Chapter 3 ADT Unsorted List Yanjun Li CS
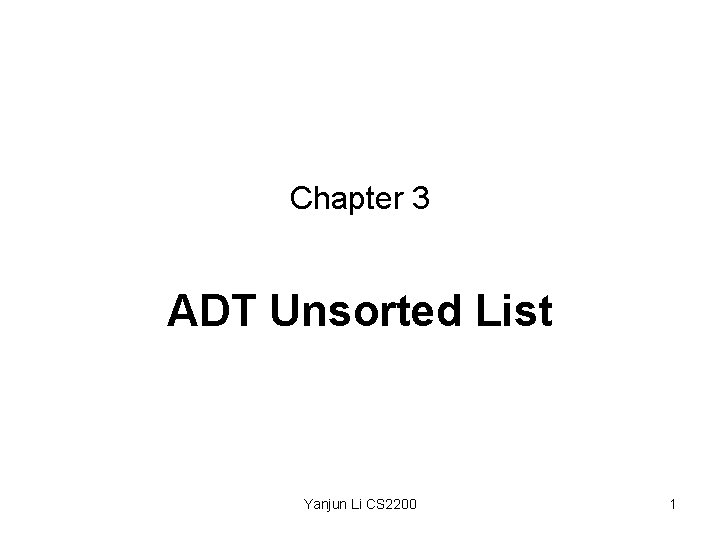
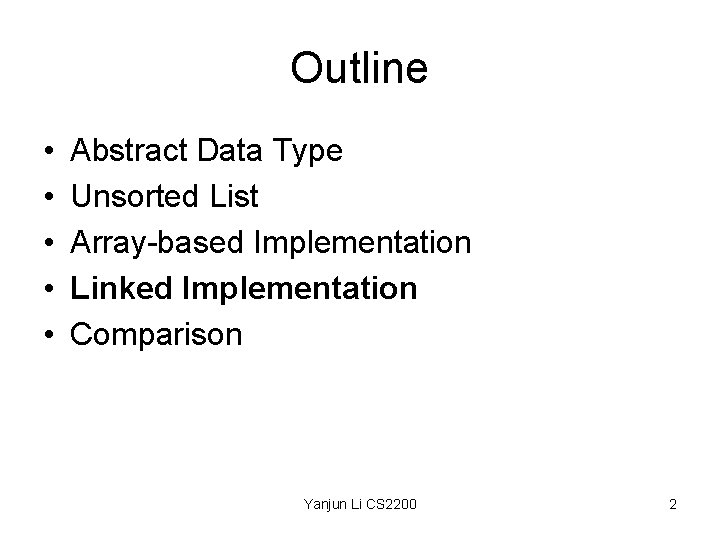
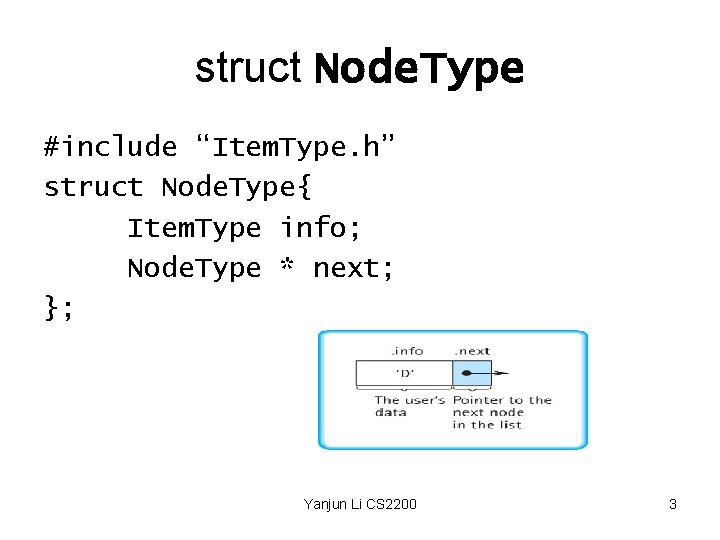
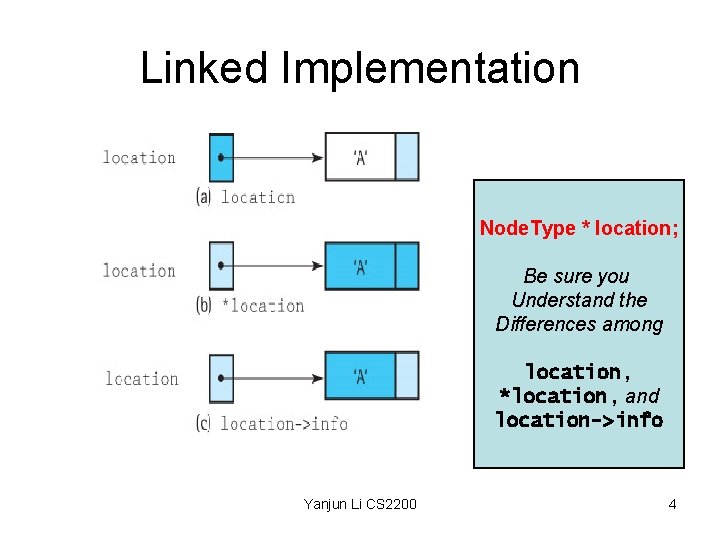
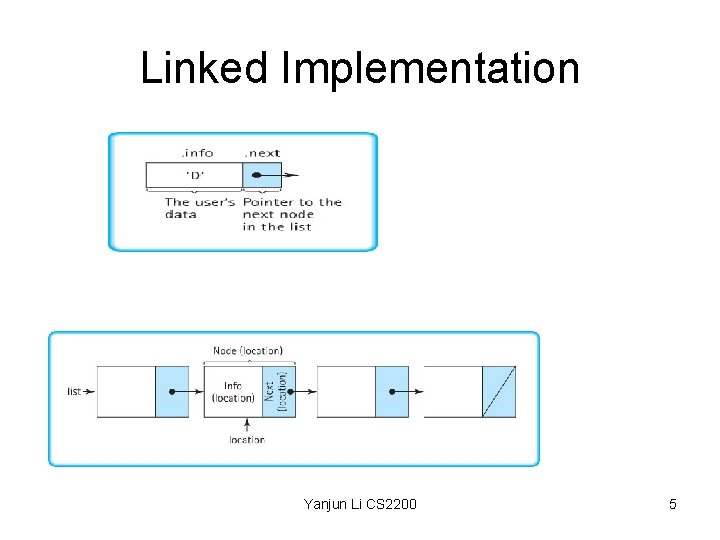
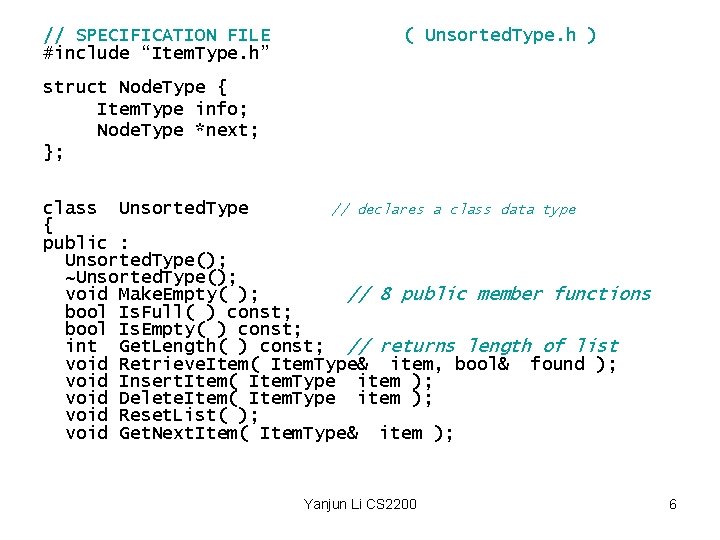
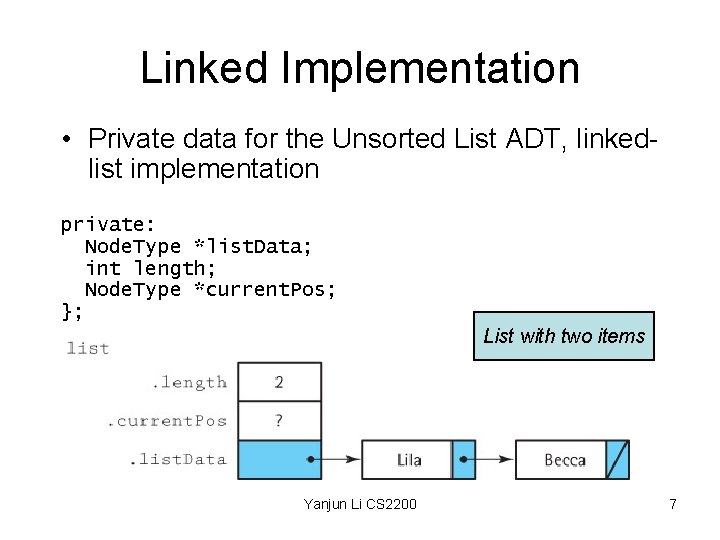
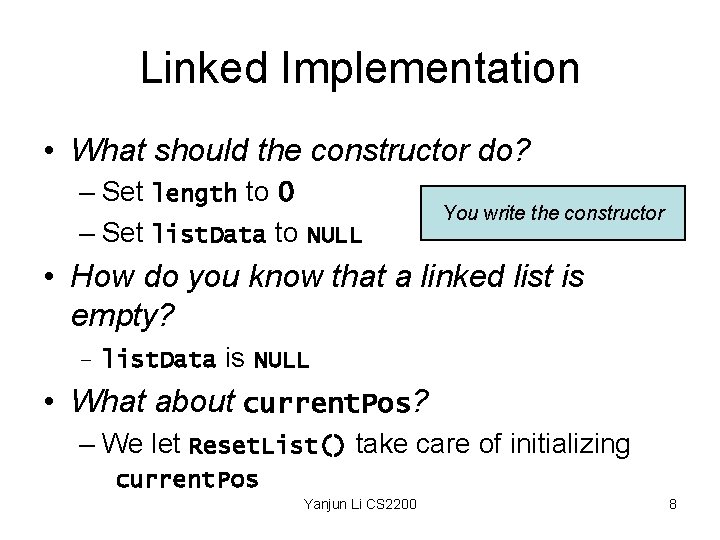
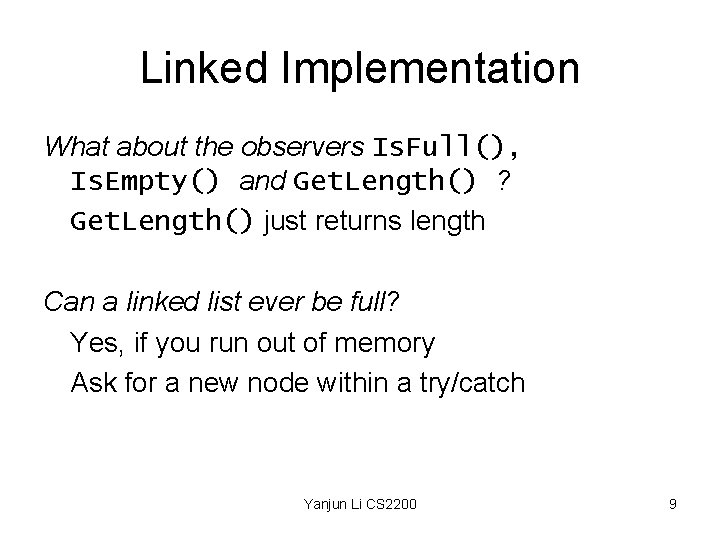
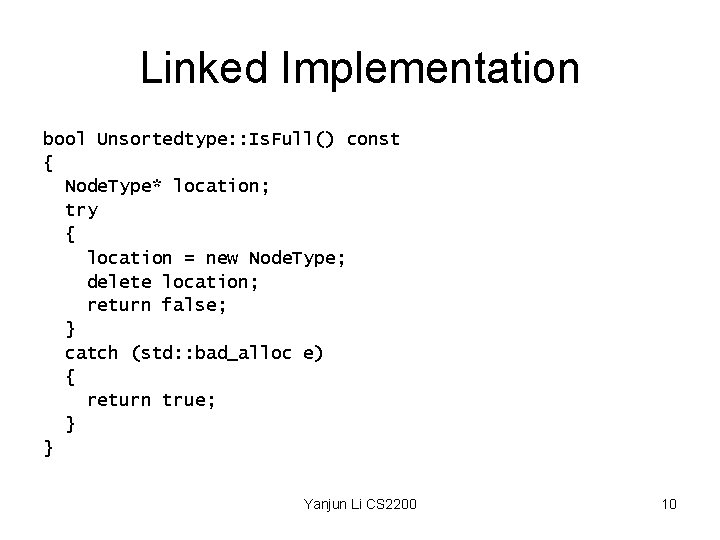
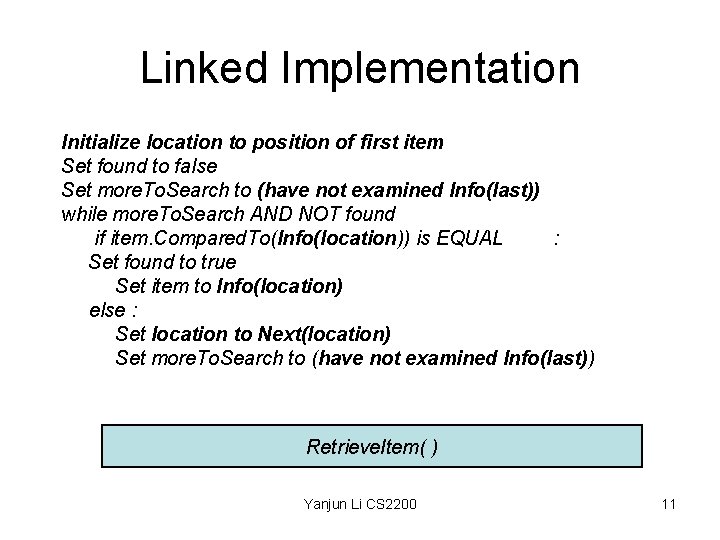
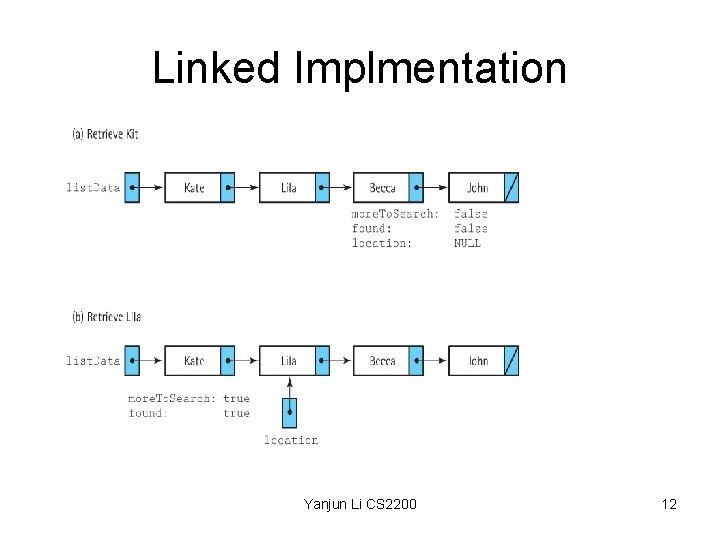
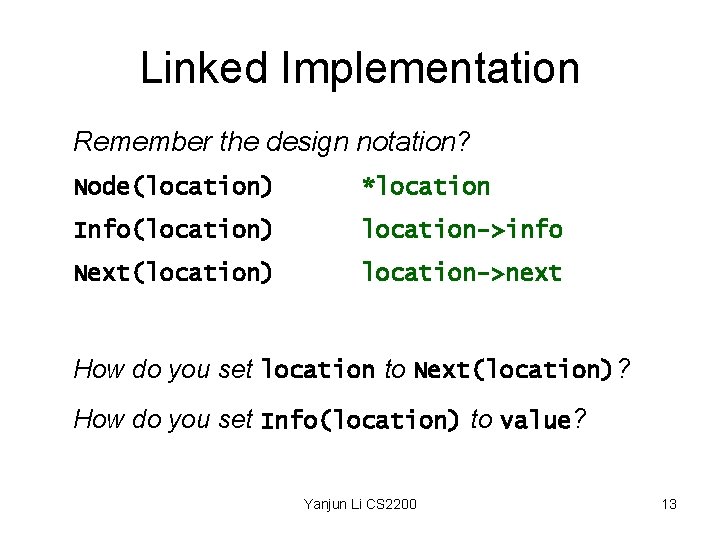
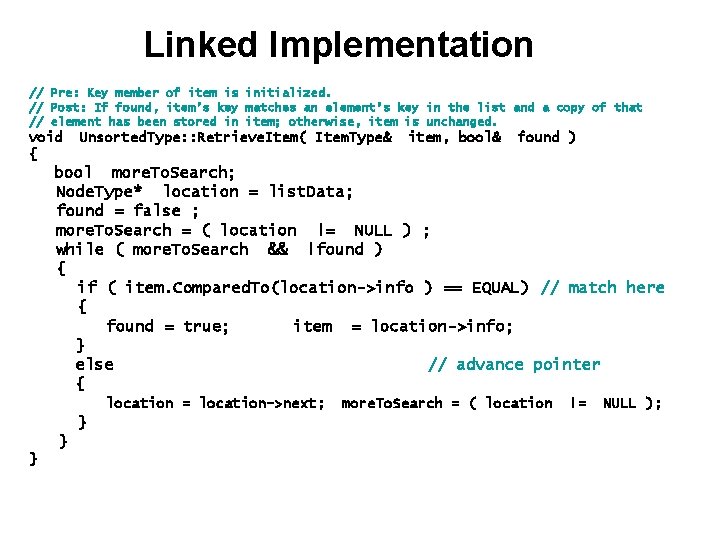
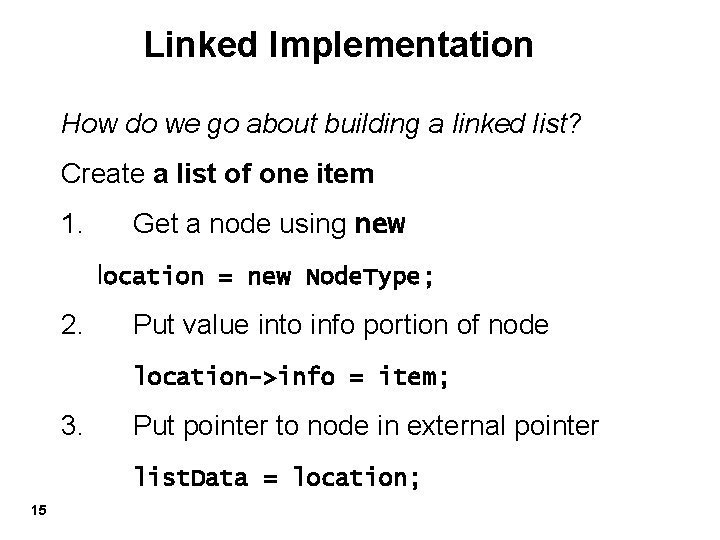
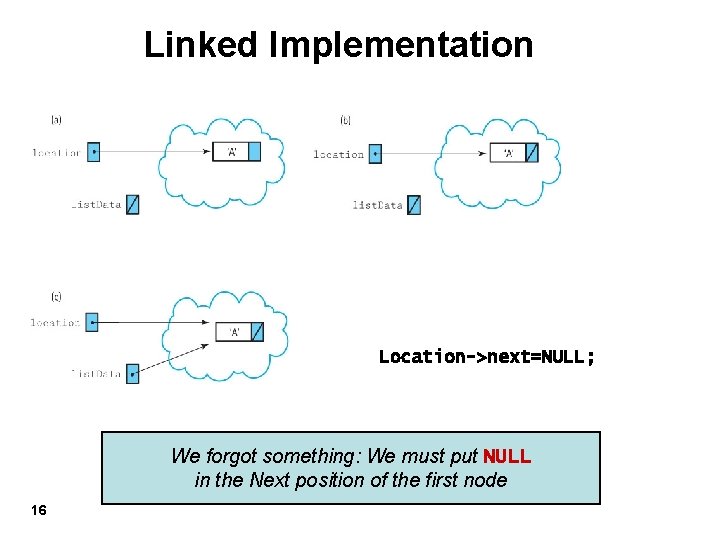
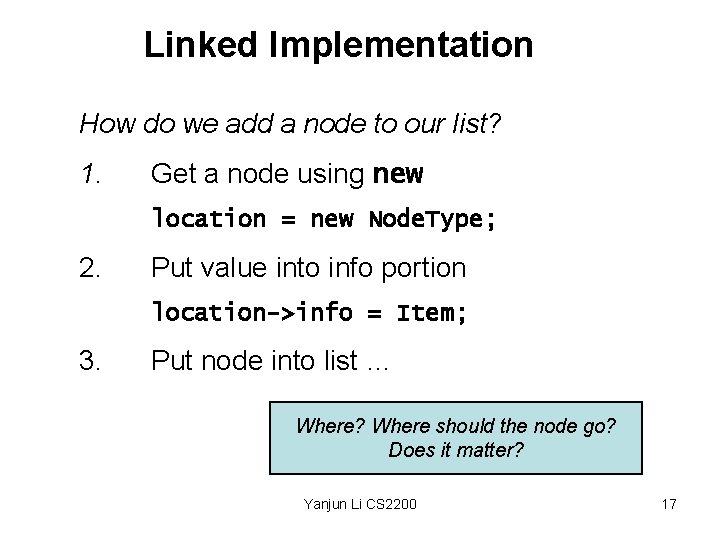
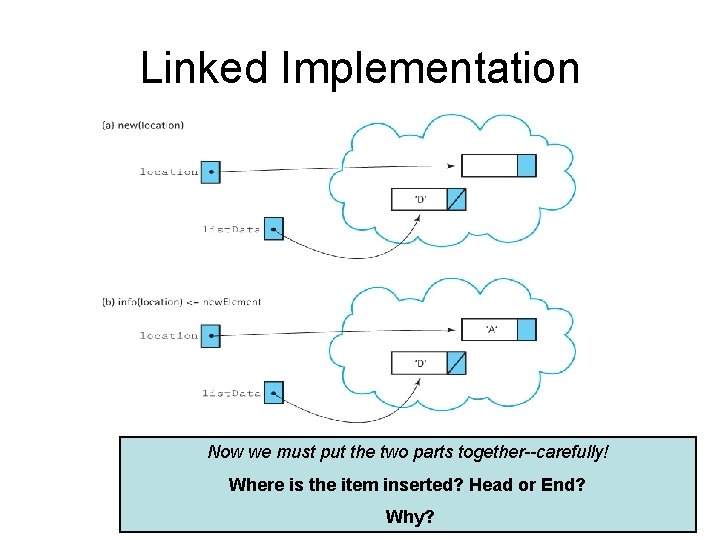
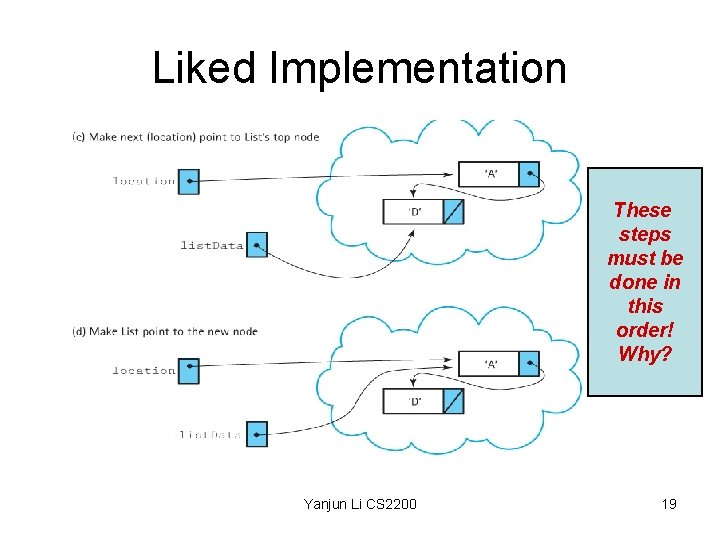
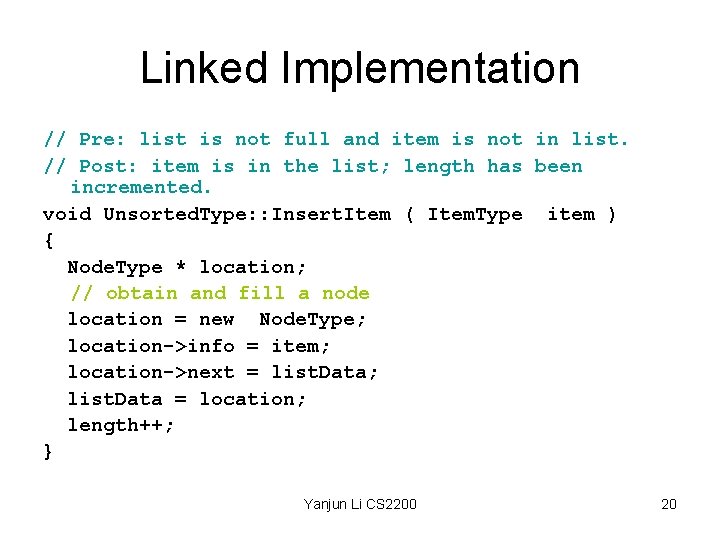
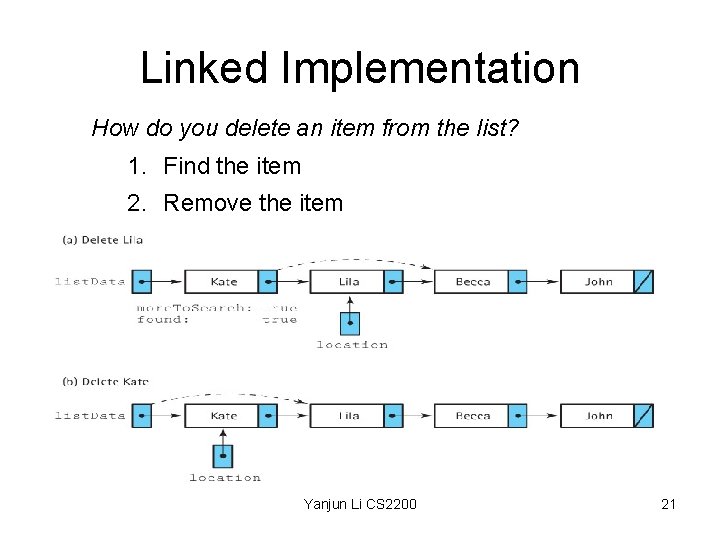
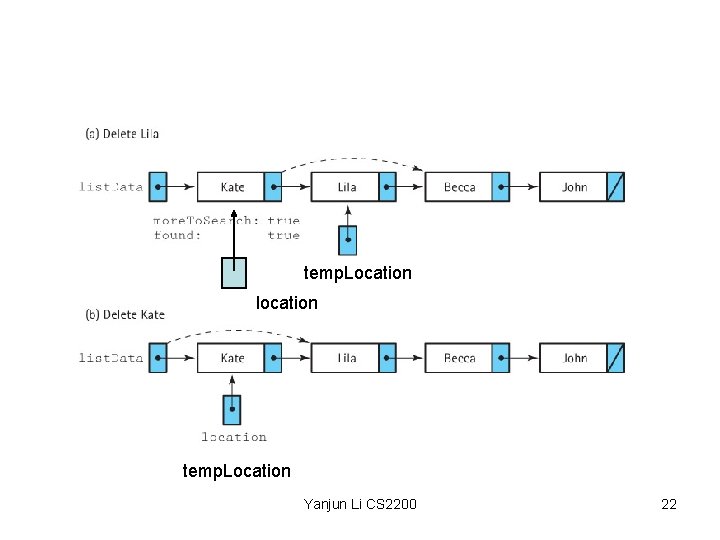
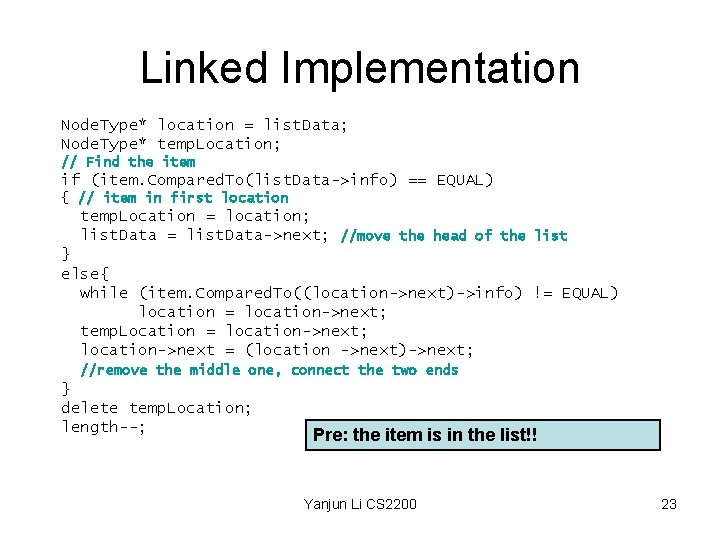
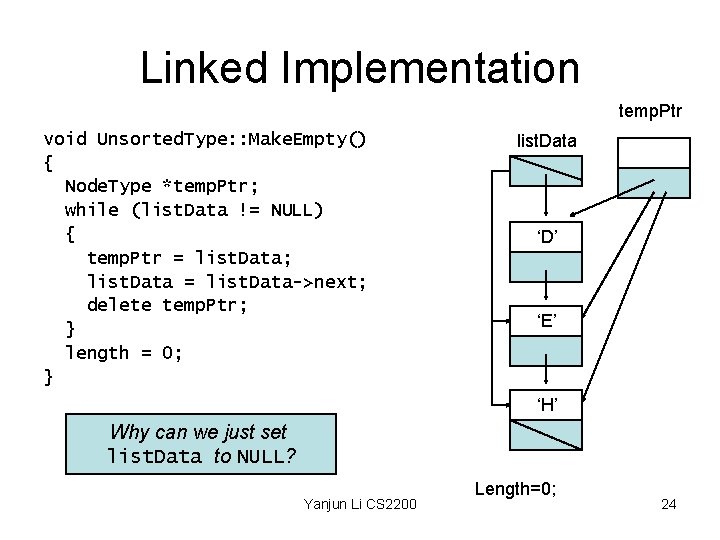
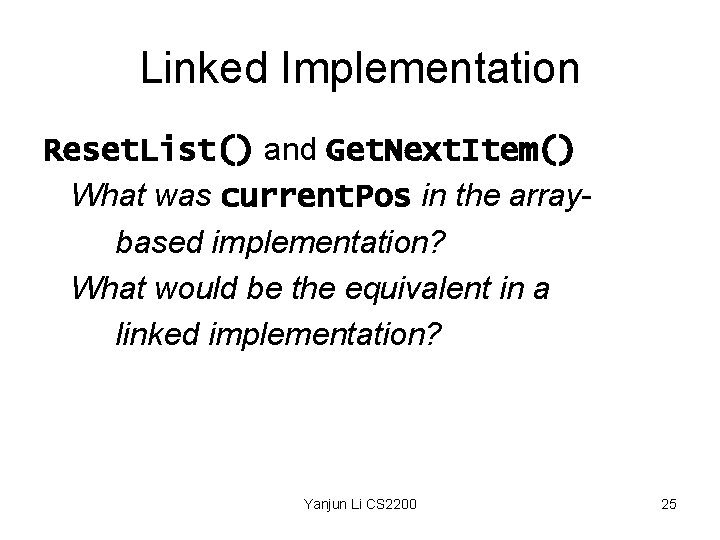
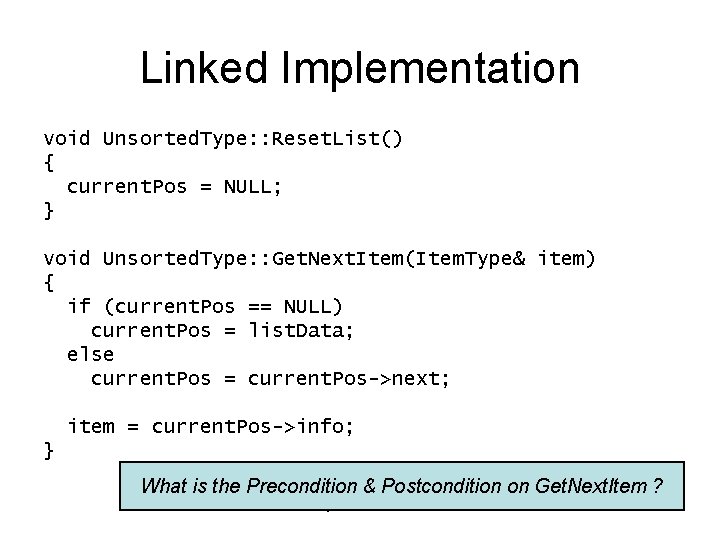
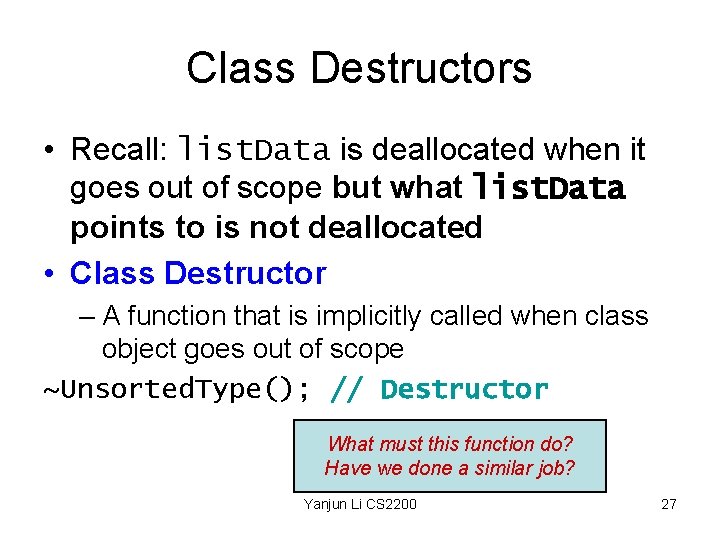
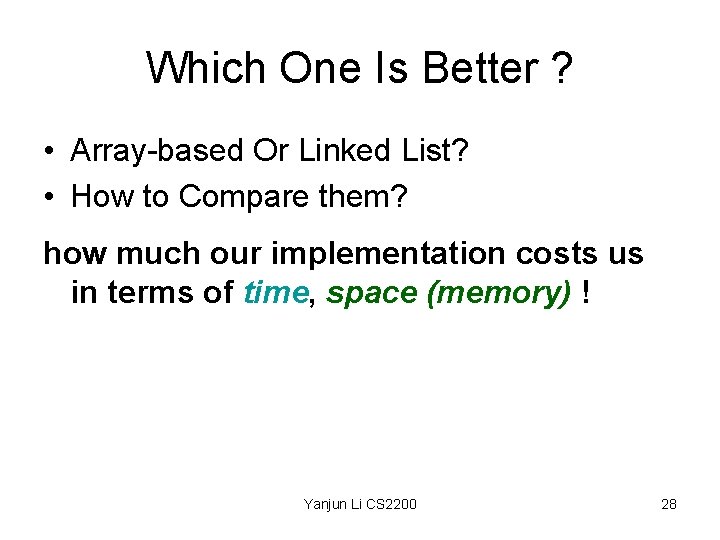
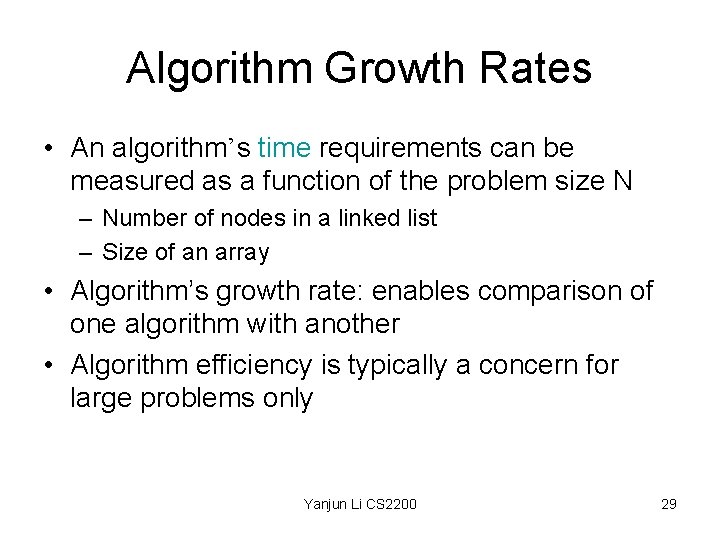
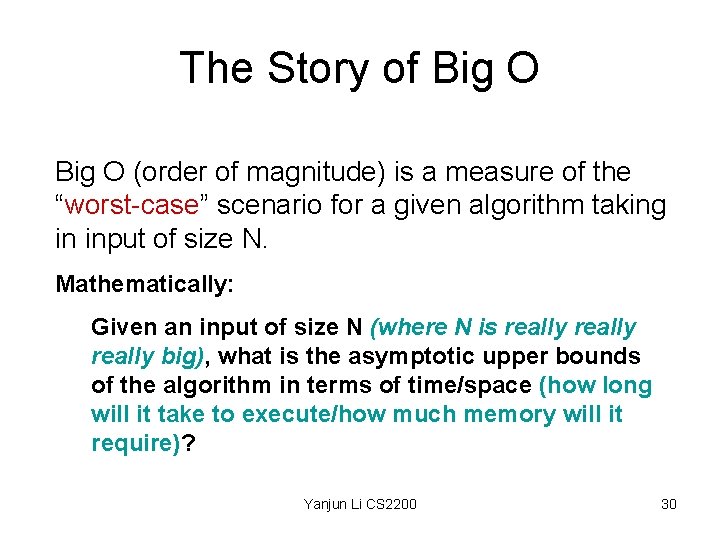
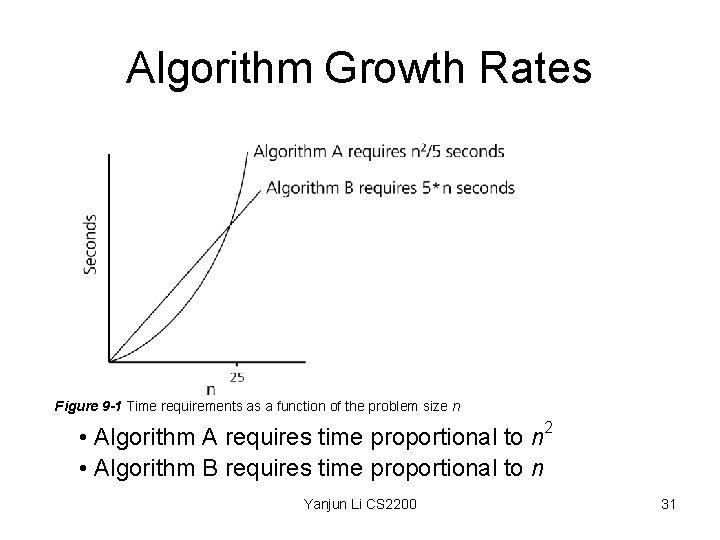
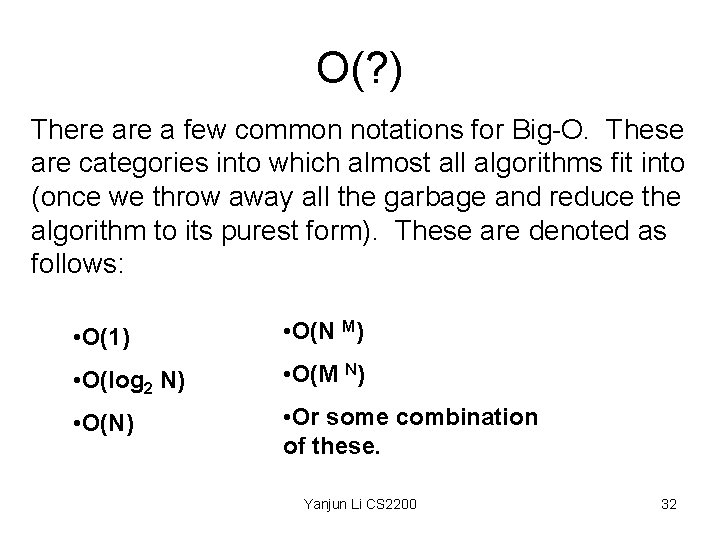
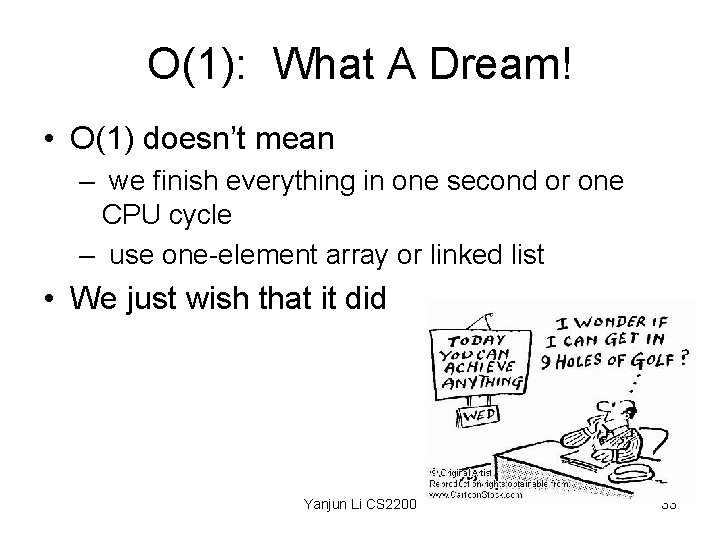
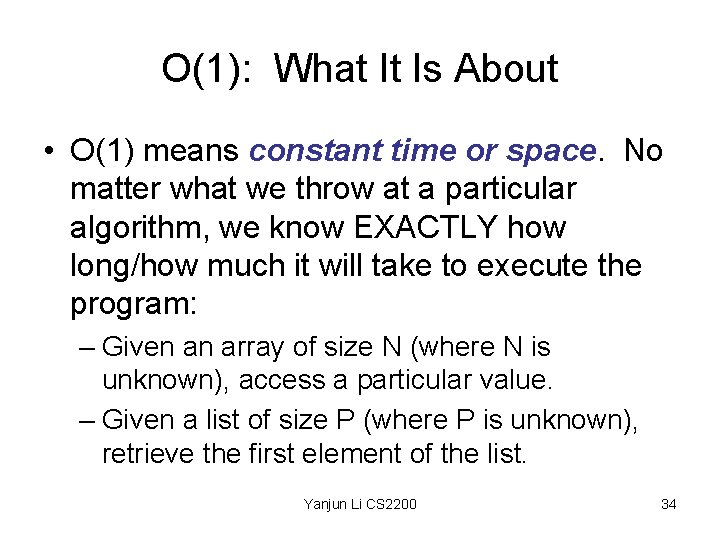
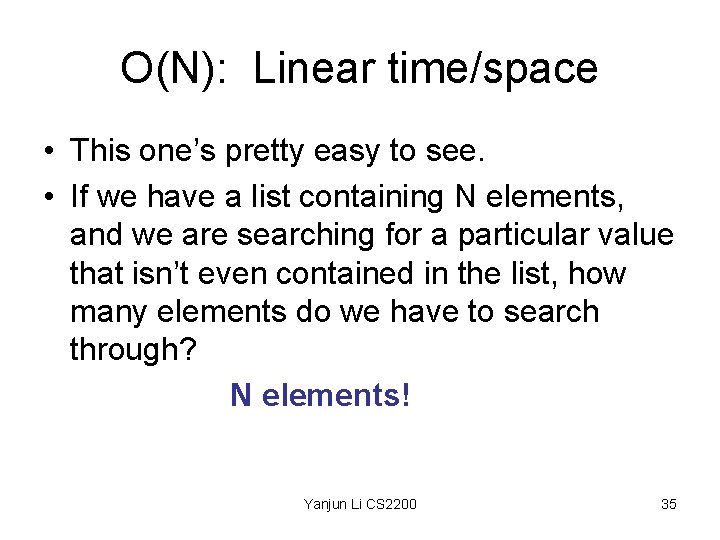
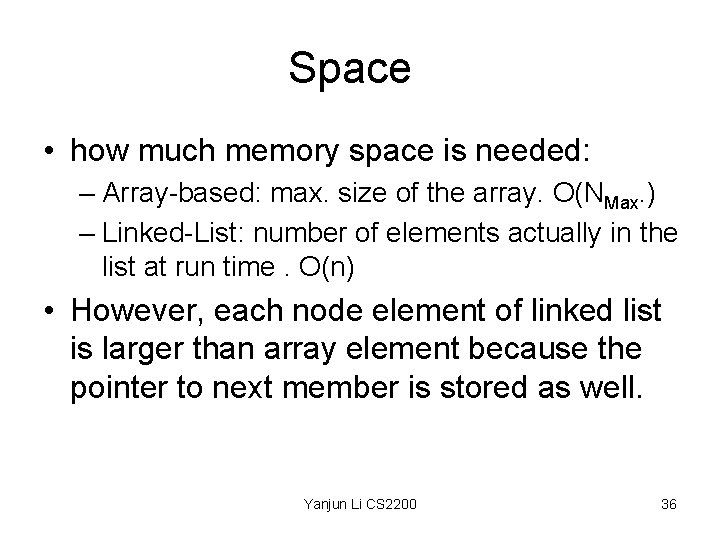
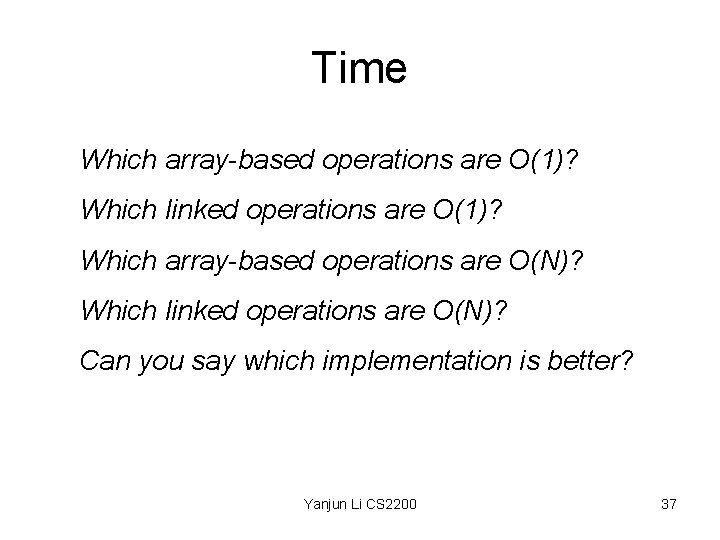
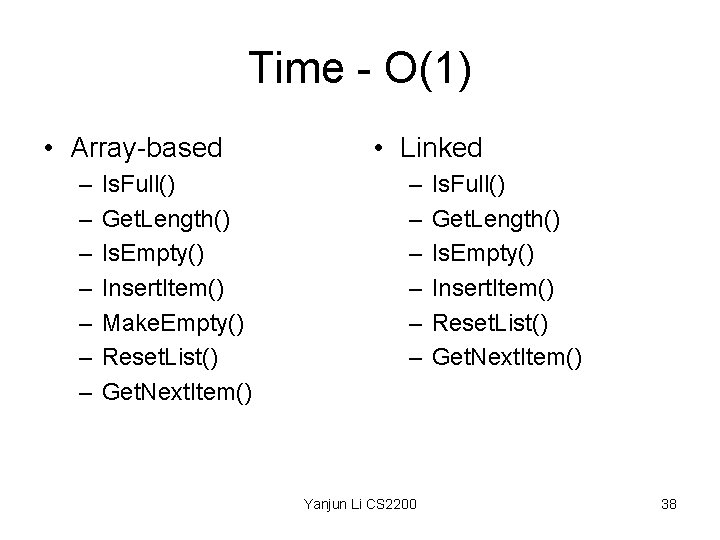
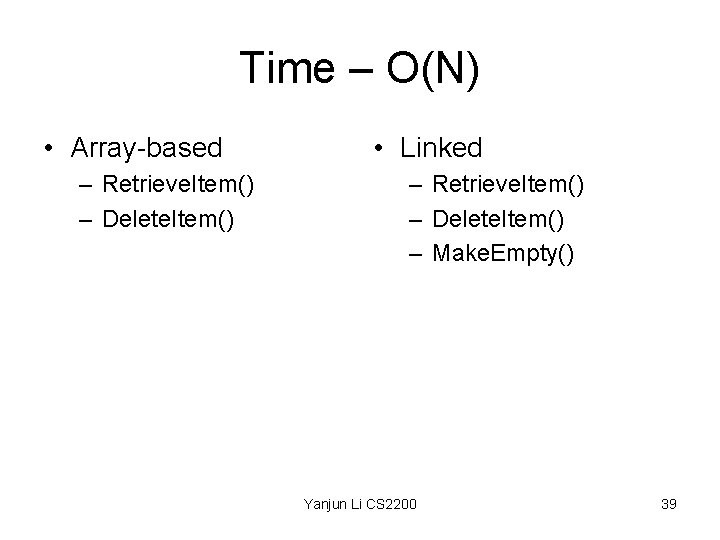
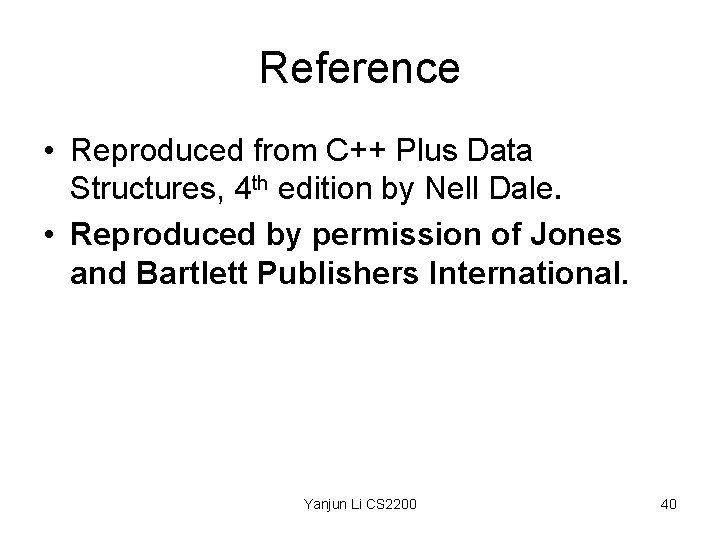
- Slides: 40
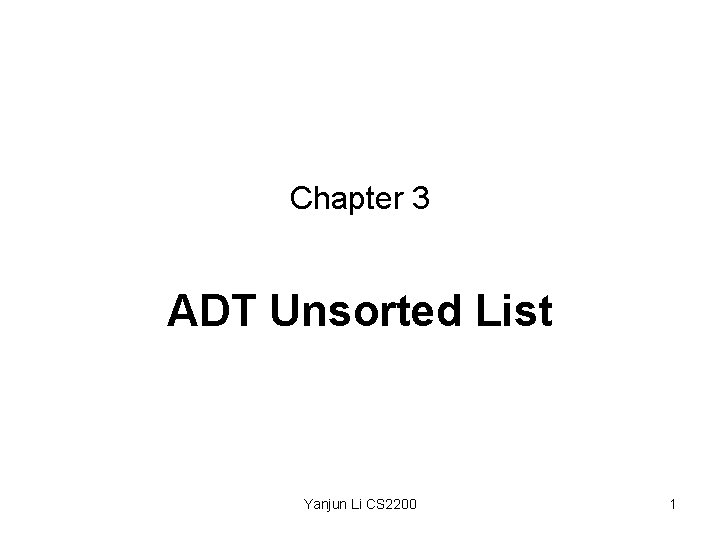
Chapter 3 ADT Unsorted List Yanjun Li CS 2200 1
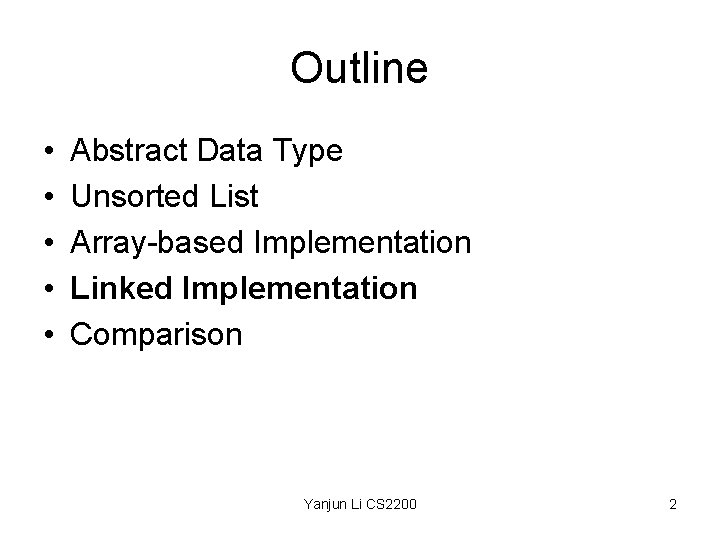
Outline • • • Abstract Data Type Unsorted List Array-based Implementation Linked Implementation Comparison Yanjun Li CS 2200 2
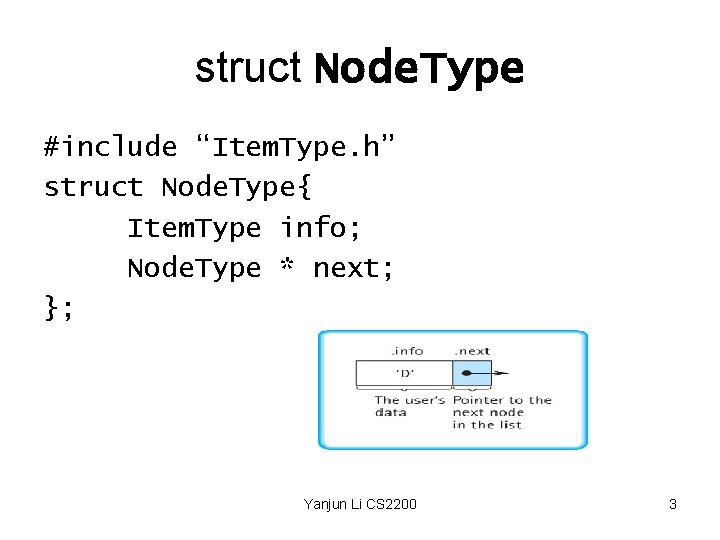
struct Node. Type #include “Item. Type. h” struct Node. Type{ Item. Type info; Node. Type * next; }; Yanjun Li CS 2200 3
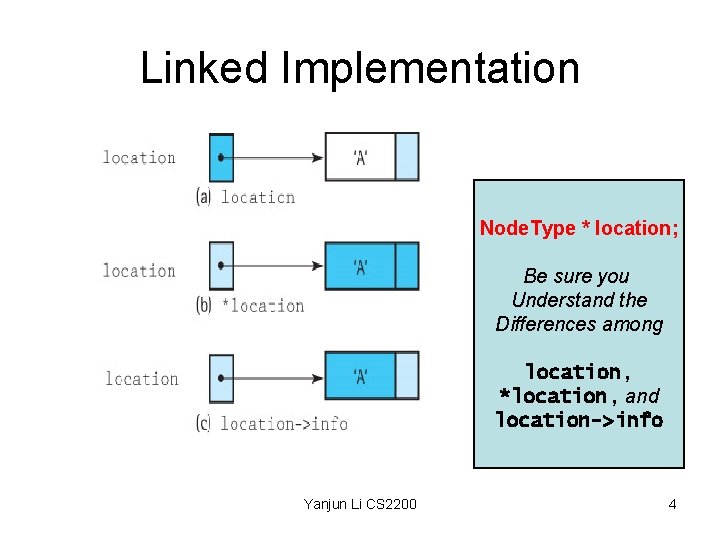
Linked Implementation Node. Type * location; Be sure you Understand the Differences among location, *location, and location->info Yanjun Li CS 2200 4
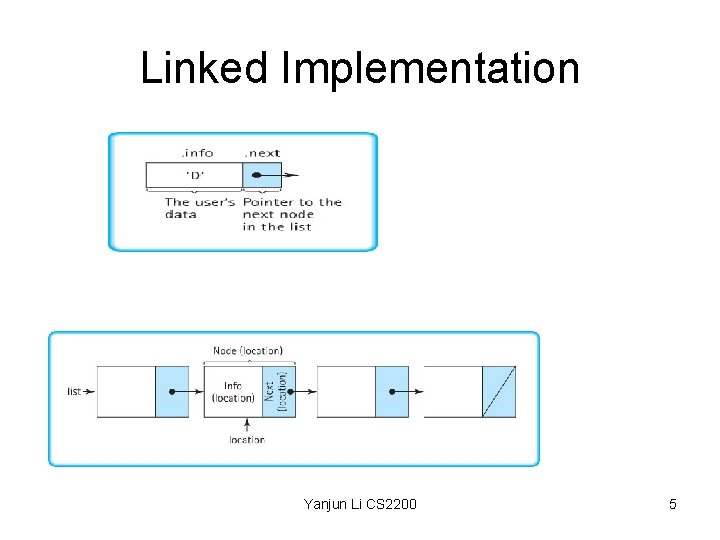
Linked Implementation Yanjun Li CS 2200 5
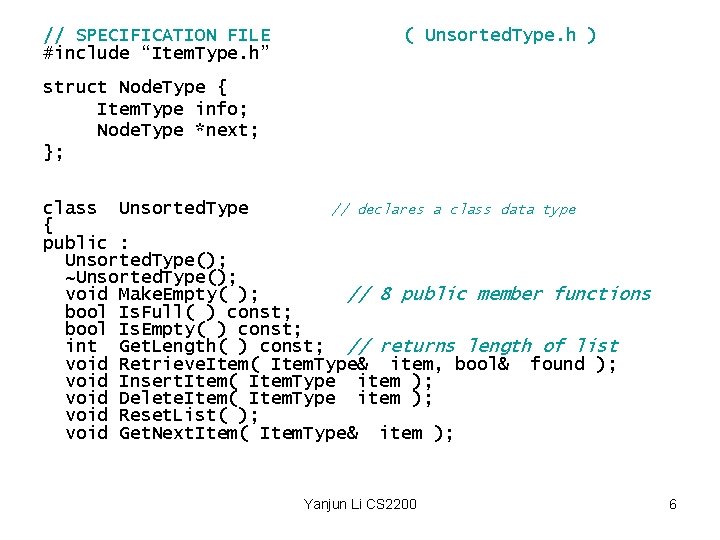
// SPECIFICATION FILE #include “Item. Type. h” ( Unsorted. Type. h ) struct Node. Type { Item. Type info; Node. Type *next; }; class Unsorted. Type // declares a class data type { public : Unsorted. Type(); ~Unsorted. Type(); void Make. Empty( ); // 8 public member functions bool Is. Full( ) const; bool Is. Empty( ) const; int Get. Length( ) const; // returns length of list void Retrieve. Item( Item. Type& item, bool& found ); void Insert. Item( Item. Type item ); void Delete. Item( Item. Type item ); void Reset. List( ); void Get. Next. Item( Item. Type& item ); Yanjun Li CS 2200 6
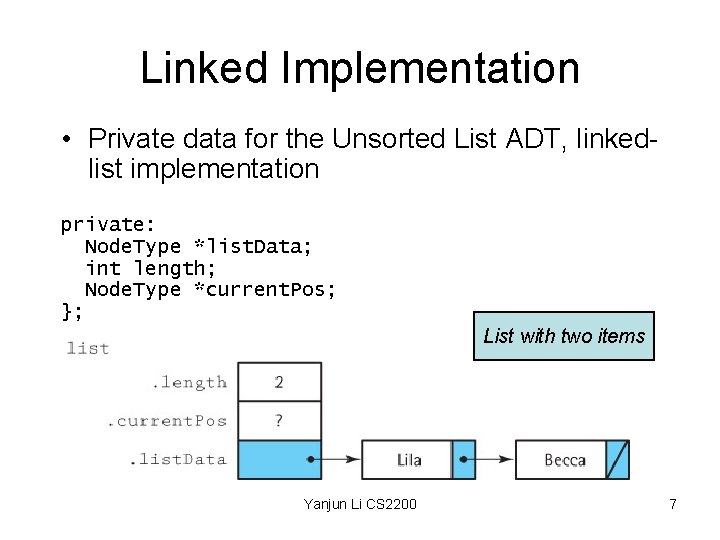
Linked Implementation • Private data for the Unsorted List ADT, linkedlist implementation private: Node. Type *list. Data; int length; Node. Type *current. Pos; }; List with two items Yanjun Li CS 2200 7
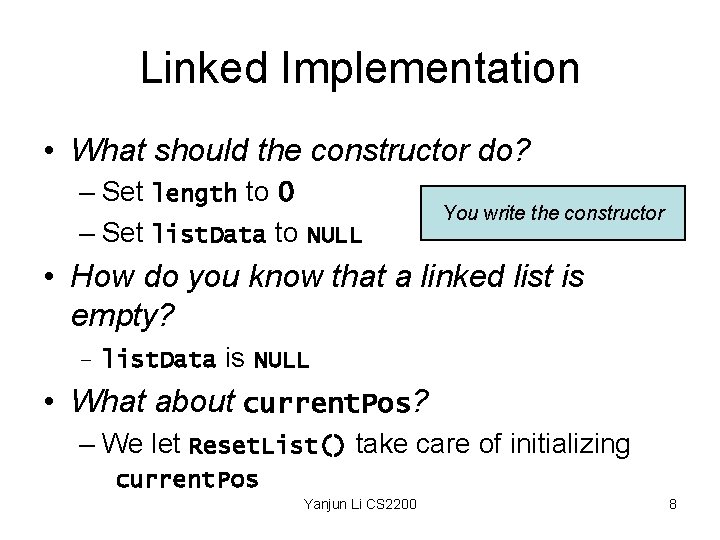
Linked Implementation • What should the constructor do? – Set length to 0 – Set list. Data to NULL You write the constructor • How do you know that a linked list is empty? – list. Data is NULL • What about current. Pos? – We let Reset. List() take care of initializing current. Pos Yanjun Li CS 2200 8
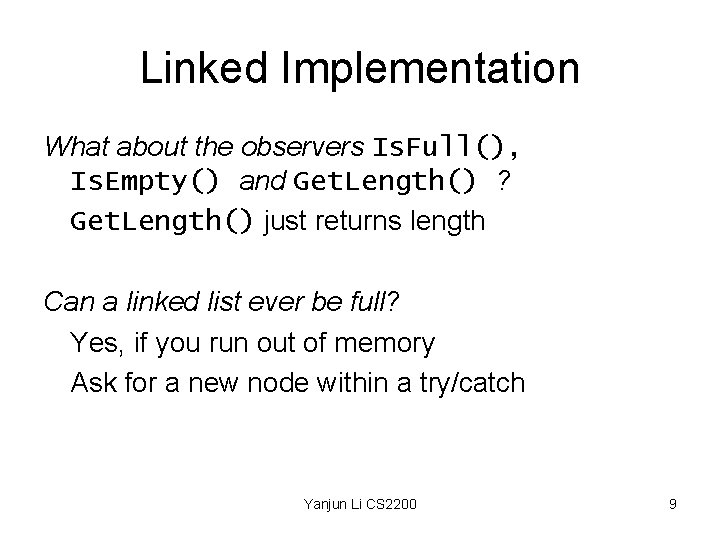
Linked Implementation What about the observers Is. Full(), Is. Empty() and Get. Length() ? Get. Length() just returns length Can a linked list ever be full? Yes, if you run out of memory Ask for a new node within a try/catch Yanjun Li CS 2200 9
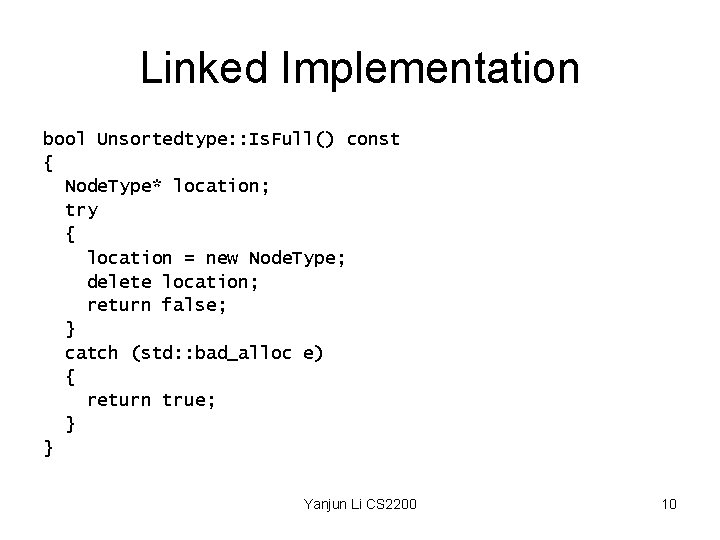
Linked Implementation bool Unsortedtype: : Is. Full() const { Node. Type* location; try { location = new Node. Type; delete location; return false; } catch (std: : bad_alloc e) { return true; } } Yanjun Li CS 2200 10
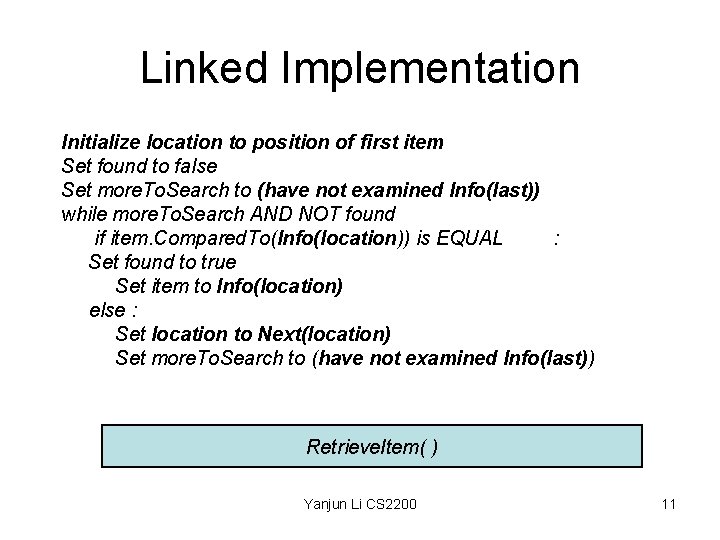
Linked Implementation Initialize location to position of first item Set found to false Set more. To. Search to (have not examined Info(last)) while more. To. Search AND NOT found if item. Compared. To(Info(location)) is EQUAL : Set found to true Set item to Info(location) else : Set location to Next(location) Set more. To. Search to (have not examined Info(last)) Retrieve. Item( ) Yanjun Li CS 2200 11
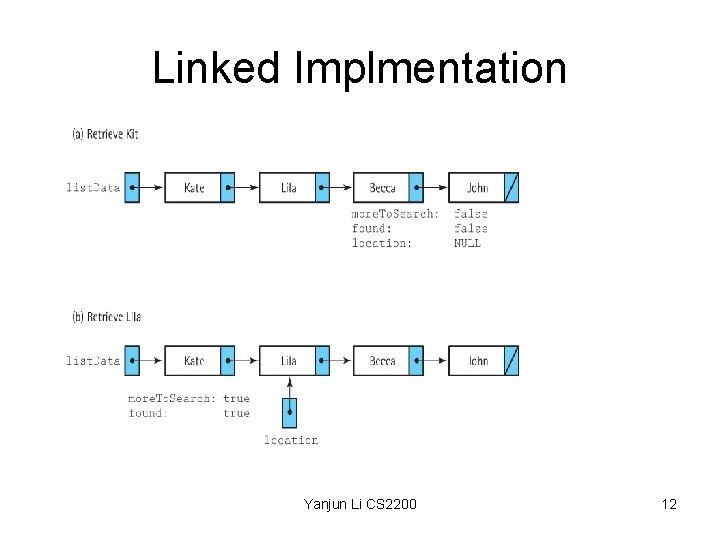
Linked Implmentation Yanjun Li CS 2200 12
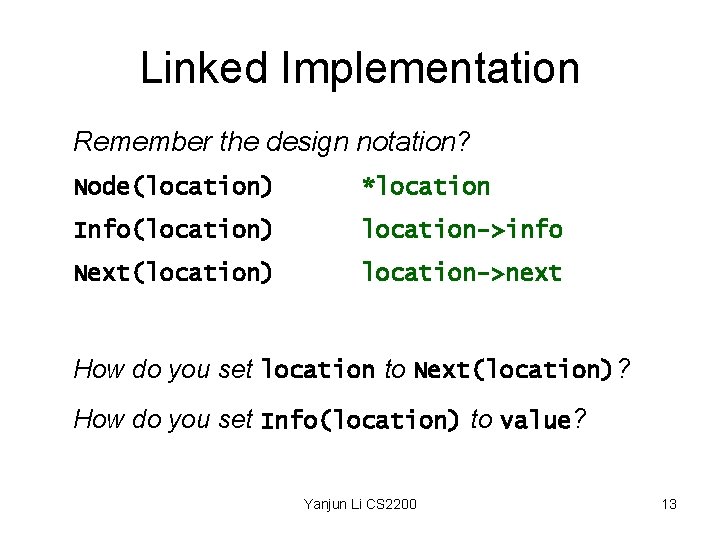
Linked Implementation Remember the design notation? Node(location) *location Info(location) location->info Next(location) location->next How do you set location to Next(location)? How do you set Info(location) to value? Yanjun Li CS 2200 13
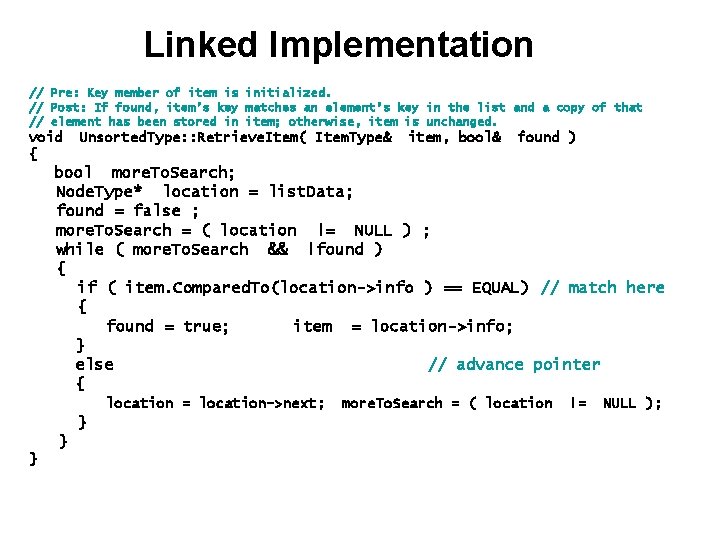
Linked Implementation // Pre: Key member of item is initialized. // Post: If found, item’s key matches an element’s key in the list and a copy of that // element has been stored in item; otherwise, item is unchanged. void { Unsorted. Type: : Retrieve. Item( Item. Type& item, bool& found ) bool more. To. Search; Node. Type* location = list. Data; found = false ; more. To. Search = ( location != NULL ) ; while ( more. To. Search && !found ) { if ( item. Compared. To(location->info ) == EQUAL) // match here { found = true; item = location->info; } else // advance pointer { location = location->next; more. To. Search = ( location != NULL ); } } } Yanjun Li CS 2200 14
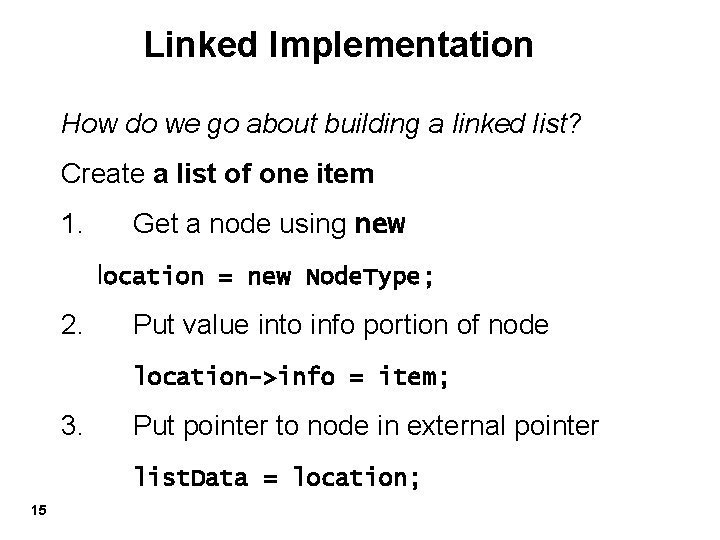
Linked Implementation How do we go about building a linked list? Create a list of one item 1. Get a node using new location = new Node. Type; 2. Put value into info portion of node location->info = item; 3. Put pointer to node in external pointer list. Data = location; 15 Yanjun Li CS 2200 15
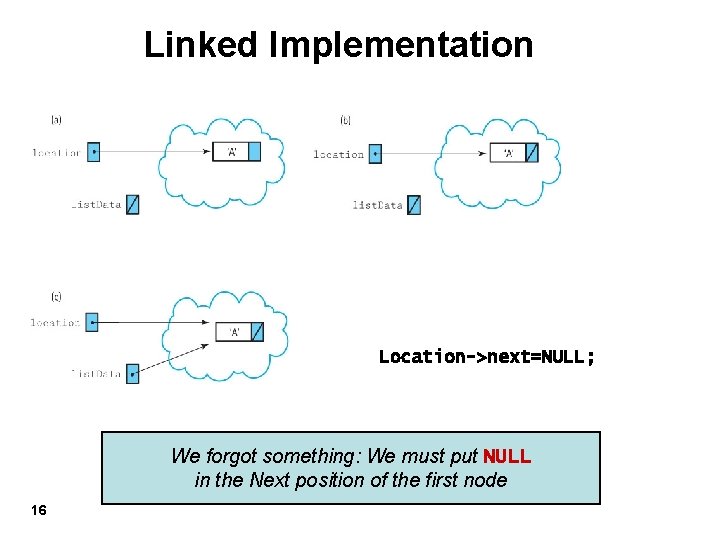
Linked Implementation Location->next=NULL; We forgot something: We must put NULL in the Next position of the first node 16 Yanjun Li CS 2200 16
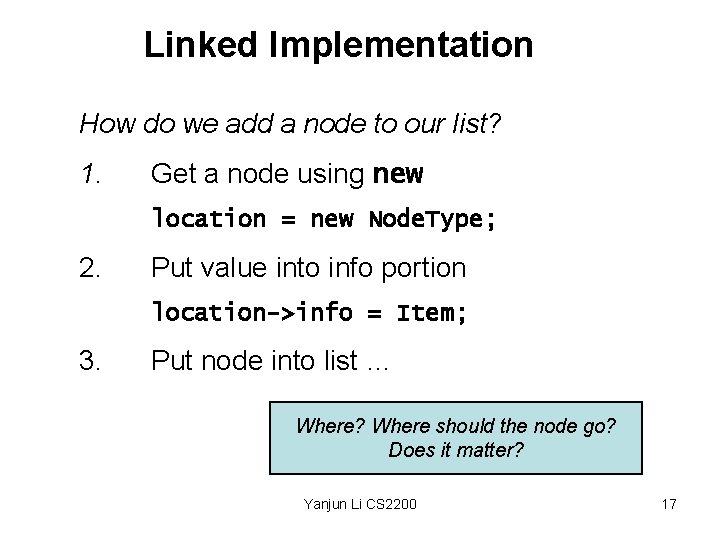
Linked Implementation How do we add a node to our list? 1. Get a node using new location = new Node. Type; 2. Put value into info portion location->info = Item; 3. Put node into list … Where? Where should the node go? Does it matter? Yanjun Li CS 2200 17
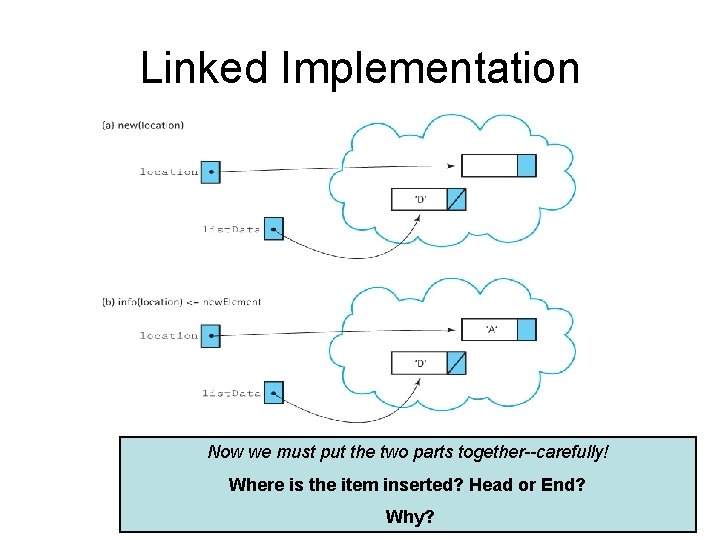
Linked Implementation Now we must put the two parts together--carefully! Where is the item inserted? Head or End? Yanjun Li CS 2200 Why? 18
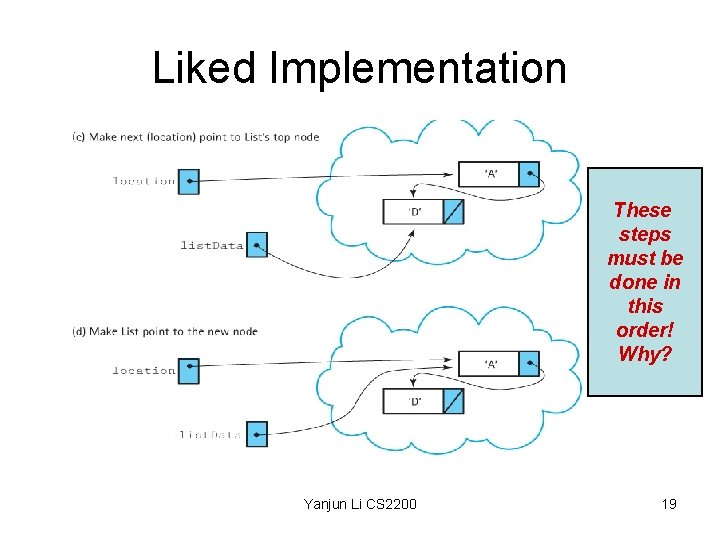
Liked Implementation These steps must be done in this order! Why? Yanjun Li CS 2200 19
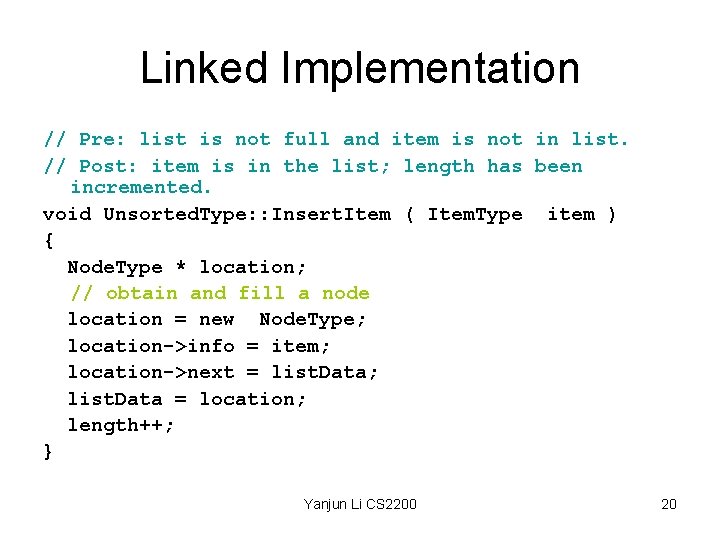
Linked Implementation // Pre: list is not full and item is not in list. // Post: item is in the list; length has been incremented. void Unsorted. Type: : Insert. Item ( Item. Type item ) { Node. Type * location; // obtain and fill a node location = new Node. Type; location->info = item; location->next = list. Data; list. Data = location; length++; } Yanjun Li CS 2200 20
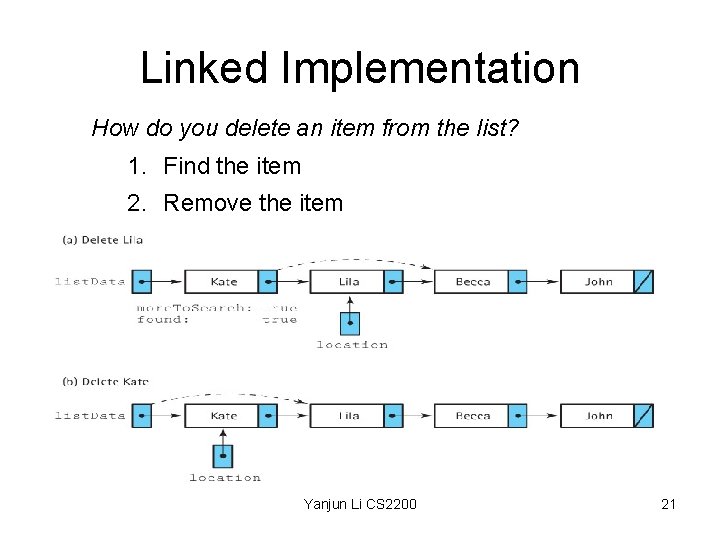
Linked Implementation How do you delete an item from the list? 1. Find the item 2. Remove the item Yanjun Li CS 2200 21
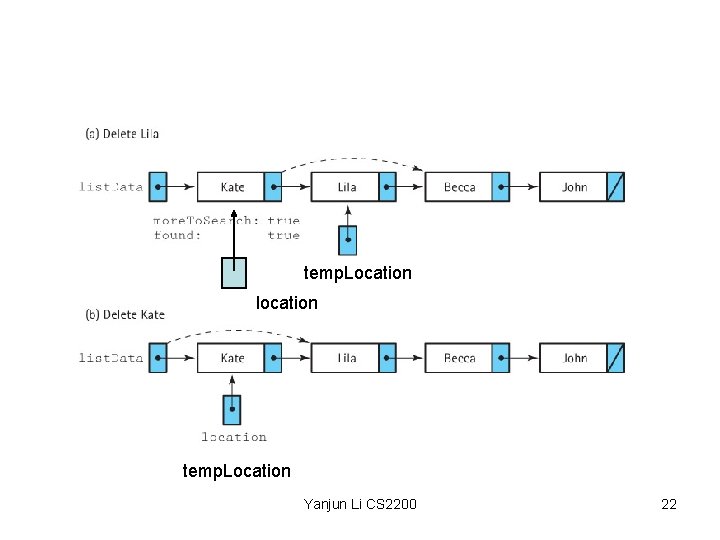
temp. Location location temp. Location Yanjun Li CS 2200 22
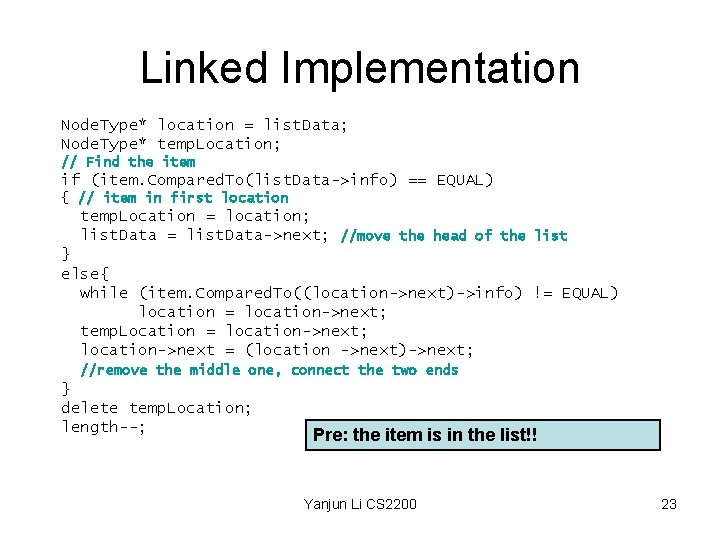
Linked Implementation Node. Type* location = list. Data; Node. Type* temp. Location; // Find the item if (item. Compared. To(list. Data->info) == EQUAL) { // item in first location temp. Location = location; list. Data = list. Data->next; //move the head of the list } else{ while (item. Compared. To((location->next)->info) != EQUAL) location = location->next; temp. Location = location->next; location->next = (location ->next)->next; //remove the middle one, connect the two ends } delete temp. Location; length--; Pre: the item is in the list!! Yanjun Li CS 2200 23
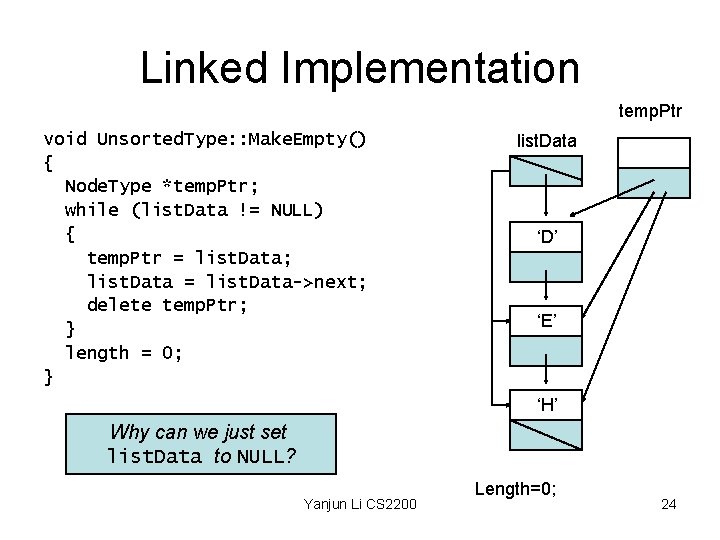
Linked Implementation temp. Ptr void Unsorted. Type: : Make. Empty() { Node. Type *temp. Ptr; while (list. Data != NULL) { temp. Ptr = list. Data; list. Data = list. Data->next; delete temp. Ptr; } length = 0; } list. Data ‘D’ ‘E’ ‘H’ Why can we just set list. Data to NULL? Yanjun Li CS 2200 Length=0; 24
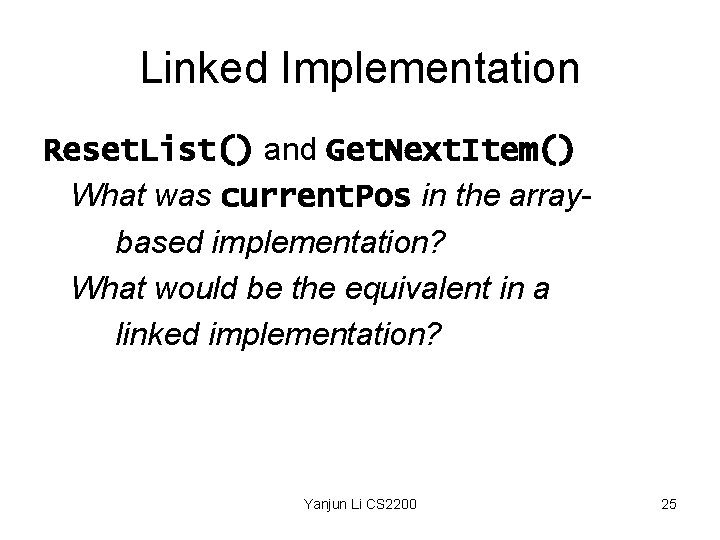
Linked Implementation Reset. List() and Get. Next. Item() What was current. Pos in the arraybased implementation? What would be the equivalent in a linked implementation? Yanjun Li CS 2200 25
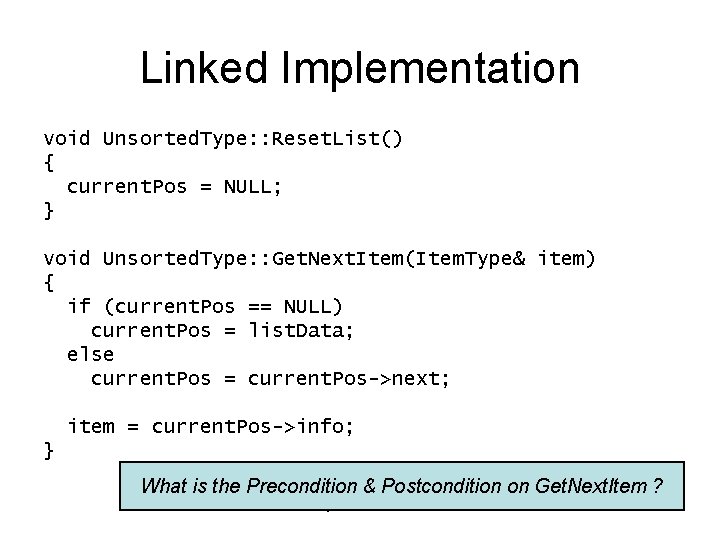
Linked Implementation void Unsorted. Type: : Reset. List() { current. Pos = NULL; } void Unsorted. Type: : Get. Next. Item(Item. Type& item) { if (current. Pos == NULL) current. Pos = list. Data; else current. Pos = current. Pos->next; item = current. Pos->info; } What is the Precondition & Postcondition on Get. Next. Item ? Yanjun Li CS 2200 26
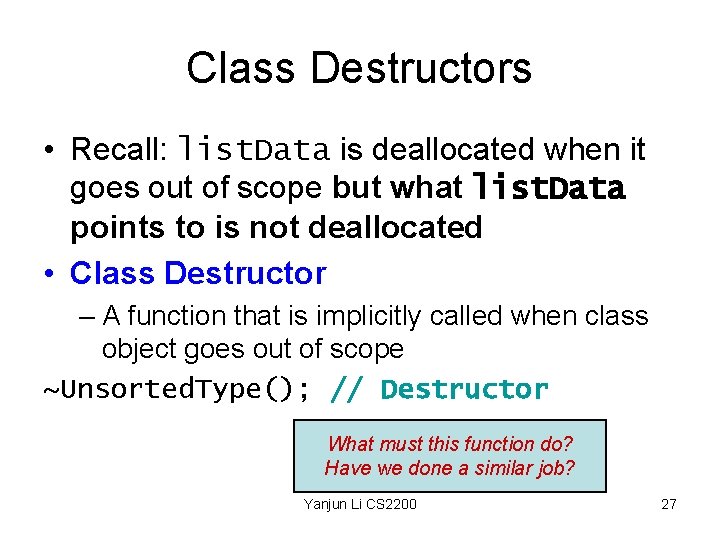
Class Destructors • Recall: list. Data is deallocated when it goes out of scope but what list. Data points to is not deallocated • Class Destructor – A function that is implicitly called when class object goes out of scope ~Unsorted. Type(); // Destructor What must this function do? Have we done a similar job? Yanjun Li CS 2200 27
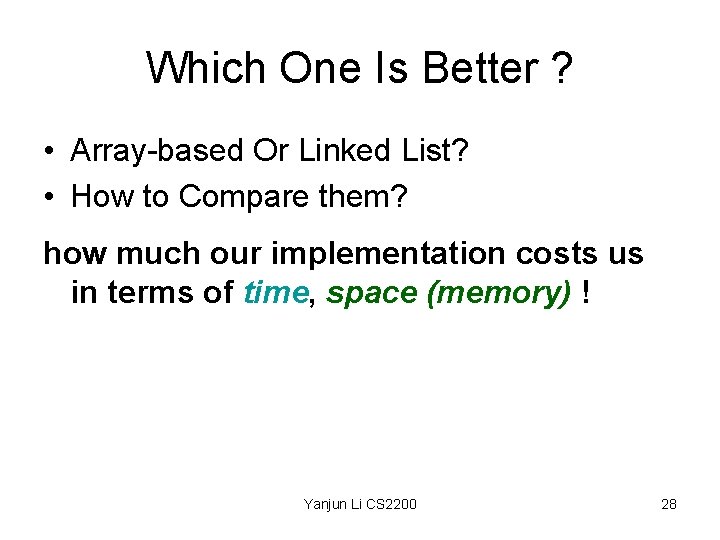
Which One Is Better ? • Array-based Or Linked List? • How to Compare them? how much our implementation costs us in terms of time, space (memory) ! Yanjun Li CS 2200 28
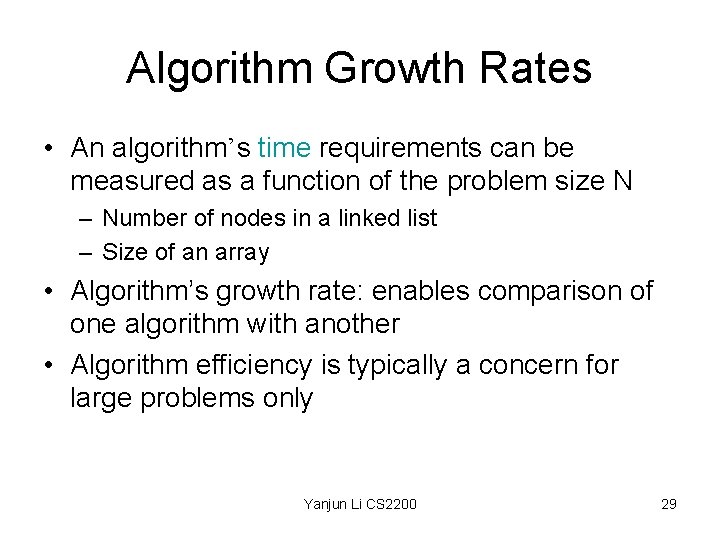
Algorithm Growth Rates • An algorithm’s time requirements can be measured as a function of the problem size N – Number of nodes in a linked list – Size of an array • Algorithm’s growth rate: enables comparison of one algorithm with another • Algorithm efficiency is typically a concern for large problems only Yanjun Li CS 2200 29
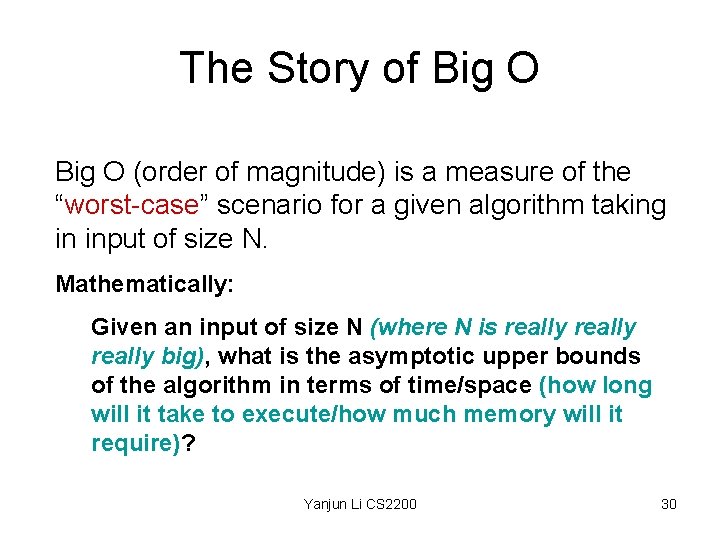
The Story of Big O (order of magnitude) is a measure of the “worst-case” scenario for a given algorithm taking in input of size N. Mathematically: Given an input of size N (where N is really big), what is the asymptotic upper bounds of the algorithm in terms of time/space (how long will it take to execute/how much memory will it require)? Yanjun Li CS 2200 30
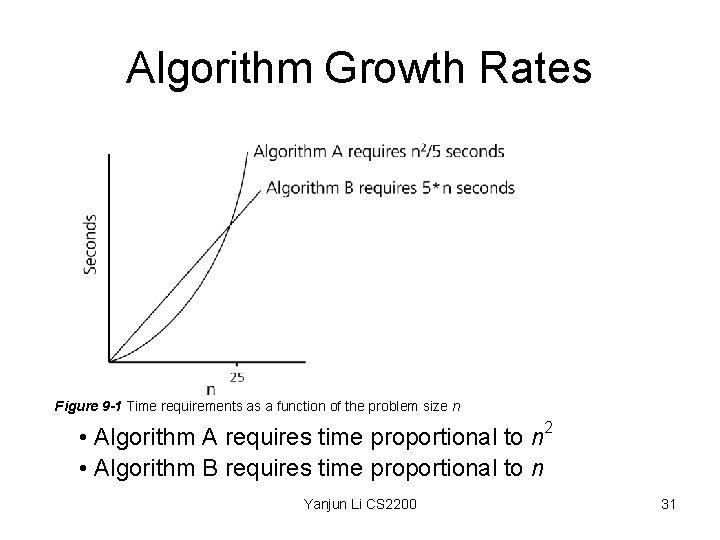
Algorithm Growth Rates Figure 9 -1 Time requirements as a function of the problem size n • Algorithm A requires time proportional to n 2 • Algorithm B requires time proportional to n Yanjun Li CS 2200 31
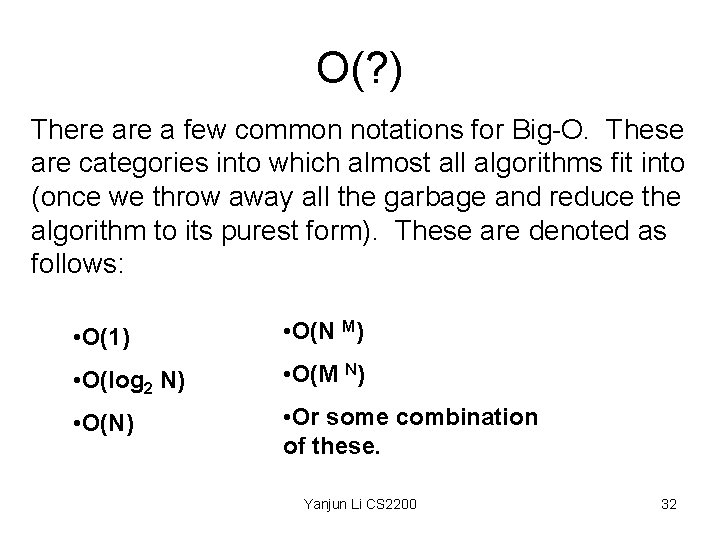
O(? ) There a few common notations for Big-O. These are categories into which almost all algorithms fit into (once we throw away all the garbage and reduce the algorithm to its purest form). These are denoted as follows: • O(1) • O(N M) • O(log 2 N) • O(M N) • O(N) • Or some combination of these. Yanjun Li CS 2200 32
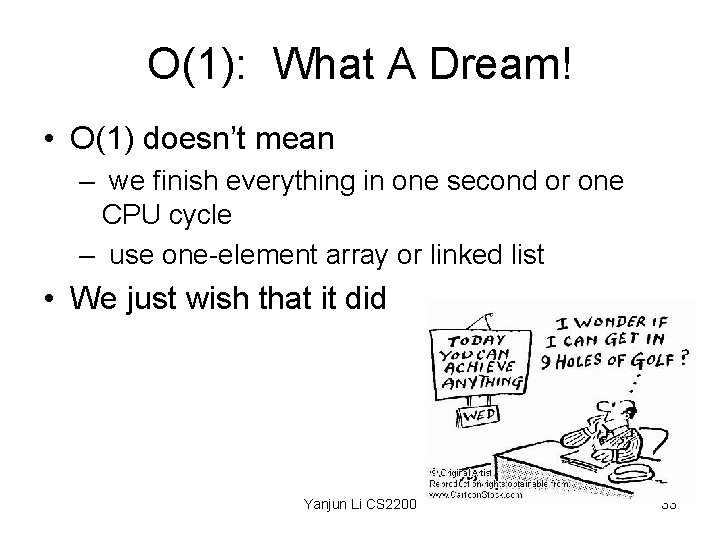
O(1): What A Dream! • O(1) doesn’t mean – we finish everything in one second or one CPU cycle – use one-element array or linked list • We just wish that it did Yanjun Li CS 2200 33
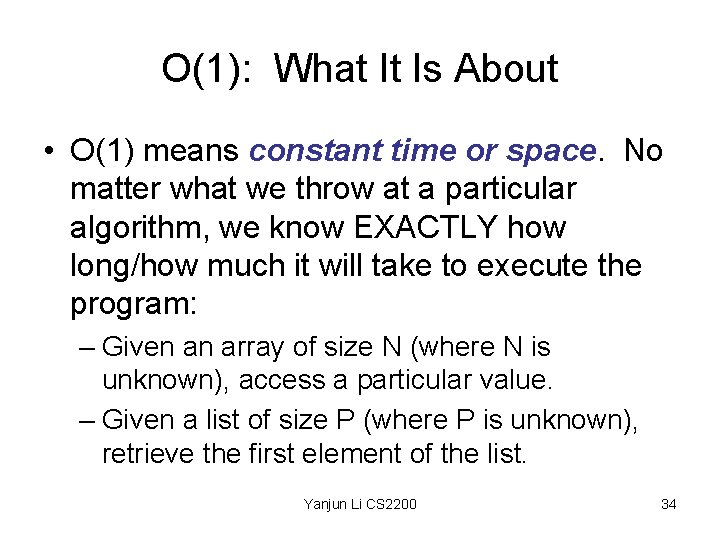
O(1): What It Is About • O(1) means constant time or space. No matter what we throw at a particular algorithm, we know EXACTLY how long/how much it will take to execute the program: – Given an array of size N (where N is unknown), access a particular value. – Given a list of size P (where P is unknown), retrieve the first element of the list. Yanjun Li CS 2200 34
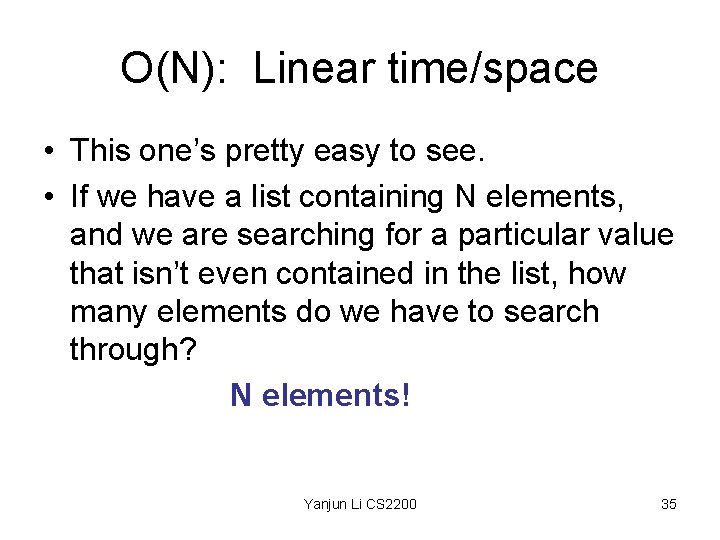
O(N): Linear time/space • This one’s pretty easy to see. • If we have a list containing N elements, and we are searching for a particular value that isn’t even contained in the list, how many elements do we have to search through? N elements! Yanjun Li CS 2200 35
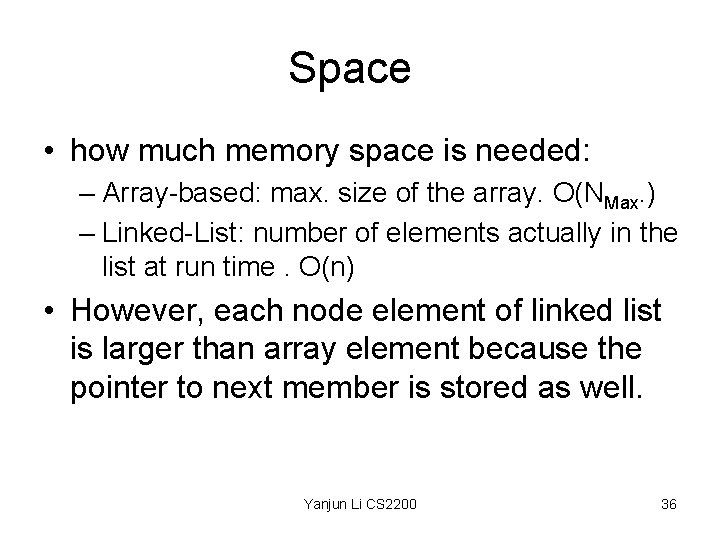
Space • how much memory space is needed: – Array-based: max. size of the array. O(NMax. ) – Linked-List: number of elements actually in the list at run time. O(n) • However, each node element of linked list is larger than array element because the pointer to next member is stored as well. Yanjun Li CS 2200 36
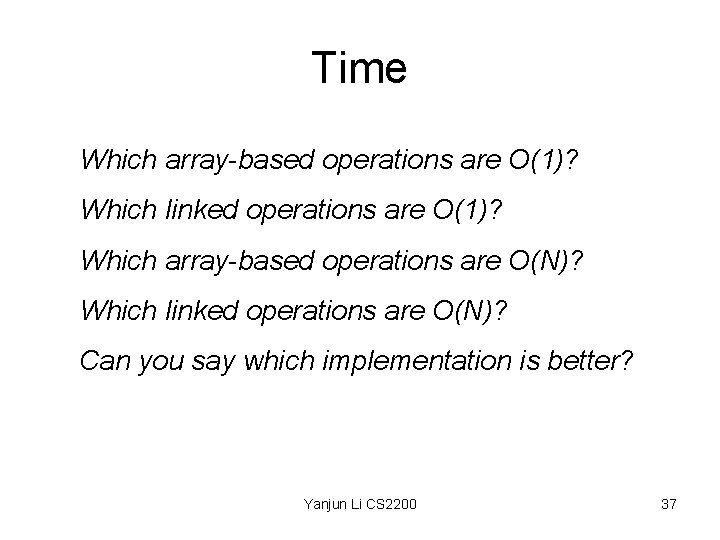
Time Which array-based operations are O(1)? Which linked operations are O(1)? Which array-based operations are O(N)? Which linked operations are O(N)? Can you say which implementation is better? Yanjun Li CS 2200 37
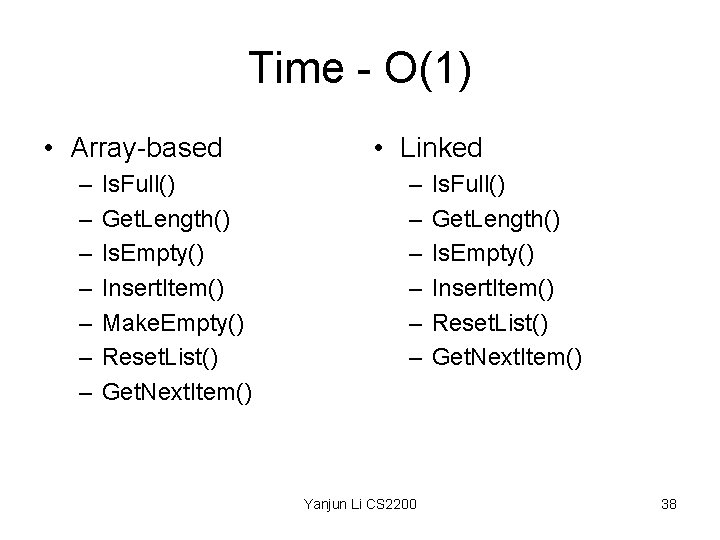
Time - O(1) • Array-based – – – – Is. Full() Get. Length() Is. Empty() Insert. Item() Make. Empty() Reset. List() Get. Next. Item() • Linked – – – Yanjun Li CS 2200 Is. Full() Get. Length() Is. Empty() Insert. Item() Reset. List() Get. Next. Item() 38
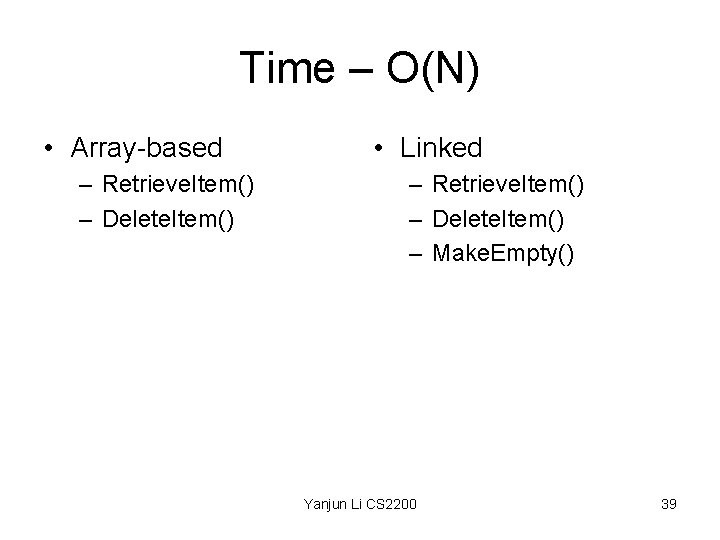
Time – O(N) • Array-based – Retrieve. Item() – Delete. Item() • Linked – Retrieve. Item() – Delete. Item() – Make. Empty() Yanjun Li CS 2200 39
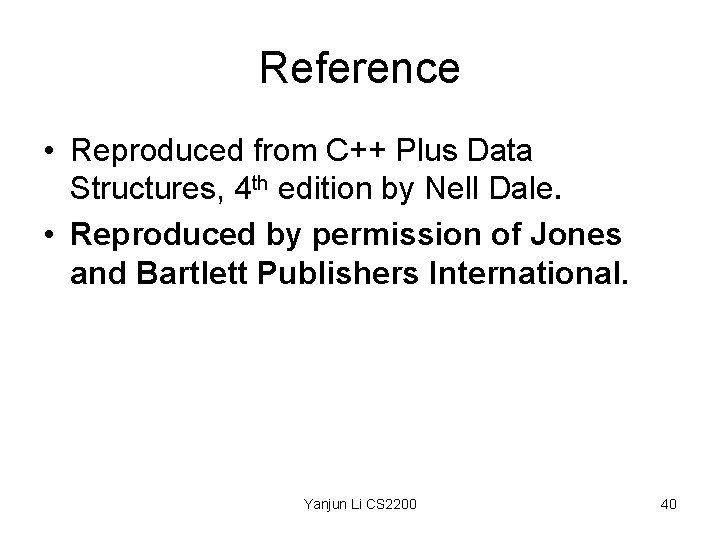
Reference • Reproduced from C++ Plus Data Structures, 4 th edition by Nell Dale. • Reproduced by permission of Jones and Bartlett Publishers International. Yanjun Li CS 2200 40