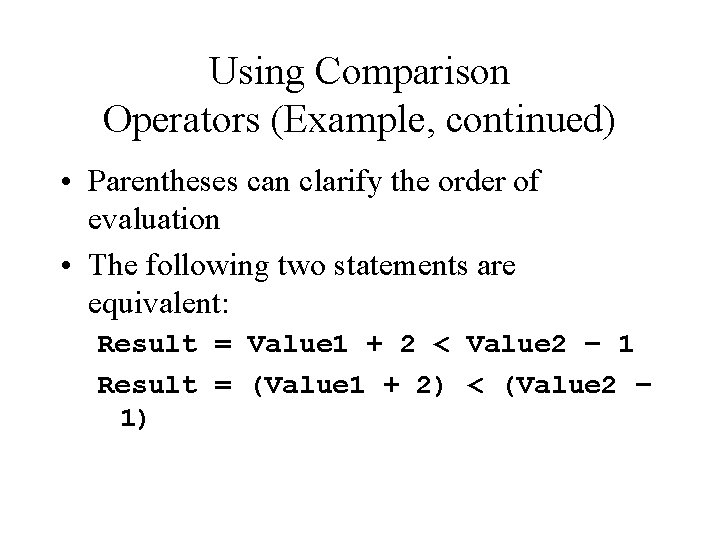
Using Comparison Operators (Example, continued) • Parentheses can clarify the order of evaluation • The following two statements are equivalent: Result = Value 1 + 2 < Value 2 – 1 Result = (Value 1 + 2) < (Value 2 – 1)
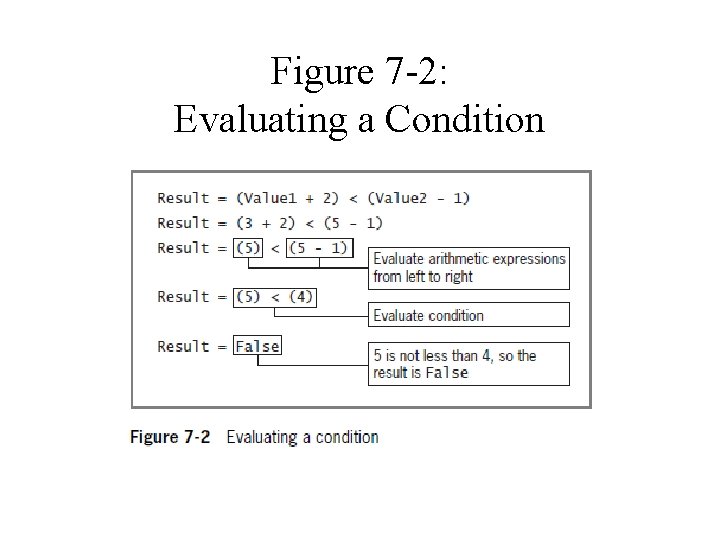
Figure 7 -2: Evaluating a Condition
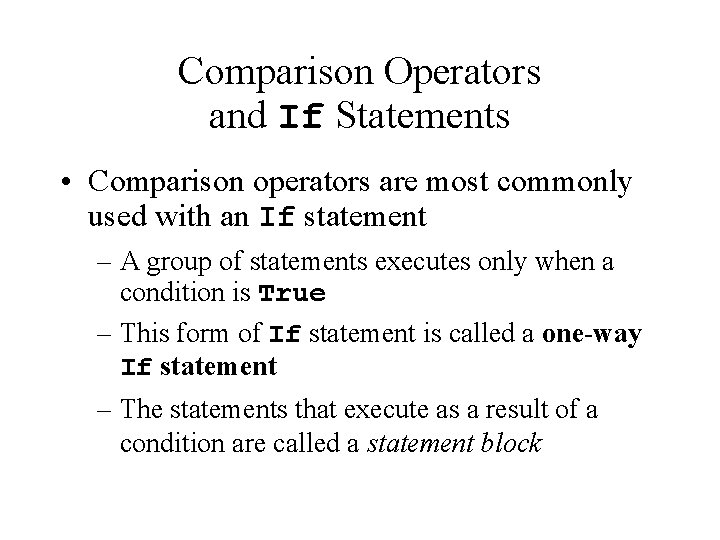
Comparison Operators and If Statements • Comparison operators are most commonly used with an If statement – A group of statements executes only when a condition is True – This form of If statement is called a one-way If statement – The statements that execute as a result of a condition are called a statement block
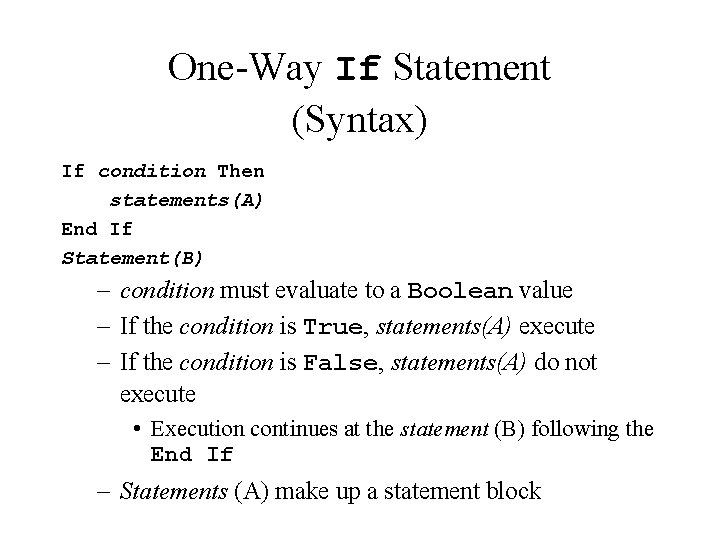
One-Way If Statement (Syntax) If condition Then statements(A) End If Statement(B) – condition must evaluate to a Boolean value – If the condition is True, statements(A) execute – If the condition is False, statements(A) do not execute • Execution continues at the statement (B) following the End If – Statements (A) make up a statement block
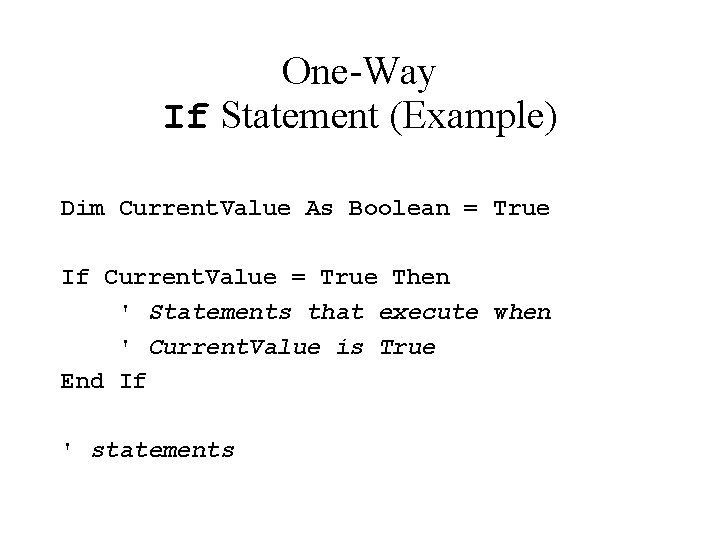
One-Way If Statement (Example) Dim Current. Value As Boolean = True If Current. Value = True Then ' Statements that execute when ' Current. Value is True End If ' statements
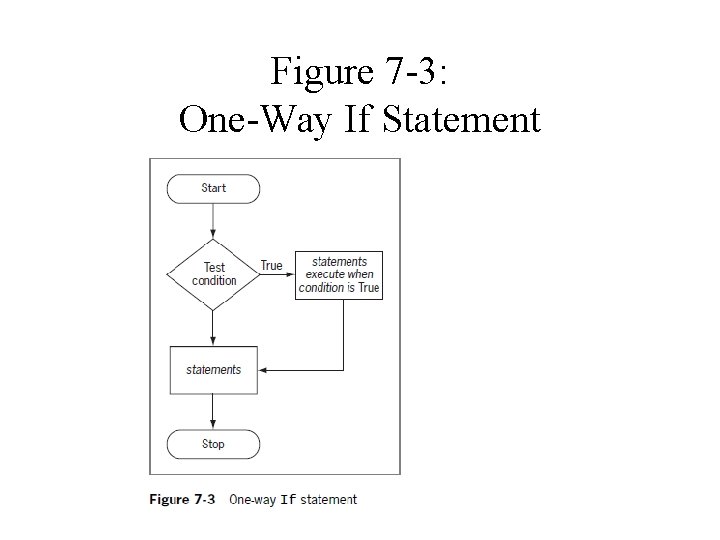
Figure 7 -3: One-Way If Statement
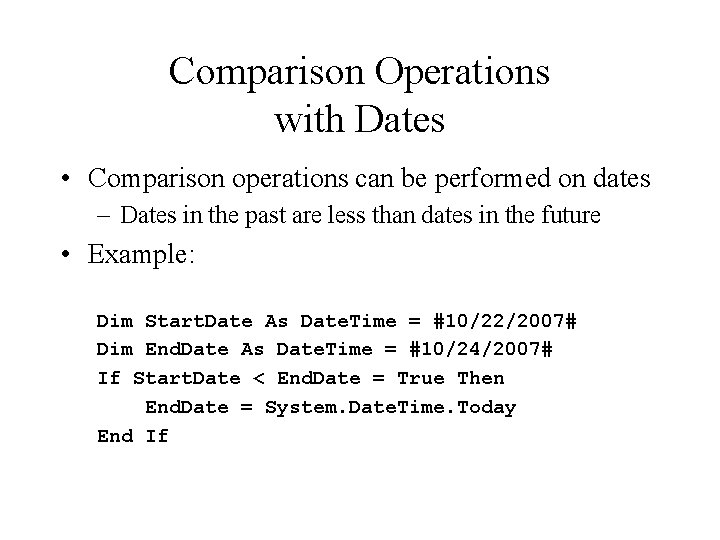
Comparison Operations with Dates • Comparison operations can be performed on dates – Dates in the past are less than dates in the future • Example: Dim Start. Date As Date. Time = #10/22/2007# Dim End. Date As Date. Time = #10/24/2007# If Start. Date < End. Date = True Then End. Date = System. Date. Time. Today End If
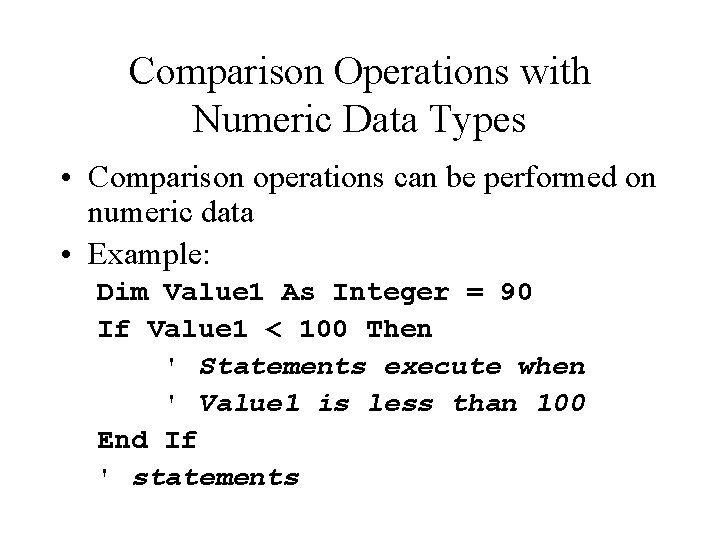
Comparison Operations with Numeric Data Types • Comparison operations can be performed on numeric data • Example: Dim Value 1 As Integer = 90 If Value 1 < 100 Then ' Statements execute when ' Value 1 is less than 100 End If ' statements
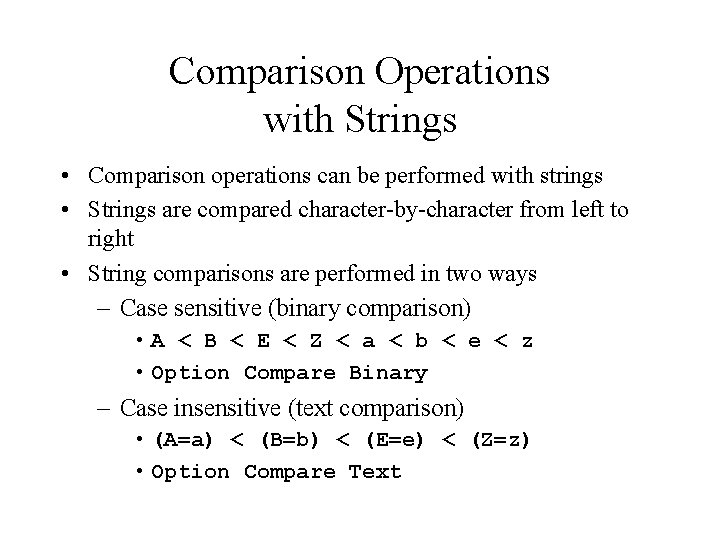
Comparison Operations with Strings • Comparison operations can be performed with strings • Strings are compared character-by-character from left to right • String comparisons are performed in two ways – Case sensitive (binary comparison) • A < B < E < Z < a < b < e < z • Option Compare Binary – Case insensitive (text comparison) • (A=a) < (B=b) < (E=e) < (Z=z) • Option Compare Text
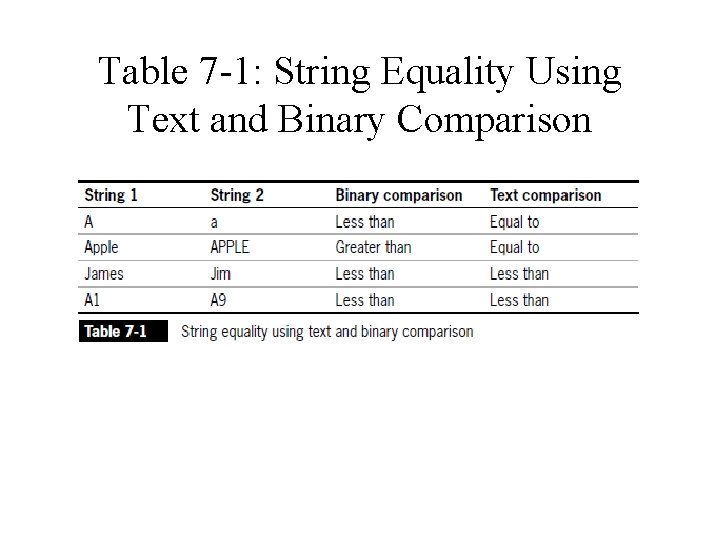
Table 7 -1: String Equality Using Text and Binary Comparison
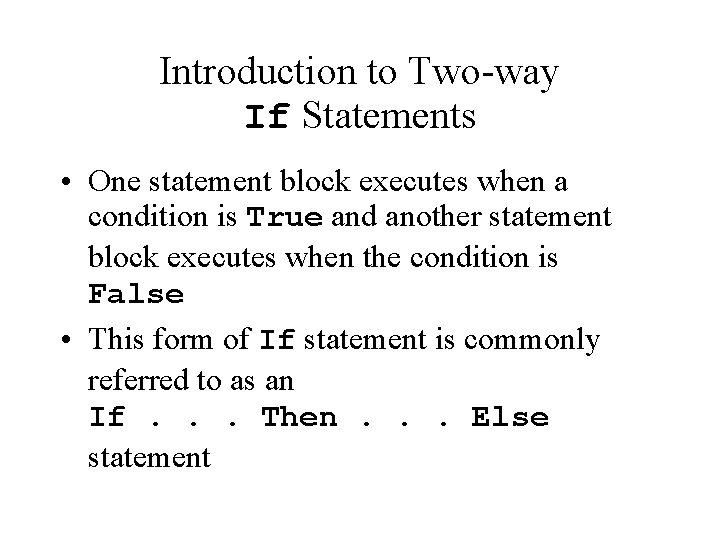
Introduction to Two-way If Statements • One statement block executes when a condition is True and another statement block executes when the condition is False • This form of If statement is commonly referred to as an If. . . Then. . . Else statement
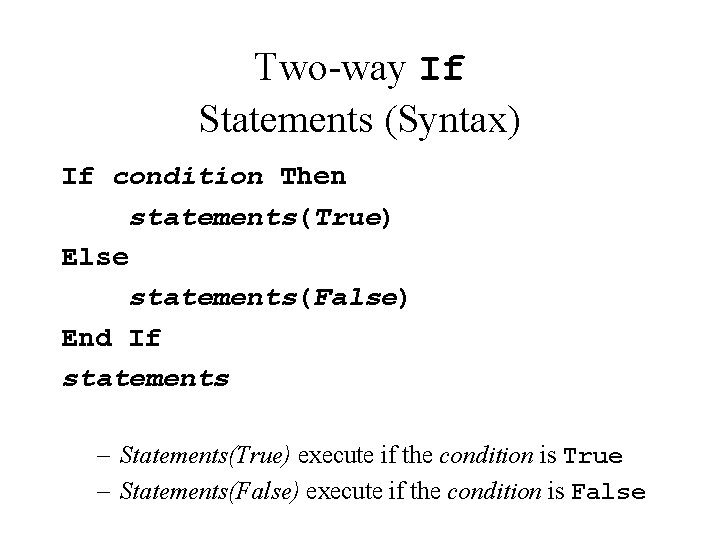
Two-way If Statements (Syntax) If condition Then statements(True) Else statements(False) End If statements – Statements(True) execute if the condition is True – Statements(False) execute if the condition is False
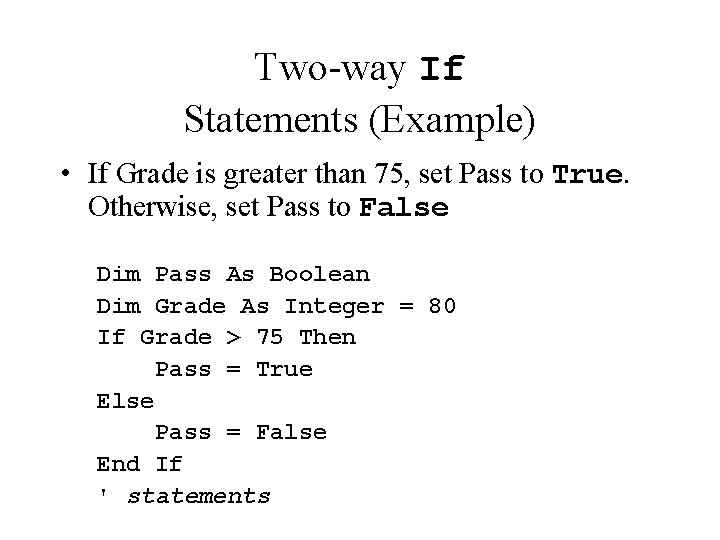
Two-way If Statements (Example) • If Grade is greater than 75, set Pass to True. Otherwise, set Pass to False Dim Pass As Boolean Dim Grade As Integer = 80 If Grade > 75 Then Pass = True Else Pass = False End If ' statements
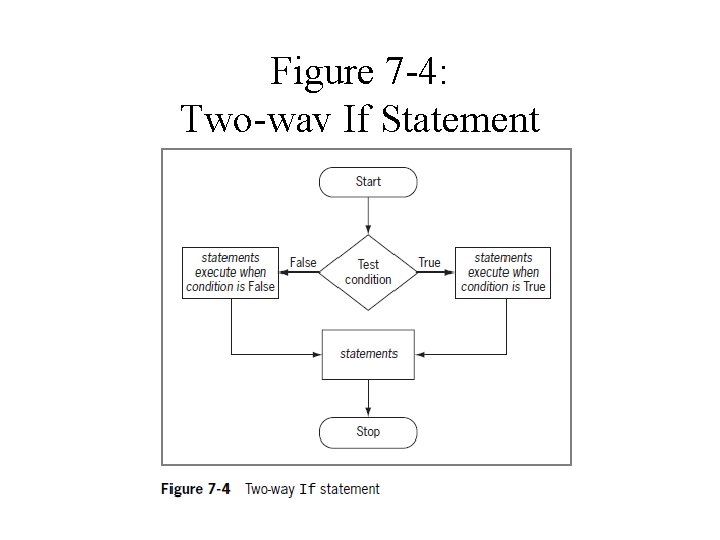
Figure 7 -4: Two-way If Statement
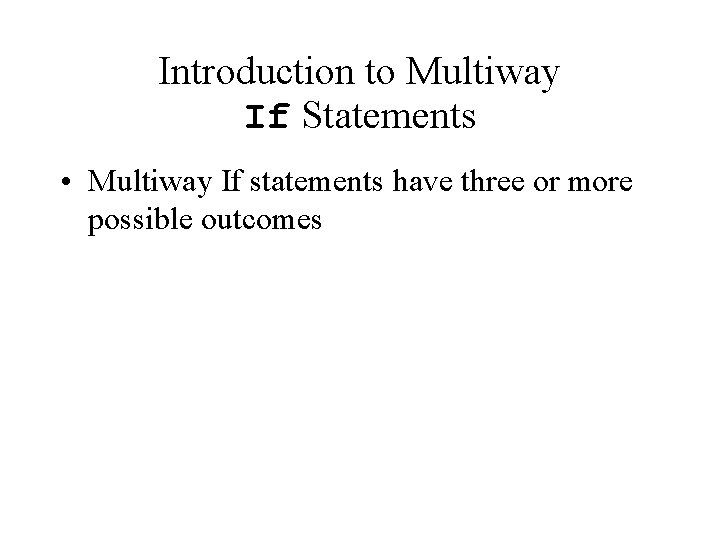
Introduction to Multiway If Statements • Multiway If statements have three or more possible outcomes
![Multiway If Statements Syntax If condition 1 Then statements Else If condition 2 Then Multiway If Statements (Syntax) If condition 1 Then [statements] [Else. If condition 2 Then](https://slidetodoc.com/presentation_image_h2/3a5d678195b084fa4240981954bf9a8b/image-26.jpg)
Multiway If Statements (Syntax) If condition 1 Then [statements] [Else. If condition 2 Then [elseif. Statements]] [Else] [else. Statements]] End If statements
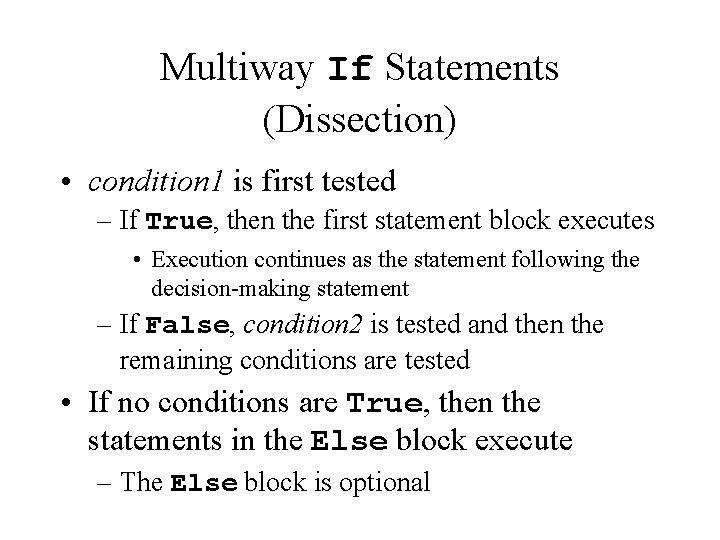
Multiway If Statements (Dissection) • condition 1 is first tested – If True, then the first statement block executes • Execution continues as the statement following the decision-making statement – If False, condition 2 is tested and then the remaining conditions are tested • If no conditions are True, then the statements in the Else block execute – The Else block is optional
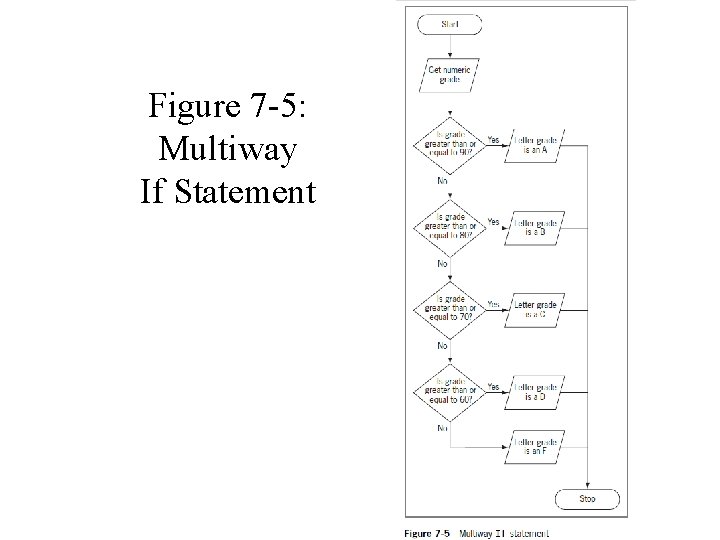
Figure 7 -5: Multiway If Statement
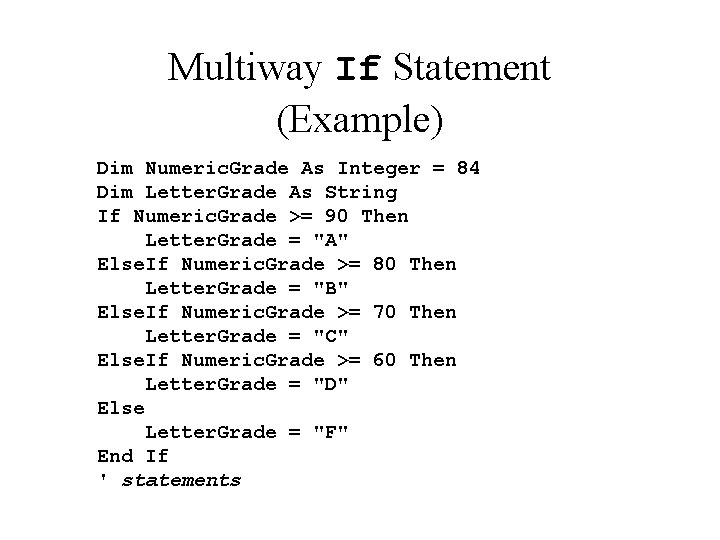
Multiway If Statement (Example) Dim Numeric. Grade As Integer = 84 Dim Letter. Grade As String If Numeric. Grade >= 90 Then Letter. Grade = "A" Else. If Numeric. Grade >= 80 Then Letter. Grade = "B" Else. If Numeric. Grade >= 70 Then Letter. Grade = "C" Else. If Numeric. Grade >= 60 Then Letter. Grade = "D" Else Letter. Grade = "F" End If ' statements
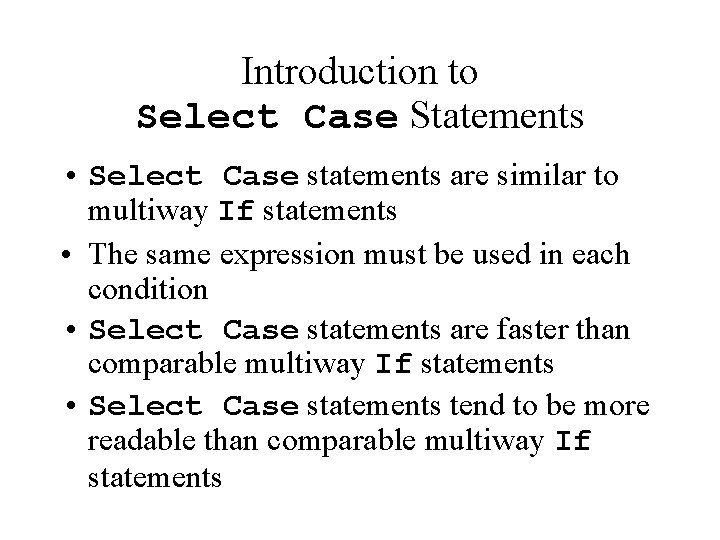
Introduction to Select Case Statements • Select Case statements are similar to multiway If statements • The same expression must be used in each condition • Select Case statements are faster than comparable multiway If statements • Select Case statements tend to be more readable than comparable multiway If statements
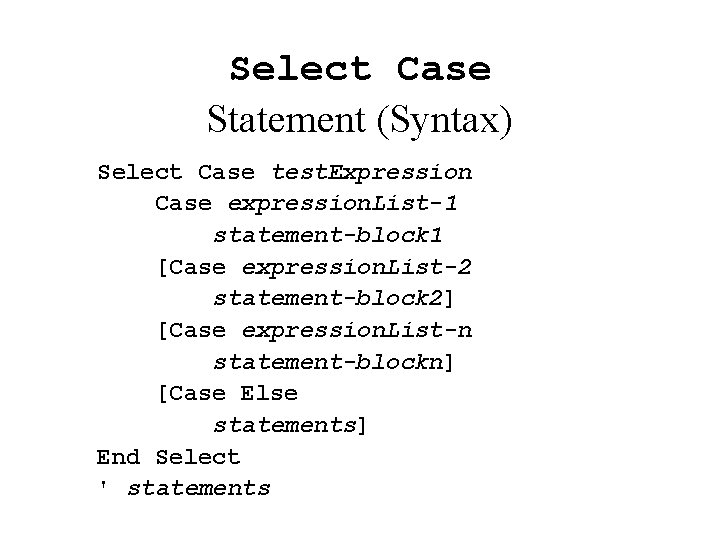
Select Case Statement (Syntax) Select Case test. Expression Case expression. List-1 statement-block 1 [Case expression. List-2 statement-block 2] [Case expression. List-n statement-blockn] [Case Else statements] End Select ' statements
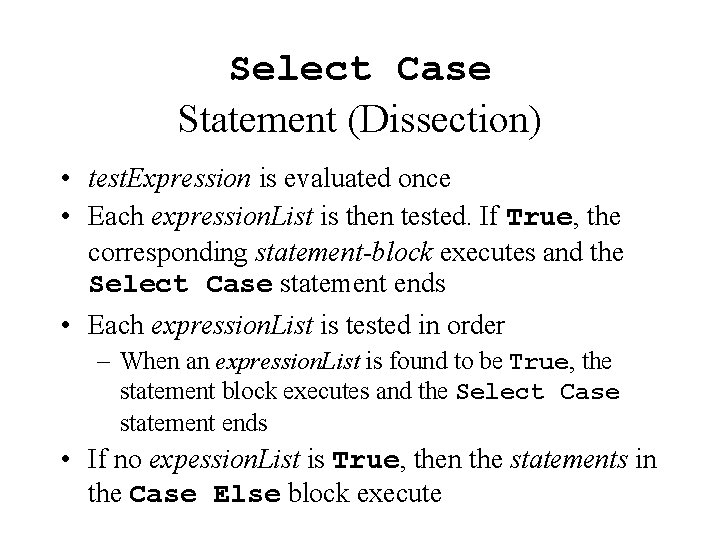
Select Case Statement (Dissection) • test. Expression is evaluated once • Each expression. List is then tested. If True, the corresponding statement-block executes and the Select Case statement ends • Each expression. List is tested in order – When an expression. List is found to be True, the statement block executes and the Select Case statement ends • If no expession. List is True, then the statements in the Case Else block execute
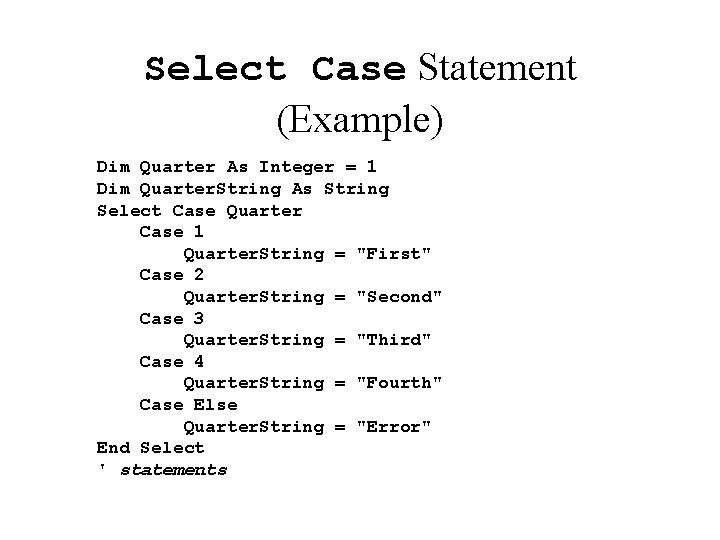
Select Case Statement (Example) Dim Quarter As Integer = 1 Dim Quarter. String As String Select Case Quarter Case 1 Quarter. String = "First" Case 2 Quarter. String = "Second" Case 3 Quarter. String = "Third" Case 4 Quarter. String = "Fourth" Case Else Quarter. String = "Error" End Select ' statements
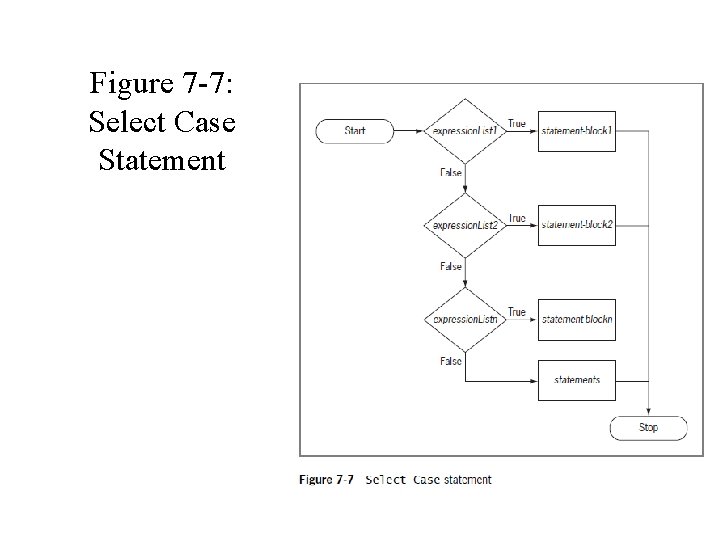
Figure 7 -7: Select Case Statement
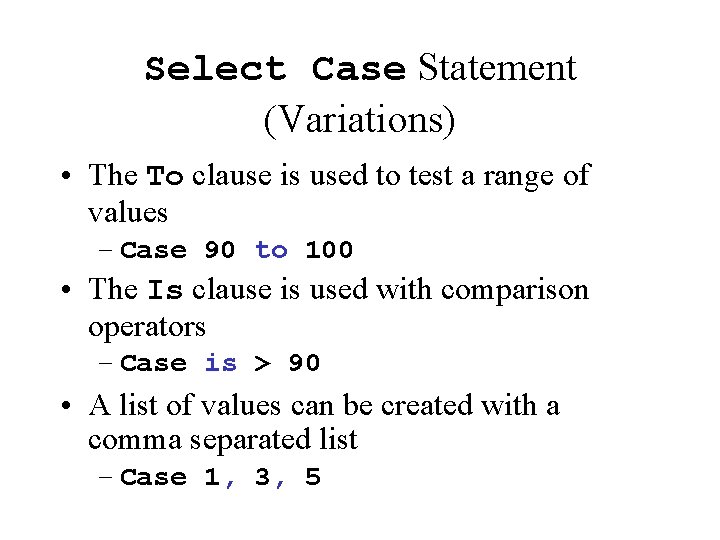
Select Case Statement (Variations) • The To clause is used to test a range of values – Case 90 to 100 • The Is clause is used with comparison operators – Case is > 90 • A list of values can be created with a comma separated list – Case 1, 3, 5
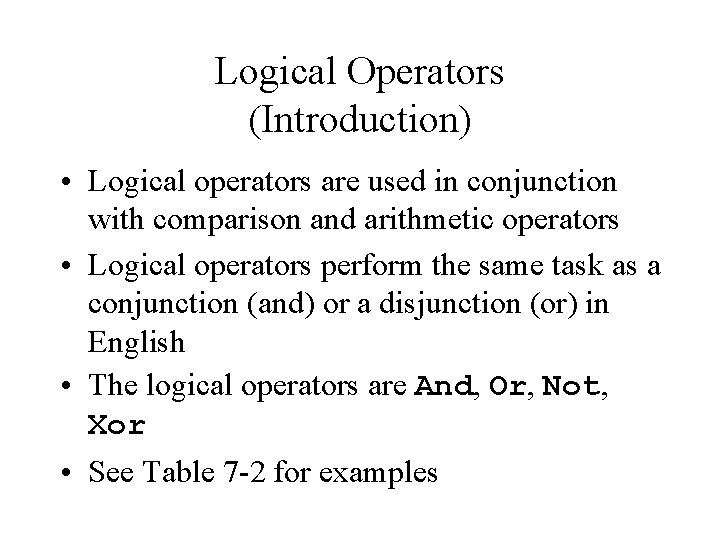
Logical Operators (Introduction) • Logical operators are used in conjunction with comparison and arithmetic operators • Logical operators perform the same task as a conjunction (and) or a disjunction (or) in English • The logical operators are And, Or, Not, Xor • See Table 7 -2 for examples
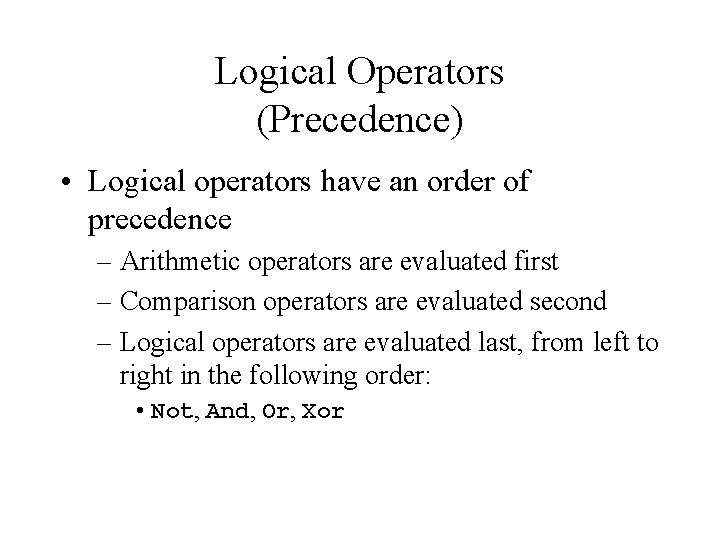
Logical Operators (Precedence) • Logical operators have an order of precedence – Arithmetic operators are evaluated first – Comparison operators are evaluated second – Logical operators are evaluated last, from left to right in the following order: • Not, And, Or, Xor
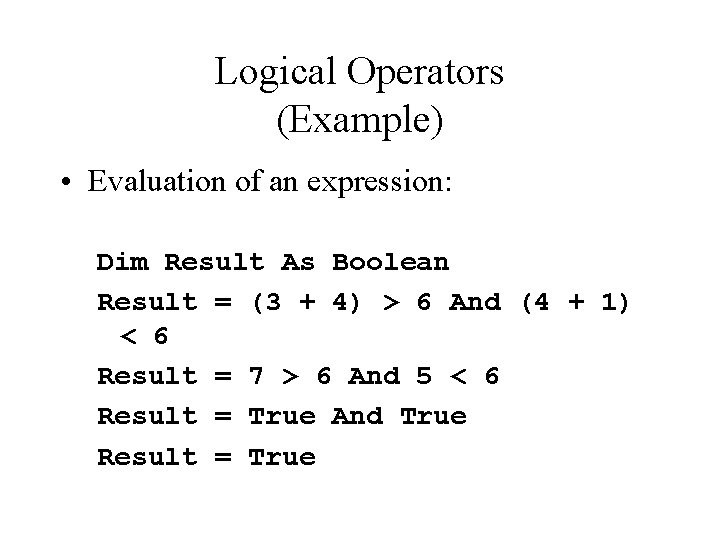
Logical Operators (Example) • Evaluation of an expression: Dim Result As Boolean Result = (3 + 4) > 6 And (4 + 1) < 6 Result = 7 > 6 And 5 < 6 Result = True And True Result = True
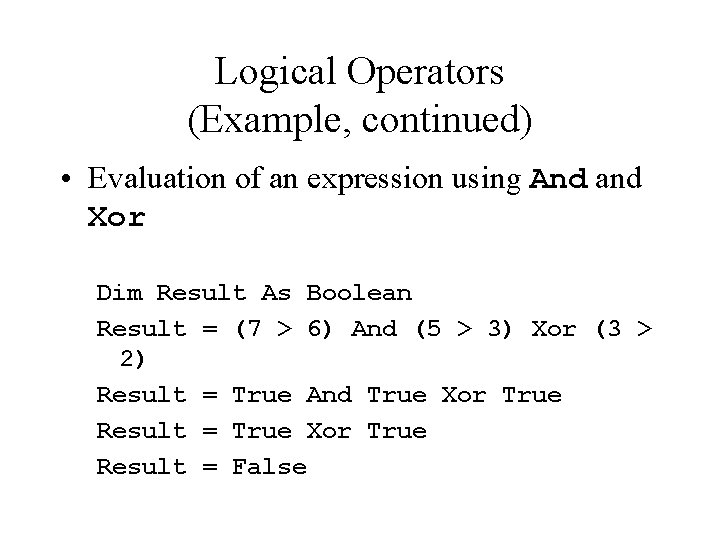
Logical Operators (Example, continued) • Evaluation of an expression using And and Xor Dim Result As Boolean Result = (7 > 6) And (5 > 3) Xor (3 > 2) Result = True And True Xor True Result = False
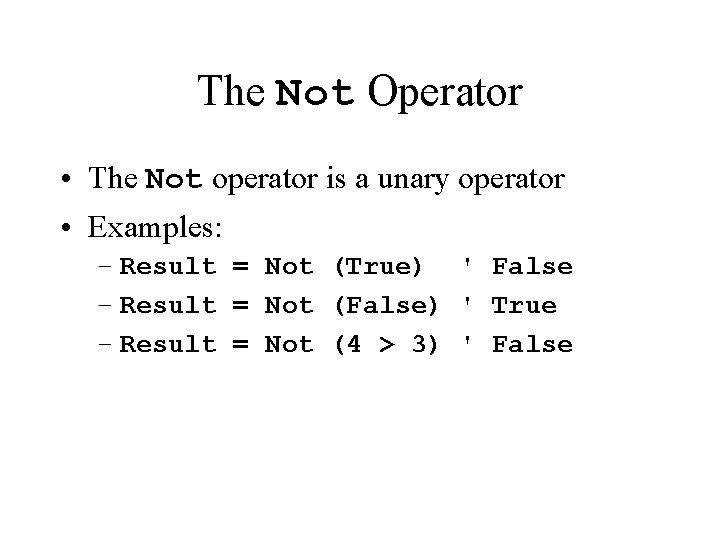
The Not Operator • The Not operator is a unary operator • Examples: – Result = Not (True) ' False – Result = Not (False) ' True – Result = Not (4 > 3) ' False
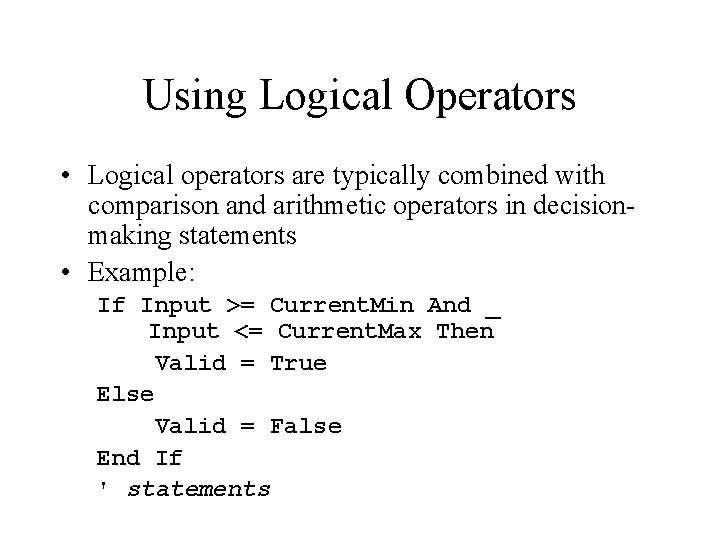
Using Logical Operators • Logical operators are typically combined with comparison and arithmetic operators in decisionmaking statements • Example: If Input >= Current. Min And _ Input <= Current. Max Then Valid = True Else Valid = False End If ' statements
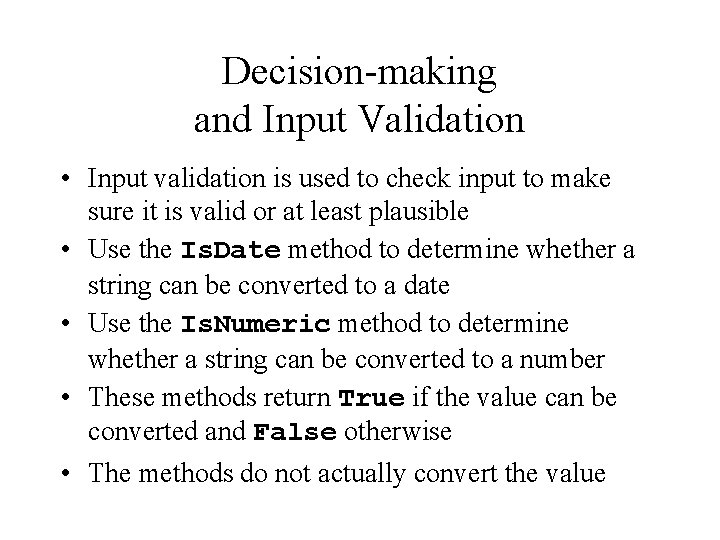
Decision-making and Input Validation • Input validation is used to check input to make sure it is valid or at least plausible • Use the Is. Date method to determine whether a string can be converted to a date • Use the Is. Numeric method to determine whether a string can be converted to a number • These methods return True if the value can be converted and False otherwise • The methods do not actually convert the value
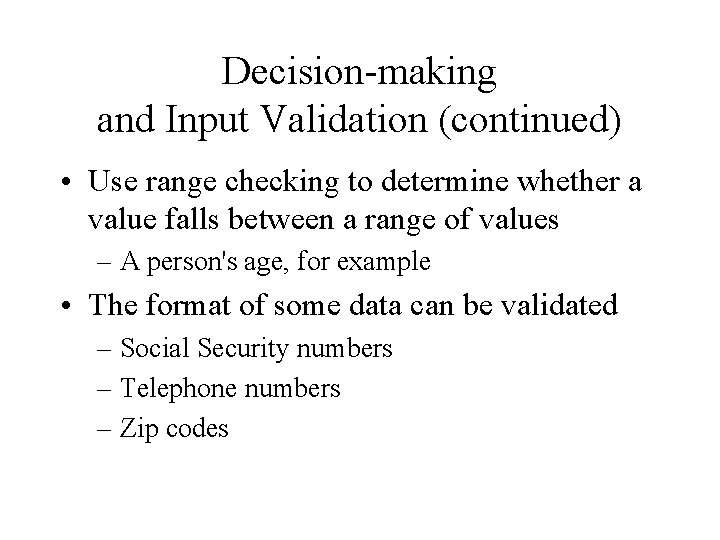
Decision-making and Input Validation (continued) • Use range checking to determine whether a value falls between a range of values – A person's age, for example • The format of some data can be validated – Social Security numbers – Telephone numbers – Zip codes
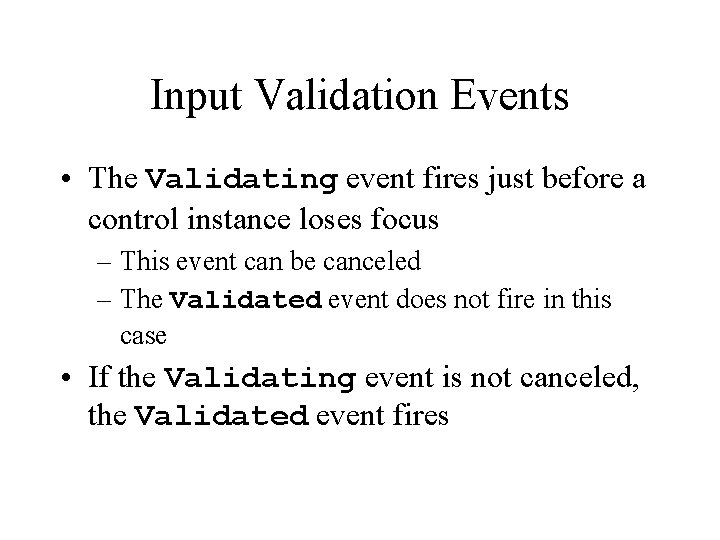
Input Validation Events • The Validating event fires just before a control instance loses focus – This event can be canceled – The Validated event does not fire in this case • If the Validating event is not canceled, the Validated event fires
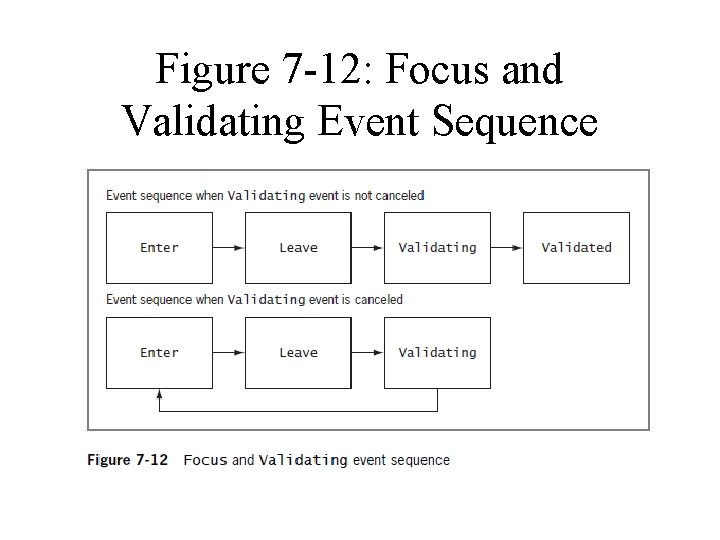
Figure 7 -12: Focus and Validating Event Sequence
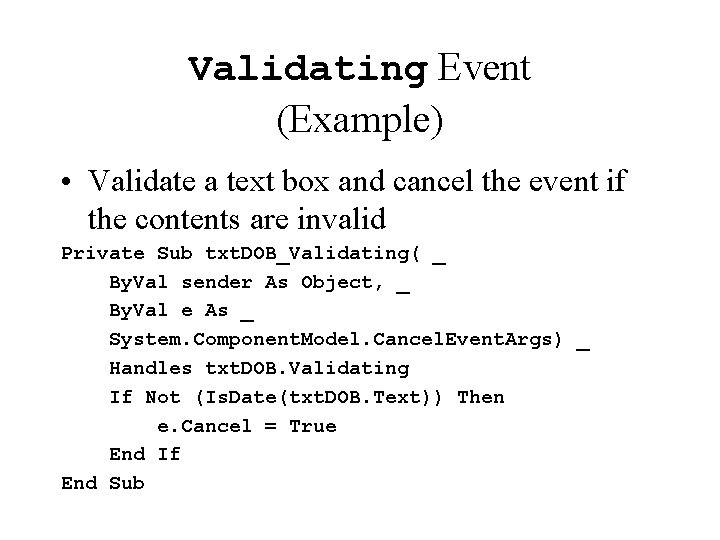
Validating Event (Example) • Validate a text box and cancel the event if the contents are invalid Private Sub txt. DOB_Validating( _ By. Val sender As Object, _ By. Val e As _ System. Component. Model. Cancel. Event. Args) _ Handles txt. DOB. Validating If Not (Is. Date(txt. DOB. Text)) Then e. Cancel = True End If End Sub
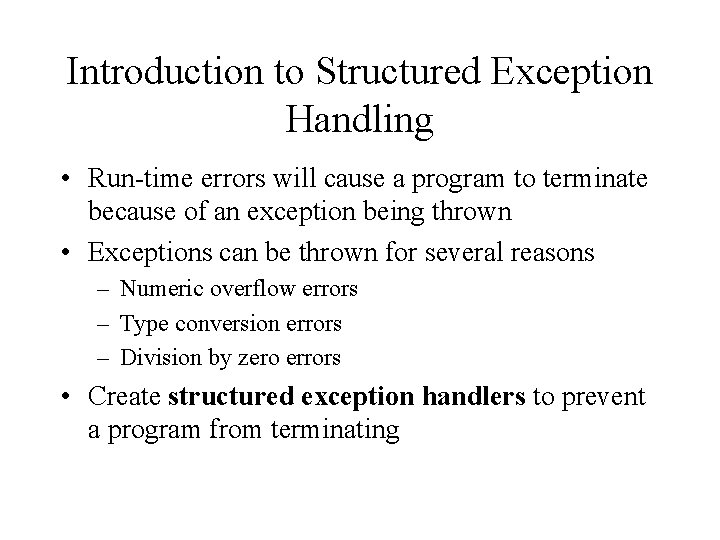
Introduction to Structured Exception Handling • Run-time errors will cause a program to terminate because of an exception being thrown • Exceptions can be thrown for several reasons – Numeric overflow errors – Type conversion errors – Division by zero errors • Create structured exception handlers to prevent a program from terminating
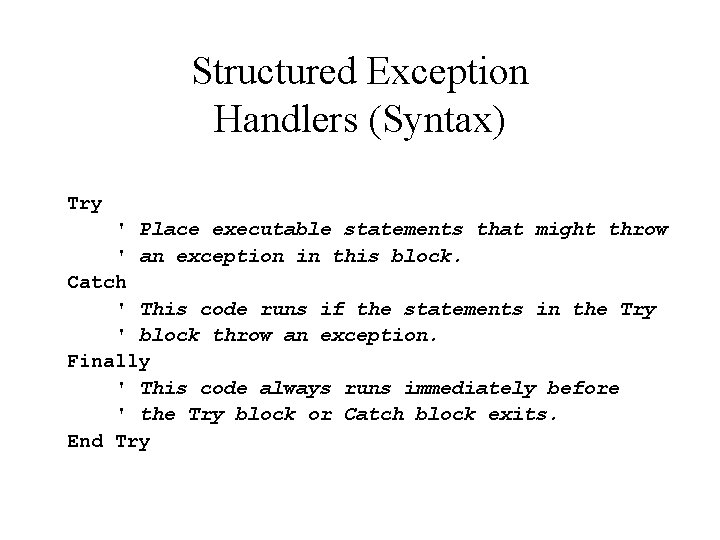
Structured Exception Handlers (Syntax) Try ' Place executable statements that might throw ' an exception in this block. Catch ' This code runs if the statements in the Try ' block throw an exception. Finally ' This code always runs immediately before ' the Try block or Catch block exits. End Try
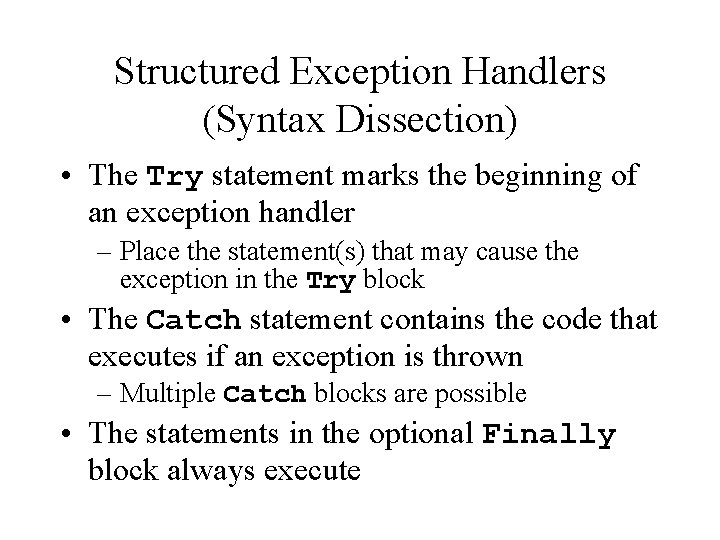
Structured Exception Handlers (Syntax Dissection) • The Try statement marks the beginning of an exception handler – Place the statement(s) that may cause the exception in the Try block • The Catch statement contains the code that executes if an exception is thrown – Multiple Catch blocks are possible • The statements in the optional Finally block always execute
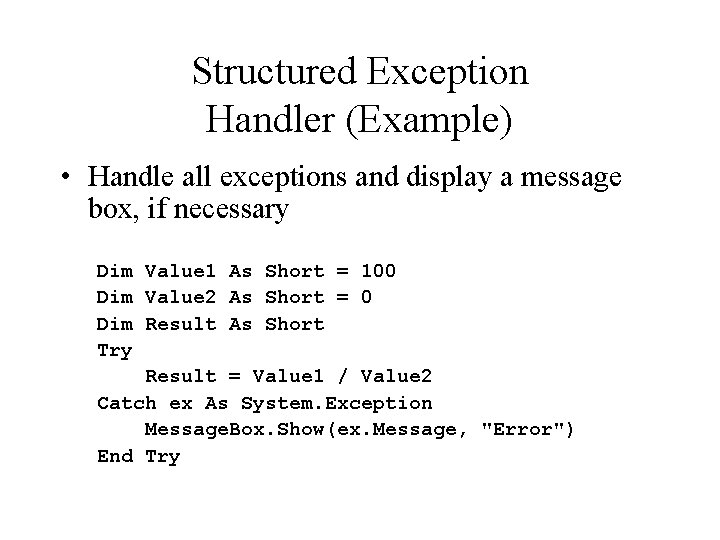
Structured Exception Handler (Example) • Handle all exceptions and display a message box, if necessary Dim Value 1 As Short = 100 Dim Value 2 As Short = 0 Dim Result As Short Try Result = Value 1 / Value 2 Catch ex As System. Exception Message. Box. Show(ex. Message, "Error") End Try
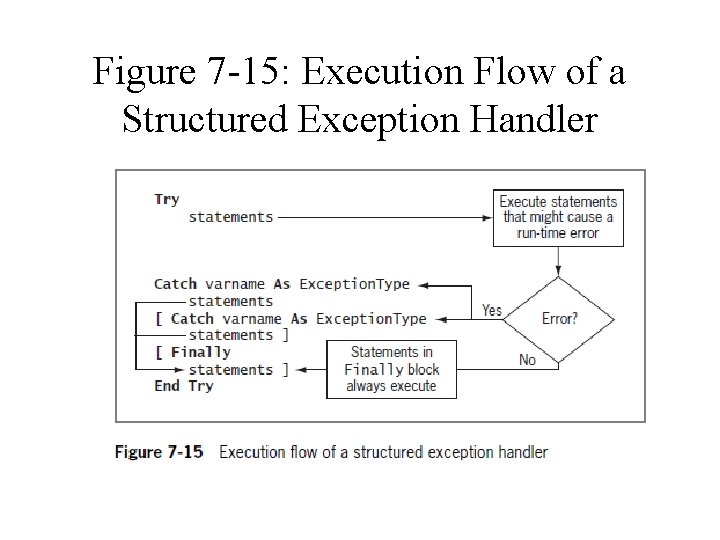
Figure 7 -15: Execution Flow of a Structured Exception Handler
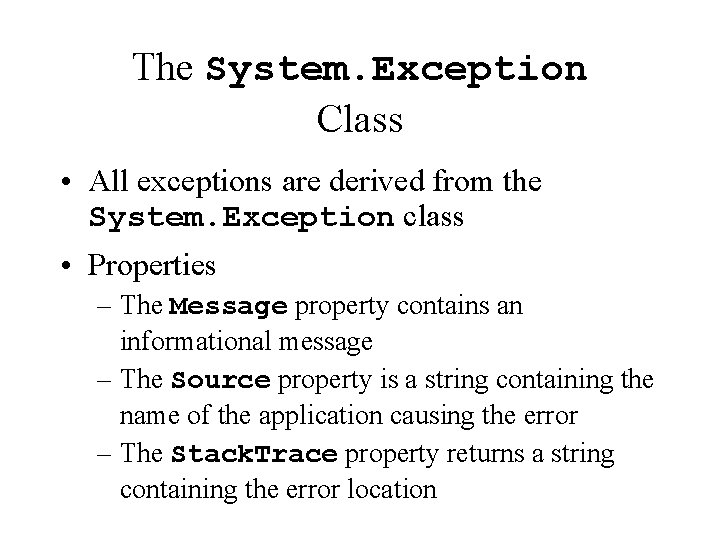
The System. Exception Class • All exceptions are derived from the System. Exception class • Properties – The Message property contains an informational message – The Source property is a string containing the name of the application causing the error – The Stack. Trace property returns a string containing the error location
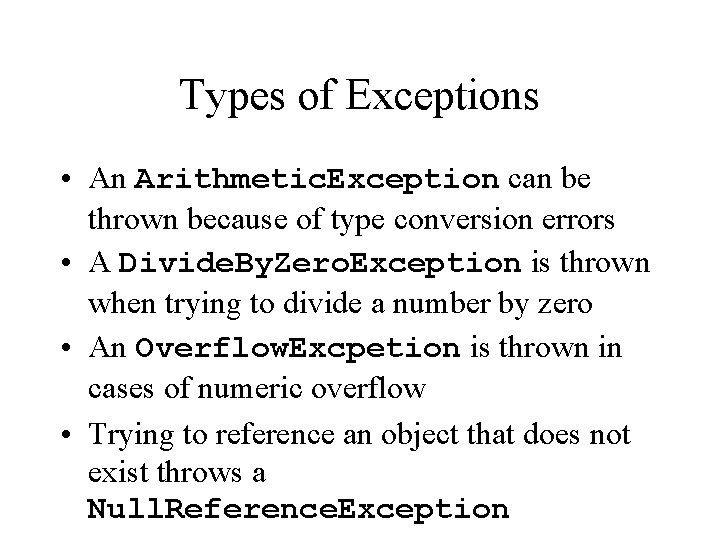
Types of Exceptions • An Arithmetic. Exception can be thrown because of type conversion errors • A Divide. By. Zero. Exception is thrown when trying to divide a number by zero • An Overflow. Excpetion is thrown in cases of numeric overflow • Trying to reference an object that does not exist throws a Null. Reference. Exception