Chapter 3 Expressions and Interactivity Csc 125 Introduction
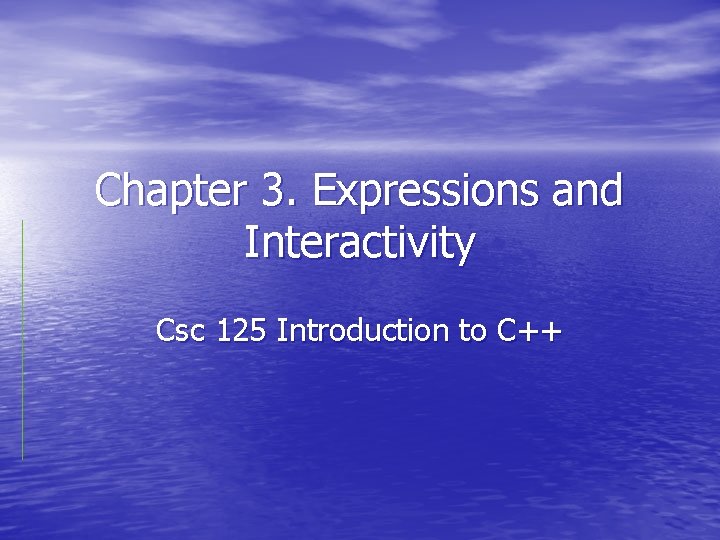
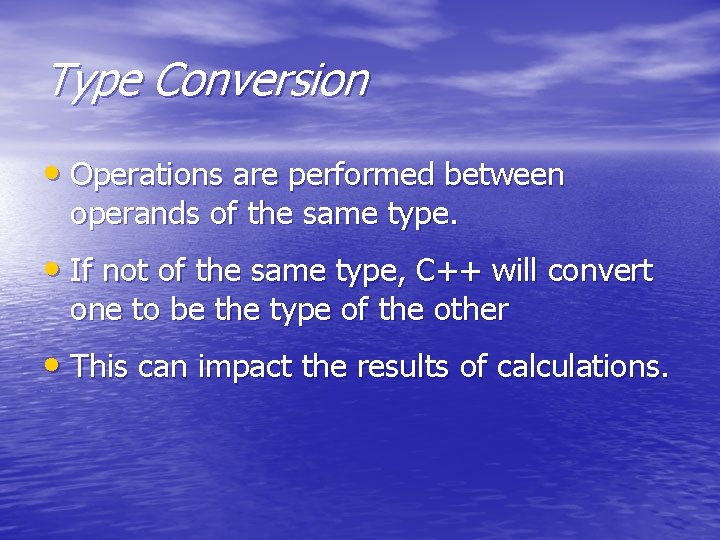
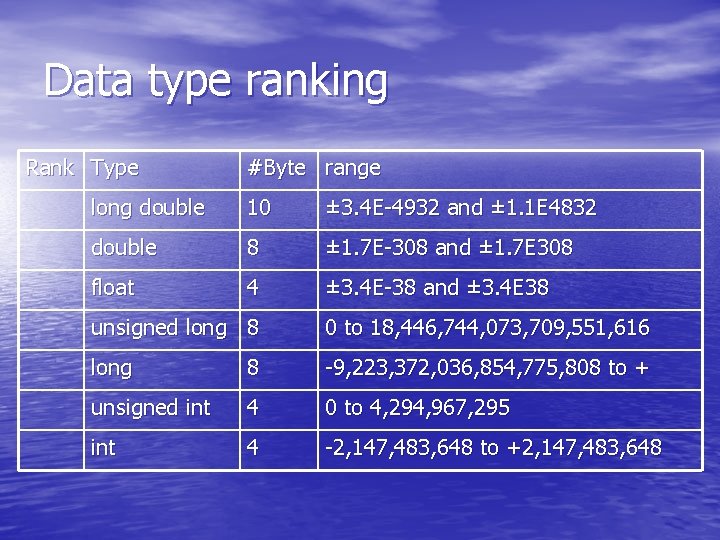
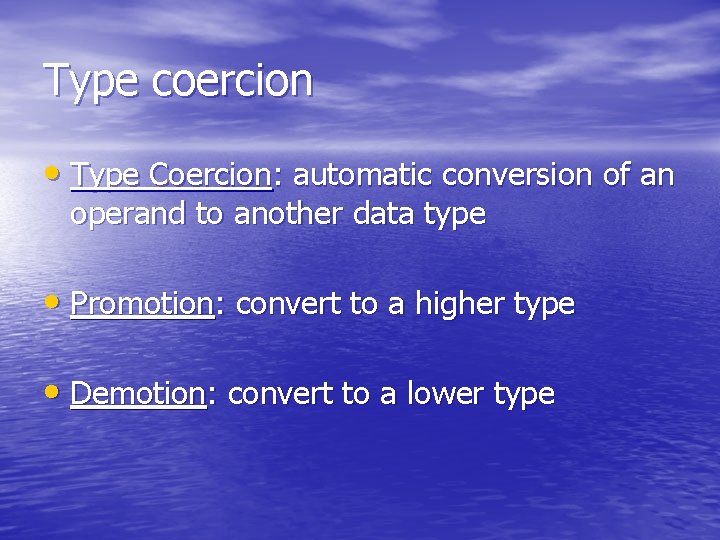
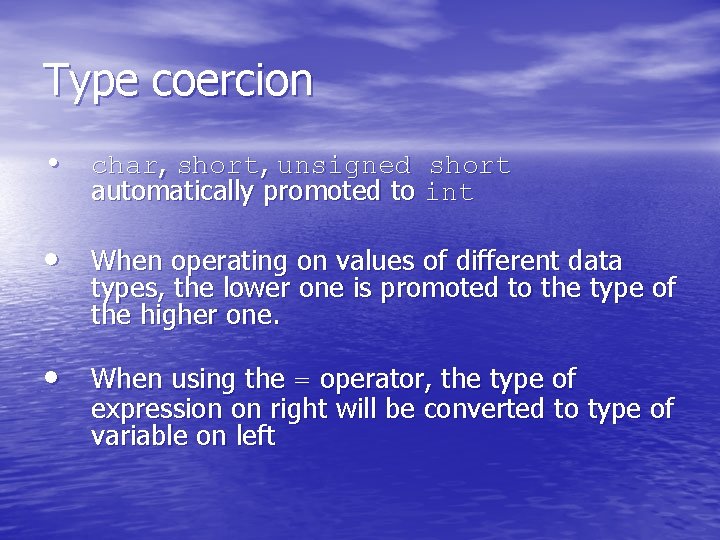
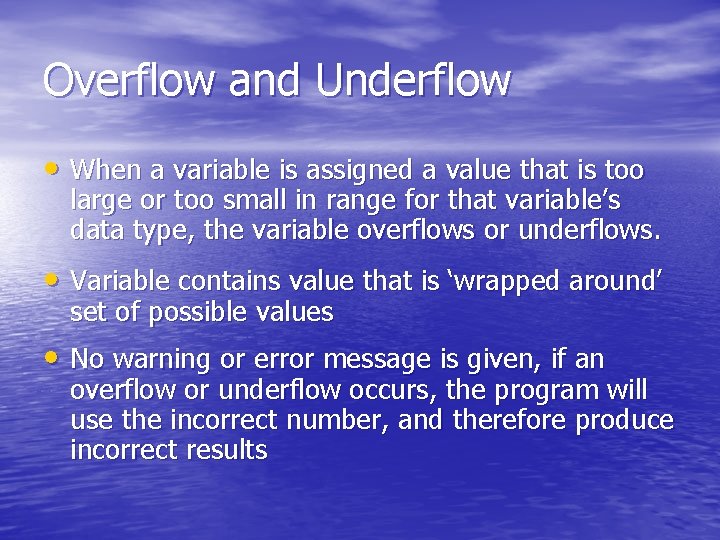
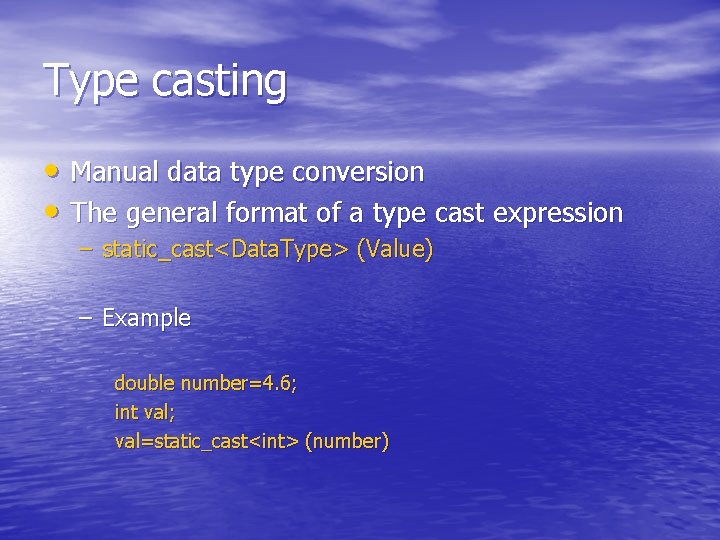
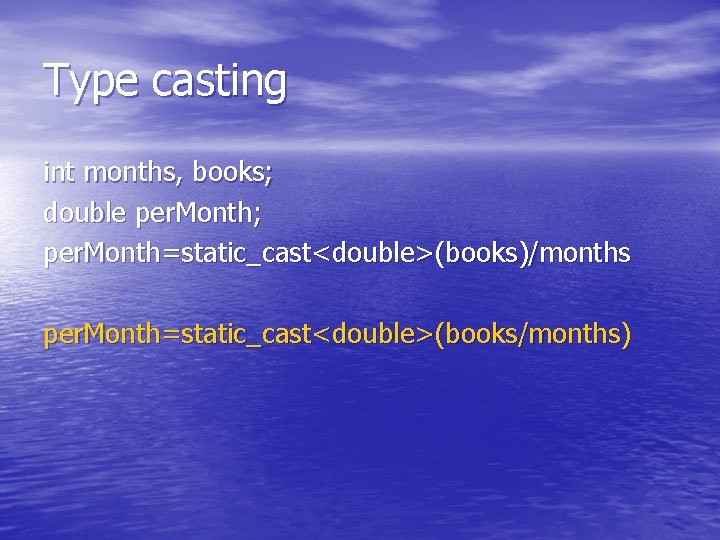
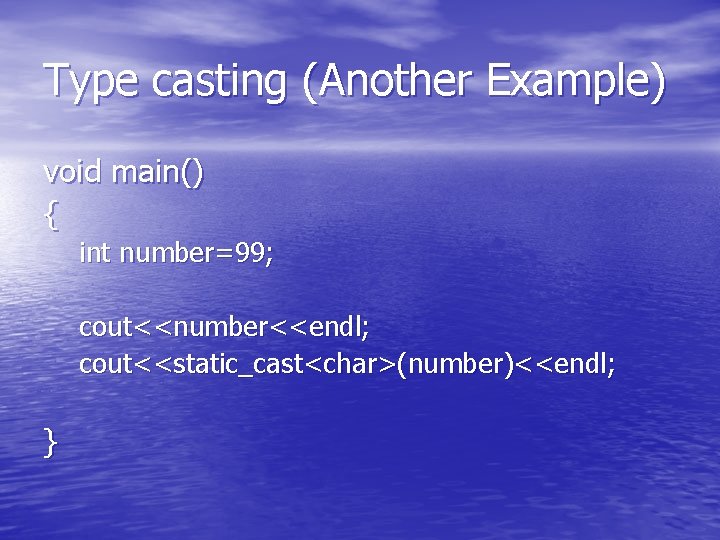
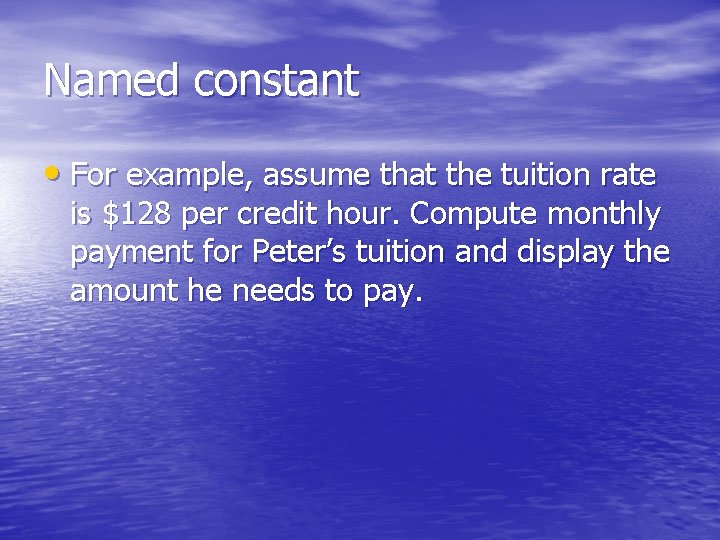
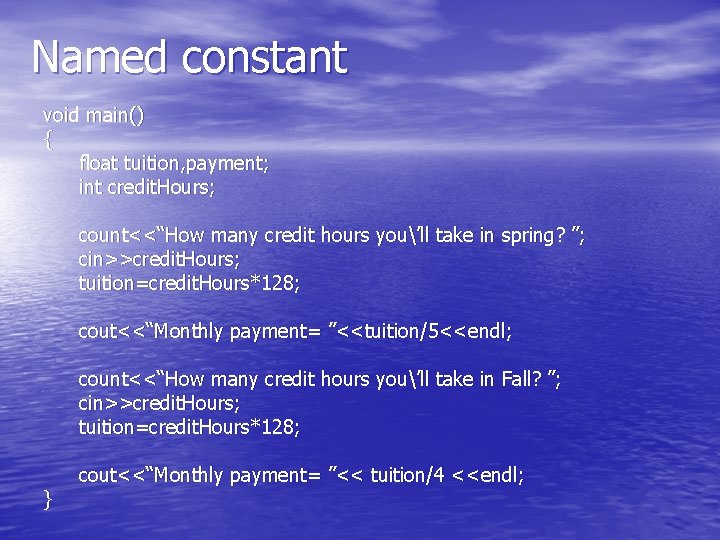
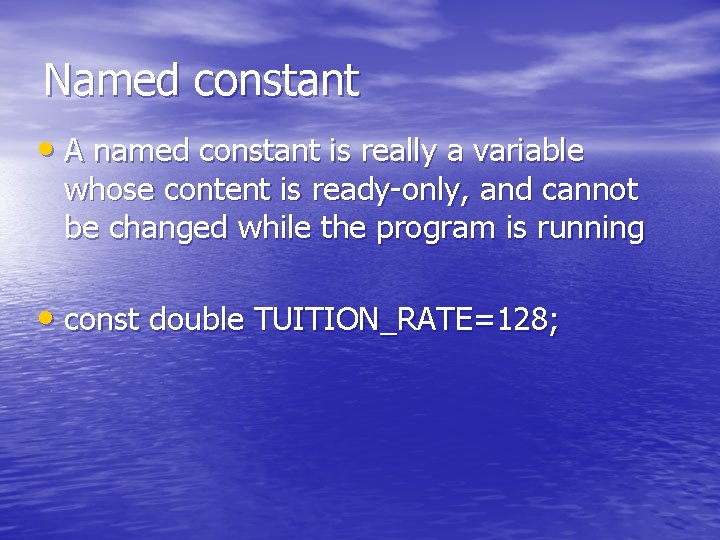
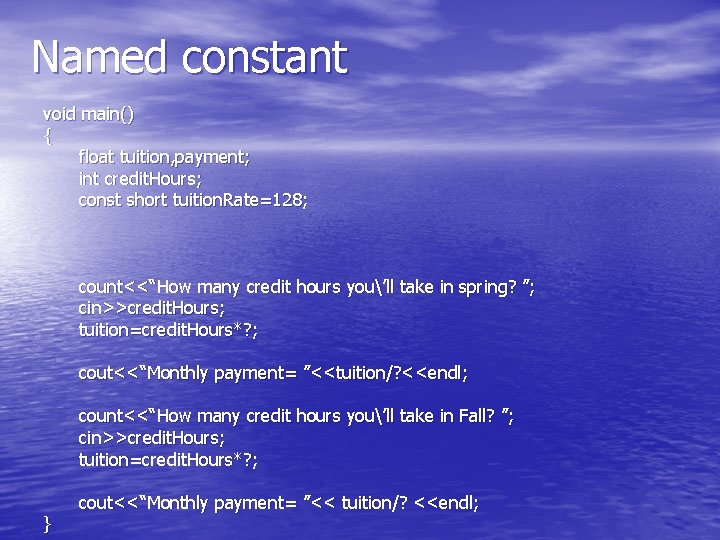
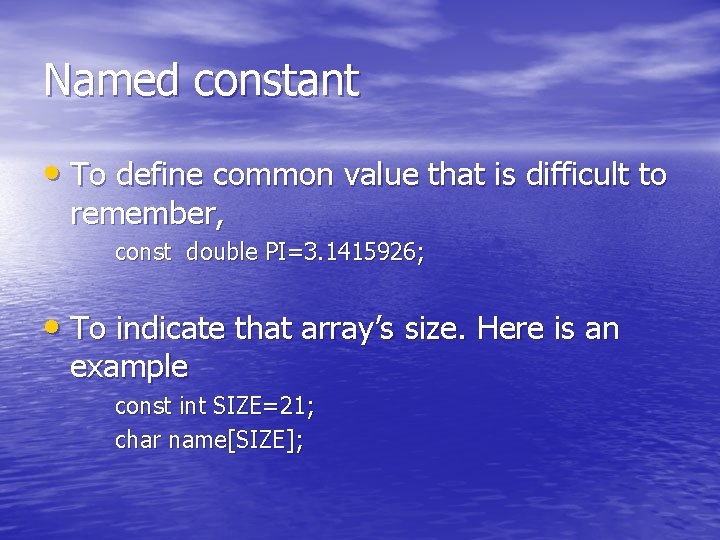
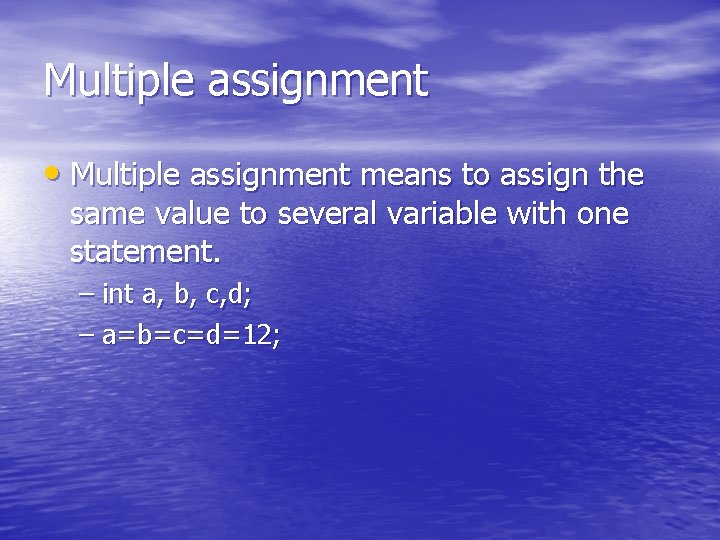
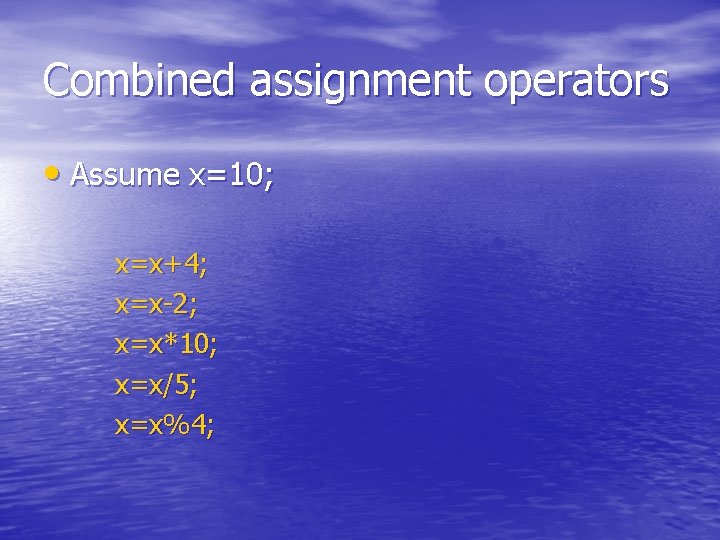
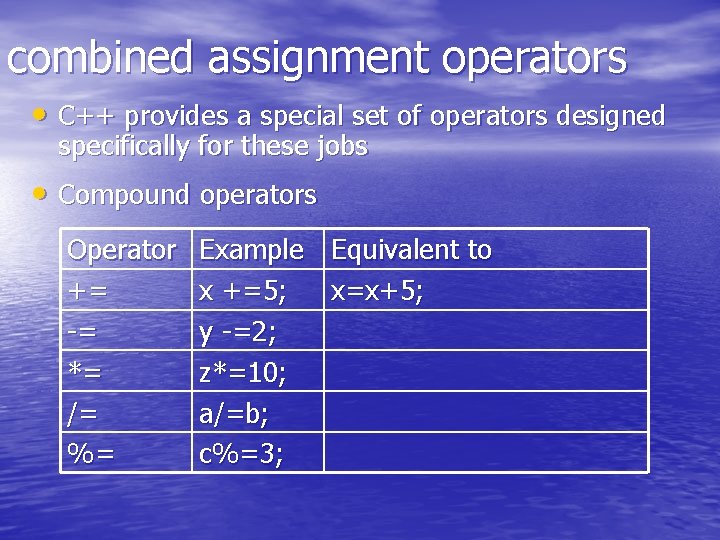
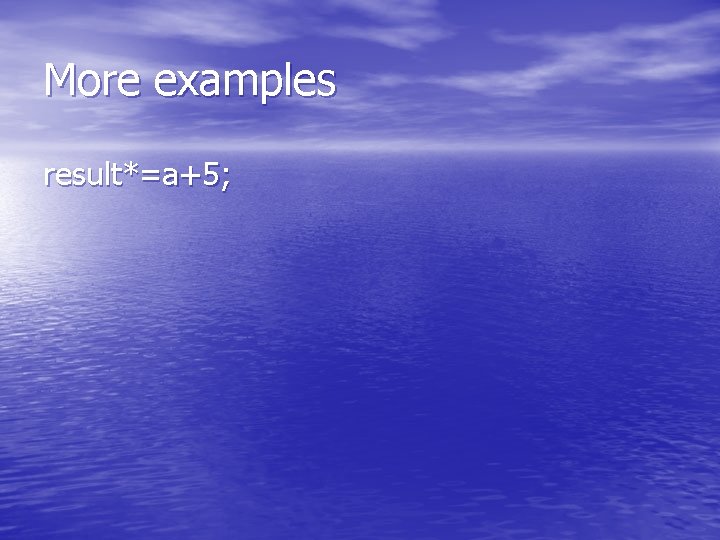
- Slides: 18
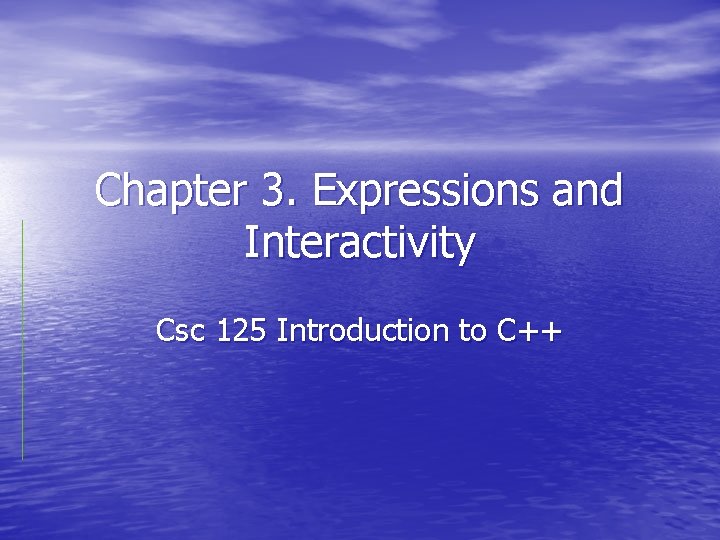
Chapter 3. Expressions and Interactivity Csc 125 Introduction to C++
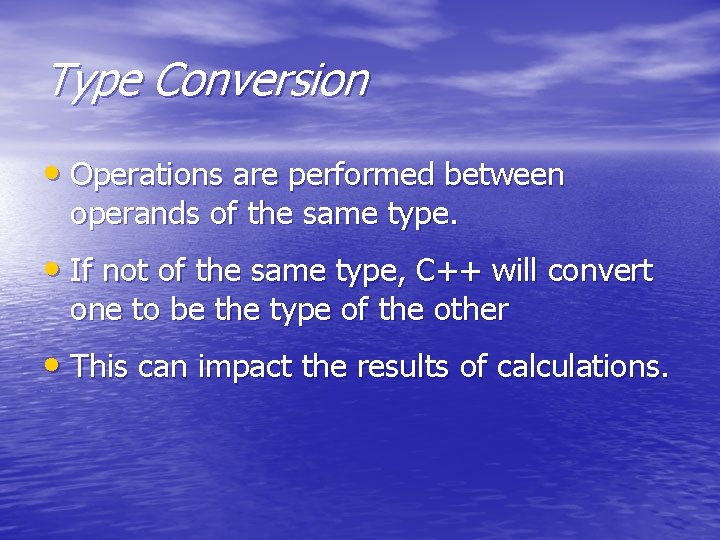
Type Conversion • Operations are performed between operands of the same type. • If not of the same type, C++ will convert one to be the type of the other • This can impact the results of calculations.
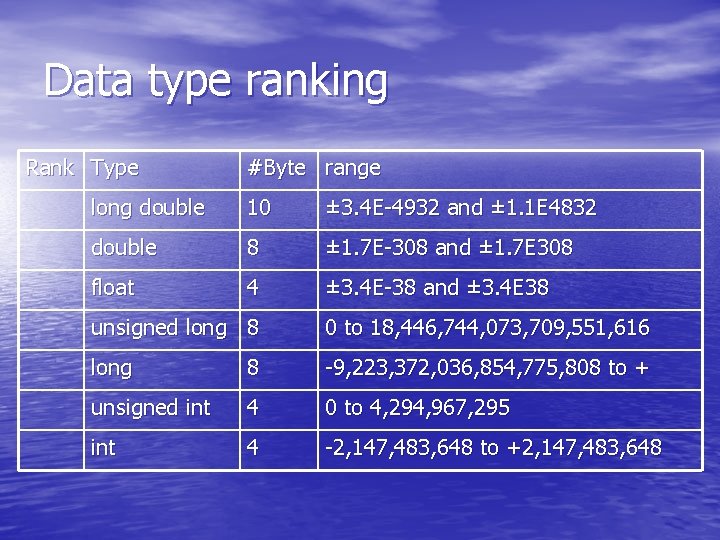
Data type ranking Rank Type #Byte range long double 10 ± 3. 4 E-4932 and ± 1. 1 E 4832 double 8 ± 1. 7 E-308 and ± 1. 7 E 308 float 4 ± 3. 4 E-38 and ± 3. 4 E 38 unsigned long 8 0 to 18, 446, 744, 073, 709, 551, 616 long 8 -9, 223, 372, 036, 854, 775, 808 to + unsigned int 4 0 to 4, 294, 967, 295 int 4 -2, 147, 483, 648 to +2, 147, 483, 648
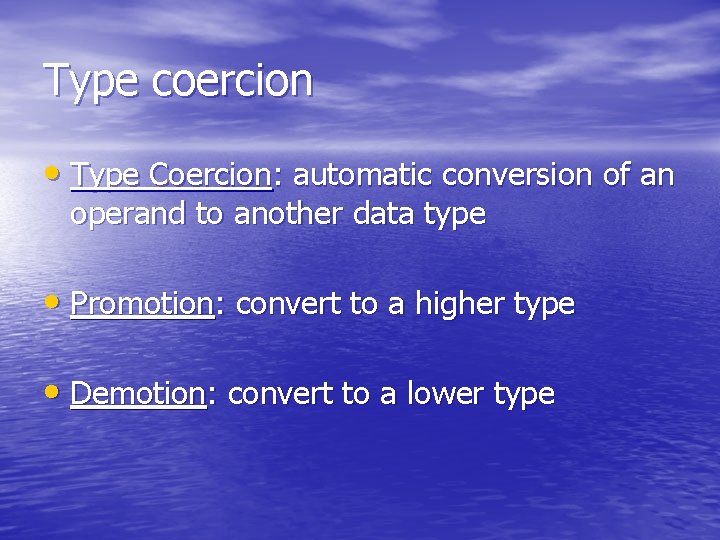
Type coercion • Type Coercion: automatic conversion of an operand to another data type • Promotion: convert to a higher type • Demotion: convert to a lower type
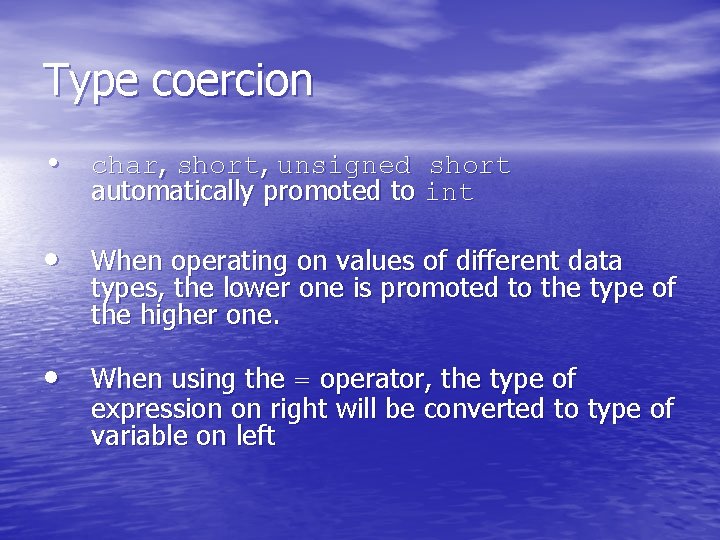
Type coercion • char, short, unsigned short automatically promoted to int • When operating on values of different data types, the lower one is promoted to the type of the higher one. • When using the = operator, the type of expression on right will be converted to type of variable on left
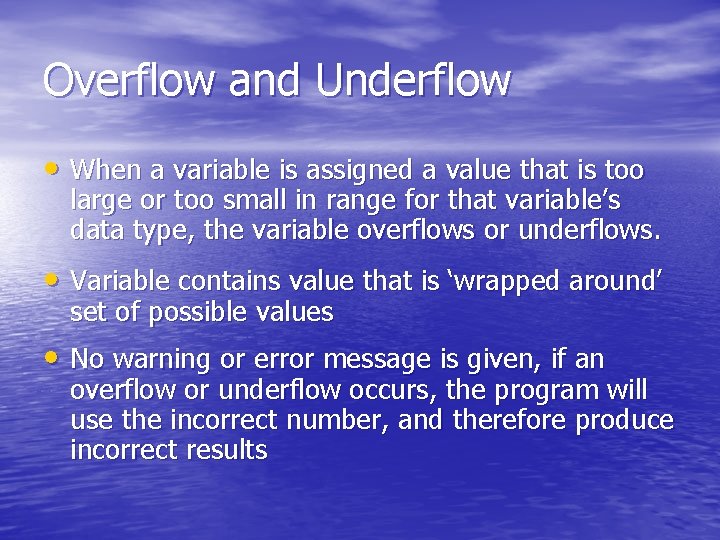
Overflow and Underflow • When a variable is assigned a value that is too large or too small in range for that variable’s data type, the variable overflows or underflows. • Variable contains value that is ‘wrapped around’ set of possible values • No warning or error message is given, if an overflow or underflow occurs, the program will use the incorrect number, and therefore produce incorrect results
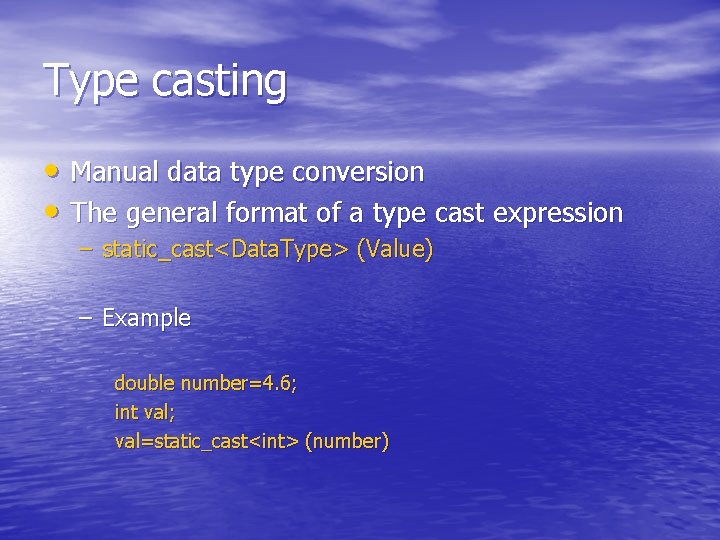
Type casting • Manual data type conversion • The general format of a type cast expression – static_cast<Data. Type> (Value) – Example double number=4. 6; int val; val=static_cast<int> (number)
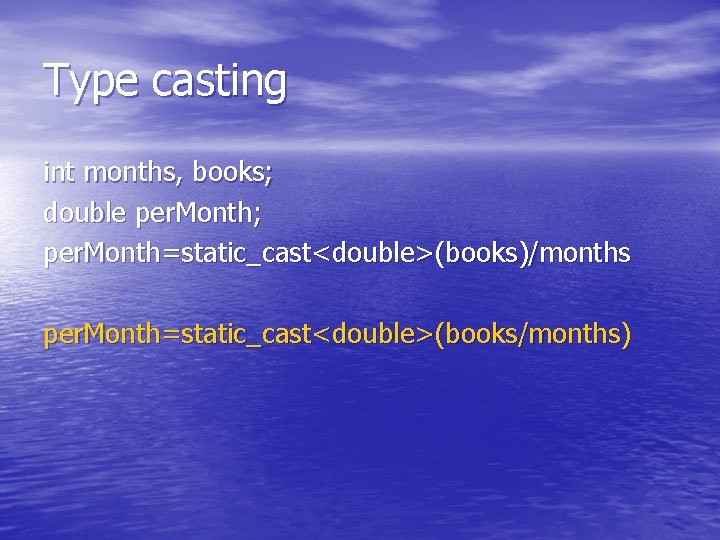
Type casting int months, books; double per. Month; per. Month=static_cast<double>(books)/months per. Month=static_cast<double>(books/months)
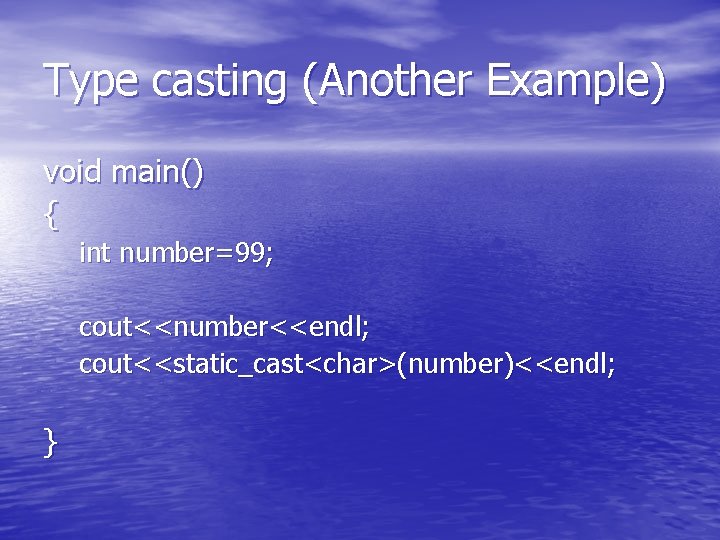
Type casting (Another Example) void main() { int number=99; cout<<number<<endl; cout<<static_cast<char>(number)<<endl; }
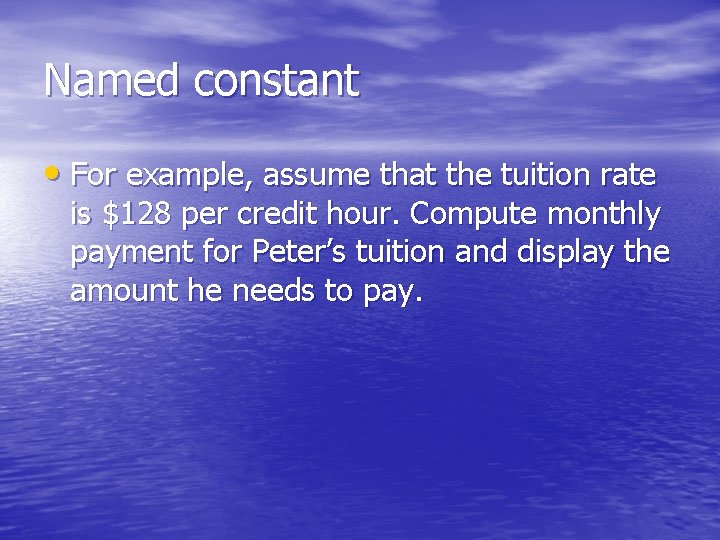
Named constant • For example, assume that the tuition rate is $128 per credit hour. Compute monthly payment for Peter’s tuition and display the amount he needs to pay.
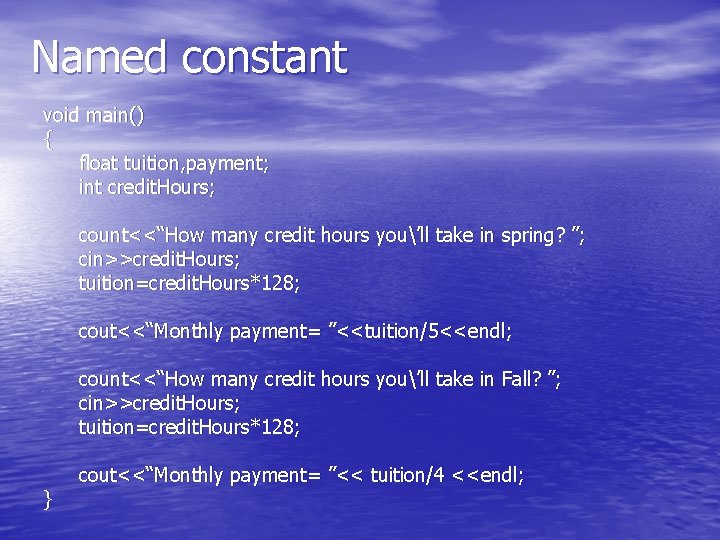
Named constant void main() { float tuition, payment; int credit. Hours; count<<“How many credit hours you’ll take in spring? ”; cin>>credit. Hours; tuition=credit. Hours*128; cout<<“Monthly payment= ”<<tuition/5<<endl; count<<“How many credit hours you’ll take in Fall? ”; cin>>credit. Hours; tuition=credit. Hours*128; } cout<<“Monthly payment= ”<< tuition/4 <<endl;
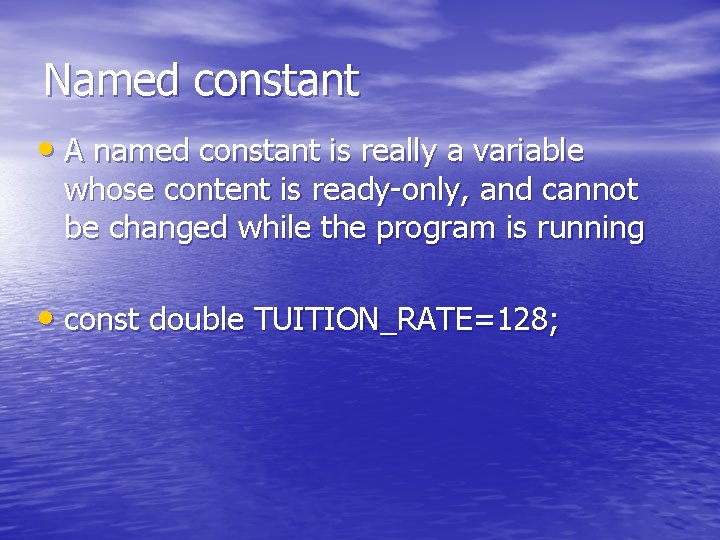
Named constant • A named constant is really a variable whose content is ready-only, and cannot be changed while the program is running • const double TUITION_RATE=128;
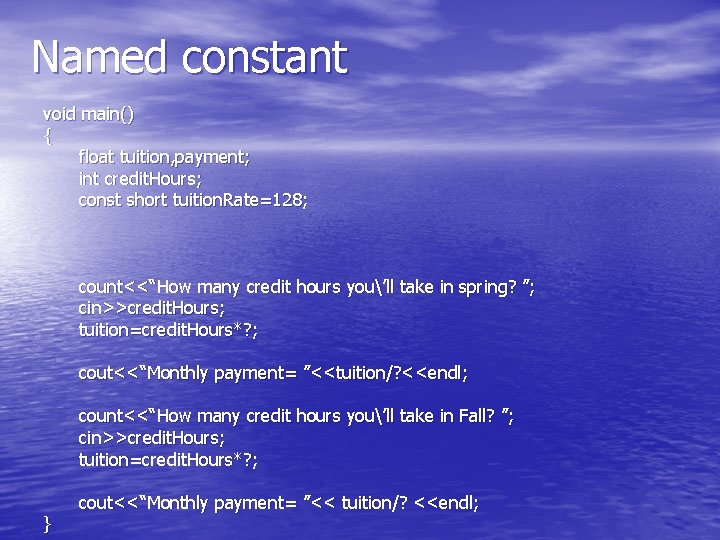
Named constant void main() { float tuition, payment; int credit. Hours; const short tuition. Rate=128; count<<“How many credit hours you’ll take in spring? ”; cin>>credit. Hours; tuition=credit. Hours*? ; cout<<“Monthly payment= ”<<tuition/? <<endl; count<<“How many credit hours you’ll take in Fall? ”; cin>>credit. Hours; tuition=credit. Hours*? ; } cout<<“Monthly payment= ”<< tuition/? <<endl;
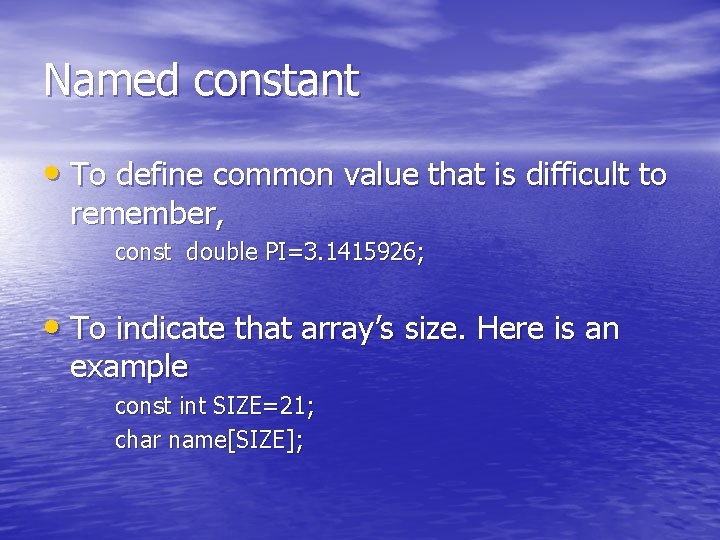
Named constant • To define common value that is difficult to remember, const double PI=3. 1415926; • To indicate that array’s size. Here is an example const int SIZE=21; char name[SIZE];
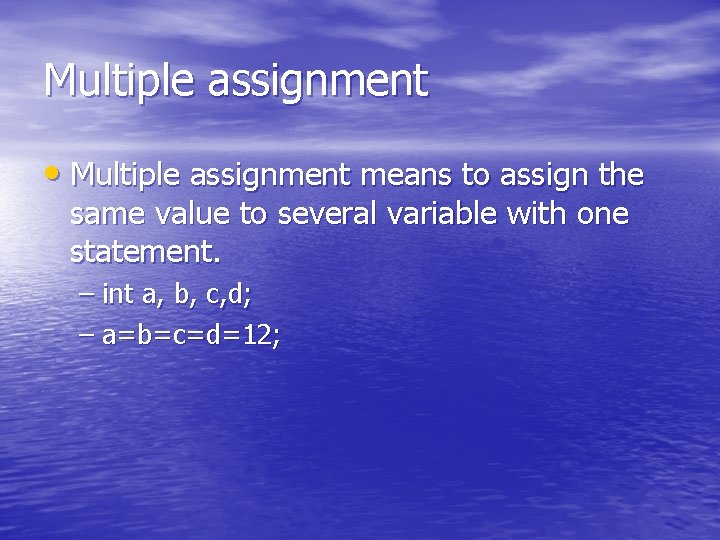
Multiple assignment • Multiple assignment means to assign the same value to several variable with one statement. – int a, b, c, d; – a=b=c=d=12;
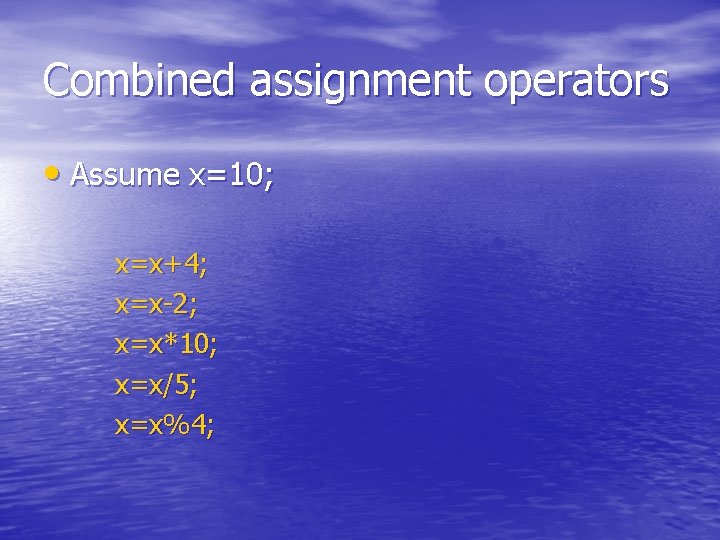
Combined assignment operators • Assume x=10; x=x+4; x=x-2; x=x*10; x=x/5; x=x%4;
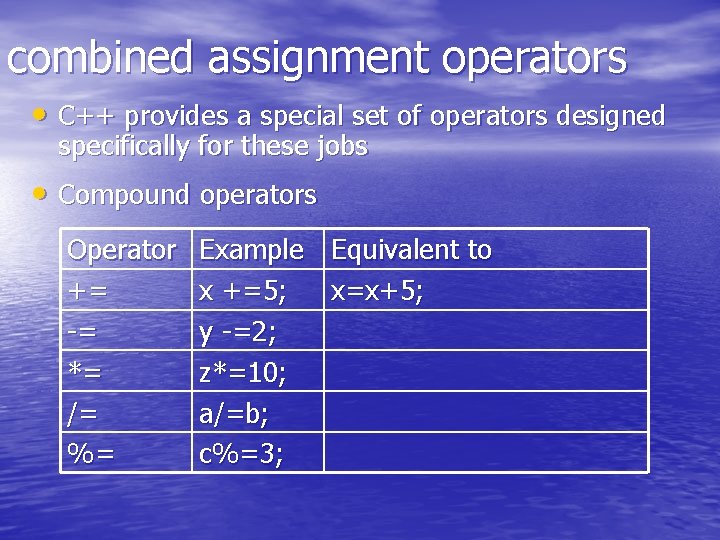
combined assignment operators • C++ provides a special set of operators designed specifically for these jobs • Compound operators Operator += -= *= /= %= Example Equivalent to x +=5; x=x+5; y -=2; z*=10; a/=b; c%=3;
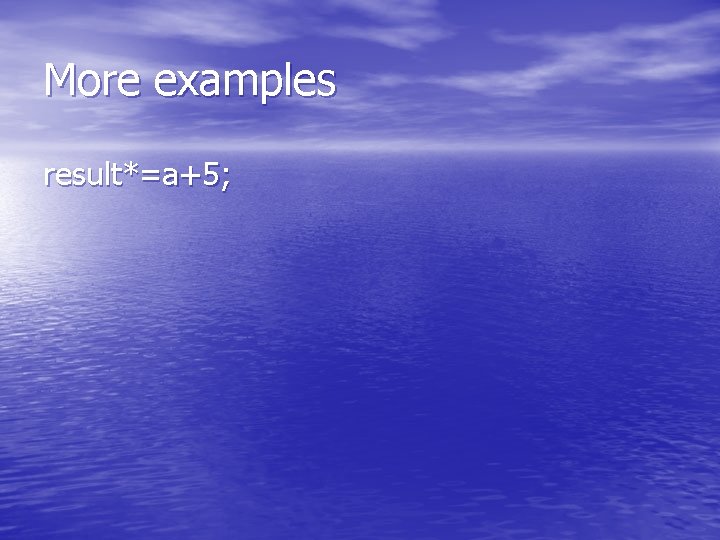
More examples result*=a+5;