Arithmetic Logic Unit ALU Discussion D 4 6
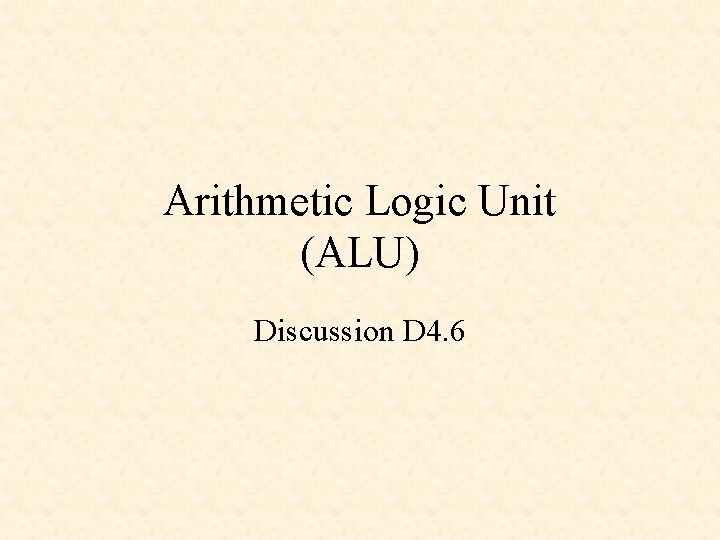
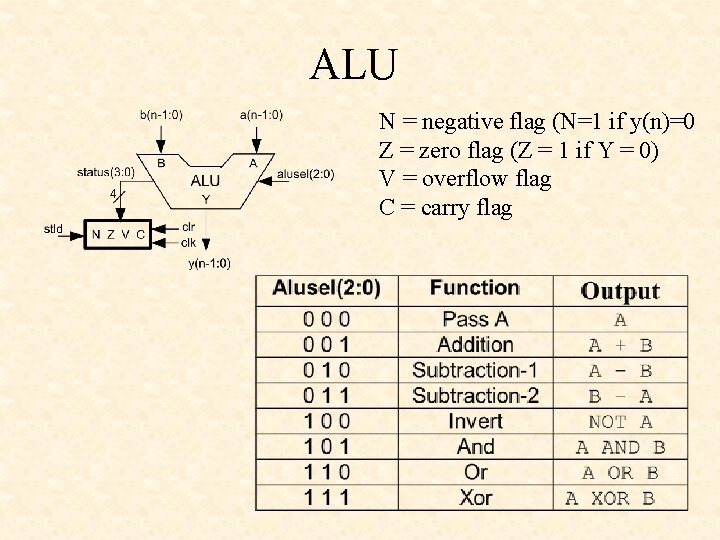
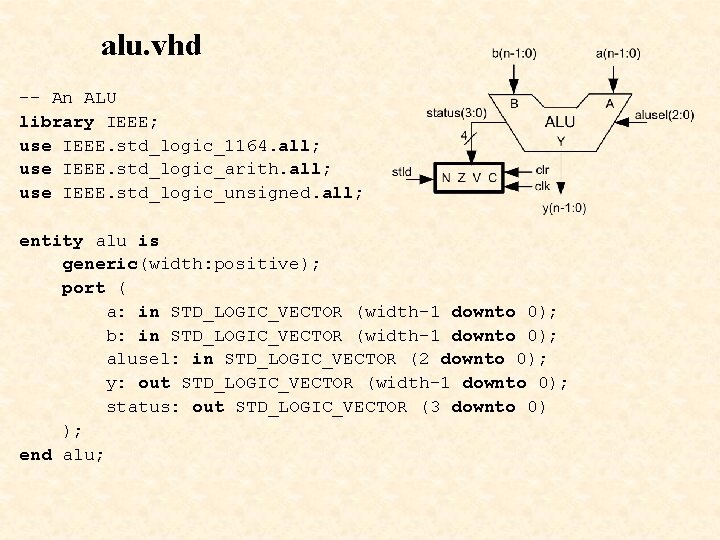
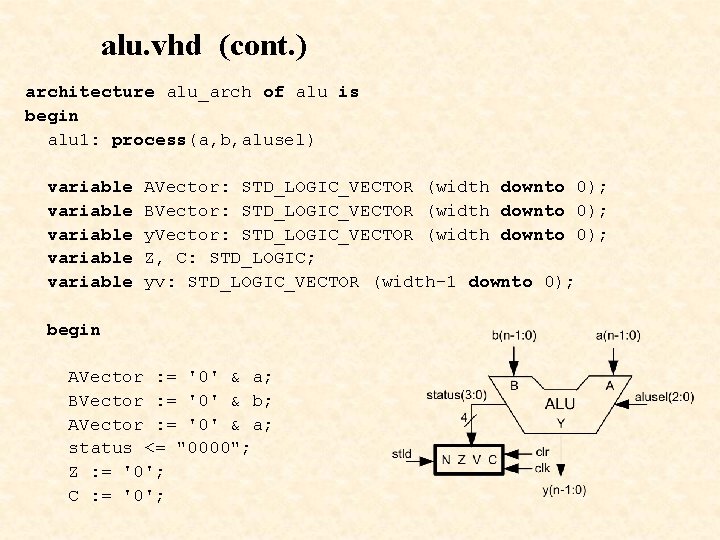
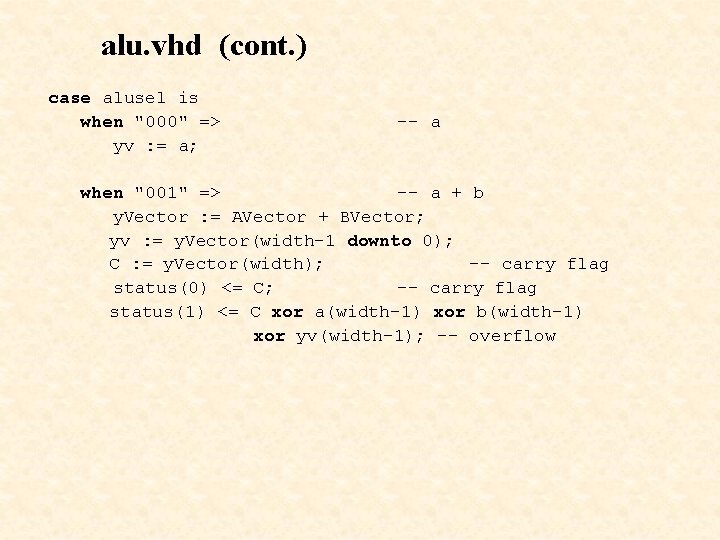
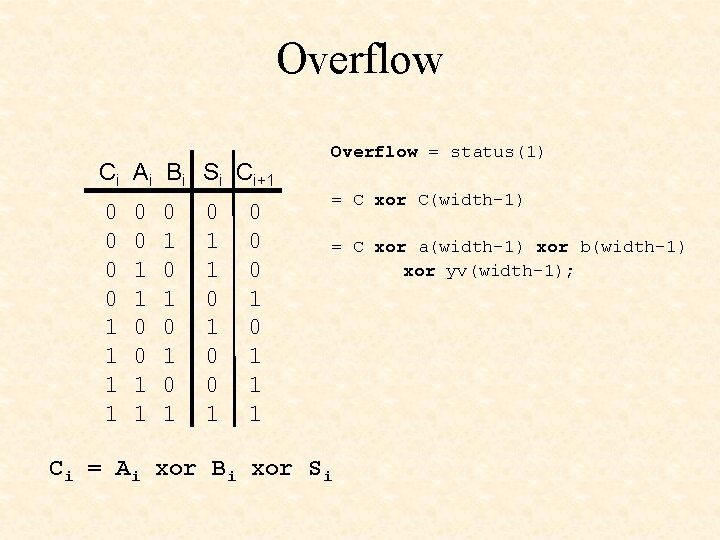
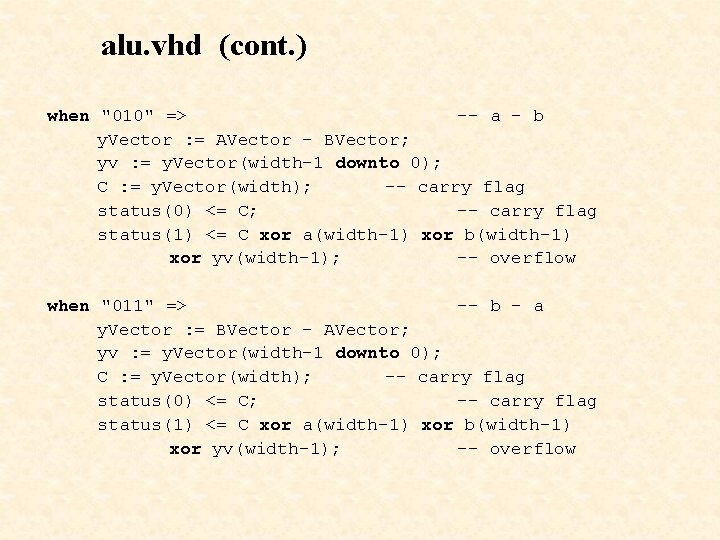
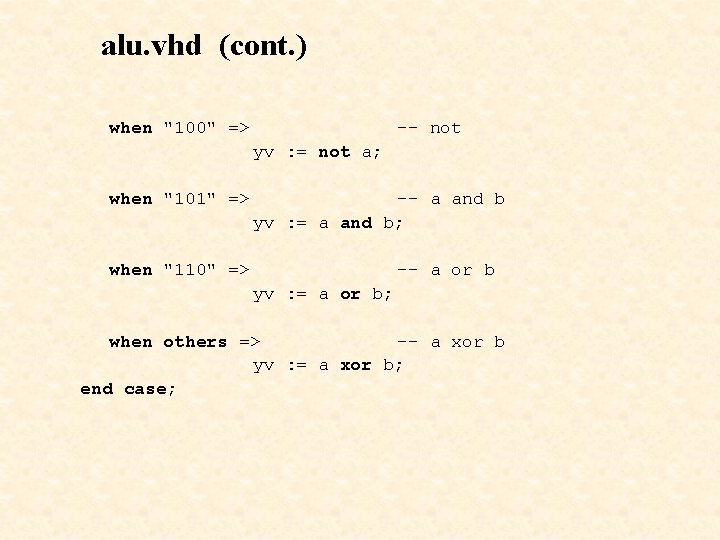
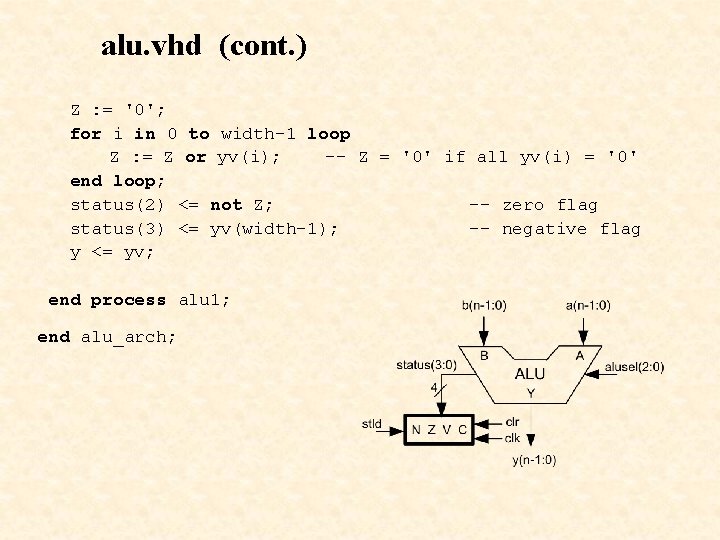
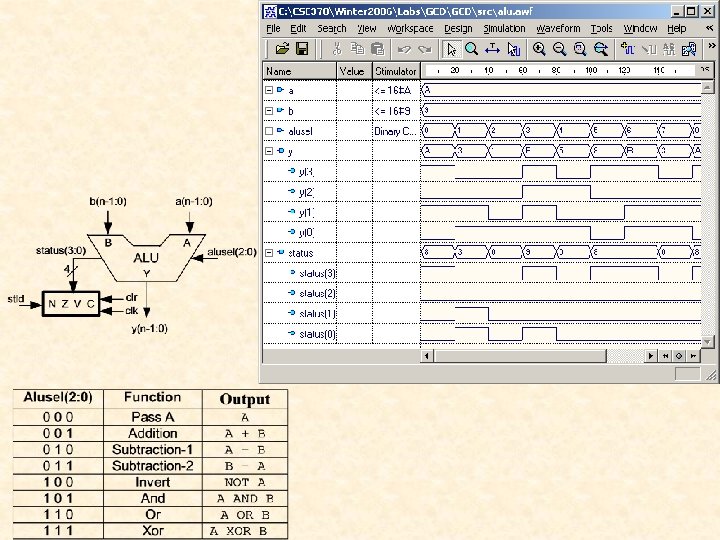
- Slides: 10
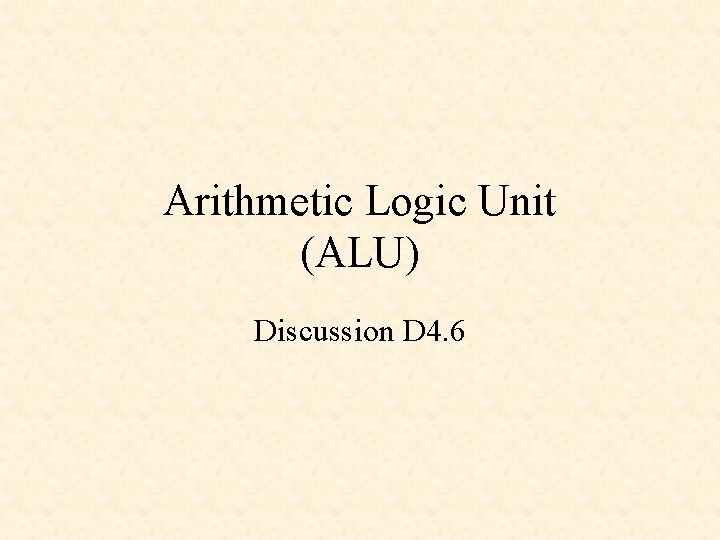
Arithmetic Logic Unit (ALU) Discussion D 4. 6
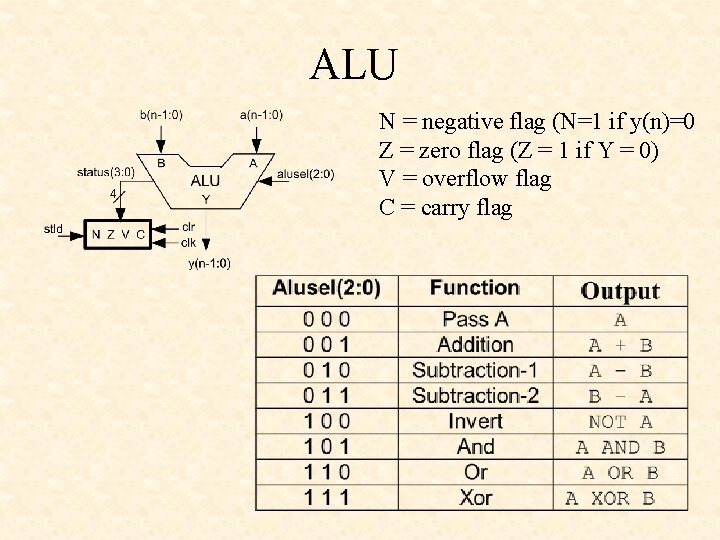
ALU N = negative flag (N=1 if y(n)=0 Z = zero flag (Z = 1 if Y = 0) V = overflow flag C = carry flag
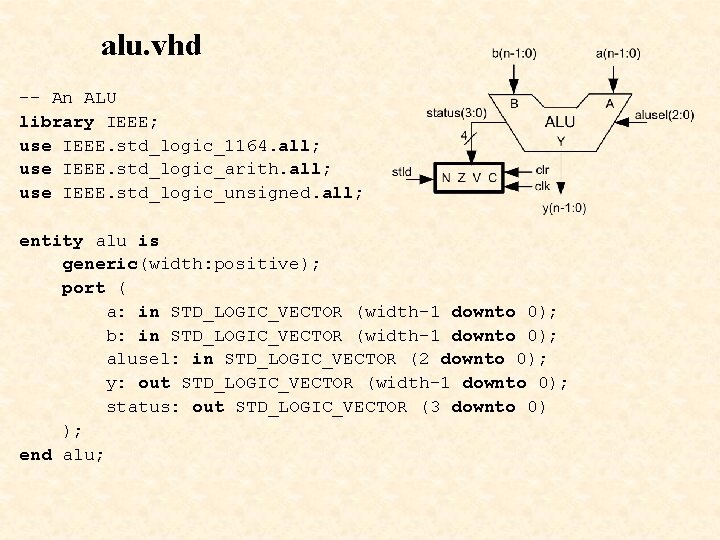
alu. vhd -- An ALU library IEEE; use IEEE. std_logic_1164. all; use IEEE. std_logic_arith. all; use IEEE. std_logic_unsigned. all; entity alu is generic(width: positive); port ( a: in STD_LOGIC_VECTOR (width-1 downto 0); b: in STD_LOGIC_VECTOR (width-1 downto 0); alusel: in STD_LOGIC_VECTOR (2 downto 0); y: out STD_LOGIC_VECTOR (width-1 downto 0); status: out STD_LOGIC_VECTOR (3 downto 0) ); end alu;
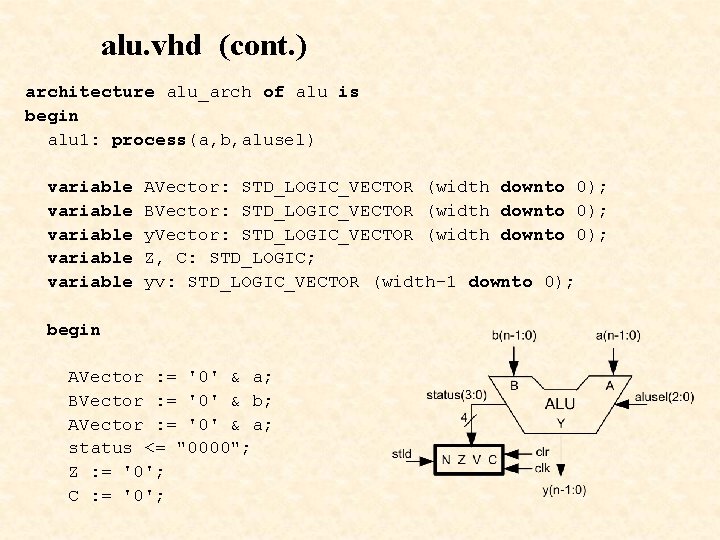
alu. vhd (cont. ) architecture alu_arch of alu is begin alu 1: process(a, b, alusel) variable variable AVector: STD_LOGIC_VECTOR (width downto 0); BVector: STD_LOGIC_VECTOR (width downto 0); y. Vector: STD_LOGIC_VECTOR (width downto 0); Z, C: STD_LOGIC; yv: STD_LOGIC_VECTOR (width-1 downto 0); begin AVector : = '0' & a; BVector : = '0' & b; AVector : = '0' & a; status <= "0000"; Z : = '0'; C : = '0';
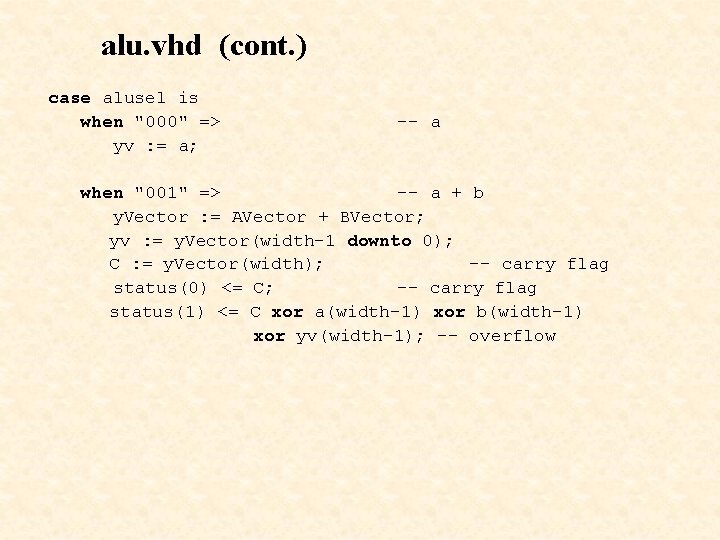
alu. vhd (cont. ) case alusel is when "000" => yv : = a; -- a when "001" => -- a + b y. Vector : = AVector + BVector; yv : = y. Vector(width-1 downto 0); C : = y. Vector(width); -- carry flag status(0) <= C; -- carry flag status(1) <= C xor a(width-1) xor b(width-1) xor yv(width-1); -- overflow
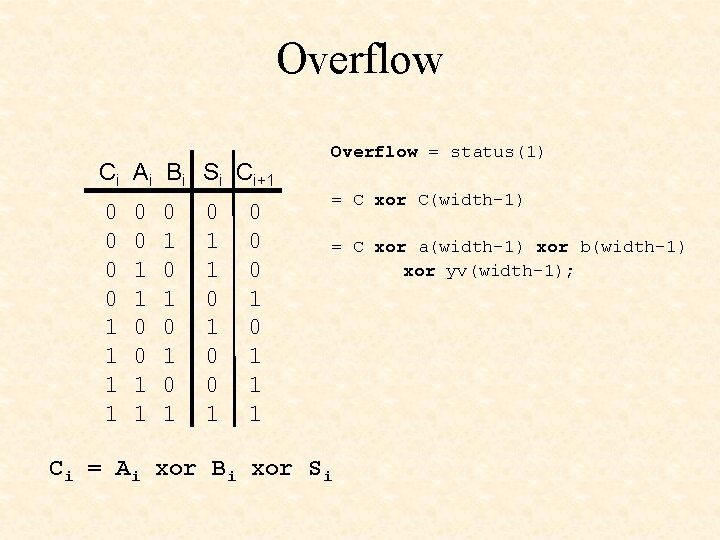
Overflow Ci Ai Bi Si Ci+1 0 0 1 1 0 1 0 1 0 1 1 0 0 0 1 1 1 Overflow = status(1) = C xor C(width-1) = C xor a(width-1) xor b(width-1) xor yv(width-1); Ci = Ai xor Bi xor Si
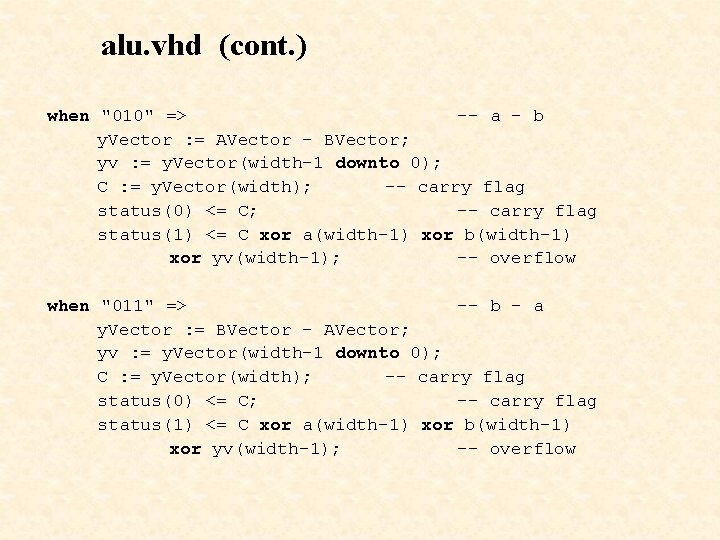
alu. vhd (cont. ) when "010" => -- a - b y. Vector : = AVector - BVector; yv : = y. Vector(width-1 downto 0); C : = y. Vector(width); -- carry flag status(0) <= C; -- carry flag status(1) <= C xor a(width-1) xor b(width-1) xor yv(width-1); -- overflow when "011" => -- b - a y. Vector : = BVector - AVector; yv : = y. Vector(width-1 downto 0); C : = y. Vector(width); -- carry flag status(0) <= C; -- carry flag status(1) <= C xor a(width-1) xor b(width-1) xor yv(width-1); -- overflow
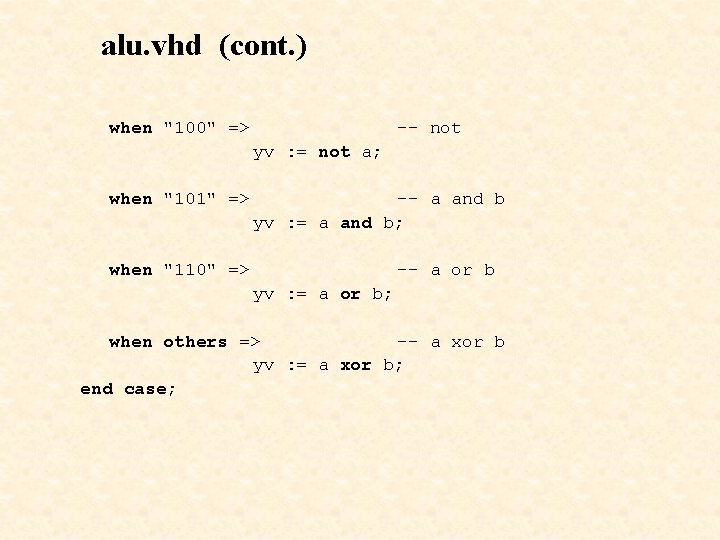
alu. vhd (cont. ) when "100" => -- not yv : = not a; when "101" => -- a and b yv : = a and b; when "110" => -- a or b yv : = a or b; when others => -- a xor b yv : = a xor b; end case;
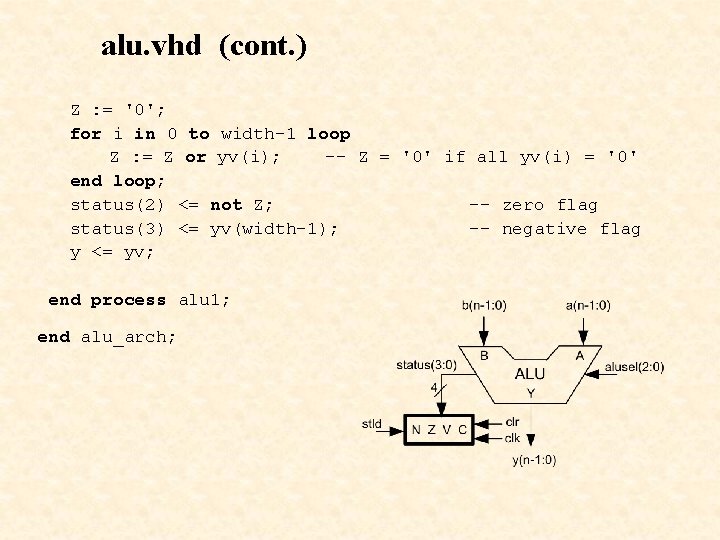
alu. vhd (cont. ) Z : = '0'; for i in 0 to width-1 loop Z : = Z or yv(i); -- Z = '0' if all yv(i) = '0' end loop; status(2) <= not Z; -- zero flag status(3) <= yv(width-1); -- negative flag y <= yv; end process alu 1; end alu_arch;
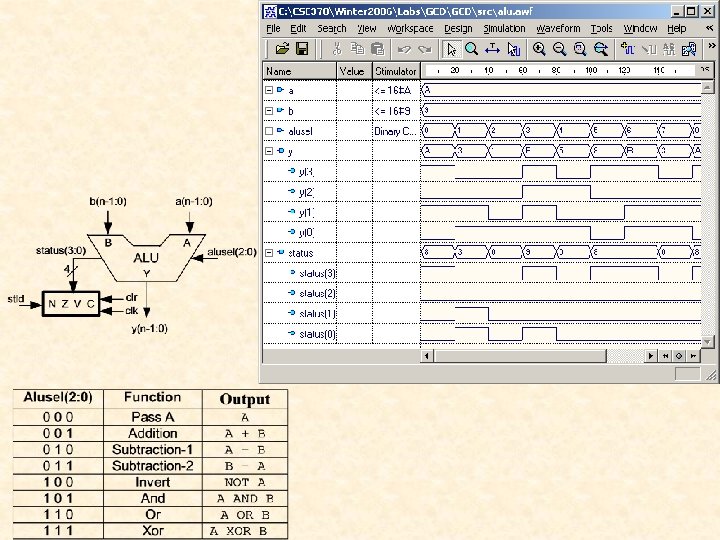