Chapter 20 Lists Stacks Queues and Priority Queues
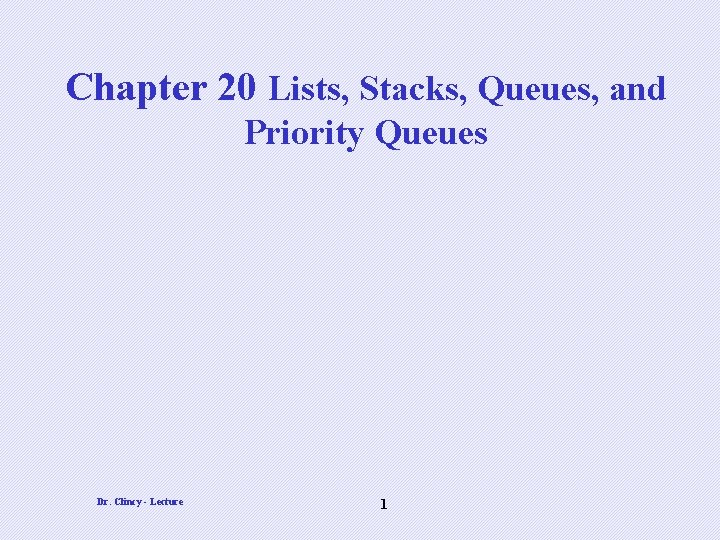
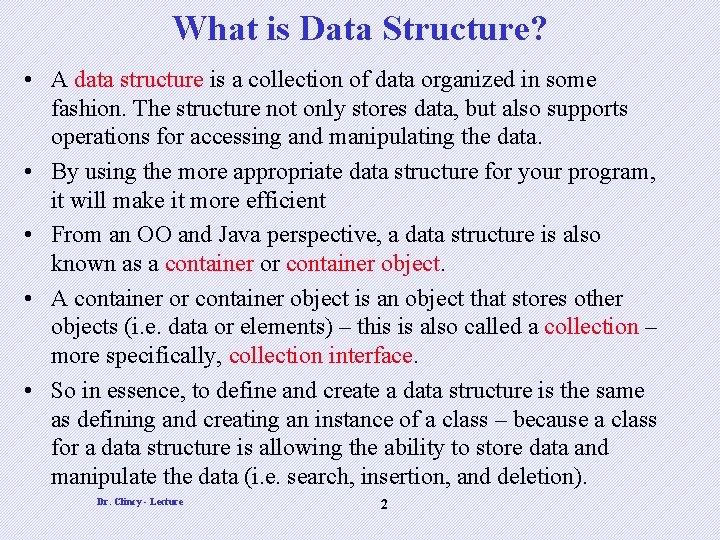
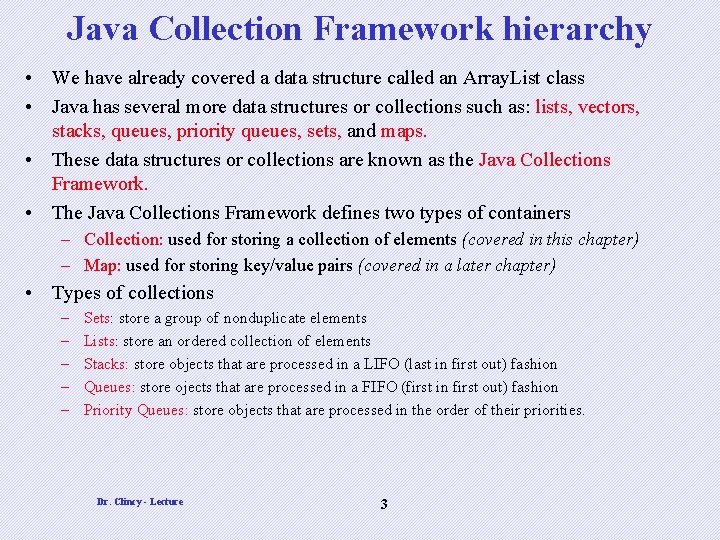
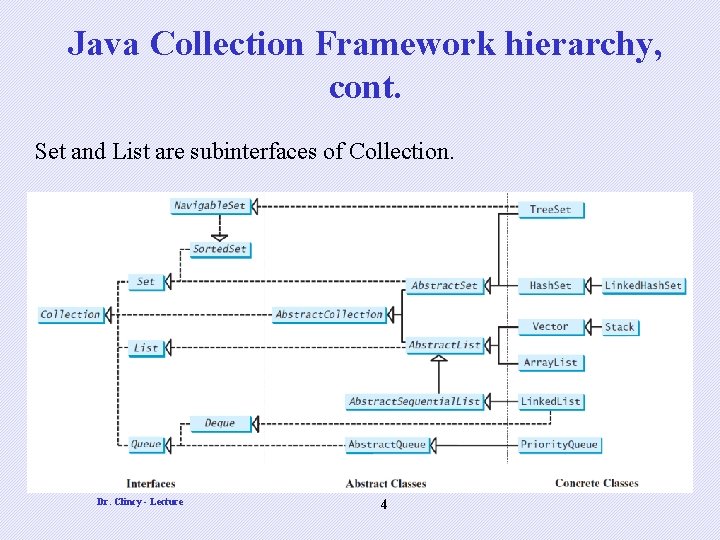
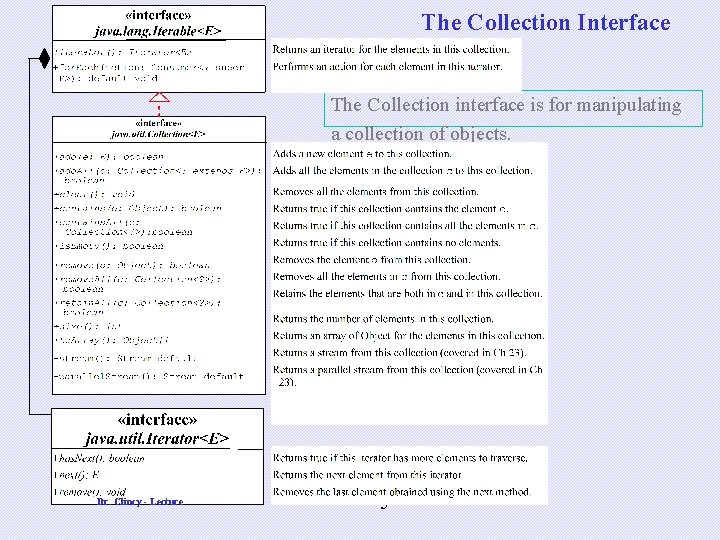
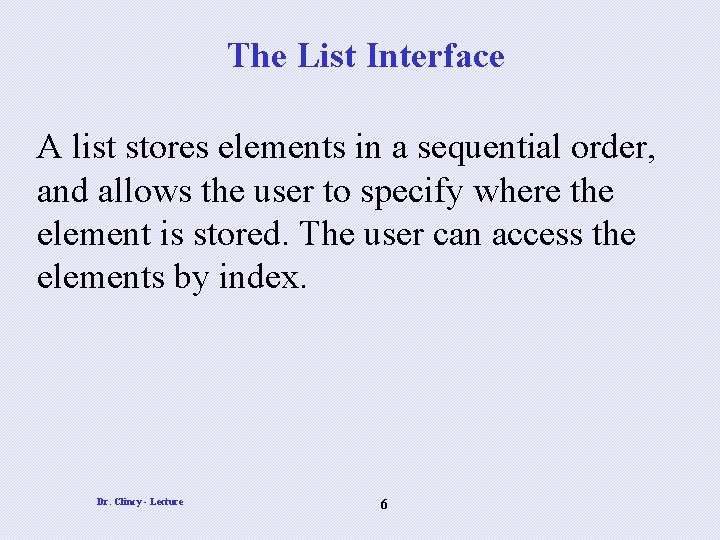
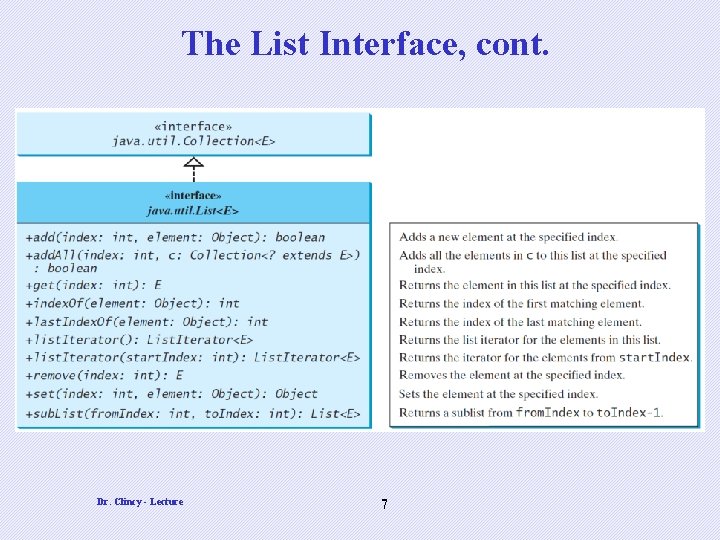
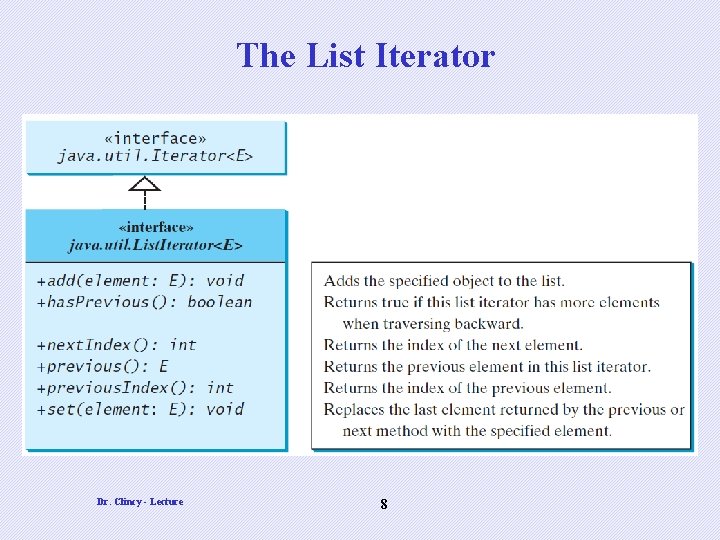
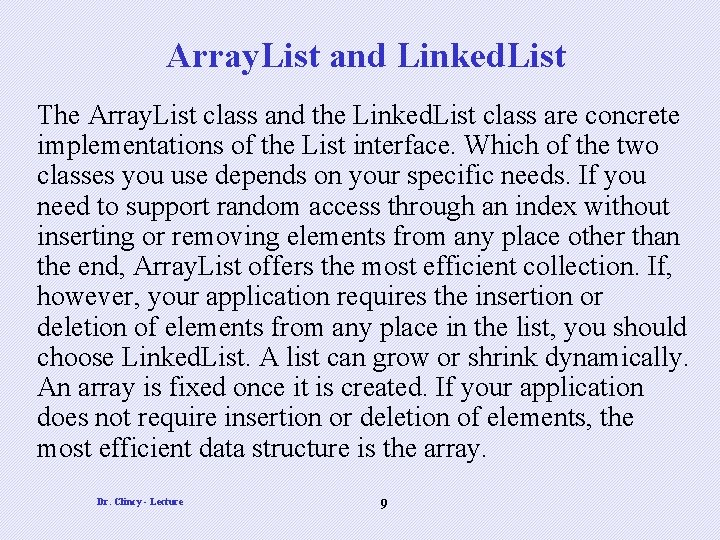
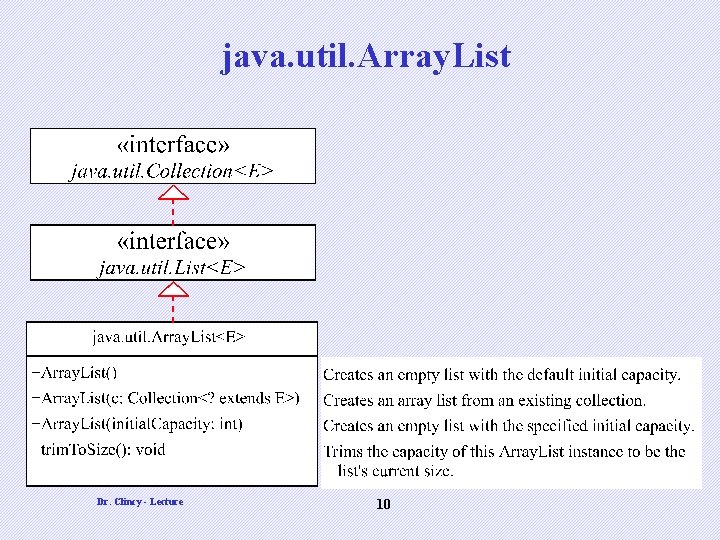
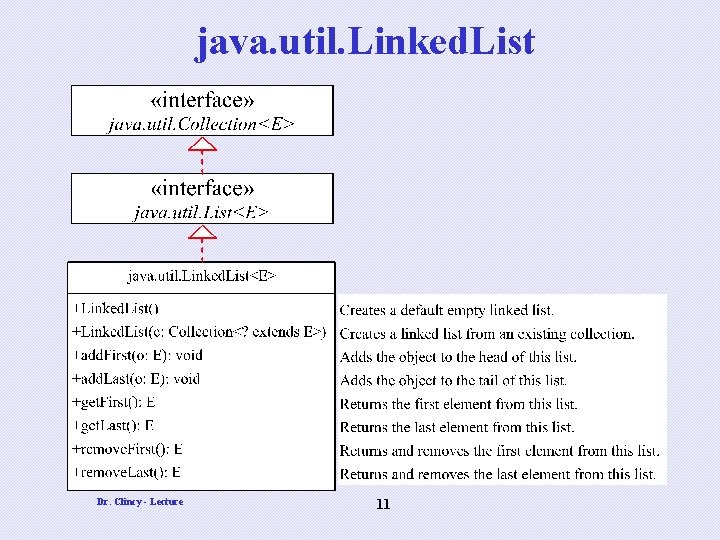
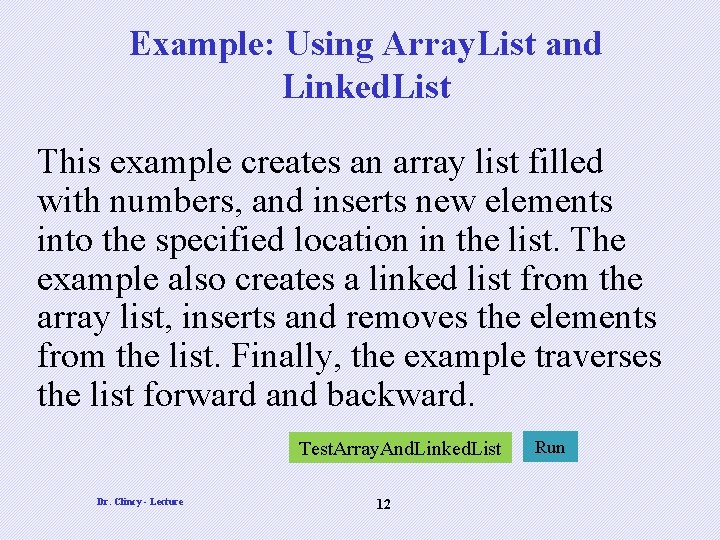
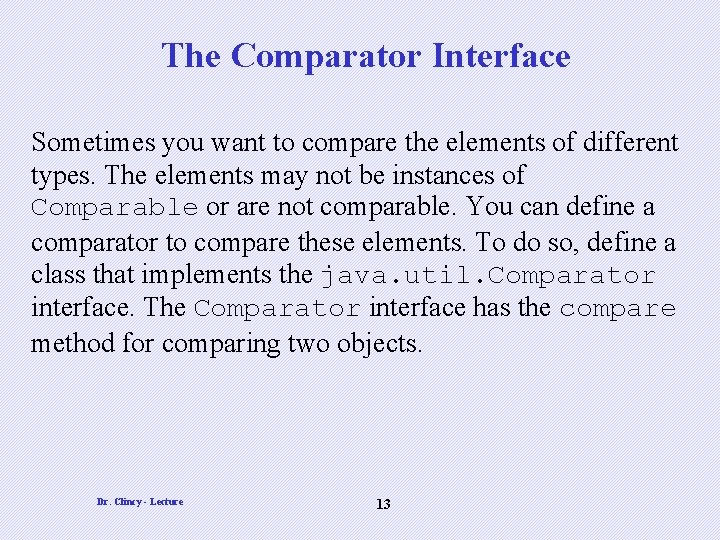
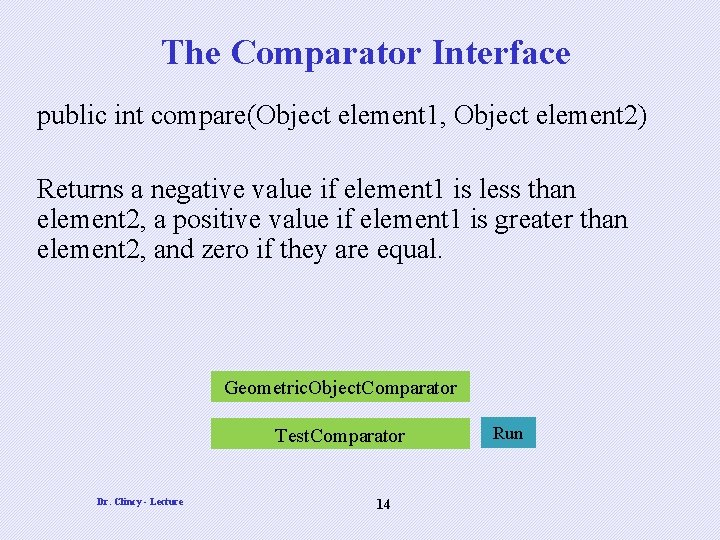
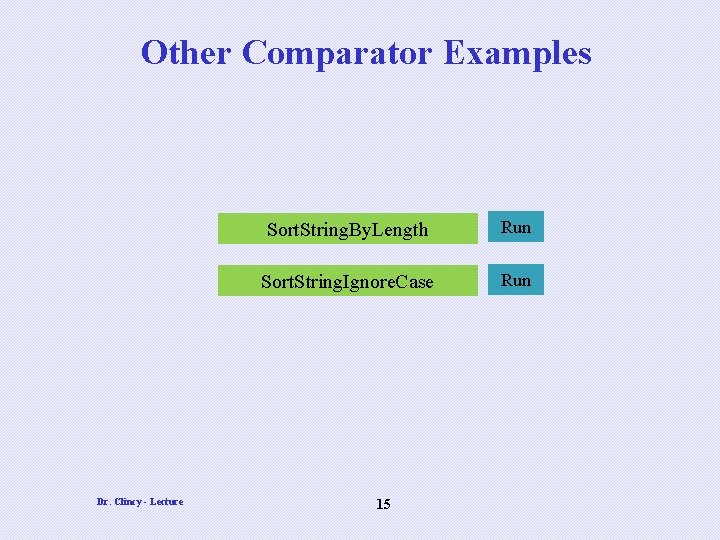
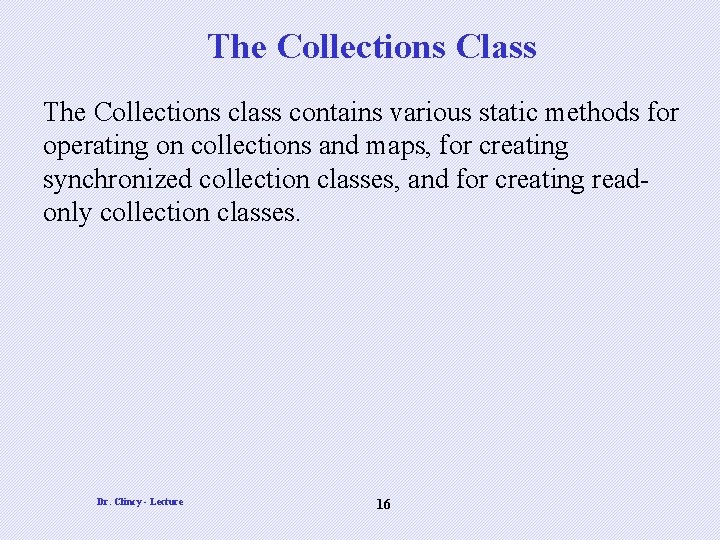
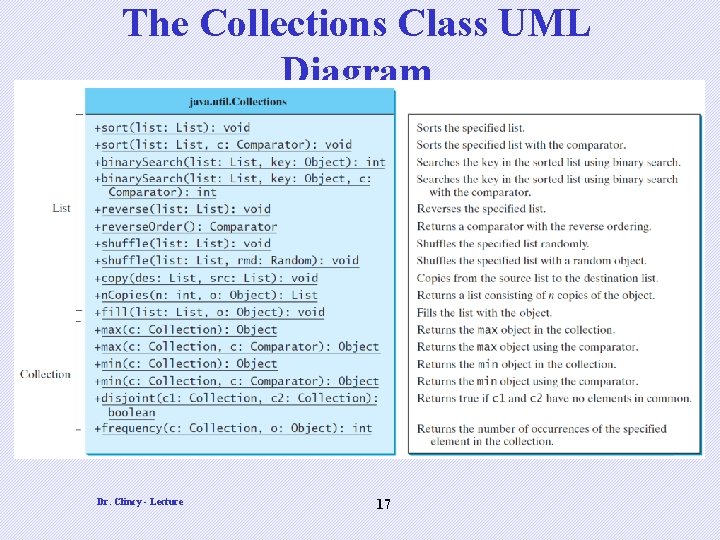
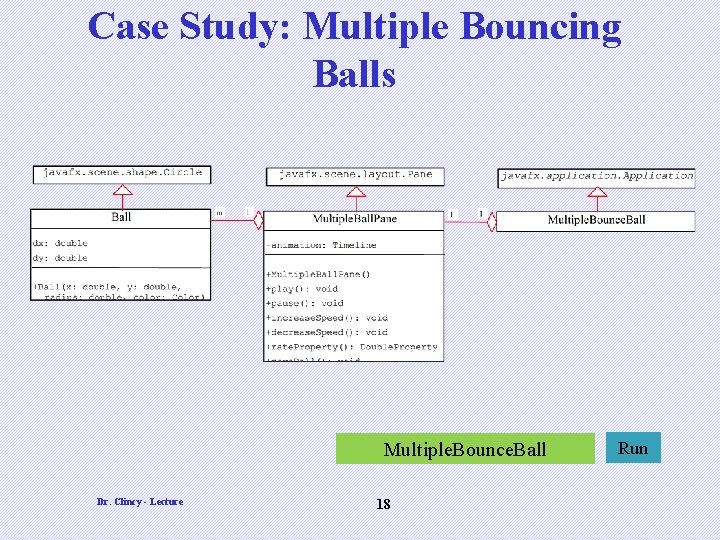
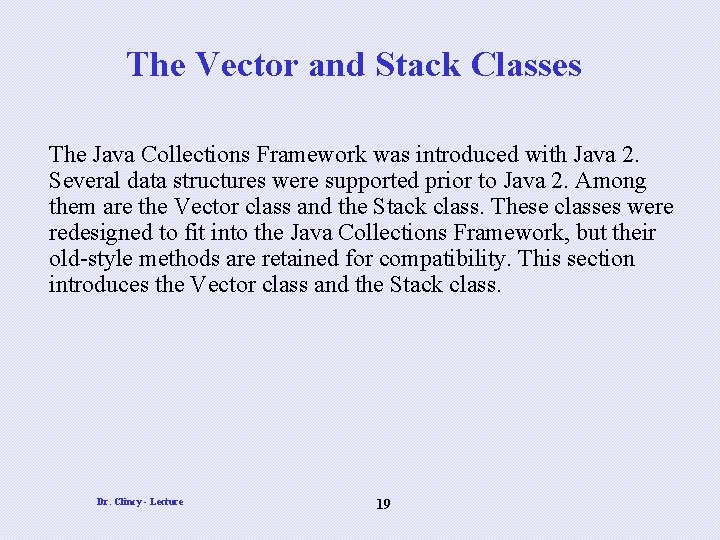
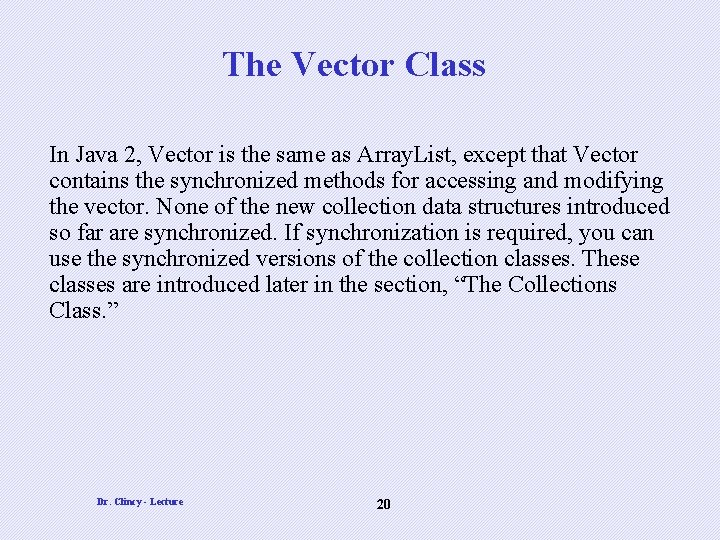
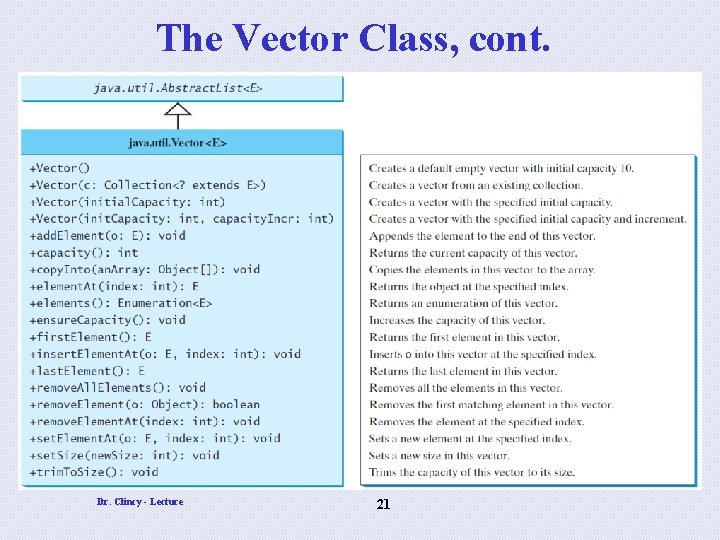
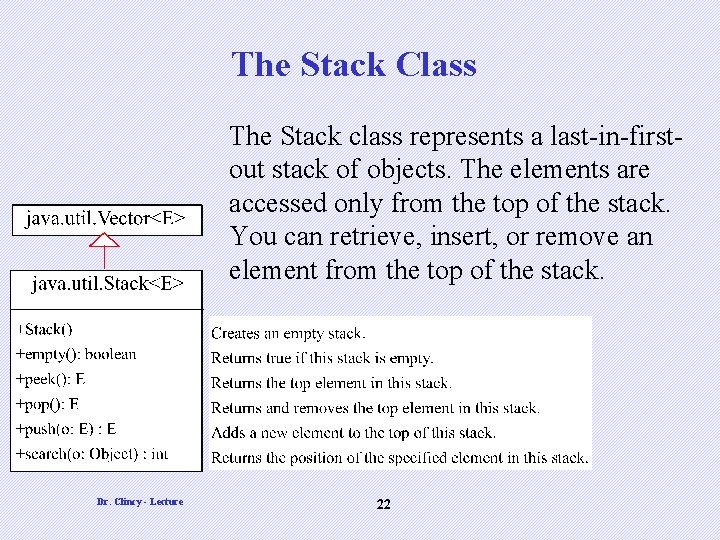
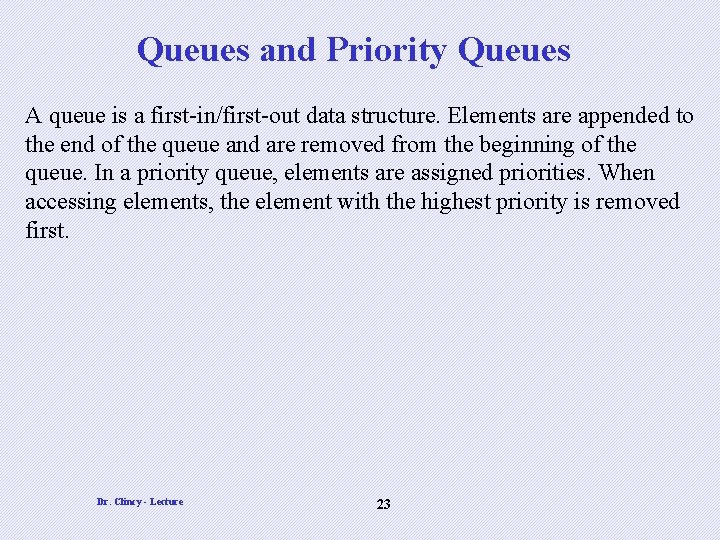
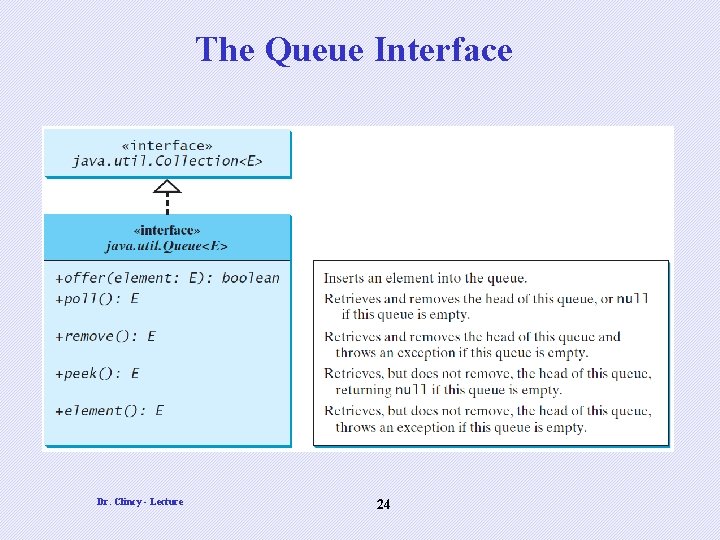
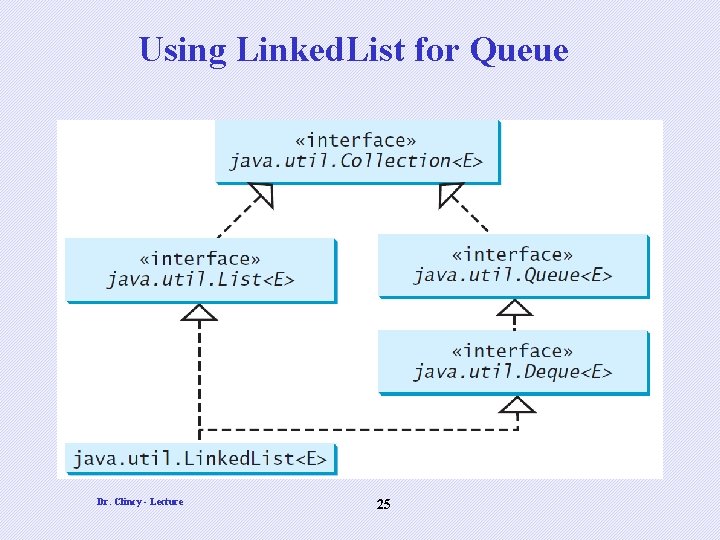
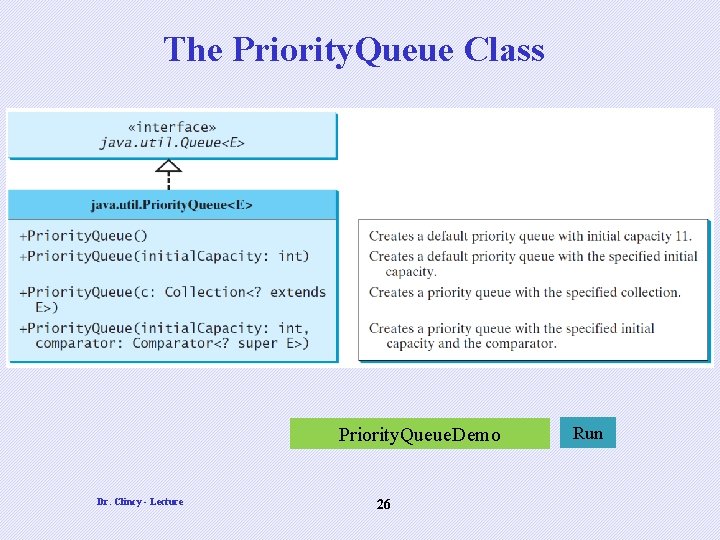
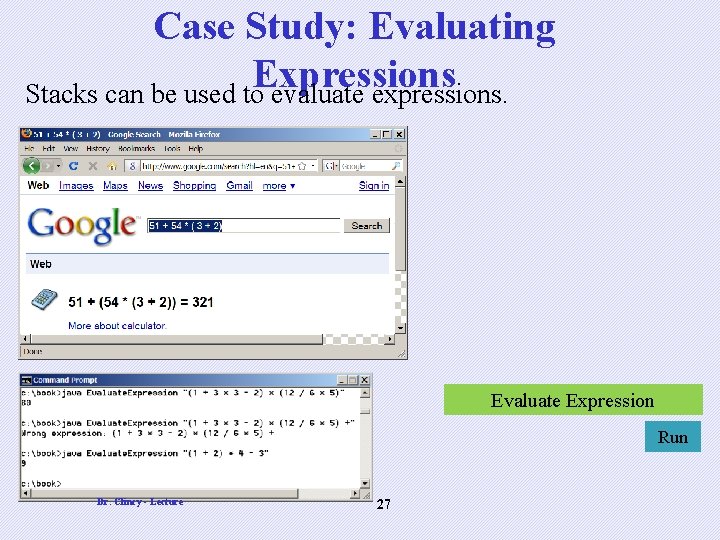
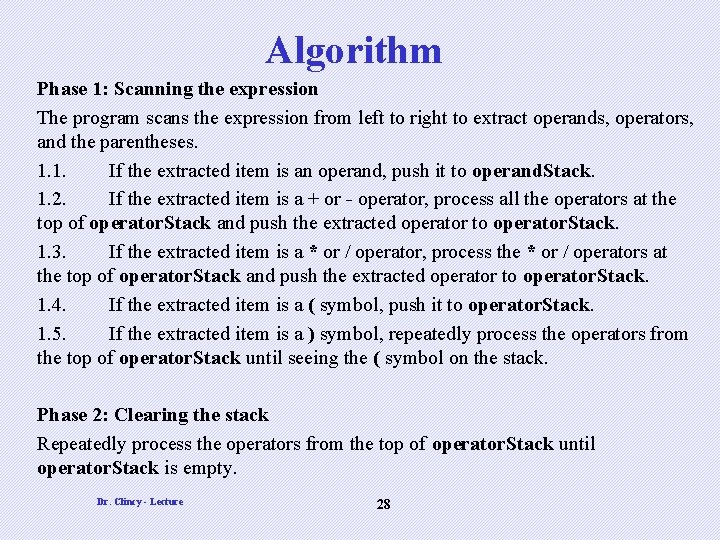
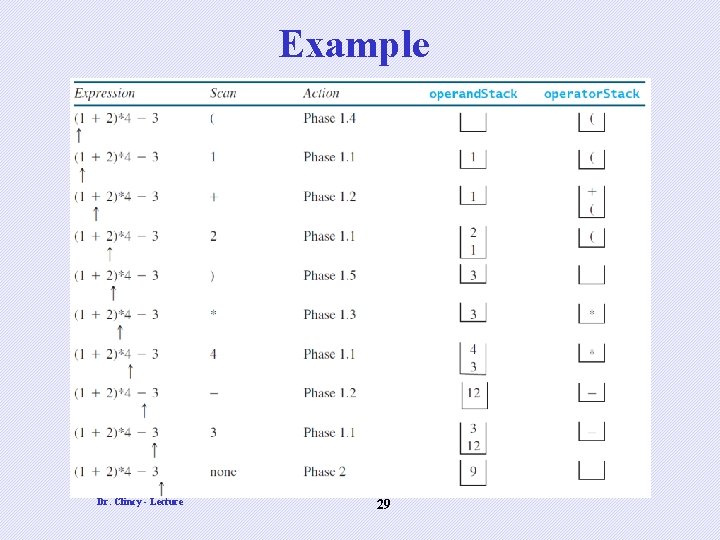
- Slides: 29
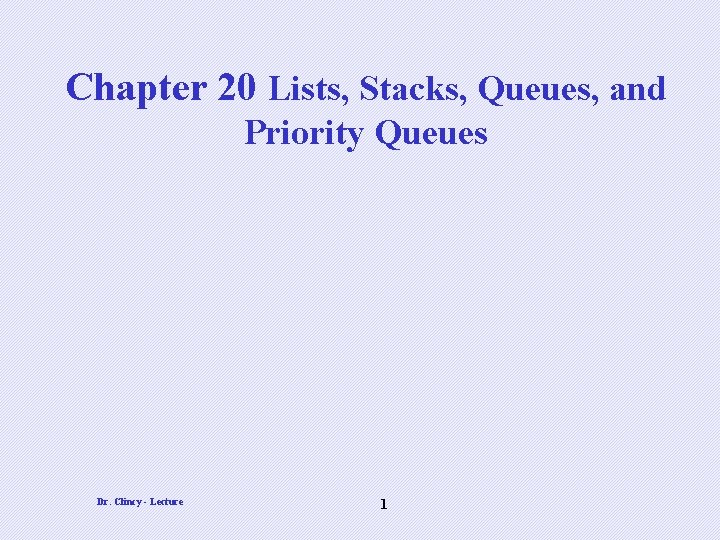
Chapter 20 Lists, Stacks, Queues, and Priority Queues Dr. Clincy - Lecture 1
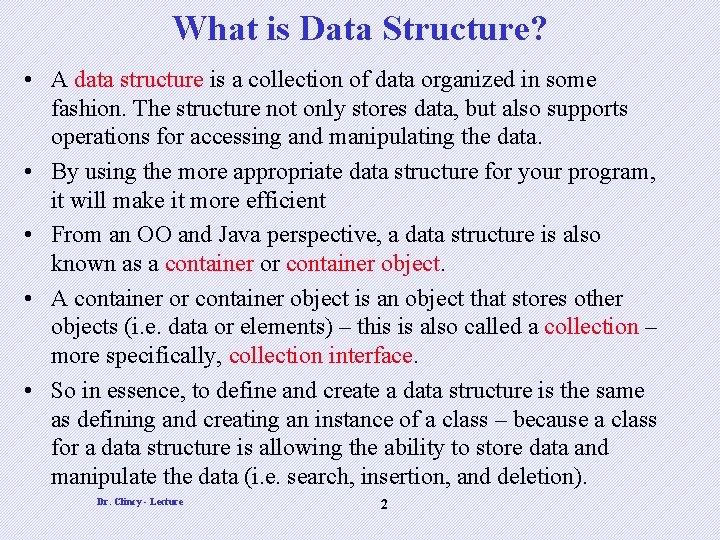
What is Data Structure? • A data structure is a collection of data organized in some fashion. The structure not only stores data, but also supports operations for accessing and manipulating the data. • By using the more appropriate data structure for your program, it will make it more efficient • From an OO and Java perspective, a data structure is also known as a container or container object. • A container or container object is an object that stores other objects (i. e. data or elements) – this is also called a collection – more specifically, collection interface. • So in essence, to define and create a data structure is the same as defining and creating an instance of a class – because a class for a data structure is allowing the ability to store data and manipulate the data (i. e. search, insertion, and deletion). Dr. Clincy - Lecture 2
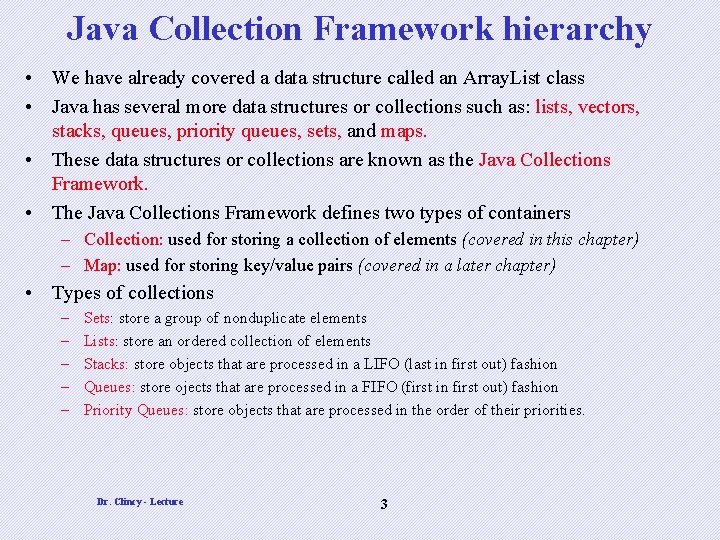
Java Collection Framework hierarchy • We have already covered a data structure called an Array. List class • Java has several more data structures or collections such as: lists, vectors, stacks, queues, priority queues, sets, and maps. • These data structures or collections are known as the Java Collections Framework. • The Java Collections Framework defines two types of containers – Collection: used for storing a collection of elements (covered in this chapter) – Map: used for storing key/value pairs (covered in a later chapter) • Types of collections – – – Sets: store a group of nonduplicate elements Lists: store an ordered collection of elements Stacks: store objects that are processed in a LIFO (last in first out) fashion Queues: store ojects that are processed in a FIFO (first in first out) fashion Priority Queues: store objects that are processed in the order of their priorities. Dr. Clincy - Lecture 3
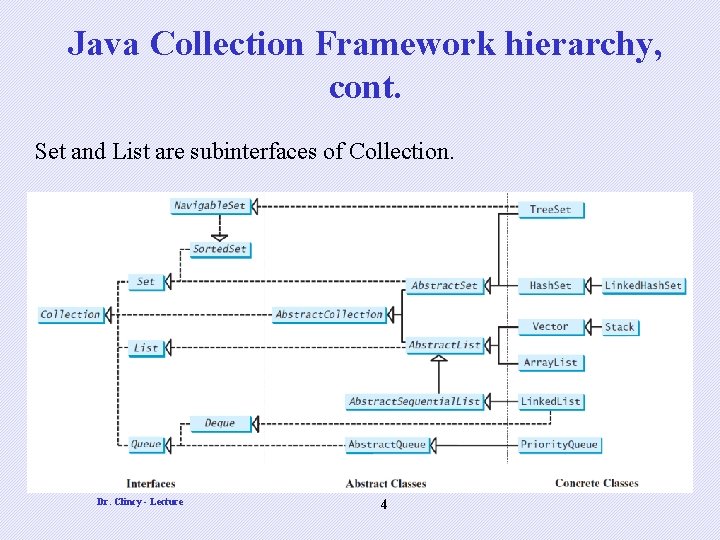
Java Collection Framework hierarchy, cont. Set and List are subinterfaces of Collection. Dr. Clincy - Lecture 4
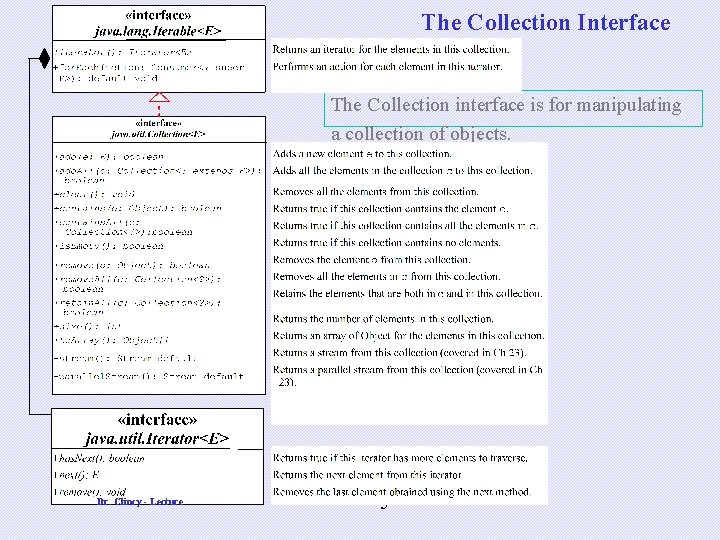
The Collection Interface The Collection interface is for manipulating a collection of objects. Dr. Clincy - Lecture 5
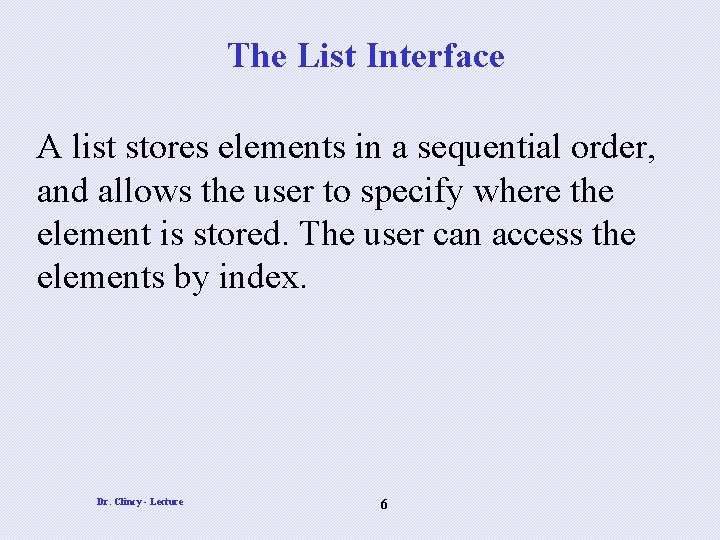
The List Interface A list stores elements in a sequential order, and allows the user to specify where the element is stored. The user can access the elements by index. Dr. Clincy - Lecture 6
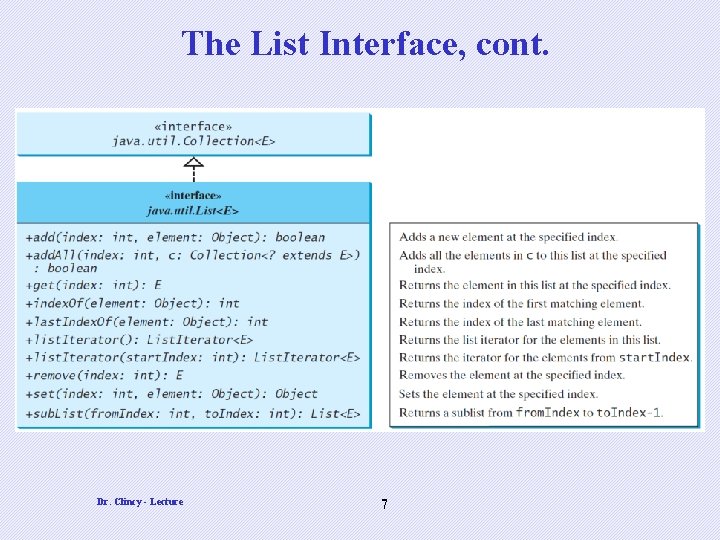
The List Interface, cont. Dr. Clincy - Lecture 7
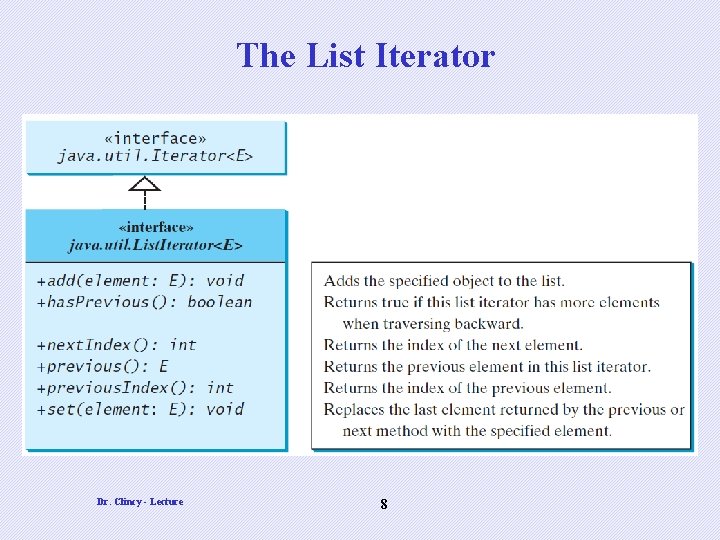
The List Iterator Dr. Clincy - Lecture 8
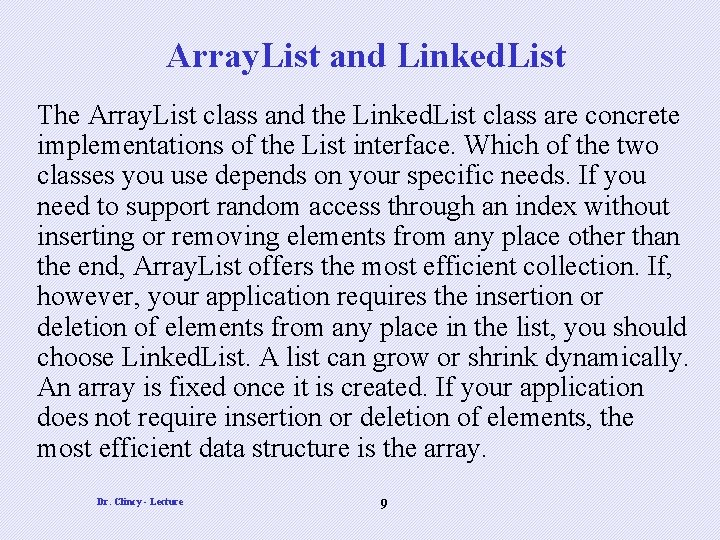
Array. List and Linked. List The Array. List class and the Linked. List class are concrete implementations of the List interface. Which of the two classes you use depends on your specific needs. If you need to support random access through an index without inserting or removing elements from any place other than the end, Array. List offers the most efficient collection. If, however, your application requires the insertion or deletion of elements from any place in the list, you should choose Linked. List. A list can grow or shrink dynamically. An array is fixed once it is created. If your application does not require insertion or deletion of elements, the most efficient data structure is the array. Dr. Clincy - Lecture 9
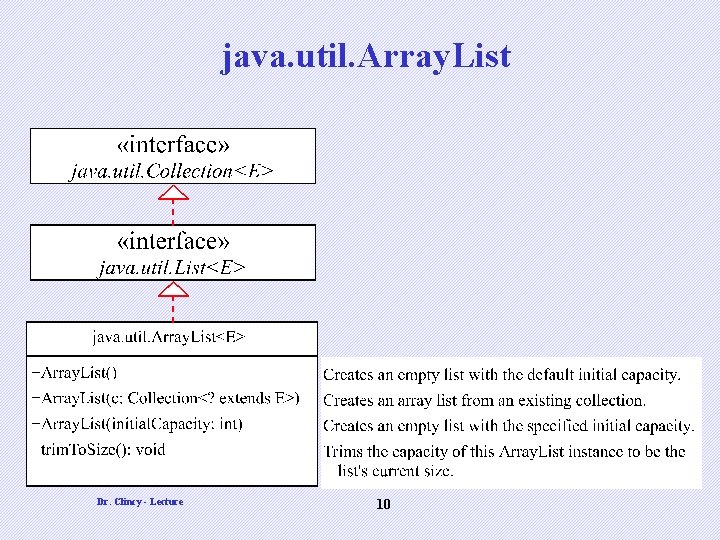
java. util. Array. List Dr. Clincy - Lecture 10
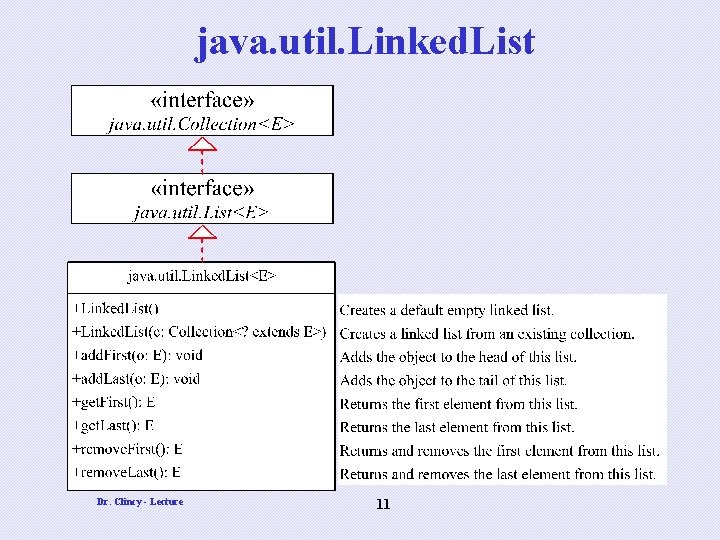
java. util. Linked. List Dr. Clincy - Lecture 11
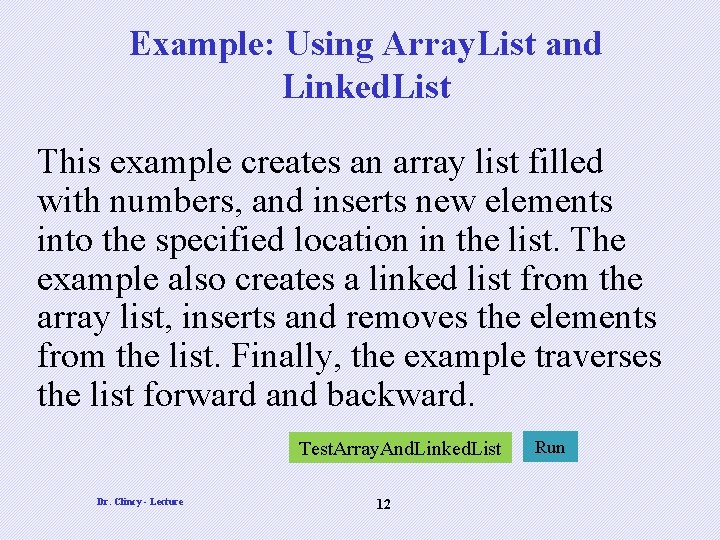
Example: Using Array. List and Linked. List This example creates an array list filled with numbers, and inserts new elements into the specified location in the list. The example also creates a linked list from the array list, inserts and removes the elements from the list. Finally, the example traverses the list forward and backward. Test. Array. And. Linked. List Dr. Clincy - Lecture 12 Run
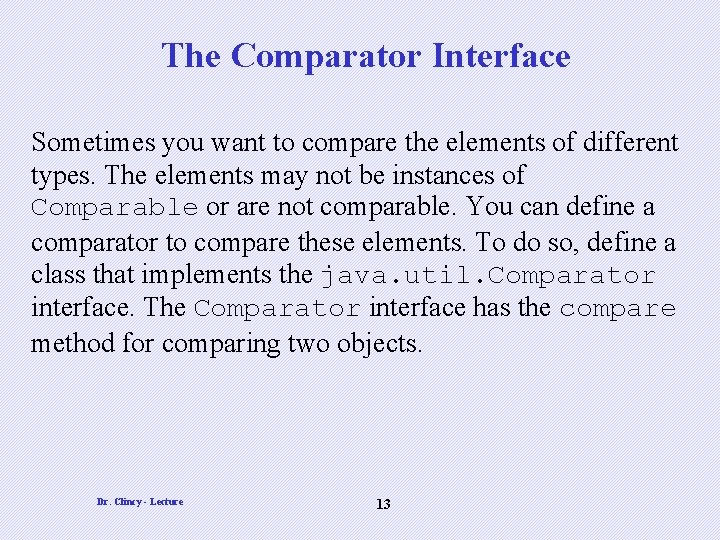
The Comparator Interface Sometimes you want to compare the elements of different types. The elements may not be instances of Comparable or are not comparable. You can define a comparator to compare these elements. To do so, define a class that implements the java. util. Comparator interface. The Comparator interface has the compare method for comparing two objects. Dr. Clincy - Lecture 13
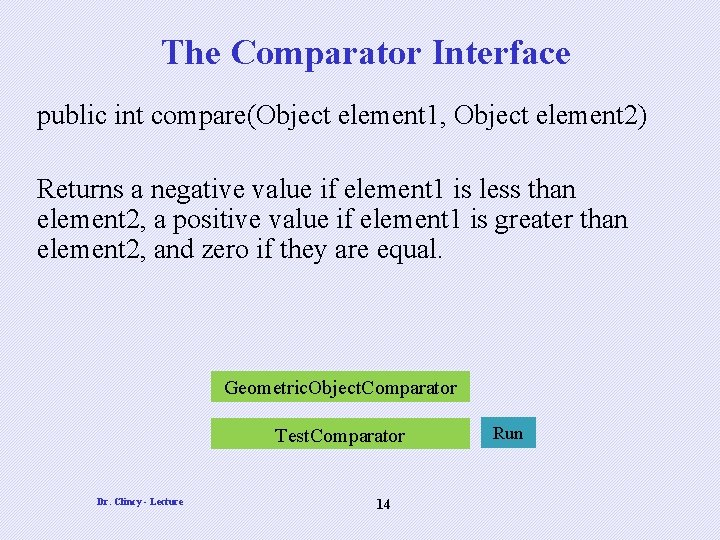
The Comparator Interface public int compare(Object element 1, Object element 2) Returns a negative value if element 1 is less than element 2, a positive value if element 1 is greater than element 2, and zero if they are equal. Geometric. Object. Comparator Test. Comparator Dr. Clincy - Lecture 14 Run
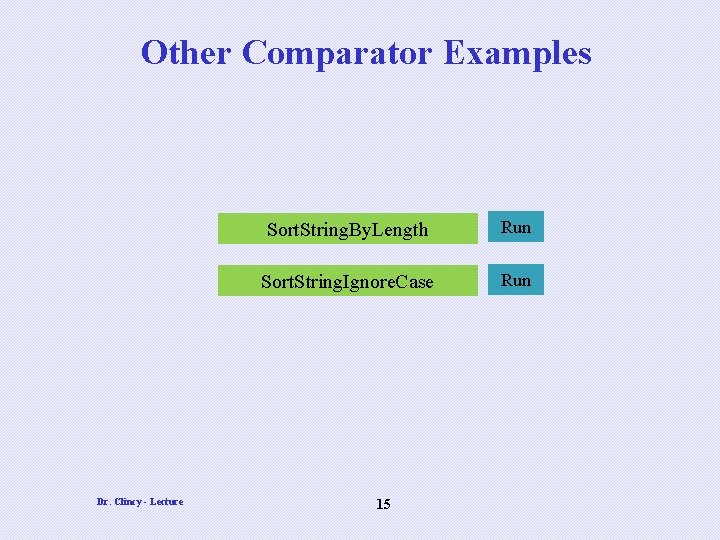
Other Comparator Examples Dr. Clincy - Lecture Sort. String. By. Length Run Sort. String. Ignore. Case Run 15
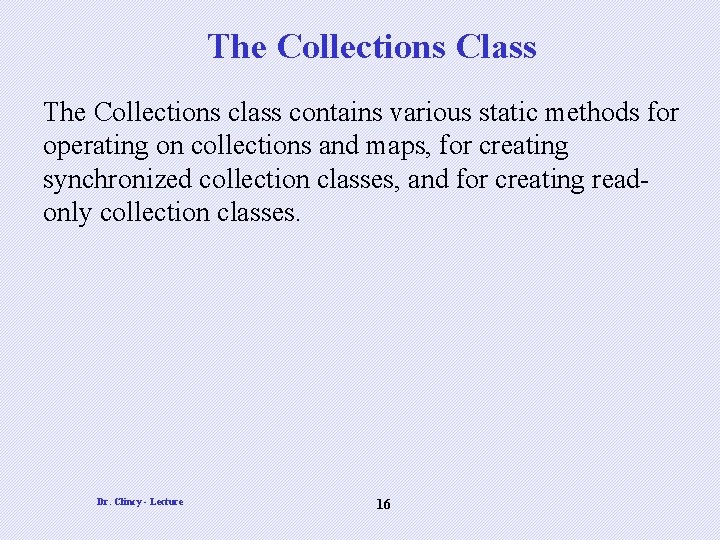
The Collections Class The Collections class contains various static methods for operating on collections and maps, for creating synchronized collection classes, and for creating readonly collection classes. Dr. Clincy - Lecture 16
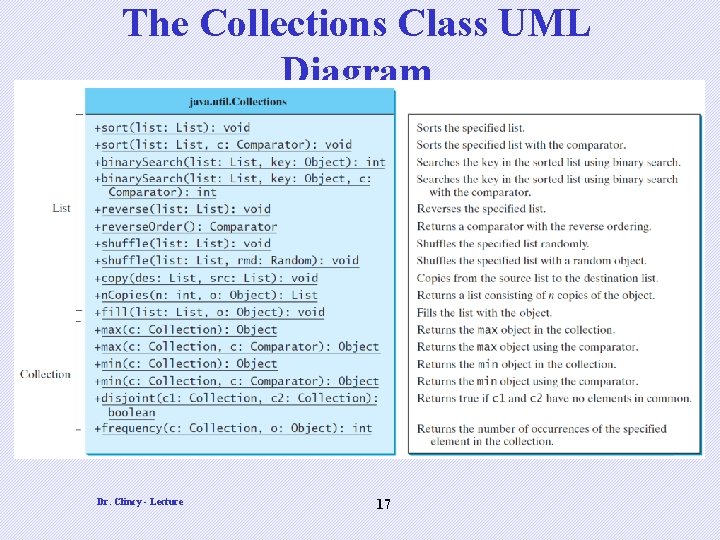
The Collections Class UML Diagram Dr. Clincy - Lecture 17
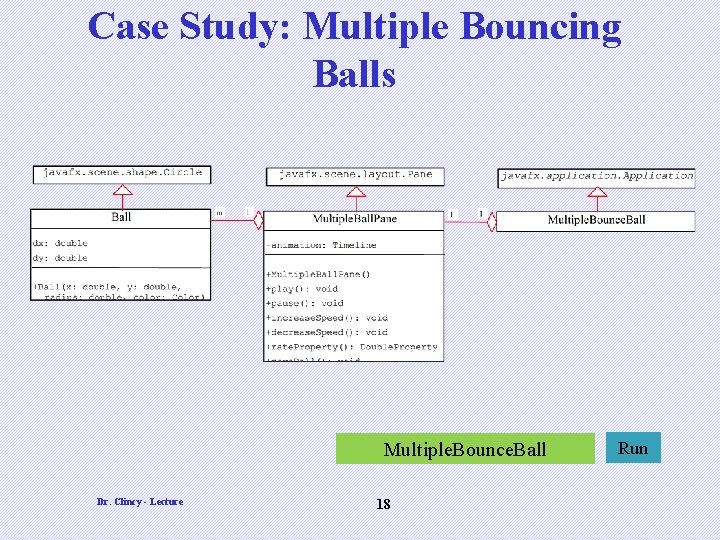
Case Study: Multiple Bouncing Balls Multiple. Bounce. Ball Dr. Clincy - Lecture 18 Run
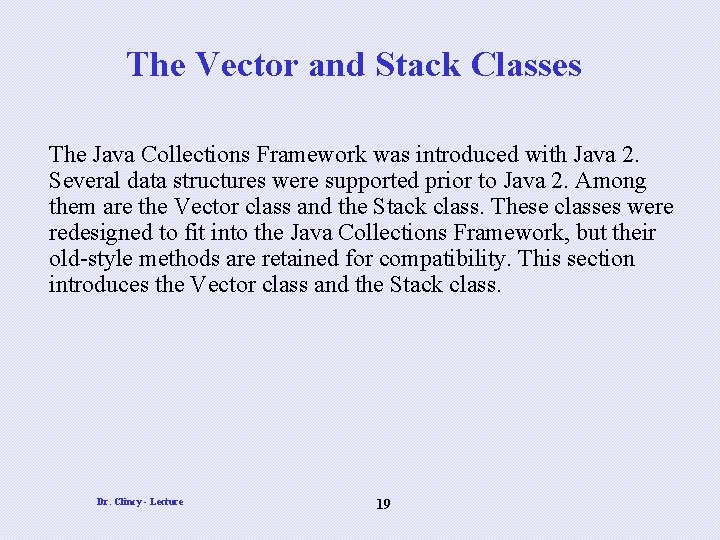
The Vector and Stack Classes The Java Collections Framework was introduced with Java 2. Several data structures were supported prior to Java 2. Among them are the Vector class and the Stack class. These classes were redesigned to fit into the Java Collections Framework, but their old-style methods are retained for compatibility. This section introduces the Vector class and the Stack class. Dr. Clincy - Lecture 19
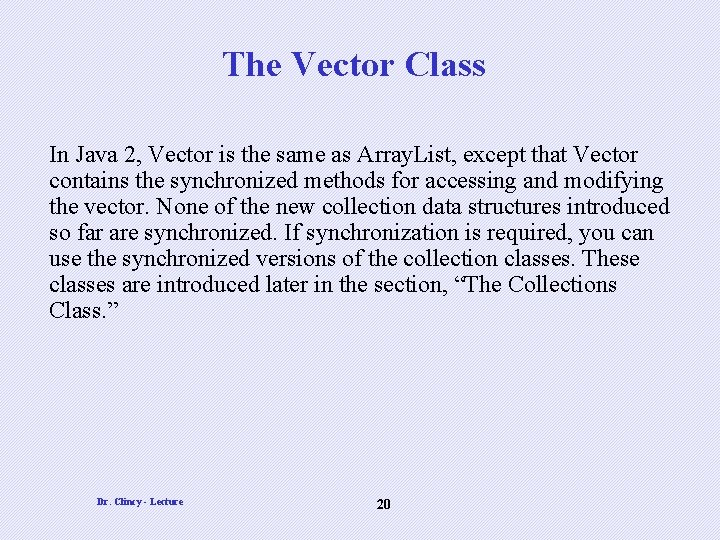
The Vector Class In Java 2, Vector is the same as Array. List, except that Vector contains the synchronized methods for accessing and modifying the vector. None of the new collection data structures introduced so far are synchronized. If synchronization is required, you can use the synchronized versions of the collection classes. These classes are introduced later in the section, “The Collections Class. ” Dr. Clincy - Lecture 20
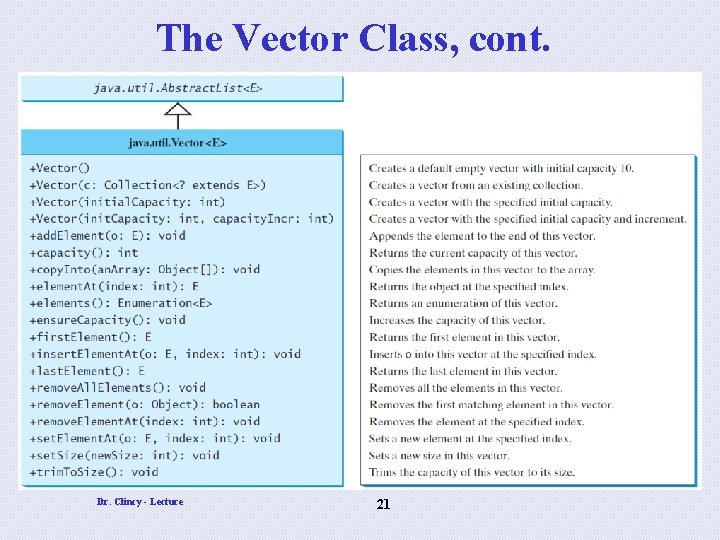
The Vector Class, cont. Dr. Clincy - Lecture 21
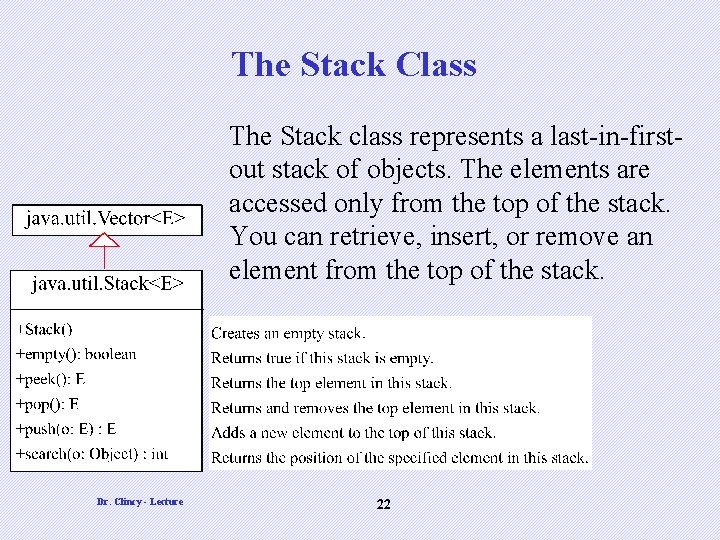
The Stack Class The Stack class represents a last-in-firstout stack of objects. The elements are accessed only from the top of the stack. You can retrieve, insert, or remove an element from the top of the stack. Dr. Clincy - Lecture 22
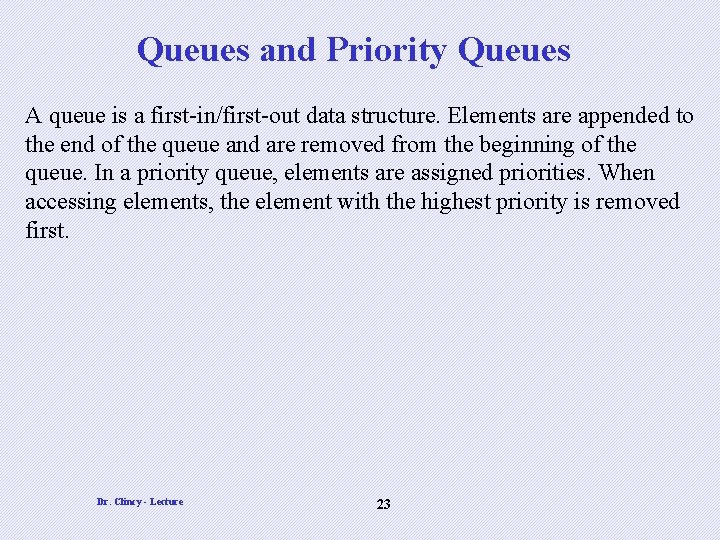
Queues and Priority Queues A queue is a first-in/first-out data structure. Elements are appended to the end of the queue and are removed from the beginning of the queue. In a priority queue, elements are assigned priorities. When accessing elements, the element with the highest priority is removed first. Dr. Clincy - Lecture 23
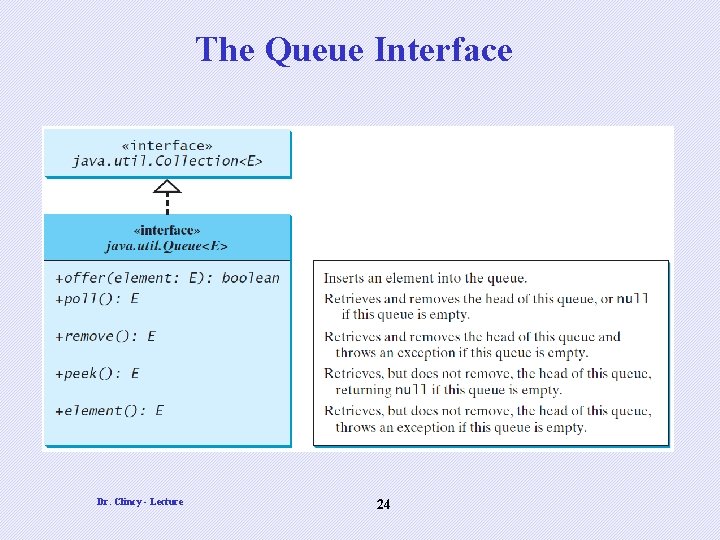
The Queue Interface Dr. Clincy - Lecture 24
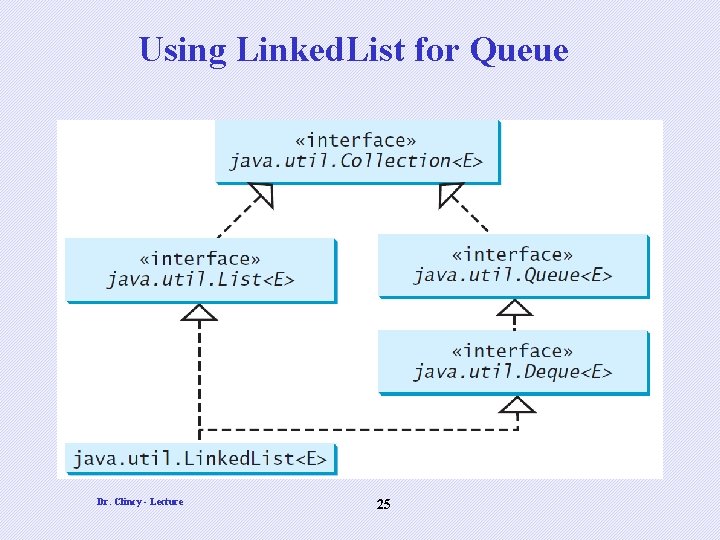
Using Linked. List for Queue Dr. Clincy - Lecture 25
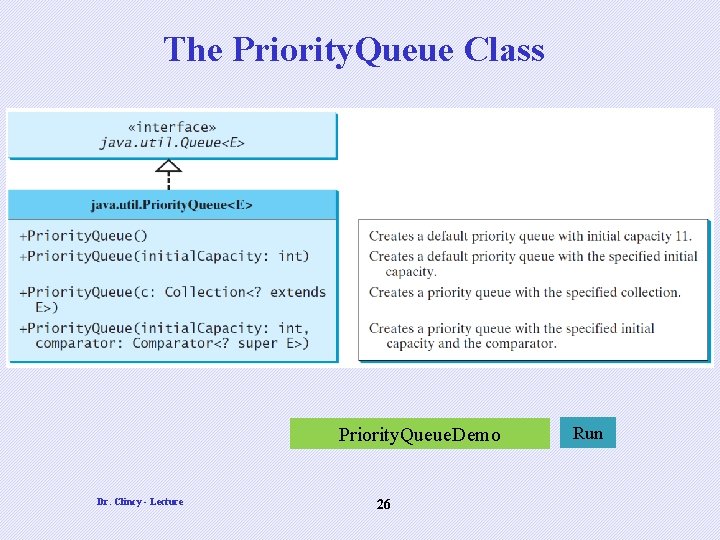
The Priority. Queue Class Priority. Queue. Demo Dr. Clincy - Lecture 26 Run
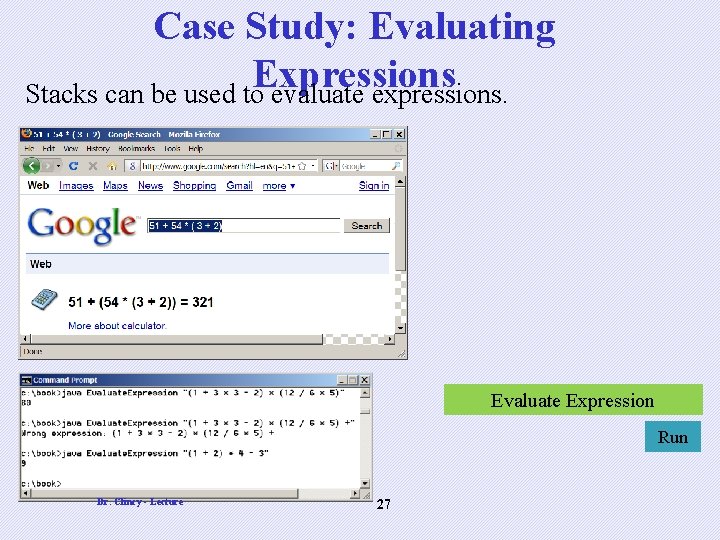
Case Study: Evaluating Expressions Stacks can be used to evaluate expressions. Evaluate Expression Run Dr. Clincy - Lecture 27
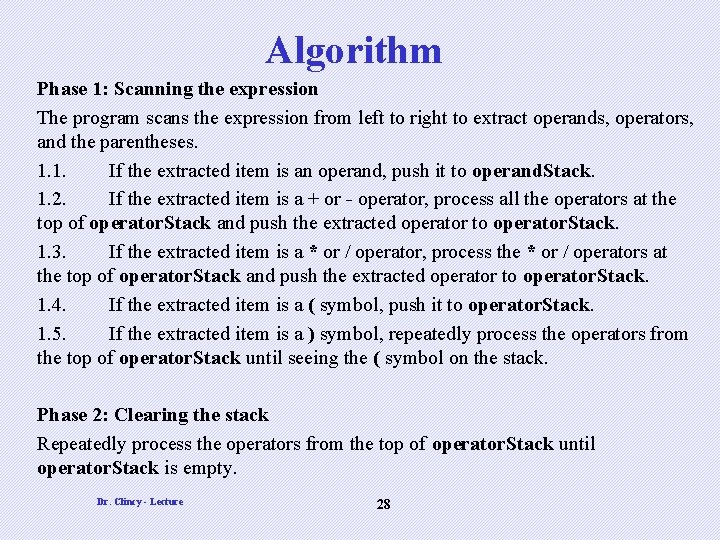
Algorithm Phase 1: Scanning the expression The program scans the expression from left to right to extract operands, operators, and the parentheses. 1. 1. If the extracted item is an operand, push it to operand. Stack. 1. 2. If the extracted item is a + or - operator, process all the operators at the top of operator. Stack and push the extracted operator to operator. Stack. 1. 3. If the extracted item is a * or / operator, process the * or / operators at the top of operator. Stack and push the extracted operator to operator. Stack. 1. 4. If the extracted item is a ( symbol, push it to operator. Stack. 1. 5. If the extracted item is a ) symbol, repeatedly process the operators from the top of operator. Stack until seeing the ( symbol on the stack. Phase 2: Clearing the stack Repeatedly process the operators from the top of operator. Stack until operator. Stack is empty. Dr. Clincy - Lecture 28
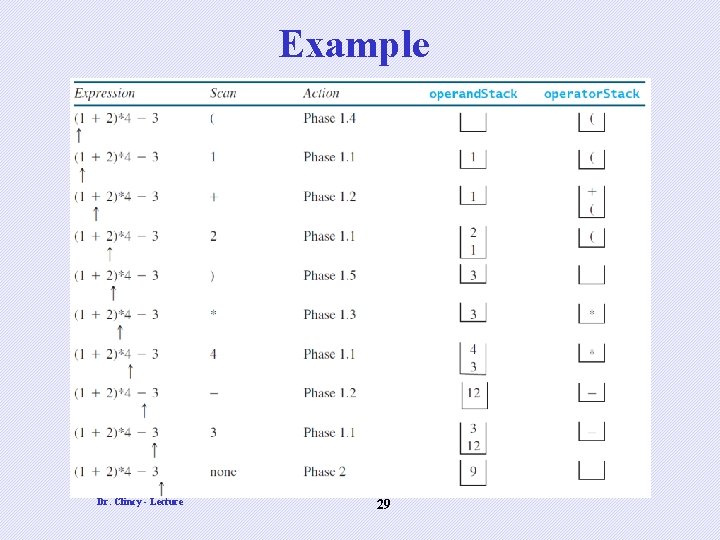
Example Dr. Clincy - Lecture 29