Ch 19 State Pattern Ch 19 State Pattern
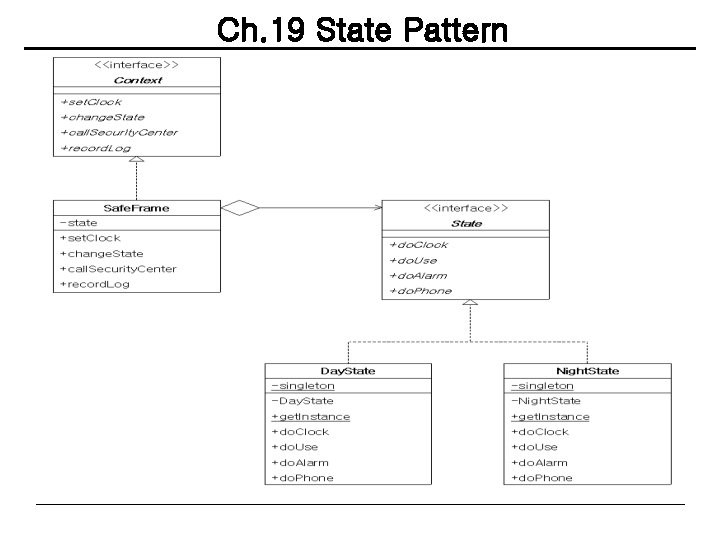
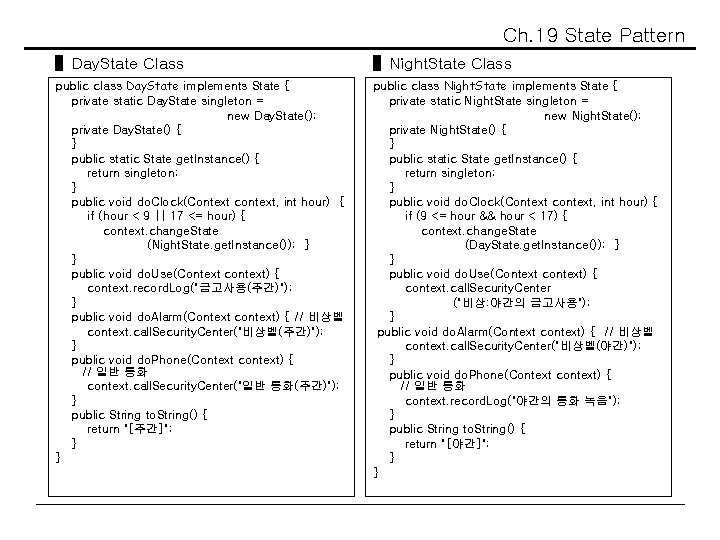
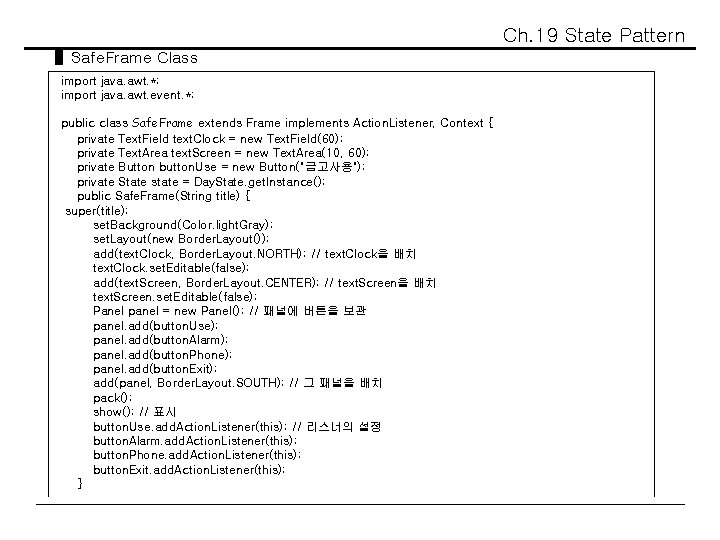
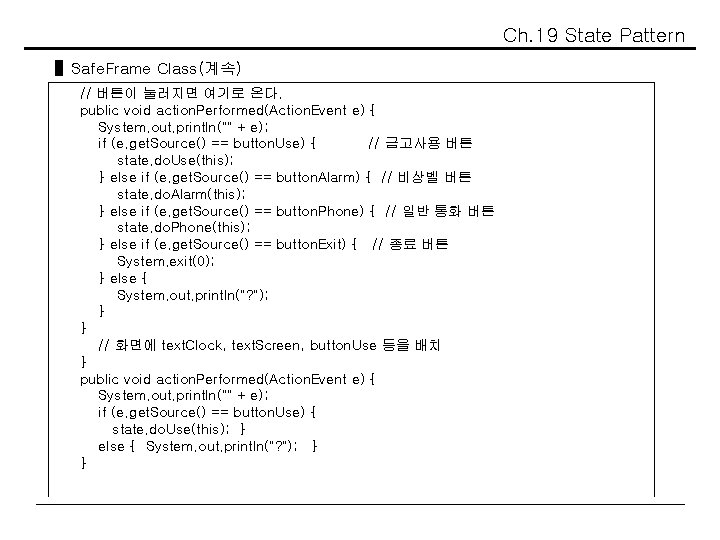
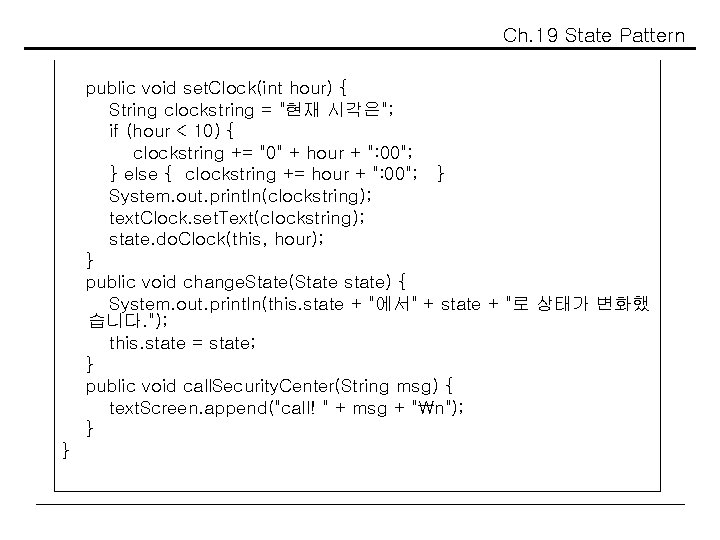
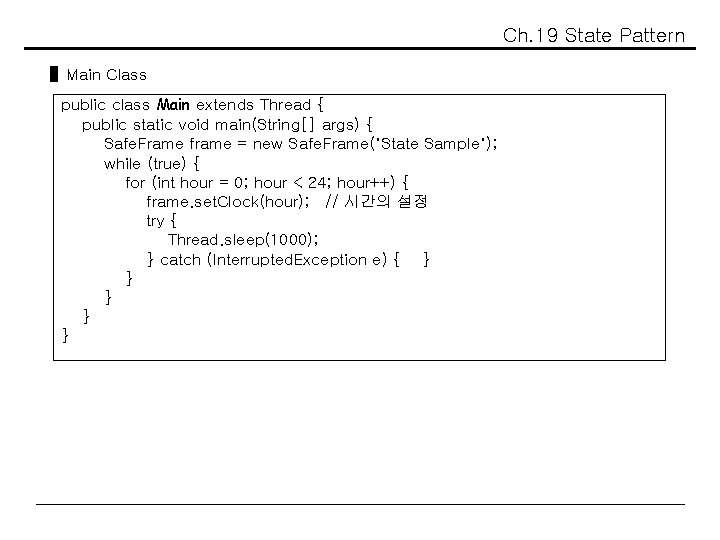
- Slides: 6
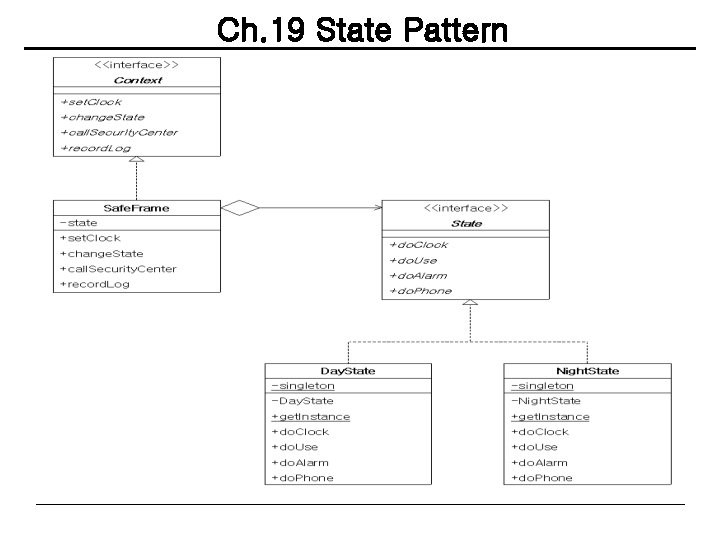
Ch. 19 State Pattern
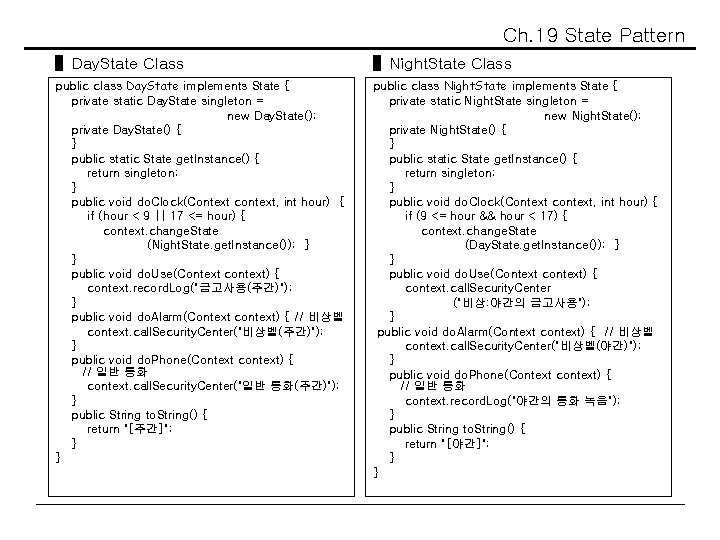
Ch. 19 State Pattern ▌Day. State Class ▌Night. State Class public class Day. State implements State { private static Day. State singleton = new Day. State(); private Day. State() { } public static State get. Instance() { return singleton; } public void do. Clock(Context context, int hour) { if (hour < 9 || 17 <= hour) { context. change. State (Night. State. get. Instance()); } } public void do. Use(Context context) { context. record. Log("금고사용(주간)"); } public void do. Alarm(Context context) { // 비상벨 context. call. Security. Center("비상벨(주간)"); } public void do. Phone(Context context) { // 일반 통화 context. call. Security. Center("일반 통화(주간)"); } public String to. String() { return "[주간]"; } } public class Night. State implements State { private static Night. State singleton = new Night. State(); private Night. State() { } public static State get. Instance() { return singleton; } public void do. Clock(Context context, int hour) { if (9 <= hour && hour < 17) { context. change. State (Day. State. get. Instance()); } } public void do. Use(Context context) { context. call. Security. Center ("비상: 야간의 금고사용"); } public void do. Alarm(Context context) { // 비상벨 context. call. Security. Center("비상벨(야간)"); } public void do. Phone(Context context) { // 일반 통화 context. record. Log("야간의 통화 녹음"); } public String to. String() { return "[야간]"; } }
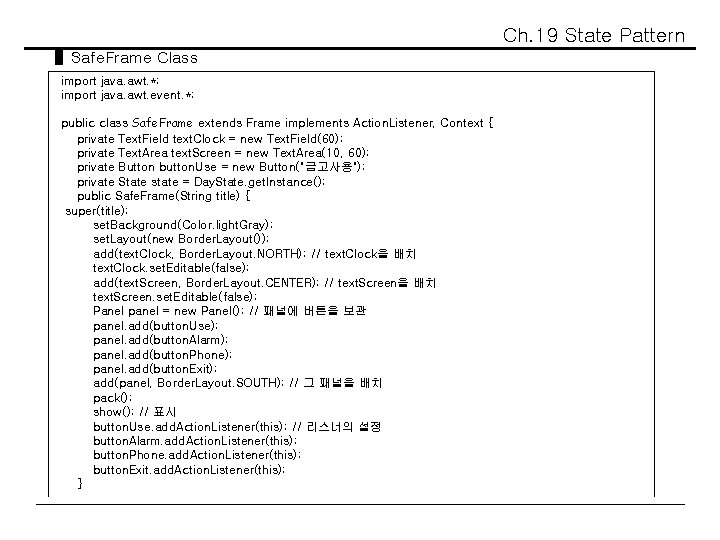
Ch. 19 State Pattern ▌Safe. Frame Class import java. awt. *; import java. awt. event. *; public class Safe. Frame extends Frame implements Action. Listener, Context { private Text. Field text. Clock = new Text. Field(60); private Text. Area text. Screen = new Text. Area(10, 60); private Button button. Use = new Button("금고사용"); private State state = Day. State. get. Instance(); public Safe. Frame(String title) { super(title); set. Background(Color. light. Gray); set. Layout(new Border. Layout()); add(text. Clock, Border. Layout. NORTH); // text. Clock을 배치 text. Clock. set. Editable(false); add(text. Screen, Border. Layout. CENTER); // text. Screen을 배치 text. Screen. set. Editable(false); Panel panel = new Panel(); // 패널에 버튼을 보관 panel. add(button. Use); panel. add(button. Alarm); panel. add(button. Phone); panel. add(button. Exit); add(panel, Border. Layout. SOUTH); // 그 패널을 배치 pack(); show(); // 표시 button. Use. add. Action. Listener(this); // 리스너의 설정 button. Alarm. add. Action. Listener(this); button. Phone. add. Action. Listener(this); button. Exit. add. Action. Listener(this); }
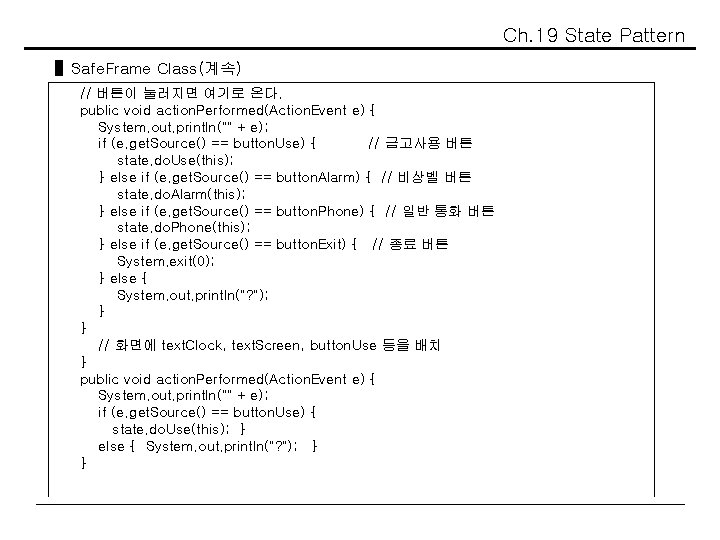
Ch. 19 State Pattern ▌Safe. Frame Class(계속) // 버튼이 눌러지면 여기로 온다. public void action. Performed(Action. Event e) { System. out. println("" + e); if (e. get. Source() == button. Use) { // 금고사용 버튼 state. do. Use(this); } else if (e. get. Source() == button. Alarm) { // 비상벨 버튼 state. do. Alarm(this); } else if (e. get. Source() == button. Phone) { // 일반 통화 버튼 state. do. Phone(this); } else if (e. get. Source() == button. Exit) { // 종료 버튼 System. exit(0); } else { System. out. println("? "); } } // 화면에 text. Clock, text. Screen, button. Use 등을 배치 } public void action. Performed(Action. Event e) { System. out. println("" + e); if (e. get. Source() == button. Use) { state. do. Use(this); } else { System. out. println("? "); } }
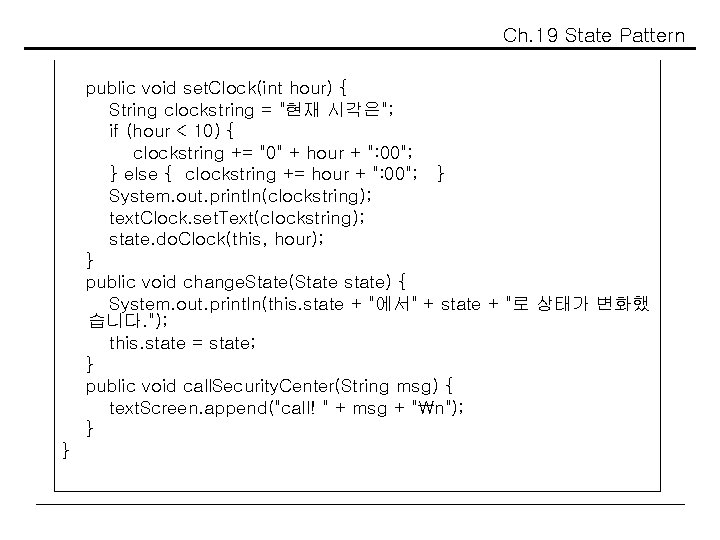
Ch. 19 State Pattern public void set. Clock(int hour) { String clockstring = "현재 시각은"; if (hour < 10) { clockstring += "0" + hour + ": 00"; } else { clockstring += hour + ": 00"; } System. out. println(clockstring); text. Clock. set. Text(clockstring); state. do. Clock(this, hour); } public void change. State(State state) { System. out. println(this. state + "에서" + state + "로 상태가 변화했 습니다. "); this. state = state; } public void call. Security. Center(String msg) { text. Screen. append("call! " + msg + "n"); } }
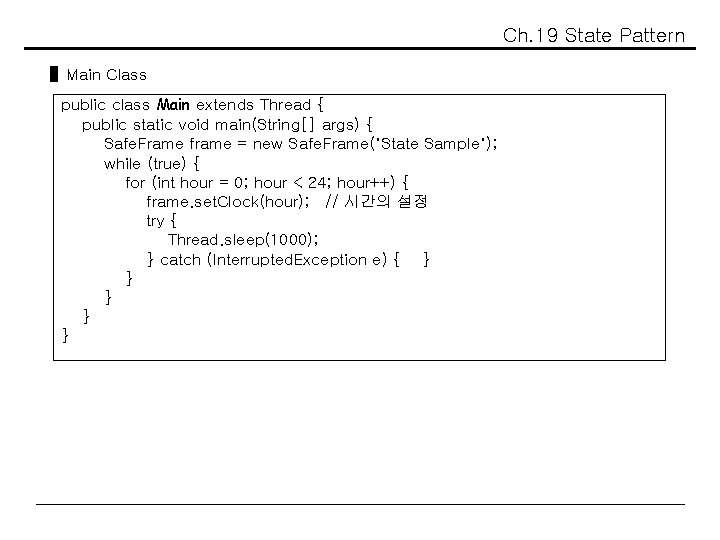
Ch. 19 State Pattern ▌Main Class public class Main extends Thread { public static void main(String[] args) { Safe. Frame frame = new Safe. Frame("State Sample"); while (true) { for (int hour = 0; hour < 24; hour++) { frame. set. Clock(hour); // 시간의 설정 try { Thread. sleep(1000); } catch (Interrupted. Exception e) { } } }