Arrays Array Array Our Very First Data Structure
![Arrays [Array, Array] Arrays [Array, Array]](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-1.jpg)
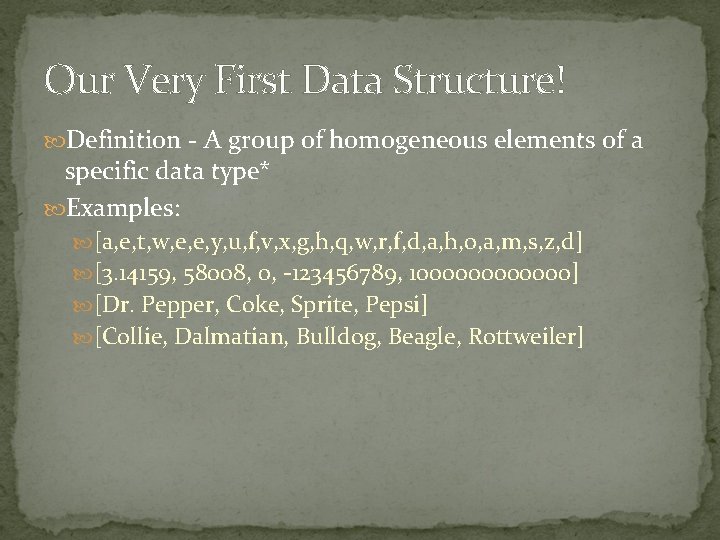
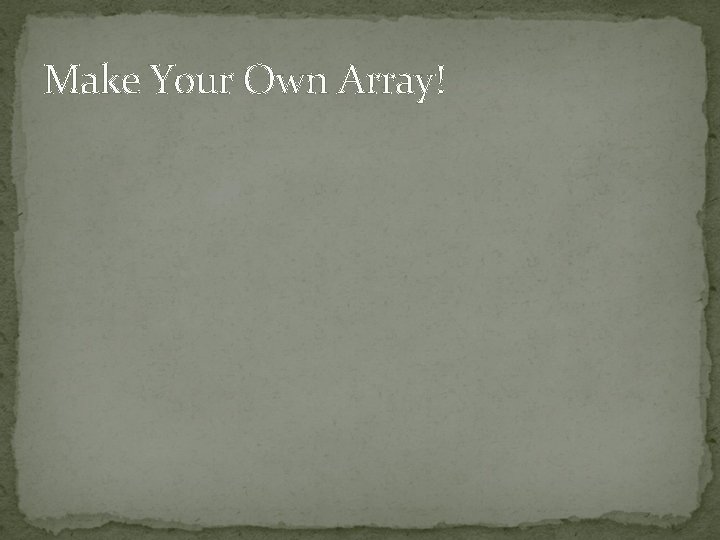
![Declaration An array is declared as follows: variable_type[] variable_name; Examples: int[] costumer. IDs; double[] Declaration An array is declared as follows: variable_type[] variable_name; Examples: int[] costumer. IDs; double[]](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-4.jpg)
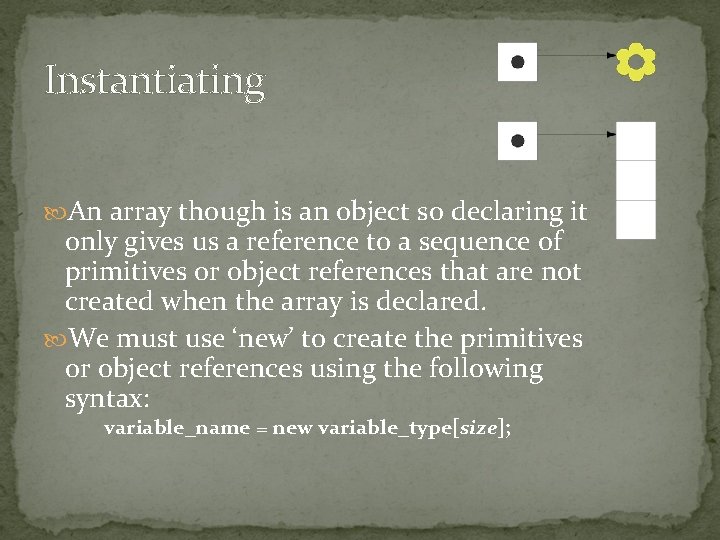
![Array Size variable_name = new variable_type[size]; In this declaration, size is the number of Array Size variable_name = new variable_type[size]; In this declaration, size is the number of](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-6.jpg)
![Instantiating Examples int[] costumer. IDs; costumer. IDs = new int[1000]; double[] change. Jars; change. Instantiating Examples int[] costumer. IDs; costumer. IDs = new int[1000]; double[] change. Jars; change.](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-7.jpg)
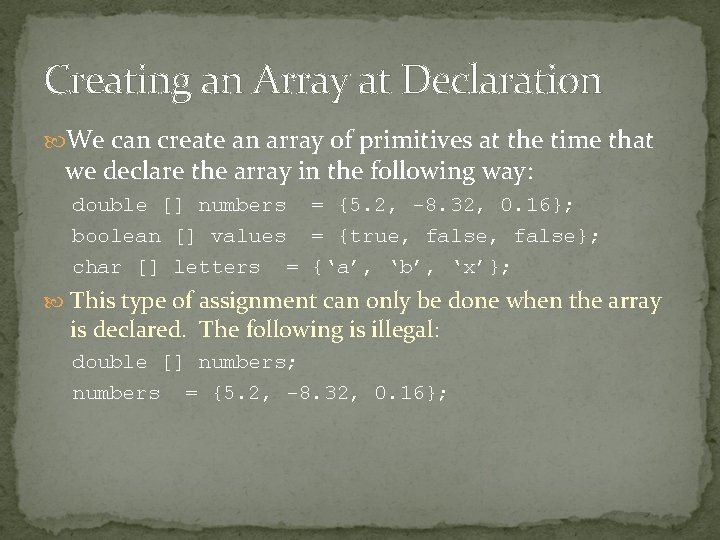
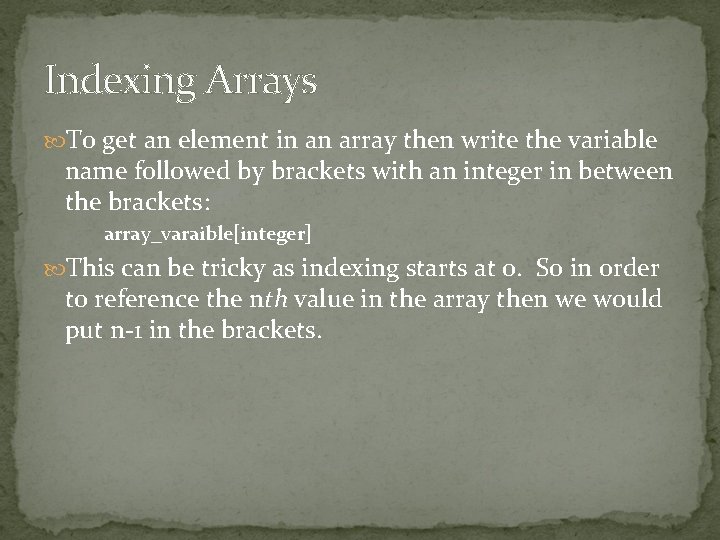
![Indexing Examples We have the following array: double [] numbers = {5. 2, -8. Indexing Examples We have the following array: double [] numbers = {5. 2, -8.](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-10.jpg)
![Indexing Activity rainbow = [red, orange, yellow, green, blue] rainbow[ 0 ] == ? Indexing Activity rainbow = [red, orange, yellow, green, blue] rainbow[ 0 ] == ?](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-11.jpg)
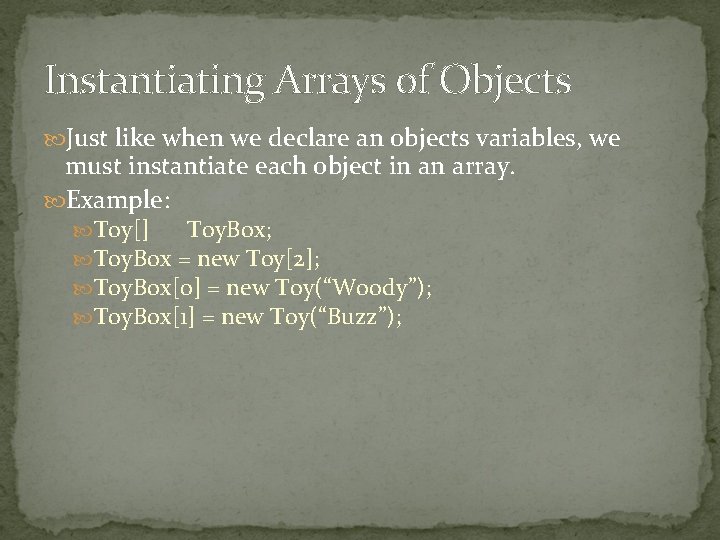
![Daisy Array Example Step 1 – Declare the array Daisy[] Daisy. Set; Step 2 Daisy Array Example Step 1 – Declare the array Daisy[] Daisy. Set; Step 2](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-13.jpg)
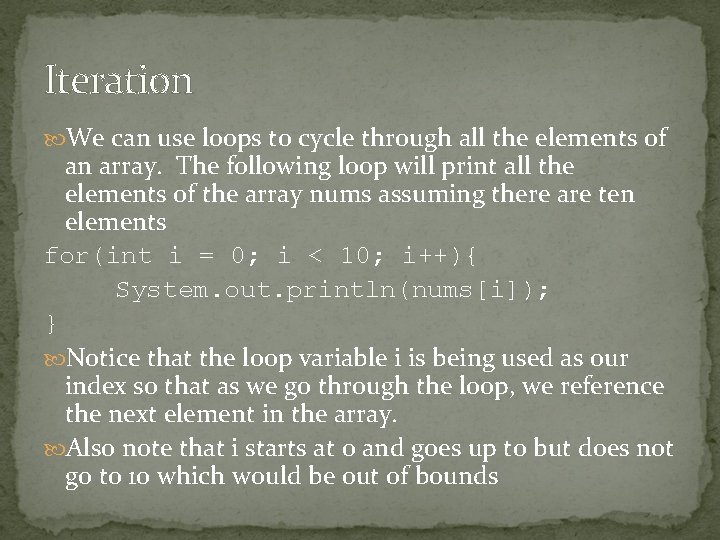
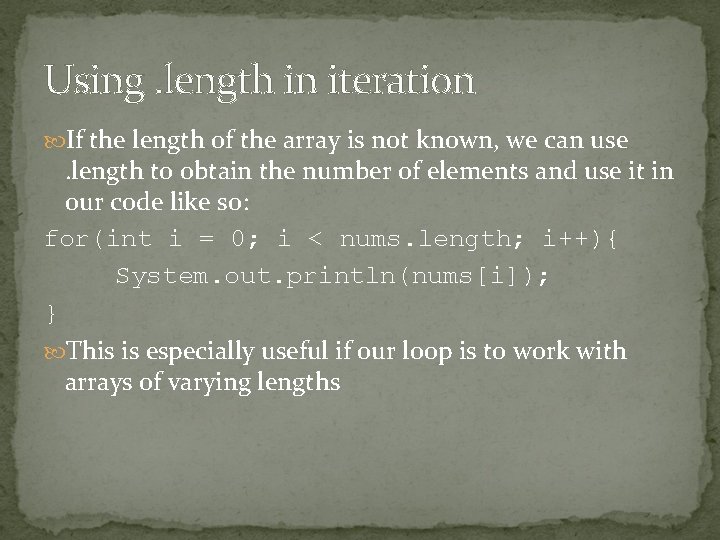
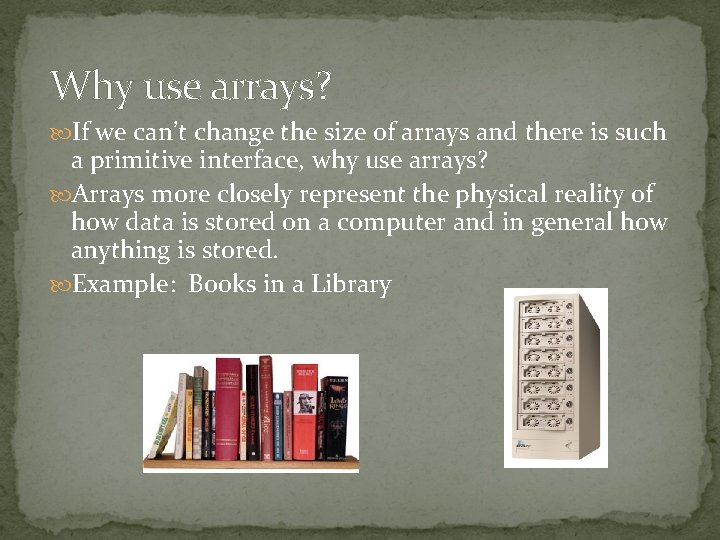
- Slides: 16
![Arrays Array Array Arrays [Array, Array]](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-1.jpg)
Arrays [Array, Array]
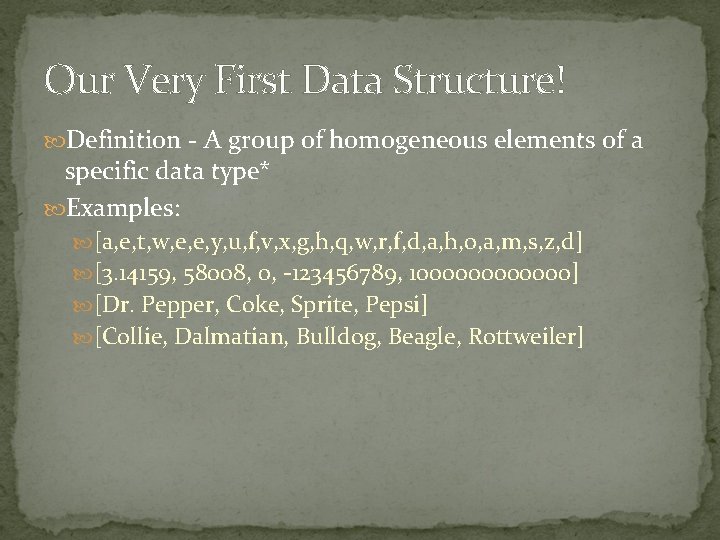
Our Very First Data Structure! Definition - A group of homogeneous elements of a specific data type* Examples: [a, e, t, w, e, e, y, u, f, v, x, g, h, q, w, r, f, d, a, h, o, a, m, s, z, d] [3. 14159, 58008, 0, -123456789, 1000000] [Dr. Pepper, Coke, Sprite, Pepsi] [Collie, Dalmatian, Bulldog, Beagle, Rottweiler]
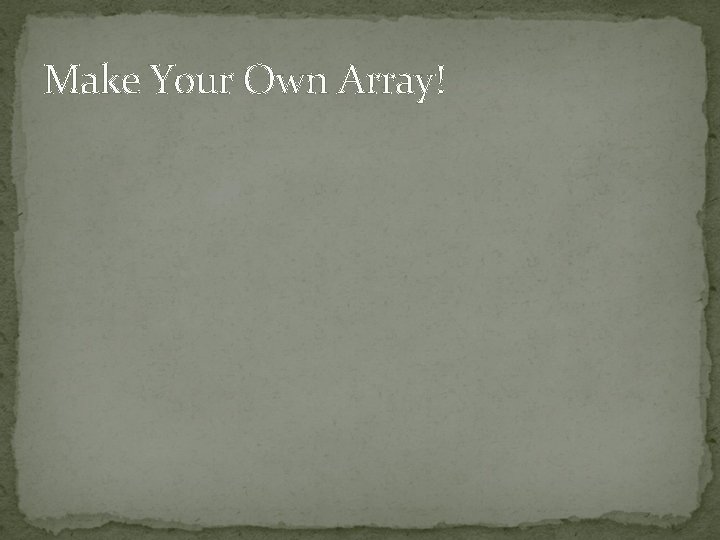
Make Your Own Array!
![Declaration An array is declared as follows variabletype variablename Examples int costumer IDs double Declaration An array is declared as follows: variable_type[] variable_name; Examples: int[] costumer. IDs; double[]](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-4.jpg)
Declaration An array is declared as follows: variable_type[] variable_name; Examples: int[] costumer. IDs; double[] change. Jars; String[] NFLteam. Names;
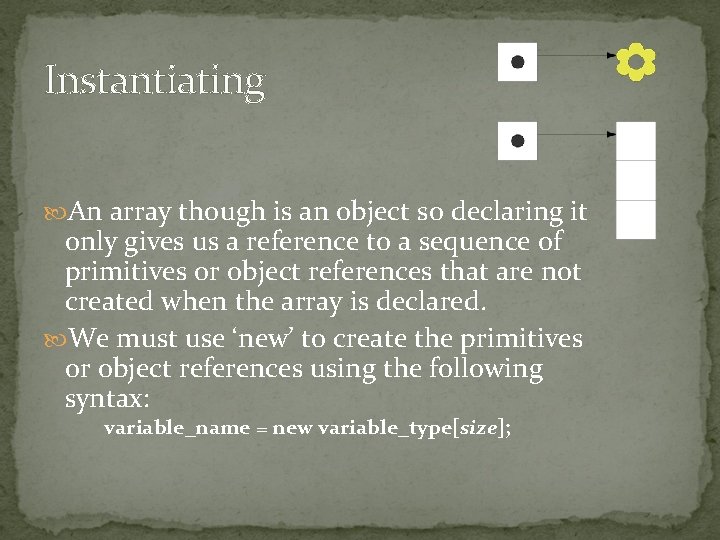
Instantiating An array though is an object so declaring it only gives us a reference to a sequence of primitives or object references that are not created when the array is declared. We must use ‘new’ to create the primitives or object references using the following syntax: variable_name = new variable_type[size];
![Array Size variablename new variabletypesize In this declaration size is the number of Array Size variable_name = new variable_type[size]; In this declaration, size is the number of](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-6.jpg)
Array Size variable_name = new variable_type[size]; In this declaration, size is the number of objects or primitives being created. size and must be an integer. Size of an array is set when the array is created(instantiated). Once set, the size of an array can not be changed! There are other data structures though that will let us change the size
![Instantiating Examples int costumer IDs costumer IDs new int1000 double change Jars change Instantiating Examples int[] costumer. IDs; costumer. IDs = new int[1000]; double[] change. Jars; change.](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-7.jpg)
Instantiating Examples int[] costumer. IDs; costumer. IDs = new int[1000]; double[] change. Jars; change. Jars = new double[6]; String[] NFLteam. Names; NFLteam. Names = new String[32];
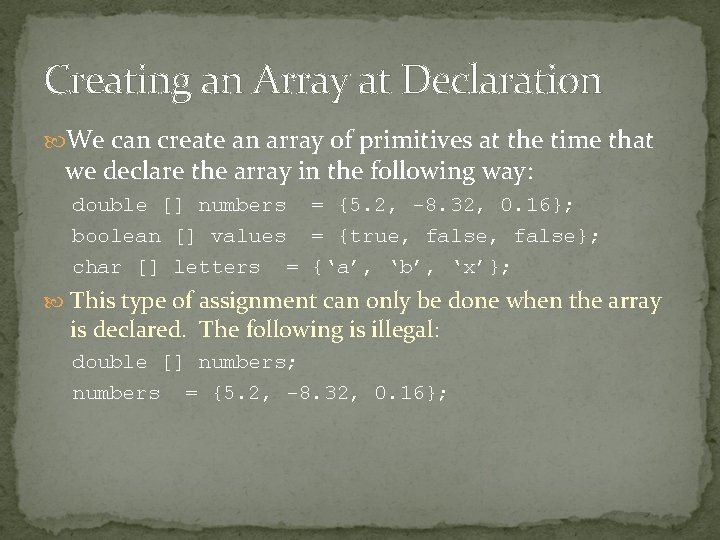
Creating an Array at Declaration We can create an array of primitives at the time that we declare the array in the following way: double [] numbers = {5. 2, -8. 32, 0. 16}; boolean [] values = {true, false}; char [] letters = {‘a’, ‘b’, ‘x’}; This type of assignment can only be done when the array is declared. The following is illegal: double [] numbers; numbers = {5. 2, -8. 32, 0. 16};
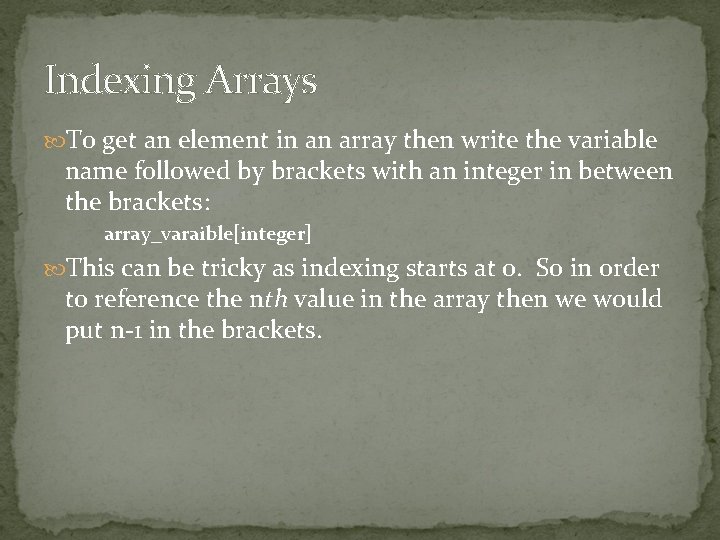
Indexing Arrays To get an element in an array then write the variable name followed by brackets with an integer in between the brackets: array_varaible[integer] This can be tricky as indexing starts at 0. So in order to reference the nth value in the array then we would put n-1 in the brackets.
![Indexing Examples We have the following array double numbers 5 2 8 Indexing Examples We have the following array: double [] numbers = {5. 2, -8.](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-10.jpg)
Indexing Examples We have the following array: double [] numbers = {5. 2, -8. 32, 0. 16}; Then numbers[0] == 5. 2 numbers[1] == -8. 32 numbers[2] == 0. 16 Any integer greater than 2 or less than 0 is out of bounds for this array.
![Indexing Activity rainbow red orange yellow green blue rainbow 0 Indexing Activity rainbow = [red, orange, yellow, green, blue] rainbow[ 0 ] == ?](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-11.jpg)
Indexing Activity rainbow = [red, orange, yellow, green, blue] rainbow[ 0 ] == ? red rainbow[ 3 ] == ? green rainbow[ 4? ] == blue rainbow[ 3? ] == green rainbow[ 1? ] == orange
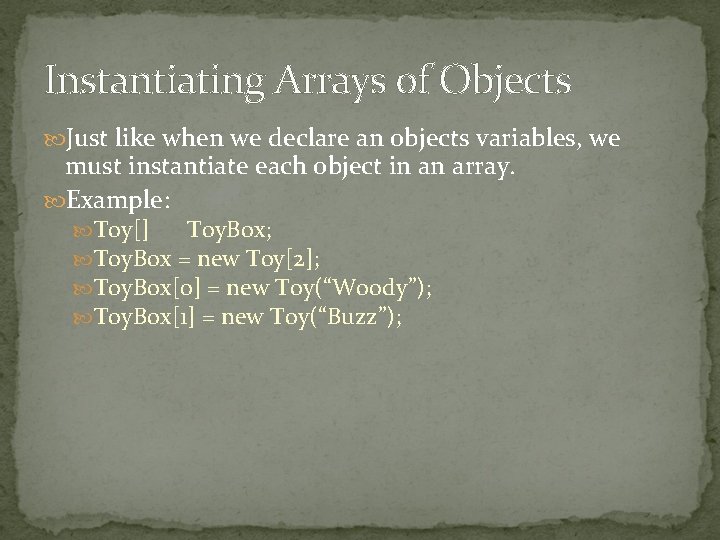
Instantiating Arrays of Objects Just like when we declare an objects variables, we must instantiate each object in an array. Example: Toy[] Toy. Box; Toy. Box = new Toy[2]; Toy. Box[0] = new Toy(“Woody”); Toy. Box[1] = new Toy(“Buzz”);
![Daisy Array Example Step 1 Declare the array Daisy Daisy Set Step 2 Daisy Array Example Step 1 – Declare the array Daisy[] Daisy. Set; Step 2](https://slidetodoc.com/presentation_image/bb58a1ec8db63d231fdfebe8844b2868/image-13.jpg)
Daisy Array Example Step 1 – Declare the array Daisy[] Daisy. Set; Step 2 – Instantiating Array Daisy. Set = new Daisy[3]; Step 3 – Instantiate Objects in the Array Daisy. Set[0] = new Daisy(); Daisy. Set[1] = new Daisy(); Daisy. Set[2] = new Daisy();
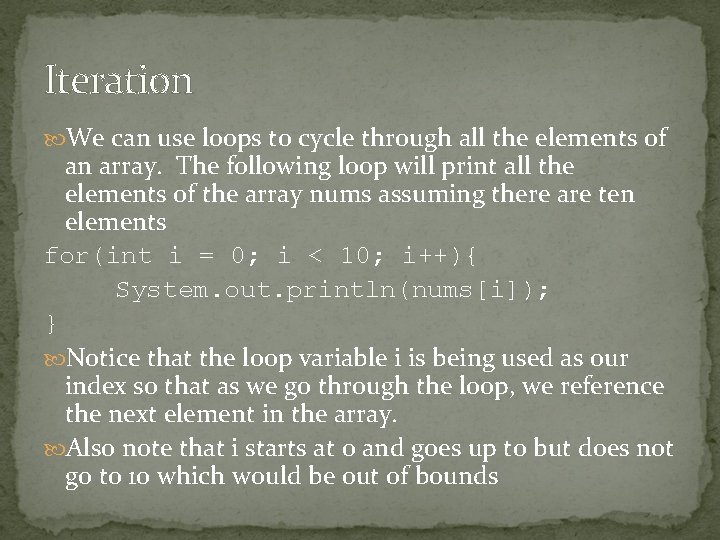
Iteration We can use loops to cycle through all the elements of an array. The following loop will print all the elements of the array nums assuming there are ten elements for(int i = 0; i < 10; i++){ System. out. println(nums[i]); } Notice that the loop variable i is being used as our index so that as we go through the loop, we reference the next element in the array. Also note that i starts at 0 and goes up to but does not go to 10 which would be out of bounds
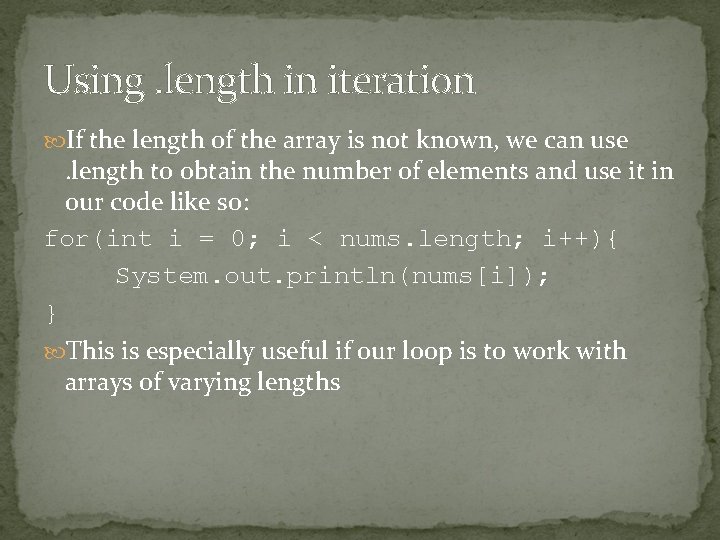
Using. length in iteration If the length of the array is not known, we can use . length to obtain the number of elements and use it in our code like so: for(int i = 0; i < nums. length; i++){ System. out. println(nums[i]); } This is especially useful if our loop is to work with arrays of varying lengths
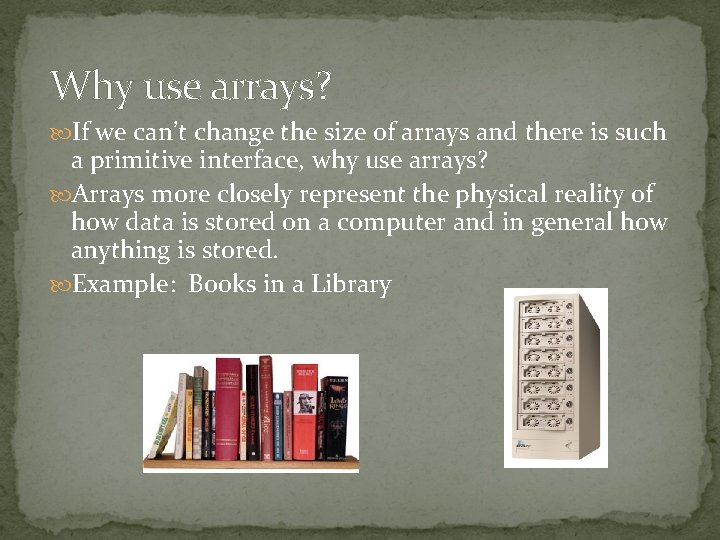
Why use arrays? If we can’t change the size of arrays and there is such a primitive interface, why use arrays? Arrays more closely represent the physical reality of how data is stored on a computer and in general how anything is stored. Example: Books in a Library