ARRAYS 01204111 Computer Programming Group 350 352 Dept
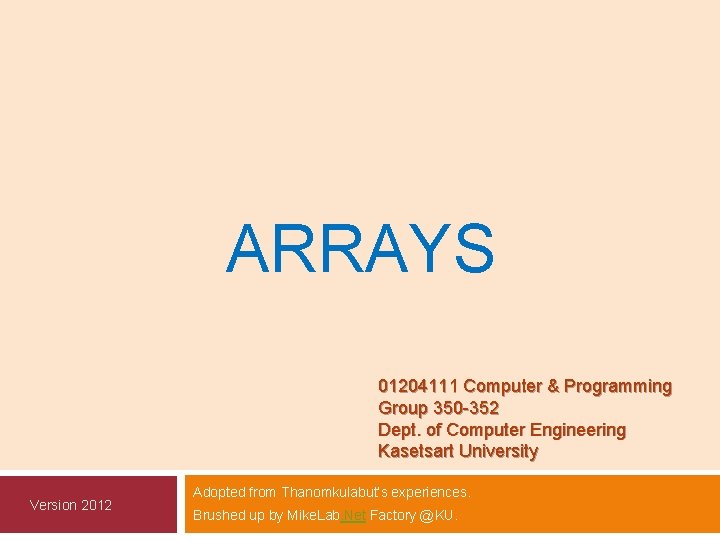
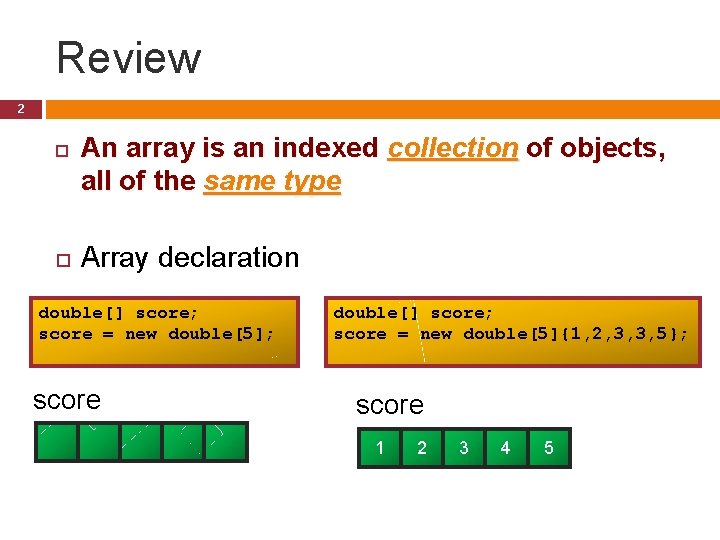
![Review (2) 3 Declaration only int Declaration & creation int [] ai; [] ai Review (2) 3 Declaration only int Declaration & creation int [] ai; [] ai](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-3.jpg)
![Review (3) 4 Access array elements int [] score = new int[4]; score 0 Review (3) 4 Access array elements int [] score = new int[4]; score 0](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-4.jpg)
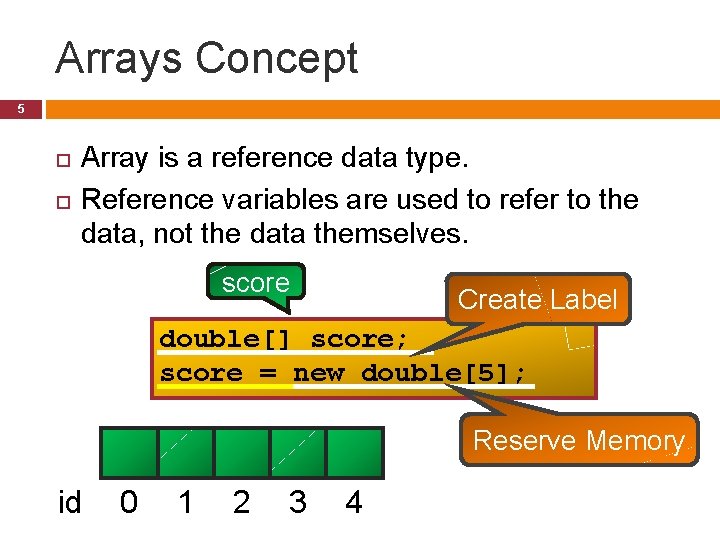
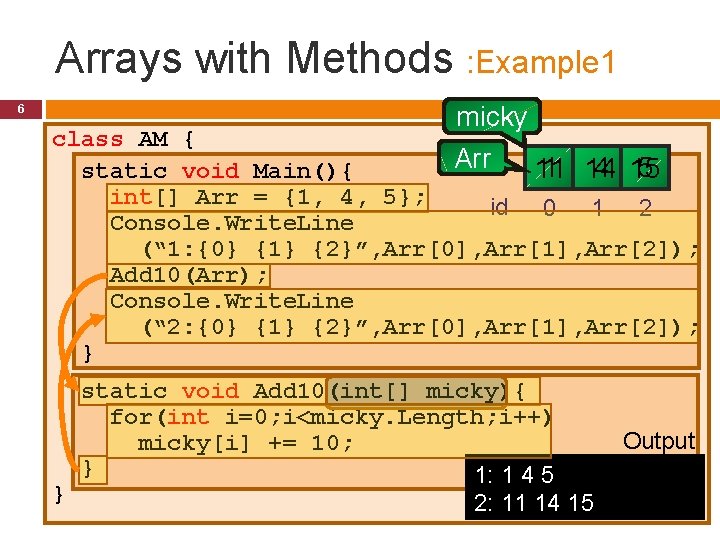
![Arrays with Methods : Example 2 7 static void Main(){ data info Nobi char[] Arrays with Methods : Example 2 7 static void Main(){ data info Nobi char[]](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-7.jpg)
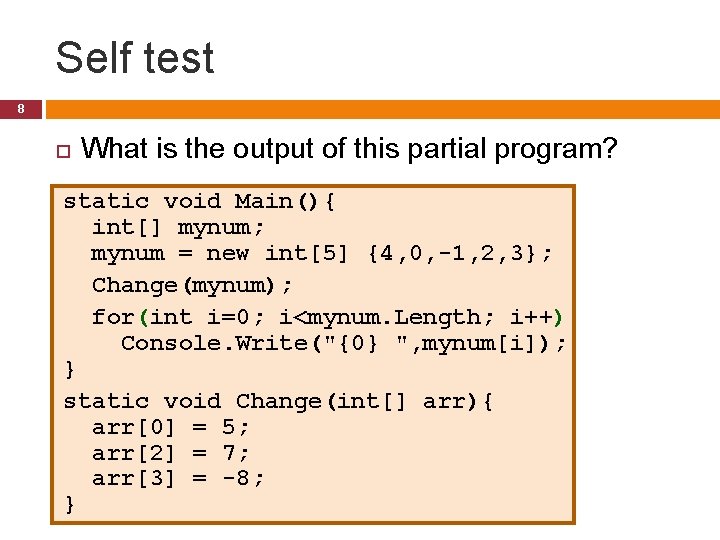
![Example 1 9 static void Main(){ char[] name 1, name 2; name 1 = Example 1 9 static void Main(){ char[] name 1, name 2; name 1 =](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-9.jpg)
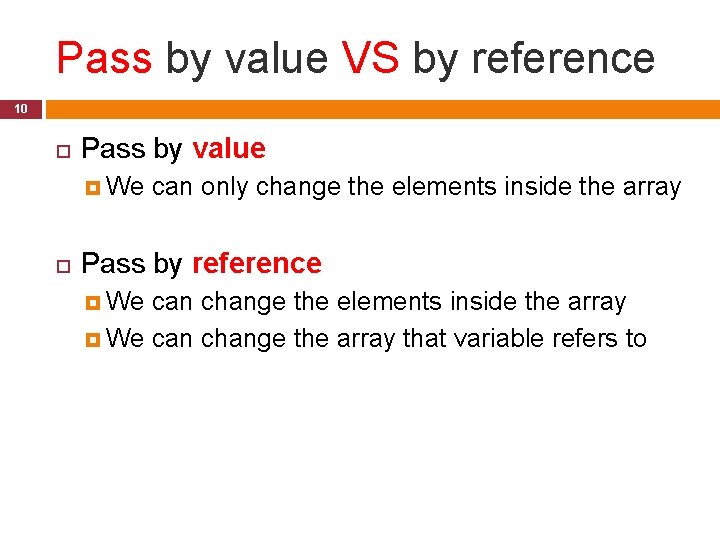
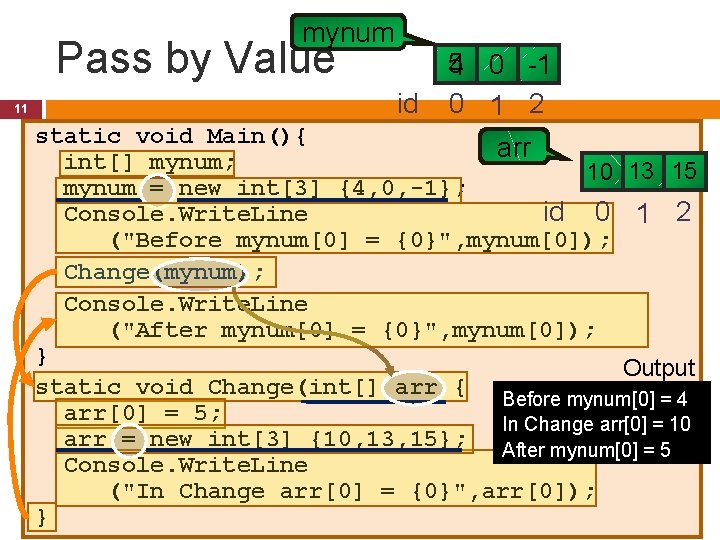
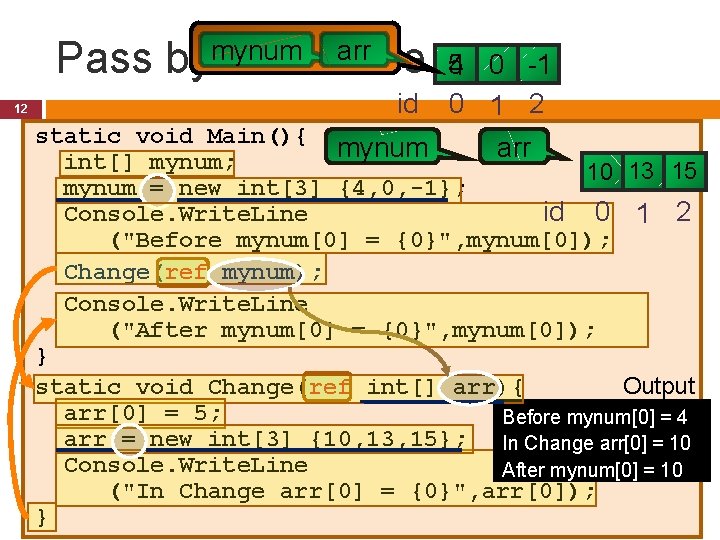
![13 Multi-dimensional Array (introduction) If you want to keep score of 50 students double[] 13 Multi-dimensional Array (introduction) If you want to keep score of 50 students double[]](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-13.jpg)
![14 Multi-dimensional Array Declaration 1 dimension <type> [] <name>; int [] score; Multi-dimensional 2 14 Multi-dimensional Array Declaration 1 dimension <type> [] <name>; int [] score; Multi-dimensional 2](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-14.jpg)
![Multi-dimensional Array Declaration 15 1 Dimension score <name> = new <type>[<dim size>]; score = Multi-dimensional Array Declaration 15 1 Dimension score <name> = new <type>[<dim size>]; score =](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-15.jpg)
![16 Multi-dimensional Array Declaration with Initialization 1 Dimension int[] score 6 1 3 score 16 Multi-dimensional Array Declaration with Initialization 1 Dimension int[] score 6 1 3 score](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-16.jpg)
![Index of Multi-dimensional Array 17 int[, ] score = { {5, 3, 1}, {9, Index of Multi-dimensional Array 17 int[, ] score = { {5, 3, 1}, {9,](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-17.jpg)
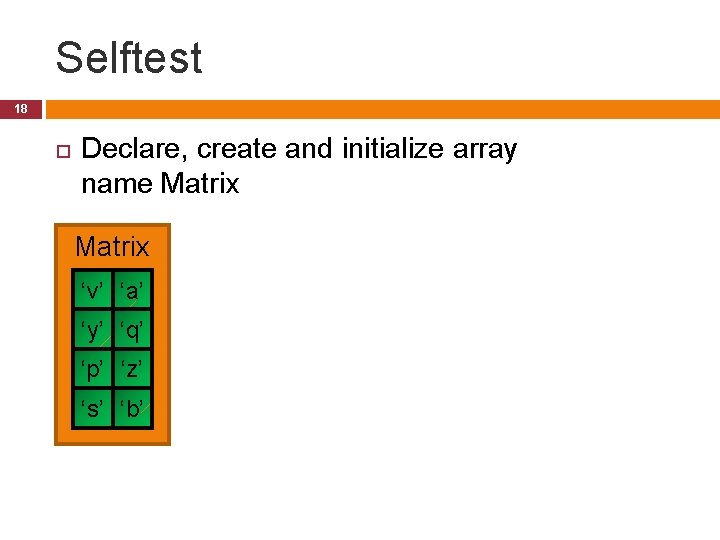
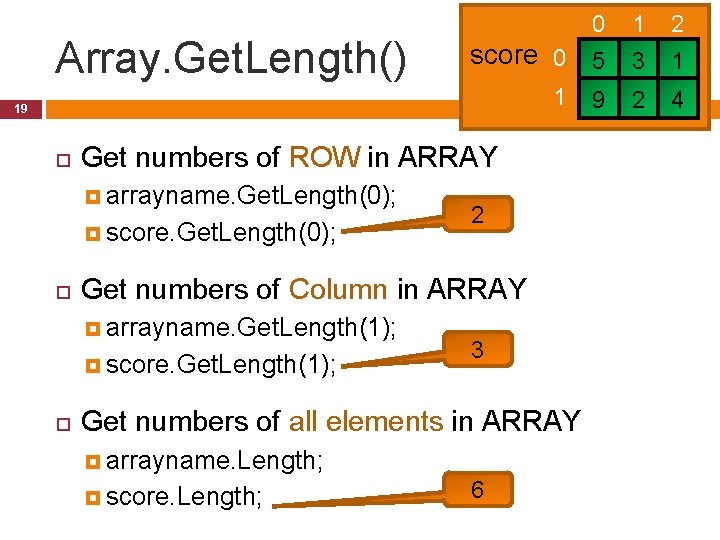
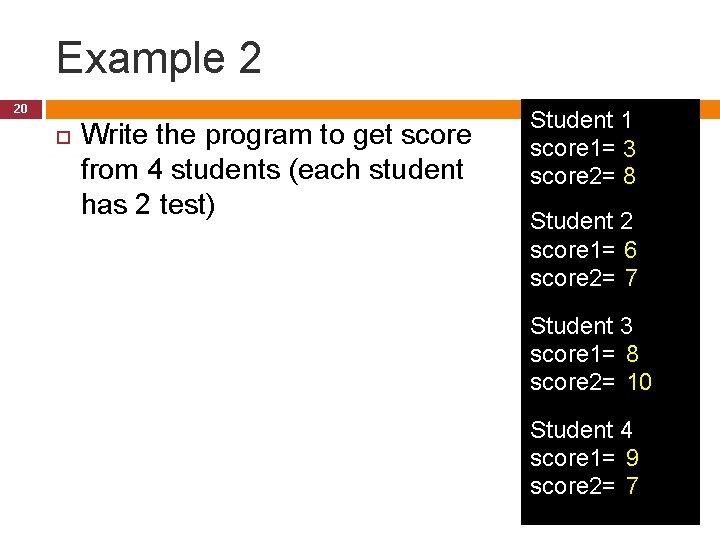
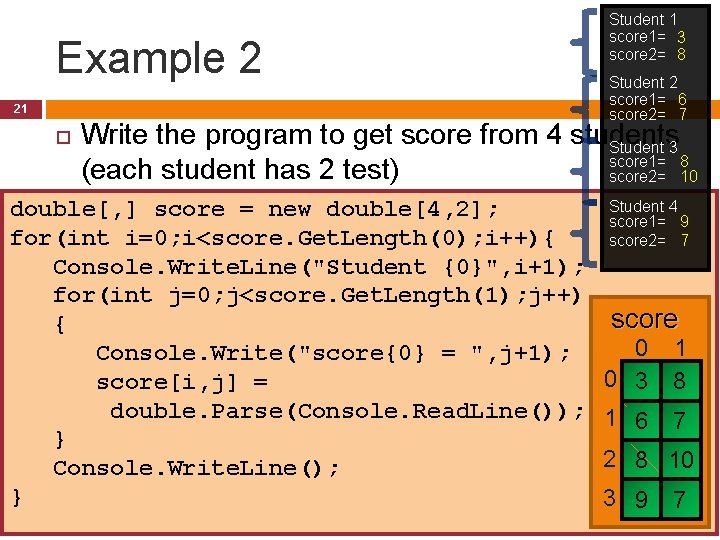
![Example 2 with Method 22 static void Main(){ double [, ] arr; arr = Example 2 with Method 22 static void Main(){ double [, ] arr; arr =](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-22.jpg)
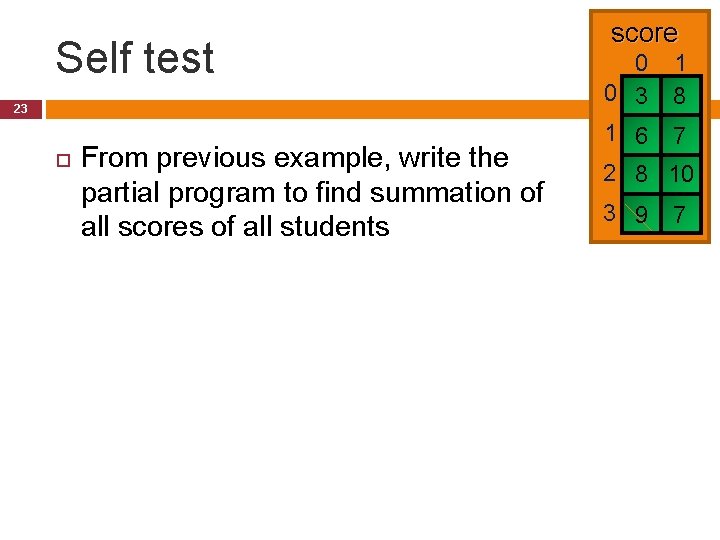
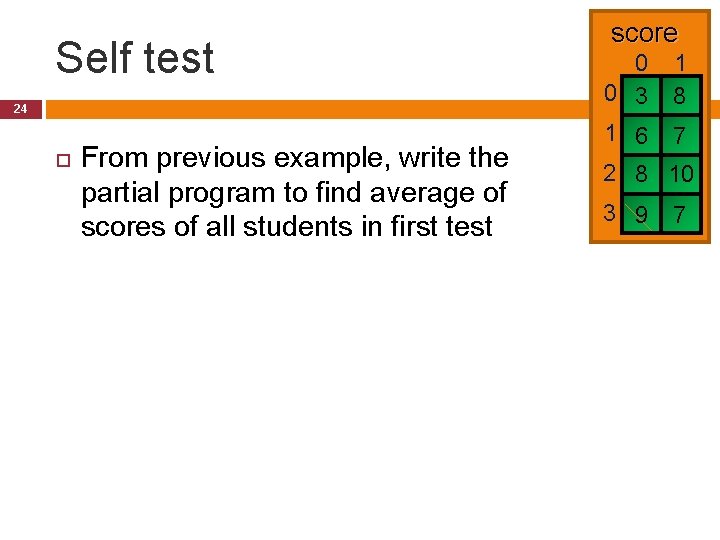
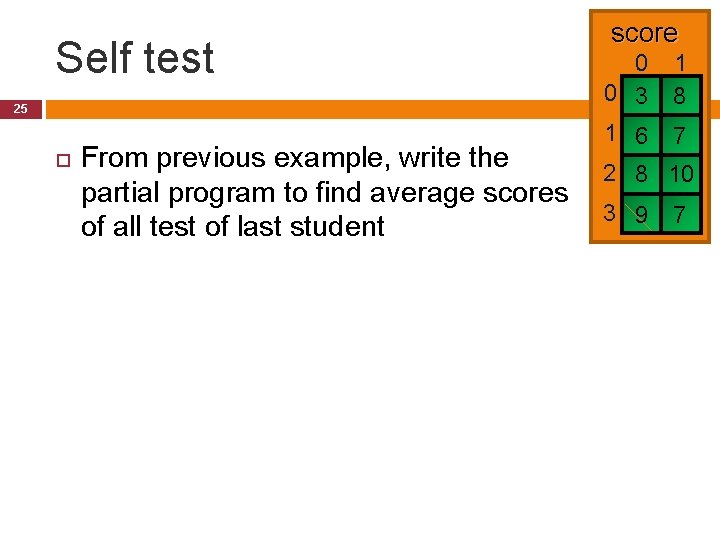
![How to find Array’s #dimension? By property Rank int [] matrix = new int[5]; How to find Array’s #dimension? By property Rank int [] matrix = new int[5];](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-26.jpg)
- Slides: 26
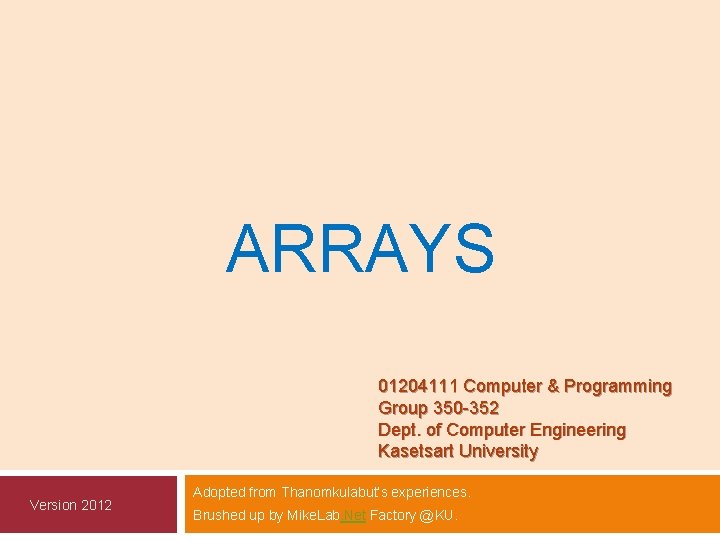
ARRAYS 01204111 Computer & Programming Group 350 -352 Dept. of Computer Engineering Kasetsart University Version 2012 Adopted from Thanomkulabut’s experiences. Brushed up by Mike. Lab. Net Factory @KU.
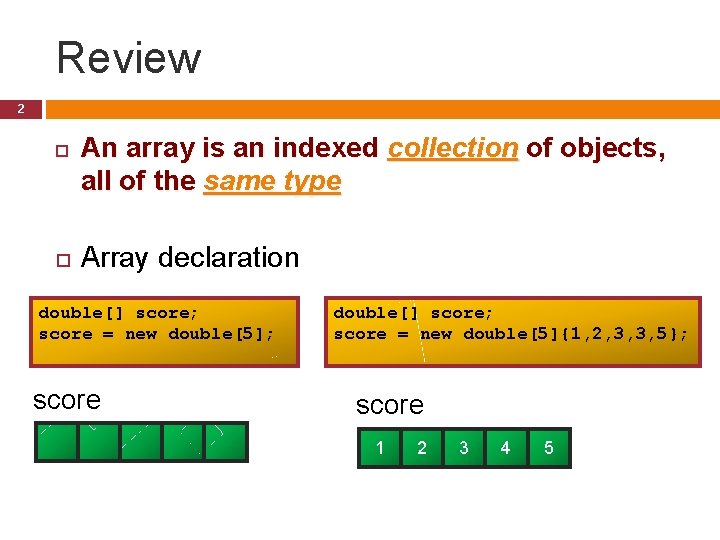
Review 2 An array is an indexed collection of objects, all of the same type Array declaration double[] score; score = new double[5]; score double[] score; score = new double[5]{1, 2, 3, 3, 5}; score 1 2 3 4 5
![Review 2 3 Declaration only int Declaration creation int ai ai Review (2) 3 Declaration only int Declaration & creation int [] ai; [] ai](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-3.jpg)
Review (2) 3 Declaration only int Declaration & creation int [] ai; [] ai = new int[4]; Declaration & creation & initialization int [] ai = new int[4] {1, 2, 3, 4}; int [] ai = new int[] {1, 2, 3, 4}; int [] ai = {1, 2, 3, 4};
![Review 3 4 Access array elements int score new int4 score 0 Review (3) 4 Access array elements int [] score = new int[4]; score 0](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-4.jpg)
Review (3) 4 Access array elements int [] score = new int[4]; score 0 -3 1 2 3 4 7 score[0] = -3; score[2+1] = 7; Score[3 -1] = score[0]+score[3] Console. Write. Line(score[2]); Runtime Error!! score[4] = 5; 4
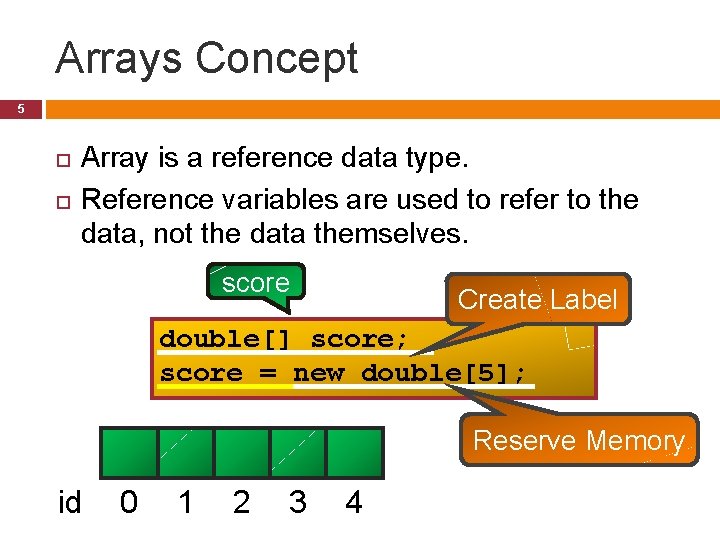
Arrays Concept 5 Array is a reference data type. Reference variables are used to refer to the data, not the data themselves. score Create Label double[] score; score = new double[5]; Reserve Memory id 0 1 2 3 4
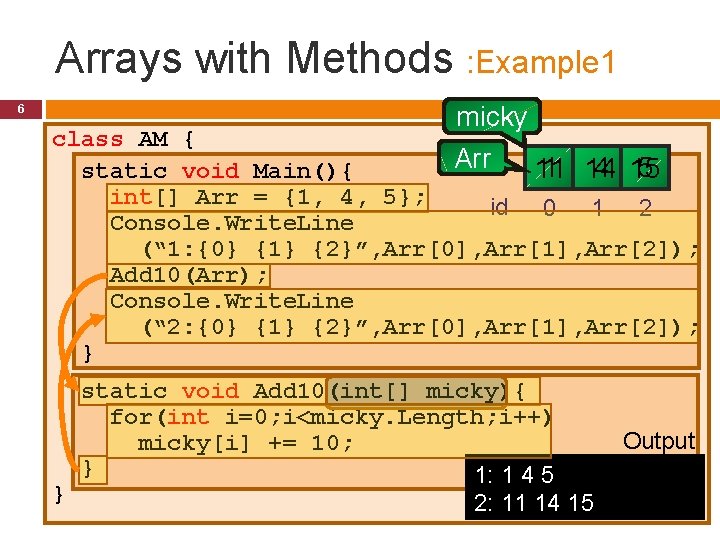
Arrays with Methods : Example 1 6 micky Arr 1 14 4 15 5 11 class AM { static void Main(){ int[] Arr = {1, 4, 5}; id 0 1 2 Console. Write. Line (“ 1: {0} {1} {2}”, Arr[0], Arr[1], Arr[2]); Add 10(Arr); Console. Write. Line (“ 2: {0} {1} {2}”, Arr[0], Arr[1], Arr[2]); } static void Add 10(int[] micky){ for(int i=0; i<micky. Length; i++) micky[i] += 10; } 1: 1 4 5 } 2: 11 14 15 Output
![Arrays with Methods Example 2 7 static void Main data info Nobi char Arrays with Methods : Example 2 7 static void Main(){ data info Nobi char[]](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-7.jpg)
Arrays with Methods : Example 2 7 static void Main(){ data info Nobi char[] data; info data = Read. Array(4); B e a r show. Array(data); N=4 } id 0 1 2 3 static char[] Read. Array(int N){ char[] info = new char[N]; for(int i=0; i<N; i++) info[i] = char. Parse(Console. Read. Line()); return info; } B e static void show. Array(char[] Nobi){ a for(int i=0; i<Nobi. Length; i++) r Console. Write(Nobi[i]); Monitor Be ar }
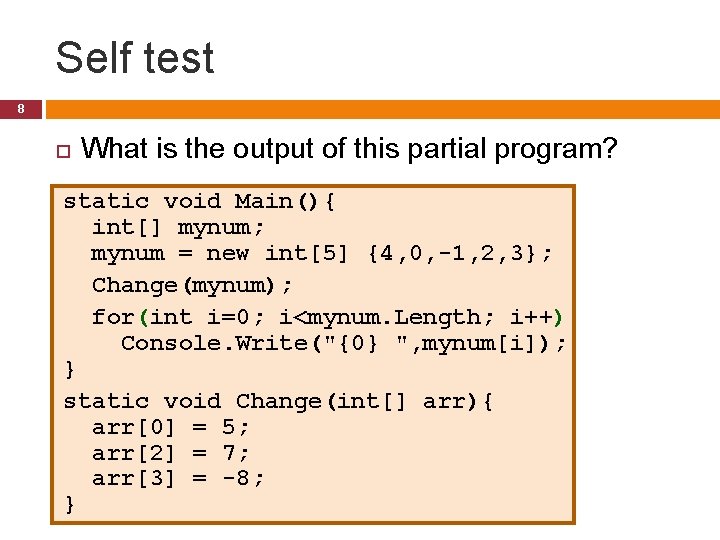
Self test 8 What is the output of this partial program? static void Main(){ int[] mynum; mynum = new int[5] {4, 0, -1, 2, 3}; Change(mynum); for(int i=0; i<mynum. Length; i++) Console. Write("{0} ", mynum[i]); } static void Change(int[] arr){ arr[0] = 5; arr[2] = 7; arr[3] = -8; }
![Example 1 9 static void Main char name 1 name 2 name 1 Example 1 9 static void Main(){ char[] name 1, name 2; name 1 =](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-9.jpg)
Example 1 9 static void Main(){ char[] name 1, name 2; name 1 = new char[4] {'N', 'O', 'B', 'I'}; What the output of this partial program? name 2 is = reverse(name 1); show. Arr(name 2); } static char[] reverse(char[] arr){ int j=0; char[] arr_re = new char[arr. Length]; for(int i=arr. Length-1; i>=0; i--){ arr_re[j] = arr[i]; Output j++; NOBI } return arr_re; IBON } static void show. Arr(char[] arr){ foreach(char x in arr) Console. Write(x); Console. Write. Line(); }
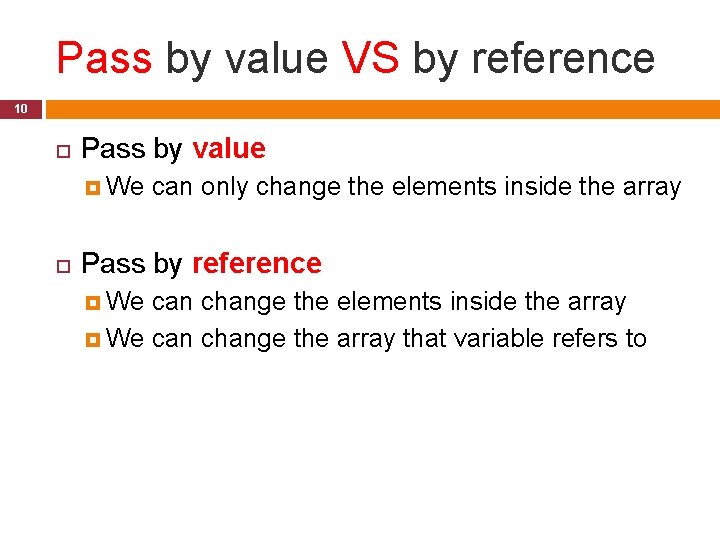
Pass by value VS by reference 10 Pass by value We can only change the elements inside the array Pass by reference We can change the elements inside the array We can change the array that variable refers to
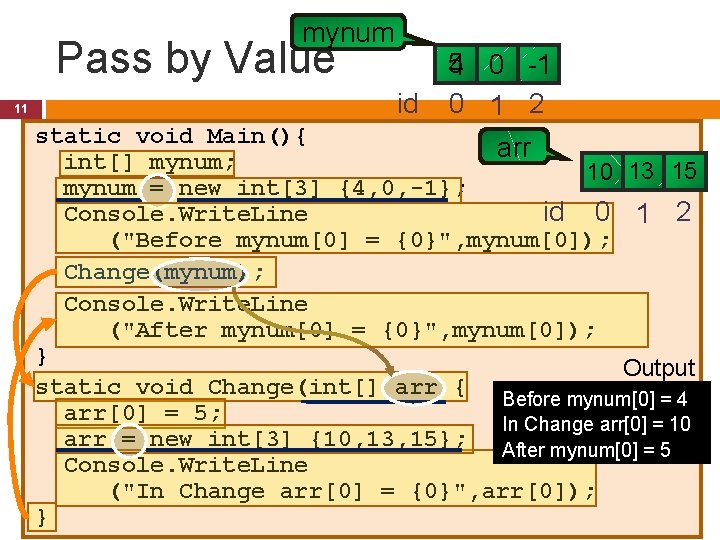
mynum Pass by Value 11 id 5 4 0 -1 0 1 2 arr static void Main(){ int[] mynum; 10 13 15 mynum = new int[3] {4, 0, -1}; id 0 1 2 Console. Write. Line ("Before mynum[0] = {0}", mynum[0]); Change(mynum); Console. Write. Line ("After mynum[0] = {0}", mynum[0]); } Output static void Change(int[] arr){ Before mynum[0] = 4 arr[0] = 5; In Change arr[0] = 10 arr = new int[3] {10, 13, 15}; After mynum[0] = 5 Console. Write. Line ("In Change arr[0] = {0}", arr[0]); }
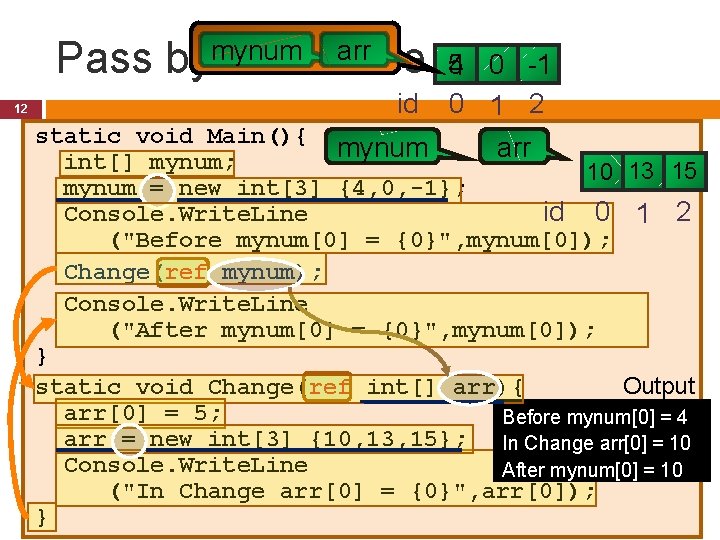
Pass mynum arr by Reference 5 4 0 -1 id 0 1 2 12 static void Main(){ mynum arr int[] mynum; 10 13 15 mynum = new int[3] {4, 0, -1}; id 0 Console. Write. Line ("Before mynum[0] = {0}", mynum[0]); Change(ref mynum); Console. Write. Line ("After mynum[0] = {0}", mynum[0]); 1 2 } Output static void Change(ref int[] arr){ arr[0] = 5; Before mynum[0] = 4 arr = new int[3] {10, 13, 15}; In Change arr[0] = 10 Console. Write. Line After mynum[0] = 10 ("In Change arr[0] = {0}", arr[0]); }
![13 Multidimensional Array introduction If you want to keep score of 50 students double 13 Multi-dimensional Array (introduction) If you want to keep score of 50 students double[]](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-13.jpg)
13 Multi-dimensional Array (introduction) If you want to keep score of 50 students double[] score = new double[50]; If each student have 10 test double[] score 0 = new double[50]; double[] score 1 = new double[50]; double[] score 2 = new double[50]; double[] score 9 = new double[50];
![14 Multidimensional Array Declaration 1 dimension type name int score Multidimensional 2 14 Multi-dimensional Array Declaration 1 dimension <type> [] <name>; int [] score; Multi-dimensional 2](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-14.jpg)
14 Multi-dimensional Array Declaration 1 dimension <type> [] <name>; int [] score; Multi-dimensional 2 Dimension <type> [ , ] <name>; int [ , ] score; 3 Dimension <type> [ , , ] <name>; int [ , , ] score;
![Multidimensional Array Declaration 15 1 Dimension score name new typedim size score Multi-dimensional Array Declaration 15 1 Dimension score <name> = new <type>[<dim size>]; score =](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-15.jpg)
Multi-dimensional Array Declaration 15 1 Dimension score <name> = new <type>[<dim size>]; score = new int[4]; score 2 Dimension <name> = new <type>[<dim 1 size >, <dim 2 size>]; score = new int[4, 2]; score 3 Dimension <name> = new <type>[<dim 1 size>, <dim 2 size>, <dim 3 size>]; score = new int[4, 2, 3];
![16 Multidimensional Array Declaration with Initialization 1 Dimension int score 6 1 3 score 16 Multi-dimensional Array Declaration with Initialization 1 Dimension int[] score 6 1 3 score](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-16.jpg)
16 Multi-dimensional Array Declaration with Initialization 1 Dimension int[] score 6 1 3 score = new int[3] {6, 1, 3}; 2 Dimension int [, ] score = new int[2, 3] { {1, 8, 4} , {3, 6, 9} }; int [, ] score = { {1, 8, 4} , {3, 6, 9} }; score 1 8 4 3 6 9
![Index of Multidimensional Array 17 int score 5 3 1 9 Index of Multi-dimensional Array 17 int[, ] score = { {5, 3, 1}, {9,](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-17.jpg)
Index of Multi-dimensional Array 17 int[, ] score = { {5, 3, 1}, {9, 2, 4} }; score[0, 1] = 7; score[2 -1 , 1+1] = 0; Console. Write(score[0, 0]); 0 score 0 53 1 5 for(int i = 0; i<=2 ; i++) score[0, i] = 3; Console. Write(score. Length); 6 9 1 7 3 2 2 0 4 1 3
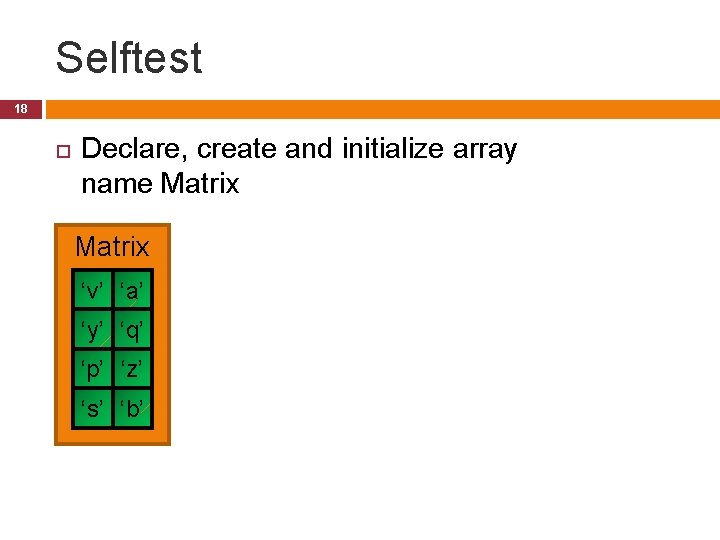
Selftest 18 Declare, create and initialize array name Matrix ‘v’ ‘a’ ‘y’ ‘q’ ‘p’ ‘z’ ‘s’ ‘b’ Format I char[, ] Matrix; Matrix = new char[4, 2]{ {'v', 'a'}, {'y', 'q'}, {'p', 'z'}, {'s', 'b'} };
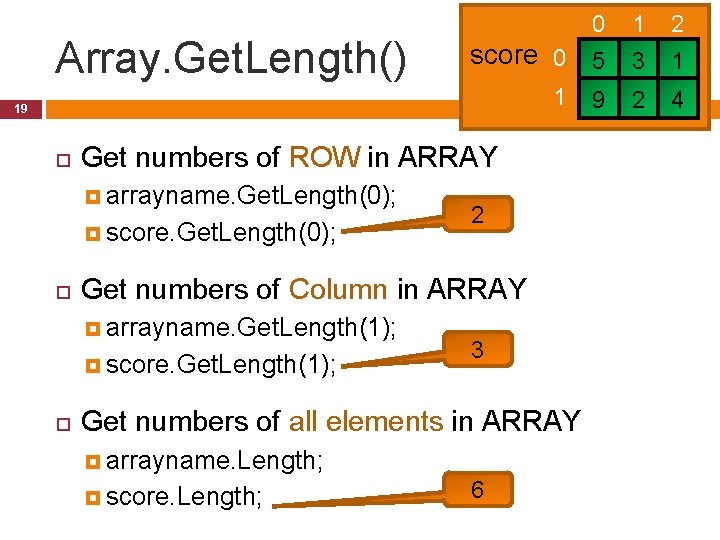
Array. Get. Length() 0 1 Get numbers of ROW in ARRAY arrayname. Get. Length(0); score. Get. Length(1); 2 Get numbers of Column in ARRAY arrayname. Get. Length(1); 3 Get numbers of all elements in ARRAY arrayname. Length; score. Length; 2 score 0 5 3 1 19 1 6 9 2 4
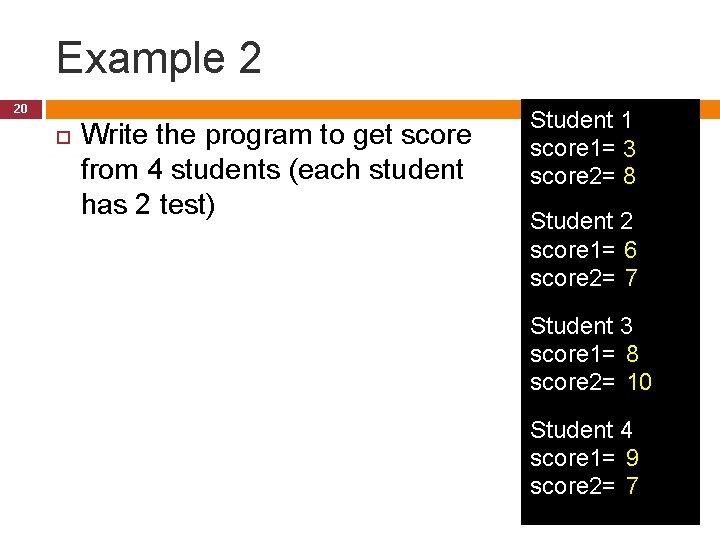
Example 2 20 Write the program to get score from 4 students (each student has 2 test) Student 1 score 1= 3 score 2= 8 Student 2 score 1= 6 score 2= 7 Student 3 score 1= 8 score 2= 10 Student 4 score 1= 9 score 2= 7
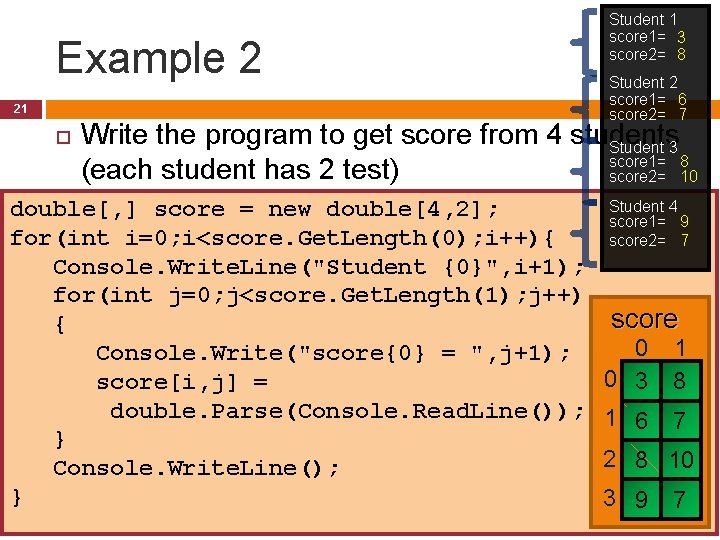
Example 2 21 Student 1 score 1= 3 score 2= 8 Student 2 score 1= 6 score 2= 7 Write the program to get score from 4 students Student 3 score 1= 8 (each student has 2 test) score 2= 10 double[, ] score = new double[4, 2]; for(int i=0; i<score. Get. Length(0); i++){ Console. Write. Line("Student {0}", i+1); for(int j=0; j<score. Get. Length(1); j++) { Console. Write("score{0} = ", j+1); score[i, j] = double. Parse(Console. Read. Line()); } Console. Write. Line(); } Student 4 score 1= 9 score 2= 7 score 0 0 3 1 8 1 6 7 2 8 10 3 9 7
![Example 2 with Method 22 static void Main double arr arr Example 2 with Method 22 static void Main(){ double [, ] arr; arr =](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-22.jpg)
Example 2 with Method 22 static void Main(){ double [, ] arr; arr = Read. Array 2(2, 4); } static double[, ] Read. Array 2(int row, int col){ double[, ] score = new double[4, 2]; for(int i=0; i<score. Get. Length(0); i++){ Console. Write. Line("Student {0}", i+1); for(int j=0; j<score. Get. Length(1); j++){ Console. Write("score{0} = ", j+1); score[i, j] = double. Parse(Console. Read. Line()); } Console. Write. Line(); } return score; }
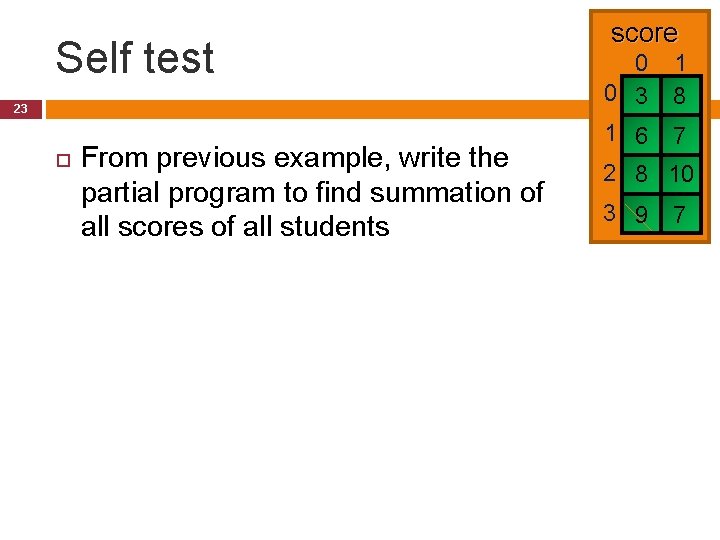
Self test 23 From previous example, write the partial program to find summation of all scores of all students score 0 0 3 1 8 1 6 7 2 8 10 3 9 double sum = 0; for(int i=0; i<score. Get. Length(0); i++){ for(int j=0; j<score. Get. Length(1); j++){ sum += score[i, j]; } } Console. Write. Line("Sumation = {0}", sum); 7
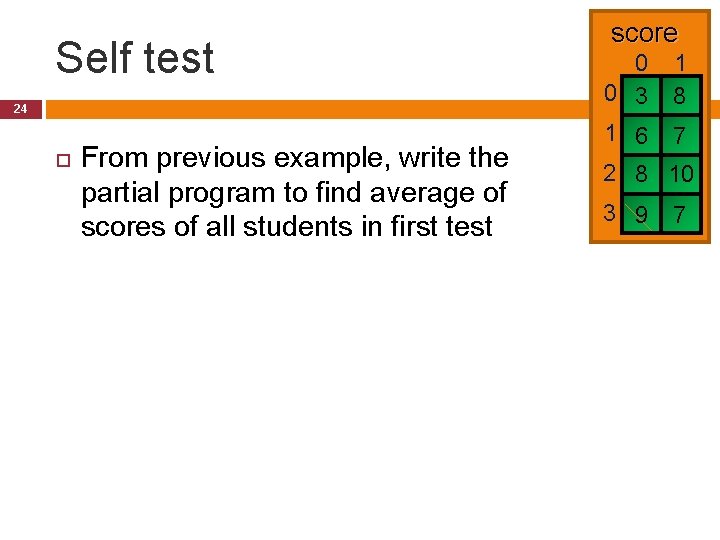
Self test 24 From previous example, write the partial program to find average of scores of all students in first test score 0 0 3 1 8 1 6 7 2 8 10 3 9 double sum = 0; for(int i=0; i<score. Get. Length(0); i++){ sum += score[i, 0]; } double avg = sum/score. Get. Length(0); Console. Write. Line ("Average of First test = {0}", avg); 7
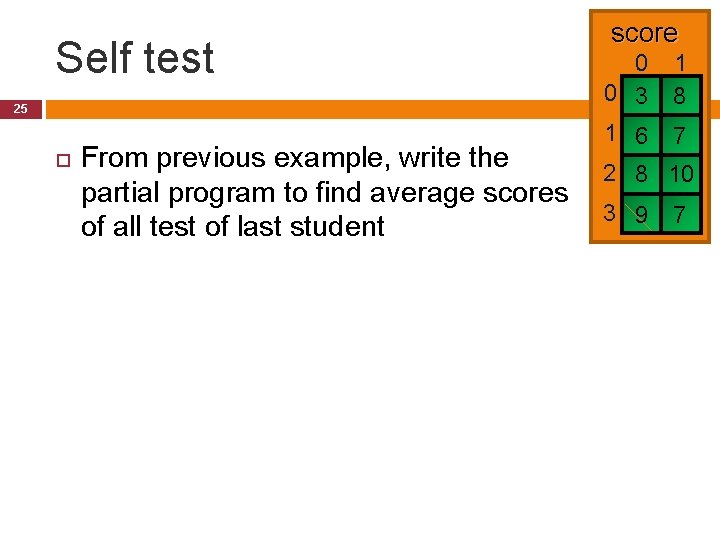
Self test 25 From previous example, write the partial program to find average scores of all test of last student score 0 0 3 1 8 1 6 7 2 8 10 3 9 double sum = 0; for(int j=0; j<score. Get. Length(1); j++){ sum += score[3, j]; } double avg = sum/score. Get. Length(1); Console. Write. Line ("Average of last student = {0}", avg); 7
![How to find Arrays dimension By property Rank int matrix new int5 How to find Array’s #dimension? By property Rank int [] matrix = new int[5];](https://slidetodoc.com/presentation_image_h/b43c1ee431efdfecb75cfbc1047b68f4/image-26.jpg)
How to find Array’s #dimension? By property Rank int [] matrix = new int[5]; Console. Write. Line(matrix. Rank); 1 int [, ] matrix = new int[5, 2]; Console. Write. Line(matrix. Rank); 2 int [, , ] matrix = new int[5, 2, 3]; Console. Write. Line(matrix. Rank); 3
01204111
01204111
Https://pixabay
Computer science arrays
A computer company receives 350 applications
One hot state assignment
It 352
352 formação
Comp 352
Aci 352
Troop 352
Aae 352
Exam feedback examples
Os 352
Os 352
Coen 352
Opwekking 464
Ece 352
Tmk 352
Ece 352
Os 352
Csc 352
Scm 352
Parallel arrays examples
Array of arrays c++
Ragged array
Veteork