Chapter 20 Lists Stacks Queues and Priority Queues
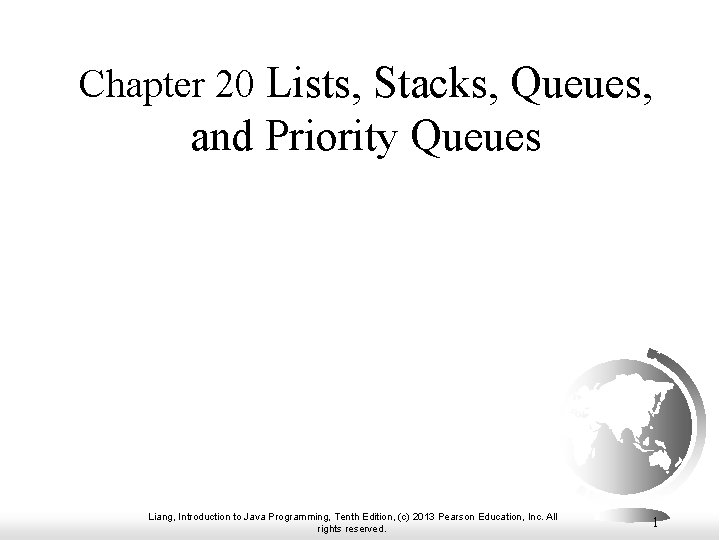
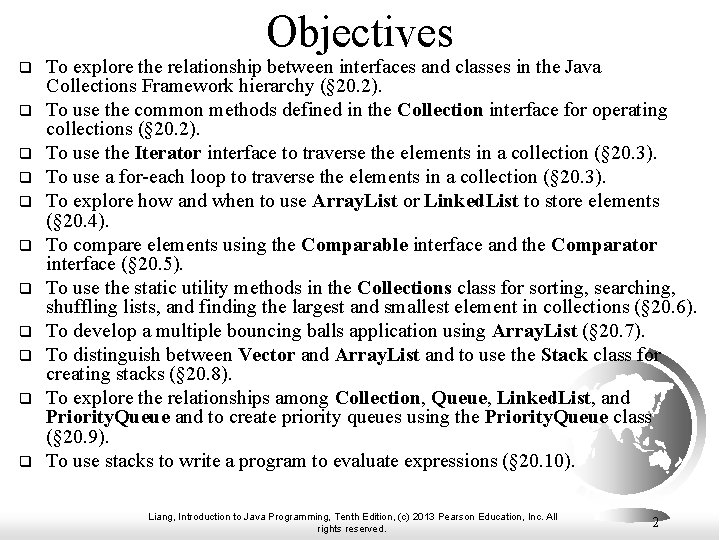
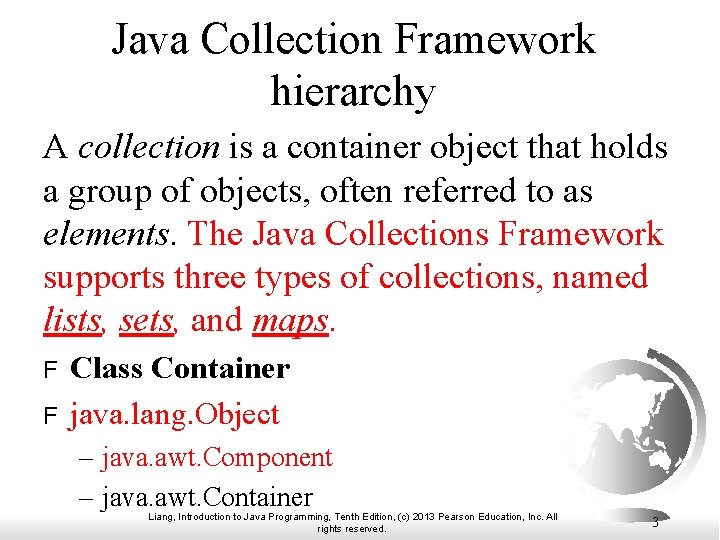
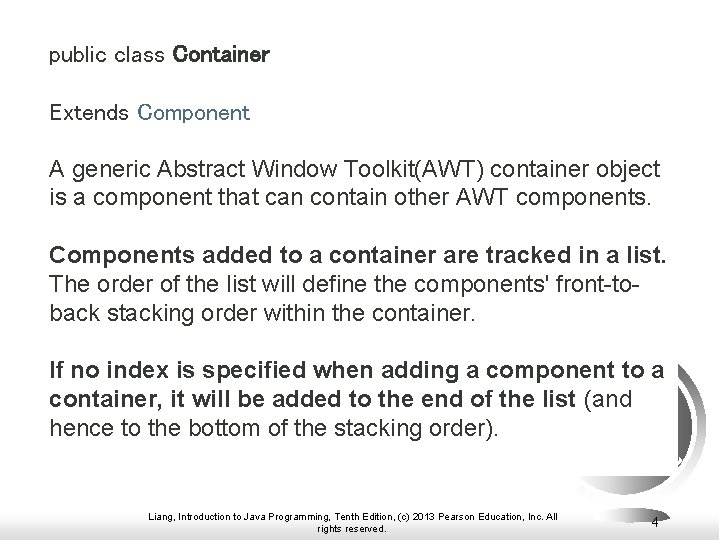
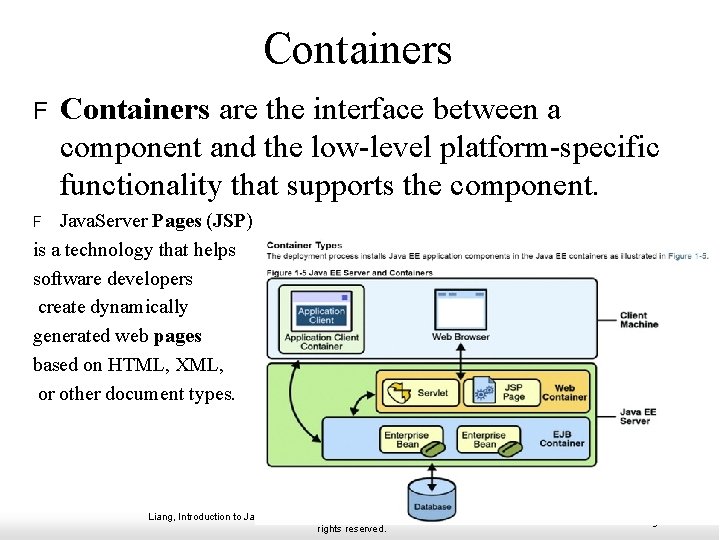
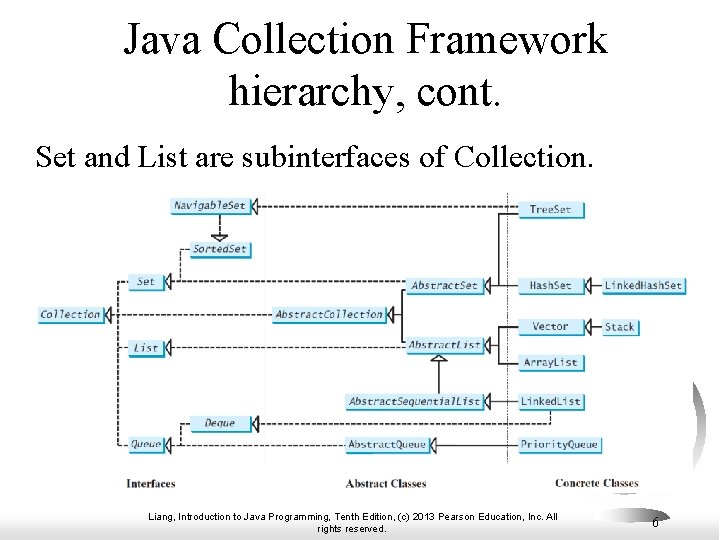
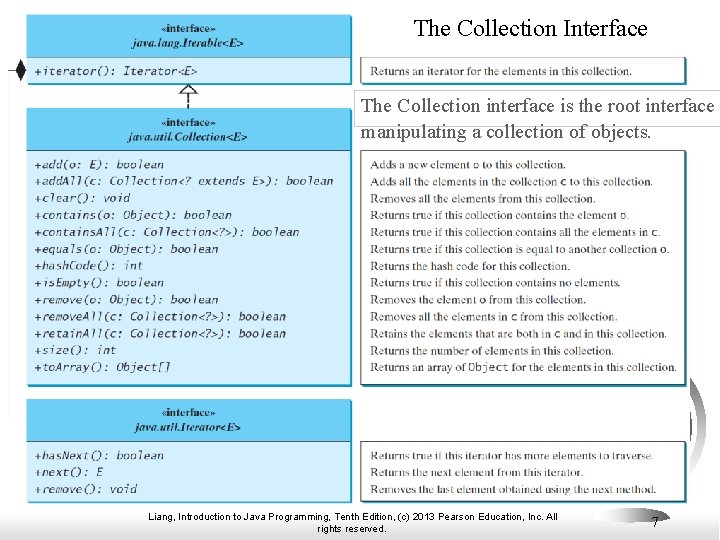
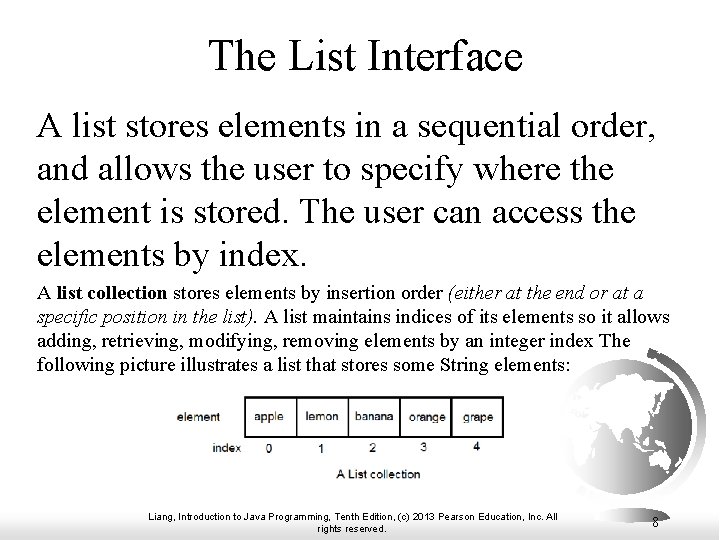
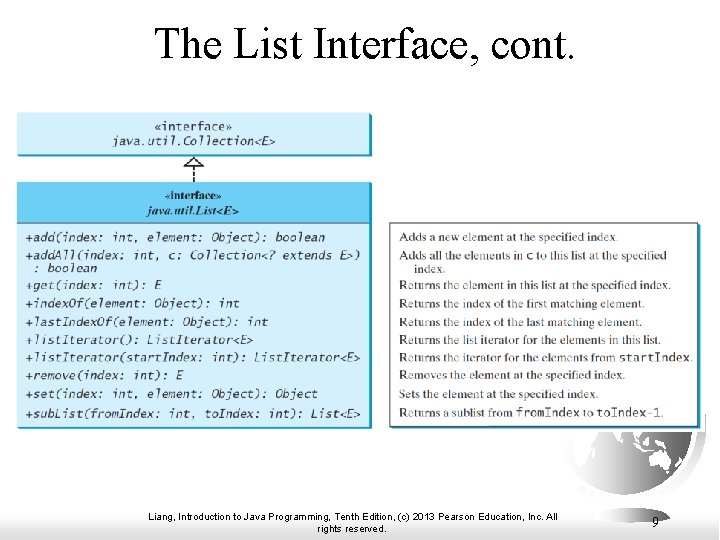
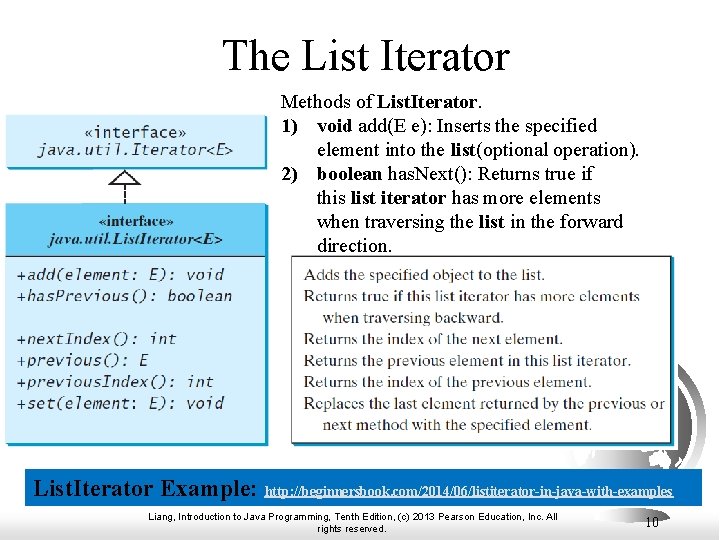
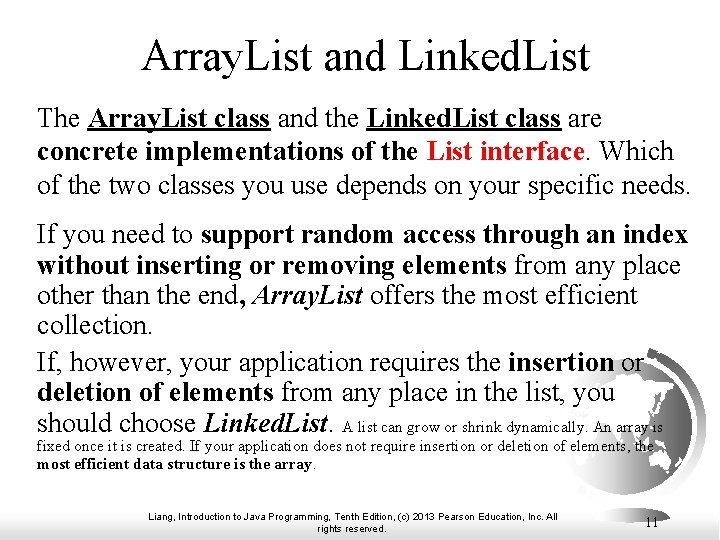
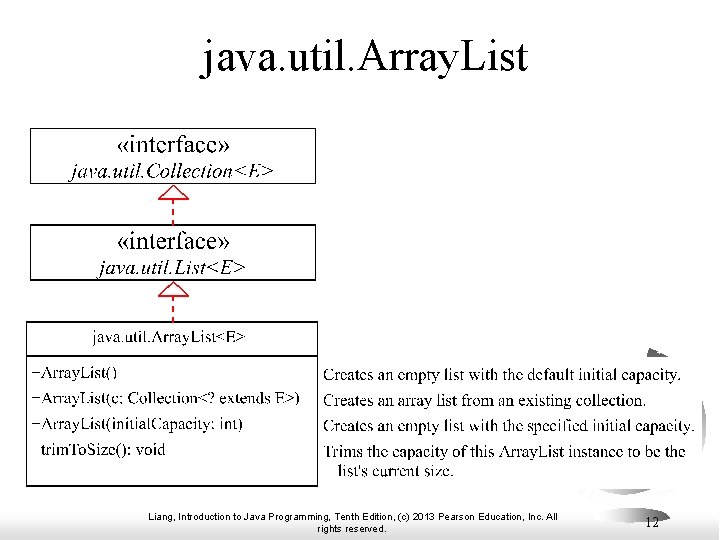
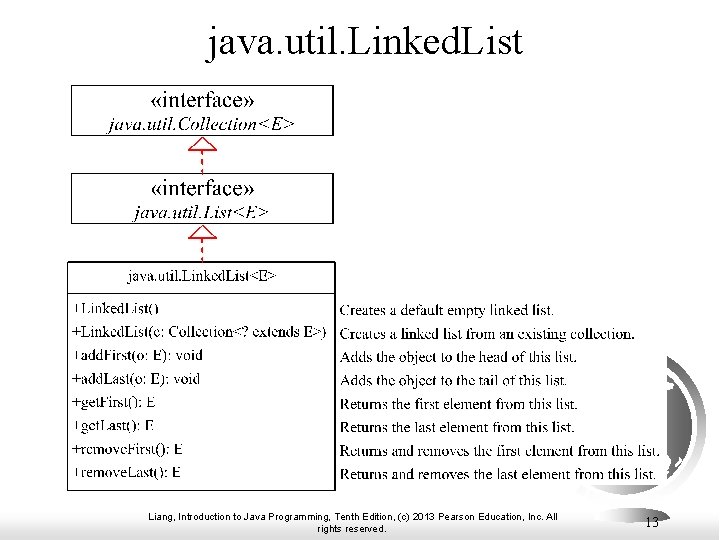
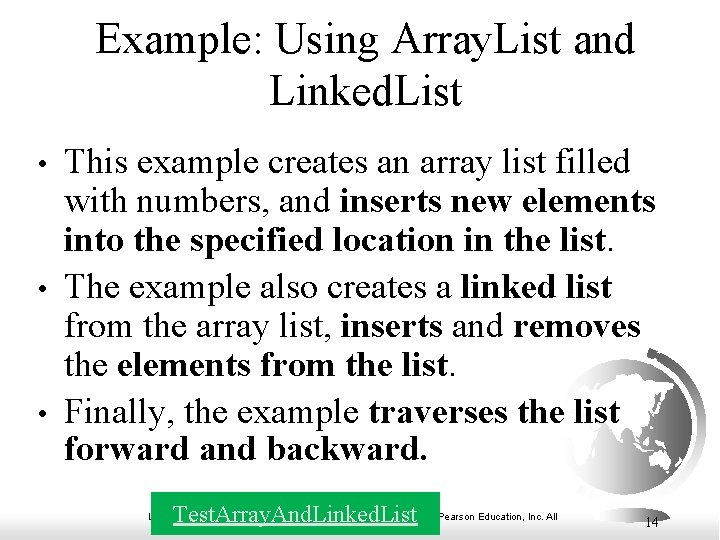
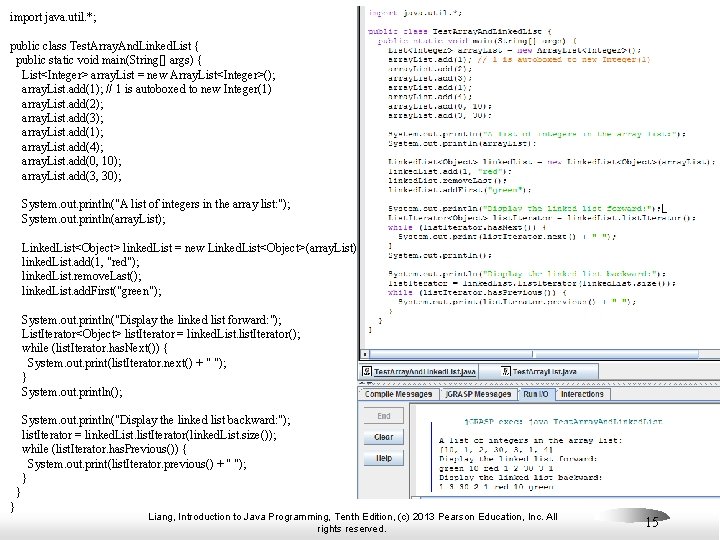
![public class Test. Array. List { public static void main(String[] args) { // Create public class Test. Array. List { public static void main(String[] args) { // Create](https://slidetodoc.com/presentation_image_h2/5f384ed4766067848e70329fc779d291/image-16.jpg)
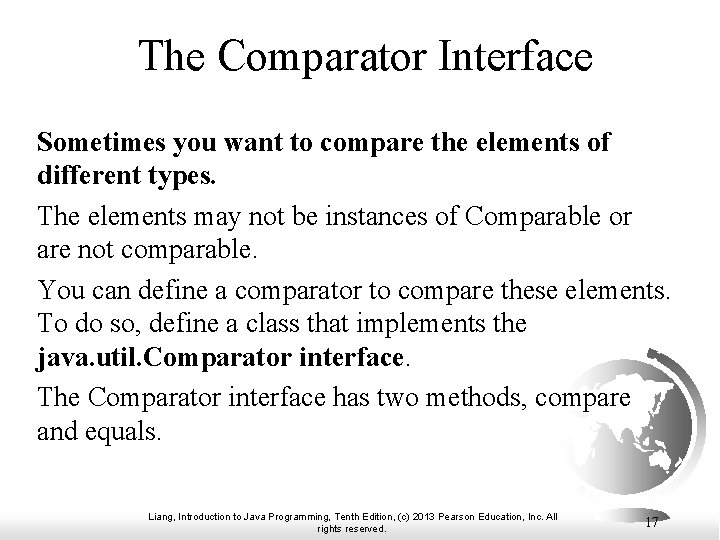
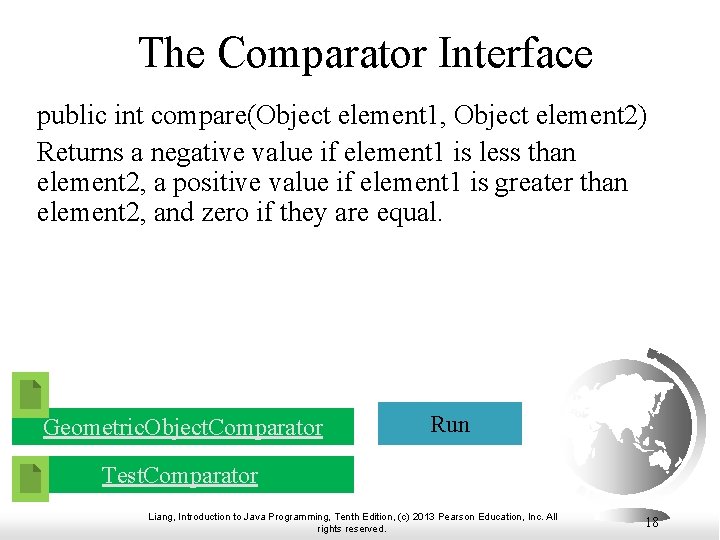
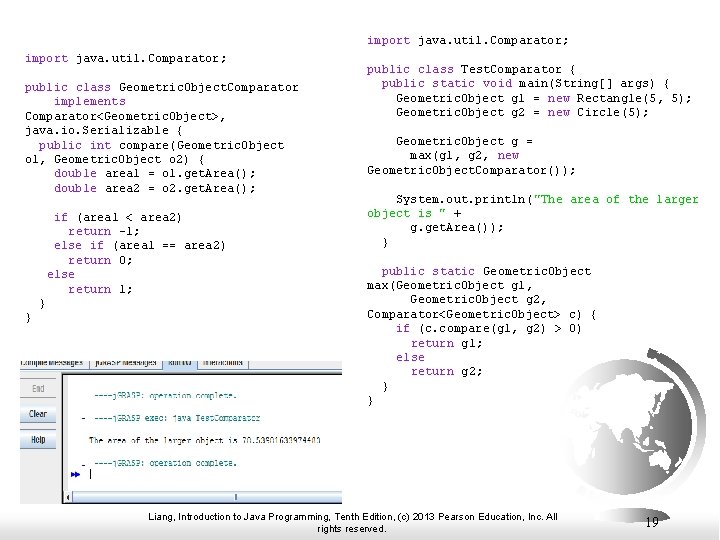
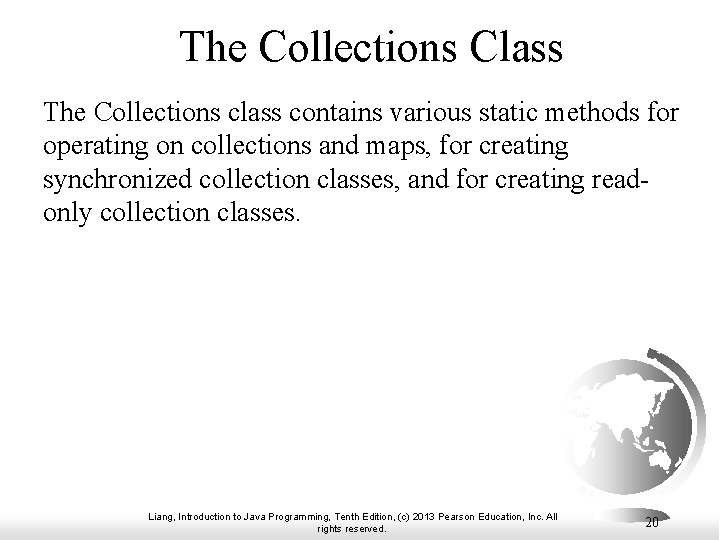
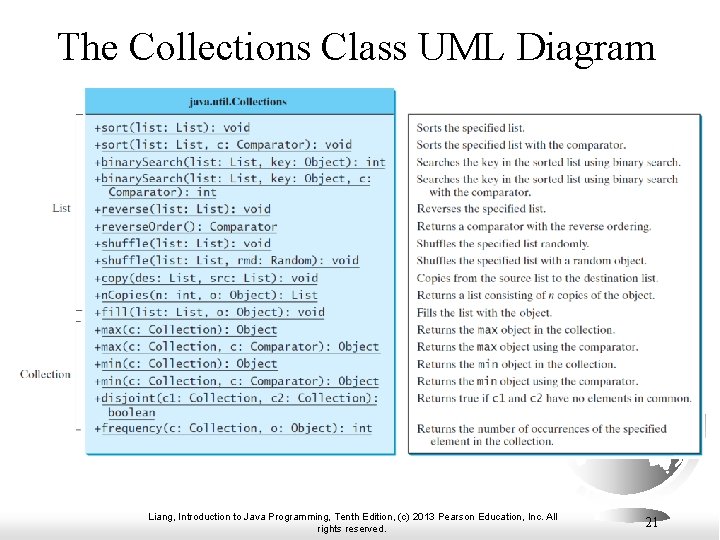
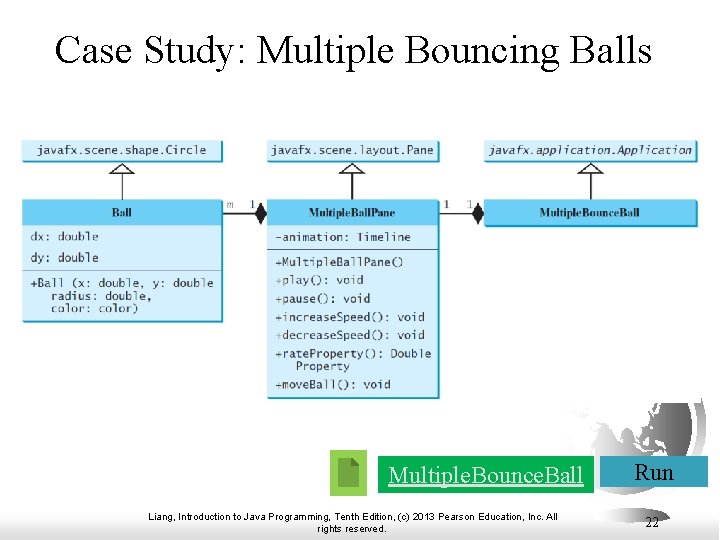
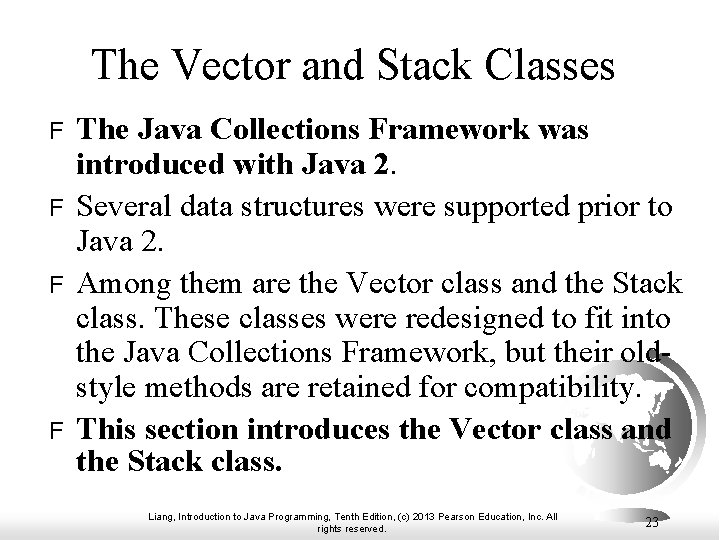
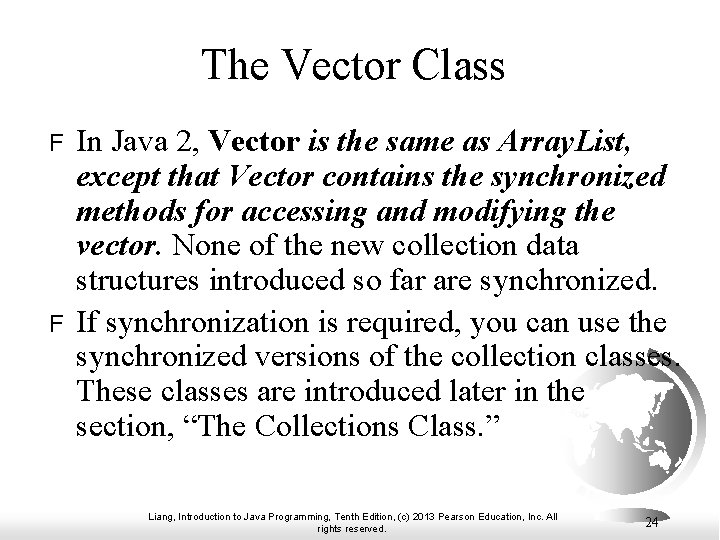
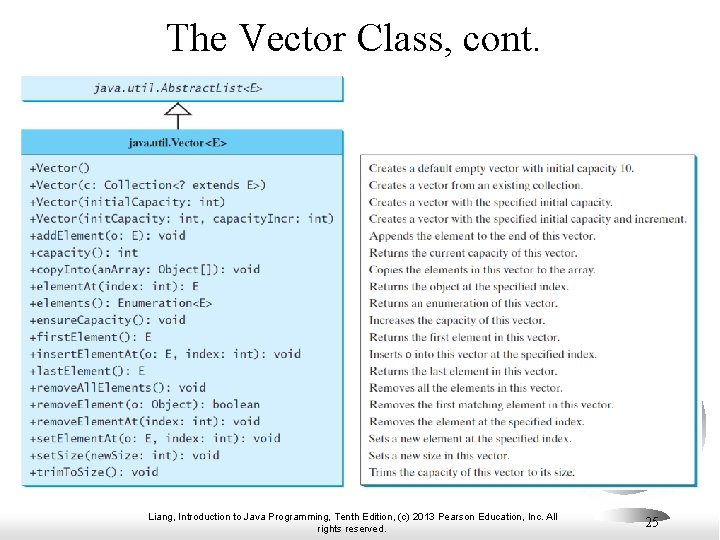
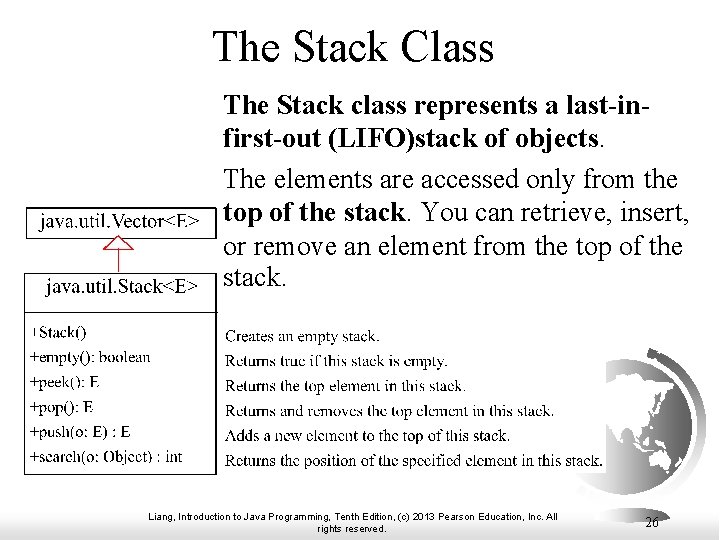
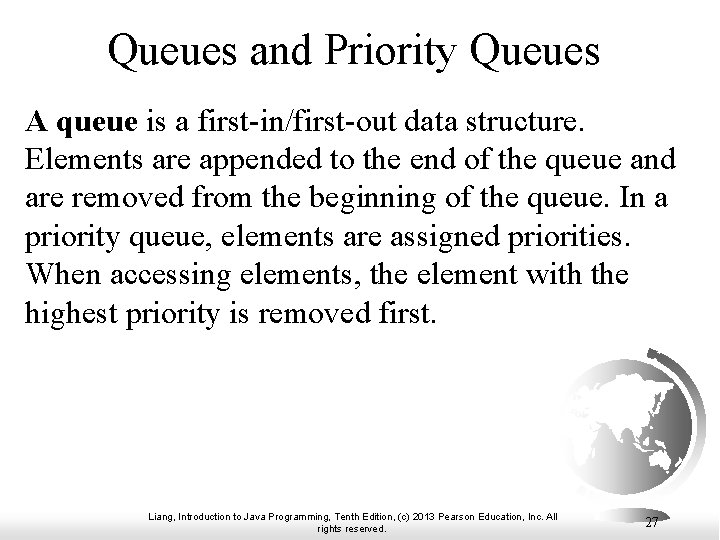
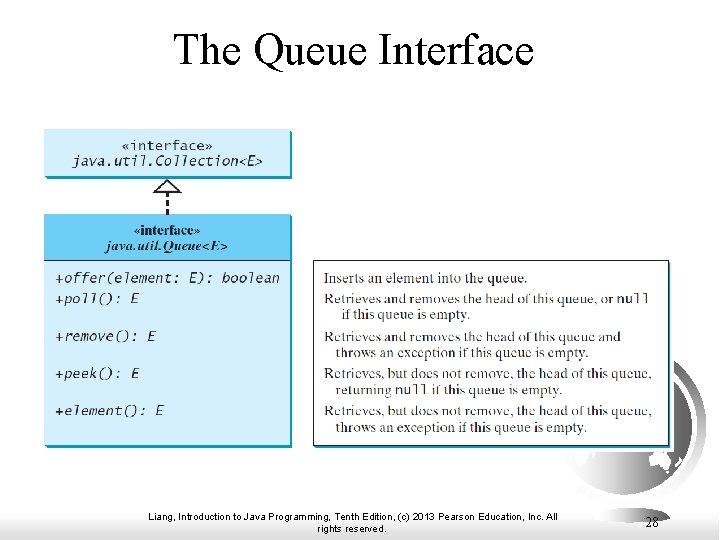
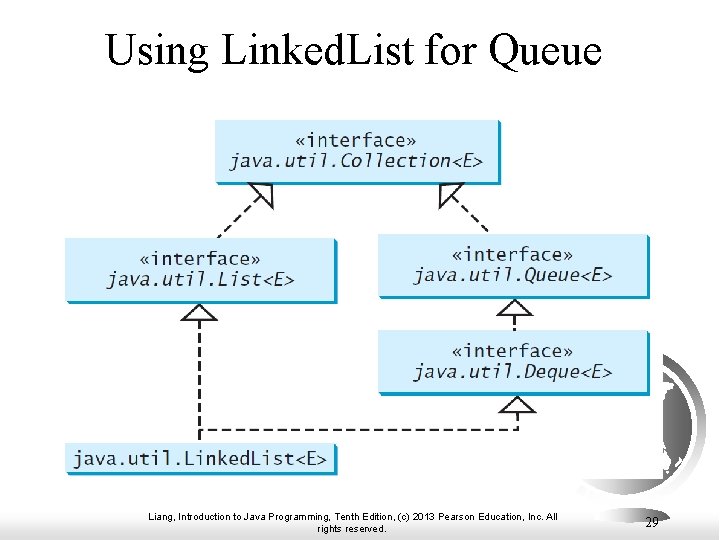
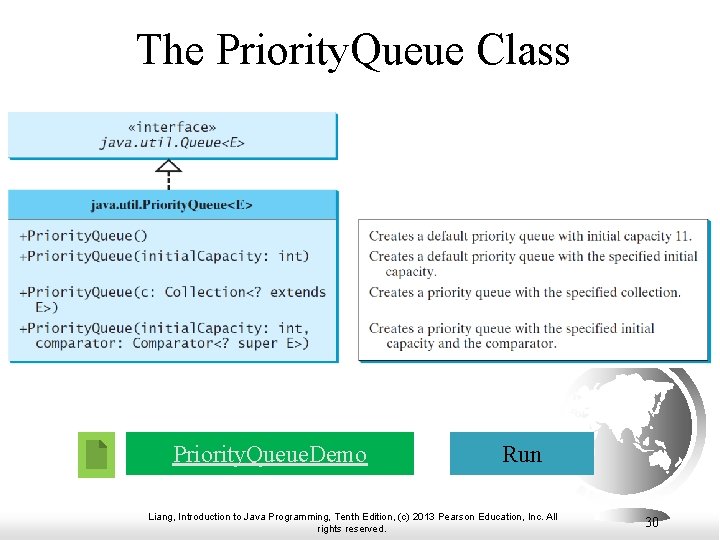
![import java. util. *; public class Priority. Queue. Demo { public static void main(String[] import java. util. *; public class Priority. Queue. Demo { public static void main(String[]](https://slidetodoc.com/presentation_image_h2/5f384ed4766067848e70329fc779d291/image-31.jpg)
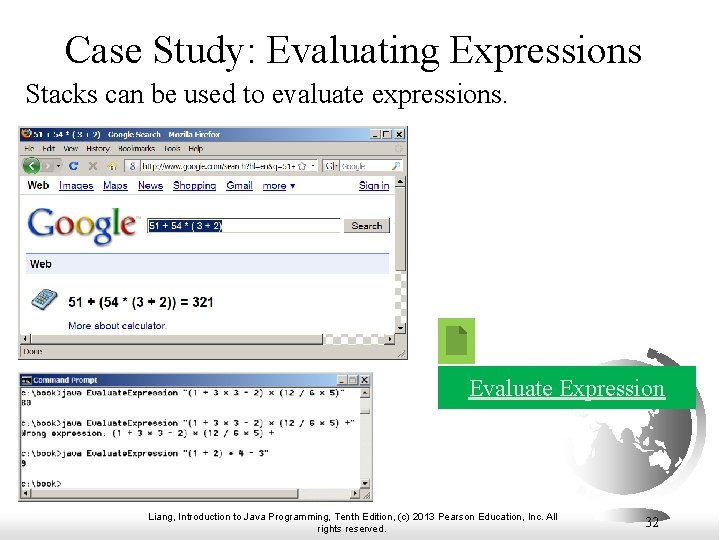
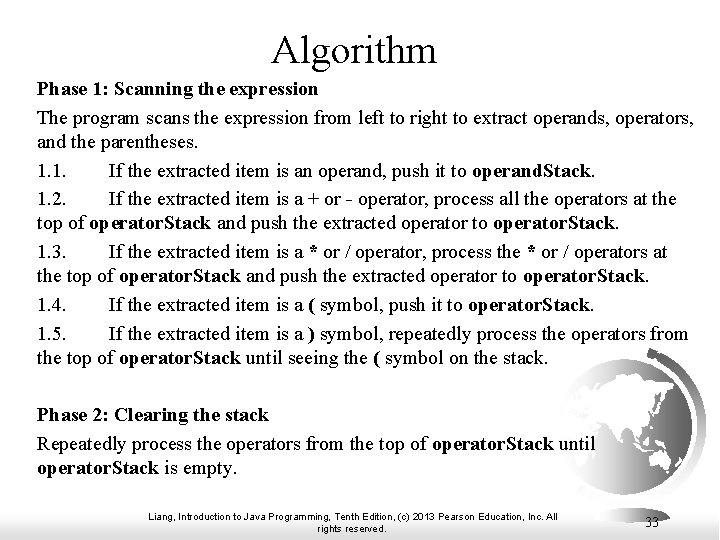
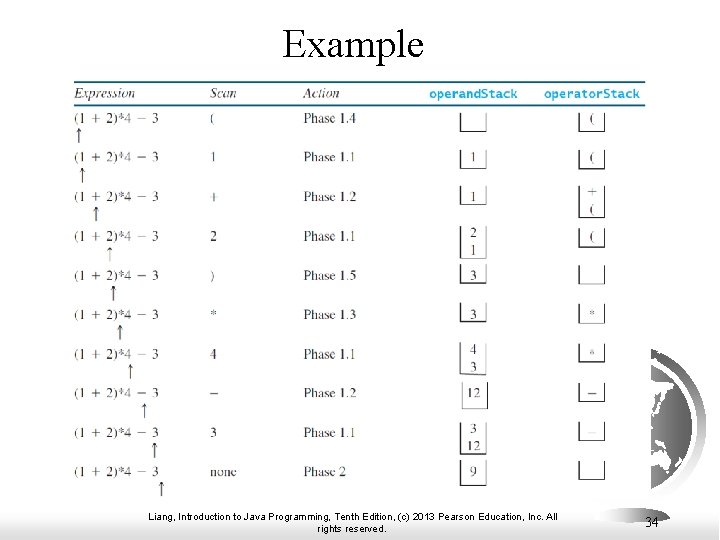
- Slides: 34
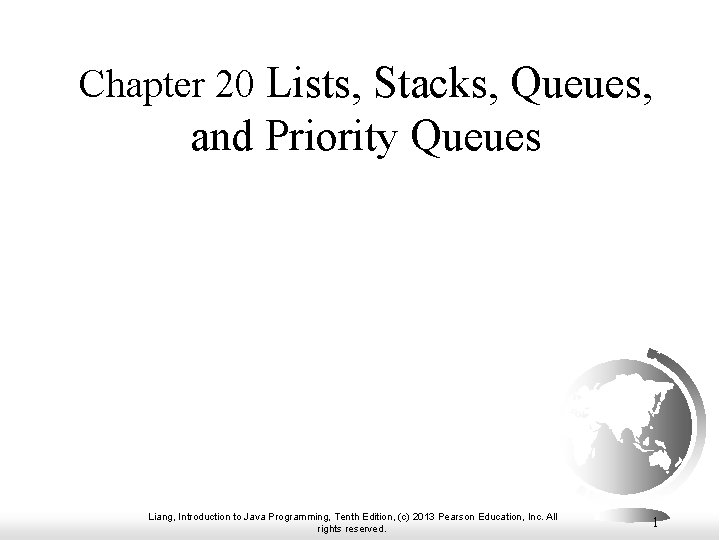
Chapter 20 Lists, Stacks, Queues, and Priority Queues Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 1
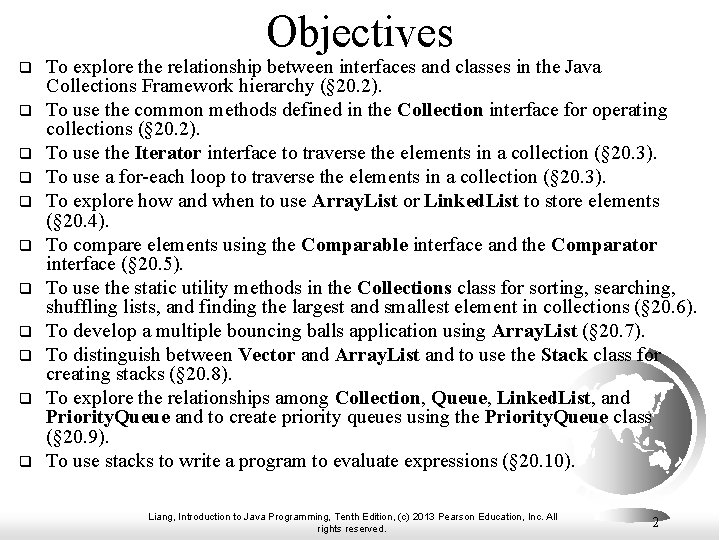
Objectives q q q To explore the relationship between interfaces and classes in the Java Collections Framework hierarchy (§ 20. 2). To use the common methods defined in the Collection interface for operating collections (§ 20. 2). To use the Iterator interface to traverse the elements in a collection (§ 20. 3). To use a for-each loop to traverse the elements in a collection (§ 20. 3). To explore how and when to use Array. List or Linked. List to store elements (§ 20. 4). To compare elements using the Comparable interface and the Comparator interface (§ 20. 5). To use the static utility methods in the Collections class for sorting, searching, shuffling lists, and finding the largest and smallest element in collections (§ 20. 6). To develop a multiple bouncing balls application using Array. List (§ 20. 7). To distinguish between Vector and Array. List and to use the Stack class for creating stacks (§ 20. 8). To explore the relationships among Collection, Queue, Linked. List, and Priority. Queue and to create priority queues using the Priority. Queue class (§ 20. 9). To use stacks to write a program to evaluate expressions (§ 20. 10). Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 2
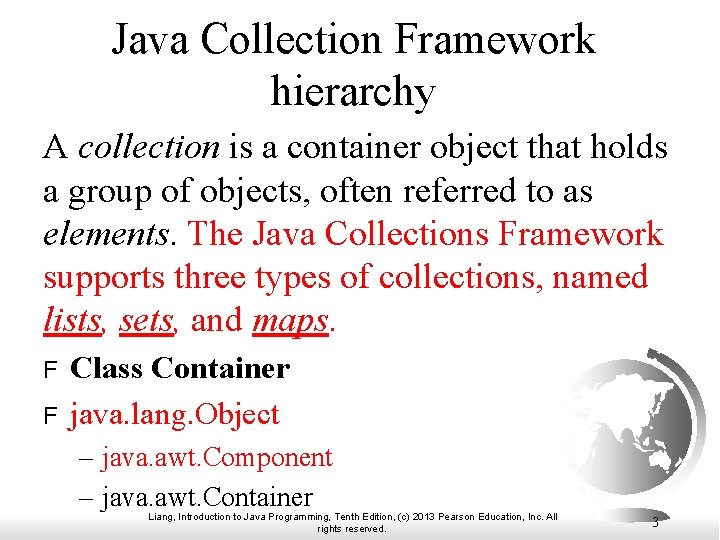
Java Collection Framework hierarchy A collection is a container object that holds a group of objects, often referred to as elements. The Java Collections Framework supports three types of collections, named lists, sets, and maps. F F Class Container java. lang. Object – java. awt. Component – java. awt. Container Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 3
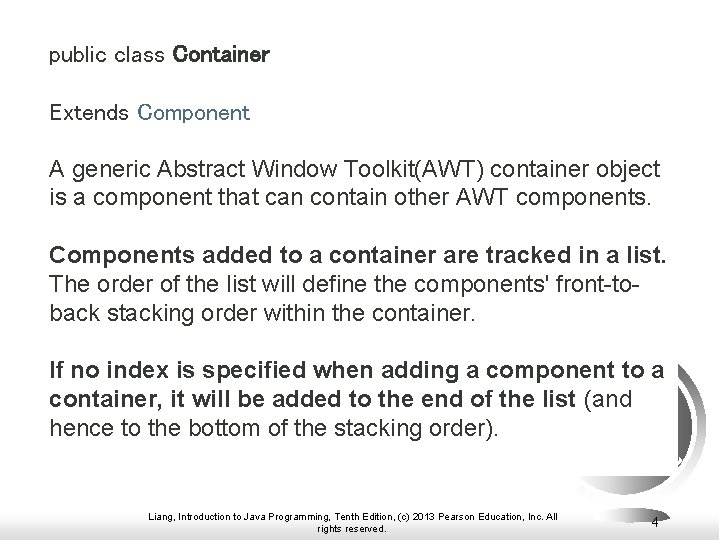
public class Container Extends Component A generic Abstract Window Toolkit(AWT) container object is a component that can contain other AWT components. Components added to a container are tracked in a list. The order of the list will define the components' front-toback stacking order within the container. If no index is specified when adding a component to a container, it will be added to the end of the list (and hence to the bottom of the stacking order). Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 4
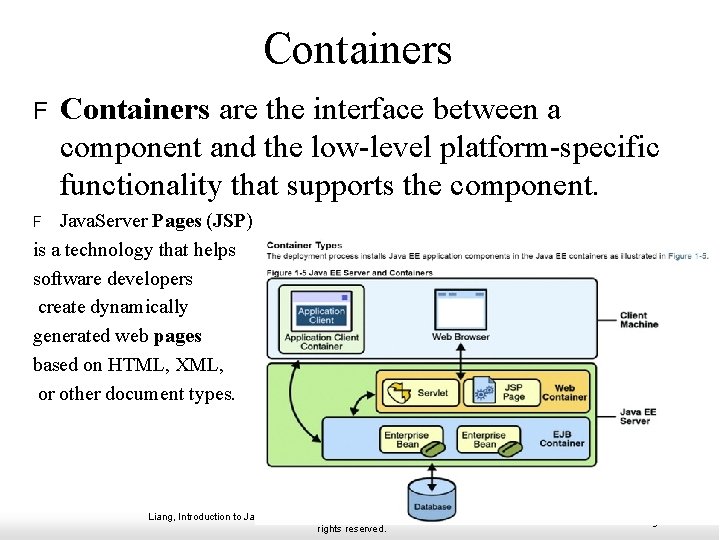
Containers F Containers are the interface between a component and the low-level platform-specific functionality that supports the component. Java. Server Pages (JSP) is a technology that helps software developers create dynamically generated web pages based on HTML, XML, or other document types. F Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 5
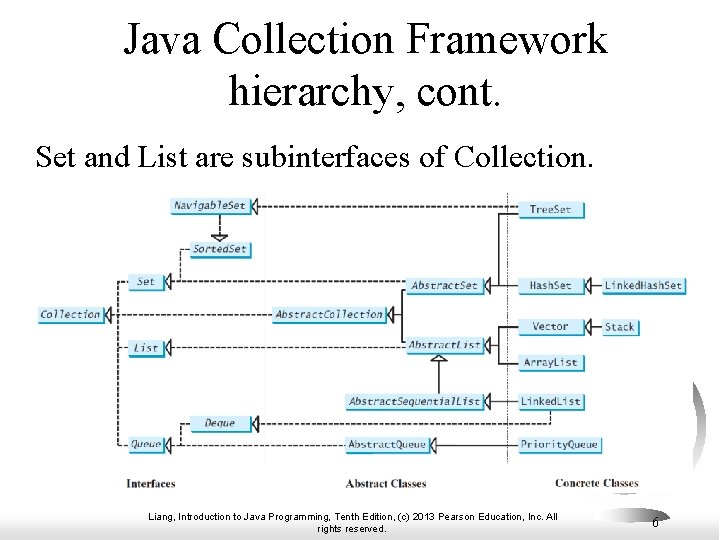
Java Collection Framework hierarchy, cont. Set and List are subinterfaces of Collection. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 6
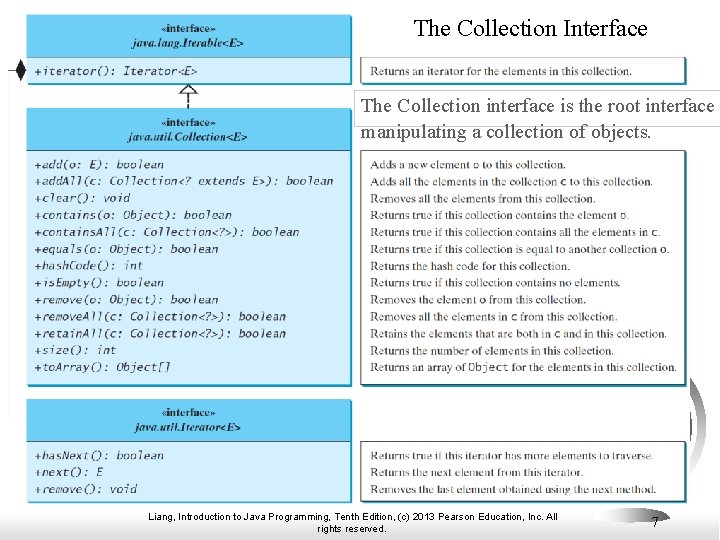
The Collection Interface The Collection interface is the root interface manipulating a collection of objects. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 7
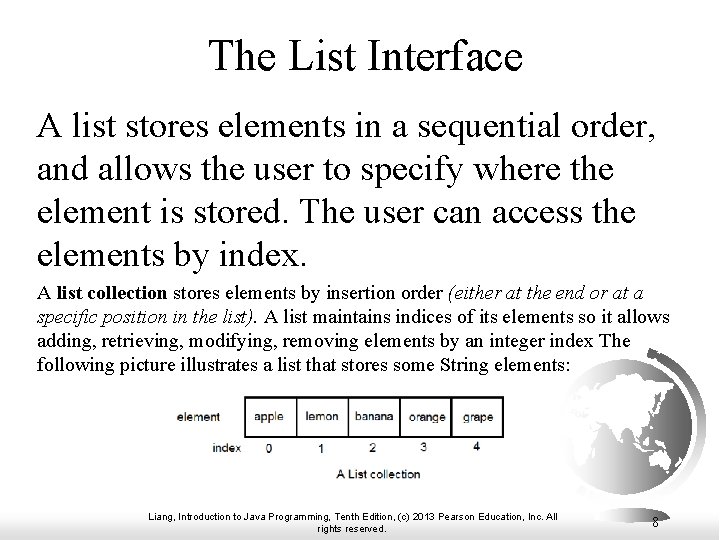
The List Interface A list stores elements in a sequential order, and allows the user to specify where the element is stored. The user can access the elements by index. A list collection stores elements by insertion order (either at the end or at a specific position in the list). A list maintains indices of its elements so it allows adding, retrieving, modifying, removing elements by an integer index The following picture illustrates a list that stores some String elements: Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 8
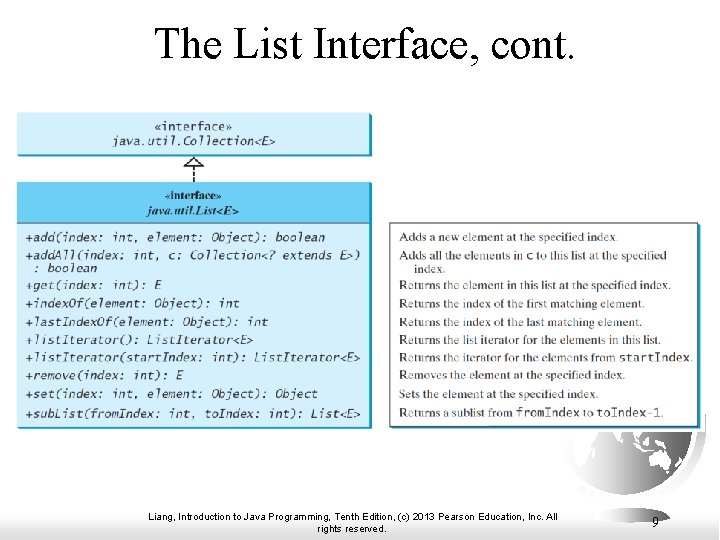
The List Interface, cont. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 9
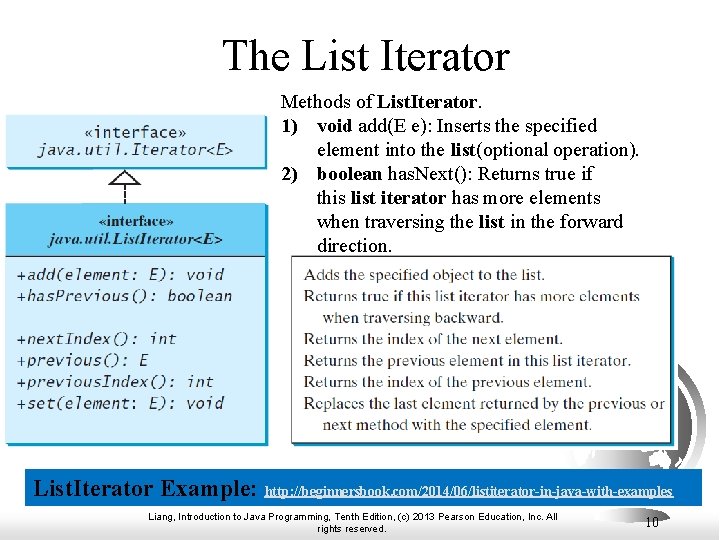
The List Iterator Methods of List. Iterator. 1) void add(E e): Inserts the specified element into the list(optional operation). 2) boolean has. Next(): Returns true if this list iterator has more elements when traversing the list in the forward direction. List. Iterator Example: http: //beginnersbook. com/2014/06/listiterator-in-java-with-examples Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 10
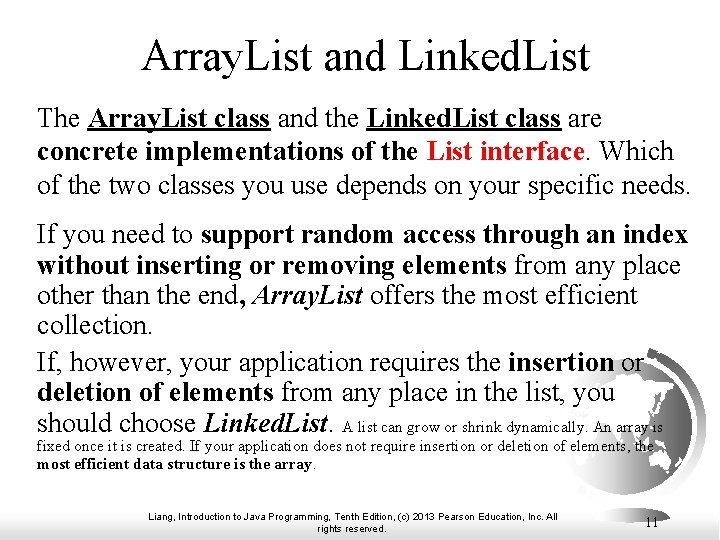
Array. List and Linked. List The Array. List class and the Linked. List class are concrete implementations of the List interface. Which of the two classes you use depends on your specific needs. If you need to support random access through an index without inserting or removing elements from any place other than the end, Array. List offers the most efficient collection. If, however, your application requires the insertion or deletion of elements from any place in the list, you should choose Linked. List. A list can grow or shrink dynamically. An array is fixed once it is created. If your application does not require insertion or deletion of elements, the most efficient data structure is the array. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 11
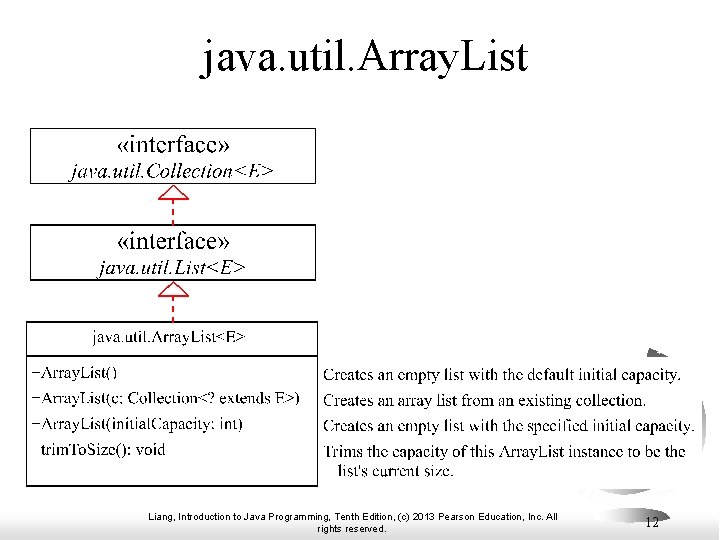
java. util. Array. List Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 12
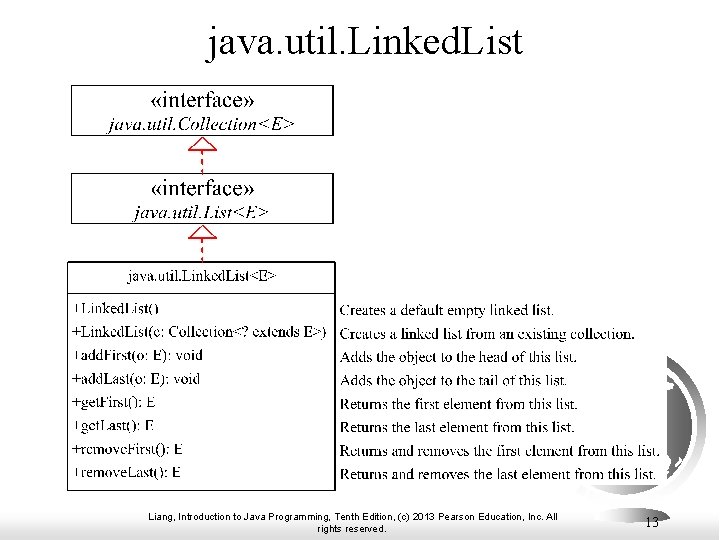
java. util. Linked. List Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 13
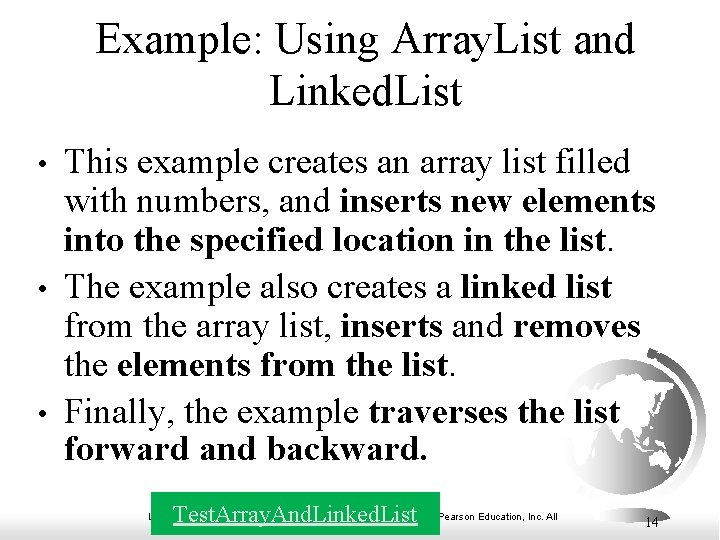
Example: Using Array. List and Linked. List • • • This example creates an array list filled with numbers, and inserts new elements into the specified location in the list. The example also creates a linked list from the array list, inserts and removes the elements from the list. Finally, the example traverses the list forward and backward. Test. Array. And. Linked. List Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 14
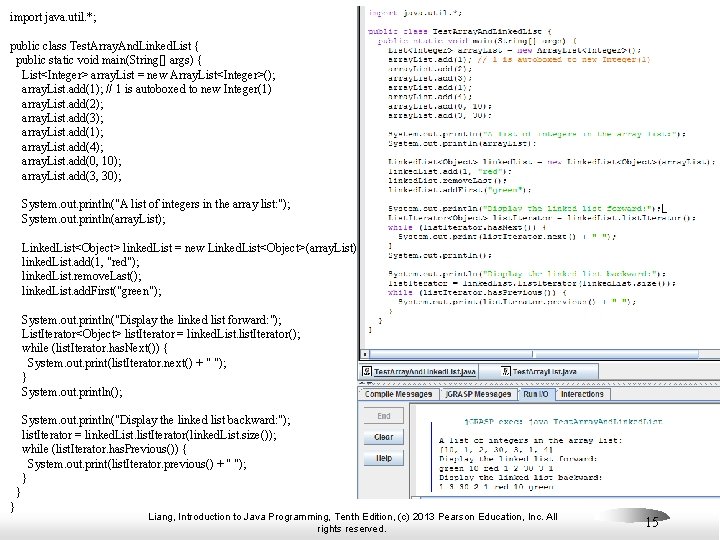
import java. util. *; public class Test. Array. And. Linked. List { public static void main(String[] args) { List<Integer> array. List = new Array. List<Integer>(); array. List. add(1); // 1 is autoboxed to new Integer(1) array. List. add(2); array. List. add(3); array. List. add(1); array. List. add(4); array. List. add(0, 10); array. List. add(3, 30); System. out. println("A list of integers in the array list: "); System. out. println(array. List); Linked. List<Object> linked. List = new Linked. List<Object>(array. List); linked. List. add(1, "red"); linked. List. remove. Last(); linked. List. add. First("green"); System. out. println("Display the linked list forward: "); List. Iterator<Object> list. Iterator = linked. List. list. Iterator(); while (list. Iterator. has. Next()) { System. out. print(list. Iterator. next() + " "); } System. out. println(); System. out. println("Display the linked list backward: "); list. Iterator = linked. List. list. Iterator(linked. List. size()); while (list. Iterator. has. Previous()) { System. out. print(list. Iterator. previous() + " "); } } } Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 15
![public class Test Array List public static void mainString args Create public class Test. Array. List { public static void main(String[] args) { // Create](https://slidetodoc.com/presentation_image_h2/5f384ed4766067848e70329fc779d291/image-16.jpg)
public class Test. Array. List { public static void main(String[] args) { // Create a list to store cities java. util. Array. List city. List = new java. util. Array. List(); // Remove a city at index 1 city. List. remove(1); // contains [London, Xian, Paris, Seoul, Tokyo] // Add some cities in the list city. List. add("London"); // city. List now contains [London] city. List. add("Denver"); // city. List now contains [London, Denver] city. List. add("Paris"); // city. List now contains [London, Denver, Paris] city. List. add("Miami"); // city. List now contains [London, Denver, Paris, Miami] city. List. add("Seoul"); // contains [London, Denver, Paris, Miami, Seoul] city. List. add("Tokyo"); // contains [London, Denver, Paris, Miami, Seoul, Tokyo] System. out. println("List size? " + city. List. size()); System. out. println("Is Miami in the list? " + city. List. contains("Miami")); System. out. println("The location of Denver in the list? " + city. List. index. Of("Denver")); System. out. println("Is the list empty? " + city. List. is. Empty()); // Print false // Display the contents in the list System. out. println(city. List. to. String()); // Display the contents in the list in reverse order for (int i = city. List. size() - 1; i >= 0; i--) System. out. print(city. List. get(i) + " "); System. out. println(); // Create a list to store two circles java. util. Array. List list = new java. util. Array. List(); // Add two circles list. add(new Circle 4(2)); list. add(new Circle 4(3)); // Display the area of the first circle in the list System. out. println("The area of the circle? " + ((Circle 4)list. get(0)). get. Area()); } } // Insert a new city at index 2 city. List. add(2, "Xian"); // contains [London, Denver, Xian, Paris, Miami, Seoul, Tokyo] // Remove a city from the list city. List. remove("Miami"); // contains [London, Denver, Xian, Paris, Seoul, Tokyo] Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 16
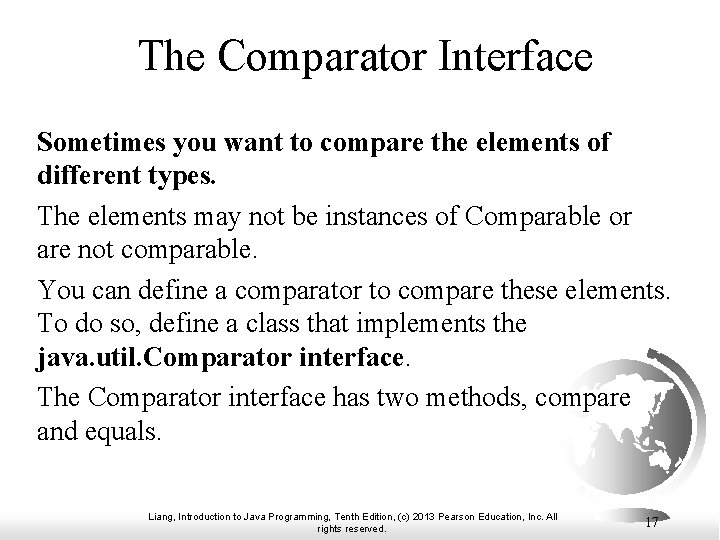
The Comparator Interface Sometimes you want to compare the elements of different types. The elements may not be instances of Comparable or are not comparable. You can define a comparator to compare these elements. To do so, define a class that implements the java. util. Comparator interface. The Comparator interface has two methods, compare and equals. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 17
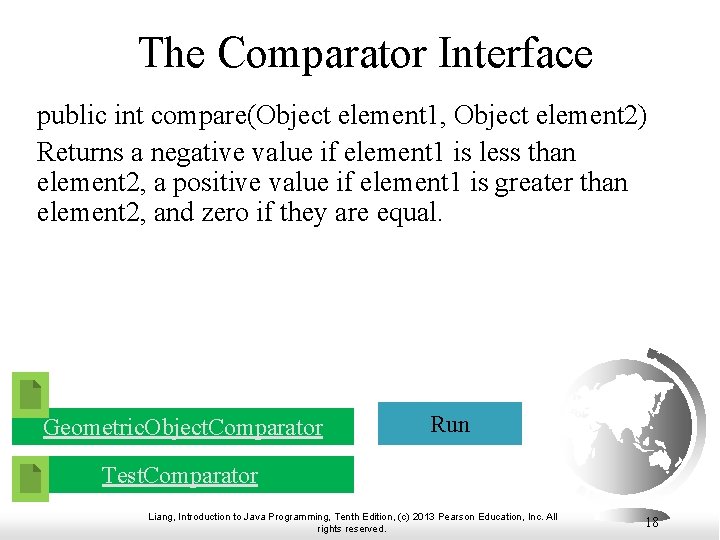
The Comparator Interface public int compare(Object element 1, Object element 2) Returns a negative value if element 1 is less than element 2, a positive value if element 1 is greater than element 2, and zero if they are equal. Geometric. Object. Comparator Run Test. Comparator Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 18
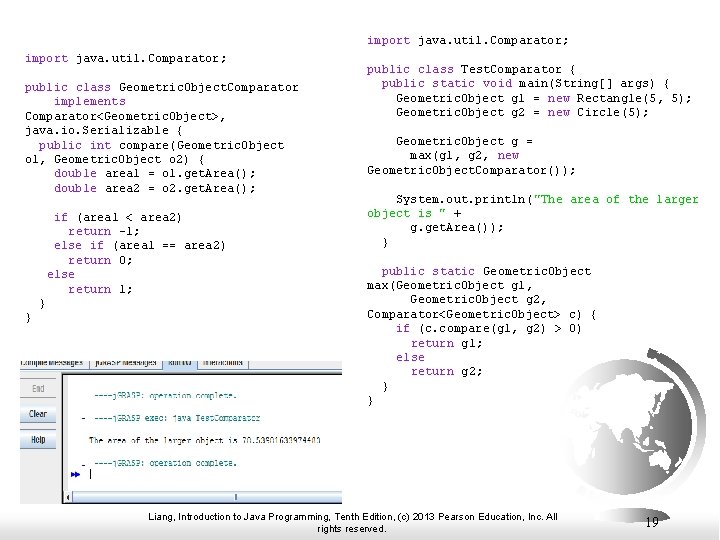
import java. util. Comparator; public class Geometric. Object. Comparator implements Comparator<Geometric. Object>, java. io. Serializable { public int compare(Geometric. Object o 1, Geometric. Object o 2) { double area 1 = o 1. get. Area(); double area 2 = o 2. get. Area(); if (area 1 < area 2) return -1; else if (area 1 == area 2) return 0; else return 1; } } public class Test. Comparator { public static void main(String[] args) { Geometric. Object g 1 = new Rectangle(5, 5); Geometric. Object g 2 = new Circle(5); Geometric. Object g = max(g 1, g 2, new Geometric. Object. Comparator()); System. out. println("The area of the larger object is " + g. get. Area()); } public static Geometric. Object max(Geometric. Object g 1, Geometric. Object g 2, Comparator<Geometric. Object> c) { if (c. compare(g 1, g 2) > 0) return g 1; else return g 2; } } Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 19
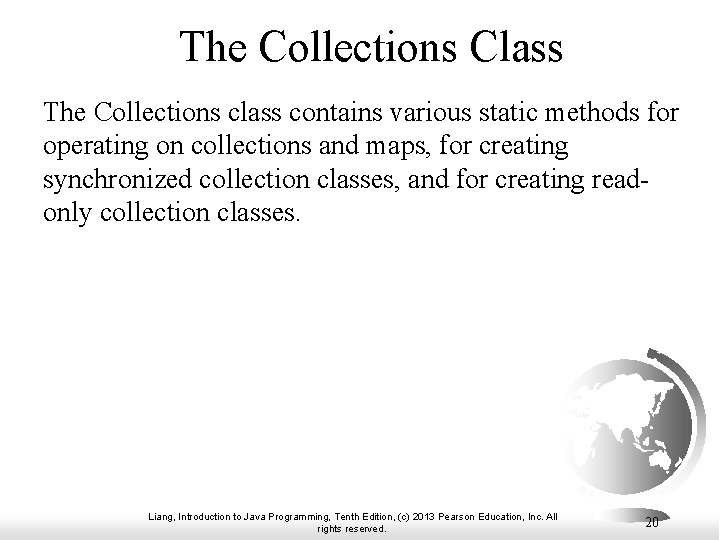
The Collections Class The Collections class contains various static methods for operating on collections and maps, for creating synchronized collection classes, and for creating readonly collection classes. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 20
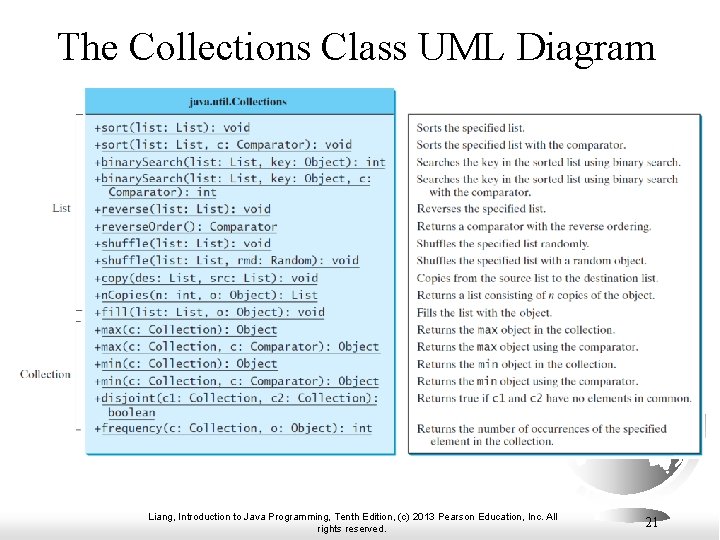
The Collections Class UML Diagram Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 21
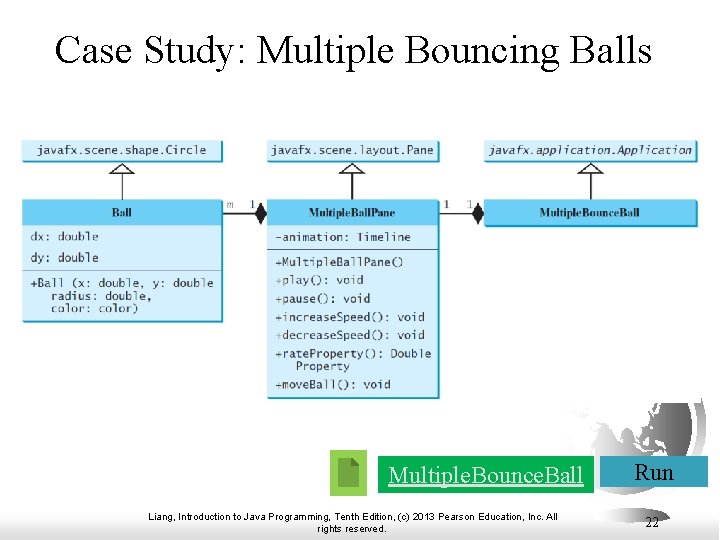
Case Study: Multiple Bouncing Balls Multiple. Bounce. Ball Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. Run 22
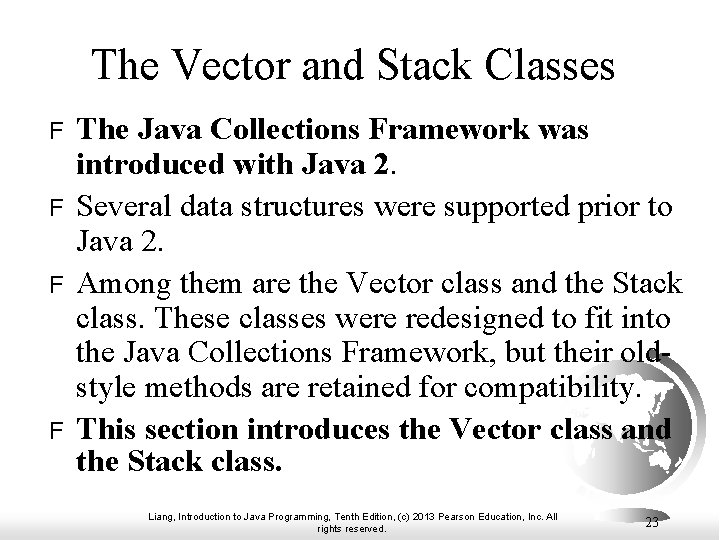
The Vector and Stack Classes F F The Java Collections Framework was introduced with Java 2. Several data structures were supported prior to Java 2. Among them are the Vector class and the Stack class. These classes were redesigned to fit into the Java Collections Framework, but their oldstyle methods are retained for compatibility. This section introduces the Vector class and the Stack class. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 23
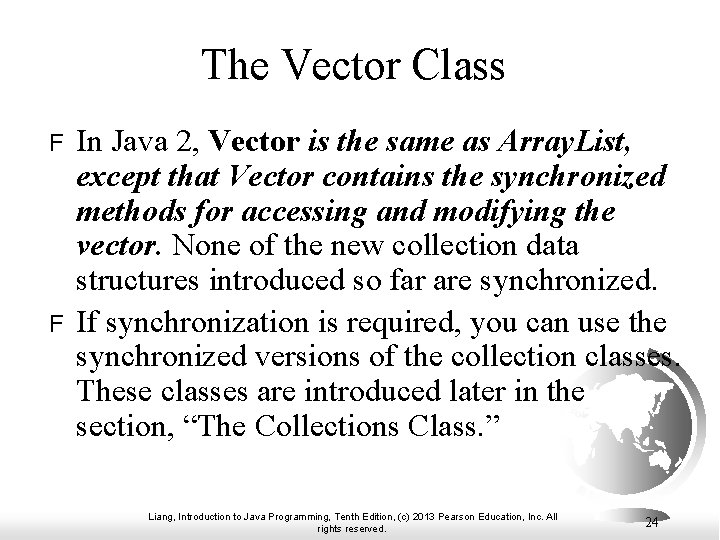
The Vector Class F F In Java 2, Vector is the same as Array. List, except that Vector contains the synchronized methods for accessing and modifying the vector. None of the new collection data structures introduced so far are synchronized. If synchronization is required, you can use the synchronized versions of the collection classes. These classes are introduced later in the section, “The Collections Class. ” Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 24
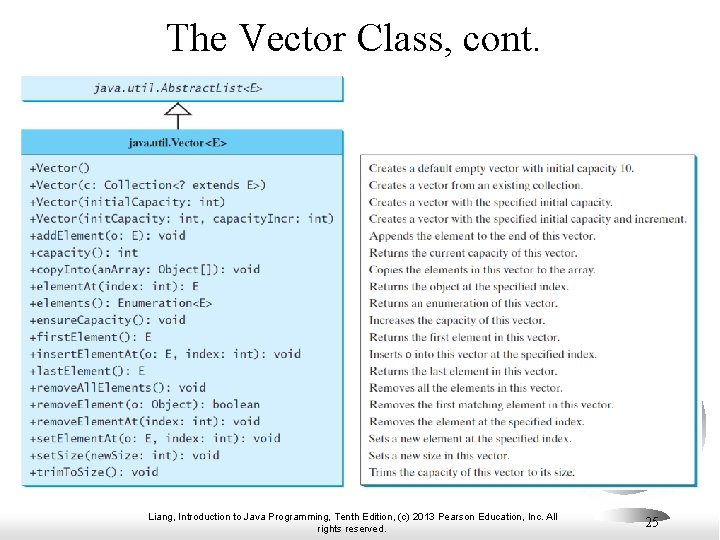
The Vector Class, cont. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 25
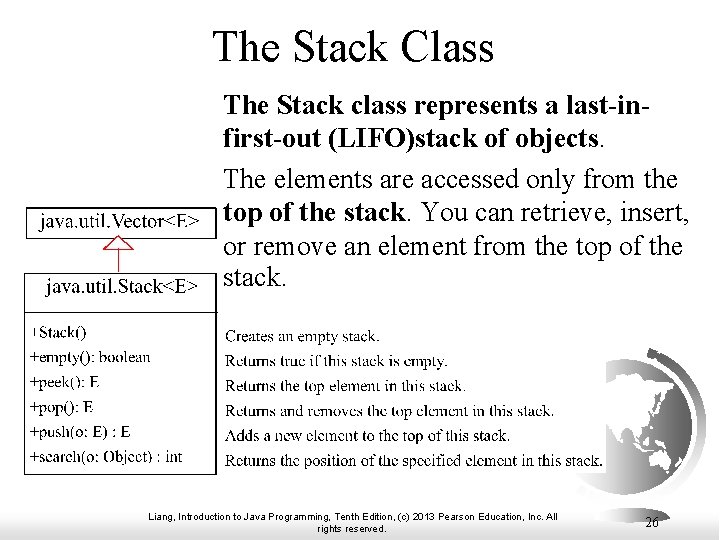
The Stack Class The Stack class represents a last-infirst-out (LIFO)stack of objects. The elements are accessed only from the top of the stack. You can retrieve, insert, or remove an element from the top of the stack. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 26
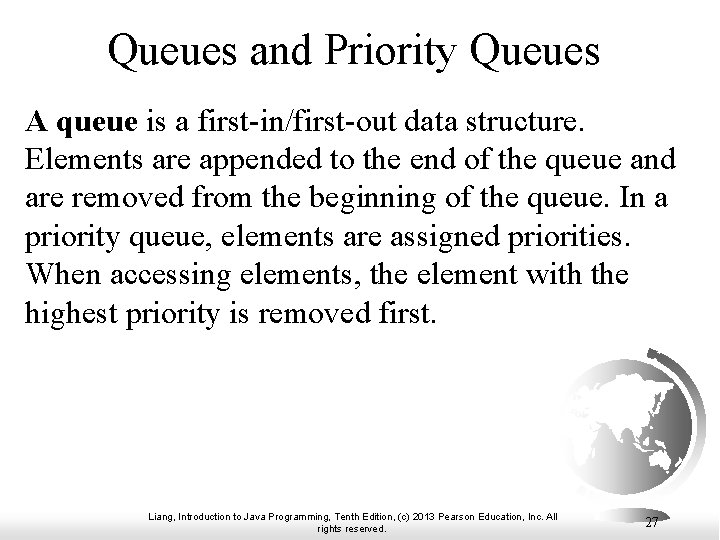
Queues and Priority Queues A queue is a first-in/first-out data structure. Elements are appended to the end of the queue and are removed from the beginning of the queue. In a priority queue, elements are assigned priorities. When accessing elements, the element with the highest priority is removed first. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 27
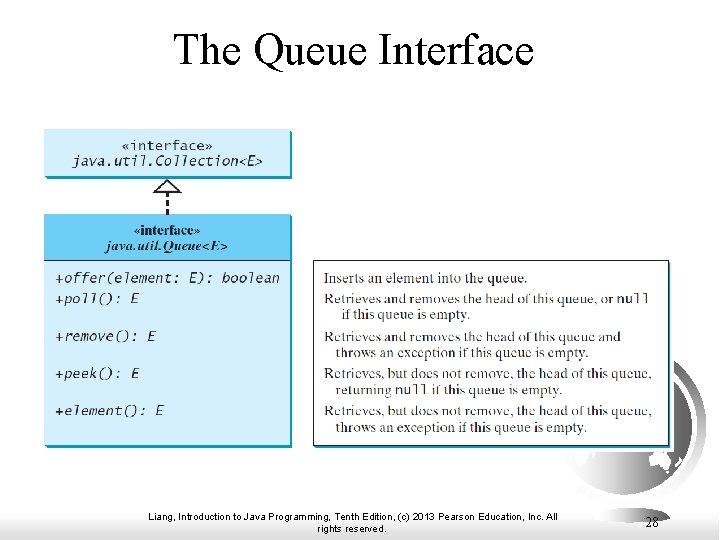
The Queue Interface Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 28
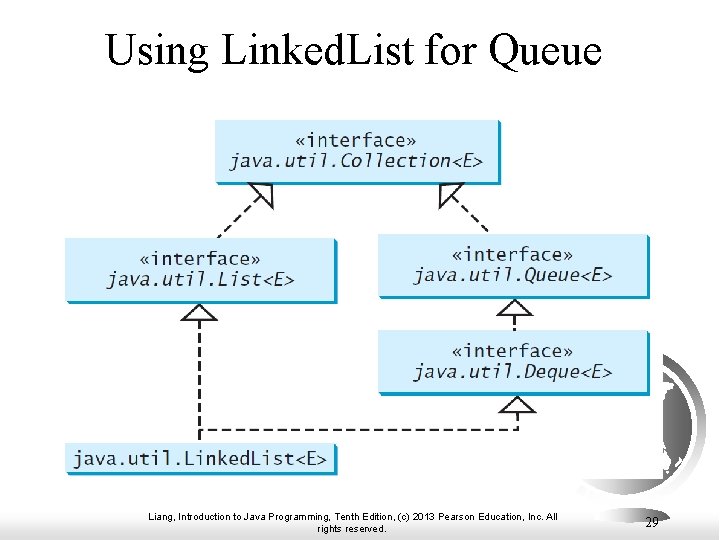
Using Linked. List for Queue Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 29
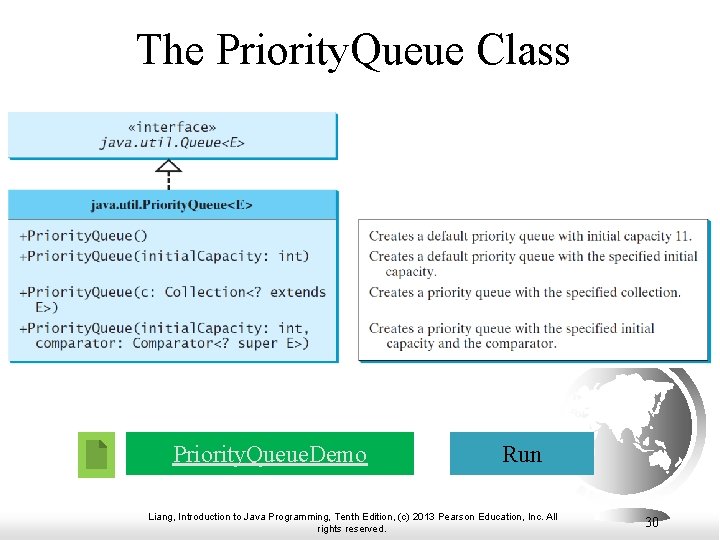
The Priority. Queue Class Priority. Queue. Demo Run Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 30
![import java util public class Priority Queue Demo public static void mainString import java. util. *; public class Priority. Queue. Demo { public static void main(String[]](https://slidetodoc.com/presentation_image_h2/5f384ed4766067848e70329fc779d291/image-31.jpg)
import java. util. *; public class Priority. Queue. Demo { public static void main(String[] args) { Priority. Queue<String> queue 1 = new Priority. Queue<String>(); queue 1. offer("Oklahoma"); queue 1. offer("Indiana"); queue 1. offer("Georgia"); queue 1. offer("Texas"); System. out. println("Priority queue using Comparable: "); while (queue 1. size() > 0) { System. out. print(queue 1. remove() + " "); } Priority. Queue<String> queue 2 = new Priority. Queue<String>( 4, Collections. reverse. Order()); queue 2. offer("Oklahoma"); queue 2. offer("Indiana"); queue 2. offer("Georgia"); queue 2. offer("Texas"); System. out. println("n. Priority queue using Comparator: "); while (queue 2. size() > 0) { System. out. print(queue 2. remove() + " "); } } } Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 31
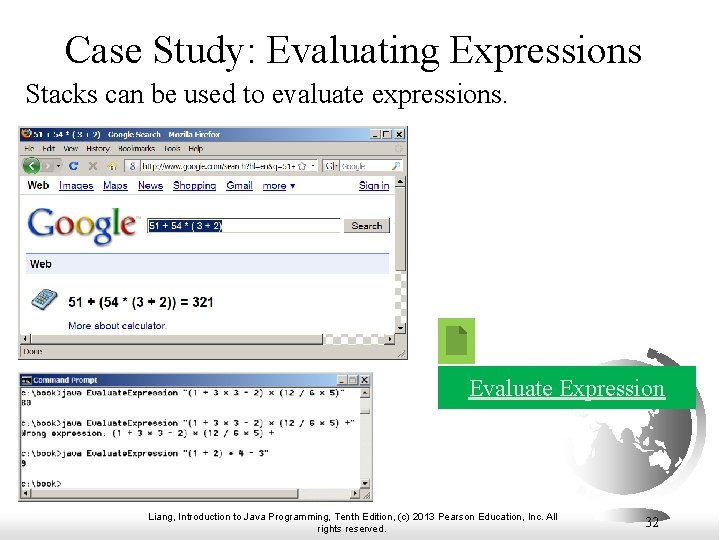
Case Study: Evaluating Expressions Stacks can be used to evaluate expressions. Evaluate Expression Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 32
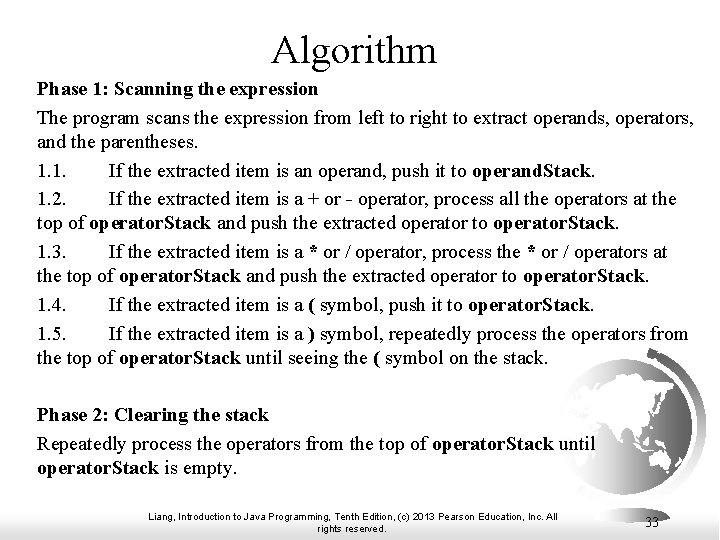
Algorithm Phase 1: Scanning the expression The program scans the expression from left to right to extract operands, operators, and the parentheses. 1. 1. If the extracted item is an operand, push it to operand. Stack. 1. 2. If the extracted item is a + or - operator, process all the operators at the top of operator. Stack and push the extracted operator to operator. Stack. 1. 3. If the extracted item is a * or / operator, process the * or / operators at the top of operator. Stack and push the extracted operator to operator. Stack. 1. 4. If the extracted item is a ( symbol, push it to operator. Stack. 1. 5. If the extracted item is a ) symbol, repeatedly process the operators from the top of operator. Stack until seeing the ( symbol on the stack. Phase 2: Clearing the stack Repeatedly process the operators from the top of operator. Stack until operator. Stack is empty. Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 33
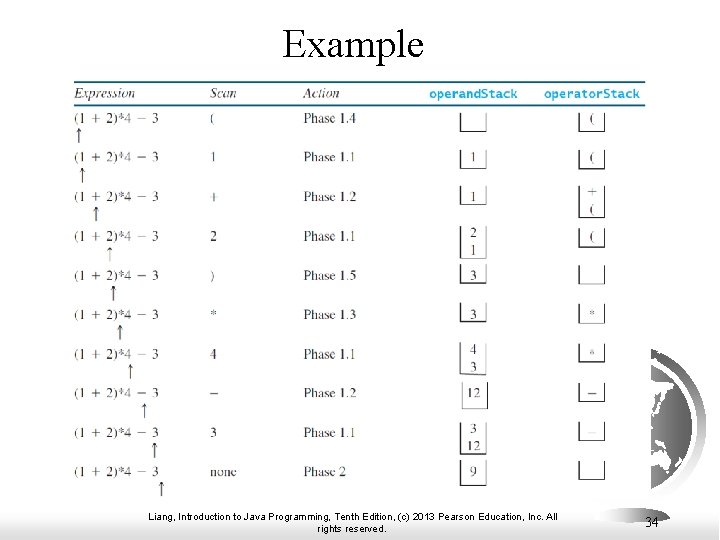
Example Liang, Introduction to Java Programming, Tenth Edition, (c) 2013 Pearson Education, Inc. All rights reserved. 34