C First Steps C First Steps Overview General
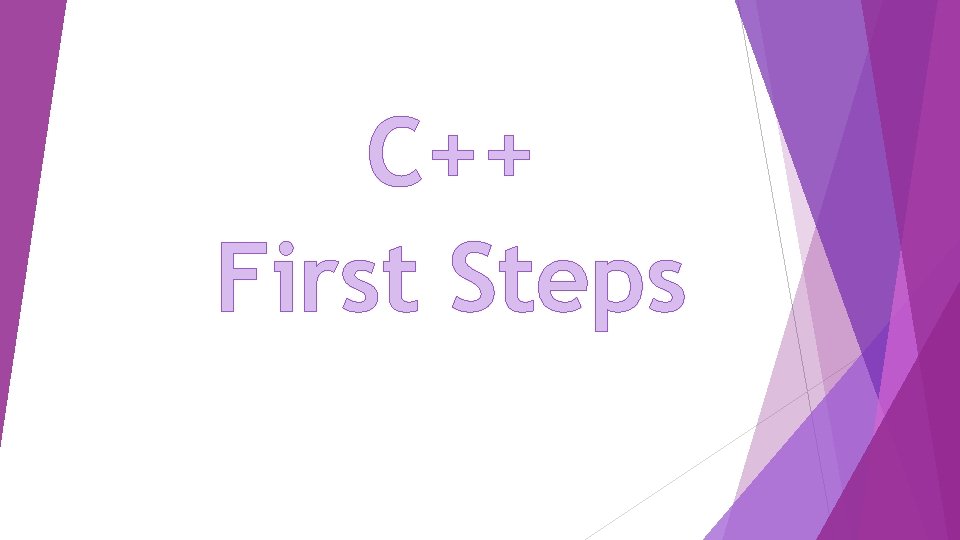
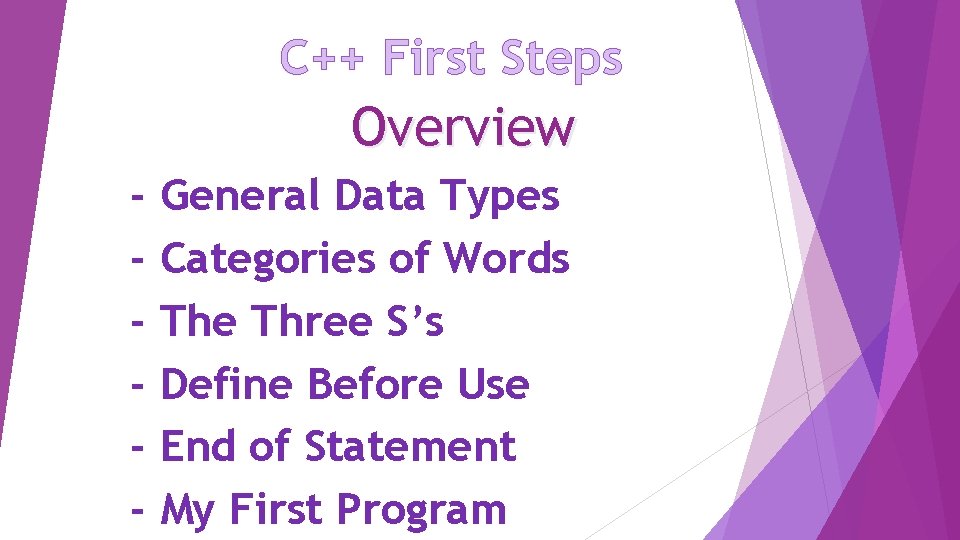
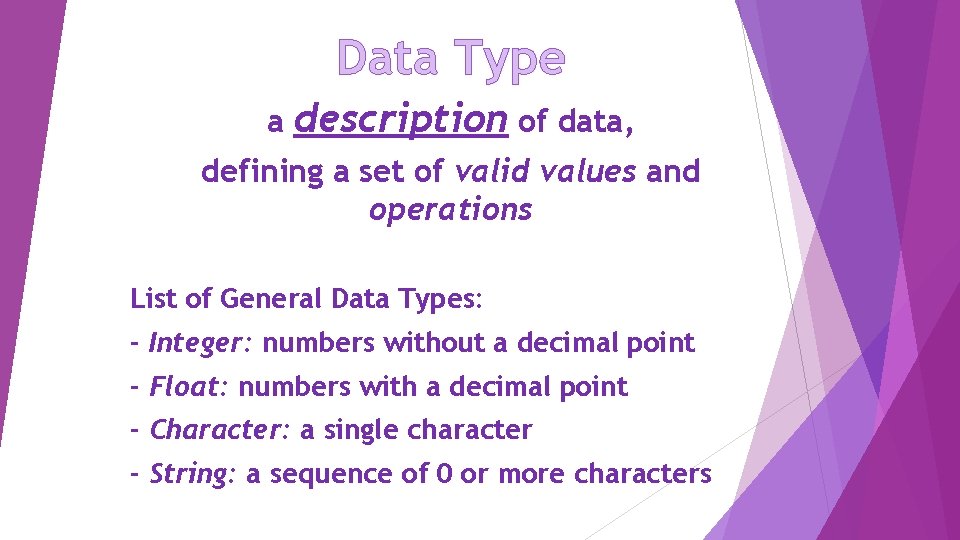
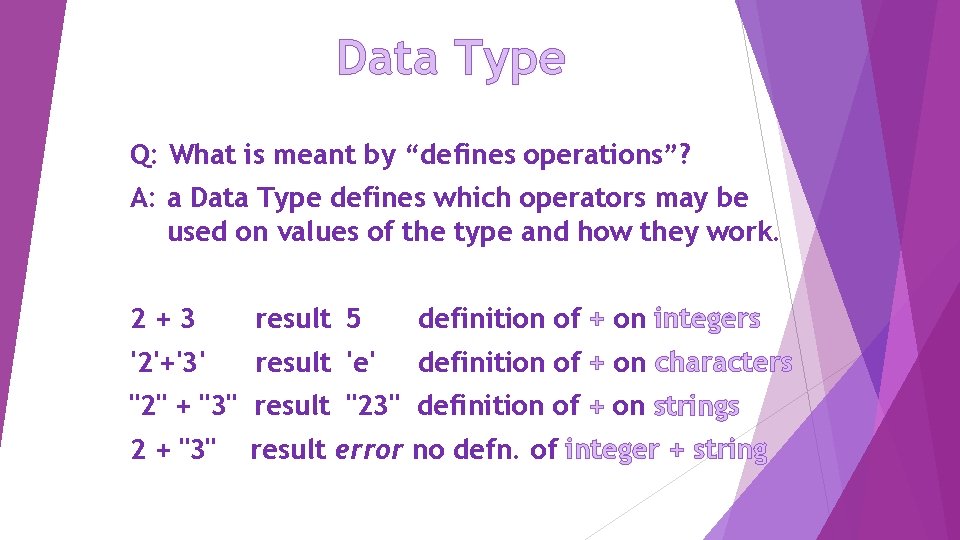
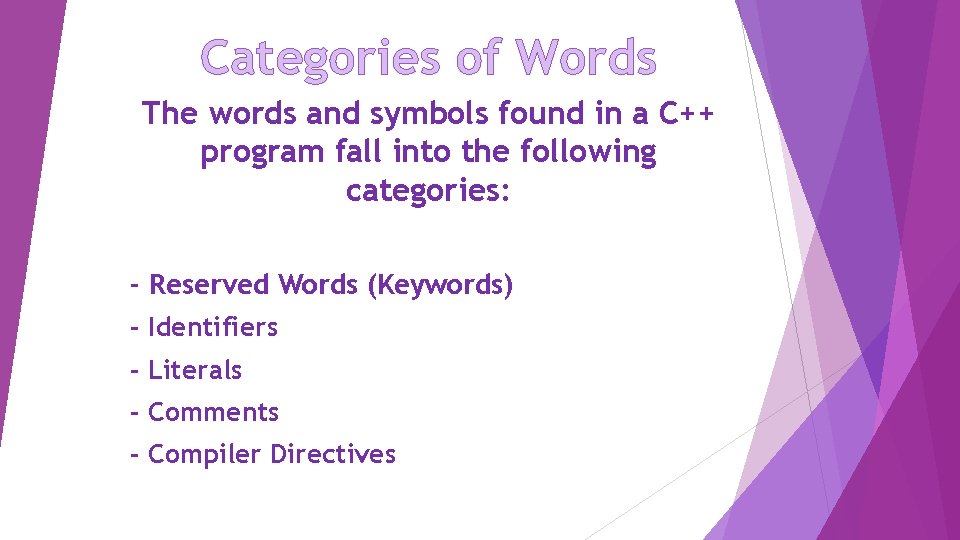
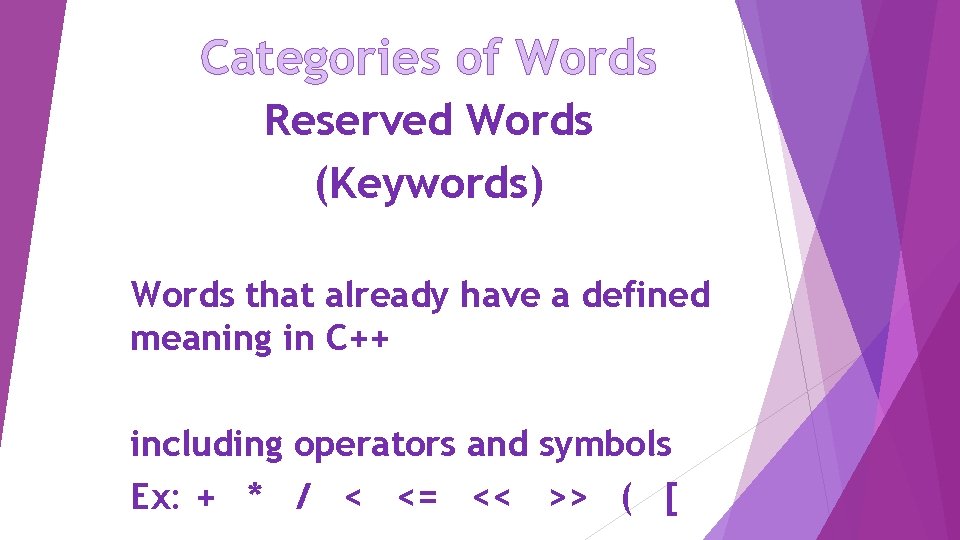
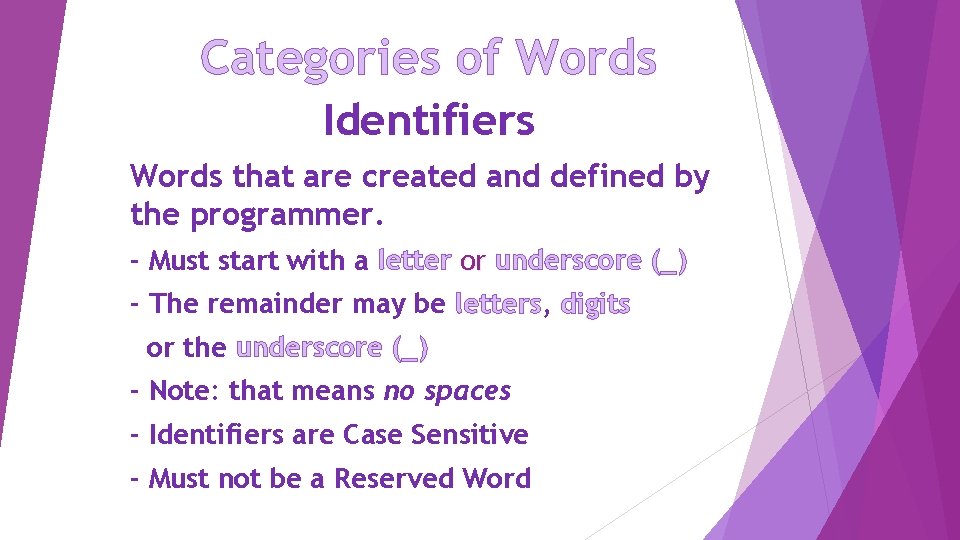
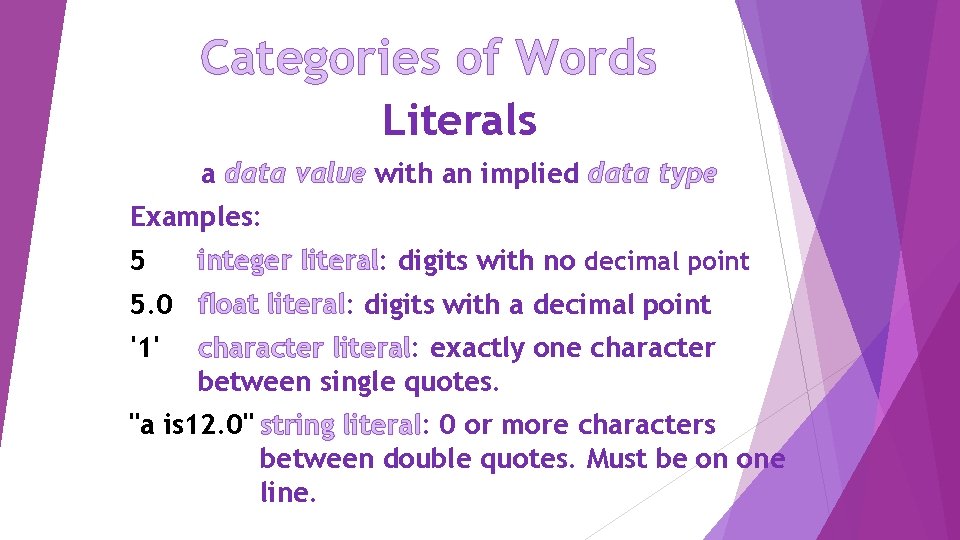
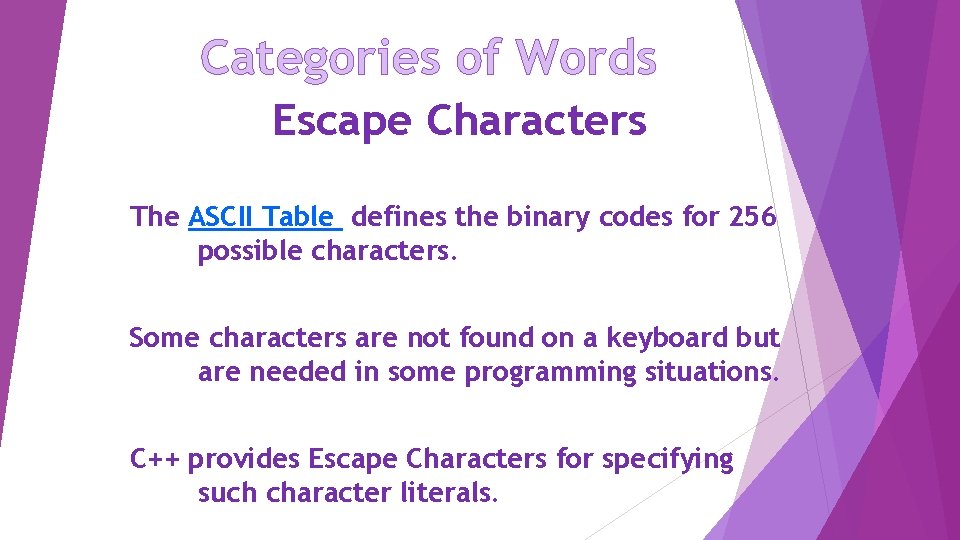
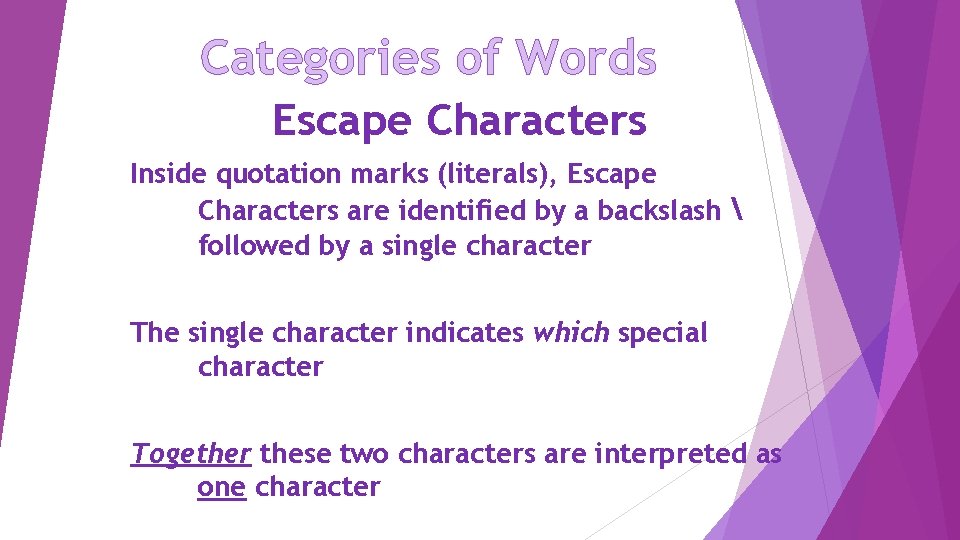
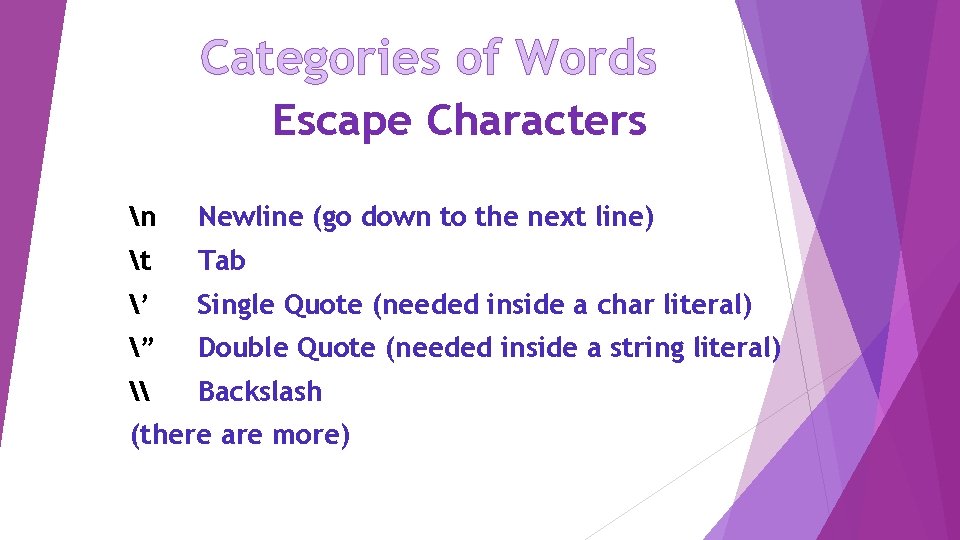
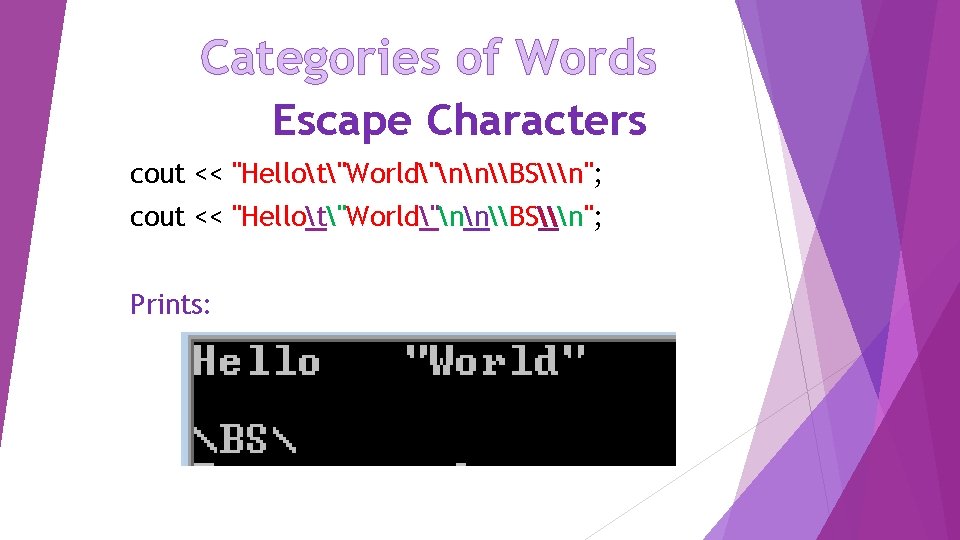
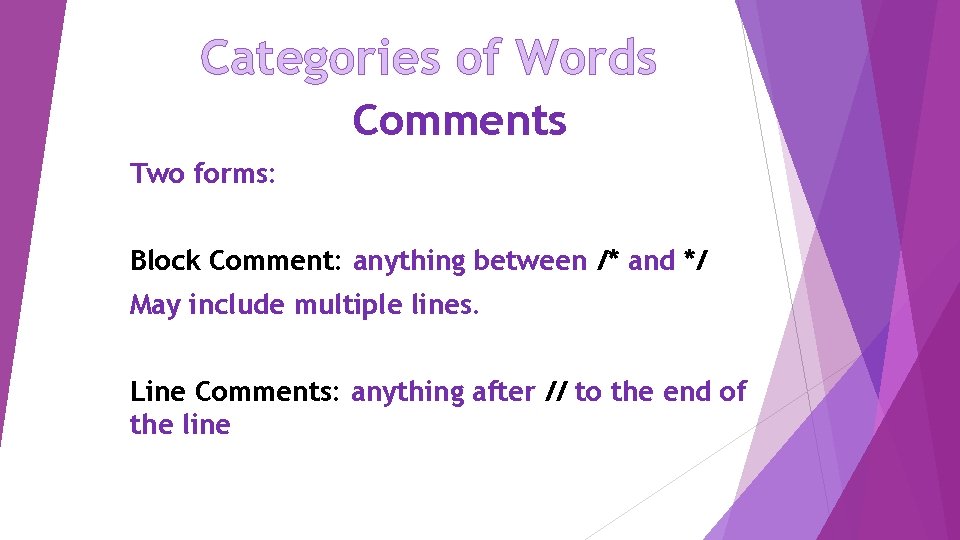
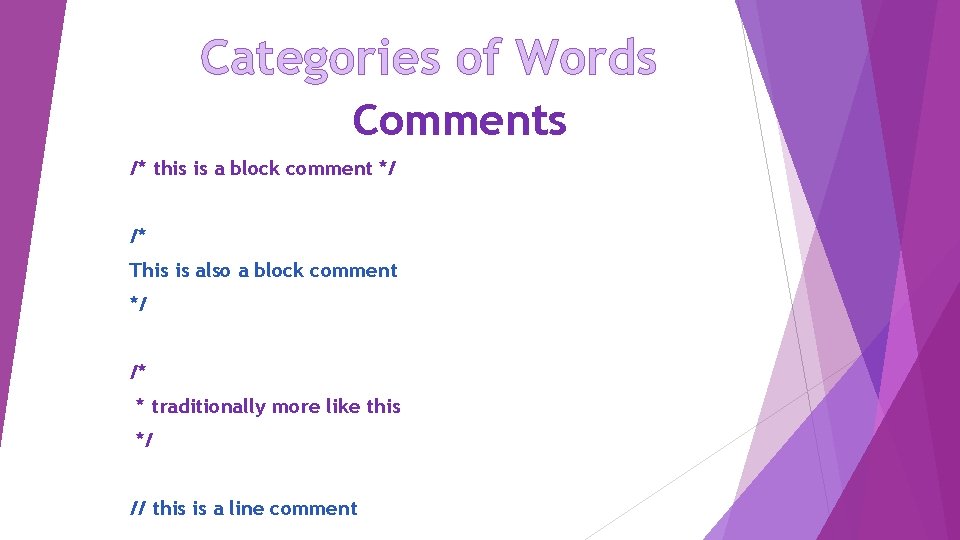
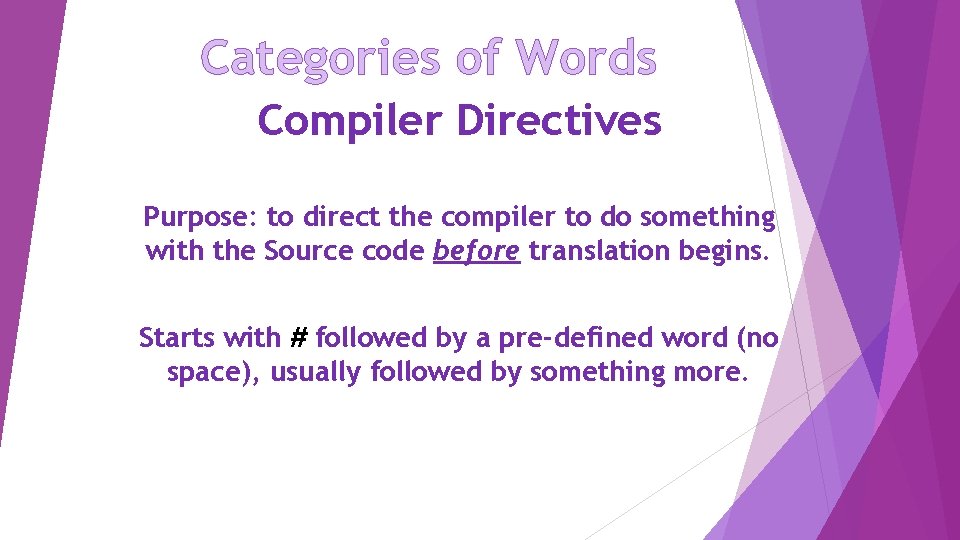
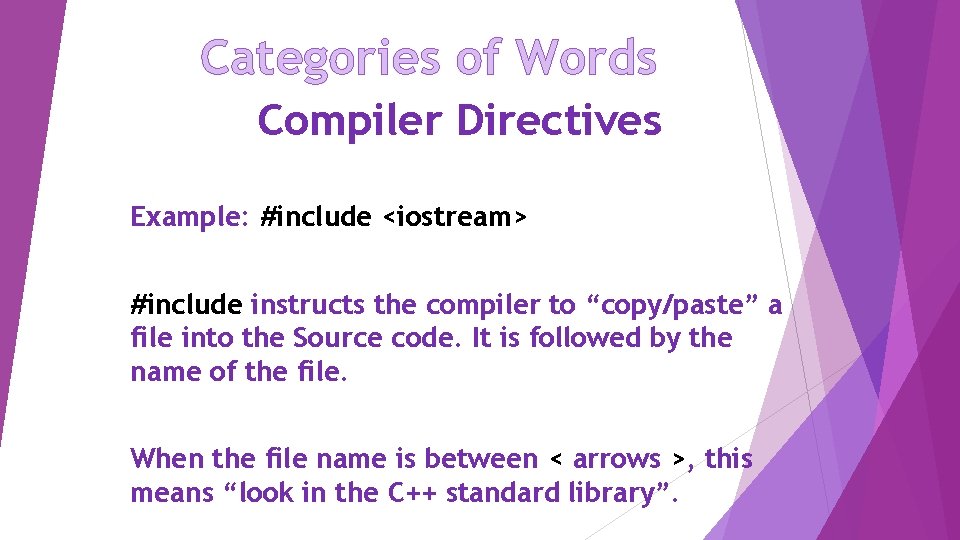
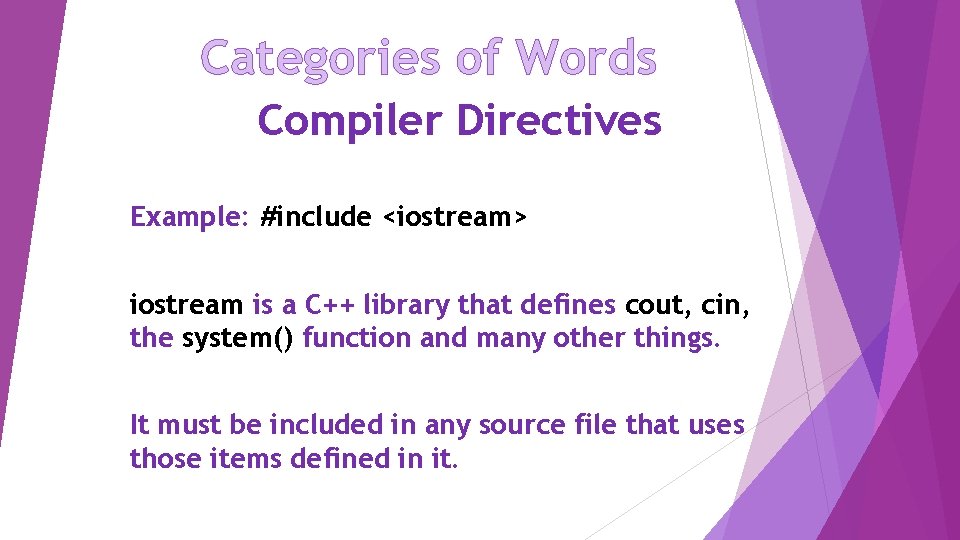
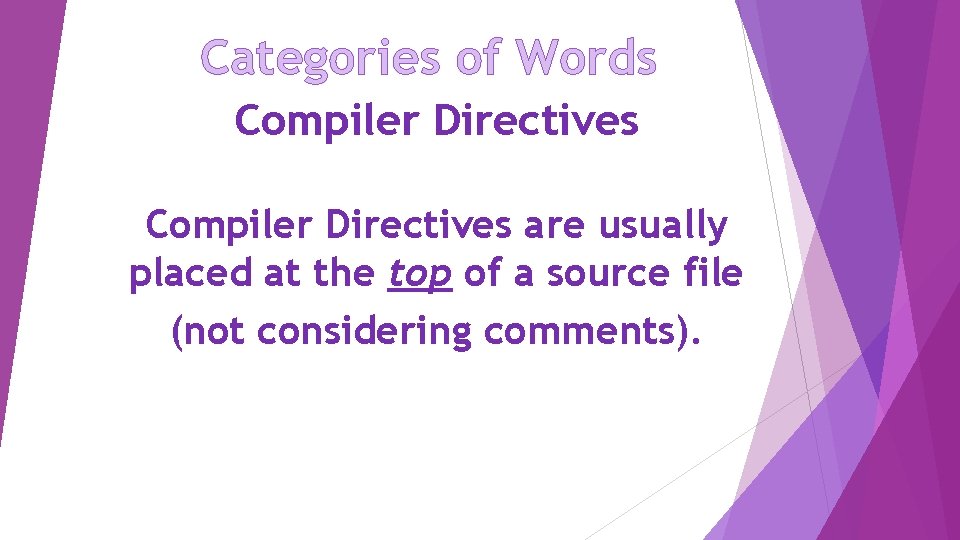
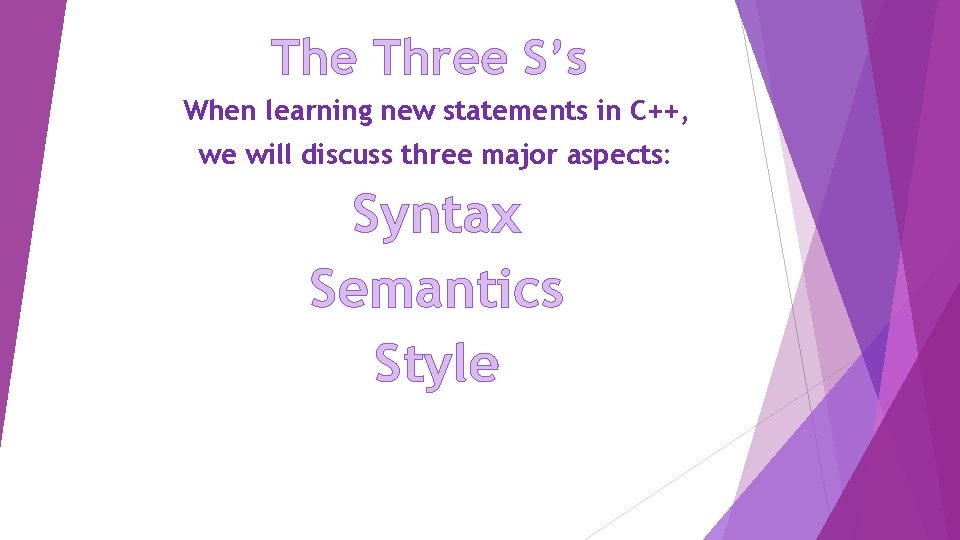
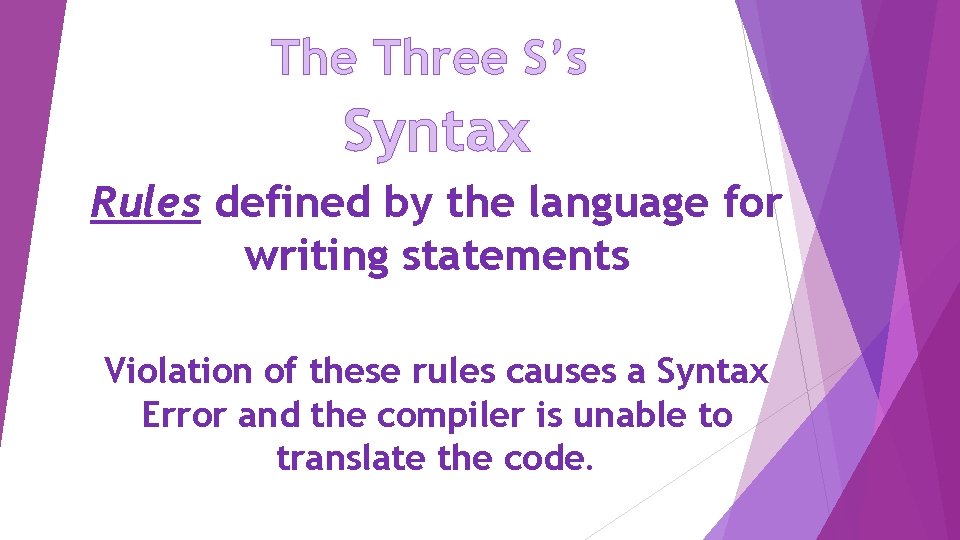
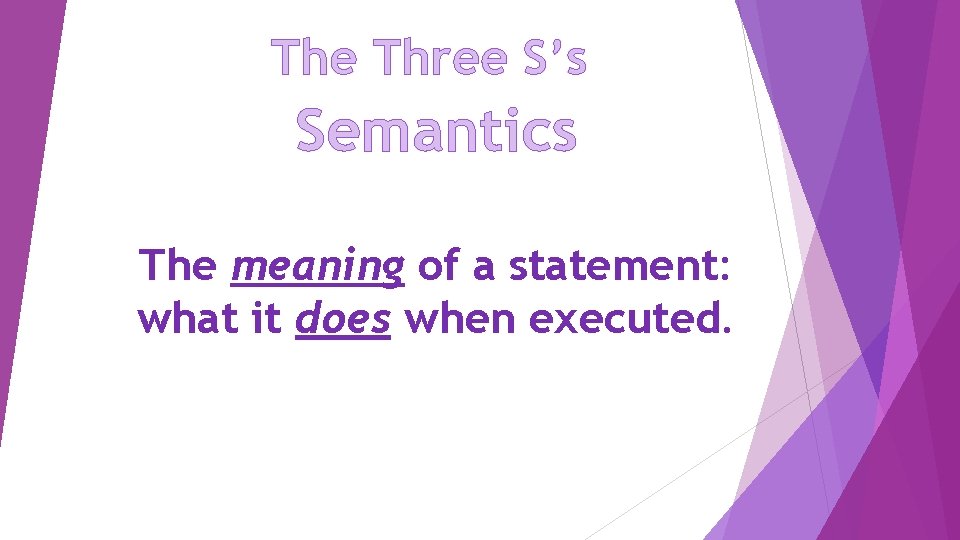
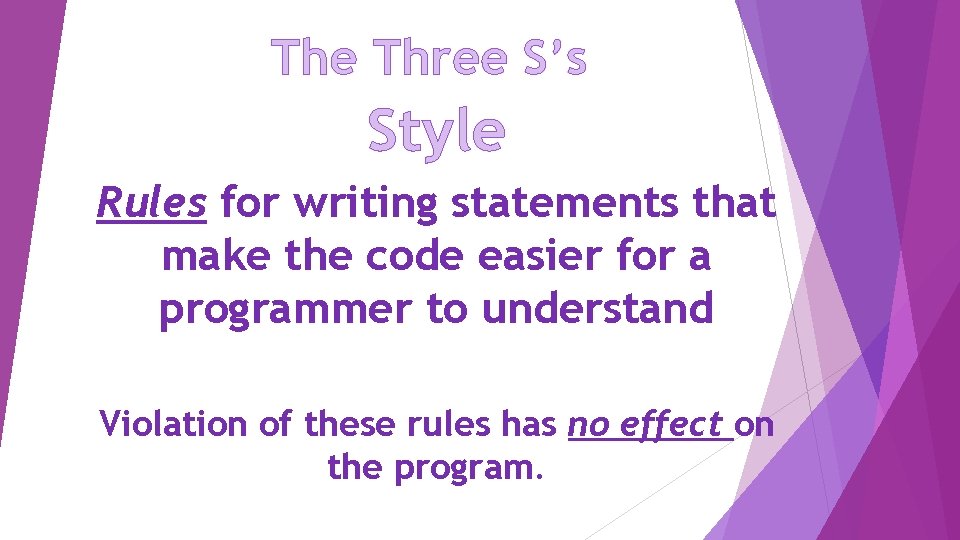
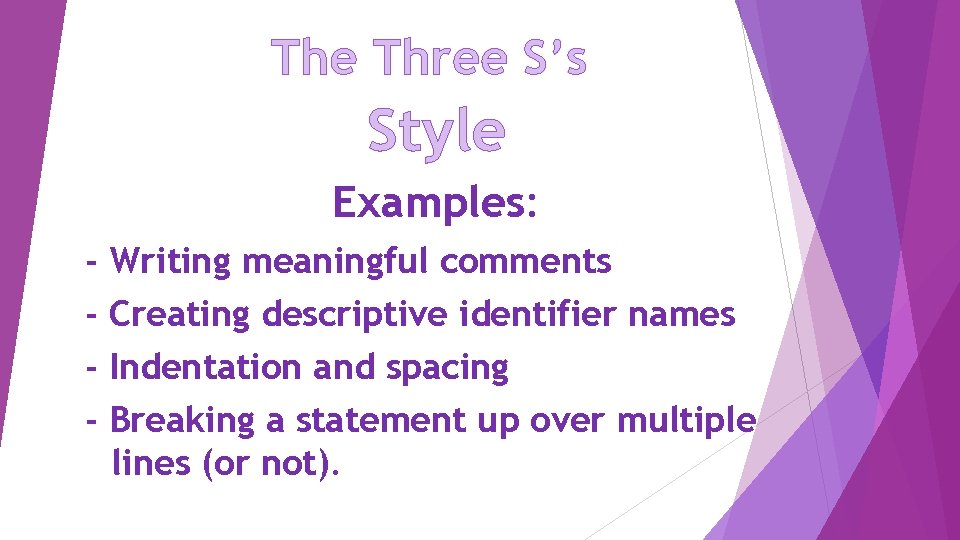
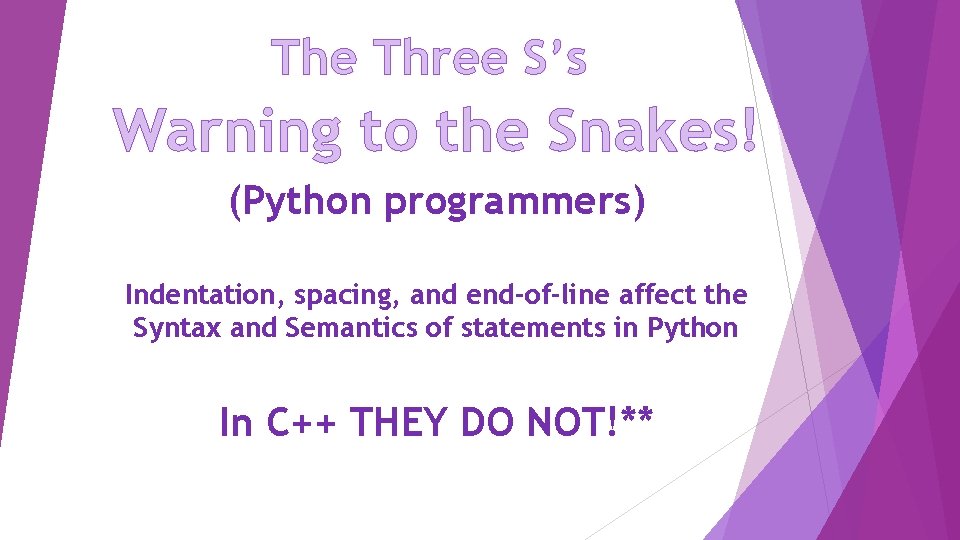
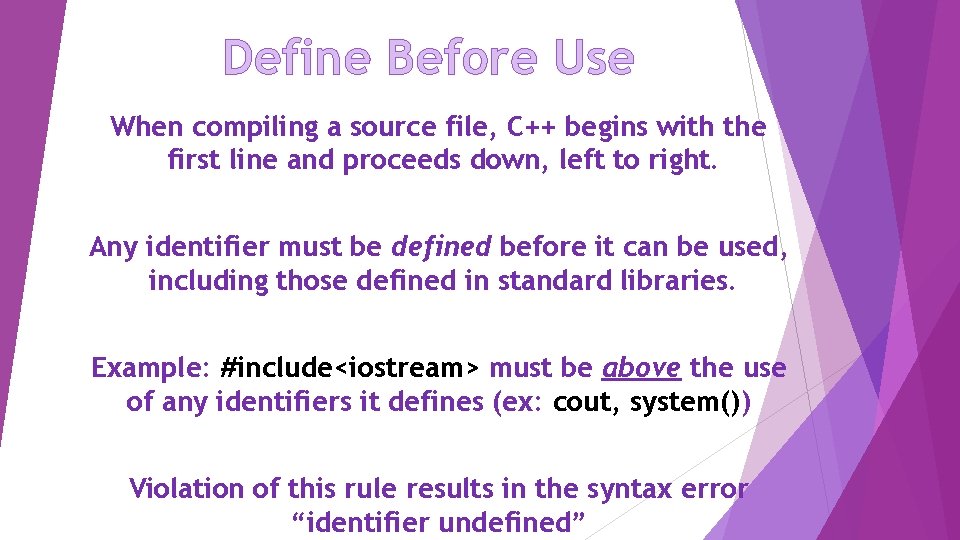
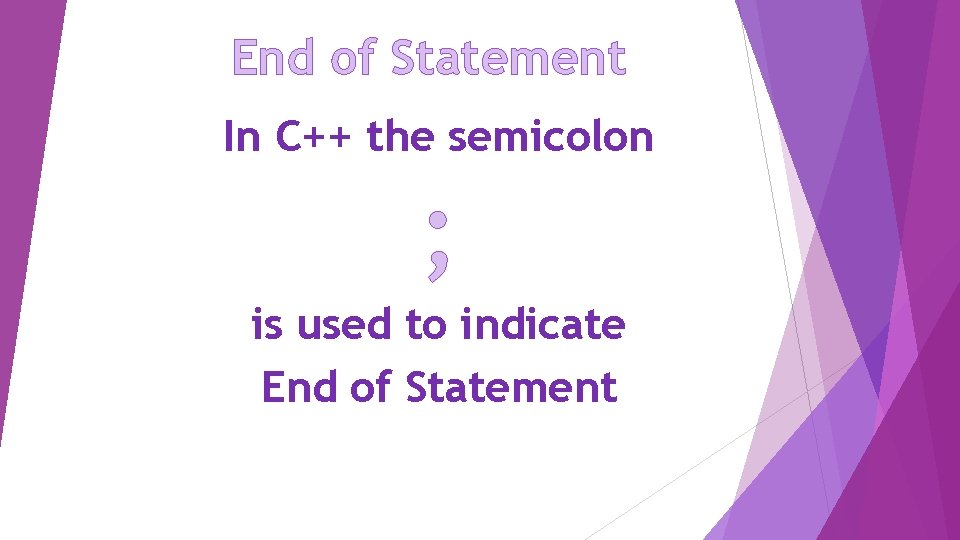
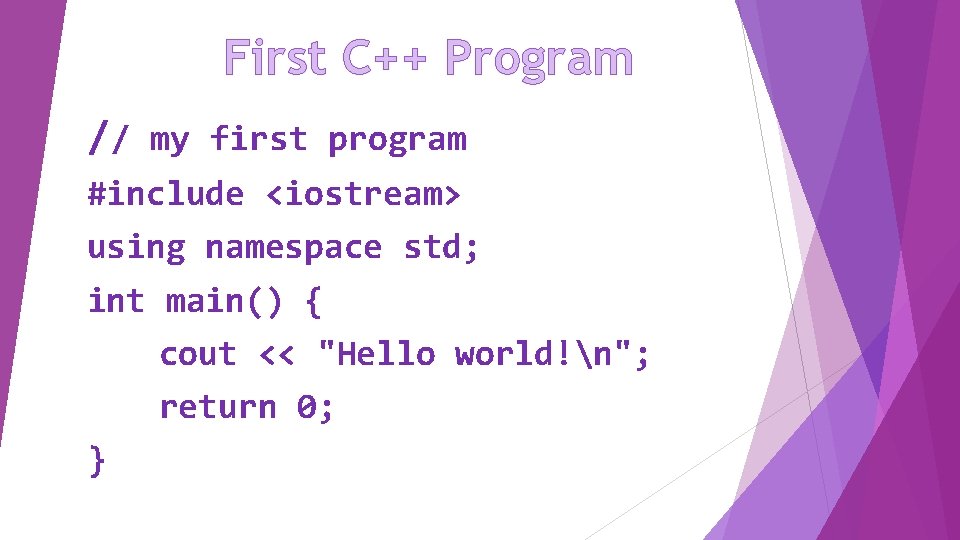
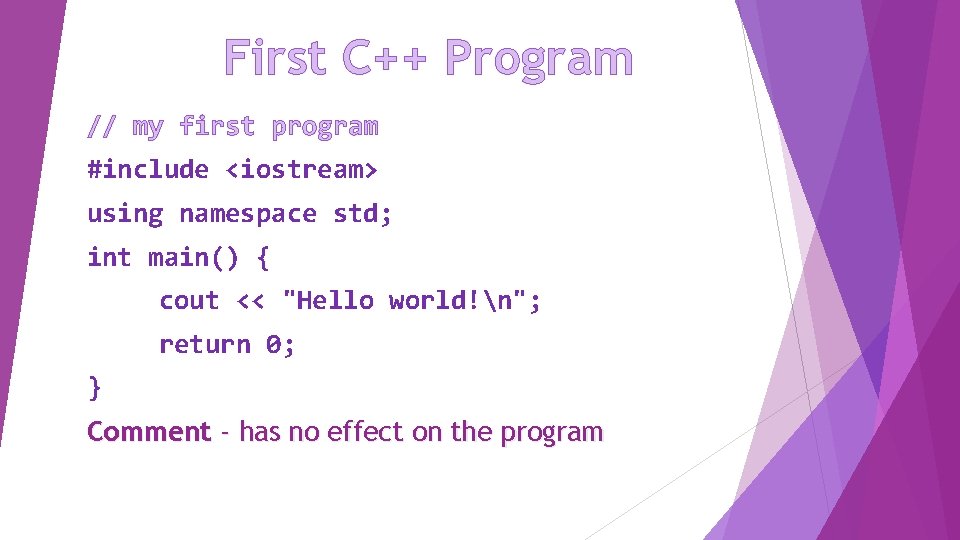
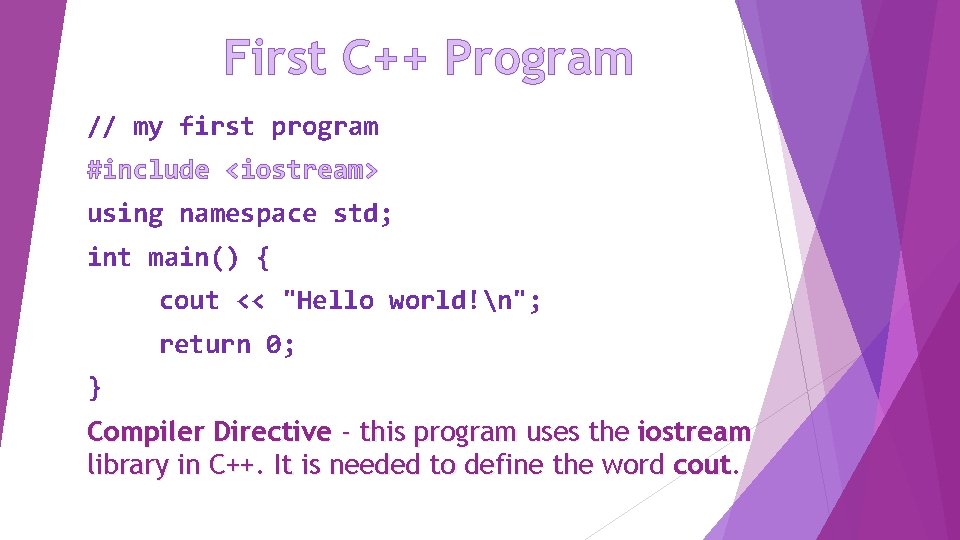
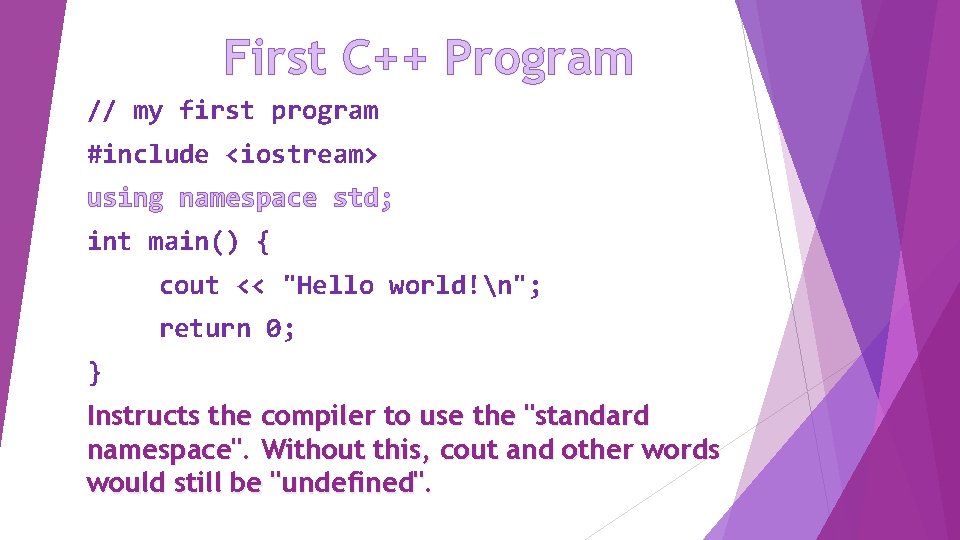
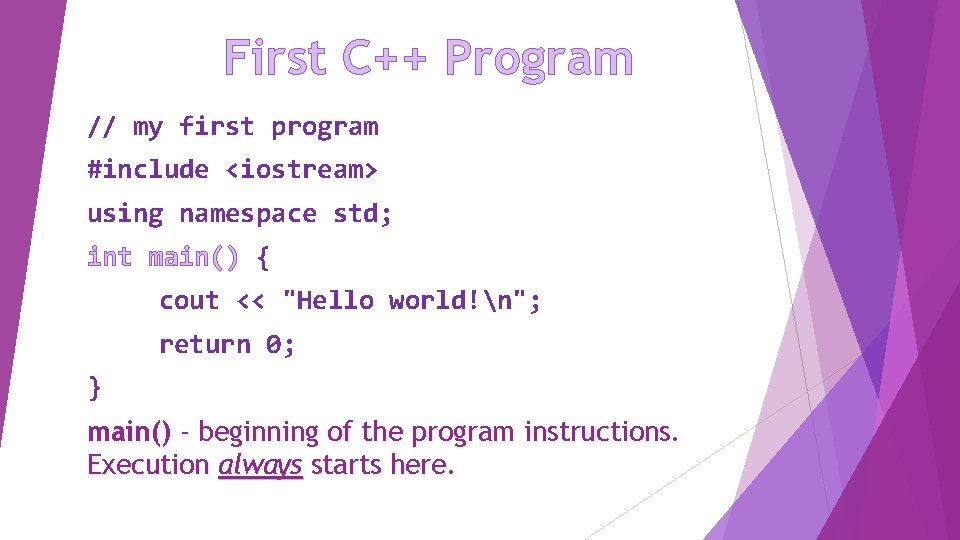
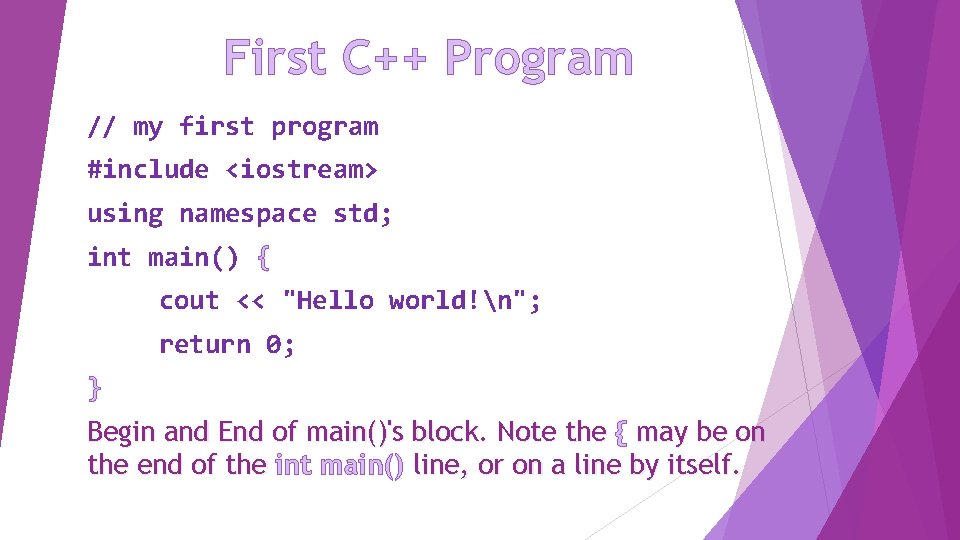
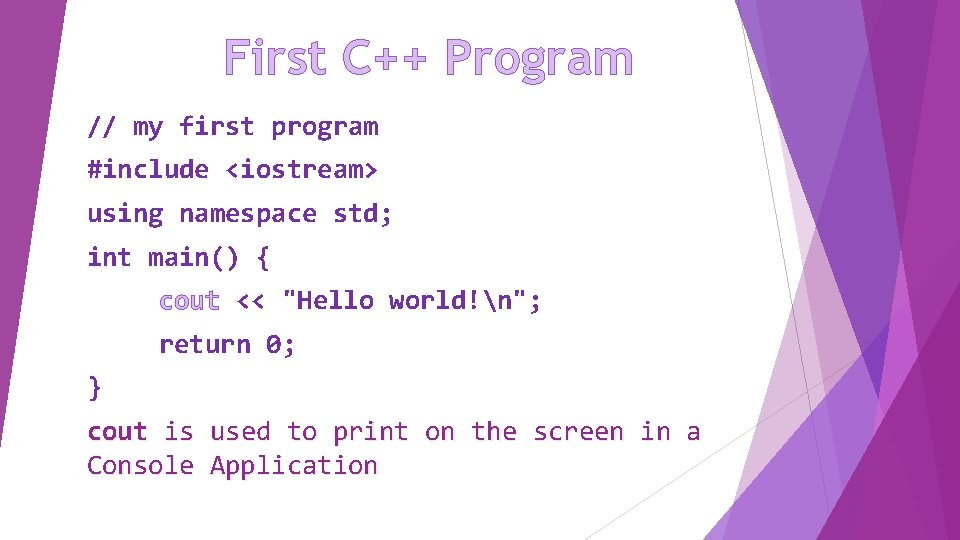
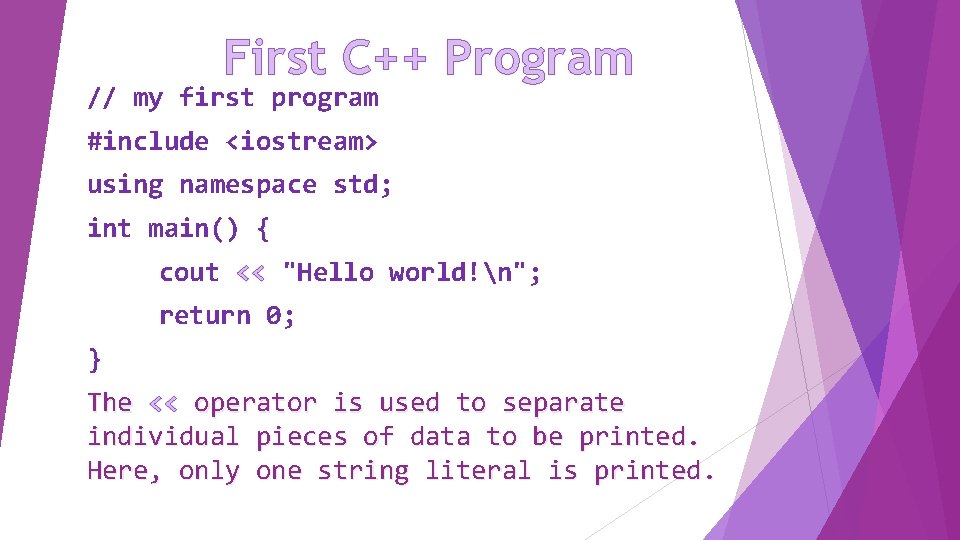
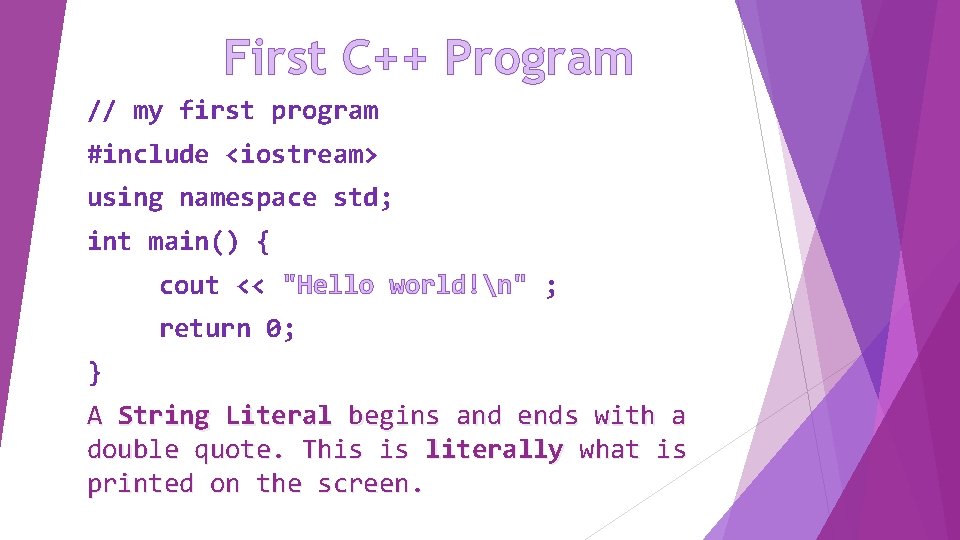
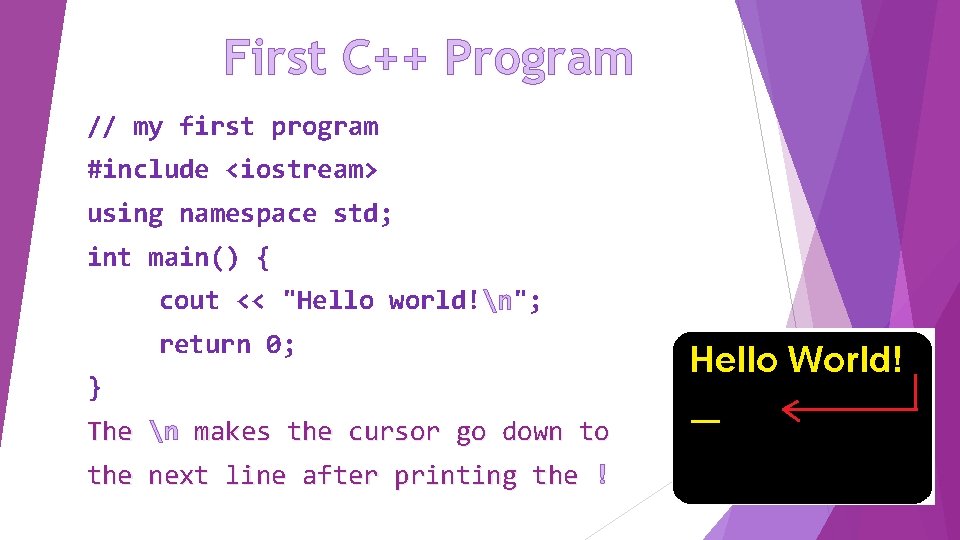
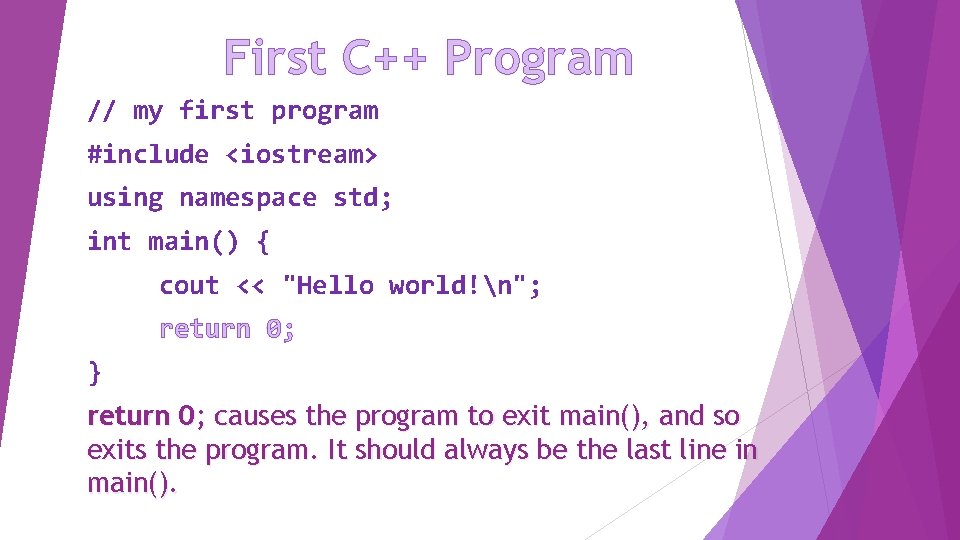
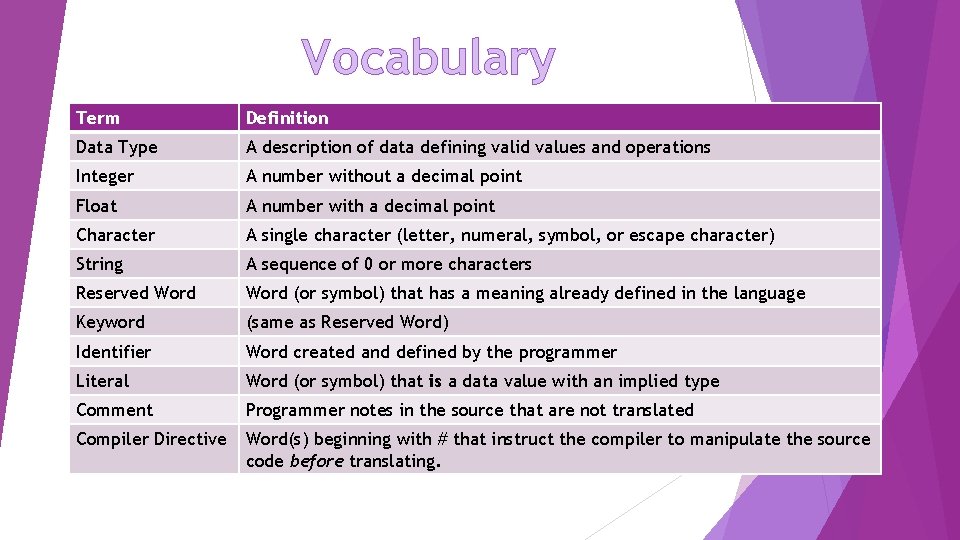
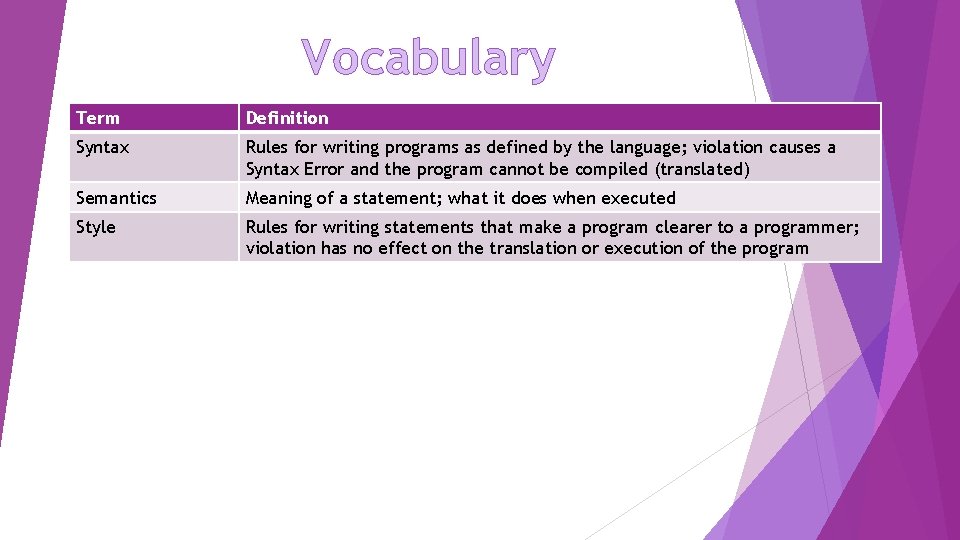
- Slides: 39
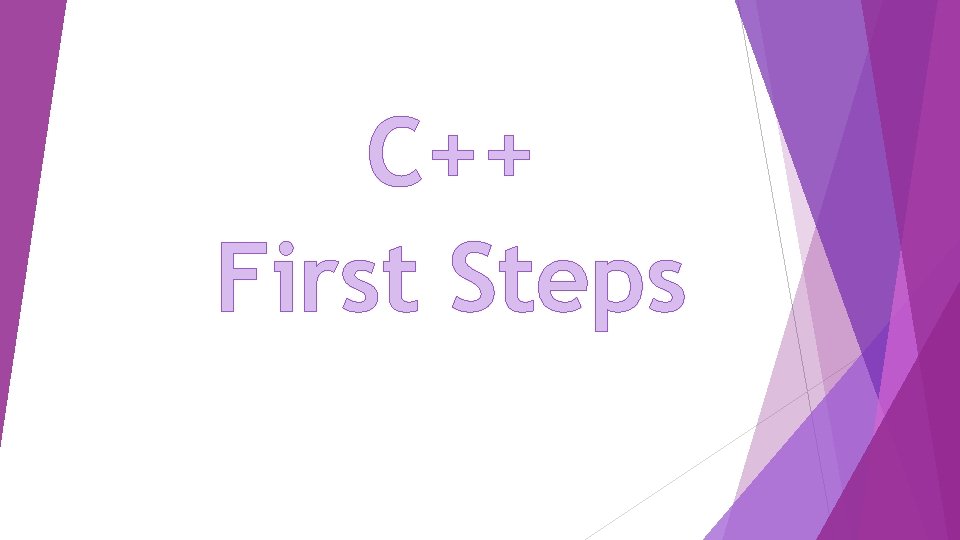
C++ First Steps
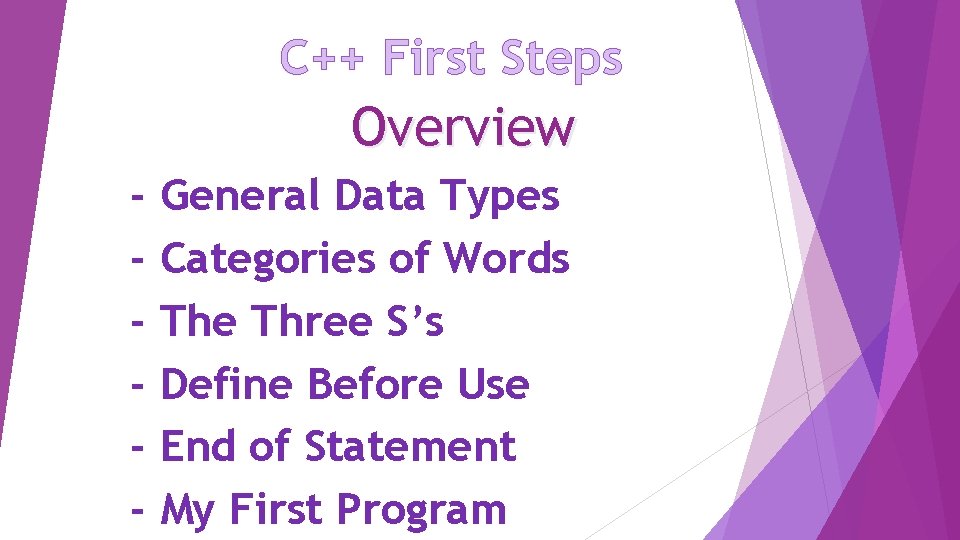
C++ First Steps Overview - General Data Types Categories of Words The Three S’s Define Before Use End of Statement My First Program
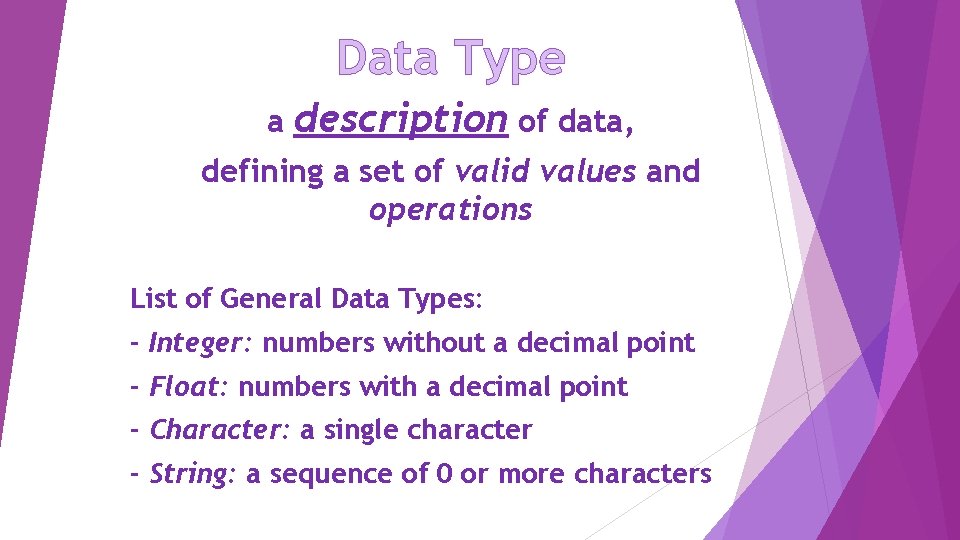
Data Type a description of data, defining a set of valid values and operations List of General Data Types: - Integer: numbers without a decimal point - Float: numbers with a decimal point - Character: a single character - String: a sequence of 0 or more characters
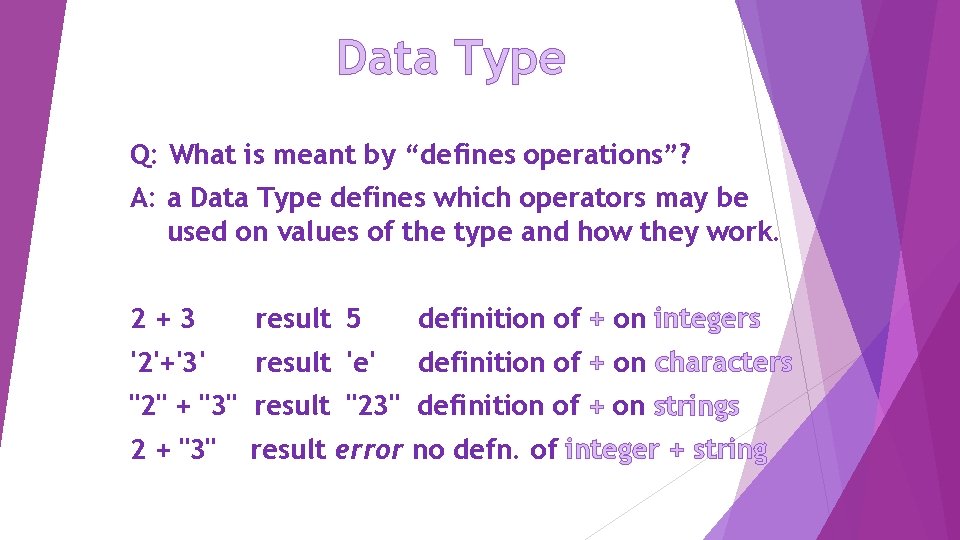
Data Type Q: What is meant by “defines operations”? A: a Data Type defines which operators may be used on values of the type and how they work. 2+3 result 5 definition of + on integers '2'+'3' result 'e' definition of + on characters "2" + "3" result "23" definition of + on strings 2 + "3" result error no defn. of integer + string
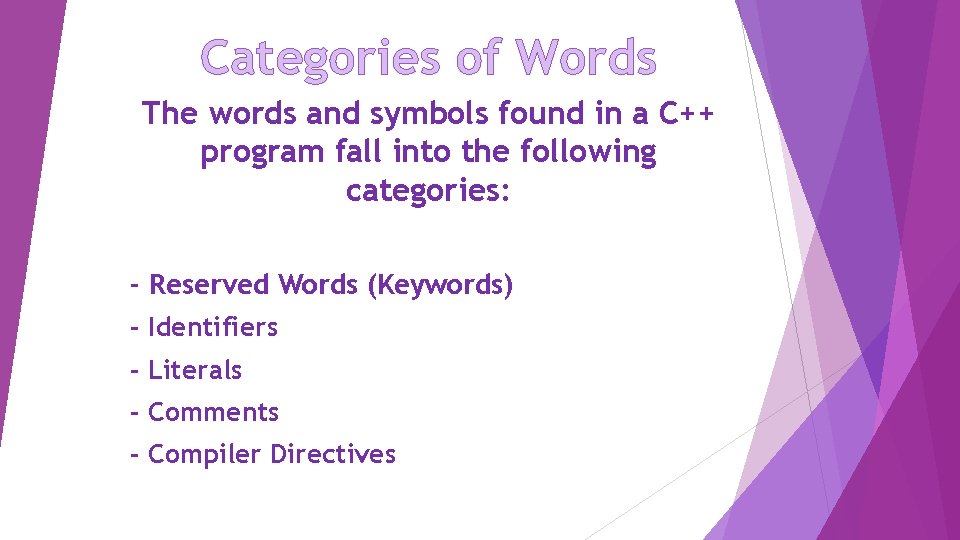
Categories of Words The words and symbols found in a C++ program fall into the following categories: - Reserved Words (Keywords) - Identifiers - Literals - Comments - Compiler Directives
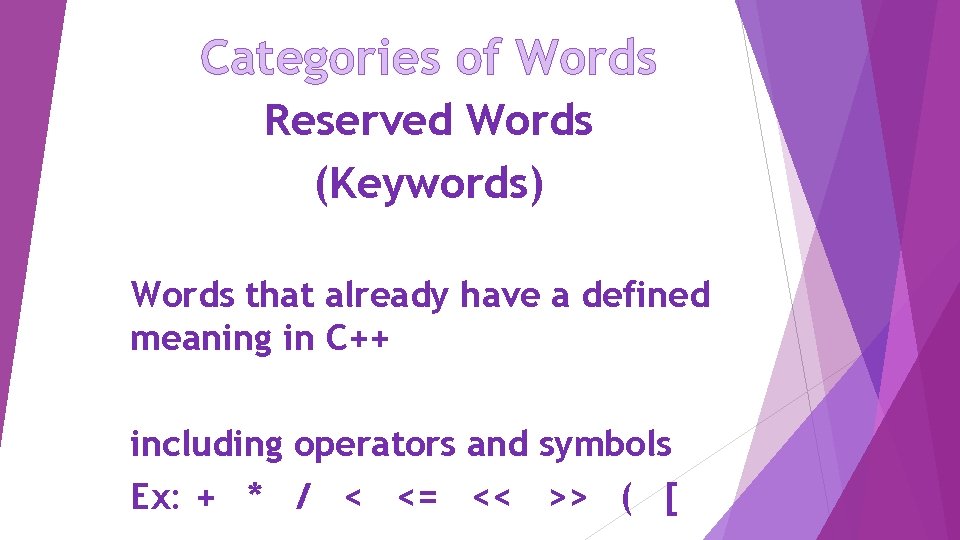
Categories of Words Reserved Words (Keywords) Words that already have a defined meaning in C++ including operators and symbols Ex: + * / < <= << >> ( [
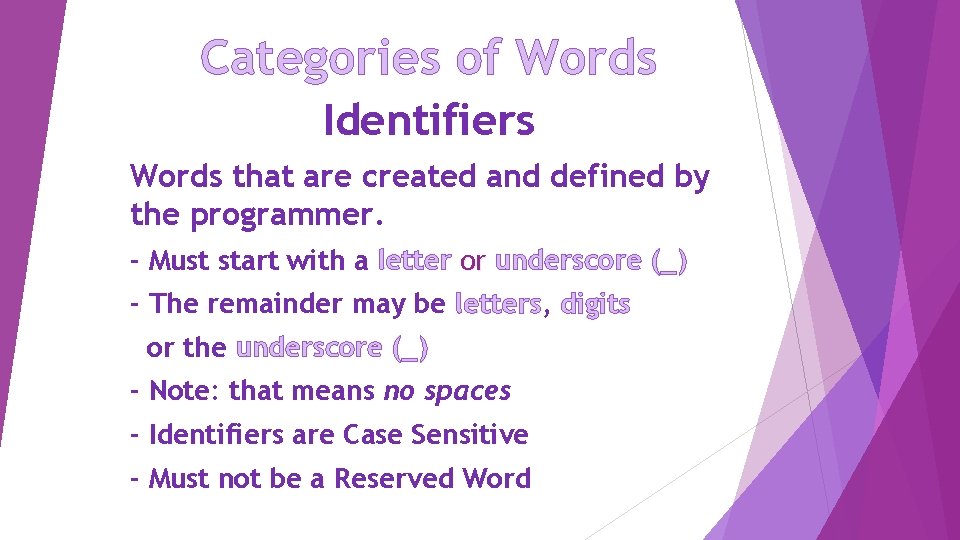
Categories of Words Identifiers Words that are created and defined by the programmer. - Must start with a letter or underscore (_) - The remainder may be letters, digits or the underscore (_) - Note: that means no spaces - Identifiers are Case Sensitive - Must not be a Reserved Word
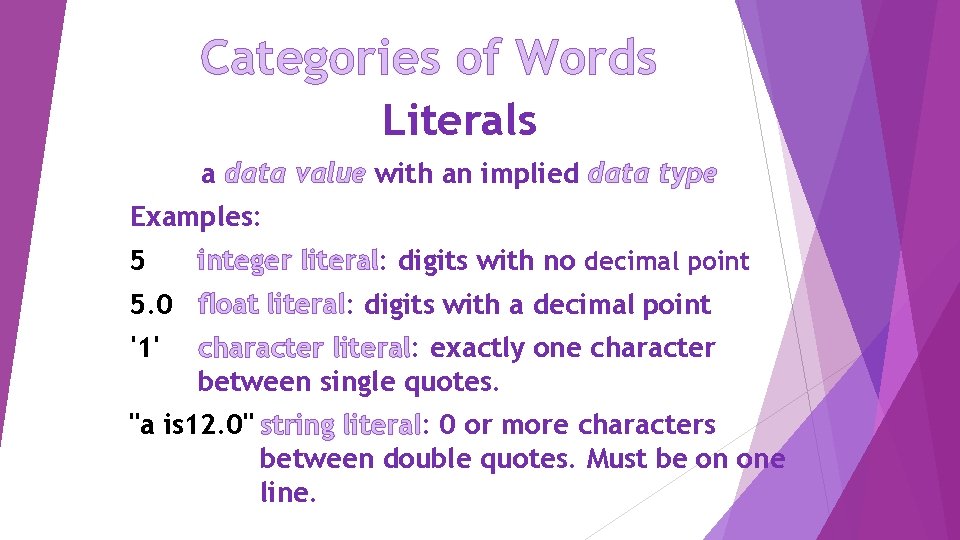
Categories of Words Literals a data value with an implied data type Examples: 5 integer literal: digits with no decimal point 5. 0 float literal: digits with a decimal point '1' character literal: exactly one character between single quotes. "a is 12. 0" string literal: 0 or more characters between double quotes. Must be on one line.
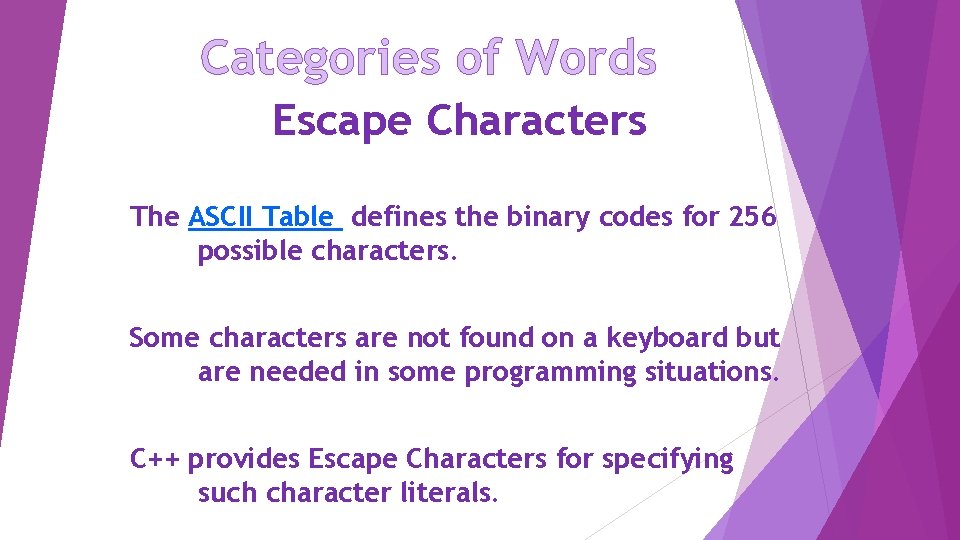
Categories of Words Escape Characters The ASCII Table defines the binary codes for 256 possible characters. Some characters are not found on a keyboard but are needed in some programming situations. C++ provides Escape Characters for specifying such character literals.
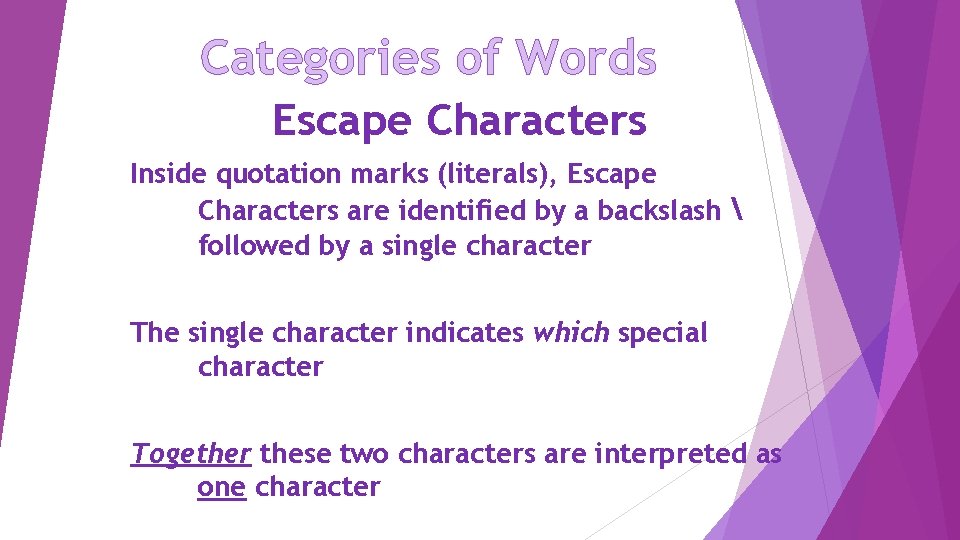
Categories of Words Escape Characters Inside quotation marks (literals), Escape Characters are identified by a backslash followed by a single character The single character indicates which special character Together these two characters are interpreted as one character
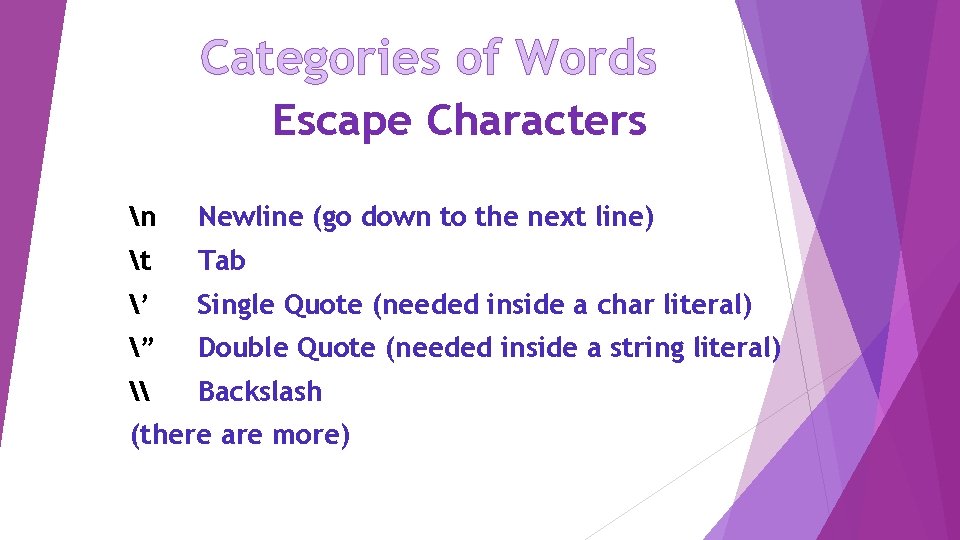
Categories of Words Escape Characters n Newline (go down to the next line) t Tab ’ Single Quote (needed inside a char literal) ” Double Quote (needed inside a string literal) \ Backslash (there are more)
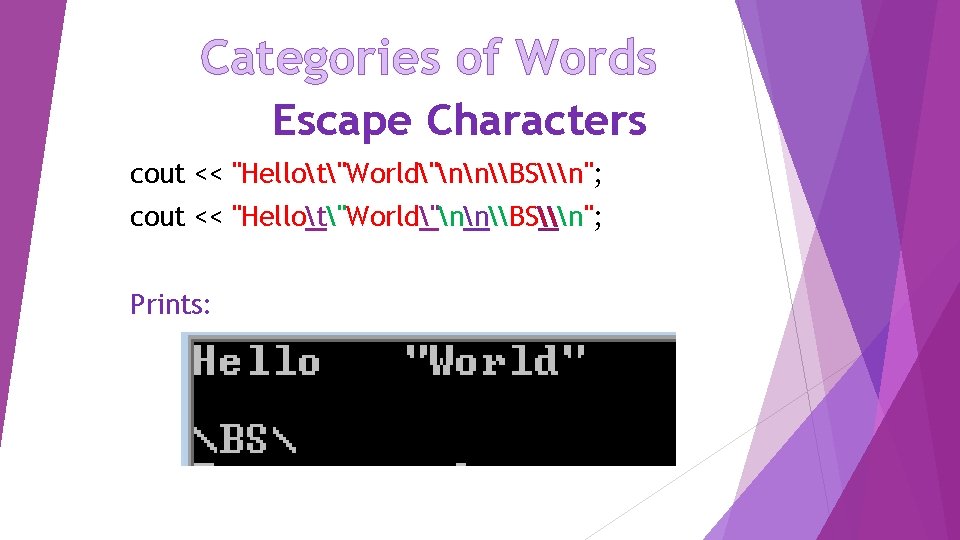
Categories of Words Escape Characters cout << "Hellot"World"nn\BS\n"; Prints:
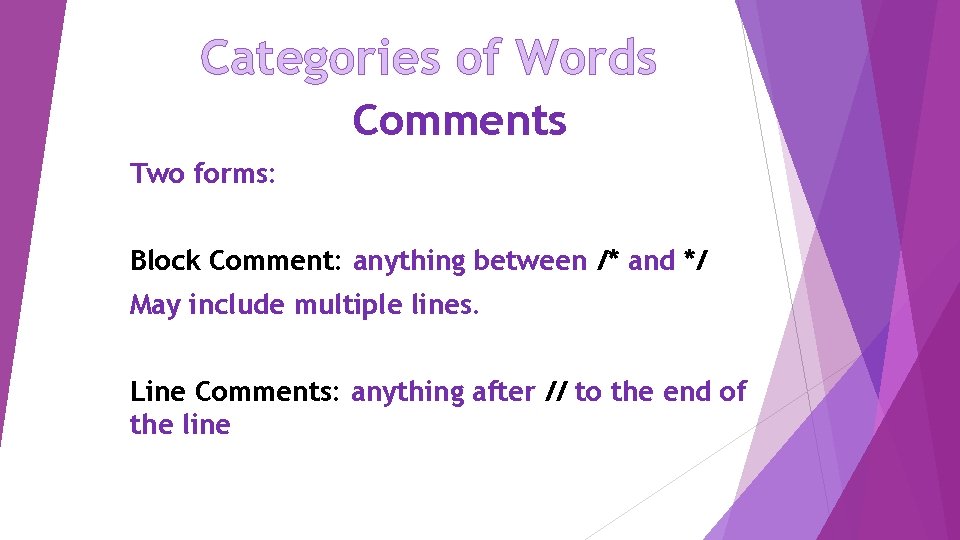
Categories of Words Comments Two forms: Block Comment: anything between /* and */ May include multiple lines. Line Comments: anything after // to the end of the line
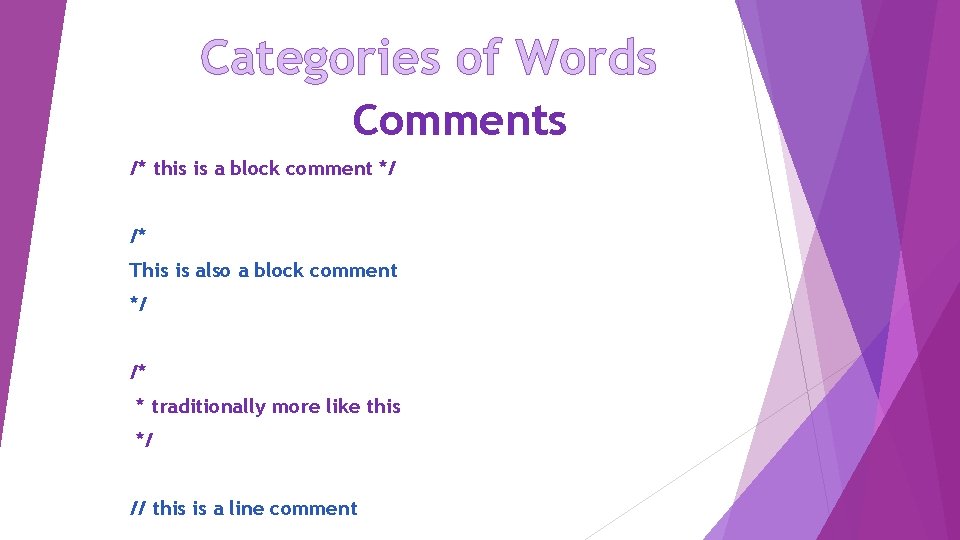
Categories of Words Comments /* this is a block comment */ /* This is also a block comment */ /* * traditionally more like this */ // this is a line comment
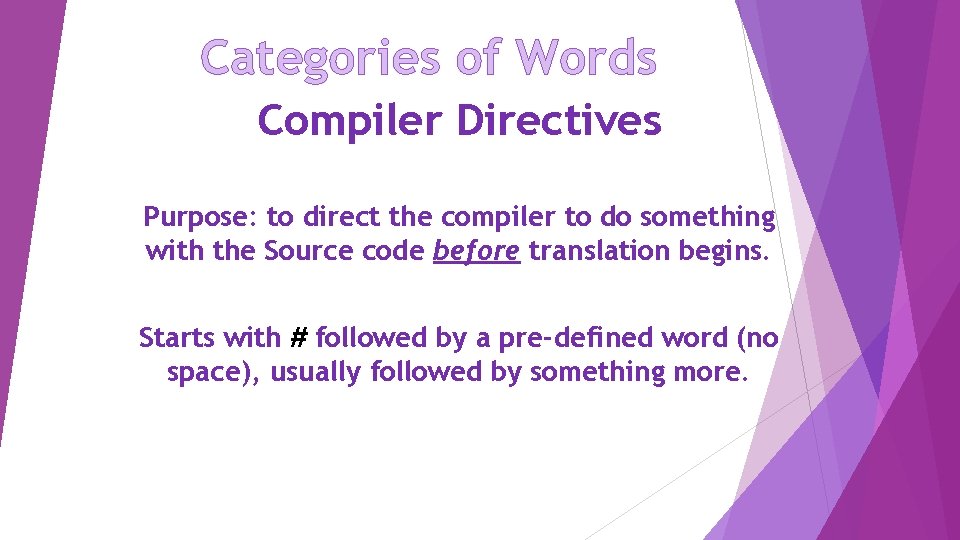
Categories of Words Compiler Directives Purpose: to direct the compiler to do something with the Source code before translation begins. Starts with # followed by a pre-defined word (no space), usually followed by something more.
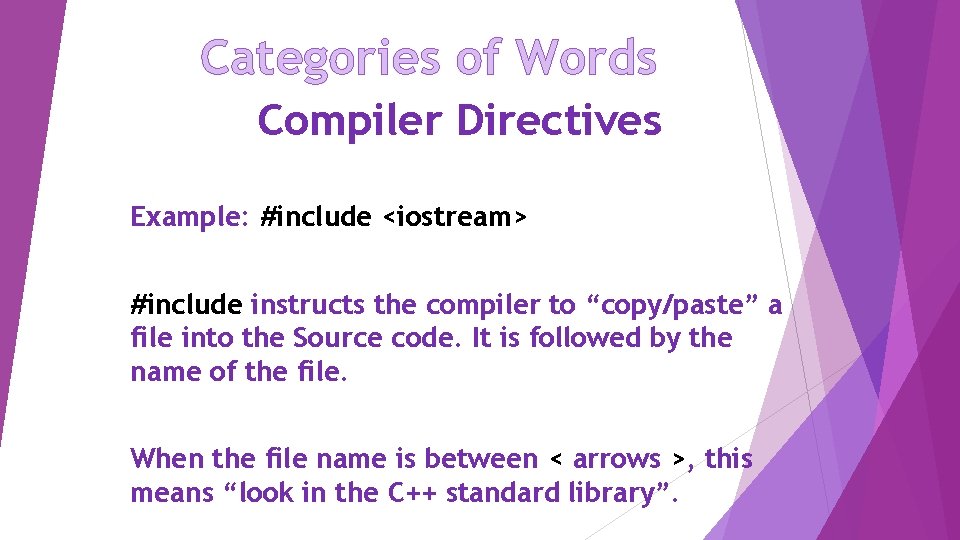
Categories of Words Compiler Directives Example: #include <iostream> #include instructs the compiler to “copy/paste” a file into the Source code. It is followed by the name of the file. When the file name is between < arrows >, this means “look in the C++ standard library”.
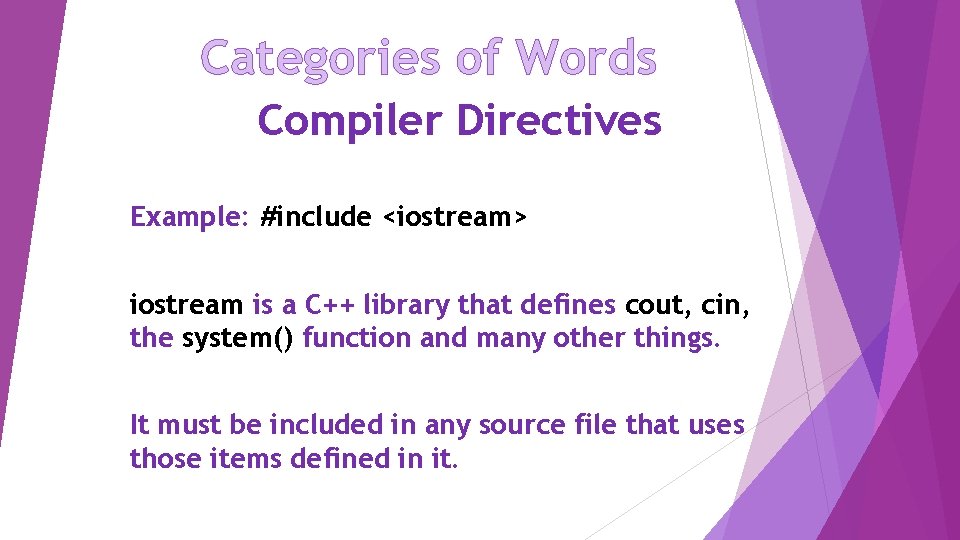
Categories of Words Compiler Directives Example: #include <iostream> iostream is a C++ library that defines cout, cin, the system() function and many other things. It must be included in any source file that uses those items defined in it.
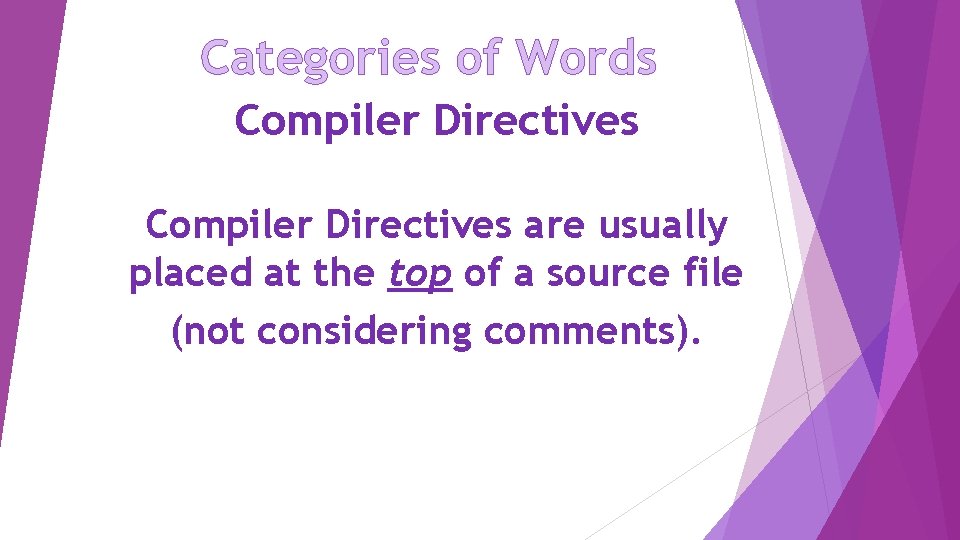
Categories of Words Compiler Directives are usually placed at the top of a source file (not considering comments).
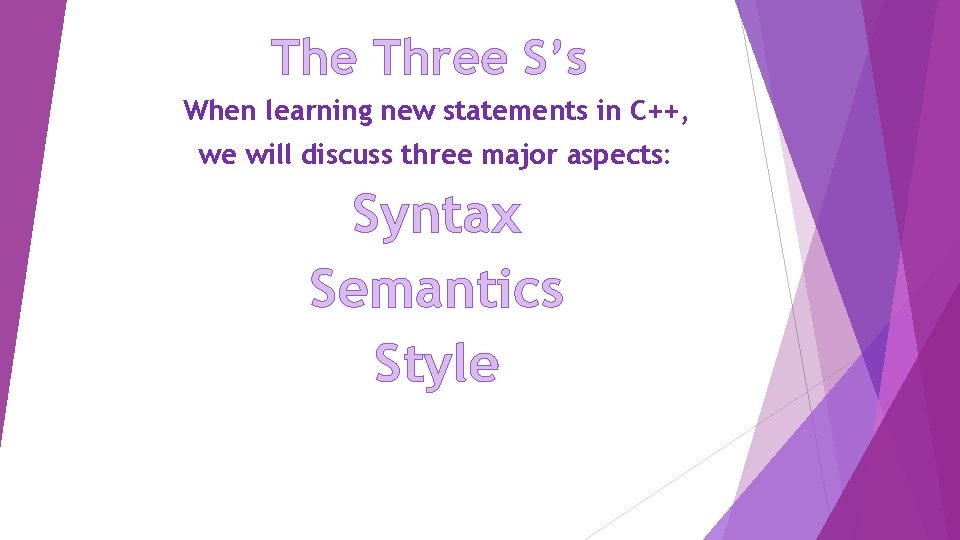
The Three S’s When learning new statements in C++, we will discuss three major aspects: Syntax Semantics Style
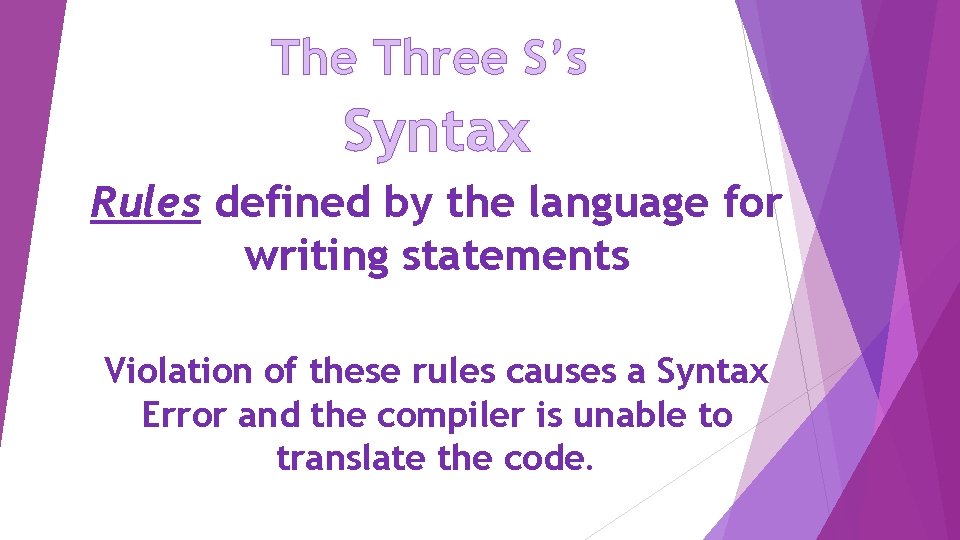
The Three S’s Syntax Rules defined by the language for writing statements Violation of these rules causes a Syntax Error and the compiler is unable to translate the code.
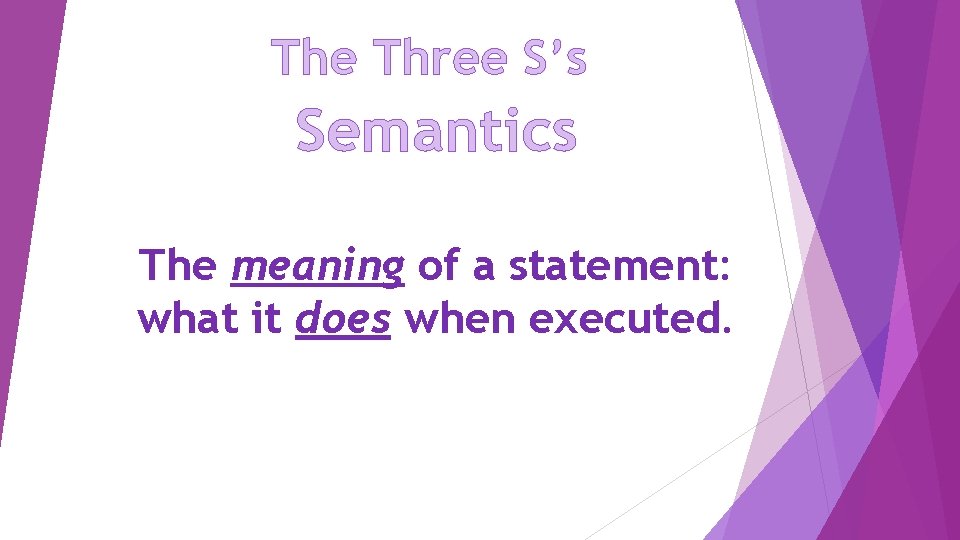
The Three S’s Semantics The meaning of a statement: what it does when executed.
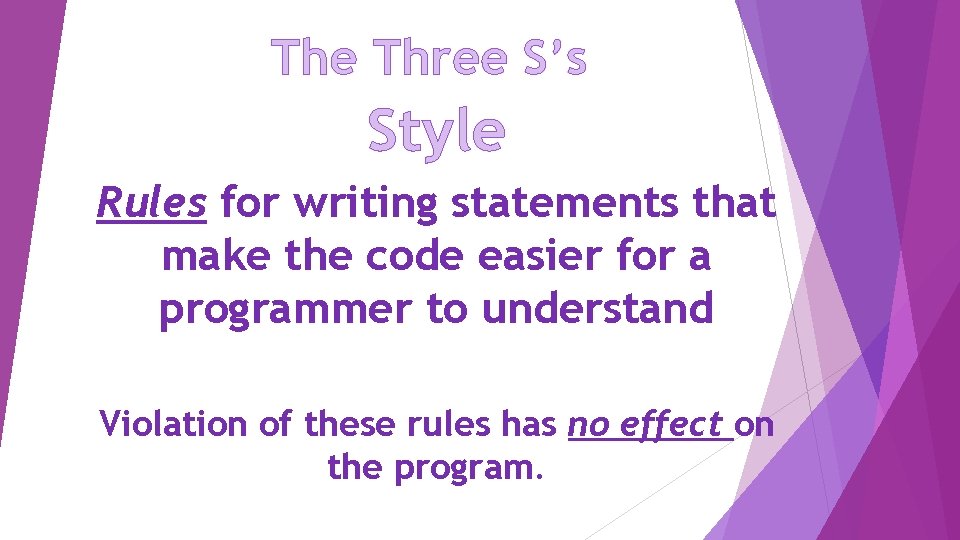
The Three S’s Style Rules for writing statements that make the code easier for a programmer to understand Violation of these rules has no effect on the program.
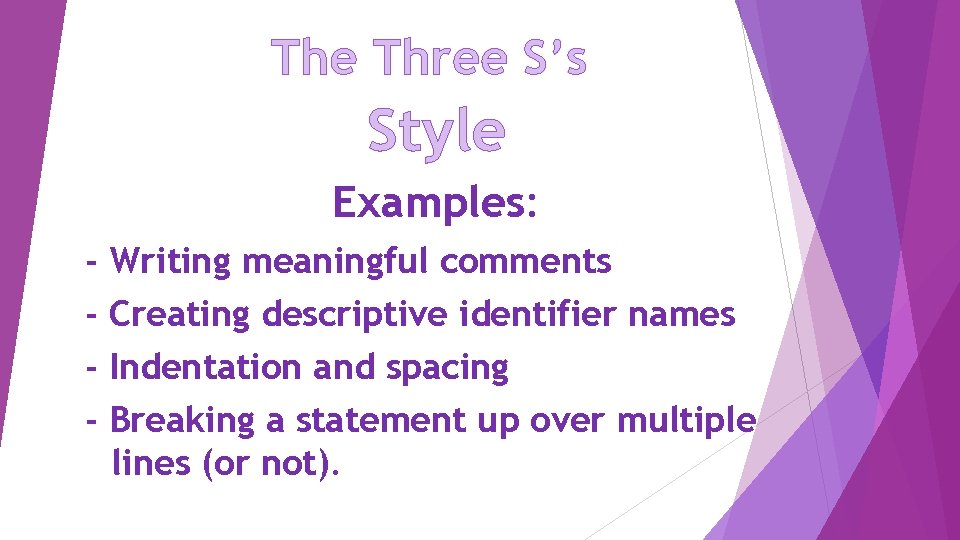
The Three S’s Style Examples: - Writing meaningful comments Creating descriptive identifier names Indentation and spacing Breaking a statement up over multiple lines (or not).
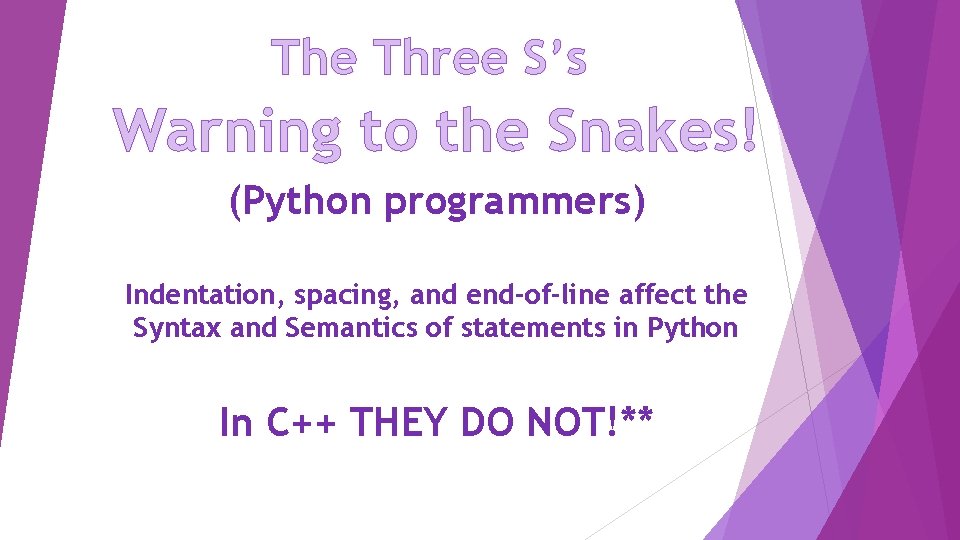
The Three S’s Warning to the Snakes! (Python programmers) Indentation, spacing, and end-of-line affect the Syntax and Semantics of statements in Python In C++ THEY DO NOT!**
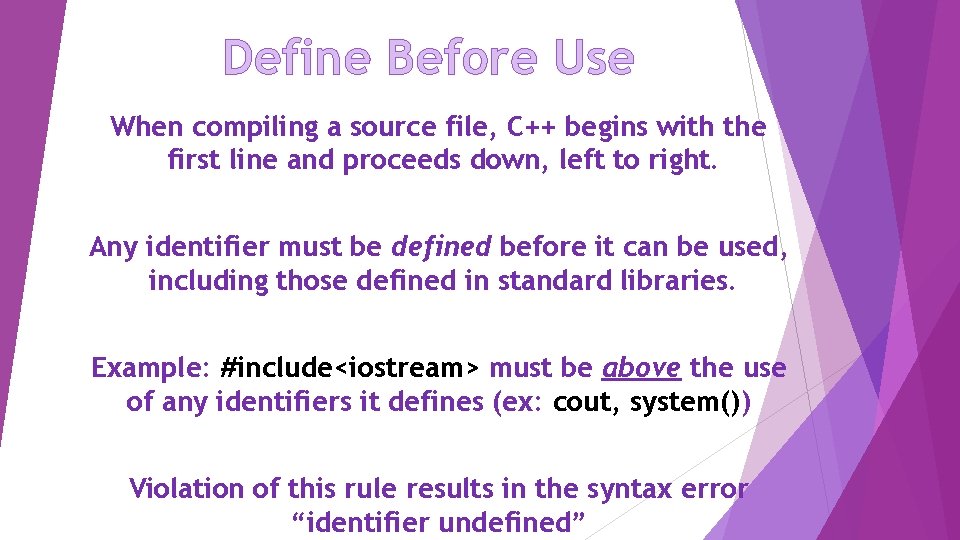
Define Before Use When compiling a source file, C++ begins with the first line and proceeds down, left to right. Any identifier must be defined before it can be used, including those defined in standard libraries. Example: #include<iostream> must be above the use of any identifiers it defines (ex: cout, system()) Violation of this rule results in the syntax error “identifier undefined”
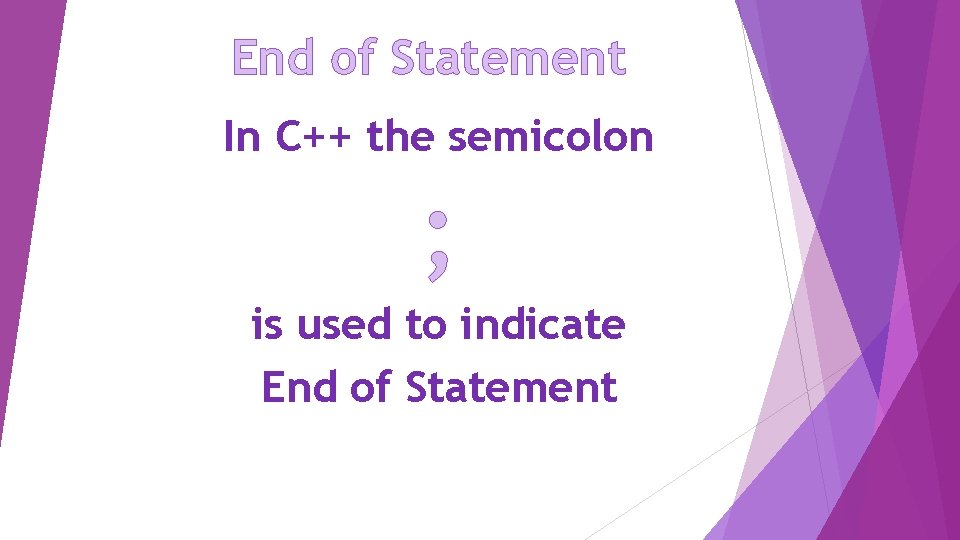
End of Statement In C++ the semicolon ; is used to indicate End of Statement
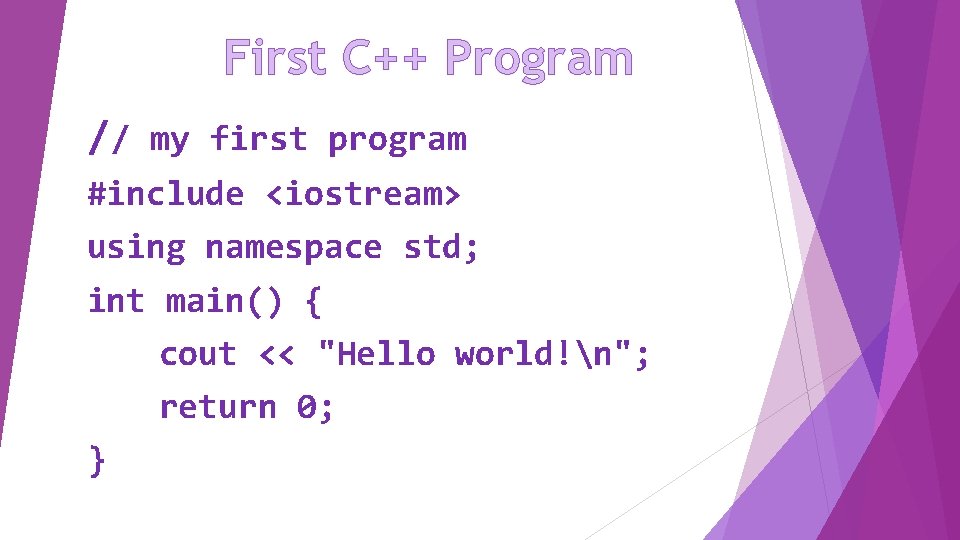
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; }
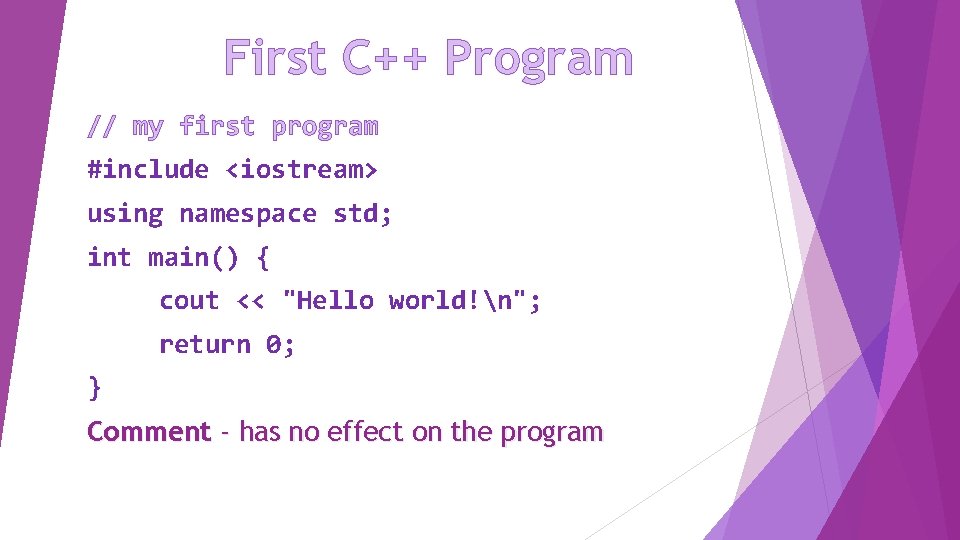
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; } Comment - has no effect on the program
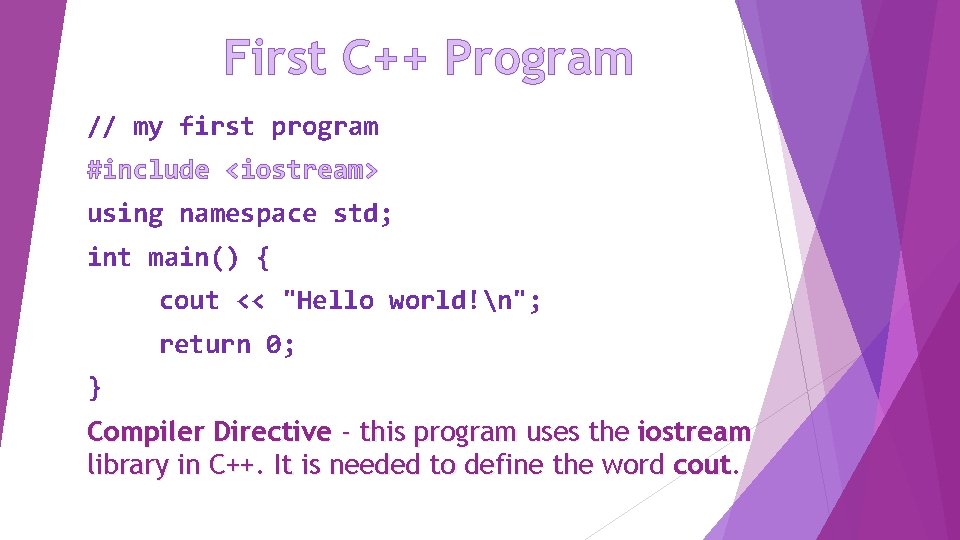
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; } Compiler Directive - this program uses the iostream library in C++. It is needed to define the word cout.
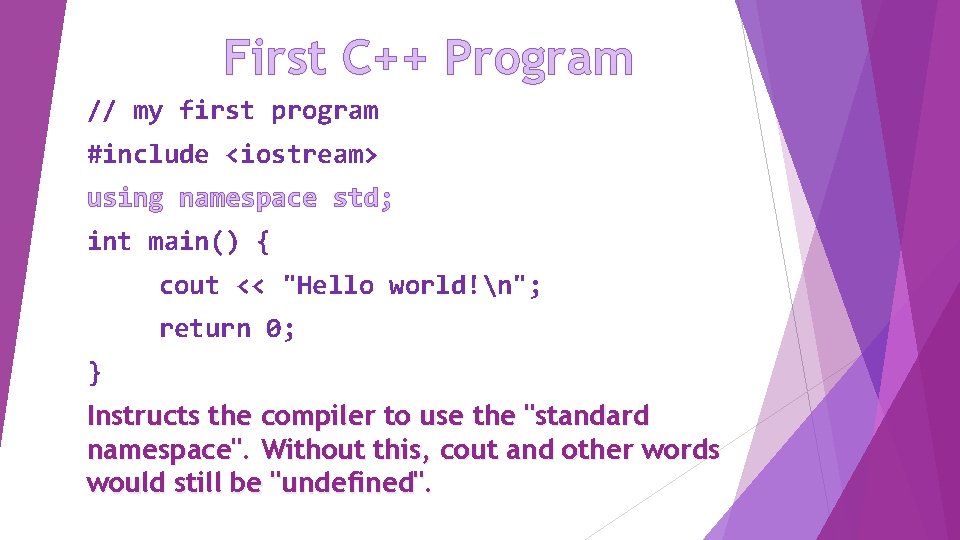
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; } Instructs the compiler to use the "standard namespace". Without this, cout and other words would still be "undefined".
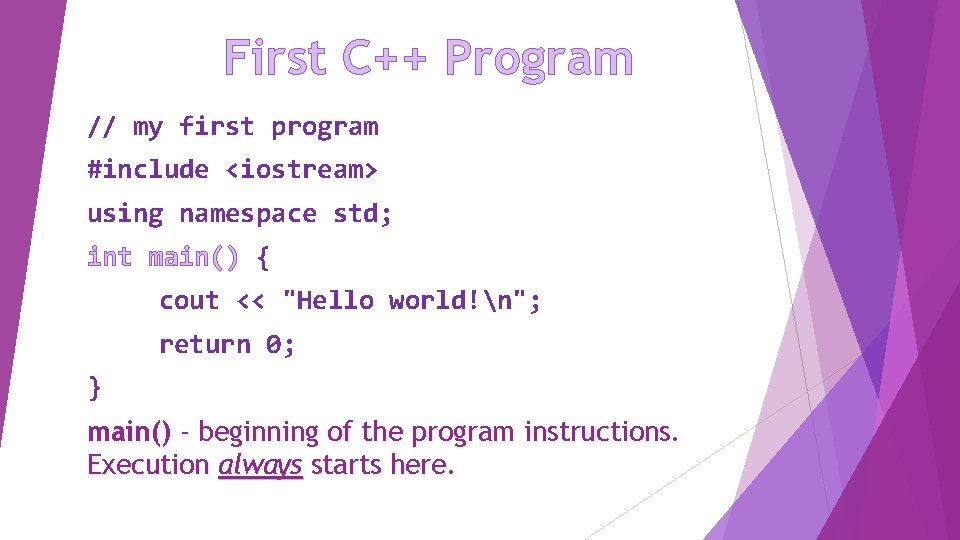
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; } main() - beginning of the program instructions. Execution always starts here.
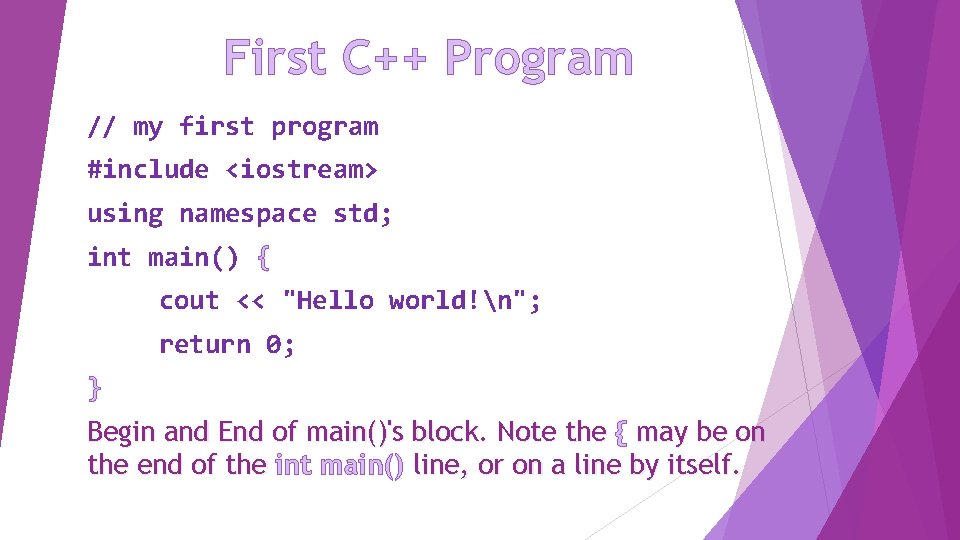
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; } Begin and End of main()'s block. Note the { may be on the end of the int main() line, or on a line by itself.
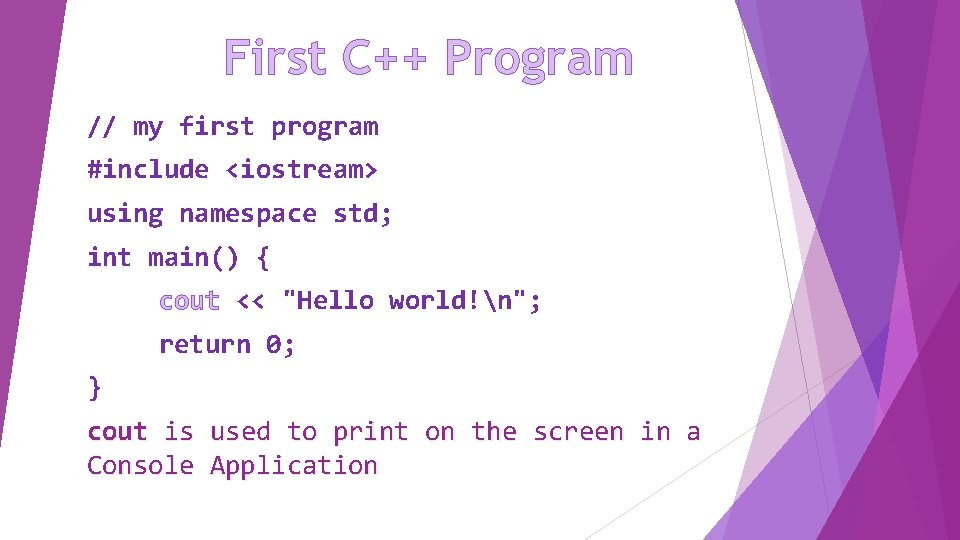
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; } cout is used to print on the screen in a Console Application
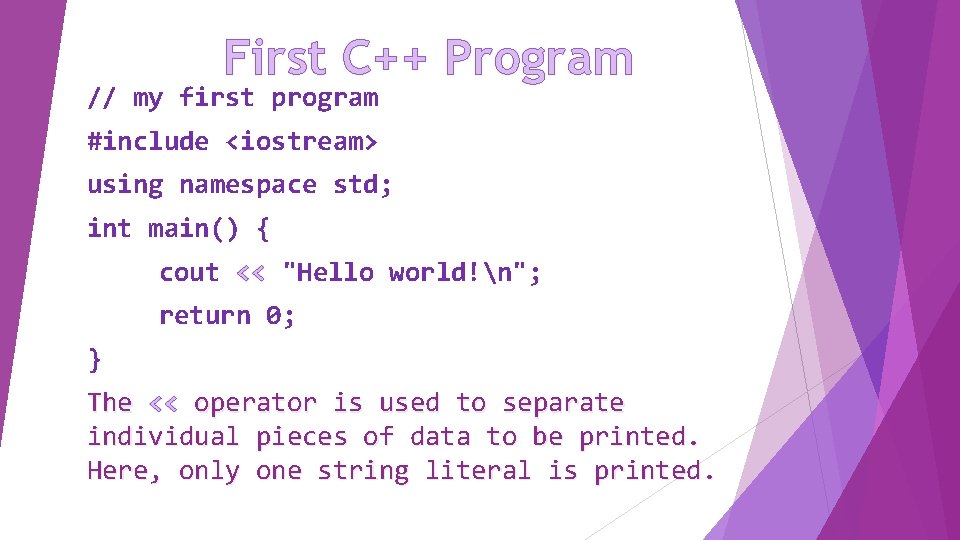
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; } The << operator is used to separate individual pieces of data to be printed. Here, only one string literal is printed.
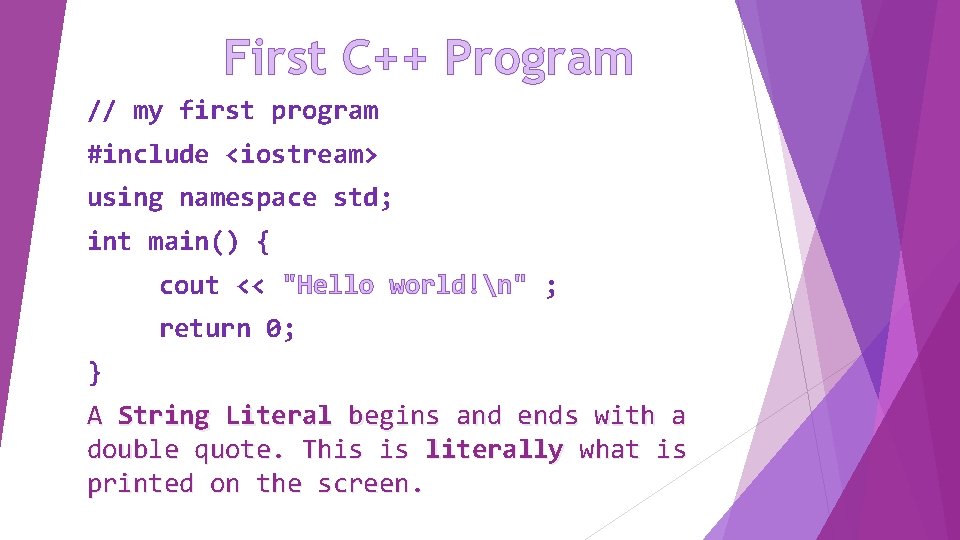
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n" ; return 0; } A String Literal begins and ends with a double quote. This is literally what is printed on the screen.
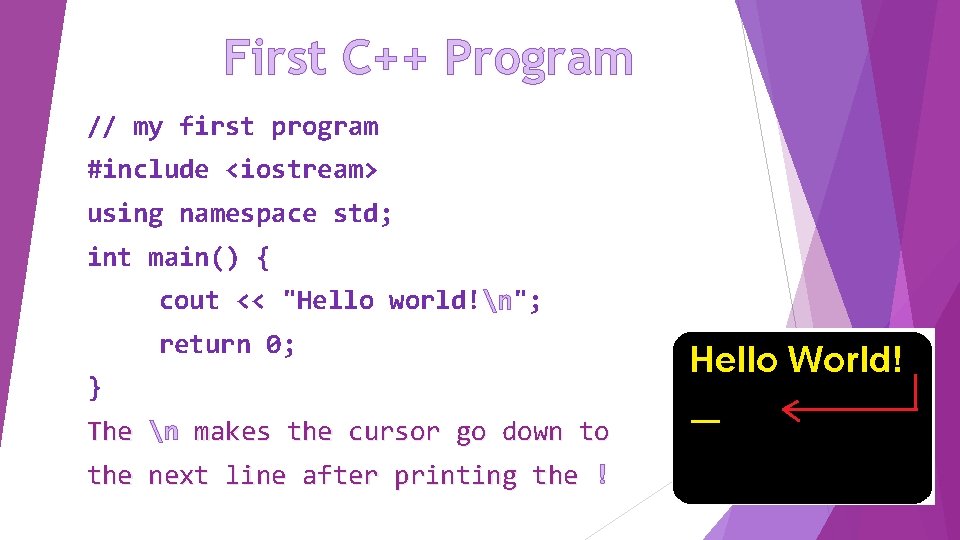
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; } The n makes the cursor go down to the next line after printing the !
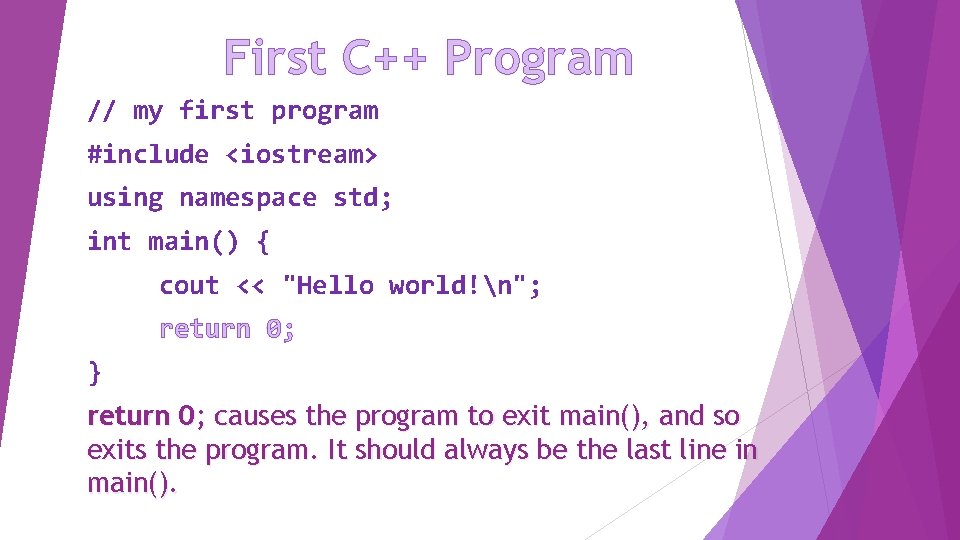
First C++ Program // my first program #include <iostream> using namespace std; int main() { cout << "Hello world!n"; return 0; } return 0; causes the program to exit main(), and so exits the program. It should always be the last line in main().
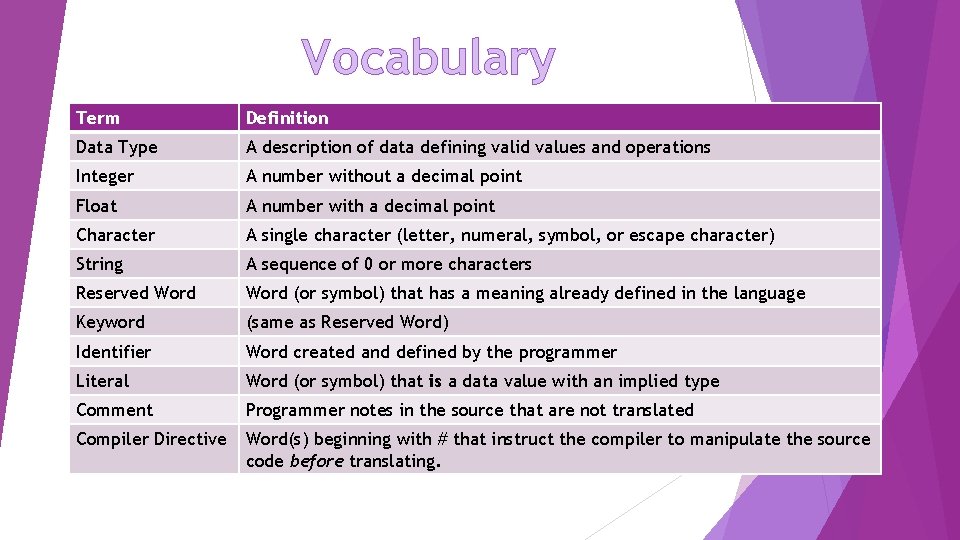
Vocabulary Term Definition Data Type A description of data defining valid values and operations Integer A number without a decimal point Float A number with a decimal point Character A single character (letter, numeral, symbol, or escape character) String A sequence of 0 or more characters Reserved Word (or symbol) that has a meaning already defined in the language Keyword (same as Reserved Word) Identifier Word created and defined by the programmer Literal Word (or symbol) that is a data value with an implied type Comment Programmer notes in the source that are not translated Compiler Directive Word(s) beginning with # that instruct the compiler to manipulate the source code before translating.
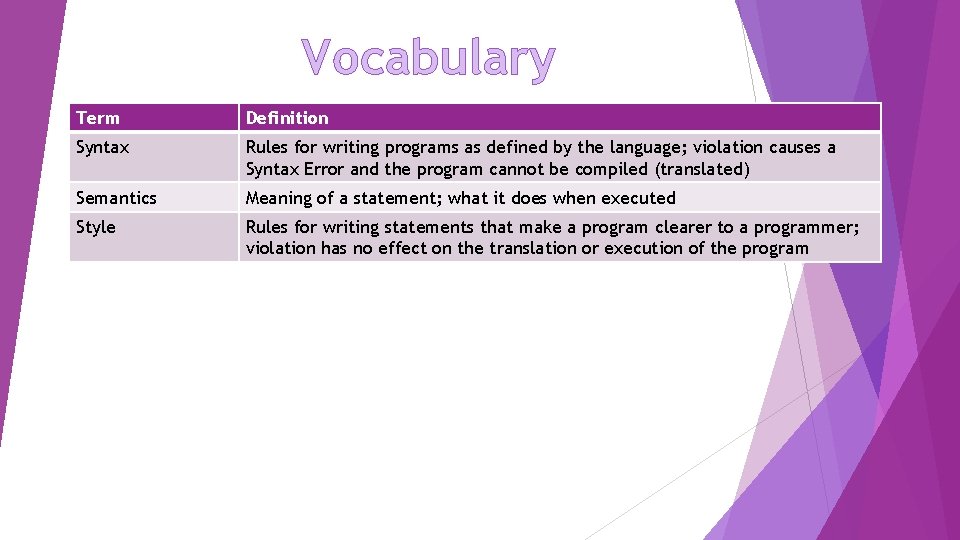
Vocabulary Term Definition Syntax Rules for writing programs as defined by the language; violation causes a Syntax Error and the program cannot be compiled (translated) Semantics Meaning of a statement; what it does when executed Style Rules for writing statements that make a program clearer to a programmer; violation has no effect on the translation or execution of the program