Arrays and Collections Intro to the Java Collections
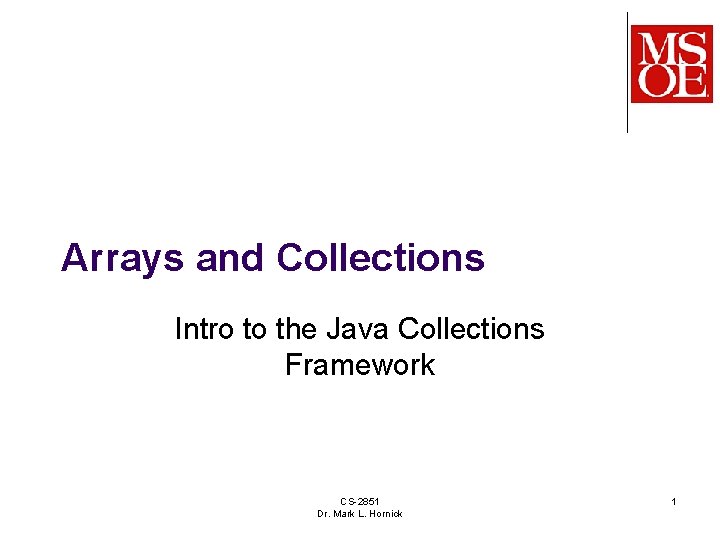
![Java Array Review l Declaration of an array: <datatype>[] <variable>; <datatype> <variable>[]; l // Java Array Review l Declaration of an array: <datatype>[] <variable>; <datatype> <variable>[]; l //](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-2.jpg)
![The distinction between declaration and creation int[] scores; // or int scores[] After Declaration The distinction between declaration and creation int[] scores; // or int scores[] After Declaration](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-3.jpg)
![The distinction between declaration and creation int[] scores; // or int scores[] scores = The distinction between declaration and creation int[] scores; // or int scores[] scores =](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-4.jpg)
![After assigning values to the array elements: int[] scores; // or int scores[] scores After assigning values to the array elements: int[] scores; // or int scores[] scores](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-5.jpg)
![Another approach: Simultaneous declaration creation, and initialization of an array int[] scores ={8, 7, Another approach: Simultaneous declaration creation, and initialization of an array int[] scores ={8, 7,](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-6.jpg)
![Declaring an array of objects Person[] people; people = new Person[3]; The people array Declaring an array of objects Person[] people; people = new Person[3]; The people array](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-7.jpg)
![Creating an array of objects Person[] people; people = new Person[3]; people[0] = new Creating an array of objects Person[] people; people = new Person[3]; people[0] = new](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-8.jpg)
![Another way of looking at it Code Person[ ] people; people = new Person[20]; Another way of looking at it Code Person[ ] people; people = new Person[20];](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-9.jpg)
![Simultaneous declaration creation, and initialization of an object array Person[] people ={new Person(A), new Simultaneous declaration creation, and initialization of an object array Person[] people ={new Person(A), new](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-10.jpg)
![Element Deletion int index= 1; A people[index] = null; people 0 A Delete Person Element Deletion int index= 1; A people[index] = null; people 0 A Delete Person](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-11.jpg)
![Element Insertion (? ) int index= 1; A people[index] = new Person(E); What happens? Element Insertion (? ) int index= 1; A people[index] = new Person(E); What happens?](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-12.jpg)
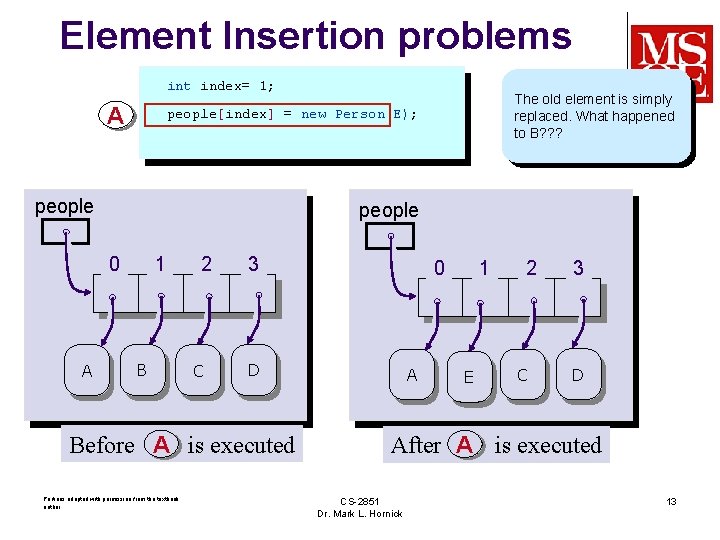
- Slides: 13
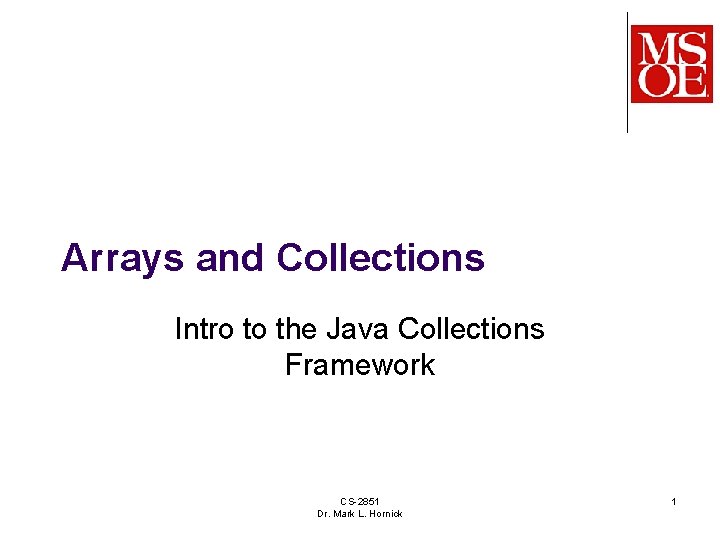
Arrays and Collections Intro to the Java Collections Framework CS-2851 Dr. Mark L. Hornick 1
![Java Array Review l Declaration of an array datatype variable datatype variable l Java Array Review l Declaration of an array: <datatype>[] <variable>; <datatype> <variable>[]; l //](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-2.jpg)
Java Array Review l Declaration of an array: <datatype>[] <variable>; <datatype> <variable>[]; l // approach 1 // approach 2 Creation of the actual array: <variable> = new <datatype>[<size>]; Variation 1 Variation 2 int[] scores; int scores[]; scores = new int[12]; Arrays are created like objects! Portions adapted with permission from the textbook author. CS-2851 Dr. Mark L. Hornick 2
![The distinction between declaration and creation int scores or int scores After Declaration The distinction between declaration and creation int[] scores; // or int scores[] After Declaration](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-3.jpg)
The distinction between declaration and creation int[] scores; // or int scores[] After Declaration of an array, a variable that references an array is created somewhere in memory But since there’s no actual array (yet), the variable has a value of null. Start of Memory … scores null … End of Memory
![The distinction between declaration and creation int scores or int scores scores The distinction between declaration and creation int[] scores; // or int scores[] scores =](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-4.jpg)
The distinction between declaration and creation int[] scores; // or int scores[] scores = new int[12]; After Creation of the array, the memory for the array elements is allocated and the variable is assigned to reference the first element of the array All elements of the array are initialized (in this case) to 0. Portions adapted with permission from the textbook author. CS-2851 Dr. Mark L. Hornick … scores addr 0 0 0 … 4
![After assigning values to the array elements int scores or int scores scores After assigning values to the array elements: int[] scores; // or int scores[] scores](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-5.jpg)
After assigning values to the array elements: int[] scores; // or int scores[] scores = new int[12]; for( int i=0; i<scores. length; i++ ) scores[i] = i; scores 0 1 2 3 4 5 6 7 8 9 10 11 5 The index of the first position in an array is 0. CS-2851 Dr. Mark L. Hornick
![Another approach Simultaneous declaration creation and initialization of an array int scores 8 7 Another approach: Simultaneous declaration creation, and initialization of an array int[] scores ={8, 7,](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-6.jpg)
Another approach: Simultaneous declaration creation, and initialization of an array int[] scores ={8, 7, 10, 4, 5, 6, 5, 8, 9, 10, 5, 8}; scores 0 1 2 3 4 5 6 7 8 9 10 11 8 7 10 4 5 6 5 8 9 10 5 8 scores[2] Portions adapted with permission from the textbook author. CS-2851 Dr. Mark L. Hornick This indexed expression refers to the element at position #2 6
![Declaring an array of objects Person people people new Person3 The people array Declaring an array of objects Person[] people; people = new Person[3]; The people array](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-7.jpg)
Declaring an array of objects Person[] people; people = new Person[3]; The people array is actually an array of references to Person objects After Creation of the array, the memory for the array elements is allocated and the variable is assigned to reference the first element of the array … people addr null All elements of the array are initialized (in this case) to null. … Portions adapted with permission from the textbook author. CS-2851 Dr. Mark L. Hornick 7
![Creating an array of objects Person people people new Person3 people0 new Creating an array of objects Person[] people; people = new Person[3]; people[0] = new](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-8.jpg)
Creating an array of objects Person[] people; people = new Person[3]; people[0] = new Person(A); When a Person object is created, the object is placed somewhere in memory. The new operator returns this address in memory. After initializing an array element to reference an instance of a Person object, the array element is assigned to reference to the Person object … people addr null Person … Portions adapted with permission from the textbook author. CS-2851 Dr. Mark L. Hornick 8
![Another way of looking at it Code Person people people new Person20 Another way of looking at it Code Person[ ] people; people = new Person[20];](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-9.jpg)
Another way of looking at it Code Person[ ] people; people = new Person[20]; C people[0] = new Person(A); One Person object is created and the reference to this object is placed in position 0. people person 0 State of Memory 1 2 3 4 16 17 18 19 Person A Portions adapted with permission from the textbook author. After C is executed CS-2851 Dr. Mark L. Hornick 9
![Simultaneous declaration creation and initialization of an object array Person people new PersonA new Simultaneous declaration creation, and initialization of an object array Person[] people ={new Person(A), new](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-10.jpg)
Simultaneous declaration creation, and initialization of an object array Person[] people ={new Person(A), new Person(B), new Person(C)}; people 0 1 Person A B Portions adapted with permission from the textbook author. 2 Person C CS-2851 Dr. Mark L. Hornick 10
![Element Deletion int index 1 A peopleindex null people 0 A Delete Person Element Deletion int index= 1; A people[index] = null; people 0 A Delete Person](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-11.jpg)
Element Deletion int index= 1; A people[index] = null; people 0 A Delete Person B by setting the reference in position 1 to null. What problem does this introduce? 1 B 2 C 3 D Before A is executed Portions adapted with permission from the textbook author. 0 A 1 2 C 3 D After A is executed CS-2851 Dr. Mark L. Hornick 11
![Element Insertion int index 1 A peopleindex new PersonE What happens Element Insertion (? ) int index= 1; A people[index] = new Person(E); What happens?](https://slidetodoc.com/presentation_image_h/08c4f3fa7ad67544a7c0f9af49a04b42/image-12.jpg)
Element Insertion (? ) int index= 1; A people[index] = new Person(E); What happens? ? people 0 A 1 B 2 C 3 D Before A is executed Portions adapted with permission from the textbook author. CS-2851 Dr. Mark L. Hornick 12
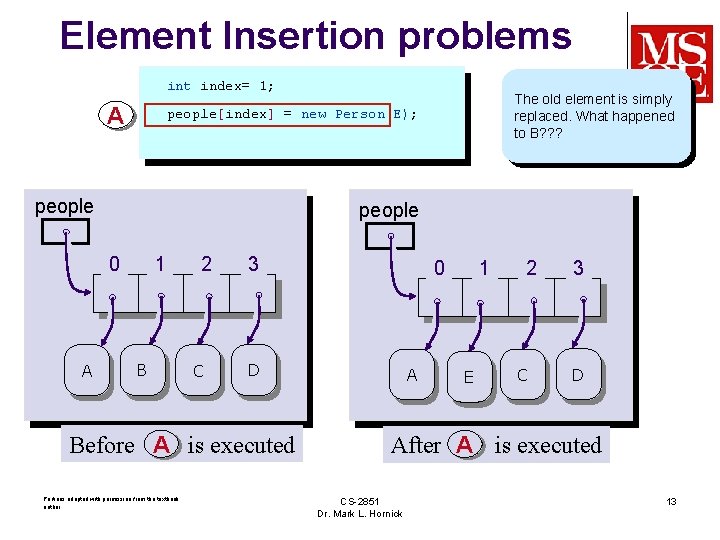
Element Insertion problems int index= 1; A people 0 A The old element is simply replaced. What happened to B? ? ? people[index] = new Person(E); 1 B 2 C 3 D Before A is executed Portions adapted with permission from the textbook author. 0 A 1 E 2 C 3 D After A is executed CS-2851 Dr. Mark L. Hornick 13