Arrays Numeric array An array with a numeric
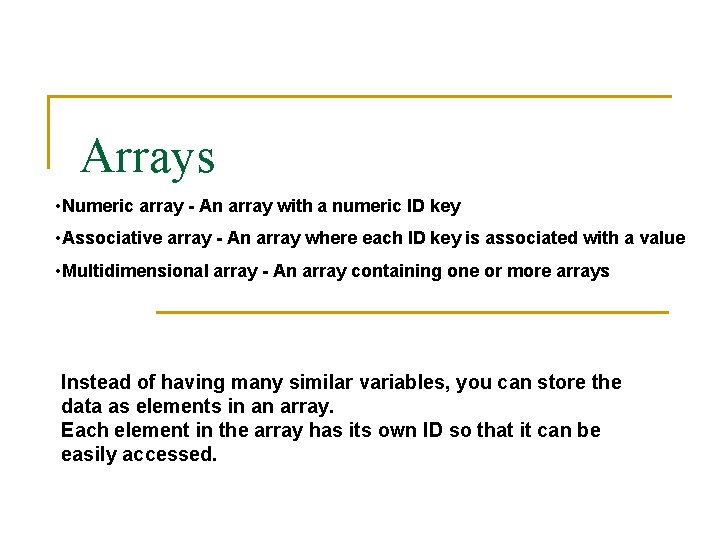
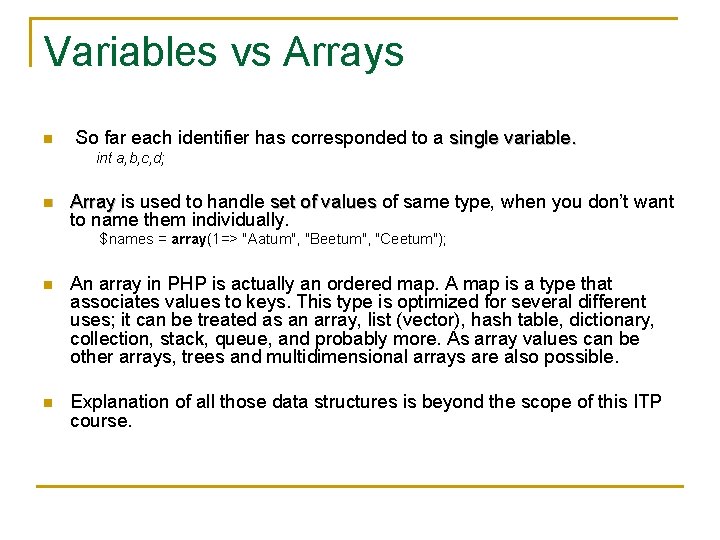
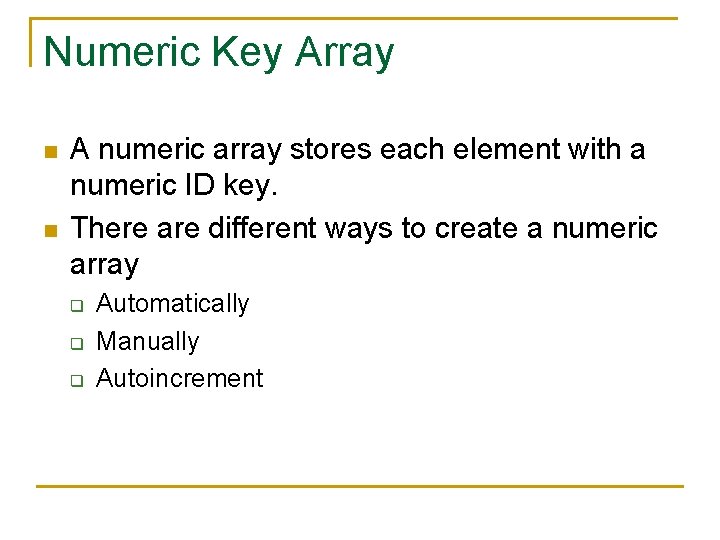
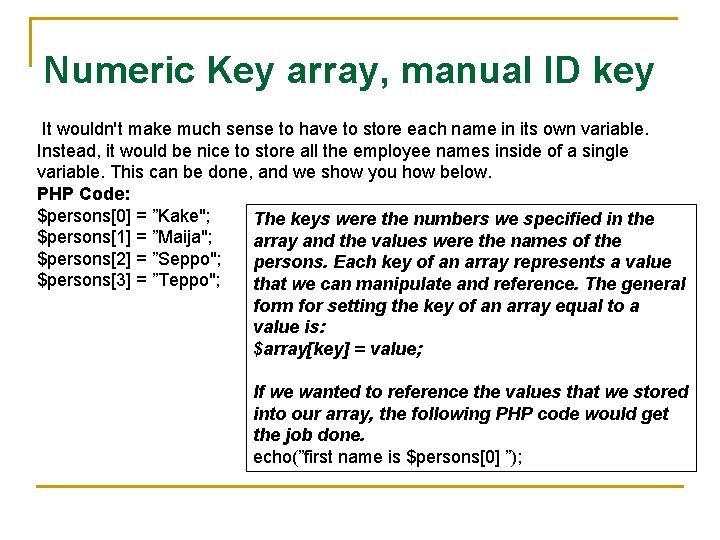
![Variations, numeric //1. Initializing Arrays key //Index auto generation $person[]= "Kalle Aaltonen"; //Autoincrement $person[]= Variations, numeric //1. Initializing Arrays key //Index auto generation $person[]= "Kalle Aaltonen"; //Autoincrement $person[]=](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-5.jpg)
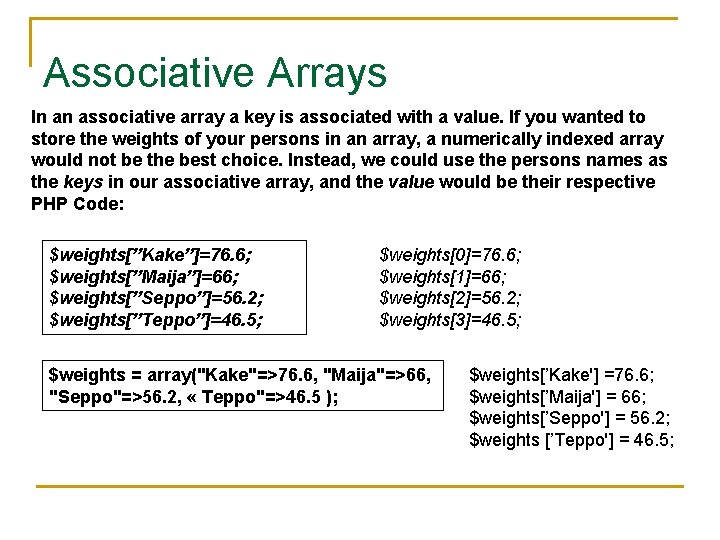
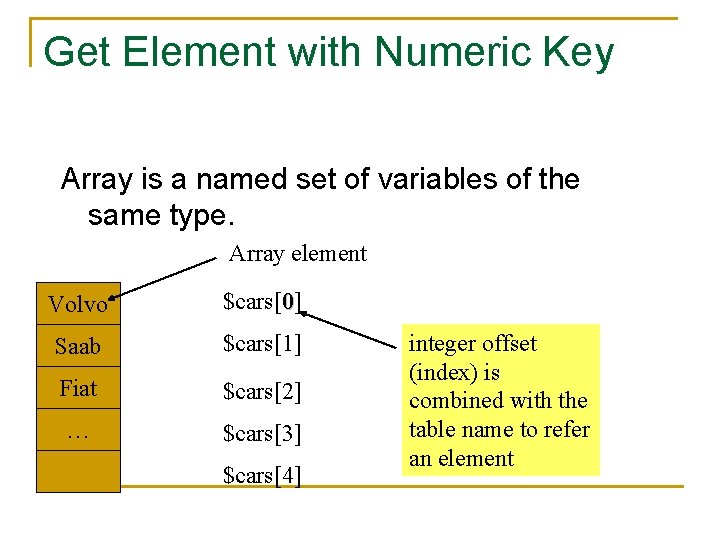
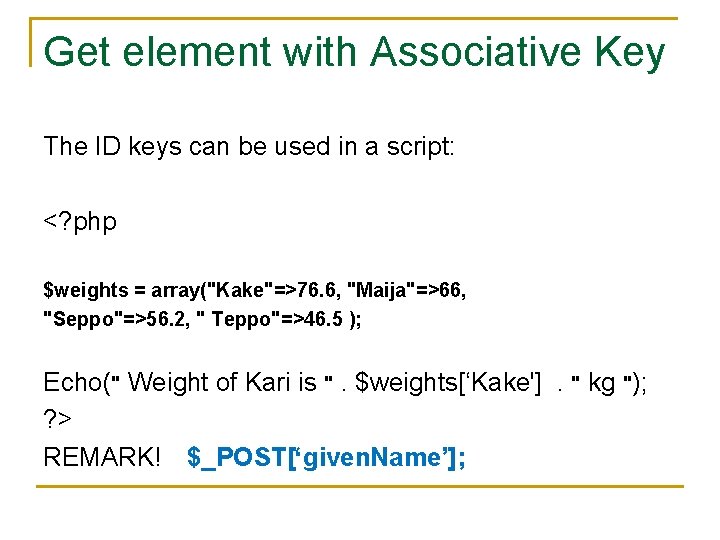
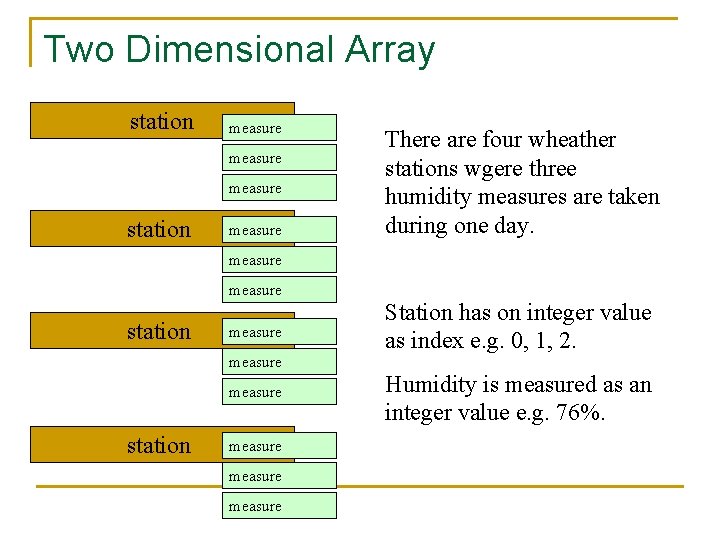
![station index = 0 humidity[][] humidity[0][0] = 65 humidity[0][1] = 75 humidity[0][2] = 85 station index = 0 humidity[][] humidity[0][0] = 65 humidity[0][1] = 75 humidity[0][2] = 85](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-10.jpg)
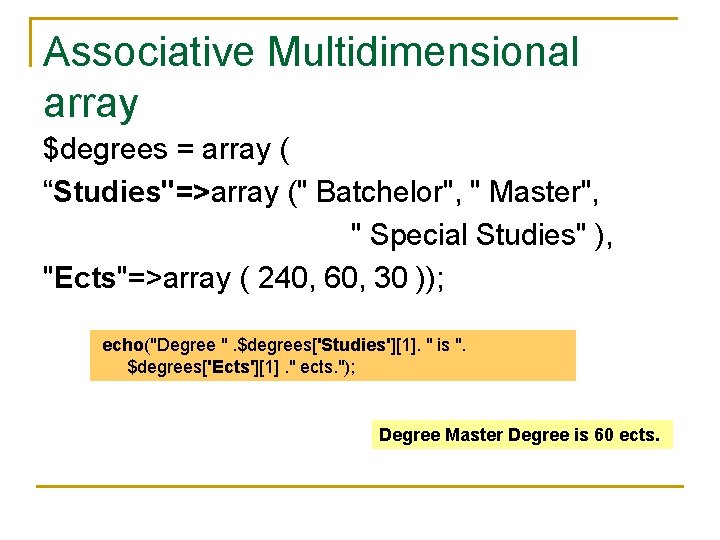
![Separate Arrays firstname[] lastname[] points[] [0] [1] [2] [3] [4] Use index to combine Separate Arrays firstname[] lastname[] points[] [0] [1] [2] [3] [4] Use index to combine](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-12.jpg)
![Multiple Arrays person[] weight[] height[] bmi[] [0] [1] [2] [3] Multiple Arrays person[] weight[] height[] bmi[] [0] [1] [2] [3]](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-13.jpg)
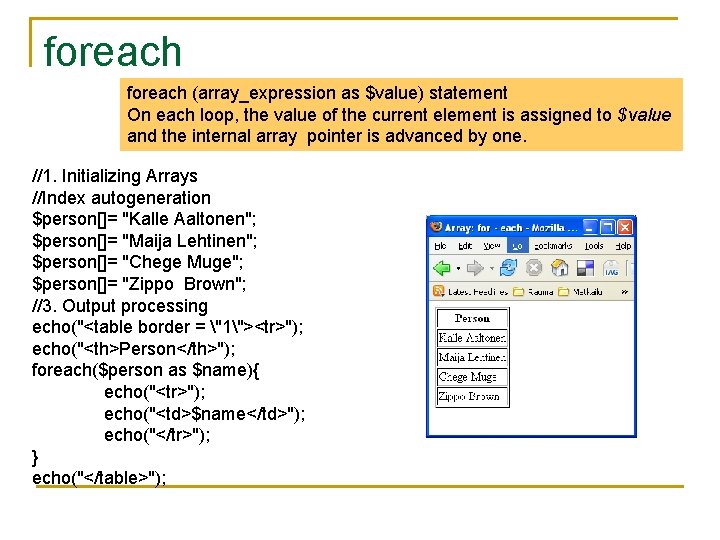
![count() //2. Calculation for($i=0; $i<count($person); $i++){ $bmi[$i]=$weight[$i]*$height[$i]; } Loop counter $i is used as count() //2. Calculation for($i=0; $i<count($person); $i++){ $bmi[$i]=$weight[$i]*$height[$i]; } Loop counter $i is used as](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-15.jpg)
![Multidimensional, assosiative //1. Initializing Multidimensional //name, weight, height $team[0]= array('name' => 'Kalle Aaltonen', 'weight'=>89. Multidimensional, assosiative //1. Initializing Multidimensional //name, weight, height $team[0]= array('name' => 'Kalle Aaltonen', 'weight'=>89.](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-16.jpg)
![Multidimensional, for //Loop all rows (=person) for($i=0; $i<count($team); $i++){ echo("<tr>"); echo("<td>". $team[$i]["name"]. "</td><td>". $team[$i]["weight"]. Multidimensional, for //Loop all rows (=person) for($i=0; $i<count($team); $i++){ echo("<tr>"); echo("<td>". $team[$i]["name"]. "</td><td>". $team[$i]["weight"].](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-17.jpg)
![Multidimensional, foreach /Loop all rows (=person) foreach( $team as $person){ echo("<tr>"); echo("<td>". $person["name"]. "</td><td>". Multidimensional, foreach /Loop all rows (=person) foreach( $team as $person){ echo("<tr>"); echo("<td>". $person["name"]. "</td><td>".](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-18.jpg)
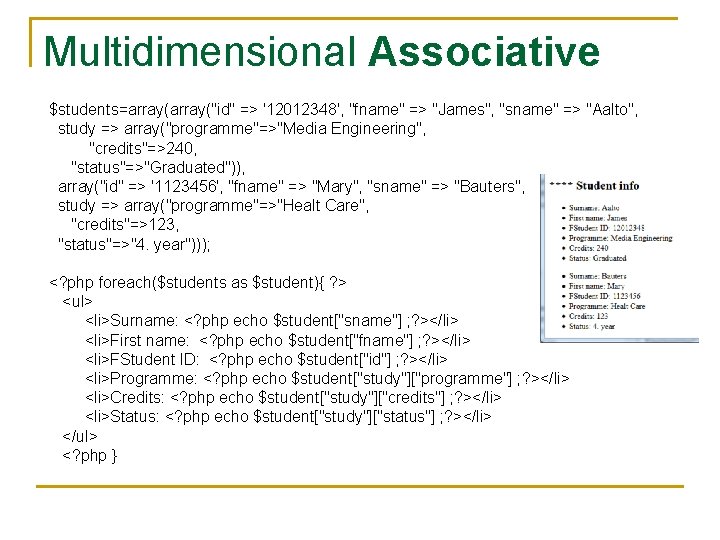
![Multidimensional, associative, new element on the fly //1. Initializing Multidimensional //name, weight, height $team[0]= Multidimensional, associative, new element on the fly //1. Initializing Multidimensional //name, weight, height $team[0]=](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-20.jpg)
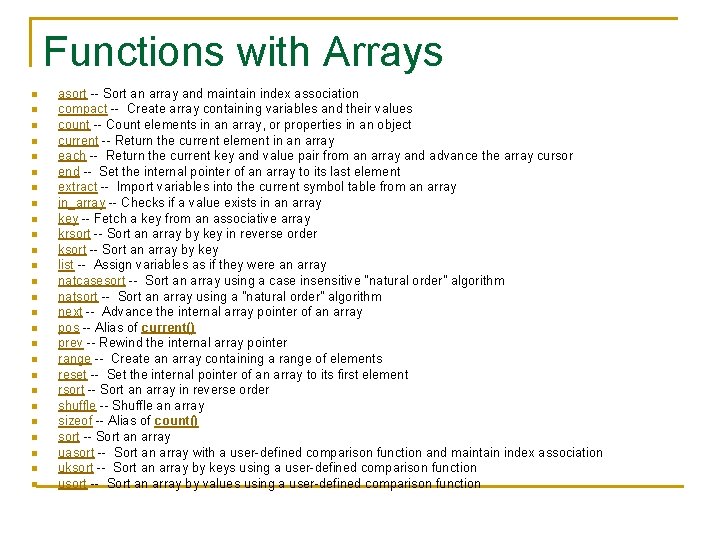
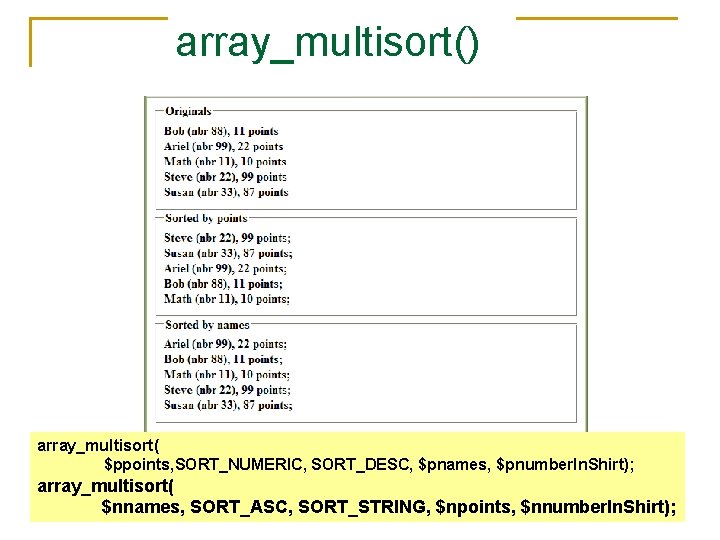
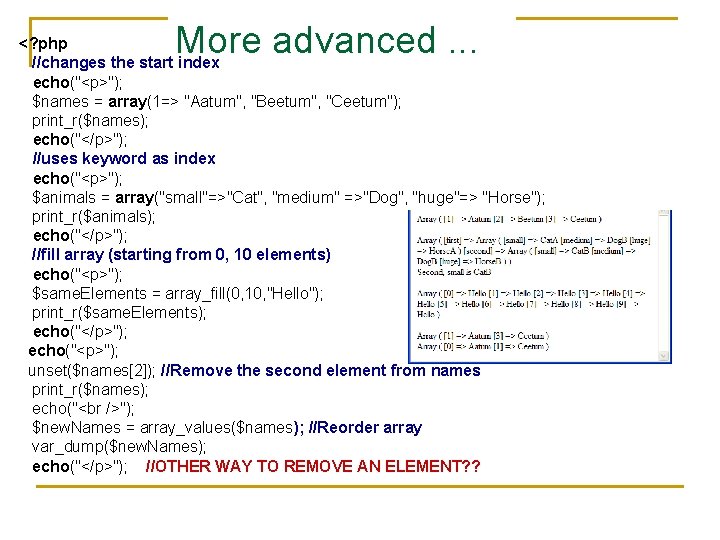
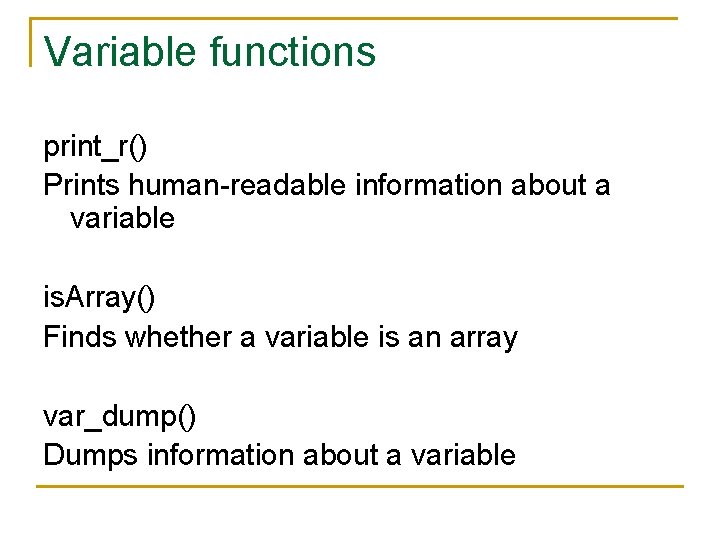
![Forms and Arrays for($i=0; $i<PERSONS; $i++){ echo("<tr>". "<td><input type="text" name=“given. Names[]“ /></td>". "<td><input type="text" Forms and Arrays for($i=0; $i<PERSONS; $i++){ echo("<tr>". "<td><input type="text" name=“given. Names[]“ /></td>". "<td><input type="text"](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-25.jpg)
- Slides: 25
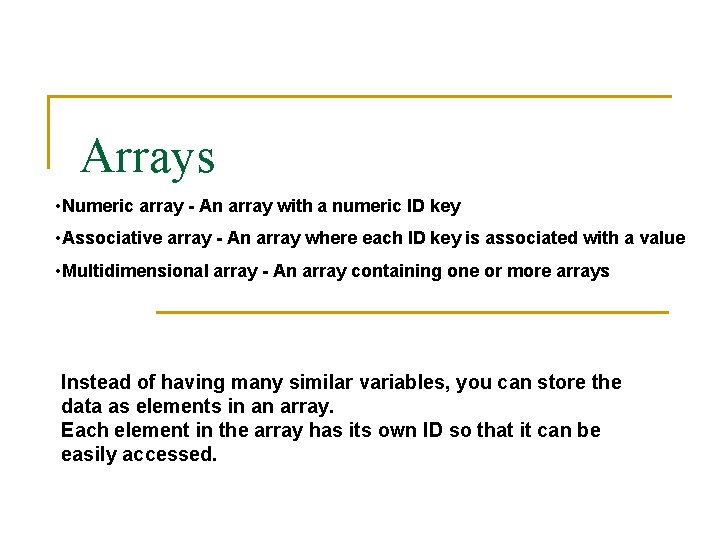
Arrays • Numeric array - An array with a numeric ID key • Associative array - An array where each ID key is associated with a value • Multidimensional array - An array containing one or more arrays Instead of having many similar variables, you can store the data as elements in an array. Each element in the array has its own ID so that it can be easily accessed.
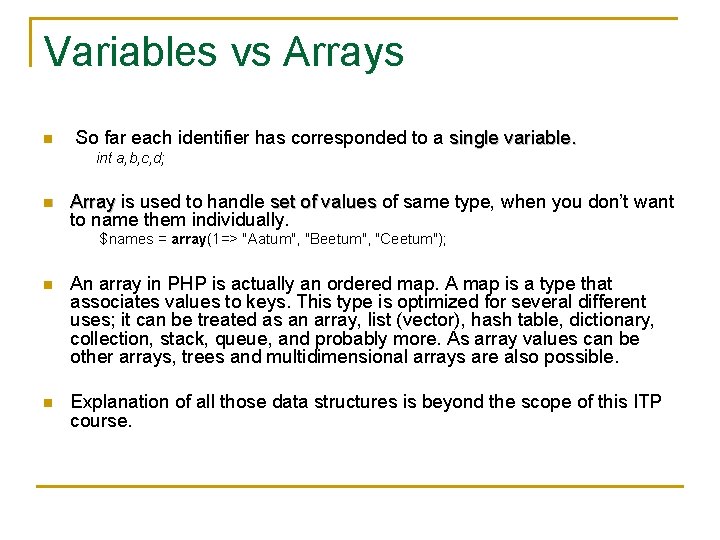
Variables vs Arrays n So far each identifier has corresponded to a single variable. int a, b, c, d; n Array is used to handle set of values of same type, when you don’t want to name them individually. $names = array(1=> "Aatum", "Beetum", "Ceetum"); n An array in PHP is actually an ordered map. A map is a type that associates values to keys. This type is optimized for several different uses; it can be treated as an array, list (vector), hash table, dictionary, collection, stack, queue, and probably more. As array values can be other arrays, trees and multidimensional arrays are also possible. n Explanation of all those data structures is beyond the scope of this ITP course.
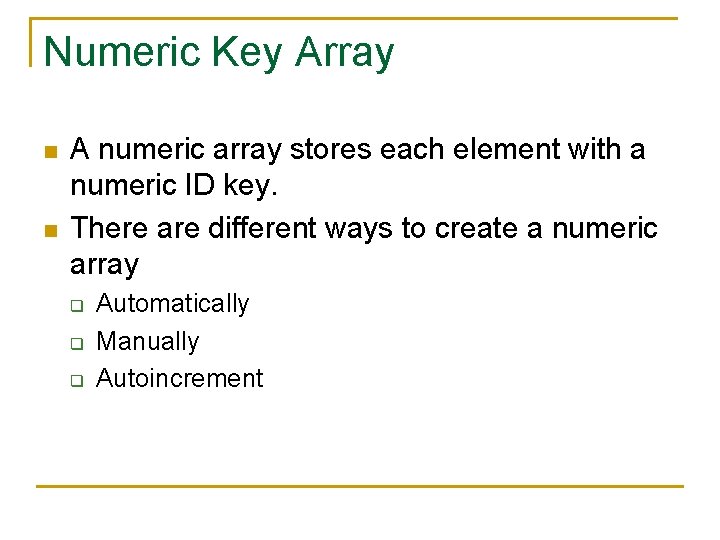
Numeric Key Array n n A numeric array stores each element with a numeric ID key. There are different ways to create a numeric array q q q Automatically Manually Autoincrement
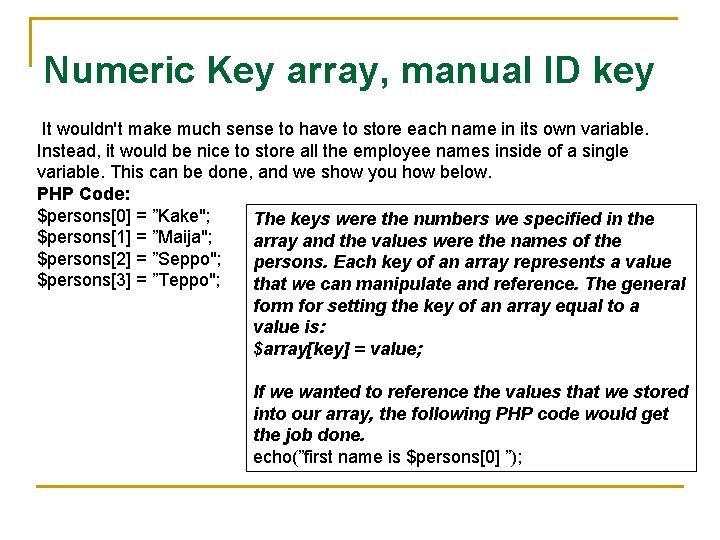
Numeric Key array, manual ID key It wouldn't make much sense to have to store each name in its own variable. Instead, it would be nice to store all the employee names inside of a single variable. This can be done, and we show you how below. PHP Code: $persons[0] = ”Kake"; The keys were the numbers we specified in the $persons[1] = ”Maija"; array and the values were the names of the $persons[2] = ”Seppo"; persons. Each key of an array represents a value $persons[3] = ”Teppo"; that we can manipulate and reference. The general form for setting the key of an array equal to a value is: $array[key] = value; If we wanted to reference the values that we stored into our array, the following PHP code would get the job done. echo(”first name is $persons[0] ”);
![Variations numeric 1 Initializing Arrays key Index auto generation person Kalle Aaltonen Autoincrement person Variations, numeric //1. Initializing Arrays key //Index auto generation $person[]= "Kalle Aaltonen"; //Autoincrement $person[]=](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-5.jpg)
Variations, numeric //1. Initializing Arrays key //Index auto generation $person[]= "Kalle Aaltonen"; //Autoincrement $person[]= "Maija Lehtinen"; $person[]= "Chege Muge"; $person[]= "Zippo Brown"; //Indexed $weight[0]=89. 5; //Manual $weight[1]=78. 4; $weight[2]=67. 3; $weight[3]=56. 2; //List $height= array( 1. 80, 1. 90, 1. 70, 1. 67 , 1. 90); //Automatically See the lecture examples //3. Output processing for($i=0; $i<count($person); $i++){ echo("<tr>"); echo("<td>$person[$i]</td><td>$weight[$i]</td><td>$height[$i]</td>"); echo("</tr>"); }
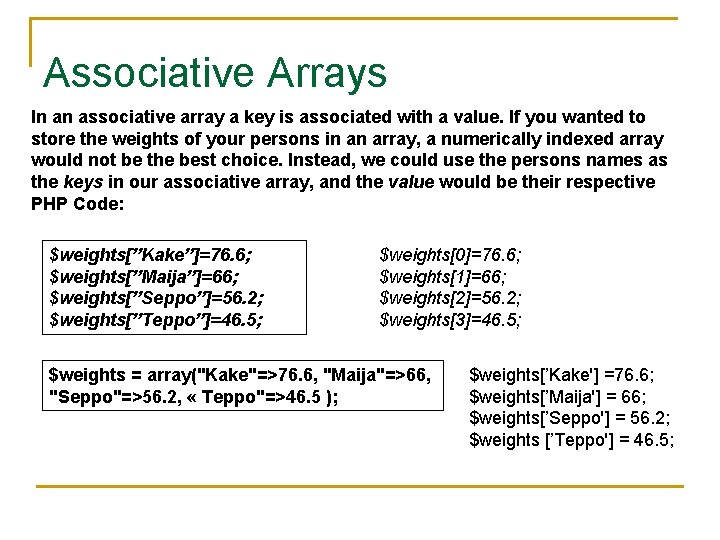
Associative Arrays In an associative array a key is associated with a value. If you wanted to store the weights of your persons in an array, a numerically indexed array would not be the best choice. Instead, we could use the persons names as the keys in our associative array, and the value would be their respective PHP Code: $weights[”Kake”]=76. 6; $weights[”Maija”]=66; $weights[”Seppo”]=56. 2; $weights[”Teppo”]=46. 5; $weights[0]=76. 6; $weights[1]=66; $weights[2]=56. 2; $weights[3]=46. 5; $weights = array("Kake"=>76. 6, "Maija"=>66, "Seppo"=>56. 2, « Teppo"=>46. 5 ); $weights[’Kake'] =76. 6; $weights[’Maija'] = 66; $weights[’Seppo'] = 56. 2; $weights [’Teppo'] = 46. 5;
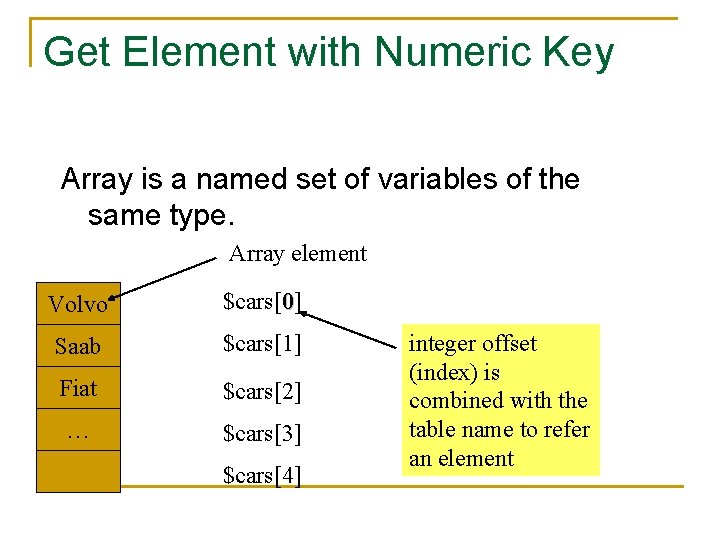
Get Element with Numeric Key Array is a named set of variables of the same type. Array element Volvo $cars[0] Saab $cars[1] Fiat $cars[2] … $cars[3] $cars[4] integer offset (index) is combined with the table name to refer an element
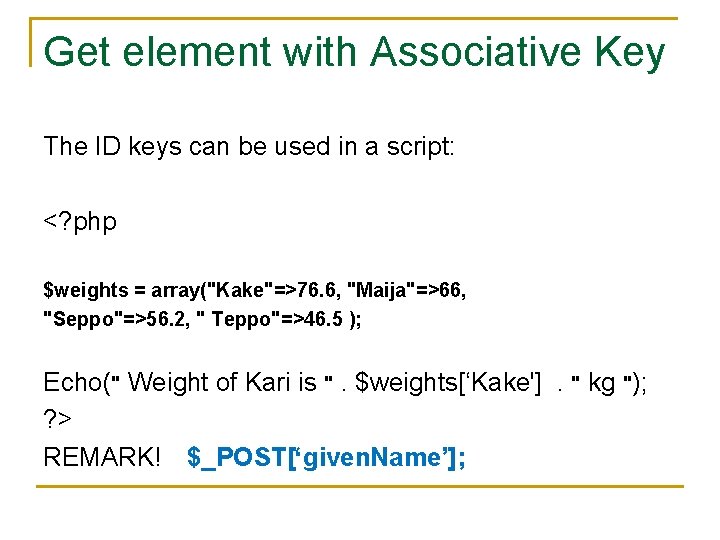
Get element with Associative Key The ID keys can be used in a script: <? php $weights = array("Kake"=>76. 6, "Maija"=>66, "Seppo"=>56. 2, " Teppo"=>46. 5 ); Echo(" Weight of Kari is ". $weights[‘Kake']. " kg "); ? > REMARK! $_POST[‘given. Name’];
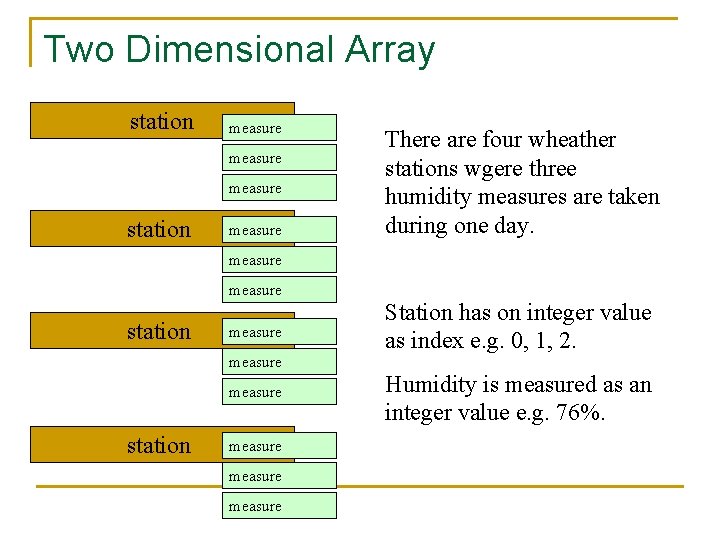
Two Dimensional Array station measure station measure There are four wheather stations wgere three humidity measures are taken during one day. measure station measure Station has on integer value as index e. g. 0, 1, 2. Humidity is measured as an integer value e. g. 76%.
![station index 0 humidity humidity00 65 humidity01 75 humidity02 85 station index = 0 humidity[][] humidity[0][0] = 65 humidity[0][1] = 75 humidity[0][2] = 85](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-10.jpg)
station index = 0 humidity[][] humidity[0][0] = 65 humidity[0][1] = 75 humidity[0][2] = 85 station index = 1 humidity[1][0] = 66 humidity[1][1] = 76 humidity[1][2] = 86 station index = 2 humidity[2][0] = 67 humidity[2][1] = 77 humidity[2][2] = 87 station index = 3 humidity[3][0] = 68 …. int [][] humidity = new int [4][3]; [0] [1] [2] [3] 65 66 67 68 75 76 77 78 85 86 87 88 humidity[2][1]
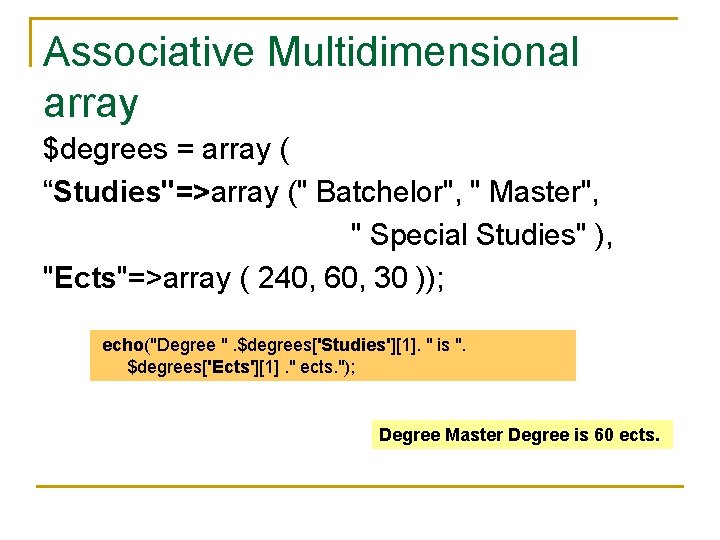
Associative Multidimensional array $degrees = array ( “Studies"=>array (" Batchelor", " Master", " Special Studies" ), "Ects"=>array ( 240, 60, 30 )); echo("Degree ". $degrees['Studies'][1]. " is ". $degrees['Ects'][1]. " ects. "); Degree Master Degree is 60 ects.
![Separate Arrays firstname lastname points 0 1 2 3 4 Use index to combine Separate Arrays firstname[] lastname[] points[] [0] [1] [2] [3] [4] Use index to combine](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-12.jpg)
Separate Arrays firstname[] lastname[] points[] [0] [1] [2] [3] [4] Use index to combine information from separate arrays!
![Multiple Arrays person weight height bmi 0 1 2 3 Multiple Arrays person[] weight[] height[] bmi[] [0] [1] [2] [3]](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-13.jpg)
Multiple Arrays person[] weight[] height[] bmi[] [0] [1] [2] [3]
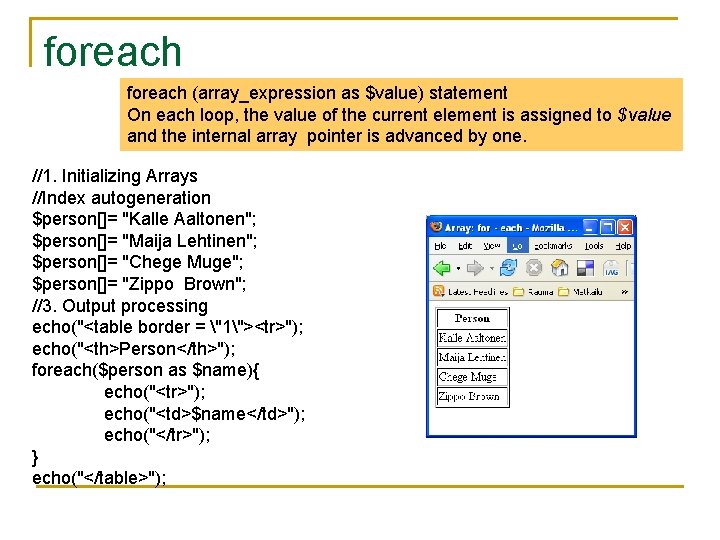
foreach (array_expression as $value) statement On each loop, the value of the current element is assigned to $value and the internal array pointer is advanced by one. //1. Initializing Arrays //Index autogeneration $person[]= "Kalle Aaltonen"; $person[]= "Maija Lehtinen"; $person[]= "Chege Muge"; $person[]= "Zippo Brown"; //3. Output processing echo("<table border = "1"><tr>"); echo("<th>Person</th>"); foreach($person as $name){ echo("<tr>"); echo("<td>$name</td>"); echo("</tr>"); } echo("</table>");
![count 2 Calculation fori0 icountperson i bmiiweightiheighti Loop counter i is used as count() //2. Calculation for($i=0; $i<count($person); $i++){ $bmi[$i]=$weight[$i]*$height[$i]; } Loop counter $i is used as](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-15.jpg)
count() //2. Calculation for($i=0; $i<count($person); $i++){ $bmi[$i]=$weight[$i]*$height[$i]; } Loop counter $i is used as index to array element. . //3. Output processing for($i=0; $i<count($person); $i++){ echo("<tr>"); echo("<td>$person[$i]</td><td>$weight[$i]</td><td>$height[$i]</td><td>$bmi[$i]</td>"); echo("</tr>"); } Method count () returns the number of elements.
![Multidimensional assosiative 1 Initializing Multidimensional name weight height team0 arrayname Kalle Aaltonen weight89 Multidimensional, assosiative //1. Initializing Multidimensional //name, weight, height $team[0]= array('name' => 'Kalle Aaltonen', 'weight'=>89.](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-16.jpg)
Multidimensional, assosiative //1. Initializing Multidimensional //name, weight, height $team[0]= array('name' => 'Kalle Aaltonen', 'weight'=>89. 5, 'height'=>1. 80); $team[1]= array('name' => 'Chege Muge', 'weight'=>78. 4, 'height'=>1. 90); $team[2]= array('name' => 'Maija Lehtinen', 'weight'=>67. 3, 'height'=>1. 70); $team[3]= array('name' => 'Zippo brown', 'weight'=>56. 2, 'height'=>1. 67); $team[4]= array('name' => 'Tedd Todd', 'weight'=>45. 1, 'height'=>1. 64); $team[5]= array('name' => 'Tina Turner', 'weight'=>34. 0, 'height'=>1. 51); //2. Calculation, adding one more row = bmi for($i=0; $i<count($team); $i++){ $team[$i]['bmi']=$team[$i]['weight'] / ($team[$i]['height'] *$team[$i]['height']) ; }
![Multidimensional for Loop all rows person fori0 icountteam i echotr echotd teaminame tdtd teamiweight Multidimensional, for //Loop all rows (=person) for($i=0; $i<count($team); $i++){ echo("<tr>"); echo("<td>". $team[$i]["name"]. "</td><td>". $team[$i]["weight"].](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-17.jpg)
Multidimensional, for //Loop all rows (=person) for($i=0; $i<count($team); $i++){ echo("<tr>"); echo("<td>". $team[$i]["name"]. "</td><td>". $team[$i]["weight"]. "</td><td>". $team[$i]["height"]. "</td><td>". $team[$i]["bmi"]. "</td>"); echo("</tr>"); }
![Multidimensional foreach Loop all rows person foreach team as person echotr echotd personname tdtd Multidimensional, foreach /Loop all rows (=person) foreach( $team as $person){ echo("<tr>"); echo("<td>". $person["name"]. "</td><td>".](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-18.jpg)
Multidimensional, foreach /Loop all rows (=person) foreach( $team as $person){ echo("<tr>"); echo("<td>". $person["name"]. "</td><td>". $person["weight"]. "</td> <td>". $person["height"]. "</td><td>". $person["bmi"]. "</td>"); echo("</tr>"); }
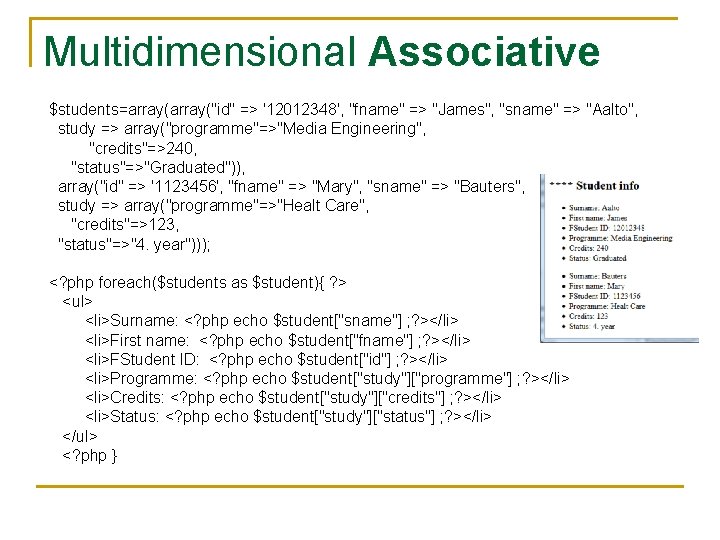
Multidimensional Associative $students=array("id" => '12012348', "fname" => "James", "sname" => "Aalto", study => array("programme"=>"Media Engineering", "credits"=>240, "status"=>"Graduated")), array("id" => '1123456', "fname" => "Mary", "sname" => "Bauters", study => array("programme"=>"Healt Care", "credits"=>123, "status"=>"4. year"))); <? php foreach($students as $student){ ? > <ul> <li>Surname: <? php echo $student["sname"] ; ? ></li> <li>First name: <? php echo $student["fname"] ; ? ></li> <li>FStudent ID: <? php echo $student["id"] ; ? ></li> <li>Programme: <? php echo $student["study"]["programme"] ; ? ></li> <li>Credits: <? php echo $student["study"]["credits"] ; ? ></li> <li>Status: <? php echo $student["study"]["status"] ; ? ></li> </ul> <? php }
![Multidimensional associative new element on the fly 1 Initializing Multidimensional name weight height team0 Multidimensional, associative, new element on the fly //1. Initializing Multidimensional //name, weight, height $team[0]=](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-20.jpg)
Multidimensional, associative, new element on the fly //1. Initializing Multidimensional //name, weight, height $team[0]= array('name' => 'Kalle Aaltonen', 'weight'=>89. 5, 'height'=>1. 80); $team[1]= array('name' => 'Chege Muge', 'weight'=>78. 4, 'height'=>1. 90); $team[2]= array('name' => 'Maija Lehtinen', 'weight'=>67. 3, 'height'=>1. 70); $team[3]= array('name' => 'Zippo brown', 'weight'=>56. 2, 'height'=>1. 67); $team[4]= array('name' => 'Tedd Todd', 'weight'=>45. 1, 'height'=>1. 64); $team[5]= array('name' => 'Tina Turner', 'weight'=>34. 0, 'height'=>1. 51); echo(" <h 2>**** BMI on the fly</h 2>"); for($i=0; $i<count($team); $i++){ $team[$i]['bmi']=$team[$i]['weight'] / ($team[$i]['height'] *$team[$i]['height']) ; } var_dump($team);
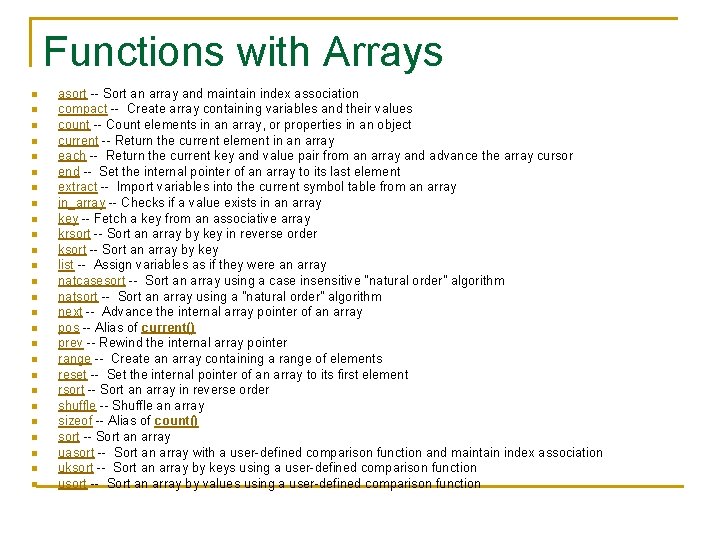
Functions with Arrays n n n n n n n asort -- Sort an array and maintain index association compact -- Create array containing variables and their values count -- Count elements in an array, or properties in an object current -- Return the current element in an array each -- Return the current key and value pair from an array and advance the array cursor end -- Set the internal pointer of an array to its last element extract -- Import variables into the current symbol table from an array in_array -- Checks if a value exists in an array key -- Fetch a key from an associative array krsort -- Sort an array by key in reverse order ksort -- Sort an array by key list -- Assign variables as if they were an array natcasesort -- Sort an array using a case insensitive "natural order" algorithm natsort -- Sort an array using a "natural order" algorithm next -- Advance the internal array pointer of an array pos -- Alias of current() prev -- Rewind the internal array pointer range -- Create an array containing a range of elements reset -- Set the internal pointer of an array to its first element rsort -- Sort an array in reverse order shuffle -- Shuffle an array sizeof -- Alias of count() sort -- Sort an array uasort -- Sort an array with a user-defined comparison function and maintain index association uksort -- Sort an array by keys using a user-defined comparison function usort -- Sort an array by values using a user-defined comparison function
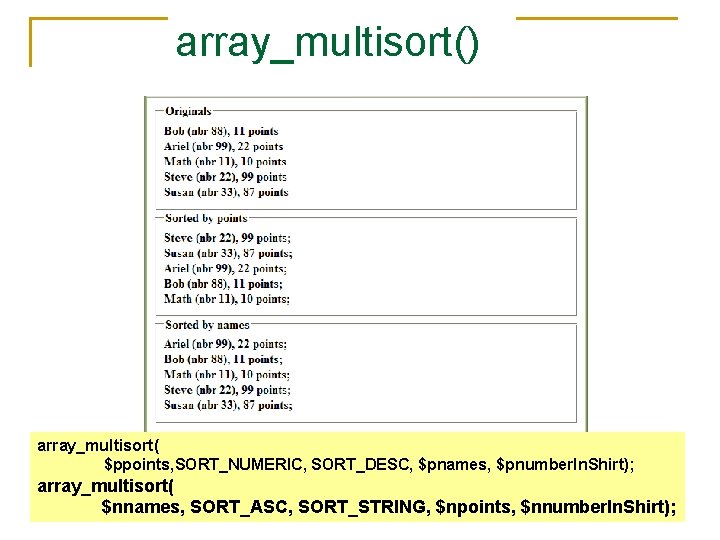
array_multisort() array_multisort( $ppoints, SORT_NUMERIC, SORT_DESC, $pnames, $pnumber. In. Shirt); array_multisort( $nnames, SORT_ASC, SORT_STRING, $npoints, $nnumber. In. Shirt);
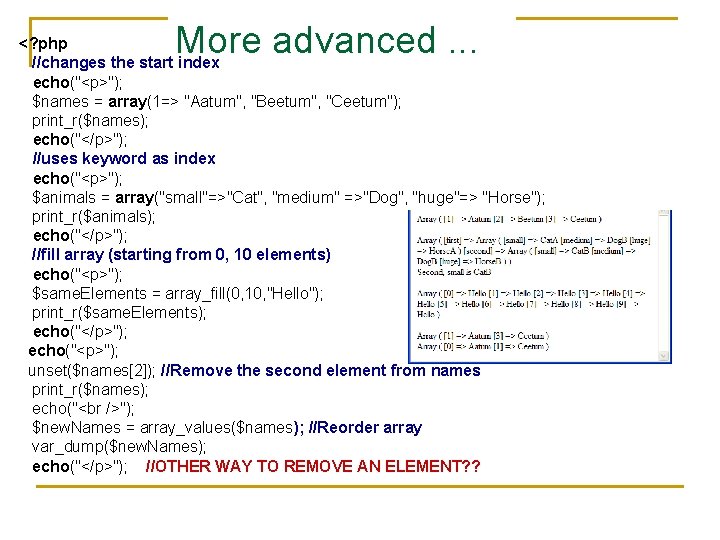
More advanced. . . <? php //changes the start index echo("<p>"); $names = array(1=> "Aatum", "Beetum", "Ceetum"); print_r($names); echo("</p>"); //uses keyword as index echo("<p>"); $animals = array("small"=>"Cat", "medium" =>"Dog", "huge"=> "Horse"); print_r($animals); echo("</p>"); //fill array (starting from 0, 10 elements) echo("<p>"); $same. Elements = array_fill(0, 10, "Hello"); print_r($same. Elements); echo("</p>"); echo("<p>"); unset($names[2]); //Remove the second element from names print_r($names); echo(" "); $new. Names = array_values($names); //Reorder array var_dump($new. Names); echo("</p>"); //OTHER WAY TO REMOVE AN ELEMENT? ?
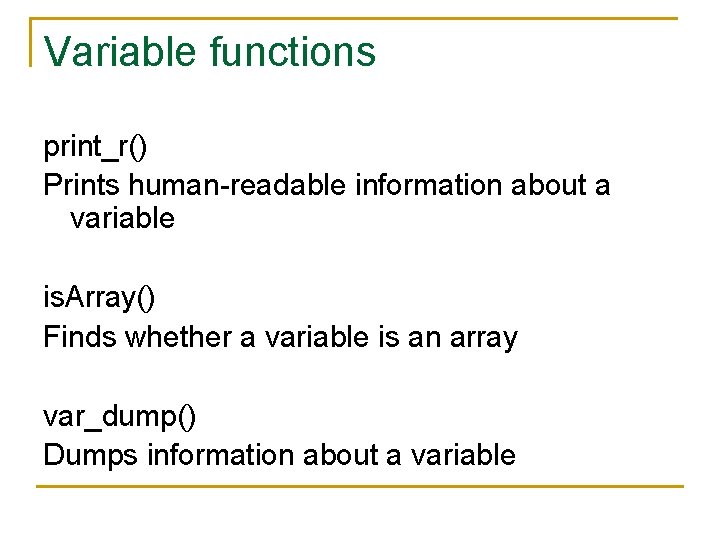
Variable functions print_r() Prints human-readable information about a variable is. Array() Finds whether a variable is an array var_dump() Dumps information about a variable
![Forms and Arrays fori0 iPERSONS i echotr tdinput typetext namegiven Names td tdinput typetext Forms and Arrays for($i=0; $i<PERSONS; $i++){ echo("<tr>". "<td><input type="text" name=“given. Names[]“ /></td>". "<td><input type="text"](https://slidetodoc.com/presentation_image_h2/7f0372ca8a1dad15e79685c4ba74757c/image-25.jpg)
Forms and Arrays for($i=0; $i<PERSONS; $i++){ echo("<tr>". "<td><input type="text" name=“given. Names[]“ /></td>". "<td><input type="text" name=“give. Weights[]“/></td>". "<td><input type="text" name=“given. Heights[]“/></td>". "</tr>"); $names = $_POST[”given. Names”]; } $heights = $_POST[”given. Heights”]; $weights = $_POST[”given. Weights”]; echo("<table border="box""); echo("<tr><th>Person</th><th>Height (m)</th><th>Weight (kg)</th><th>BM Index</th>". "<th>Description</th></tr>"); for($i=0; $i<count($names); $i++){ echo("<tr><td>$names[$i]</td><td>$heights[$i]</td>". "<td>$weights[$i]</td><td>$formatted. Index[$i]</td>". "<td>$bmi. Text[$i]</td></tr>"); } echo("</table>");