Array and String 1 Array is a collection
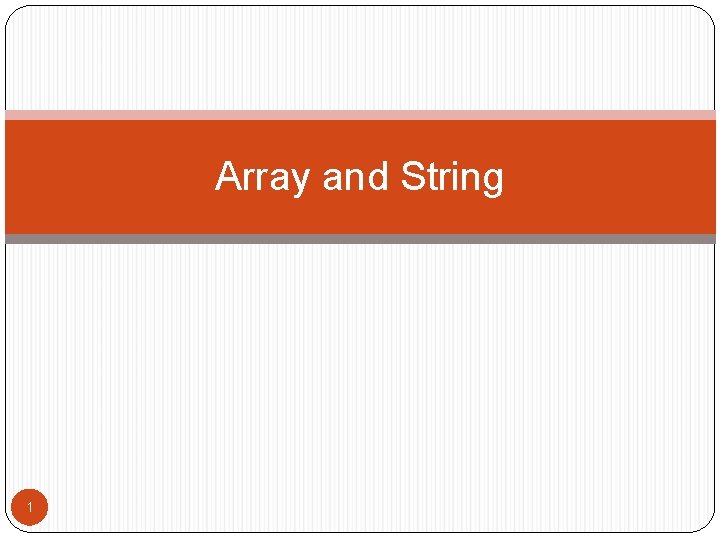
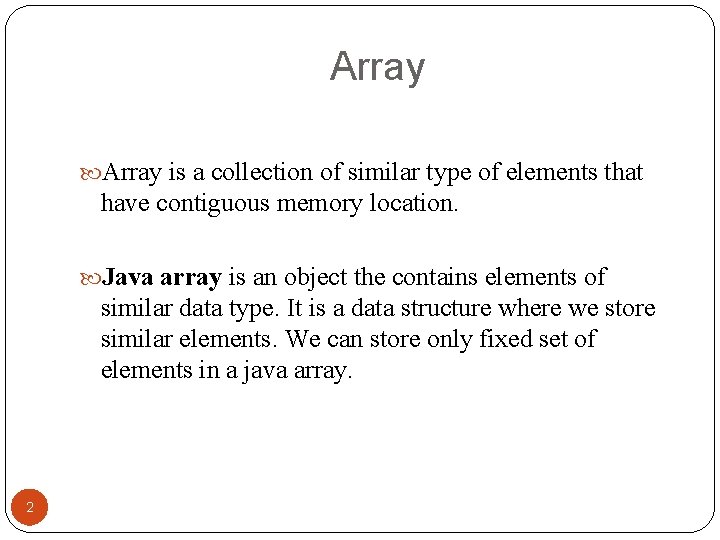
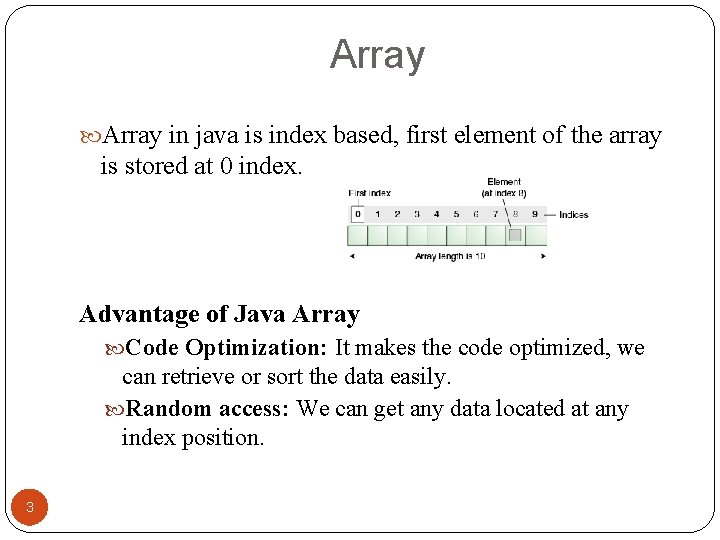
![Syntax to Declare an Array in java data. Type[] arr; (or) data. Type []arr; Syntax to Declare an Array in java data. Type[] arr; (or) data. Type []arr;](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-4.jpg)
![Declare arrays byte[] an. Array. Of. Bytes; short[] an. Array. Of. Shorts; long[] an. Declare arrays byte[] an. Array. Of. Bytes; short[] an. Array. Of. Shorts; long[] an.](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-5.jpg)
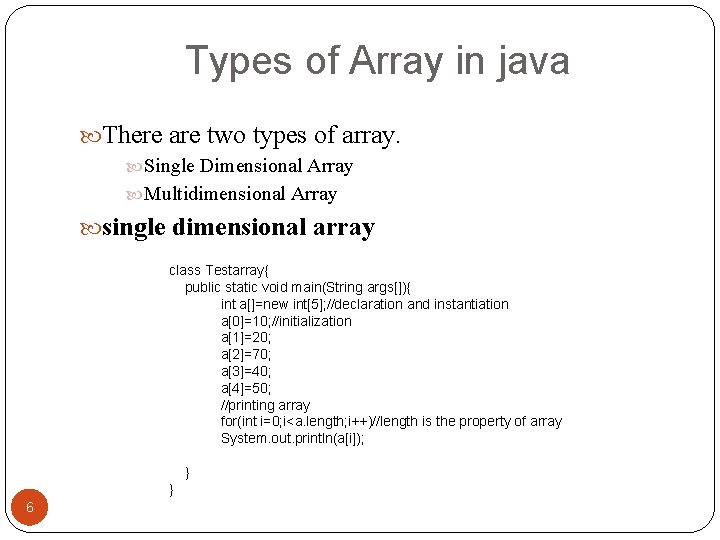
![Initializing array // create an array of integers an. Array = new int[10]; an. Initializing array // create an array of integers an. Array = new int[10]; an.](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-7.jpg)
![Example of Array class Array. Demo { public static void main(String[] args) { // Example of Array class Array. Demo { public static void main(String[] args) { //](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-8.jpg)
![Instantiation and Initialization of Array int a[]={33, 3, 4, 5}; //declaration, instantiation and initialization Instantiation and Initialization of Array int a[]={33, 3, 4, 5}; //declaration, instantiation and initialization](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-9.jpg)
![Passing Array to method class Testarray 2{ static void min(int arr[]){ int min=arr[0]; for(int Passing Array to method class Testarray 2{ static void min(int arr[]){ int min=arr[0]; for(int](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-10.jpg)
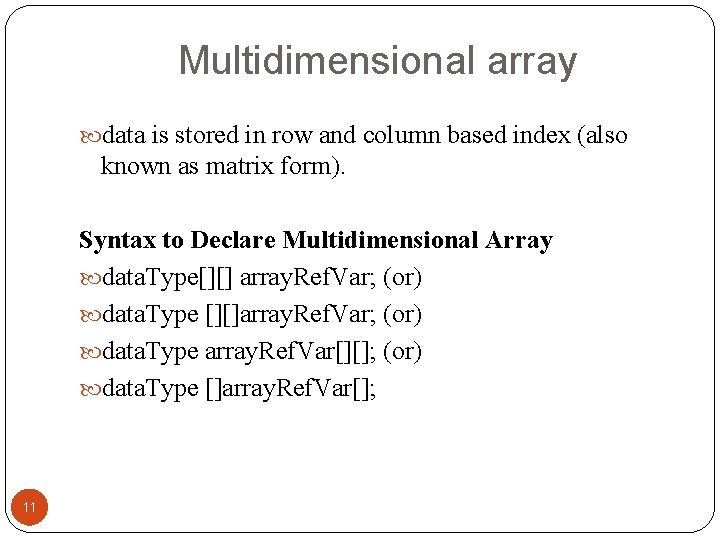
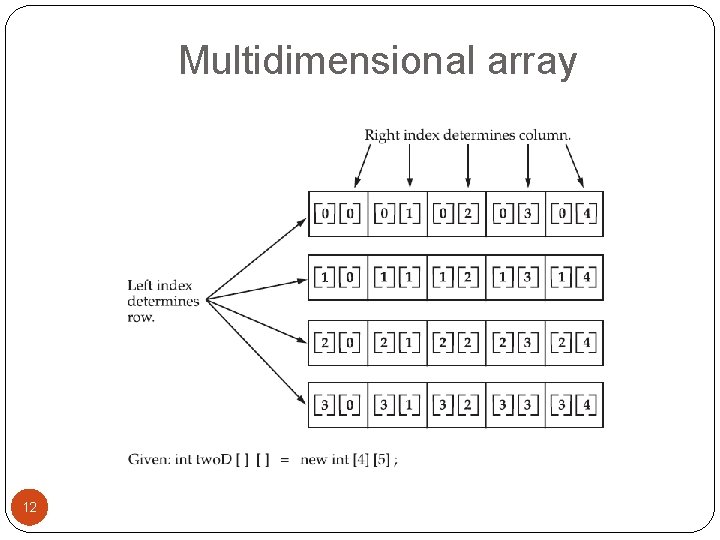
![Multidimensional array int[][] arr=new int[3][3]; //3 row and 3 column initialize arr[0][0]=1; arr[0][1]=2; arr[0][2]=3; Multidimensional array int[][] arr=new int[3][3]; //3 row and 3 column initialize arr[0][0]=1; arr[0][1]=2; arr[0][2]=3;](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-13.jpg)
![Multidimensional array long[][][] beans = new long[5][10][30]; long[][][] beans = new long[3][][]; // Three Multidimensional array long[][][] beans = new long[5][10][30]; long[][][] beans = new long[3][][]; // Three](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-14.jpg)
![Example of Multidimensional array class Testarray 3{ public static void main(String args[]){ //declaring and Example of Multidimensional array class Testarray 3{ public static void main(String args[]){ //declaring and](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-15.jpg)
![Example of Multidimensional array class Two. DAgain { public static void main(String args[]) { Example of Multidimensional array class Two. DAgain { public static void main(String args[]) {](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-16.jpg)
![Initialization of Array using Method double[] data = new double[50]; import java. util. Arrays; Initialization of Array using Method double[] data = new double[50]; import java. util. Arrays;](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-17.jpg)
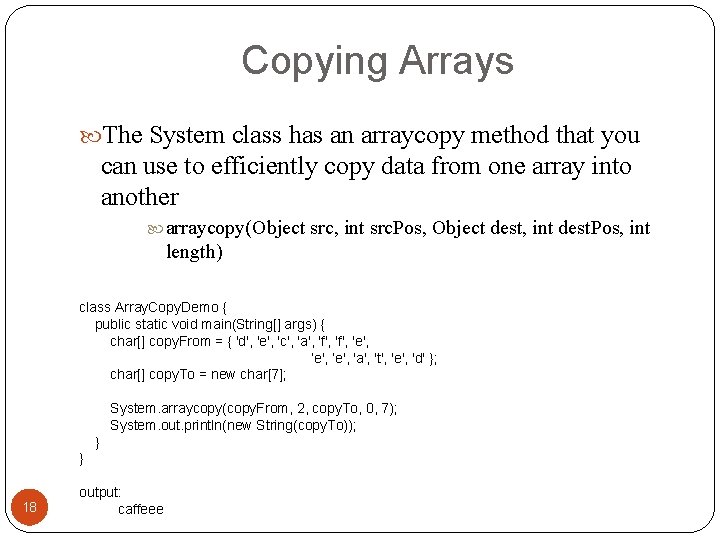
![Addition of 2 matrices class Testarray 5{ public static void main(String args[]){ //creating two Addition of 2 matrices class Testarray 5{ public static void main(String args[]){ //creating two](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-19.jpg)
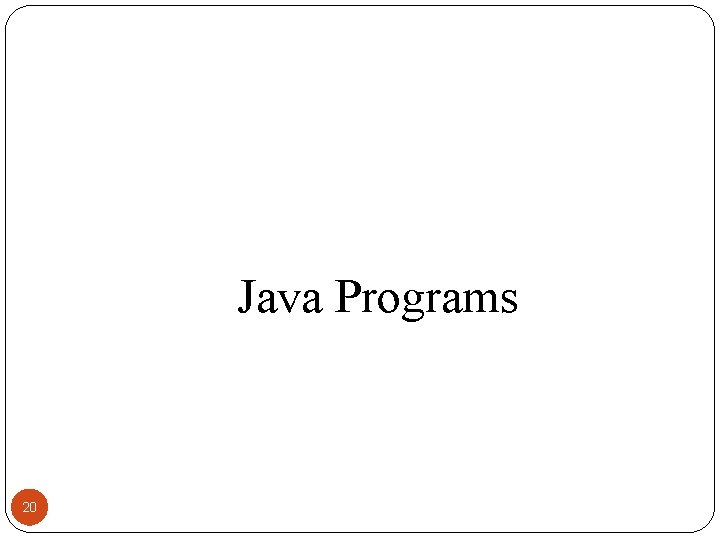
- Slides: 20
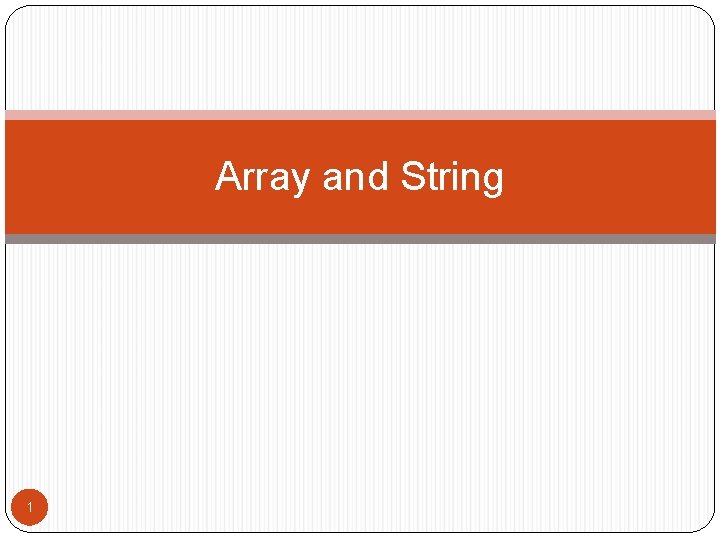
Array and String 1
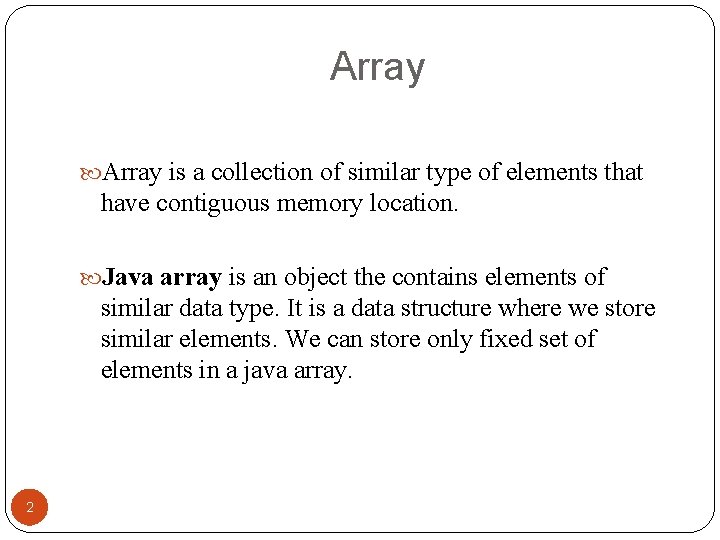
Array is a collection of similar type of elements that have contiguous memory location. Java array is an object the contains elements of similar data type. It is a data structure where we store similar elements. We can store only fixed set of elements in a java array. 2
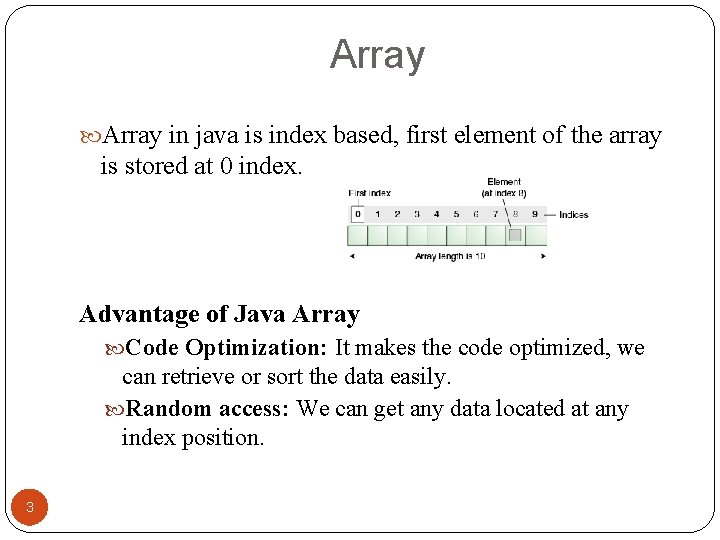
Array in java is index based, first element of the array is stored at 0 index. Advantage of Java Array Code Optimization: It makes the code optimized, we can retrieve or sort the data easily. Random access: We can get any data located at any index position. 3
![Syntax to Declare an Array in java data Type arr or data Type arr Syntax to Declare an Array in java data. Type[] arr; (or) data. Type []arr;](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-4.jpg)
Syntax to Declare an Array in java data. Type[] arr; (or) data. Type []arr; (or) data. Type arr[]; Instantiation of an Array array. Ref. Var=new datatype[size]; 4
![Declare arrays byte an Array Of Bytes short an Array Of Shorts long an Declare arrays byte[] an. Array. Of. Bytes; short[] an. Array. Of. Shorts; long[] an.](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-5.jpg)
Declare arrays byte[] an. Array. Of. Bytes; short[] an. Array. Of. Shorts; long[] an. Array. Of. Longs; float[] an. Array. Of. Floats; double[] an. Array. Of. Doubles; boolean[] an. Array. Of. Booleans; char[] an. Array. Of. Chars; String[] an. Array. Of. Strings; 5
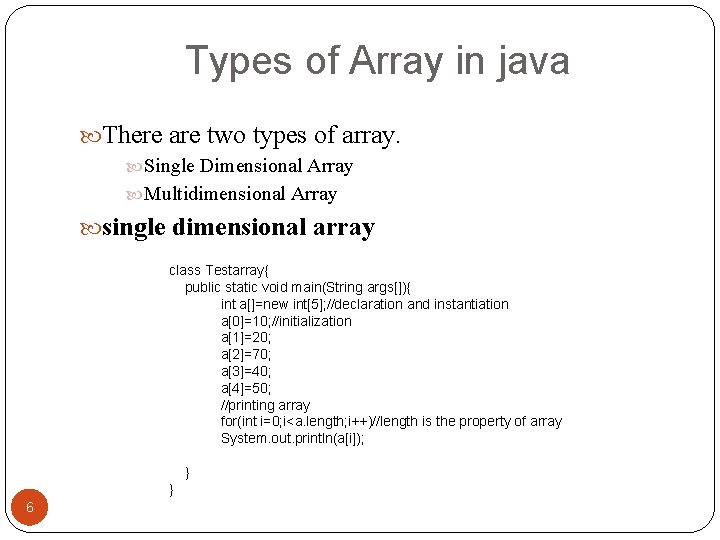
Types of Array in java There are two types of array. Single Dimensional Array Multidimensional Array single dimensional array class Testarray{ public static void main(String args[]){ int a[]=new int[5]; //declaration and instantiation a[0]=10; //initialization a[1]=20; a[2]=70; a[3]=40; a[4]=50; //printing array for(int i=0; i<a. length; i++)//length is the property of array System. out. println(a[i]); } } 6
![Initializing array create an array of integers an Array new int10 an Initializing array // create an array of integers an. Array = new int[10]; an.](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-7.jpg)
Initializing array // create an array of integers an. Array = new int[10]; an. Array[0] = 100; // initialize first element an. Array[1] = 200; // initialize second element 7
![Example of Array class Array Demo public static void mainString args Example of Array class Array. Demo { public static void main(String[] args) { //](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-8.jpg)
Example of Array class Array. Demo { public static void main(String[] args) { // declares an array of integers int[] an. Array; // allocates memory for 5 integers an. Array = new int[5]; // initialize first element an. Array[0] = 100; // initialize second element an. Array[1] = 200; // and so forth an. Array[2] = 300; an. Array[3] = 400; an. Array[4] = 500; ; System. out. println("Element at index 0: “ System. out. println("Element at index 1: “ System. out. println("Element at index 2: “ System. out. println("Element at index 3: “ System. out. println("Element at index 4: “ } 8 } + an. Array[0]); + an. Array[1]); + an. Array[2]); + an. Array[3]); + an. Array[4]);
![Instantiation and Initialization of Array int a33 3 4 5 declaration instantiation and initialization Instantiation and Initialization of Array int a[]={33, 3, 4, 5}; //declaration, instantiation and initialization](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-9.jpg)
Instantiation and Initialization of Array int a[]={33, 3, 4, 5}; //declaration, instantiation and initialization class Testarray 1{ public static void main(String args[]){ int a[]={33, 3, 4, 5}; //declaration, instantiation and initialization //printing array for(int i=0; i<a. length; i++)//length is the property of array System. out. println(a[i]); }} 9
![Passing Array to method class Testarray 2 static void minint arr int minarr0 forint Passing Array to method class Testarray 2{ static void min(int arr[]){ int min=arr[0]; for(int](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-10.jpg)
Passing Array to method class Testarray 2{ static void min(int arr[]){ int min=arr[0]; for(int i=1; i<arr. length; i++) if(min>arr[i]) min=arr[i]; System. out. println(min); } public static void main(String args[]){ int a[]={33, 3, 4, 5}; min(a); //passing array to method 10 }}
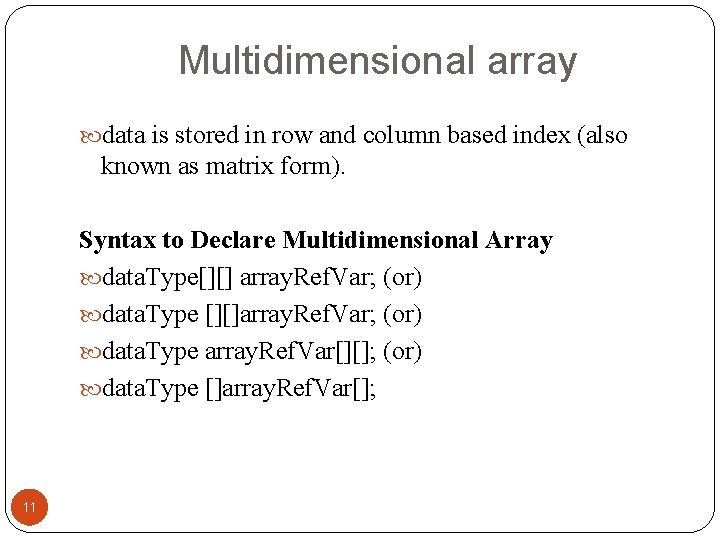
Multidimensional array data is stored in row and column based index (also known as matrix form). Syntax to Declare Multidimensional Array data. Type[][] array. Ref. Var; (or) data. Type [][]array. Ref. Var; (or) data. Type array. Ref. Var[][]; (or) data. Type []array. Ref. Var[]; 11
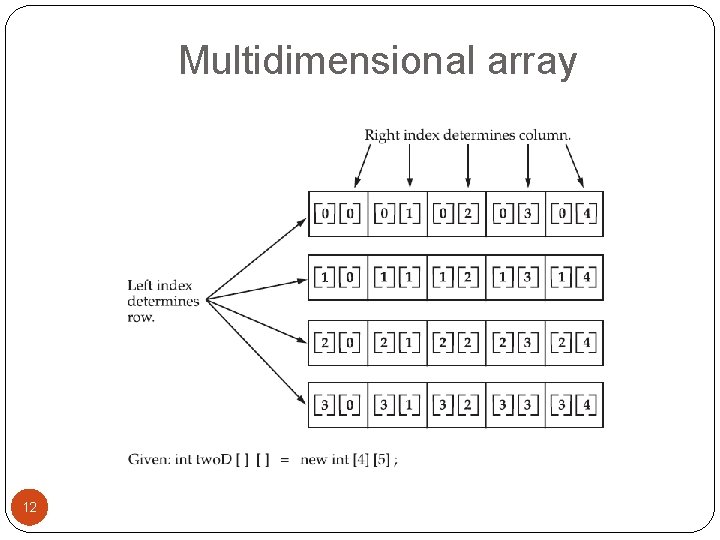
Multidimensional array 12
![Multidimensional array int arrnew int33 3 row and 3 column initialize arr001 arr012 arr023 Multidimensional array int[][] arr=new int[3][3]; //3 row and 3 column initialize arr[0][0]=1; arr[0][1]=2; arr[0][2]=3;](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-13.jpg)
Multidimensional array int[][] arr=new int[3][3]; //3 row and 3 column initialize arr[0][0]=1; arr[0][1]=2; arr[0][2]=3; arr[1][0]=4; arr[1][1]=5; arr[1][2]=6; arr[2][0]=7; arr[2][1]=8; arr[2][2]=9; 13
![Multidimensional array long beans new long51030 long beans new long3 Three Multidimensional array long[][][] beans = new long[5][10][30]; long[][][] beans = new long[3][][]; // Three](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-14.jpg)
Multidimensional array long[][][] beans = new long[5][10][30]; long[][][] beans = new long[3][][]; // Three two-dimensional arrays beans[0] = new long[4][]; beans[1] = new long[2][]; beans[2] = new long[5][]; 14
![Example of Multidimensional array class Testarray 3 public static void mainString args declaring and Example of Multidimensional array class Testarray 3{ public static void main(String args[]){ //declaring and](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-15.jpg)
Example of Multidimensional array class Testarray 3{ public static void main(String args[]){ //declaring and initializing 2 D array int arr[][]={{1, 2, 3}, {2, 4, 5}, {4, 4, 5}}; //printing 2 D array for(int i=0; i<3; i++){ for(int j=0; j<3; j++){ System. out. print(arr[i][j]+" "); } System. out. println(); } }} 15 Output: 1 2 3 245 445
![Example of Multidimensional array class Two DAgain public static void mainString args Example of Multidimensional array class Two. DAgain { public static void main(String args[]) {](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-16.jpg)
Example of Multidimensional array class Two. DAgain { public static void main(String args[]) { int two. D[][] = new int[4][]; two. D[0] = new int[1]; two. D[1] = new int[2]; two. D[2] = new int[3]; two. D[3] = new int[4]; int i, j, k = 0; for(i=0; i<4; i++) for(j=0; j<i+1; j++) { two. D[i][j] = k; k++; } for(i=0; i<4; i++) { for(j=0; j<i+1; j++) System. out. print(two. D[i][j] + " "); System. out. println(); } } } 16 output: 0 12 345 6789
![Initialization of Array using Method double data new double50 import java util Arrays Initialization of Array using Method double[] data = new double[50]; import java. util. Arrays;](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-17.jpg)
Initialization of Array using Method double[] data = new double[50]; import java. util. Arrays; Arrays. fill(data, 1. 0); 17
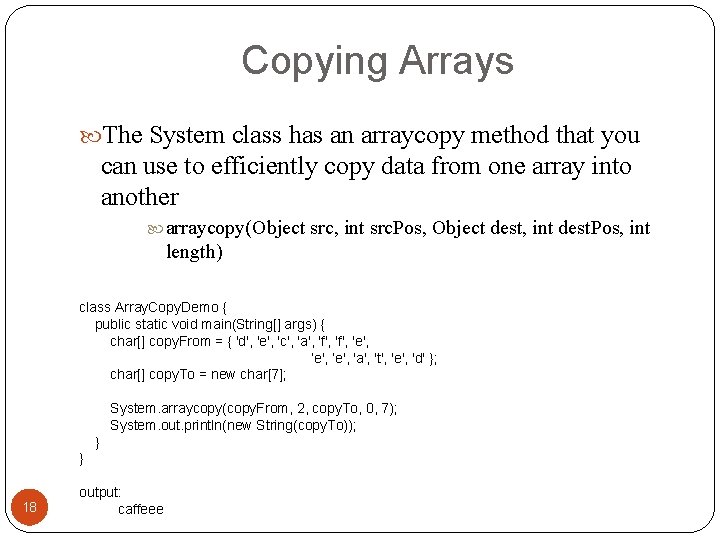
Copying Arrays The System class has an arraycopy method that you can use to efficiently copy data from one array into another arraycopy(Object src, int src. Pos, Object dest, int dest. Pos, int length) class Array. Copy. Demo { public static void main(String[] args) { char[] copy. From = { 'd', 'e', 'c', 'a', 'f', 'e', ‘e', 'a', 't', 'e', 'd' }; char[] copy. To = new char[7]; System. arraycopy(copy. From, 2, copy. To, 0, 7); System. out. println(new String(copy. To)); } } 18 output: caffeee
![Addition of 2 matrices class Testarray 5 public static void mainString args creating two Addition of 2 matrices class Testarray 5{ public static void main(String args[]){ //creating two](https://slidetodoc.com/presentation_image/dc663de7195e72d7d1101c67925fc6e5/image-19.jpg)
Addition of 2 matrices class Testarray 5{ public static void main(String args[]){ //creating two matrices int a[][]={{1, 3, 4}, {3, 4, 5}}; int b[][]={{1, 3, 4}, {3, 4, 5}}; //creating another matrix to store the sum of two matrices int c[][]=new int[2][3]; //adding and printing addition of 2 matrices for(int i=0; i<2; i++){ for(int j=0; j<3; j++){ c[i][j]=a[i][j]+b[i][j]; System. out. print(c[i][j]+" "); } System. out. println(); //new line } }} 19 Output: 2 6 8 10
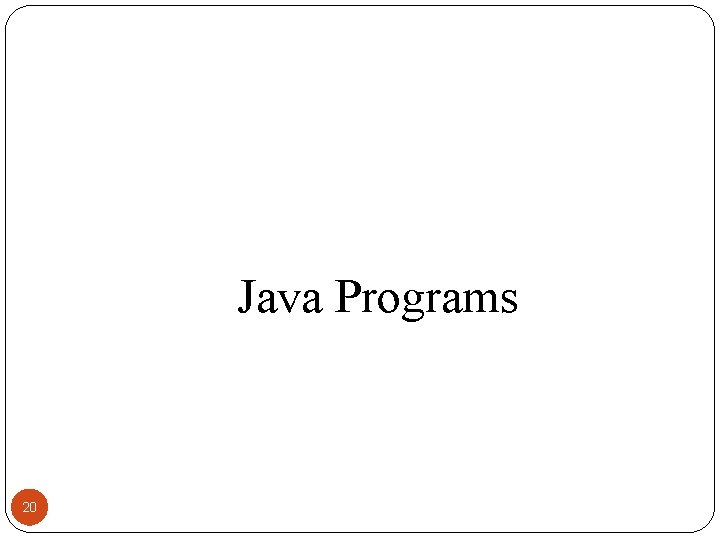
Java Programs 20