Chapter 2 Array and String Array Definition of
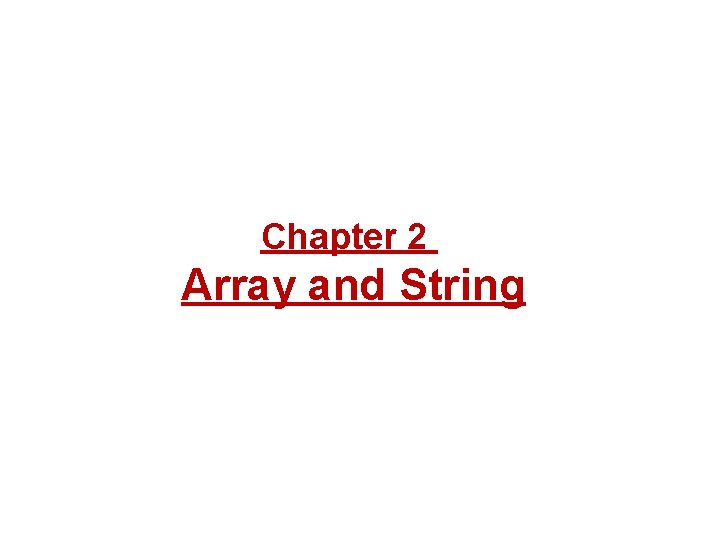
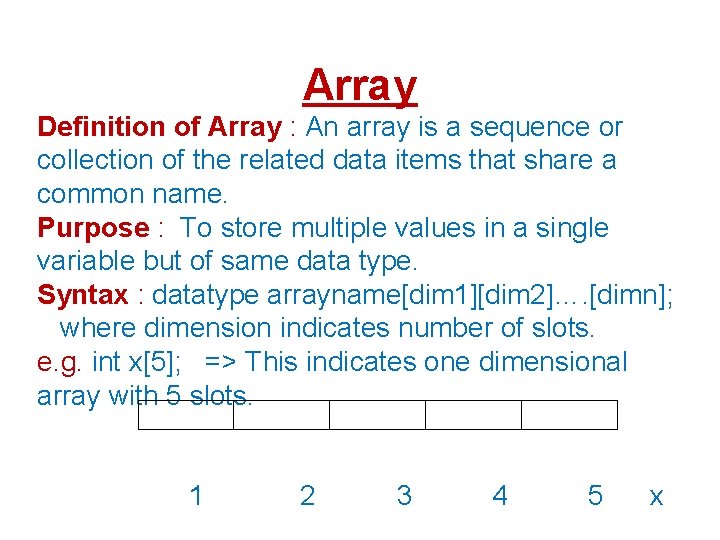
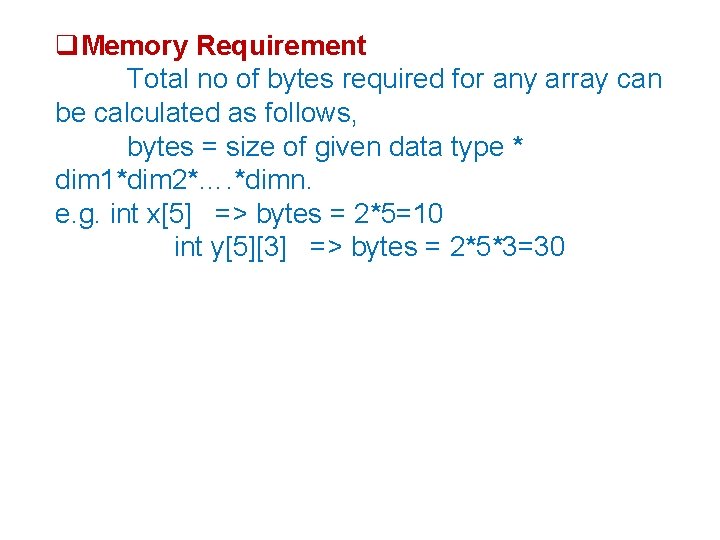
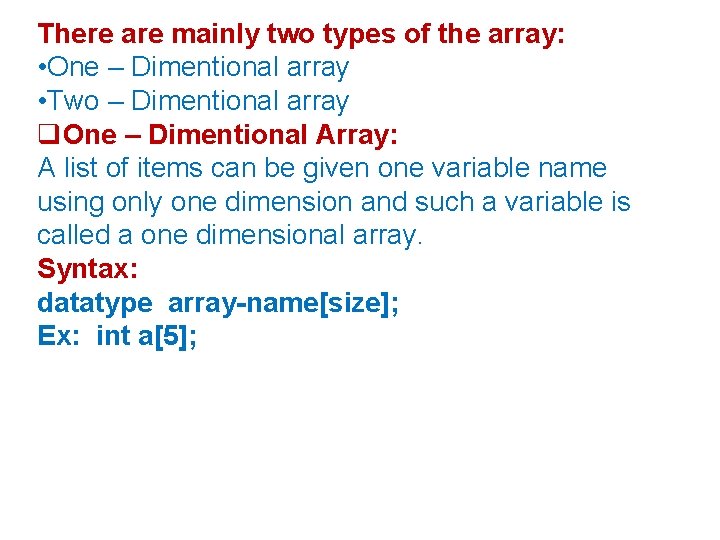
![• To access particular slot syntax: arrayname[logical slotno] e. g. a[0]- content of • To access particular slot syntax: arrayname[logical slotno] e. g. a[0]- content of](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-5.jpg)
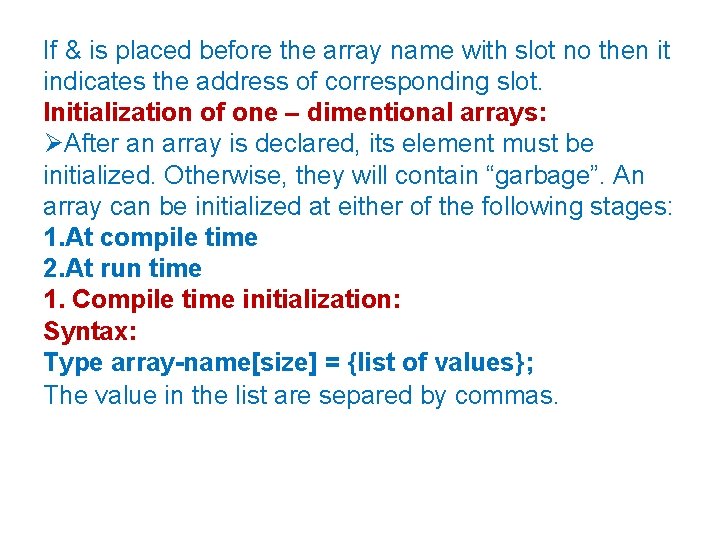
![Ex: int a[3] = {0, 0, 0} ; int a[3] = {10, 20, 30, Ex: int a[3] = {0, 0, 0} ; int a[3] = {10, 20, 30,](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-7.jpg)
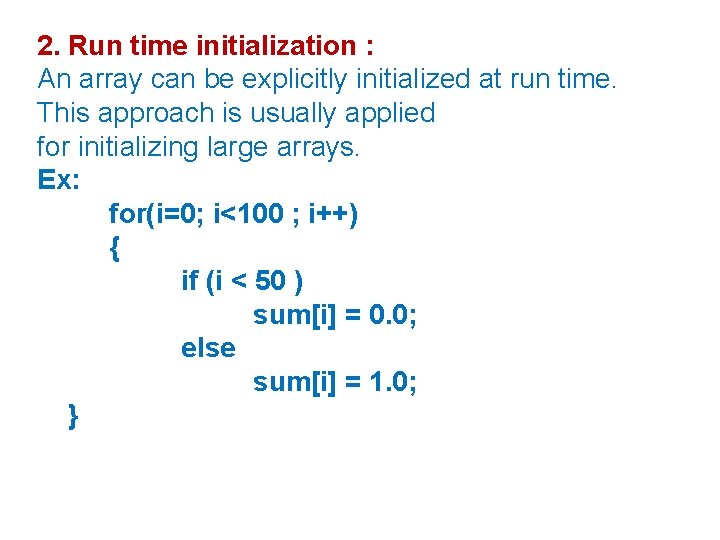
![Ex: int x[3]; scanf (“%d%d%d”, &x[0], &x[1], &x[2] ) ; Will initialize array elements Ex: int x[3]; scanf (“%d%d%d”, &x[0], &x[1], &x[2] ) ; Will initialize array elements](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-9.jpg)
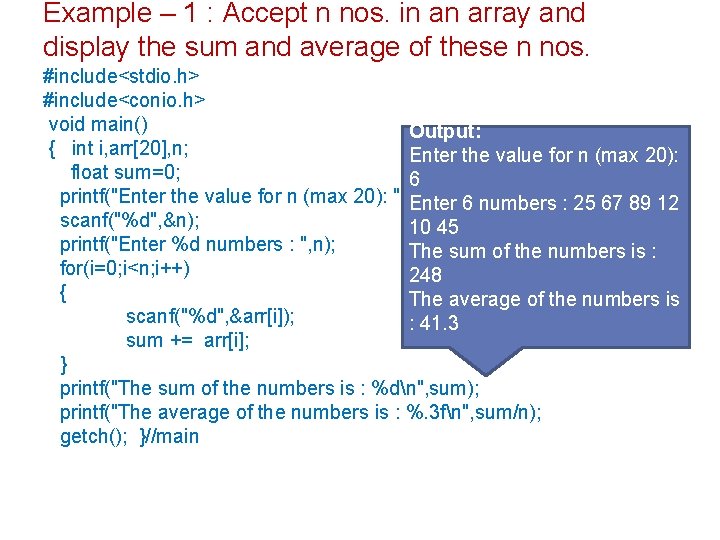
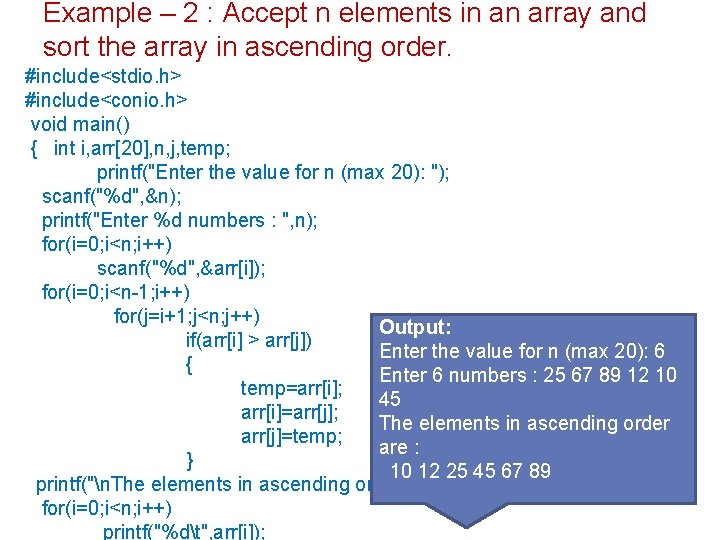
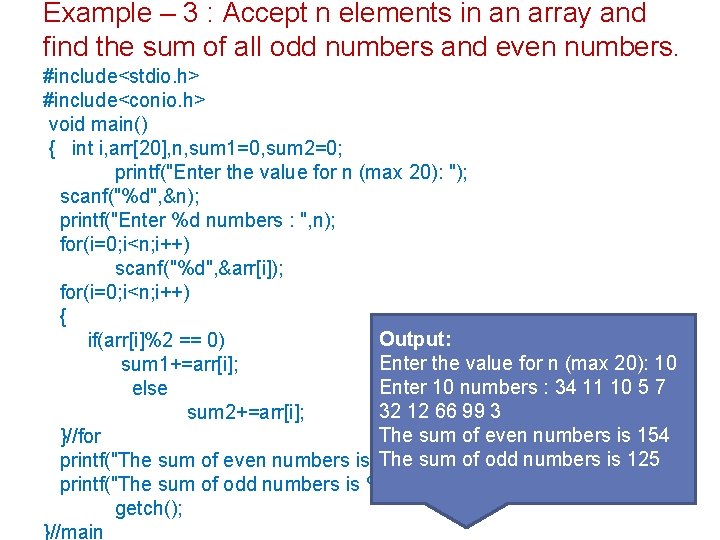
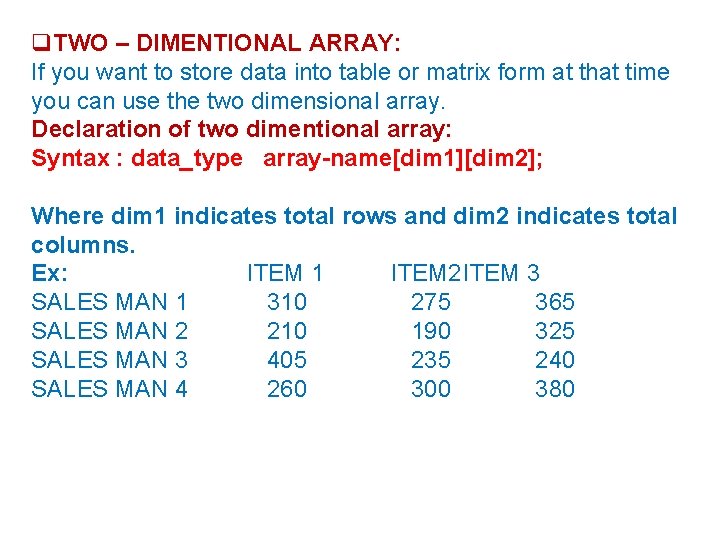
![Memory representation of the two dimentional array for v[4][3]. column 0 column 1 column Memory representation of the two dimentional array for v[4][3]. column 0 column 1 column](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-14.jpg)
![Initializing two dimentional array: Ex: int table[2][3] = {0, 0, 0, 1, 1, 1}; Initializing two dimentional array: Ex: int table[2][3] = {0, 0, 0, 1, 1, 1};](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-15.jpg)
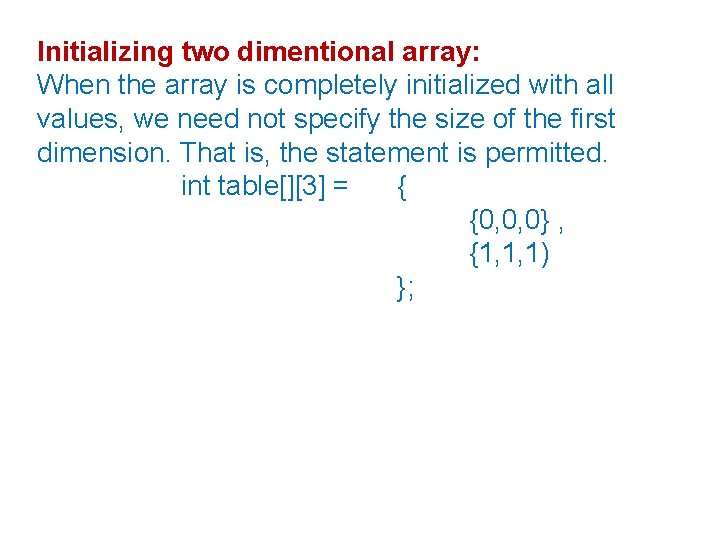
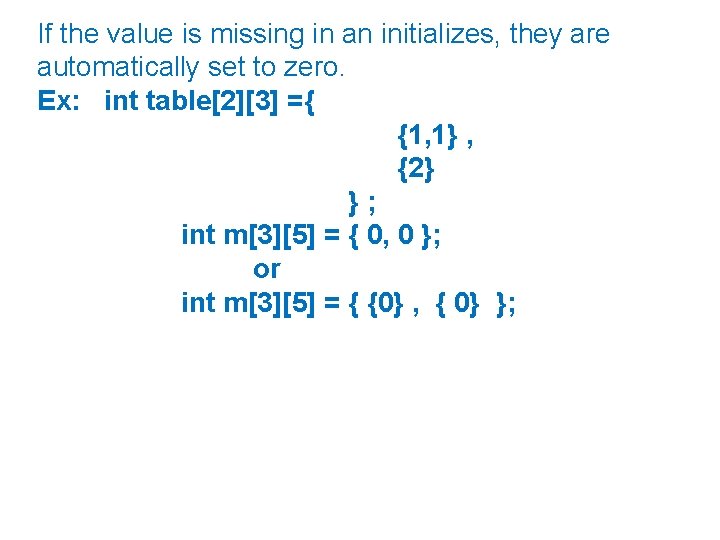
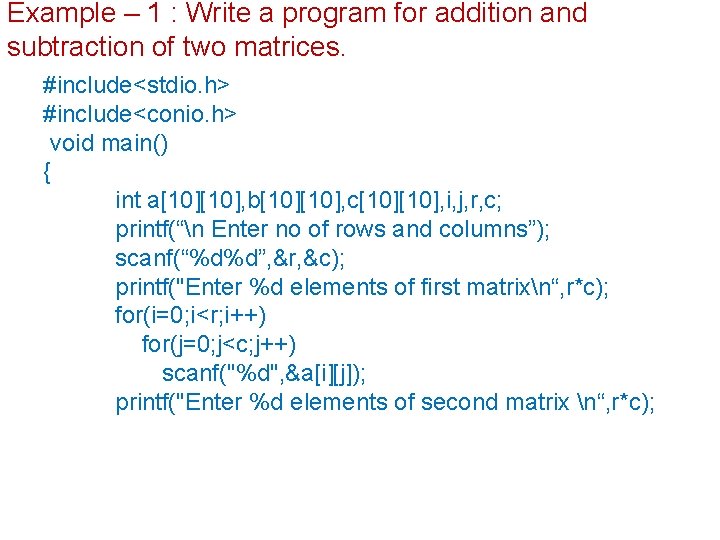
![for(i=0; i<r; i++) for(j=0; j<c; j++) scanf("%d", &b[i][j]); for(i=0; i<r; i++) for(j=0; j<c; j++) for(i=0; i<r; i++) for(j=0; j<c; j++) scanf("%d", &b[i][j]); for(i=0; i<r; i++) for(j=0; j<c; j++)](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-19.jpg)
![for(i=0; i<r; i++) for(j=0; j<c; j++) c[i][j] = a[i][j]-b[i][j]; printf("The subtraction of the two for(i=0; i<r; i++) for(j=0; j<c; j++) c[i][j] = a[i][j]-b[i][j]; printf("The subtraction of the two](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-20.jpg)
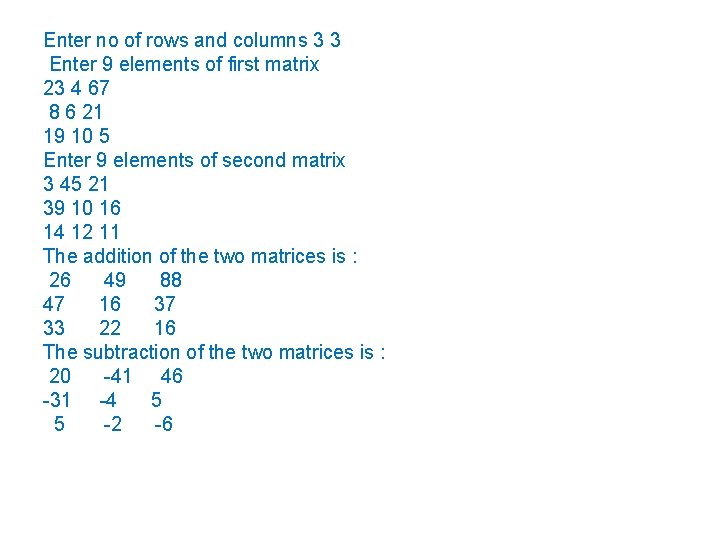
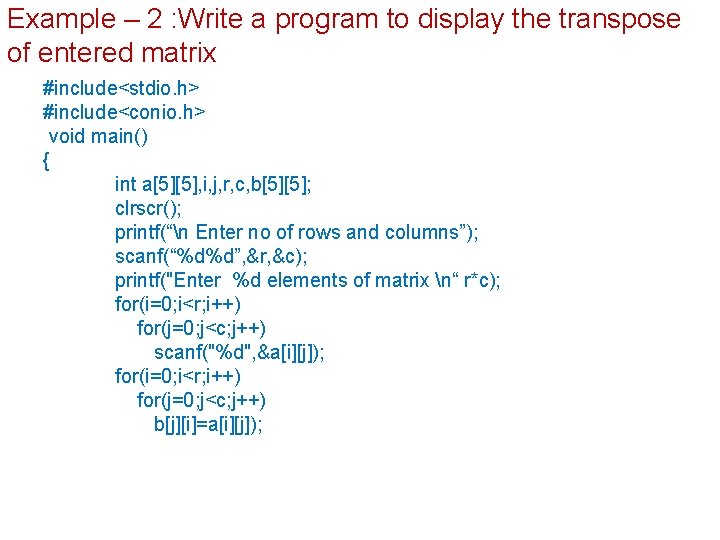
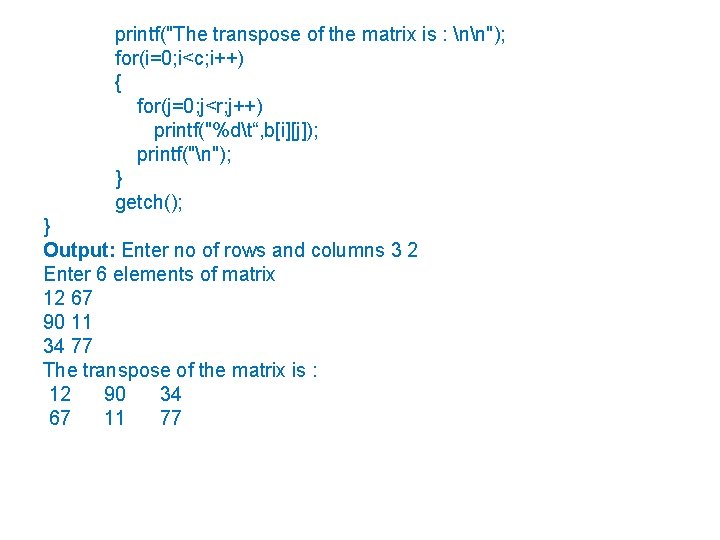
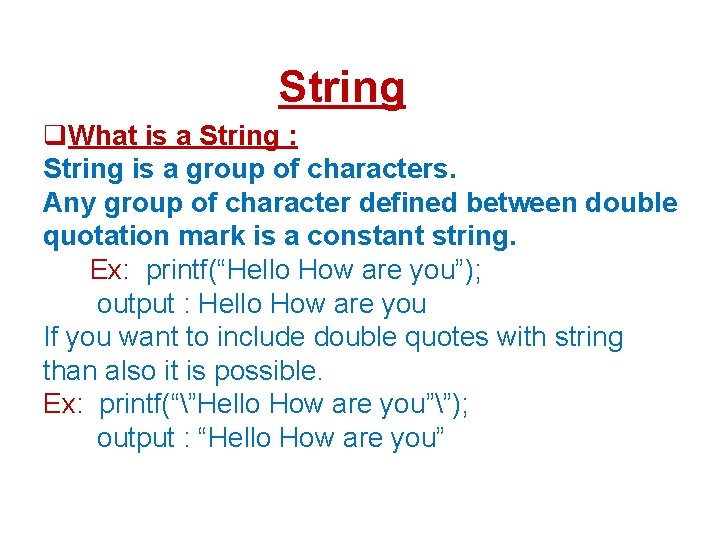
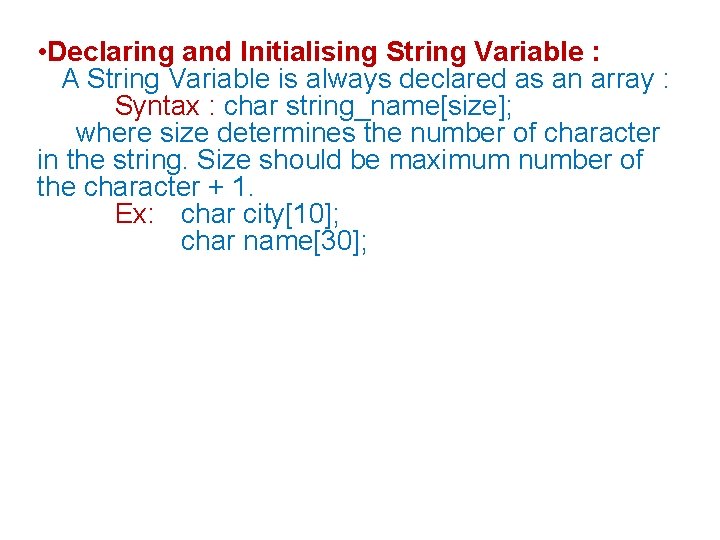
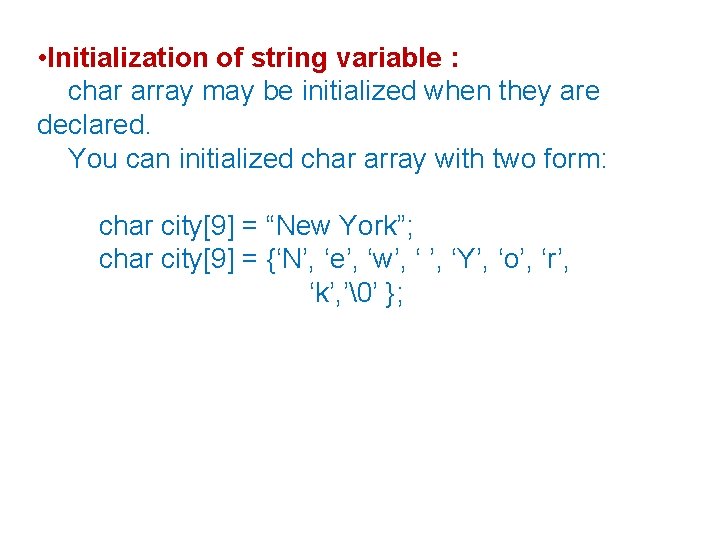
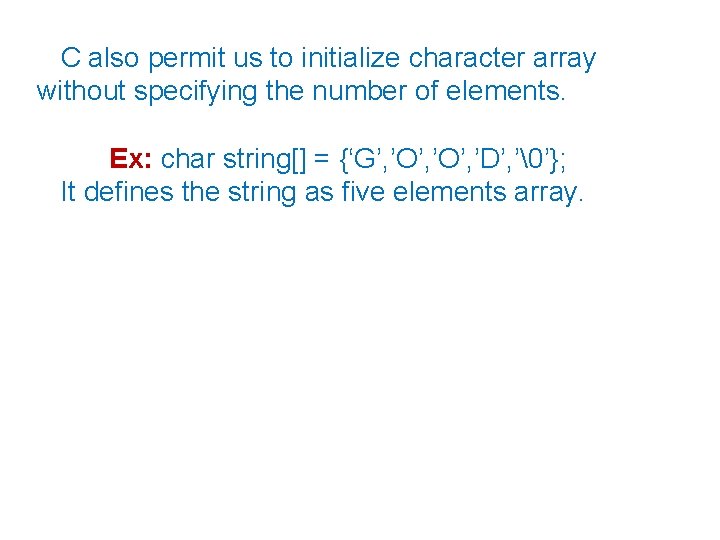
![• Reading String : Ex: char address[15]; scanf(“%s”, address); (& sign is not • Reading String : Ex: char address[15]; scanf(“%s”, address); (& sign is not](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-28.jpg)
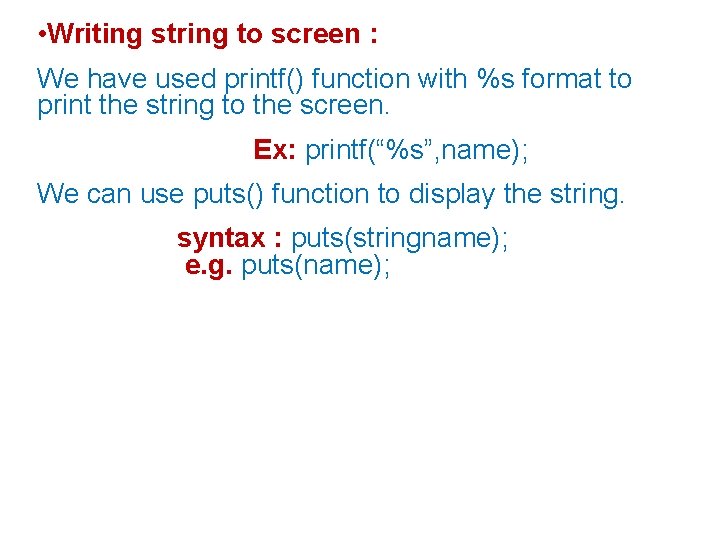
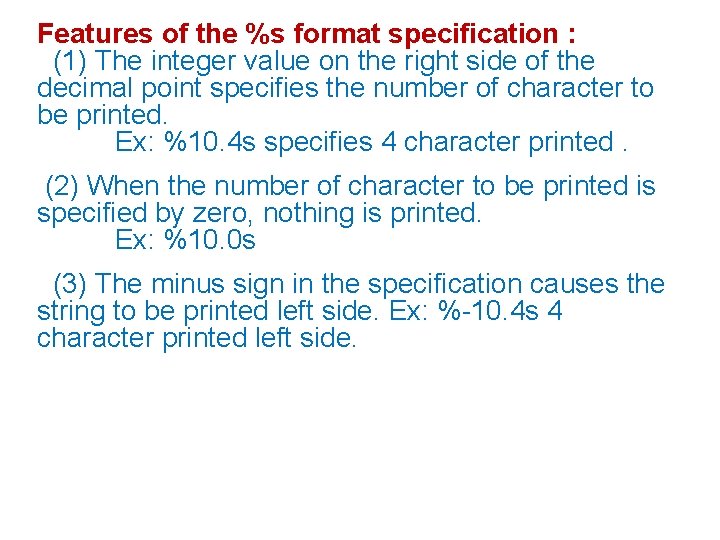
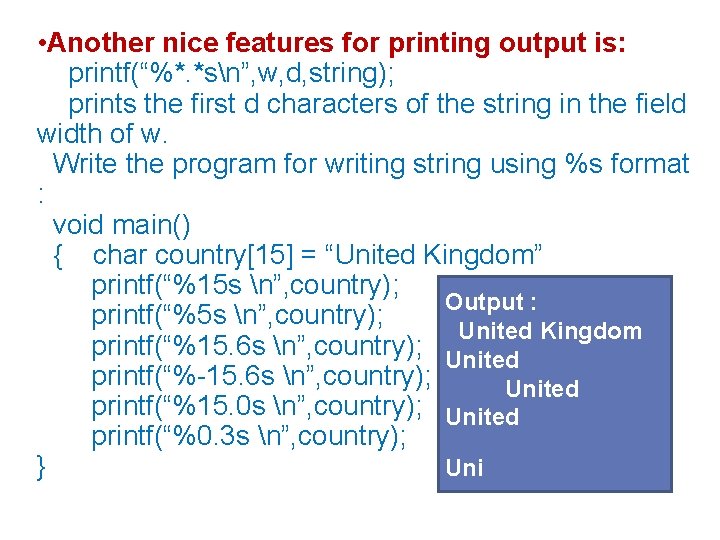
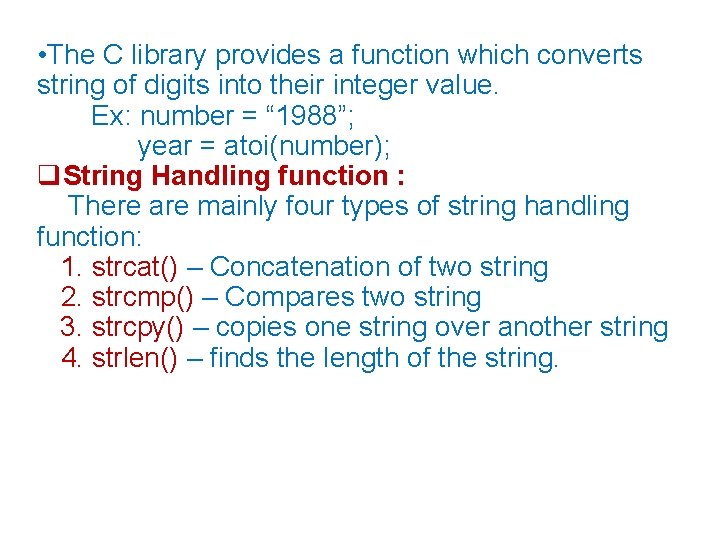
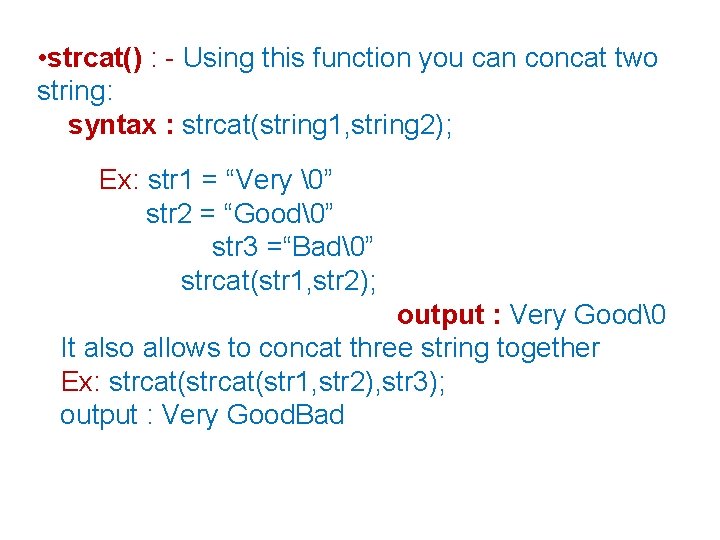
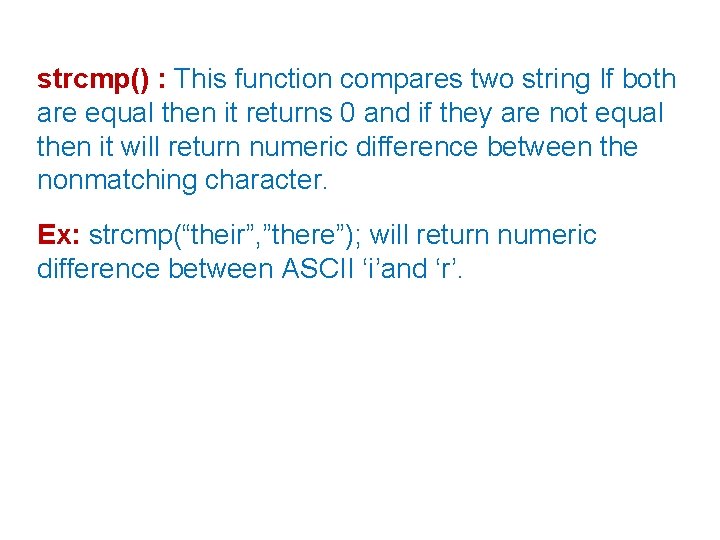
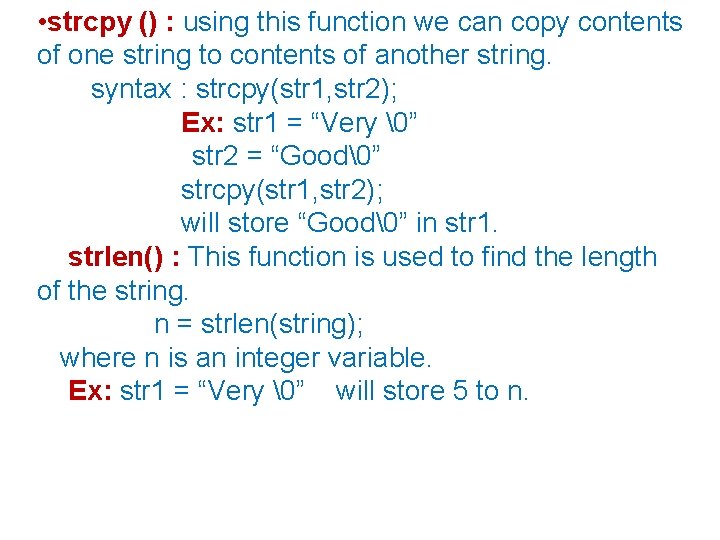
- Slides: 35
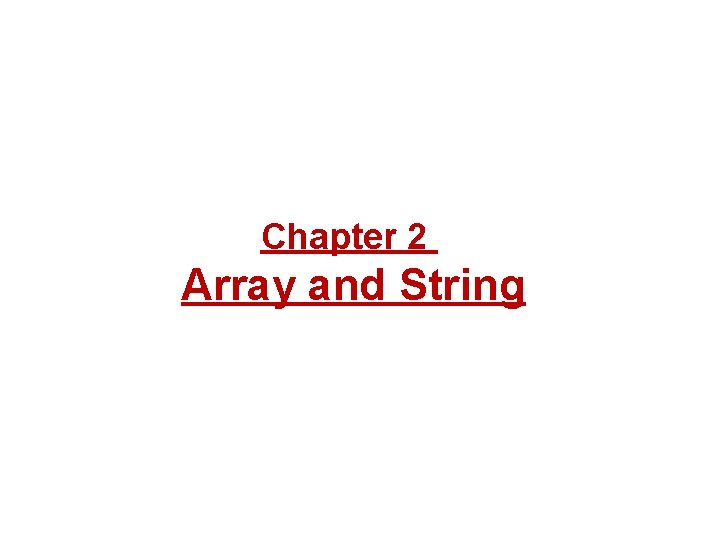
Chapter 2 Array and String
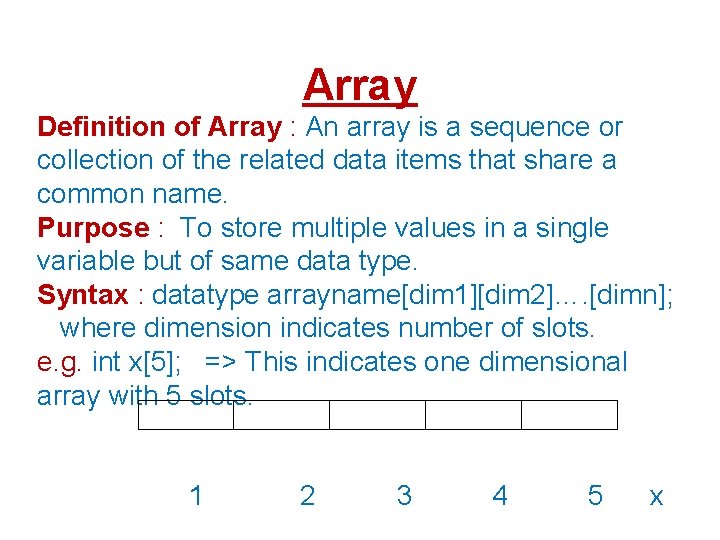
Array Definition of Array : An array is a sequence or collection of the related data items that share a common name. Purpose : To store multiple values in a single variable but of same data type. Syntax : datatype arrayname[dim 1][dim 2]…. [dimn]; where dimension indicates number of slots. e. g. int x[5]; => This indicates one dimensional array with 5 slots. 1 2 3 4 5 x
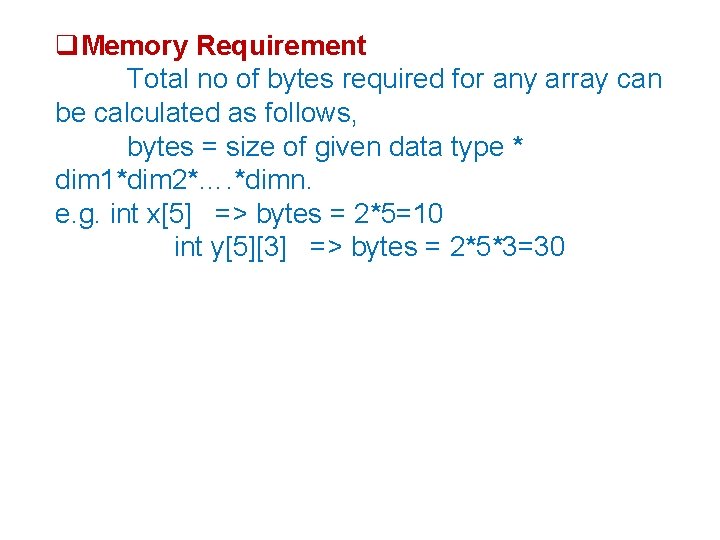
Memory Requirement Total no of bytes required for any array can be calculated as follows, bytes = size of given data type * dim 1*dim 2*…. *dimn. e. g. int x[5] => bytes = 2*5=10 int y[5][3] => bytes = 2*5*3=30
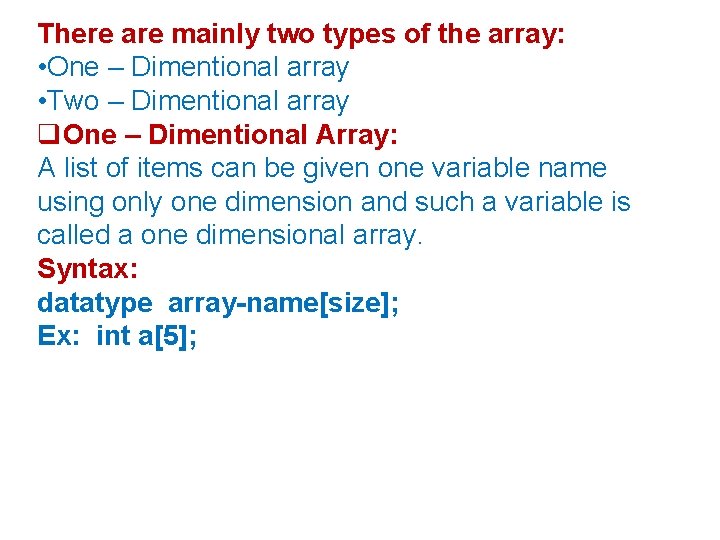
There are mainly two types of the array: • One – Dimentional array • Two – Dimentional array One – Dimentional Array: A list of items can be given one variable name using only one dimension and such a variable is called a one dimensional array. Syntax: datatype array-name[size]; Ex: int a[5];
![To access particular slot syntax arraynamelogical slotno e g a0 content of • To access particular slot syntax: arrayname[logical slotno] e. g. a[0]- content of](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-5.jpg)
• To access particular slot syntax: arrayname[logical slotno] e. g. a[0]- content of 0 th slot(logical)
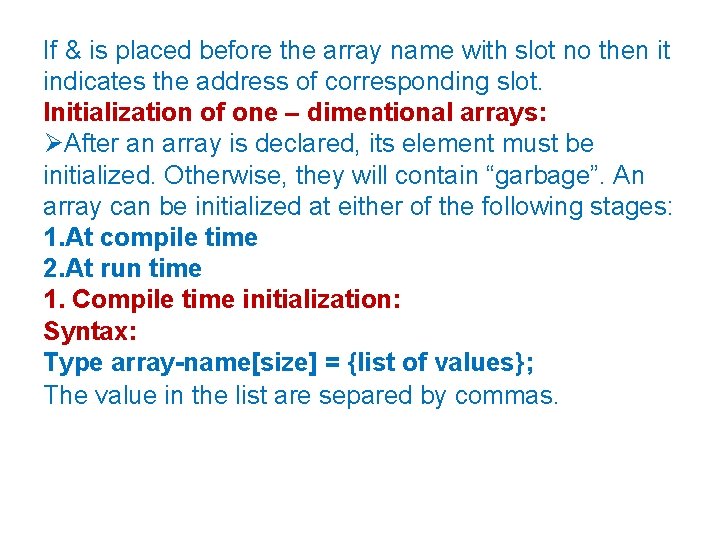
If & is placed before the array name with slot no then it indicates the address of corresponding slot. Initialization of one – dimentional arrays: After an array is declared, its element must be initialized. Otherwise, they will contain “garbage”. An array can be initialized at either of the following stages: 1. At compile time 2. At run time 1. Compile time initialization: Syntax: Type array-name[size] = {list of values}; The value in the list are separed by commas.
![Ex int a3 0 0 0 int a3 10 20 30 Ex: int a[3] = {0, 0, 0} ; int a[3] = {10, 20, 30,](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-7.jpg)
Ex: int a[3] = {0, 0, 0} ; int a[3] = {10, 20, 30, 40} ; will not work. It is illegal in C. Ex: int counter[] = {1, 1, 1, 1} counter array contain four element with initial value 1.
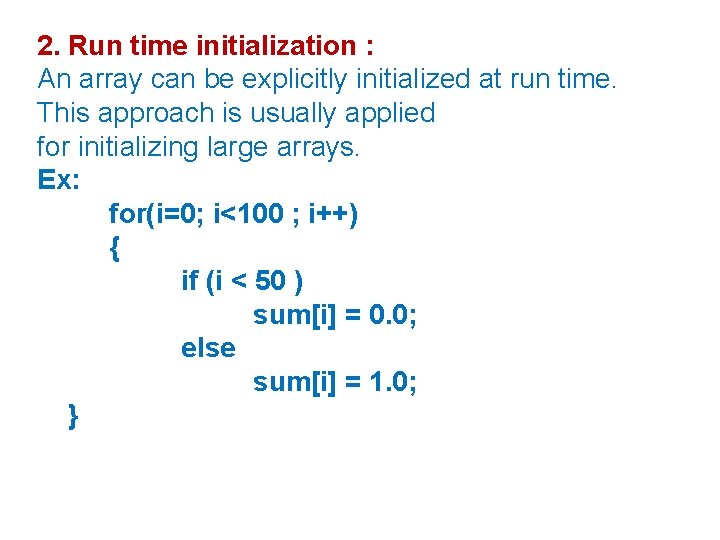
2. Run time initialization : An array can be explicitly initialized at run time. This approach is usually applied for initializing large arrays. Ex: for(i=0; i<100 ; i++) { if (i < 50 ) sum[i] = 0. 0; else sum[i] = 1. 0; }
![Ex int x3 scanf ddd x0 x1 x2 Will initialize array elements Ex: int x[3]; scanf (“%d%d%d”, &x[0], &x[1], &x[2] ) ; Will initialize array elements](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-9.jpg)
Ex: int x[3]; scanf (“%d%d%d”, &x[0], &x[1], &x[2] ) ; Will initialize array elements with the values entered through the keyboard.
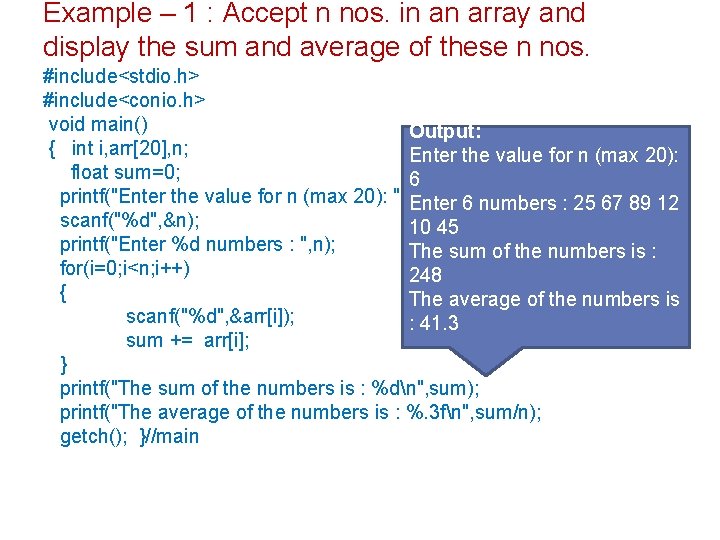
Example – 1 : Accept n nos. in an array and display the sum and average of these n nos. #include<stdio. h> #include<conio. h> void main() Output: { int i, arr[20], n; Enter the value for n (max 20): float sum=0; 6 printf("Enter the value for n (max 20): "); Enter 6 numbers : 25 67 89 12 scanf("%d", &n); 10 45 printf("Enter %d numbers : ", n); The sum of the numbers is : for(i=0; i<n; i++) 248 { The average of the numbers is scanf("%d", &arr[i]); : 41. 3 sum += arr[i]; } printf("The sum of the numbers is : %dn", sum); printf("The average of the numbers is : %. 3 fn", sum/n); getch(); }//main
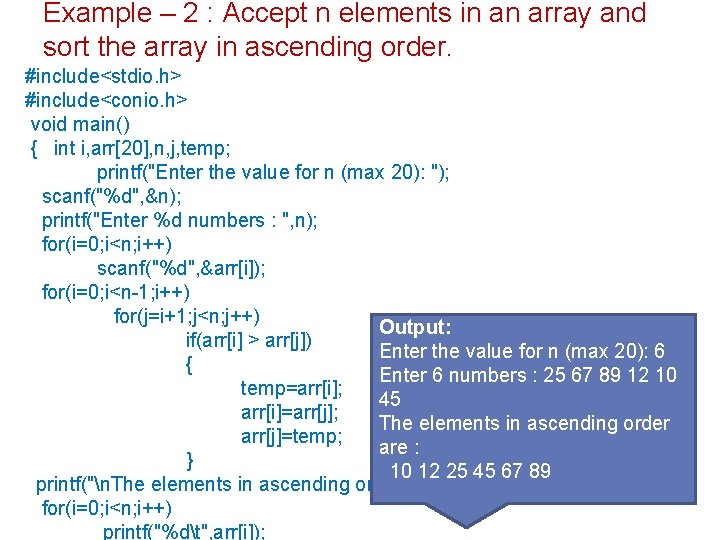
Example – 2 : Accept n elements in an array and sort the array in ascending order. #include<stdio. h> #include<conio. h> void main() { int i, arr[20], n, j, temp; printf("Enter the value for n (max 20): "); scanf("%d", &n); printf("Enter %d numbers : ", n); for(i=0; i<n; i++) scanf("%d", &arr[i]); for(i=0; i<n-1; i++) for(j=i+1; j<n; j++) Output: if(arr[i] > arr[j]) Enter the value for n (max 20): 6 { Enter 6 numbers : 25 67 89 12 10 temp=arr[i]; 45 arr[i]=arr[j]; The elements in ascending order arr[j]=temp; are : } 10 12 25 45 67 89 printf("n. The elements in ascending order are : n"); for(i=0; i<n; i++) printf("%dt", arr[i]);
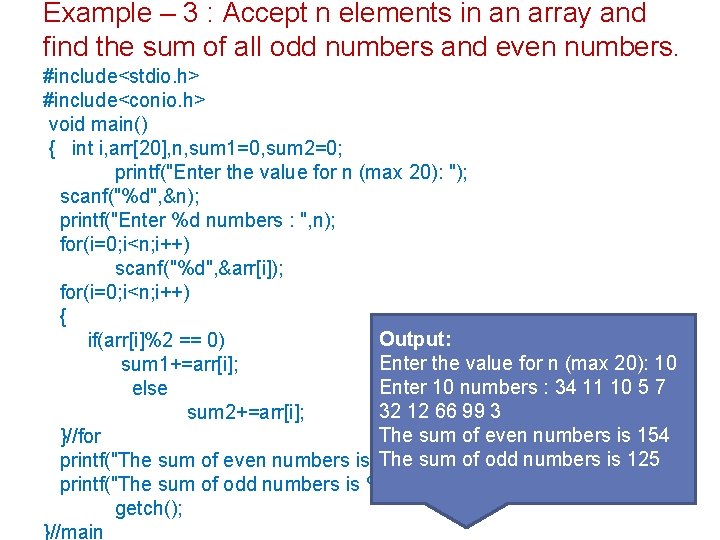
Example – 3 : Accept n elements in an array and find the sum of all odd numbers and even numbers. #include<stdio. h> #include<conio. h> void main() { int i, arr[20], n, sum 1=0, sum 2=0; printf("Enter the value for n (max 20): "); scanf("%d", &n); printf("Enter %d numbers : ", n); for(i=0; i<n; i++) scanf("%d", &arr[i]); for(i=0; i<n; i++) { Output: if(arr[i]%2 == 0) Enter the value for n (max 20): 10 sum 1+=arr[i]; Enter 10 numbers : 34 11 10 5 7 else 32 12 66 99 3 sum 2+=arr[i]; The sum of even numbers is 154 }//for The sum of odd numbers is 125 printf("The sum of even numbers is %dn", sum 1); printf("The sum of odd numbers is %dn", sum 2); getch(); }//main
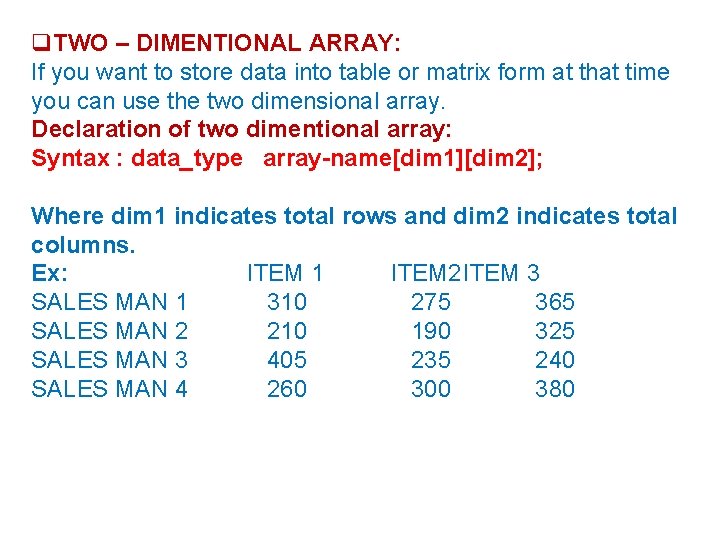
TWO – DIMENTIONAL ARRAY: If you want to store data into table or matrix form at that time you can use the two dimensional array. Declaration of two dimentional array: Syntax : data_type array-name[dim 1][dim 2]; Where dim 1 indicates total rows and dim 2 indicates total columns. Ex: ITEM 1 ITEM 2 ITEM 3 SALES MAN 1 310 275 365 SALES MAN 2 210 190 325 SALES MAN 3 405 235 240 SALES MAN 4 260 300 380
![Memory representation of the two dimentional array for v43 column 0 column 1 column Memory representation of the two dimentional array for v[4][3]. column 0 column 1 column](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-14.jpg)
Memory representation of the two dimentional array for v[4][3]. column 0 column 1 column 2 310 [0][0] 275 [0][1] 365 [0][2] Row 0 Row 1 [1][0] 10 405 [2][0] 190 235 [2][1] [1][1] 325 [1][2] 240 [2][2] Row 2 310 [3][0] Row 3 275 [3][1] 365 [3][2]
![Initializing two dimentional array Ex int table23 0 0 0 1 1 1 Initializing two dimentional array: Ex: int table[2][3] = {0, 0, 0, 1, 1, 1};](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-15.jpg)
Initializing two dimentional array: Ex: int table[2][3] = {0, 0, 0, 1, 1, 1}; int table[2][3] = { {0, 0, 0}, {1, 1, 1} } ;
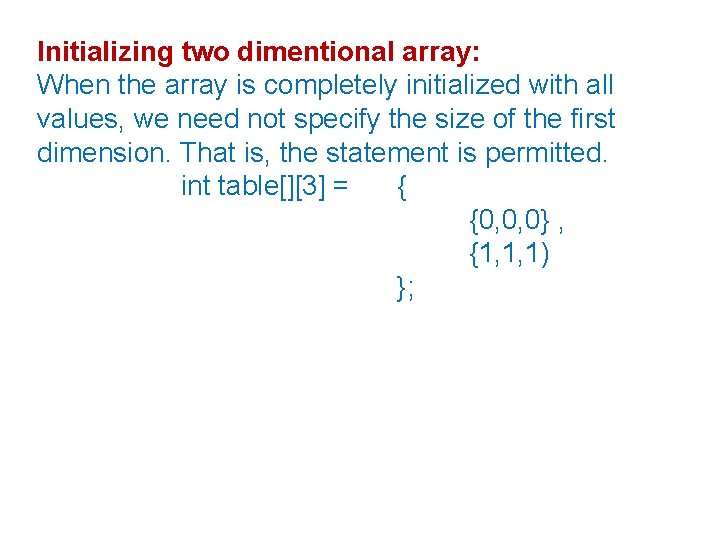
Initializing two dimentional array: When the array is completely initialized with all values, we need not specify the size of the first dimension. That is, the statement is permitted. int table[][3] = { {0, 0, 0} , {1, 1, 1) };
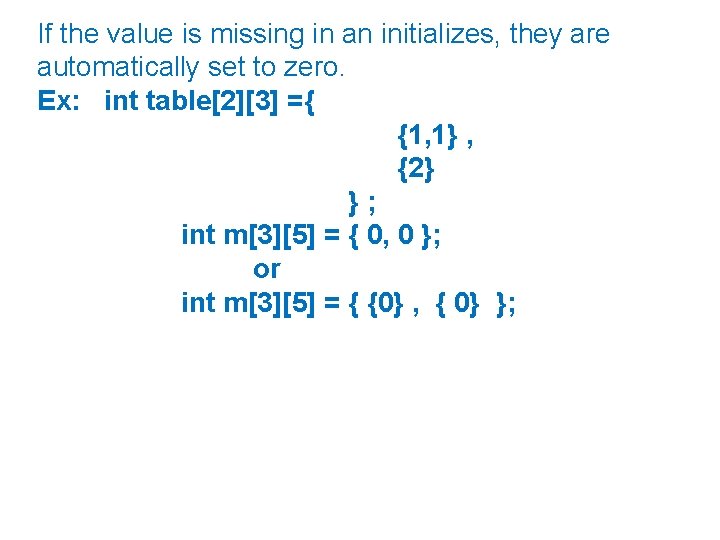
If the value is missing in an initializes, they are automatically set to zero. Ex: int table[2][3] ={ {1, 1} , {2} }; int m[3][5] = { 0, 0 }; or int m[3][5] = { {0} , { 0} };
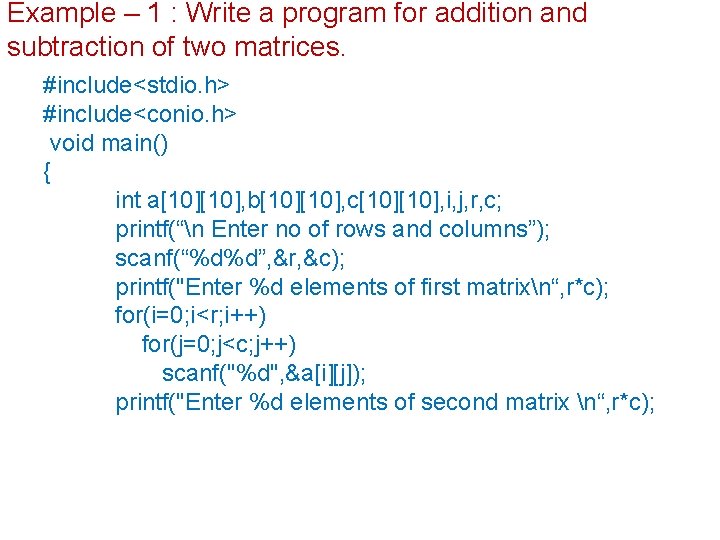
Example – 1 : Write a program for addition and subtraction of two matrices. #include<stdio. h> #include<conio. h> void main() { int a[10], b[10], c[10], i, j, r, c; printf(“n Enter no of rows and columns”); scanf(“%d%d”, &r, &c); printf("Enter %d elements of first matrixn“, r*c); for(i=0; i<r; i++) for(j=0; j<c; j++) scanf("%d", &a[i][j]); printf("Enter %d elements of second matrix n“, r*c);
![fori0 ir i forj0 jc j scanfd bij fori0 ir i forj0 jc j for(i=0; i<r; i++) for(j=0; j<c; j++) scanf("%d", &b[i][j]); for(i=0; i<r; i++) for(j=0; j<c; j++)](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-19.jpg)
for(i=0; i<r; i++) for(j=0; j<c; j++) scanf("%d", &b[i][j]); for(i=0; i<r; i++) for(j=0; j<c; j++) c[i][j] = a[i][j]+b[i][j]; printf("The addition of the two matrices is : nn"); for(i=0; i<r; i++) { for(j=0; j<c; j++) printf("%dt", c[i][j]); printf("n"); }
![fori0 ir i forj0 jc j cij aijbij printfThe subtraction of the two for(i=0; i<r; i++) for(j=0; j<c; j++) c[i][j] = a[i][j]-b[i][j]; printf("The subtraction of the two](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-20.jpg)
for(i=0; i<r; i++) for(j=0; j<c; j++) c[i][j] = a[i][j]-b[i][j]; printf("The subtraction of the two matrices is : nn"); for(i=0; i<r; i++) { for(j=0; j<c; j++) printf("%dt", c[i][j]); printf("n"); } getch(); }
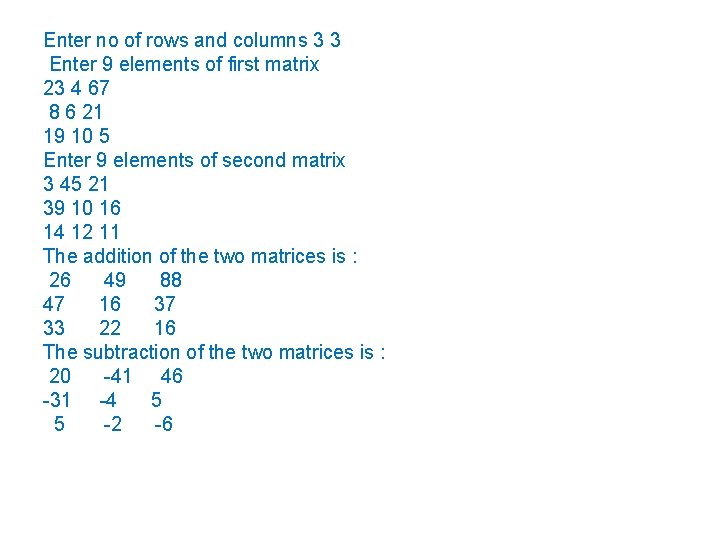
Enter no of rows and columns 3 3 Enter 9 elements of first matrix 23 4 67 8 6 21 19 10 5 Enter 9 elements of second matrix 3 45 21 39 10 16 14 12 11 The addition of the two matrices is : 26 49 88 47 16 37 33 22 16 The subtraction of the two matrices is : 20 -41 46 -31 -4 5 5 -2 -6
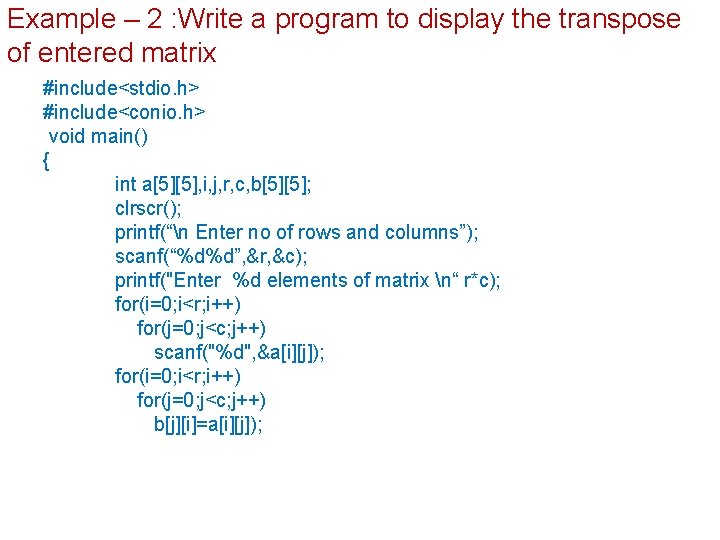
Example – 2 : Write a program to display the transpose of entered matrix #include<stdio. h> #include<conio. h> void main() { int a[5][5], i, j, r, c, b[5][5]; clrscr(); printf(“n Enter no of rows and columns”); scanf(“%d%d”, &r, &c); printf("Enter %d elements of matrix n“ r*c); for(i=0; i<r; i++) for(j=0; j<c; j++) scanf("%d", &a[i][j]); for(i=0; i<r; i++) for(j=0; j<c; j++) b[j][i]=a[i][j]);
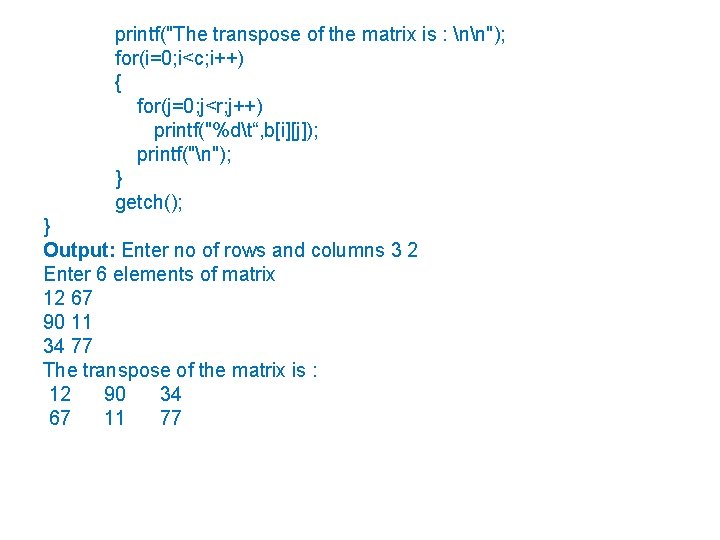
printf("The transpose of the matrix is : nn"); for(i=0; i<c; i++) { for(j=0; j<r; j++) printf("%dt“, b[i][j]); printf("n"); } getch(); } Output: Enter no of rows and columns 3 2 Enter 6 elements of matrix 12 67 90 11 34 77 The transpose of the matrix is : 12 90 34 67 11 77
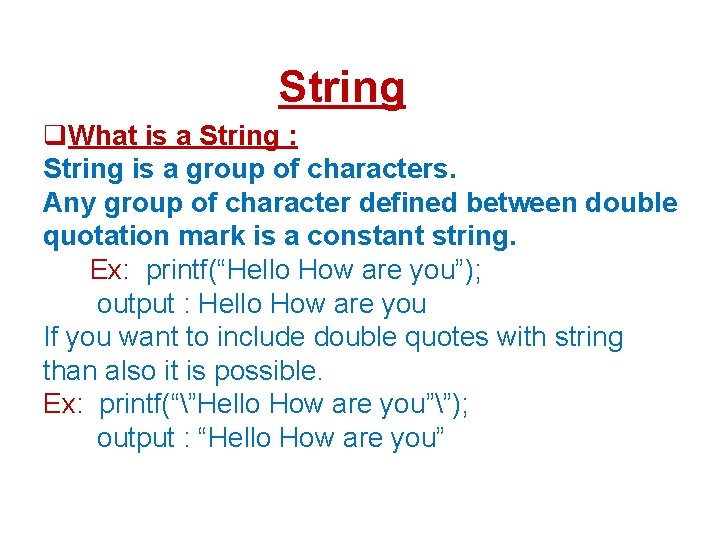
String What is a String : String is a group of characters. Any group of character defined between double quotation mark is a constant string. Ex: printf(“Hello How are you”); output : Hello How are you If you want to include double quotes with string than also it is possible. Ex: printf(“”Hello How are you””); output : “Hello How are you”
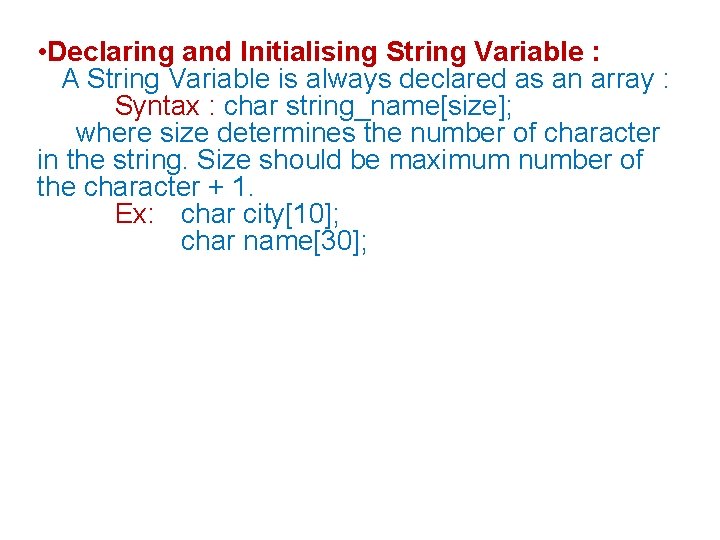
• Declaring and Initialising String Variable : A String Variable is always declared as an array : Syntax : char string_name[size]; where size determines the number of character in the string. Size should be maximum number of the character + 1. Ex: char city[10]; char name[30];
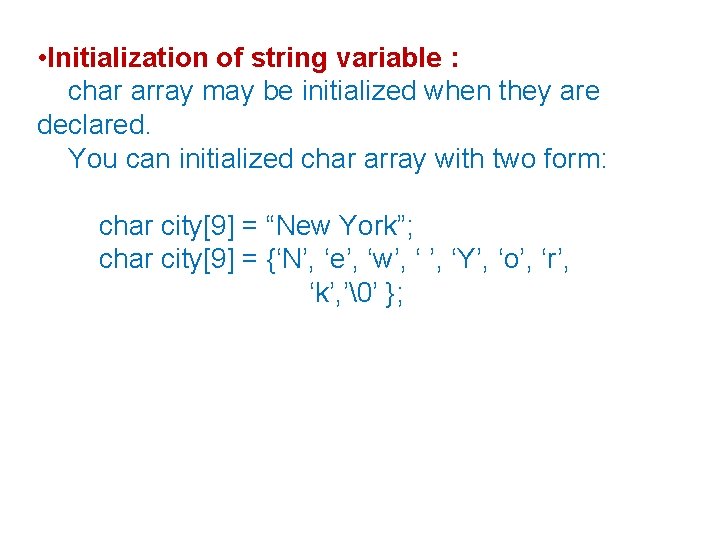
• Initialization of string variable : char array may be initialized when they are declared. You can initialized char array with two form: char city[9] = “New York”; char city[9] = {‘N’, ‘e’, ‘w’, ‘Y’, ‘o’, ‘r’, ‘k’, ’ ’ };
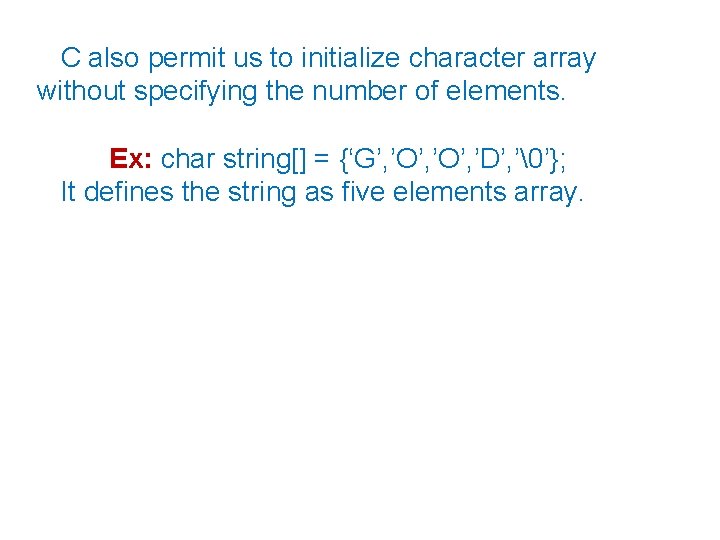
C also permit us to initialize character array without specifying the number of elements. Ex: char string[] = {‘G’, ’O’, ’D’, ’ ’}; It defines the string as five elements array.
![Reading String Ex char address15 scanfs address sign is not • Reading String : Ex: char address[15]; scanf(“%s”, address); (& sign is not](https://slidetodoc.com/presentation_image_h/2ce21db383dd046dc88f4ee2335ba93a/image-28.jpg)
• Reading String : Ex: char address[15]; scanf(“%s”, address); (& sign is not included before address) The problem with the scanf() function is that it terminates its input on the first white space it finds. Ex: If input string is “New York” then in address array stores only “New”. After New, string will be terminated. For this reason to read the string gets() function is used which reads white spaces also. Syntax: gets(arrayname); Ex. gets(address);
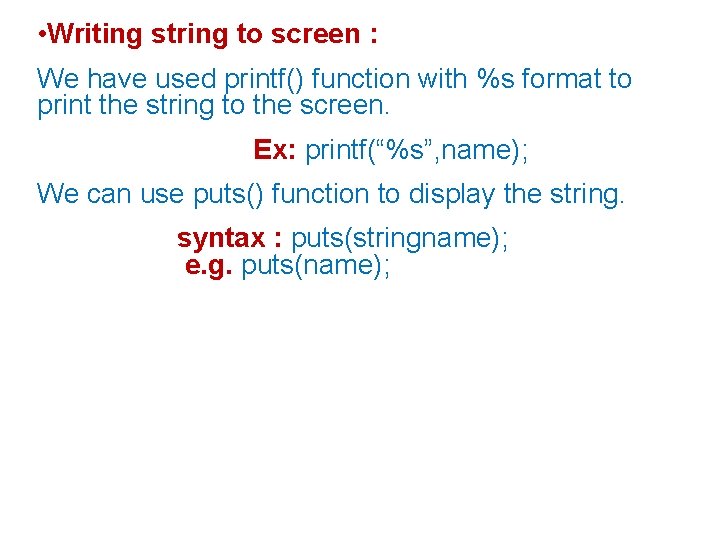
• Writing string to screen : We have used printf() function with %s format to print the string to the screen. Ex: printf(“%s”, name); We can use puts() function to display the string. syntax : puts(stringname); e. g. puts(name);
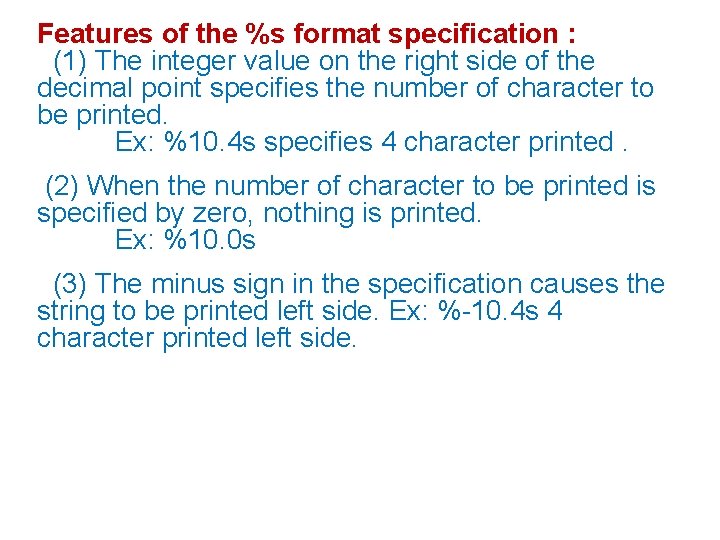
Features of the %s format specification : (1) The integer value on the right side of the decimal point specifies the number of character to be printed. Ex: %10. 4 s specifies 4 character printed. (2) When the number of character to be printed is specified by zero, nothing is printed. Ex: %10. 0 s (3) The minus sign in the specification causes the string to be printed left side. Ex: %-10. 4 s 4 character printed left side.
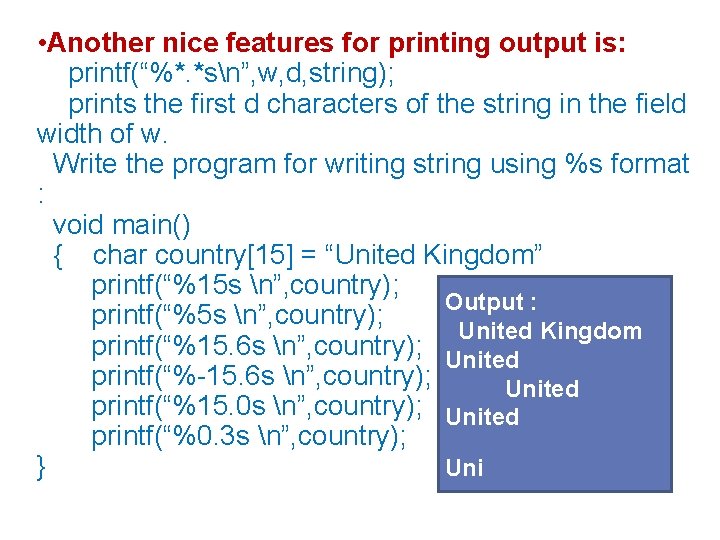
• Another nice features for printing output is: printf(“%*. *sn”, w, d, string); prints the first d characters of the string in the field width of w. Write the program for writing string using %s format : void main() { char country[15] = “United Kingdom” printf(“%15 s n”, country); Output : printf(“%5 s n”, country); United Kingdom printf(“%15. 6 s n”, country); United printf(“%-15. 6 s n”, country); United printf(“%15. 0 s n”, country); United printf(“%0. 3 s n”, country); } Uni
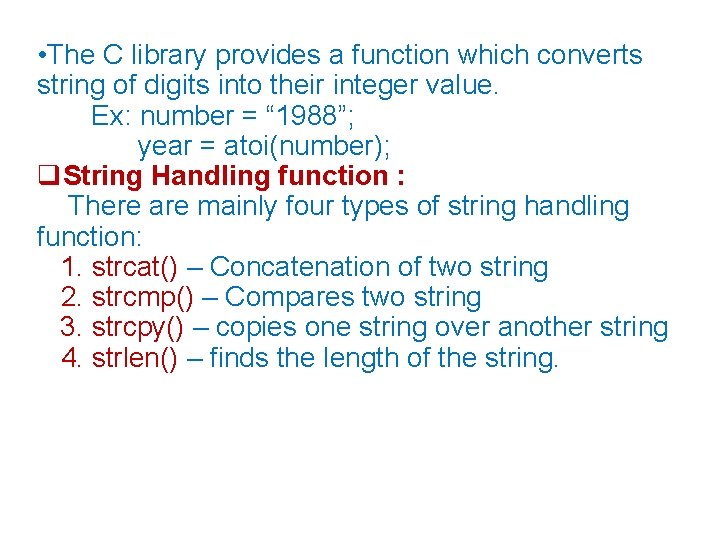
• The C library provides a function which converts string of digits into their integer value. Ex: number = “ 1988”; year = atoi(number); String Handling function : There are mainly four types of string handling function: 1. strcat() – Concatenation of two string 2. strcmp() – Compares two string 3. strcpy() – copies one string over another string 4. strlen() – finds the length of the string.
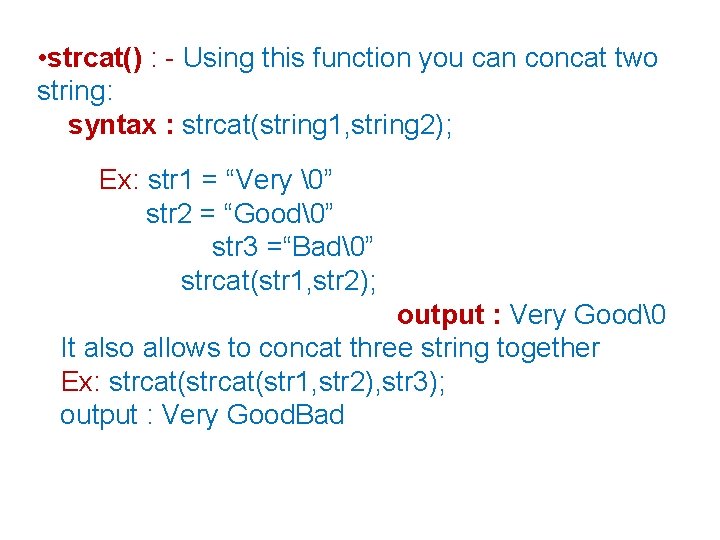
• strcat() : - Using this function you can concat two string: syntax : strcat(string 1, string 2); Ex: str 1 = “Very ” str 2 = “Good ” str 3 =“Bad ” strcat(str 1, str 2); output : Very Good It also allows to concat three string together Ex: strcat(str 1, str 2), str 3); output : Very Good. Bad
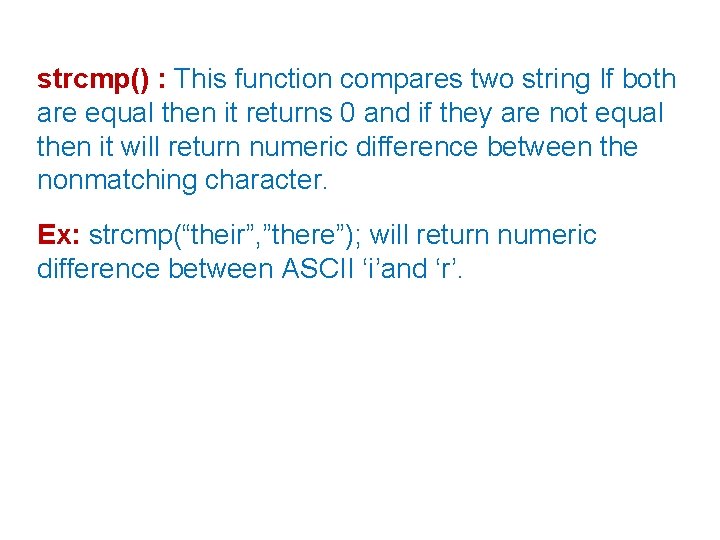
strcmp() : This function compares two string If both are equal then it returns 0 and if they are not equal then it will return numeric difference between the nonmatching character. Ex: strcmp(“their”, ”there”); will return numeric difference between ASCII ‘i’and ‘r’.
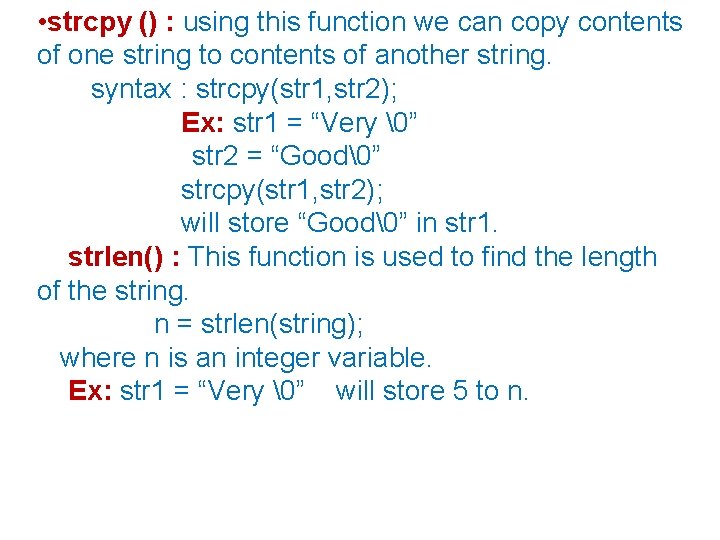
• strcpy () : using this function we can copy contents of one string to contents of another string. syntax : strcpy(str 1, str 2); Ex: str 1 = “Very ” str 2 = “Good ” strcpy(str 1, str 2); will store “Good ” in str 1. strlen() : This function is used to find the length of the string. n = strlen(string); where n is an integer variable. Ex: str 1 = “Very ” will store 5 to n.