Chapter 21 Once Is Not Enough Iteration Principles
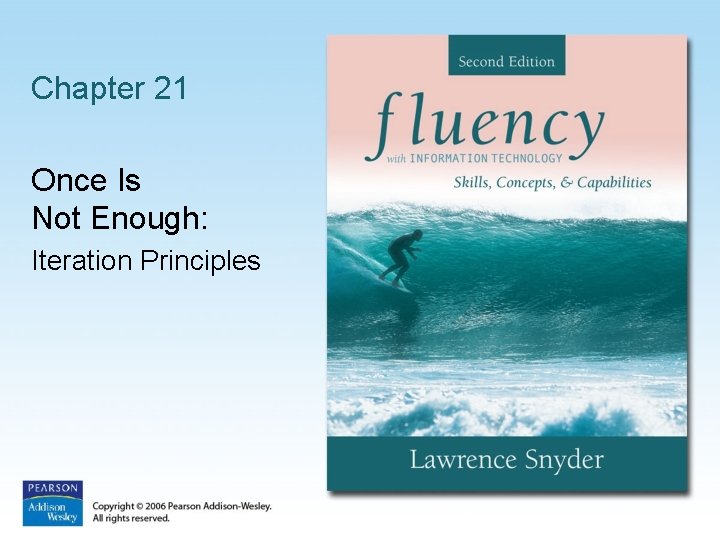
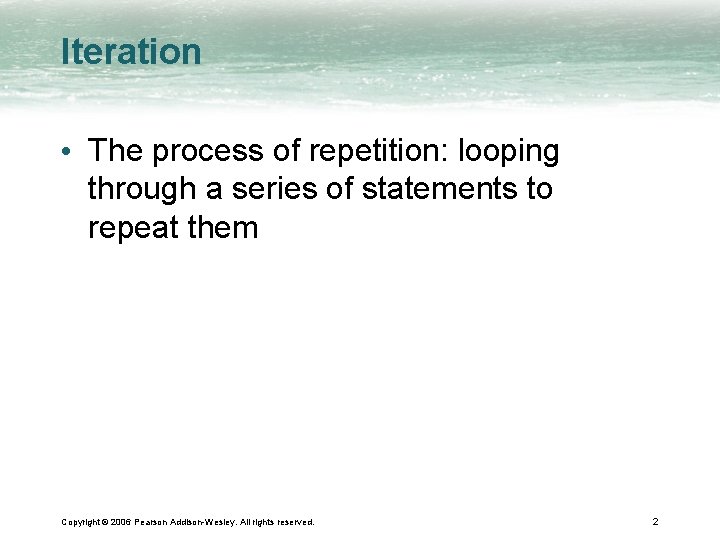
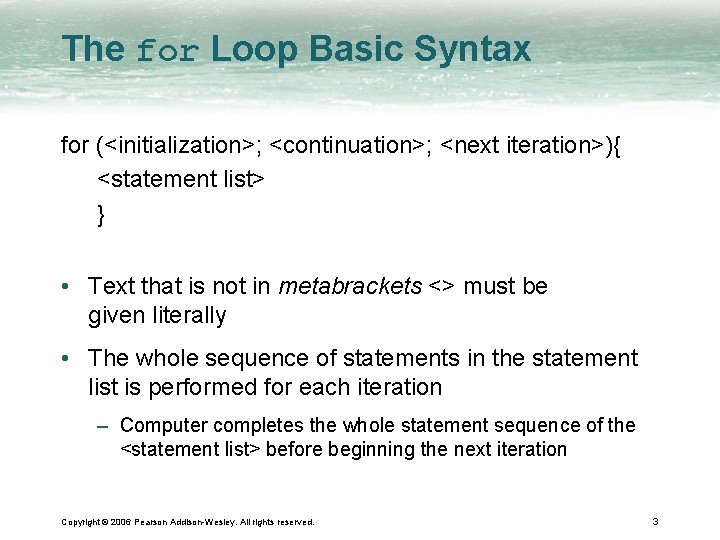
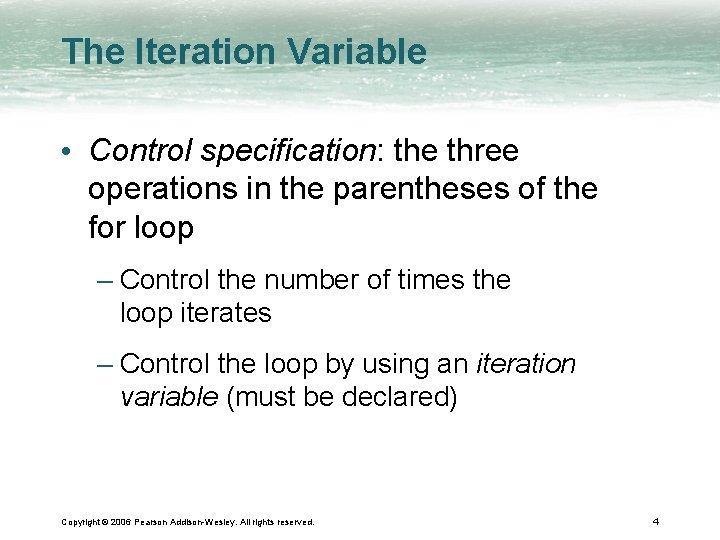
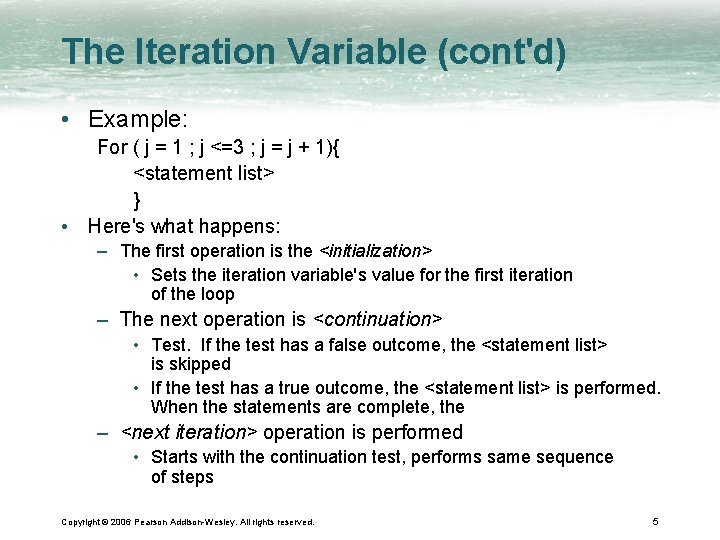
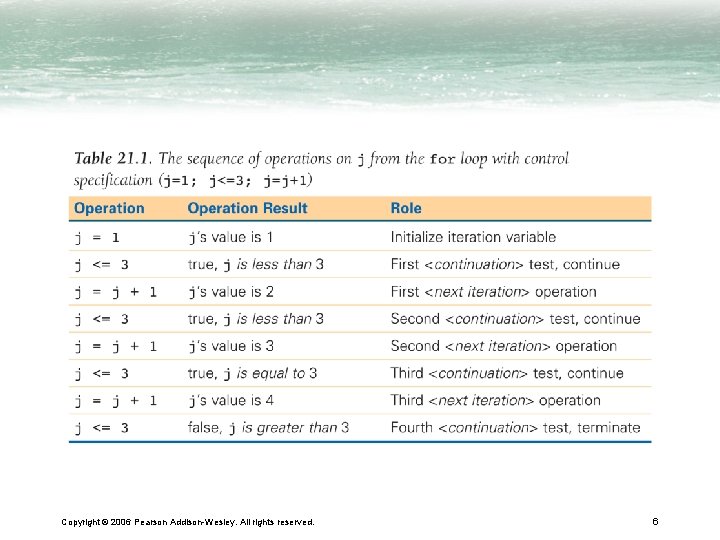
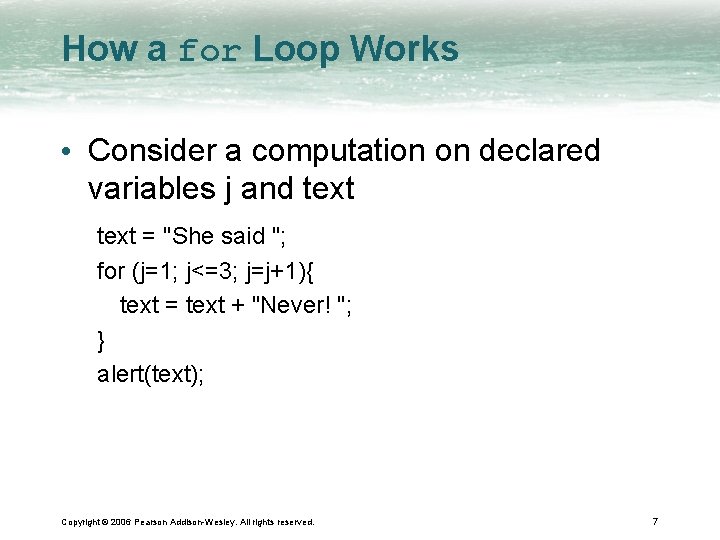
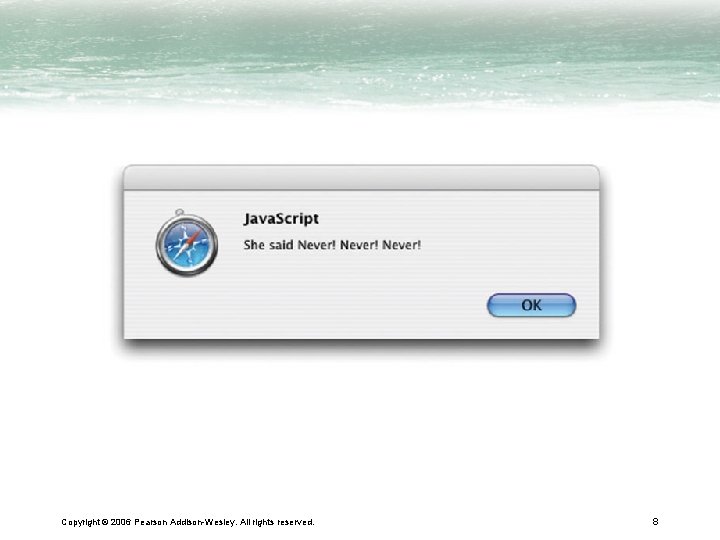
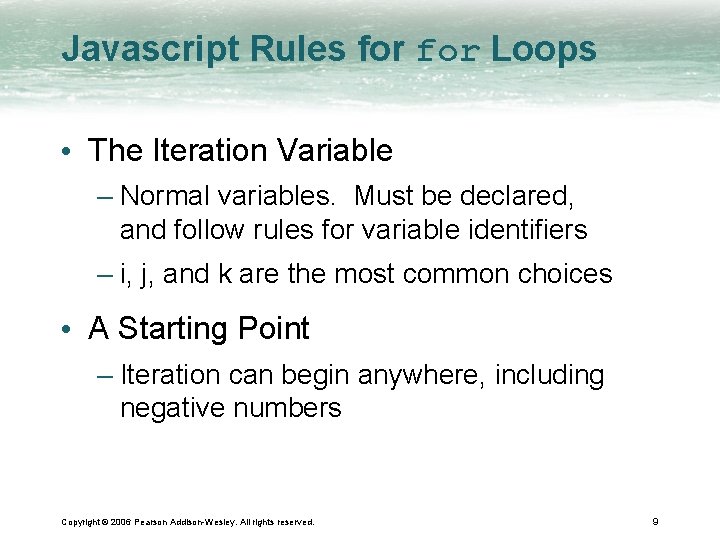
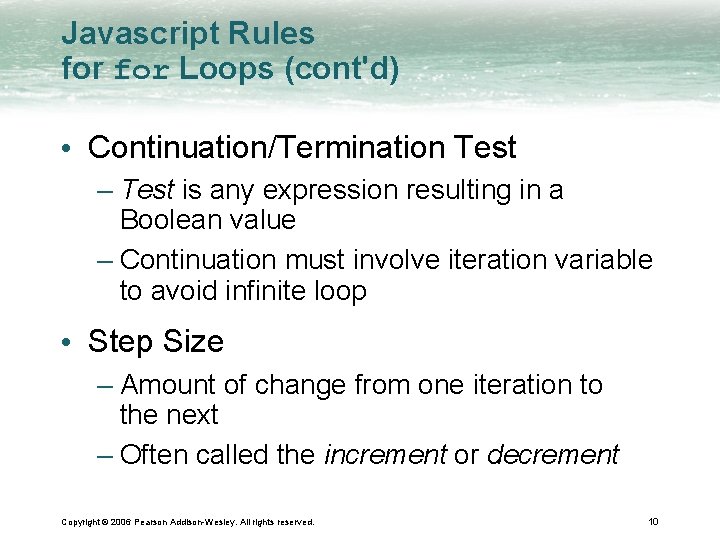
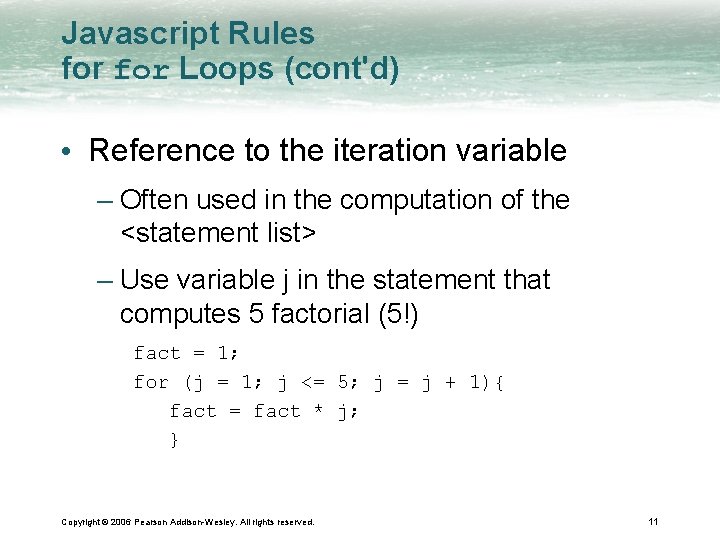
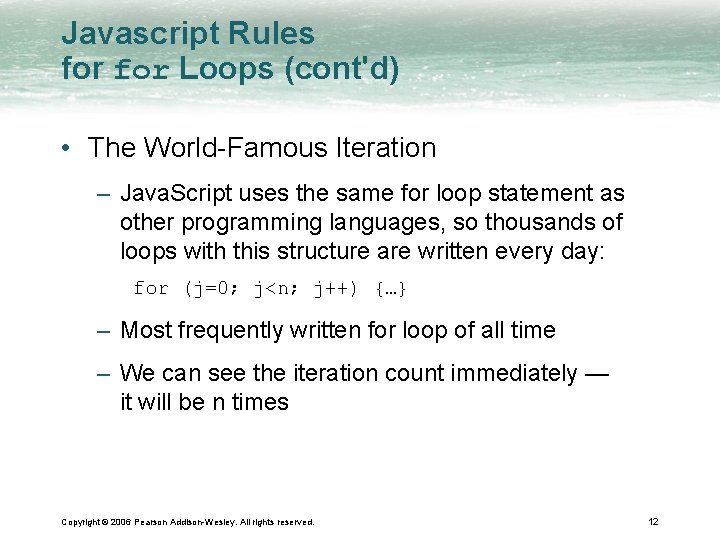
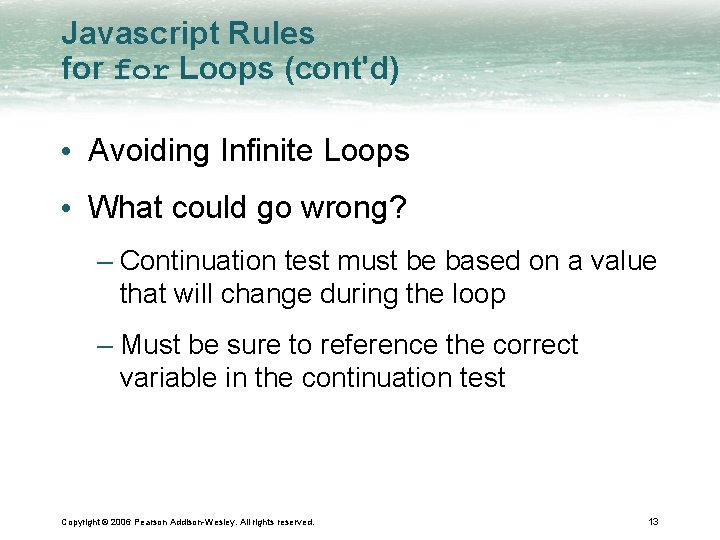
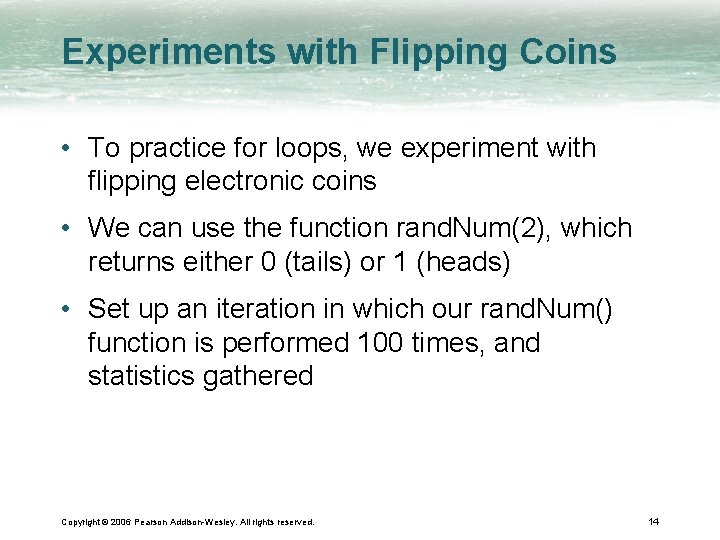
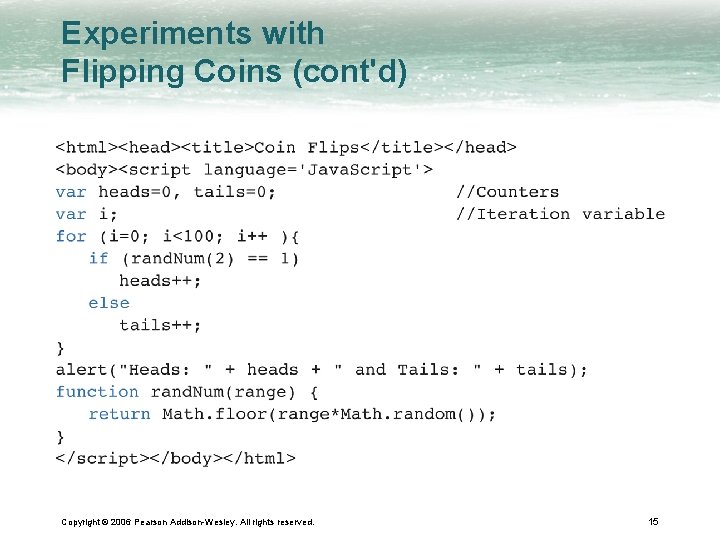
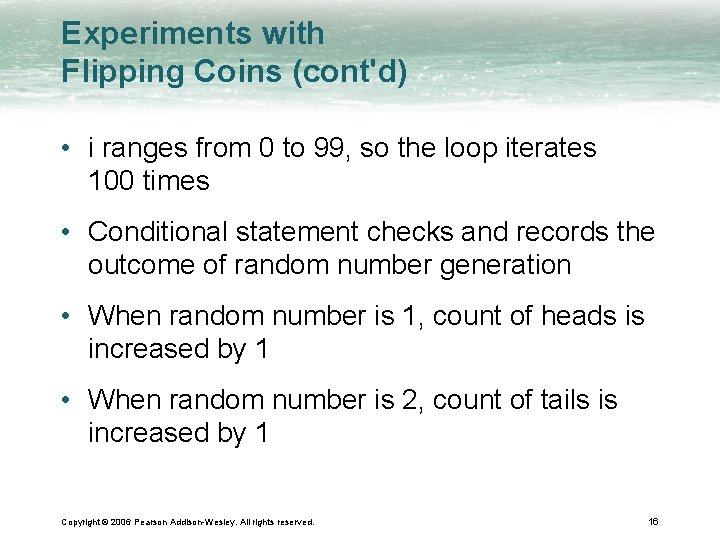
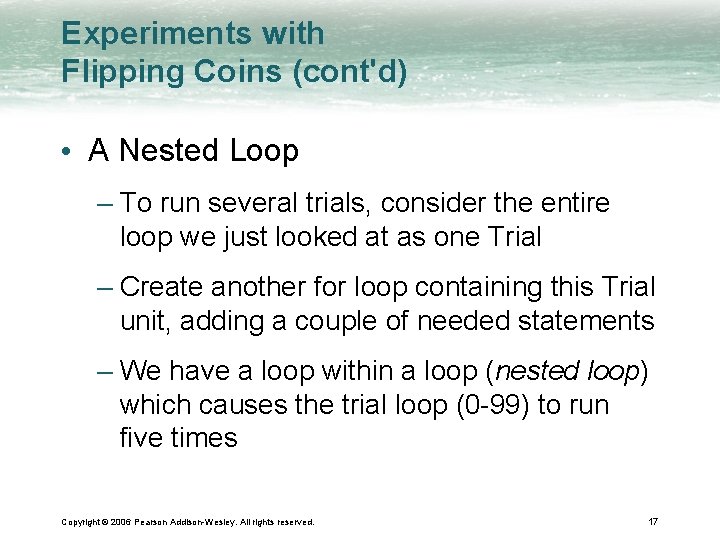
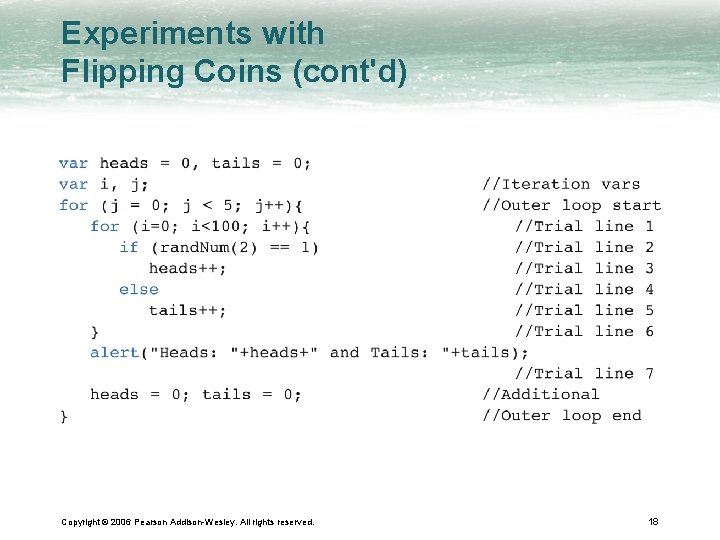
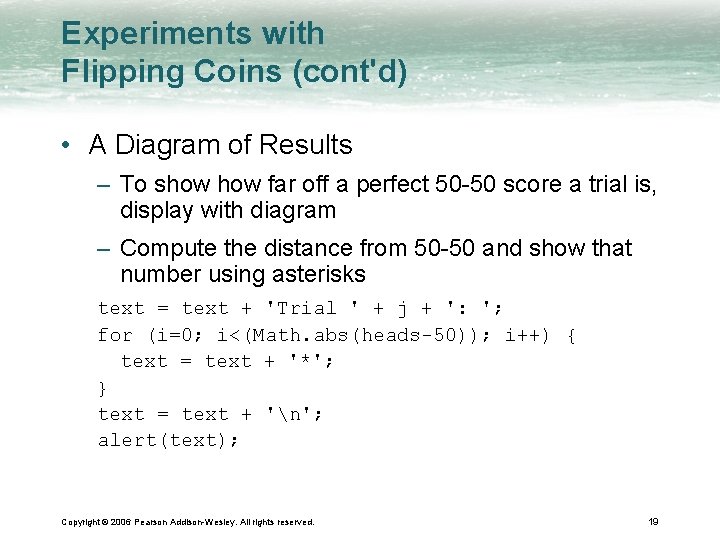
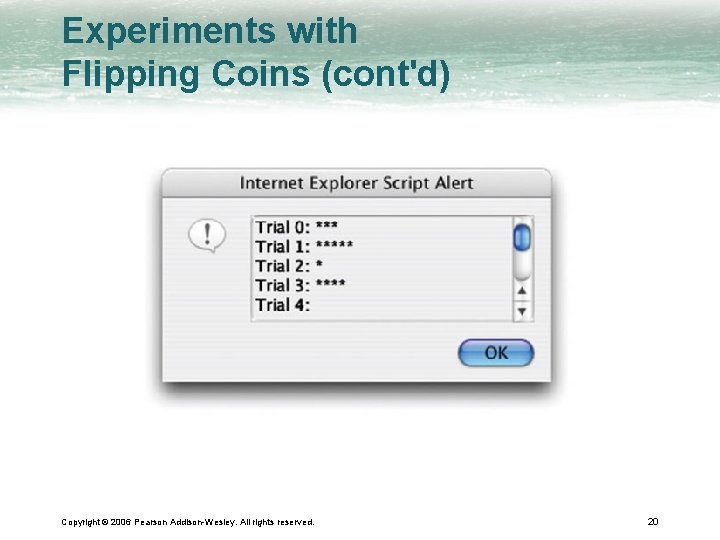
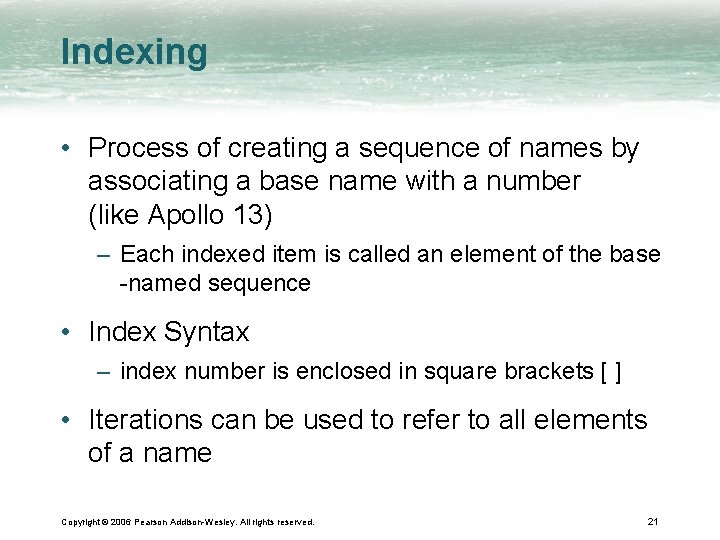
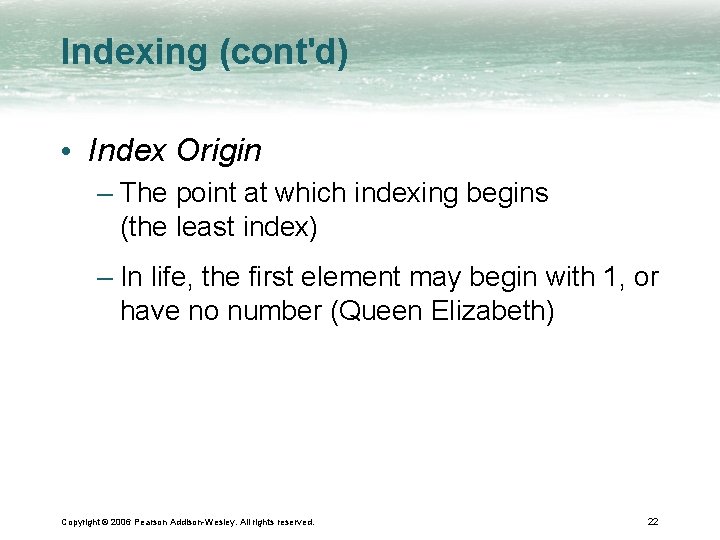
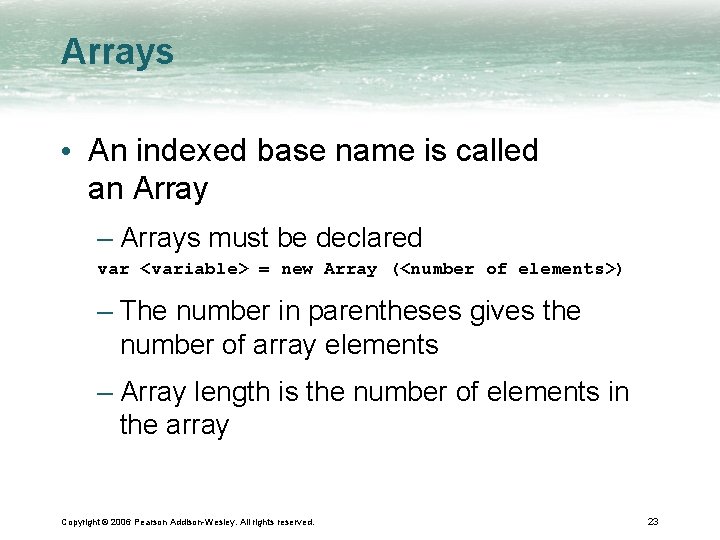
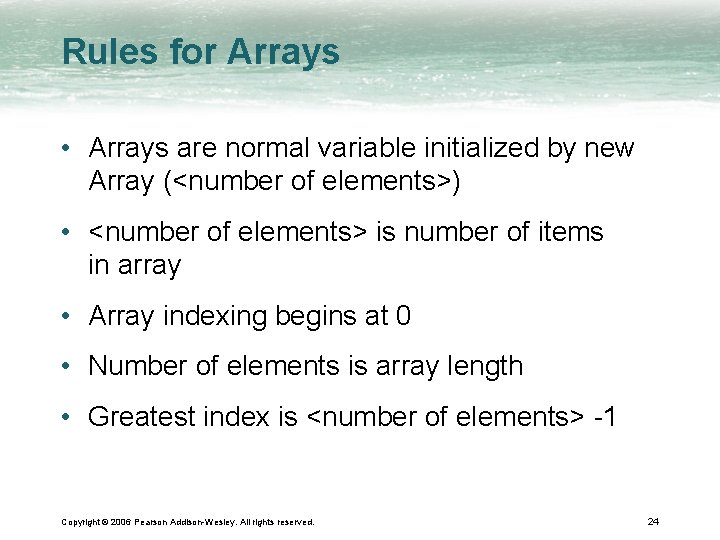
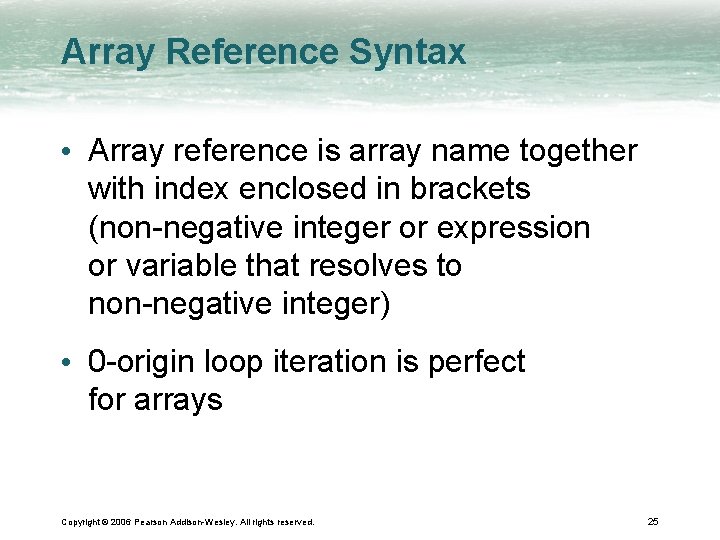
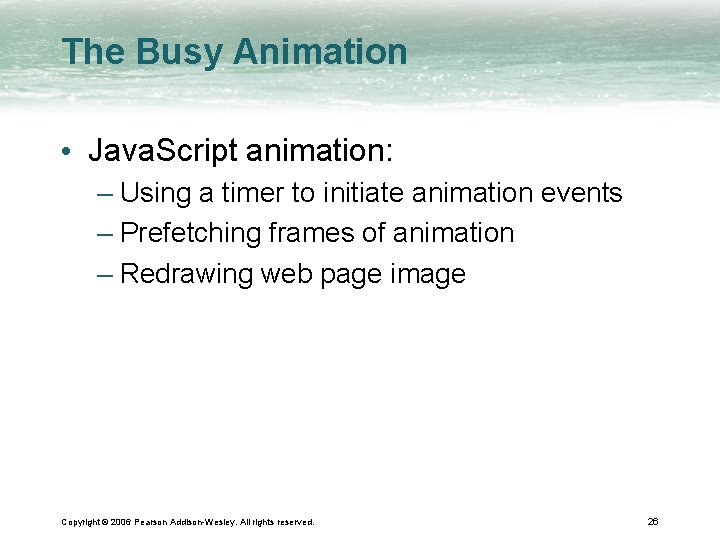
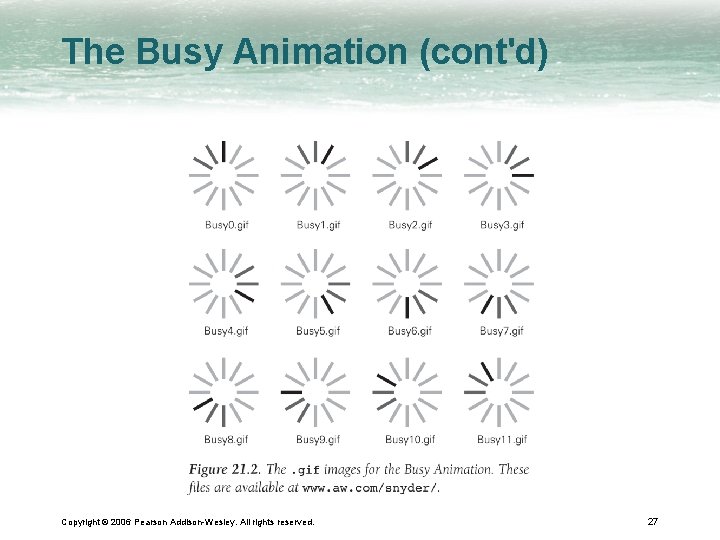
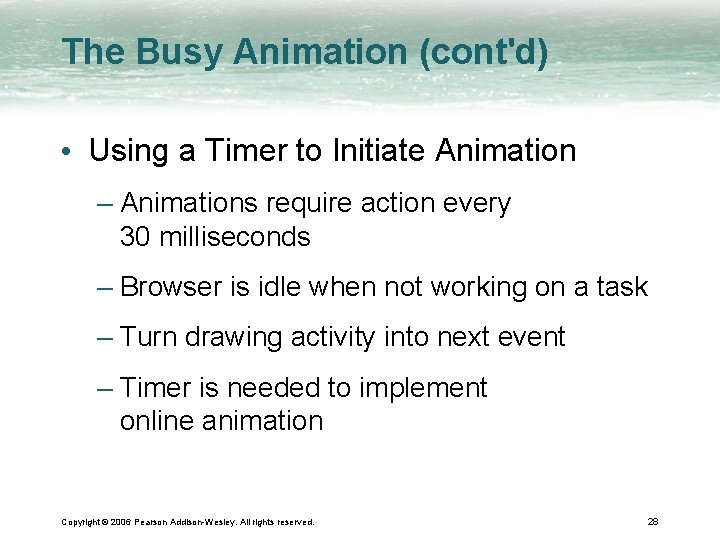
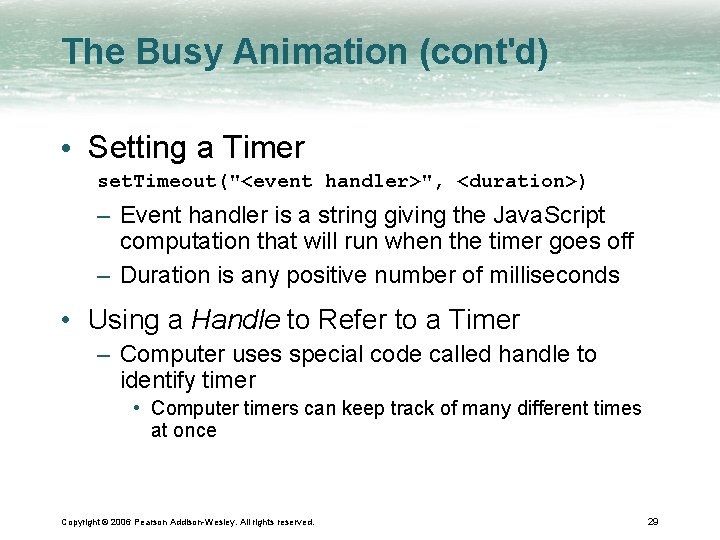
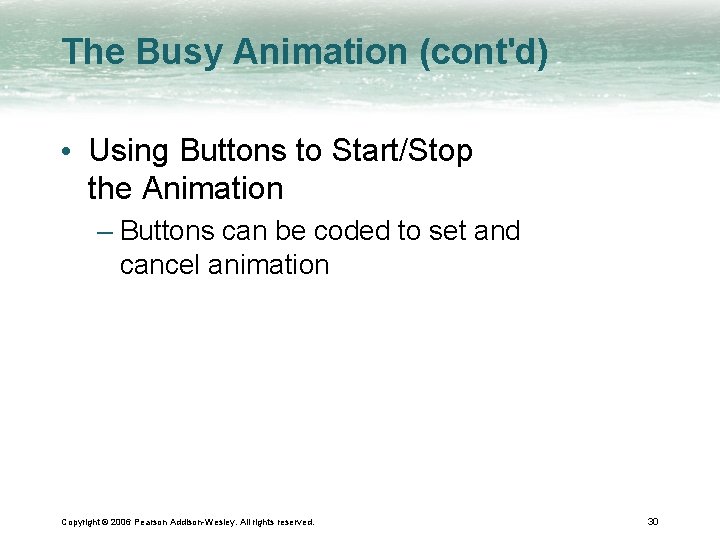
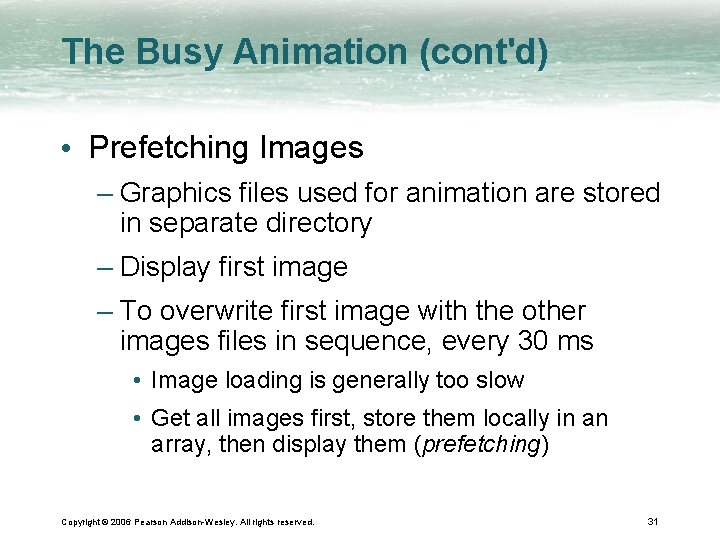
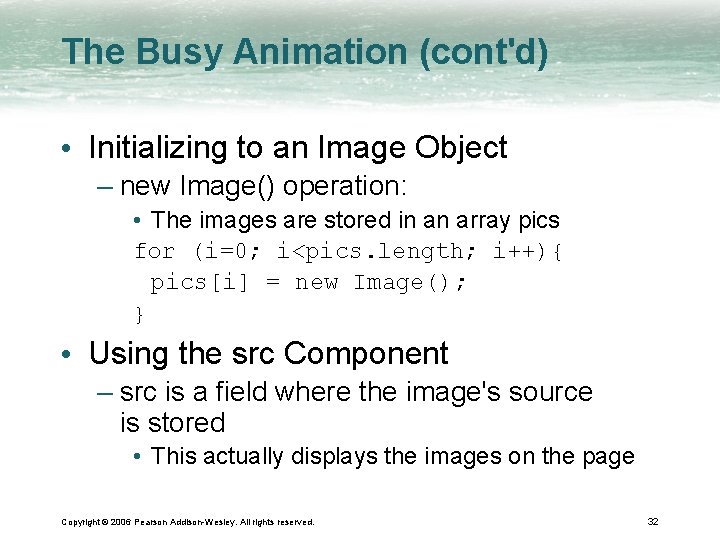
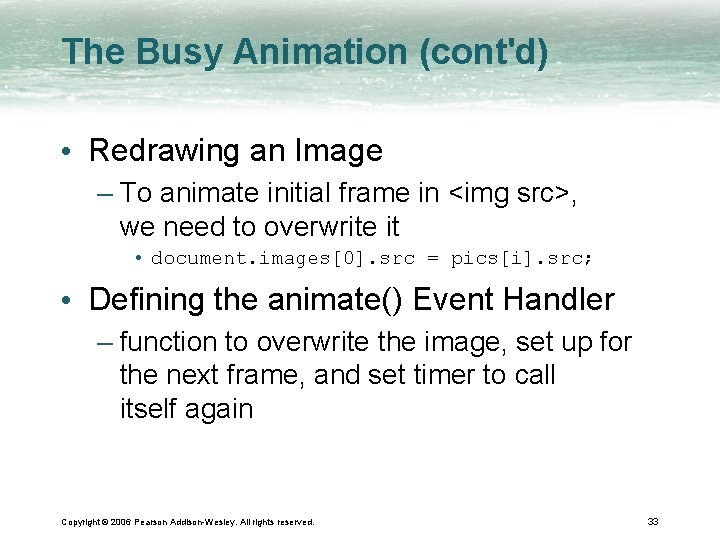
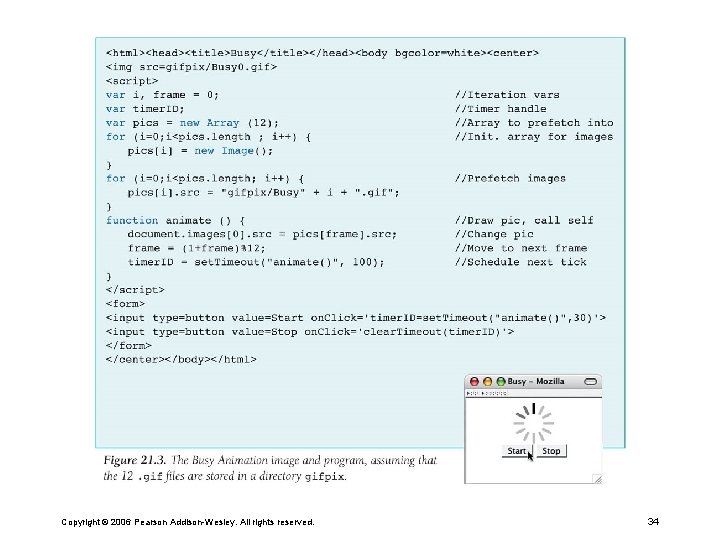
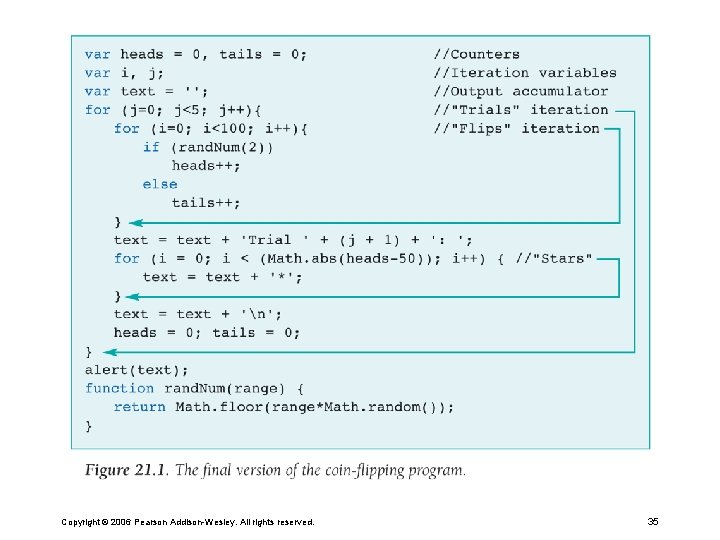
- Slides: 35
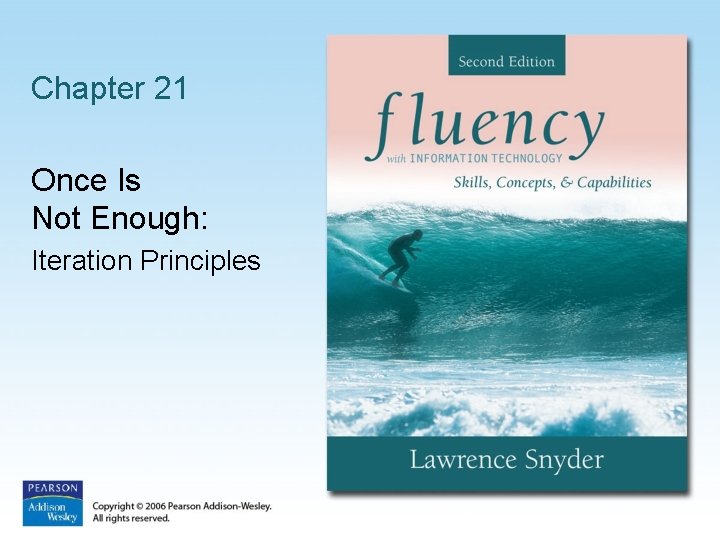
Chapter 21 Once Is Not Enough: Iteration Principles
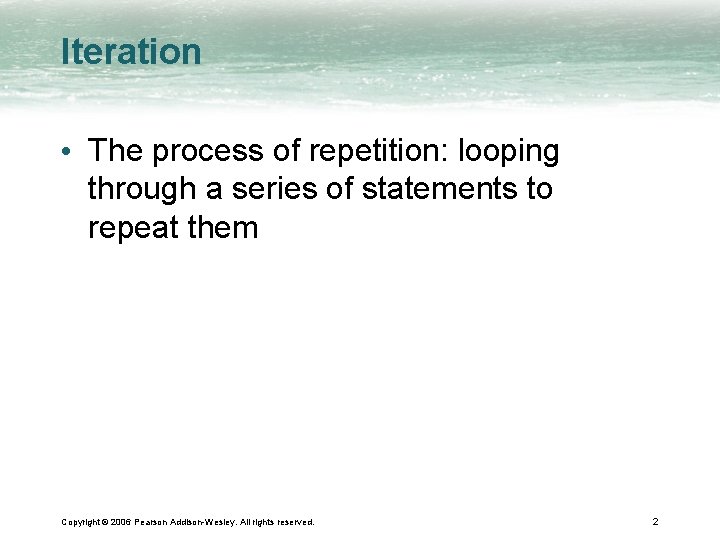
Iteration • The process of repetition: looping through a series of statements to repeat them Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 2
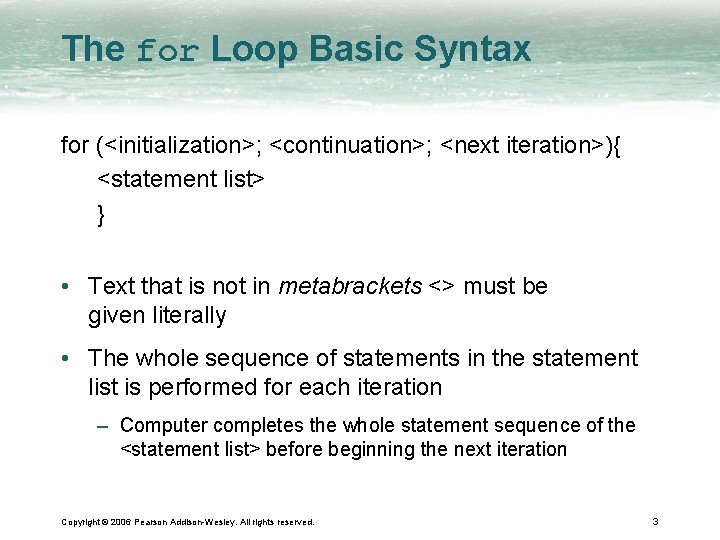
The for Loop Basic Syntax for (<initialization>; <continuation>; <next iteration>){ <statement list> } • Text that is not in metabrackets <> must be given literally • The whole sequence of statements in the statement list is performed for each iteration – Computer completes the whole statement sequence of the <statement list> before beginning the next iteration Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 3
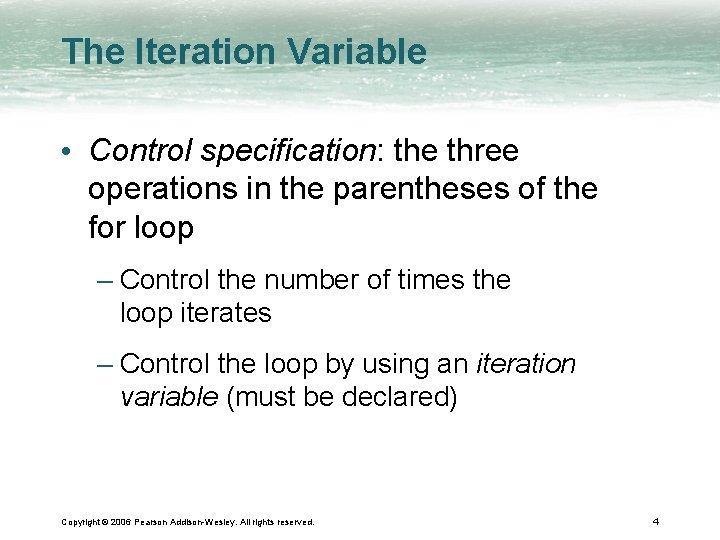
The Iteration Variable • Control specification: the three operations in the parentheses of the for loop – Control the number of times the loop iterates – Control the loop by using an iteration variable (must be declared) Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 4
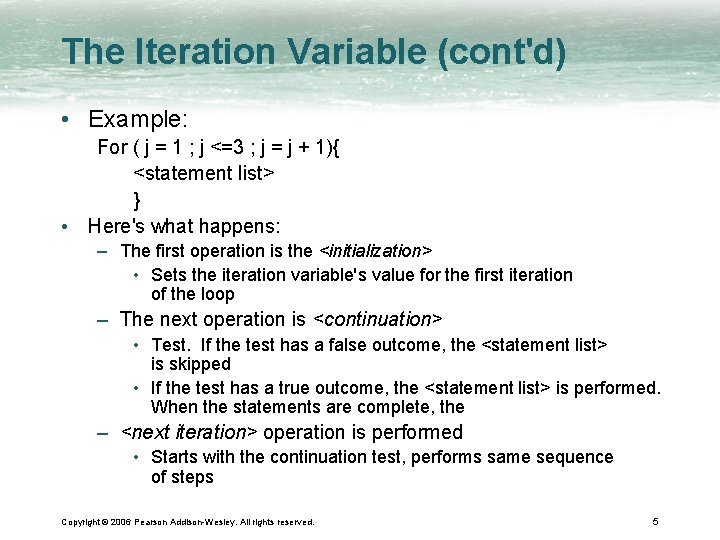
The Iteration Variable (cont'd) • Example: For ( j = 1 ; j <=3 ; j = j + 1){ <statement list> } • Here's what happens: – The first operation is the <initialization> • Sets the iteration variable's value for the first iteration of the loop – The next operation is <continuation> • Test. If the test has a false outcome, the <statement list> is skipped • If the test has a true outcome, the <statement list> is performed. When the statements are complete, the – <next iteration> operation is performed • Starts with the continuation test, performs same sequence of steps Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 5
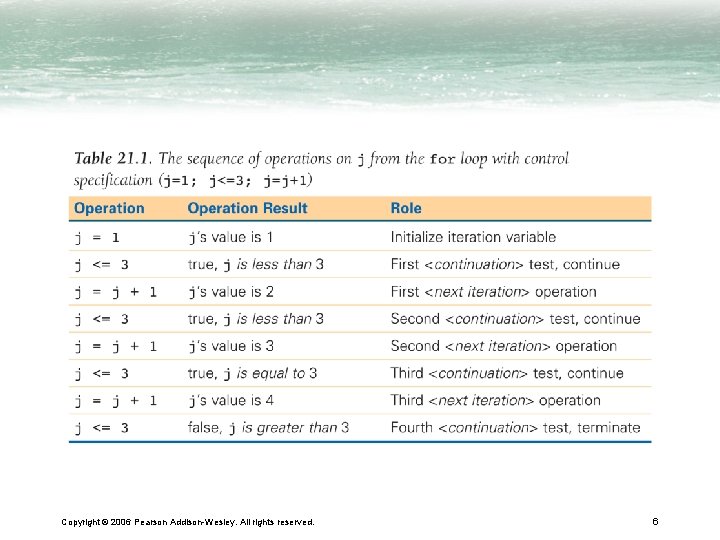
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 6
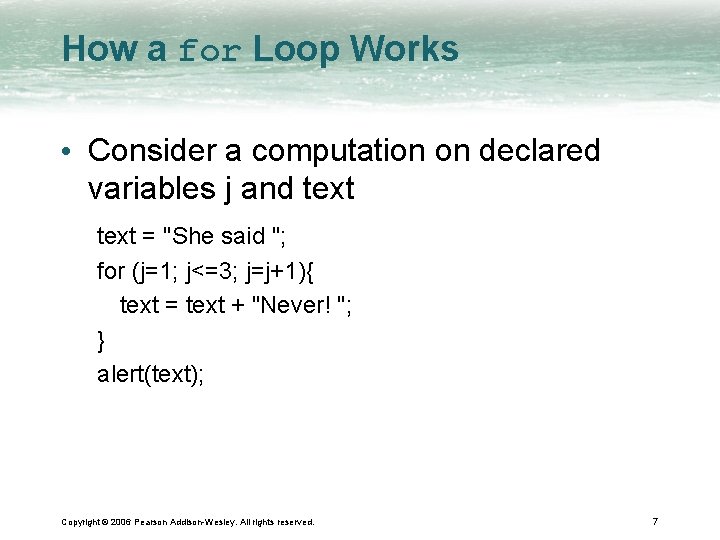
How a for Loop Works • Consider a computation on declared variables j and text = "She said "; for (j=1; j<=3; j=j+1){ text = text + "Never! "; } alert(text); Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 7
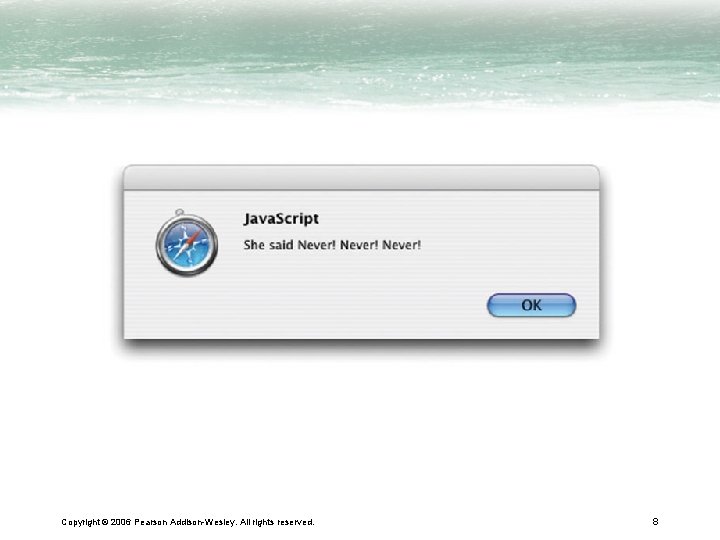
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 8
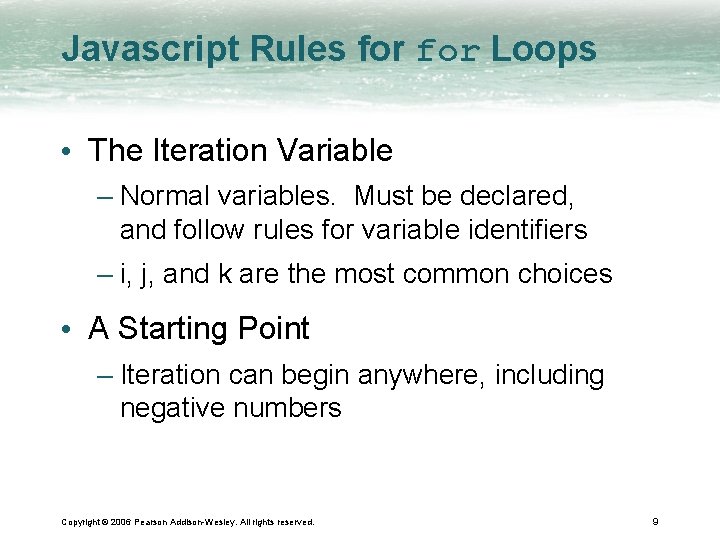
Javascript Rules for Loops • The Iteration Variable – Normal variables. Must be declared, and follow rules for variable identifiers – i, j, and k are the most common choices • A Starting Point – Iteration can begin anywhere, including negative numbers Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 9
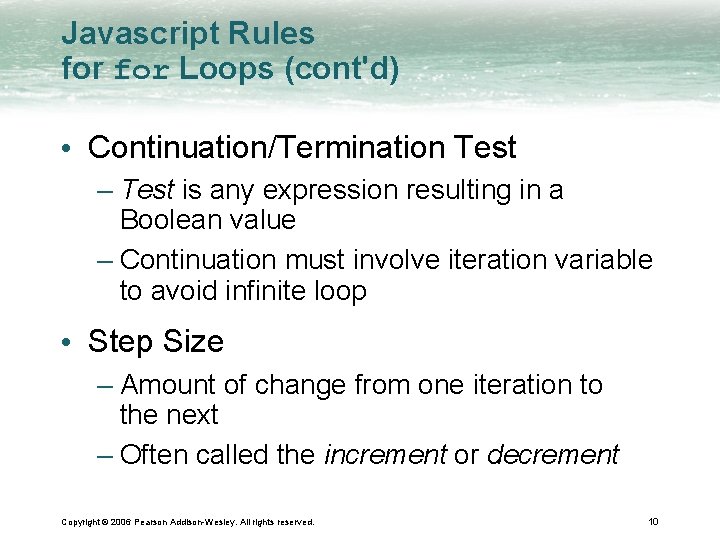
Javascript Rules for Loops (cont'd) • Continuation/Termination Test – Test is any expression resulting in a Boolean value – Continuation must involve iteration variable to avoid infinite loop • Step Size – Amount of change from one iteration to the next – Often called the increment or decrement Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 10
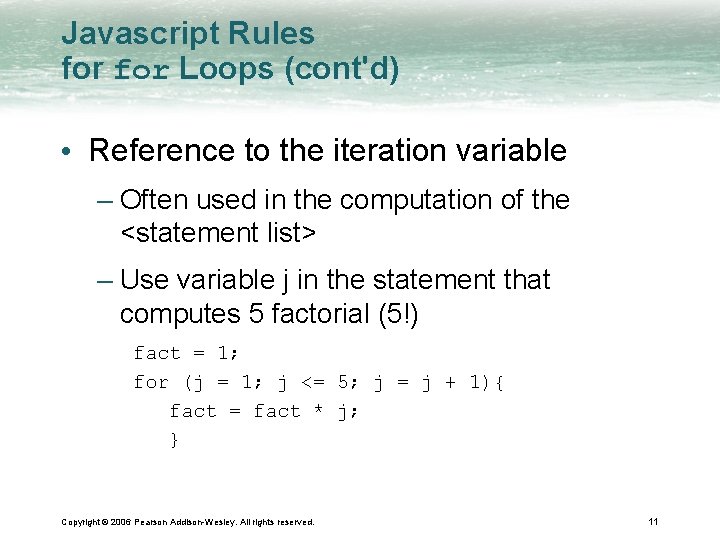
Javascript Rules for Loops (cont'd) • Reference to the iteration variable – Often used in the computation of the <statement list> – Use variable j in the statement that computes 5 factorial (5!) fact = 1; for (j = 1; j <= 5; j = j + 1){ fact = fact * j; } Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 11
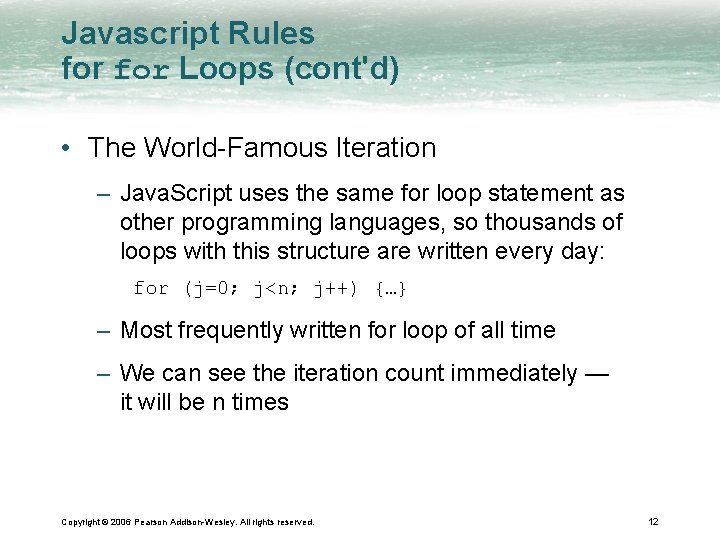
Javascript Rules for Loops (cont'd) • The World-Famous Iteration – Java. Script uses the same for loop statement as other programming languages, so thousands of loops with this structure are written every day: for (j=0; j<n; j++) {…} – Most frequently written for loop of all time – We can see the iteration count immediately — it will be n times Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 12
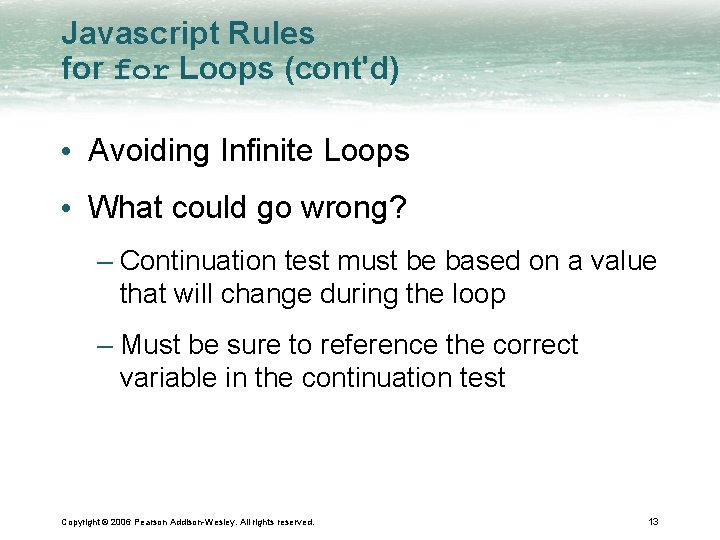
Javascript Rules for Loops (cont'd) • Avoiding Infinite Loops • What could go wrong? – Continuation test must be based on a value that will change during the loop – Must be sure to reference the correct variable in the continuation test Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 13
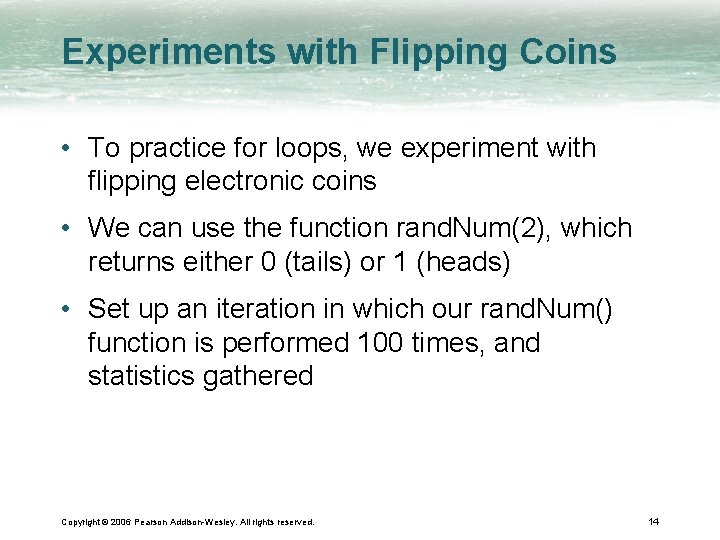
Experiments with Flipping Coins • To practice for loops, we experiment with flipping electronic coins • We can use the function rand. Num(2), which returns either 0 (tails) or 1 (heads) • Set up an iteration in which our rand. Num() function is performed 100 times, and statistics gathered Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 14
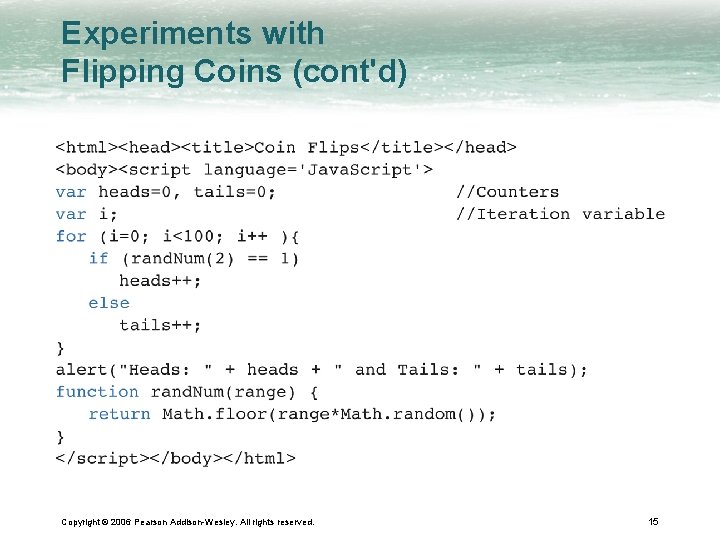
Experiments with Flipping Coins (cont'd) Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 15
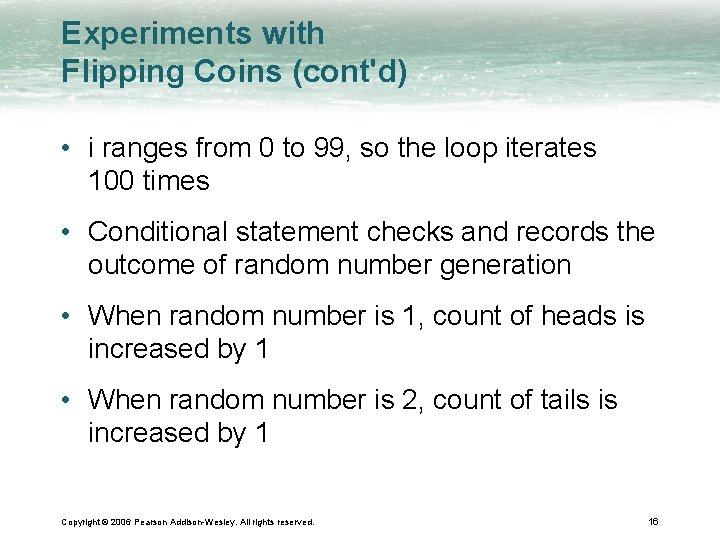
Experiments with Flipping Coins (cont'd) • i ranges from 0 to 99, so the loop iterates 100 times • Conditional statement checks and records the outcome of random number generation • When random number is 1, count of heads is increased by 1 • When random number is 2, count of tails is increased by 1 Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 16
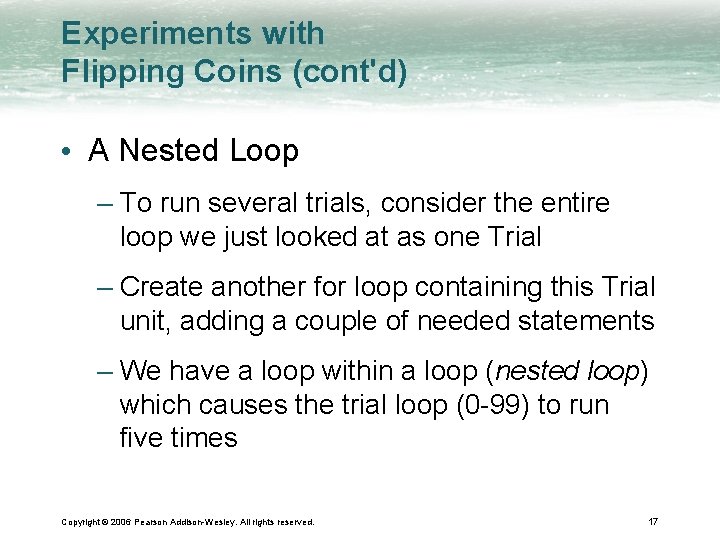
Experiments with Flipping Coins (cont'd) • A Nested Loop – To run several trials, consider the entire loop we just looked at as one Trial – Create another for loop containing this Trial unit, adding a couple of needed statements – We have a loop within a loop (nested loop) which causes the trial loop (0 -99) to run five times Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 17
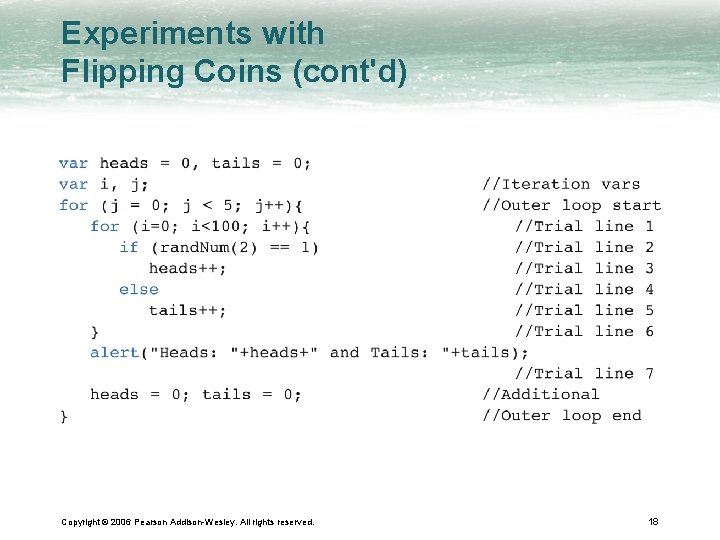
Experiments with Flipping Coins (cont'd) Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 18
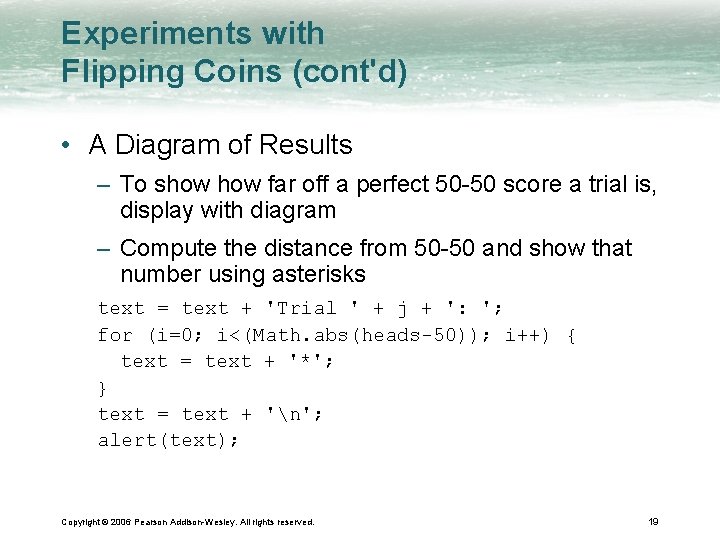
Experiments with Flipping Coins (cont'd) • A Diagram of Results – To show far off a perfect 50 -50 score a trial is, display with diagram – Compute the distance from 50 -50 and show that number using asterisks text = text + 'Trial ' + j + ': '; for (i=0; i<(Math. abs(heads-50)); i++) { text = text + '*'; } text = text + 'n'; alert(text); Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 19
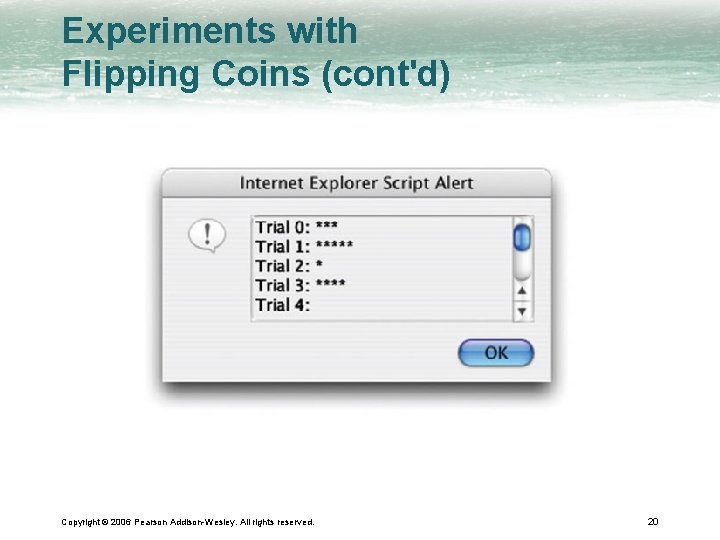
Experiments with Flipping Coins (cont'd) Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 20
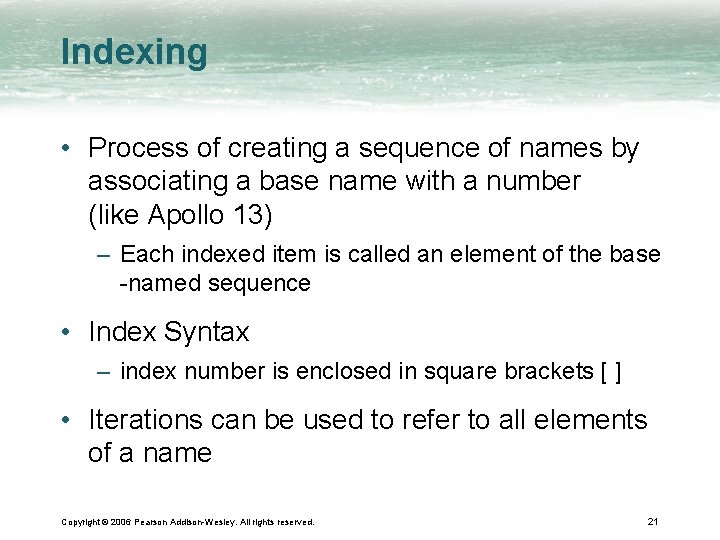
Indexing • Process of creating a sequence of names by associating a base name with a number (like Apollo 13) – Each indexed item is called an element of the base -named sequence • Index Syntax – index number is enclosed in square brackets [ ] • Iterations can be used to refer to all elements of a name Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 21
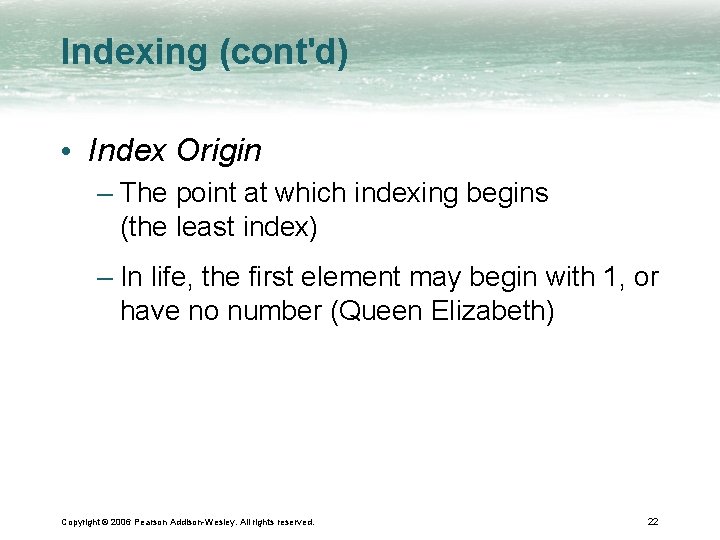
Indexing (cont'd) • Index Origin – The point at which indexing begins (the least index) – In life, the first element may begin with 1, or have no number (Queen Elizabeth) Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 22
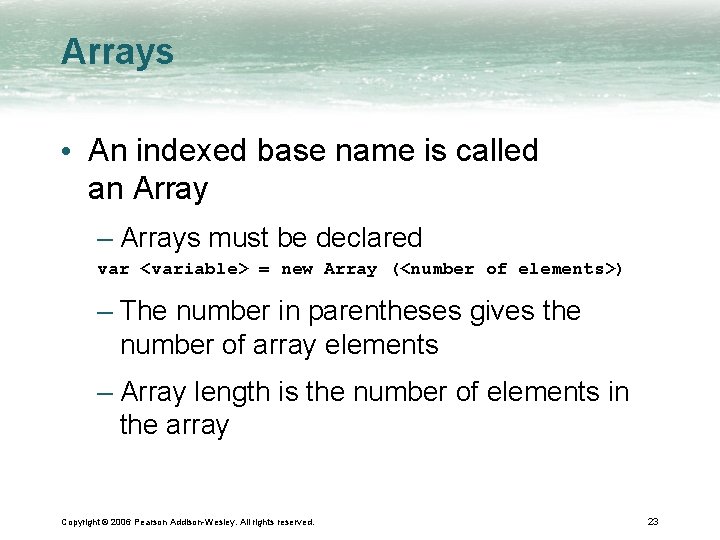
Arrays • An indexed base name is called an Array – Arrays must be declared var <variable> = new Array (<number of elements>) – The number in parentheses gives the number of array elements – Array length is the number of elements in the array Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 23
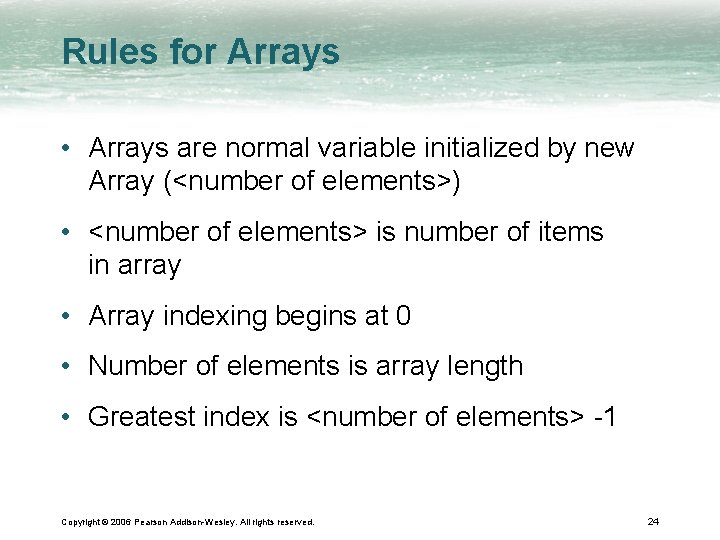
Rules for Arrays • Arrays are normal variable initialized by new Array (<number of elements>) • <number of elements> is number of items in array • Array indexing begins at 0 • Number of elements is array length • Greatest index is <number of elements> -1 Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 24
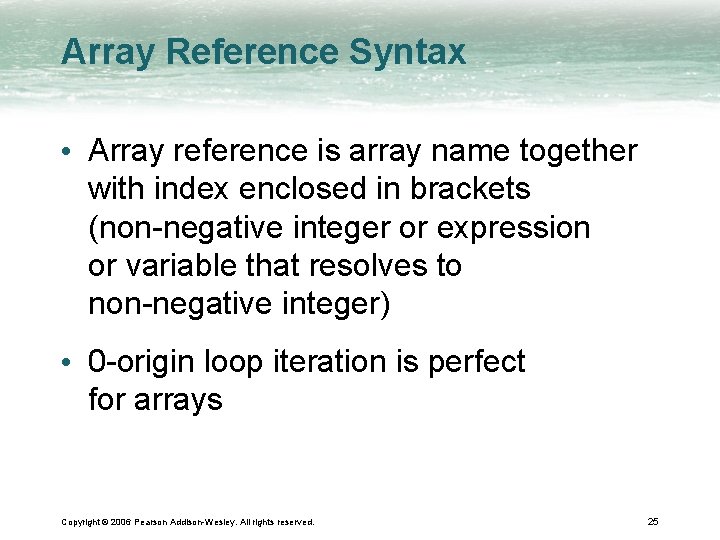
Array Reference Syntax • Array reference is array name together with index enclosed in brackets (non-negative integer or expression or variable that resolves to non-negative integer) • 0 -origin loop iteration is perfect for arrays Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 25
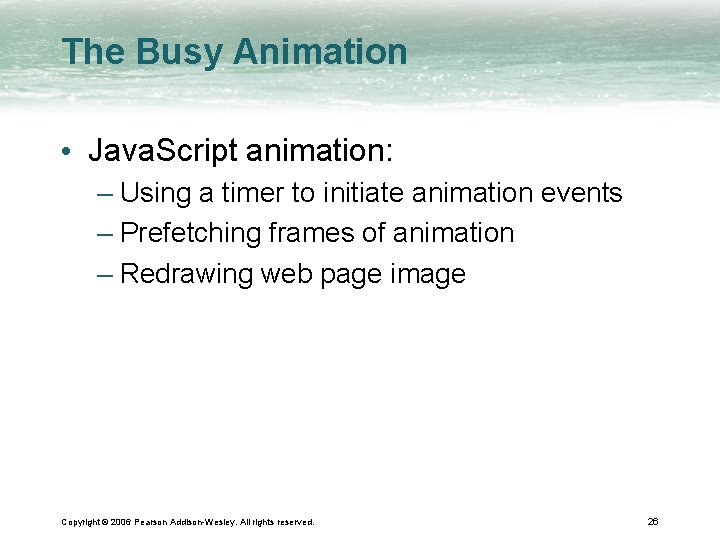
The Busy Animation • Java. Script animation: – Using a timer to initiate animation events – Prefetching frames of animation – Redrawing web page image Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 26
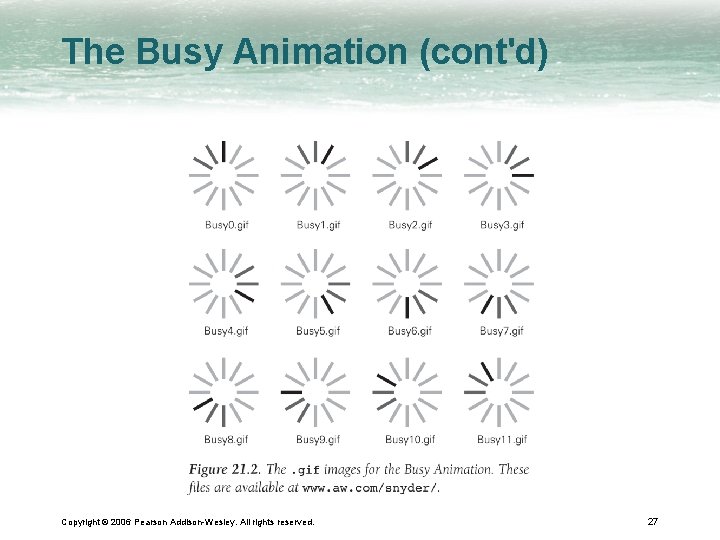
The Busy Animation (cont'd) Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 27
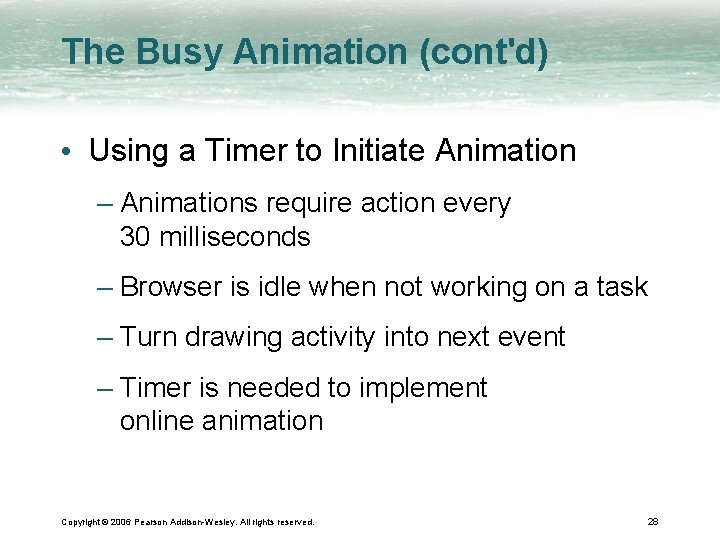
The Busy Animation (cont'd) • Using a Timer to Initiate Animation – Animations require action every 30 milliseconds – Browser is idle when not working on a task – Turn drawing activity into next event – Timer is needed to implement online animation Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 28
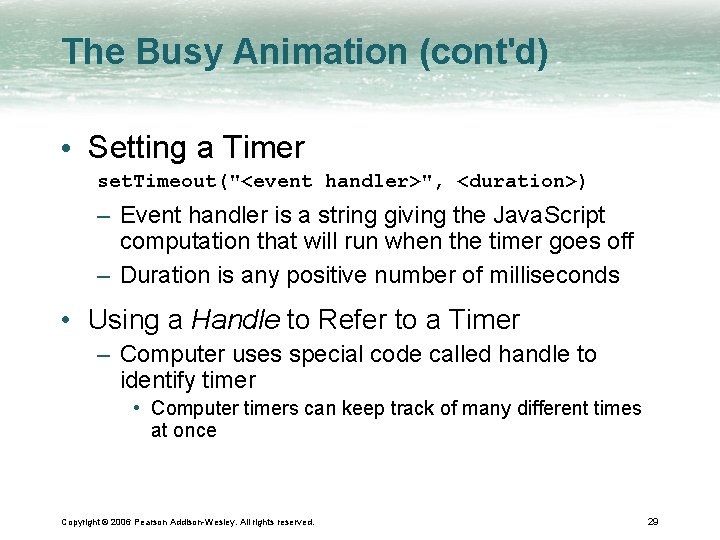
The Busy Animation (cont'd) • Setting a Timer set. Timeout("<event handler>", <duration>) – Event handler is a string giving the Java. Script computation that will run when the timer goes off – Duration is any positive number of milliseconds • Using a Handle to Refer to a Timer – Computer uses special code called handle to identify timer • Computer timers can keep track of many different times at once Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 29
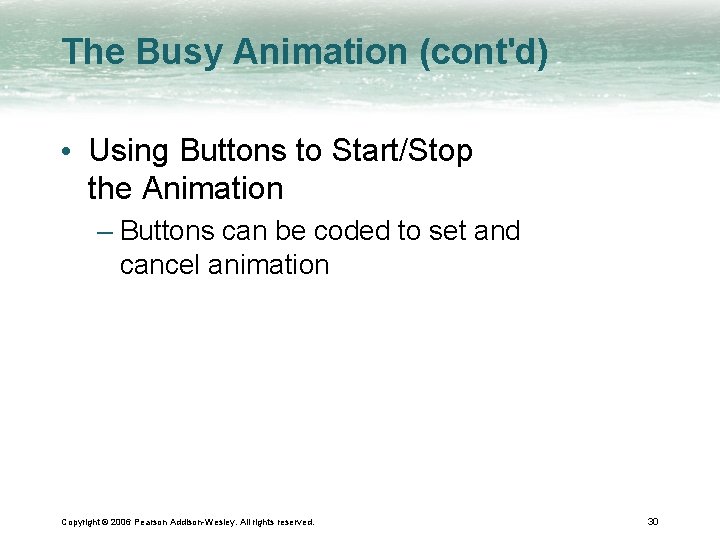
The Busy Animation (cont'd) • Using Buttons to Start/Stop the Animation – Buttons can be coded to set and cancel animation Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 30
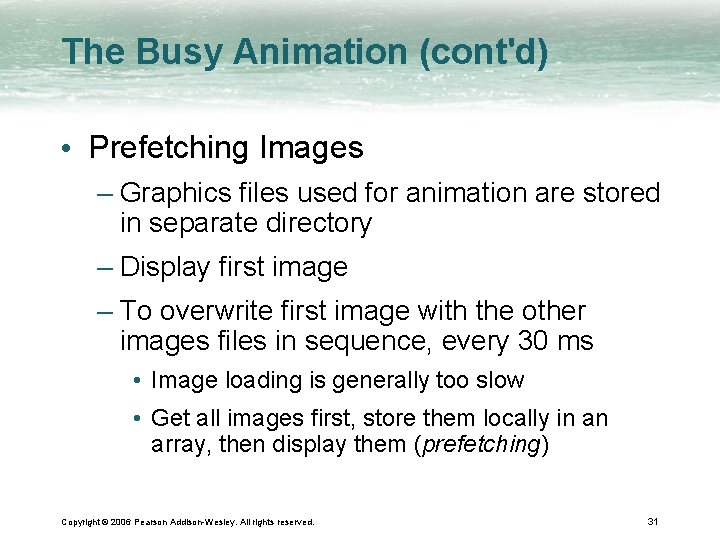
The Busy Animation (cont'd) • Prefetching Images – Graphics files used for animation are stored in separate directory – Display first image – To overwrite first image with the other images files in sequence, every 30 ms • Image loading is generally too slow • Get all images first, store them locally in an array, then display them (prefetching) Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 31
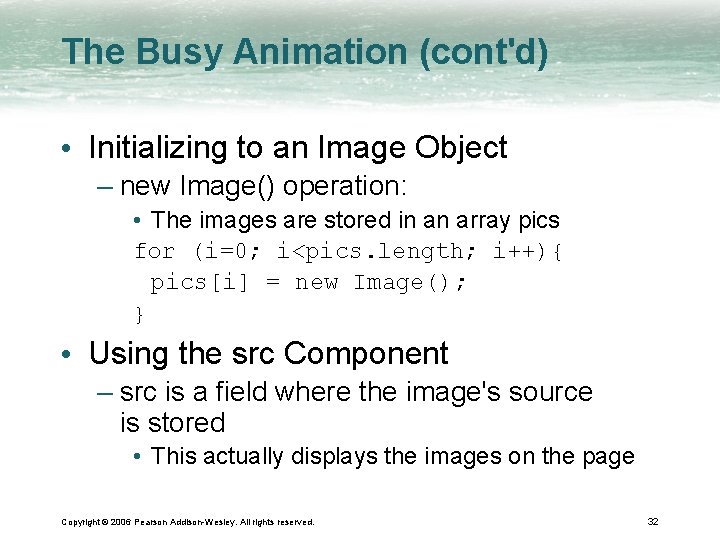
The Busy Animation (cont'd) • Initializing to an Image Object – new Image() operation: • The images are stored in an array pics for (i=0; i<pics. length; i++){ pics[i] = new Image(); } • Using the src Component – src is a field where the image's source is stored • This actually displays the images on the page Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 32
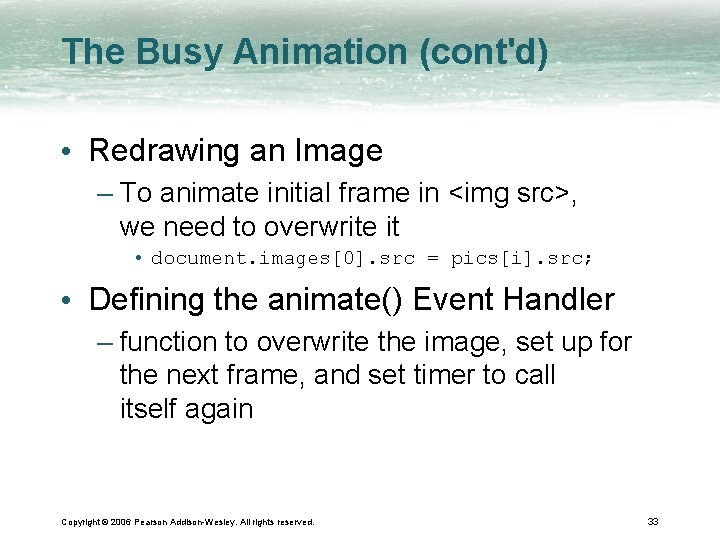
The Busy Animation (cont'd) • Redrawing an Image – To animate initial frame in <img src>, we need to overwrite it • document. images[0]. src = pics[i]. src; • Defining the animate() Event Handler – function to overwrite the image, set up for the next frame, and set timer to call itself again Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 33
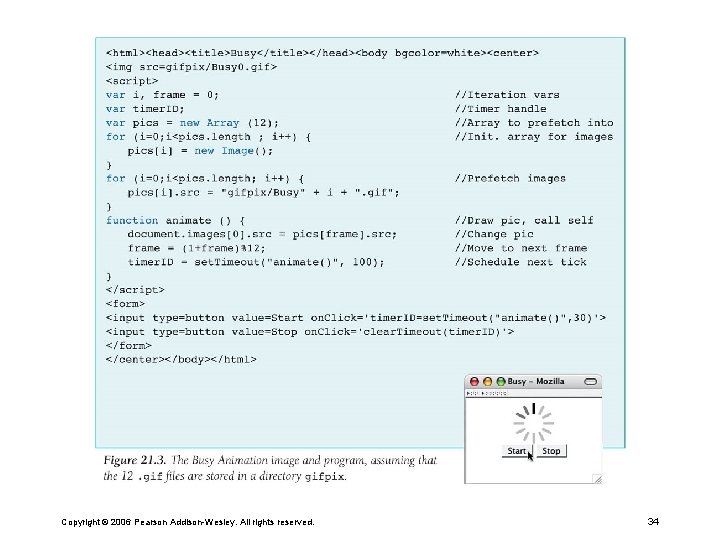
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 34
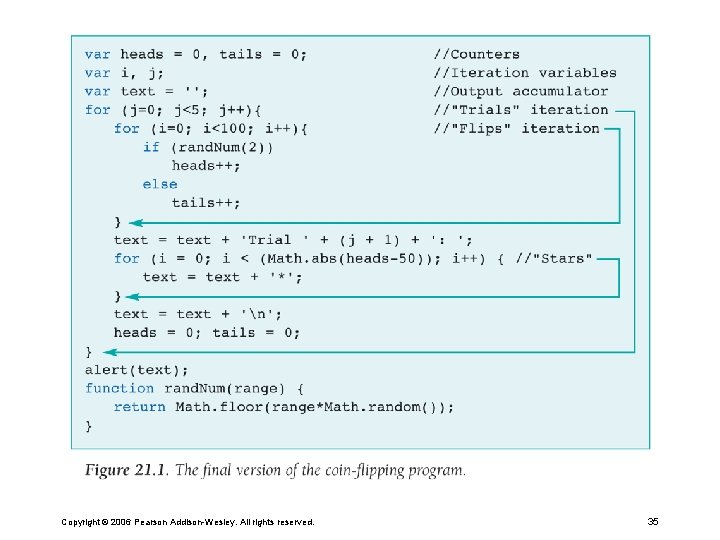
Copyright © 2006 Pearson Addison-Wesley. All rights reserved. 35