Chapter 4 Stacks www asyrani com This is
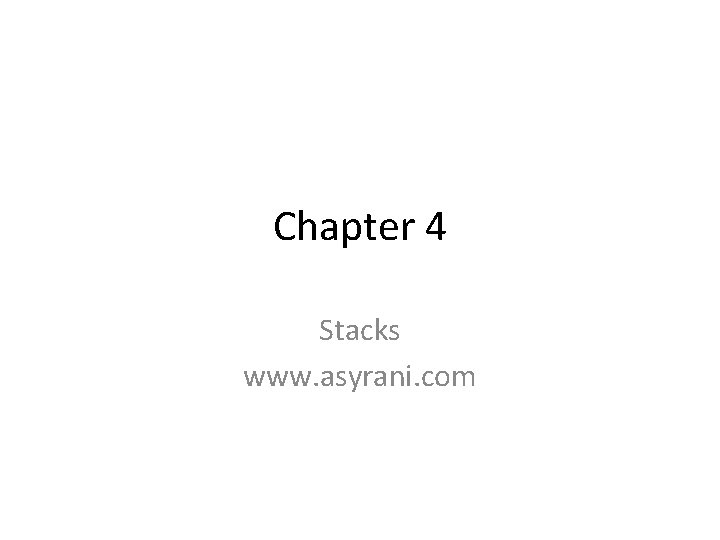
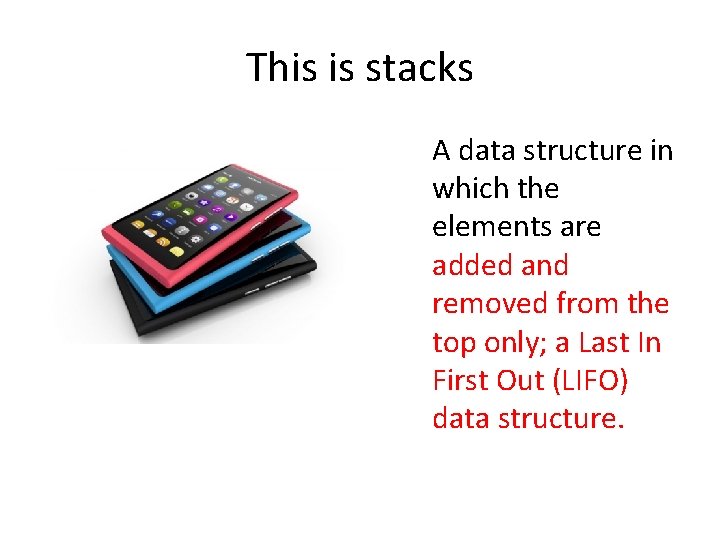
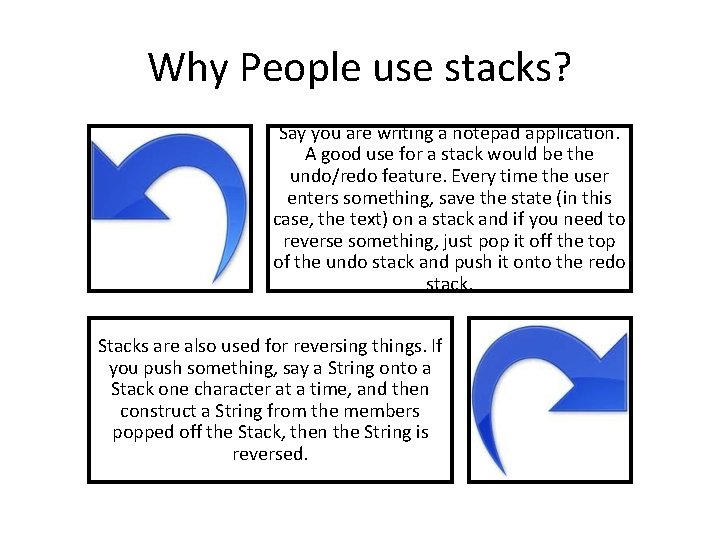
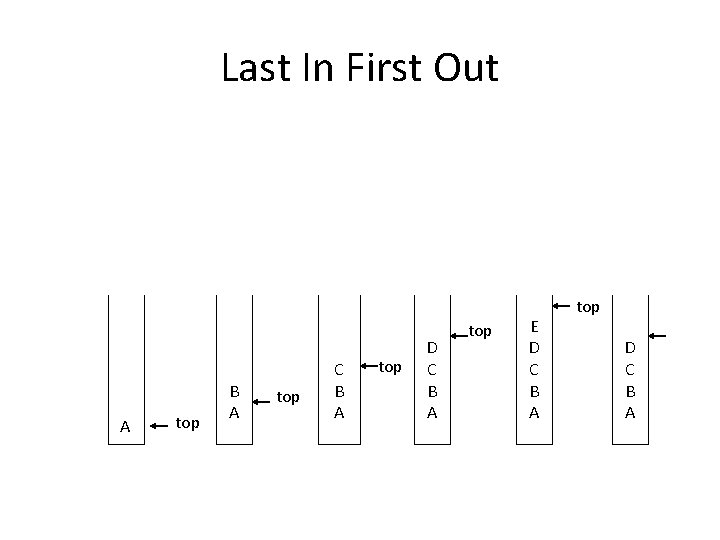
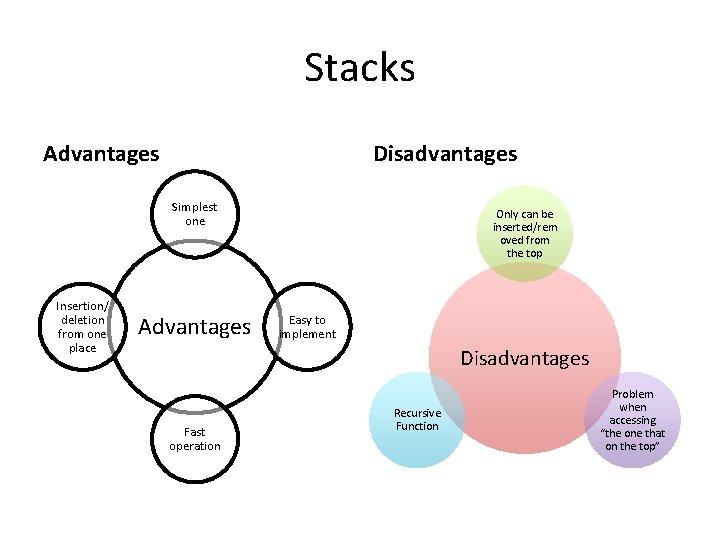
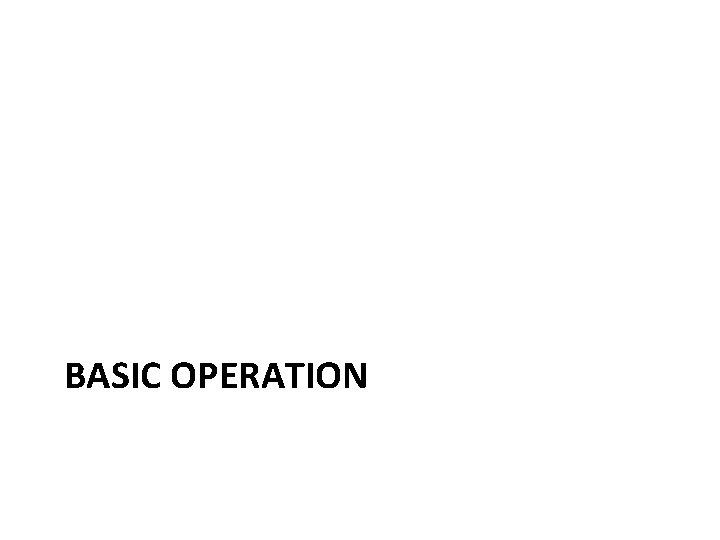
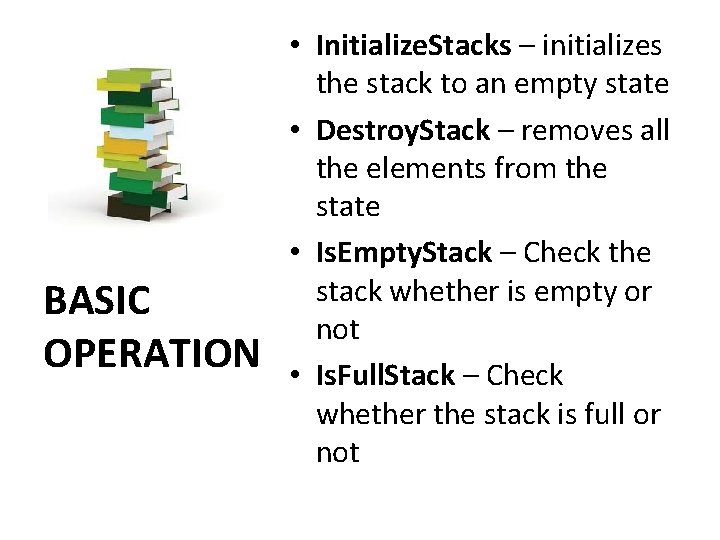
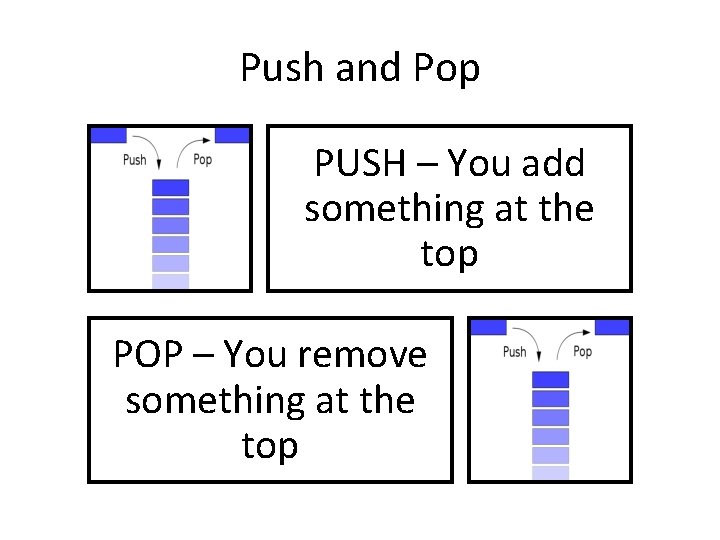
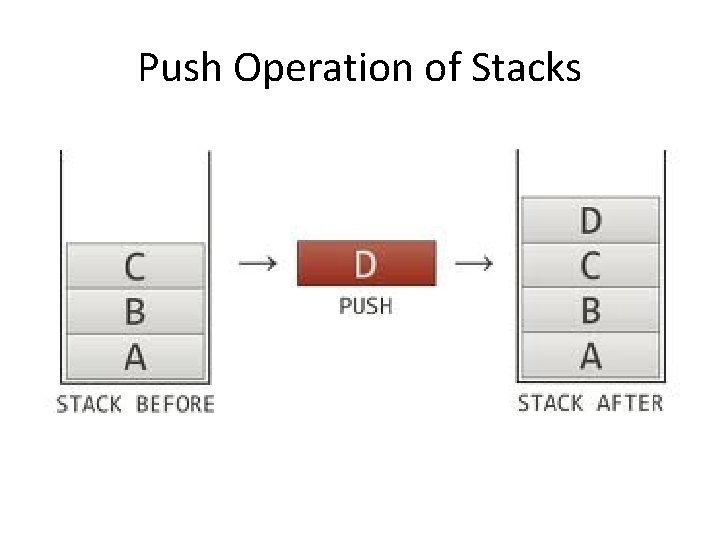
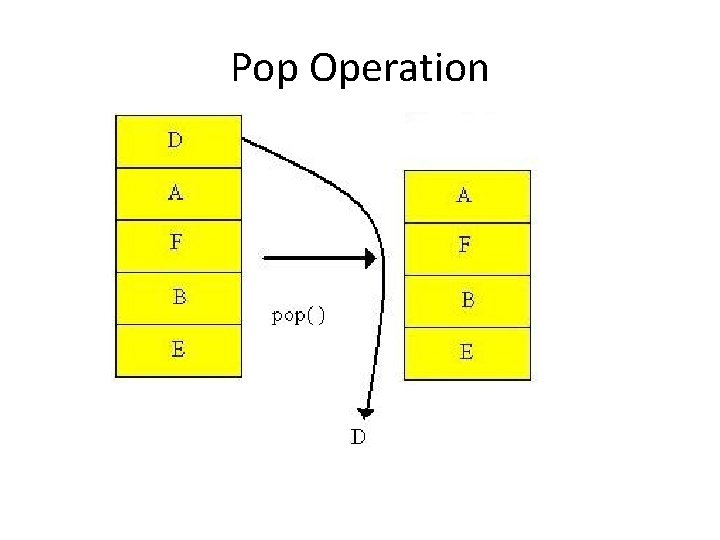
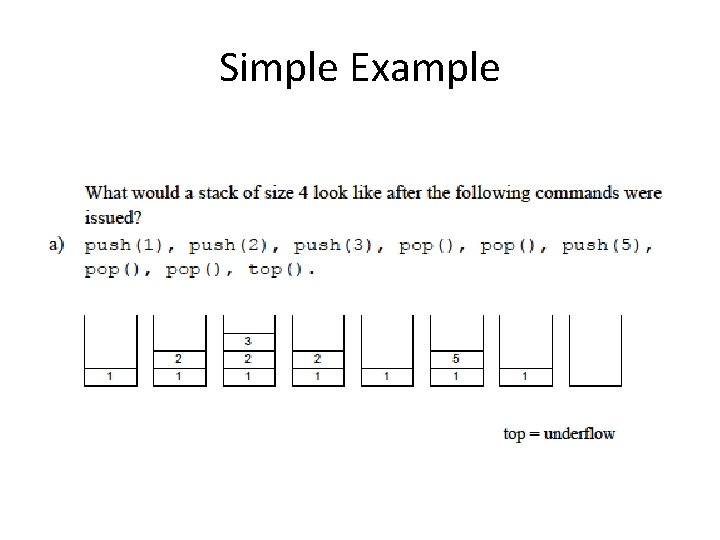
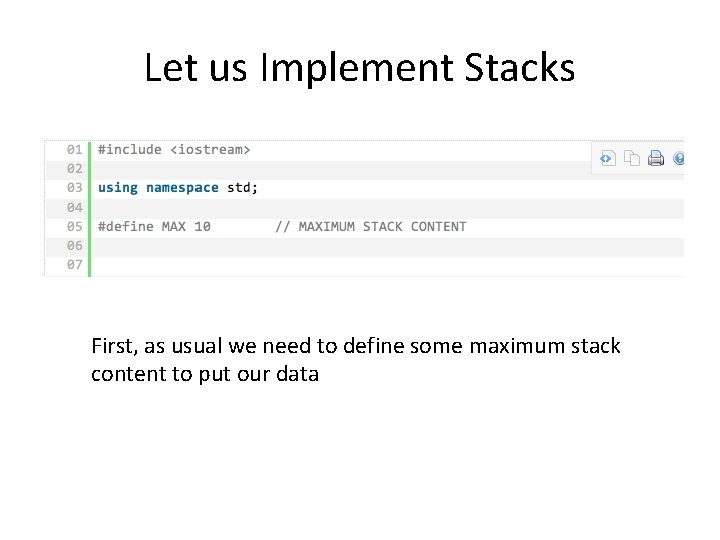
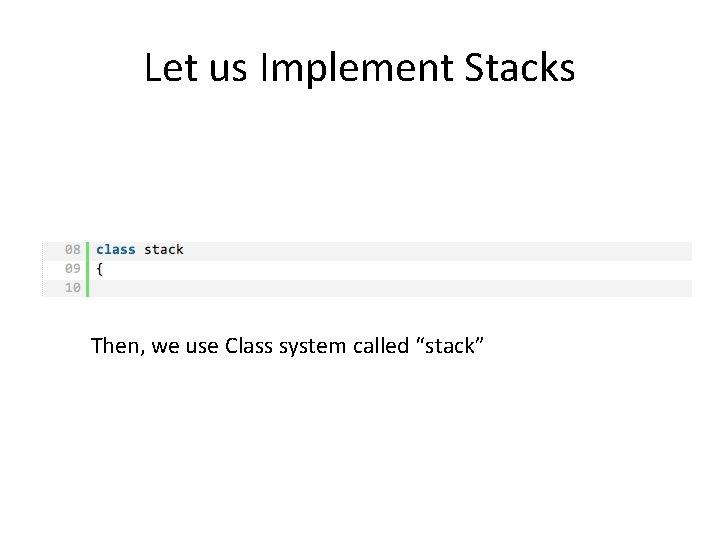
![Let us Implement Stacks We declare a private inside “stack” class - arr[MAX] : Let us Implement Stacks We declare a private inside “stack” class - arr[MAX] :](https://slidetodoc.com/presentation_image_h/ae95104481aac74b58d6ffee20c98e95/image-14.jpg)
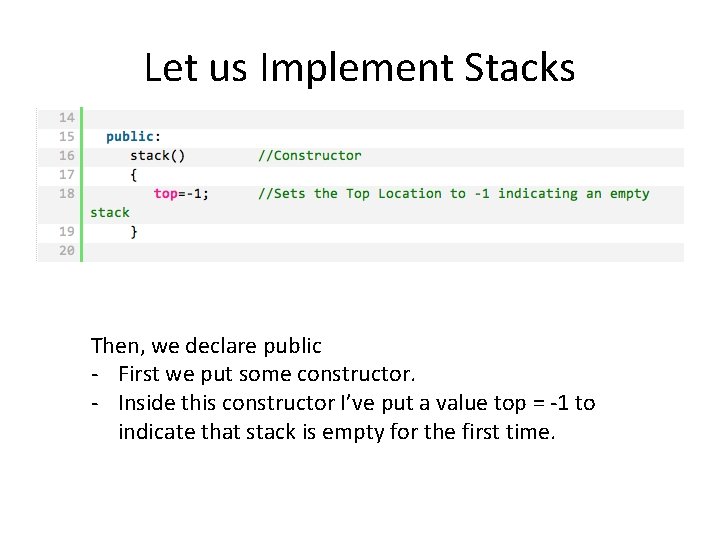
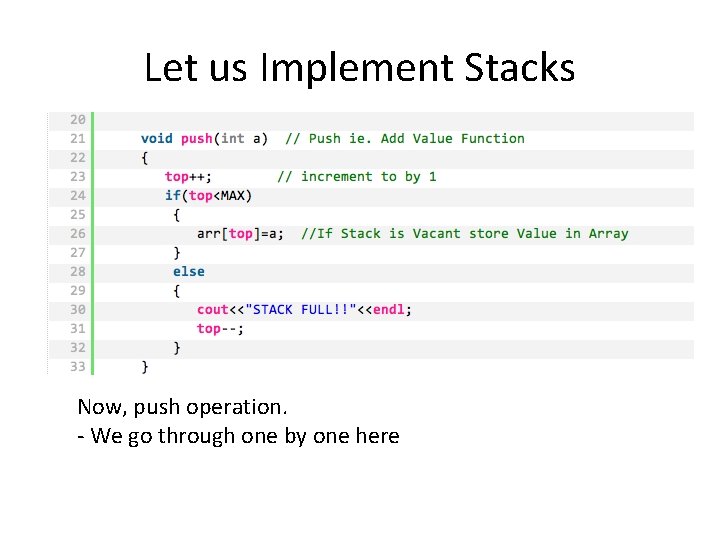
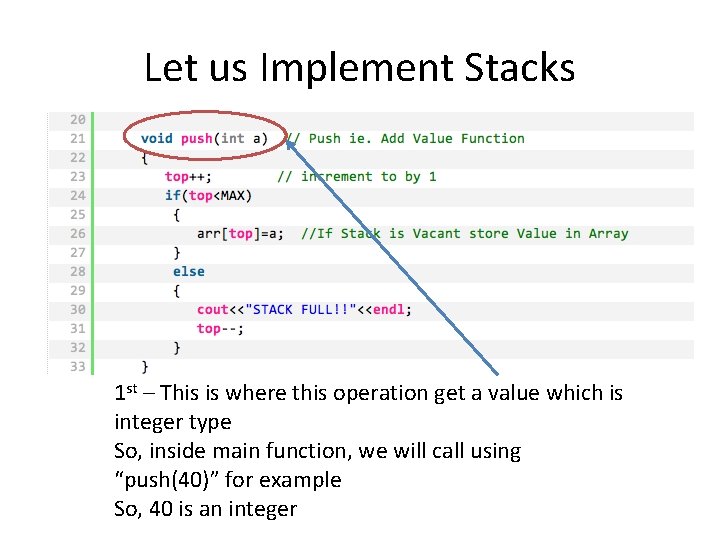
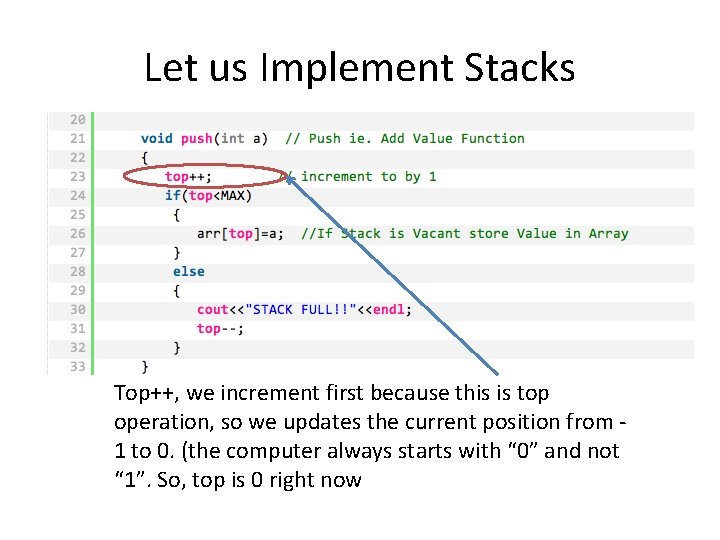
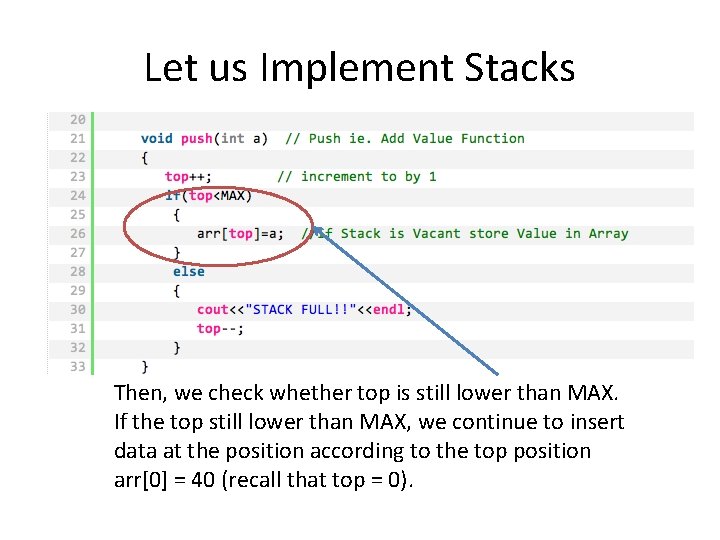
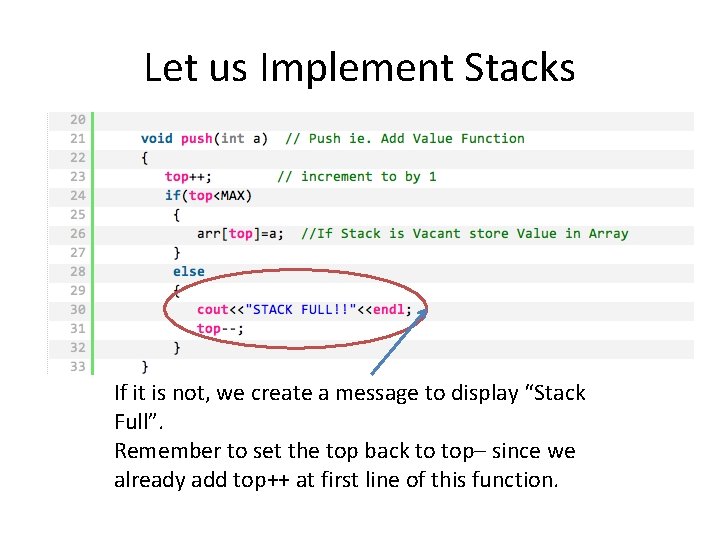
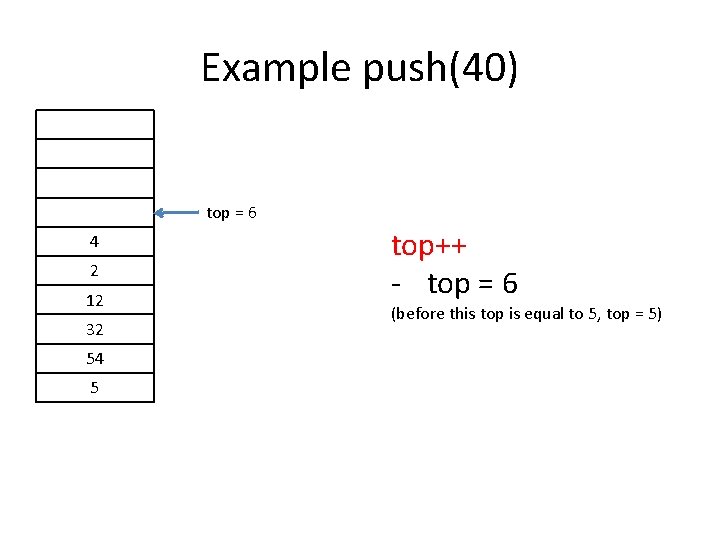
![Example push(40) top = 6 4 2 12 32 54 5 if(top<MAX) { arr[top]=a; Example push(40) top = 6 4 2 12 32 54 5 if(top<MAX) { arr[top]=a;](https://slidetodoc.com/presentation_image_h/ae95104481aac74b58d6ffee20c98e95/image-22.jpg)
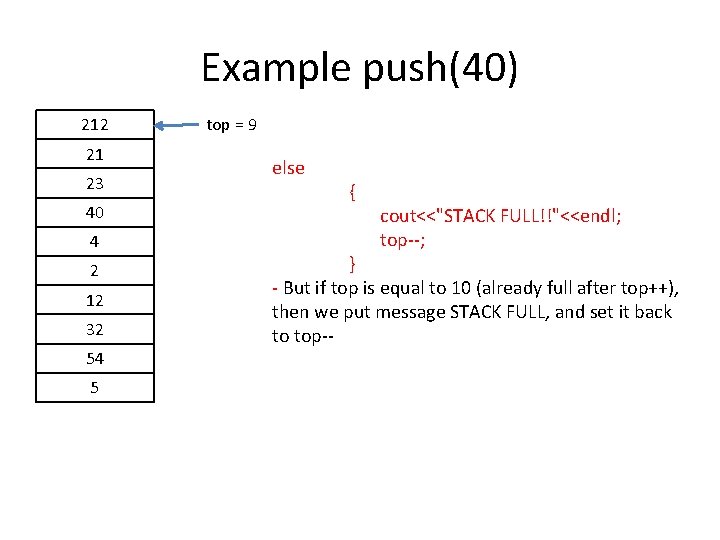
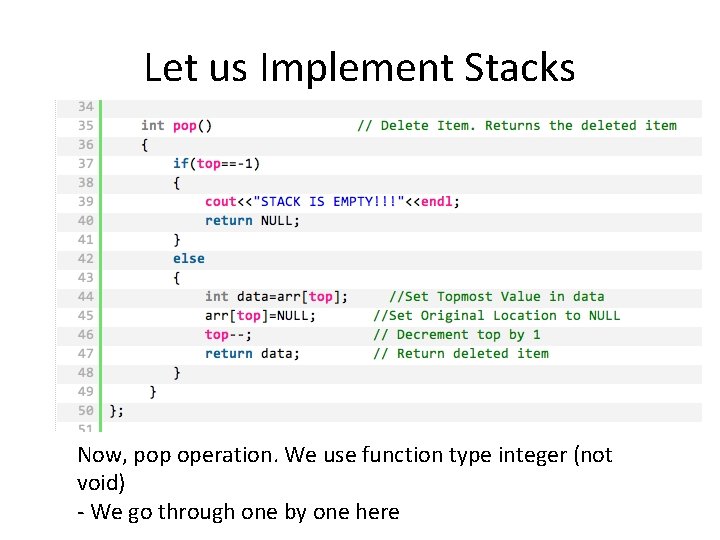
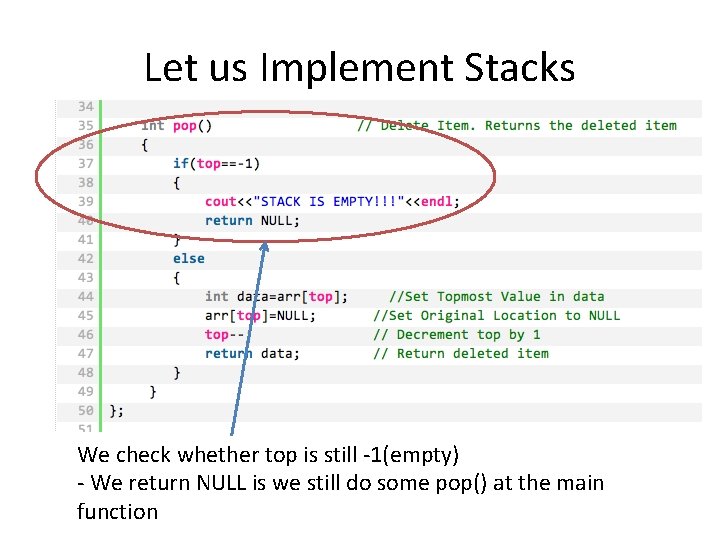
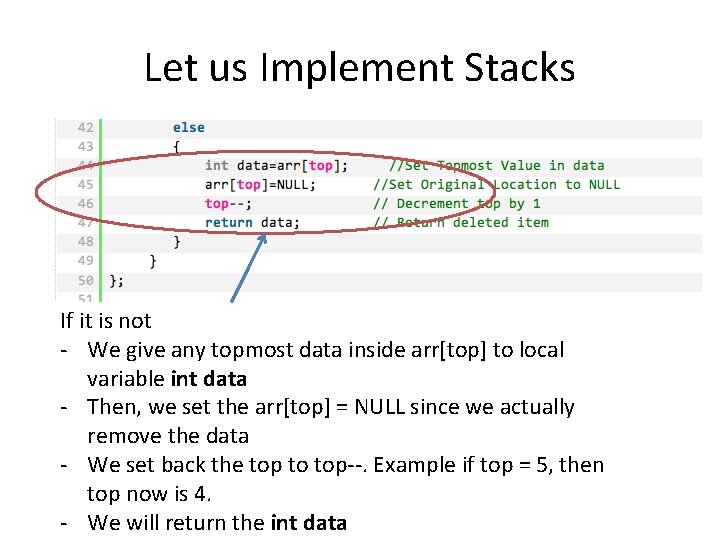
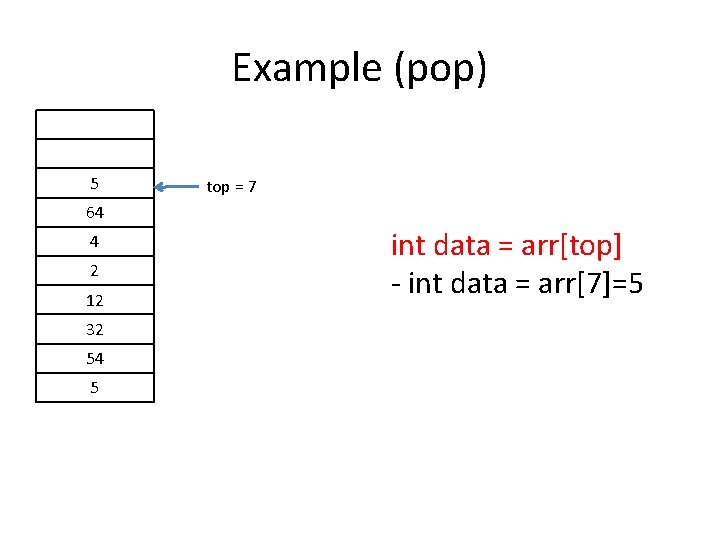
![Example (pop) top = 7 64 4 2 12 32 54 5 arr[top]= NULL Example (pop) top = 7 64 4 2 12 32 54 5 arr[top]= NULL](https://slidetodoc.com/presentation_image_h/ae95104481aac74b58d6ffee20c98e95/image-28.jpg)
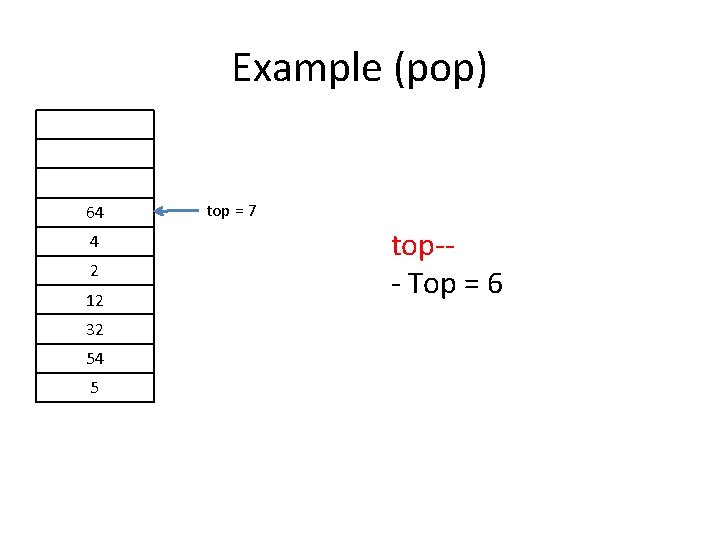
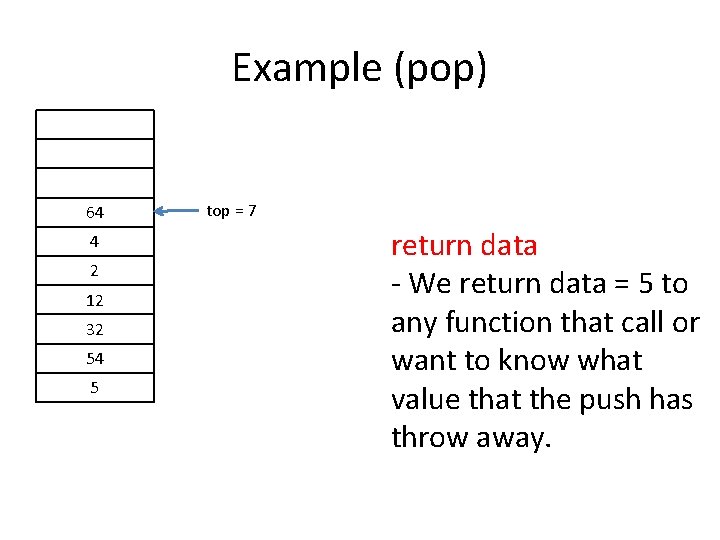
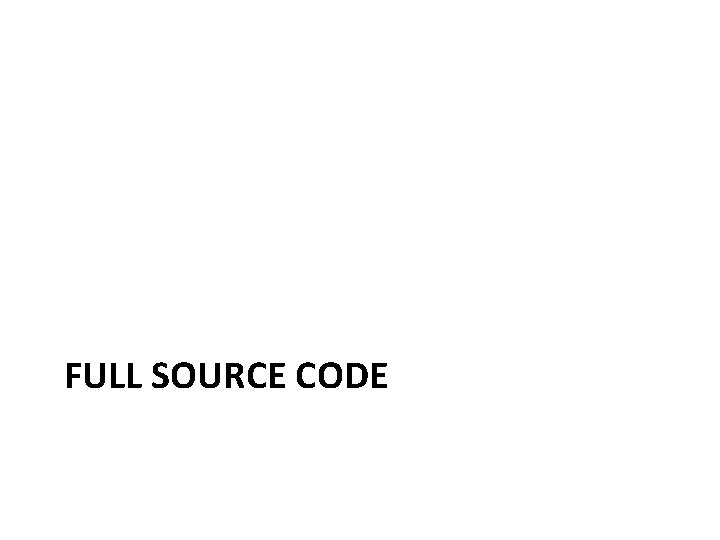
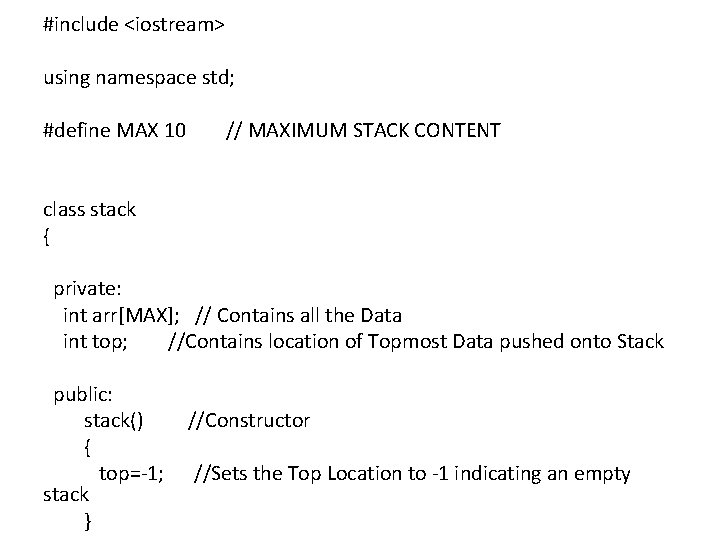
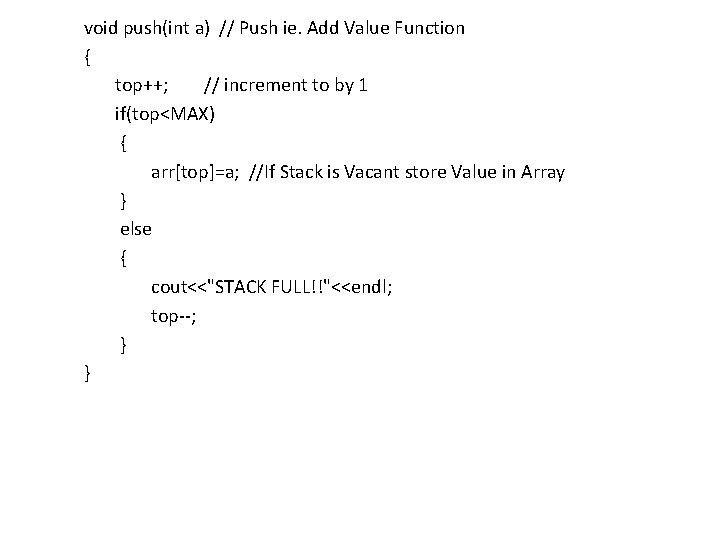
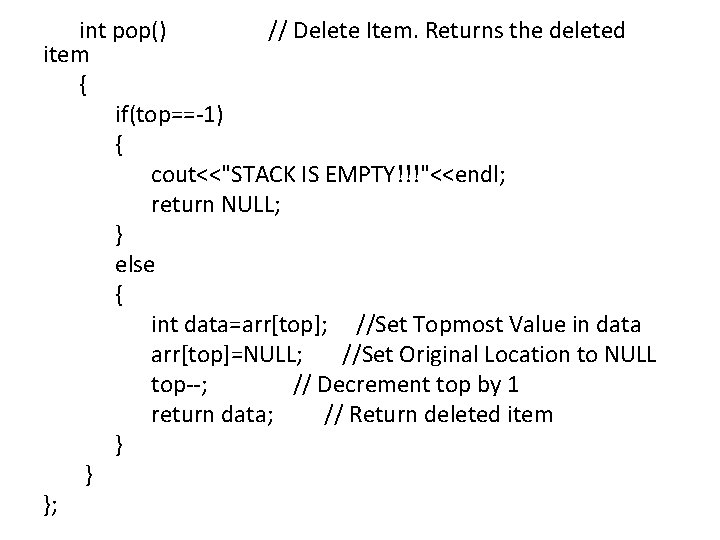
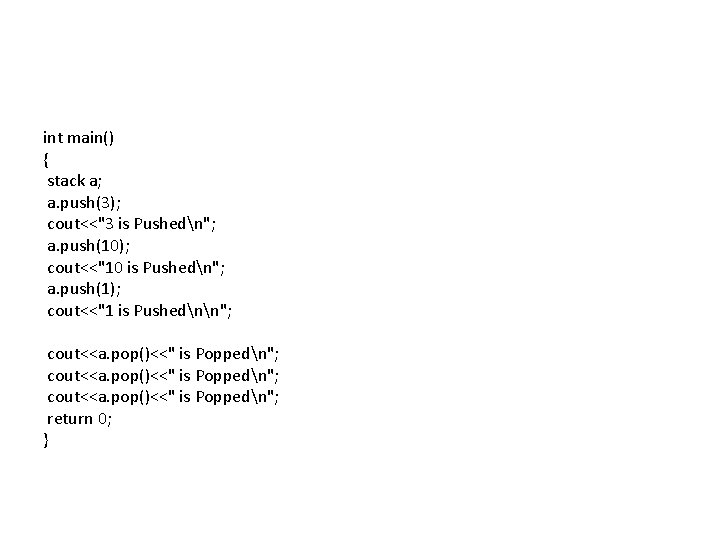
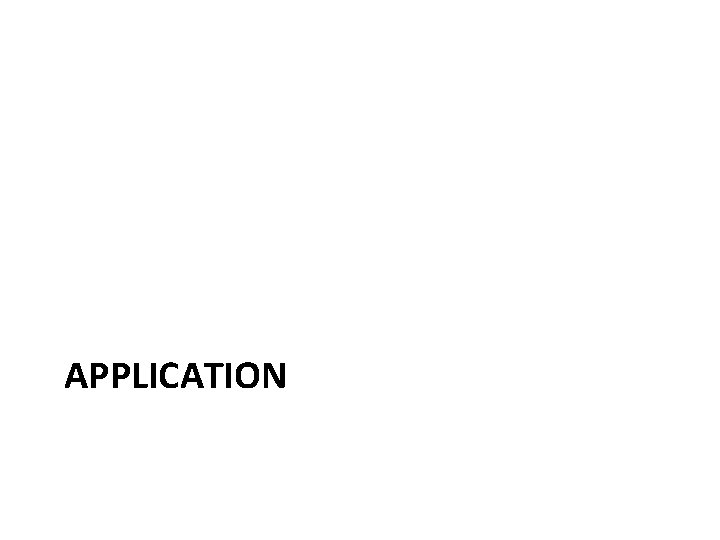
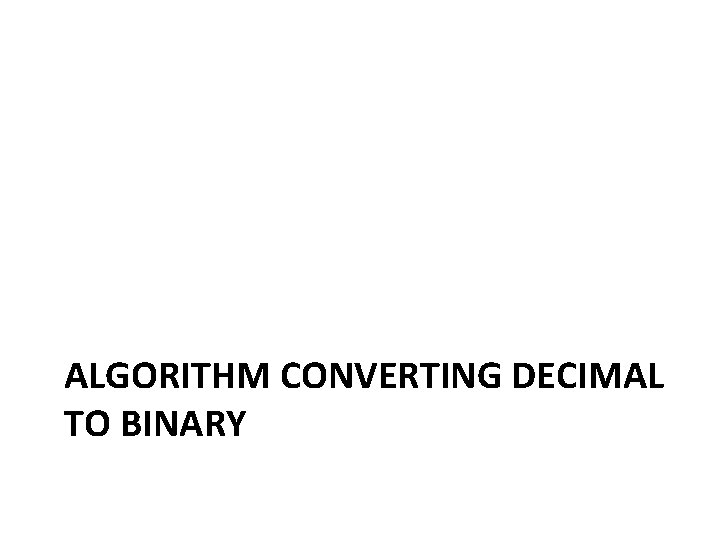
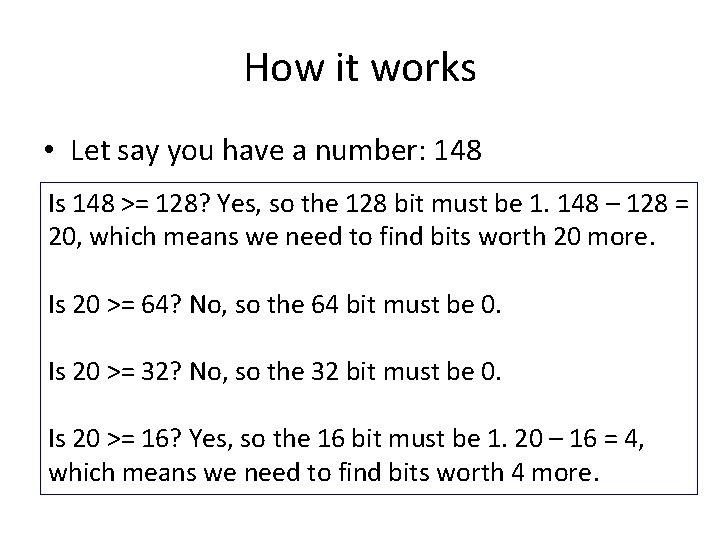
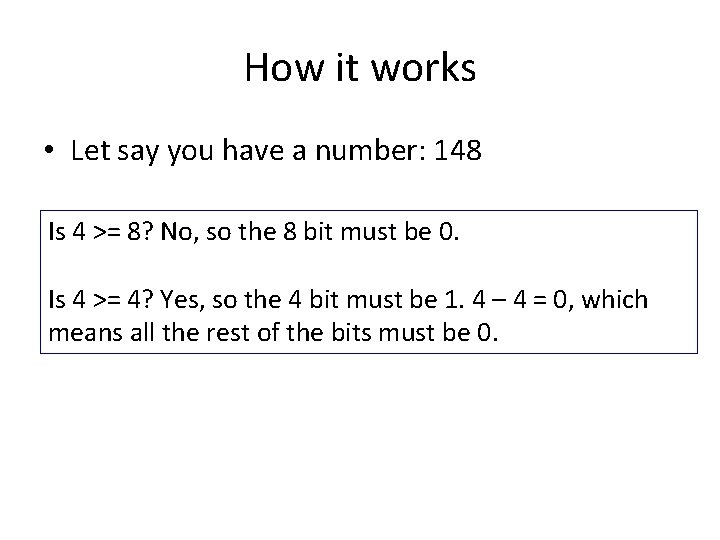
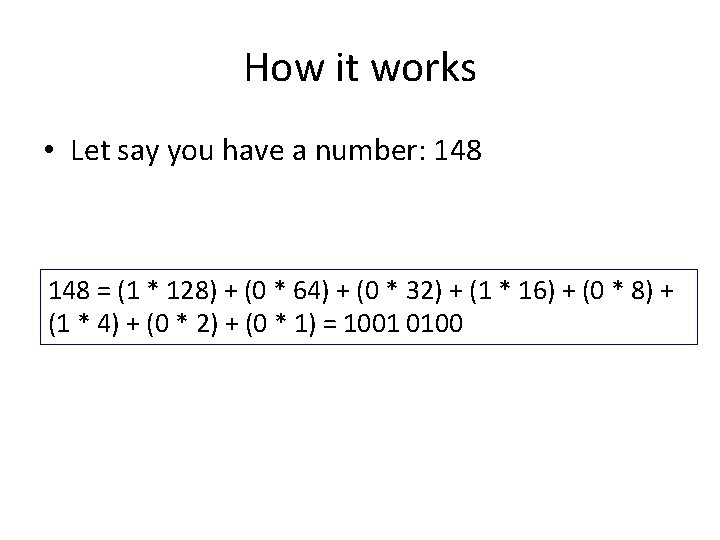
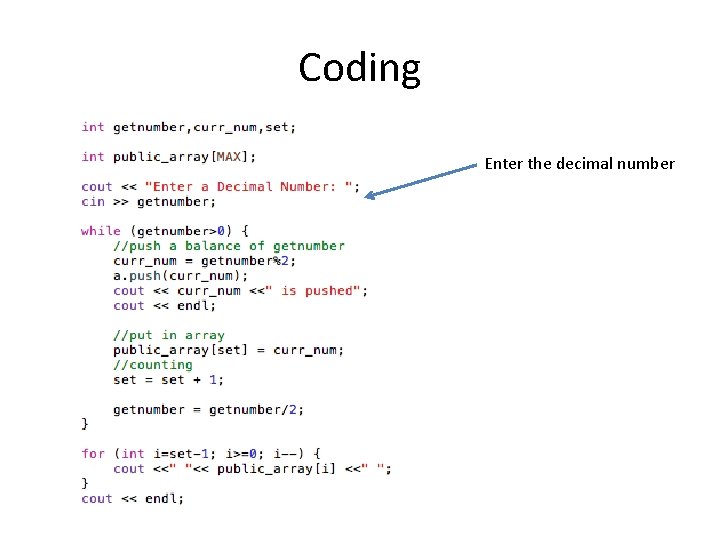
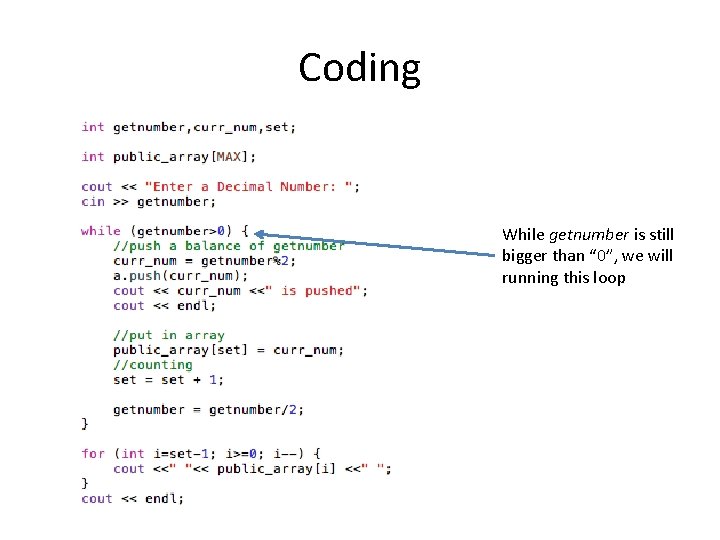
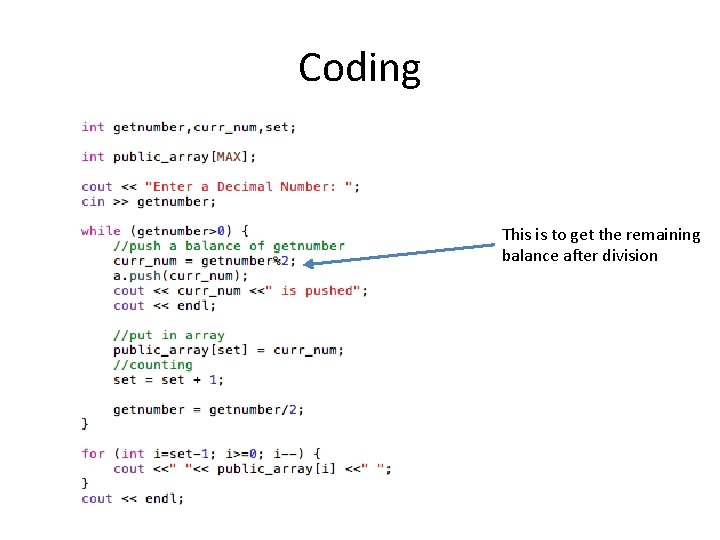
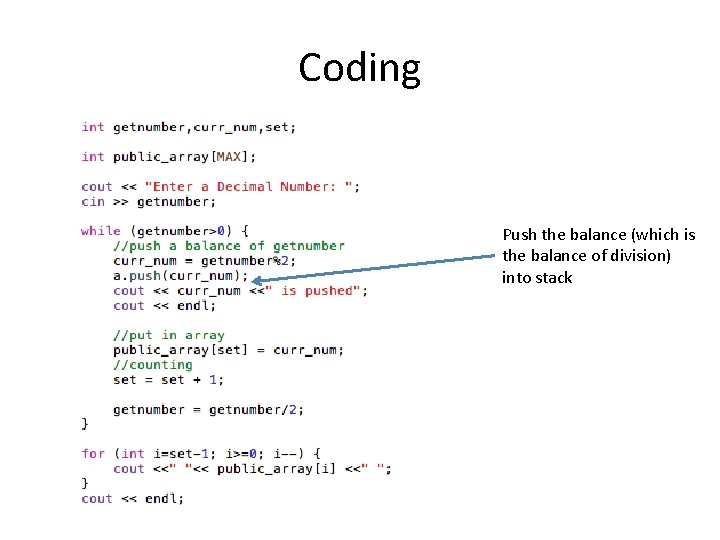
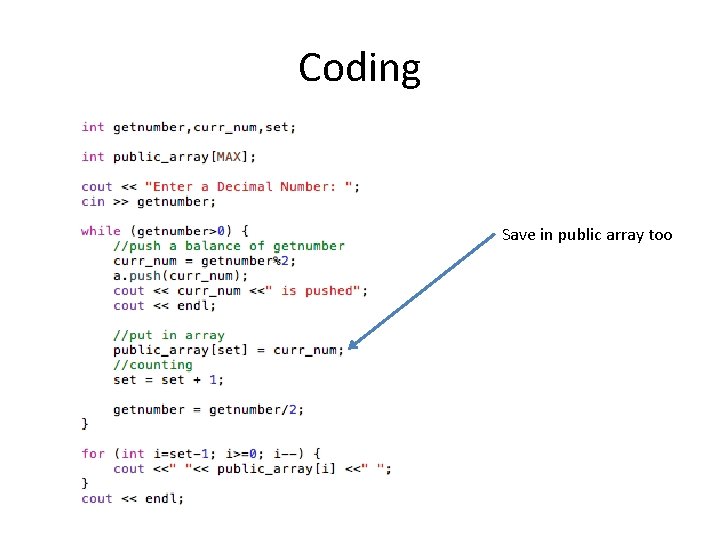
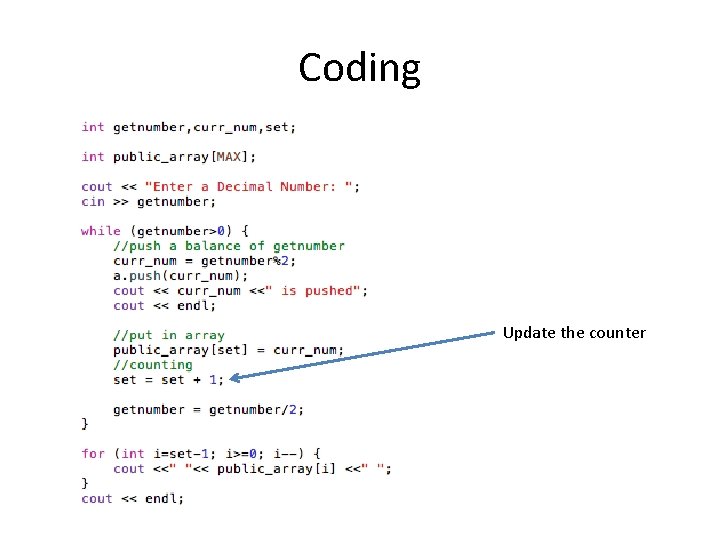
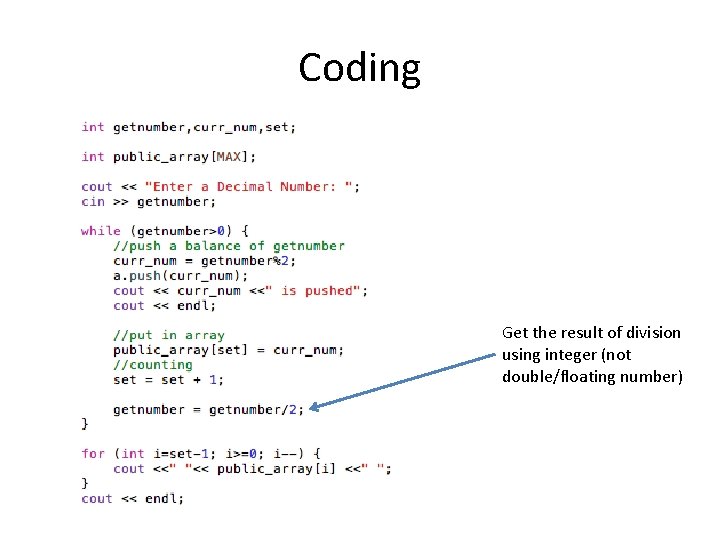
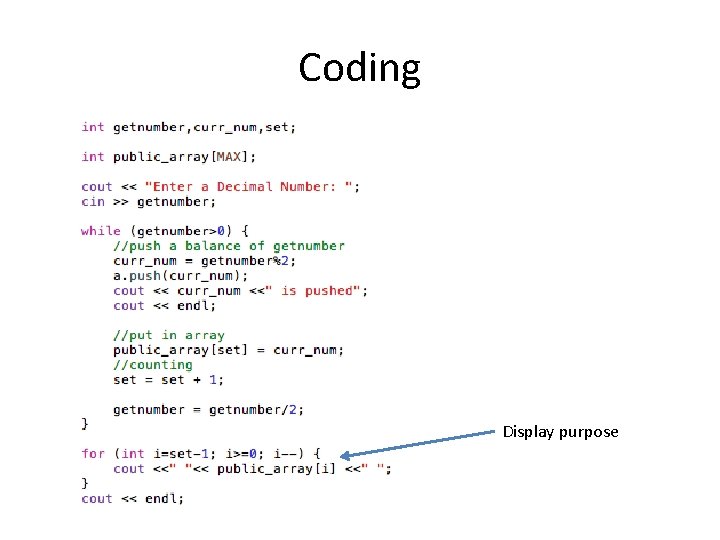
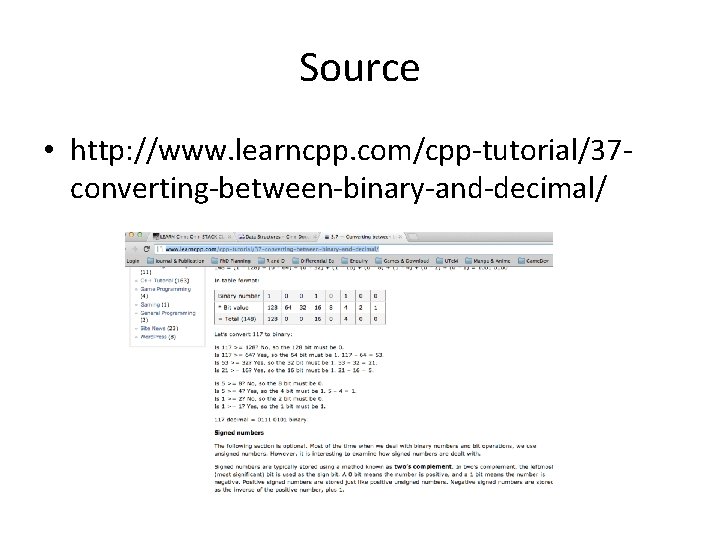
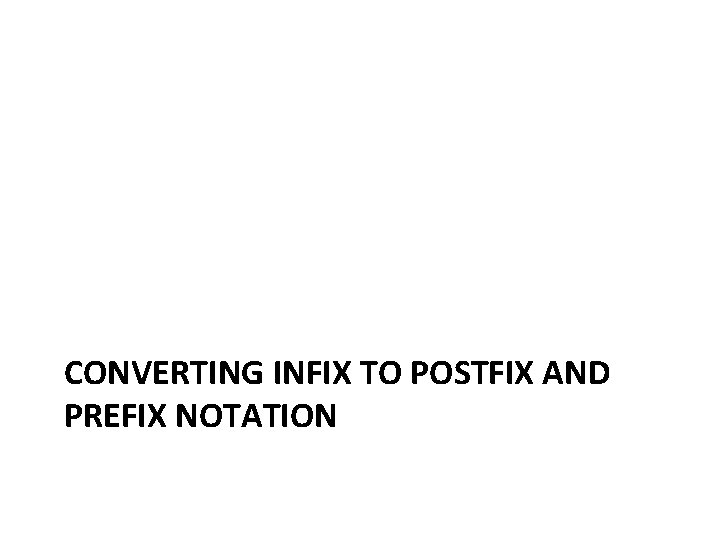
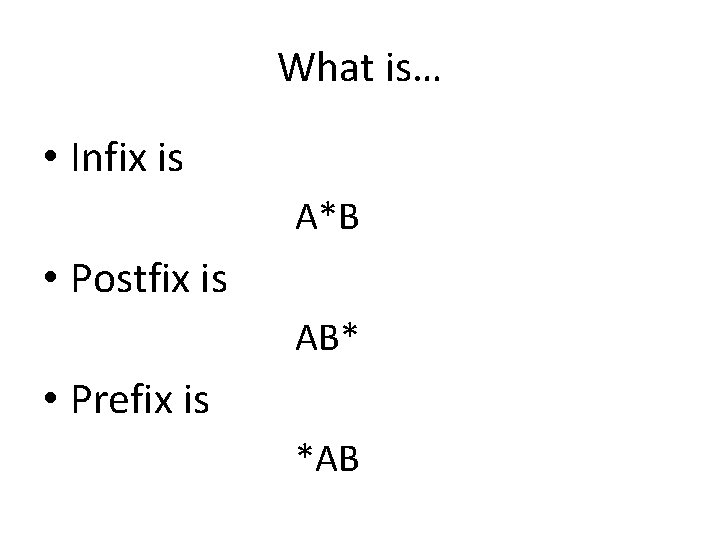
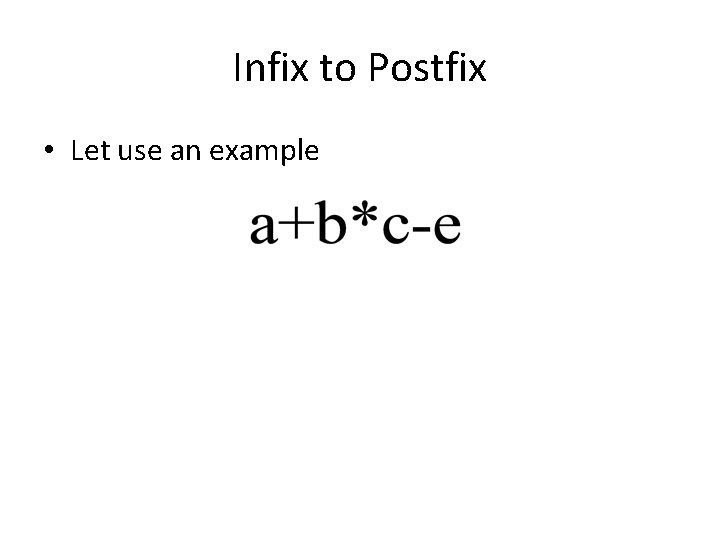
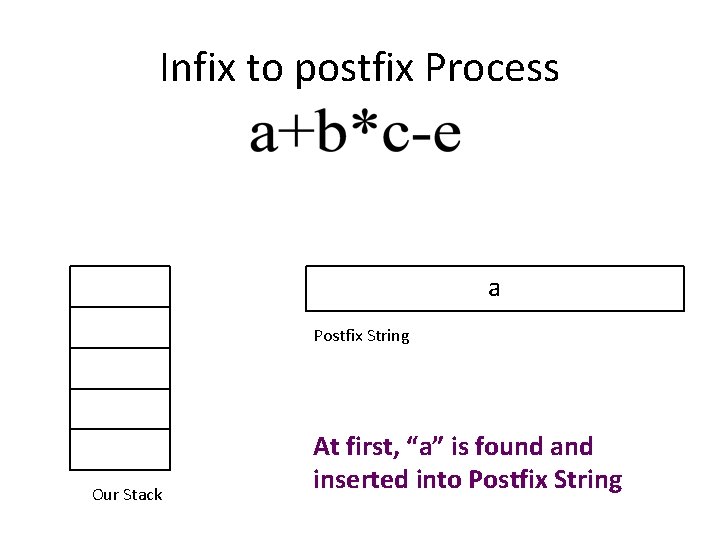
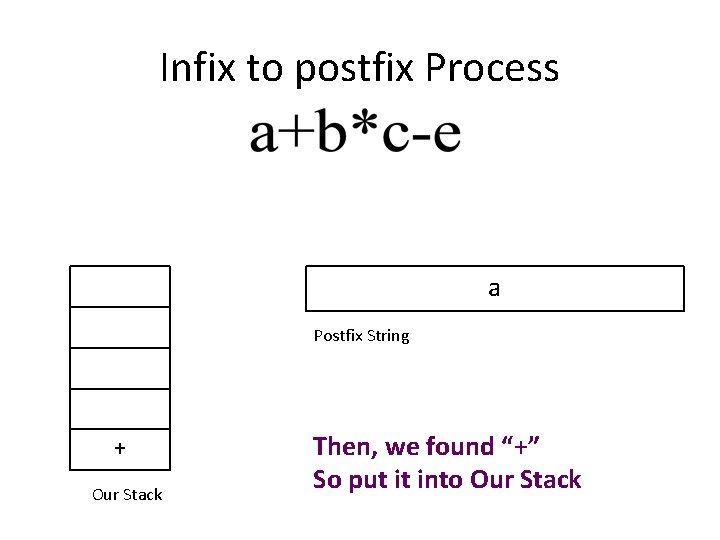
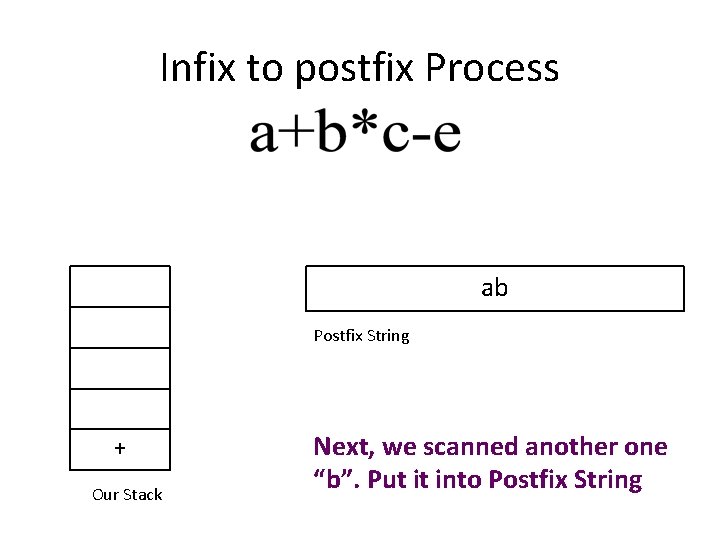
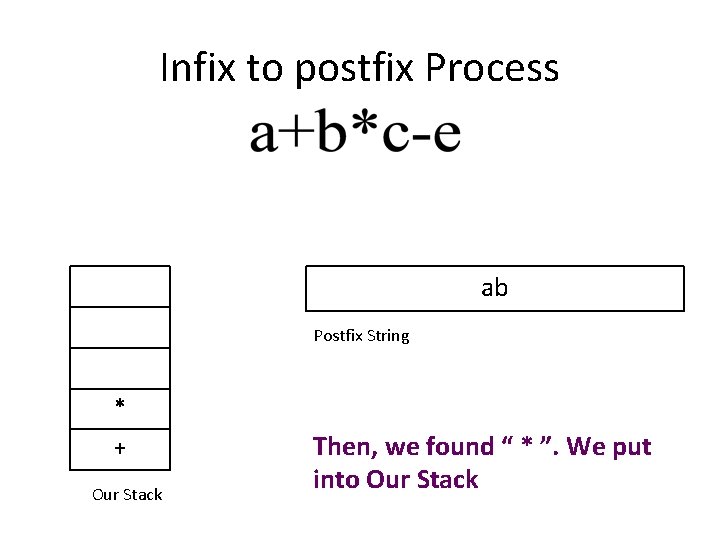
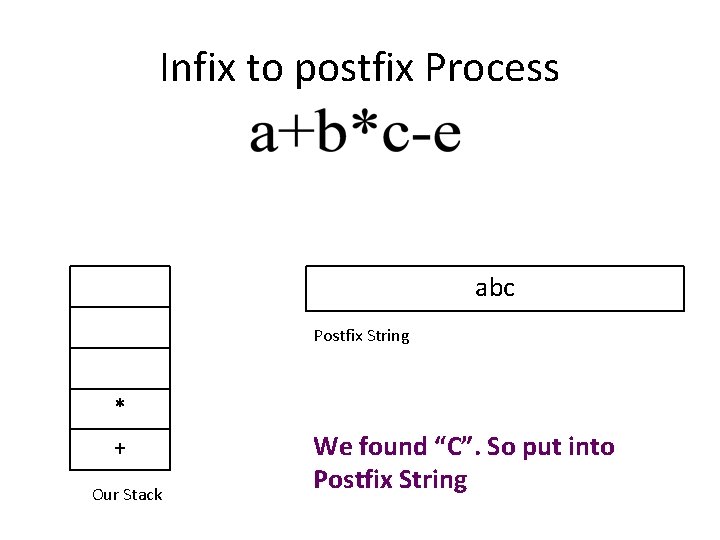
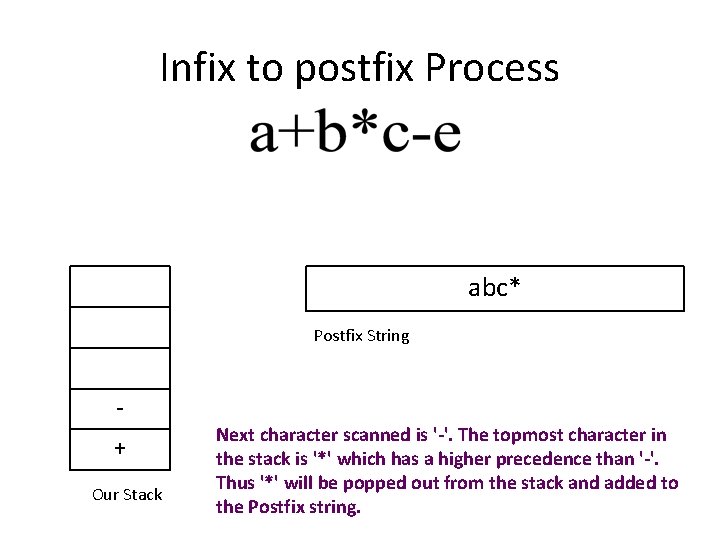
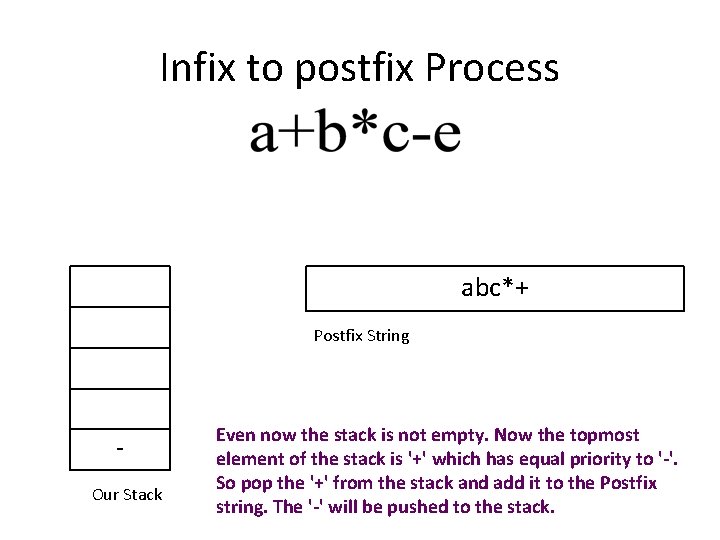
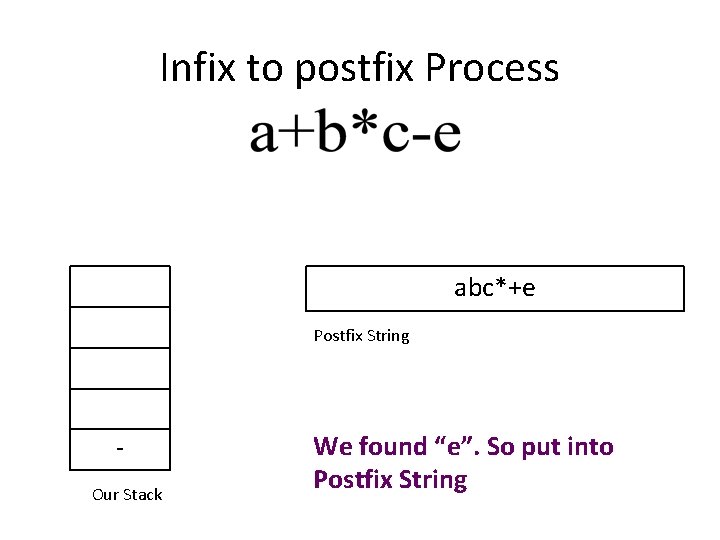
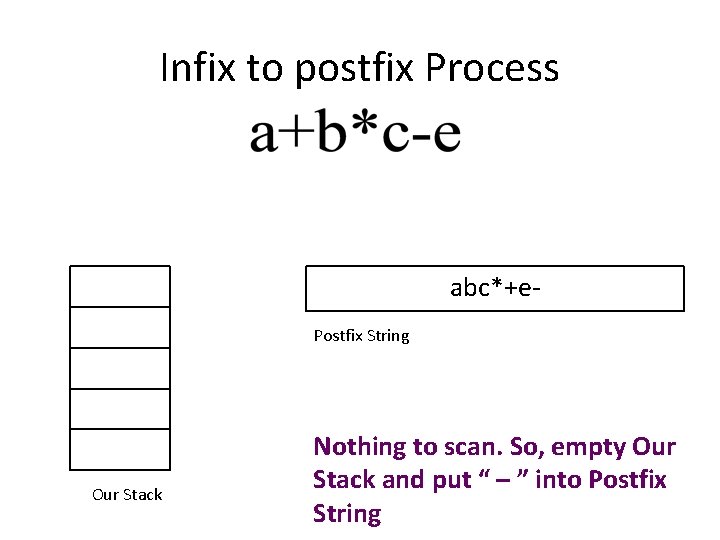
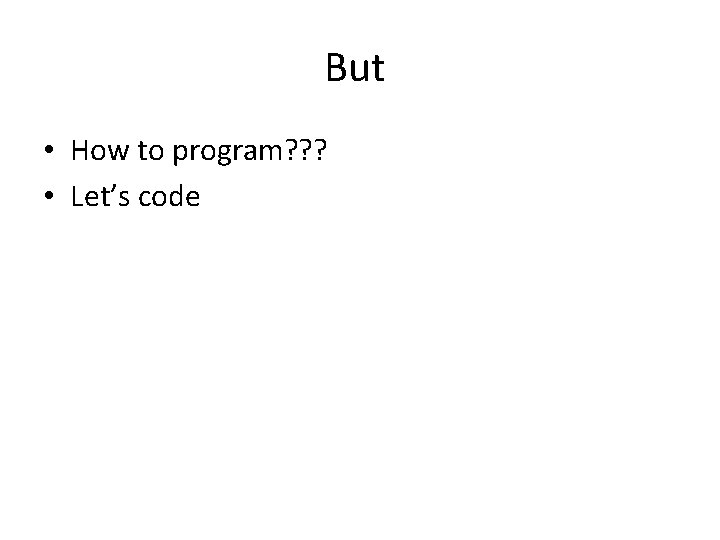
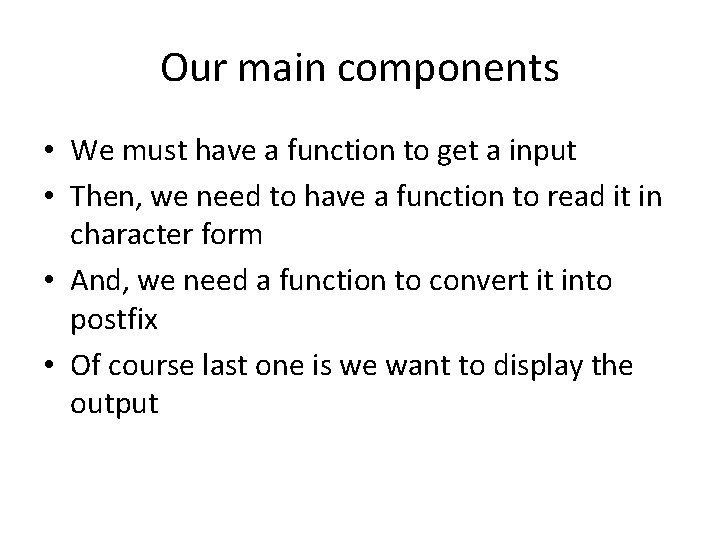
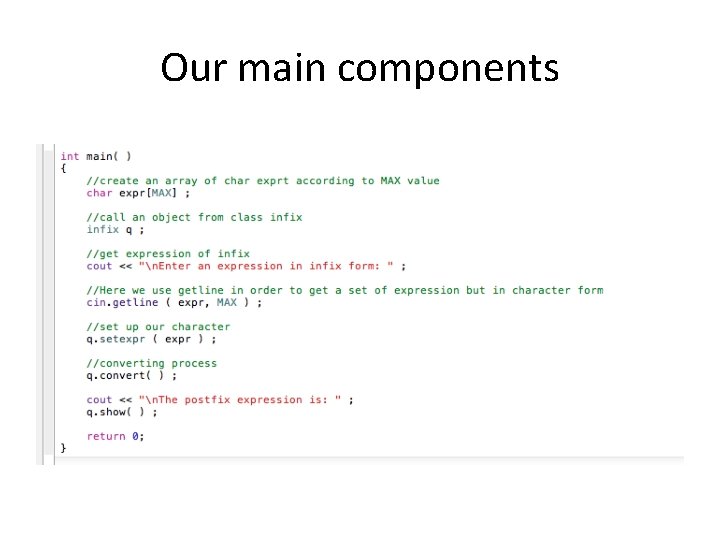
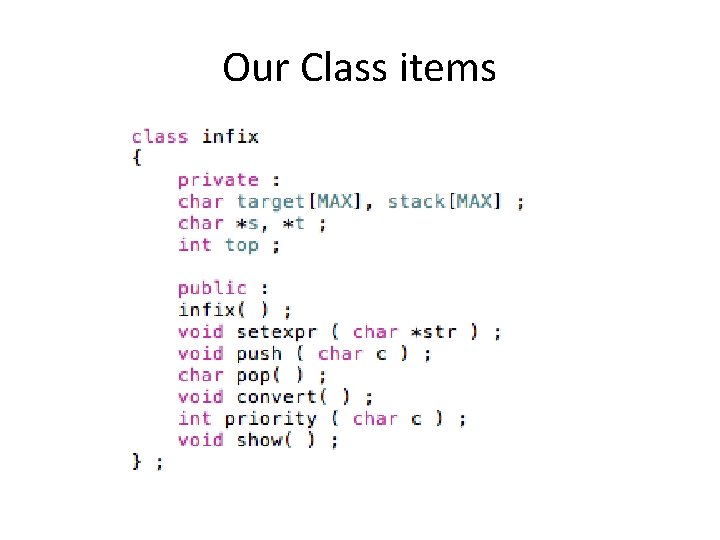
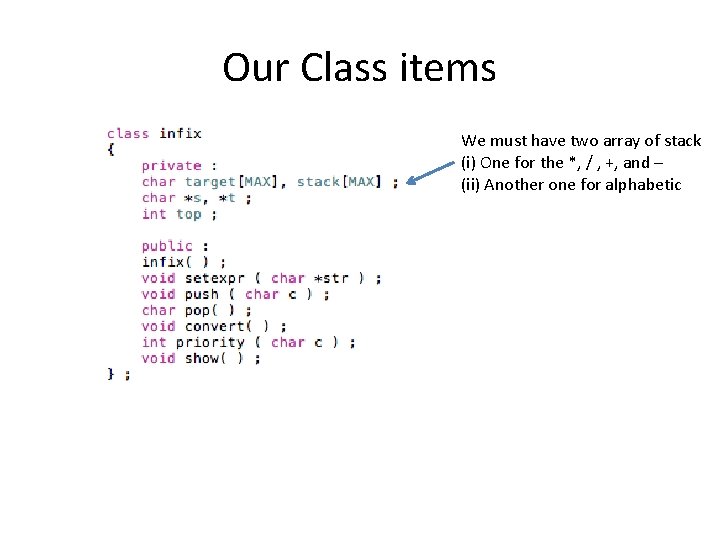
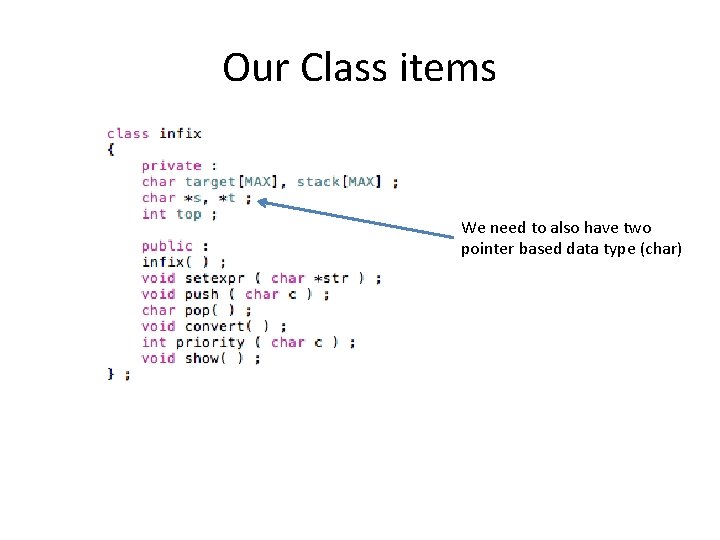
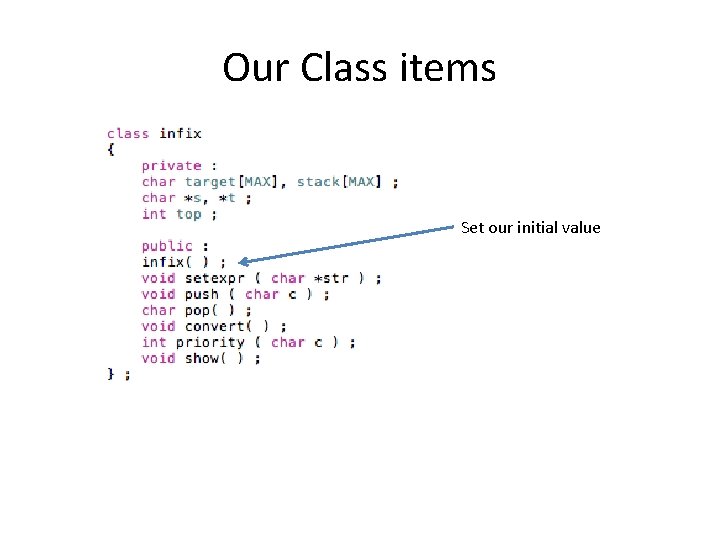
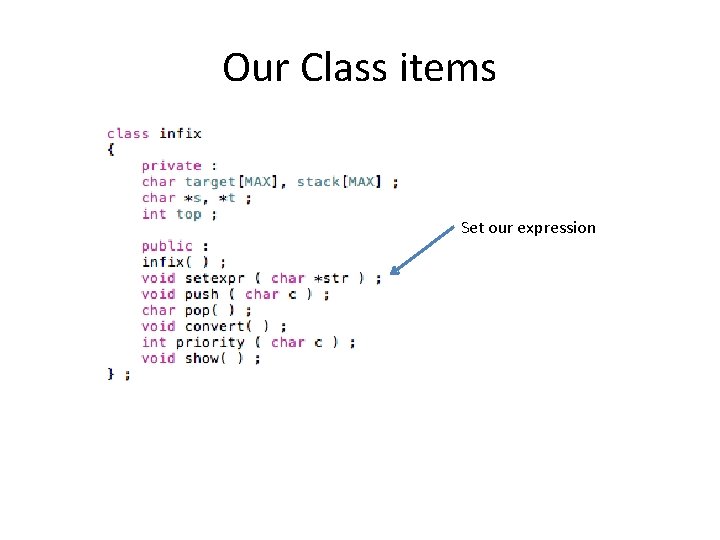
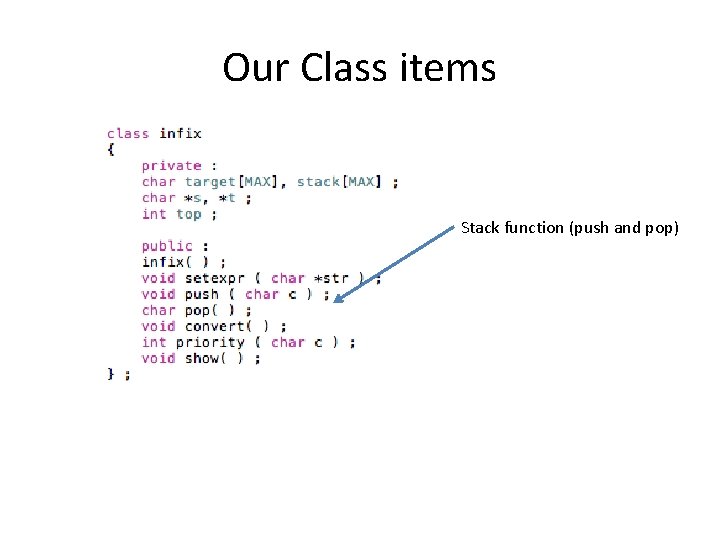
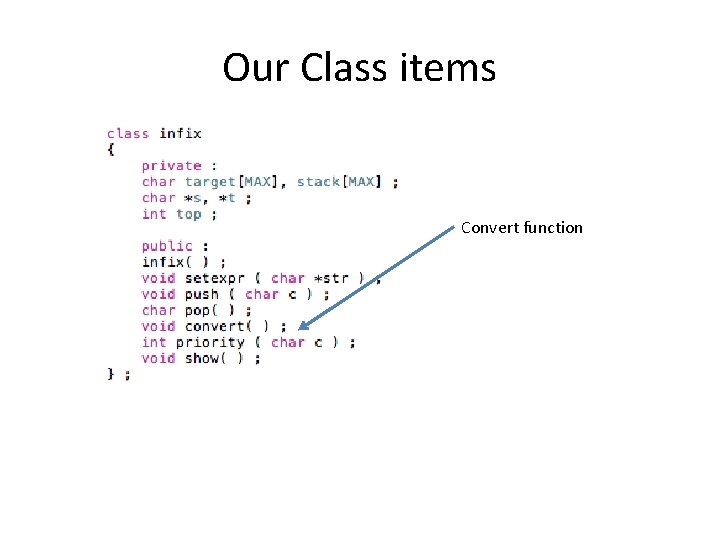
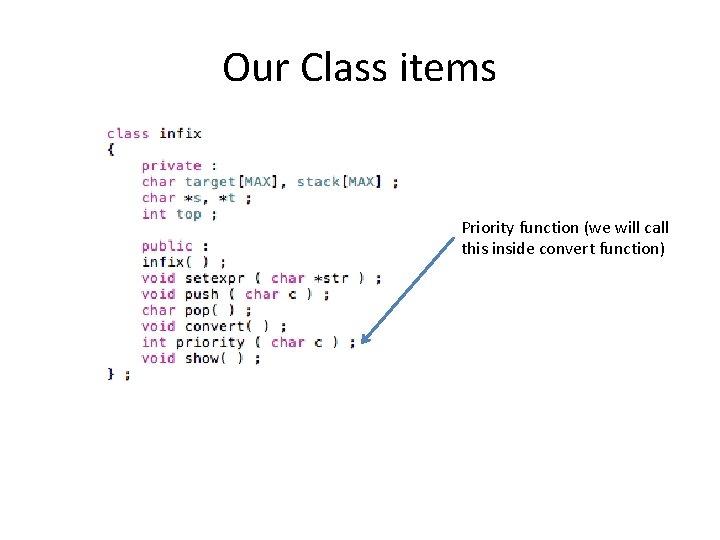
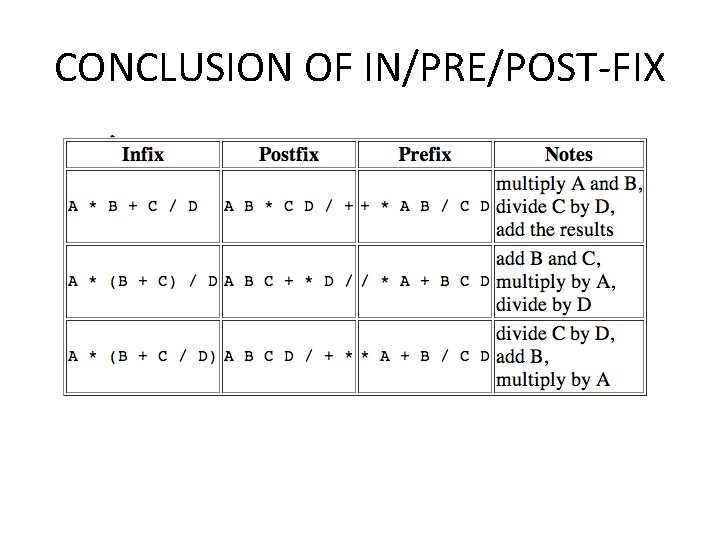
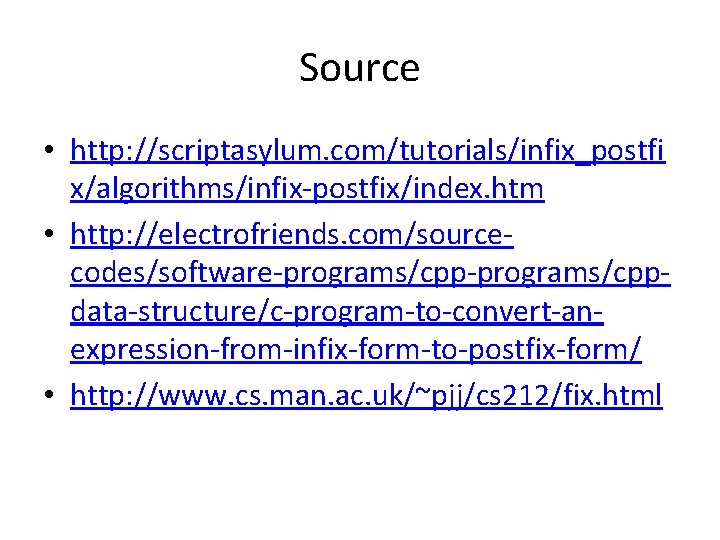
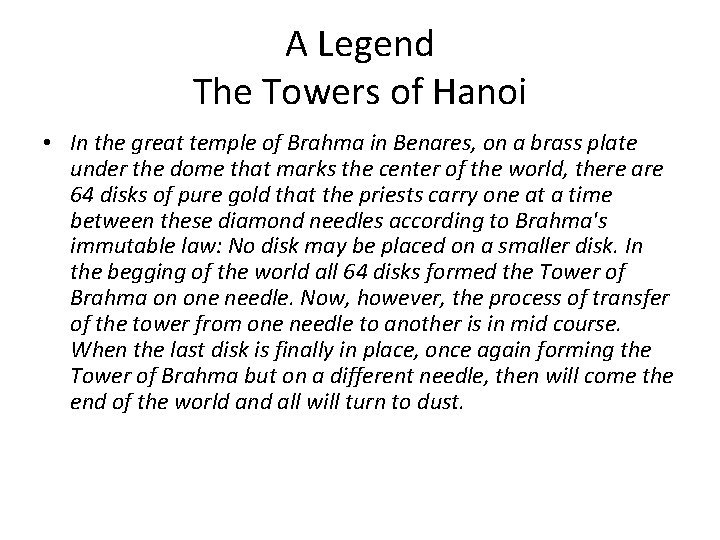
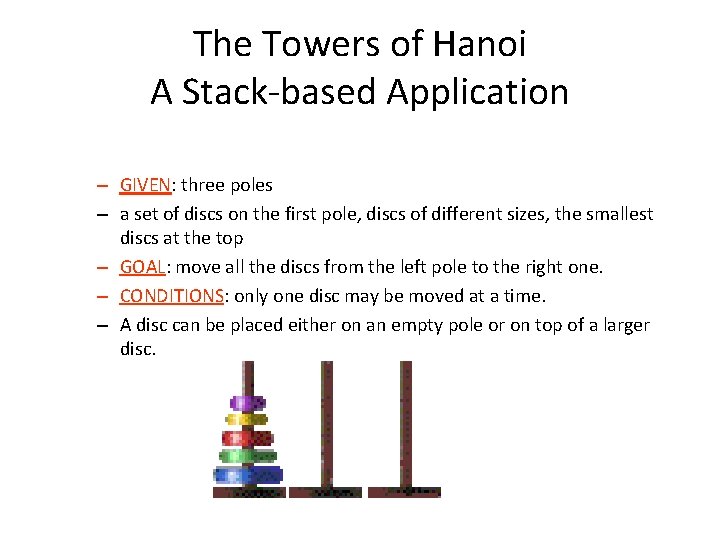
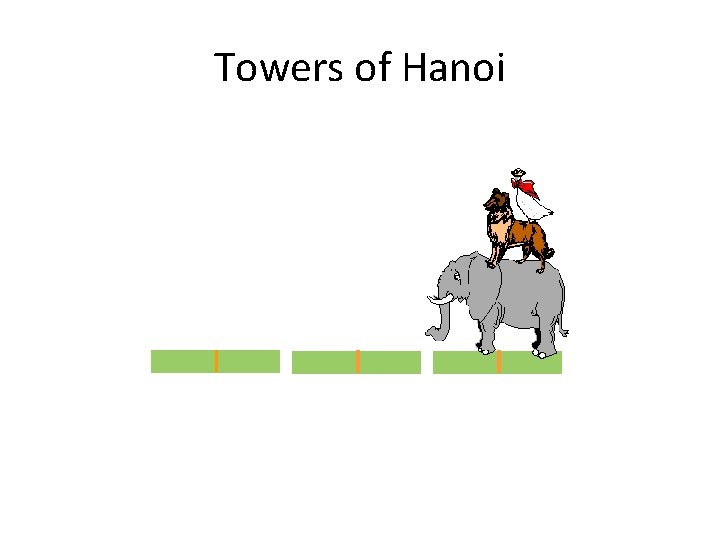
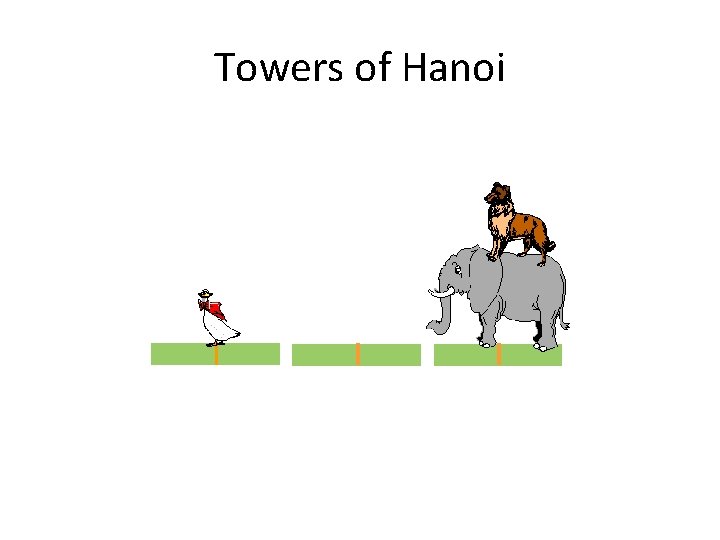
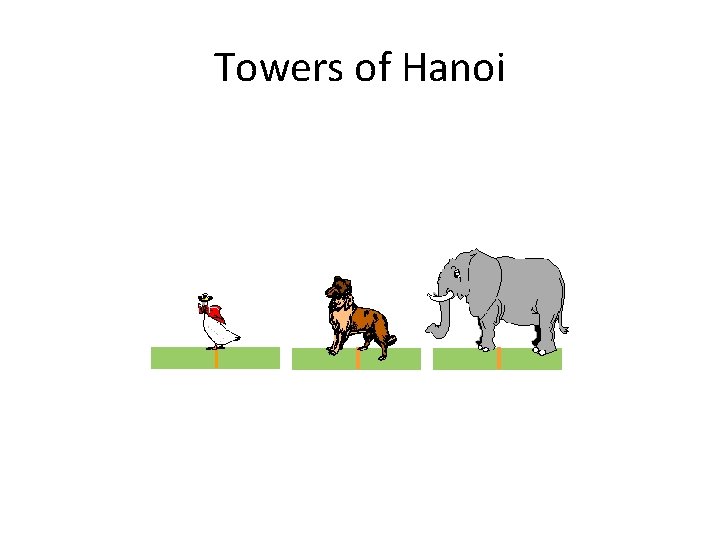
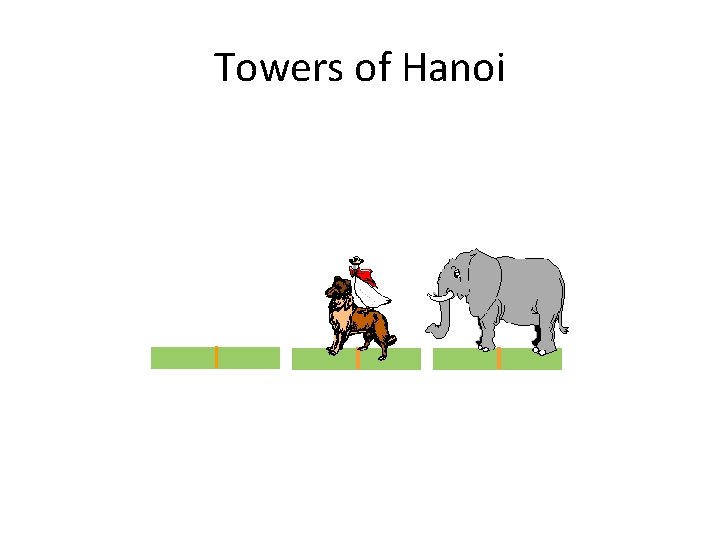
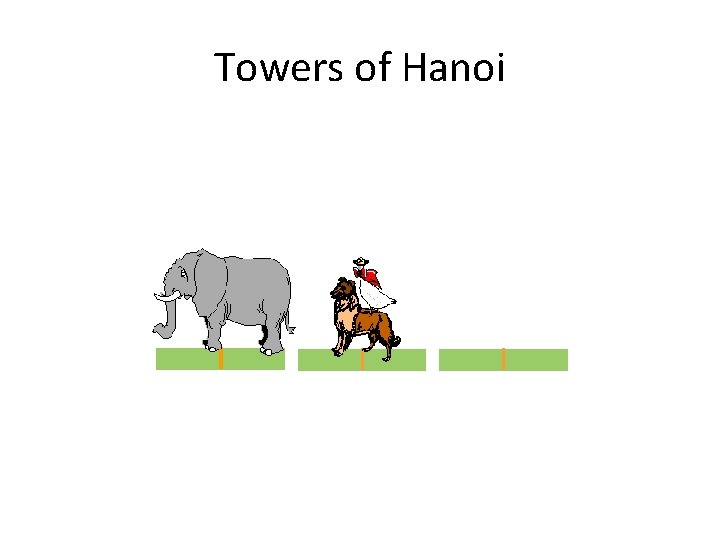
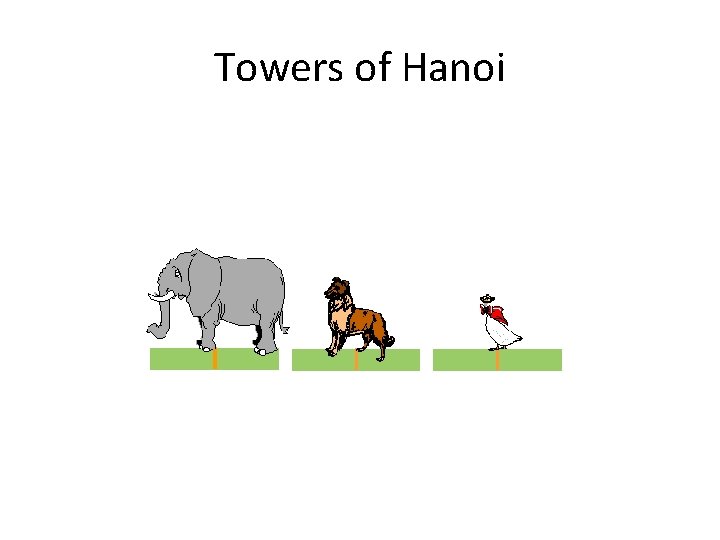
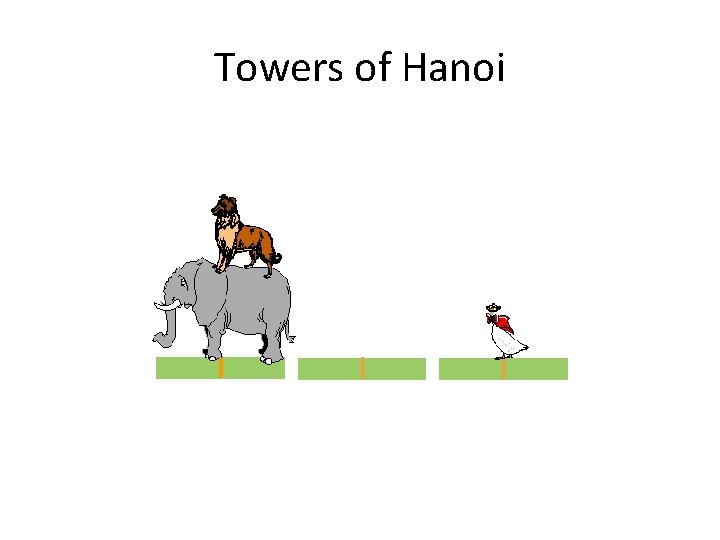
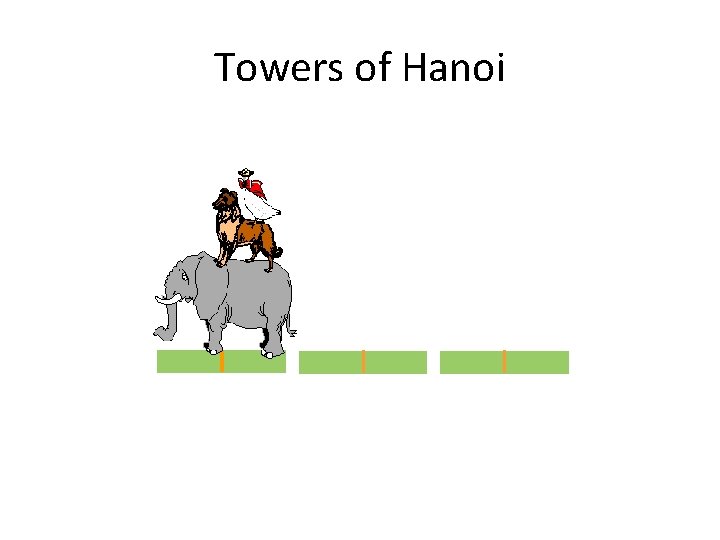
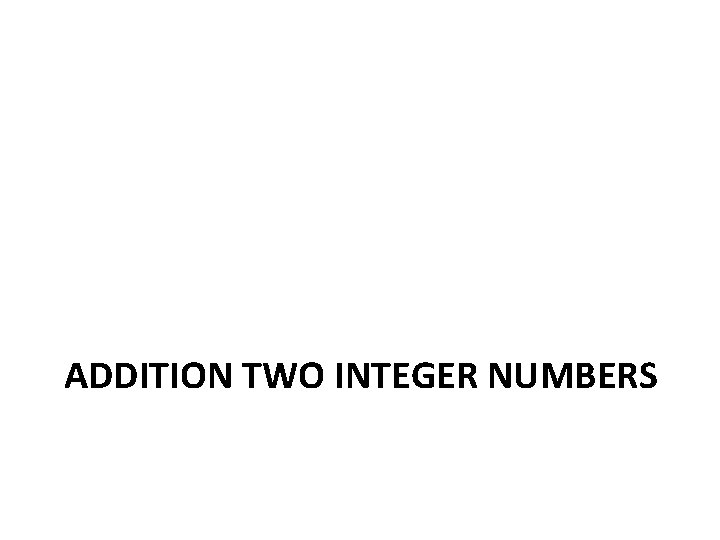
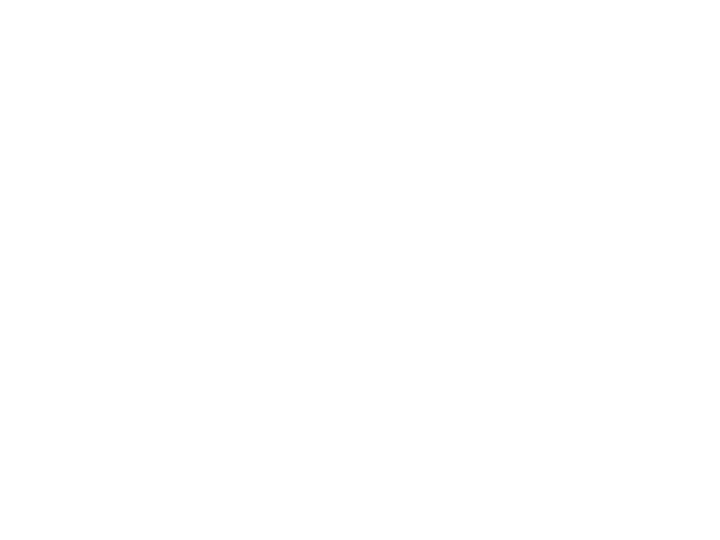
- Slides: 86
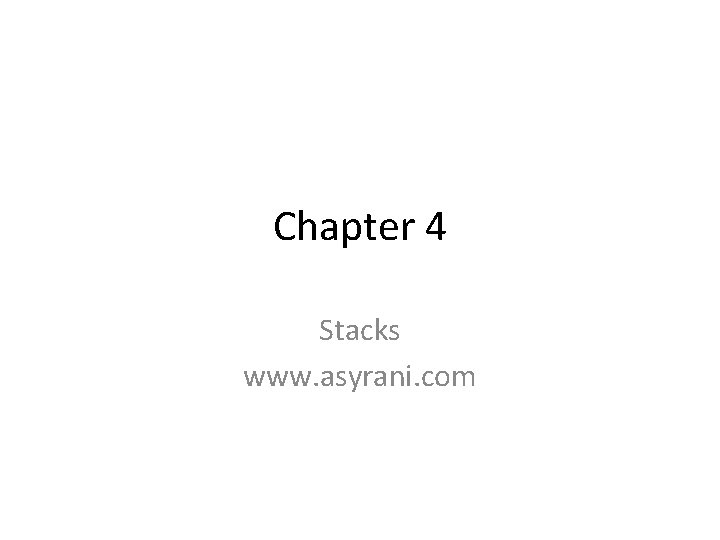
Chapter 4 Stacks www. asyrani. com
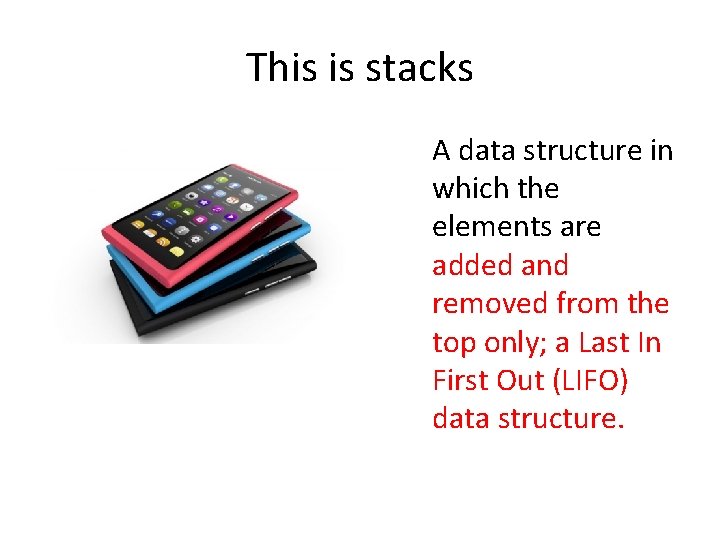
This is stacks A data structure in which the elements are added and removed from the top only; a Last In First Out (LIFO) data structure.
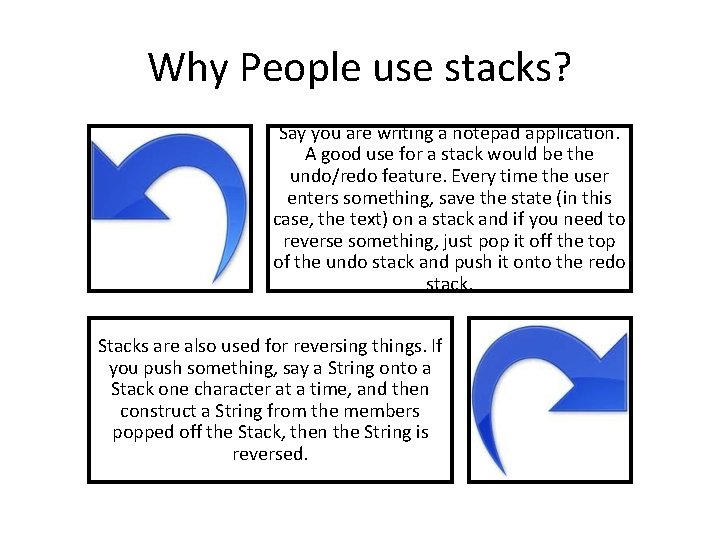
Why People use stacks? Say you are writing a notepad application. A good use for a stack would be the undo/redo feature. Every time the user enters something, save the state (in this case, the text) on a stack and if you need to reverse something, just pop it off the top of the undo stack and push it onto the redo stack. Stacks are also used for reversing things. If you push something, say a String onto a Stack one character at a time, and then construct a String from the members popped off the Stack, then the String is reversed.
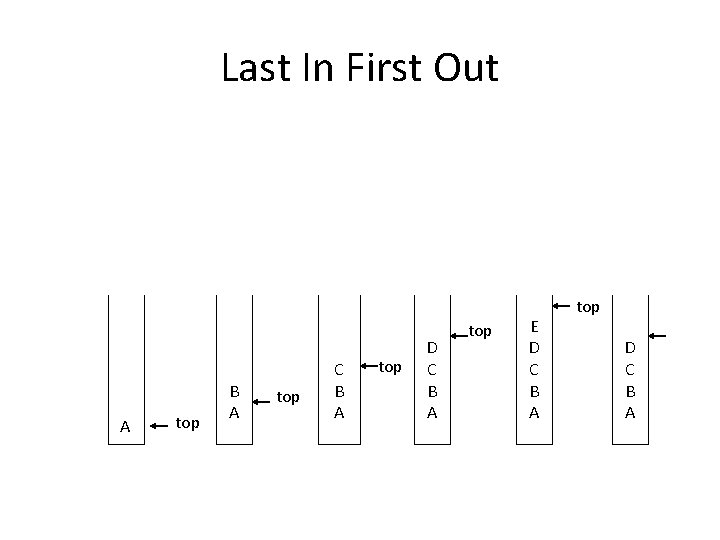
Last In First Out A top B A top C B A top D C B A top E D C B A top D C B A
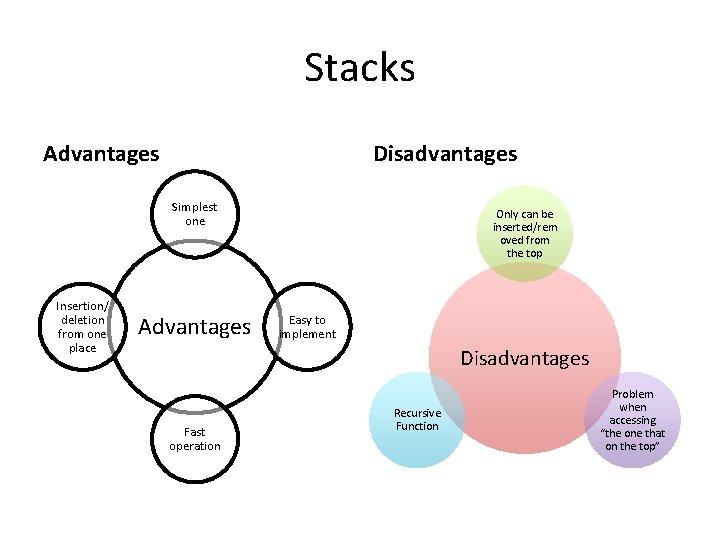
Stacks Advantages Disadvantages Simplest one Insertion/ deletion from one place Advantages Only can be inserted/rem oved from the top Easy to implement Disadvantages Fast operation Recursive Function Problem when accessing “the one that on the top”
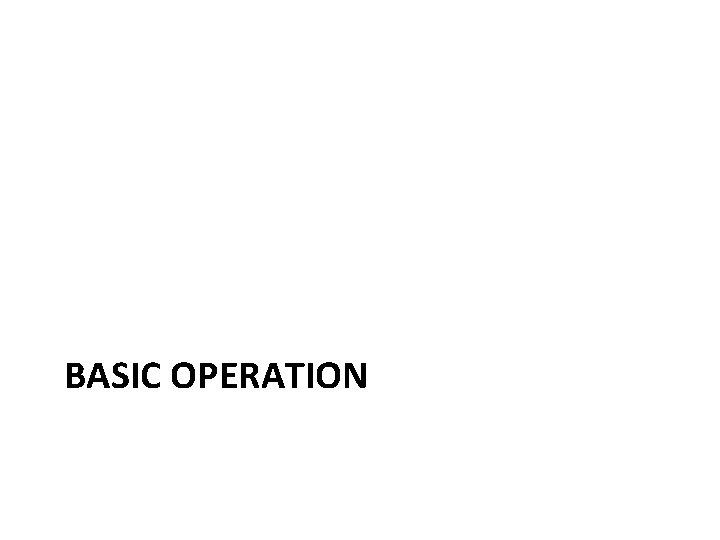
BASIC OPERATION
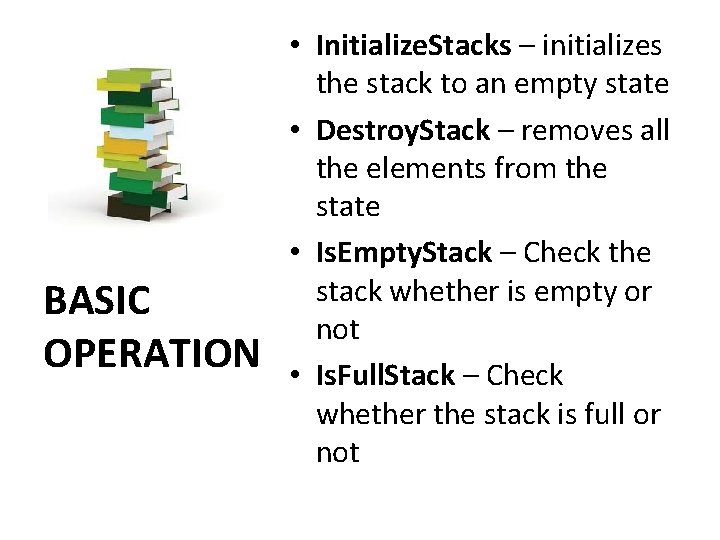
BASIC OPERATION • Initialize. Stacks – initializes the stack to an empty state • Destroy. Stack – removes all the elements from the state • Is. Empty. Stack – Check the stack whether is empty or not • Is. Full. Stack – Check whether the stack is full or not
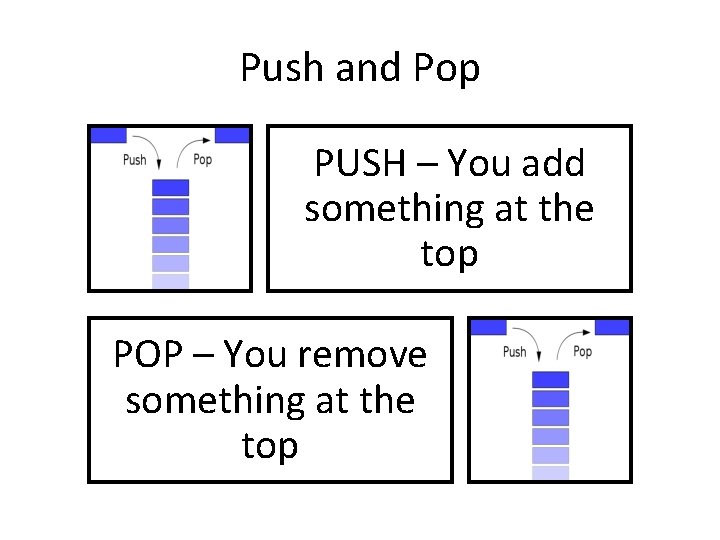
Push and Pop PUSH – You add something at the top POP – You remove something at the top
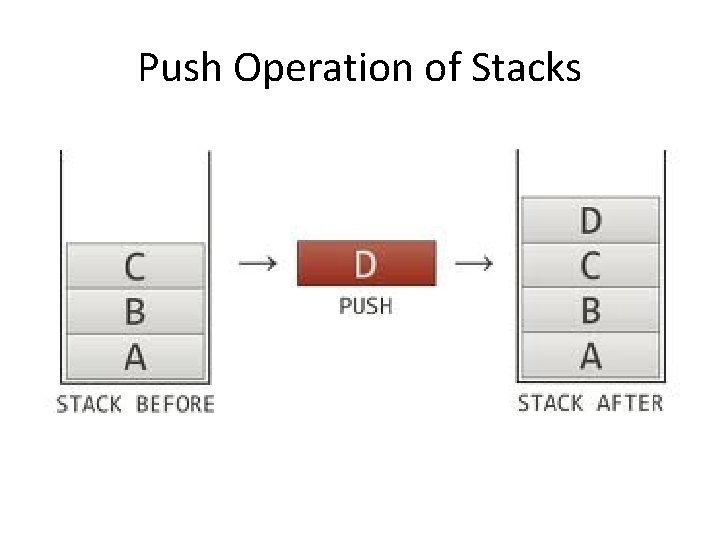
Push Operation of Stacks
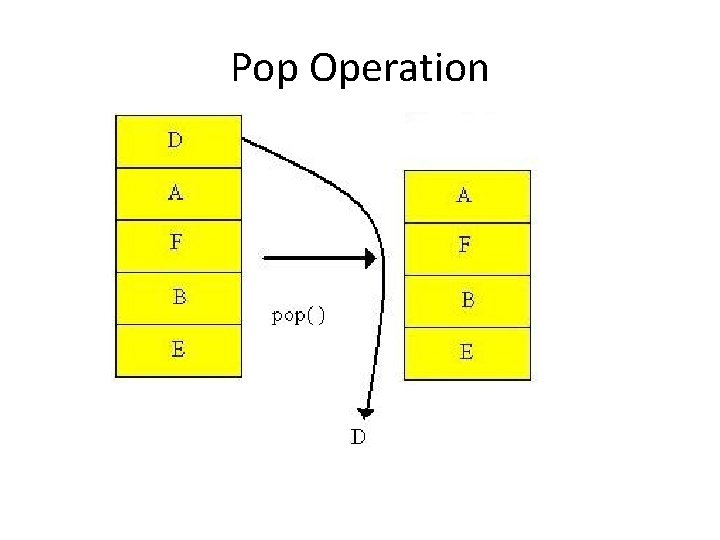
Pop Operation
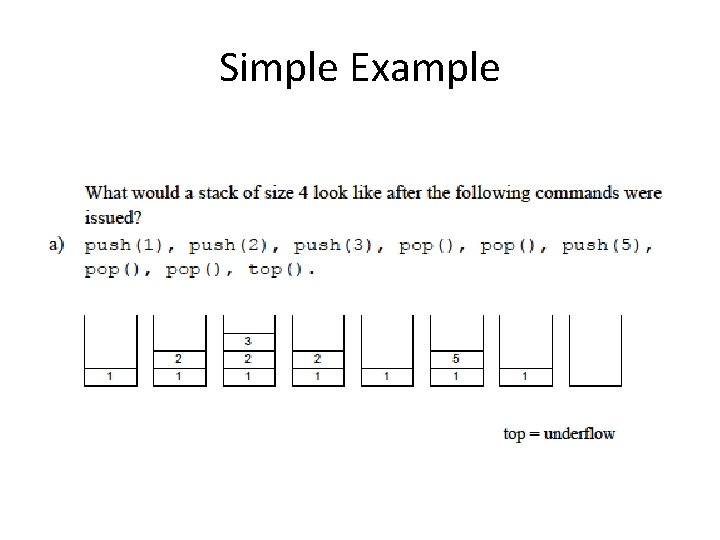
Simple Example
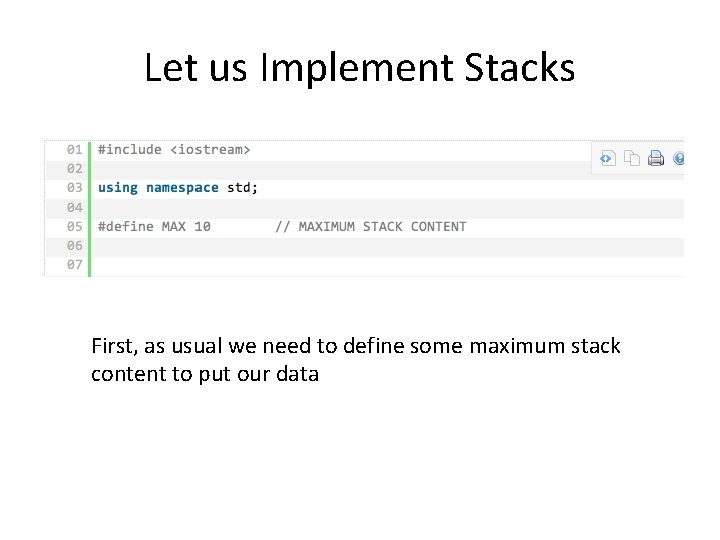
Let us Implement Stacks First, as usual we need to define some maximum stack content to put our data
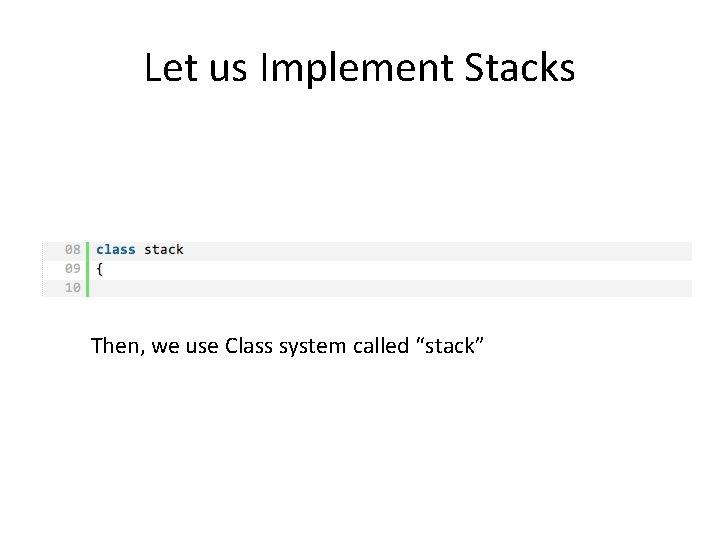
Let us Implement Stacks Then, we use Class system called “stack”
![Let us Implement Stacks We declare a private inside stack class arrMAX Let us Implement Stacks We declare a private inside “stack” class - arr[MAX] :](https://slidetodoc.com/presentation_image_h/ae95104481aac74b58d6ffee20c98e95/image-14.jpg)
Let us Implement Stacks We declare a private inside “stack” class - arr[MAX] : this is where we put all the data - top : we assign our “leader” here.
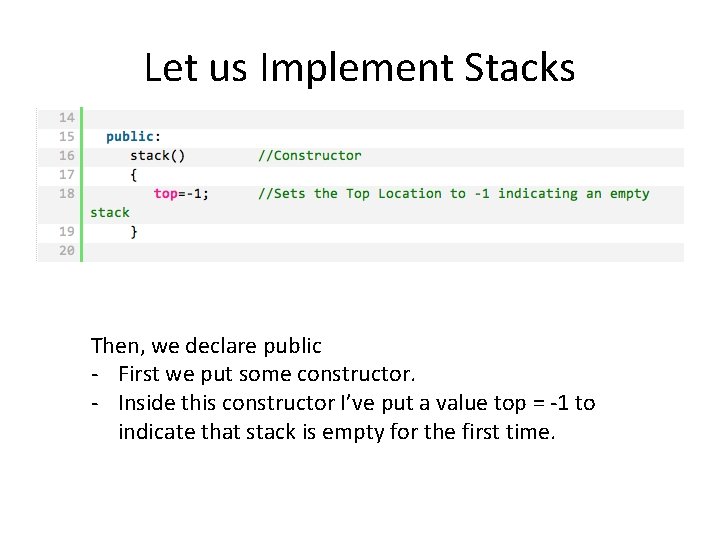
Let us Implement Stacks Then, we declare public - First we put some constructor. - Inside this constructor I’ve put a value top = -1 to indicate that stack is empty for the first time.
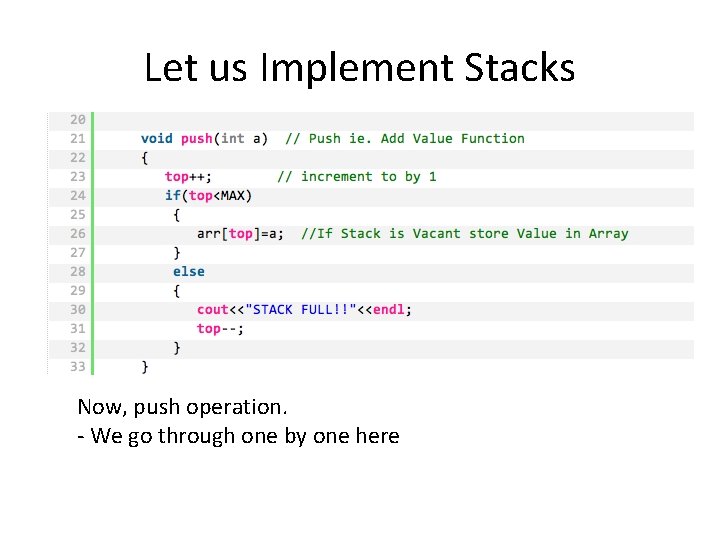
Let us Implement Stacks Now, push operation. - We go through one by one here
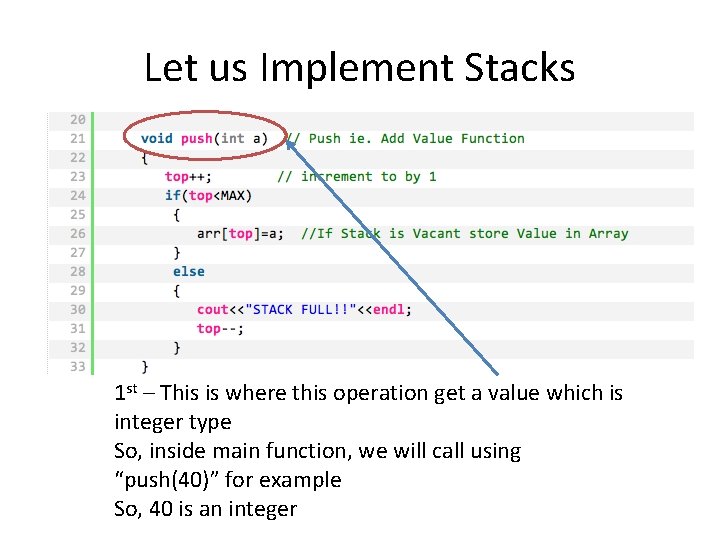
Let us Implement Stacks 1 st – This is where this operation get a value which is integer type So, inside main function, we will call using “push(40)” for example So, 40 is an integer
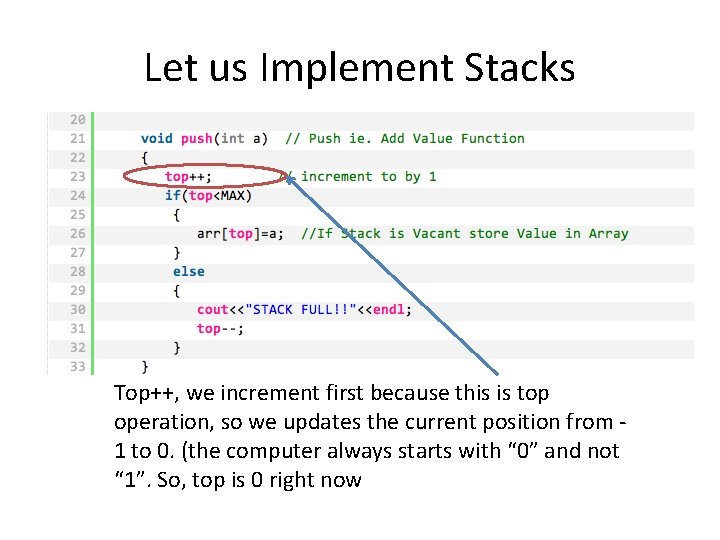
Let us Implement Stacks Top++, we increment first because this is top operation, so we updates the current position from 1 to 0. (the computer always starts with “ 0” and not “ 1”. So, top is 0 right now
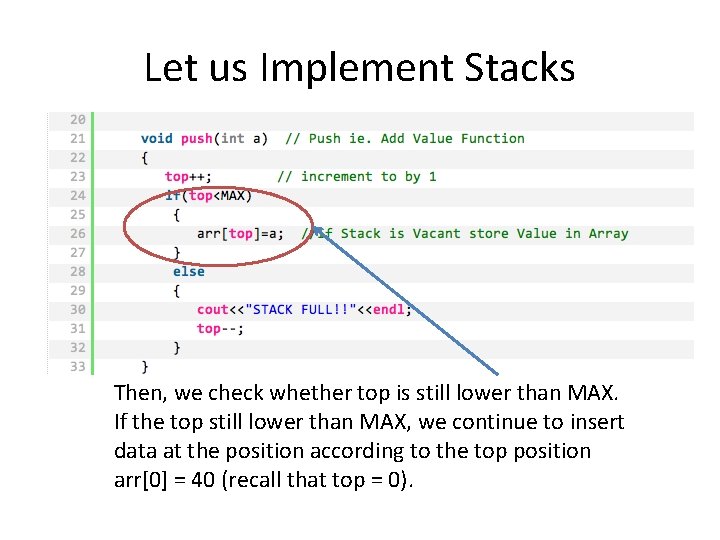
Let us Implement Stacks Then, we check whether top is still lower than MAX. If the top still lower than MAX, we continue to insert data at the position according to the top position arr[0] = 40 (recall that top = 0).
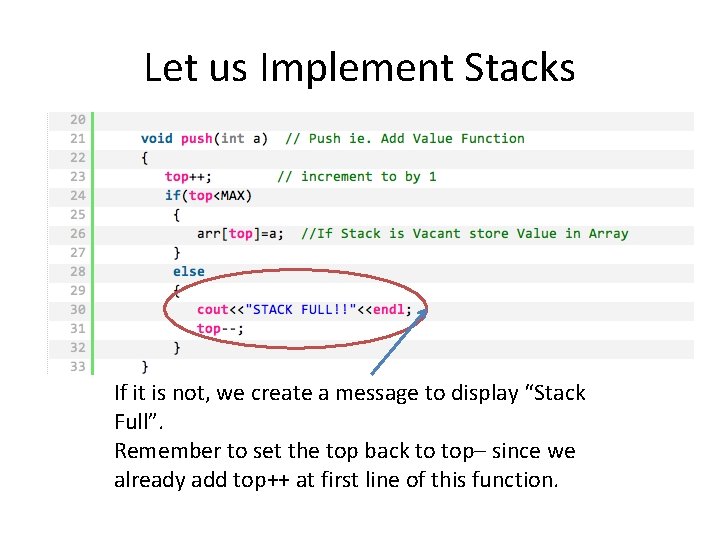
Let us Implement Stacks If it is not, we create a message to display “Stack Full”. Remember to set the top back to top– since we already add top++ at first line of this function.
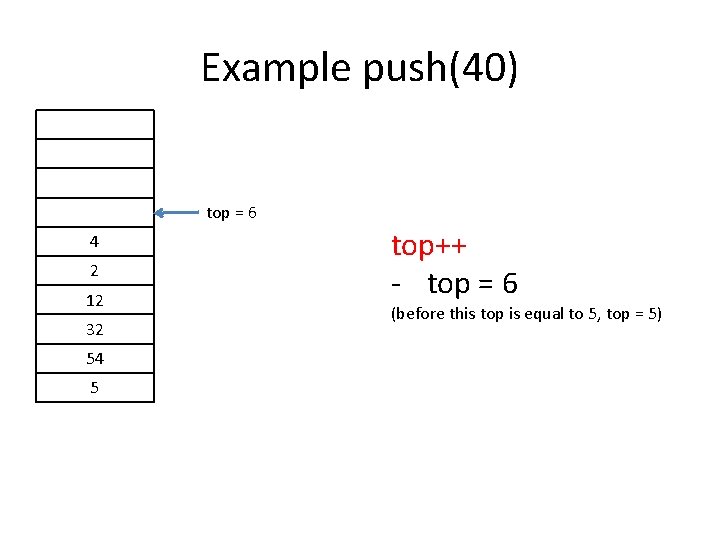
Example push(40) top = 6 4 2 12 32 54 5 top++ - top = 6 (before this top is equal to 5, top = 5)
![Example push40 top 6 4 2 12 32 54 5 iftopMAX arrtopa Example push(40) top = 6 4 2 12 32 54 5 if(top<MAX) { arr[top]=a;](https://slidetodoc.com/presentation_image_h/ae95104481aac74b58d6ffee20c98e95/image-22.jpg)
Example push(40) top = 6 4 2 12 32 54 5 if(top<MAX) { arr[top]=a; } - Yes, top is still lower than MAX which is 10. - So, arr[6] = 40 (since we receive a = 40)
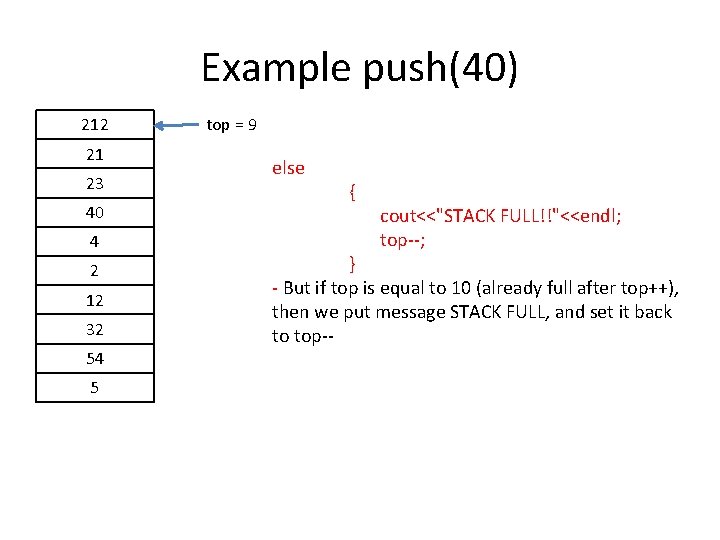
Example push(40) 212 21 23 40 4 2 12 32 54 5 top = 9 else { cout<<"STACK FULL!!"<<endl; top--; } - But if top is equal to 10 (already full after top++), then we put message STACK FULL, and set it back to top--
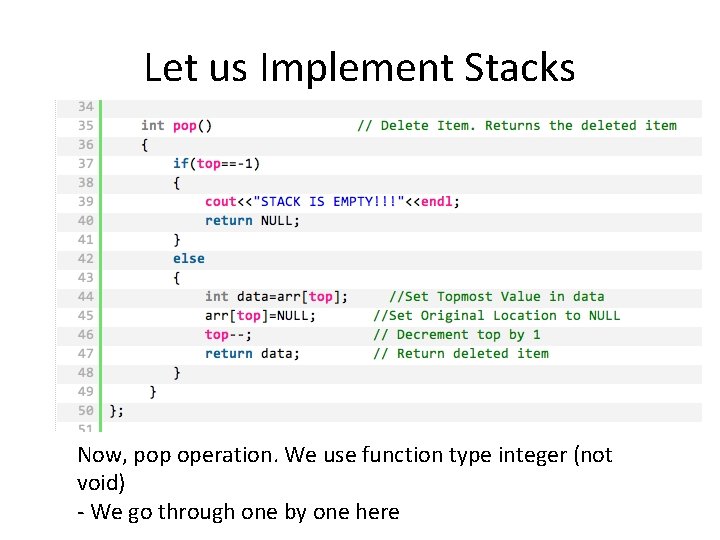
Let us Implement Stacks Now, pop operation. We use function type integer (not void) - We go through one by one here
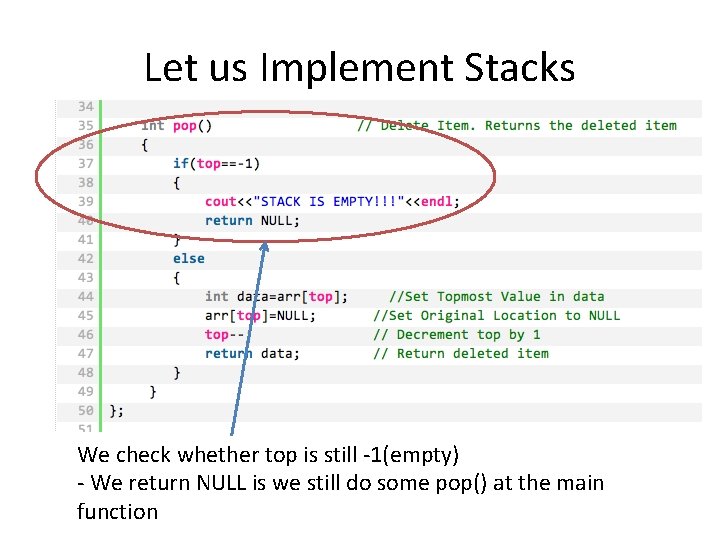
Let us Implement Stacks We check whether top is still -1(empty) - We return NULL is we still do some pop() at the main function
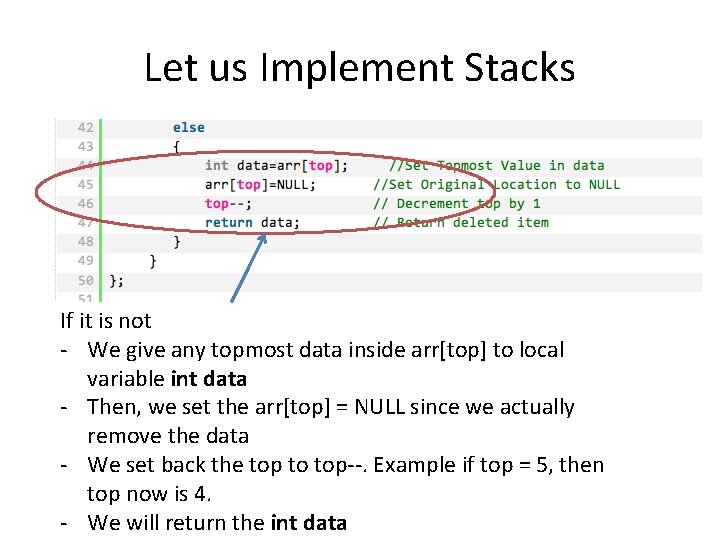
Let us Implement Stacks If it is not - We give any topmost data inside arr[top] to local variable int data - Then, we set the arr[top] = NULL since we actually remove the data - We set back the top to top--. Example if top = 5, then top now is 4. - We will return the int data
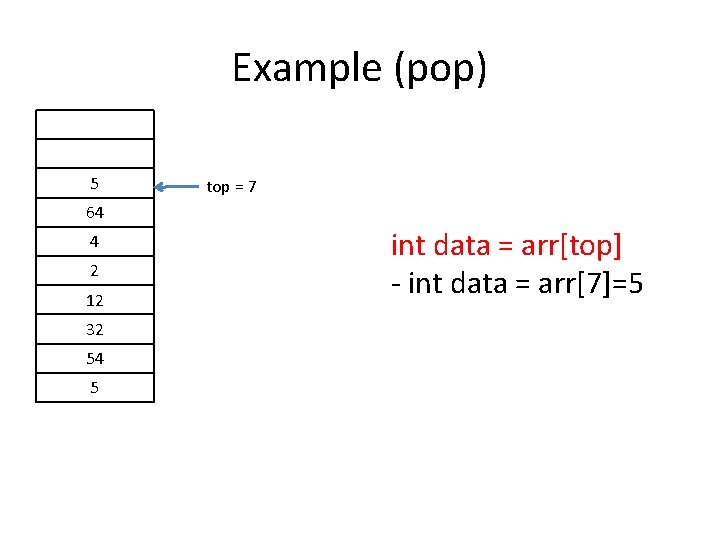
Example (pop) 5 top = 7 64 4 2 12 32 54 5 int data = arr[top] - int data = arr[7]=5
![Example pop top 7 64 4 2 12 32 54 5 arrtop NULL Example (pop) top = 7 64 4 2 12 32 54 5 arr[top]= NULL](https://slidetodoc.com/presentation_image_h/ae95104481aac74b58d6ffee20c98e95/image-28.jpg)
Example (pop) top = 7 64 4 2 12 32 54 5 arr[top]= NULL - arr[7] = NOTHING INSIDE
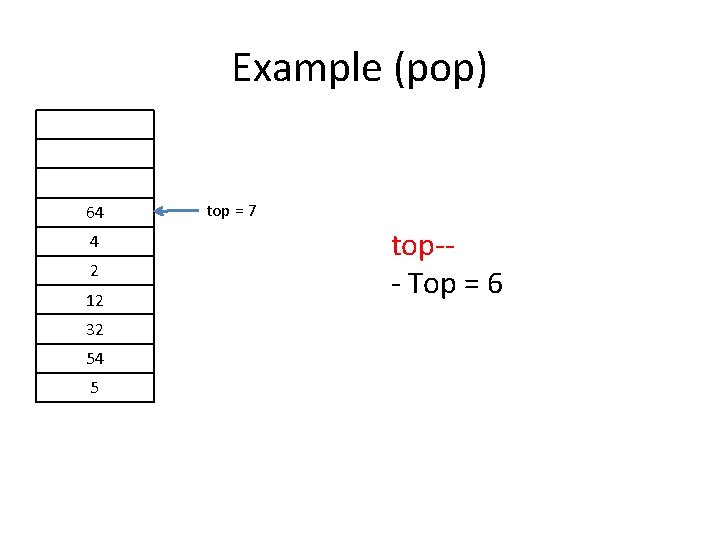
Example (pop) 64 4 2 12 32 54 5 top = 7 top-- Top = 6
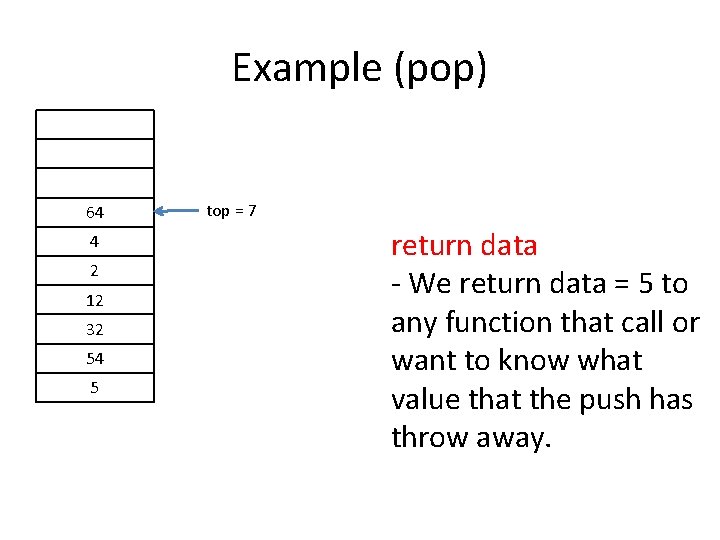
Example (pop) 64 4 2 12 32 54 5 top = 7 return data - We return data = 5 to any function that call or want to know what value that the push has throw away.
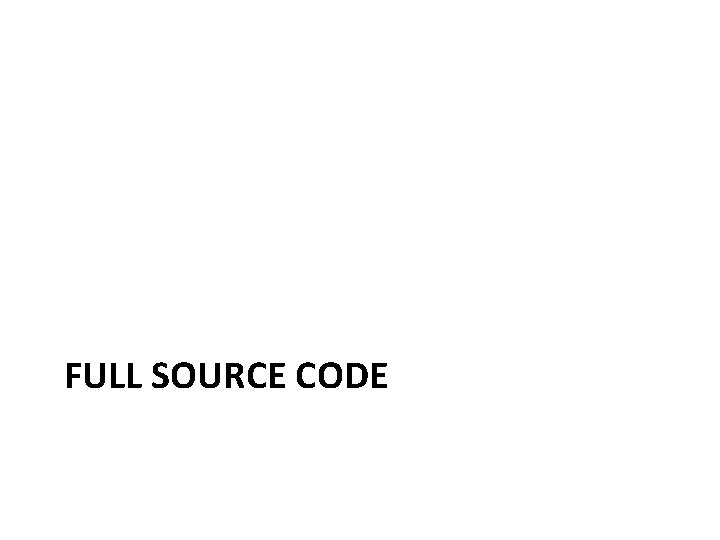
FULL SOURCE CODE
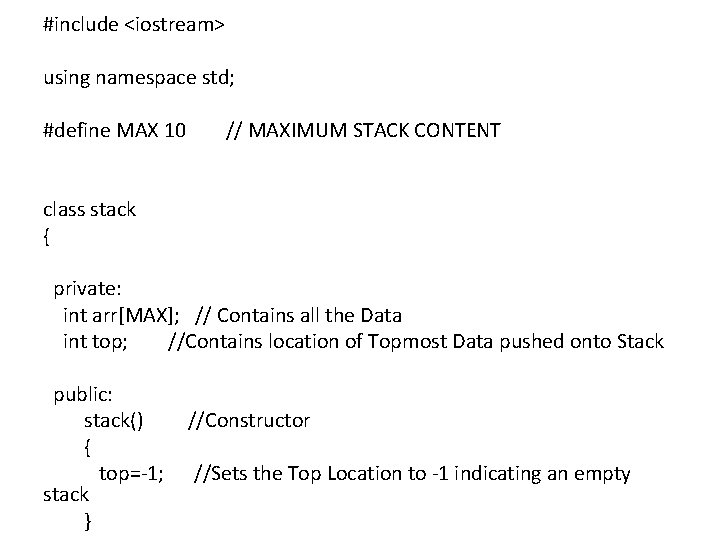
#include <iostream> using namespace std; #define MAX 10 // MAXIMUM STACK CONTENT class stack { private: int arr[MAX]; // Contains all the Data int top; //Contains location of Topmost Data pushed onto Stack public: stack() { top=-1; stack } //Constructor //Sets the Top Location to -1 indicating an empty
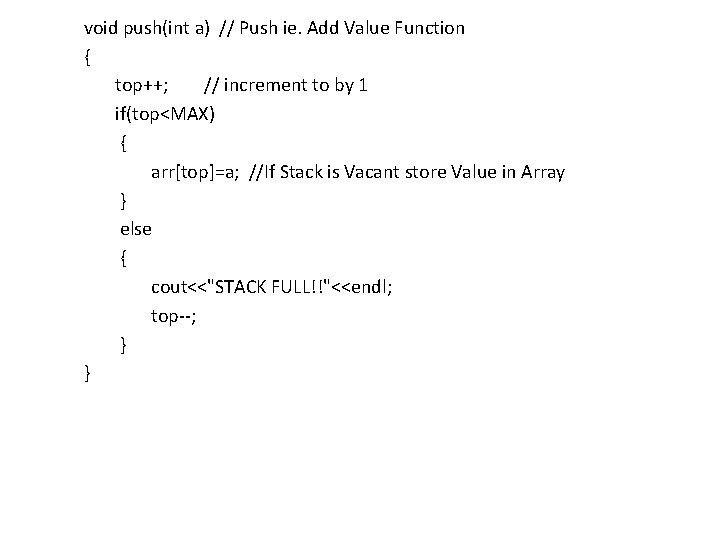
void push(int a) // Push ie. Add Value Function { top++; // increment to by 1 if(top<MAX) { arr[top]=a; //If Stack is Vacant store Value in Array } else { cout<<"STACK FULL!!"<<endl; top--; } }
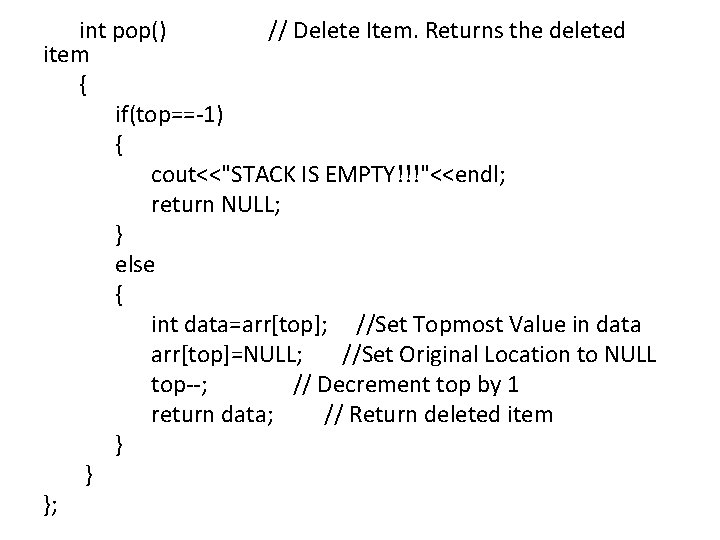
int pop() // Delete Item. Returns the deleted item { if(top==-1) { cout<<"STACK IS EMPTY!!!"<<endl; return NULL; } else { int data=arr[top]; //Set Topmost Value in data arr[top]=NULL; //Set Original Location to NULL top--; // Decrement top by 1 return data; // Return deleted item } } };
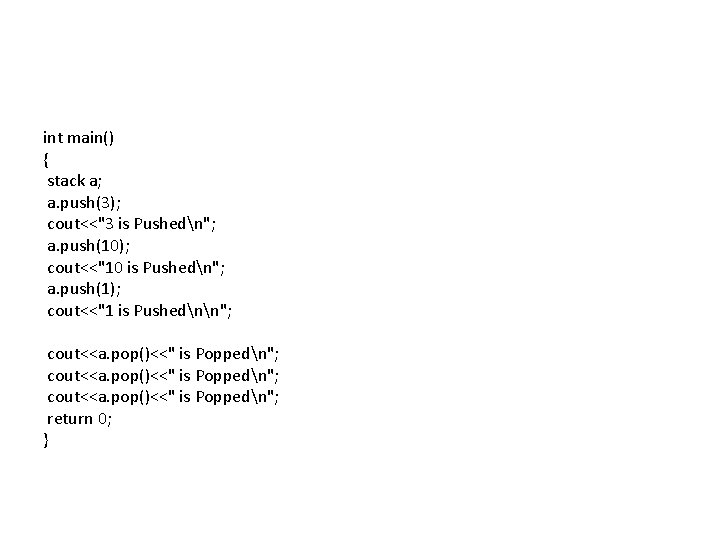
int main() { stack a; a. push(3); cout<<"3 is Pushedn"; a. push(10); cout<<"10 is Pushedn"; a. push(1); cout<<"1 is Pushednn"; cout<<a. pop()<<" is Poppedn"; return 0; }
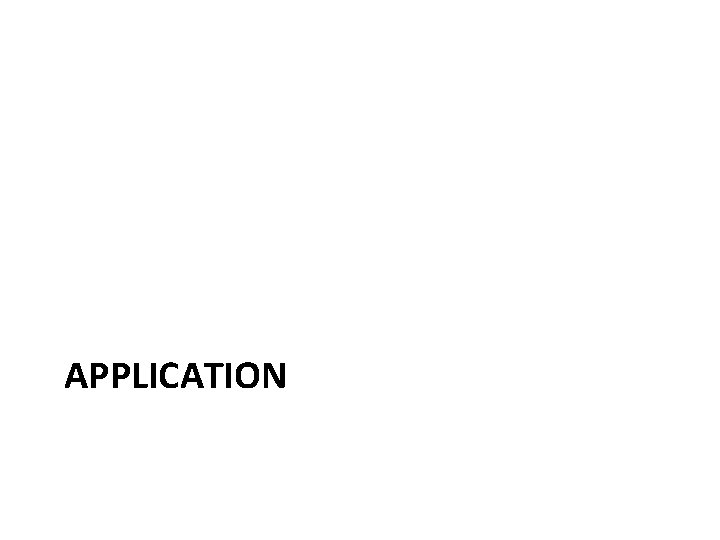
APPLICATION
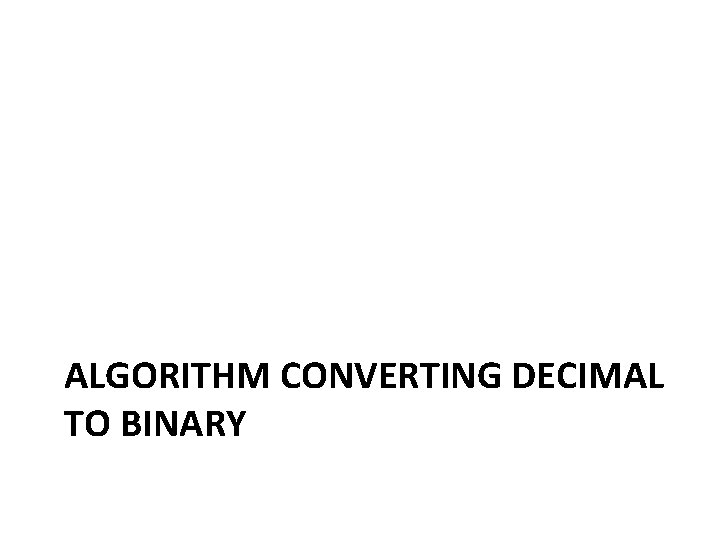
ALGORITHM CONVERTING DECIMAL TO BINARY
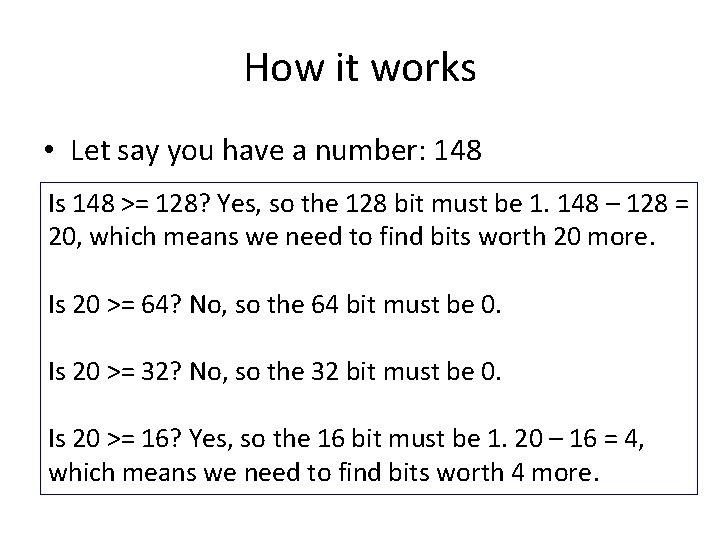
How it works • Let say you have a number: 148 Is 148 >= 128? Yes, so the 128 bit must be 1. 148 – 128 = 20, which means we need to find bits worth 20 more. Is 20 >= 64? No, so the 64 bit must be 0. Is 20 >= 32? No, so the 32 bit must be 0. Is 20 >= 16? Yes, so the 16 bit must be 1. 20 – 16 = 4, which means we need to find bits worth 4 more.
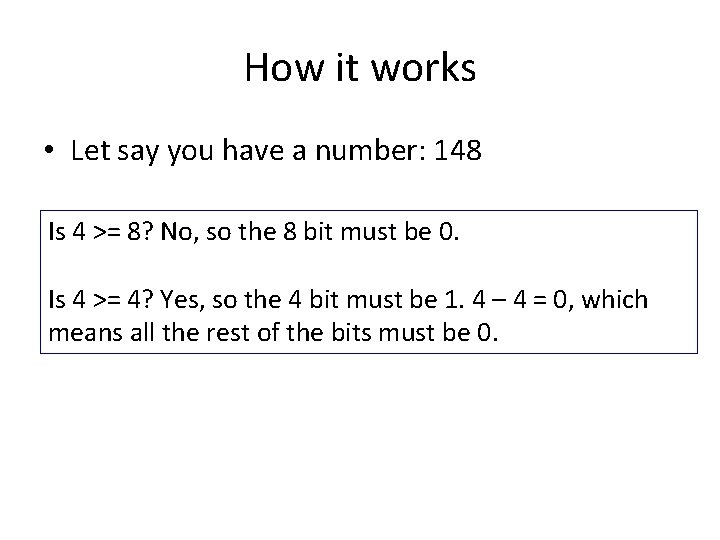
How it works • Let say you have a number: 148 Is 4 >= 8? No, so the 8 bit must be 0. Is 4 >= 4? Yes, so the 4 bit must be 1. 4 – 4 = 0, which means all the rest of the bits must be 0.
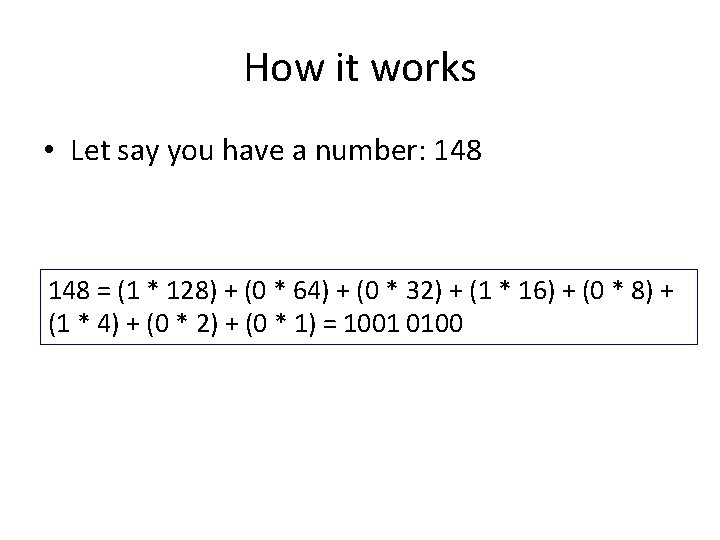
How it works • Let say you have a number: 148 = (1 * 128) + (0 * 64) + (0 * 32) + (1 * 16) + (0 * 8) + (1 * 4) + (0 * 2) + (0 * 1) = 1001 0100
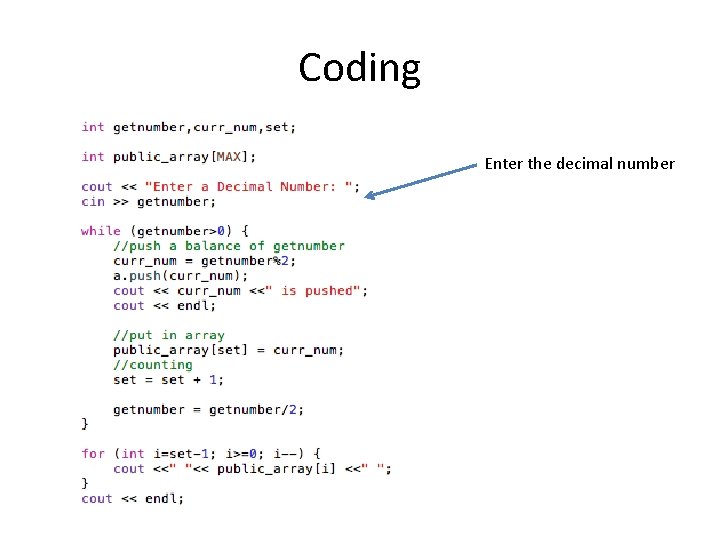
Coding Enter the decimal number
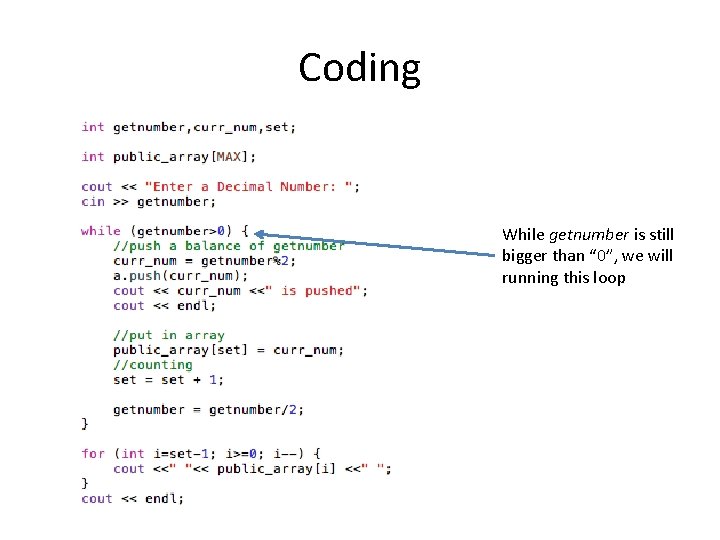
Coding While getnumber is still bigger than “ 0”, we will running this loop
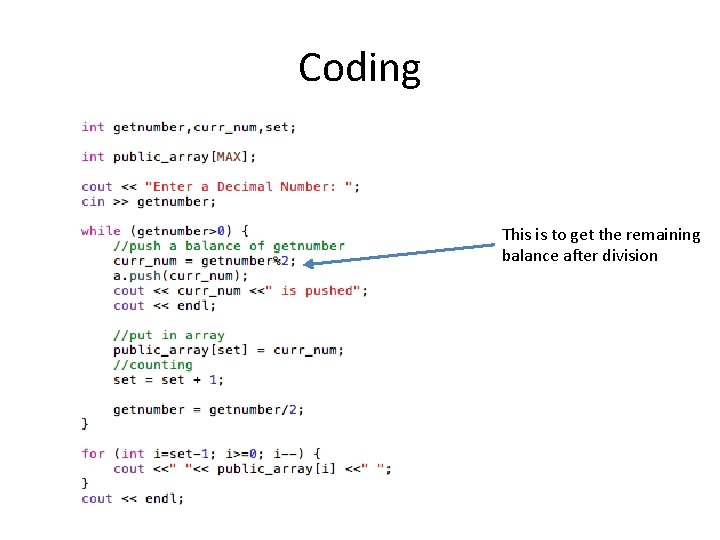
Coding This is to get the remaining balance after division
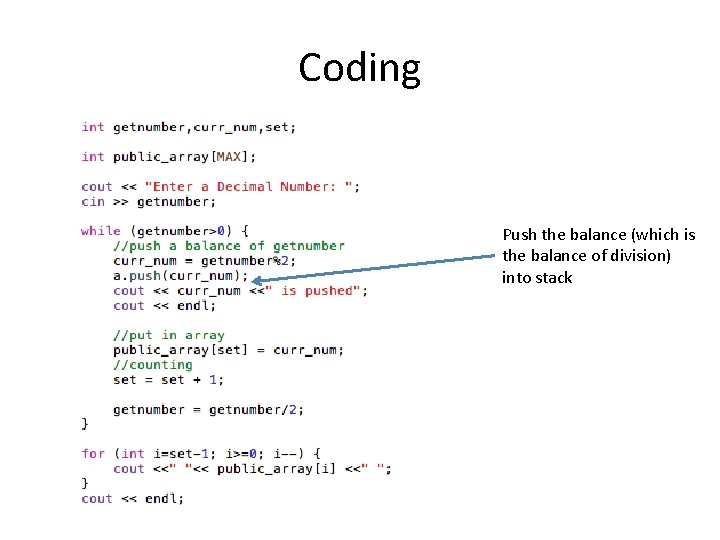
Coding Push the balance (which is the balance of division) into stack
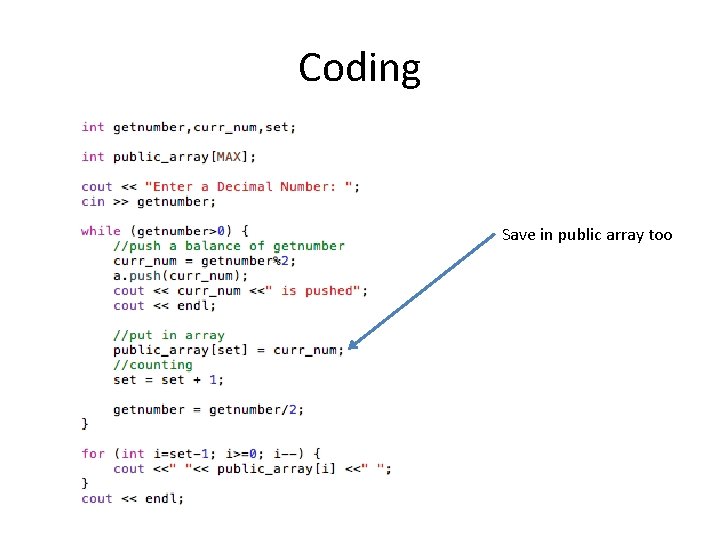
Coding Save in public array too
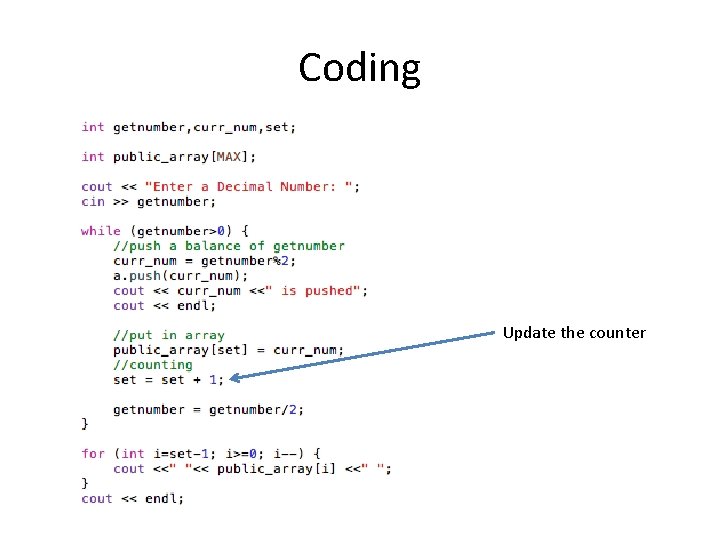
Coding Update the counter
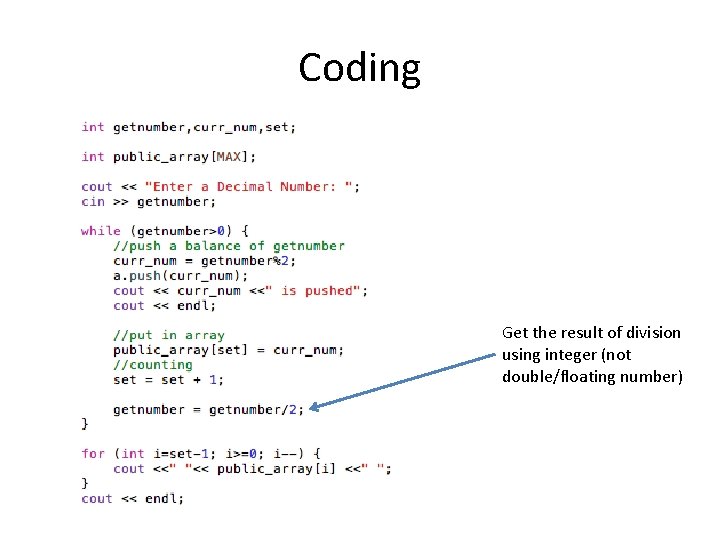
Coding Get the result of division using integer (not double/floating number)
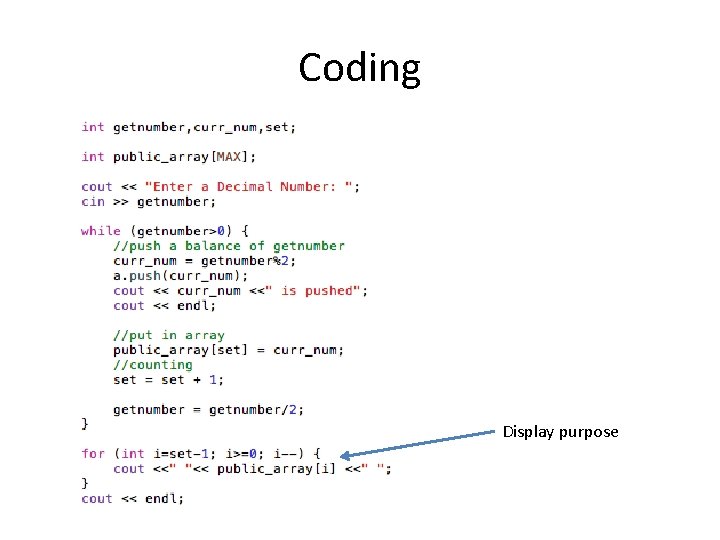
Coding Display purpose
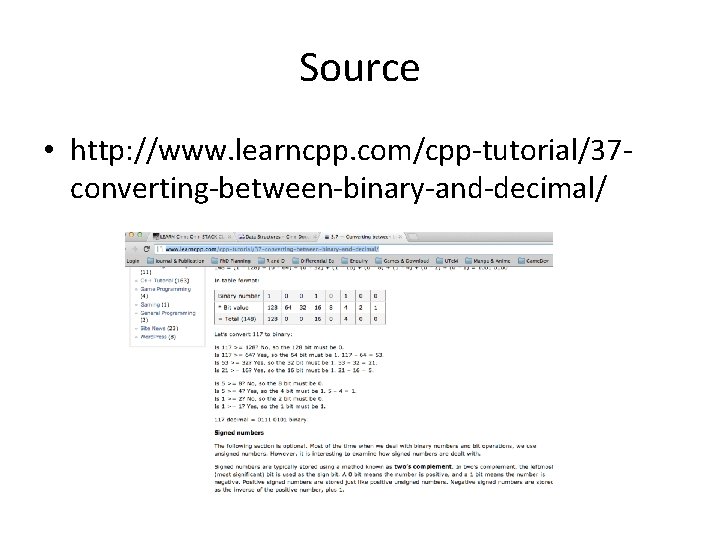
Source • http: //www. learncpp. com/cpp-tutorial/37 converting-between-binary-and-decimal/
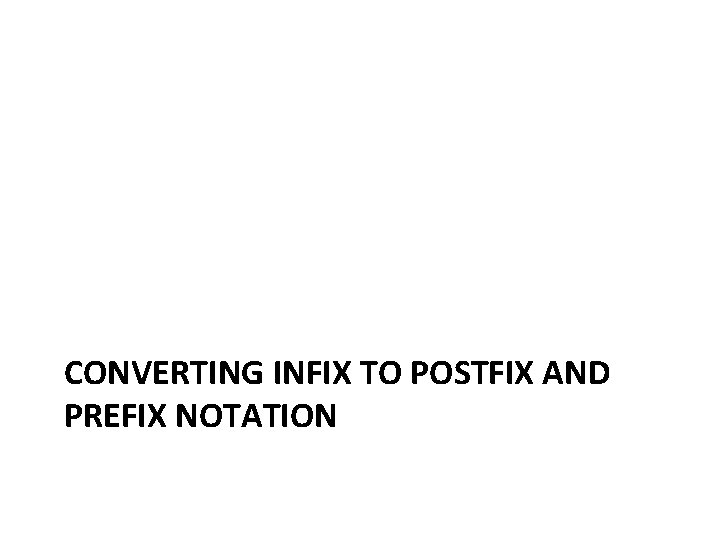
CONVERTING INFIX TO POSTFIX AND PREFIX NOTATION
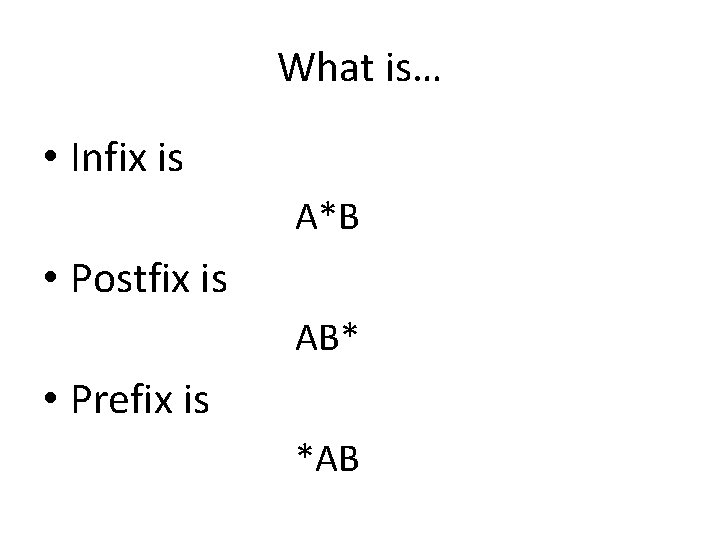
What is… • Infix is A*B • Postfix is AB* • Prefix is *AB
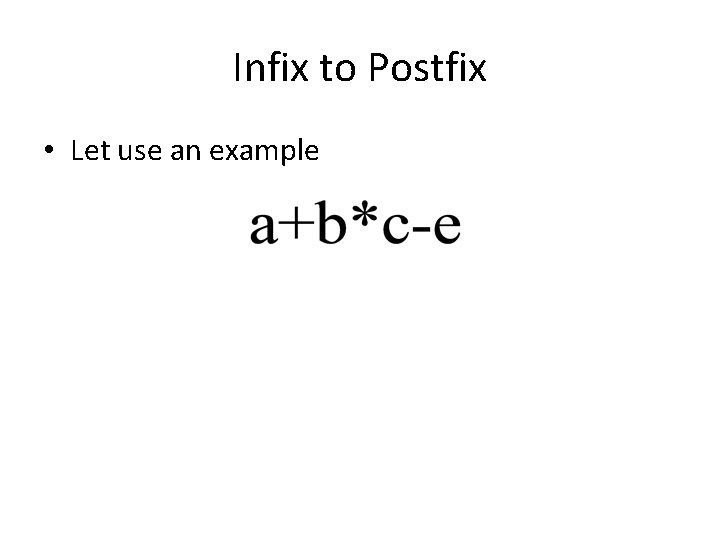
Infix to Postfix • Let use an example
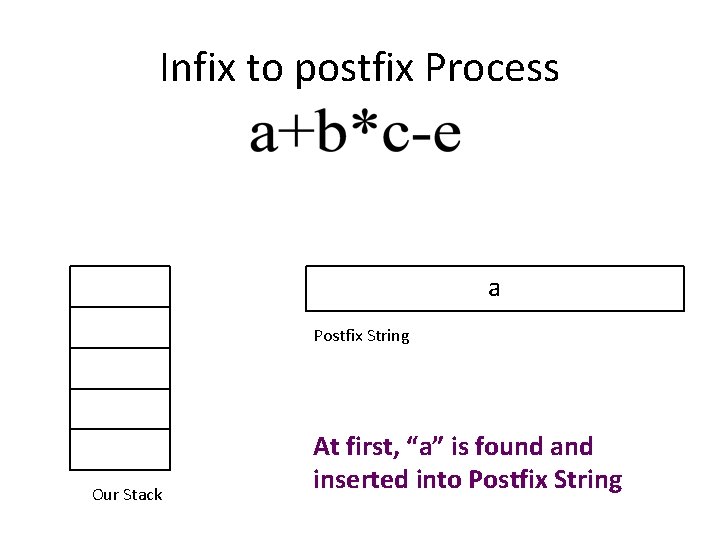
Infix to postfix Process a Postfix String Our Stack At first, “a” is found and inserted into Postfix String
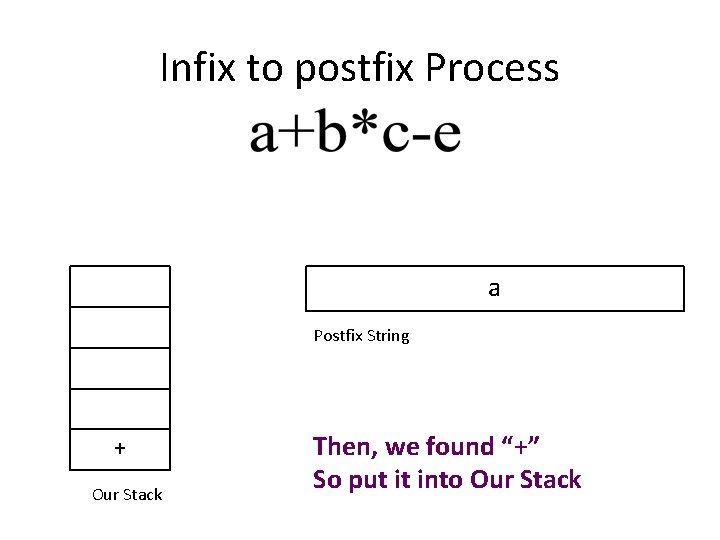
Infix to postfix Process a Postfix String + Our Stack Then, we found “+” So put it into Our Stack
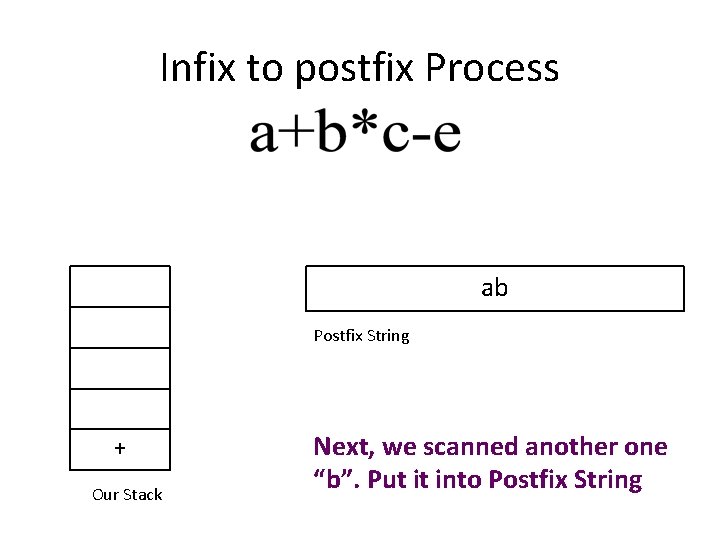
Infix to postfix Process ab Postfix String + Our Stack Next, we scanned another one “b”. Put it into Postfix String
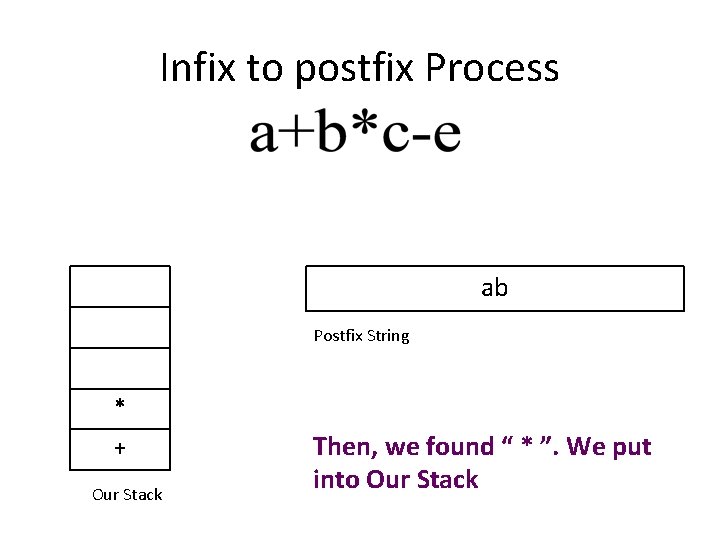
Infix to postfix Process ab Postfix String * + Our Stack Then, we found “ * ”. We put into Our Stack
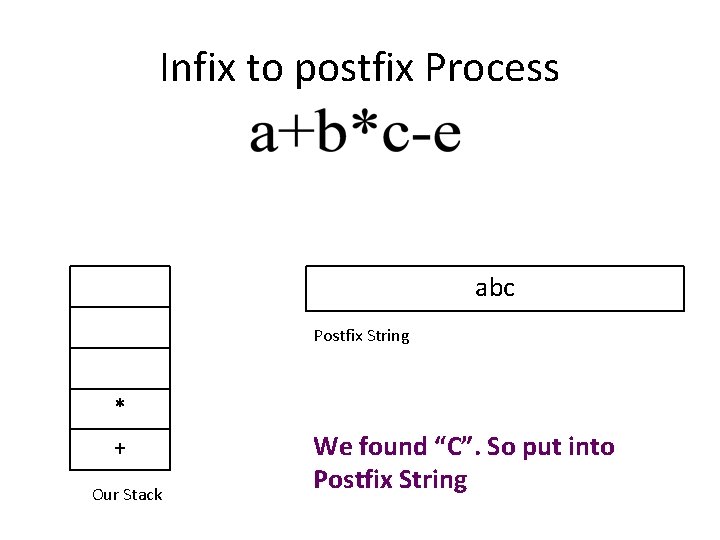
Infix to postfix Process abc Postfix String * + Our Stack We found “C”. So put into Postfix String
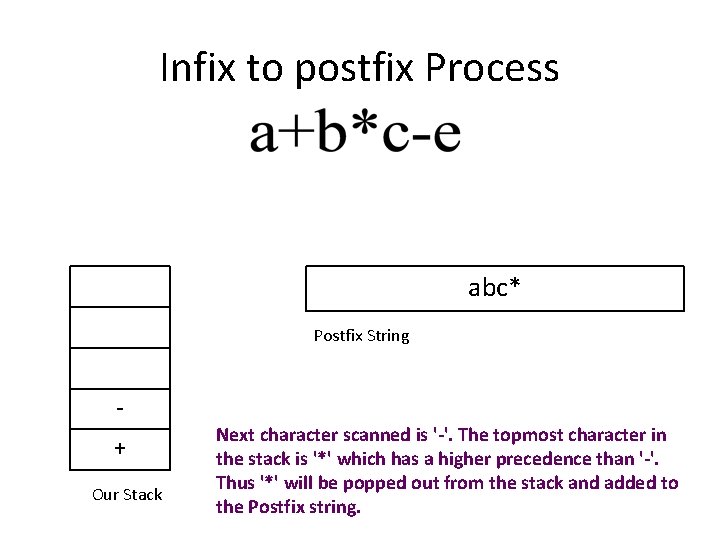
Infix to postfix Process abc* Postfix String + Our Stack Next character scanned is '-'. The topmost character in the stack is '*' which has a higher precedence than '-'. Thus '*' will be popped out from the stack and added to the Postfix string.
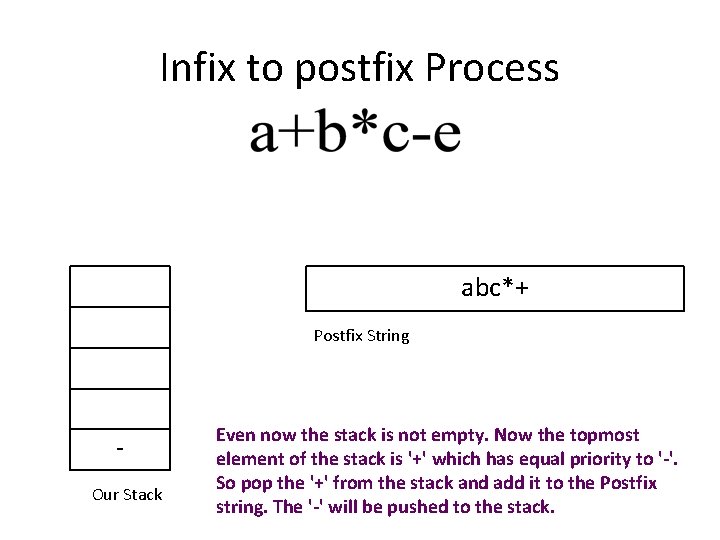
Infix to postfix Process abc*+ Postfix String Our Stack Even now the stack is not empty. Now the topmost element of the stack is '+' which has equal priority to '-'. So pop the '+' from the stack and add it to the Postfix string. The '-' will be pushed to the stack.
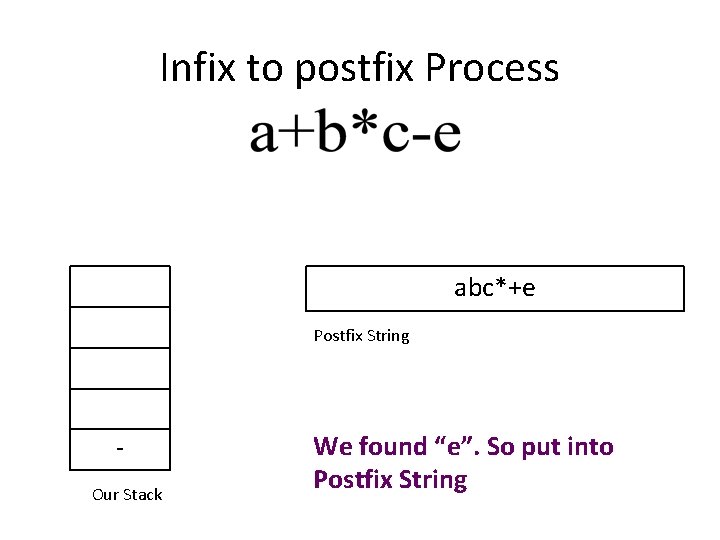
Infix to postfix Process abc*+e Postfix String Our Stack We found “e”. So put into Postfix String
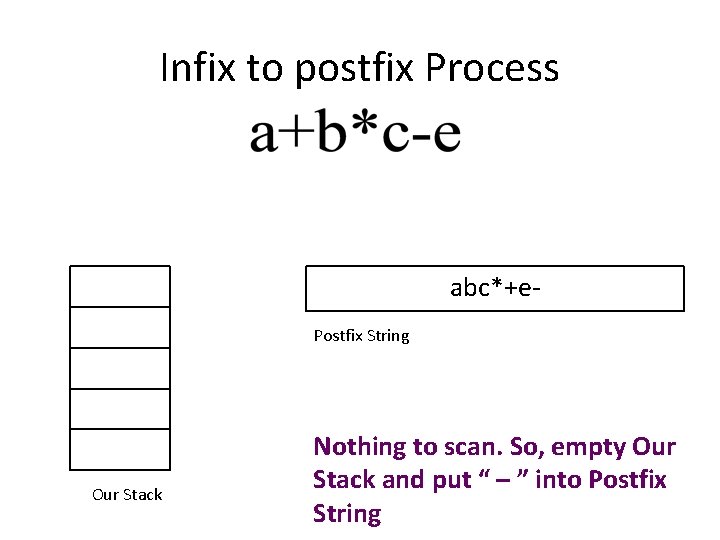
Infix to postfix Process abc*+e. Postfix String Our Stack Nothing to scan. So, empty Our Stack and put “ – ” into Postfix String
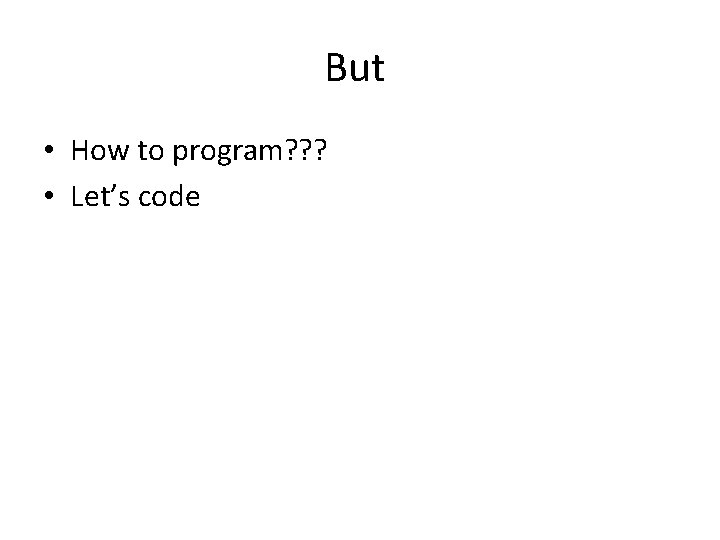
But • How to program? ? ? • Let’s code
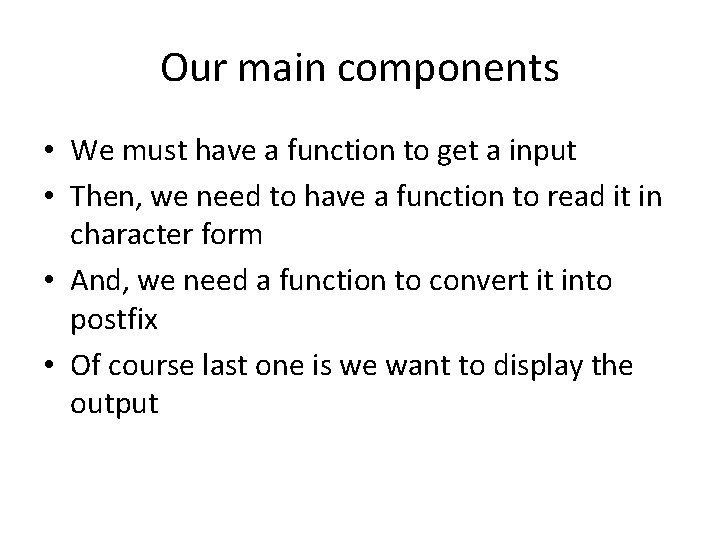
Our main components • We must have a function to get a input • Then, we need to have a function to read it in character form • And, we need a function to convert it into postfix • Of course last one is we want to display the output
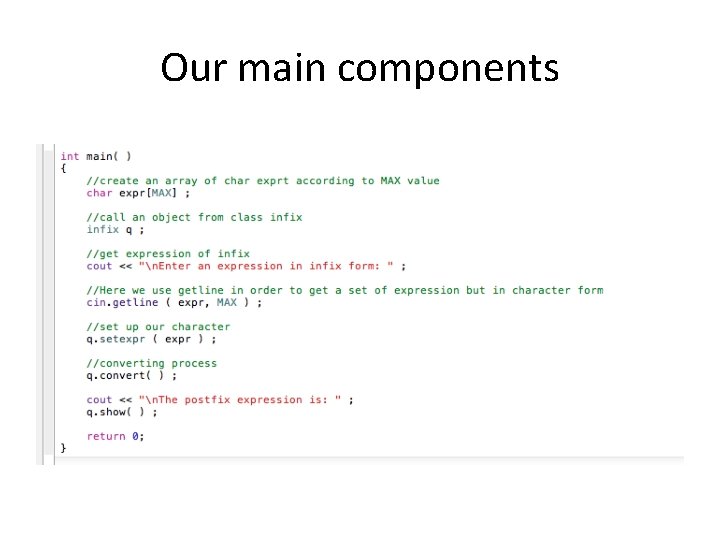
Our main components
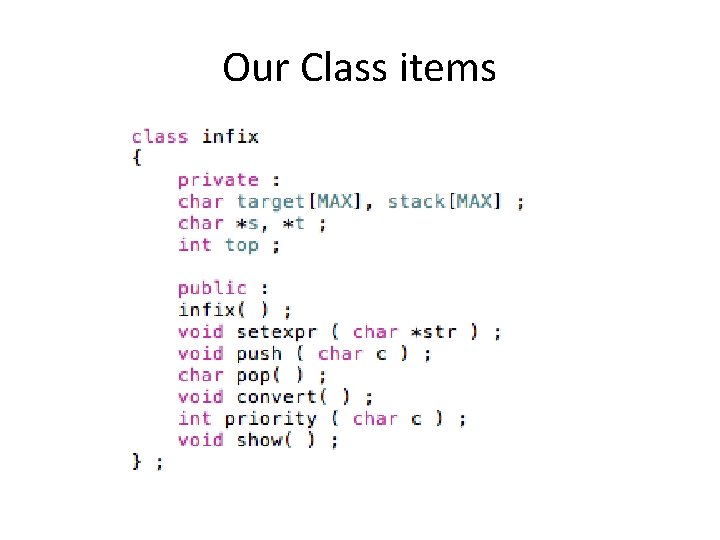
Our Class items
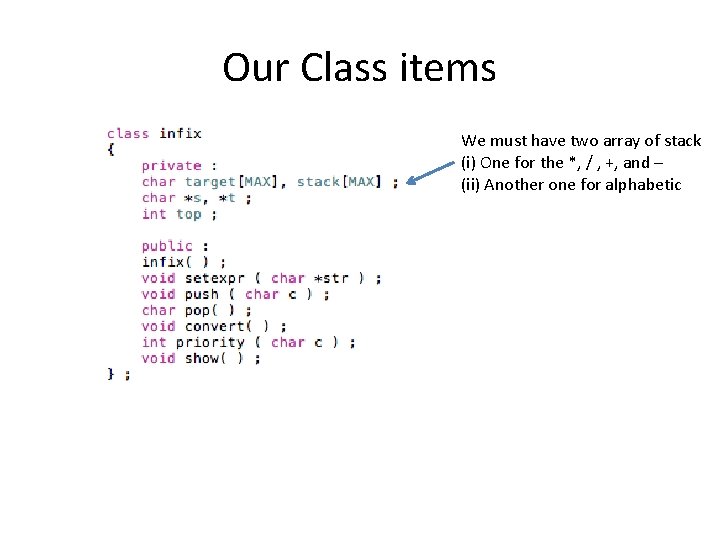
Our Class items We must have two array of stack (i) One for the *, / , +, and – (ii) Another one for alphabetic
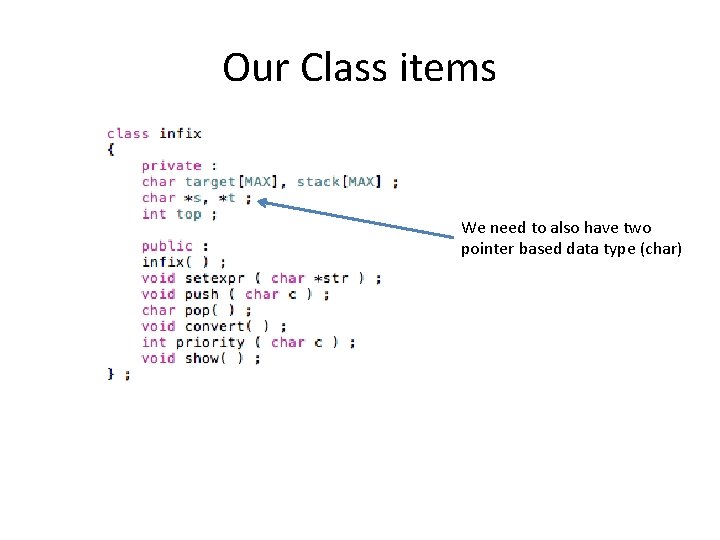
Our Class items We need to also have two pointer based data type (char)
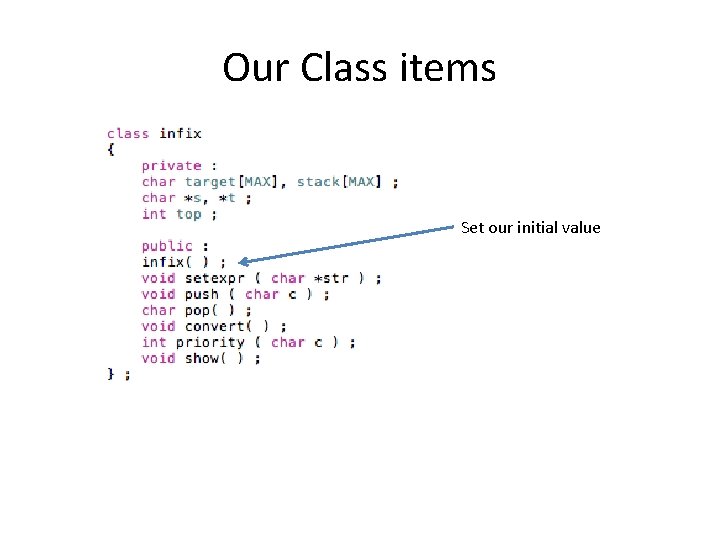
Our Class items Set our initial value
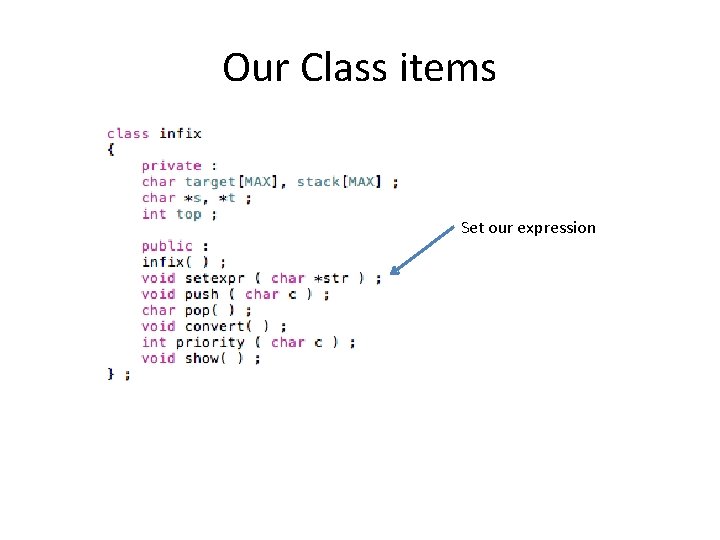
Our Class items Set our expression
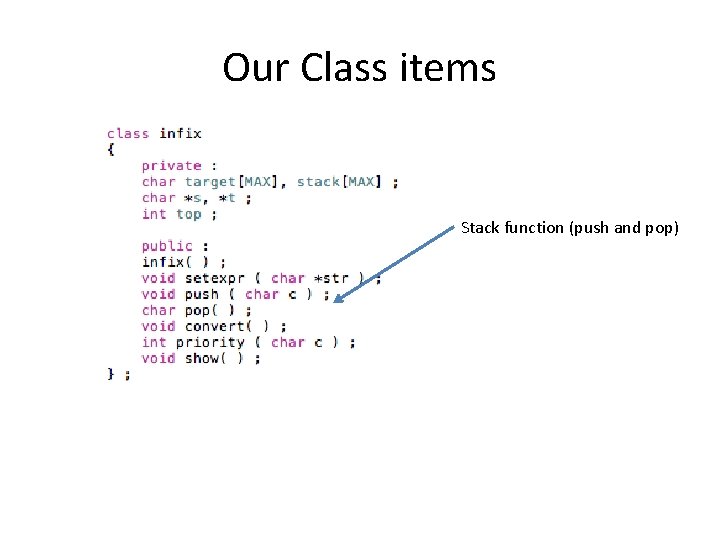
Our Class items Stack function (push and pop)
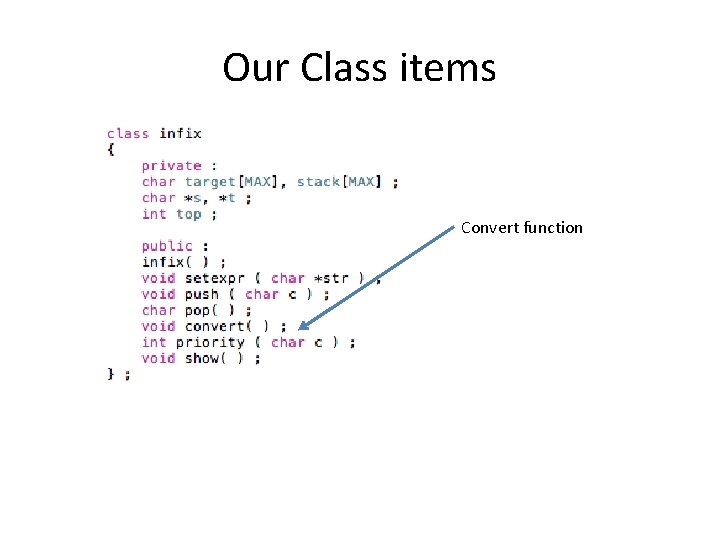
Our Class items Convert function
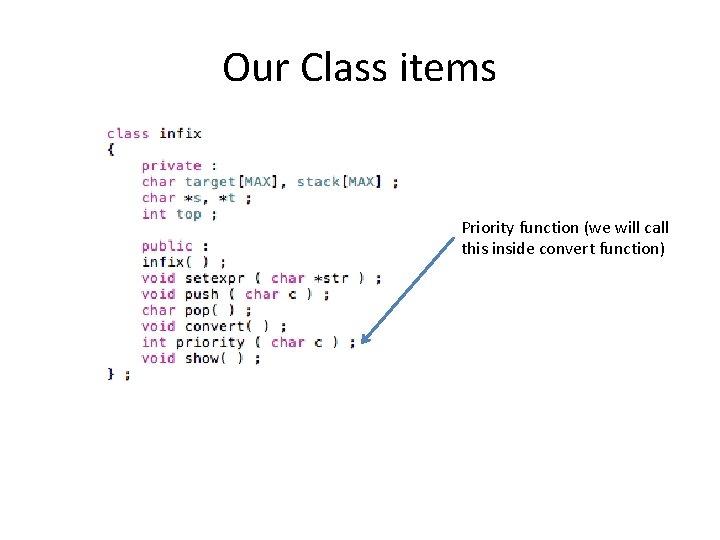
Our Class items Priority function (we will call this inside convert function)
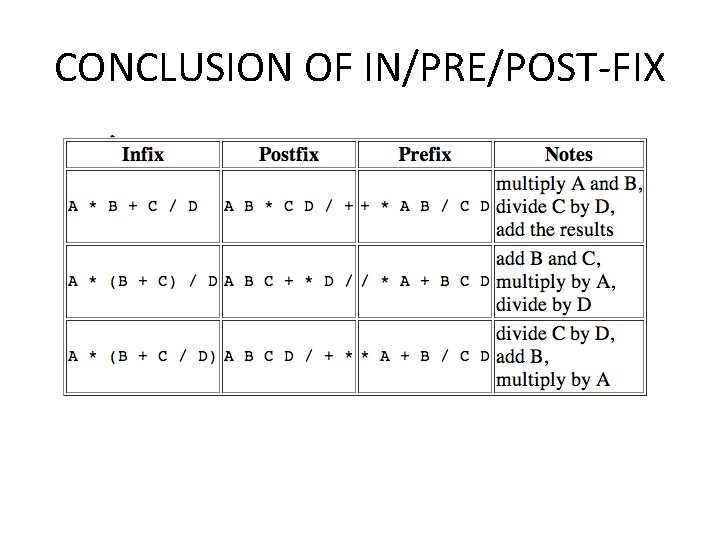
CONCLUSION OF IN/PRE/POST-FIX
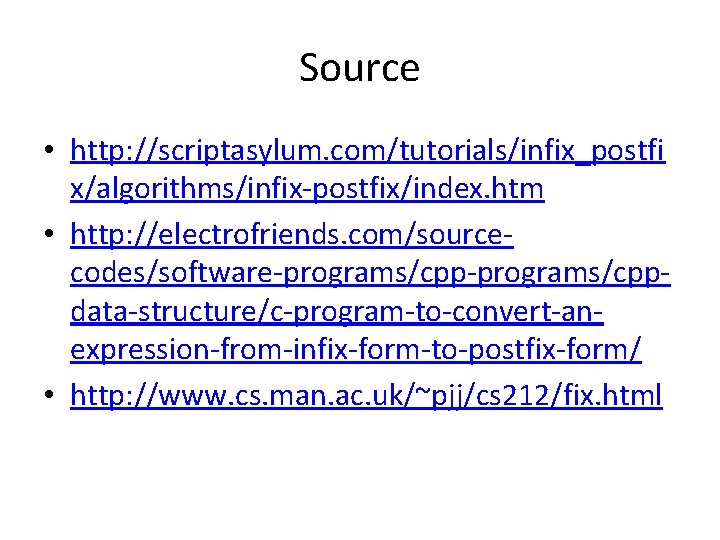
Source • http: //scriptasylum. com/tutorials/infix_postfi x/algorithms/infix-postfix/index. htm • http: //electrofriends. com/sourcecodes/software-programs/cppdata-structure/c-program-to-convert-anexpression-from-infix-form-to-postfix-form/ • http: //www. cs. man. ac. uk/~pjj/cs 212/fix. html
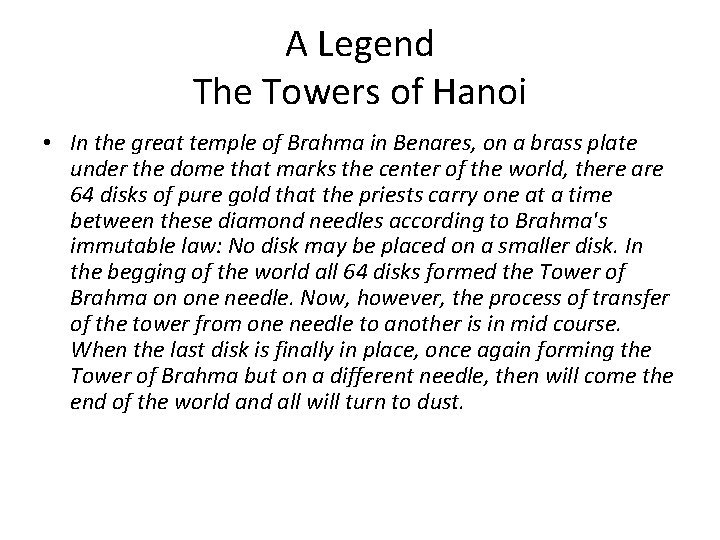
A Legend The Towers of Hanoi • In the great temple of Brahma in Benares, on a brass plate under the dome that marks the center of the world, there are 64 disks of pure gold that the priests carry one at a time between these diamond needles according to Brahma's immutable law: No disk may be placed on a smaller disk. In the begging of the world all 64 disks formed the Tower of Brahma on one needle. Now, however, the process of transfer of the tower from one needle to another is in mid course. When the last disk is finally in place, once again forming the Tower of Brahma but on a different needle, then will come the end of the world and all will turn to dust.
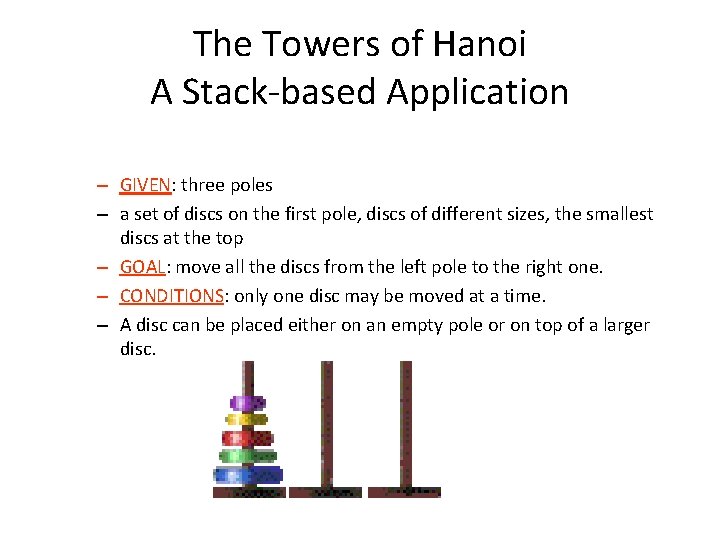
The Towers of Hanoi A Stack-based Application – GIVEN: three poles – a set of discs on the first pole, discs of different sizes, the smallest discs at the top – GOAL: move all the discs from the left pole to the right one. – CONDITIONS: only one disc may be moved at a time. – A disc can be placed either on an empty pole or on top of a larger disc.
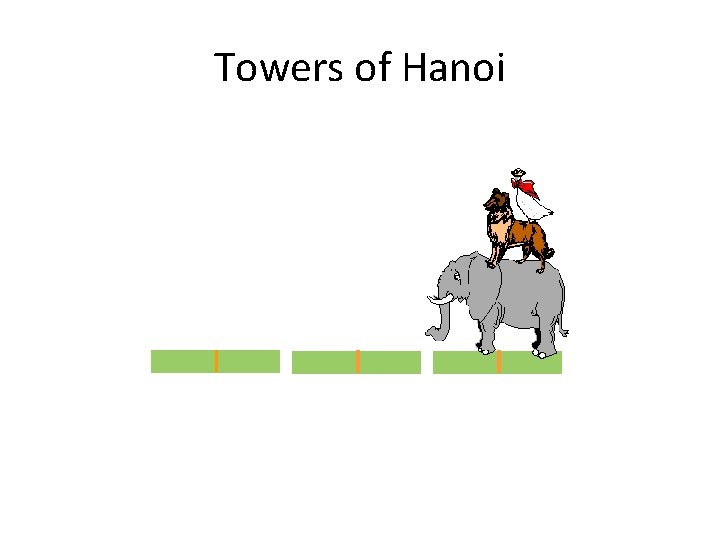
Towers of Hanoi
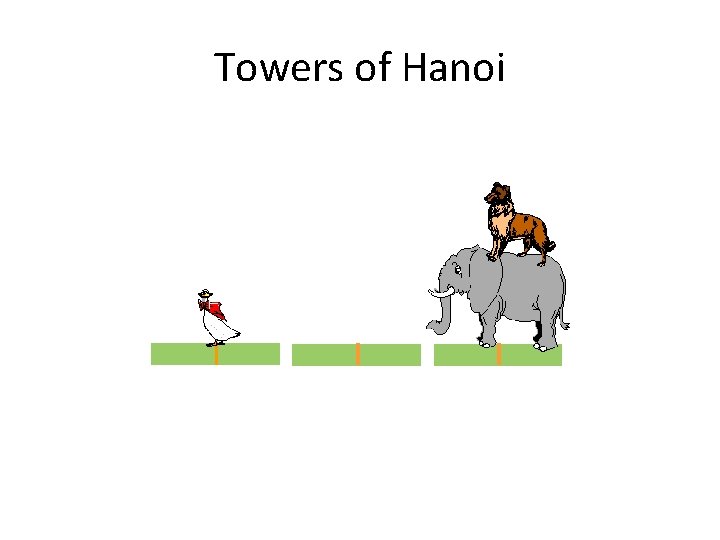
Towers of Hanoi
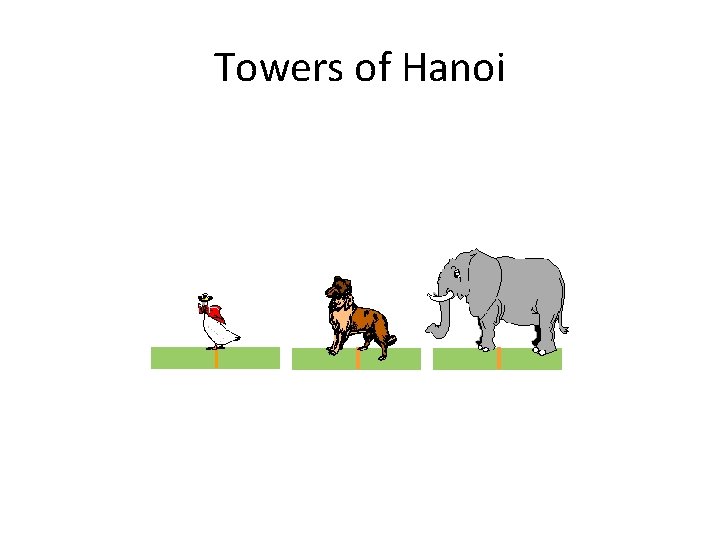
Towers of Hanoi
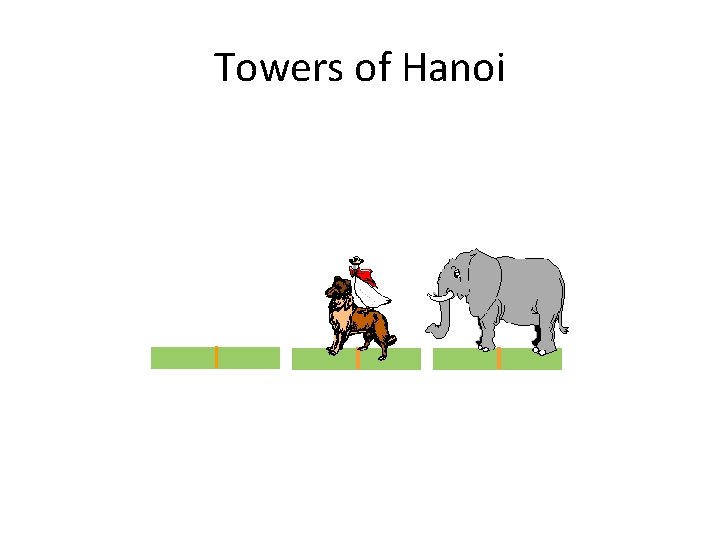
Towers of Hanoi
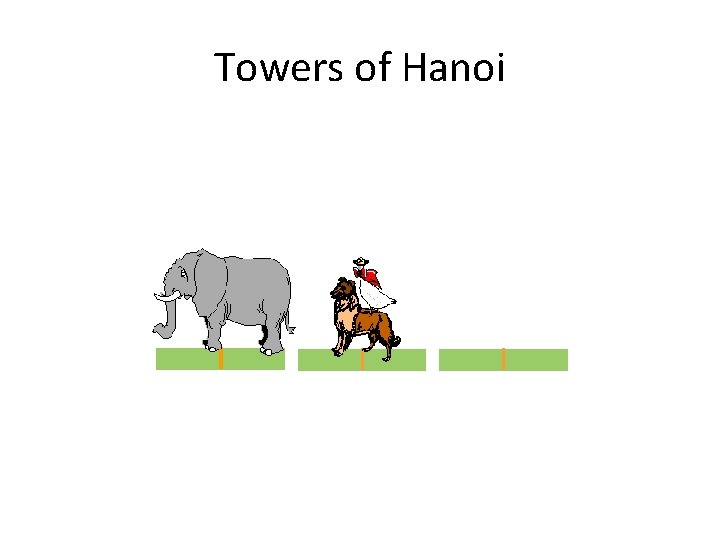
Towers of Hanoi
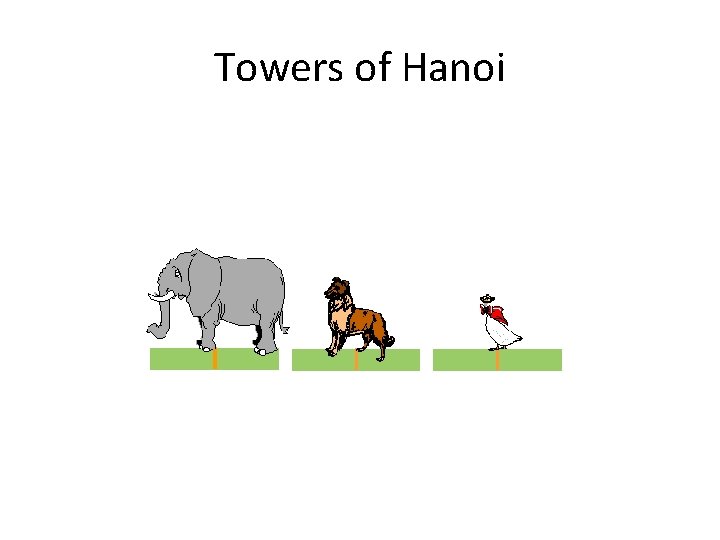
Towers of Hanoi
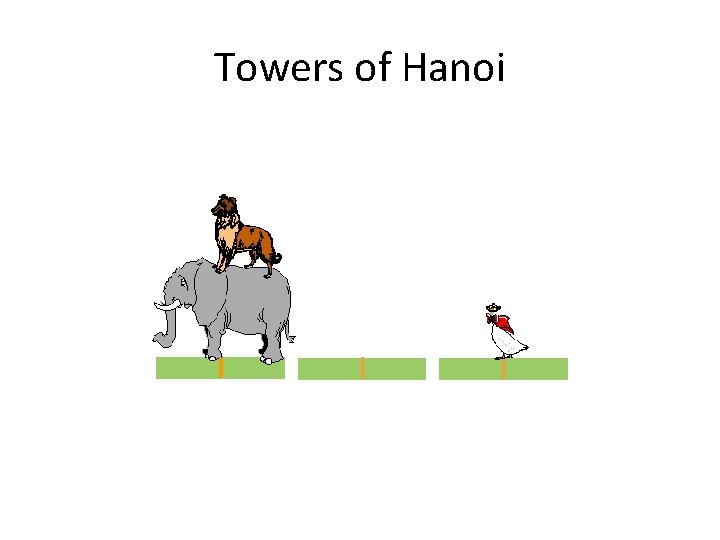
Towers of Hanoi
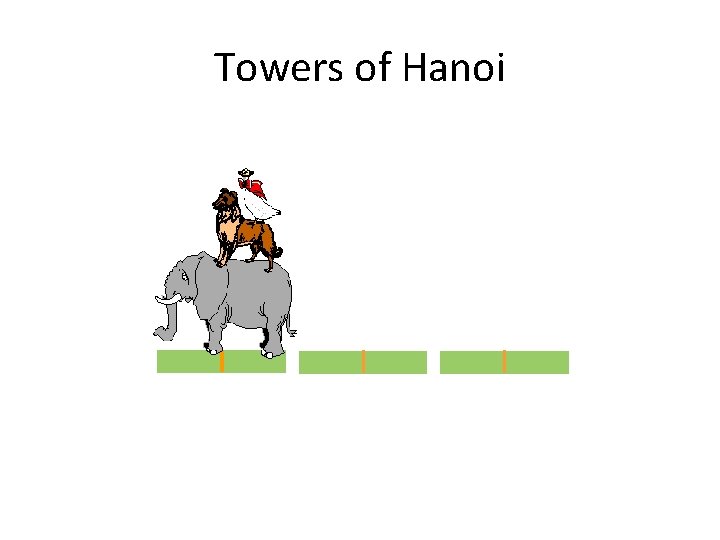
Towers of Hanoi
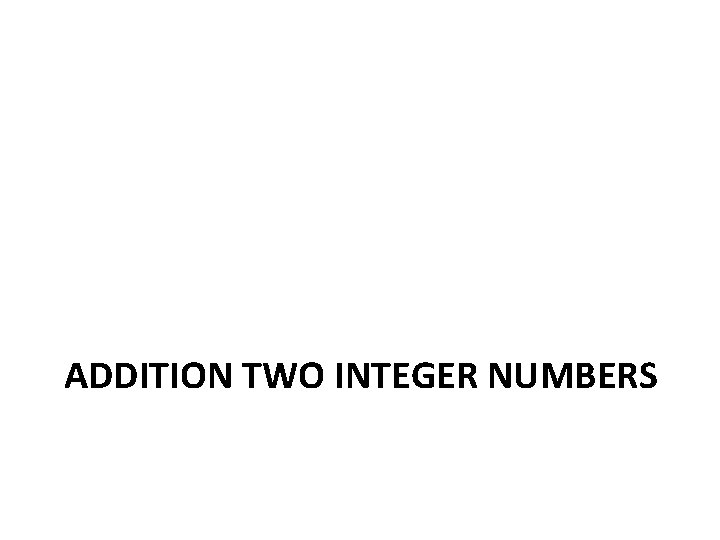
ADDITION TWO INTEGER NUMBERS
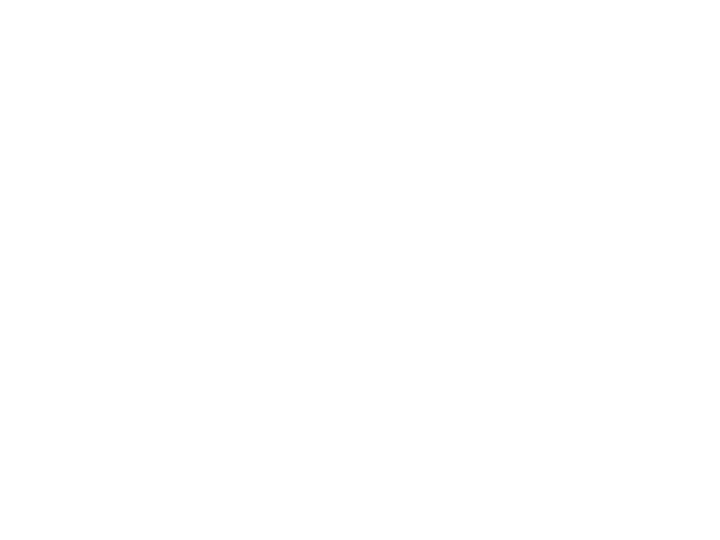