Arithmetic and Logical Instructions Multiplication and Division Multiplication
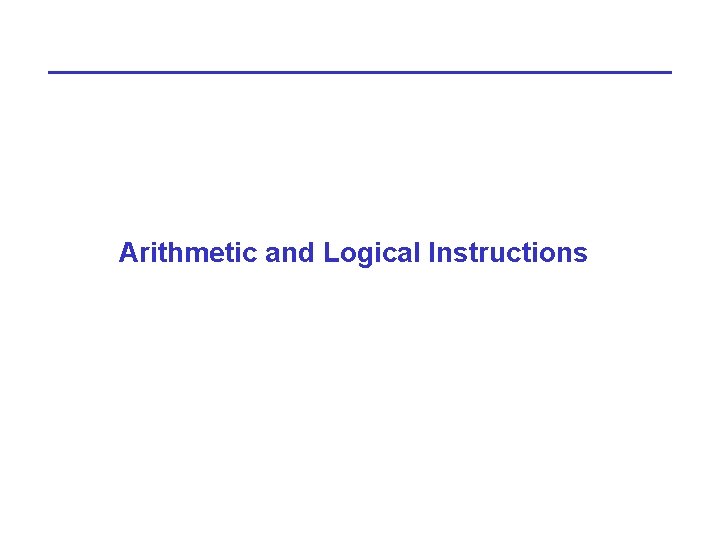
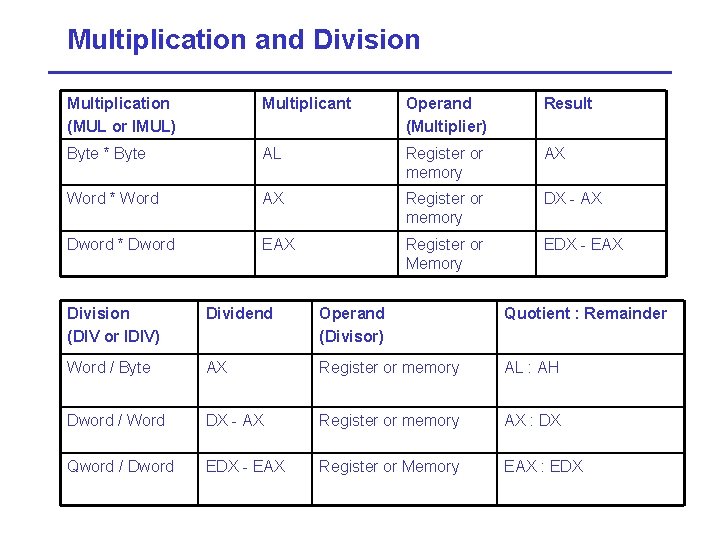
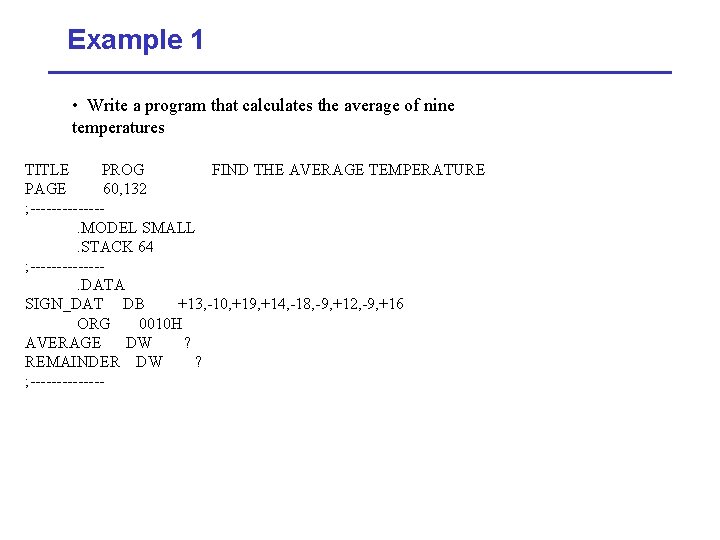
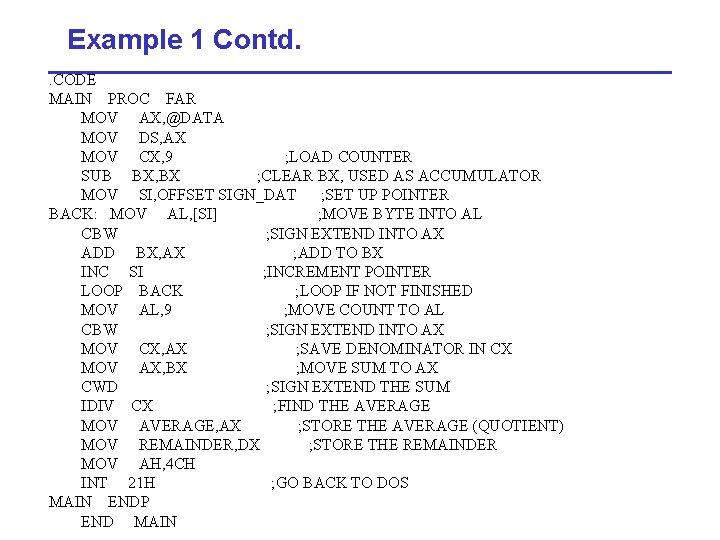
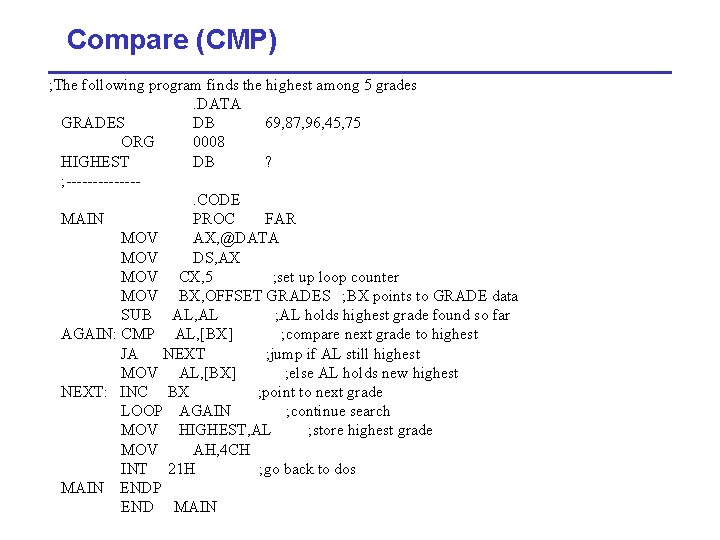
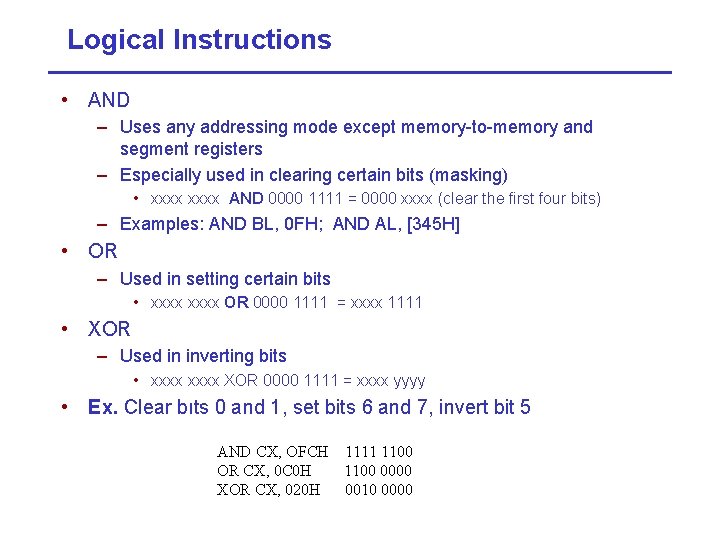
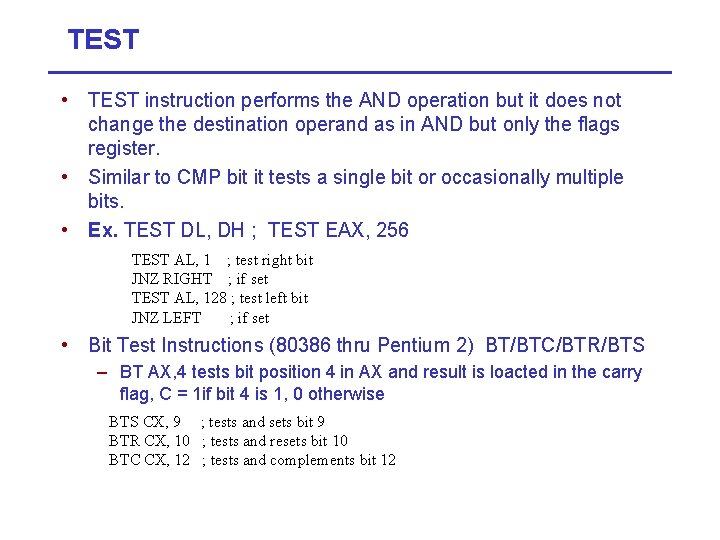
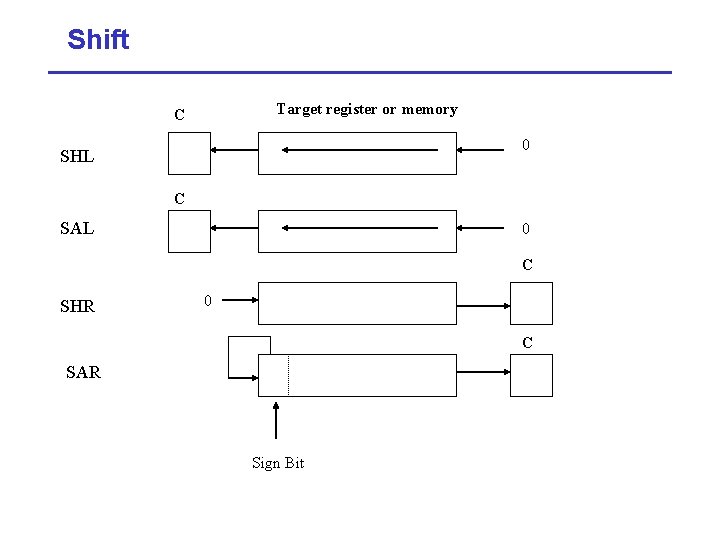
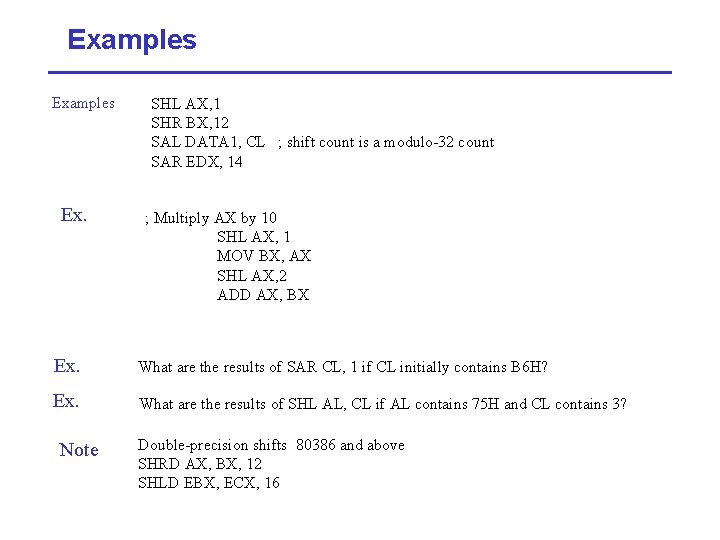
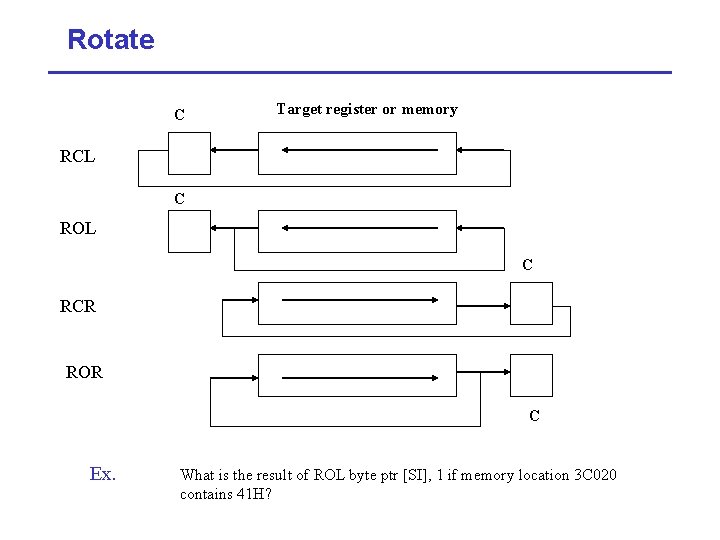
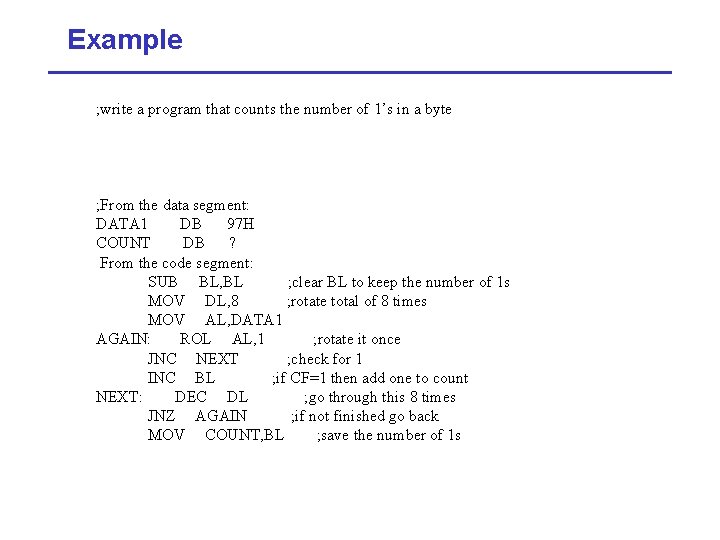
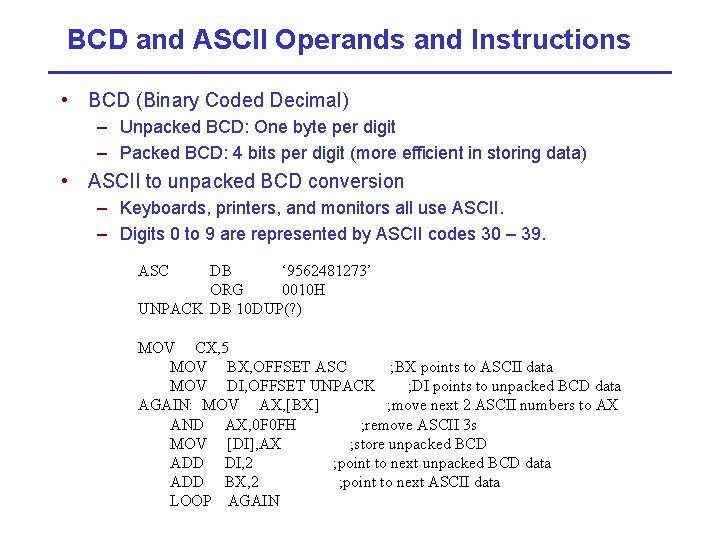
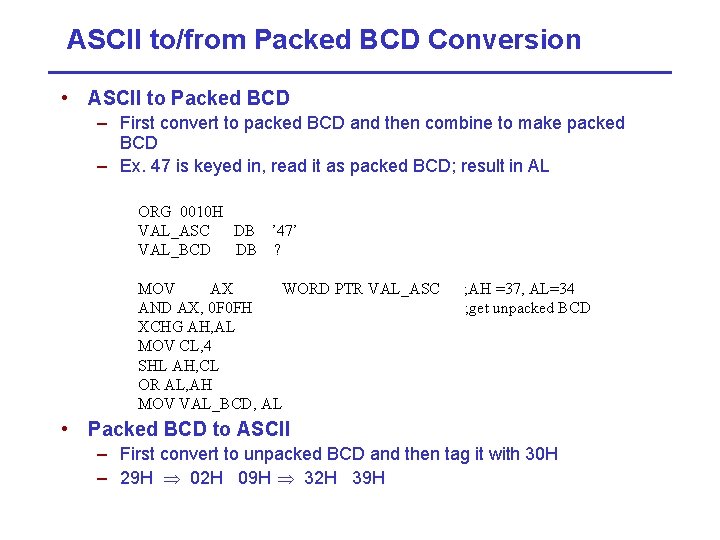
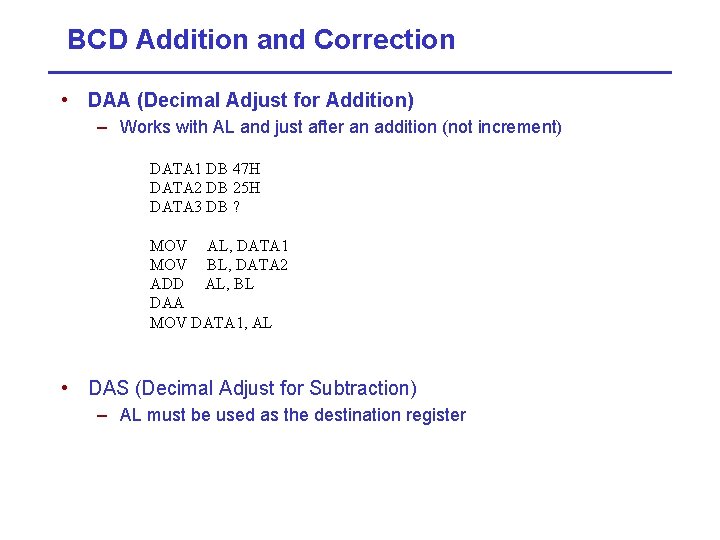
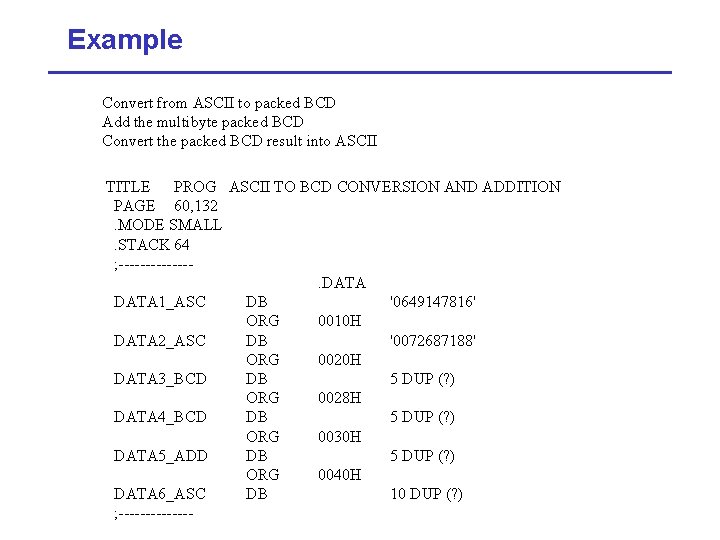
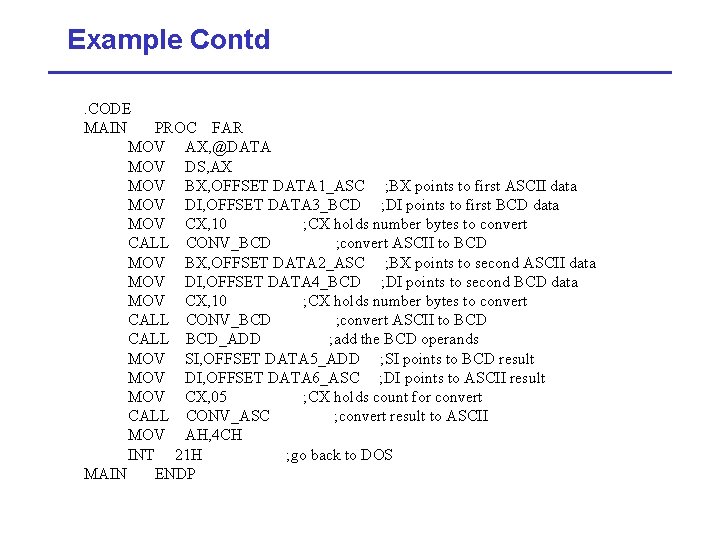
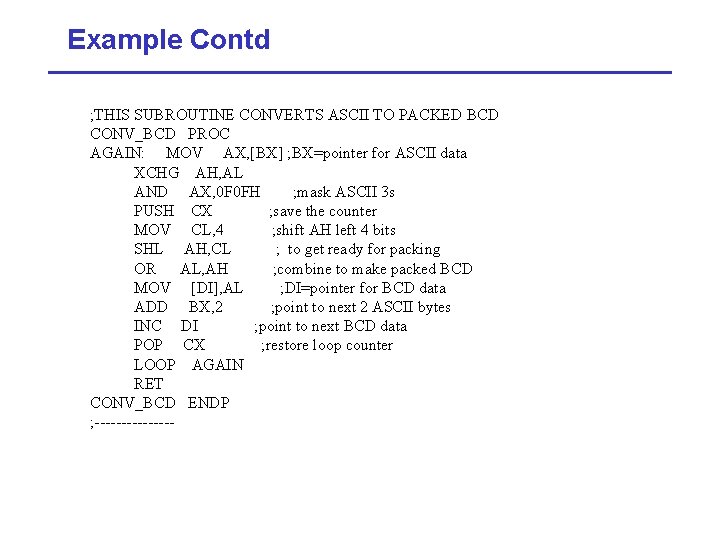
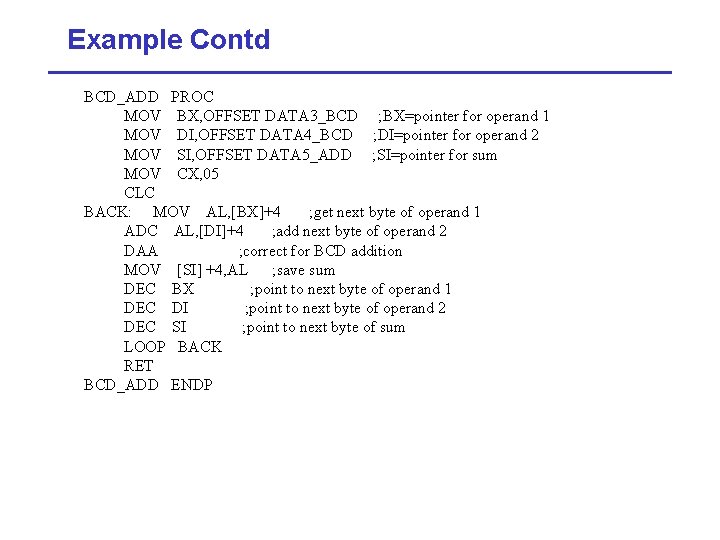
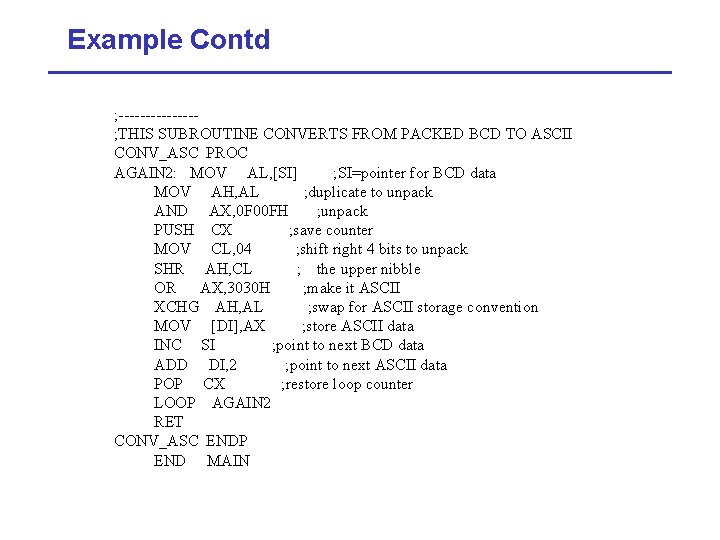
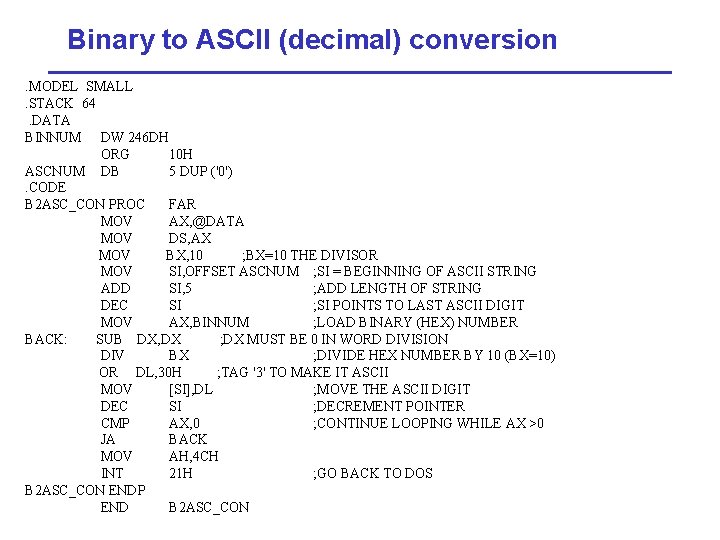
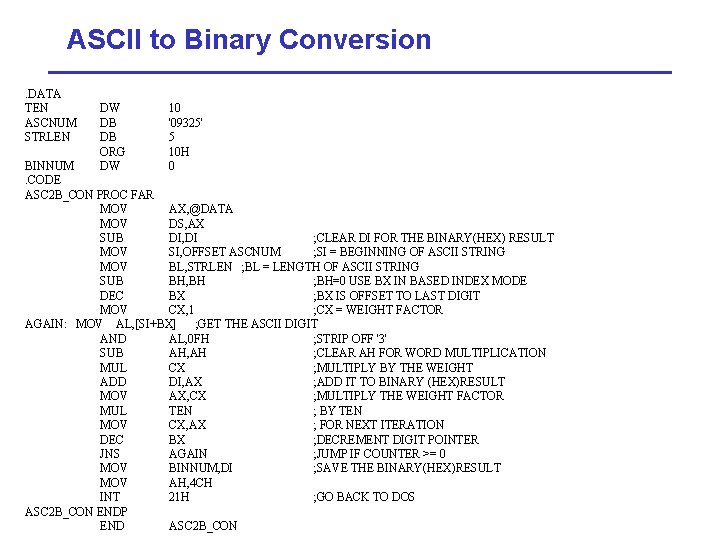
- Slides: 21
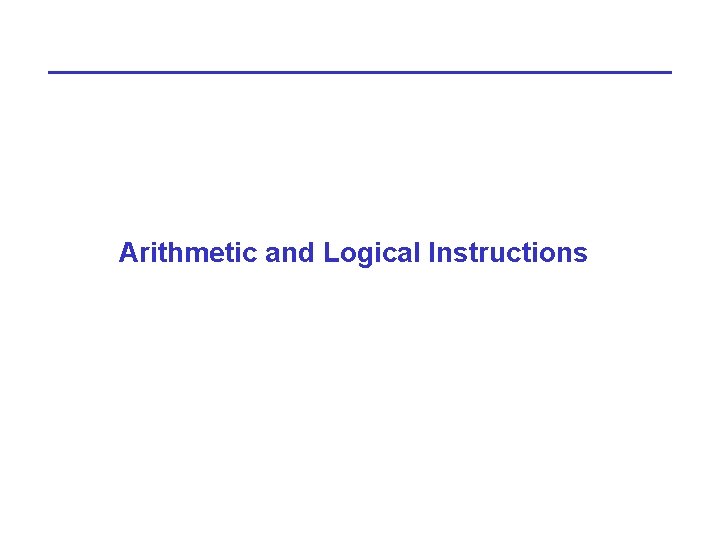
Arithmetic and Logical Instructions
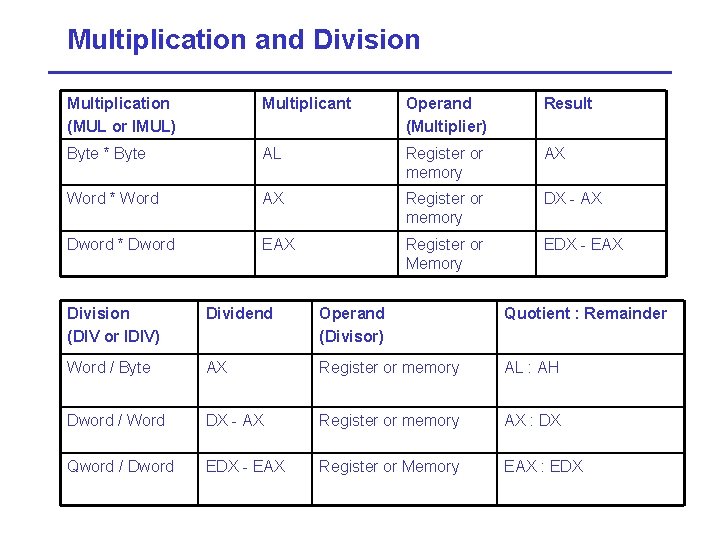
Multiplication and Division Multiplication (MUL or IMUL) Multiplicant Operand (Multiplier) Result Byte * Byte AL Register or memory AX Word * Word AX Register or memory DX - AX Dword * Dword EAX Register or Memory EDX - EAX Division (DIV or IDIV) Dividend Operand (Divisor) Quotient : Remainder Word / Byte AX Register or memory AL : AH Dword / Word DX - AX Register or memory AX : DX Qword / Dword EDX - EAX Register or Memory EAX : EDX
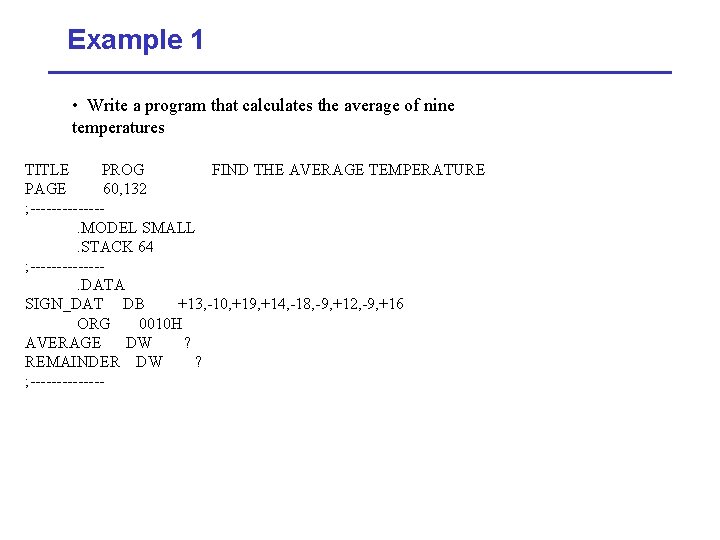
Example 1 • Write a program that calculates the average of nine temperatures TITLE PROG FIND THE AVERAGE TEMPERATURE PAGE 60, 132 ; -------. MODEL SMALL. STACK 64 ; -------. DATA SIGN_DAT DB +13, -10, +19, +14, -18, -9, +12, -9, +16 ORG 0010 H AVERAGE DW ? REMAINDER DW ? ; -------
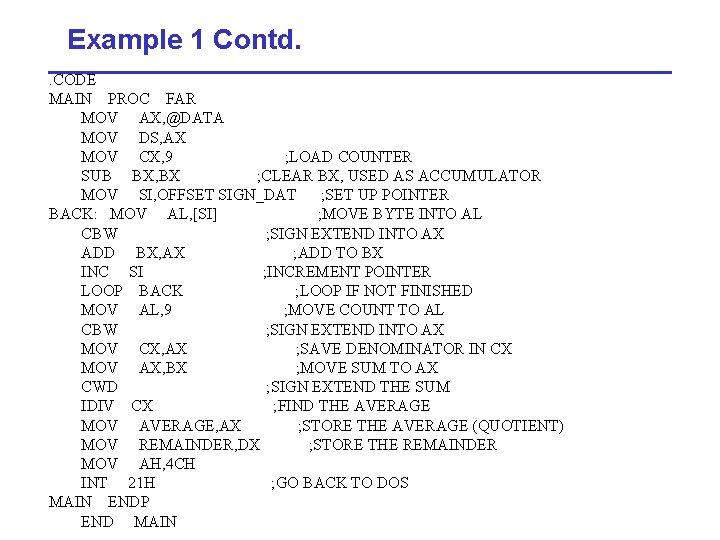
Example 1 Contd. . CODE MAIN PROC FAR MOV AX, @DATA MOV DS, AX MOV CX, 9 ; LOAD COUNTER SUB BX, BX ; CLEAR BX, USED AS ACCUMULATOR MOV SI, OFFSET SIGN_DAT ; SET UP POINTER BACK: MOV AL, [SI] ; MOVE BYTE INTO AL CBW ; SIGN EXTEND INTO AX ADD BX, AX ; ADD TO BX INC SI ; INCREMENT POINTER LOOP BACK ; LOOP IF NOT FINISHED MOV AL, 9 ; MOVE COUNT TO AL CBW ; SIGN EXTEND INTO AX MOV CX, AX ; SAVE DENOMINATOR IN CX MOV AX, BX ; MOVE SUM TO AX CWD ; SIGN EXTEND THE SUM IDIV CX ; FIND THE AVERAGE MOV AVERAGE, AX ; STORE THE AVERAGE (QUOTIENT) MOV REMAINDER, DX ; STORE THE REMAINDER MOV AH, 4 CH INT 21 H ; GO BACK TO DOS MAIN ENDP END MAIN
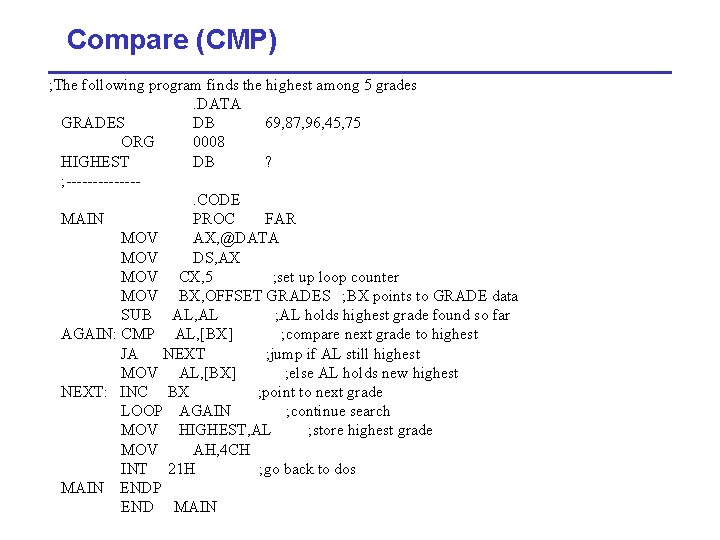
Compare (CMP) ; The following program finds the highest among 5 grades. DATA GRADES DB 69, 87, 96, 45, 75 ORG 0008 HIGHEST DB ? ; -------. CODE MAIN PROC FAR MOV AX, @DATA MOV DS, AX MOV CX, 5 ; set up loop counter MOV BX, OFFSET GRADES ; BX points to GRADE data SUB AL, AL ; AL holds highest grade found so far AGAIN: CMP AL, [BX] ; compare next grade to highest JA NEXT ; jump if AL still highest MOV AL, [BX] ; else AL holds new highest NEXT: INC BX ; point to next grade LOOP AGAIN ; continue search MOV HIGHEST, AL ; store highest grade MOV AH, 4 CH INT 21 H ; go back to dos MAIN ENDP END MAIN
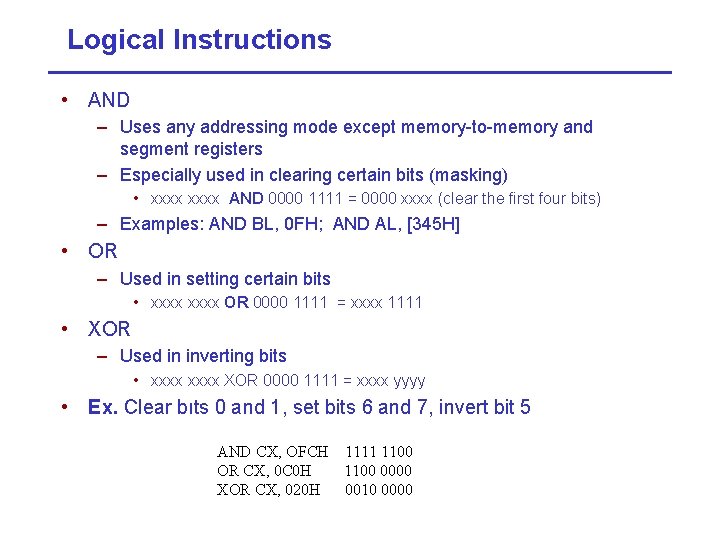
Logical Instructions • AND – Uses any addressing mode except memory-to-memory and segment registers – Especially used in clearing certain bits (masking) • xxxx AND 0000 1111 = 0000 xxxx (clear the first four bits) – Examples: AND BL, 0 FH; AND AL, [345 H] • OR – Used in setting certain bits • xxxx OR 0000 1111 = xxxx 1111 • XOR – Used in inverting bits • xxxx XOR 0000 1111 = xxxx yyyy • Ex. Clear bıts 0 and 1, set bits 6 and 7, invert bit 5 AND CX, OFCH 1111 1100 OR CX, 0 C 0 H 1100 0000 XOR CX, 020 H 0010 0000
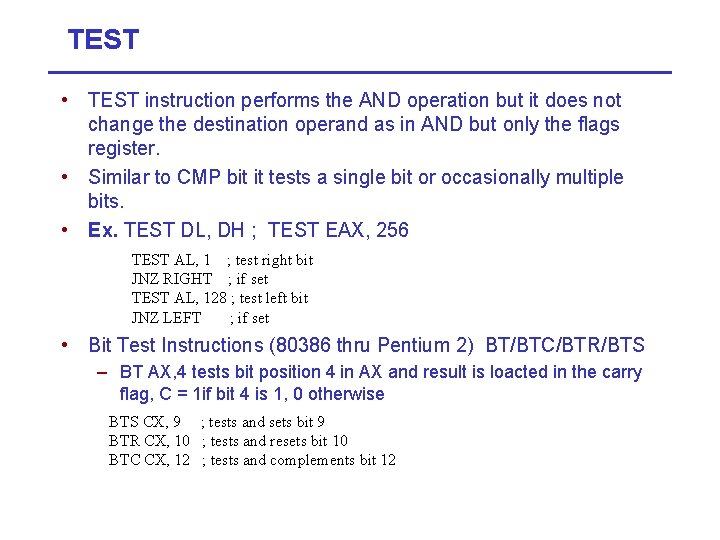
TEST • TEST instruction performs the AND operation but it does not change the destination operand as in AND but only the flags register. • Similar to CMP bit it tests a single bit or occasionally multiple bits. • Ex. TEST DL, DH ; TEST EAX, 256 TEST AL, 1 ; test right bit JNZ RIGHT ; if set TEST AL, 128 ; test left bit JNZ LEFT ; if set • Bit Test Instructions (80386 thru Pentium 2) BT/BTC/BTR/BTS – BT AX, 4 tests bit position 4 in AX and result is loacted in the carry flag, C = 1 if bit 4 is 1, 0 otherwise BTS CX, 9 ; tests and sets bit 9 BTR CX, 10 ; tests and resets bit 10 BTC CX, 12 ; tests and complements bit 12
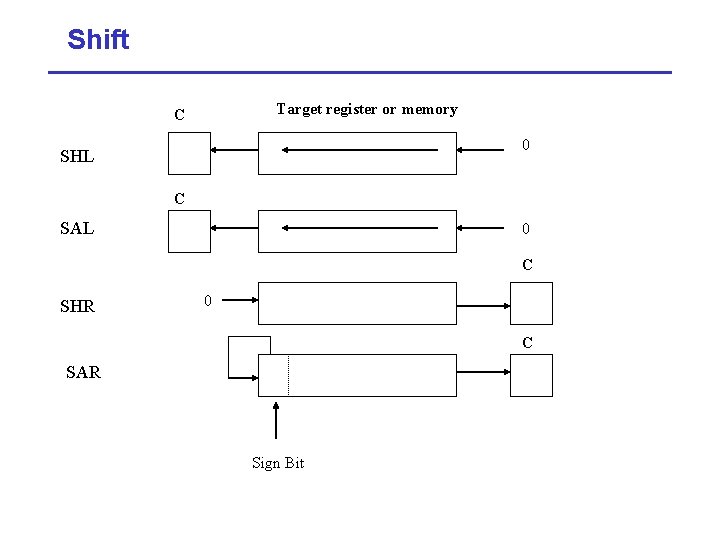
Shift Target register or memory C 0 SHL C SAL 0 C SHR 0 C SAR Sign Bit
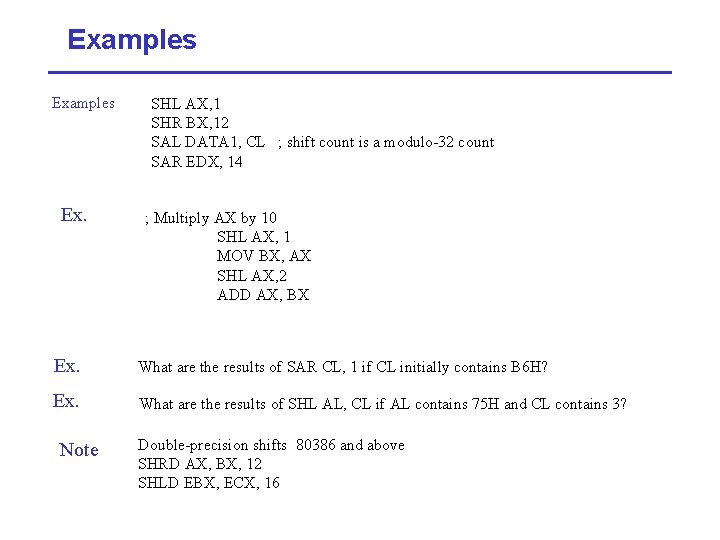
Examples Ex. SHL AX, 1 SHR BX, 12 SAL DATA 1, CL ; shift count is a modulo-32 count SAR EDX, 14 ; Multiply AX by 10 SHL AX, 1 MOV BX, AX SHL AX, 2 ADD AX, BX Ex. What are the results of SAR CL, 1 if CL initially contains B 6 H? Ex. What are the results of SHL AL, CL if AL contains 75 H and CL contains 3? Note Double-precision shifts 80386 and above SHRD AX, BX, 12 SHLD EBX, ECX, 16
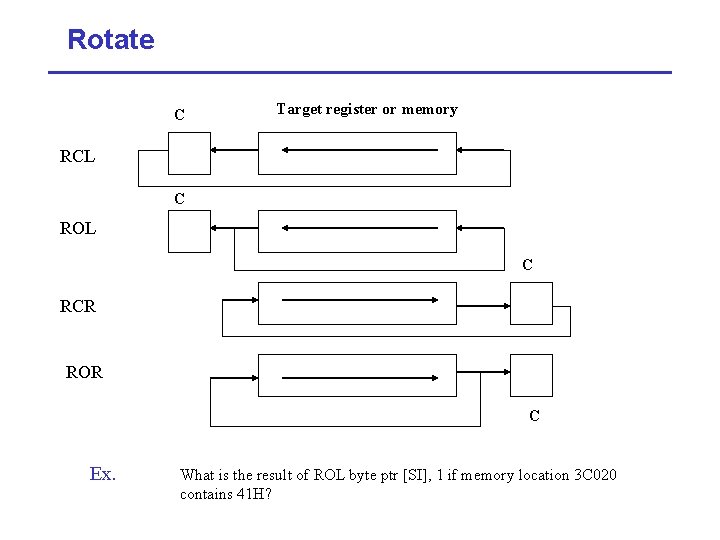
Rotate C Target register or memory RCL C ROL C RCR ROR C Ex. What is the result of ROL byte ptr [SI], 1 if memory location 3 C 020 contains 41 H?
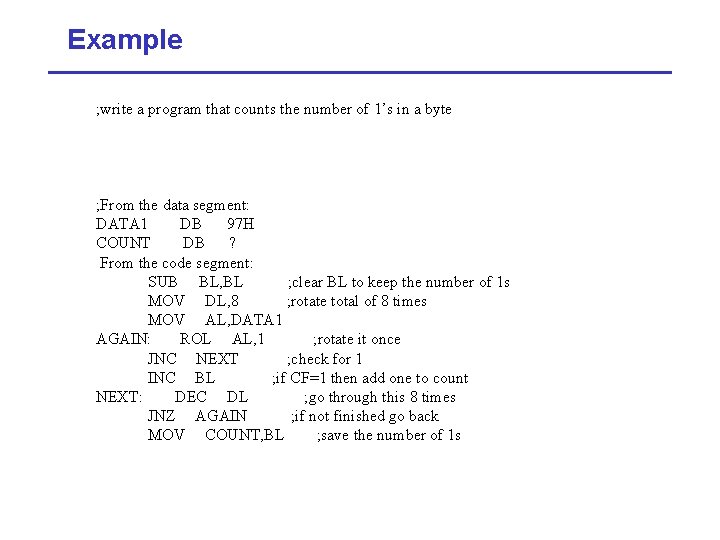
Example ; write a program that counts the number of 1’s in a byte ; From the data segment: DATA 1 DB 97 H COUNT DB ? From the code segment: SUB BL, BL ; clear BL to keep the number of 1 s MOV DL, 8 ; rotate total of 8 times MOV AL, DATA 1 AGAIN: ROL AL, 1 ; rotate it once JNC NEXT ; check for 1 INC BL ; if CF=1 then add one to count NEXT: DEC DL ; go through this 8 times JNZ AGAIN ; if not finished go back MOV COUNT, BL ; save the number of 1 s
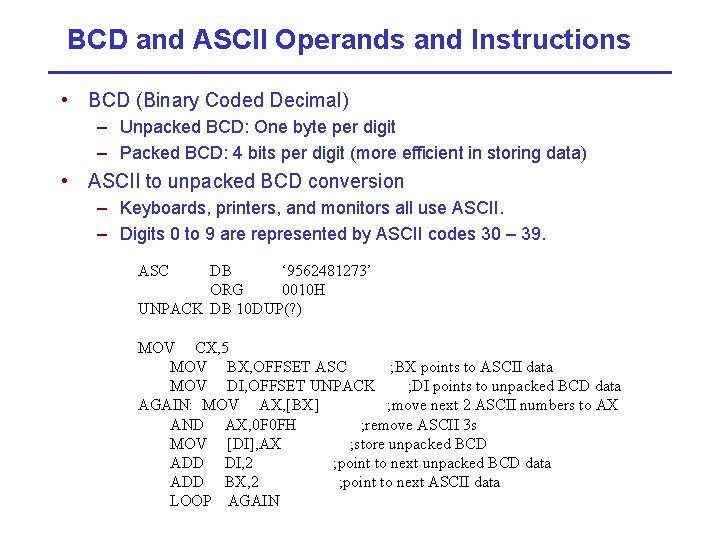
BCD and ASCII Operands and Instructions • BCD (Binary Coded Decimal) – Unpacked BCD: One byte per digit – Packed BCD: 4 bits per digit (more efficient in storing data) • ASCII to unpacked BCD conversion – Keyboards, printers, and monitors all use ASCII. – Digits 0 to 9 are represented by ASCII codes 30 – 39. ASC DB ‘ 9562481273’ ORG 0010 H UNPACK DB 10 DUP(? ) MOV CX, 5 MOV BX, OFFSET ASC ; BX points to ASCII data MOV DI, OFFSET UNPACK ; DI points to unpacked BCD data AGAIN: MOV AX, [BX] ; move next 2 ASCII numbers to AX AND AX, 0 F 0 FH ; remove ASCII 3 s MOV [DI], AX ; store unpacked BCD ADD DI, 2 ; point to next unpacked BCD data ADD BX, 2 ; point to next ASCII data LOOP AGAIN
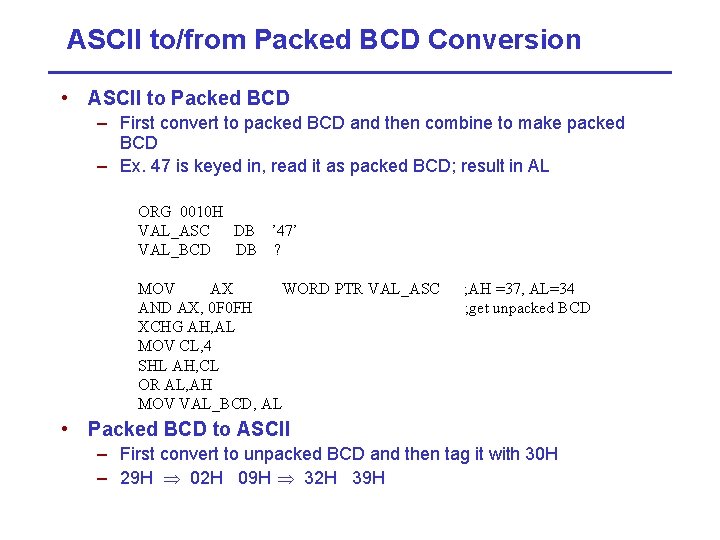
ASCII to/from Packed BCD Conversion • ASCII to Packed BCD – First convert to packed BCD and then combine to make packed BCD – Ex. 47 is keyed in, read it as packed BCD; result in AL ORG 0010 H VAL_ASC DB ’ 47’ VAL_BCD DB ? MOV AX WORD PTR VAL_ASC AND AX, 0 F 0 FH XCHG AH, AL MOV CL, 4 SHL AH, CL OR AL, AH MOV VAL_BCD, AL ; AH =37, AL=34 ; get unpacked BCD • Packed BCD to ASCII – First convert to unpacked BCD and then tag it with 30 H – 29 H 02 H 09 H 32 H 39 H
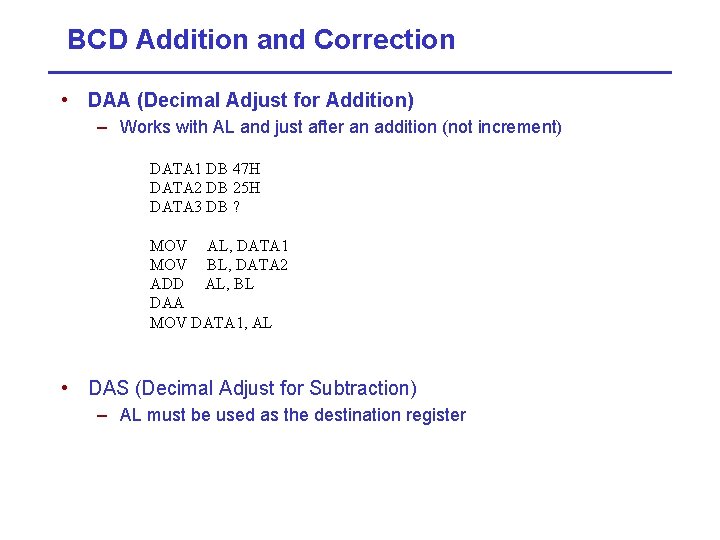
BCD Addition and Correction • DAA (Decimal Adjust for Addition) – Works with AL and just after an addition (not increment) DATA 1 DB 47 H DATA 2 DB 25 H DATA 3 DB ? MOV AL, DATA 1 MOV BL, DATA 2 ADD AL, BL DAA MOV DATA 1, AL • DAS (Decimal Adjust for Subtraction) – AL must be used as the destination register
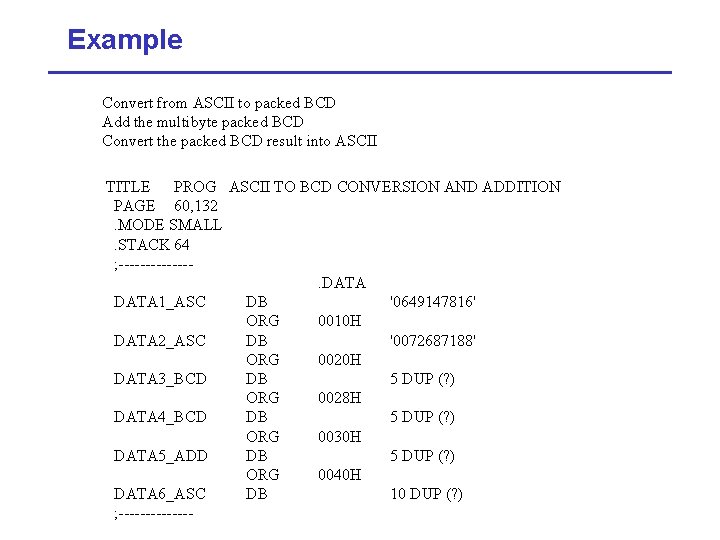
Example Convert from ASCII to packed BCD Add the multibyte packed BCD Convert the packed BCD result into ASCII TITLE PROG ASCII TO BCD CONVERSION AND ADDITION PAGE 60, 132. MODE SMALL. STACK 64 ; -------. DATA 1_ASC DB '0649147816' ORG 0010 H DATA 2_ASC DB '0072687188' ORG 0020 H DATA 3_BCD DB 5 DUP (? ) ORG 0028 H DATA 4_BCD DB 5 DUP (? ) ORG 0030 H DATA 5_ADD DB 5 DUP (? ) ORG 0040 H DATA 6_ASC DB 10 DUP (? ) ; -------
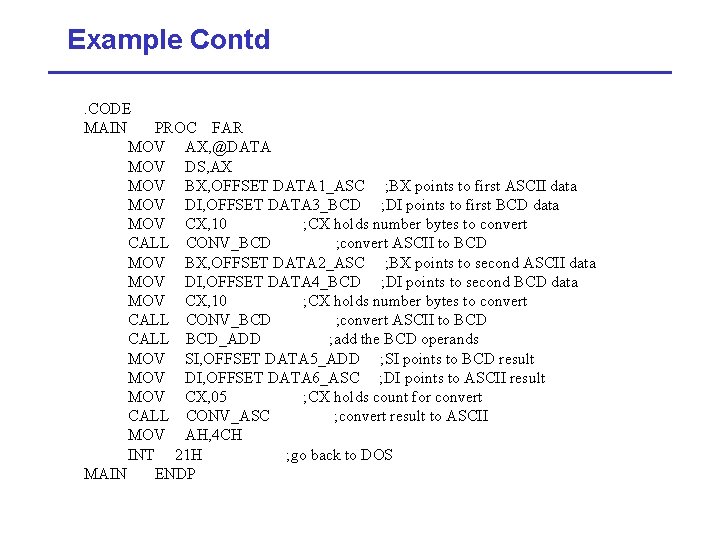
Example Contd. CODE MAIN PROC FAR MOV AX, @DATA MOV DS, AX MOV BX, OFFSET DATA 1_ASC ; BX points to first ASCII data MOV DI, OFFSET DATA 3_BCD ; DI points to first BCD data MOV CX, 10 ; CX holds number bytes to convert CALL CONV_BCD ; convert ASCII to BCD MOV BX, OFFSET DATA 2_ASC ; BX points to second ASCII data MOV DI, OFFSET DATA 4_BCD ; DI points to second BCD data MOV CX, 10 ; CX holds number bytes to convert CALL CONV_BCD ; convert ASCII to BCD CALL BCD_ADD ; add the BCD operands MOV SI, OFFSET DATA 5_ADD ; SI points to BCD result MOV DI, OFFSET DATA 6_ASC ; DI points to ASCII result MOV CX, 05 ; CX holds count for convert CALL CONV_ASC ; convert result to ASCII MOV AH, 4 CH INT 21 H ; go back to DOS MAIN ENDP
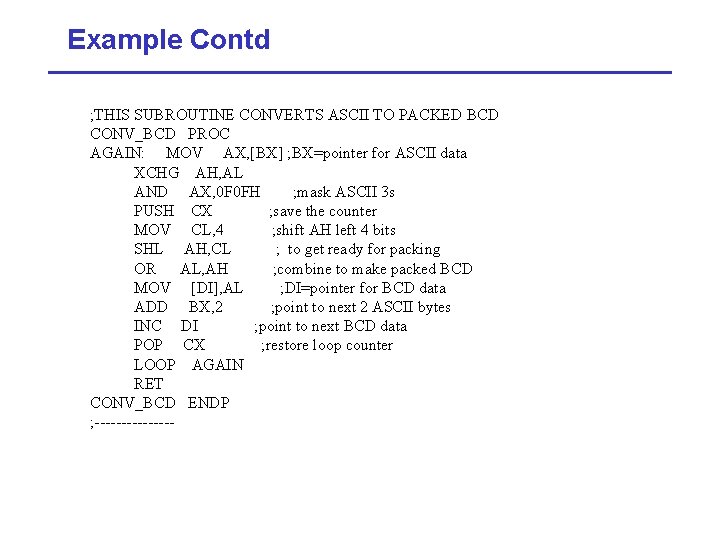
Example Contd ; THIS SUBROUTINE CONVERTS ASCII TO PACKED BCD CONV_BCD PROC AGAIN: MOV AX, [BX] ; BX=pointer for ASCII data XCHG AH, AL AND AX, 0 F 0 FH ; mask ASCII 3 s PUSH CX ; save the counter MOV CL, 4 ; shift AH left 4 bits SHL AH, CL ; to get ready for packing OR AL, AH ; combine to make packed BCD MOV [DI], AL ; DI=pointer for BCD data ADD BX, 2 ; point to next 2 ASCII bytes INC DI ; point to next BCD data POP CX ; restore loop counter LOOP AGAIN RET CONV_BCD ENDP ; --------
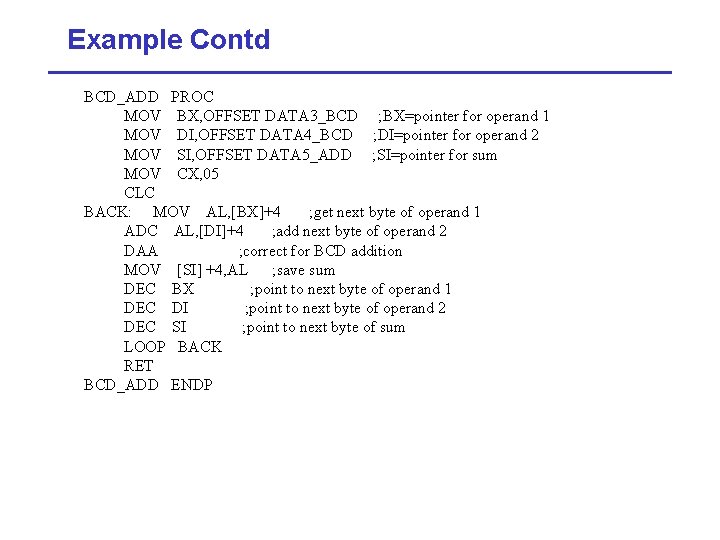
Example Contd BCD_ADD PROC MOV BX, OFFSET DATA 3_BCD ; BX=pointer for operand 1 MOV DI, OFFSET DATA 4_BCD ; DI=pointer for operand 2 MOV SI, OFFSET DATA 5_ADD ; SI=pointer for sum MOV CX, 05 CLC BACK: MOV AL, [BX]+4 ; get next byte of operand 1 ADC AL, [DI]+4 ; add next byte of operand 2 DAA ; correct for BCD addition MOV [SI] +4, AL ; save sum DEC BX ; point to next byte of operand 1 DEC DI ; point to next byte of operand 2 DEC SI ; point to next byte of sum LOOP BACK RET BCD_ADD ENDP
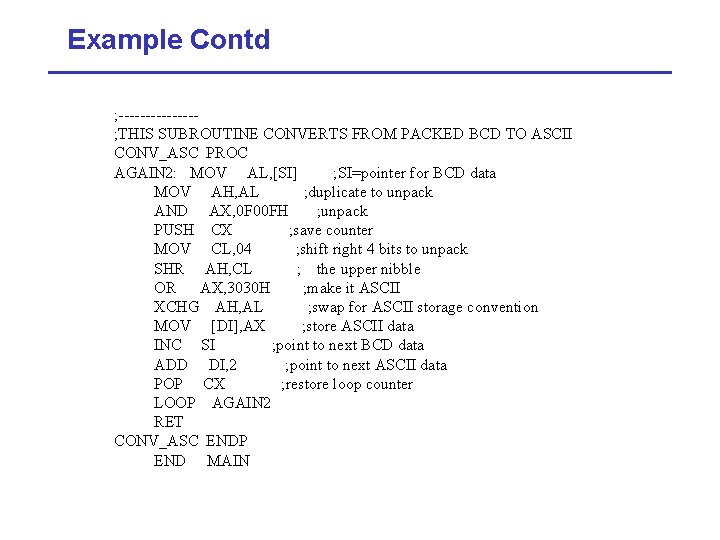
Example Contd ; -------; THIS SUBROUTINE CONVERTS FROM PACKED BCD TO ASCII CONV_ASC PROC AGAIN 2: MOV AL, [SI] ; SI=pointer for BCD data MOV AH, AL ; duplicate to unpack AND AX, 0 F 00 FH ; unpack PUSH CX ; save counter MOV CL, 04 ; shift right 4 bits to unpack SHR AH, CL ; the upper nibble OR AX, 3030 H ; make it ASCII XCHG AH, AL ; swap for ASCII storage convention MOV [DI], AX ; store ASCII data INC SI ; point to next BCD data ADD DI, 2 ; point to next ASCII data POP CX ; restore loop counter LOOP AGAIN 2 RET CONV_ASC ENDP END MAIN
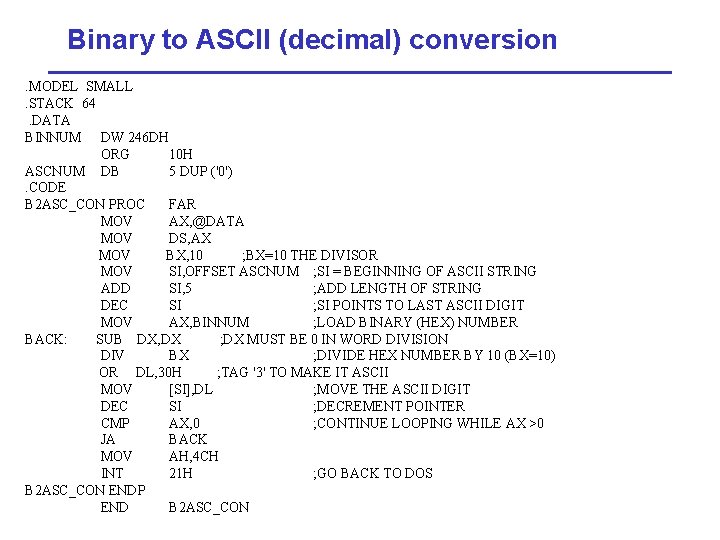
Binary to ASCII (decimal) conversion. MODEL SMALL. STACK 64. DATA BINNUM DW 246 DH ORG 10 H ASCNUM DB 5 DUP ('0'). CODE B 2 ASC_CON PROC FAR MOV AX, @DATA MOV DS, AX MOV BX, 10 ; BX=10 THE DIVISOR MOV SI, OFFSET ASCNUM ; SI = BEGINNING OF ASCII STRING ADD SI, 5 ; ADD LENGTH OF STRING DEC SI ; SI POINTS TO LAST ASCII DIGIT MOV AX, BINNUM ; LOAD BINARY (HEX) NUMBER BACK: SUB DX, DX ; DX MUST BE 0 IN WORD DIVISION DIV BX ; DIVIDE HEX NUMBER BY 10 (BX=10) OR DL, 30 H ; TAG '3' TO MAKE IT ASCII MOV [SI], DL ; MOVE THE ASCII DIGIT DEC SI ; DECREMENT POINTER CMP AX, 0 ; CONTINUE LOOPING WHILE AX >0 JA BACK MOV AH, 4 CH INT 21 H ; GO BACK TO DOS B 2 ASC_CON ENDP END B 2 ASC_CON
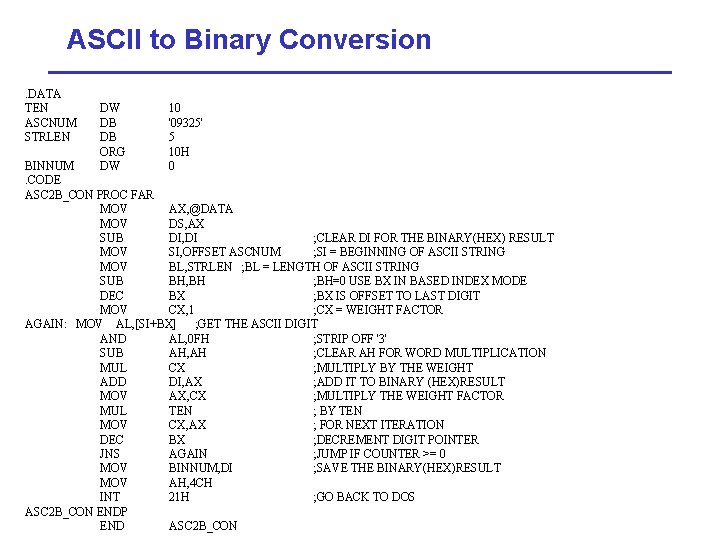
ASCII to Binary Conversion. DATA TEN ASCNUM STRLEN DW DB DB ORG DW 10 '09325' 5 10 H 0 BINNUM. CODE ASC 2 B_CON PROC FAR MOV AX, @DATA MOV DS, AX SUB DI, DI ; CLEAR DI FOR THE BINARY(HEX) RESULT MOV SI, OFFSET ASCNUM ; SI = BEGINNING OF ASCII STRING MOV BL, STRLEN ; BL = LENGTH OF ASCII STRING SUB BH, BH ; BH=0 USE BX IN BASED INDEX MODE DEC BX ; BX IS OFFSET TO LAST DIGIT MOV CX, 1 ; CX = WEIGHT FACTOR AGAIN: MOV AL, [SI+BX] ; GET THE ASCII DIGIT AND AL, 0 FH ; STRIP OFF '3' SUB AH, AH ; CLEAR AH FOR WORD MULTIPLICATION MUL CX ; MULTIPLY BY THE WEIGHT ADD DI, AX ; ADD IT TO BINARY (HEX)RESULT MOV AX, CX ; MULTIPLY THE WEIGHT FACTOR MUL TEN ; BY TEN MOV CX, AX ; FOR NEXT ITERATION DEC BX ; DECREMENT DIGIT POINTER JNS AGAIN ; JUMP IF COUNTER >= 0 MOV BINNUM, DI ; SAVE THE BINARY(HEX)RESULT MOV AH, 4 CH INT 21 H ; GO BACK TO DOS ASC 2 B_CON ENDP END ASC 2 B_CON