Ch 23 Interpreter Pattern Ch 23 Interpreter Pattern
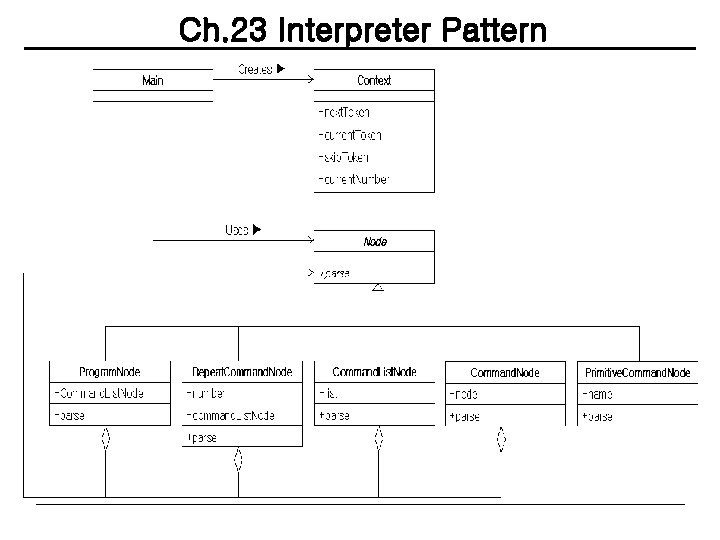
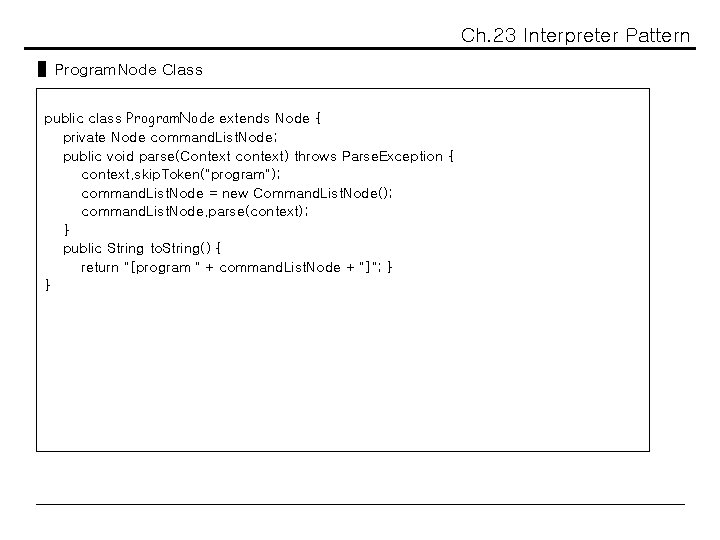
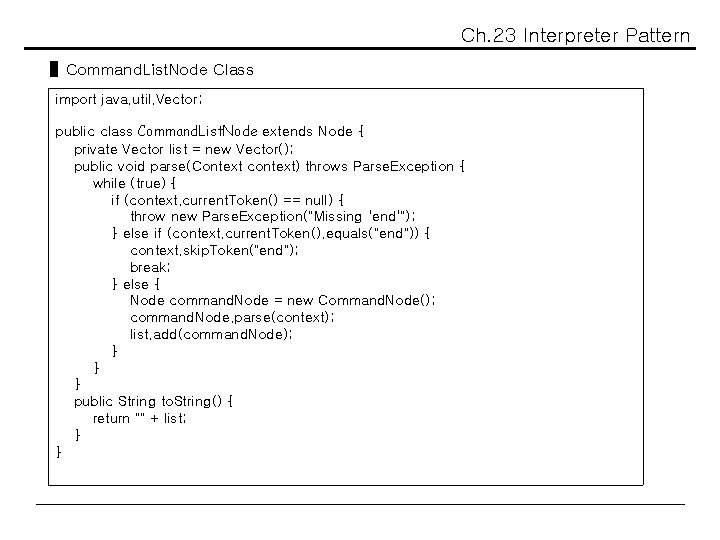
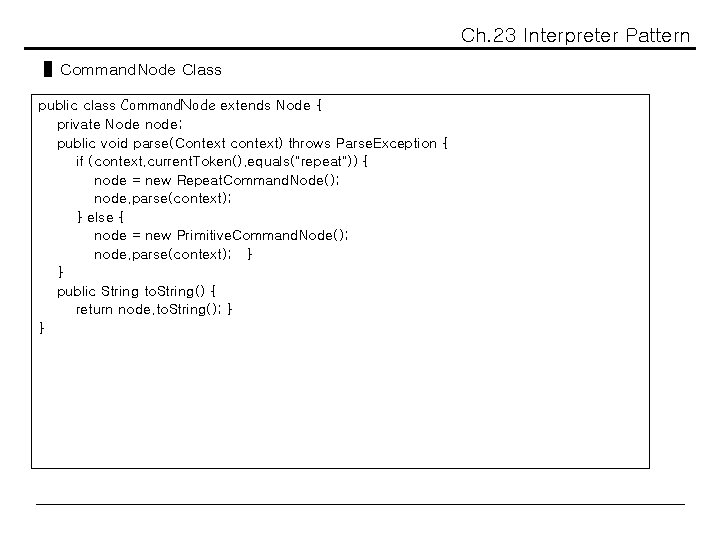
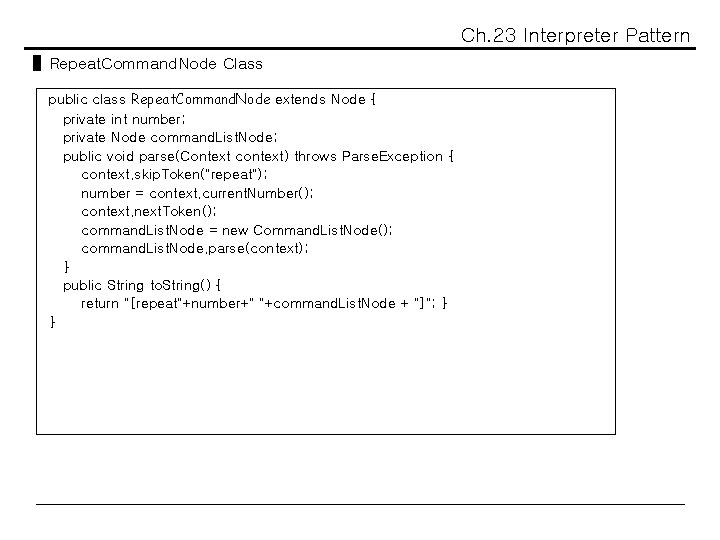
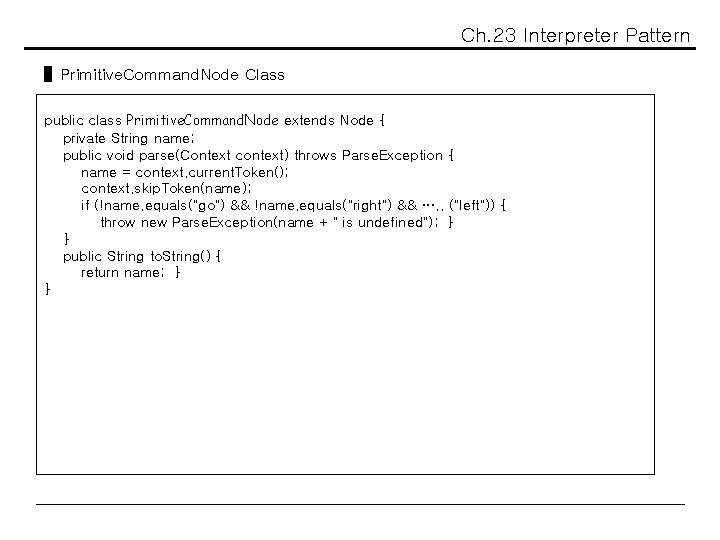
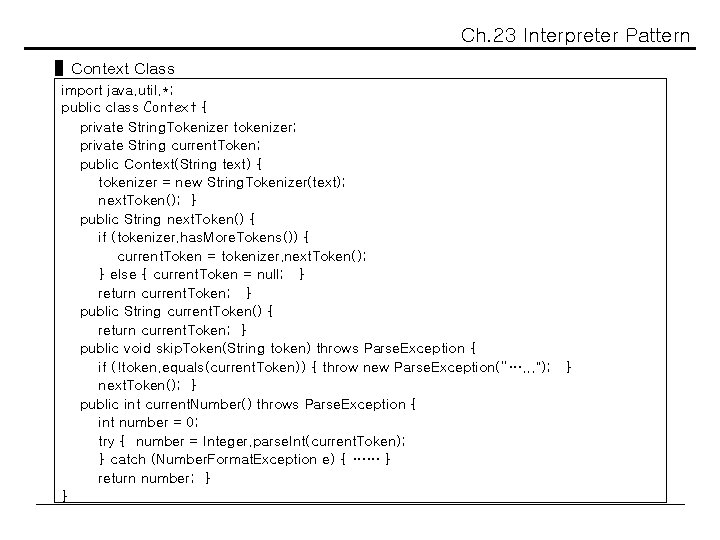
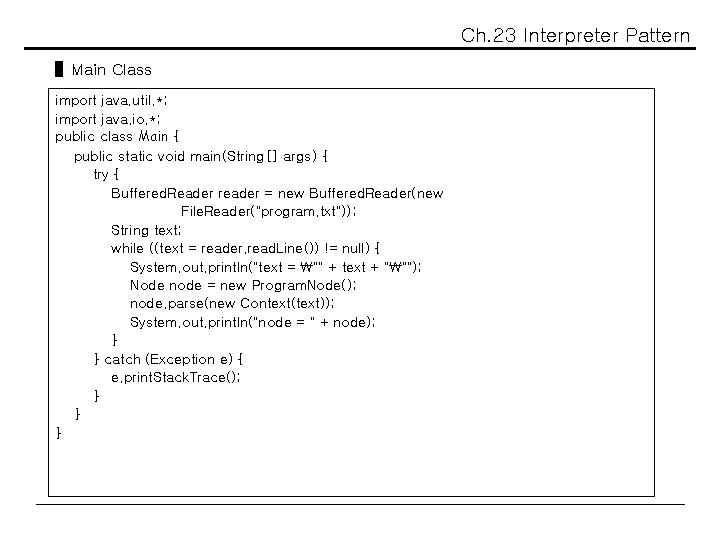
- Slides: 8
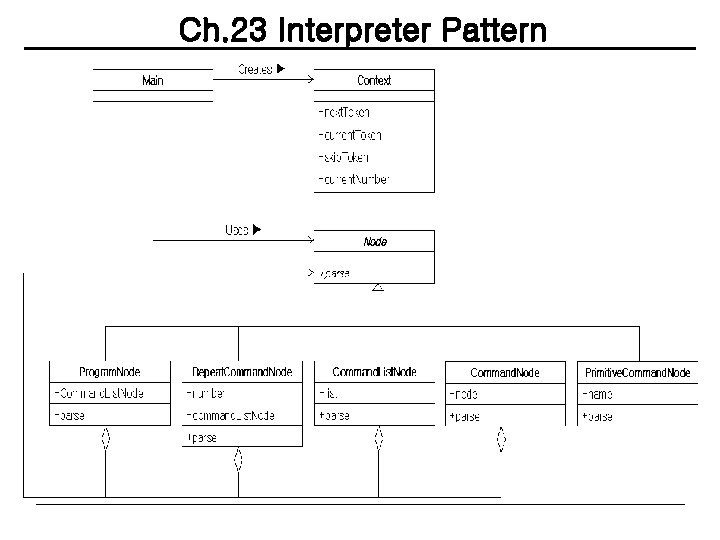
Ch. 23 Interpreter Pattern
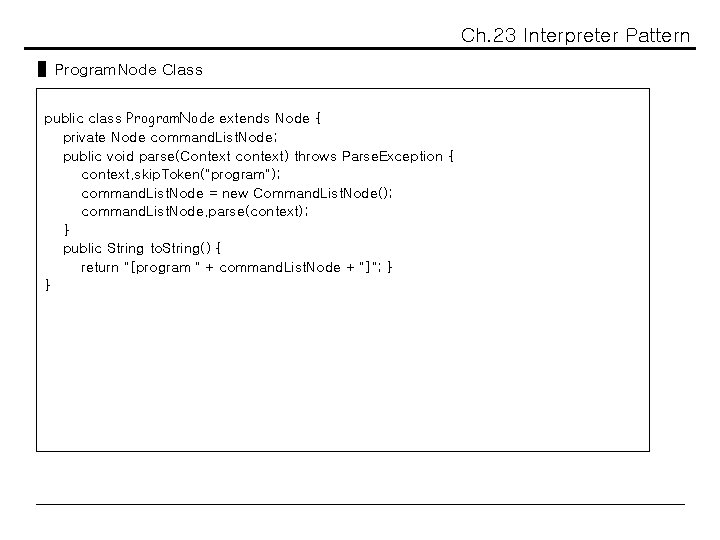
Ch. 23 Interpreter Pattern ▌Program. Node Class public class Program. Node extends Node { private Node command. List. Node; public void parse(Context context) throws Parse. Exception { context. skip. Token("program"); command. List. Node = new Command. List. Node(); command. List. Node. parse(context); } public String to. String() { return "[program " + command. List. Node + "]"; } }
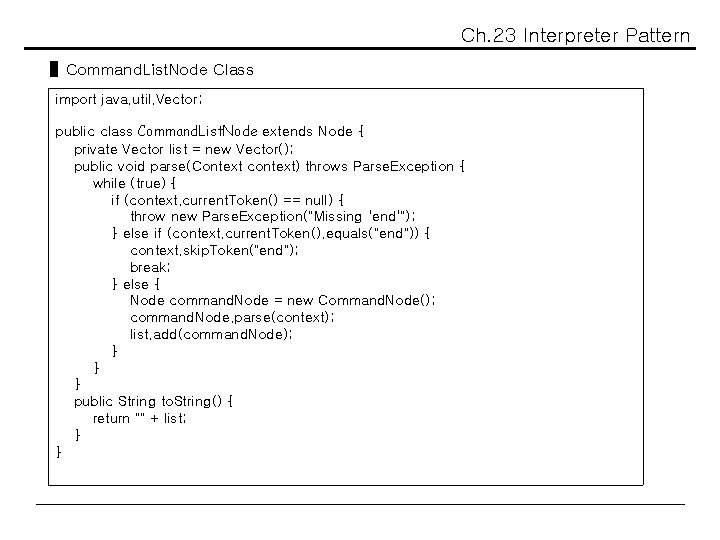
Ch. 23 Interpreter Pattern ▌Command. List. Node Class import java. util. Vector; public class Command. List. Node extends Node { private Vector list = new Vector(); public void parse(Context context) throws Parse. Exception { while (true) { if (context. current. Token() == null) { throw new Parse. Exception("Missing 'end'"); } else if (context. current. Token(). equals("end")) { context. skip. Token("end"); break; } else { Node command. Node = new Command. Node(); command. Node. parse(context); list. add(command. Node); } } } public String to. String() { return "" + list; } }
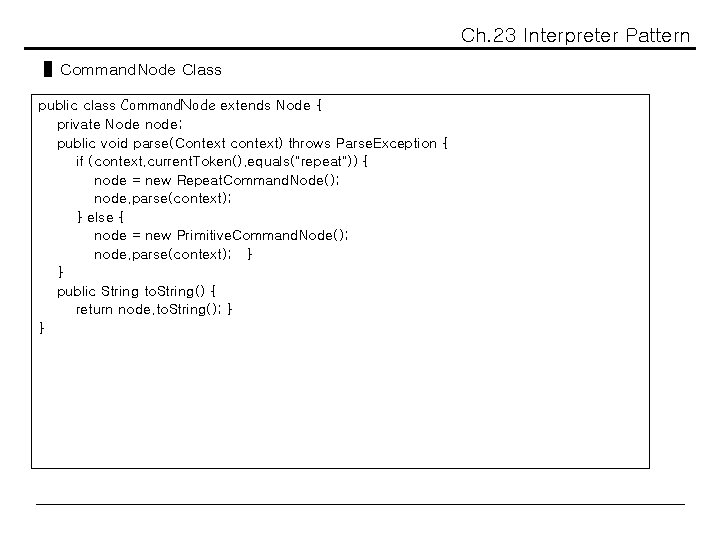
Ch. 23 Interpreter Pattern ▌Command. Node Class public class Command. Node extends Node { private Node node; public void parse(Context context) throws Parse. Exception { if (context. current. Token(). equals("repeat")) { node = new Repeat. Command. Node(); node. parse(context); } else { node = new Primitive. Command. Node(); node. parse(context); } } public String to. String() { return node. to. String(); } }
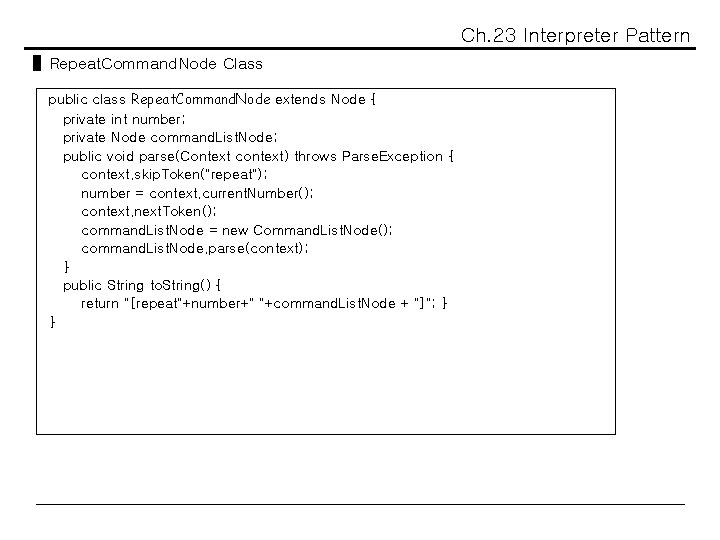
Ch. 23 Interpreter Pattern ▌Repeat. Command. Node Class public class Repeat. Command. Node extends Node { private int number; private Node command. List. Node; public void parse(Context context) throws Parse. Exception { context. skip. Token("repeat"); number = context. current. Number(); context. next. Token(); command. List. Node = new Command. List. Node(); command. List. Node. parse(context); } public String to. String() { return "[repeat"+number+" "+command. List. Node + "]"; } }
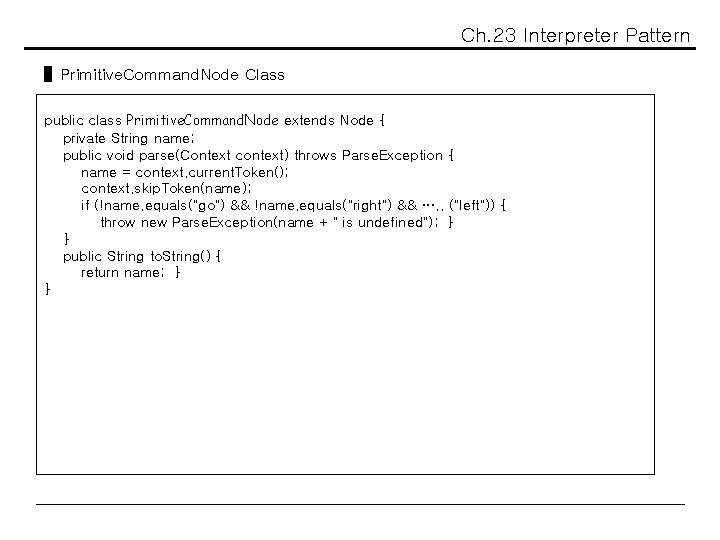
Ch. 23 Interpreter Pattern ▌Primitive. Command. Node Class public class Primitive. Command. Node extends Node { private String name; public void parse(Context context) throws Parse. Exception { name = context. current. Token(); context. skip. Token(name); if (!name. equals("go") && !name. equals("right") && …. . ("left")) { throw new Parse. Exception(name + " is undefined"); } } public String to. String() { return name; } }
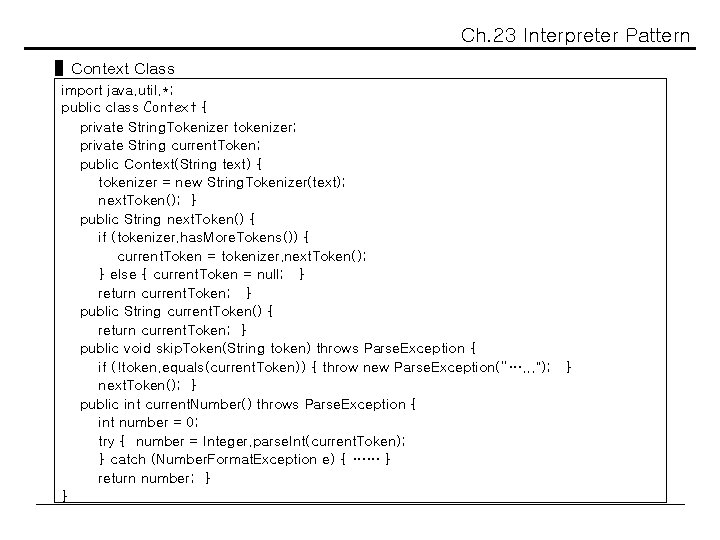
Ch. 23 Interpreter Pattern ▌Context Class import java. util. *; public class Context { private String. Tokenizer tokenizer; private String current. Token; public Context(String text) { tokenizer = new String. Tokenizer(text); next. Token(); } public String next. Token() { if (tokenizer. has. More. Tokens()) { current. Token = tokenizer. next. Token(); } else { current. Token = null; } return current. Token; } public String current. Token() { return current. Token; } public void skip. Token(String token) throws Parse. Exception { if (!token. equals(current. Token)) { throw new Parse. Exception(“…. . . "); } next. Token(); } public int current. Number() throws Parse. Exception { int number = 0; try { number = Integer. parse. Int(current. Token); } catch (Number. Format. Exception e) { …… } return number; } }
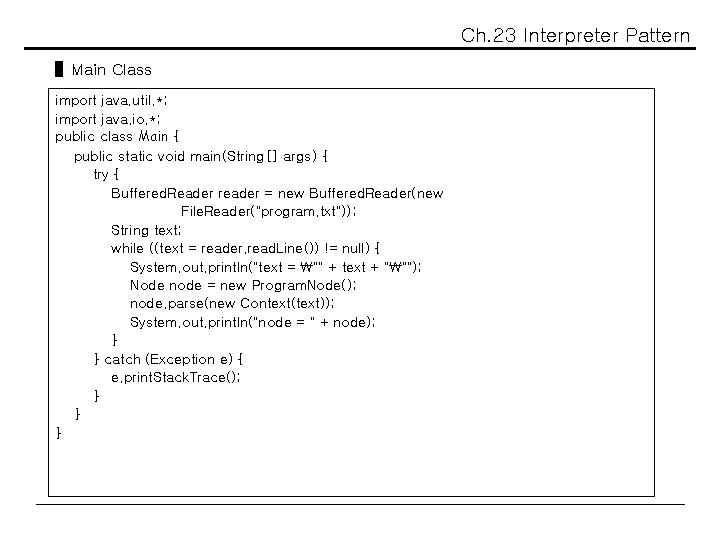
Ch. 23 Interpreter Pattern ▌Main Class import java. util. *; import java. io. *; public class Main { public static void main(String[] args) { try { Buffered. Reader reader = new Buffered. Reader(new File. Reader("program. txt")); String text; while ((text = reader. read. Line()) != null) { System. out. println("text = "" + text + """); Node node = new Program. Node(); node. parse(new Context(text)); System. out. println("node = " + node); } } catch (Exception e) { e. print. Stack. Trace(); } } }