Chapter 13 Graphical User Interface Components Part 1
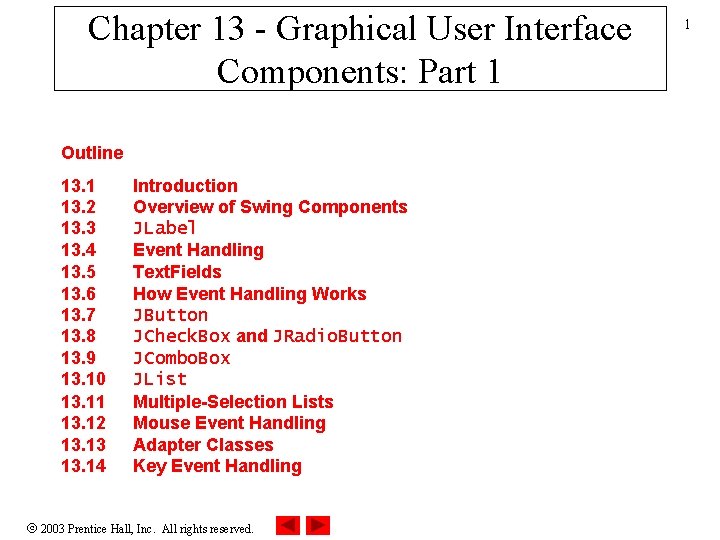
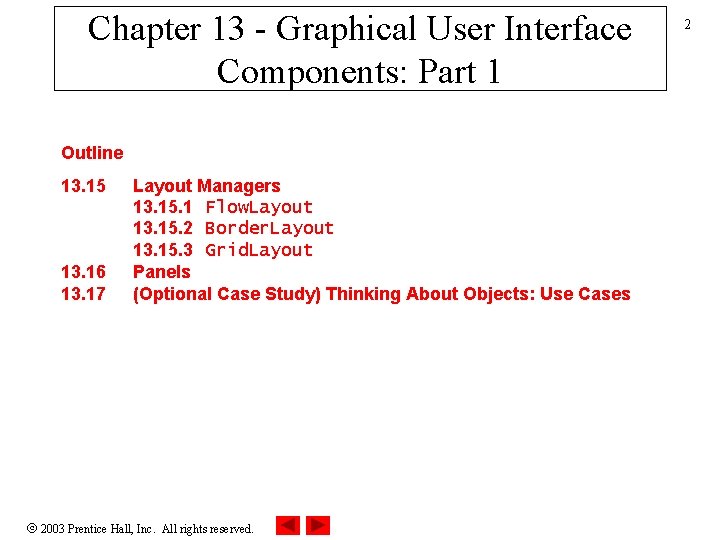
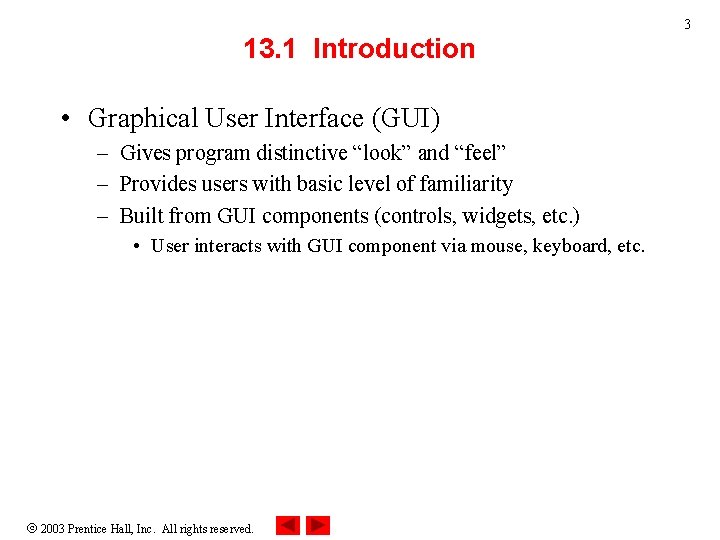
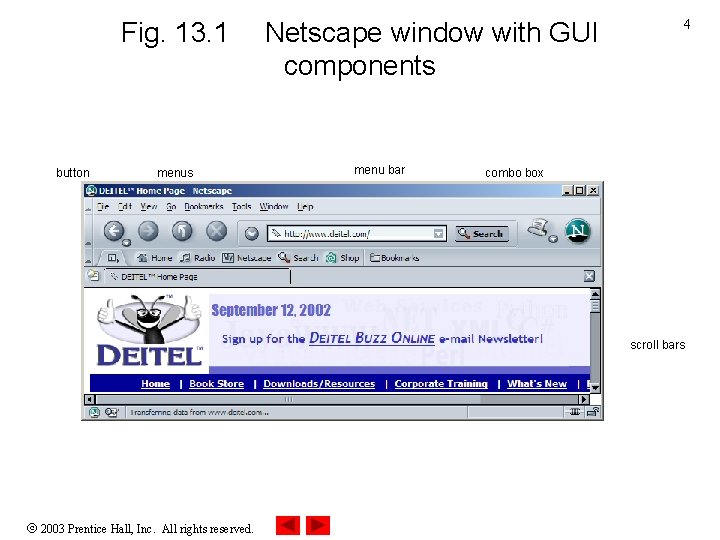
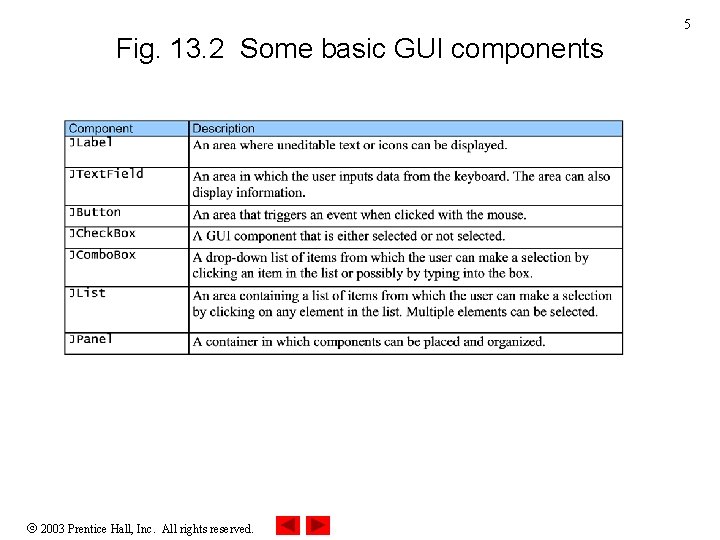
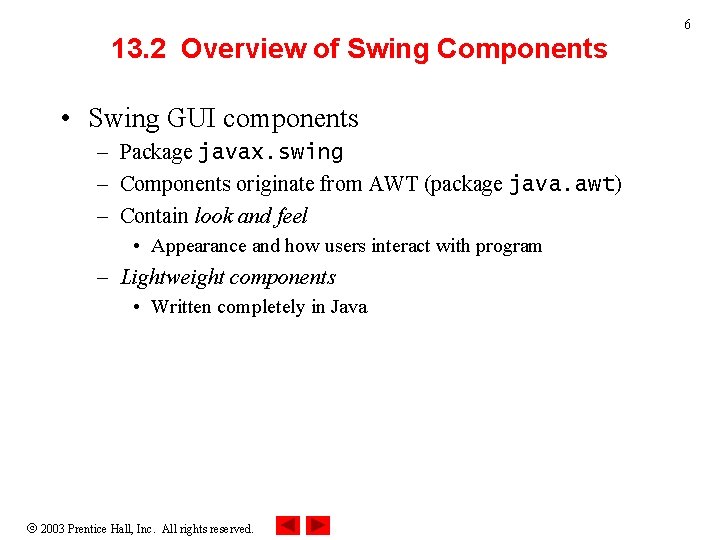
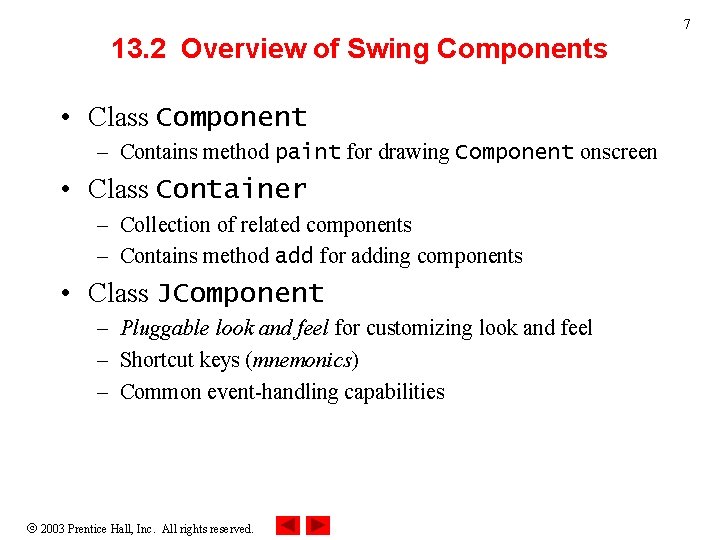
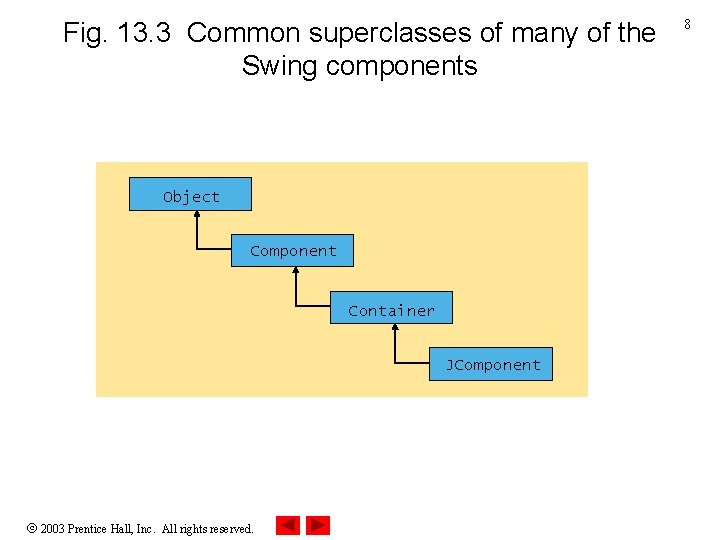
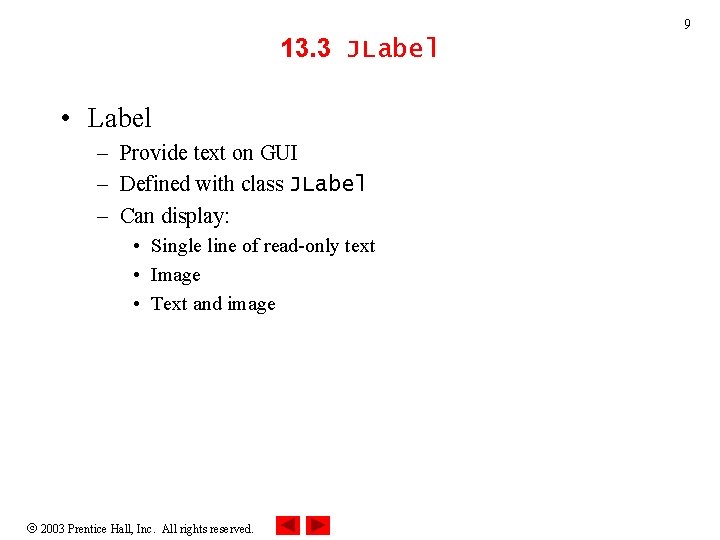
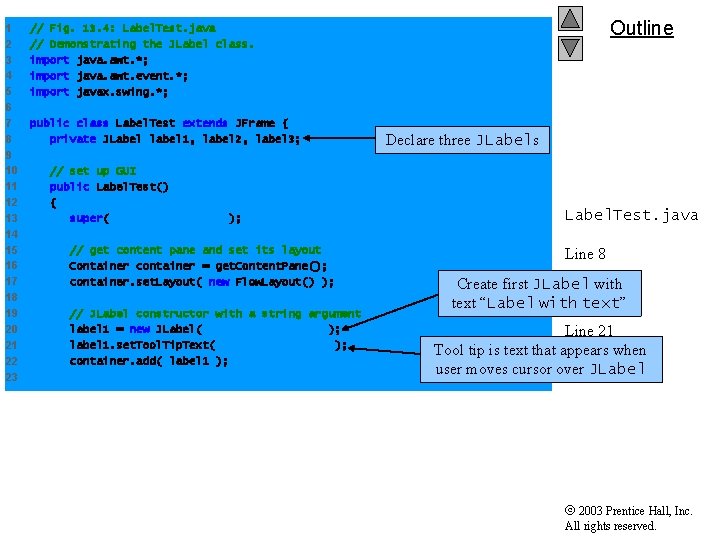
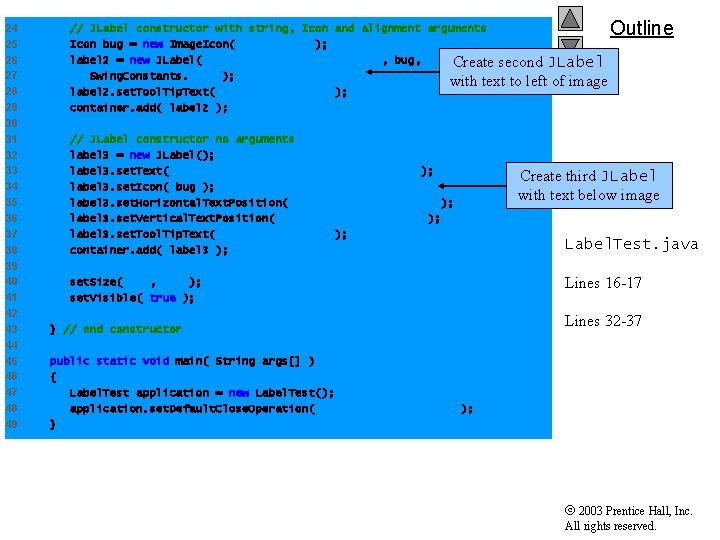
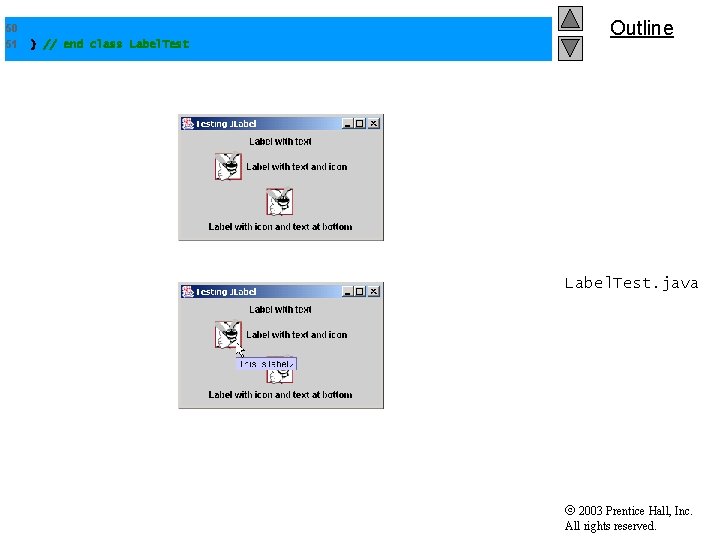
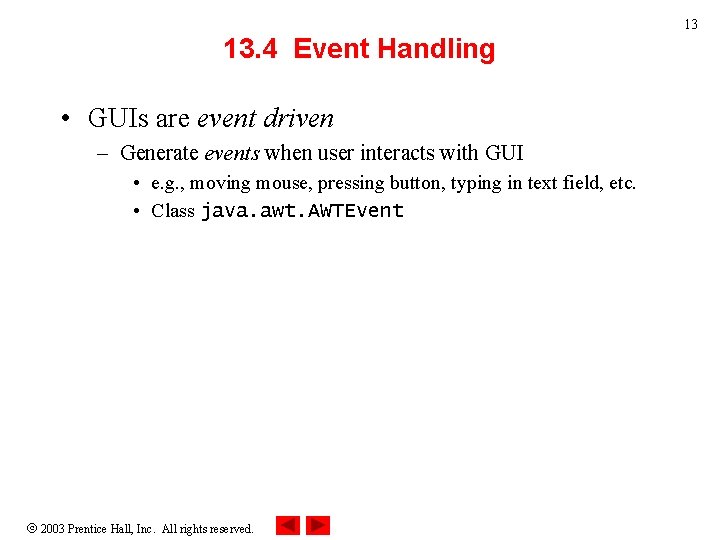
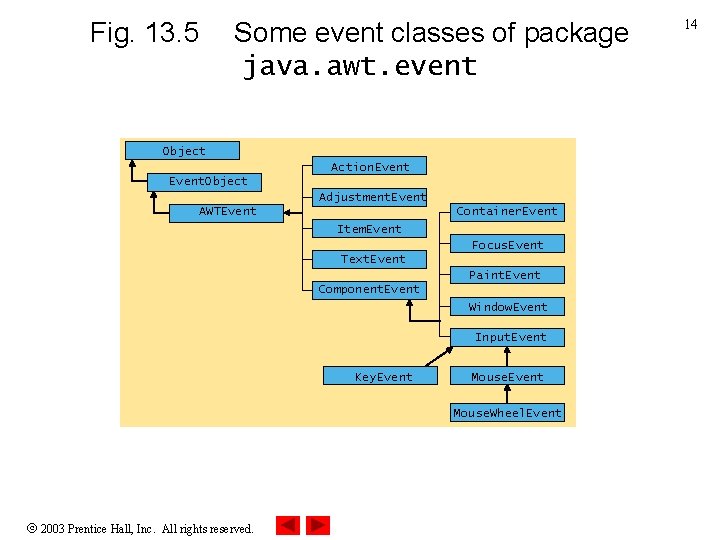
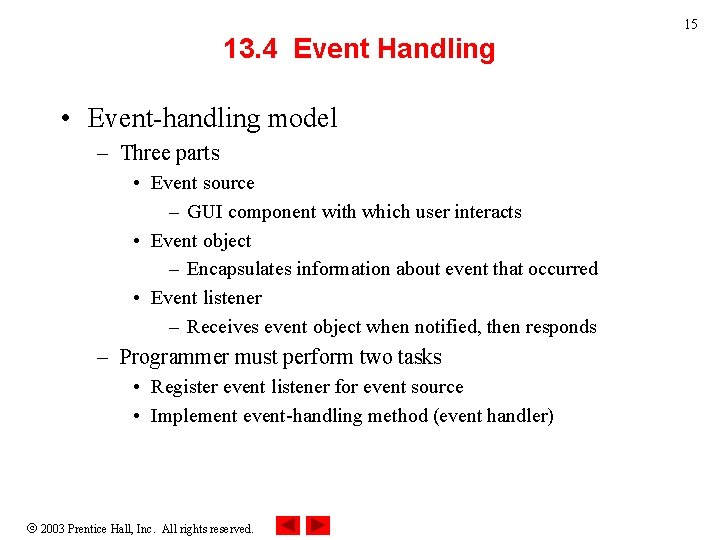
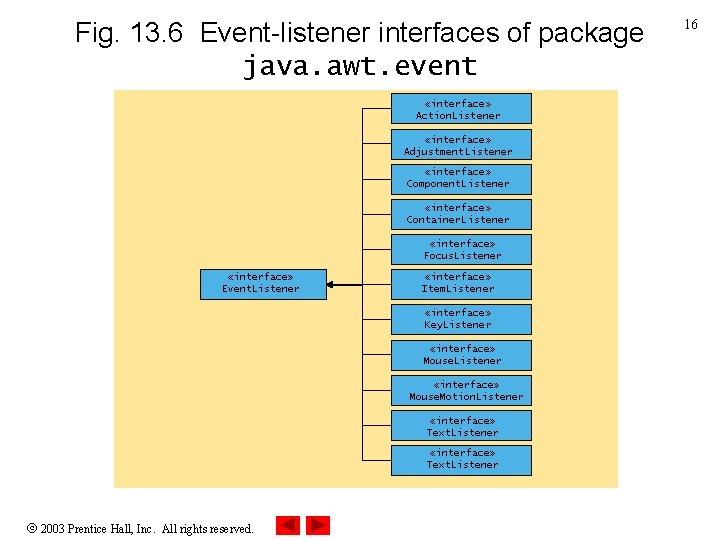
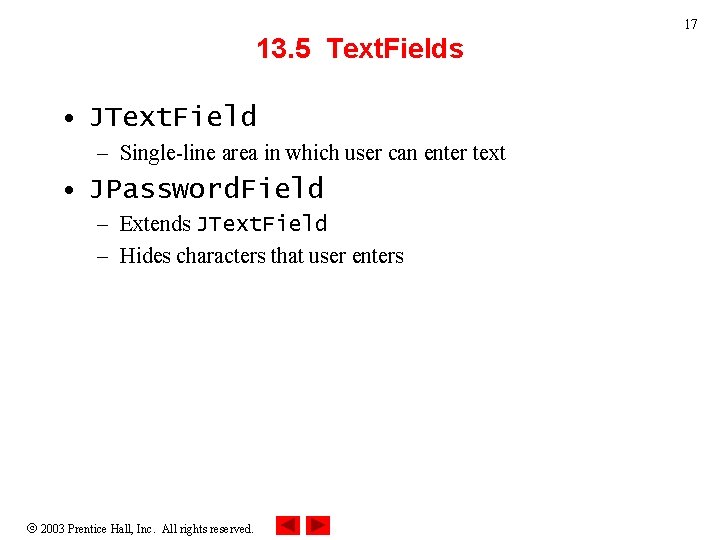
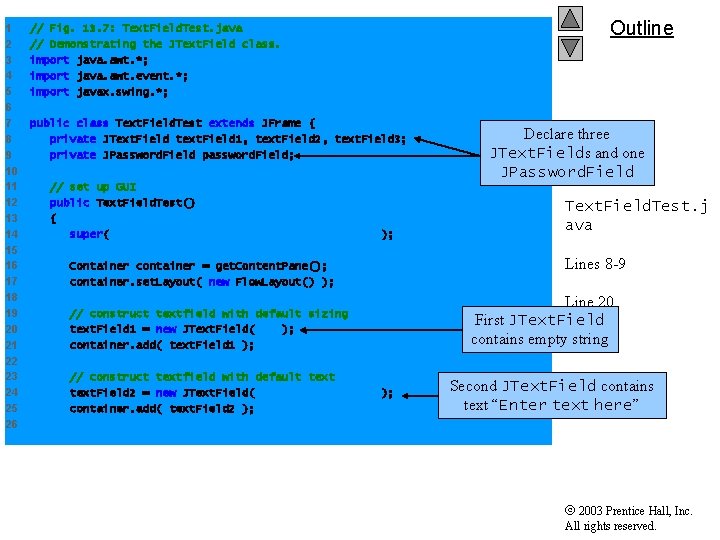
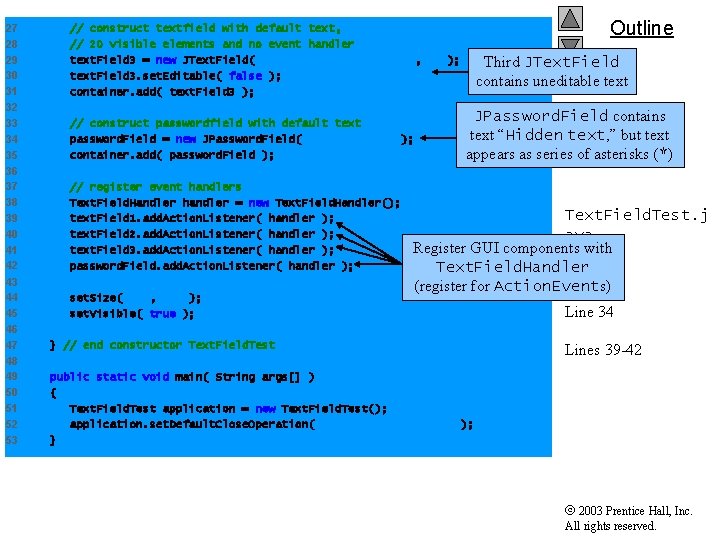
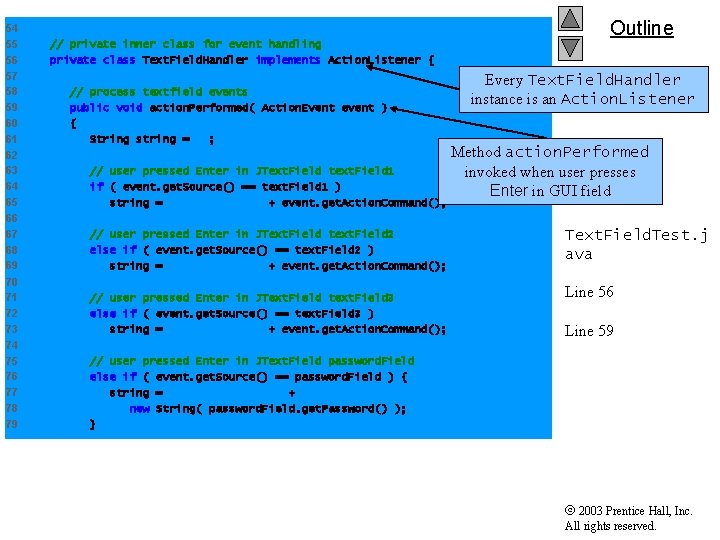
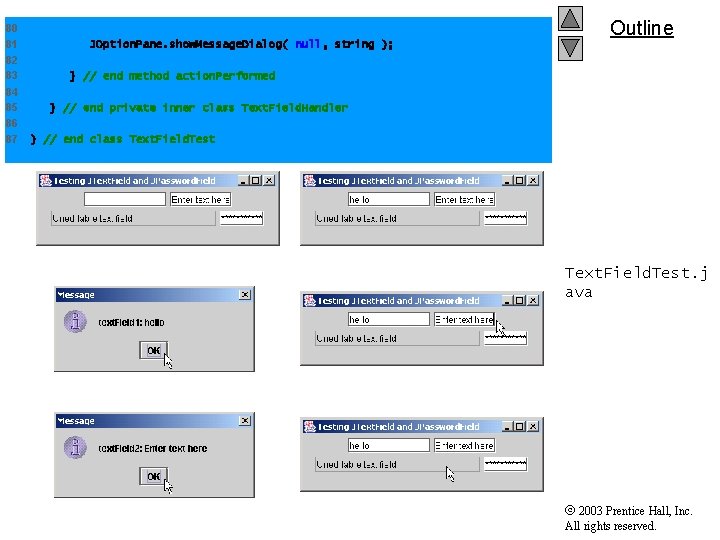
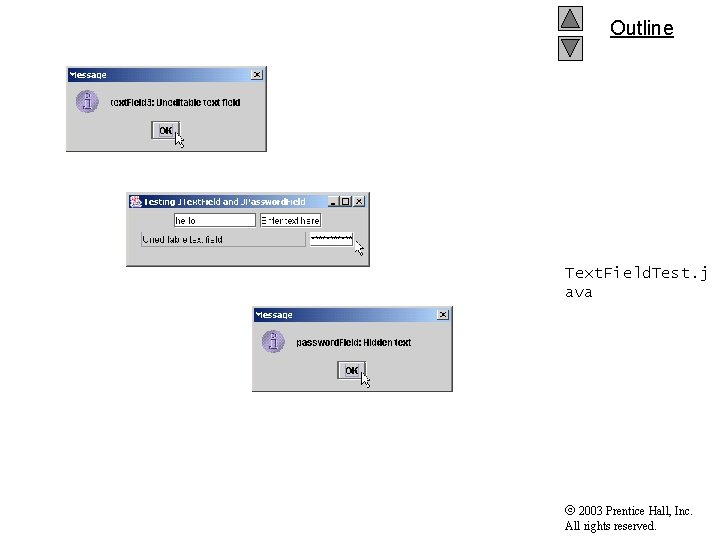
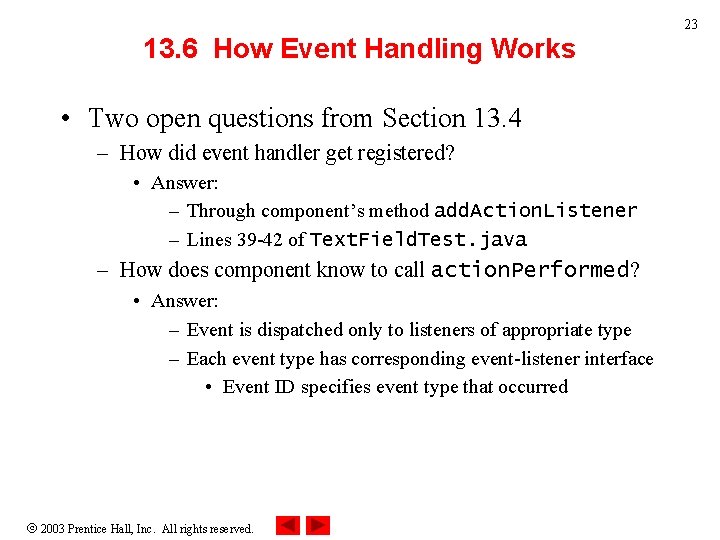
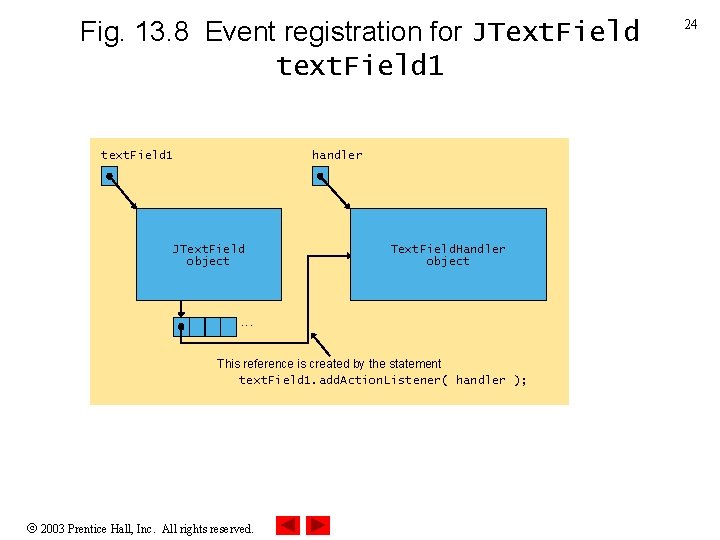
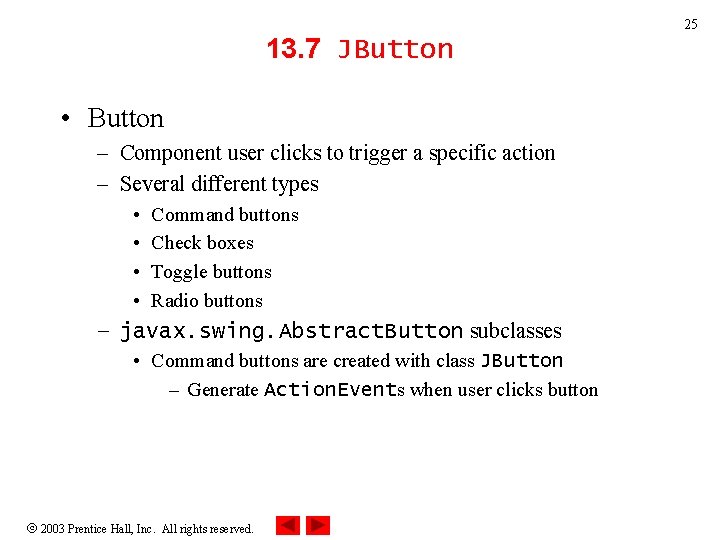
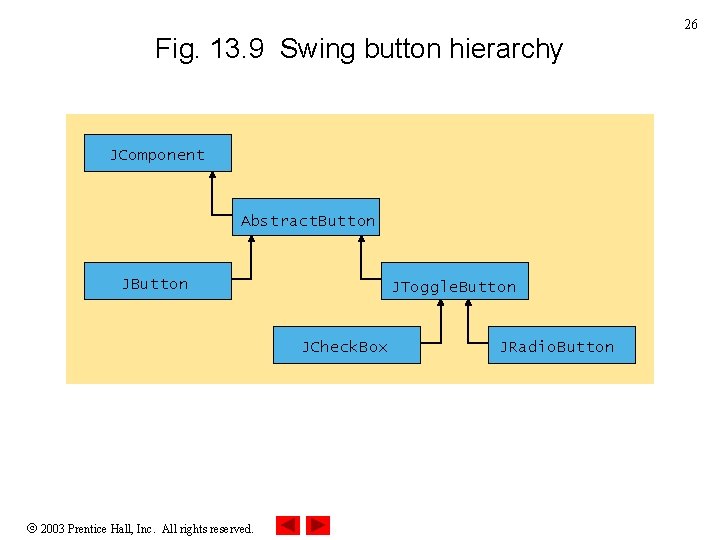
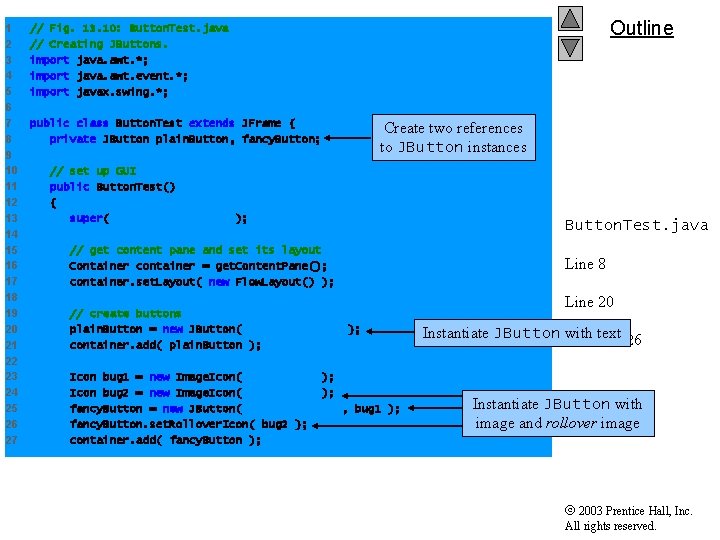
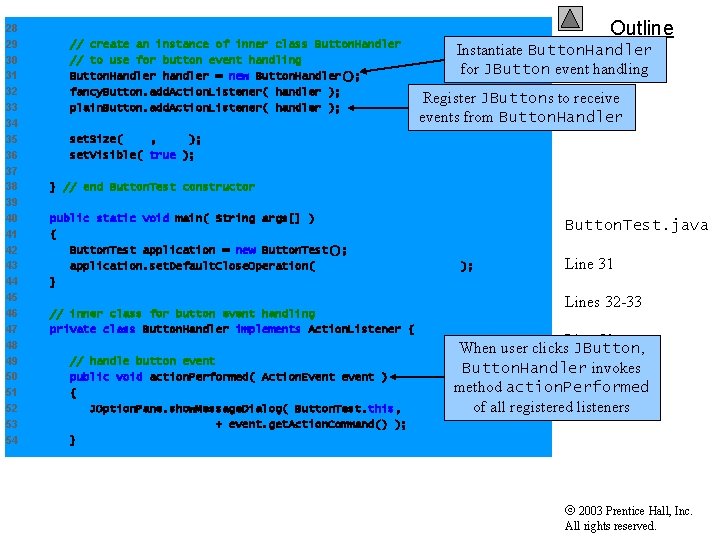
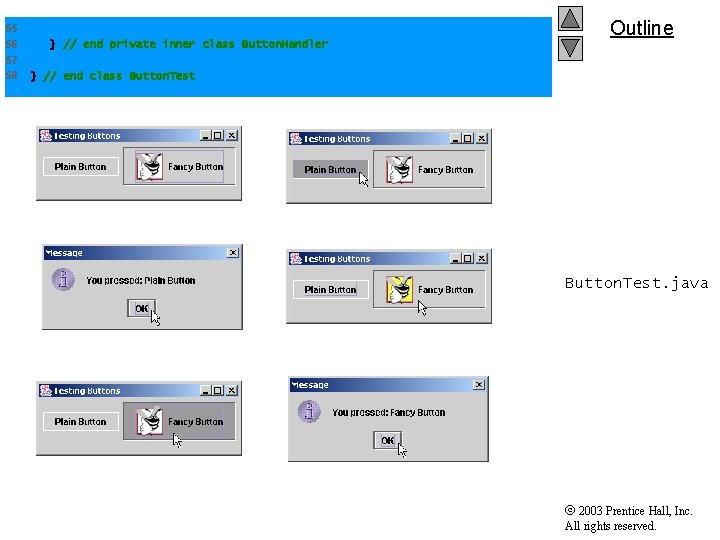
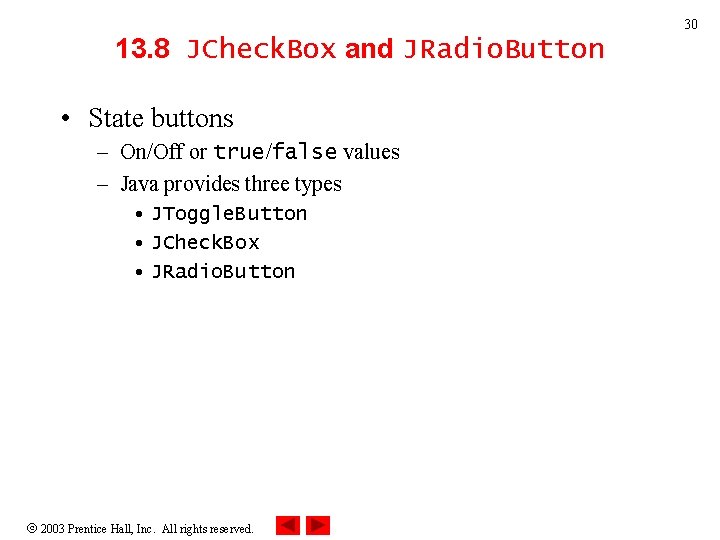
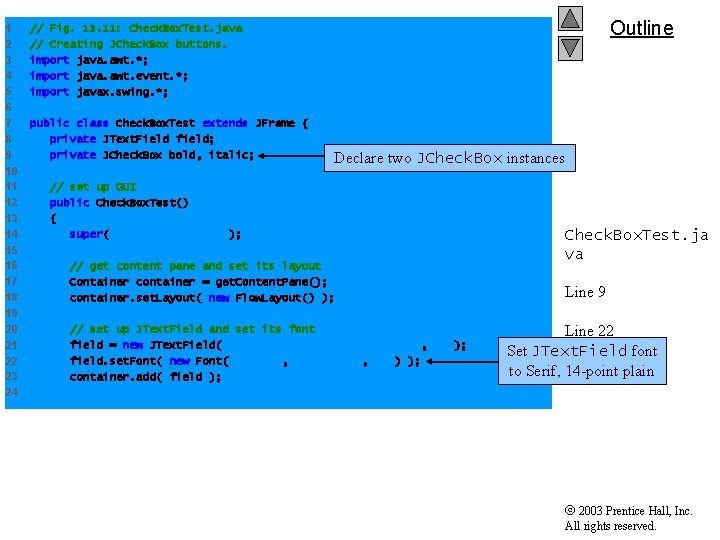
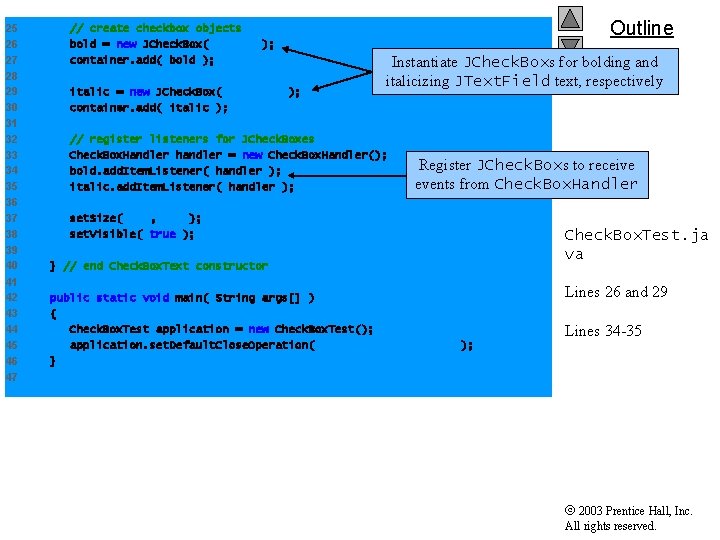
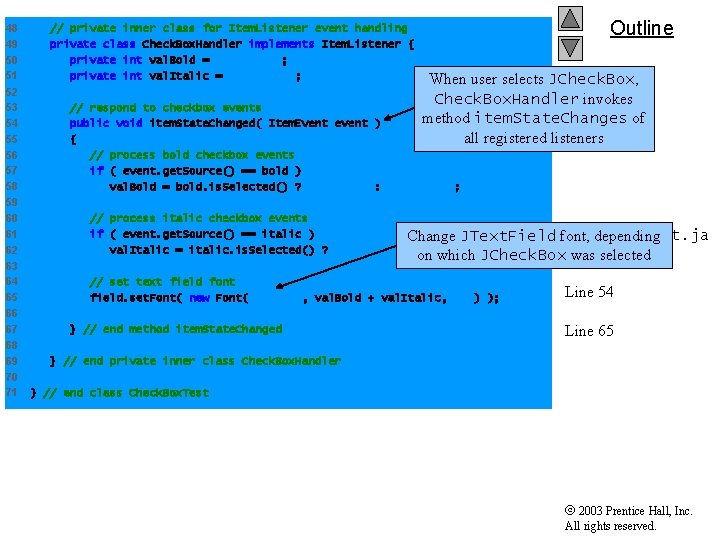
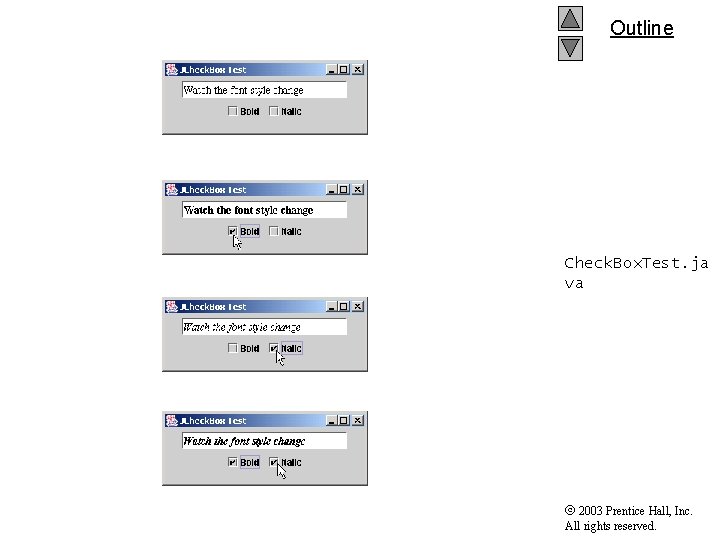
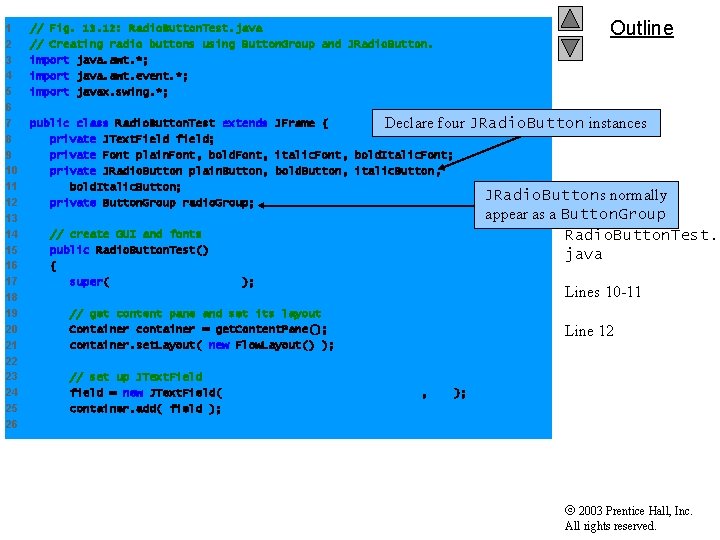
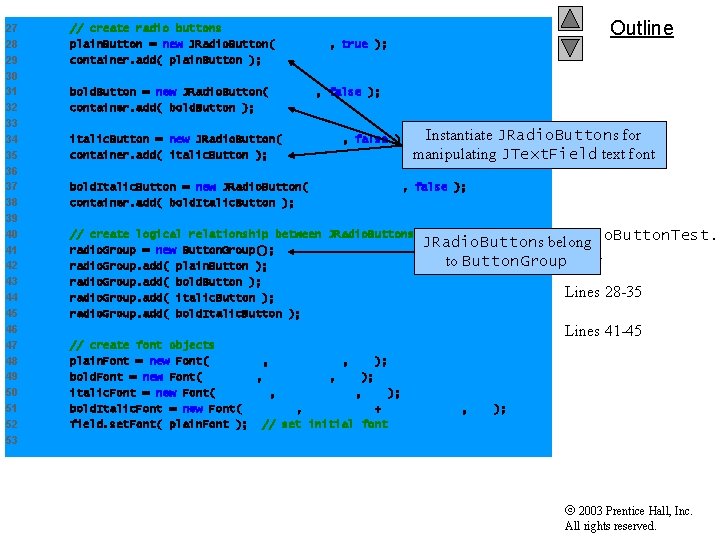
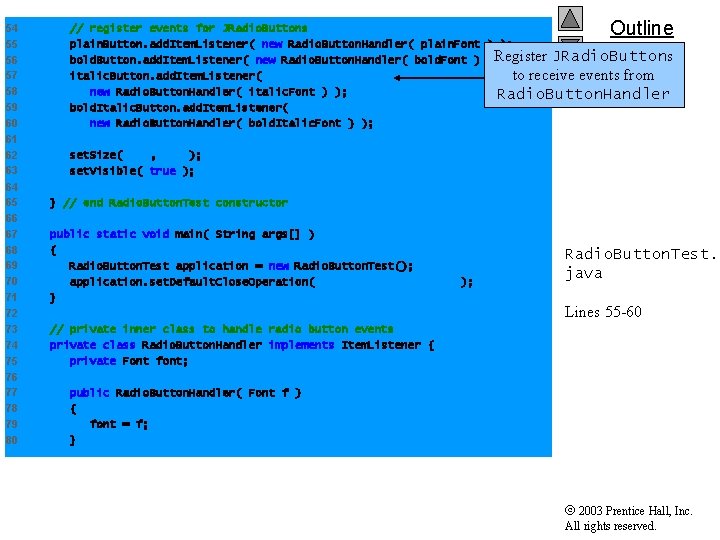
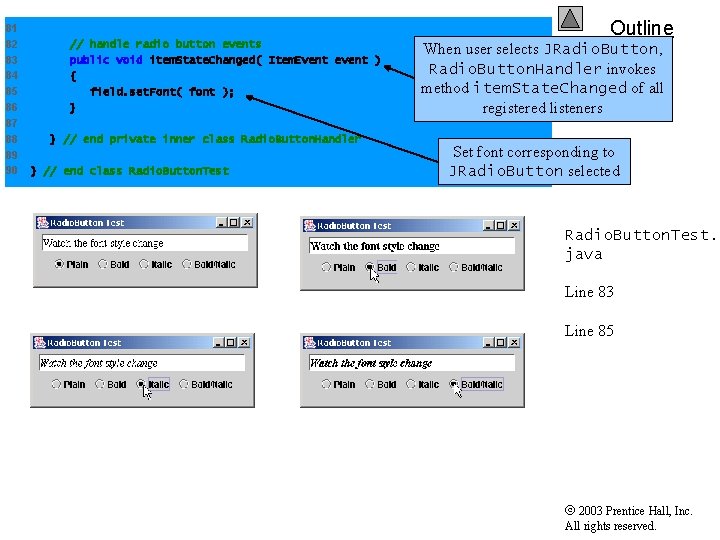
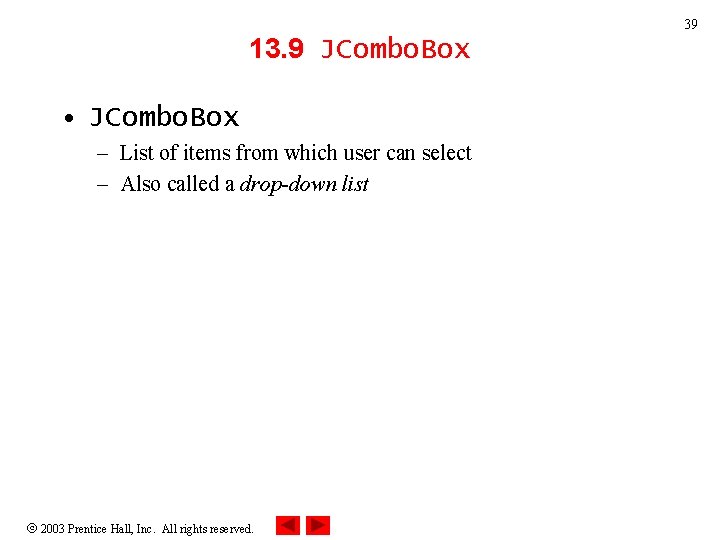
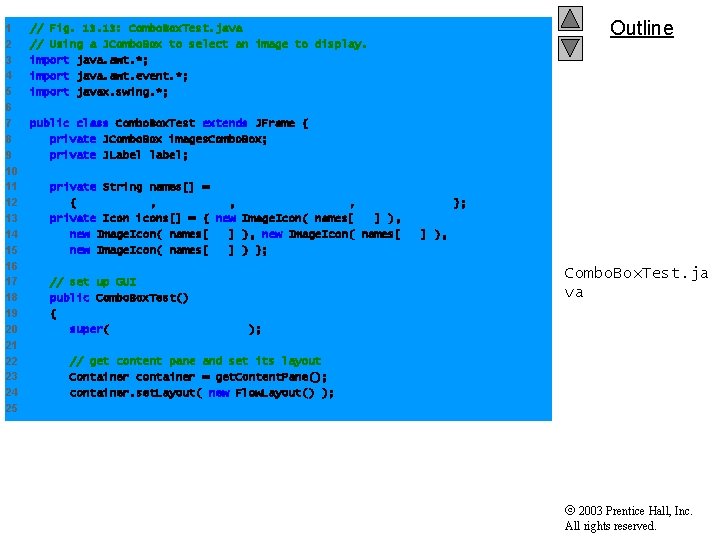
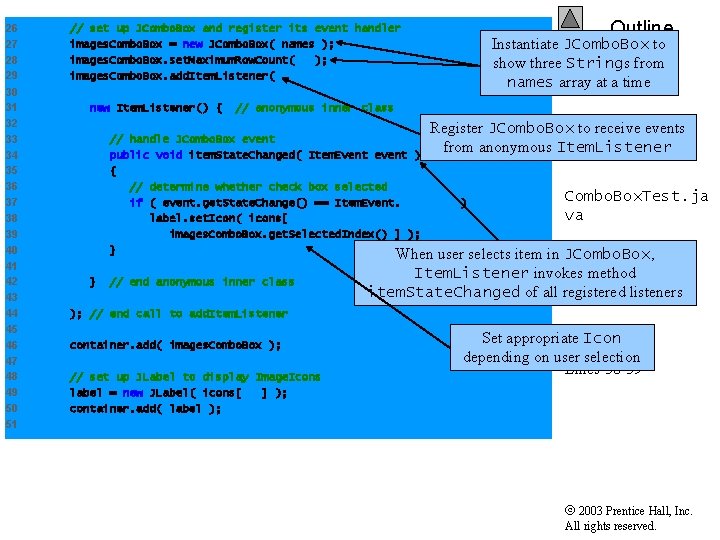
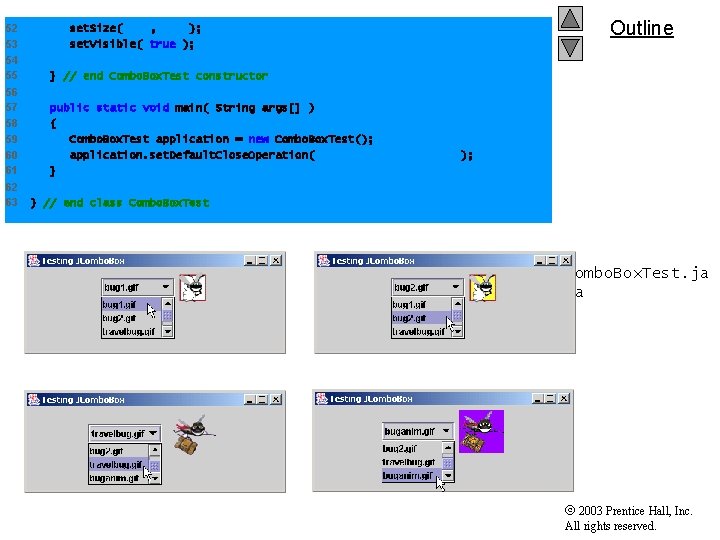
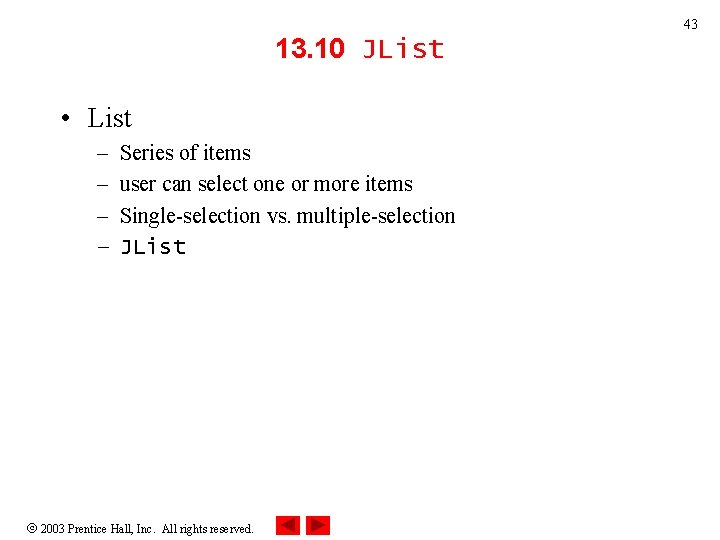
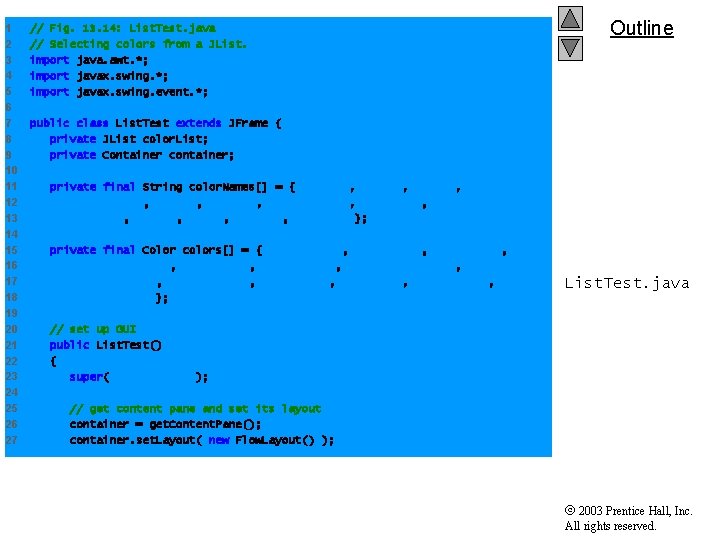
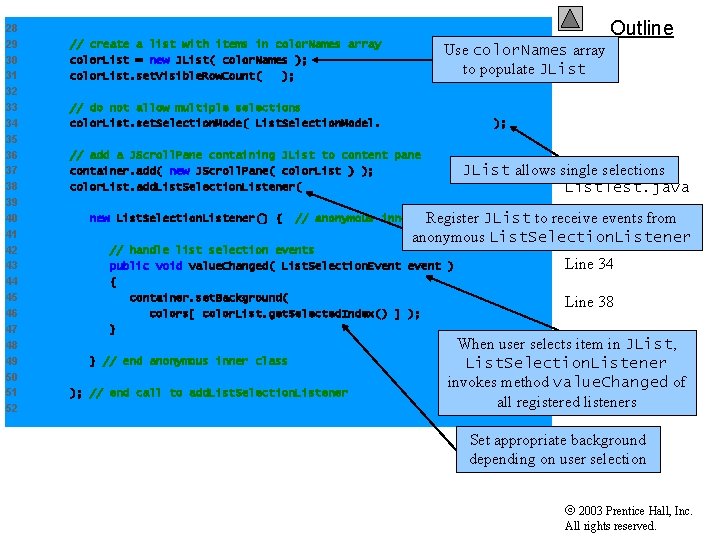
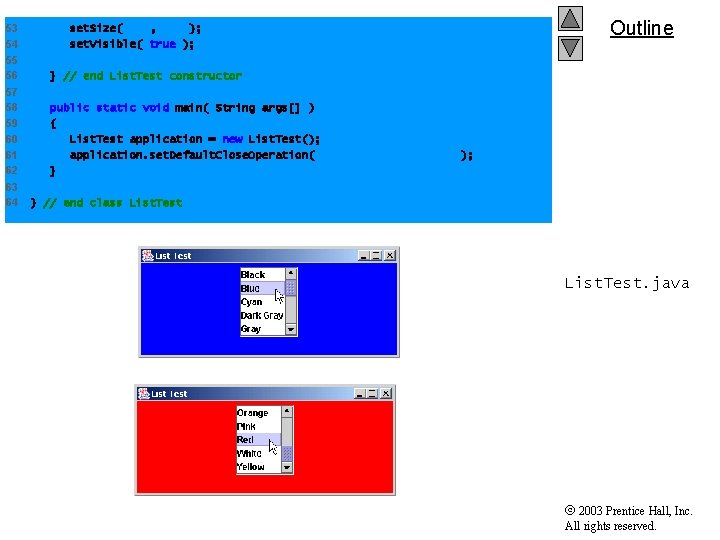
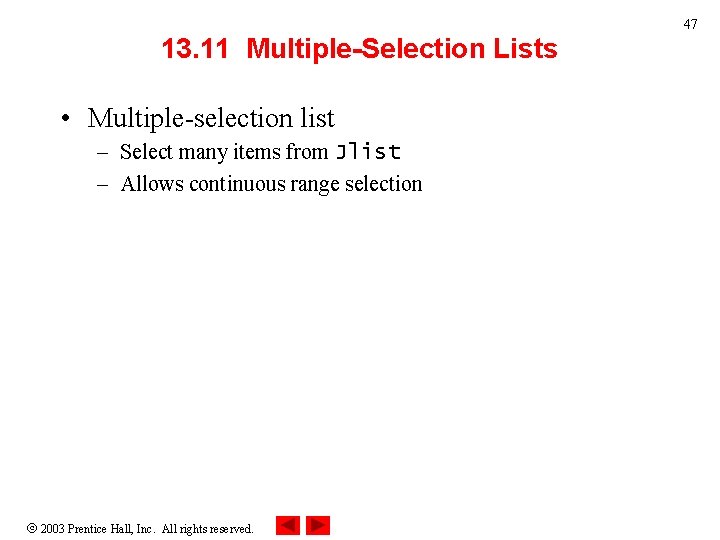
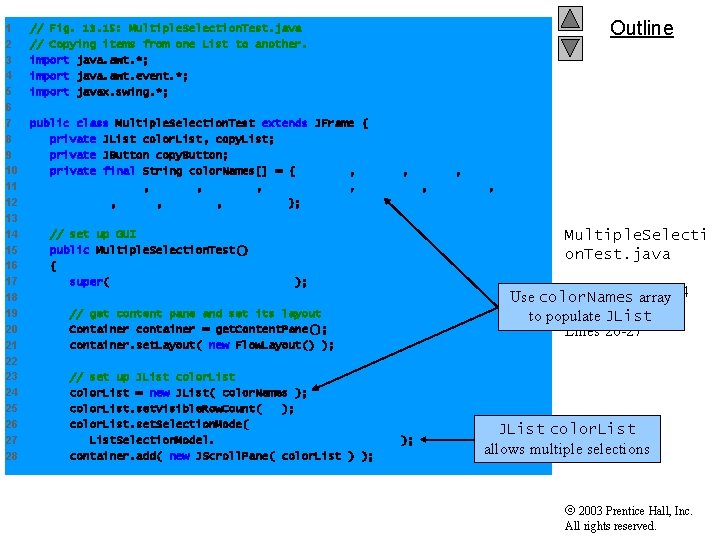
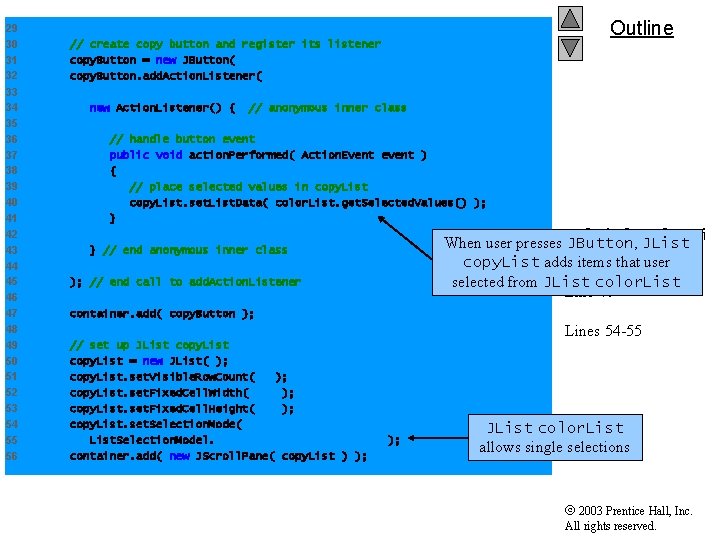
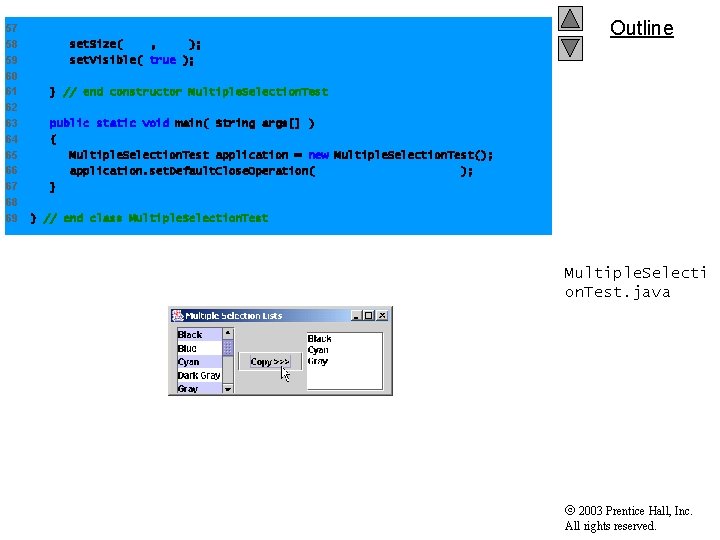
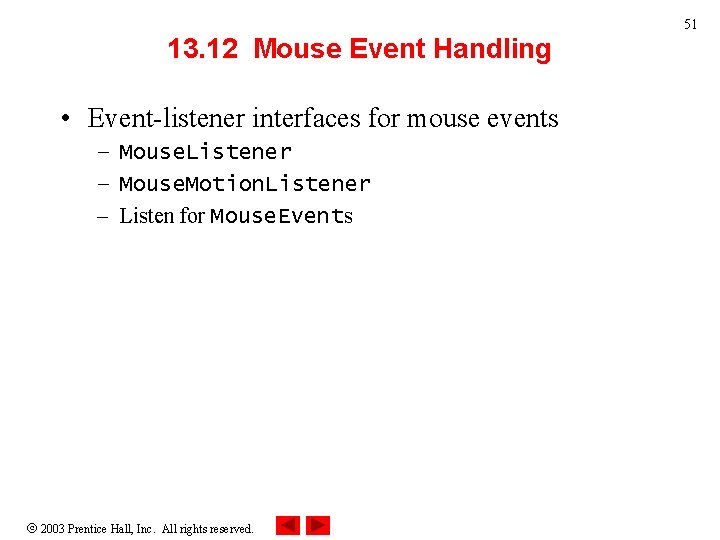
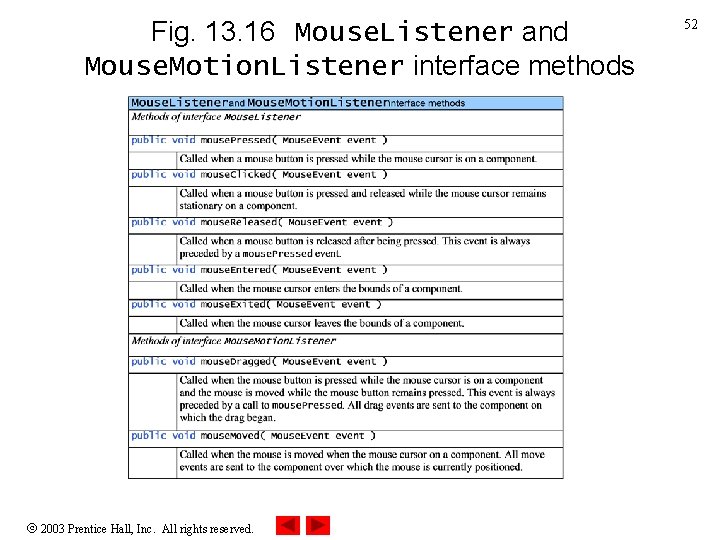
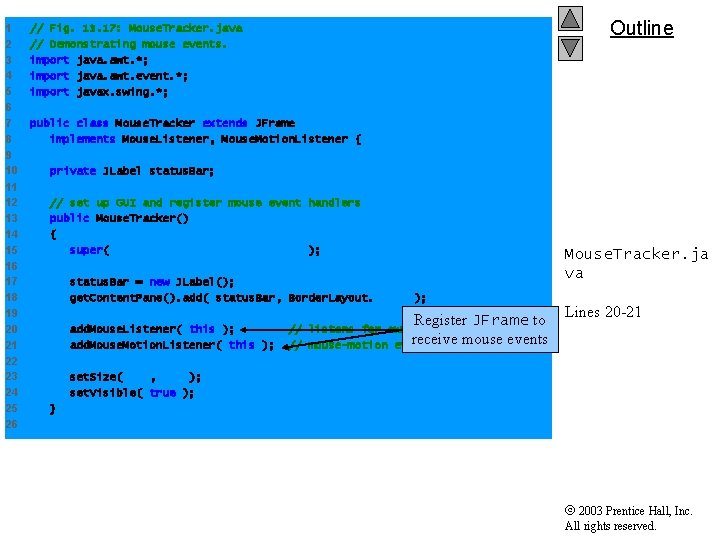
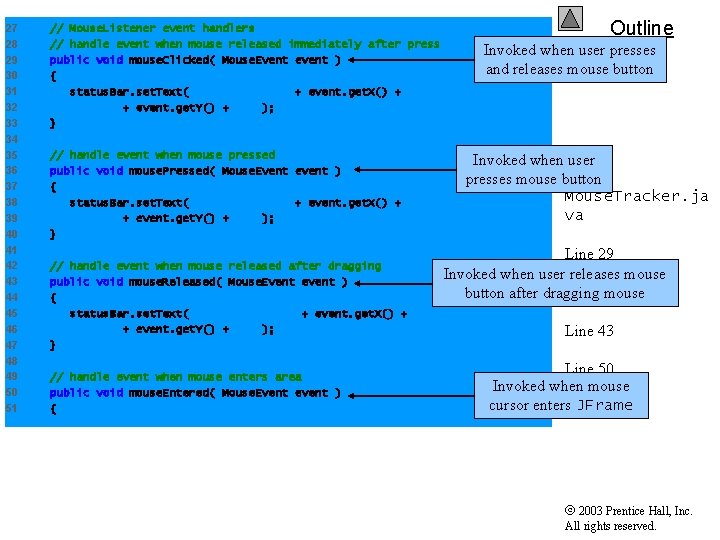
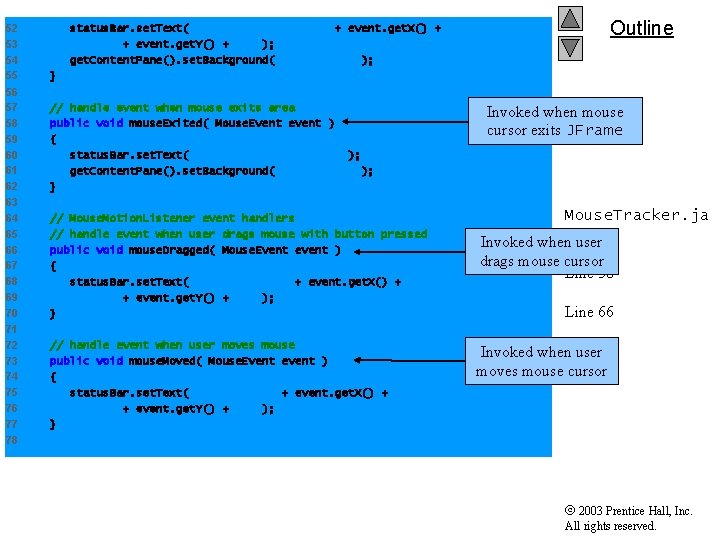
![79 80 81 82 83 84 85 public static void main( String args[] ) 79 80 81 82 83 84 85 public static void main( String args[] )](https://slidetodoc.com/presentation_image_h2/a75e4a64e3dd0a25bd045296b3dce9f9/image-56.jpg)
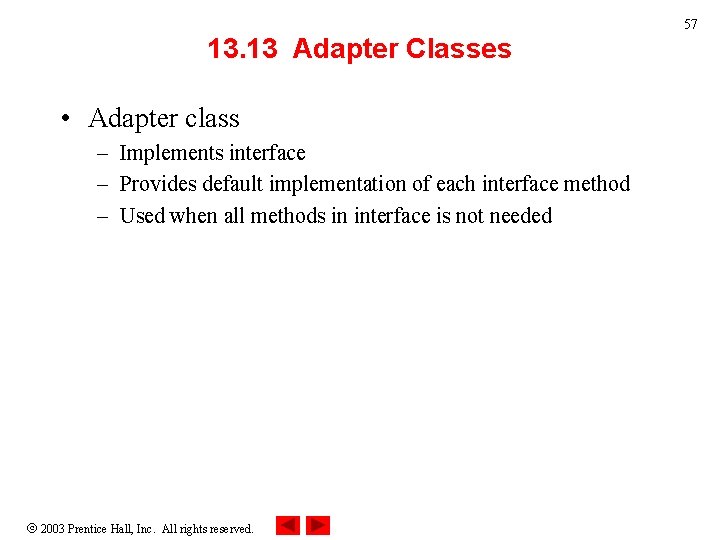
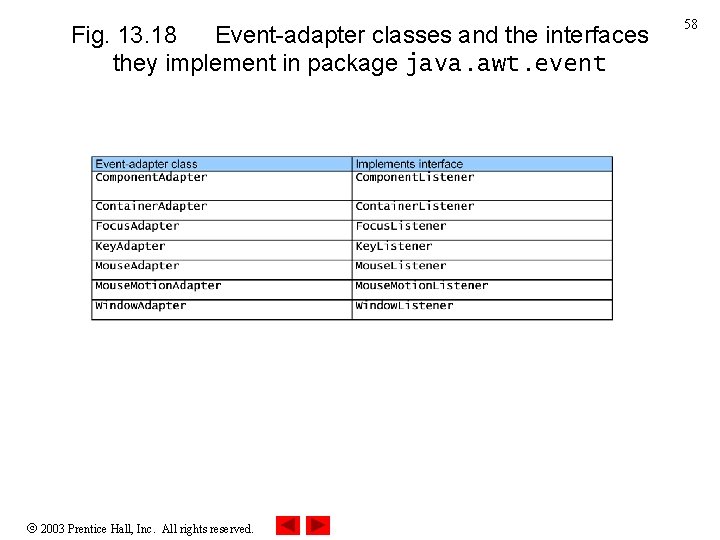
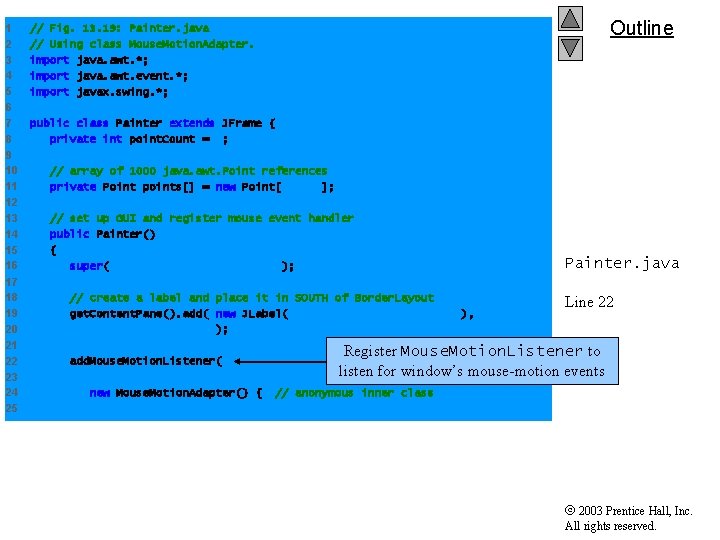
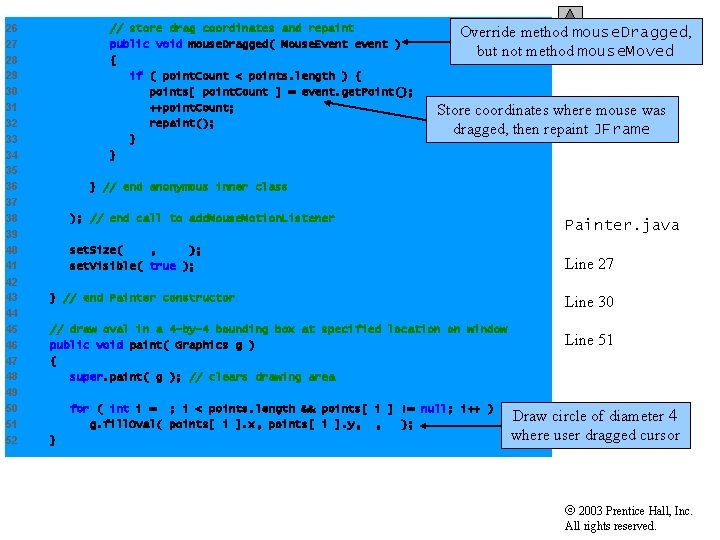
![53 54 55 56 57 58 59 60 public static void main( String args[] 53 54 55 56 57 58 59 60 public static void main( String args[]](https://slidetodoc.com/presentation_image_h2/a75e4a64e3dd0a25bd045296b3dce9f9/image-61.jpg)
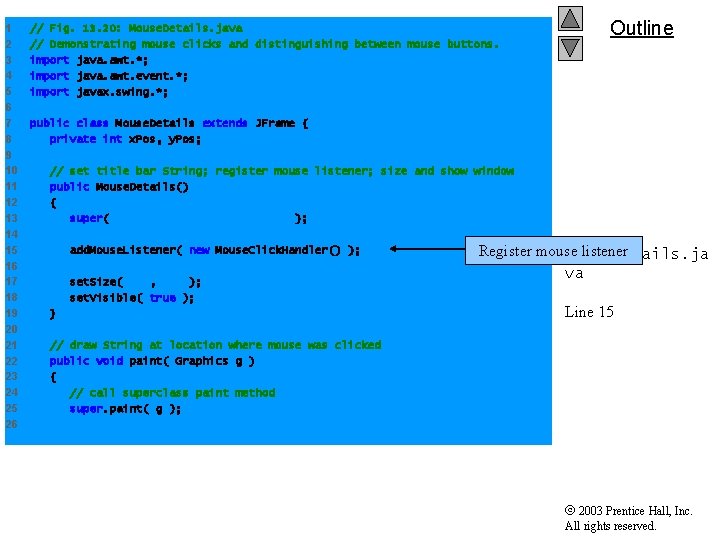
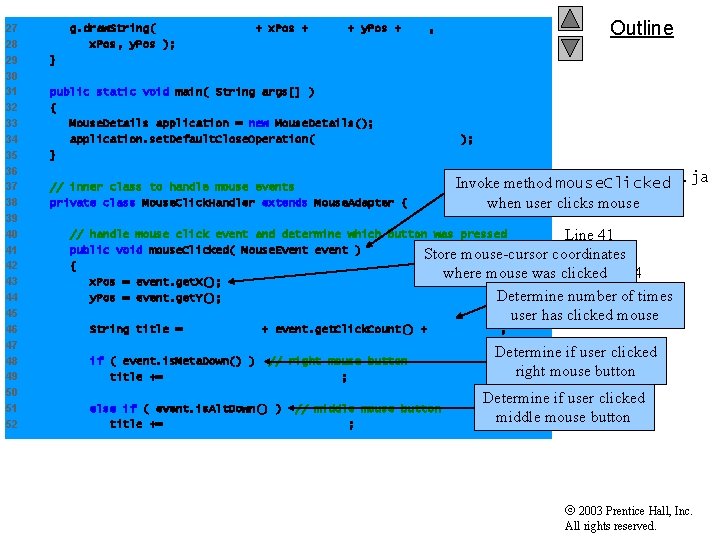
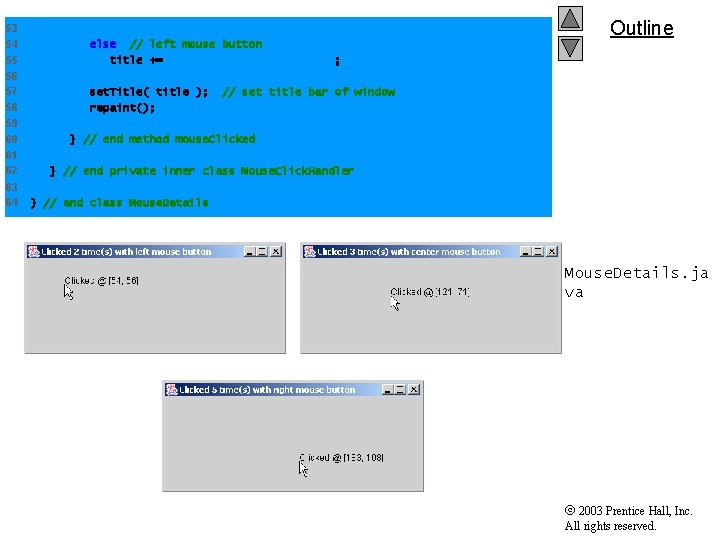
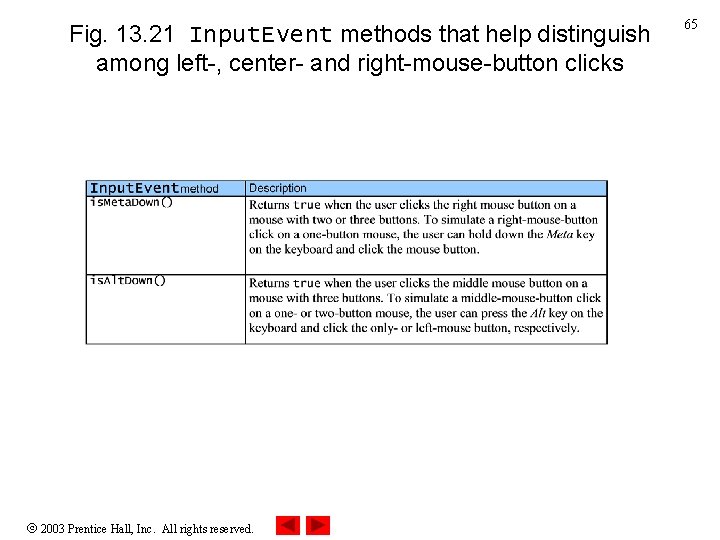
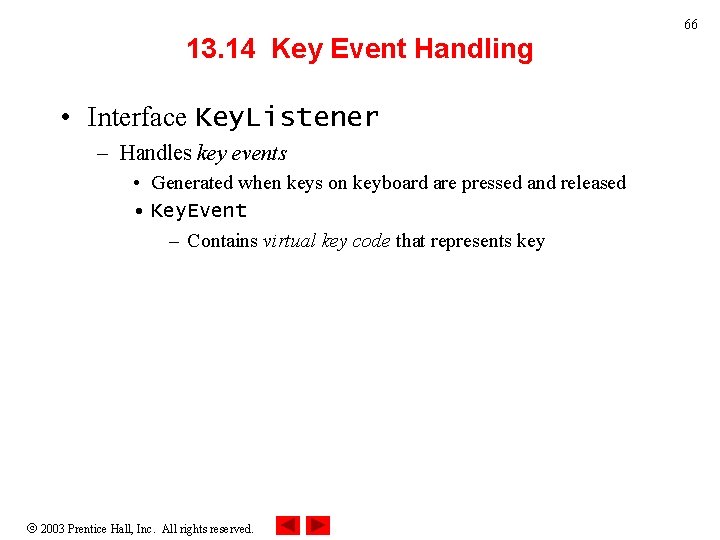
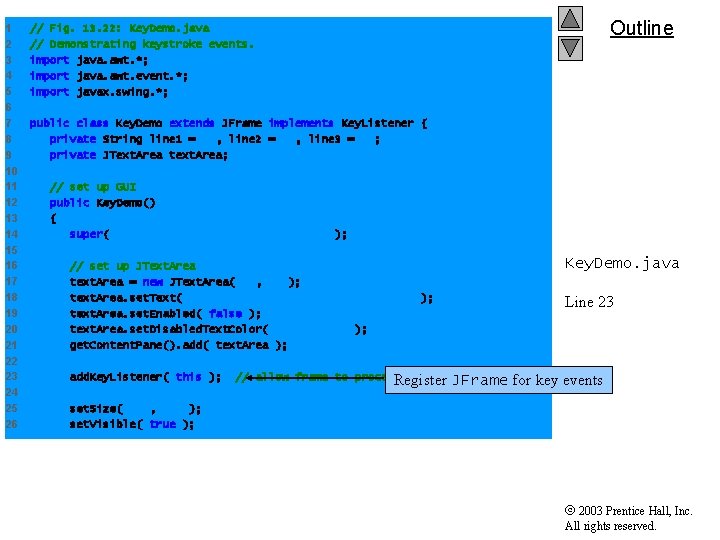
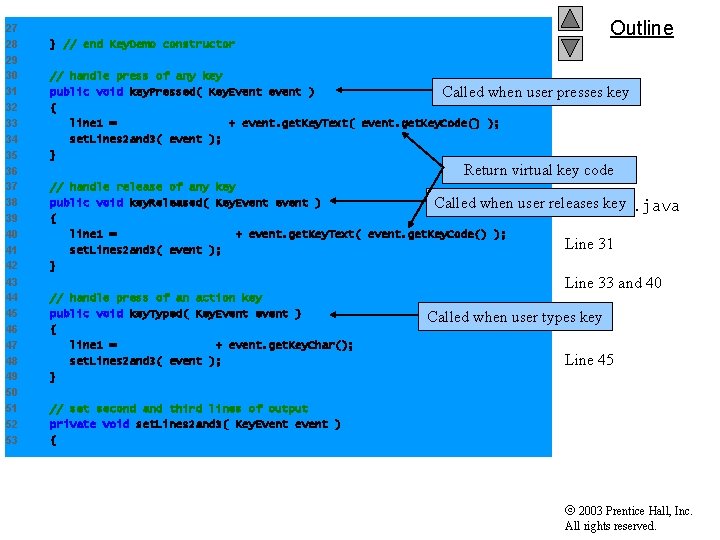
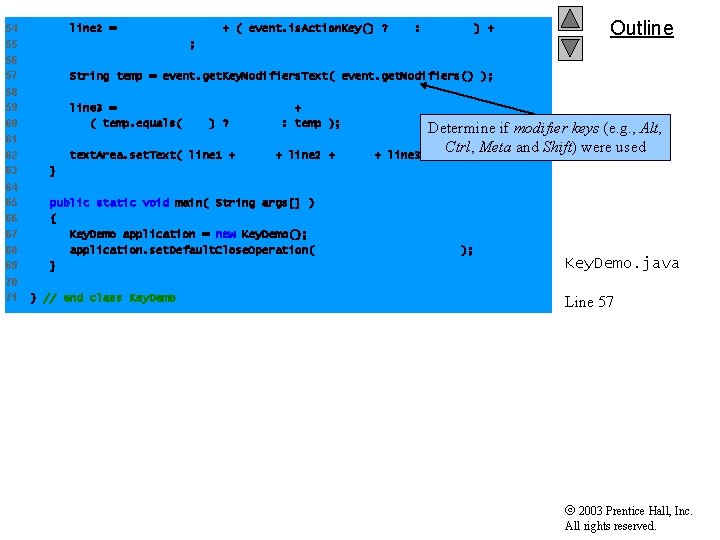
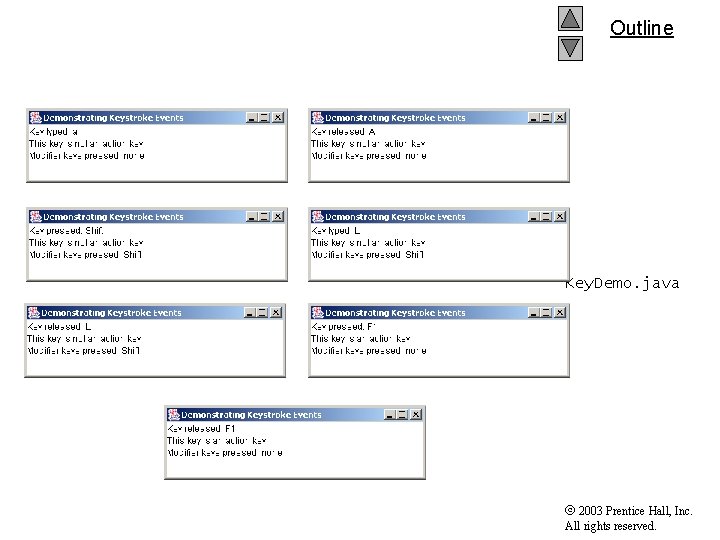
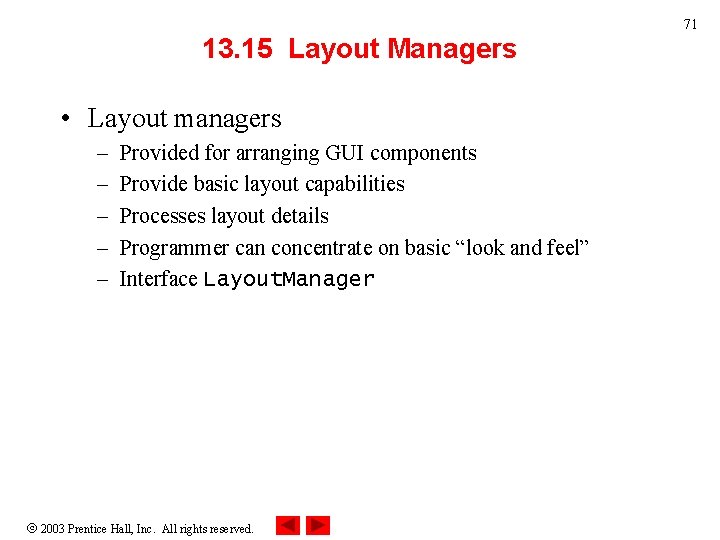
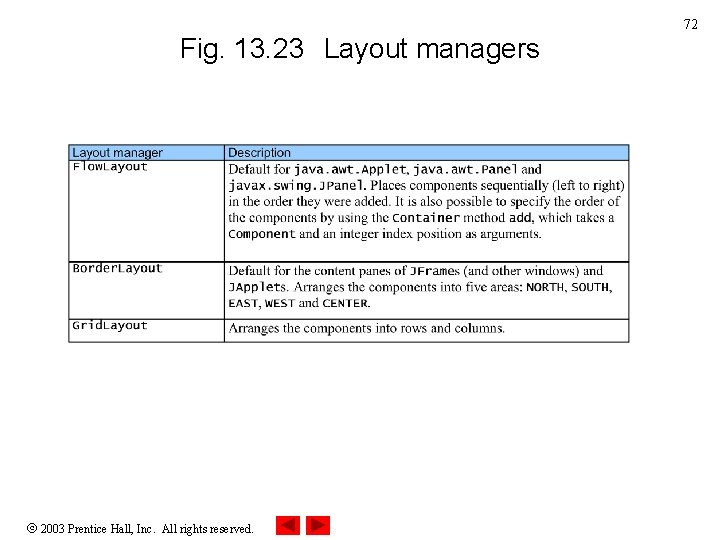
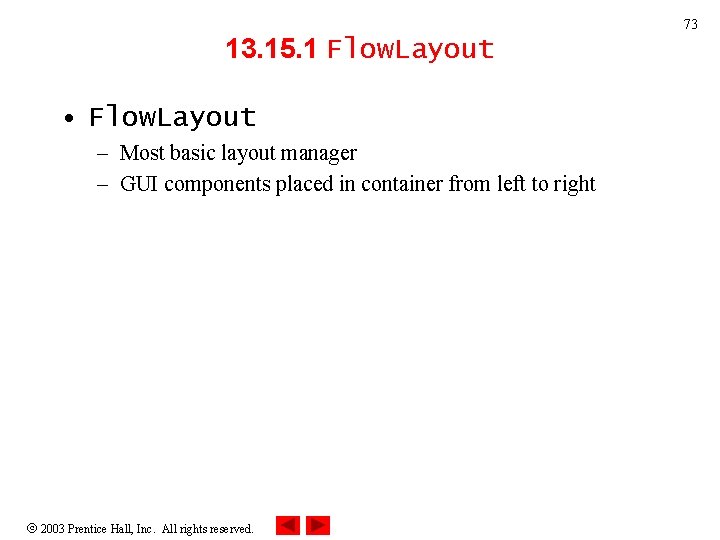
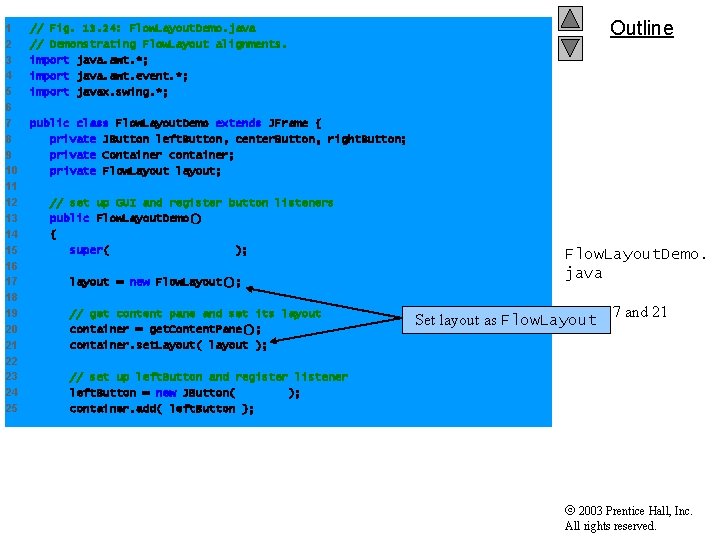
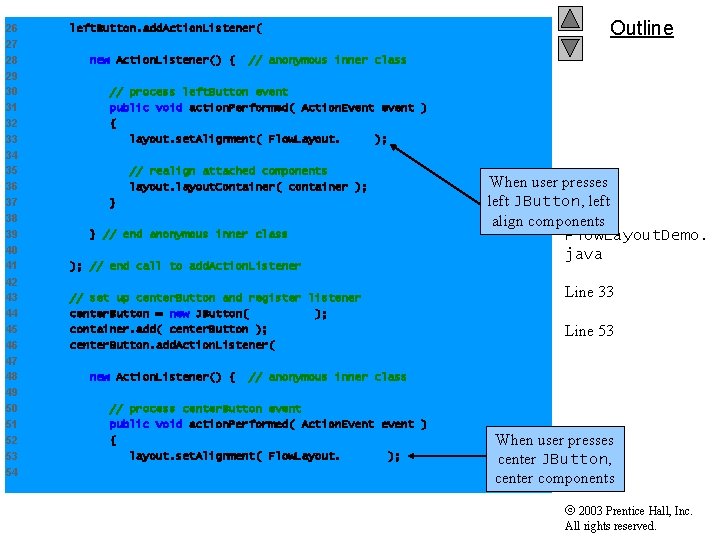
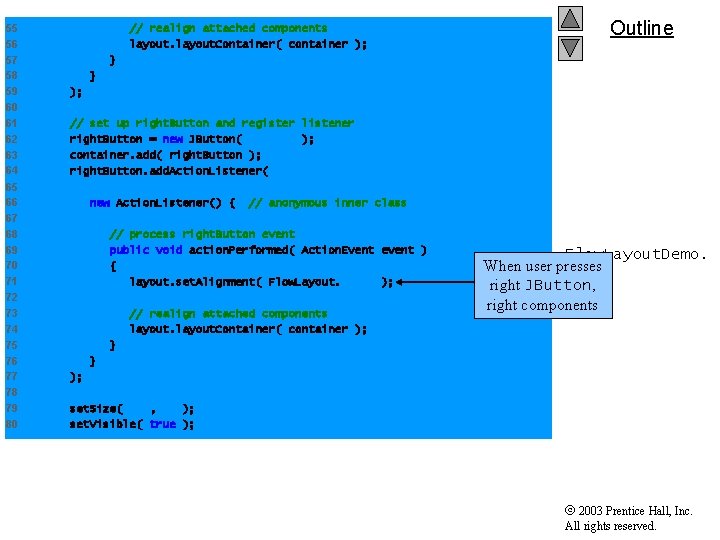
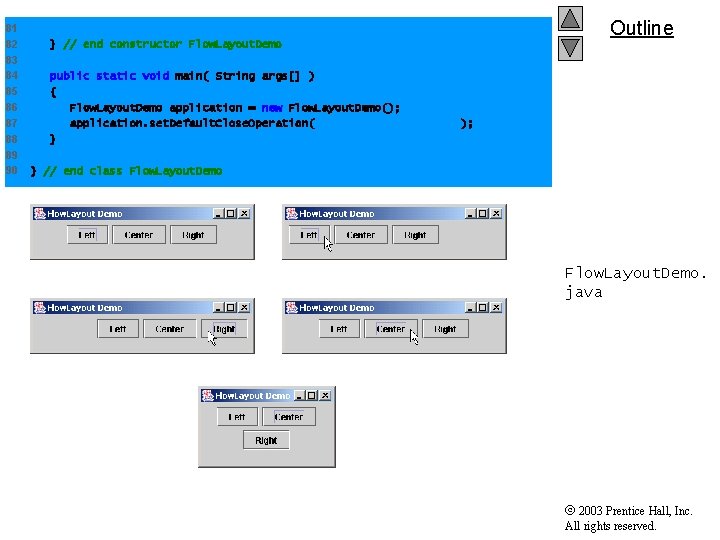
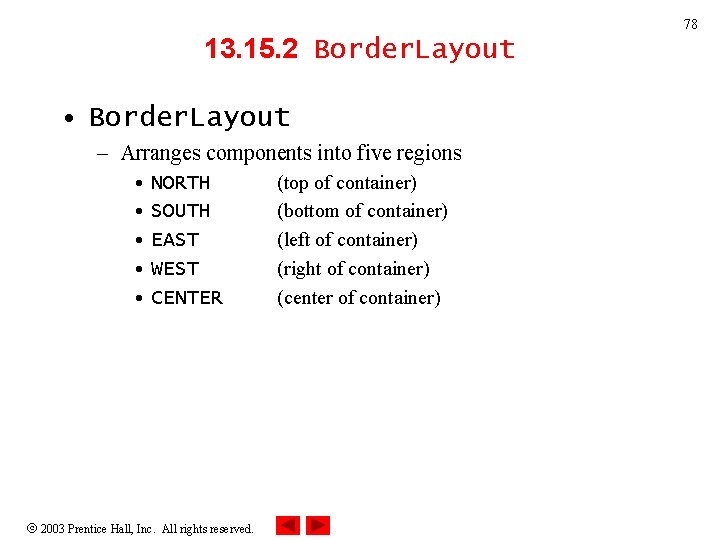
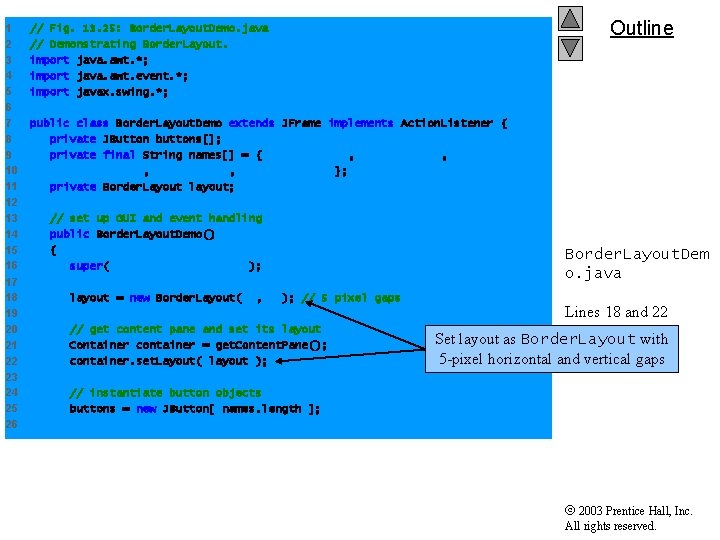
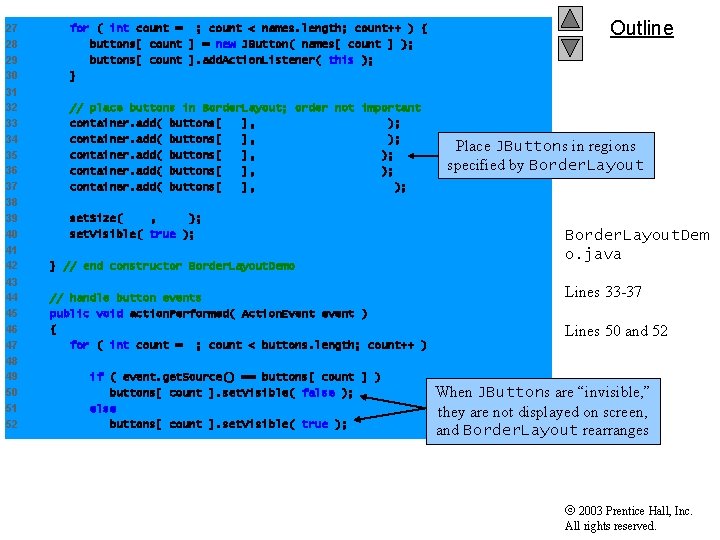
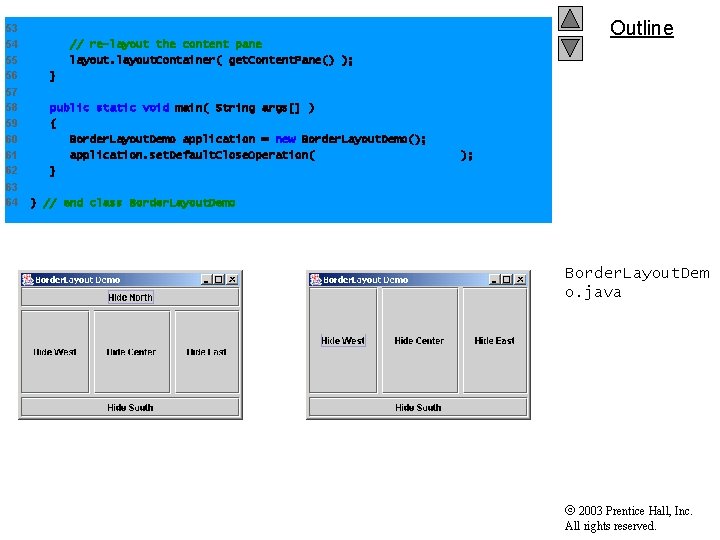
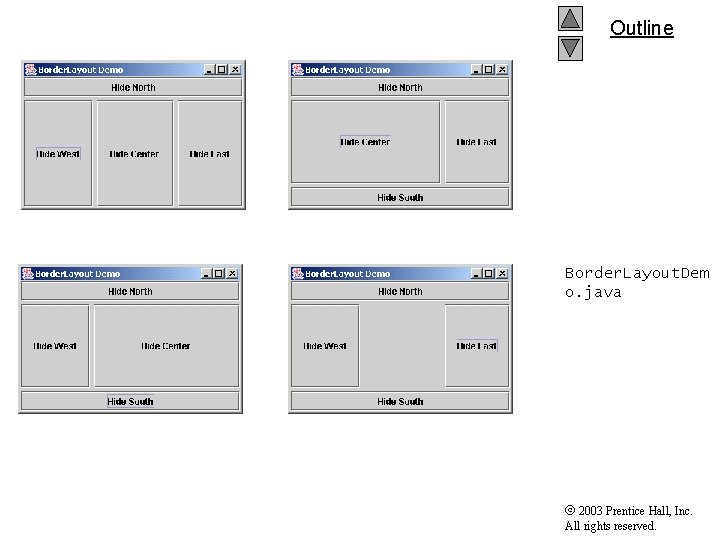
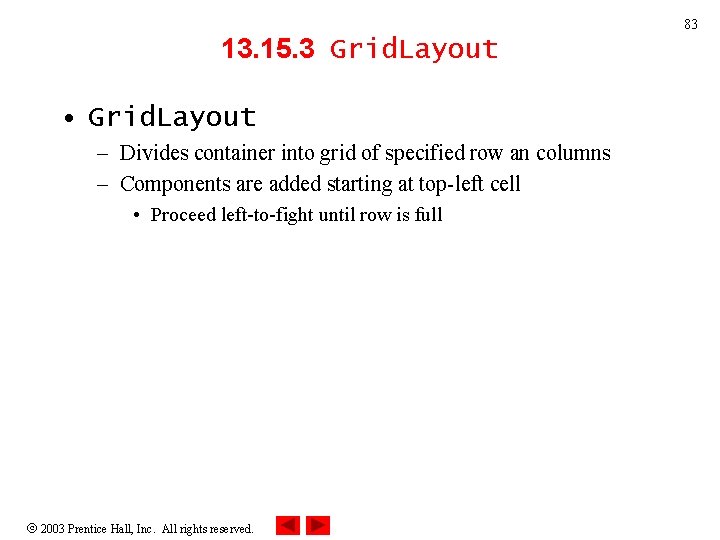
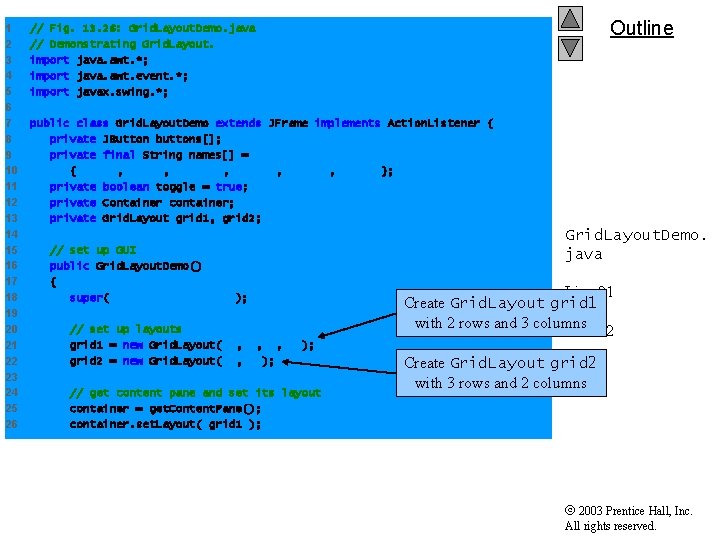
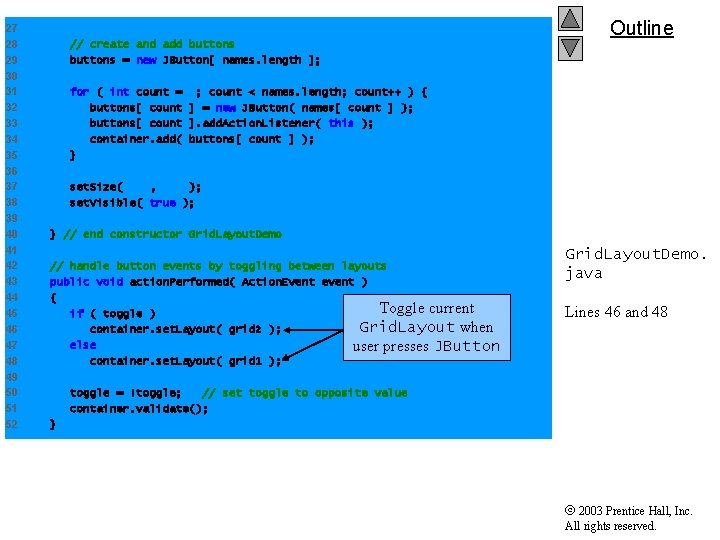
![53 54 55 56 57 58 59 60 public static void main( String args[] 53 54 55 56 57 58 59 60 public static void main( String args[]](https://slidetodoc.com/presentation_image_h2/a75e4a64e3dd0a25bd045296b3dce9f9/image-86.jpg)
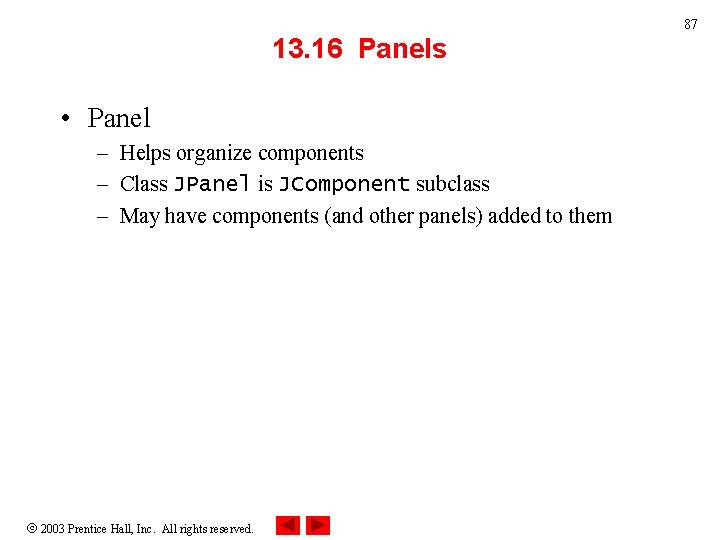
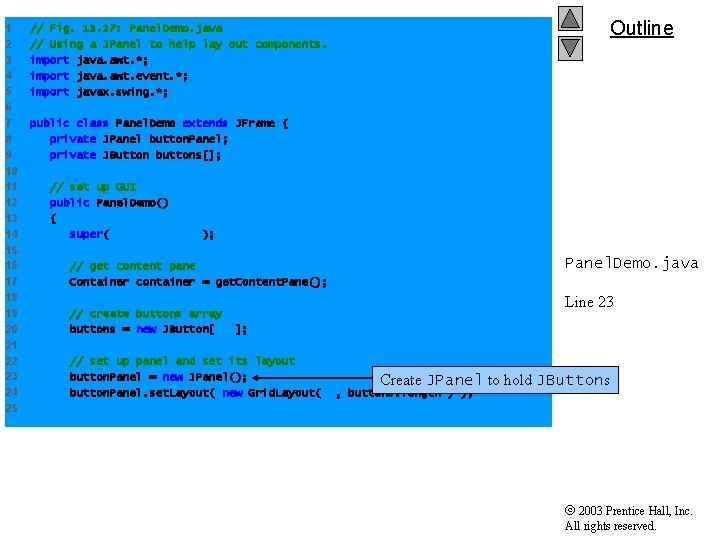
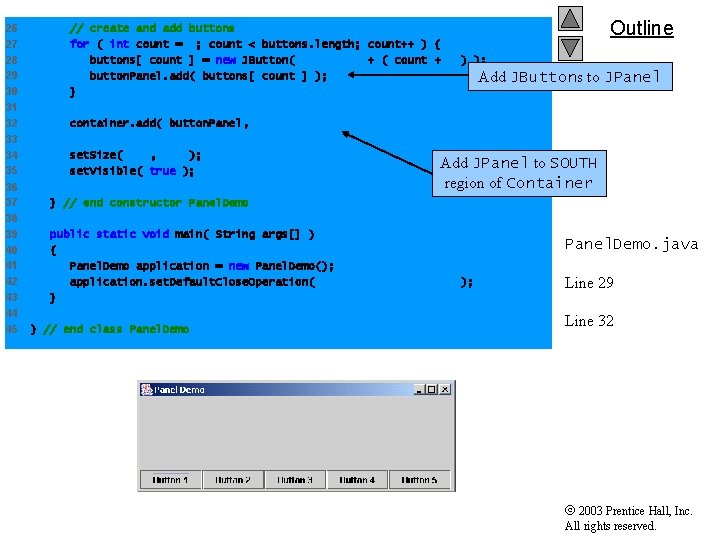
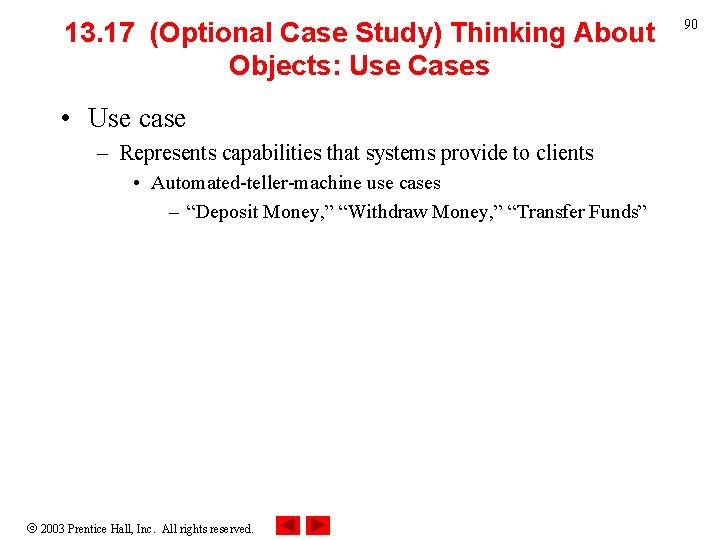
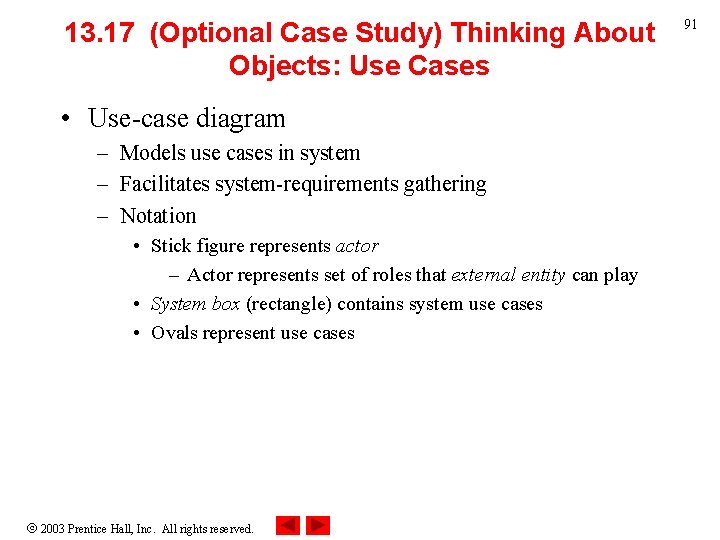
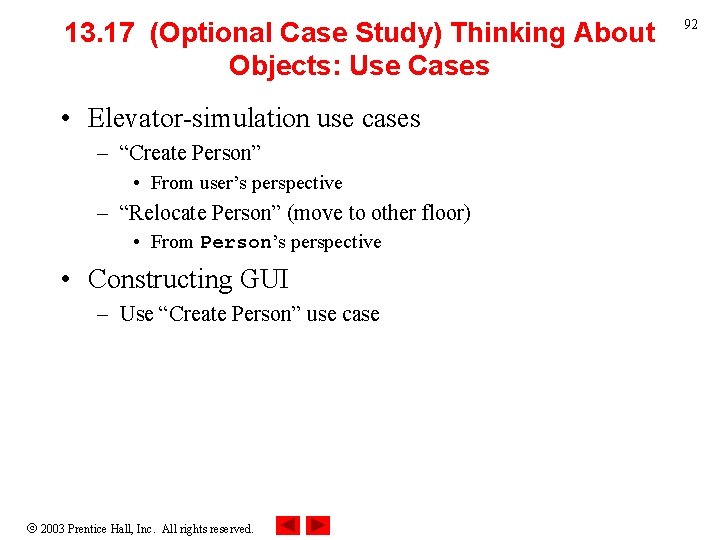
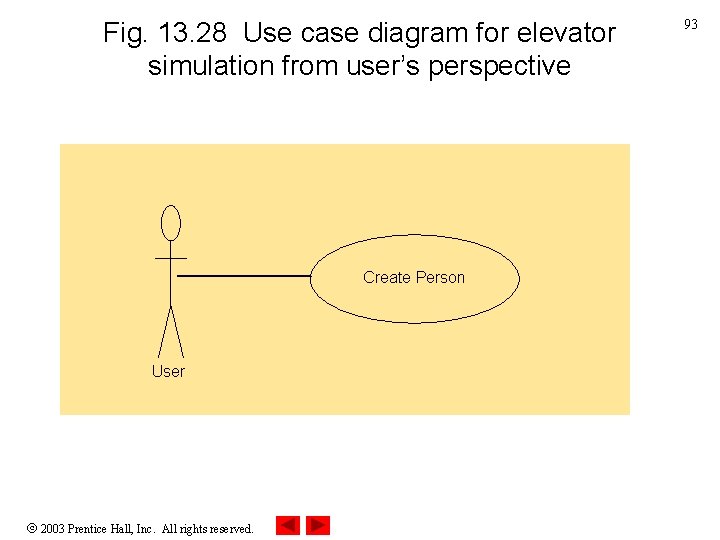
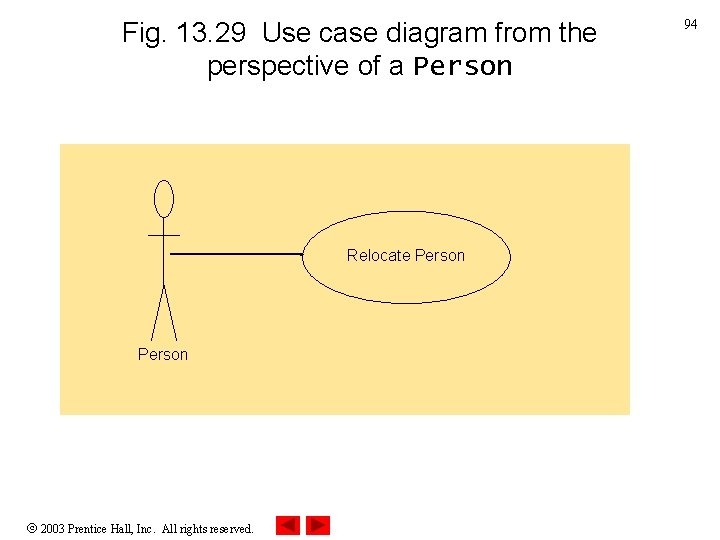
- Slides: 94
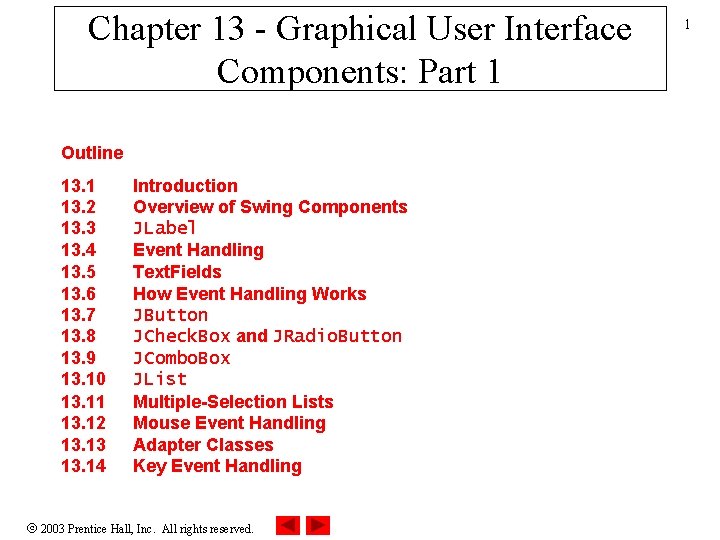
Chapter 13 - Graphical User Interface Components: Part 1 Outline 13. 1 13. 2 13. 3 13. 4 13. 5 13. 6 13. 7 13. 8 13. 9 13. 10 13. 11 13. 12 13. 13 13. 14 Introduction Overview of Swing Components JLabel Event Handling Text. Fields How Event Handling Works JButton JCheck. Box and JRadio. Button JCombo. Box JList Multiple-Selection Lists Mouse Event Handling Adapter Classes Key Event Handling 2003 Prentice Hall, Inc. All rights reserved. 1
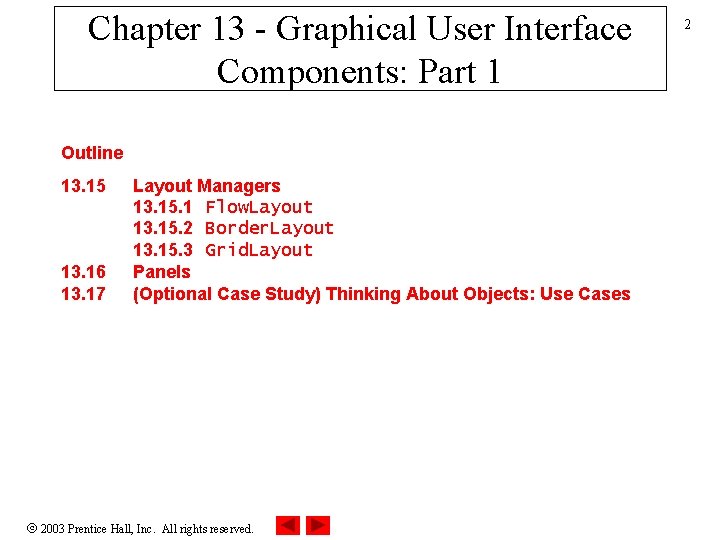
Chapter 13 - Graphical User Interface Components: Part 1 Outline 13. 15 13. 16 13. 17 Layout Managers 13. 15. 1 Flow. Layout 13. 15. 2 Border. Layout 13. 15. 3 Grid. Layout Panels (Optional Case Study) Thinking About Objects: Use Cases 2003 Prentice Hall, Inc. All rights reserved. 2
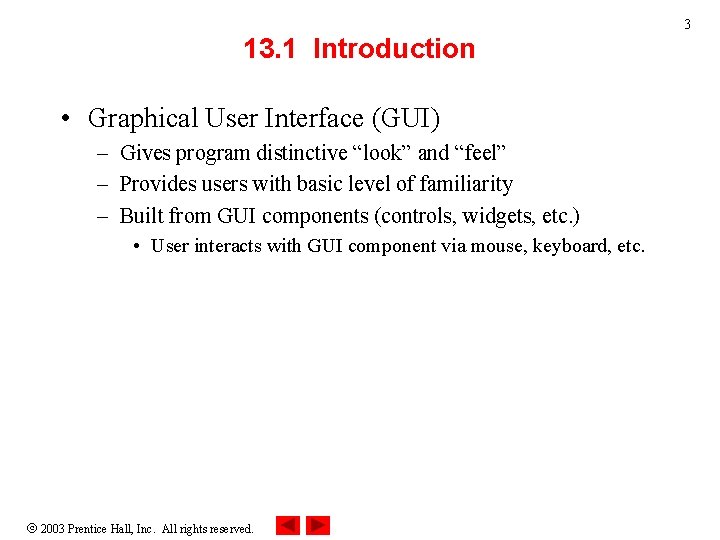
3 13. 1 Introduction • Graphical User Interface (GUI) – Gives program distinctive “look” and “feel” – Provides users with basic level of familiarity – Built from GUI components (controls, widgets, etc. ) • User interacts with GUI component via mouse, keyboard, etc. 2003 Prentice Hall, Inc. All rights reserved.
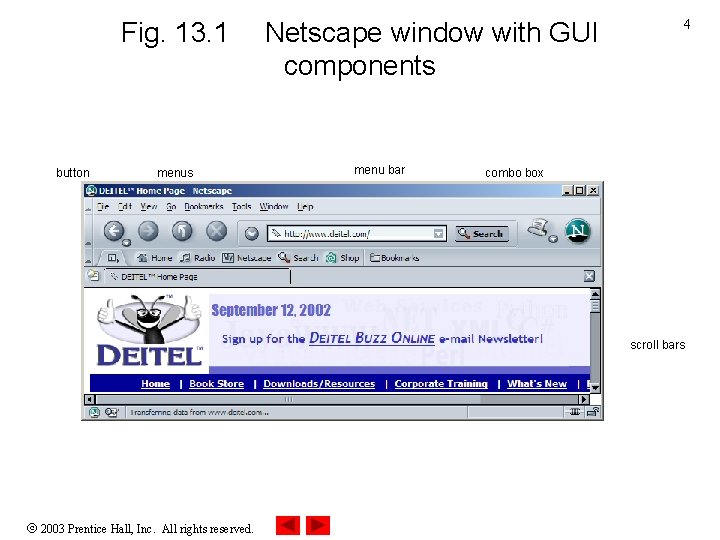
Fig. 13. 1 button menus Netscape window with GUI components menu bar 4 combo box scroll bars 2003 Prentice Hall, Inc. All rights reserved.
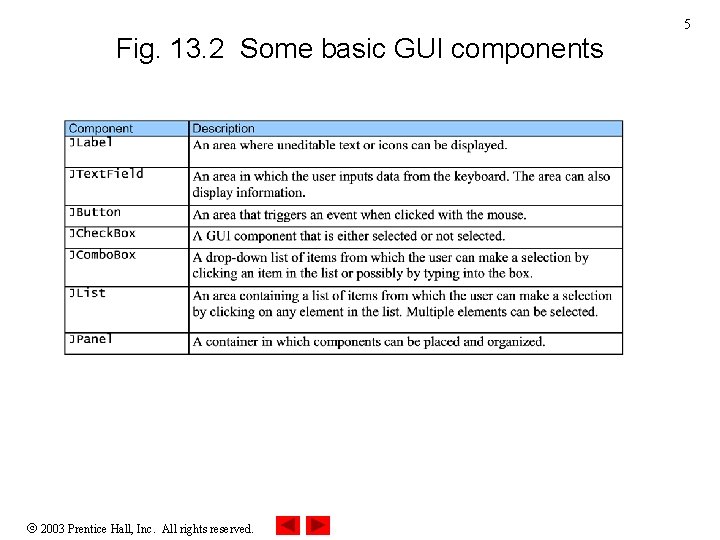
5 Fig. 13. 2 Some basic GUI components 2003 Prentice Hall, Inc. All rights reserved.
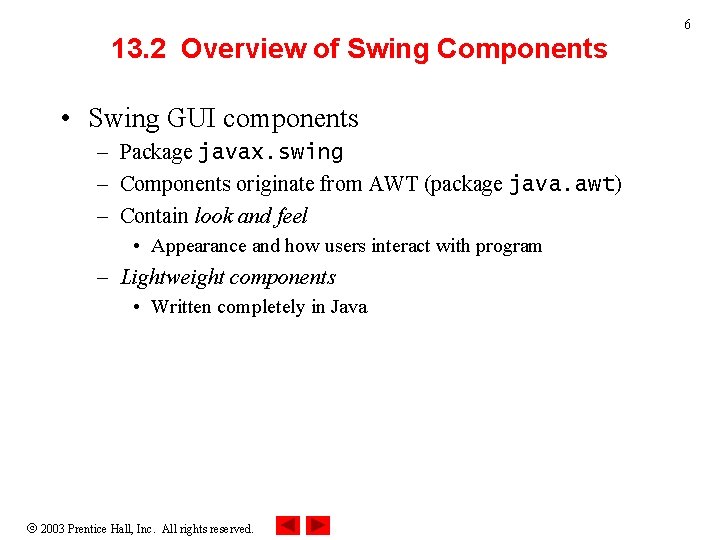
6 13. 2 Overview of Swing Components • Swing GUI components – Package javax. swing – Components originate from AWT (package java. awt) – Contain look and feel • Appearance and how users interact with program – Lightweight components • Written completely in Java 2003 Prentice Hall, Inc. All rights reserved.
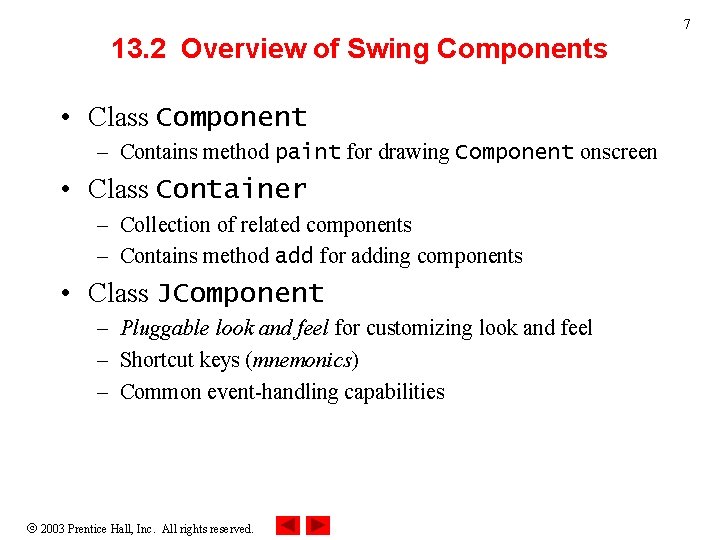
7 13. 2 Overview of Swing Components • Class Component – Contains method paint for drawing Component onscreen • Class Container – Collection of related components – Contains method add for adding components • Class JComponent – Pluggable look and feel for customizing look and feel – Shortcut keys (mnemonics) – Common event-handling capabilities 2003 Prentice Hall, Inc. All rights reserved.
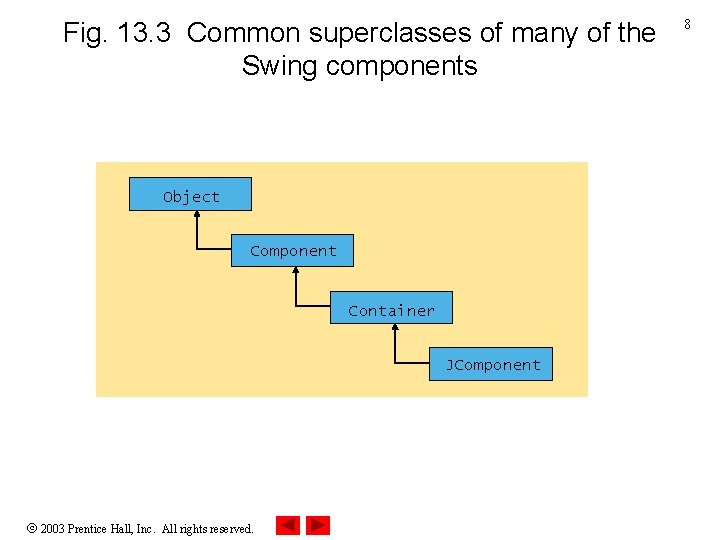
Fig. 13. 3 Common superclasses of many of the Swing components Object Component Container JComponent 2003 Prentice Hall, Inc. All rights reserved. 8
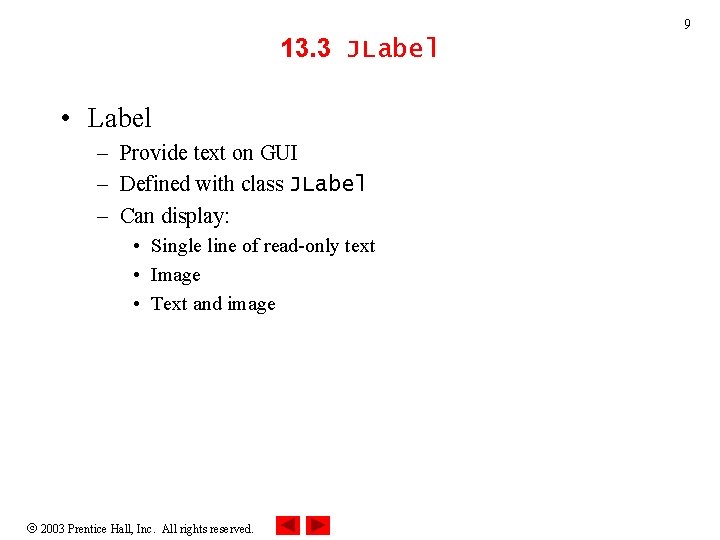
9 13. 3 JLabel • Label – Provide text on GUI – Defined with class JLabel – Can display: • Single line of read-only text • Image • Text and image 2003 Prentice Hall, Inc. All rights reserved.
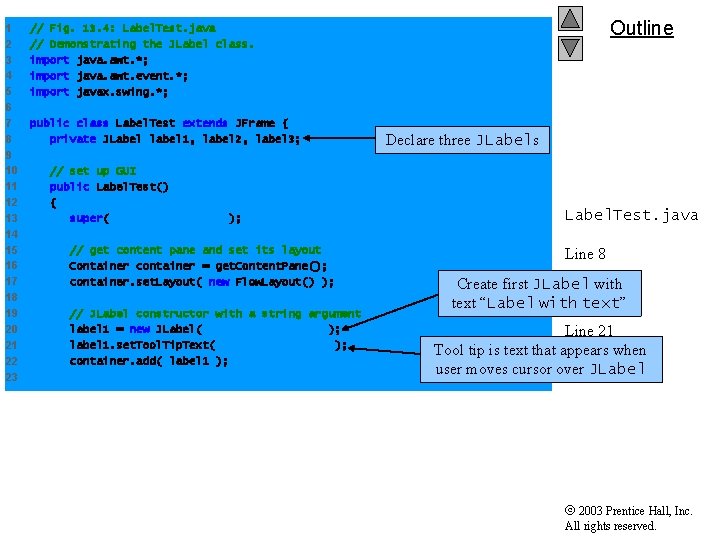
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 Outline // Fig. 13. 4: Label. Test. java // Demonstrating the JLabel class. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Label. Test extends JFrame { private JLabel label 1, label 2, label 3; // set up GUI public Label. Test() { super( "Testing JLabel" ); // get content pane and set its layout Container container = get. Content. Pane(); container. set. Layout( new Flow. Layout() ); // JLabel constructor with a string argument label 1 = new JLabel( "Label with text" ); label 1. set. Tool. Tip. Text( "This is label 1" ); container. add( label 1 ); Declare three JLabels Label. Test. java Line 8 Create first JLabel with Line 20 text “Label with text” Line 21 Tool tip is text that appears when user moves cursor over JLabel 2003 Prentice Hall, Inc. All rights reserved.
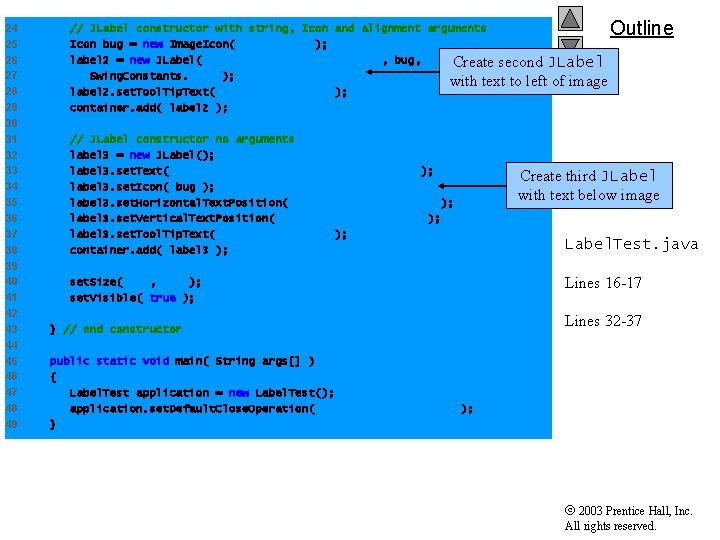
24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 // JLabel constructor with string, Icon and alignment arguments Icon bug = new Image. Icon( "bug 1. gif" ); label 2 = new JLabel( "Label with text and icon", bug, Create second JLabel Swing. Constants. LEFT ); with text to left of image label 2. set. Tool. Tip. Text( "This is label 2" ); container. add( label 2 ); // JLabel constructor no arguments label 3 = new JLabel(); label 3. set. Text( "Label with icon and text at bottom" ); label 3. set. Icon( bug ); label 3. set. Horizontal. Text. Position( Swing. Constants. CENTER ); label 3. set. Vertical. Text. Position( Swing. Constants. BOTTOM ); label 3. set. Tool. Tip. Text( "This is label 3" ); container. add( label 3 ); set. Size( 275, 170 ); set. Visible( true ); } // end constructor Outline Create third JLabel with text below image Label. Test. java Lines 16 -17 Lines 32 -37 public static void main( String args[] ) { Label. Test application = new Label. Test(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } 2003 Prentice Hall, Inc. All rights reserved.
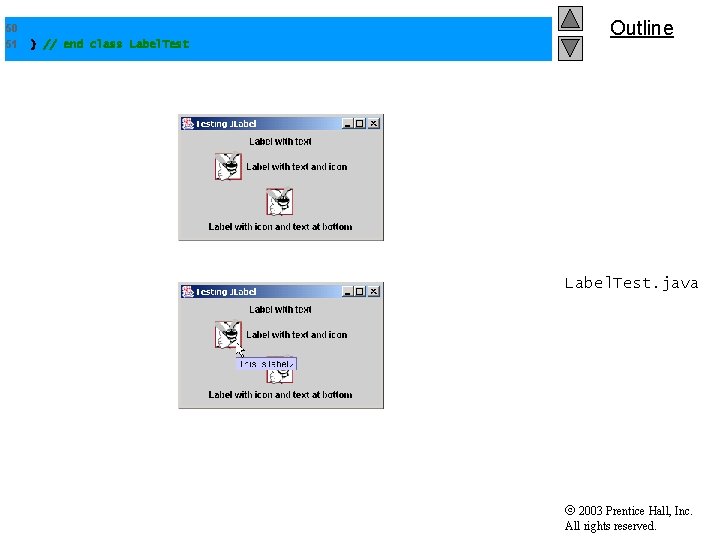
50 51 } // end class Label. Test Outline Label. Test. java 2003 Prentice Hall, Inc. All rights reserved.
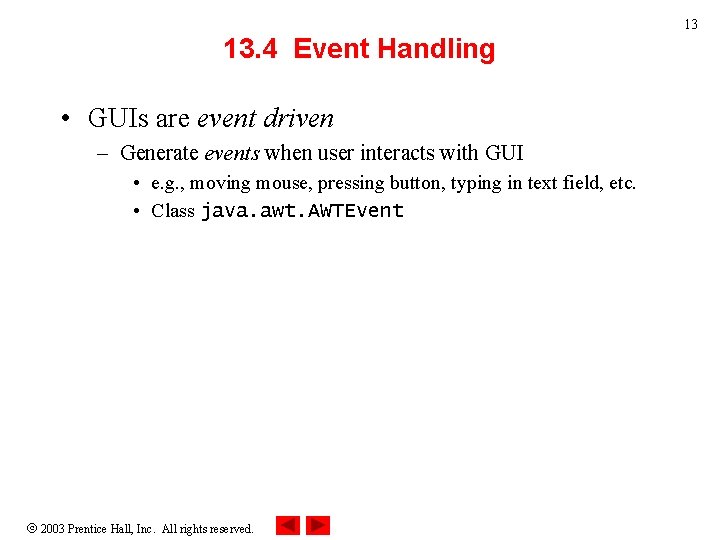
13 13. 4 Event Handling • GUIs are event driven – Generate events when user interacts with GUI • e. g. , moving mouse, pressing button, typing in text field, etc. • Class java. awt. AWTEvent 2003 Prentice Hall, Inc. All rights reserved.
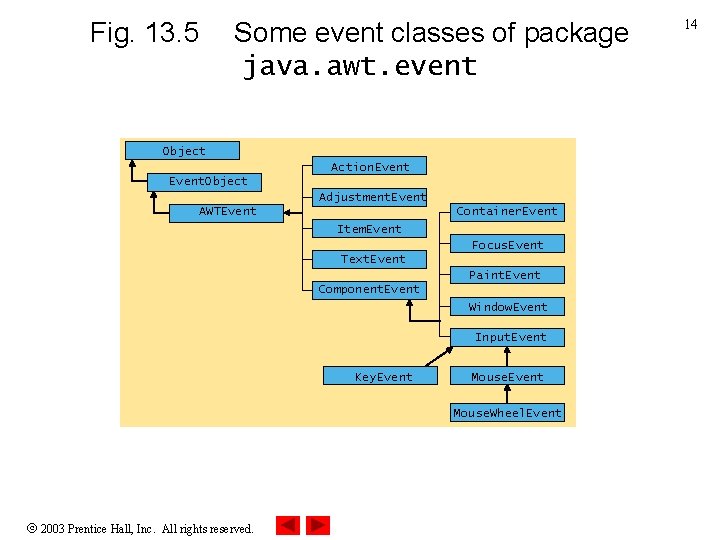
Fig. 13. 5 Some event classes of package java. awt. event Object Action. Event. Object Adjustment. Event AWTEvent Container. Event Item. Event Focus. Event Text. Event Paint. Event Component. Event Window. Event Input. Event Key. Event Mouse. Wheel. Event 2003 Prentice Hall, Inc. All rights reserved. 14
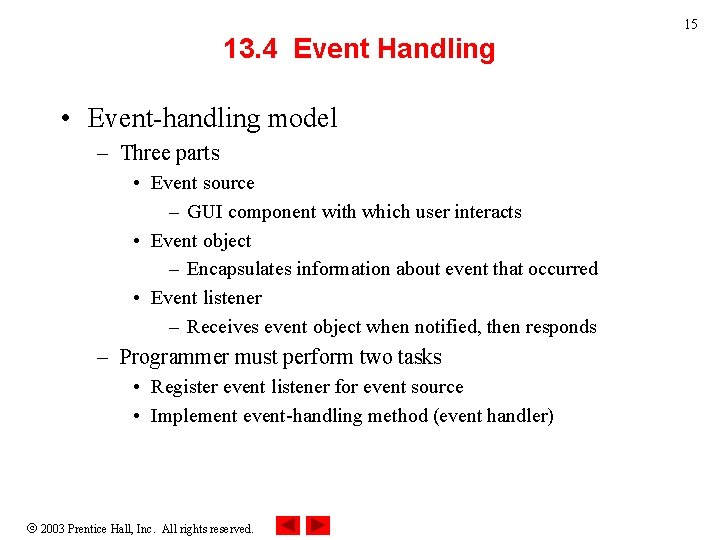
15 13. 4 Event Handling • Event-handling model – Three parts • Event source – GUI component with which user interacts • Event object – Encapsulates information about event that occurred • Event listener – Receives event object when notified, then responds – Programmer must perform two tasks • Register event listener for event source • Implement event-handling method (event handler) 2003 Prentice Hall, Inc. All rights reserved.
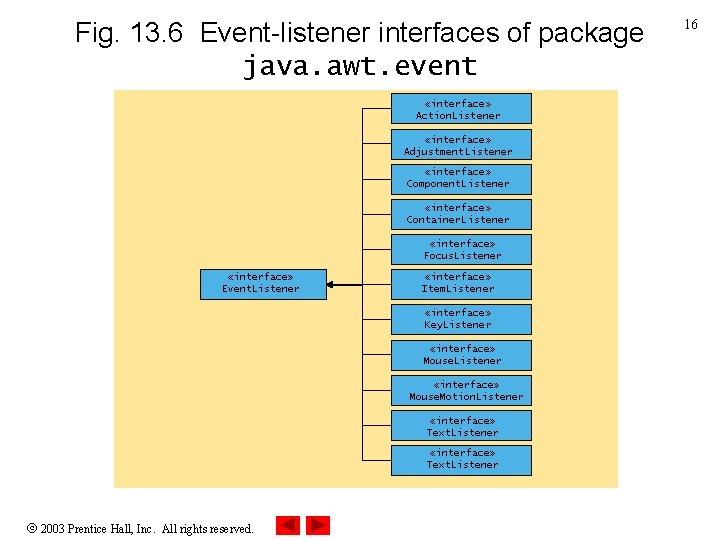
Fig. 13. 6 Event-listener interfaces of package java. awt. event interface «interface» Action. Listener interface «interface» Adjustment. Listener interface «interface» Component. Listener interface «interface» Container. Listener interface «interface» Focus. Listener interface «interface» Event. Listener «interface» interface Item. Listener interface «interface» Key. Listener interface «interface» Mouse. Motion. Listener interface «interface» Text. Listener interface «interface» Window. Listener Text. Listener 2003 Prentice Hall, Inc. All rights reserved. 16
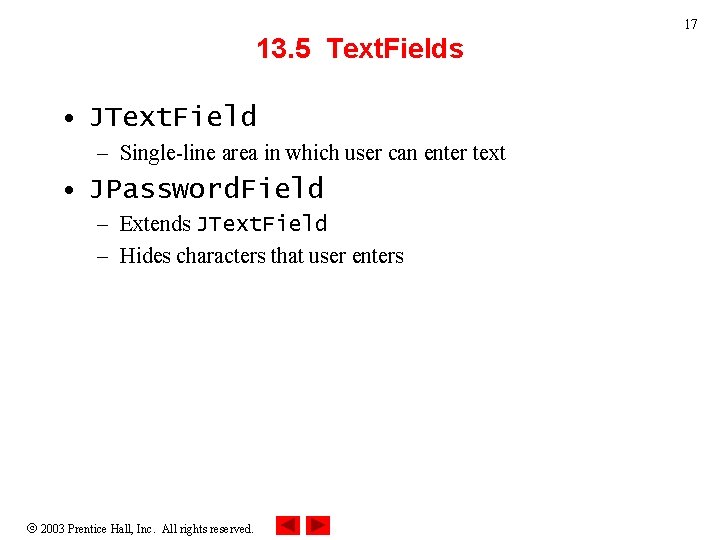
17 13. 5 Text. Fields • JText. Field – Single-line area in which user can enter text • JPassword. Field – Extends JText. Field – Hides characters that user enters 2003 Prentice Hall, Inc. All rights reserved.
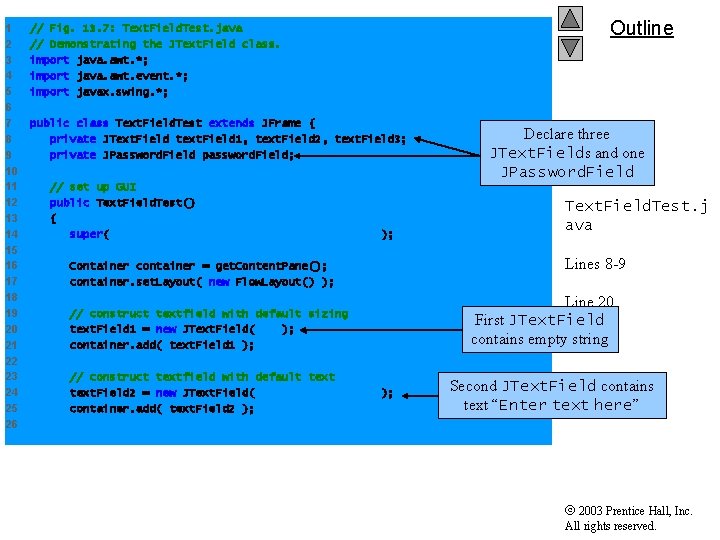
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 // Fig. 13. 7: Text. Field. Test. java // Demonstrating the JText. Field class. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Text. Field. Test extends JFrame { private JText. Field text. Field 1, text. Field 2, text. Field 3; private JPassword. Field password. Field; // set up GUI public Text. Field. Test() { super( "Testing JText. Field and JPassword. Field" ); Container container = get. Content. Pane(); container. set. Layout( new Flow. Layout() ); // construct textfield with default sizing text. Field 1 = new JText. Field( 10 ); container. add( text. Field 1 ); // construct textfield with default text. Field 2 = new JText. Field( "Enter text here" ); container. add( text. Field 2 ); Outline Declare three JText. Fields and one JPassword. Field Text. Field. Test. j ava Lines 8 -9 Line 20 First JText. Field contains empty. Line string 24 Second JText. Field contains text “Enter text here” 2003 Prentice Hall, Inc. All rights reserved.
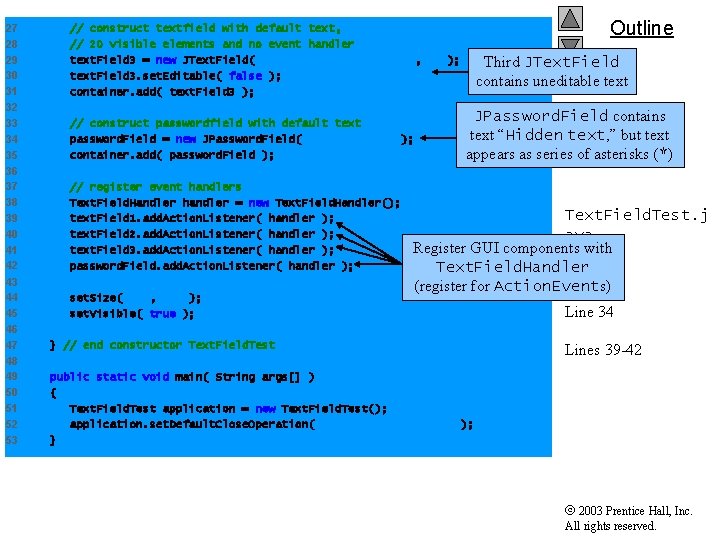
27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 Outline // construct textfield with default text, // 20 visible elements and no event handler text. Field 3 = new JText. Field( "Uneditable text field", 20 ); text. Field 3. set. Editable( false ); container. add( text. Field 3 ); // construct passwordfield with default text password. Field = new JPassword. Field( "Hidden text" ); container. add( password. Field ); // register event handlers Text. Field. Handler handler = new Text. Field. Handler(); text. Field 1. add. Action. Listener( handler ); text. Field 2. add. Action. Listener( handler ); text. Field 3. add. Action. Listener( handler ); password. Field. add. Action. Listener( handler ); set. Size( 325, 100 ); set. Visible( true ); Third JText. Field contains uneditable text JPassword. Field contains text “Hidden text, ” but text appears as series of asterisks (*) Text. Field. Test. j ava Register GUI components with Text. Field. Handler Line 30 (register for Action. Events) } // end constructor Text. Field. Test Line 34 Lines 39 -42 public static void main( String args[] ) { Text. Field. Test application = new Text. Field. Test(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } 2003 Prentice Hall, Inc. All rights reserved.
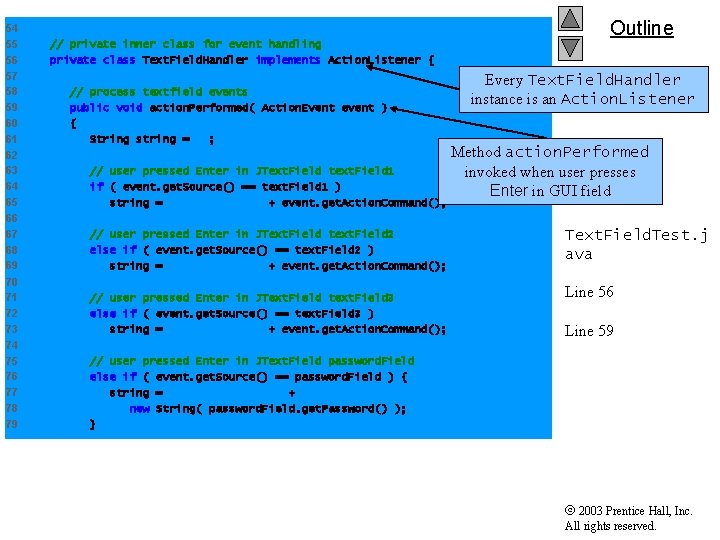
54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 // private inner class for event handling private class Text. Field. Handler implements Action. Listener { // process textfield events public void action. Performed( Action. Event event ) { String string = ""; // user pressed Enter in JText. Field text. Field 1 if ( event. get. Source() == text. Field 1 ) string = "text. Field 1: " + event. get. Action. Command(); // user pressed Enter in JText. Field text. Field 2 else if ( event. get. Source() == text. Field 2 ) string = "text. Field 2: " + event. get. Action. Command(); // user pressed Enter in JText. Field text. Field 3 else if ( event. get. Source() == text. Field 3 ) string = "text. Field 3: " + event. get. Action. Command(); Outline Every Text. Field. Handler instance is an Action. Listener Method action. Performed invoked when user presses Enter in GUI field Text. Field. Test. j ava Line 56 Line 59 // user pressed Enter in JText. Field password. Field else if ( event. get. Source() == password. Field ) { string = "password. Field: " + new String( password. Field. get. Password() ); } 2003 Prentice Hall, Inc. All rights reserved.
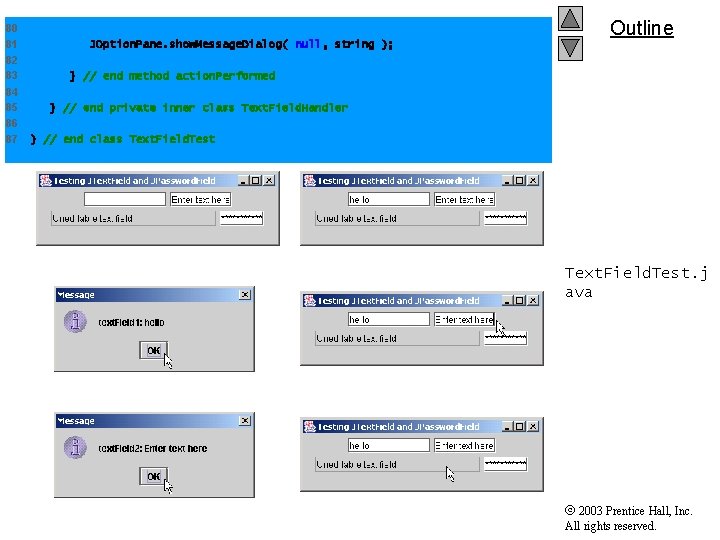
80 81 82 83 84 85 86 87 JOption. Pane. show. Message. Dialog( null, string ); Outline } // end method action. Performed } // end private inner class Text. Field. Handler } // end class Text. Field. Test. j ava 2003 Prentice Hall, Inc. All rights reserved.
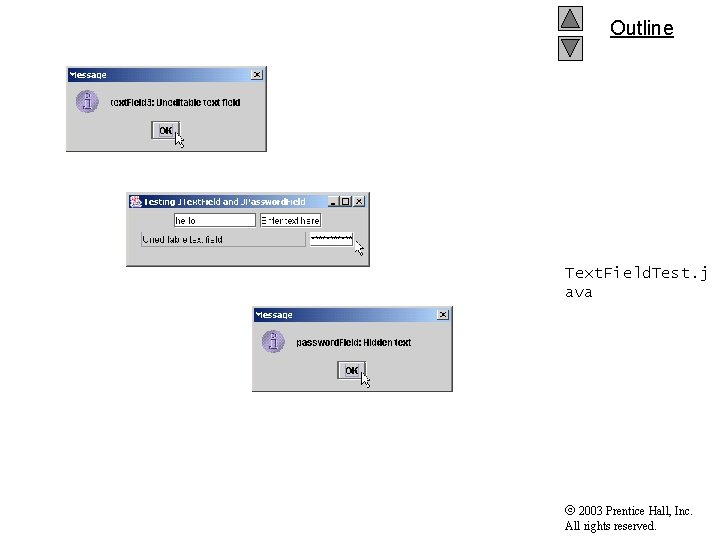
Outline Text. Field. Test. j ava 2003 Prentice Hall, Inc. All rights reserved.
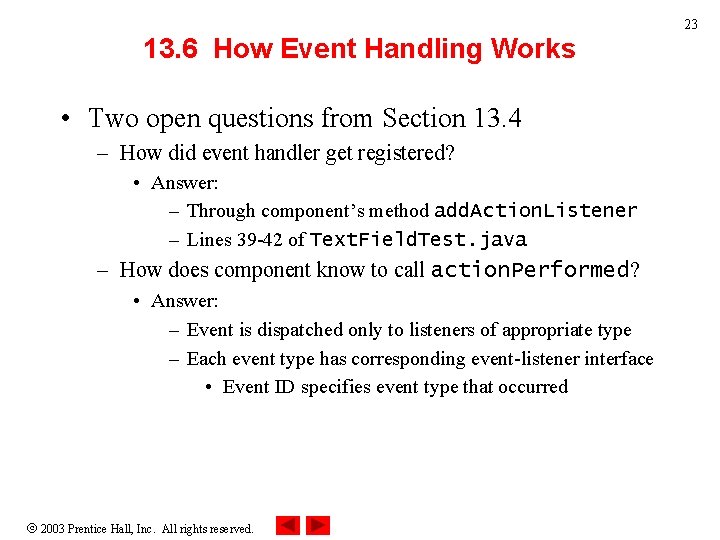
23 13. 6 How Event Handling Works • Two open questions from Section 13. 4 – How did event handler get registered? • Answer: – Through component’s method add. Action. Listener – Lines 39 -42 of Text. Field. Test. java – How does component know to call action. Performed? • Answer: – Event is dispatched only to listeners of appropriate type – Each event type has corresponding event-listener interface • Event ID specifies event type that occurred 2003 Prentice Hall, Inc. All rights reserved.
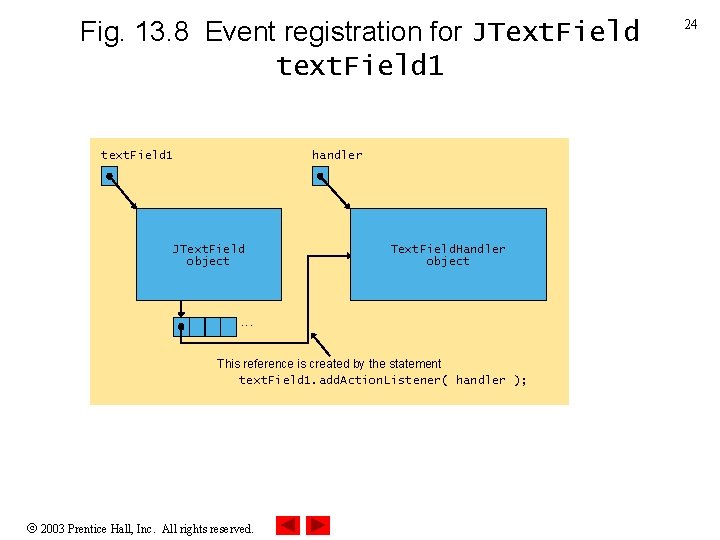
Fig. 13. 8 Event registration for JText. Field text. Field 1 handler listener. List JText. Field object public void action. Performed( Action. Event event ) { // event handled here } Text. Field. Handler object . . . This reference is created by the statement text. Field 1. add. Action. Listener( handler ); 2003 Prentice Hall, Inc. All rights reserved. 24
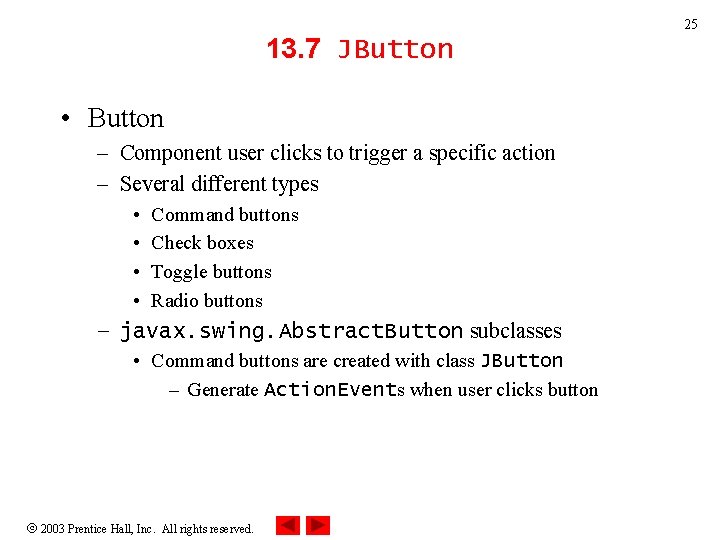
25 13. 7 JButton • Button – Component user clicks to trigger a specific action – Several different types • • Command buttons Check boxes Toggle buttons Radio buttons – javax. swing. Abstract. Button subclasses • Command buttons are created with class JButton – Generate Action. Events when user clicks button 2003 Prentice Hall, Inc. All rights reserved.
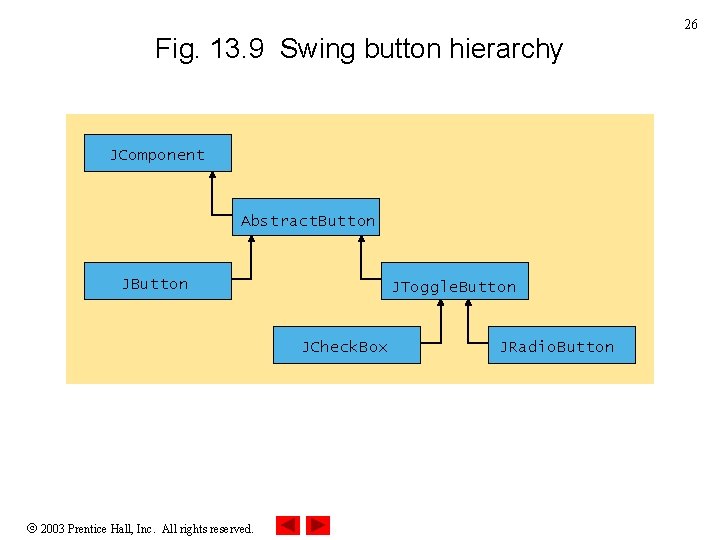
26 Fig. 13. 9 Swing button hierarchy JComponent Abstract. Button JToggle. Button JButton JToggle. Button JCheck. Box 2003 Prentice Hall, Inc. All rights reserved. JRadio. Button
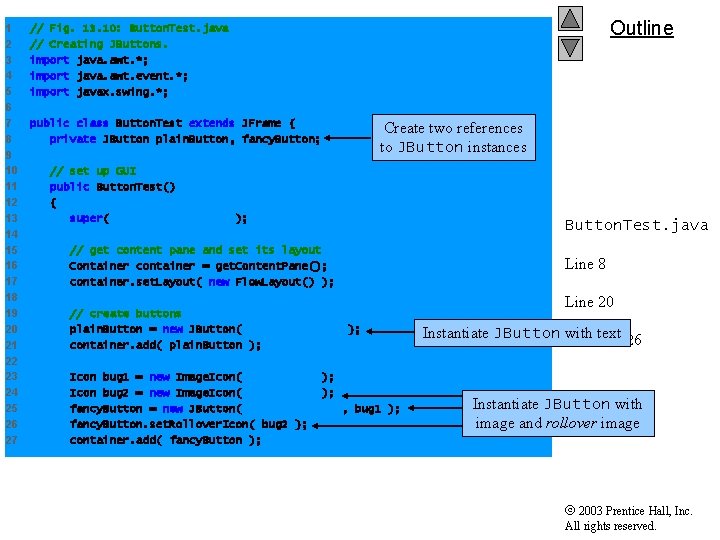
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 Outline // Fig. 13. 10: Button. Test. java // Creating JButtons. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Button. Test extends JFrame { private JButton plain. Button, fancy. Button; Create two references to JButton instances // set up GUI public Button. Test() { super( "Testing Buttons" ); // get content pane and set its layout Container container = get. Content. Pane(); container. set. Layout( new Flow. Layout() ); // create buttons plain. Button = new JButton( "Plain Button" ); container. add( plain. Button ); Icon bug 1 = new Image. Icon( "bug 1. gif" ); Icon bug 2 = new Image. Icon( "bug 2. gif" ); fancy. Button = new JButton( "Fancy Button", bug 1 ); fancy. Button. set. Rollover. Icon( bug 2 ); container. add( fancy. Button ); Button. Test. java Line 8 Line 20 Instantiate JButton with Linestext 24 -26 Instantiate JButton with image and rollover image 2003 Prentice Hall, Inc. All rights reserved.
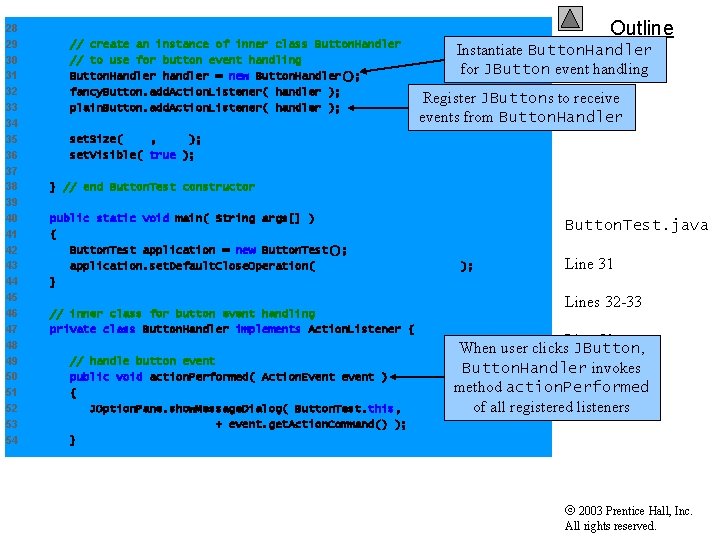
28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 // create an instance of inner class Button. Handler // to use for button event handling Button. Handler handler = new Button. Handler(); fancy. Button. add. Action. Listener( handler ); plain. Button. add. Action. Listener( handler ); Outline Instantiate Button. Handler for JButton event handling Register JButtons to receive events from Button. Handler set. Size( 275, 100 ); set. Visible( true ); } // end Button. Test constructor public static void main( String args[] ) { Button. Test application = new Button. Test(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } // inner class for button event handling private class Button. Handler implements Action. Listener { // handle button event public void action. Performed( Action. Event event ) { JOption. Pane. show. Message. Dialog( Button. Test. this, "You pressed: " + event. get. Action. Command() ); } Button. Test. java Line 31 Lines 32 -33 50 When user clicks. Line JButton, Button. Handler invokes method action. Performed of all registered listeners 2003 Prentice Hall, Inc. All rights reserved.
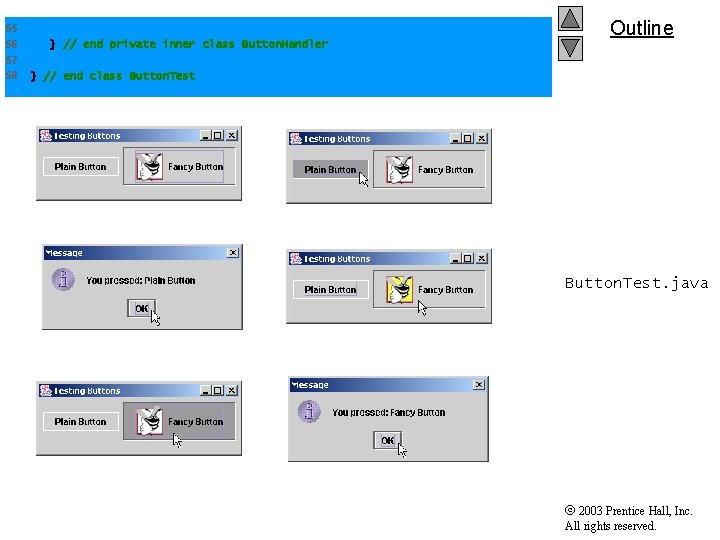
55 56 57 58 } // end private inner class Button. Handler Outline } // end class Button. Test. java 2003 Prentice Hall, Inc. All rights reserved.
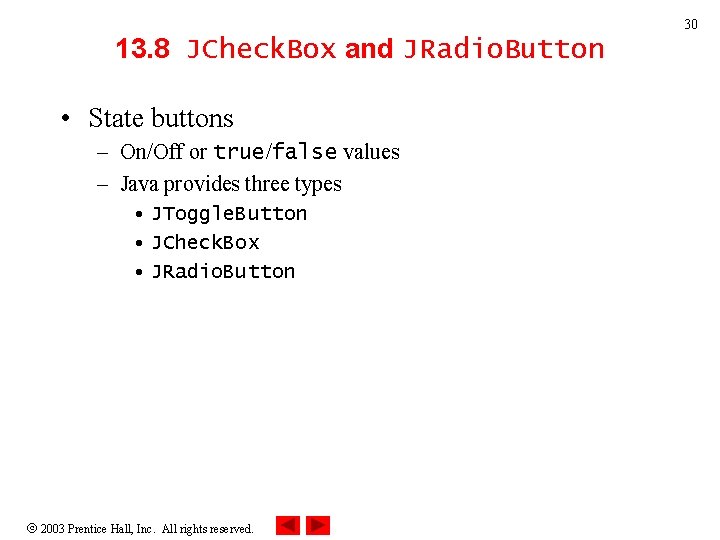
30 13. 8 JCheck. Box and JRadio. Button • State buttons – On/Off or true/false values – Java provides three types • JToggle. Button • JCheck. Box • JRadio. Button 2003 Prentice Hall, Inc. All rights reserved.
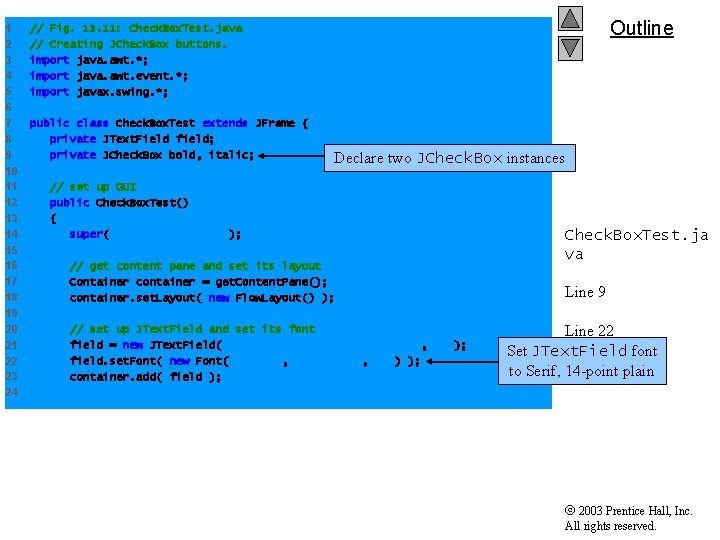
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 Outline // Fig. 13. 11: Check. Box. Test. java // Creating JCheck. Box buttons. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Check. Box. Test extends JFrame { private JText. Field field; private JCheck. Box bold, italic; Declare two JCheck. Box instances // set up GUI public Check. Box. Test() { super( "JCheck. Box Test" ); // get content pane and set its layout Container container = get. Content. Pane(); container. set. Layout( new Flow. Layout() ); // set up JText. Field and set its font field = new JText. Field( "Watch the font style change", 20 ); field. set. Font( new Font( "Serif", Font. PLAIN, 14 ) ); container. add( field ); Check. Box. Test. ja va Line 9 Line 22 Set JText. Field font to Serif, 14 -point plain 2003 Prentice Hall, Inc. All rights reserved.
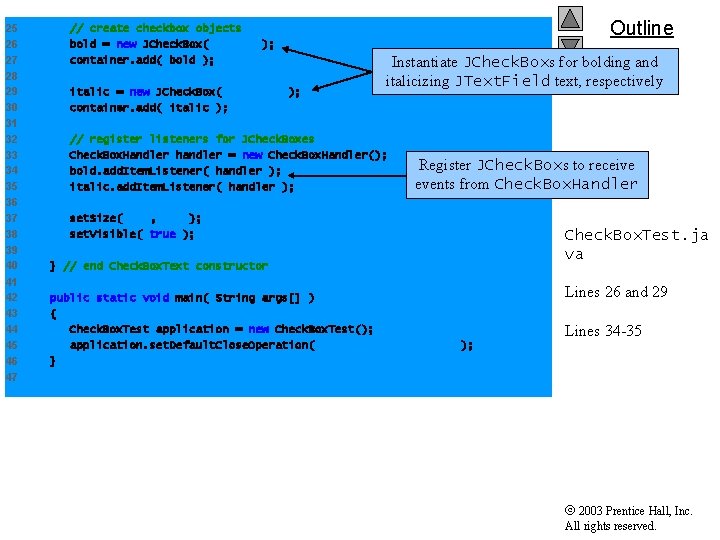
25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 // create checkbox objects bold = new JCheck. Box( "Bold" ); container. add( bold ); italic = new JCheck. Box( "Italic" ); container. add( italic ); Outline Instantiate JCheck. Boxs for bolding and italicizing JText. Field text, respectively // register listeners for JCheck. Boxes Check. Box. Handler handler = new Check. Box. Handler(); bold. add. Item. Listener( handler ); italic. add. Item. Listener( handler ); Register JCheck. Boxs to receive events from Check. Box. Handler set. Size( 275, 100 ); set. Visible( true ); } // end Check. Box. Text constructor public static void main( String args[] ) { Check. Box. Test application = new Check. Box. Test(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } Check. Box. Test. ja va Lines 26 and 29 Lines 34 -35 2003 Prentice Hall, Inc. All rights reserved.
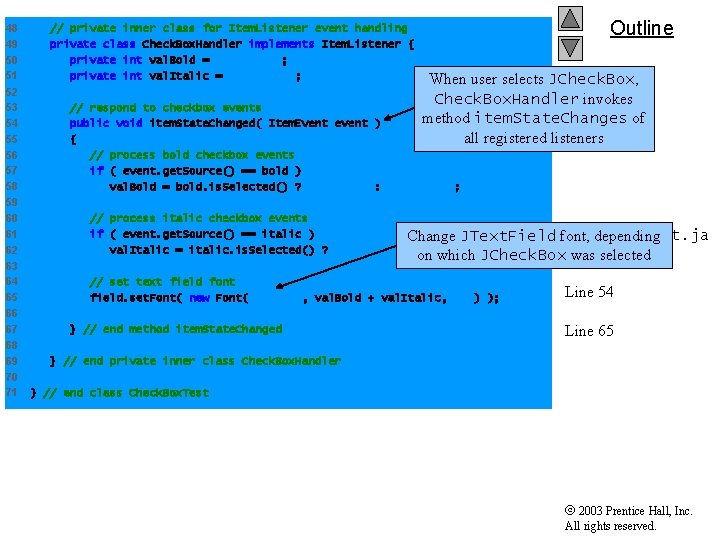
48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 // private inner class for Item. Listener event handling private class Check. Box. Handler implements Item. Listener { private int val. Bold = Font. PLAIN; private int val. Italic = Font. PLAIN; Outline When user selects JCheck. Box, Check. Box. Handler invokes method item. State. Changes of all registered listeners // respond to checkbox events public void item. State. Changed( Item. Event event ) { // process bold checkbox events if ( event. get. Source() == bold ) val. Bold = bold. is. Selected() ? Font. BOLD : Font. PLAIN; // process italic checkbox events if ( event. get. Source() == italic ) Change JText. Field val. Italic = italic. is. Selected() ? Font. ITALIC : Font. PLAIN; Check. Box. Test. ja font, depending on which JCheck. Boxva was selected // set text field font field. set. Font( new Font( "Serif", val. Bold + val. Italic, 14 ) ); } // end method item. State. Changed Line 54 Line 65 } // end private inner class Check. Box. Handler } // end class Check. Box. Test 2003 Prentice Hall, Inc. All rights reserved.
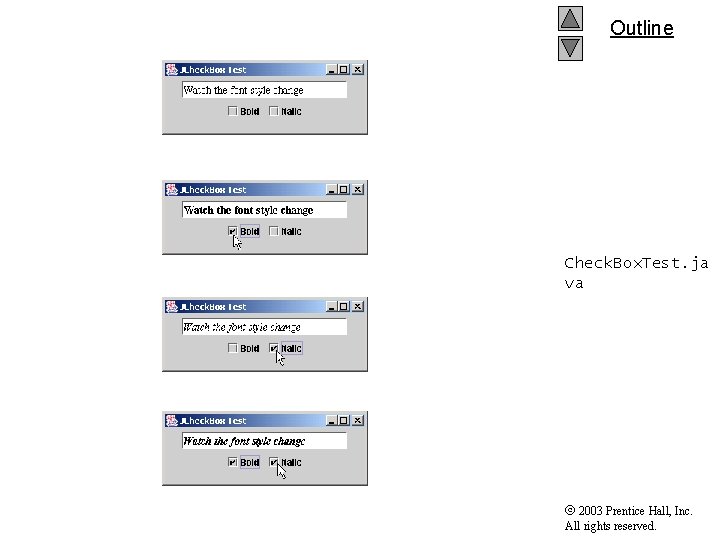
Outline Check. Box. Test. ja va 2003 Prentice Hall, Inc. All rights reserved.
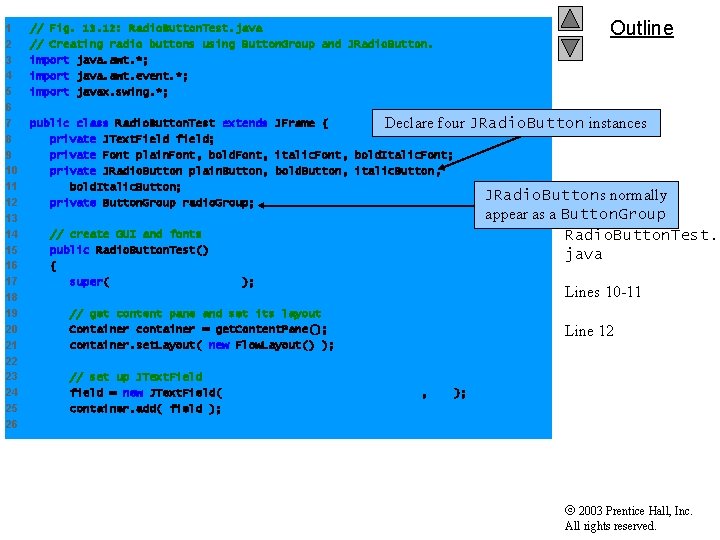
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 // Fig. 13. 12: Radio. Button. Test. java // Creating radio buttons using Button. Group and JRadio. Button. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Radio. Button. Test extends JFrame { Declare four private JText. Field field; private Font plain. Font, bold. Font, italic. Font, bold. Italic. Font; private JRadio. Button plain. Button, bold. Button, italic. Button, bold. Italic. Button; private Button. Group radio. Group; // create GUI and fonts public Radio. Button. Test() { super( "Radio. Button Test" ); // get content pane and set its layout Container container = get. Content. Pane(); container. set. Layout( new Flow. Layout() ); Outline JRadio. Button instances JRadio. Buttons normally appear as a Button. Group Radio. Button. Test. java Lines 10 -11 Line 12 // set up JText. Field field = new JText. Field( "Watch the font style change", 25 ); container. add( field ); 2003 Prentice Hall, Inc. All rights reserved.
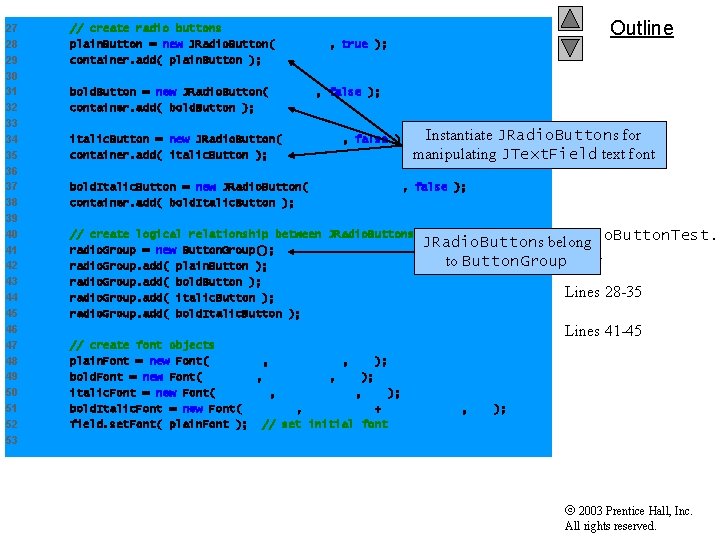
27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 Outline // create radio buttons plain. Button = new JRadio. Button( "Plain", true ); container. add( plain. Button ); bold. Button = new JRadio. Button( "Bold", false ); container. add( bold. Button ); italic. Button = new JRadio. Button( "Italic", false ); container. add( italic. Button ); Instantiate JRadio. Buttons for manipulating JText. Field text font bold. Italic. Button = new JRadio. Button( "Bold/Italic", false ); container. add( bold. Italic. Button ); // create logical relationship between JRadio. Buttons radio. Group = new Button. Group(); radio. Group. add( plain. Button ); radio. Group. add( bold. Button ); radio. Group. add( italic. Button ); radio. Group. add( bold. Italic. Button ); Radio. Button. Test. JRadio. Buttons belong to Button. Groupjava Lines 28 -35 Lines 41 -45 // create font objects plain. Font = new Font( "Serif", Font. PLAIN, 14 ); bold. Font = new Font( "Serif", Font. BOLD, 14 ); italic. Font = new Font( "Serif", Font. ITALIC, 14 ); bold. Italic. Font = new Font( "Serif", Font. BOLD + Font. ITALIC, 14 ); field. set. Font( plain. Font ); // set initial font 2003 Prentice Hall, Inc. All rights reserved.
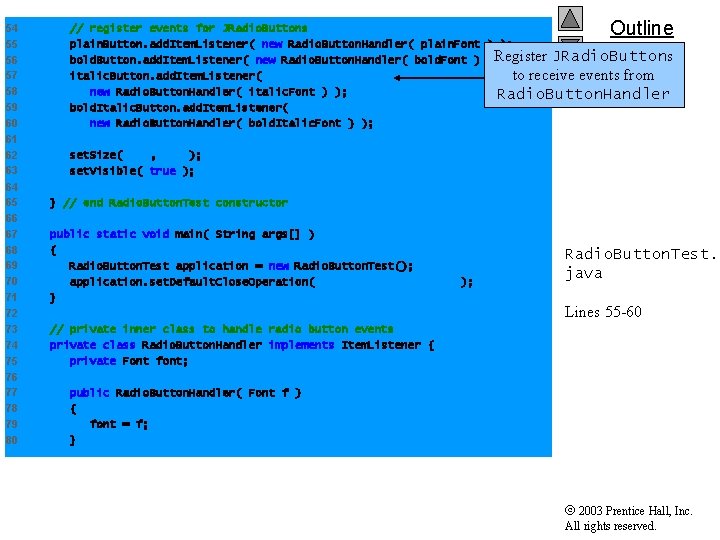
54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 Outline // register events for JRadio. Buttons plain. Button. add. Item. Listener( new Radio. Button. Handler( plain. Font ) ); Register JRadio. Buttons bold. Button. add. Item. Listener( new Radio. Button. Handler( bold. Font ) ); italic. Button. add. Item. Listener( to receive events from new Radio. Button. Handler( italic. Font ) ); Radio. Button. Handler bold. Italic. Button. add. Item. Listener( new Radio. Button. Handler( bold. Italic. Font ) ); set. Size( 300, 100 ); set. Visible( true ); } // end Radio. Button. Test constructor public static void main( String args[] ) { Radio. Button. Test application = new Radio. Button. Test(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } Radio. Button. Test. java Lines 55 -60 // private inner class to handle radio button events private class Radio. Button. Handler implements Item. Listener { private Font font; public Radio. Button. Handler( Font f ) { font = f; } 2003 Prentice Hall, Inc. All rights reserved.
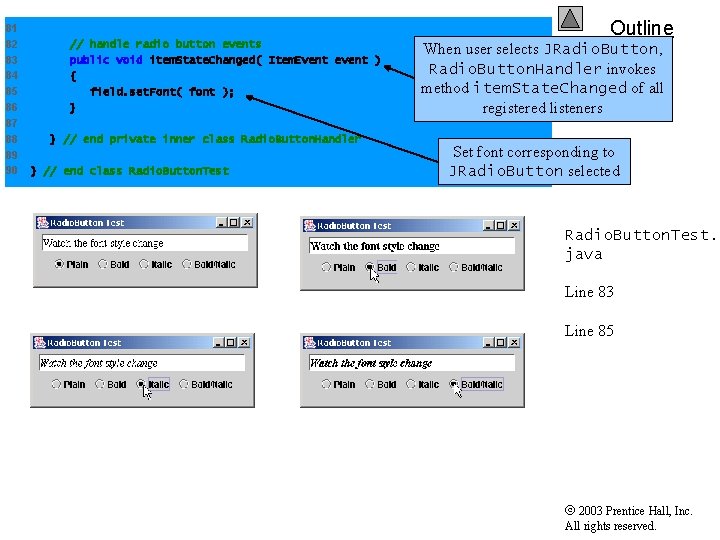
81 82 83 84 85 86 87 88 89 90 // handle radio button events public void item. State. Changed( Item. Event event ) { field. set. Font( font ); } } // end private inner class Radio. Button. Handler } // end class Radio. Button. Test Outline When user selects JRadio. Button, Radio. Button. Handler invokes method item. State. Changed of all registered listeners Set font corresponding to JRadio. Button selected Radio. Button. Test. java Line 83 Line 85 2003 Prentice Hall, Inc. All rights reserved.
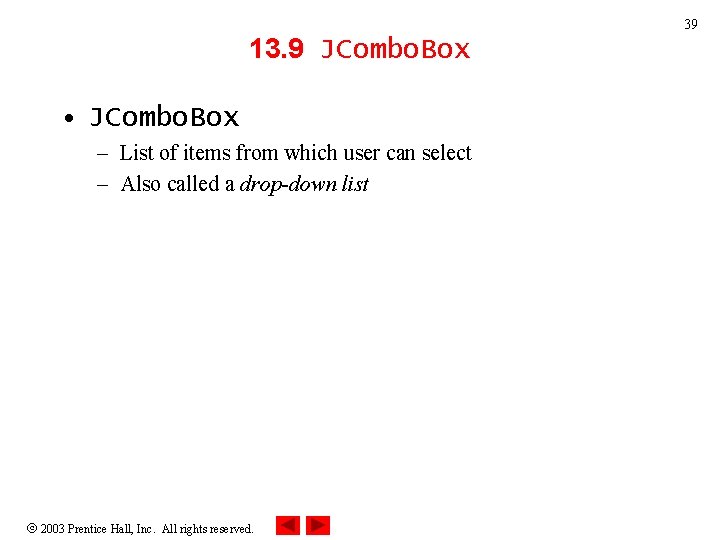
39 13. 9 JCombo. Box • JCombo. Box – List of items from which user can select – Also called a drop-down list 2003 Prentice Hall, Inc. All rights reserved.
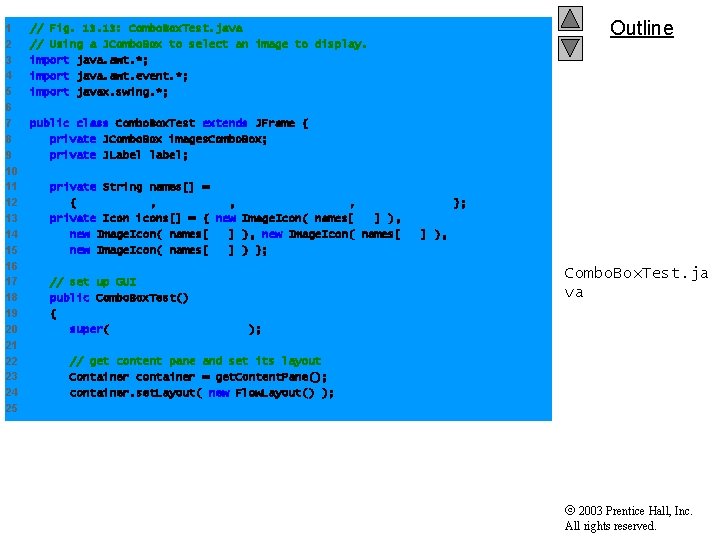
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 // Fig. 13: Combo. Box. Test. java // Using a JCombo. Box to select an image to display. import java. awt. *; import java. awt. event. *; import javax. swing. *; Outline public class Combo. Box. Test extends JFrame { private JCombo. Box images. Combo. Box; private JLabel label; private String names[] = { "bug 1. gif", "bug 2. gif", "travelbug. gif", "buganim. gif" }; private Icon icons[] = { new Image. Icon( names[ 0 ] ), new Image. Icon( names[ 1 ] ), new Image. Icon( names[ 2 ] ), new Image. Icon( names[ 3 ] ) }; // set up GUI public Combo. Box. Test() { super( "Testing JCombo. Box" ); Combo. Box. Test. ja va // get content pane and set its layout Container container = get. Content. Pane(); container. set. Layout( new Flow. Layout() ); 2003 Prentice Hall, Inc. All rights reserved.
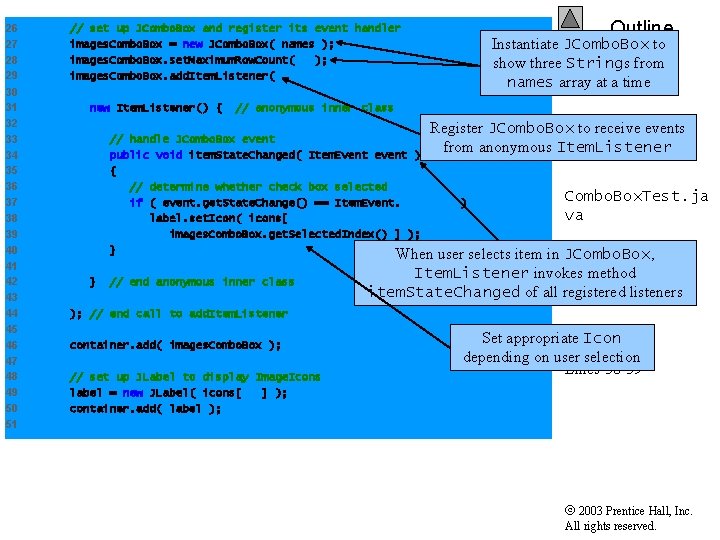
26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 // set up JCombo. Box and register its event handler images. Combo. Box = new JCombo. Box( names ); images. Combo. Box. set. Maximum. Row. Count( 3 ); images. Combo. Box. add. Item. Listener( new Item. Listener() { Outline Instantiate JCombo. Box to show three Strings from names array at a time // anonymous inner class Register JCombo. Box to receive events // handle JCombo. Box event from anonymous Item. Listener public void item. State. Changed( Item. Event event ) { // determine whether check box selected Combo. Box. Test. ja if ( event. get. State. Change() == Item. Event. SELECTED ) va label. set. Icon( icons[ images. Combo. Box. get. Selected. Index() ] ); } When user selects item in JCombo. Box, Lines 27 -28 } // end anonymous inner class Item. Listener invokes method item. State. Changed of all registered Line 29 listeners ); // end call to add. Item. Listener container. add( images. Combo. Box ); // set up JLabel to display Image. Icons label = new JLabel( icons[ 0 ] ); container. add( label ); Line 34 Set appropriate Icon depending on user selection Lines 38 -39 2003 Prentice Hall, Inc. All rights reserved.
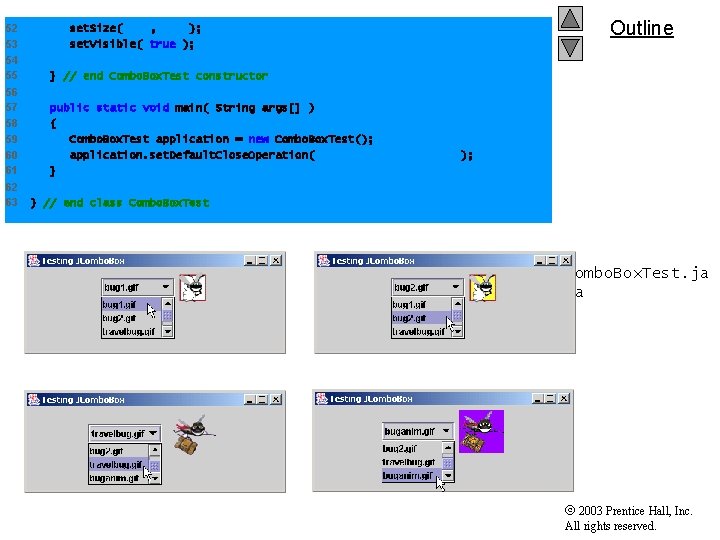
52 53 54 55 56 57 58 59 60 61 62 63 set. Size( 350, 100 ); set. Visible( true ); Outline } // end Combo. Box. Test constructor public static void main( String args[] ) { Combo. Box. Test application = new Combo. Box. Test(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } } // end class Combo. Box. Test. ja va 2003 Prentice Hall, Inc. All rights reserved.
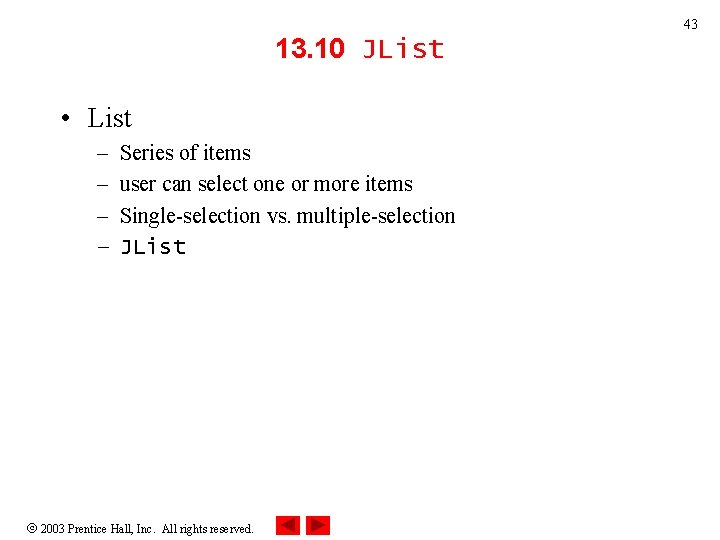
43 13. 10 JList • List – – Series of items user can select one or more items Single-selection vs. multiple-selection JList 2003 Prentice Hall, Inc. All rights reserved.
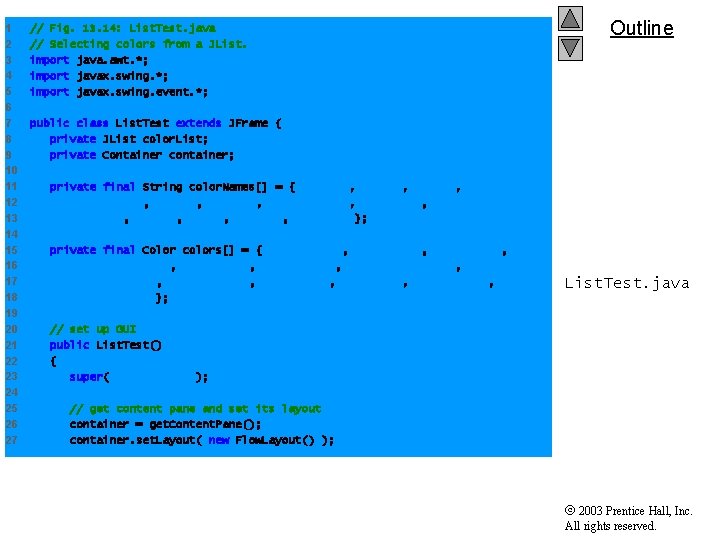
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 // Fig. 13. 14: List. Test. java // Selecting colors from a JList. import java. awt. *; import javax. swing. event. *; Outline public class List. Test extends JFrame { private JList color. List; private Container container; private final String color. Names[] = { "Black", "Blue", "Cyan", "Dark Gray", "Green", "Light Gray", "Magenta", "Orange", "Pink", "Red", "White", "Yellow" }; private final Color colors[] = { Color. BLACK, Color. BLUE, Color. CYAN, Color. DARK_GRAY, Color. GREEN, Color. LIGHT_GRAY, Color. MAGENTA, Color. ORANGE, Color. PINK, Color. RED, Color. WHITE, Color. YELLOW }; List. Test. java // set up GUI public List. Test() { super( "List Test" ); // get content pane and set its layout container = get. Content. Pane(); container. set. Layout( new Flow. Layout() ); 2003 Prentice Hall, Inc. All rights reserved.
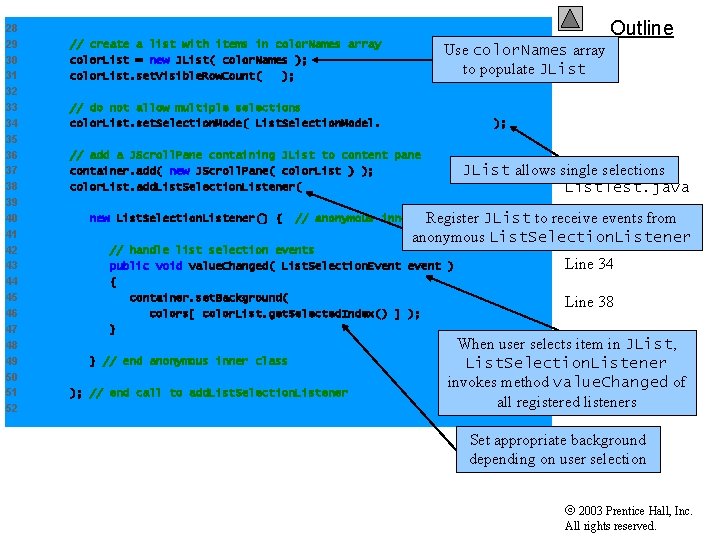
28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 Outline // create a list with items in color. Names array color. List = new JList( color. Names ); color. List. set. Visible. Row. Count( 5 ); Use color. Names array to populate JList // do not allow multiple selections color. List. set. Selection. Mode( List. Selection. Model. SINGLE_SELECTION ); // add a JScroll. Pane containing JList to content pane container. add( new JScroll. Pane( color. List ) ); color. List. add. List. Selection. Listener( new List. Selection. Listener() { JList allows single selections List. Test. java // anonymous inner class Register JList to receive events from Line 30 anonymous List. Selection. Listener // handle list selection events public void value. Changed( List. Selection. Event event ) { container. set. Background( colors[ color. List. get. Selected. Index() ] ); } } // end anonymous inner class ); // end call to add. List. Selection. Listener Line 34 Line 38 When user selects. Line item 43 in JList, List. Selection. Listener Lines 45 -46 invokes method value. Changed of all registered listeners Set appropriate background depending on user selection 2003 Prentice Hall, Inc. All rights reserved.
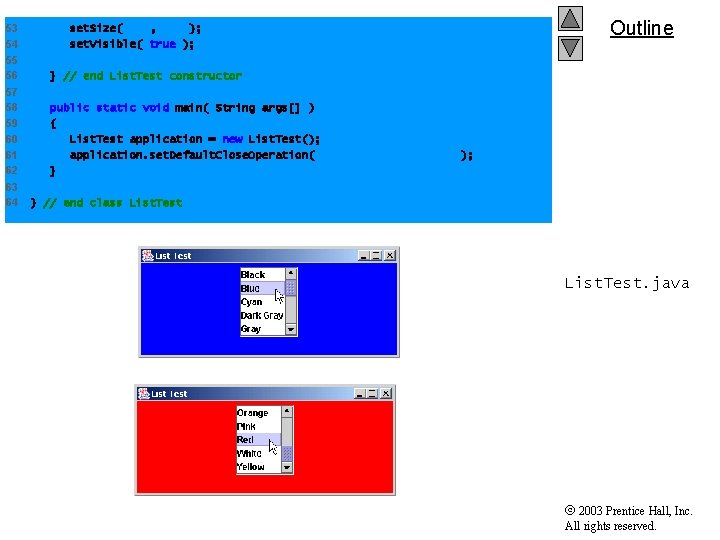
53 54 55 56 57 58 59 60 61 62 63 64 set. Size( 350, 150 ); set. Visible( true ); Outline } // end List. Test constructor public static void main( String args[] ) { List. Test application = new List. Test(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } } // end class List. Test. java 2003 Prentice Hall, Inc. All rights reserved.
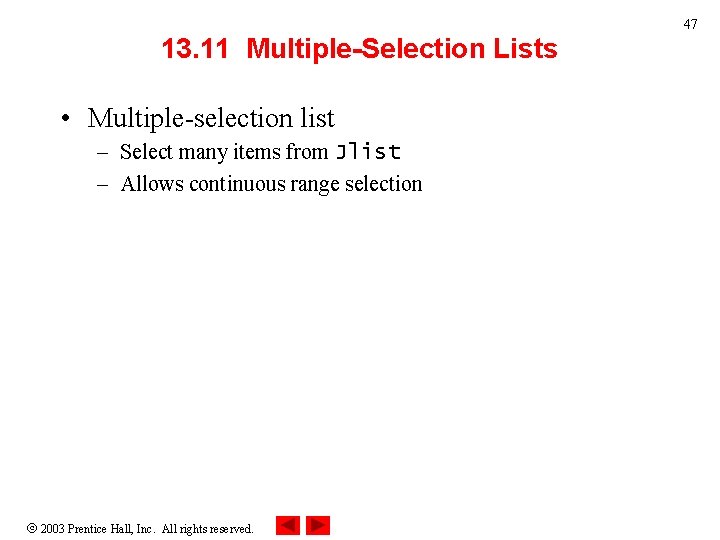
47 13. 11 Multiple-Selection Lists • Multiple-selection list – Select many items from Jlist – Allows continuous range selection 2003 Prentice Hall, Inc. All rights reserved.
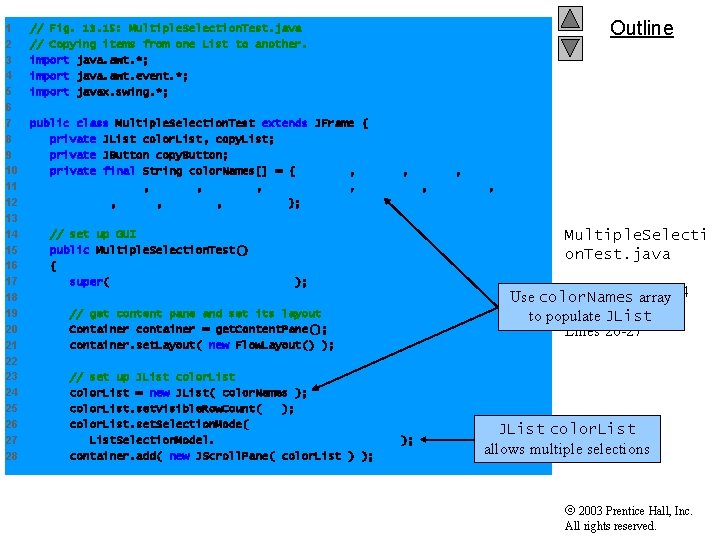
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 Outline // Fig. 13. 15: Multiple. Selection. Test. java // Copying items from one List to another. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Multiple. Selection. Test extends JFrame { private JList color. List, copy. List; private JButton copy. Button; private final String color. Names[] = { "Black", "Blue", "Cyan", "Dark Gray", "Green", "Light Gray", "Magenta", "Orange", "Pink", "Red", "White", "Yellow" }; // set up GUI public Multiple. Selection. Test() { super( "Multiple Selection Lists" ); // get content pane and set its layout Container container = get. Content. Pane(); container. set. Layout( new Flow. Layout() ); // set up JList color. List = new JList( color. Names ); color. List. set. Visible. Row. Count( 5 ); color. List. set. Selection. Mode( List. Selection. Model. MULTIPLE_INTERVAL_SELECTION ); container. add( new JScroll. Pane( color. List ) ); Multiple. Selecti on. Test. java Lines 10 -12 array and 24 Use color. Names to populate JList Lines 26 -27 JList color. List allows multiple selections 2003 Prentice Hall, Inc. All rights reserved.
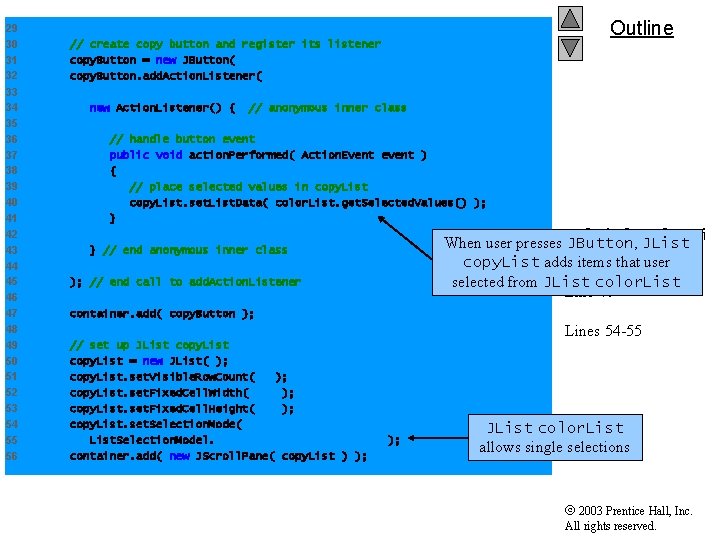
29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 Outline // create copy button and register its listener copy. Button = new JButton( "Copy >>>" ); copy. Button. add. Action. Listener( new Action. Listener() { // anonymous inner class // handle button event public void action. Performed( Action. Event event ) { // place selected values in copy. List. set. List. Data( color. List. get. Selected. Values() ); } } // end anonymous inner class ); // end call to add. Action. Listener Multiple. Selecti When user presses JButton, JList on. Test. java copy. List adds items that user selected from JList color. List Line 40 container. add( copy. Button ); Lines 54 -55 // set up JList copy. List = new JList( ); copy. List. set. Visible. Row. Count( 5 ); copy. List. set. Fixed. Cell. Width( 100 ); copy. List. set. Fixed. Cell. Height( 15 ); copy. List. set. Selection. Mode( List. Selection. Model. SINGLE_INTERVAL_SELECTION ); container. add( new JScroll. Pane( copy. List ) ); JList color. List allows single selections 2003 Prentice Hall, Inc. All rights reserved.
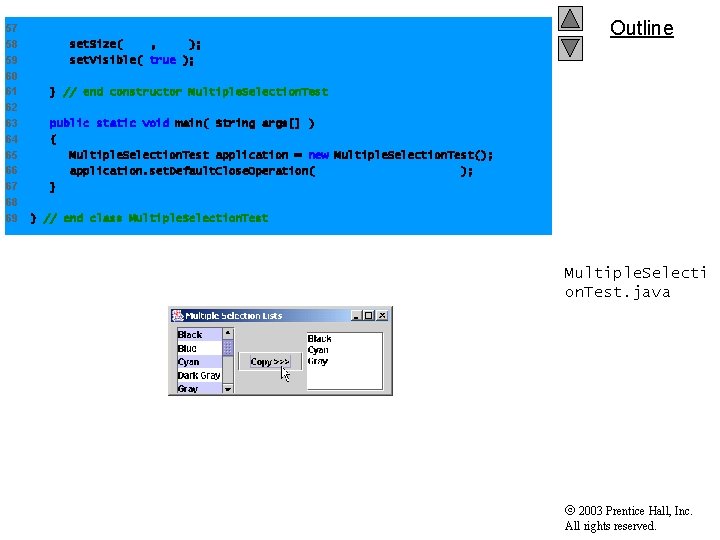
57 58 59 60 61 62 63 64 65 66 67 68 69 set. Size( 300, 130 ); set. Visible( true ); Outline } // end constructor Multiple. Selection. Test public static void main( String args[] ) { Multiple. Selection. Test application = new Multiple. Selection. Test(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } } // end class Multiple. Selection. Test Multiple. Selecti on. Test. java 2003 Prentice Hall, Inc. All rights reserved.
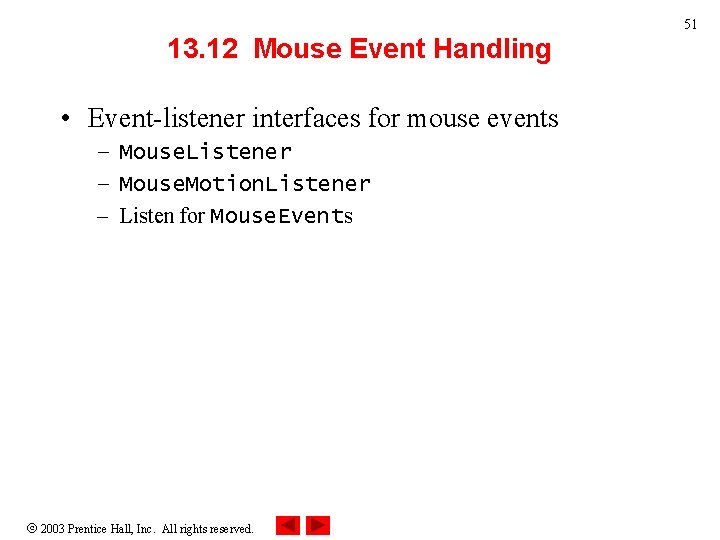
51 13. 12 Mouse Event Handling • Event-listener interfaces for mouse events – Mouse. Listener – Mouse. Motion. Listener – Listen for Mouse. Events 2003 Prentice Hall, Inc. All rights reserved.
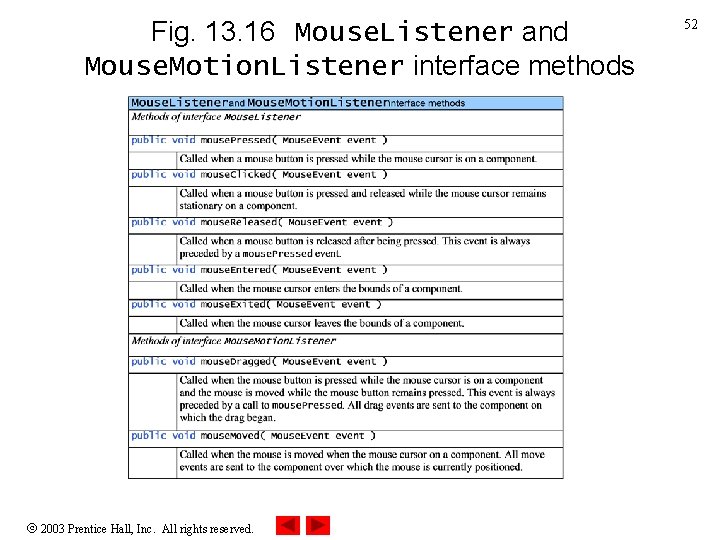
Fig. 13. 16 Mouse. Listener and Mouse. Motion. Listener interface methods 2003 Prentice Hall, Inc. All rights reserved. 52
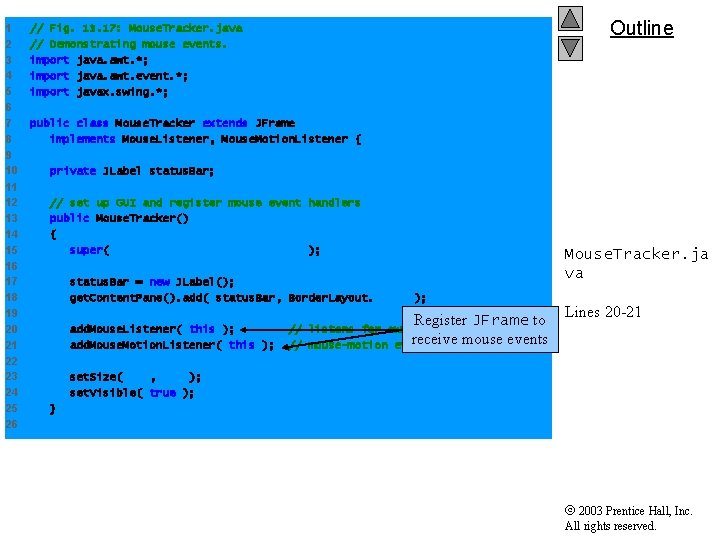
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 Outline // Fig. 13. 17: Mouse. Tracker. java // Demonstrating mouse events. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Mouse. Tracker extends JFrame implements Mouse. Listener, Mouse. Motion. Listener { private JLabel status. Bar; // set up GUI and register mouse event handlers public Mouse. Tracker() { super( "Demonstrating Mouse Events" ); status. Bar = new JLabel(); get. Content. Pane(). add( status. Bar, Border. Layout. SOUTH ); add. Mouse. Listener( this ); add. Mouse. Motion. Listener( this ); // // Register JFrame to listens for own mouse and receive mouse events mouse-motion events Mouse. Tracker. ja va Lines 20 -21 set. Size( 275, 100 ); set. Visible( true ); } 2003 Prentice Hall, Inc. All rights reserved.
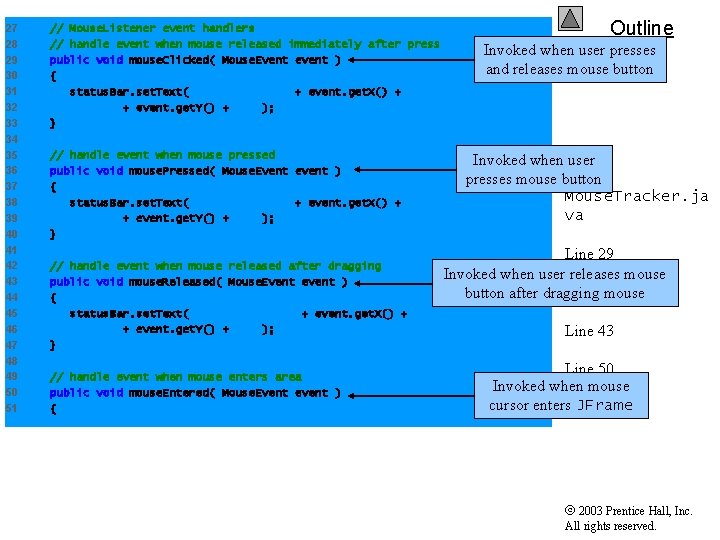
27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 // Mouse. Listener event handlers // handle event when mouse released immediately after press public void mouse. Clicked( Mouse. Event event ) { status. Bar. set. Text( "Clicked at [" + event. get. X() + ", " + event. get. Y() + "]" ); } // handle event when mouse pressed public void mouse. Pressed( Mouse. Event event ) { status. Bar. set. Text( "Pressed at [" + event. get. X() + ", " + event. get. Y() + "]" ); } // handle event when mouse released after dragging public void mouse. Released( Mouse. Event event ) { status. Bar. set. Text( "Released at [" + event. get. X() + ", " + event. get. Y() + "]" ); } // handle event when mouse enters area public void mouse. Entered( Mouse. Event event ) { Outline Invoked when user presses and releases mouse button Invoked when user presses mouse button Mouse. Tracker. ja va Line 29 Invoked when user releases mouse Line 36 button after dragging mouse Line 43 Line 50 Invoked when mouse cursor enters JFrame 2003 Prentice Hall, Inc. All rights reserved.
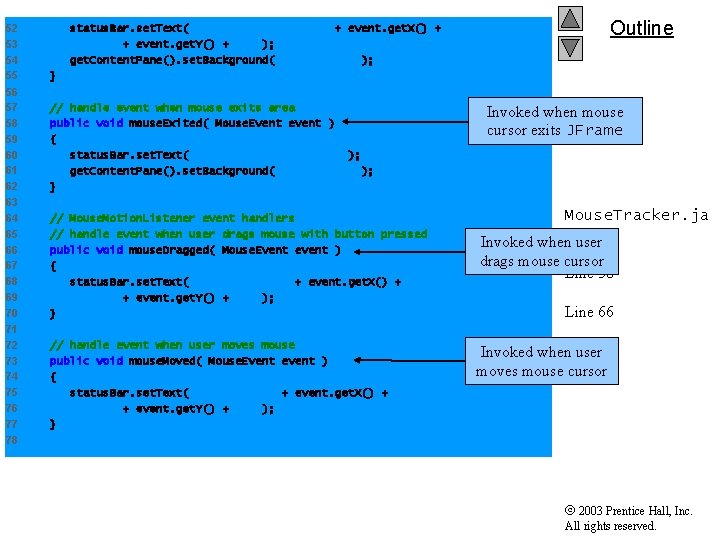
52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 status. Bar. set. Text( "Mouse entered at [" + event. get. X() + ", " + event. get. Y() + "]" ); get. Content. Pane(). set. Background( Color. GREEN ); Outline } // handle event when mouse exits area public void mouse. Exited( Mouse. Event event ) { status. Bar. set. Text( "Mouse outside window" ); get. Content. Pane(). set. Background( Color. WHITE ); } // Mouse. Motion. Listener event handlers // handle event when user drags mouse with button pressed public void mouse. Dragged( Mouse. Event event ) { status. Bar. set. Text( "Dragged at [" + event. get. X() + ", " + event. get. Y() + "]" ); } // handle event when user moves mouse public void mouse. Moved( Mouse. Event event ) { status. Bar. set. Text( "Moved at [" + event. get. X() + ", " + event. get. Y() + "]" ); } Invoked when mouse cursor exits JFrame Mouse. Tracker. ja Invoked whenva user drags mouse cursor Line 58 Line 66 Invoked when. Line user 73 moves mouse cursor 2003 Prentice Hall, Inc. All rights reserved.
![79 80 81 82 83 84 85 public static void main String args 79 80 81 82 83 84 85 public static void main( String args[] )](https://slidetodoc.com/presentation_image_h2/a75e4a64e3dd0a25bd045296b3dce9f9/image-56.jpg)
79 80 81 82 83 84 85 public static void main( String args[] ) { Mouse. Tracker application = new Mouse. Tracker(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } Outline } // end class Mouse. Tracker. ja va 2003 Prentice Hall, Inc. All rights reserved.
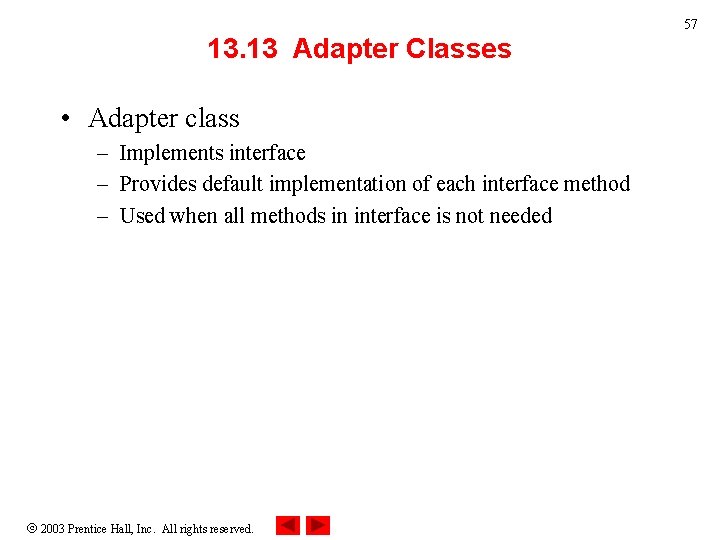
57 13. 13 Adapter Classes • Adapter class – Implements interface – Provides default implementation of each interface method – Used when all methods in interface is not needed 2003 Prentice Hall, Inc. All rights reserved.
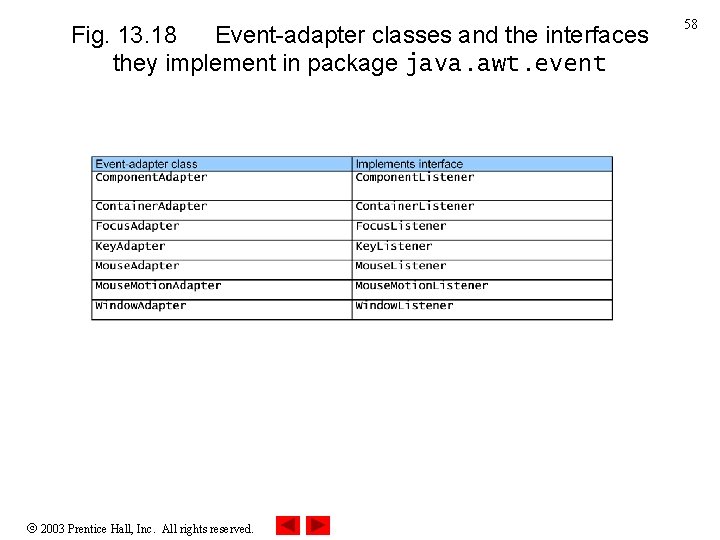
Fig. 13. 18 Event-adapter classes and the interfaces they implement in package java. awt. event 2003 Prentice Hall, Inc. All rights reserved. 58
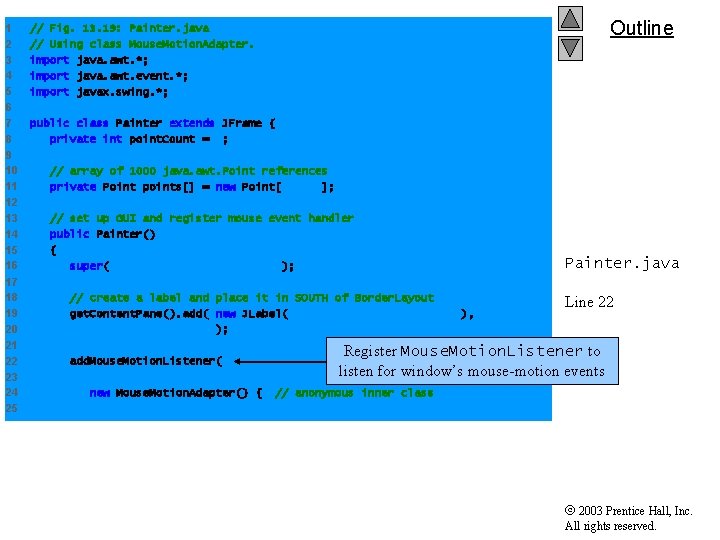
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Outline // Fig. 13. 19: Painter. java // Using class Mouse. Motion. Adapter. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Painter extends JFrame { private int point. Count = 0; // array of 1000 java. awt. Point references private Point points[] = new Point[ 1000 ]; // set up GUI and register mouse event handler public Painter() { super( "A simple paint program" ); // create a label and place it in SOUTH of Border. Layout get. Content. Pane(). add( new JLabel( "Drag the mouse to draw" ), Border. Layout. SOUTH ); add. Mouse. Motion. Listener( new Mouse. Motion. Adapter() { Painter. java Line 22 Register Mouse. Motion. Listener to listen for window’s mouse-motion events // anonymous inner class 2003 Prentice Hall, Inc. All rights reserved.
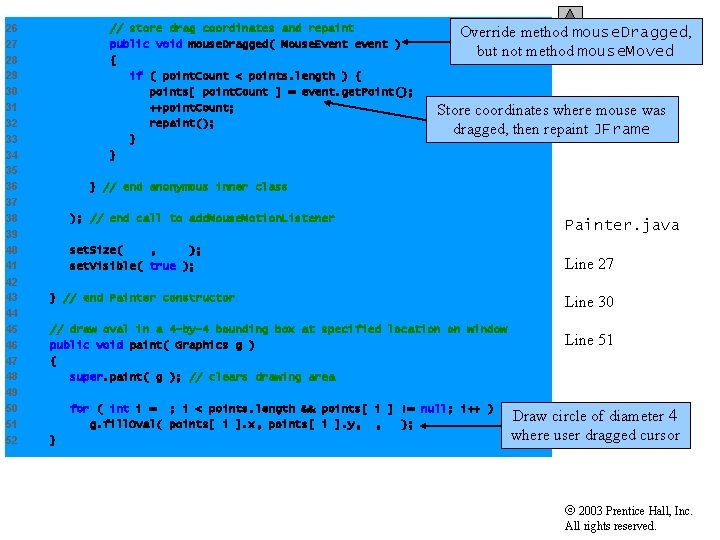
26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 // store drag coordinates and repaint public void mouse. Dragged( Mouse. Event event ) { if ( point. Count < points. length ) { points[ point. Count ] = event. get. Point(); ++point. Count; repaint(); } } Outline Override method mouse. Dragged, but not method mouse. Moved Store coordinates where mouse was dragged, then repaint JFrame } // end anonymous inner class ); // end call to add. Mouse. Motion. Listener set. Size( 300, 150 ); set. Visible( true ); } // end Painter constructor // draw oval in a 4 -by-4 bounding box at specified location on window public void paint( Graphics g ) { super. paint( g ); // clears drawing area for ( int i = 0; i < points. length && points[ i ] != null; i++ ) g. fill. Oval( points[ i ]. x, points[ i ]. y, 4, 4 ); } Painter. java Line 27 Line 30 Line 51 Draw circle of diameter 4 where user dragged cursor 2003 Prentice Hall, Inc. All rights reserved.
![53 54 55 56 57 58 59 60 public static void main String args 53 54 55 56 57 58 59 60 public static void main( String args[]](https://slidetodoc.com/presentation_image_h2/a75e4a64e3dd0a25bd045296b3dce9f9/image-61.jpg)
53 54 55 56 57 58 59 60 public static void main( String args[] ) { Painter application = new Painter(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } Outline } // end class Painter. java 2003 Prentice Hall, Inc. All rights reserved.
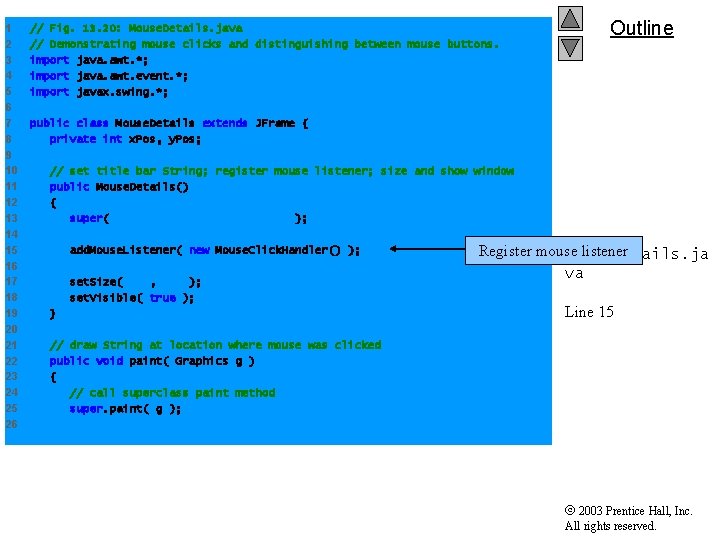
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 // Fig. 13. 20: Mouse. Details. java // Demonstrating mouse clicks and distinguishing between mouse buttons. import java. awt. *; import java. awt. event. *; import javax. swing. *; Outline public class Mouse. Details extends JFrame { private int x. Pos, y. Pos; // set title bar String; register mouse listener; size and show window public Mouse. Details() { super( "Mouse clicks and buttons" ); add. Mouse. Listener( new Mouse. Click. Handler() ); set. Size( 350, 150 ); set. Visible( true ); } Register mouse listener Mouse. Details. ja va Line 15 // draw String at location where mouse was clicked public void paint( Graphics g ) { // call superclass paint method super. paint( g ); 2003 Prentice Hall, Inc. All rights reserved.
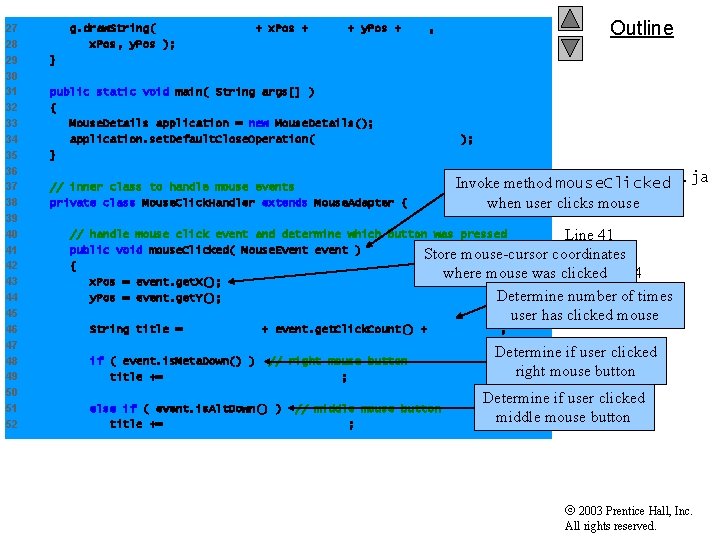
27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 Outline g. draw. String( "Clicked @ [" + x. Pos + ", " + y. Pos + "]", x. Pos, y. Pos ); } public static void main( String args[] ) { Mouse. Details application = new Mouse. Details(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } // inner class to handle mouse events private class Mouse. Click. Handler extends Mouse. Adapter { Mouse. Details. ja Invoke method mouse. Clicked va mouse when user clicks // handle mouse click event and determine which button was pressed Line 41 public void mouse. Clicked( Mouse. Event event ) Store mouse-cursor coordinates { where mouse was clicked Lines 43 -44 x. Pos = event. get. X(); Determine number of times y. Pos = event. get. Y(); 46 mouse user has Line clicked String title = "Clicked " + event. get. Click. Count() + " time(s)"; if ( event. is. Meta. Down() ) // right mouse button title += " with right mouse button"; else if ( event. is. Alt. Down() ) // middle mouse button title += " with center mouse button"; 48 clicked Determine Line if user right mouse button Line 51 Determine if user clicked middle mouse button 2003 Prentice Hall, Inc. All rights reserved.
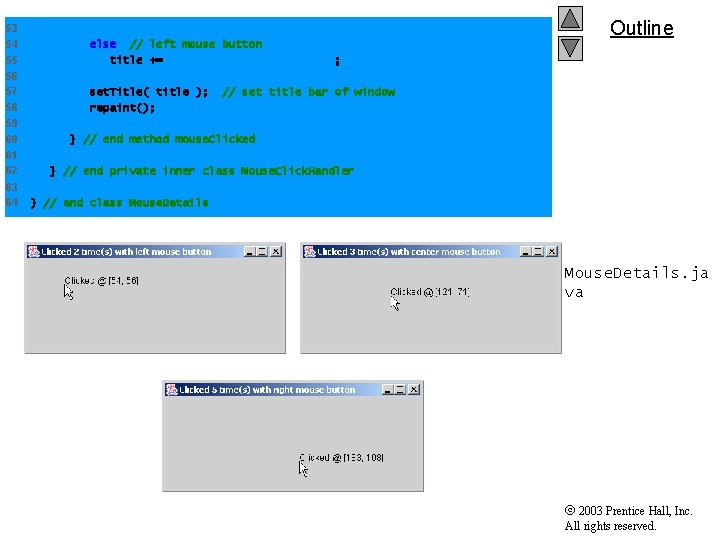
53 54 55 56 57 58 59 60 61 62 63 64 else // left mouse button title += " with left mouse button"; set. Title( title ); repaint(); Outline // set title bar of window } // end method mouse. Clicked } // end private inner class Mouse. Click. Handler } // end class Mouse. Details. ja va 2003 Prentice Hall, Inc. All rights reserved.
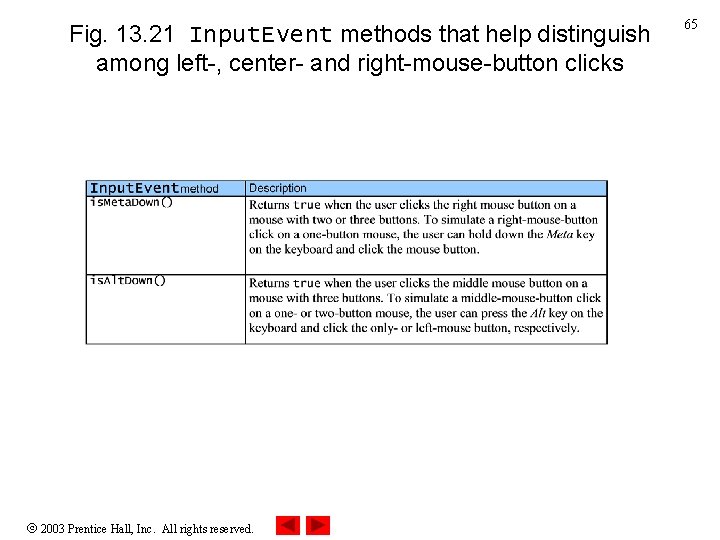
Fig. 13. 21 Input. Event methods that help distinguish among left-, center- and right-mouse-button clicks 2003 Prentice Hall, Inc. All rights reserved. 65
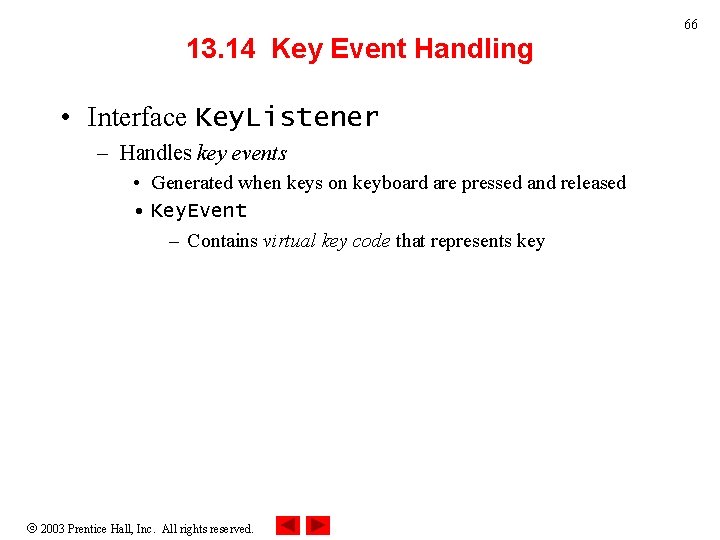
66 13. 14 Key Event Handling • Interface Key. Listener – Handles key events • Generated when keys on keyboard are pressed and released • Key. Event – Contains virtual key code that represents key 2003 Prentice Hall, Inc. All rights reserved.
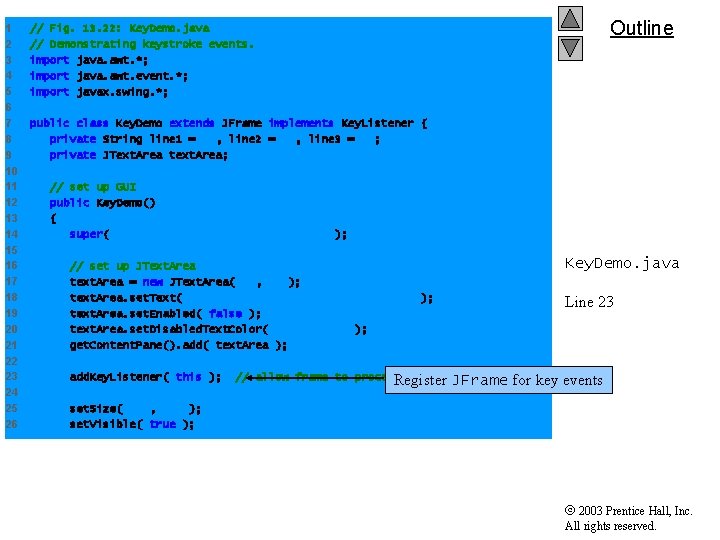
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 Outline // Fig. 13. 22: Key. Demo. java // Demonstrating keystroke events. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Key. Demo extends JFrame implements Key. Listener { private String line 1 = "", line 2 = "", line 3 = ""; private JText. Area text. Area; // set up GUI public Key. Demo() { super( "Demonstrating Keystroke Events" ); // set up JText. Area text. Area = new JText. Area( 10, 15 ); text. Area. set. Text( "Press any key on the keyboard. . . " ); text. Area. set. Enabled( false ); text. Area. set. Disabled. Text. Color( Color. BLACK ); get. Content. Pane(). add( text. Area ); add. Key. Listener( this ); // allow frame to process Key events Register JFrame Key. Demo. java Line 23 for key events set. Size( 350, 100 ); set. Visible( true ); 2003 Prentice Hall, Inc. All rights reserved.
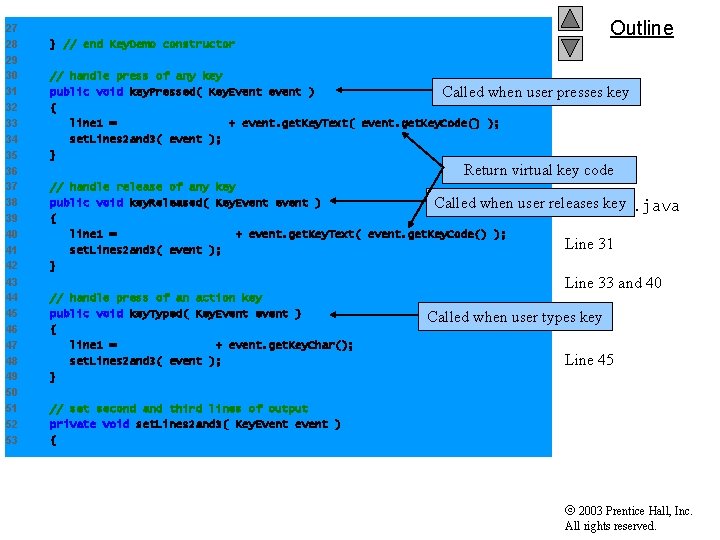
27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 Outline } // end Key. Demo constructor // handle press of any key public void key. Pressed( Key. Event event ) Called when { line 1 = "Key pressed: " + event. get. Key. Text( event. get. Key. Code() ); set. Lines 2 and 3( event ); } user presses key Return virtual key code // handle release of any key public void key. Released( Key. Event event ) Called when { line 1 = "Key released: " + event. get. Key. Text( event. get. Key. Code() ); set. Lines 2 and 3( event ); } user releases key Key. Demo. java Line 31 Line 33 and 40 // handle press of an action key public void key. Typed( Key. Event event ) { line 1 = "Key typed: " + event. get. Key. Char(); set. Lines 2 and 3( event ); } Called when user types key 38 Line 45 // set second and third lines of output private void set. Lines 2 and 3( Key. Event event ) { 2003 Prentice Hall, Inc. All rights reserved.
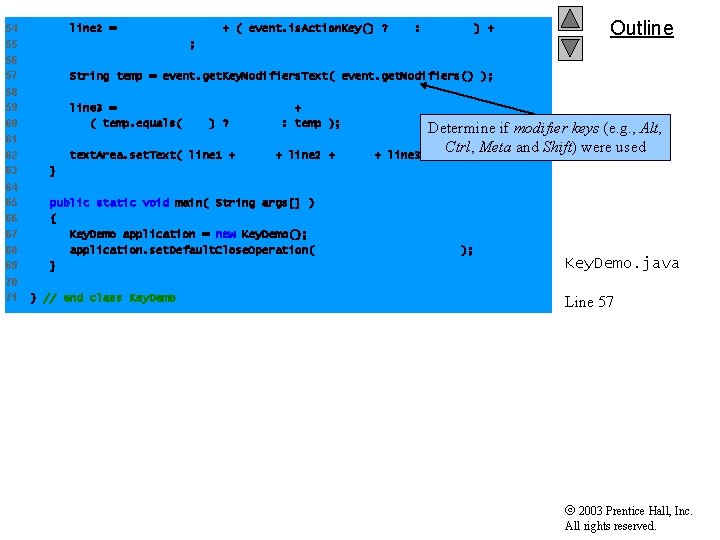
54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 line 2 = "This key is " + ( event. is. Action. Key() ? "" : "not " ) + "an action key"; Outline String temp = event. get. Key. Modifiers. Text( event. get. Modifiers() ); line 3 = "Modifier keys pressed: " + ( temp. equals( "" ) ? "none" : temp ); text. Area. set. Text( line 1 + "n" + line 2 + "n" + line 3 Determine if modifier keys (e. g. , Alt, Ctrl, Meta and Shift) were used + "n" ); } public static void main( String args[] ) { Key. Demo application = new Key. Demo(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } } // end class Key. Demo. java Line 57 2003 Prentice Hall, Inc. All rights reserved.
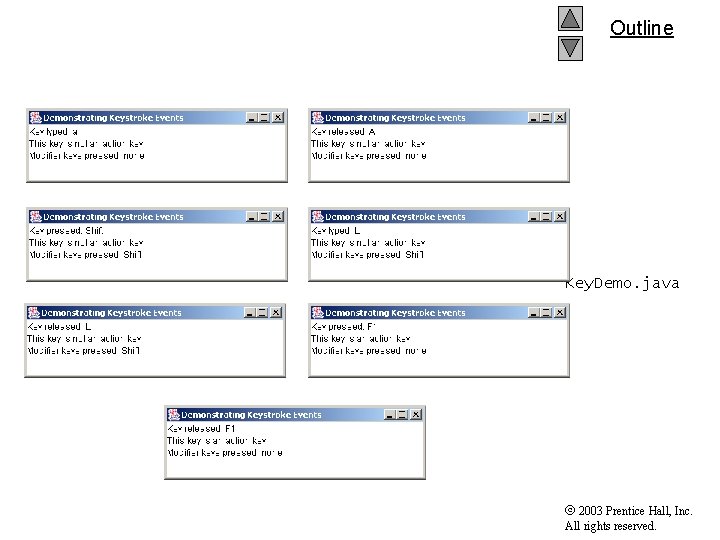
Outline Key. Demo. java 2003 Prentice Hall, Inc. All rights reserved.
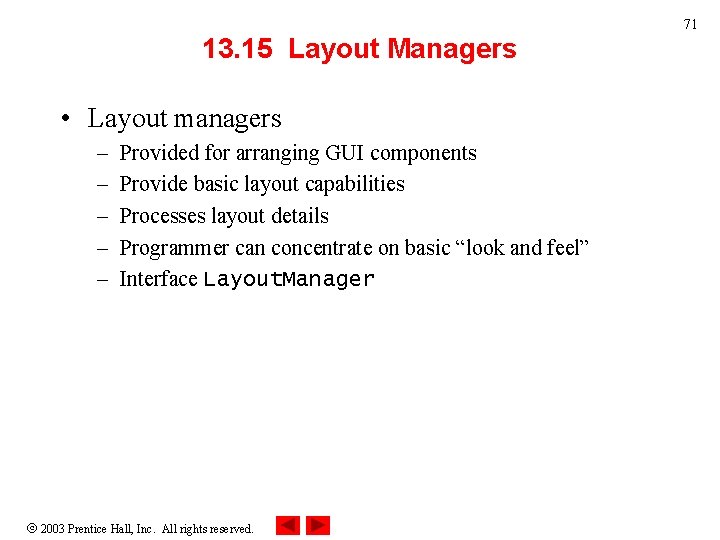
71 13. 15 Layout Managers • Layout managers – – – Provided for arranging GUI components Provide basic layout capabilities Processes layout details Programmer can concentrate on basic “look and feel” Interface Layout. Manager 2003 Prentice Hall, Inc. All rights reserved.
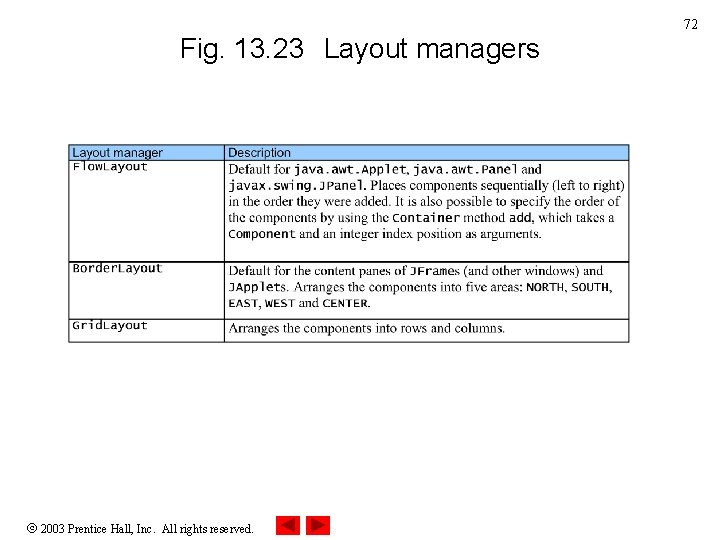
72 Fig. 13. 23 Layout managers 2003 Prentice Hall, Inc. All rights reserved.
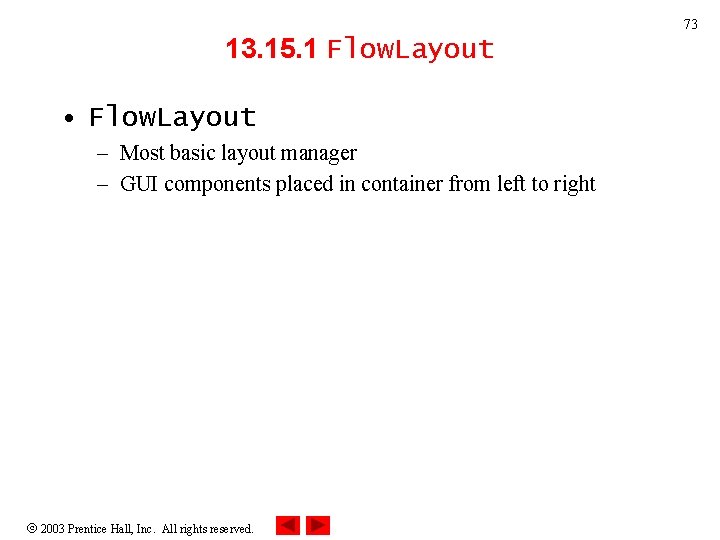
73 13. 15. 1 Flow. Layout • Flow. Layout – Most basic layout manager – GUI components placed in container from left to right 2003 Prentice Hall, Inc. All rights reserved.
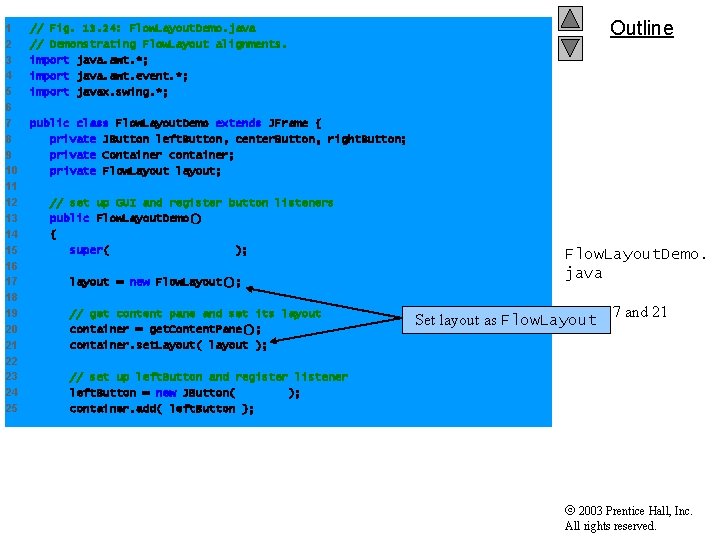
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 // Fig. 13. 24: Flow. Layout. Demo. java // Demonstrating Flow. Layout alignments. import java. awt. *; import java. awt. event. *; import javax. swing. *; Outline public class Flow. Layout. Demo extends JFrame { private JButton left. Button, center. Button, right. Button; private Container container; private Flow. Layout layout; // set up GUI and register button listeners public Flow. Layout. Demo() { super( "Flow. Layout Demo" ); layout = new Flow. Layout(); // get content pane and set its layout container = get. Content. Pane(); container. set. Layout( layout ); Flow. Layout. Demo. java Lines 17 and 21 Set layout as Flow. Layout // set up left. Button and register listener left. Button = new JButton( "Left" ); container. add( left. Button ); 2003 Prentice Hall, Inc. All rights reserved.
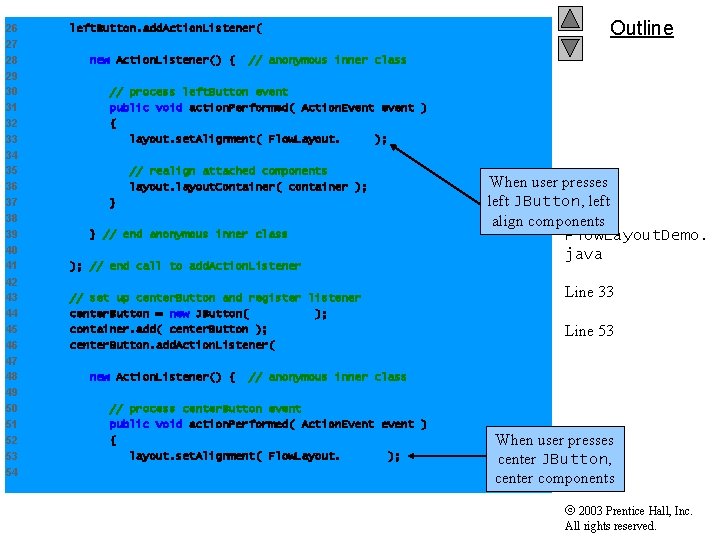
26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 left. Button. add. Action. Listener( new Action. Listener() { Outline // anonymous inner class // process left. Button event public void action. Performed( Action. Event event ) { layout. set. Alignment( Flow. Layout. LEFT ); // realign attached components layout. Container( container ); } } // end anonymous inner class ); // end call to add. Action. Listener // set up center. Button and register listener center. Button = new JButton( "Center" ); container. add( center. Button ); center. Button. add. Action. Listener( new Action. Listener() { When user presses left JButton, left align components Flow. Layout. Demo. java Line 33 Line 53 // anonymous inner class // process center. Button event public void action. Performed( Action. Event event ) { layout. set. Alignment( Flow. Layout. CENTER ); When user presses center JButton, center components 2003 Prentice Hall, Inc. All rights reserved.
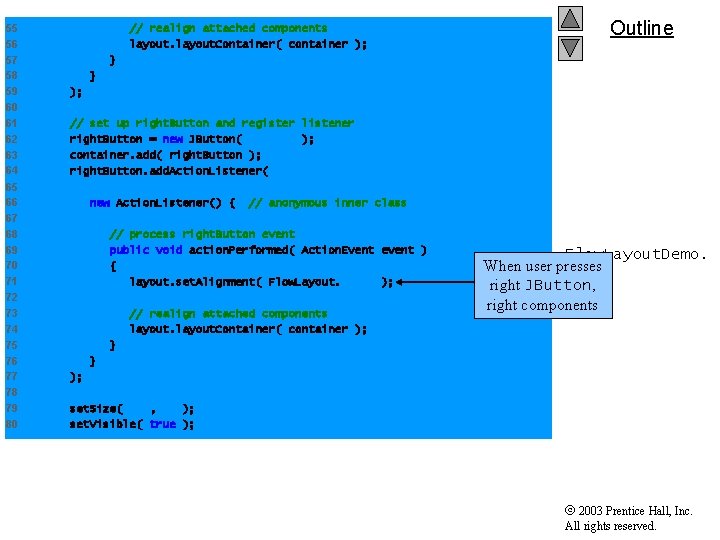
55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 // realign attached components layout. Container( container ); Outline } } ); // set up right. Button and register listener right. Button = new JButton( "Right" ); container. add( right. Button ); right. Button. add. Action. Listener( new Action. Listener() { // anonymous inner class // process right. Button event public void action. Performed( Action. Event event ) { layout. set. Alignment( Flow. Layout. RIGHT ); // realign attached components layout. Container( container ); Flow. Layout. Demo. When user presses java right JButton, right components Line 71 } } ); set. Size( 300, 75 ); set. Visible( true ); 2003 Prentice Hall, Inc. All rights reserved.
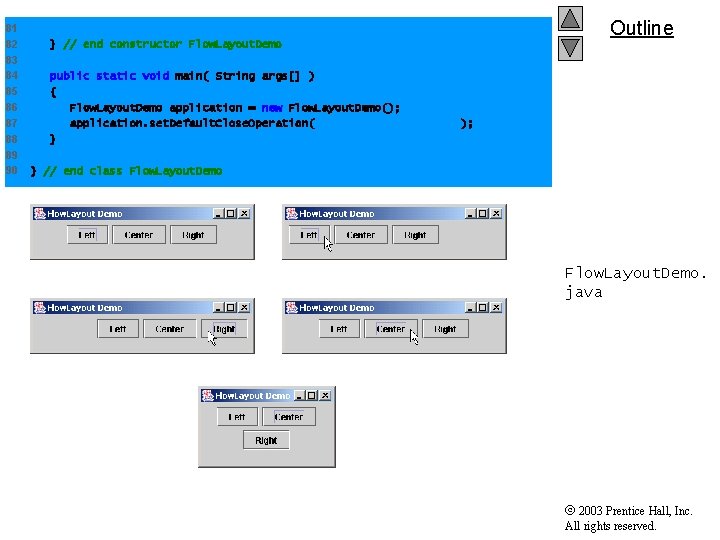
81 82 83 84 85 86 87 88 89 90 } // end constructor Flow. Layout. Demo Outline public static void main( String args[] ) { Flow. Layout. Demo application = new Flow. Layout. Demo(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } } // end class Flow. Layout. Demo. java 2003 Prentice Hall, Inc. All rights reserved.
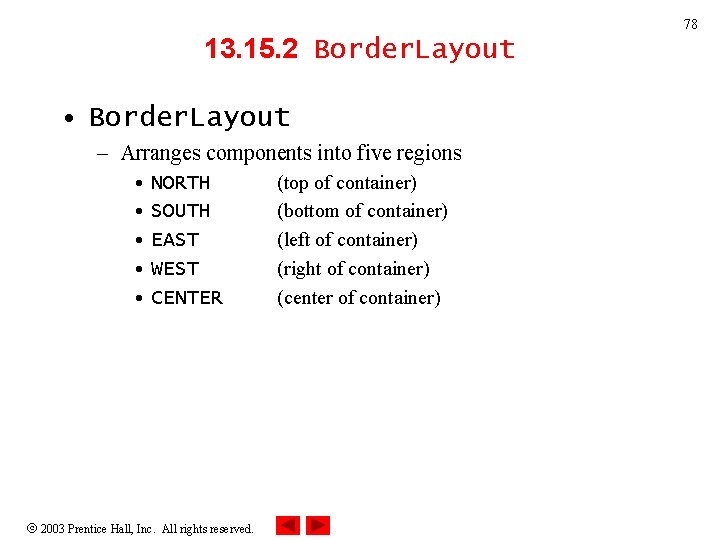
78 13. 15. 2 Border. Layout • Border. Layout – Arranges components into five regions • NORTH • SOUTH • EAST • WEST • CENTER 2003 Prentice Hall, Inc. All rights reserved. (top of container) (bottom of container) (left of container) (right of container) (center of container)
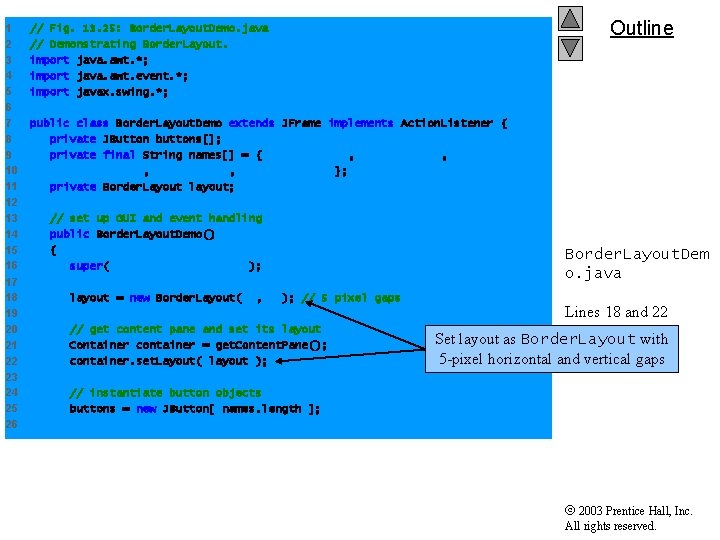
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 Outline // Fig. 13. 25: Border. Layout. Demo. java // Demonstrating Border. Layout. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Border. Layout. Demo extends JFrame implements Action. Listener { private JButton buttons[]; private final String names[] = { "Hide North", "Hide South", "Hide East", "Hide West", "Hide Center" }; private Border. Layout layout; // set up GUI and event handling public Border. Layout. Demo() { super( "Border. Layout Demo" ); layout = new Border. Layout( 5, 5 ); // 5 pixel gaps // get content pane and set its layout Container container = get. Content. Pane(); container. set. Layout( layout ); Border. Layout. Dem o. java Lines 18 and 22 Set layout as Border. Layout with 5 -pixel horizontal and vertical gaps // instantiate button objects buttons = new JButton[ names. length ]; 2003 Prentice Hall, Inc. All rights reserved.
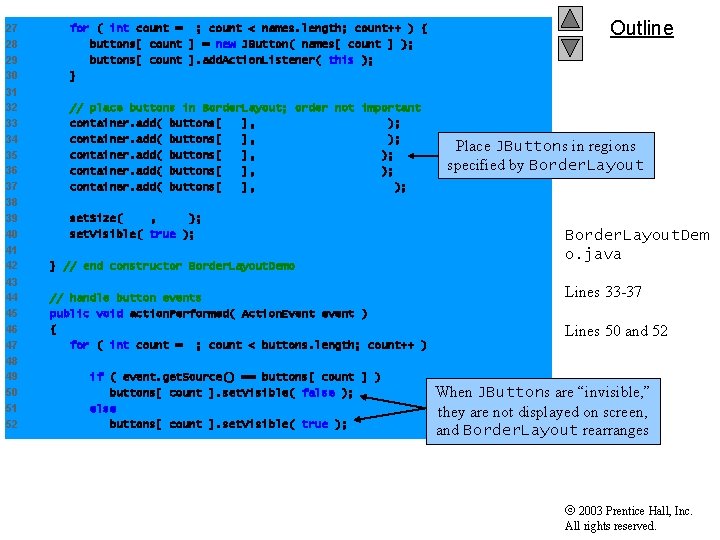
27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 for ( int count = 0; count < names. length; count++ ) { buttons[ count ] = new JButton( names[ count ] ); buttons[ count ]. add. Action. Listener( this ); } // place buttons in Border. Layout; order not important container. add( buttons[ 0 ], Border. Layout. NORTH ); container. add( buttons[ 1 ], Border. Layout. SOUTH ); container. add( buttons[ 2 ], Border. Layout. EAST ); container. add( buttons[ 3 ], Border. Layout. WEST ); container. add( buttons[ 4 ], Border. Layout. CENTER ); set. Size( 300, 200 ); set. Visible( true ); } // end constructor Border. Layout. Demo // handle button events public void action. Performed( Action. Event event ) { for ( int count = 0; count < buttons. length; count++ ) if ( event. get. Source() == buttons[ count ] ) buttons[ count ]. set. Visible( false ); else buttons[ count ]. set. Visible( true ); Outline Place JButtons in regions specified by Border. Layout. Dem o. java Lines 33 -37 Lines 50 and 52 When JButtons are “invisible, ” they are not displayed on screen, and Border. Layout rearranges 2003 Prentice Hall, Inc. All rights reserved.
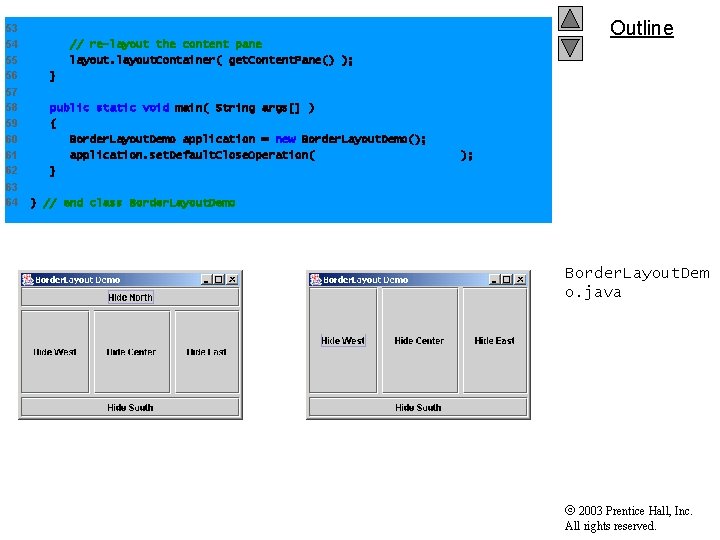
53 54 55 56 57 58 59 60 61 62 63 64 // re-layout the content pane layout. Container( get. Content. Pane() ); Outline } public static void main( String args[] ) { Border. Layout. Demo application = new Border. Layout. Demo(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } } // end class Border. Layout. Demo Border. Layout. Dem o. java 2003 Prentice Hall, Inc. All rights reserved.
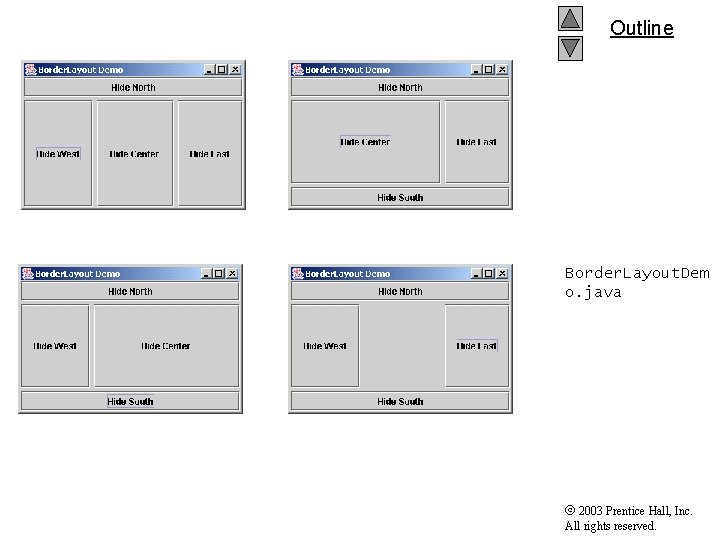
Outline Border. Layout. Dem o. java 2003 Prentice Hall, Inc. All rights reserved.
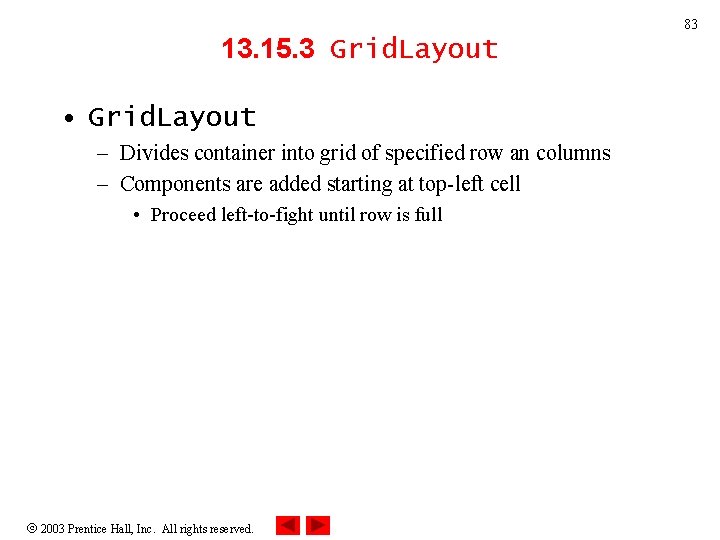
83 13. 15. 3 Grid. Layout • Grid. Layout – Divides container into grid of specified row an columns – Components are added starting at top-left cell • Proceed left-to-fight until row is full 2003 Prentice Hall, Inc. All rights reserved.
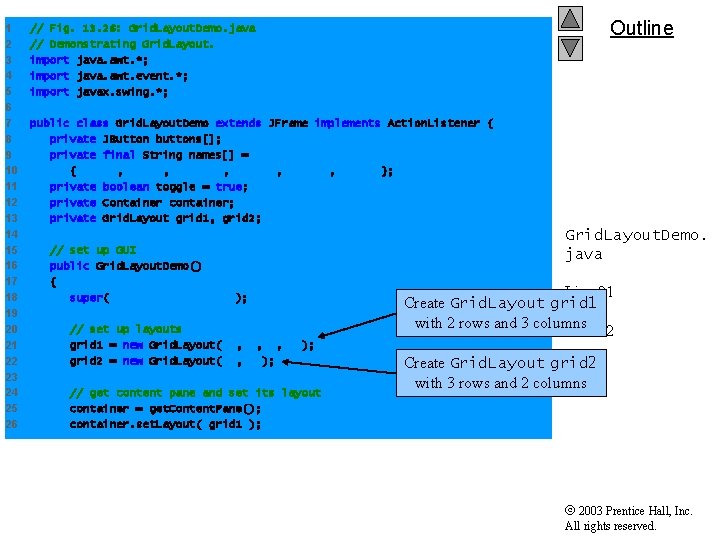
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 Outline // Fig. 13. 26: Grid. Layout. Demo. java // Demonstrating Grid. Layout. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Grid. Layout. Demo extends JFrame implements Action. Listener { private JButton buttons[]; private final String names[] = { "one", "two", "three", "four", "five", "six" }; private boolean toggle = true; private Container container; private Grid. Layout grid 1, grid 2; // set up GUI public Grid. Layout. Demo() { super( "Grid. Layout Demo" ); // set up layouts grid 1 = new Grid. Layout( 2, 3, 5, 5 ); grid 2 = new Grid. Layout( 3, 2 ); // get content pane and set its layout container = get. Content. Pane(); container. set. Layout( grid 1 ); Grid. Layout. Demo. java Line 21 Create Grid. Layout grid 1 with 2 rows and 3 columns Line 22 Create Grid. Layout grid 2 with 3 rows and 2 columns 2003 Prentice Hall, Inc. All rights reserved.
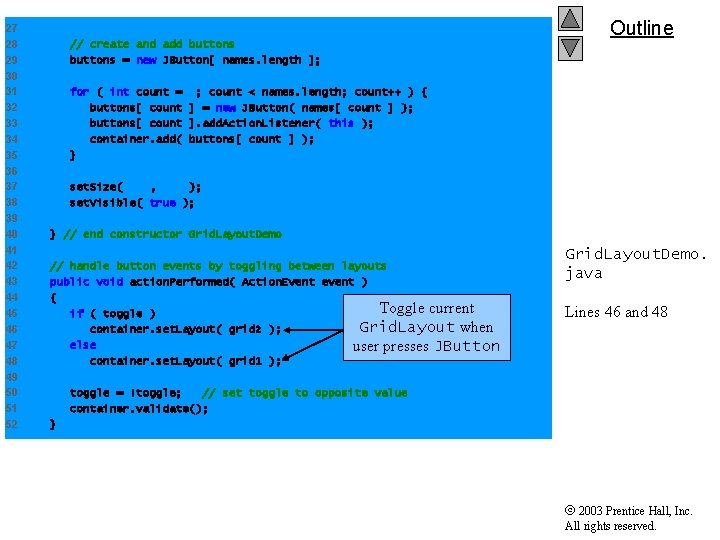
27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 // create and add buttons = new JButton[ names. length ]; for ( int count = buttons[ count container. add( } Outline 0; count < names. length; count++ ) { ] = new JButton( names[ count ] ); ]. add. Action. Listener( this ); buttons[ count ] ); set. Size( 300, 150 ); set. Visible( true ); } // end constructor Grid. Layout. Demo // handle button events by toggling between layouts public void action. Performed( Action. Event event ) { Toggle current if ( toggle ) Grid. Layout when container. set. Layout( grid 2 ); else user presses JButton container. set. Layout( grid 1 ); Grid. Layout. Demo. java Lines 46 and 48 toggle = !toggle; // set toggle to opposite value container. validate(); } 2003 Prentice Hall, Inc. All rights reserved.
![53 54 55 56 57 58 59 60 public static void main String args 53 54 55 56 57 58 59 60 public static void main( String args[]](https://slidetodoc.com/presentation_image_h2/a75e4a64e3dd0a25bd045296b3dce9f9/image-86.jpg)
53 54 55 56 57 58 59 60 public static void main( String args[] ) { Grid. Layout. Demo application = new Grid. Layout. Demo(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } Outline } // end class Grid. Layout. Demo. java 2003 Prentice Hall, Inc. All rights reserved.
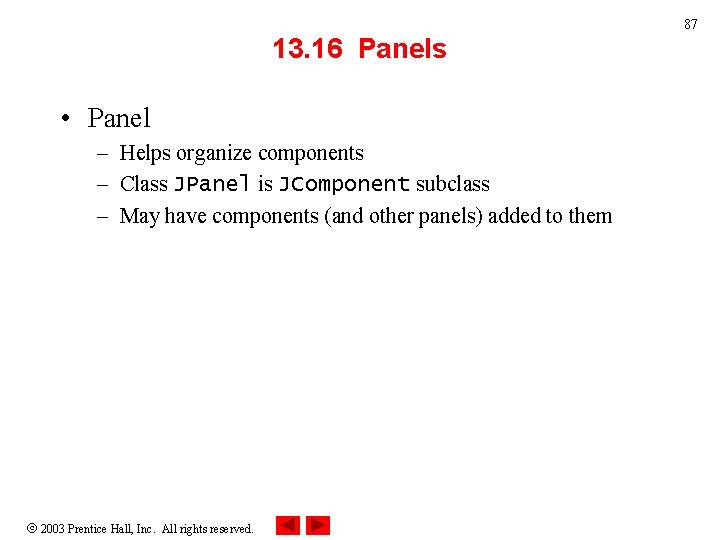
87 13. 16 Panels • Panel – Helps organize components – Class JPanel is JComponent subclass – May have components (and other panels) added to them 2003 Prentice Hall, Inc. All rights reserved.
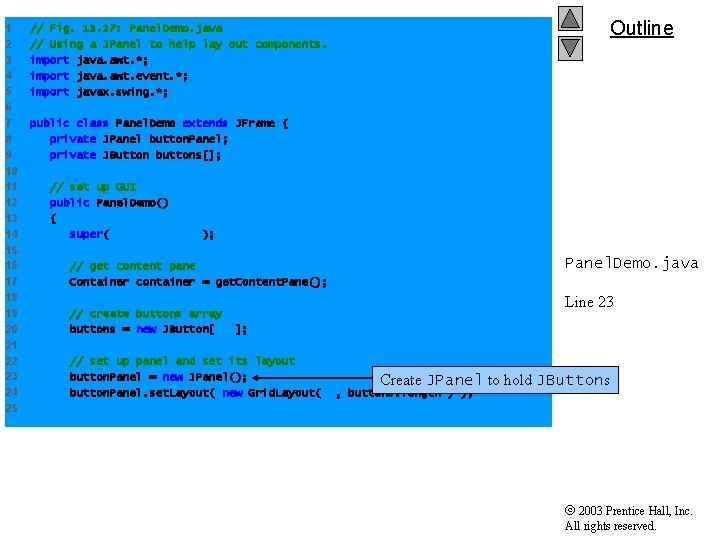
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 Outline // Fig. 13. 27: Panel. Demo. java // Using a JPanel to help lay out components. import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Panel. Demo extends JFrame { private JPanel button. Panel; private JButton buttons[]; // set up GUI public Panel. Demo() { super( "Panel Demo" ); // get content pane Container container = get. Content. Pane(); // create buttons array buttons = new JButton[ 5 ]; // set up panel and set its layout button. Panel = new JPanel(); Create JPanel button. Panel. set. Layout( new Grid. Layout( 1, buttons. length ) ); Panel. Demo. java Line 23 to hold JButtons 2003 Prentice Hall, Inc. All rights reserved.
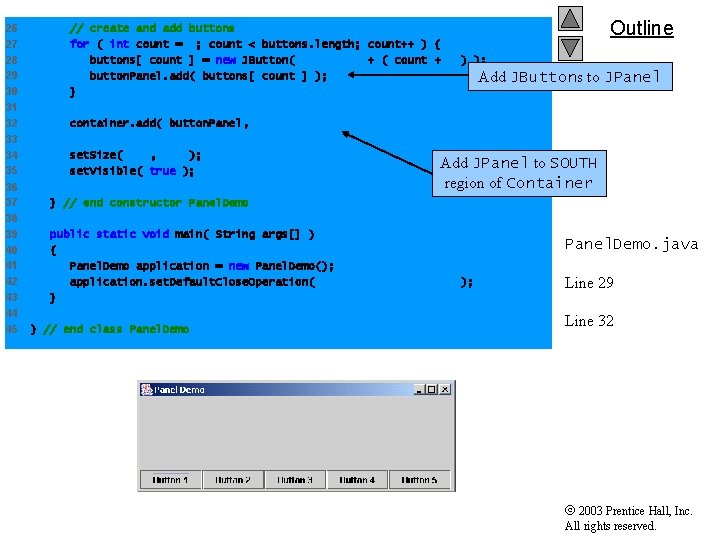
26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 // create and add buttons for ( int count = 0; count < buttons. length; count++ ) { buttons[ count ] = new JButton( "Button " + ( count + 1 ) ); button. Panel. add( buttons[ count ] ); Add } Outline JButtons to JPanel container. add( button. Panel, Border. Layout. SOUTH ); set. Size( 425, 150 ); set. Visible( true ); Add JPanel to SOUTH region of Container } // end constructor Panel. Demo public static void main( String args[] ) { Panel. Demo application = new Panel. Demo(); application. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } } // end class Panel. Demo. java Line 29 Line 32 2003 Prentice Hall, Inc. All rights reserved.
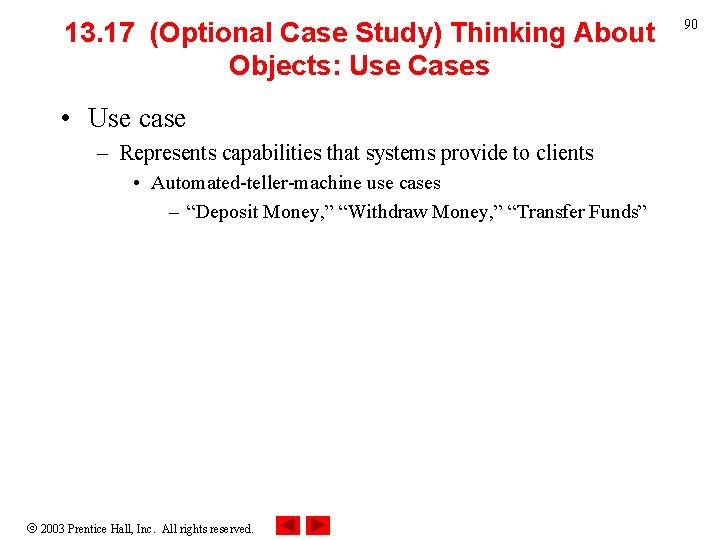
13. 17 (Optional Case Study) Thinking About Objects: Use Cases • Use case – Represents capabilities that systems provide to clients • Automated-teller-machine use cases – “Deposit Money, ” “Withdraw Money, ” “Transfer Funds” 2003 Prentice Hall, Inc. All rights reserved. 90
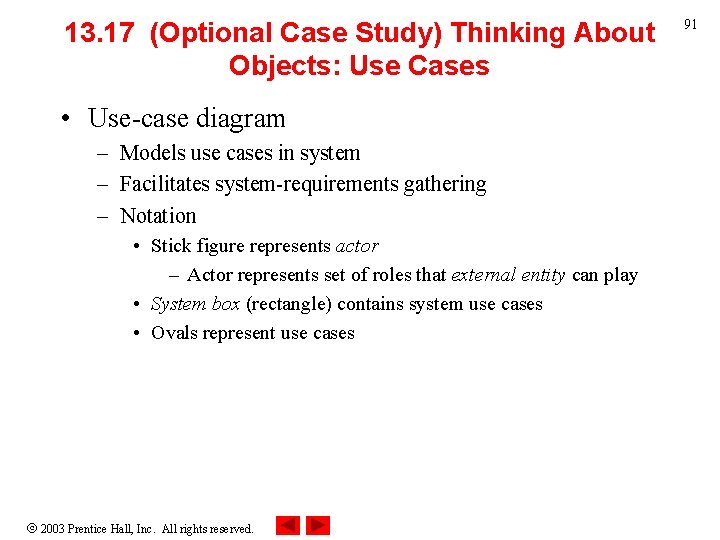
13. 17 (Optional Case Study) Thinking About Objects: Use Cases • Use-case diagram – Models use cases in system – Facilitates system-requirements gathering – Notation • Stick figure represents actor – Actor represents set of roles that external entity can play • System box (rectangle) contains system use cases • Ovals represent use cases 2003 Prentice Hall, Inc. All rights reserved. 91
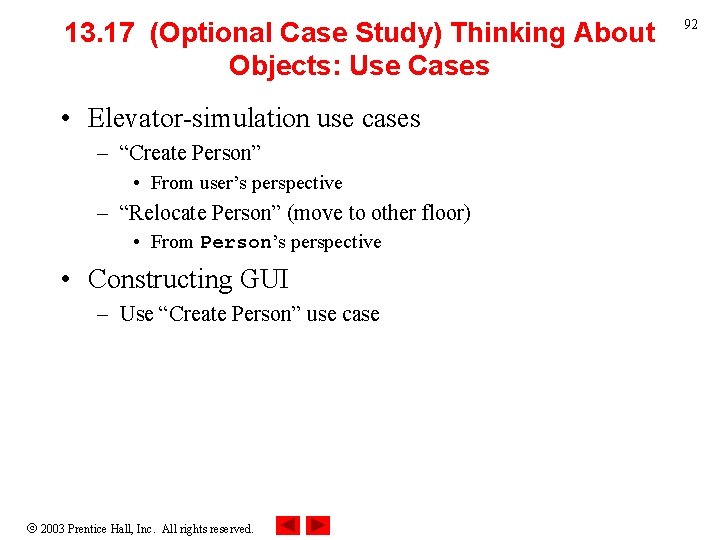
13. 17 (Optional Case Study) Thinking About Objects: Use Cases • Elevator-simulation use cases – “Create Person” • From user’s perspective – “Relocate Person” (move to other floor) • From Person’s perspective • Constructing GUI – Use “Create Person” use case 2003 Prentice Hall, Inc. All rights reserved. 92
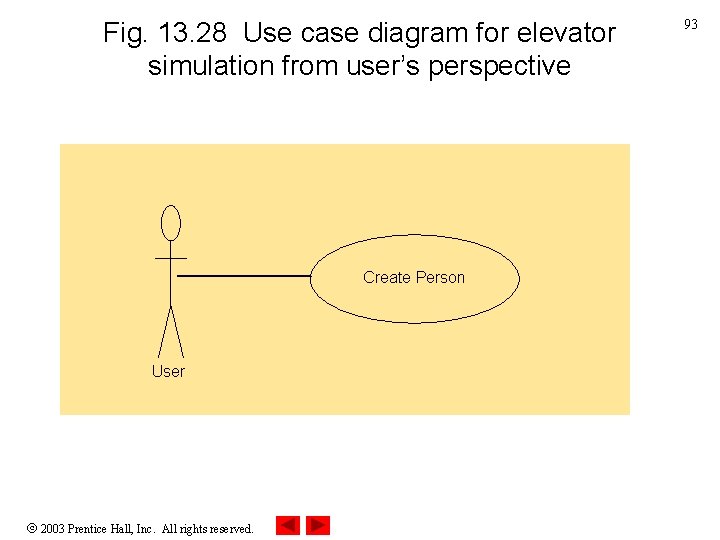
Fig. 13. 28 Use case diagram for elevator simulation from user’s perspective Create Person User 2003 Prentice Hall, Inc. All rights reserved. 93
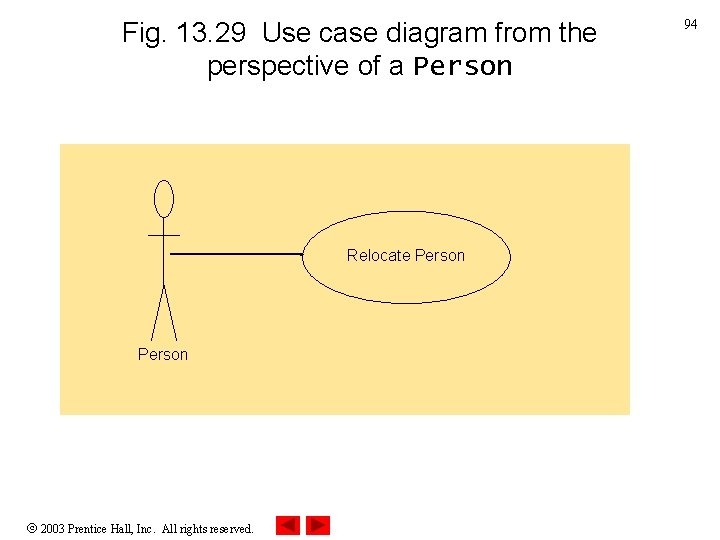
Fig. 13. 29 Use case diagram from the perspective of a Person Relocate Person 2003 Prentice Hall, Inc. All rights reserved. 94
Components of graphical user interface
Graphical user interface testing
Guidelines for data entry screen design
Principles of user interface design
What is gui
Java user interface
History of user interface
Characteristics of web interface
Gui meaning
Characteristics of graphical user interface
Graphical user interface in data structures
Characteristics of web user interface
User interface history
4 komponen dasar pembentuk user interface
Metasploit graphical interface
Grafika bmp
Idscenter
Graphical device interface with c
Ruby on rails gui
Programming graphical user interfaces in r
What is interface in java
Areas of the screen that behave as if they were independent
Office interface vs industrial interface
An interface
Yahoo user interface
Xml user interface language
Komponen user interface
Enterprise architect interface diagram
User interface design cycle
User interface importance
User interface design steps in software engineering
User interface analysis and design
Touchless touchscreen user interface
Interactible
User interface theory
What is mdi
Dibawah ini adalah komponen antar muka grafis, kecuali
Hci user interface
User interface feedback
Cognitive walkthrough vs heuristic evaluation
User interface and its types
Atm user interface design
User interface instagram
User interface design and evaluation
User interface design in system analysis and design
Interface in software engineering
User interface revit
Human interface designer
Gui is an interface between
User interface toolkit
User interface design principles in software engineering
Apa pengertian antarmuka pemakai (user interface)
User interface solutions
Python gui design
Dialog dalam konteks perancangan user interface adalah
Visualization in user interface design
Backoffice design
Frontend user interface
Recoverability in user interface design
What are the objectives of input design
Prinsip dasar user interface
User interface uses the fewest system resources:
What is os user interface
User interface design process in software engineering
Windows 7 user interface
User interface structure
Interface structure design
User interface process application block
User interface prototyping in software engineering
Task centered user interface design
User interface design in software engineering
Mengapa sistem embedded harus memiliki kehandalan sistem
Nui natural user interface
User interface hall of shame
Ben shneiderman designing the user interface
Interface between user and computer
Labview user interface design examples
Pengertian user interface
Supplier self service
Character user interface
5 tipe utama interaksi user interface
In the context of user interface designs, a heuristic is
Voice user interface
Gui characteristics
User interface design process in software engineering
Coherent ui a modern user interface
Komponen user interface
Ajax gui
Fitts law user interface design
Single user and multi user operating system
Rtos multitasking
Customer interface in business model
Part part whole addition
Unit ratio definition
Brainpop ratios