Chapter 4 InterProcess Communication IPC On The Same
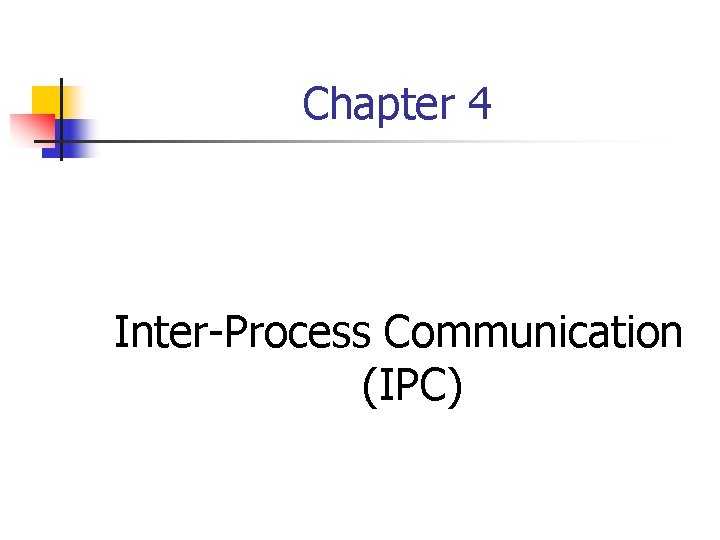
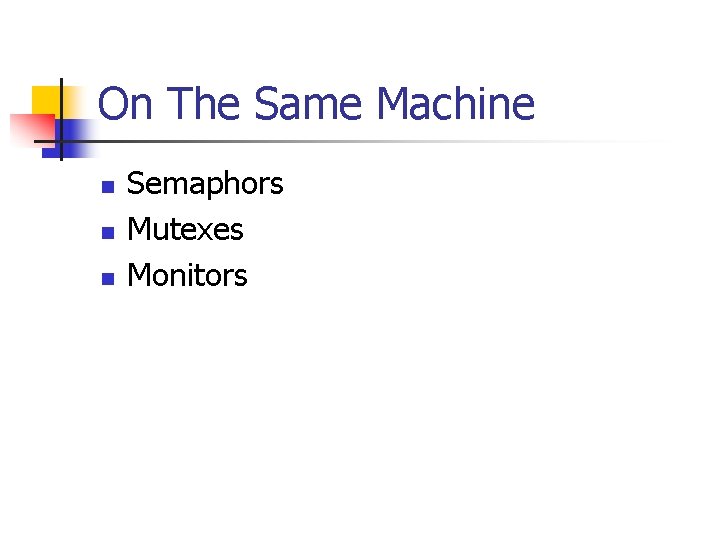
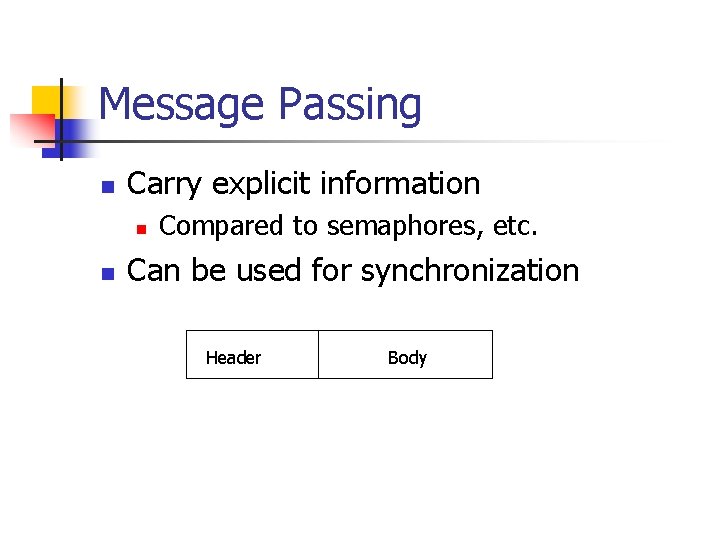
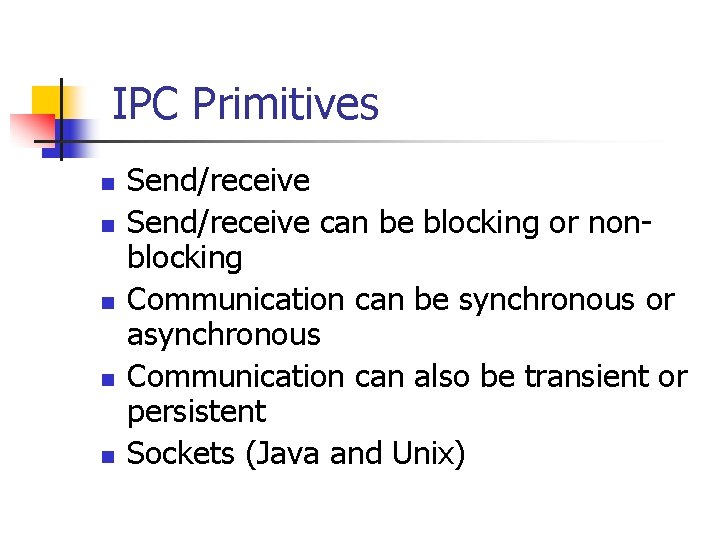
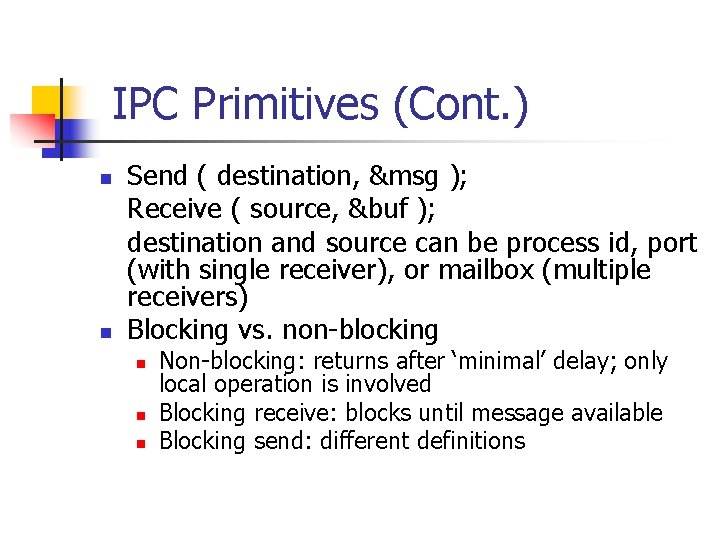
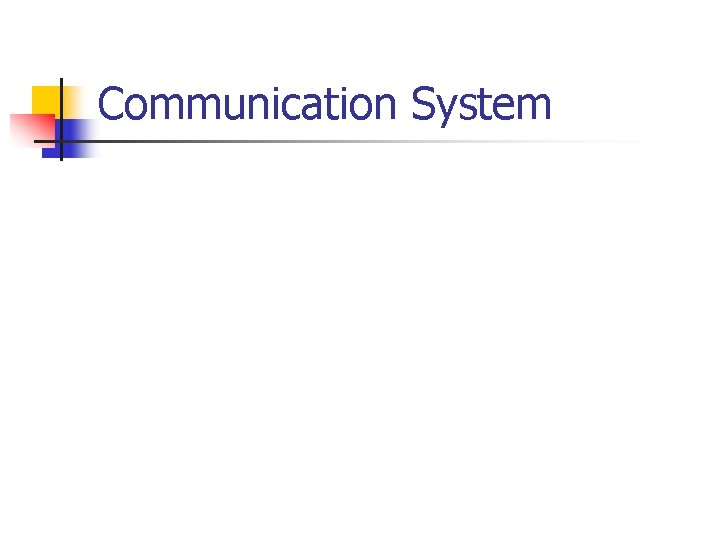
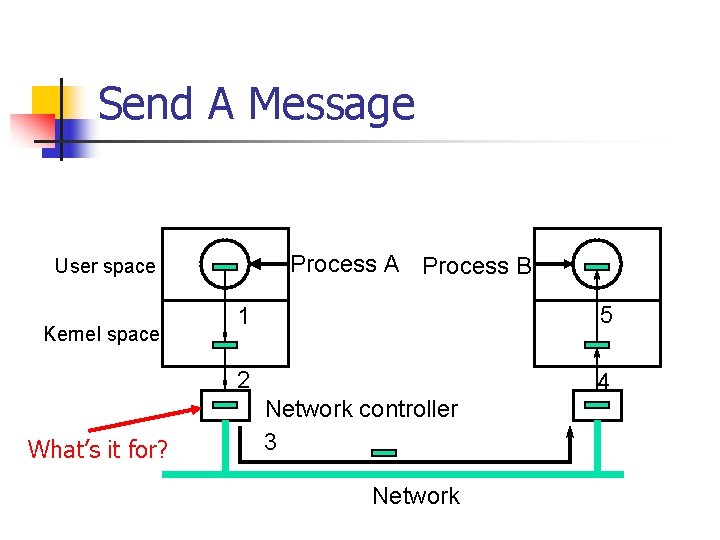
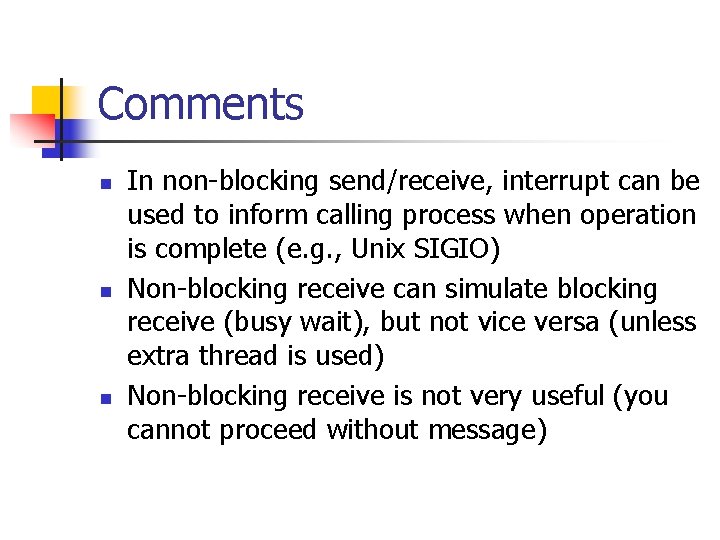
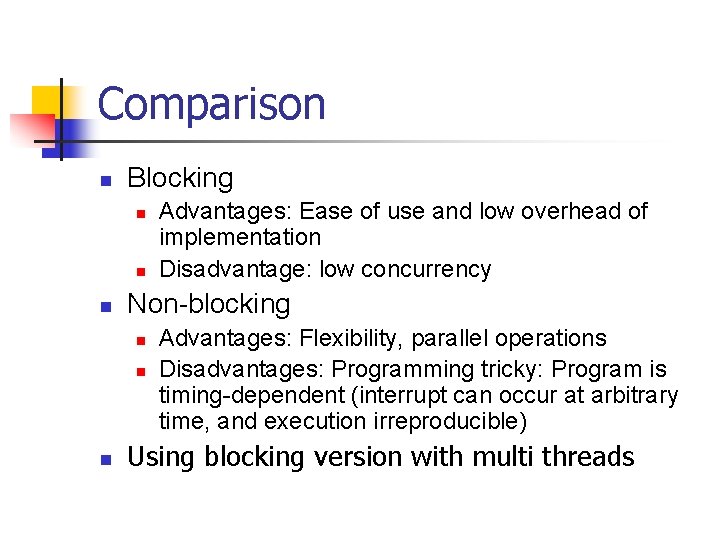
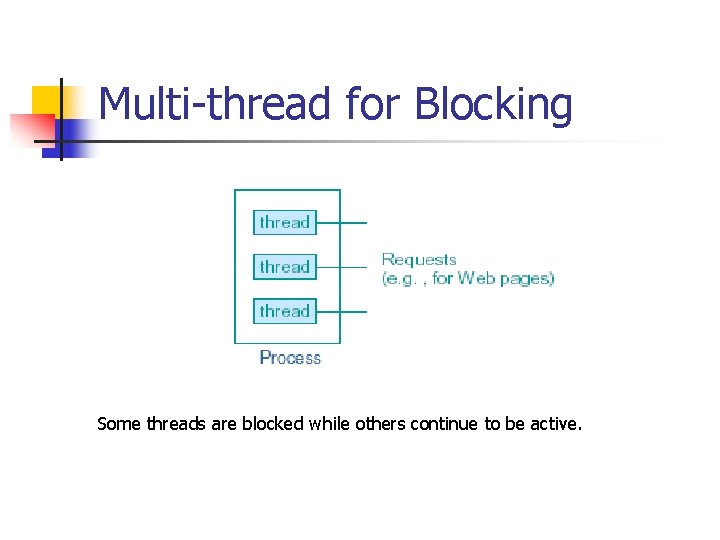
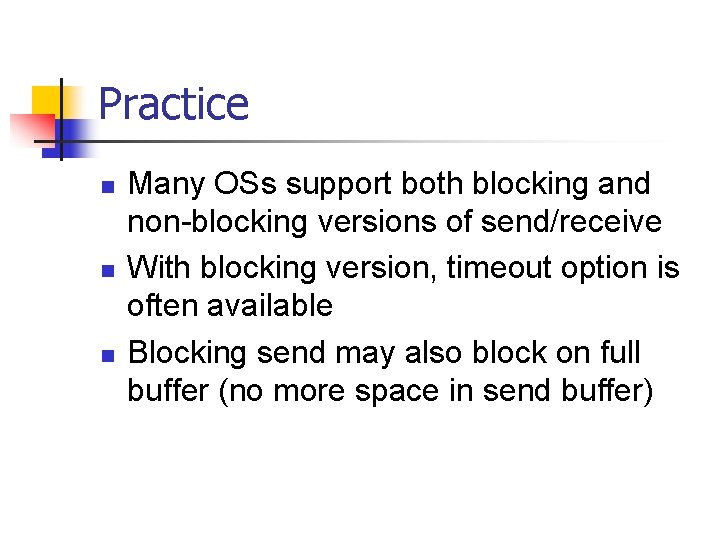
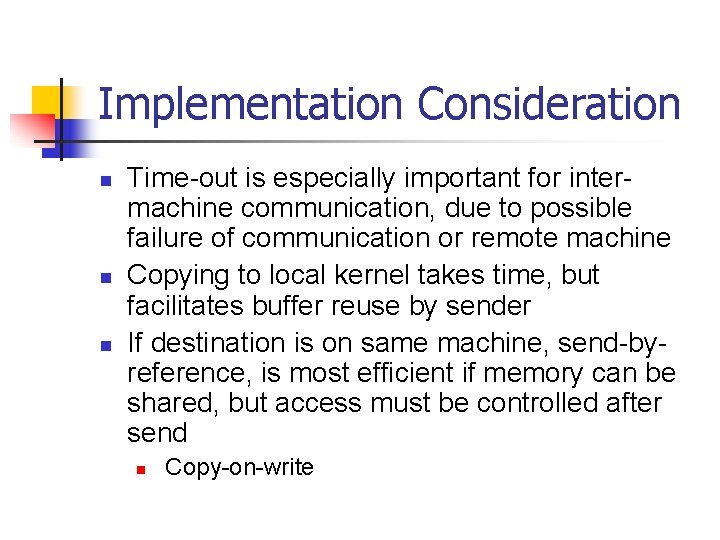
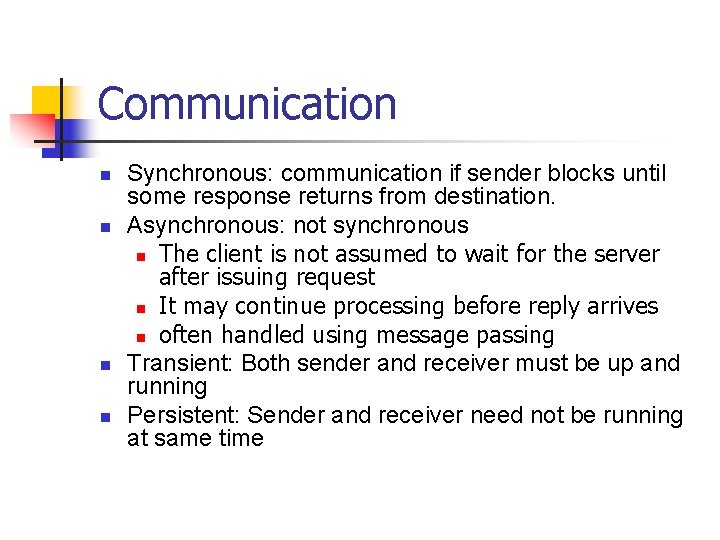
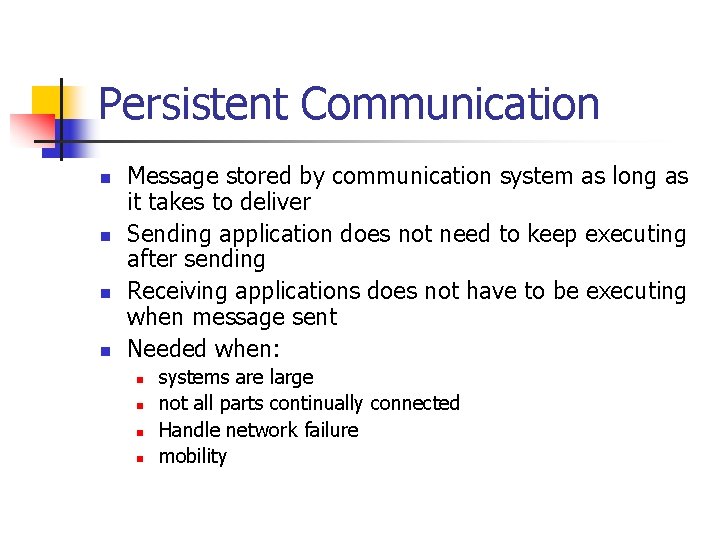
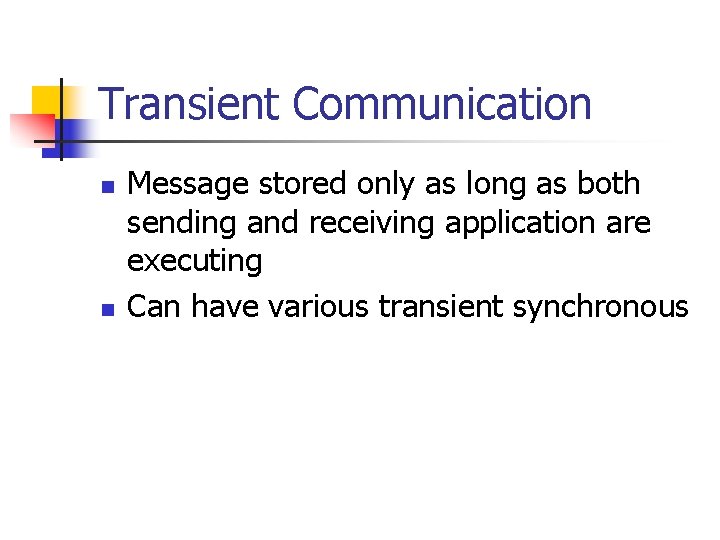
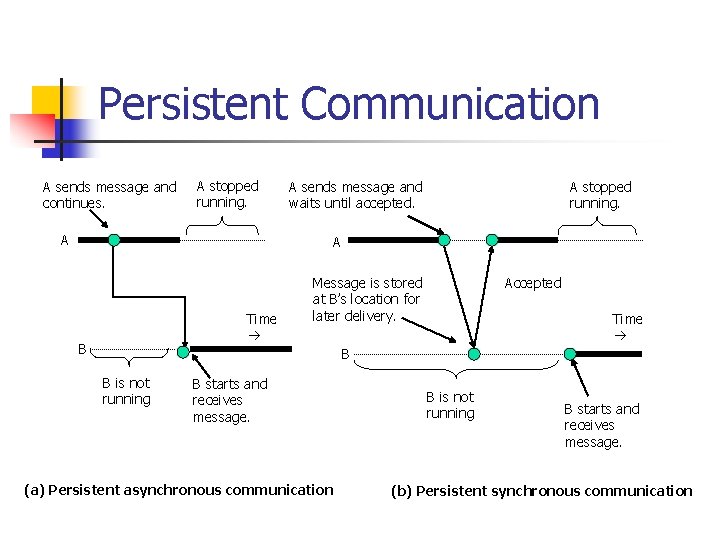
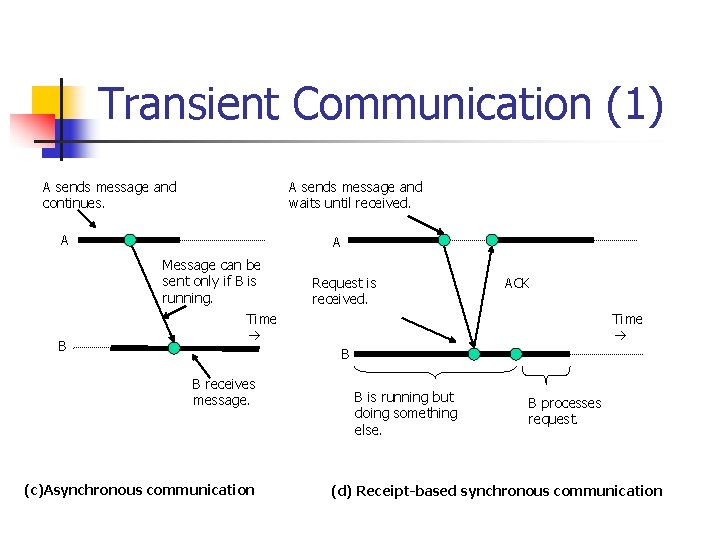
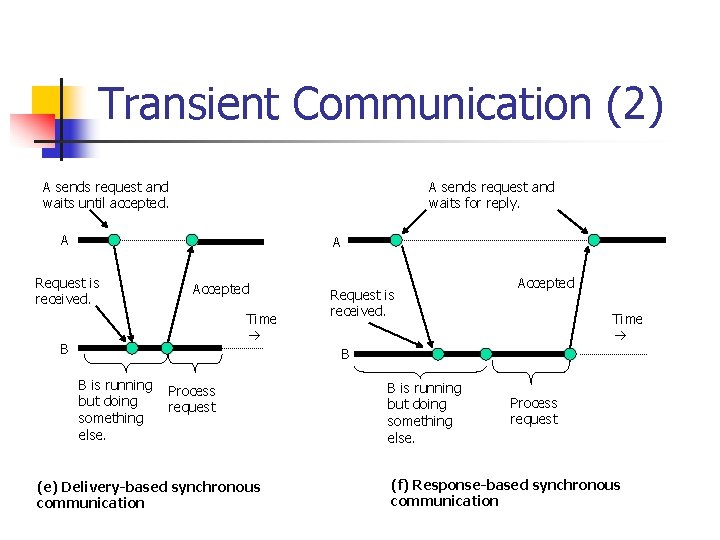
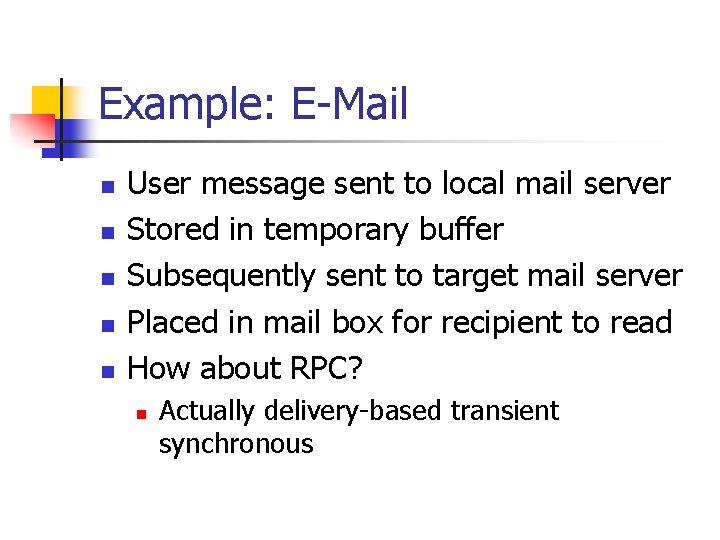
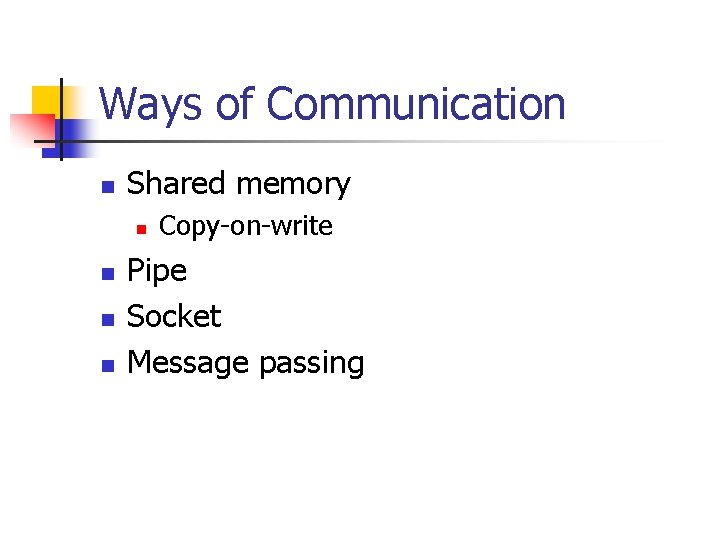
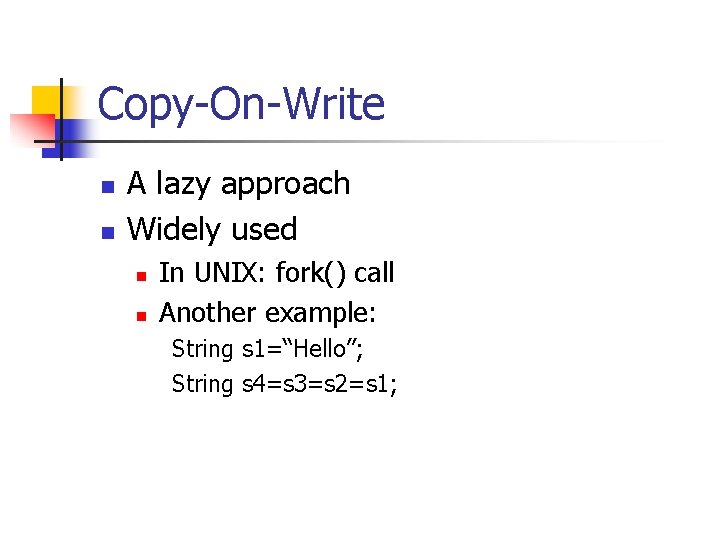
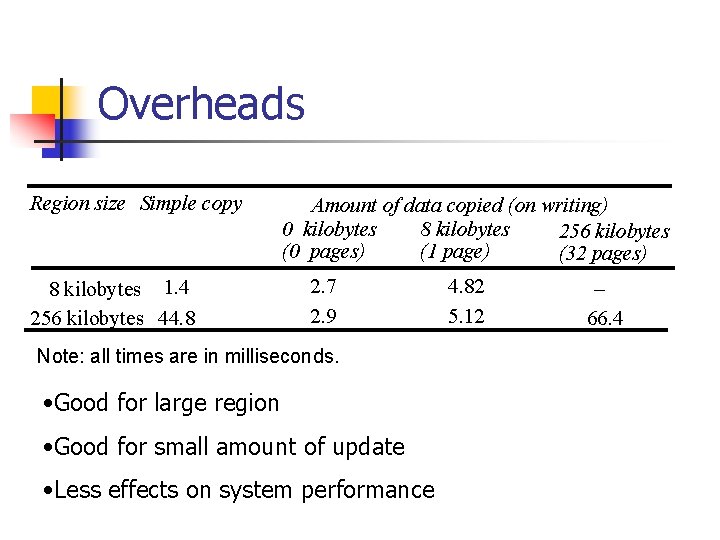
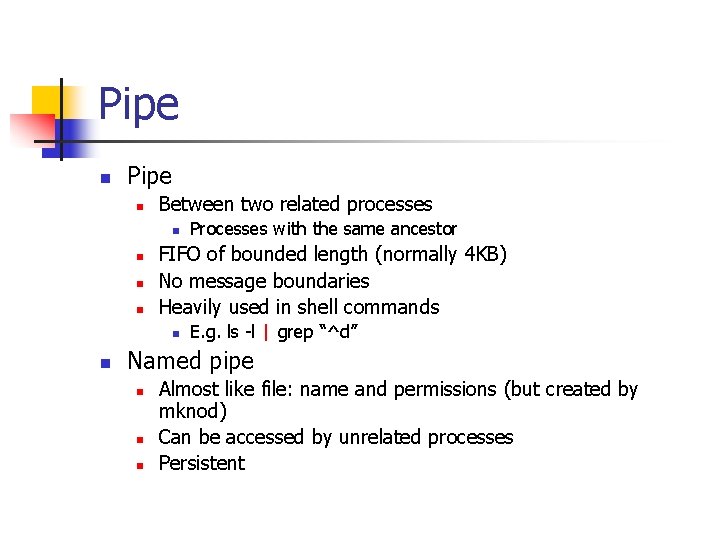
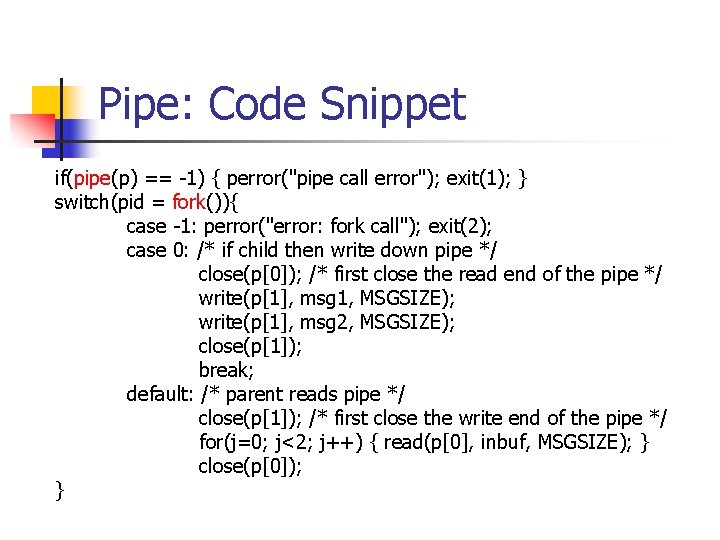
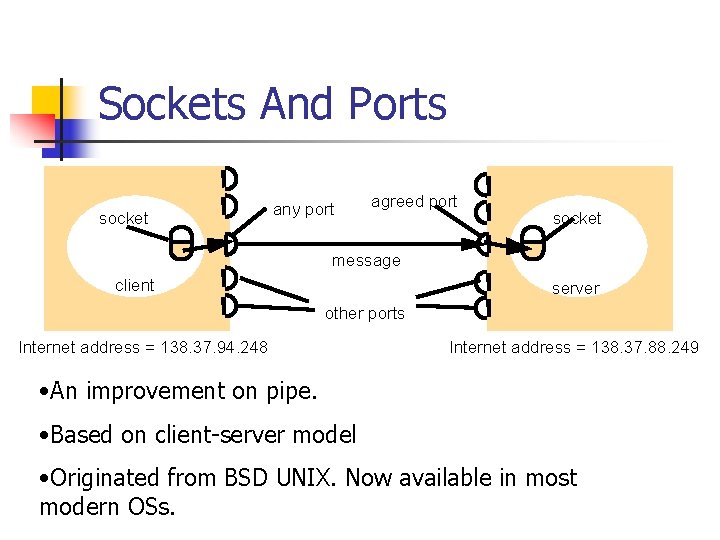
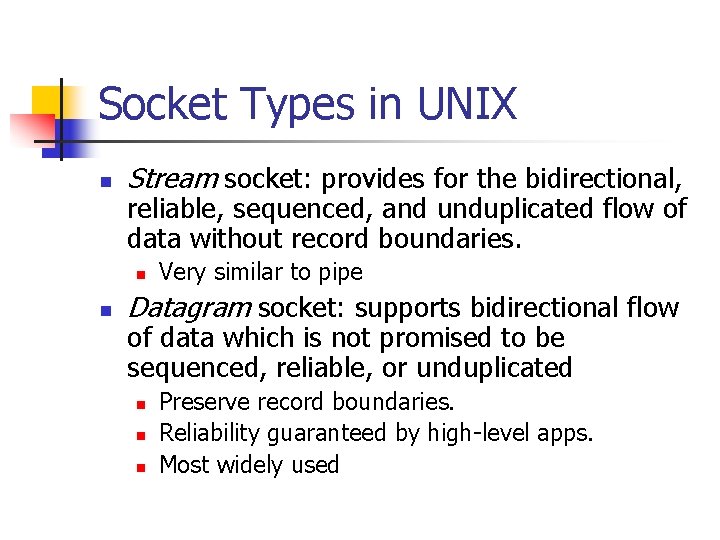
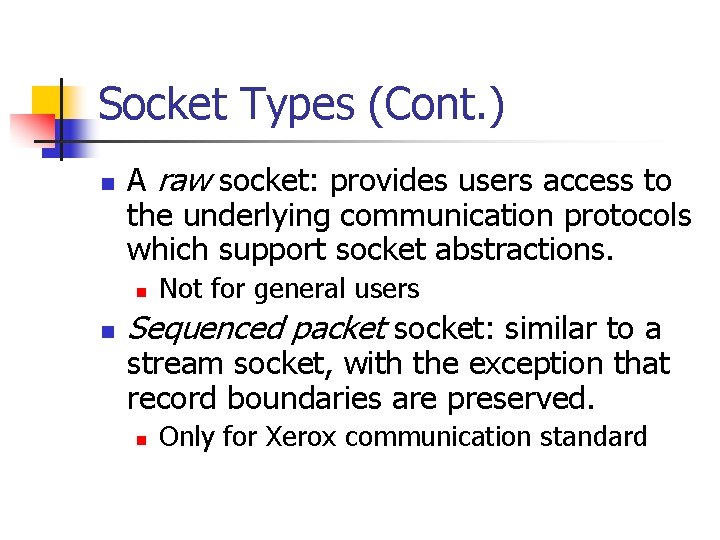
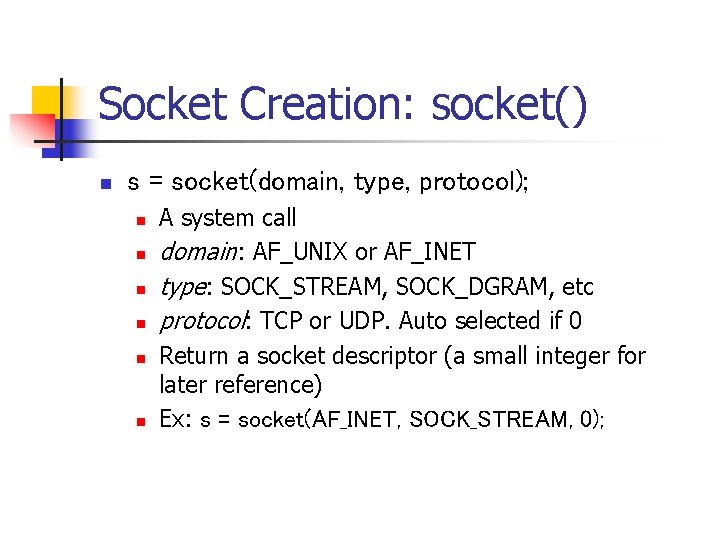
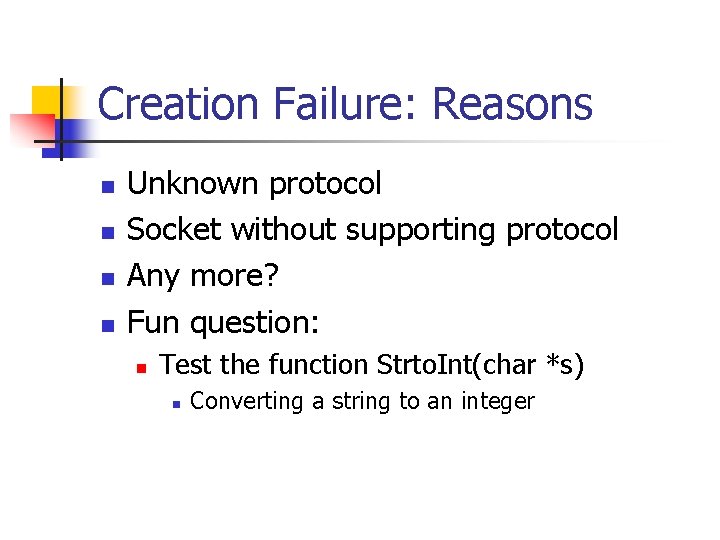
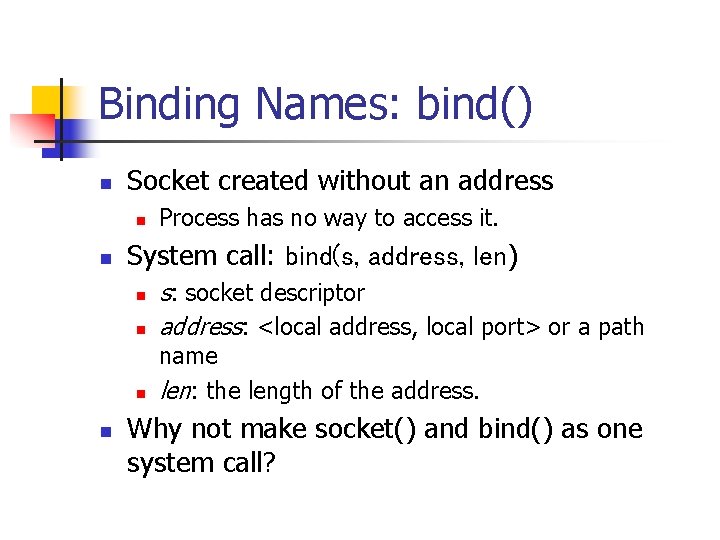
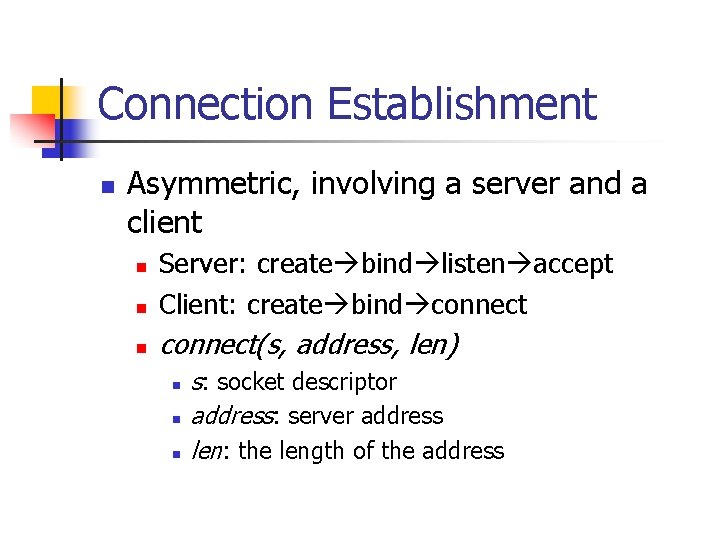
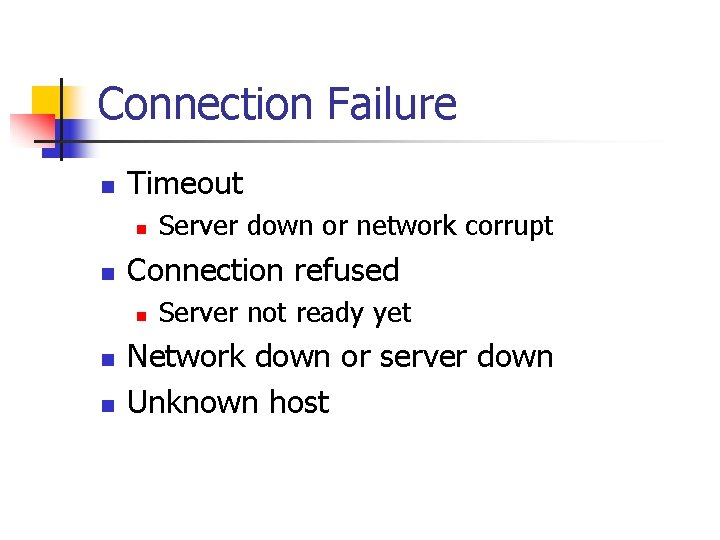
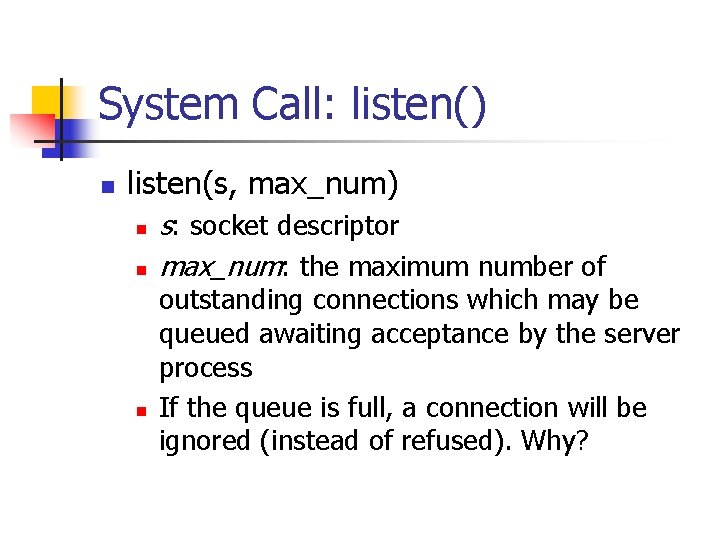
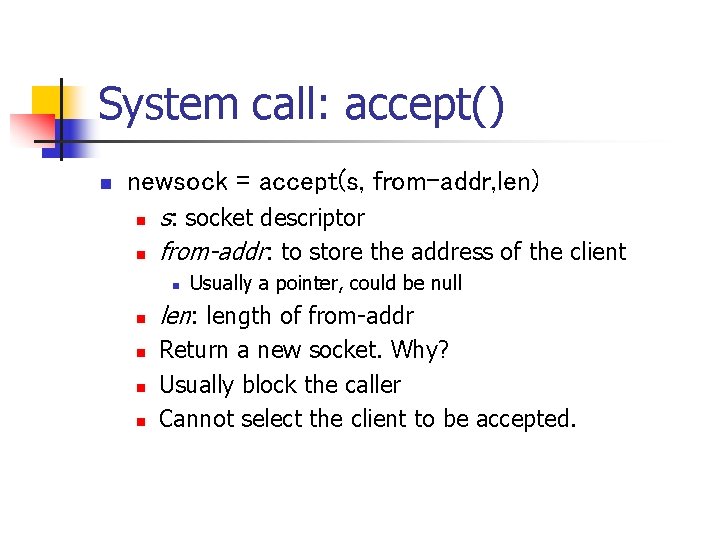
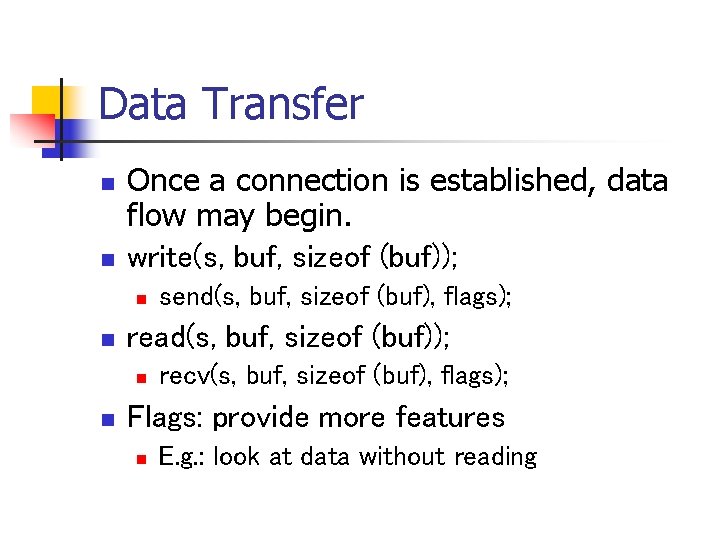
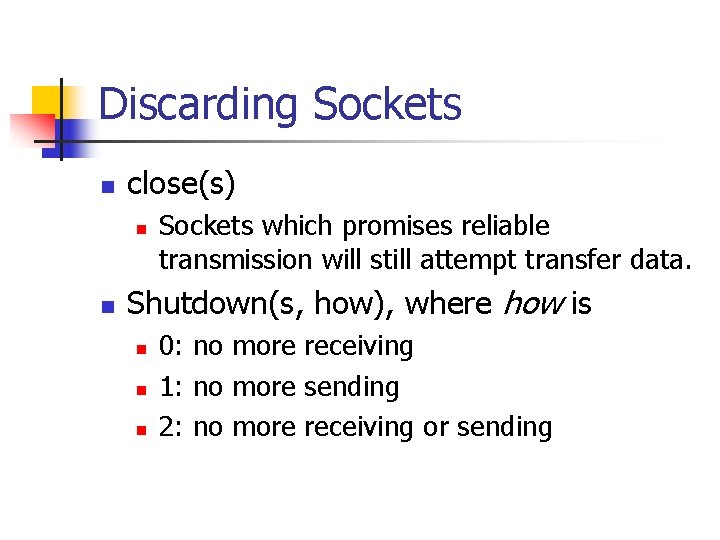
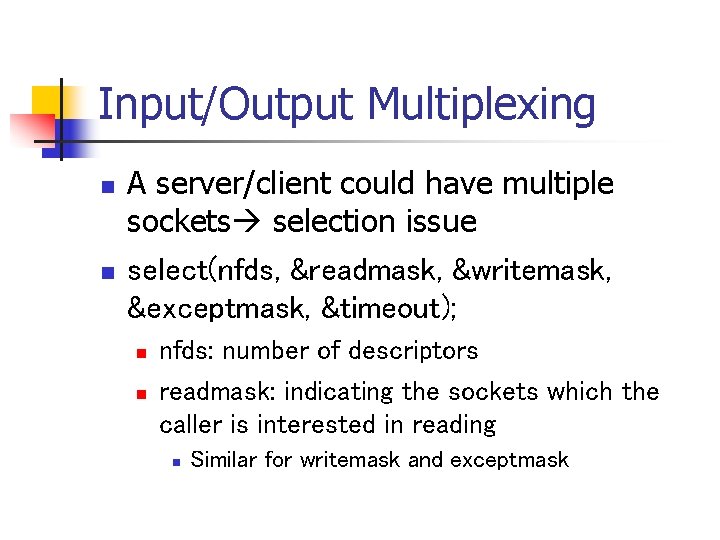
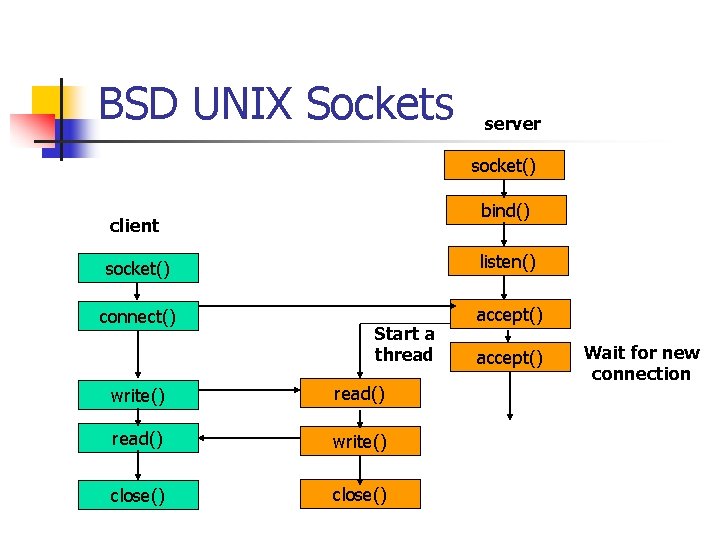
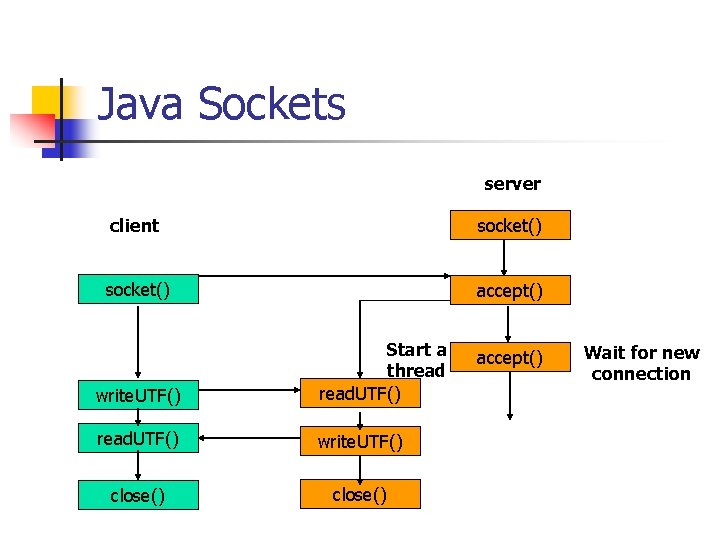
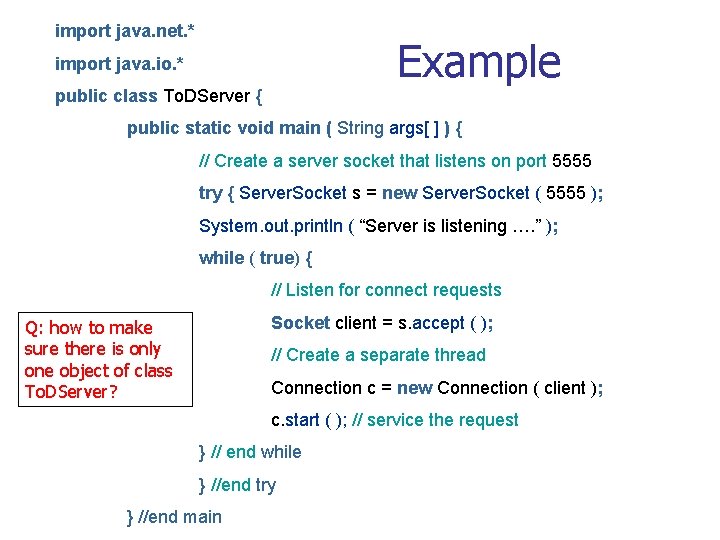
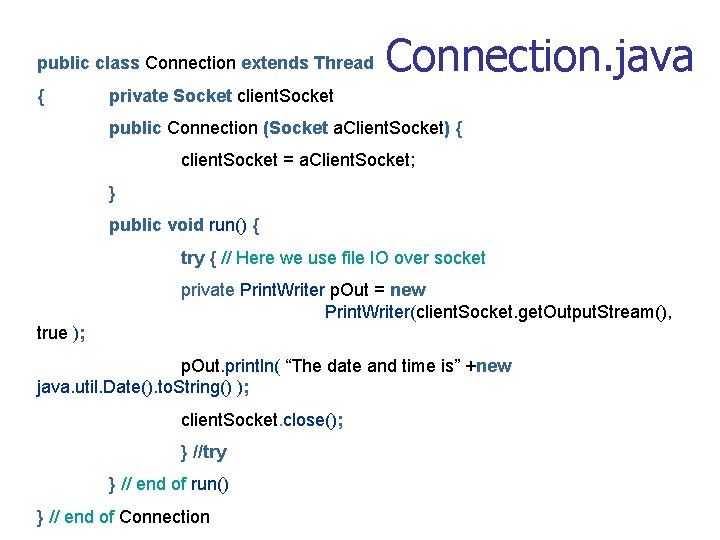
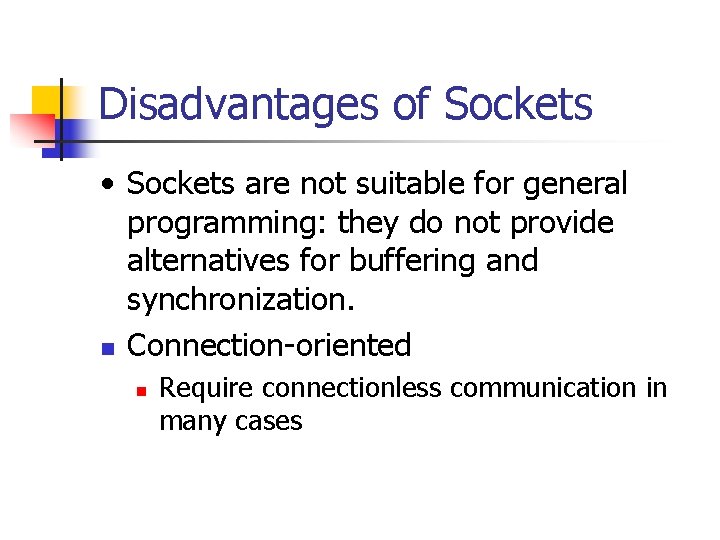
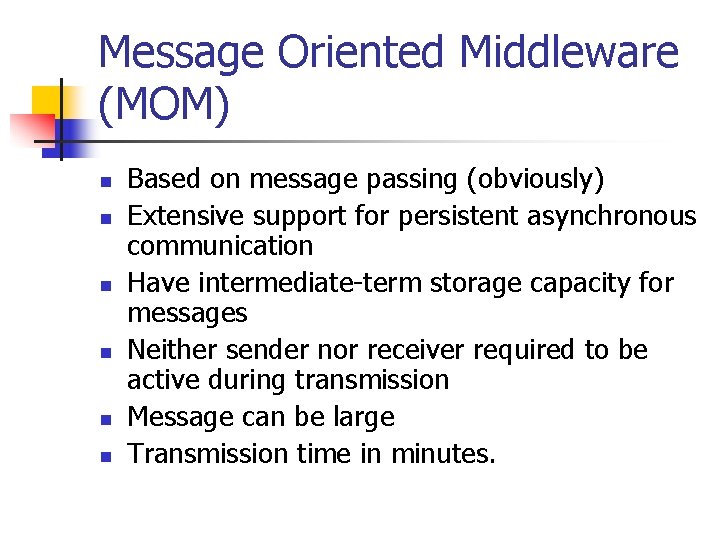
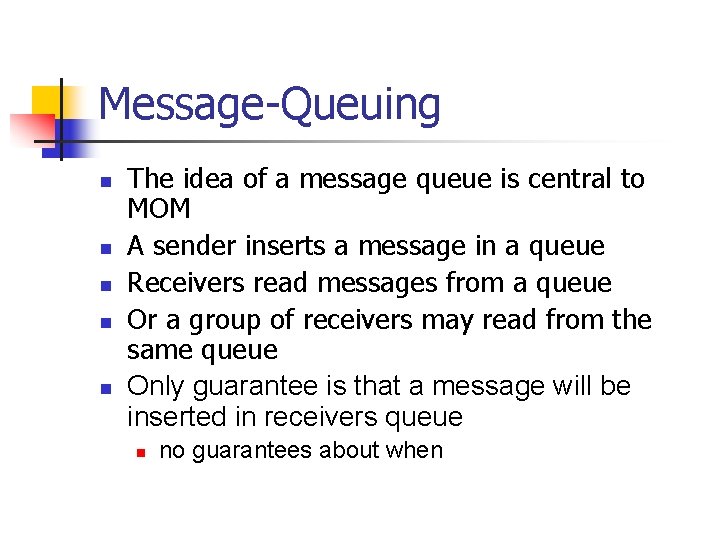
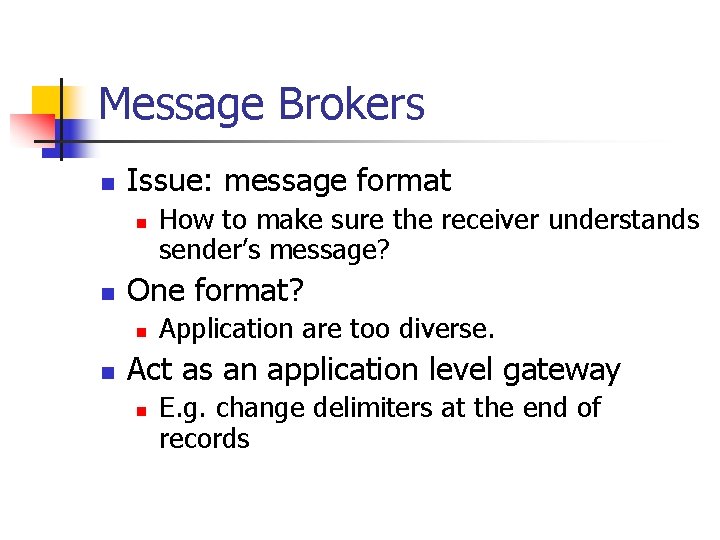
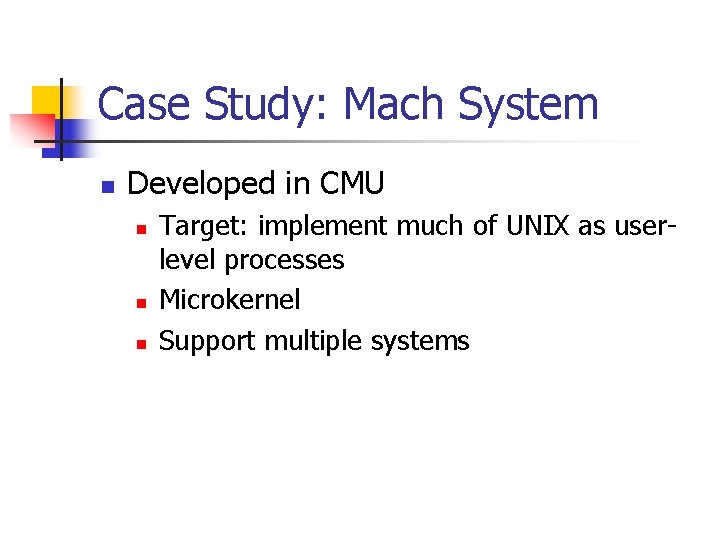
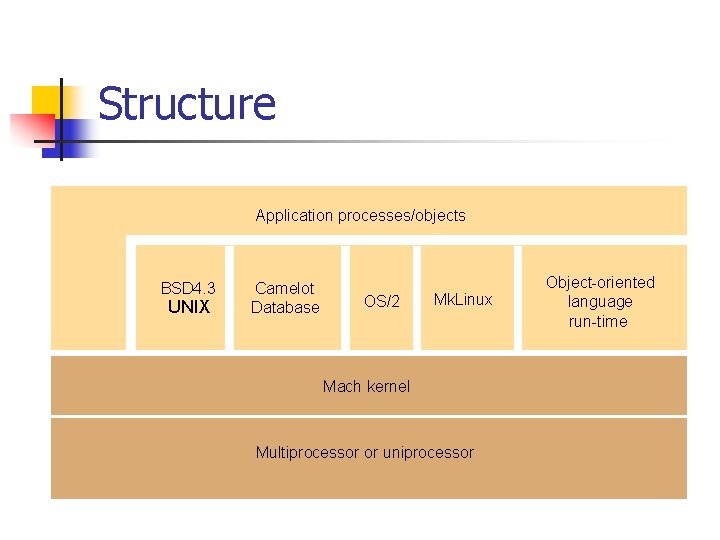
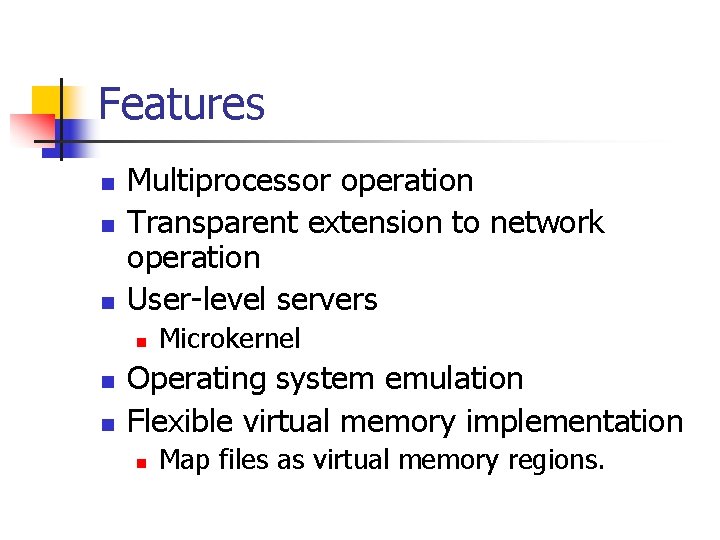
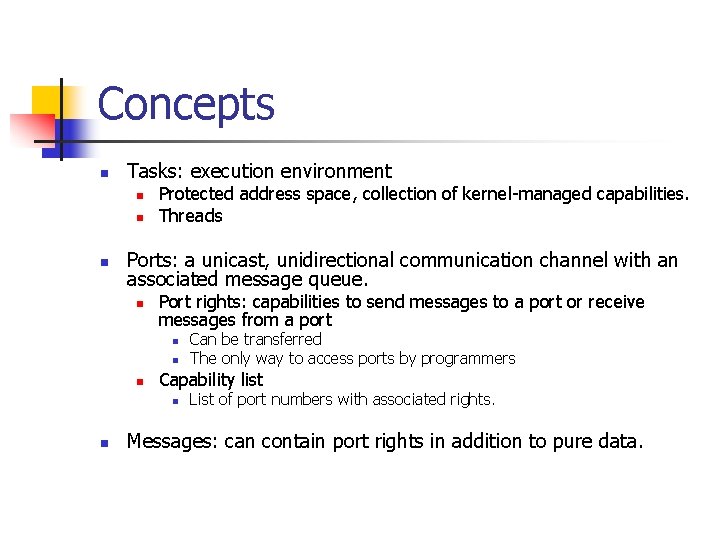
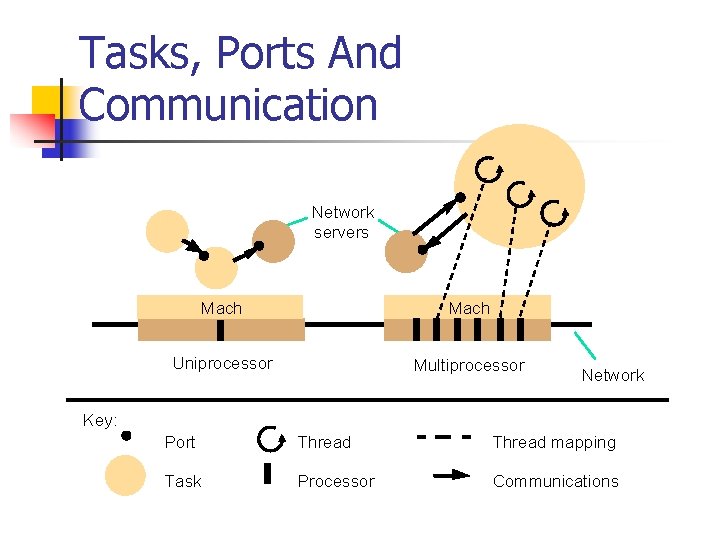
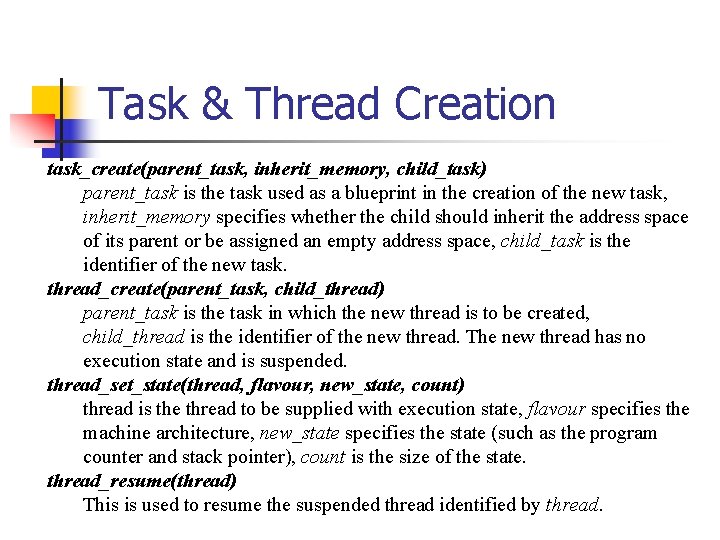
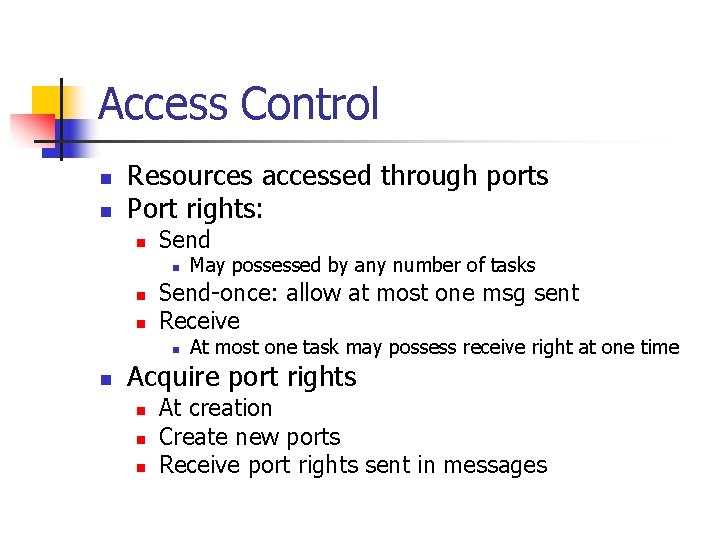
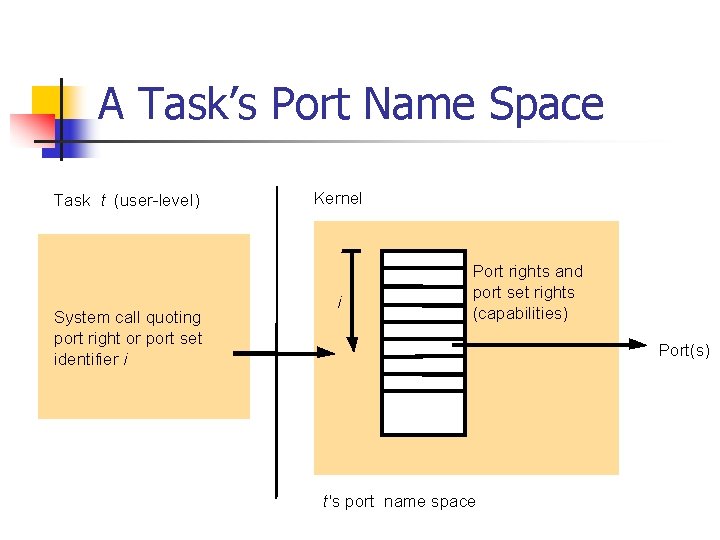
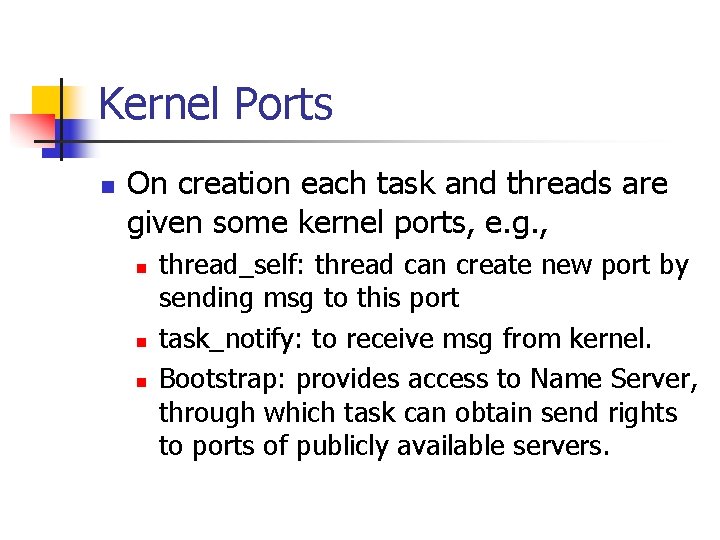
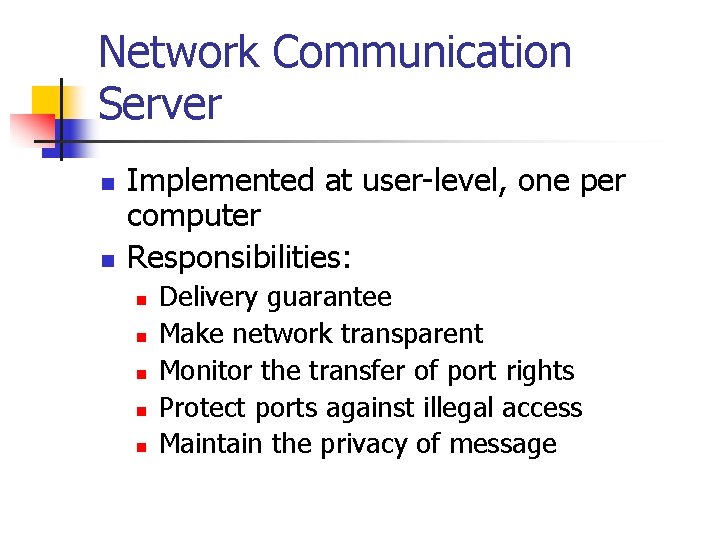
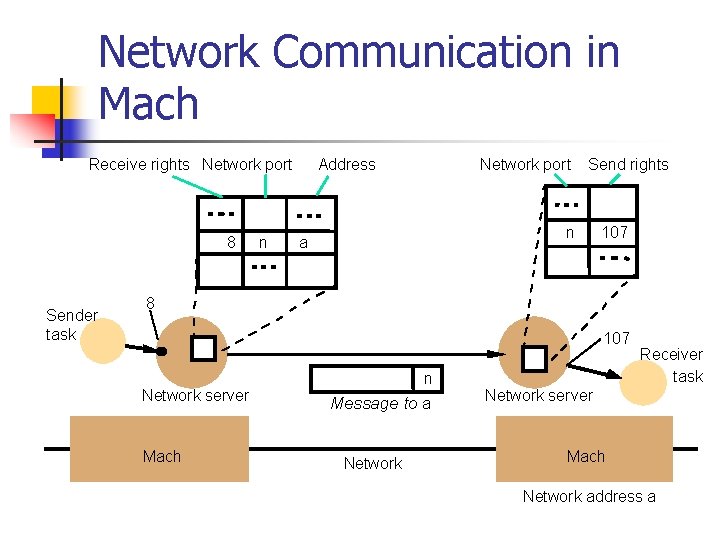
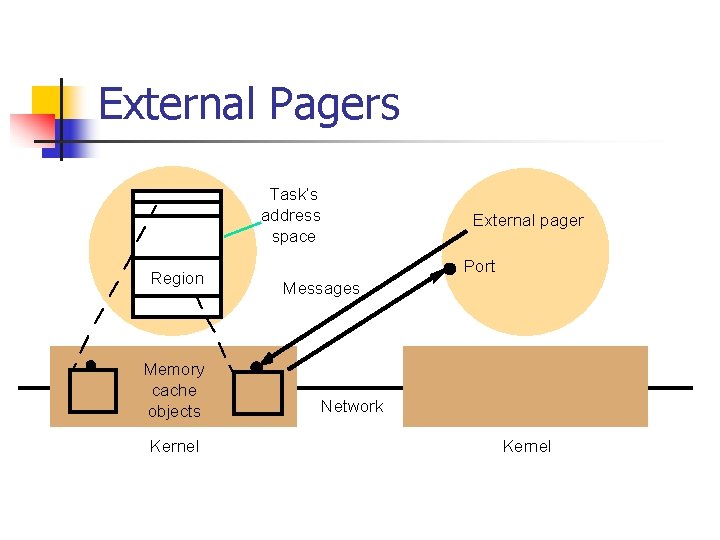
- Slides: 57
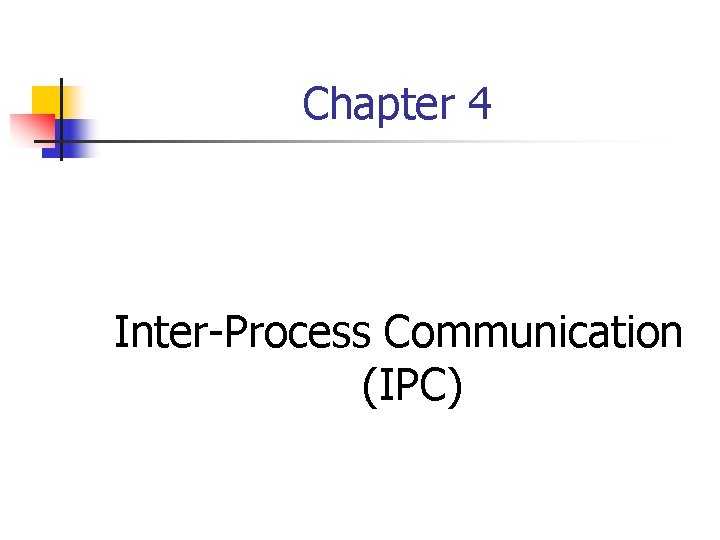
Chapter 4 Inter-Process Communication (IPC)
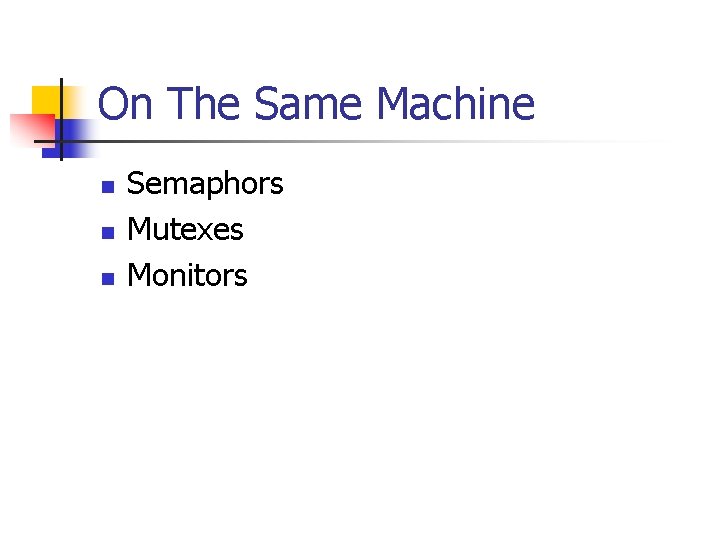
On The Same Machine n n n Semaphors Mutexes Monitors
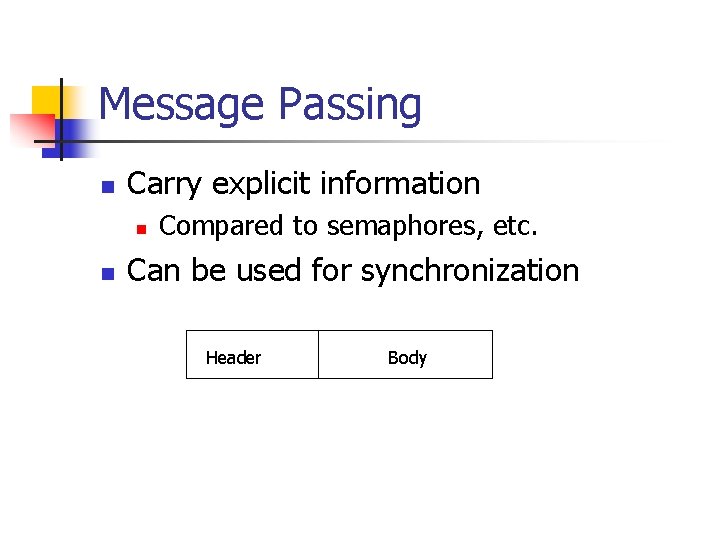
Message Passing n Carry explicit information n n Compared to semaphores, etc. Can be used for synchronization Header Body
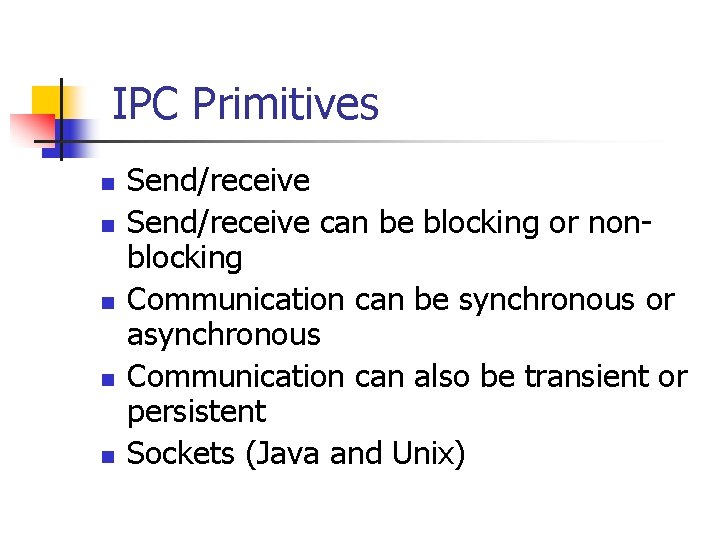
IPC Primitives n n n Send/receive can be blocking or nonblocking Communication can be synchronous or asynchronous Communication can also be transient or persistent Sockets (Java and Unix)
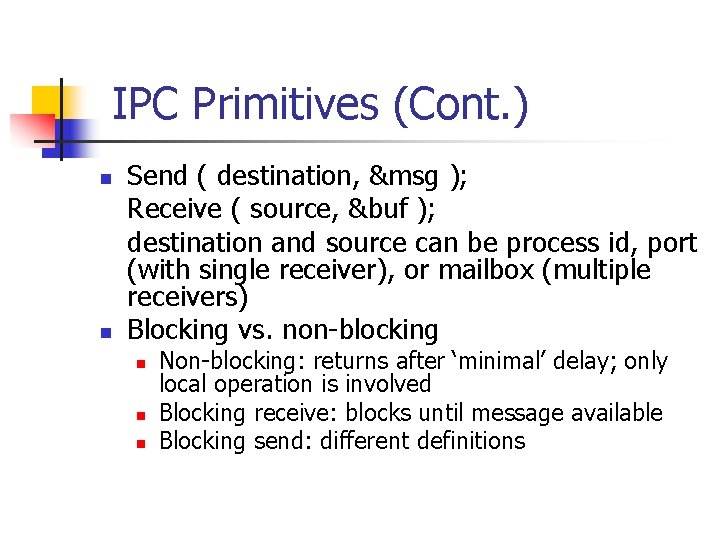
IPC Primitives (Cont. ) n n Send ( destination, &msg ); Receive ( source, &buf ); destination and source can be process id, port (with single receiver), or mailbox (multiple receivers) Blocking vs. non-blocking n n n Non-blocking: returns after ‘minimal’ delay; only local operation is involved Blocking receive: blocks until message available Blocking send: different definitions
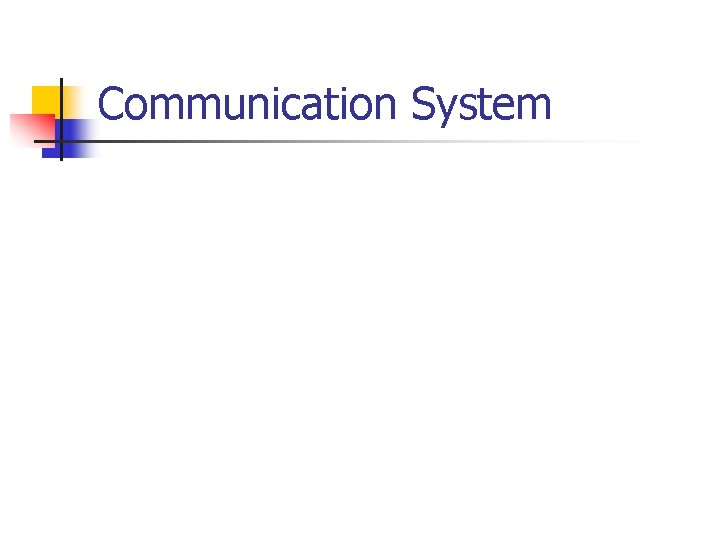
Communication System
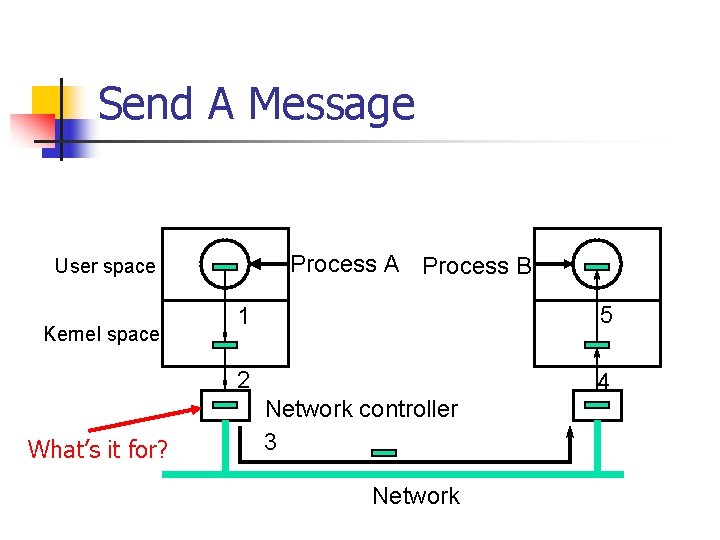
Send A Message Process A Process B User space Kernel space What’s it for? 1 5 2 4 Network controller 3 Network
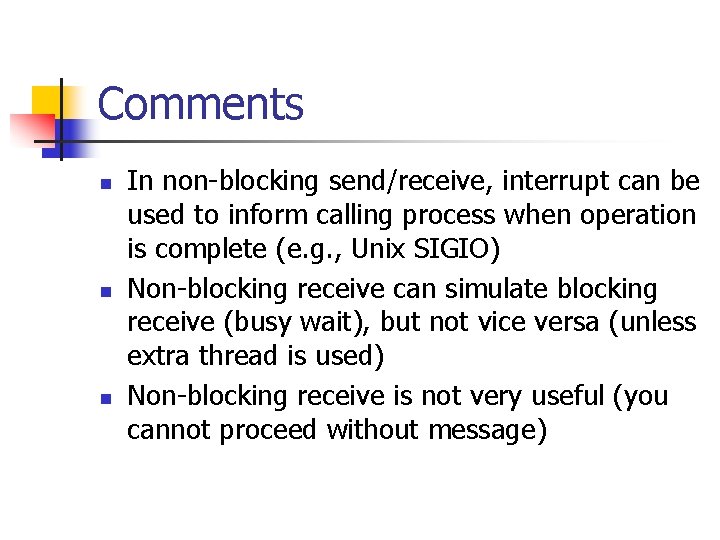
Comments n n n In non-blocking send/receive, interrupt can be used to inform calling process when operation is complete (e. g. , Unix SIGIO) Non-blocking receive can simulate blocking receive (busy wait), but not vice versa (unless extra thread is used) Non-blocking receive is not very useful (you cannot proceed without message)
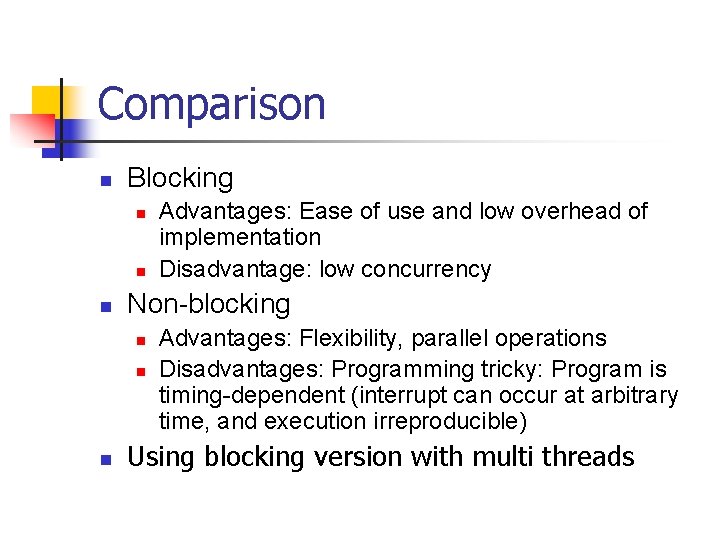
Comparison n Blocking n n n Non-blocking n n n Advantages: Ease of use and low overhead of implementation Disadvantage: low concurrency Advantages: Flexibility, parallel operations Disadvantages: Programming tricky: Program is timing-dependent (interrupt can occur at arbitrary time, and execution irreproducible) Using blocking version with multi threads
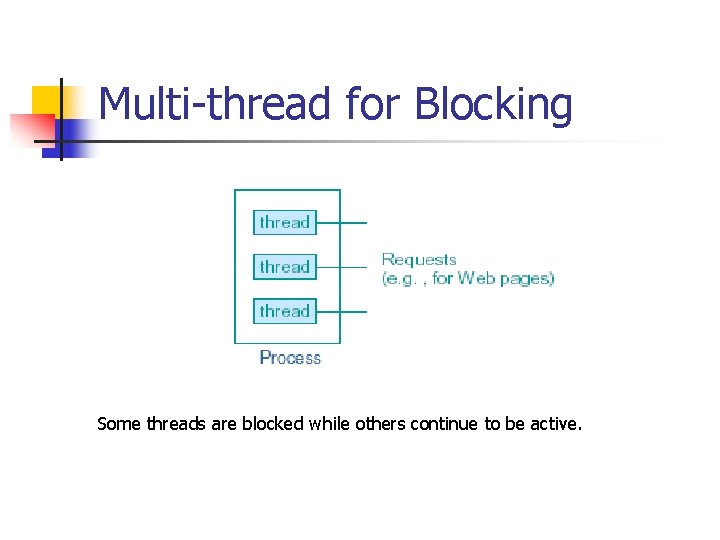
Multi-thread for Blocking Some threads are blocked while others continue to be active.
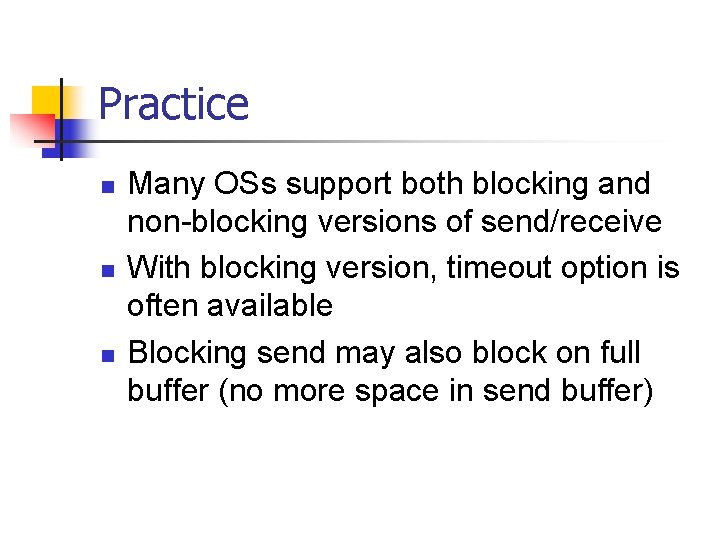
Practice n n n Many OSs support both blocking and non-blocking versions of send/receive With blocking version, timeout option is often available Blocking send may also block on full buffer (no more space in send buffer)
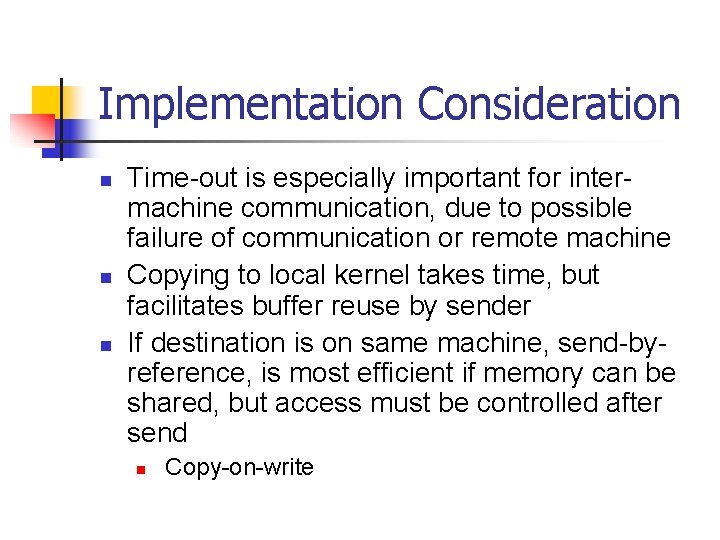
Implementation Consideration n Time-out is especially important for intermachine communication, due to possible failure of communication or remote machine Copying to local kernel takes time, but facilitates buffer reuse by sender If destination is on same machine, send-byreference, is most efficient if memory can be shared, but access must be controlled after send n Copy-on-write
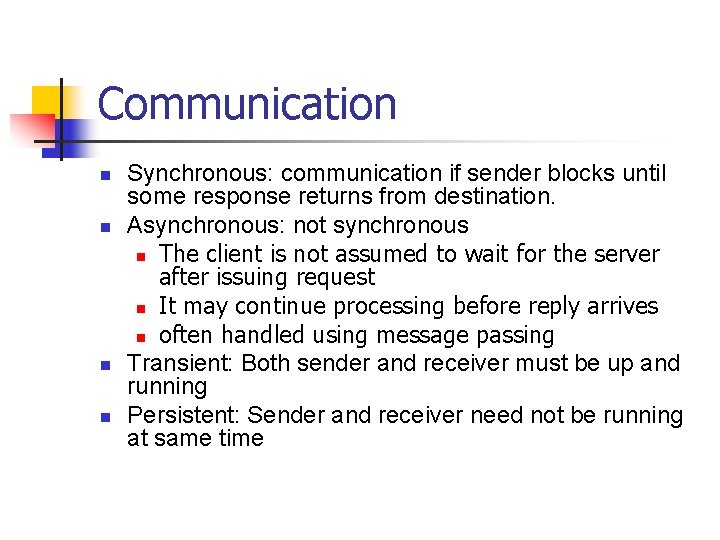
Communication n n Synchronous: communication if sender blocks until some response returns from destination. Asynchronous: not synchronous n The client is not assumed to wait for the server after issuing request n It may continue processing before reply arrives n often handled using message passing Transient: Both sender and receiver must be up and running Persistent: Sender and receiver need not be running at same time
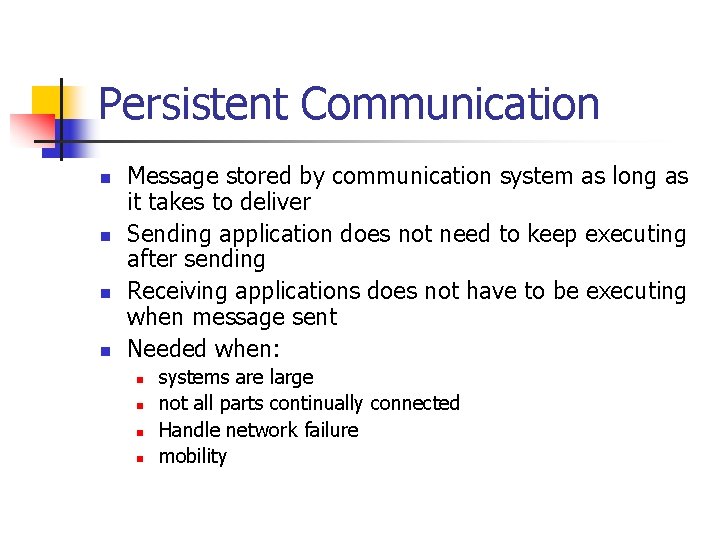
Persistent Communication n n Message stored by communication system as long as it takes to deliver Sending application does not need to keep executing after sending Receiving applications does not have to be executing when message sent Needed when: n n systems are large not all parts continually connected Handle network failure mobility
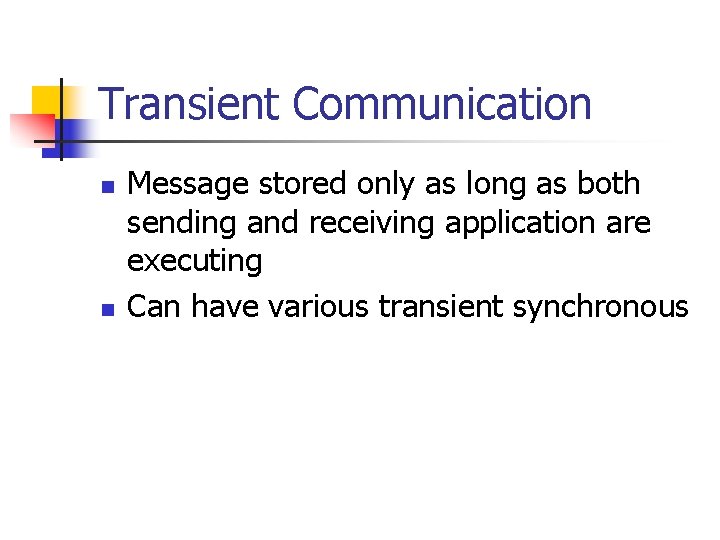
Transient Communication n n Message stored only as long as both sending and receiving application are executing Can have various transient synchronous
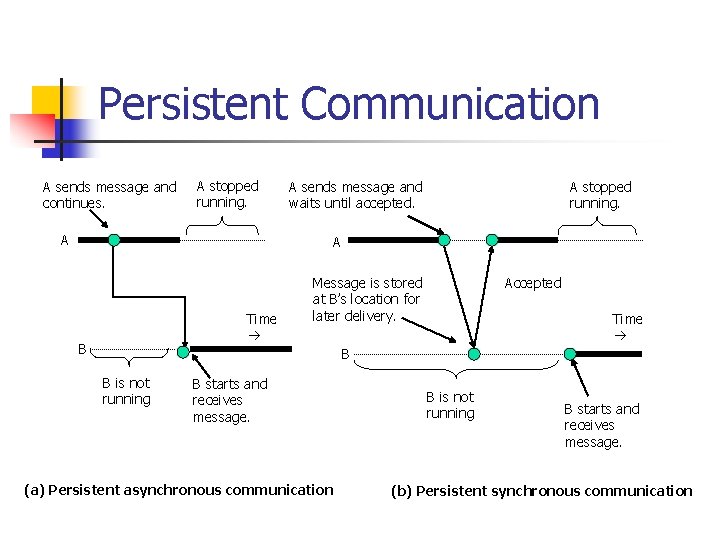
Persistent Communication A sends message and continues. A stopped running. A A sends message and waits until accepted. A stopped running. A Time B Message is stored at B’s location for later delivery. Accepted Time B B is not running B starts and receives message. (a) Persistent asynchronous communication B is not running B starts and receives message. (b) Persistent synchronous communication
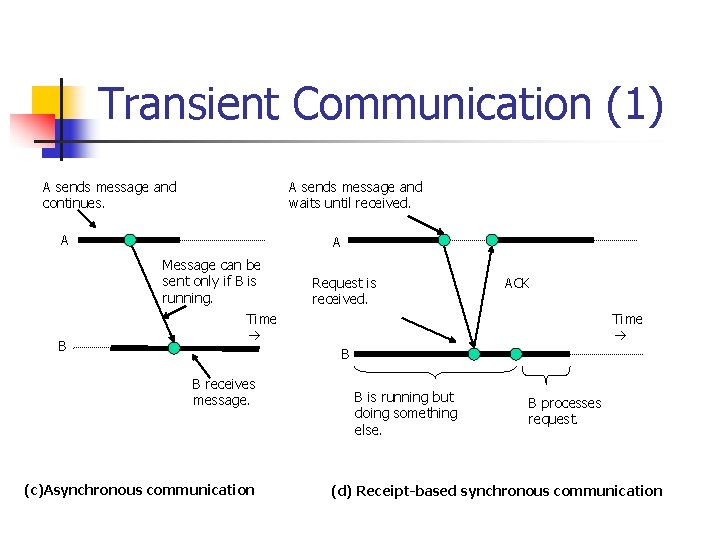
Transient Communication (1) A sends message and continues. A sends message and waits until received. A B A Message can be sent only if B is running. Time Request is received. ACK Time B B receives message. (c)Asynchronous communication B is running but doing something else. B processes request. (d) Receipt-based synchronous communication
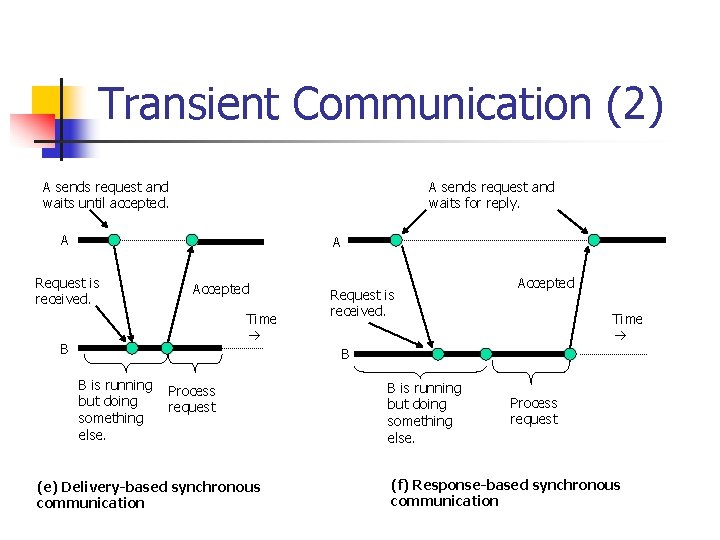
Transient Communication (2) A sends request and waits until accepted. A sends request and waits for reply. A A Request is received. Accepted Time B B is running but doing something else. Process request (e) Delivery-based synchronous communication B is running but doing something else. Process request (f) Response-based synchronous communication
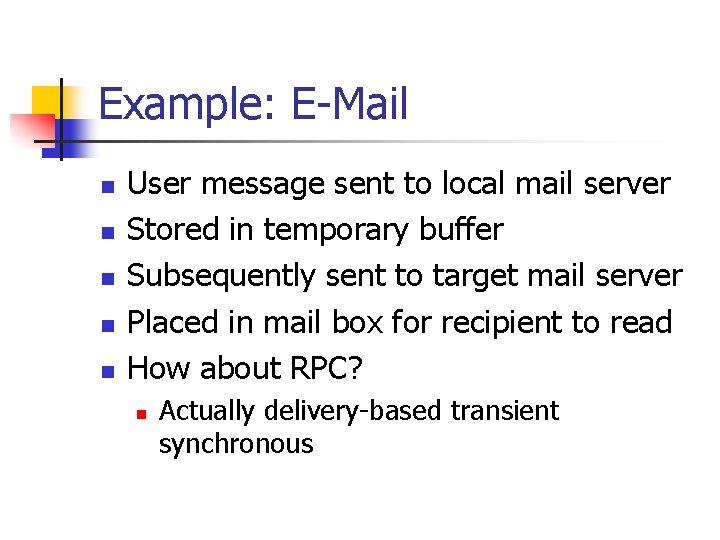
Example: E-Mail n n n User message sent to local mail server Stored in temporary buffer Subsequently sent to target mail server Placed in mail box for recipient to read How about RPC? n Actually delivery-based transient synchronous
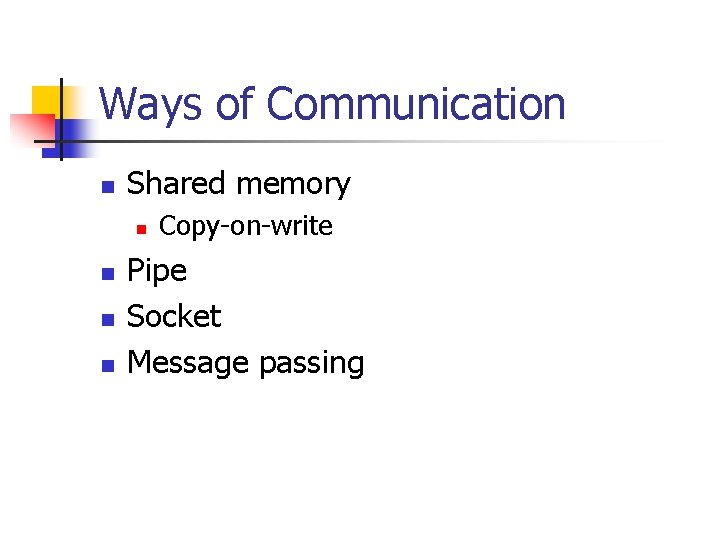
Ways of Communication n Shared memory n n Copy-on-write Pipe Socket Message passing
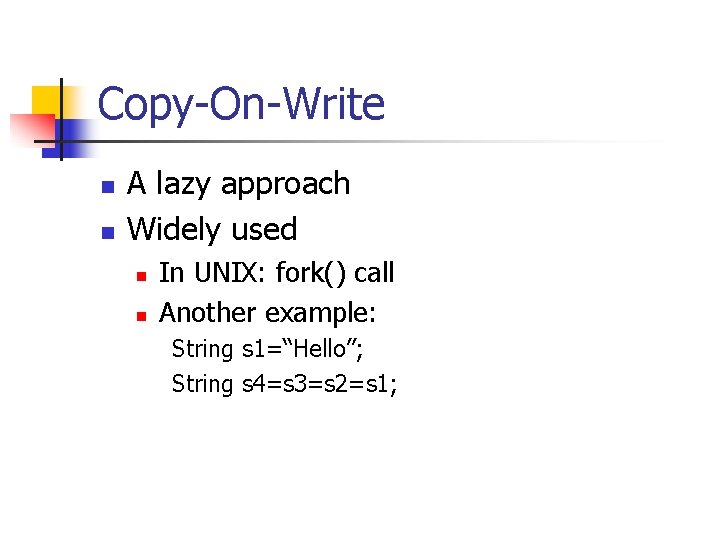
Copy-On-Write n n A lazy approach Widely used n n In UNIX: fork() call Another example: String s 1=“Hello”; String s 4=s 3=s 2=s 1;
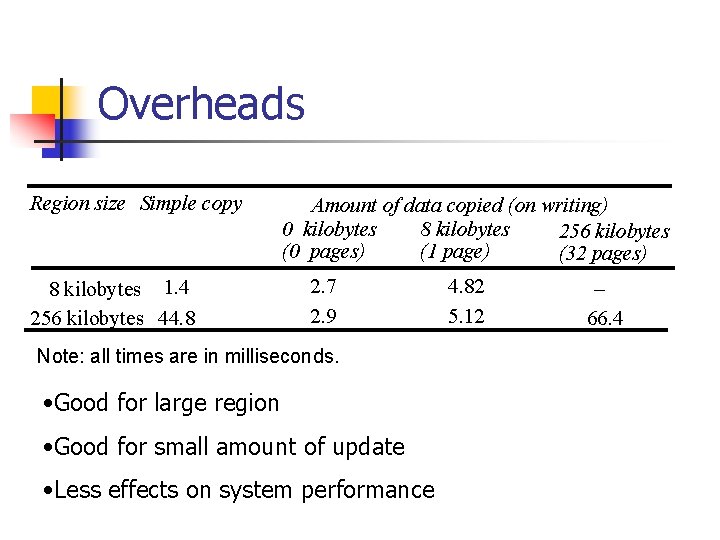
Overheads Region size Simple copy 8 kilobytes 1. 4 256 kilobytes 44. 8 Amount of data copied (on writing) 0 kilobytes 8 kilobytes 256 kilobytes (0 pages) (1 page) (32 pages) _ 2. 7 4. 82 2. 9 Note: all times are in milliseconds. • Good for large region • Good for small amount of update • Less effects on system performance 5. 12 66. 4
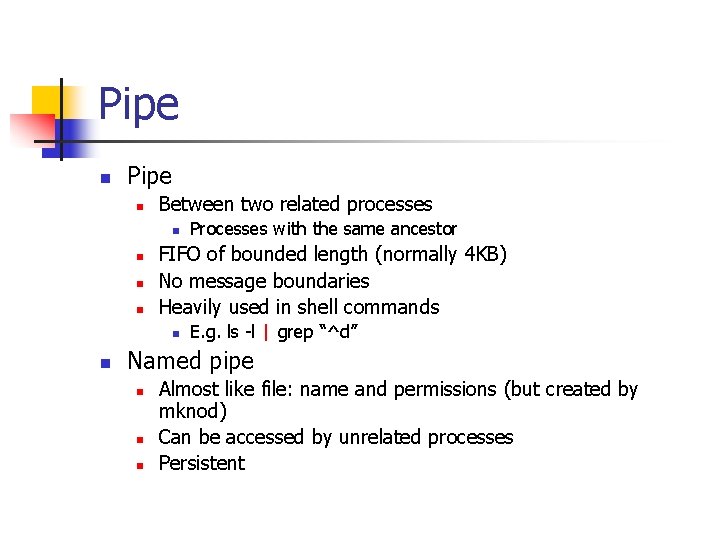
Pipe n Between two related processes n n FIFO of bounded length (normally 4 KB) No message boundaries Heavily used in shell commands n n Processes with the same ancestor E. g. ls -l | grep “^d” Named pipe n n n Almost like file: name and permissions (but created by mknod) Can be accessed by unrelated processes Persistent
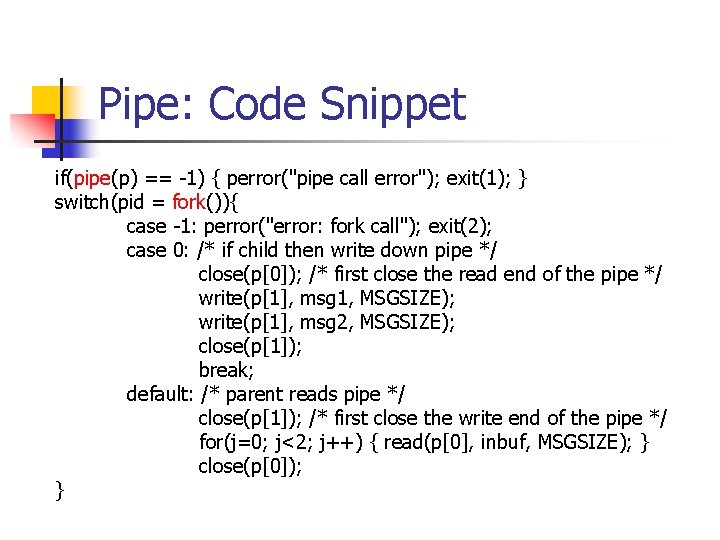
Pipe: Code Snippet if(pipe(p) == -1) { perror("pipe call error"); exit(1); } switch(pid = fork()){ case -1: perror("error: fork call"); exit(2); case 0: /* if child then write down pipe */ close(p[0]); /* first close the read end of the pipe */ write(p[1], msg 1, MSGSIZE); write(p[1], msg 2, MSGSIZE); close(p[1]); break; default: /* parent reads pipe */ close(p[1]); /* first close the write end of the pipe */ for(j=0; j<2; j++) { read(p[0], inbuf, MSGSIZE); } close(p[0]); }
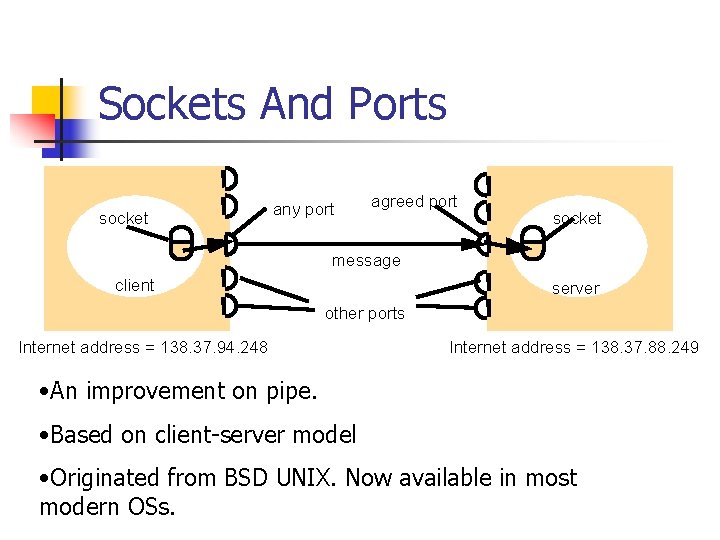
Sockets And Ports socket any port agreed port socket message client server other ports Internet address = 138. 37. 94. 248 Internet address = 138. 37. 88. 249 • An improvement on pipe. • Based on client-server model • Originated from BSD UNIX. Now available in most modern OSs.
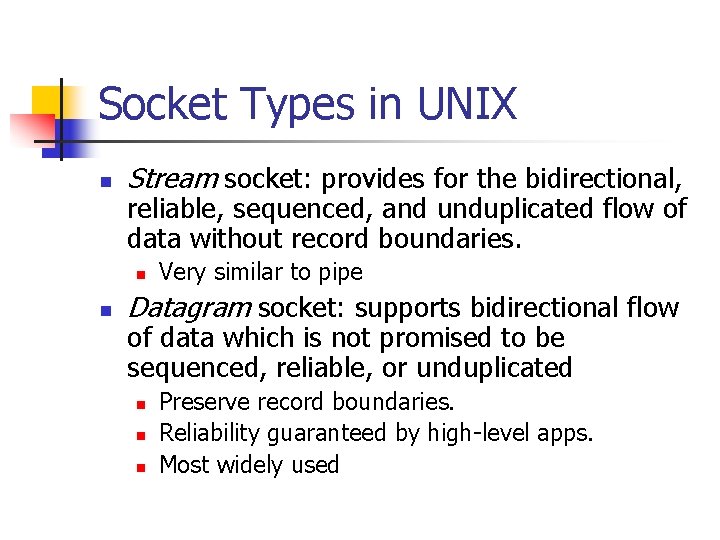
Socket Types in UNIX n Stream socket: provides for the bidirectional, reliable, sequenced, and unduplicated flow of data without record boundaries. n n Very similar to pipe Datagram socket: supports bidirectional flow of data which is not promised to be sequenced, reliable, or unduplicated n n n Preserve record boundaries. Reliability guaranteed by high-level apps. Most widely used
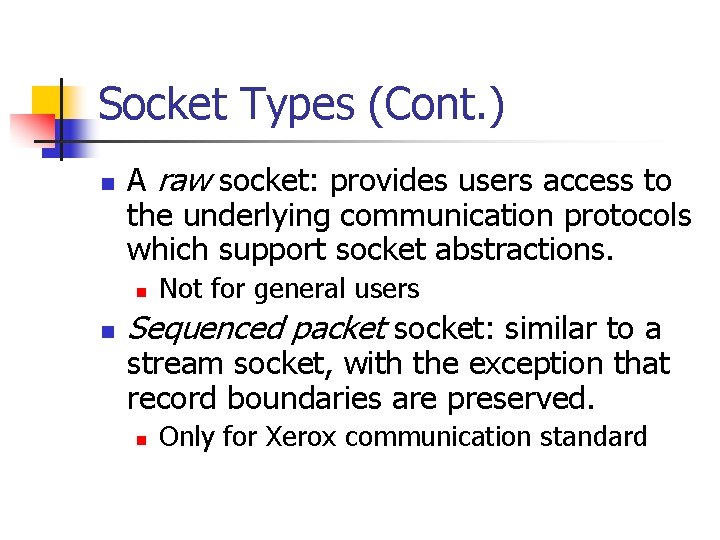
Socket Types (Cont. ) n A raw socket: provides users access to the underlying communication protocols which support socket abstractions. n n Not for general users Sequenced packet socket: similar to a stream socket, with the exception that record boundaries are preserved. n Only for Xerox communication standard
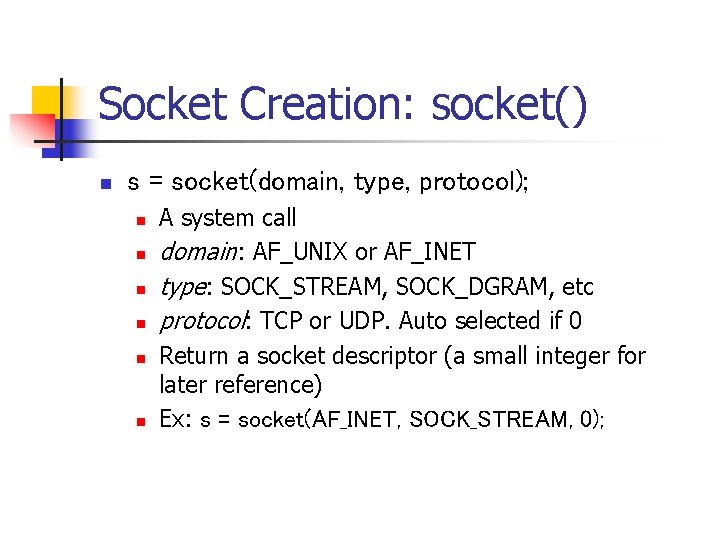
Socket Creation: socket() n s = socket(domain, type, protocol); n n n A system call domain: AF_UNIX or AF_INET type: SOCK_STREAM, SOCK_DGRAM, etc protocol: TCP or UDP. Auto selected if 0 Return a socket descriptor (a small integer for later reference) Ex: s = socket(AF_INET, SOCK_STREAM, 0);
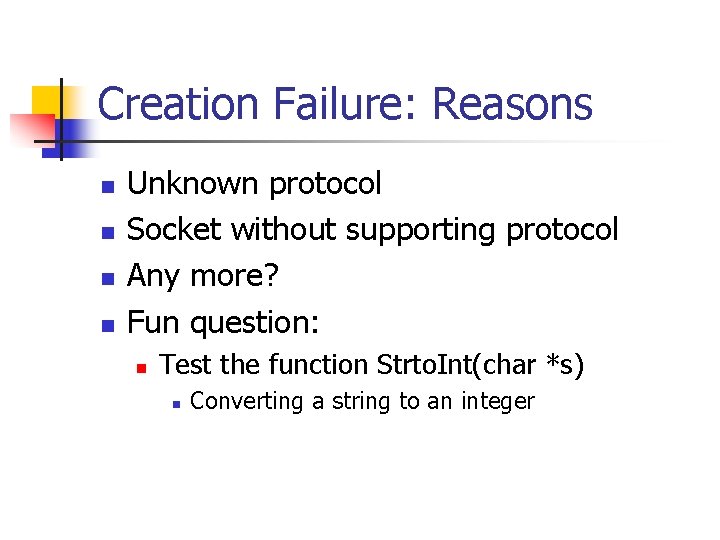
Creation Failure: Reasons n n Unknown protocol Socket without supporting protocol Any more? Fun question: n Test the function Strto. Int(char *s) n Converting a string to an integer
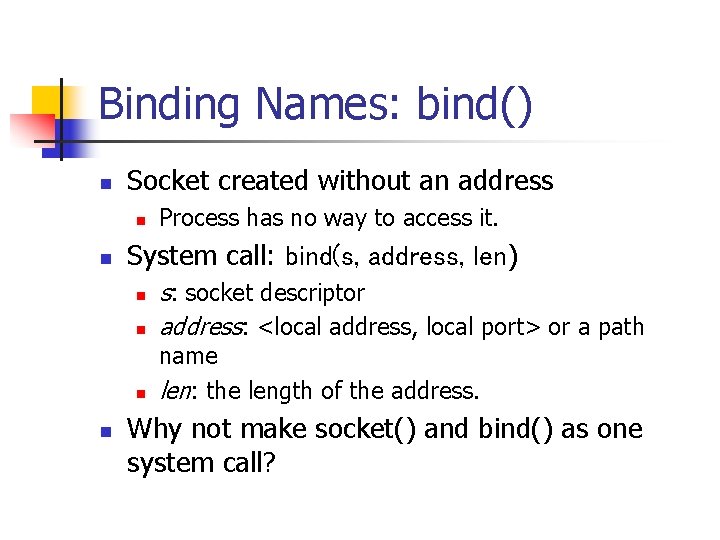
Binding Names: bind() n Socket created without an address n n System call: bind(s, address, len) n n Process has no way to access it. s: socket descriptor address: <local address, local port> or a path name len: the length of the address. Why not make socket() and bind() as one system call?
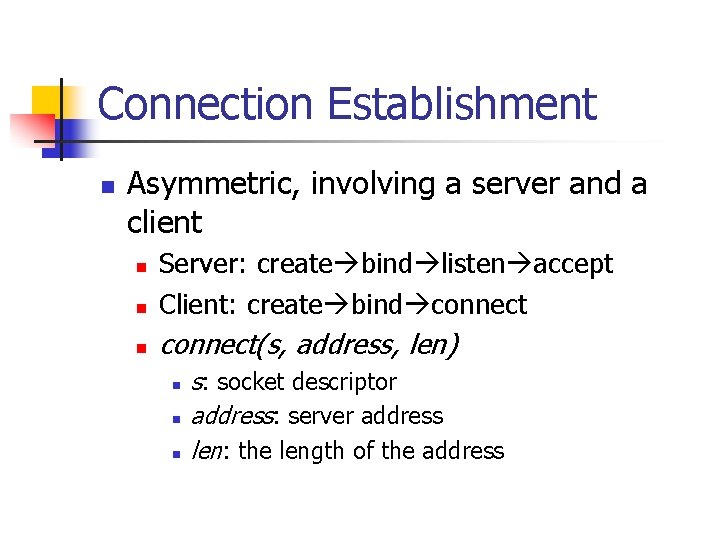
Connection Establishment n Asymmetric, involving a server and a client n Server: create bind listen accept Client: create bind connect n connect(s, address, len) n n s: socket descriptor address: server address len: the length of the address
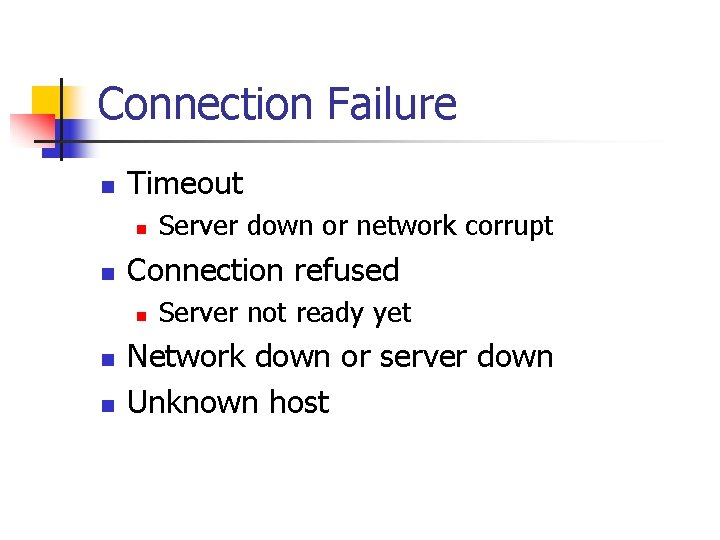
Connection Failure n Timeout n n Connection refused n n n Server down or network corrupt Server not ready yet Network down or server down Unknown host
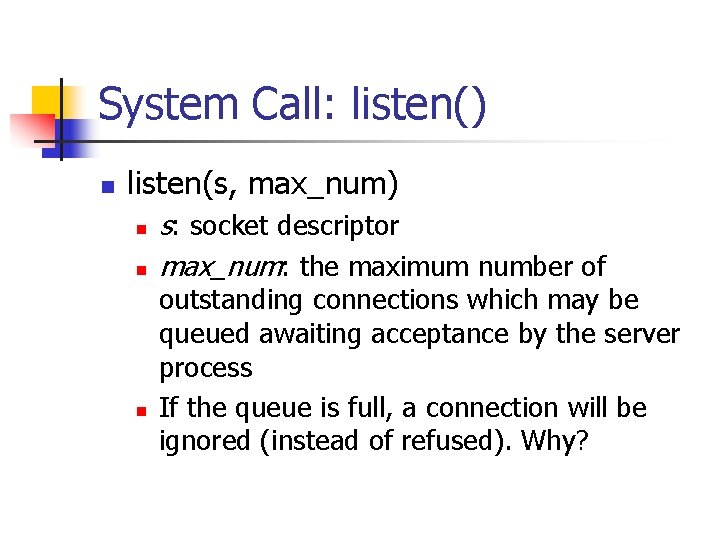
System Call: listen() n listen(s, max_num) n n n s: socket descriptor max_num: the maximum number of outstanding connections which may be queued awaiting acceptance by the server process If the queue is full, a connection will be ignored (instead of refused). Why?
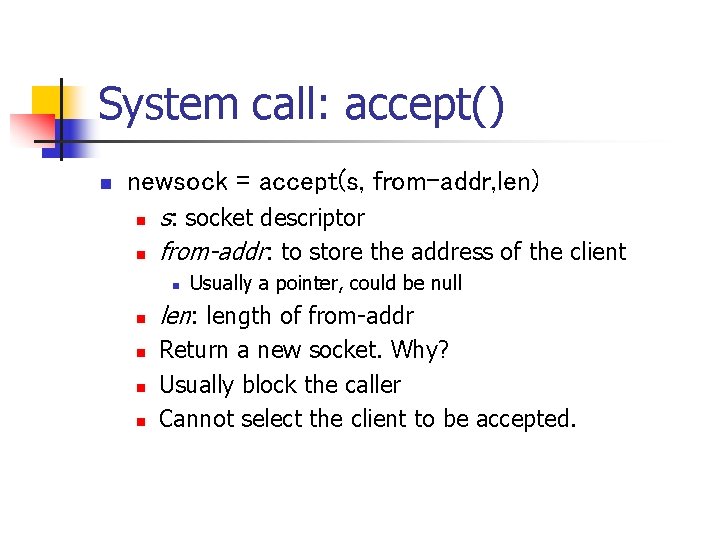
System call: accept() n newsock = accept(s, from-addr, len) n n s: socket descriptor from-addr: to store the address of the client n n n Usually a pointer, could be null len: length of from-addr Return a new socket. Why? Usually block the caller Cannot select the client to be accepted.
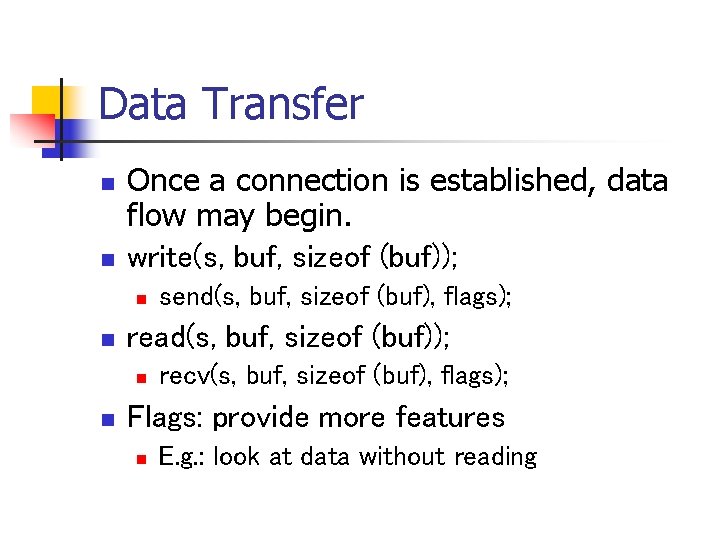
Data Transfer n n Once a connection is established, data flow may begin. write(s, buf, sizeof (buf)); n n read(s, buf, sizeof (buf)); n n send(s, buf, sizeof (buf), flags); recv(s, buf, sizeof (buf), flags); Flags: provide more features n E. g. : look at data without reading
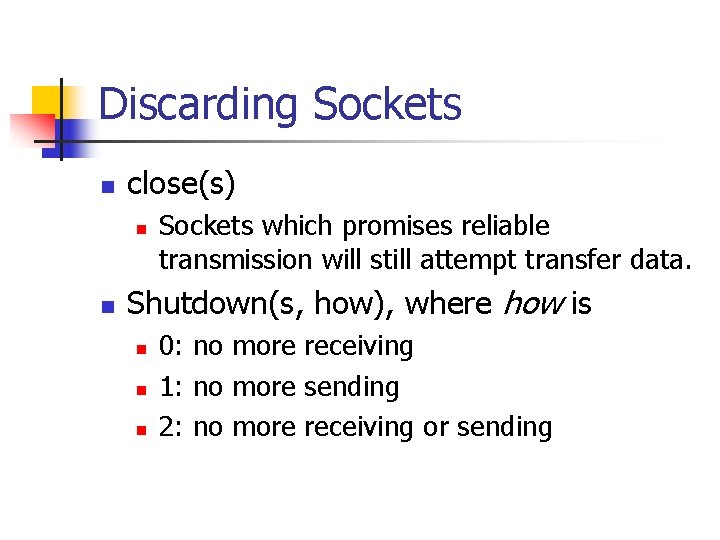
Discarding Sockets n close(s) n n Sockets which promises reliable transmission will still attempt transfer data. Shutdown(s, how), where how is n n n 0: no more receiving 1: no more sending 2: no more receiving or sending
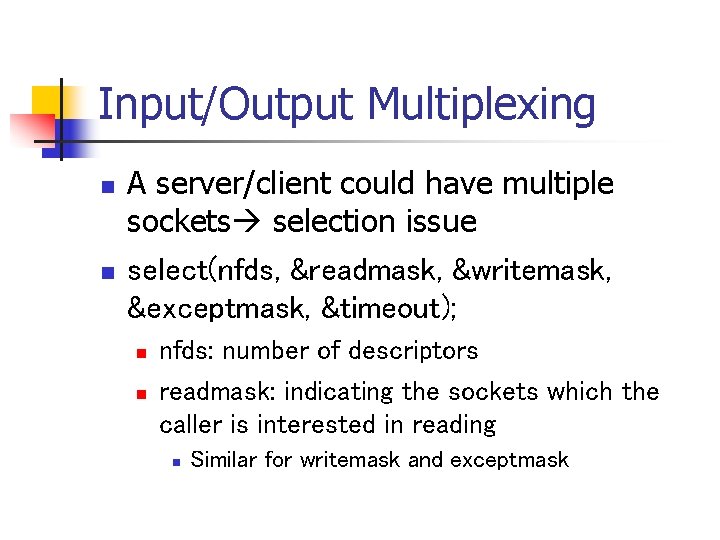
Input/Output Multiplexing n n A server/client could have multiple sockets selection issue select(nfds, &readmask, &writemask, &exceptmask, &timeout); n n nfds: number of descriptors readmask: indicating the sockets which the caller is interested in reading n Similar for writemask and exceptmask
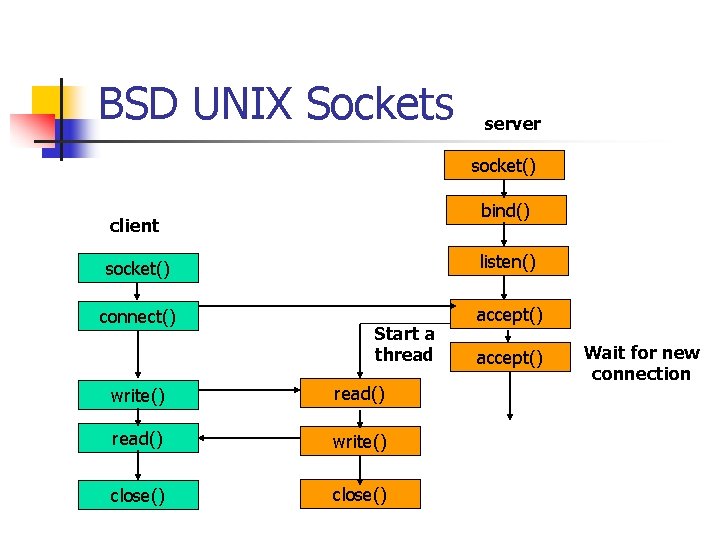
BSD UNIX Sockets server socket() bind() client socket() listen() connect() accept() Start a thread write() read() write() close() accept() Wait for new connection
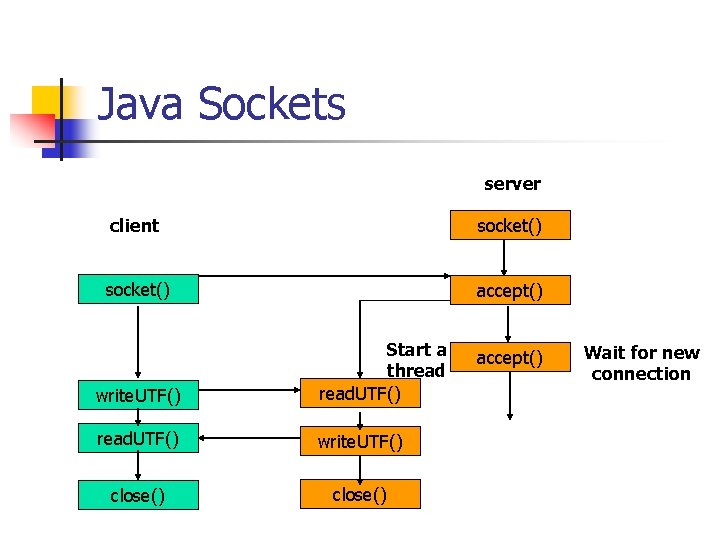
Java Sockets server client socket() accept() write. UTF() Start a thread. UTF() write. UTF() close() accept() Wait for new connection
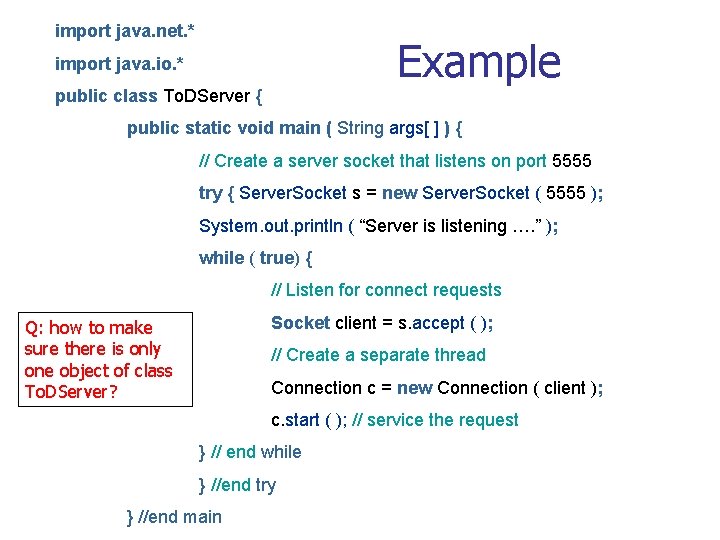
import java. net. * Example import java. io. * public class To. DServer { public static void main ( String args[ ] ) { // Create a server socket that listens on port 5555 try { Server. Socket s = new Server. Socket ( 5555 ); System. out. println ( “Server is listening …. ” ); while ( true) { // Listen for connect requests Socket client = s. accept ( ); Q: how to make sure there is only one object of class To. DServer? // Create a separate thread Connection c = new Connection ( client ); c. start ( ); // service the request } // end while } //end try } //end main
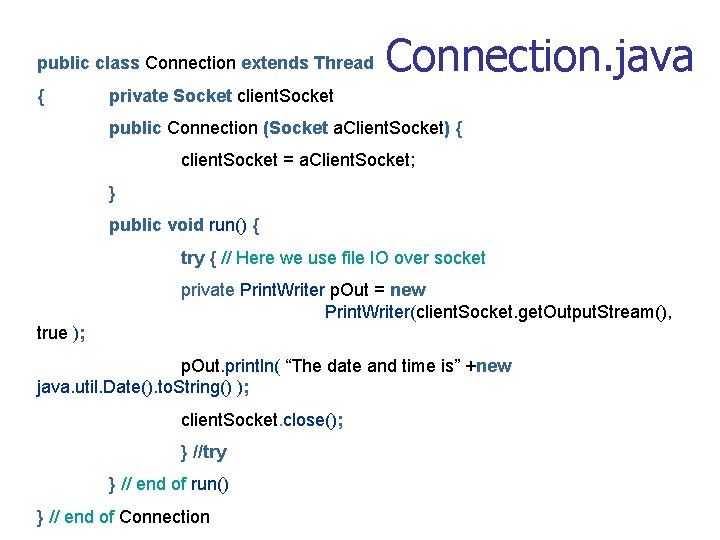
public class Connection extends Thread { Connection. java private Socket client. Socket public Connection (Socket a. Client. Socket) { client. Socket = a. Client. Socket; } public void run() { try { // Here we use file IO over socket private Print. Writer p. Out = new Print. Writer(client. Socket. get. Output. Stream(), true ); p. Out. println( “The date and time is” +new java. util. Date(). to. String() ); client. Socket. close(); } //try } // end of run() } // end of Connection
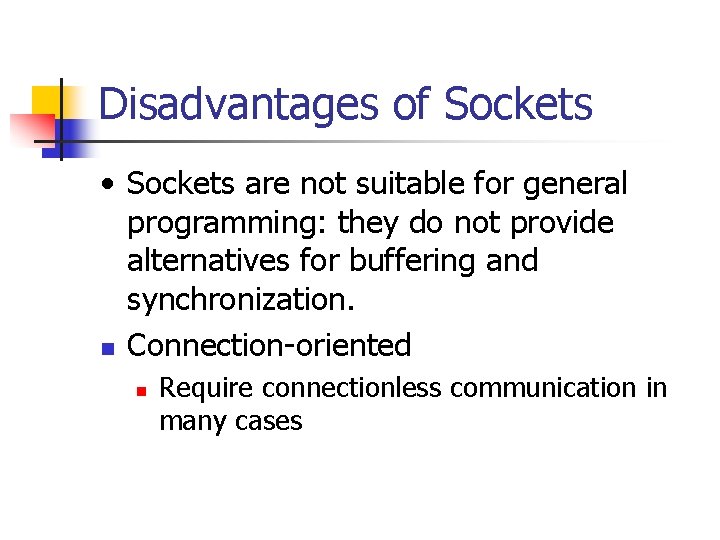
Disadvantages of Sockets • Sockets are not suitable for general programming: they do not provide alternatives for buffering and synchronization. n Connection-oriented n Require connectionless communication in many cases
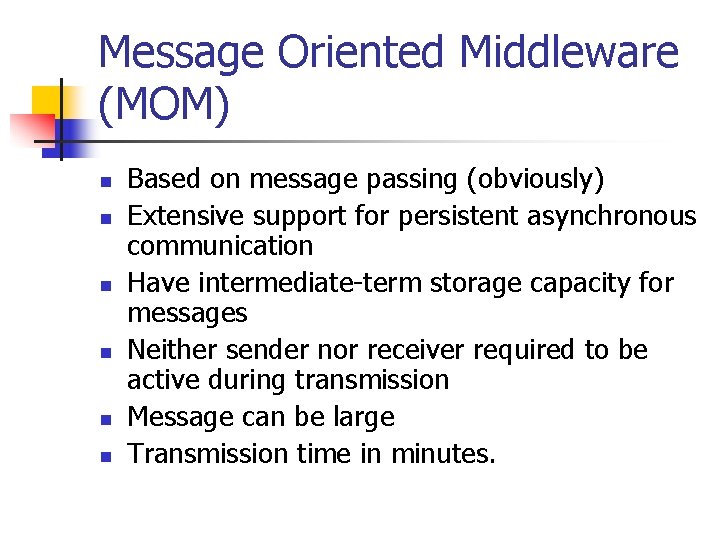
Message Oriented Middleware (MOM) n n n Based on message passing (obviously) Extensive support for persistent asynchronous communication Have intermediate-term storage capacity for messages Neither sender nor receiver required to be active during transmission Message can be large Transmission time in minutes.
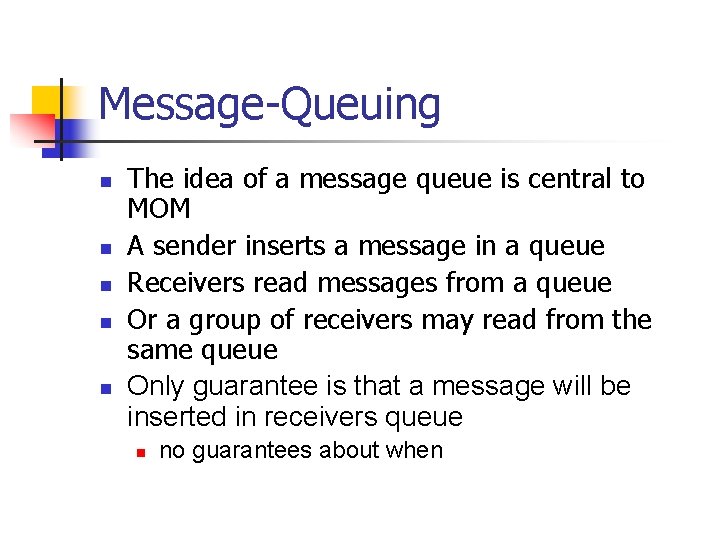
Message-Queuing n n n The idea of a message queue is central to MOM A sender inserts a message in a queue Receivers read messages from a queue Or a group of receivers may read from the same queue Only guarantee is that a message will be inserted in receivers queue n no guarantees about when
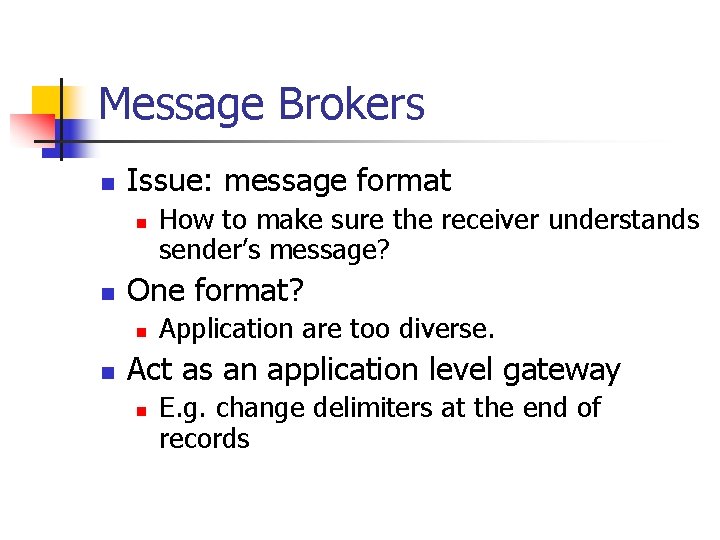
Message Brokers n Issue: message format n n One format? n n How to make sure the receiver understands sender’s message? Application are too diverse. Act as an application level gateway n E. g. change delimiters at the end of records
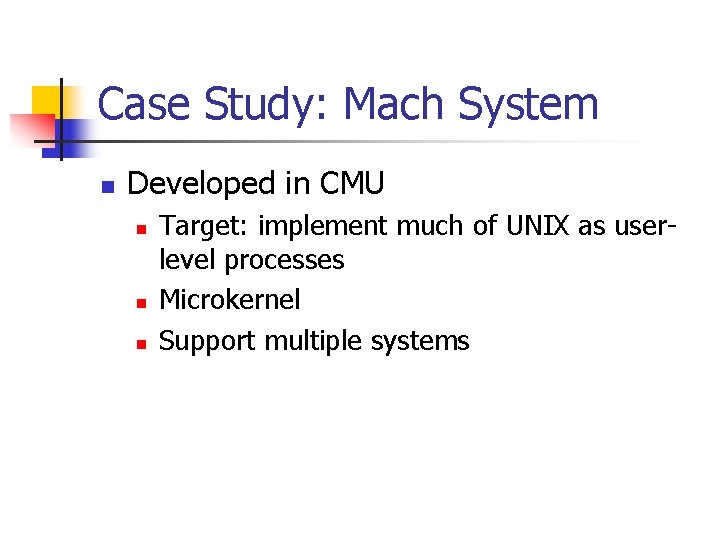
Case Study: Mach System n Developed in CMU n n n Target: implement much of UNIX as userlevel processes Microkernel Support multiple systems
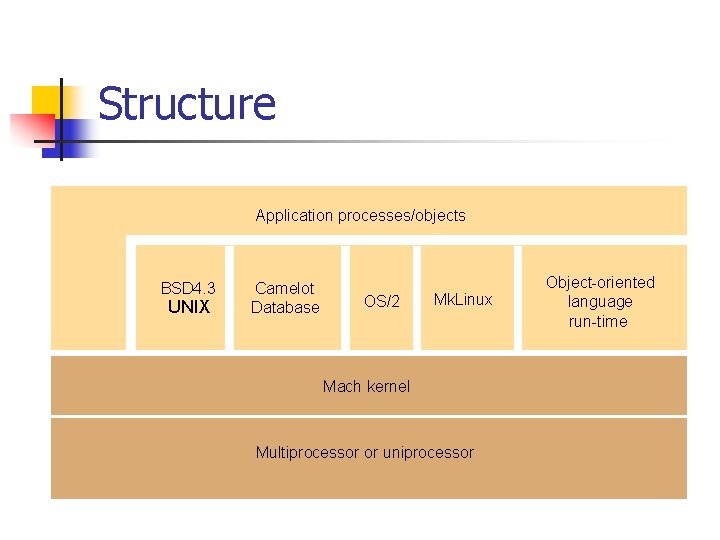
Structure Application processes/objects BSD 4. 3 UNIX Camelot Database OS/2 Mk. Linux Mach kernel Multiprocessor or uniprocessor Object-oriented language run-time
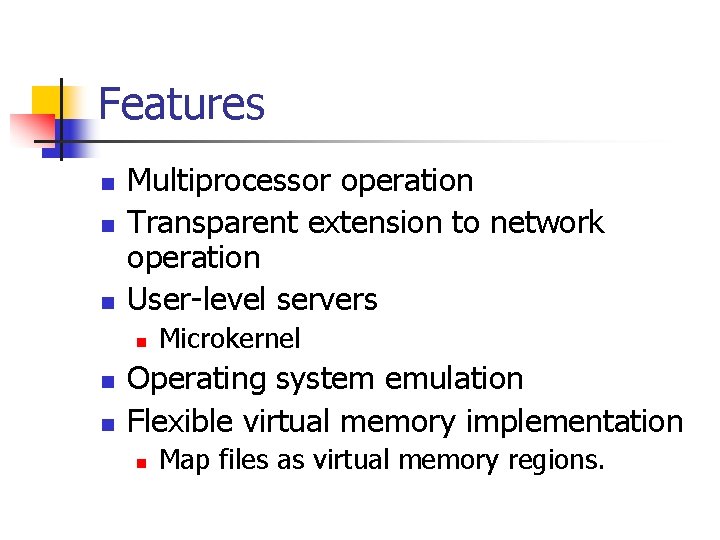
Features n n n Multiprocessor operation Transparent extension to network operation User-level servers n n n Microkernel Operating system emulation Flexible virtual memory implementation n Map files as virtual memory regions.
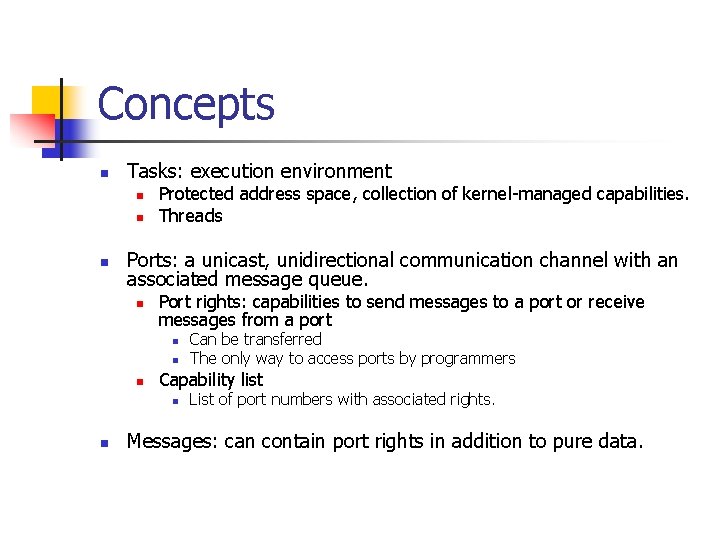
Concepts n Tasks: execution environment n n n Protected address space, collection of kernel-managed capabilities. Threads Ports: a unicast, unidirectional communication channel with an associated message queue. n Port rights: capabilities to send messages to a port or receive messages from a port n n n Capability list n n Can be transferred The only way to access ports by programmers List of port numbers with associated rights. Messages: can contain port rights in addition to pure data.
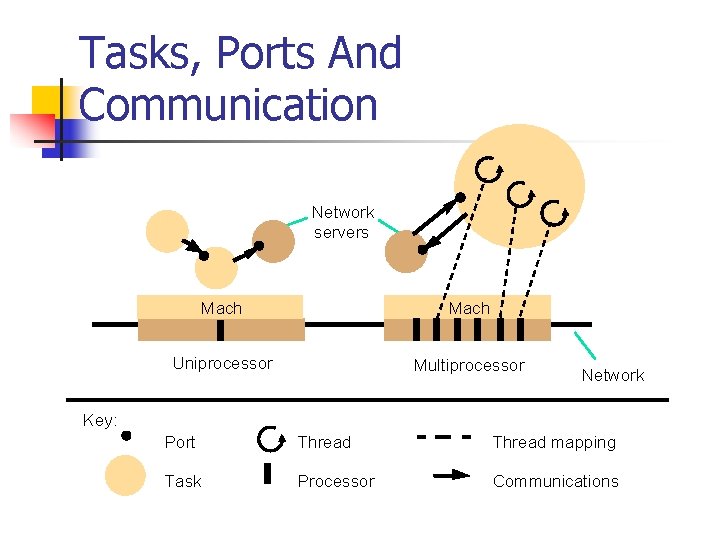
Tasks, Ports And Communication Network servers Mach Uniprocessor Multiprocessor Network Key: Port Thread mapping Task Processor Communications
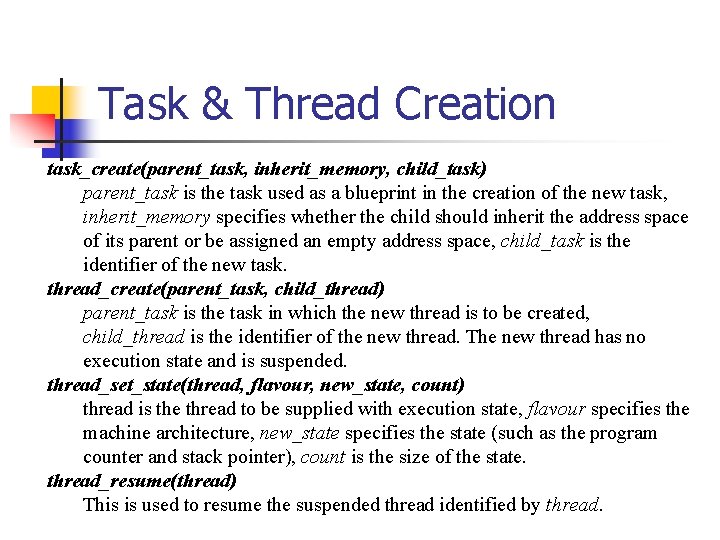
Task & Thread Creation task_create(parent_task, inherit_memory, child_task) parent_task is the task used as a blueprint in the creation of the new task, inherit_memory specifies whether the child should inherit the address space of its parent or be assigned an empty address space, child_task is the identifier of the new task. thread_create(parent_task, child_thread) parent_task is the task in which the new thread is to be created, child_thread is the identifier of the new thread. The new thread has no execution state and is suspended. thread_set_state(thread, flavour, new_state, count) thread is the thread to be supplied with execution state, flavour specifies the machine architecture, new_state specifies the state (such as the program counter and stack pointer), count is the size of the state. thread_resume(thread) This is used to resume the suspended thread identified by thread.
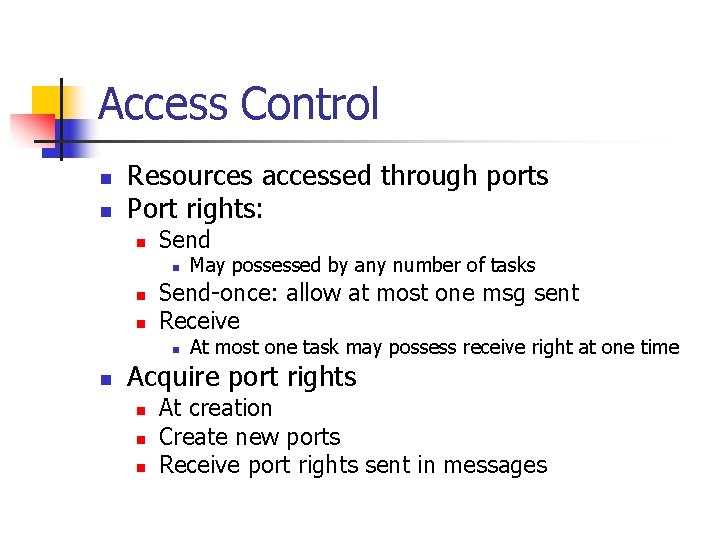
Access Control n n Resources accessed through ports Port rights: n Send n n n Send-once: allow at most one msg sent Receive n n May possessed by any number of tasks At most one task may possess receive right at one time Acquire port rights n n n At creation Create new ports Receive port rights sent in messages
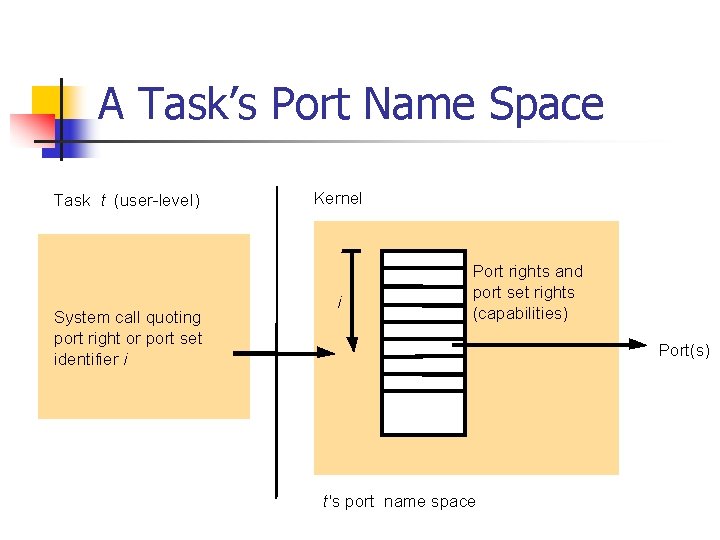
A Task’s Port Name Space Task t (user-level) System call quoting port right or port set identifier i Kernel i Port rights and port set rights (capabilities) Port(s) t 's port name space
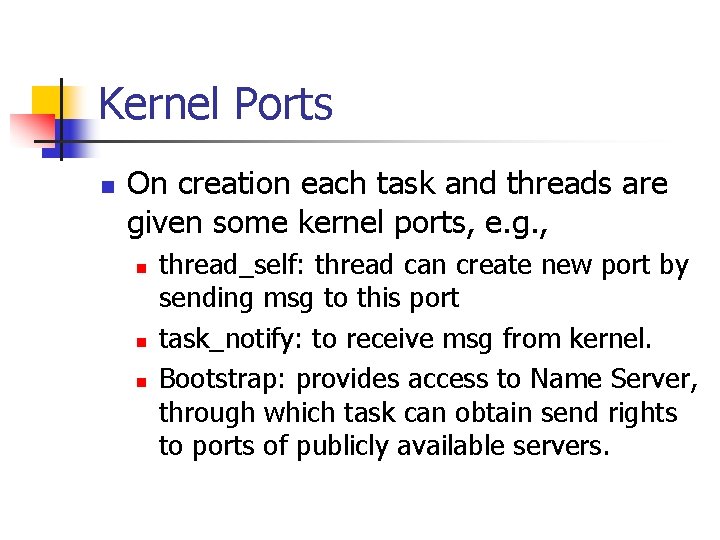
Kernel Ports n On creation each task and threads are given some kernel ports, e. g. , n n n thread_self: thread can create new port by sending msg to this port task_notify: to receive msg from kernel. Bootstrap: provides access to Name Server, through which task can obtain send rights to ports of publicly available servers.
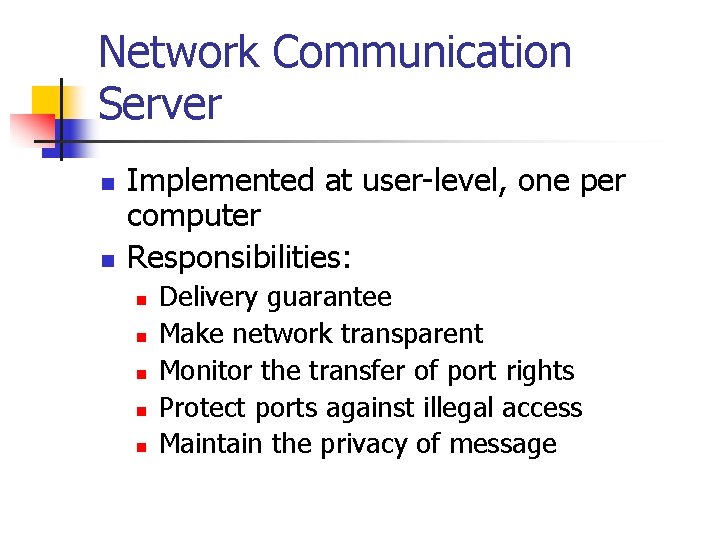
Network Communication Server n n Implemented at user-level, one per computer Responsibilities: n n n Delivery guarantee Make network transparent Monitor the transfer of port rights Protect ports against illegal access Maintain the privacy of message
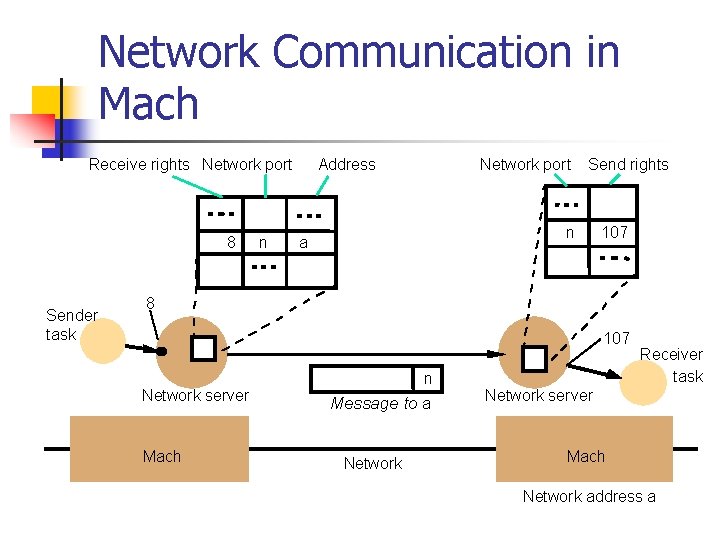
Network Communication in Mach Receive rights Network port 8 Sender task n Address Network port Send rights n a 107 8 107 Network server Mach n Message to a Network Receiver task Network server Mach Network address a
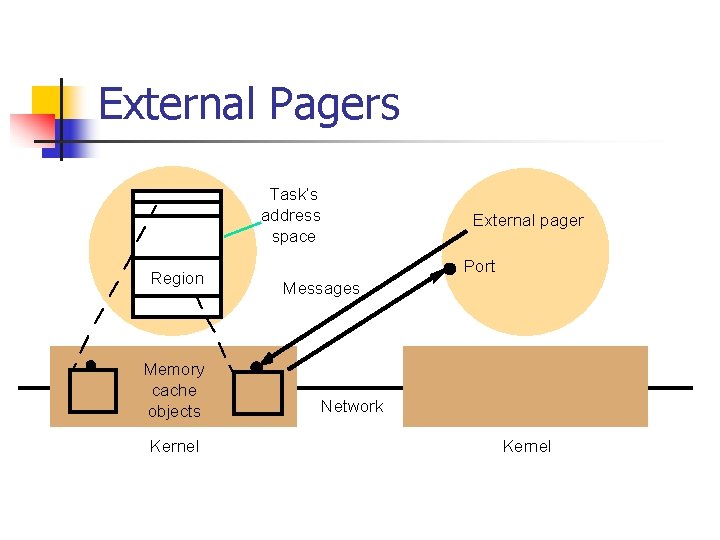
External Pagers Task’s address space Region Memory cache objects Kernel External pager Port Messages Network Kernel