c Arrays For loop While loop Do while
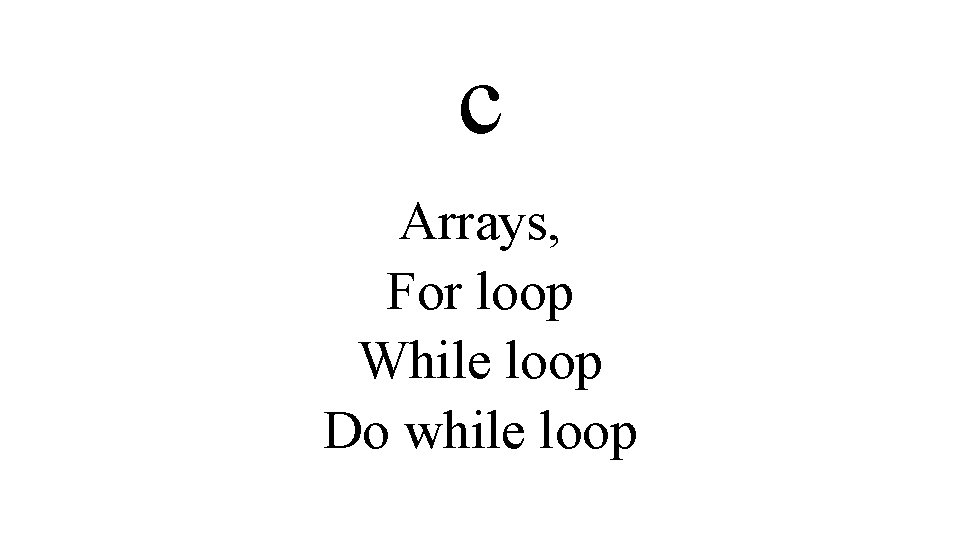
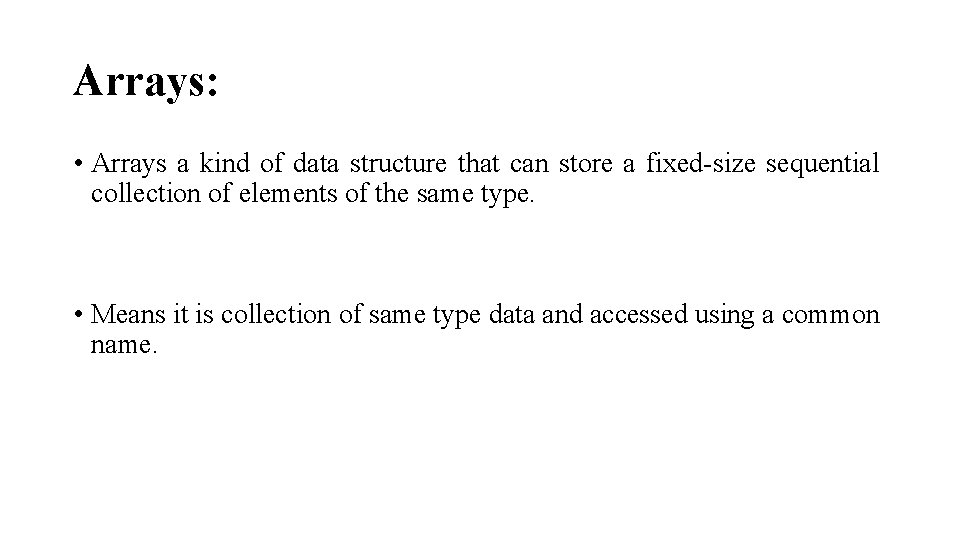
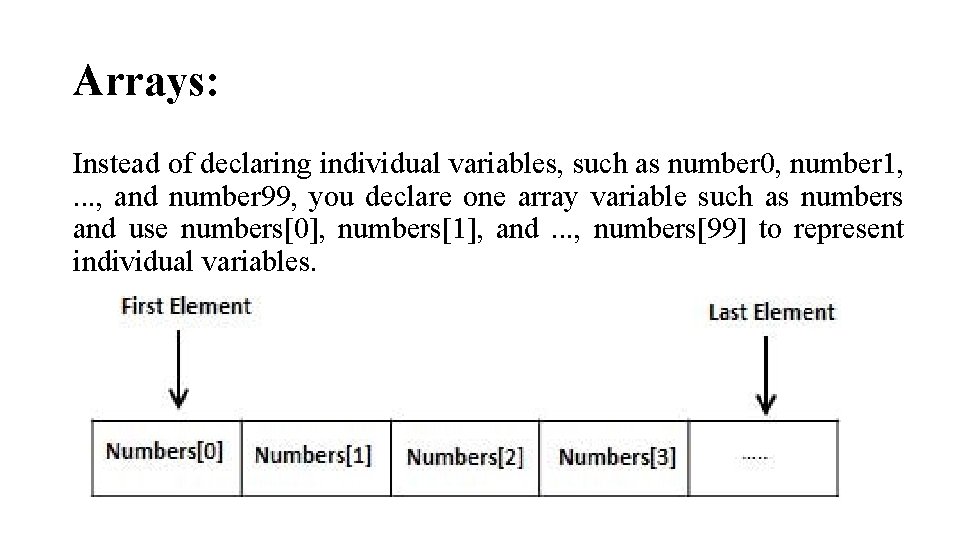
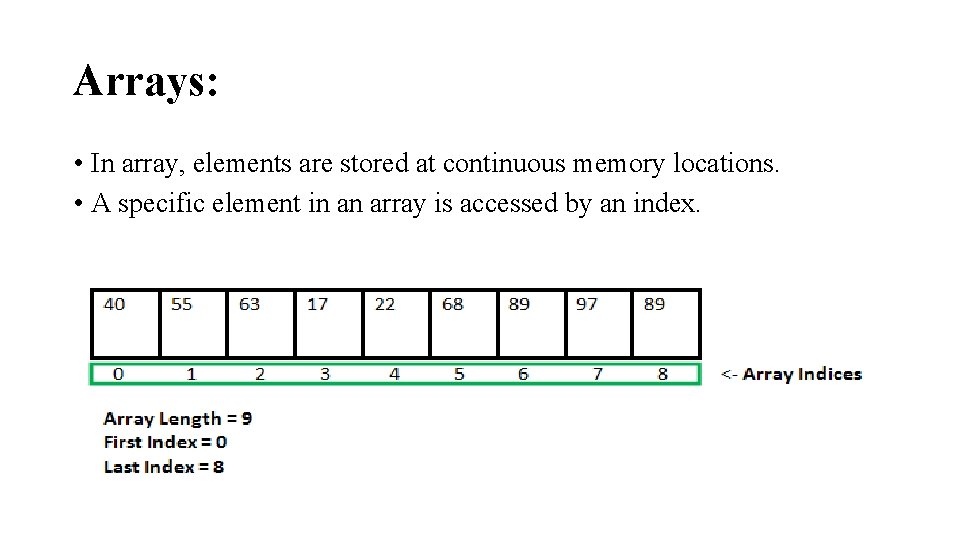
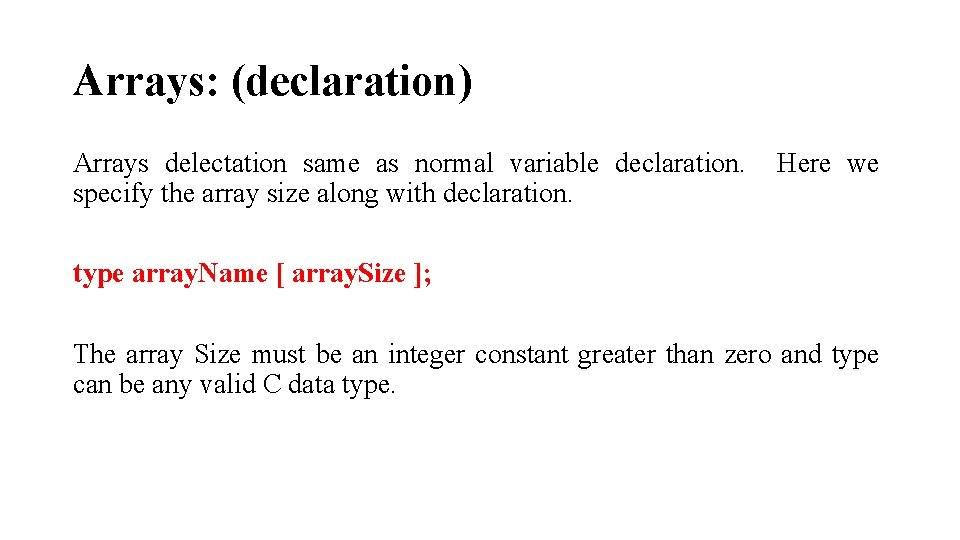
![Arrays: (declaration) Example • float mark[5]; // floating array • int a[10]; // integer Arrays: (declaration) Example • float mark[5]; // floating array • int a[10]; // integer](https://slidetodoc.com/presentation_image_h2/86de947d0039a5cd839ff34506b78d4c/image-6.jpg)
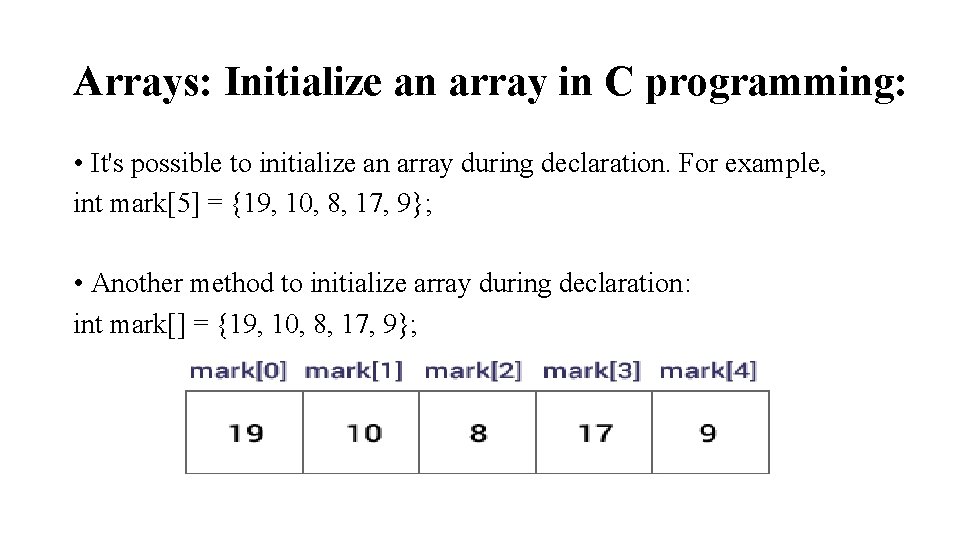
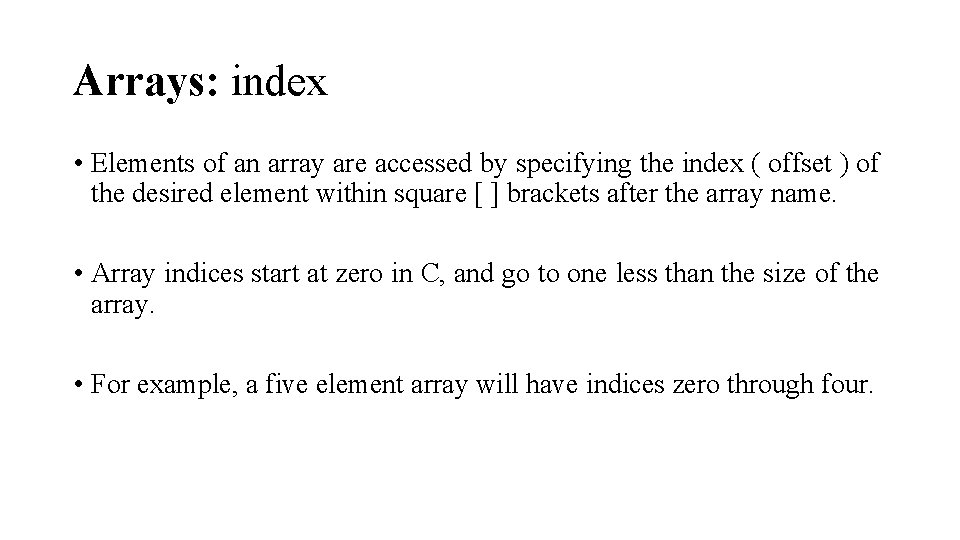
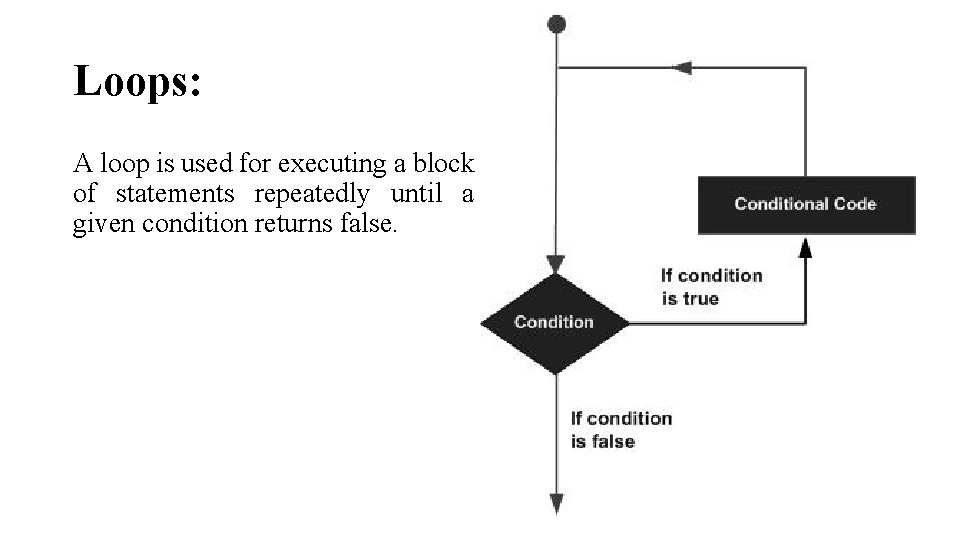
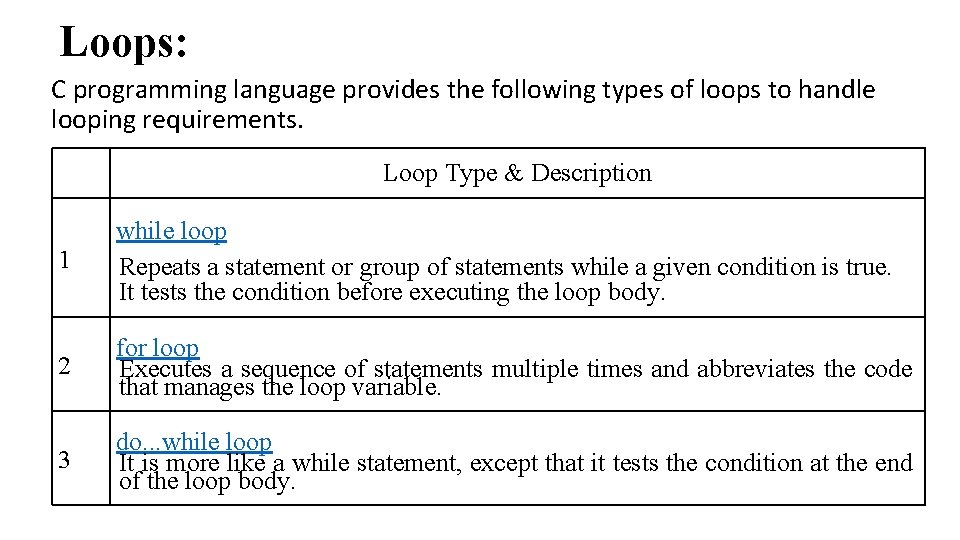
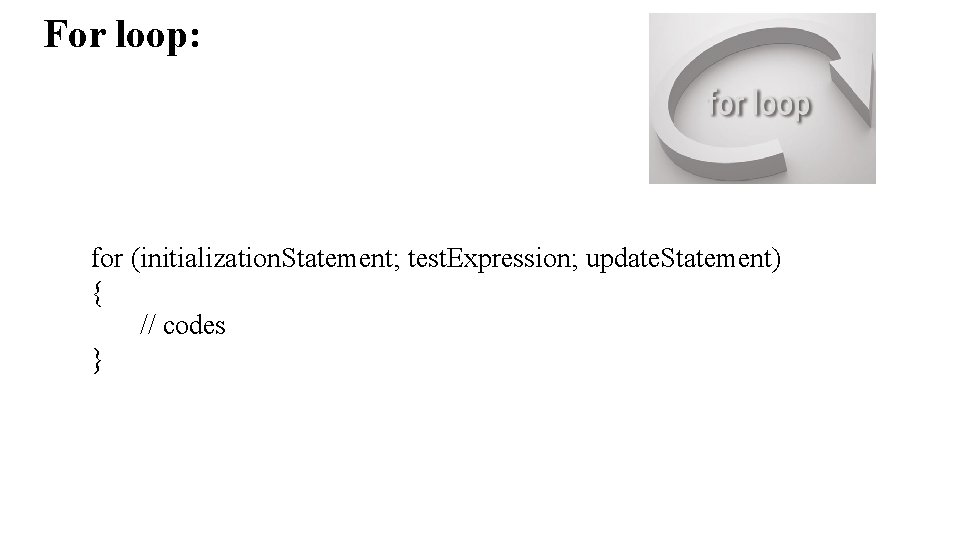
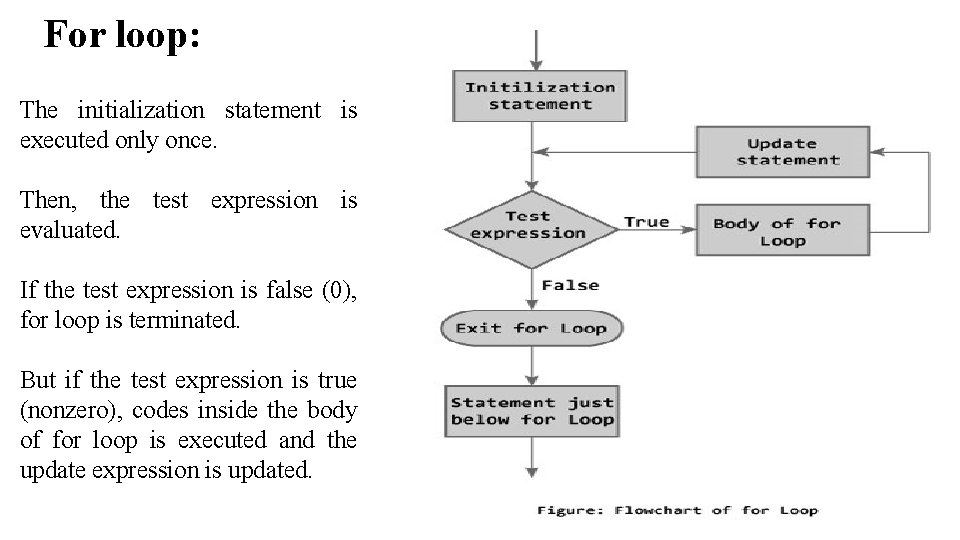
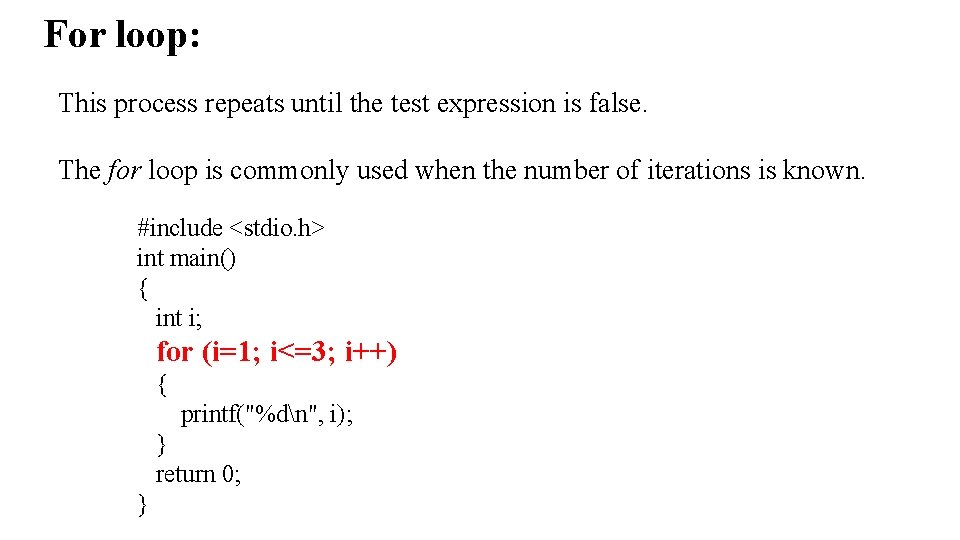
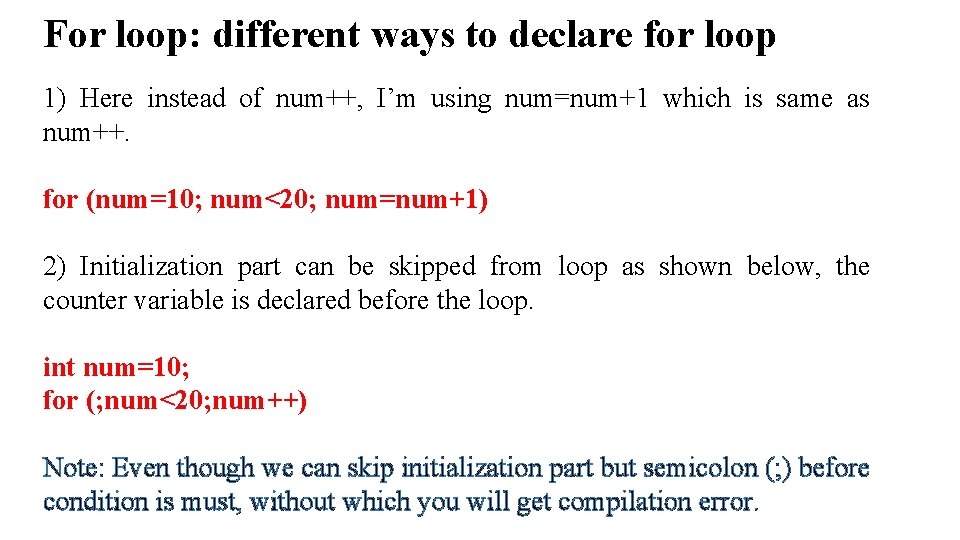
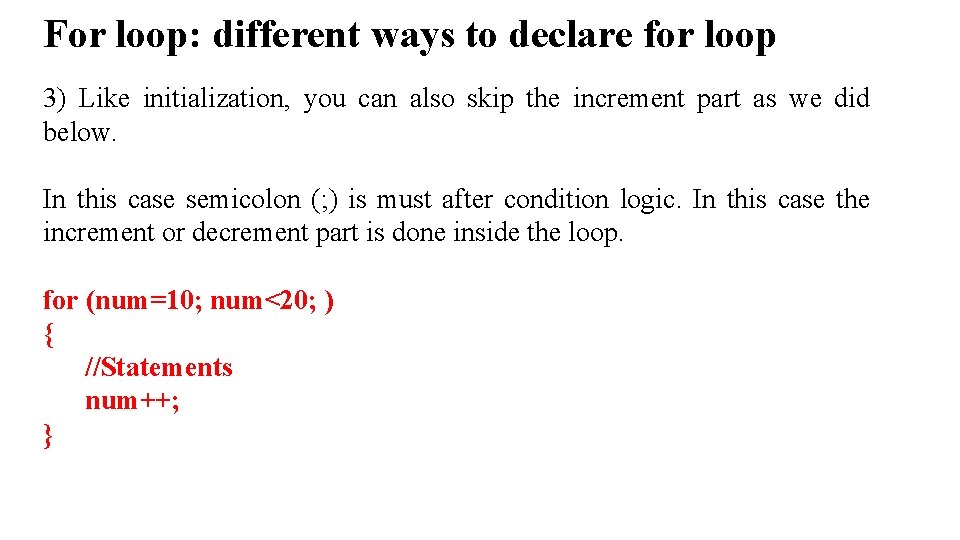
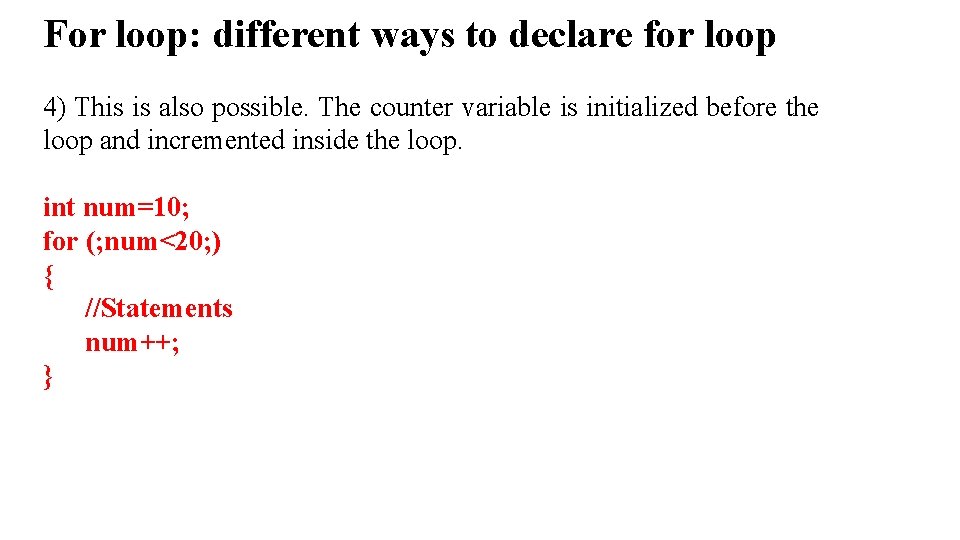
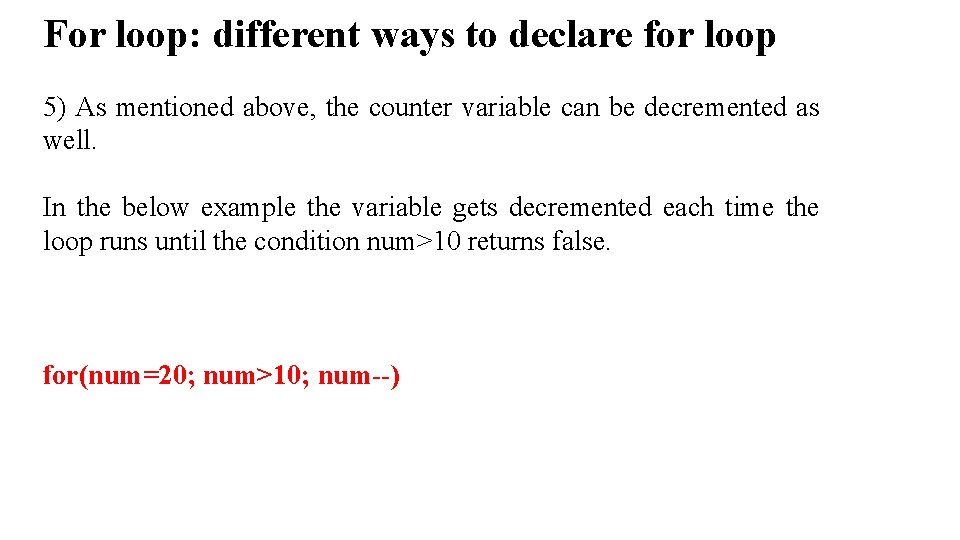
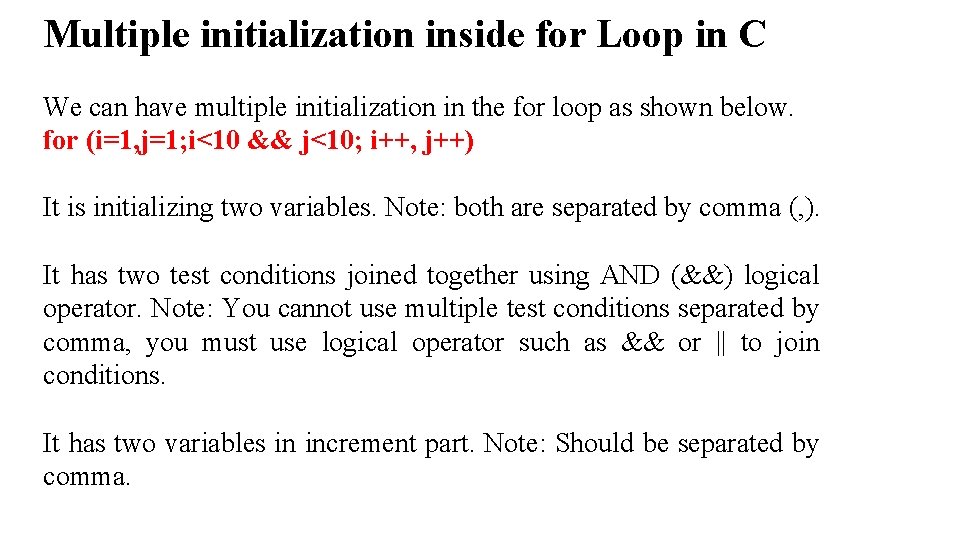
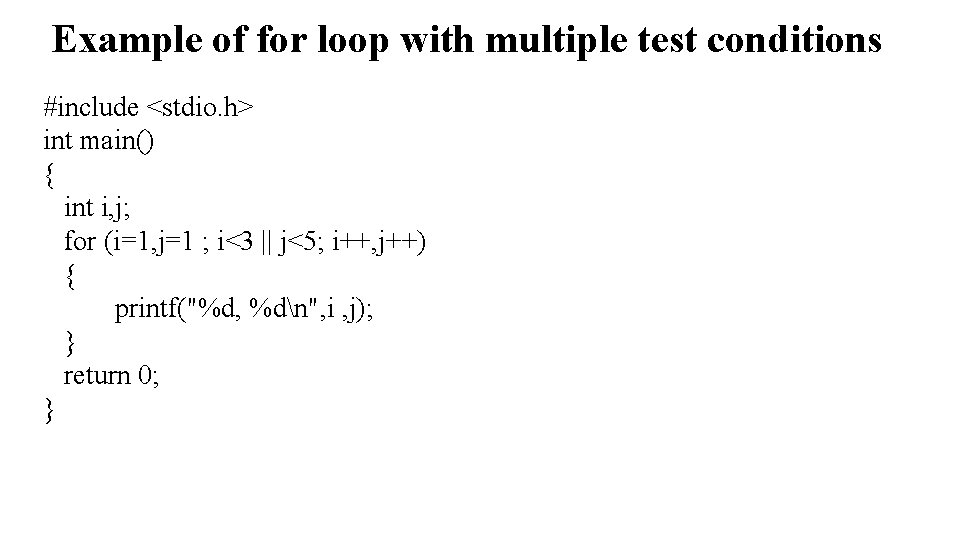
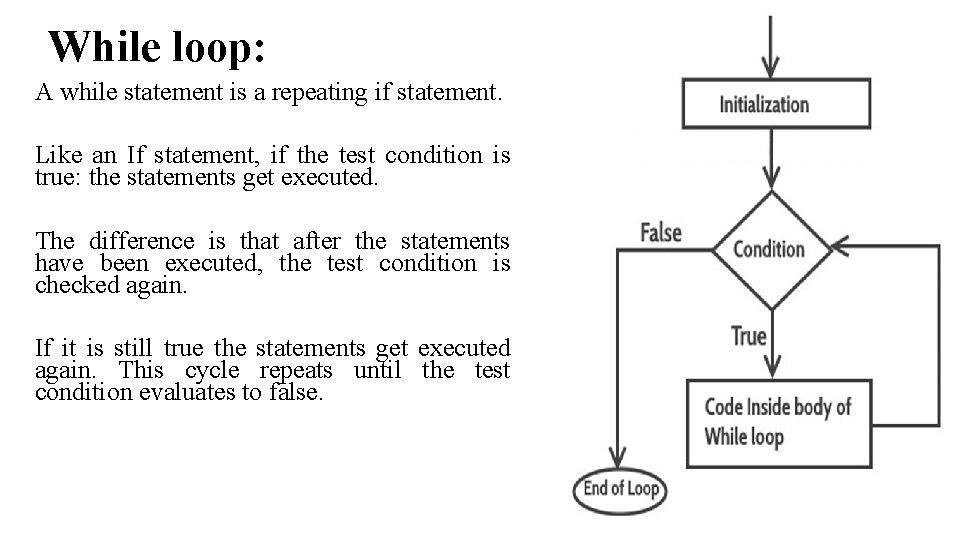
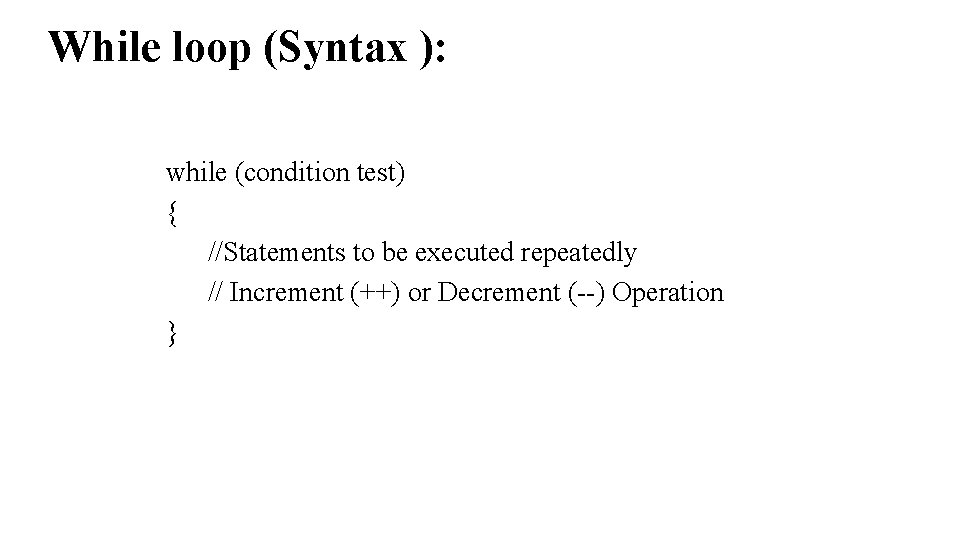
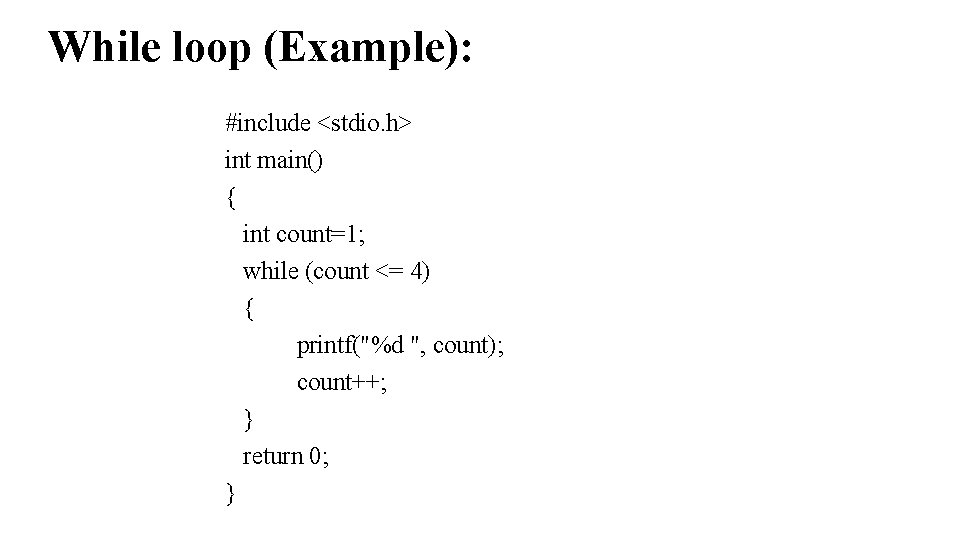
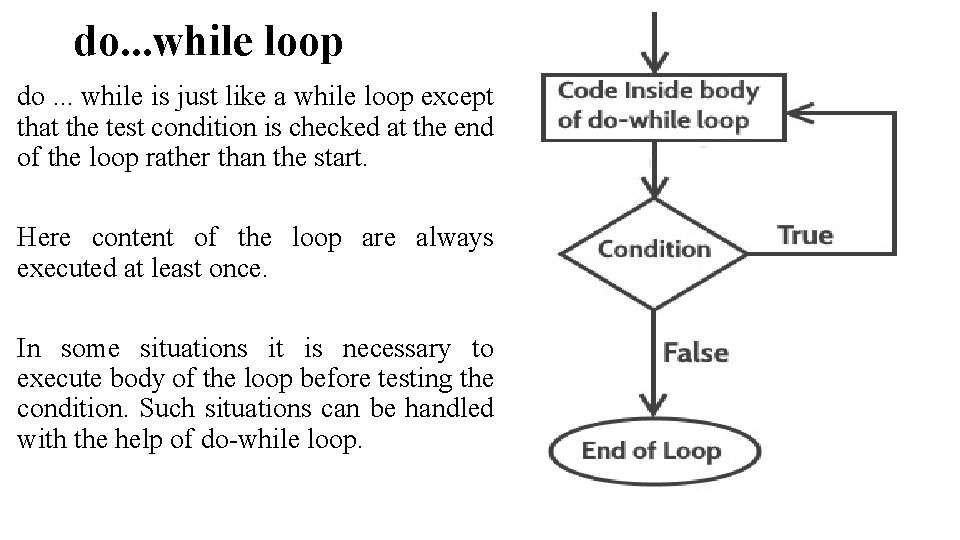
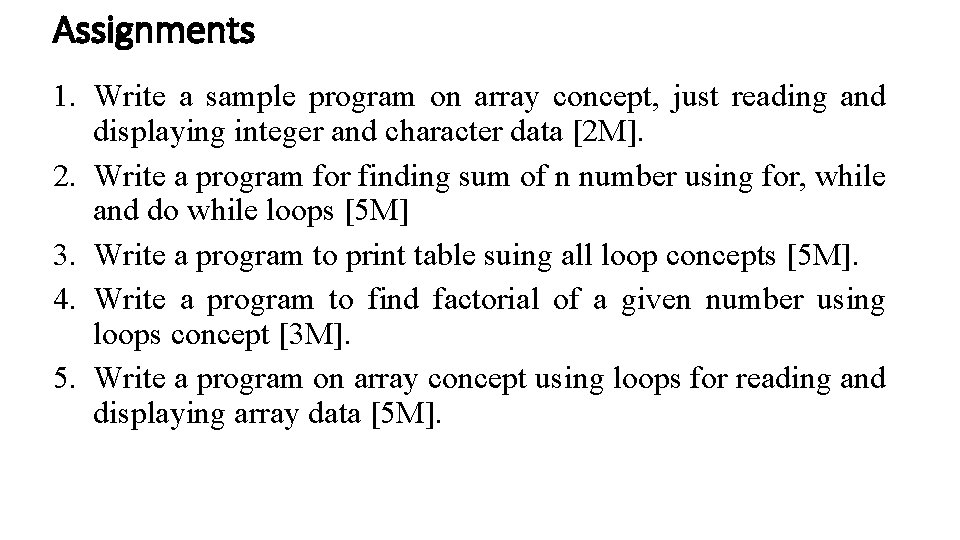
- Slides: 24
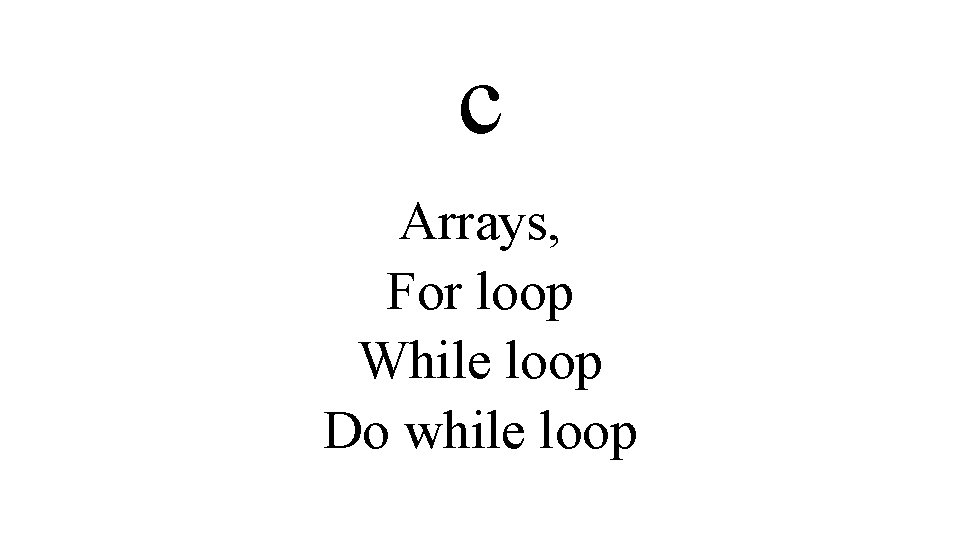
c Arrays, For loop While loop Do while loop
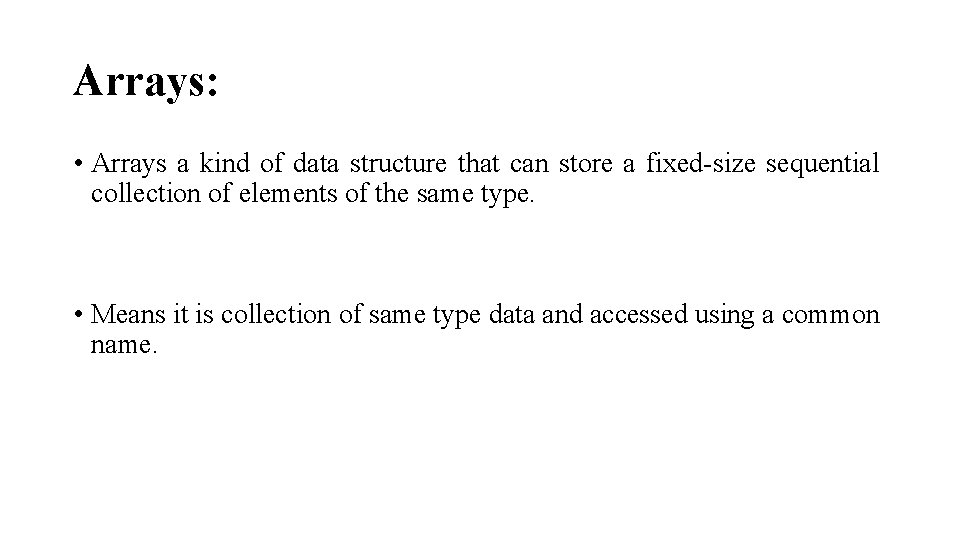
Arrays: • Arrays a kind of data structure that can store a fixed-size sequential collection of elements of the same type. • Means it is collection of same type data and accessed using a common name.
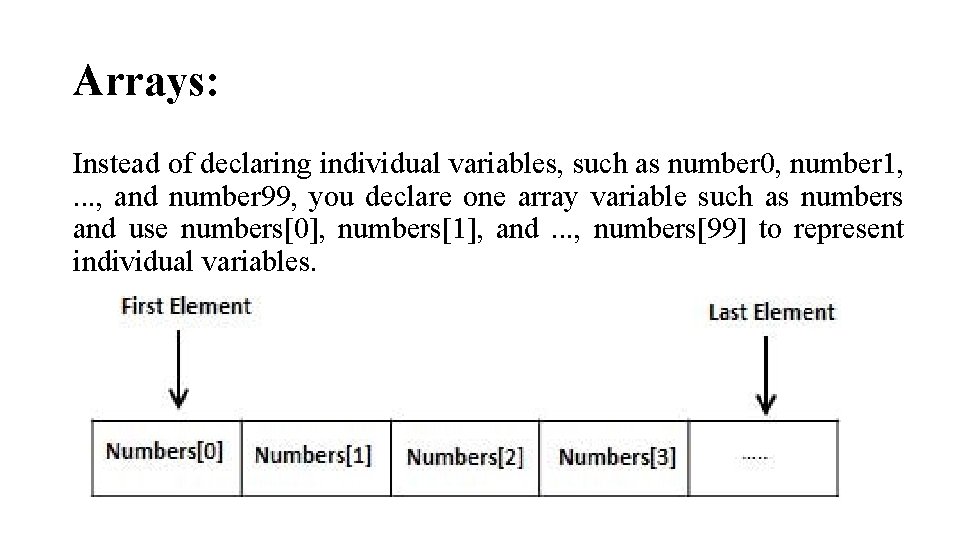
Arrays: Instead of declaring individual variables, such as number 0, number 1, . . . , and number 99, you declare one array variable such as numbers and use numbers[0], numbers[1], and. . . , numbers[99] to represent individual variables.
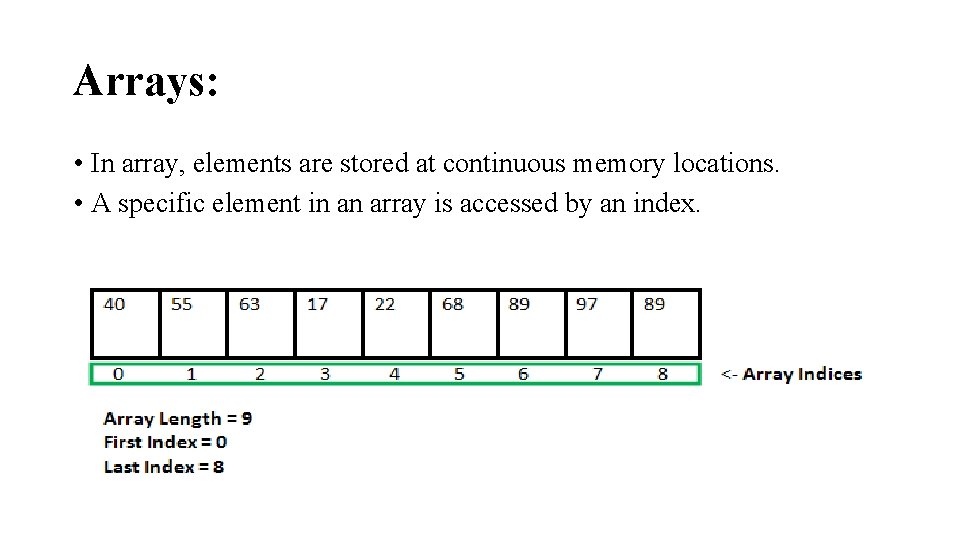
Arrays: • In array, elements are stored at continuous memory locations. • A specific element in an array is accessed by an index.
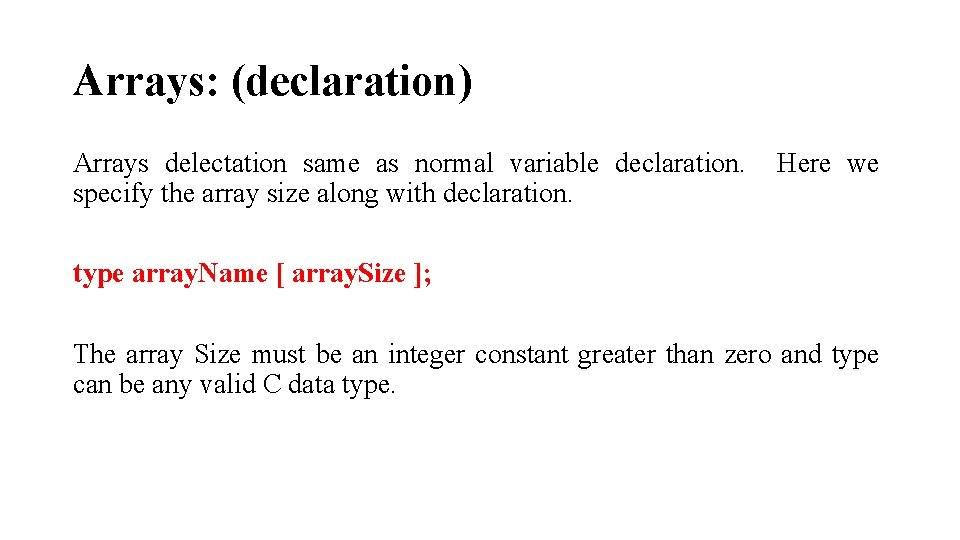
Arrays: (declaration) Arrays delectation same as normal variable declaration. specify the array size along with declaration. Here we type array. Name [ array. Size ]; The array Size must be an integer constant greater than zero and type can be any valid C data type.
![Arrays declaration Example float mark5 floating array int a10 integer Arrays: (declaration) Example • float mark[5]; // floating array • int a[10]; // integer](https://slidetodoc.com/presentation_image_h2/86de947d0039a5cd839ff34506b78d4c/image-6.jpg)
Arrays: (declaration) Example • float mark[5]; // floating array • int a[10]; // integer array • char b[10]; // character array i. e. string
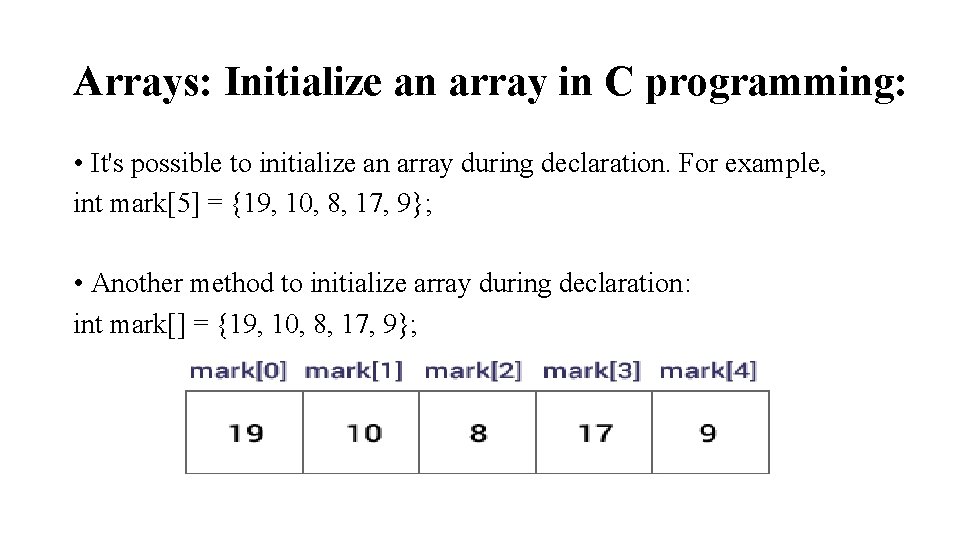
Arrays: Initialize an array in C programming: • It's possible to initialize an array during declaration. For example, int mark[5] = {19, 10, 8, 17, 9}; • Another method to initialize array during declaration: int mark[] = {19, 10, 8, 17, 9};
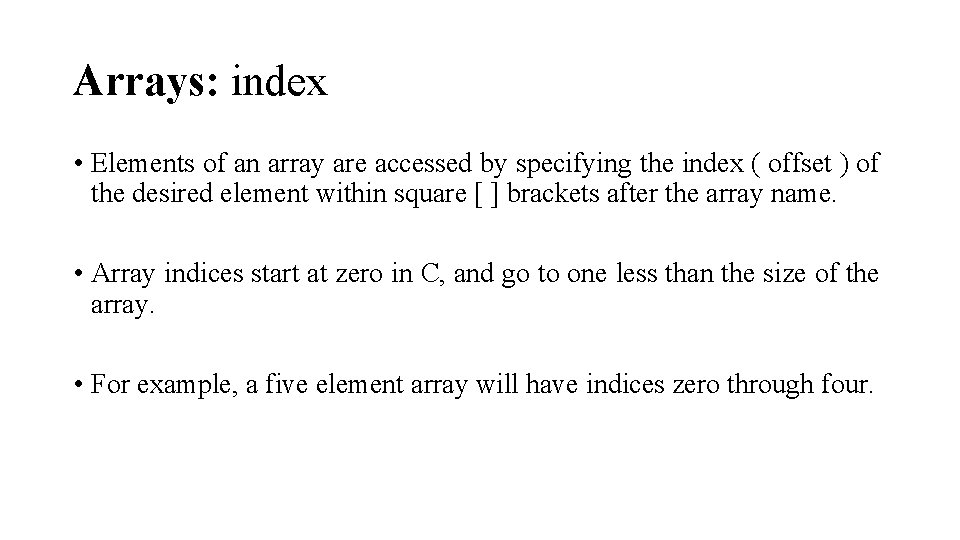
Arrays: index • Elements of an array are accessed by specifying the index ( offset ) of the desired element within square [ ] brackets after the array name. • Array indices start at zero in C, and go to one less than the size of the array. • For example, a five element array will have indices zero through four.
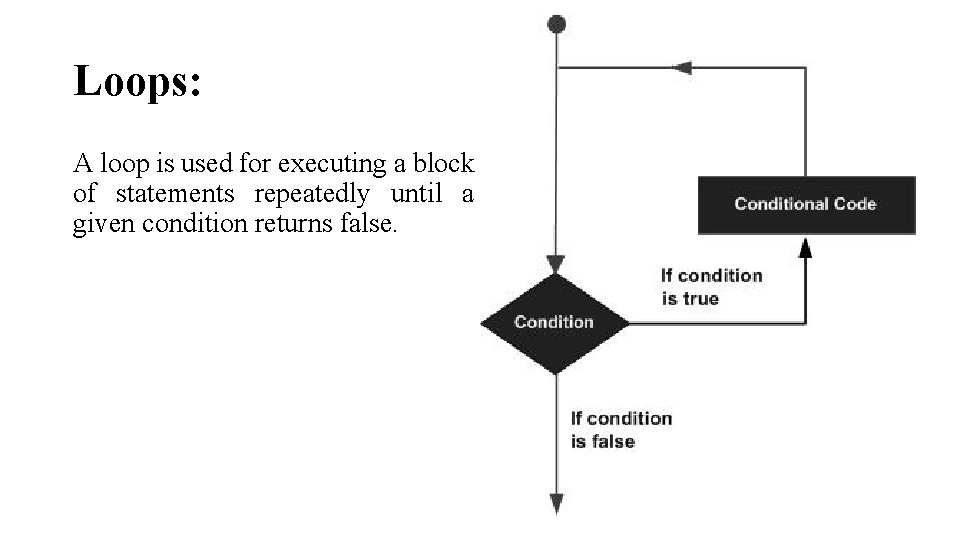
Loops: A loop is used for executing a block of statements repeatedly until a given condition returns false.
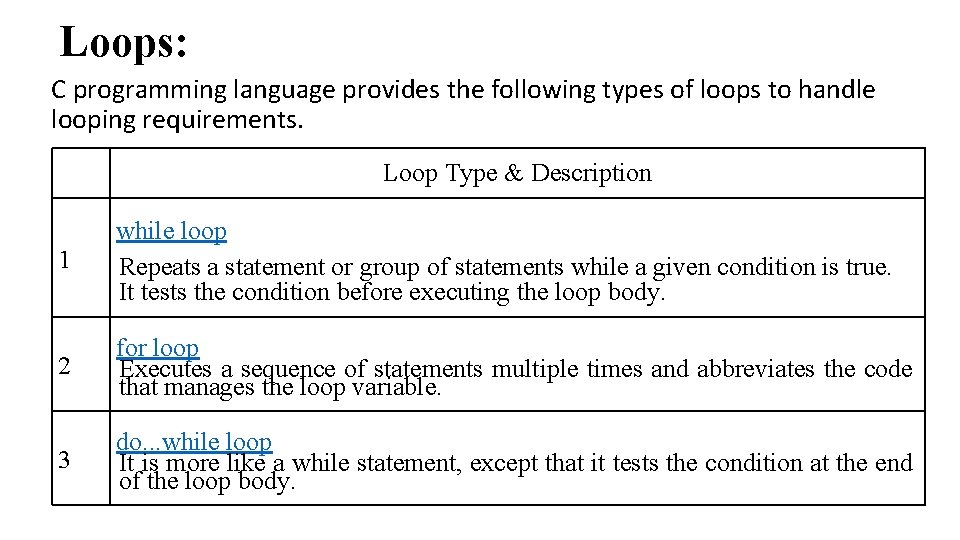
Loops: C programming language provides the following types of loops to handle looping requirements. Loop Type & Description 1 while loop Repeats a statement or group of statements while a given condition is true. It tests the condition before executing the loop body. 2 for loop Executes a sequence of statements multiple times and abbreviates the code that manages the loop variable. 3 do. . . while loop It is more like a while statement, except that it tests the condition at the end of the loop body.
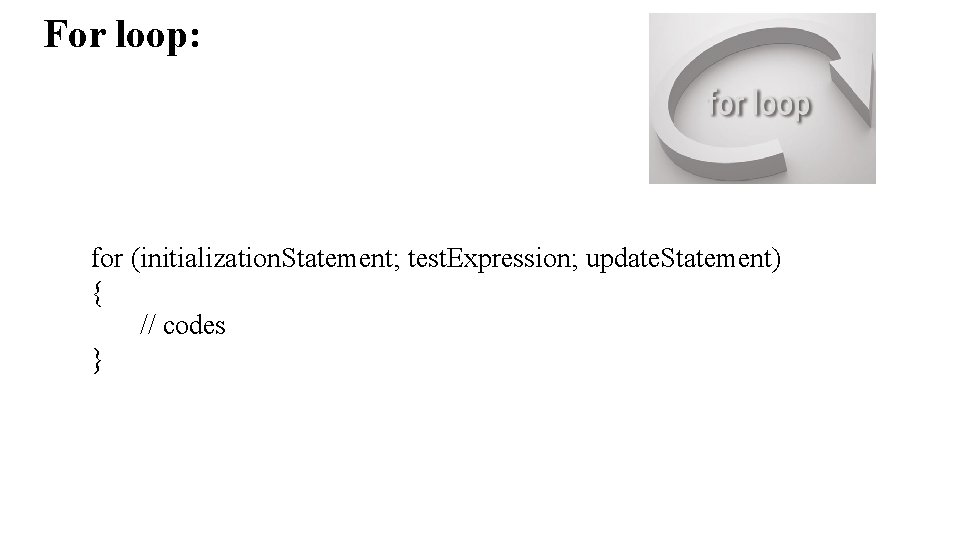
For loop: for (initialization. Statement; test. Expression; update. Statement) { // codes }
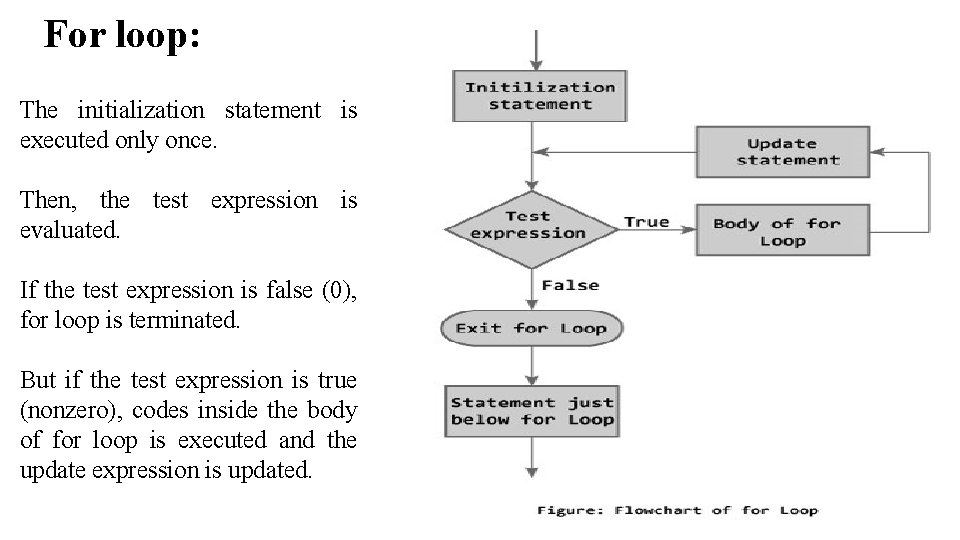
For loop: The initialization statement is executed only once. Then, the test expression is evaluated. If the test expression is false (0), for loop is terminated. But if the test expression is true (nonzero), codes inside the body of for loop is executed and the update expression is updated.
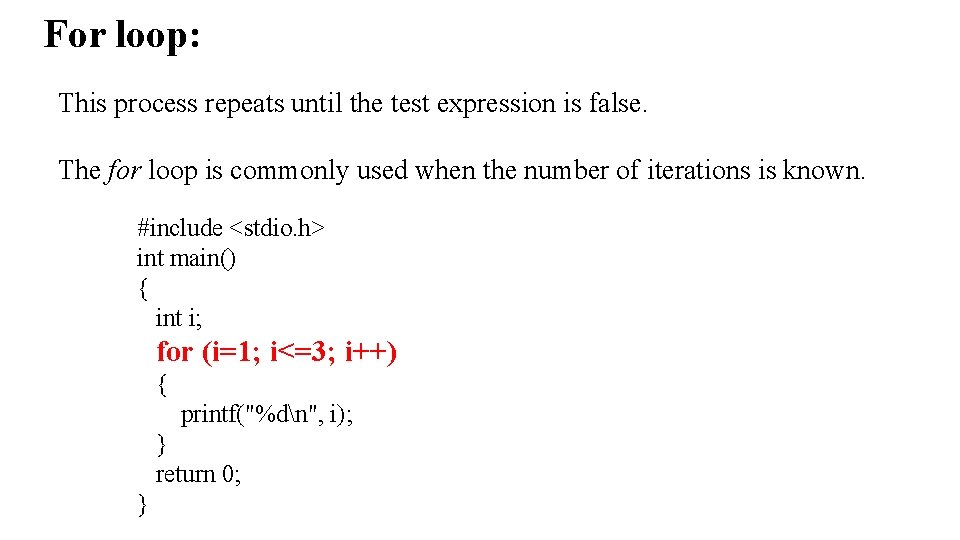
For loop: This process repeats until the test expression is false. The for loop is commonly used when the number of iterations is known. #include <stdio. h> int main() { int i; for (i=1; i<=3; i++) { printf("%dn", i); } return 0; }
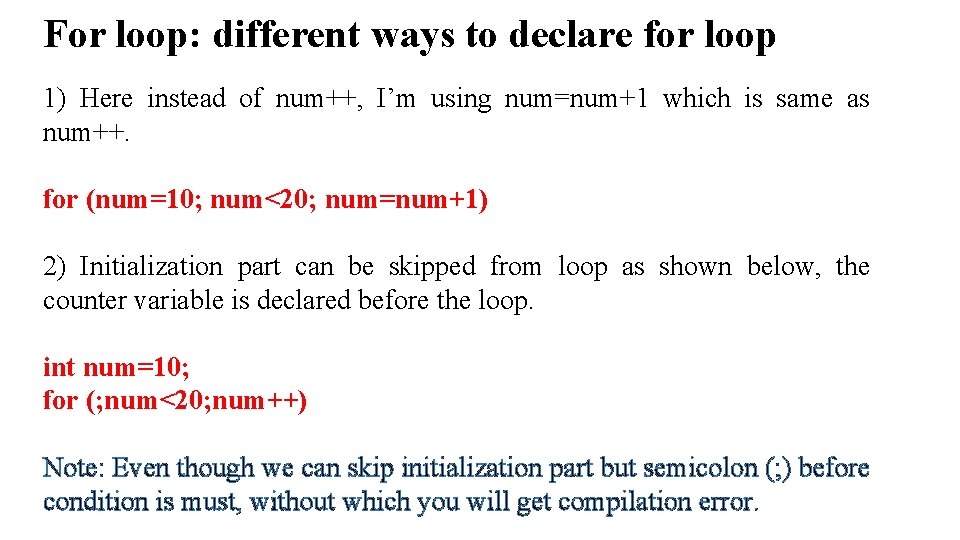
For loop: different ways to declare for loop 1) Here instead of num++, I’m using num=num+1 which is same as num++. for (num=10; num<20; num=num+1) 2) Initialization part can be skipped from loop as shown below, the counter variable is declared before the loop. int num=10; for (; num<20; num++) Note: Even though we can skip initialization part but semicolon (; ) before condition is must, without which you will get compilation error.
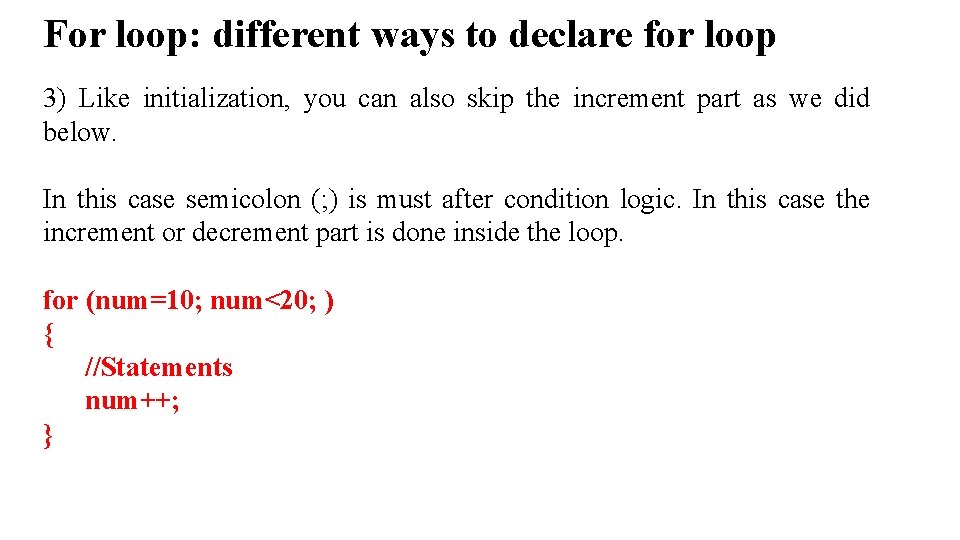
For loop: different ways to declare for loop 3) Like initialization, you can also skip the increment part as we did below. In this case semicolon (; ) is must after condition logic. In this case the increment or decrement part is done inside the loop. for (num=10; num<20; ) { //Statements num++; }
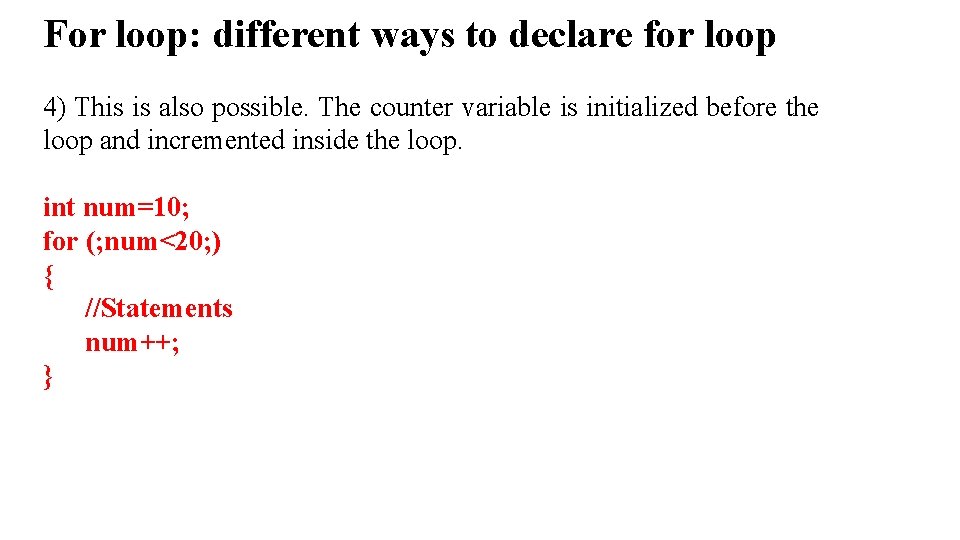
For loop: different ways to declare for loop 4) This is also possible. The counter variable is initialized before the loop and incremented inside the loop. int num=10; for (; num<20; ) { //Statements num++; }
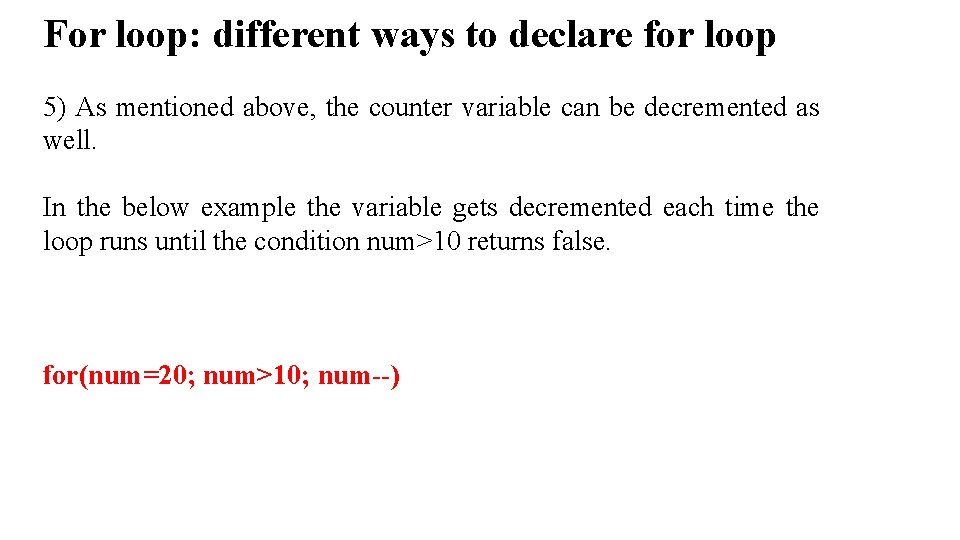
For loop: different ways to declare for loop 5) As mentioned above, the counter variable can be decremented as well. In the below example the variable gets decremented each time the loop runs until the condition num>10 returns false. for(num=20; num>10; num--)
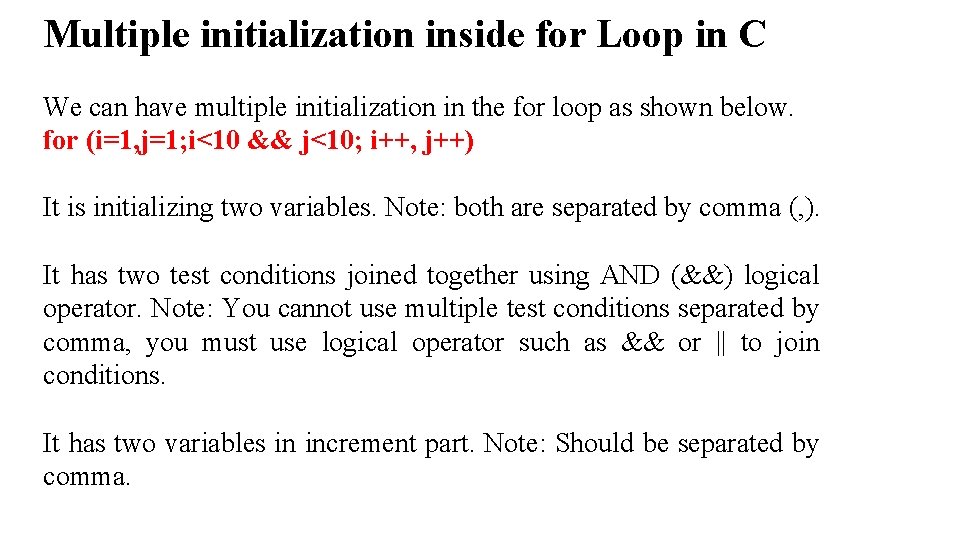
Multiple initialization inside for Loop in C We can have multiple initialization in the for loop as shown below. for (i=1, j=1; i<10 && j<10; i++, j++) It is initializing two variables. Note: both are separated by comma (, ). It has two test conditions joined together using AND (&&) logical operator. Note: You cannot use multiple test conditions separated by comma, you must use logical operator such as && or || to join conditions. It has two variables in increment part. Note: Should be separated by comma.
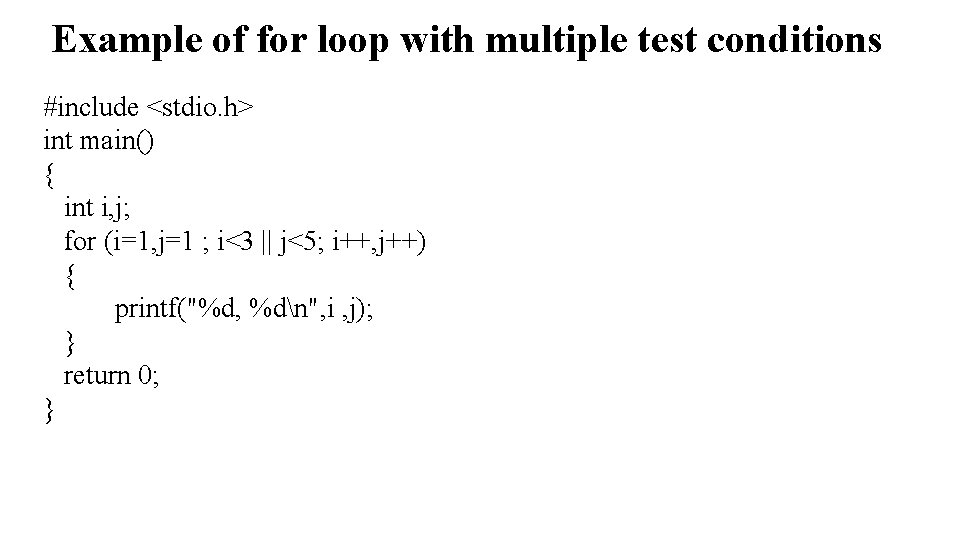
Example of for loop with multiple test conditions #include <stdio. h> int main() { int i, j; for (i=1, j=1 ; i<3 || j<5; i++, j++) { printf("%d, %dn", i , j); } return 0; }
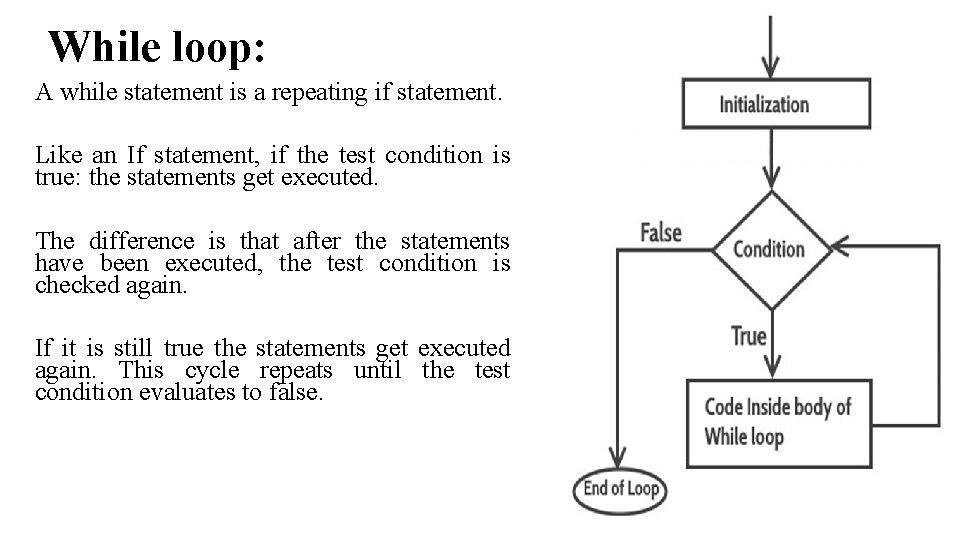
While loop: A while statement is a repeating if statement. Like an If statement, if the test condition is true: the statements get executed. The difference is that after the statements have been executed, the test condition is checked again. If it is still true the statements get executed again. This cycle repeats until the test condition evaluates to false.
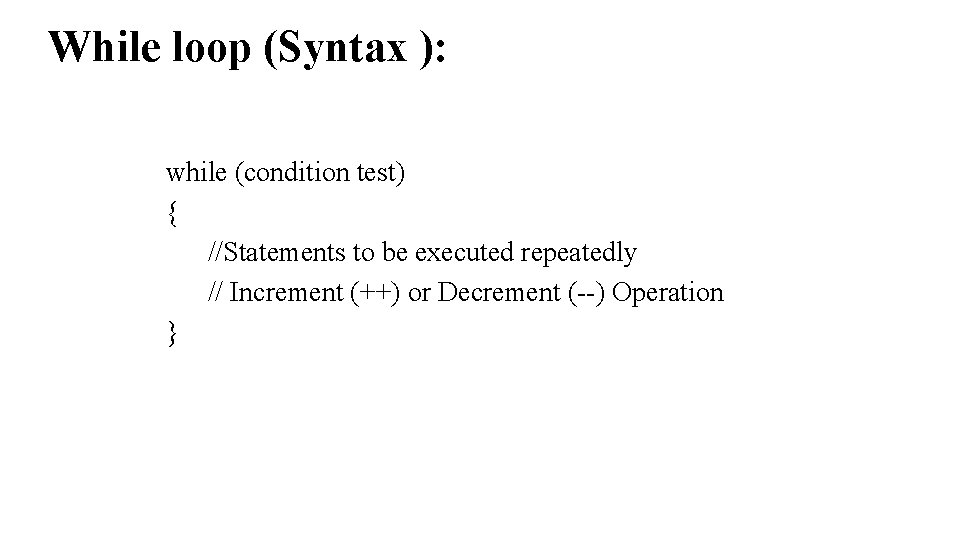
While loop (Syntax ): while (condition test) { //Statements to be executed repeatedly // Increment (++) or Decrement (--) Operation }
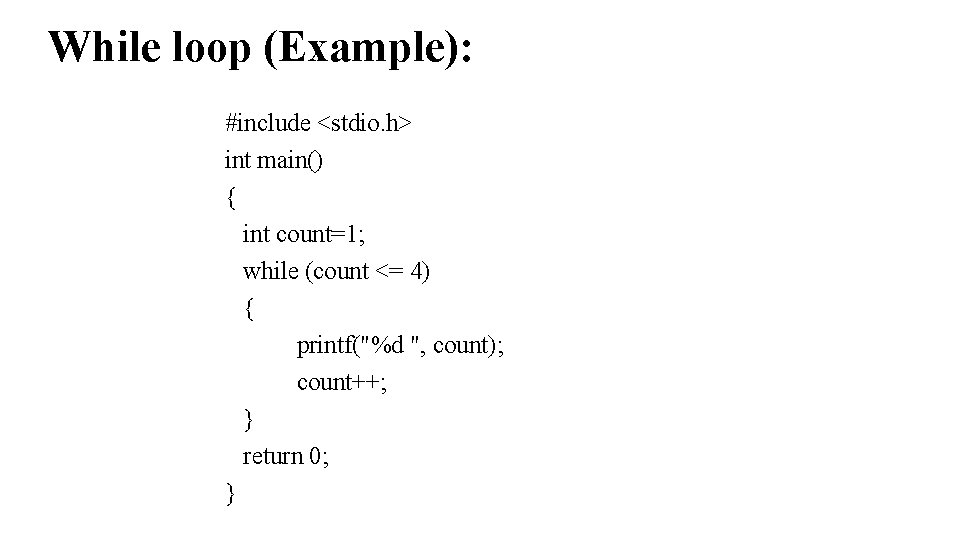
While loop (Example): #include <stdio. h> int main() { int count=1; while (count <= 4) { printf("%d ", count); count++; } return 0; }
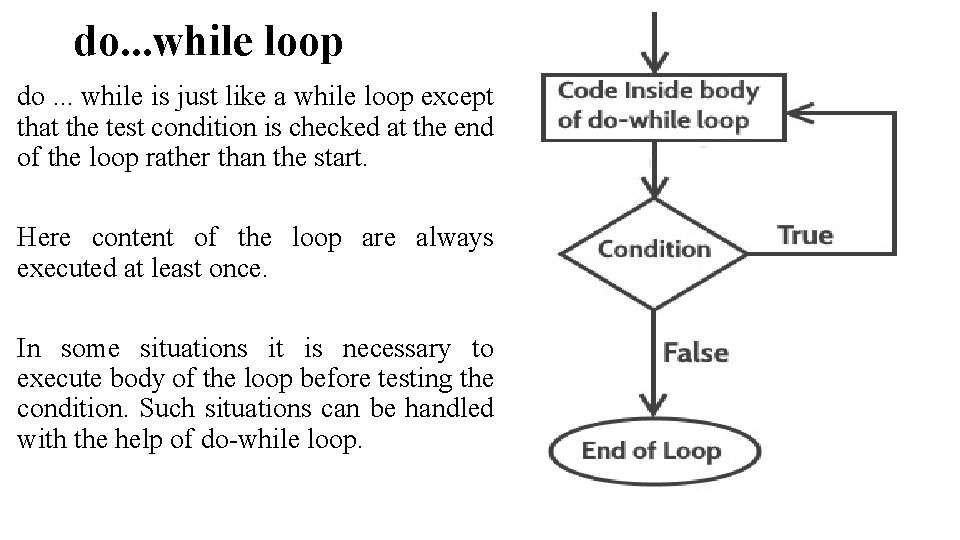
do. . . while loop do. . . while is just like a while loop except that the test condition is checked at the end of the loop rather than the start. Here content of the loop are always executed at least once. In some situations it is necessary to execute body of the loop before testing the condition. Such situations can be handled with the help of do-while loop.
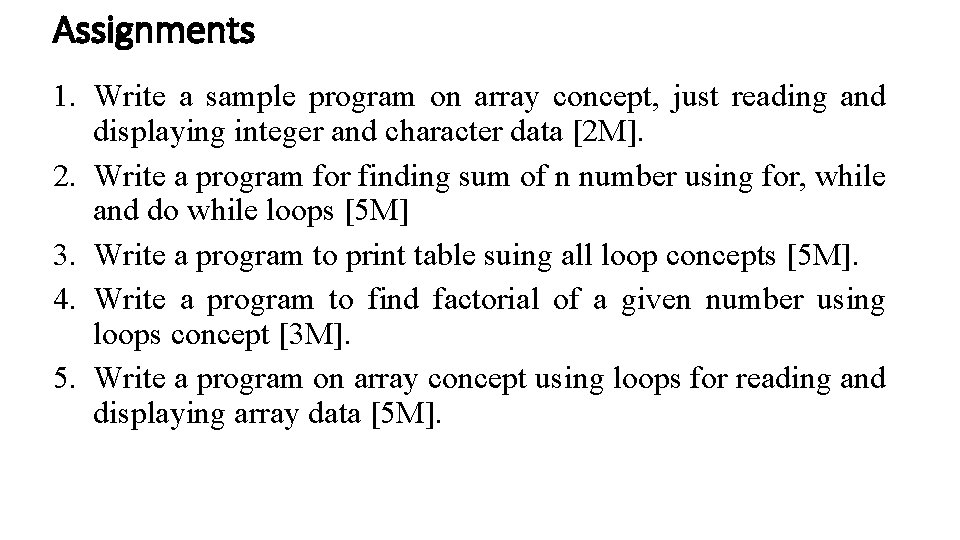
Assignments 1. Write a sample program on array concept, just reading and displaying integer and character data [2 M]. 2. Write a program for finding sum of n number using for, while and do while loops [5 M] 3. Write a program to print table suing all loop concepts [5 M]. 4. Write a program to find factorial of a given number using loops concept [3 M]. 5. Write a program on array concept using loops for reading and displaying array data [5 M].