APS 105 Functions and Pointers 1 Modularity Modularity
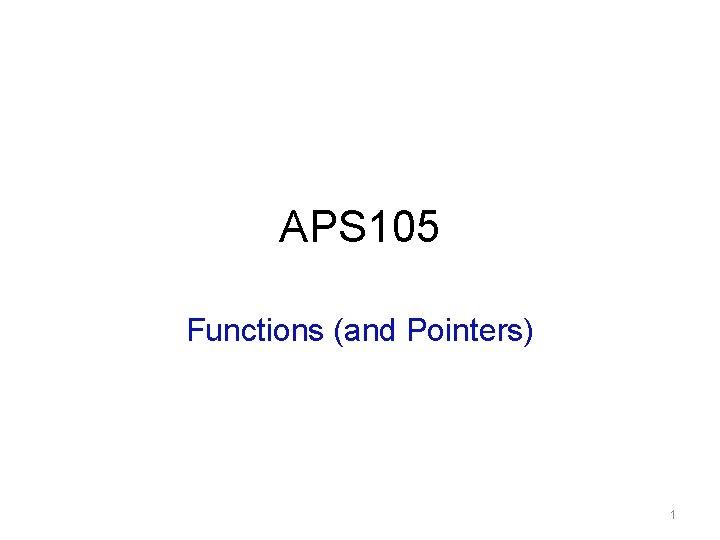
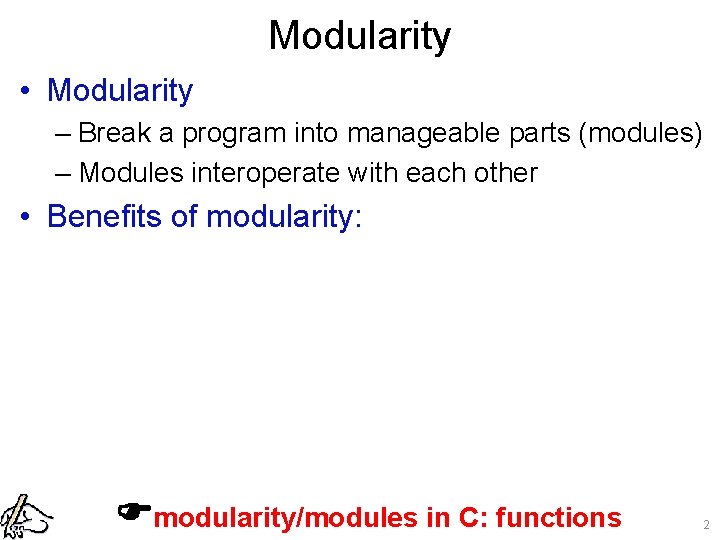
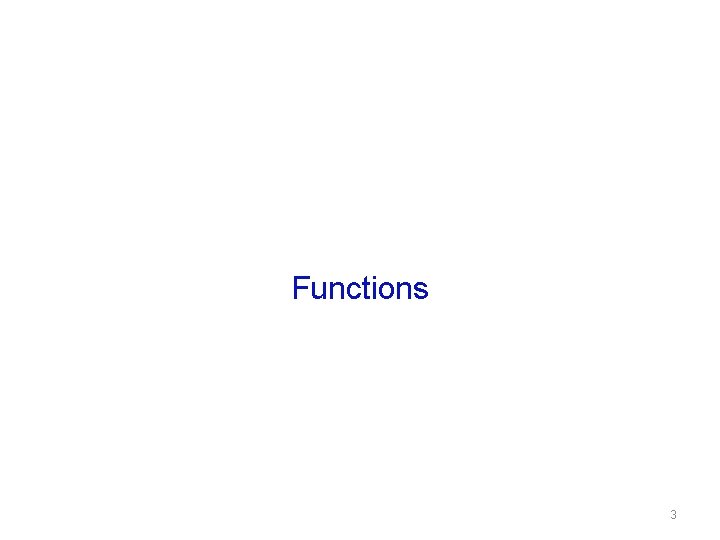
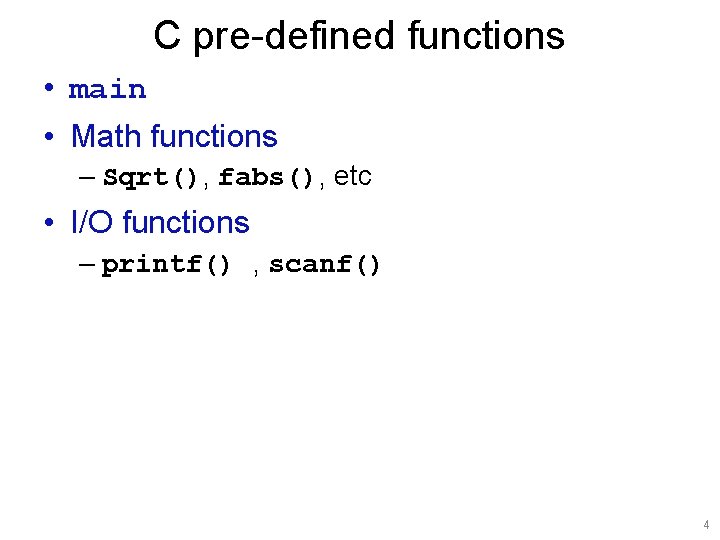
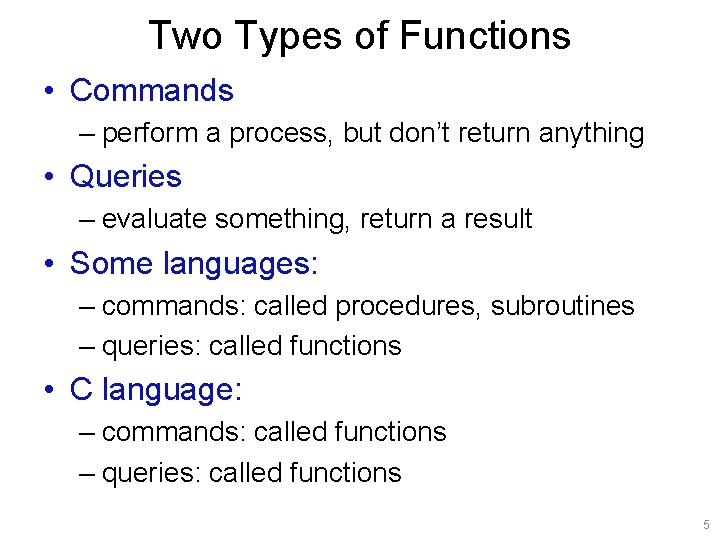
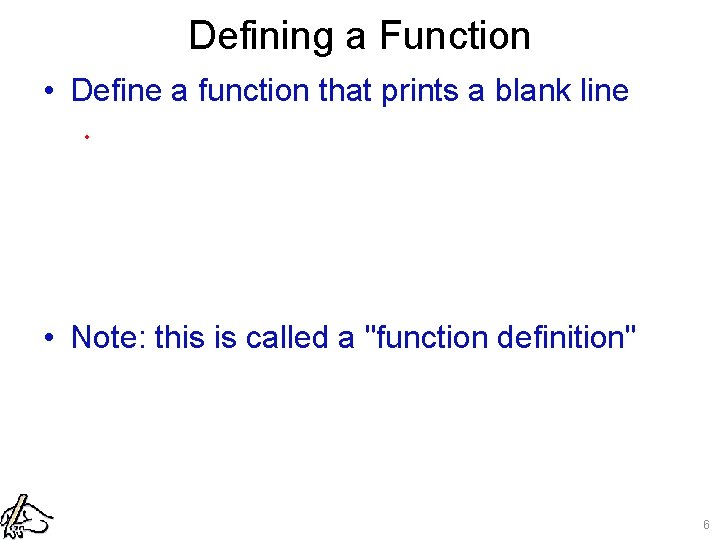
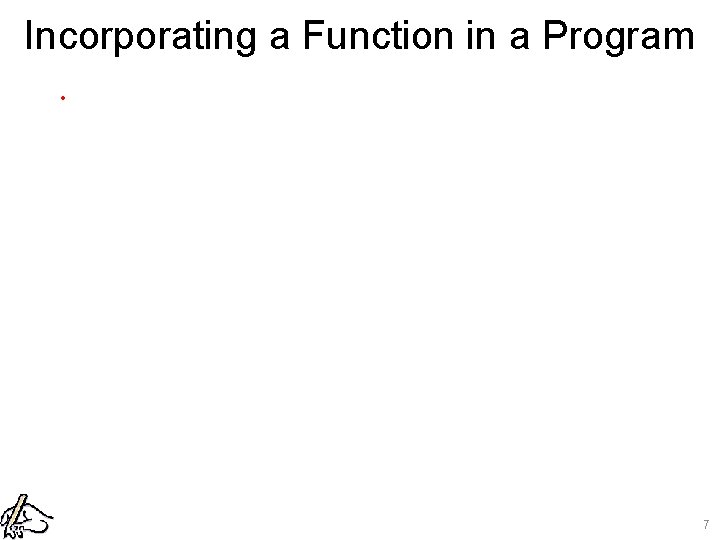
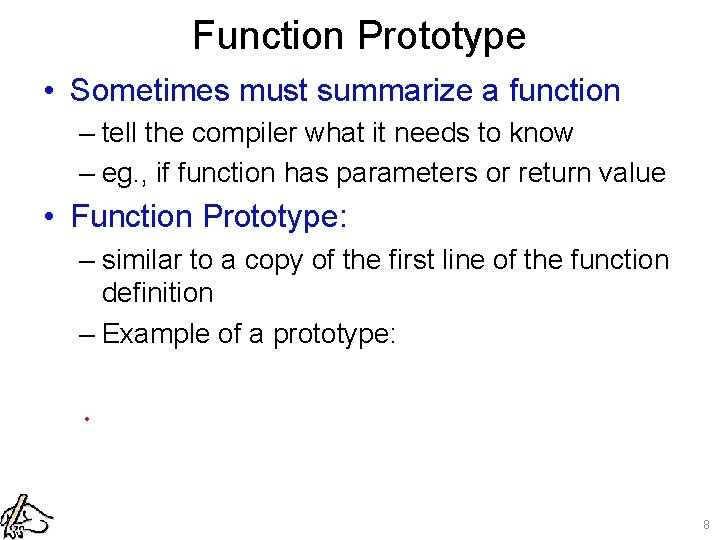
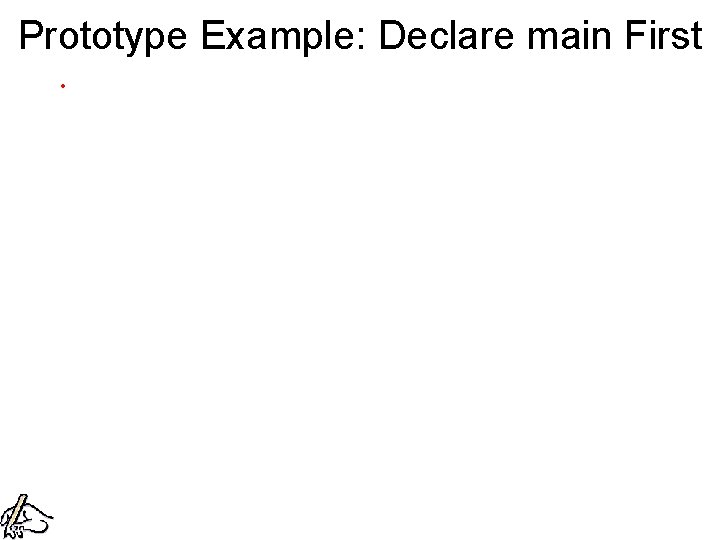
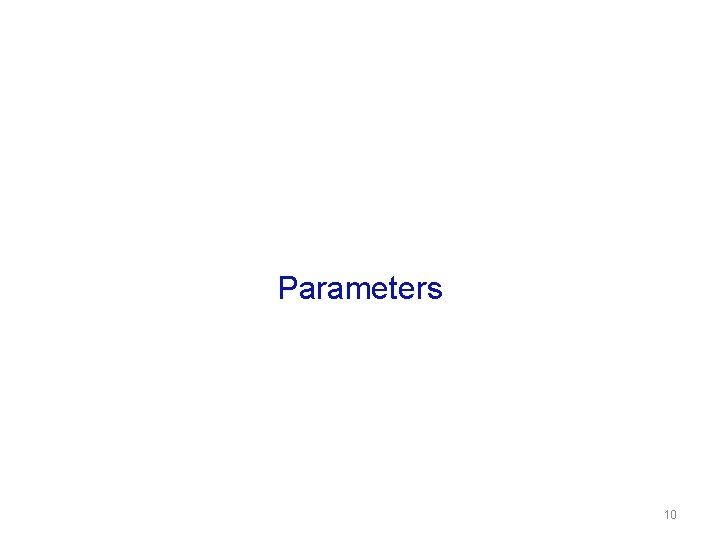
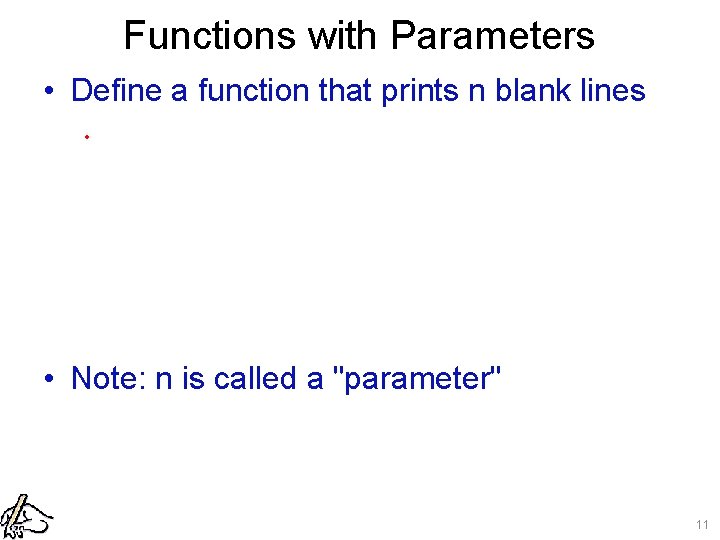
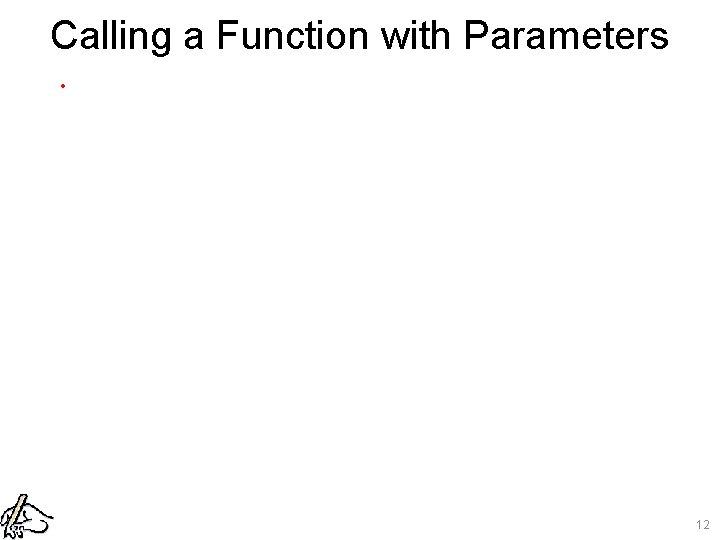
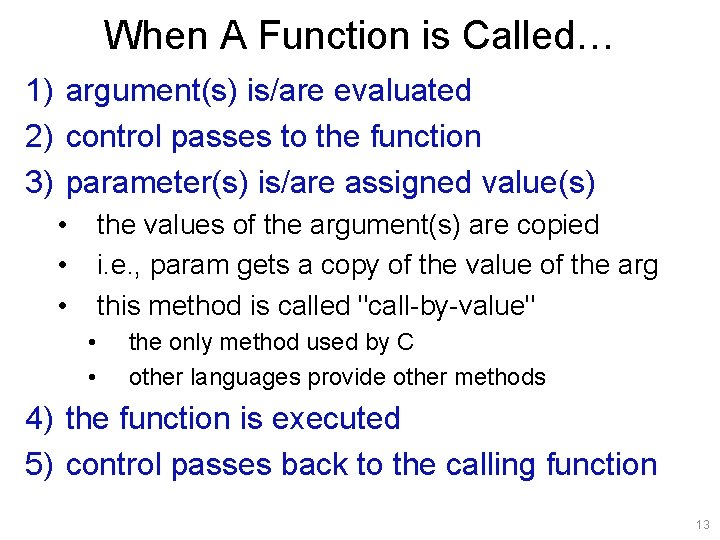
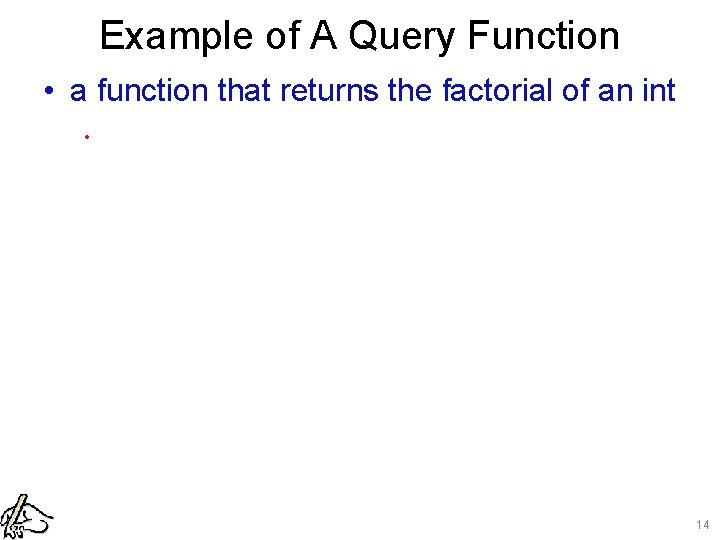
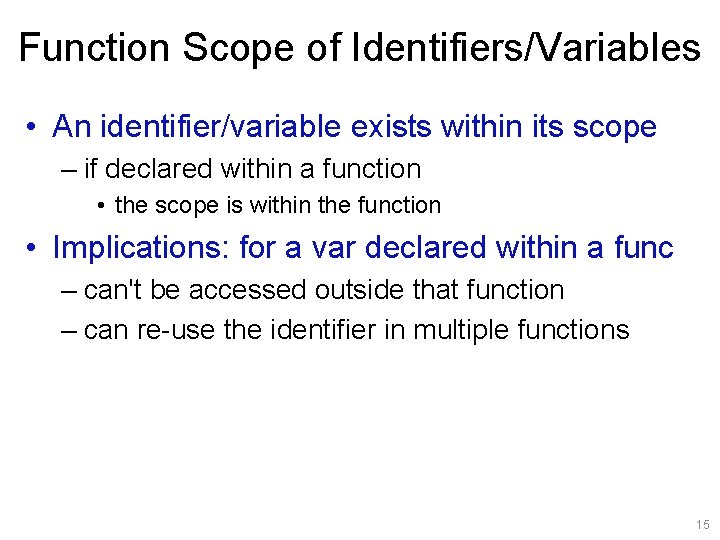
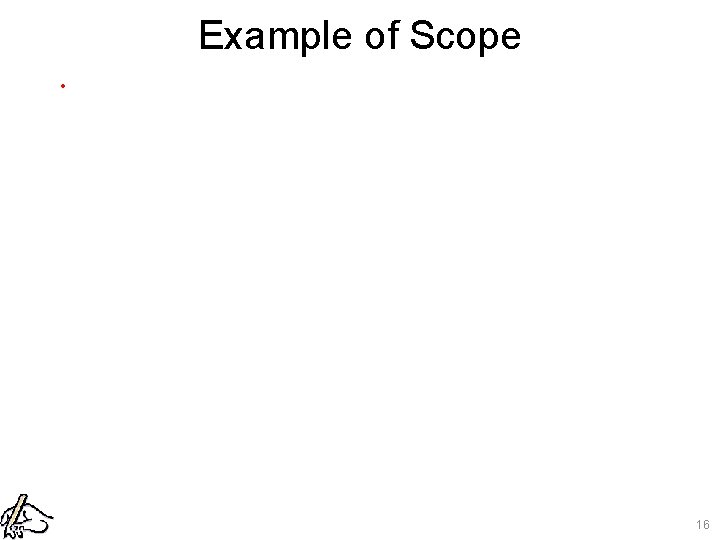
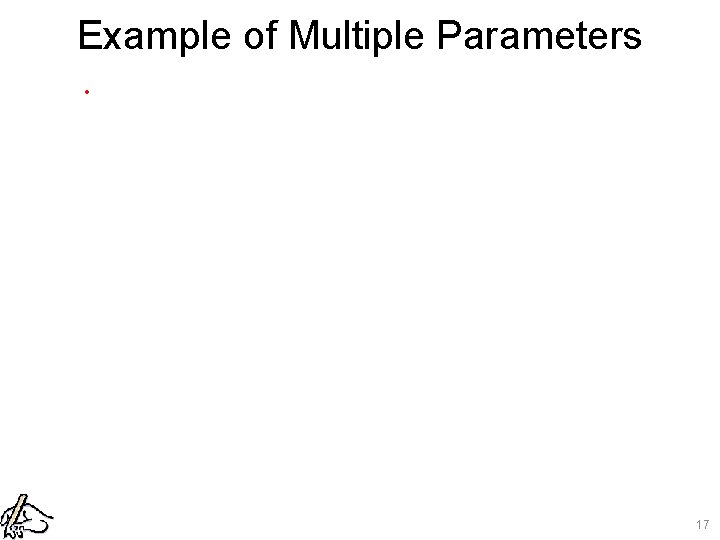
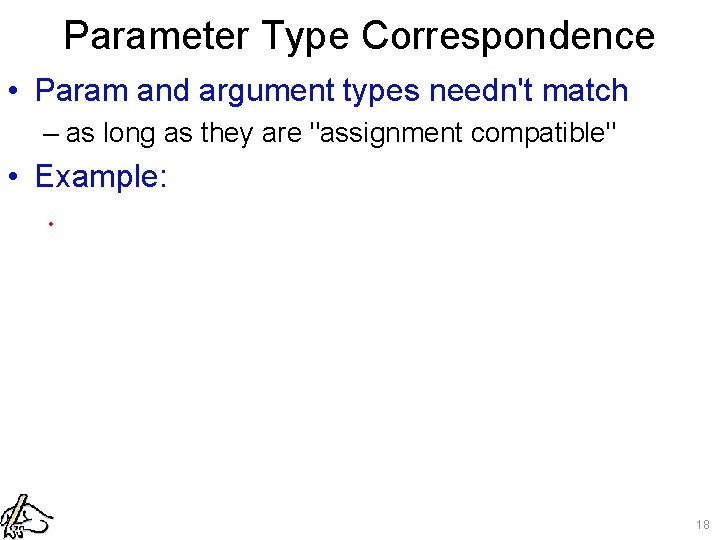
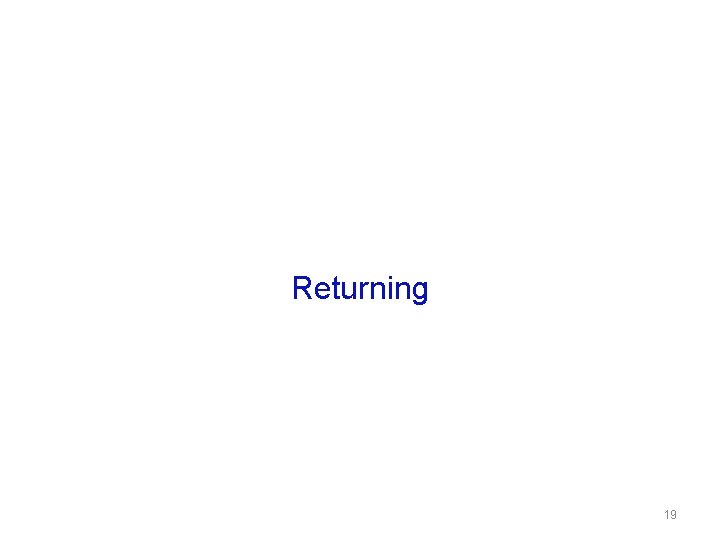
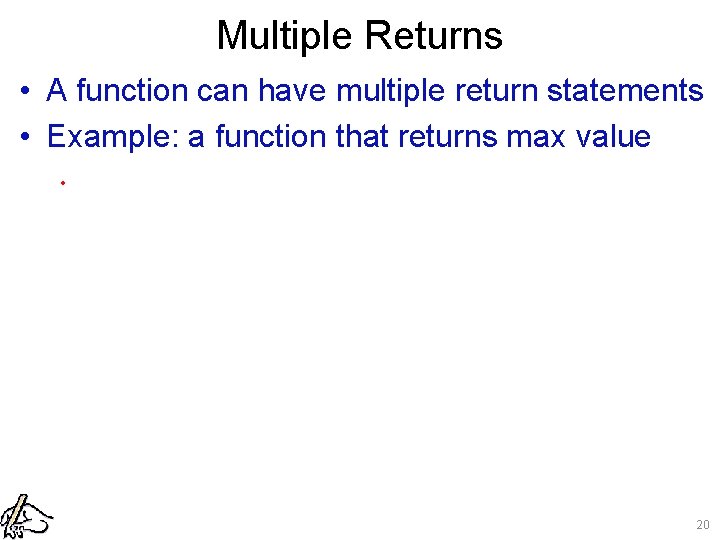
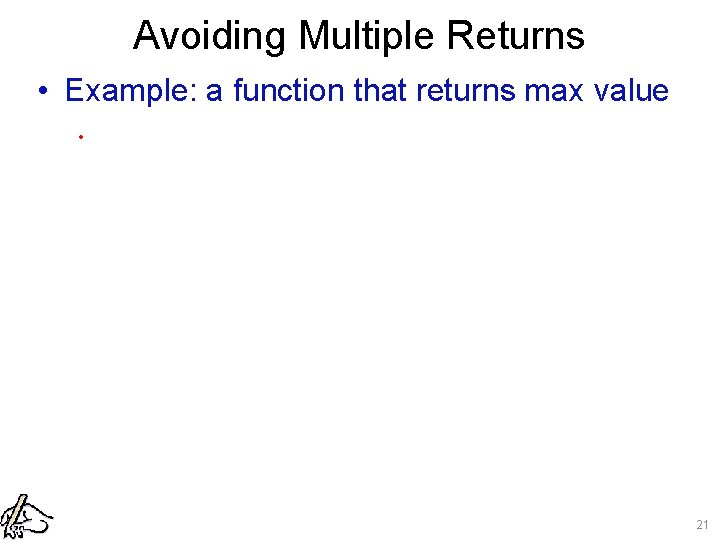
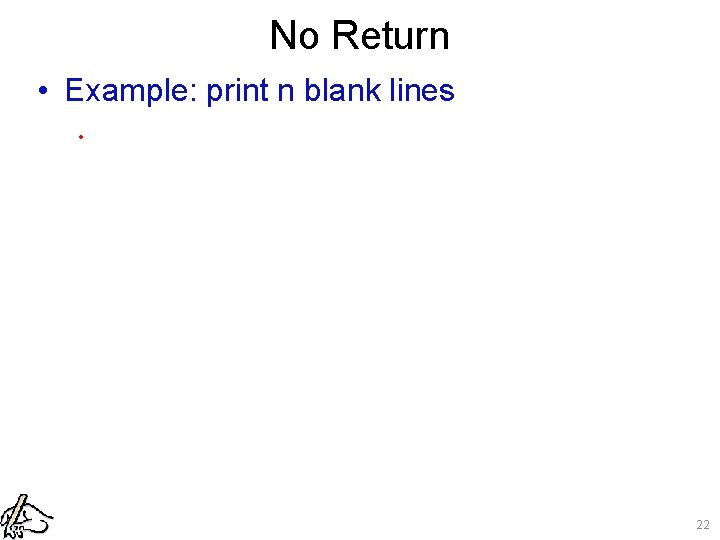
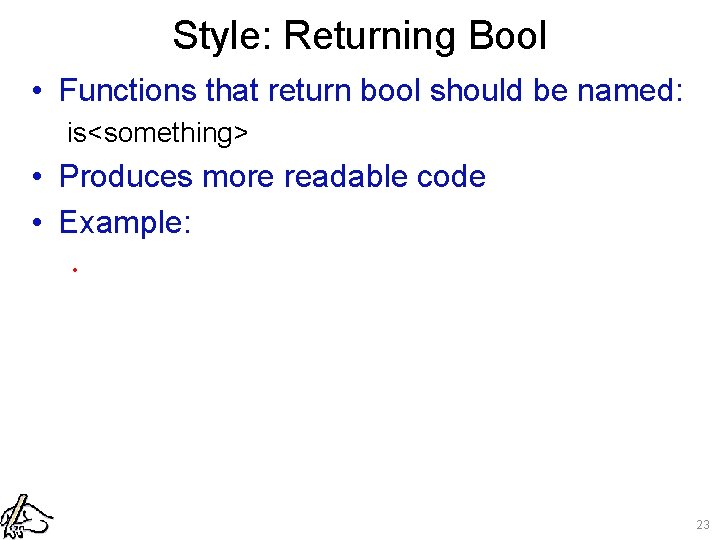
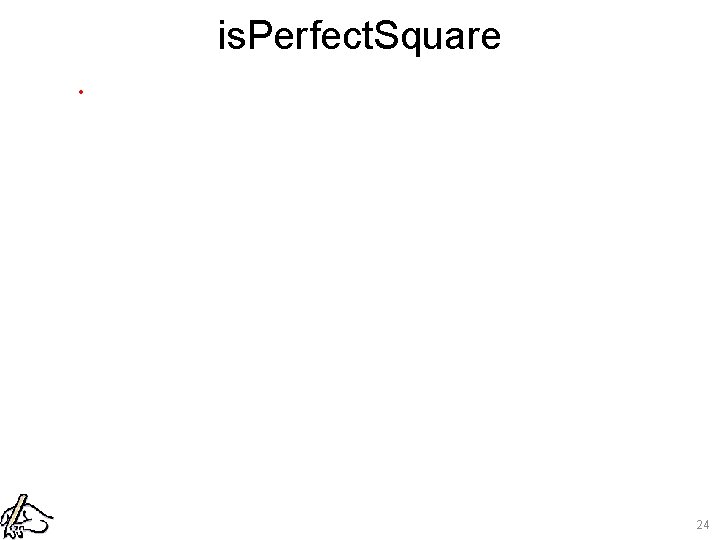
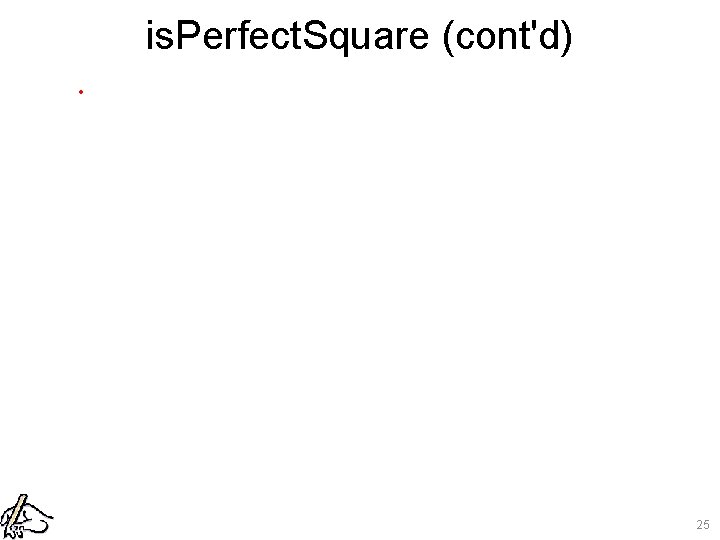
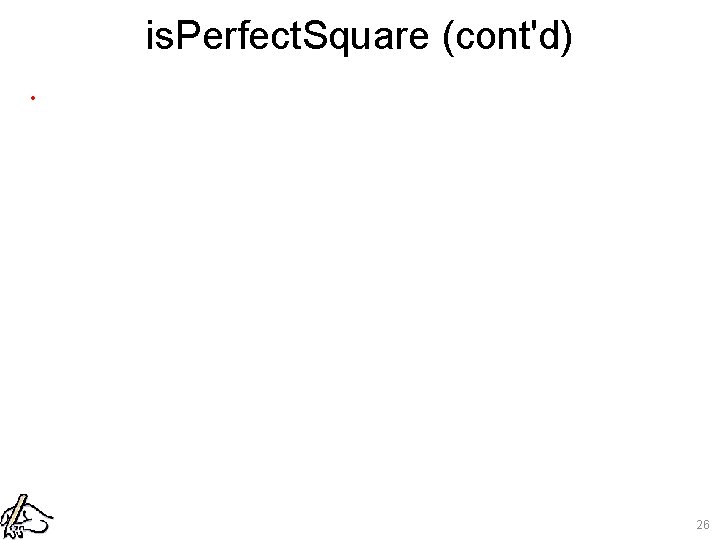
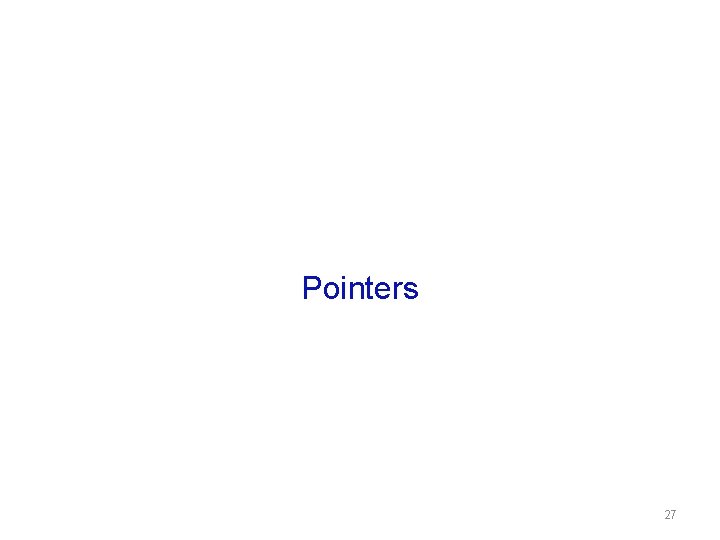
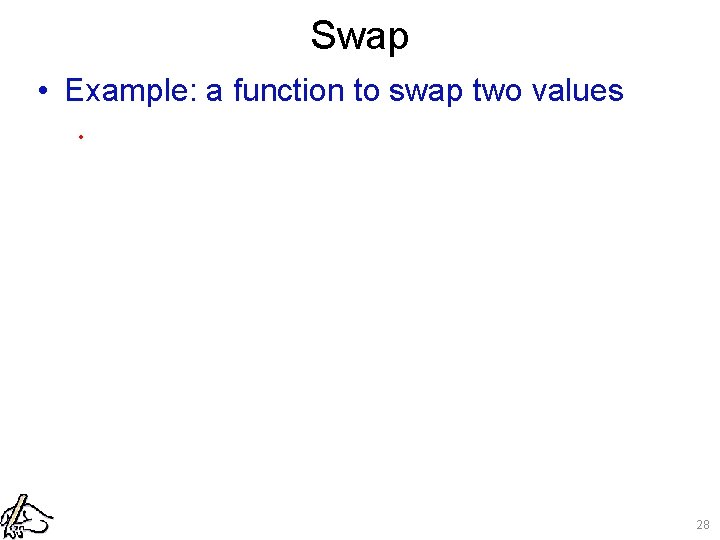
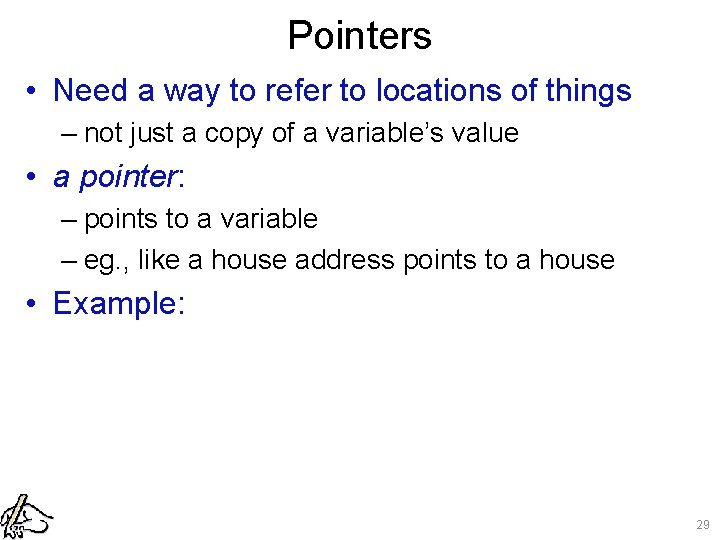
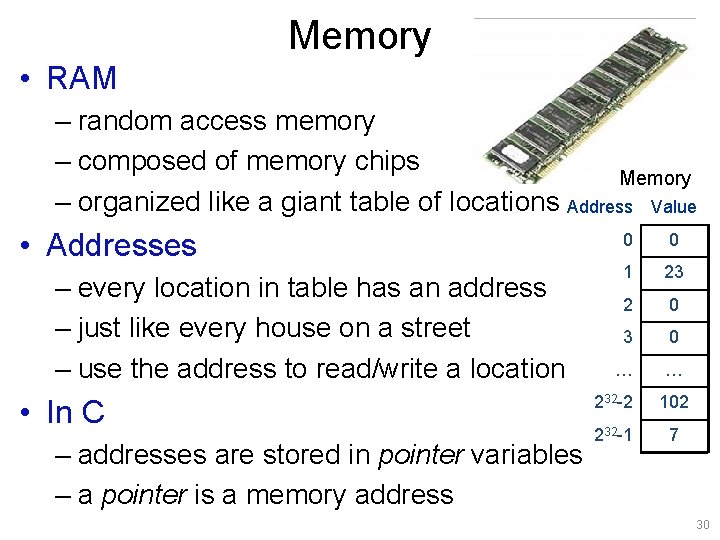
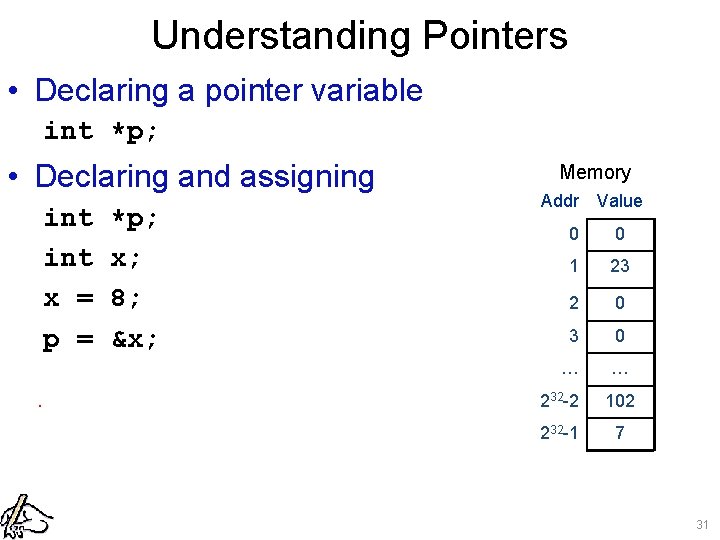
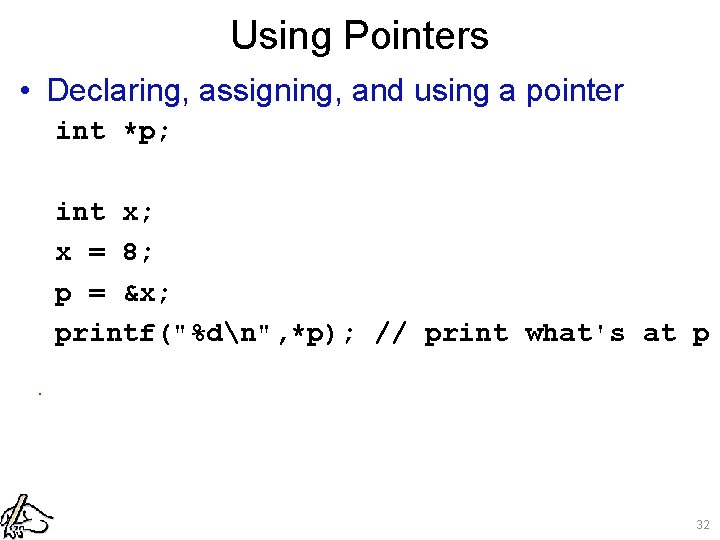
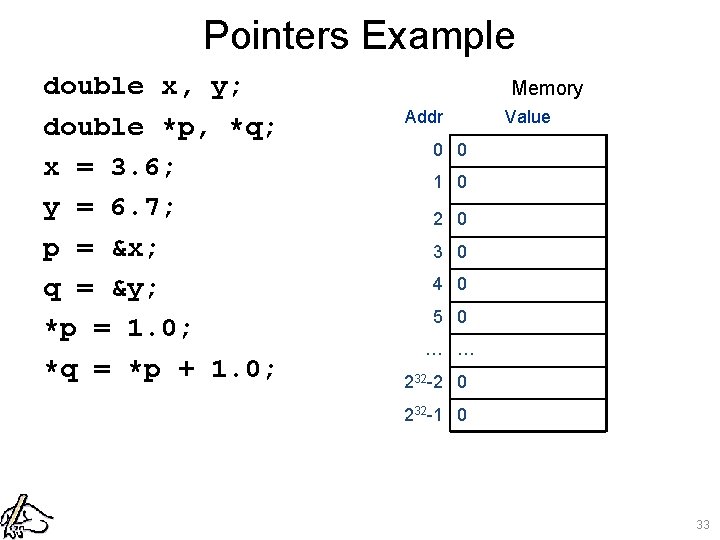
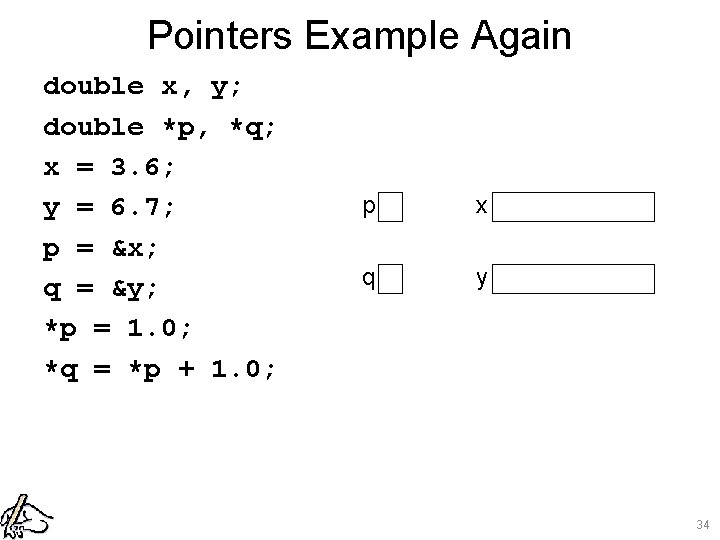
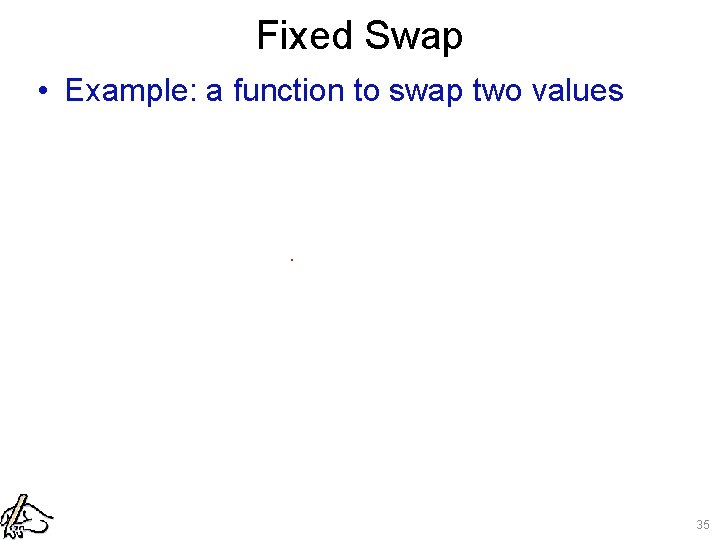
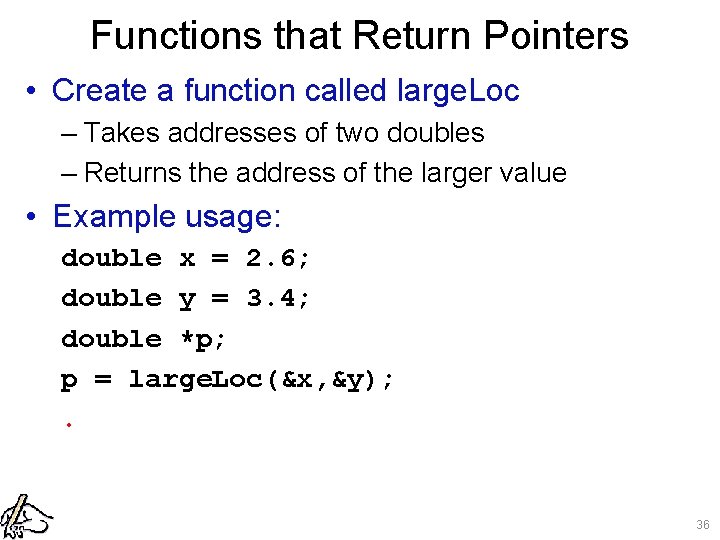
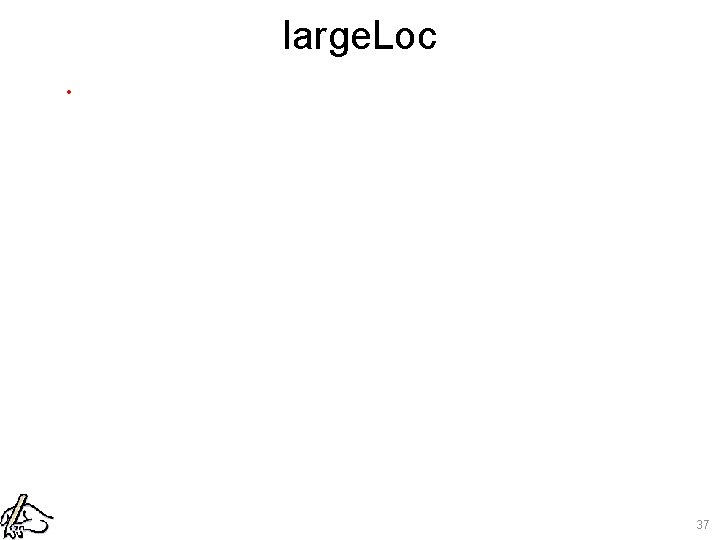
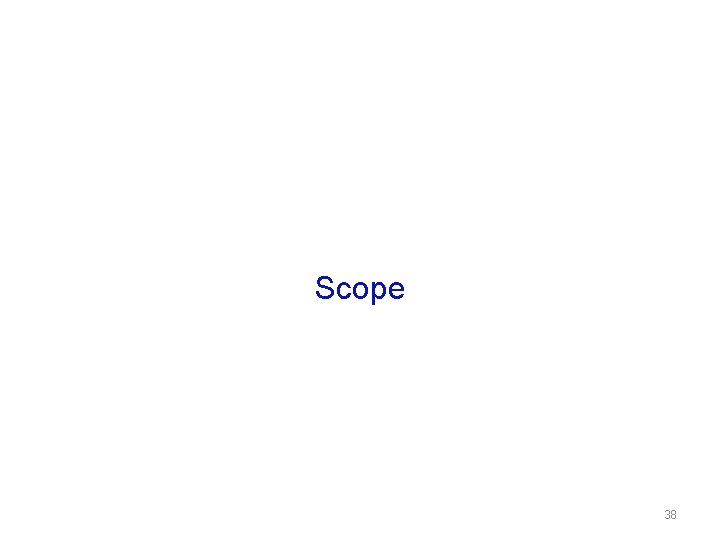
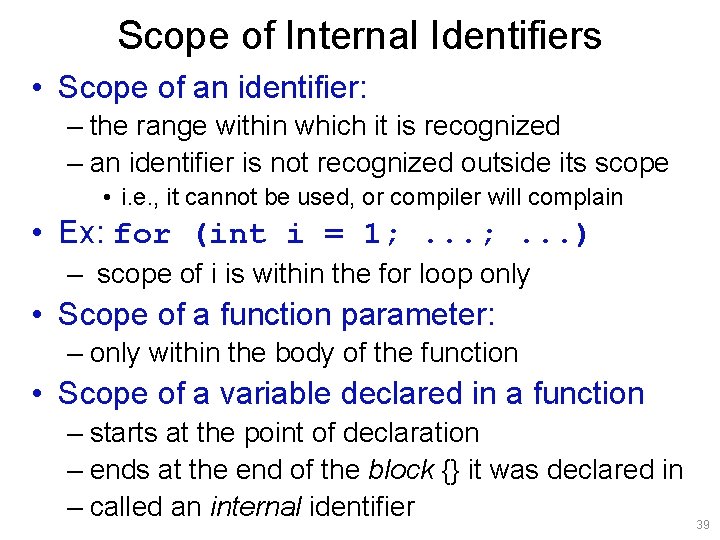
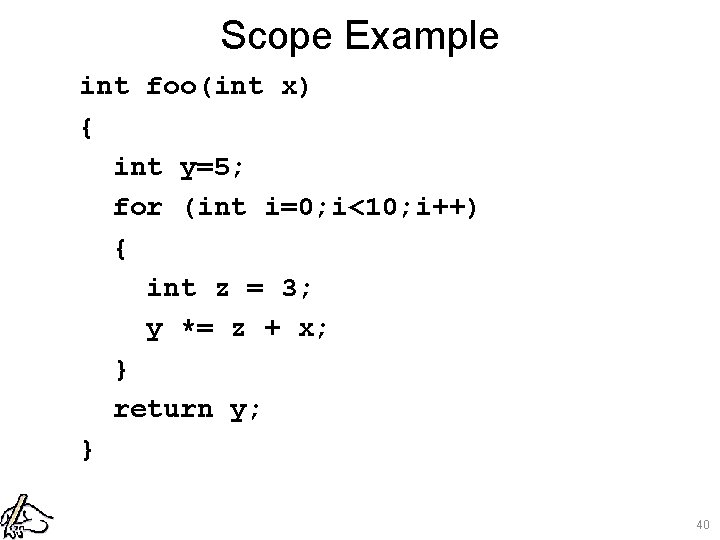
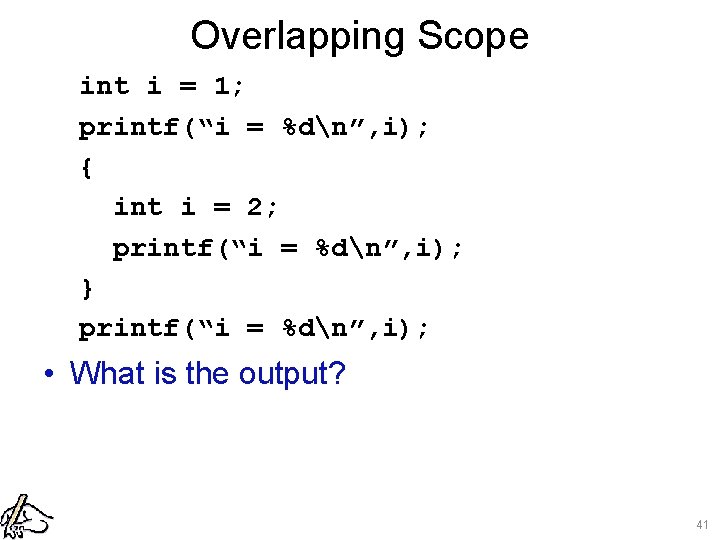
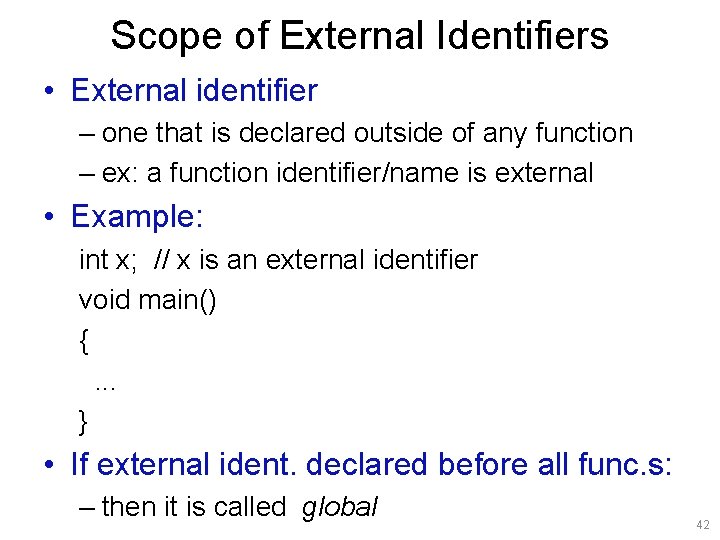
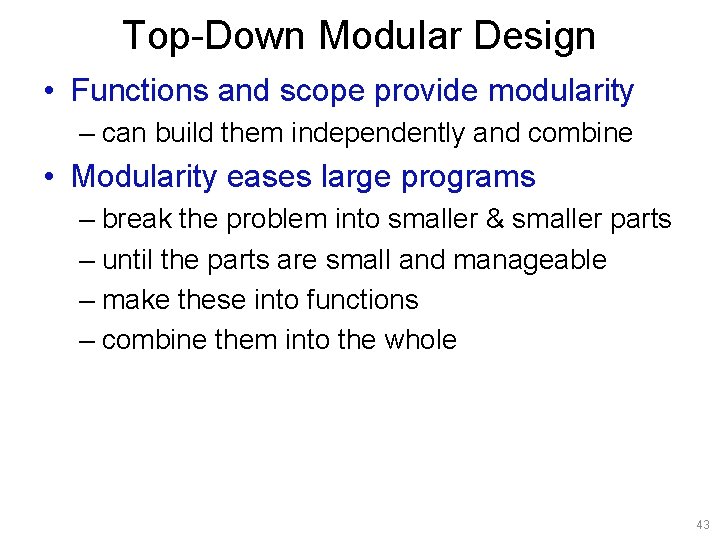
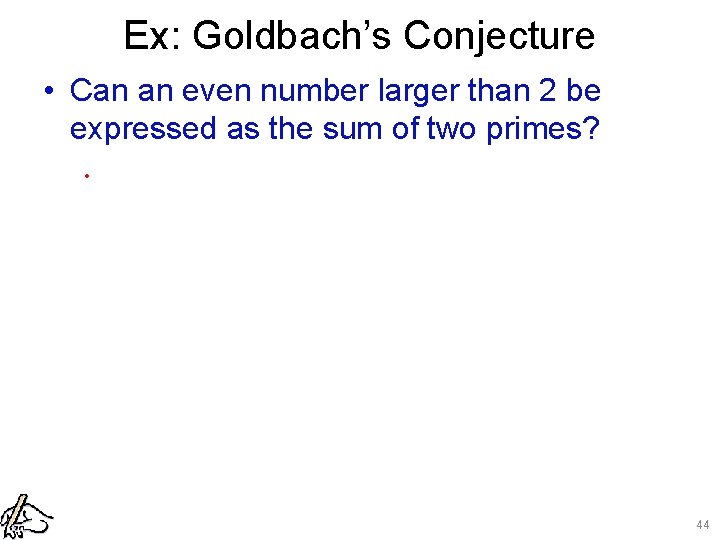
- Slides: 44
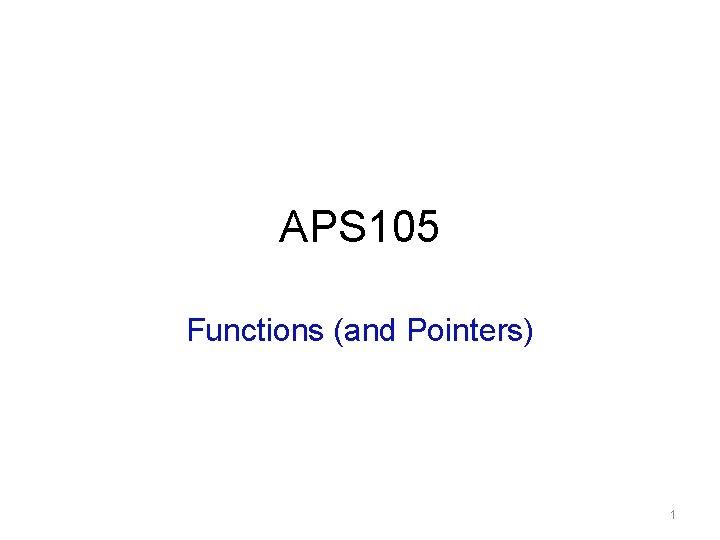
APS 105 Functions (and Pointers) 1
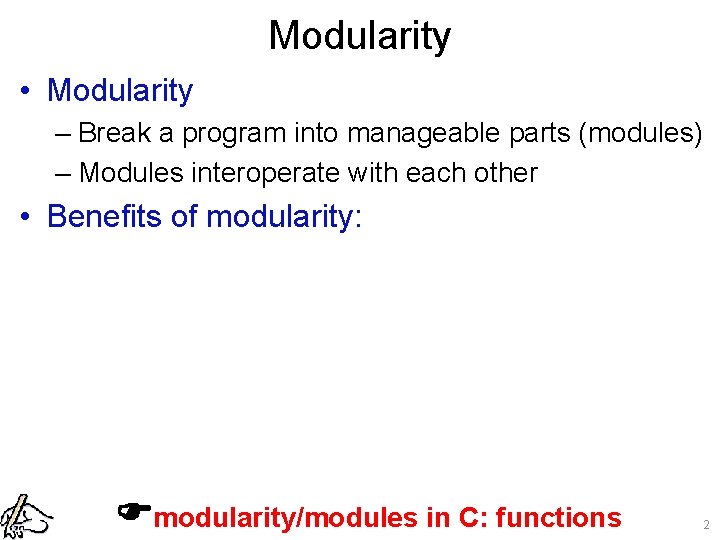
Modularity • Modularity – Break a program into manageable parts (modules) – Modules interoperate with each other • Benefits of modularity: modularity/modules in C: functions 2
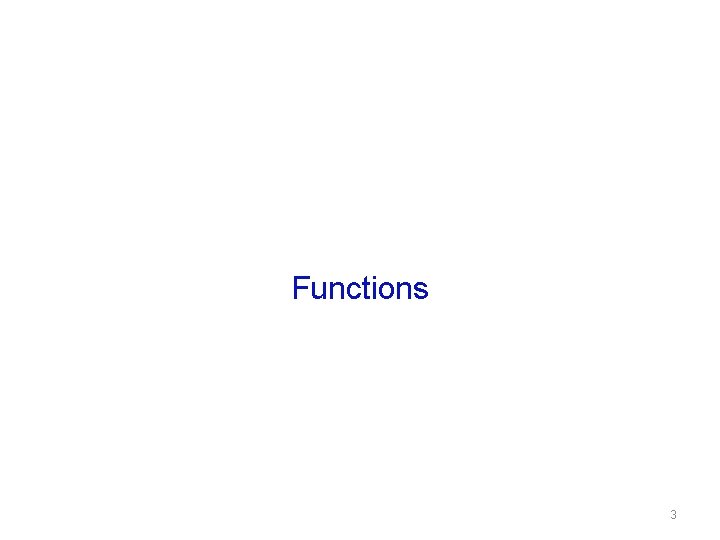
Functions 3
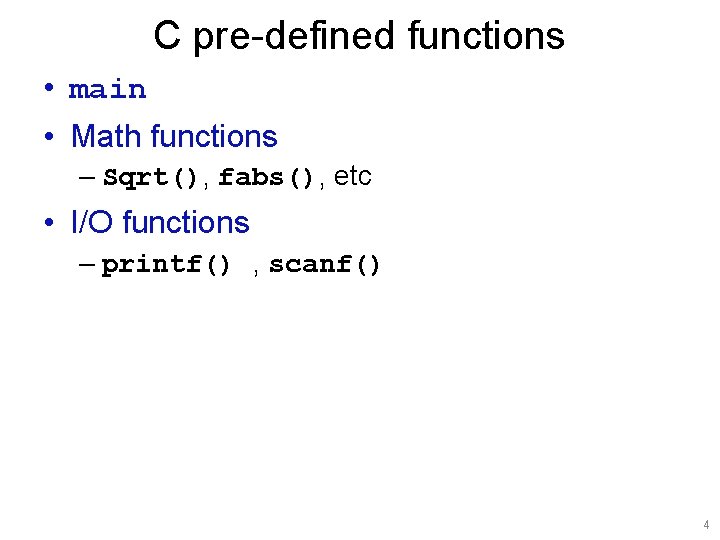
C pre-defined functions • main • Math functions – Sqrt(), fabs(), etc • I/O functions – printf() , scanf() 4
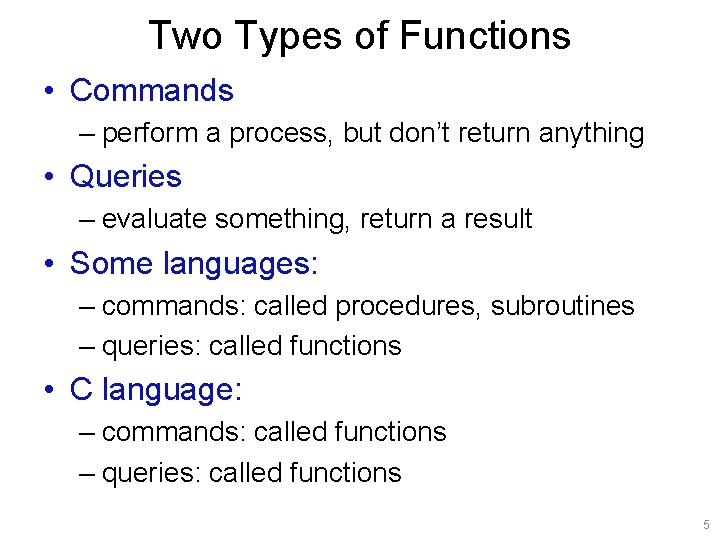
Two Types of Functions • Commands – perform a process, but don’t return anything • Queries – evaluate something, return a result • Some languages: – commands: called procedures, subroutines – queries: called functions • C language: – commands: called functions – queries: called functions 5
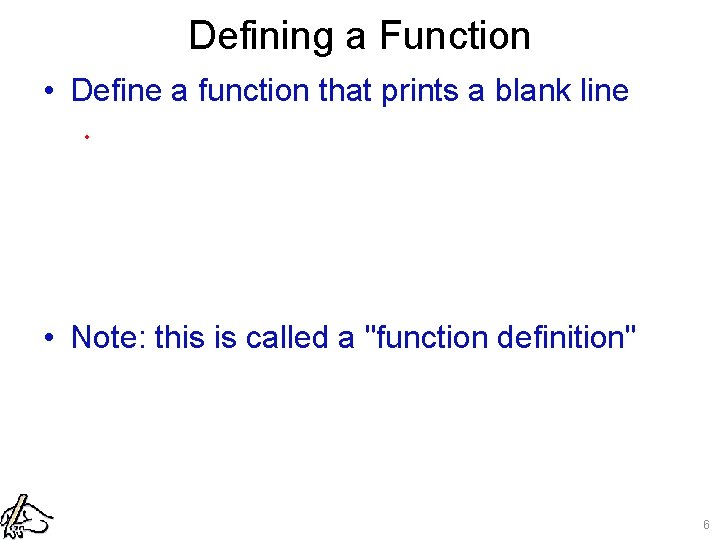
Defining a Function • Define a function that prints a blank line. • Note: this is called a "function definition" 6
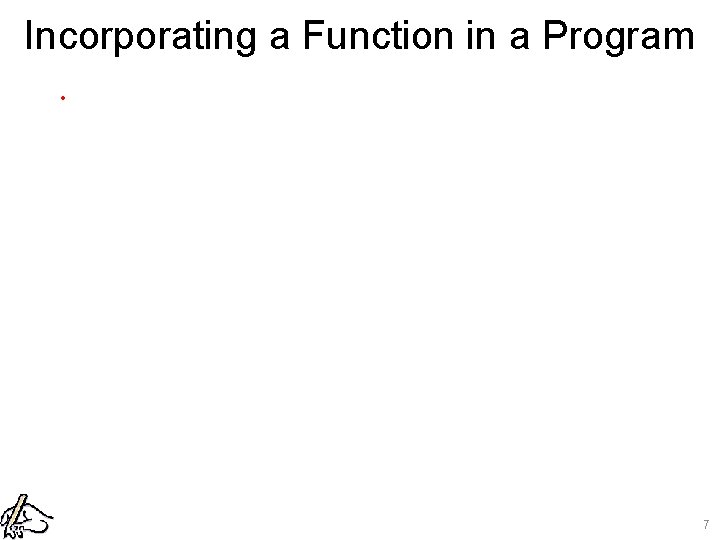
Incorporating a Function in a Program. 7
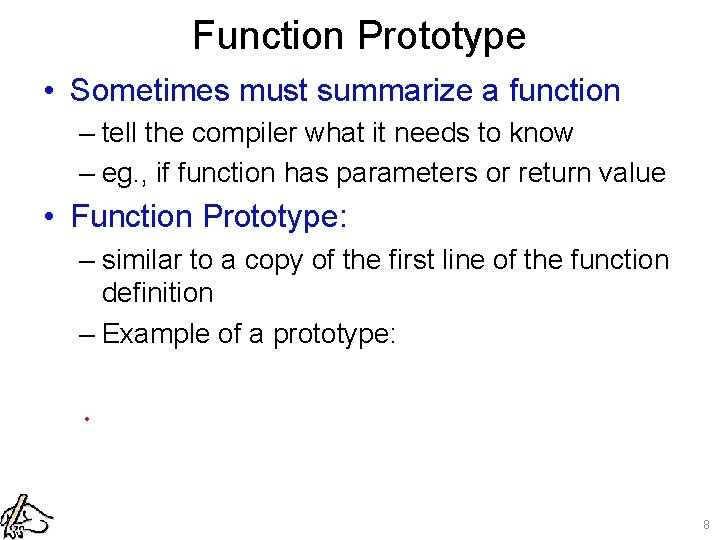
Function Prototype • Sometimes must summarize a function – tell the compiler what it needs to know – eg. , if function has parameters or return value • Function Prototype: – similar to a copy of the first line of the function definition – Example of a prototype: . 8
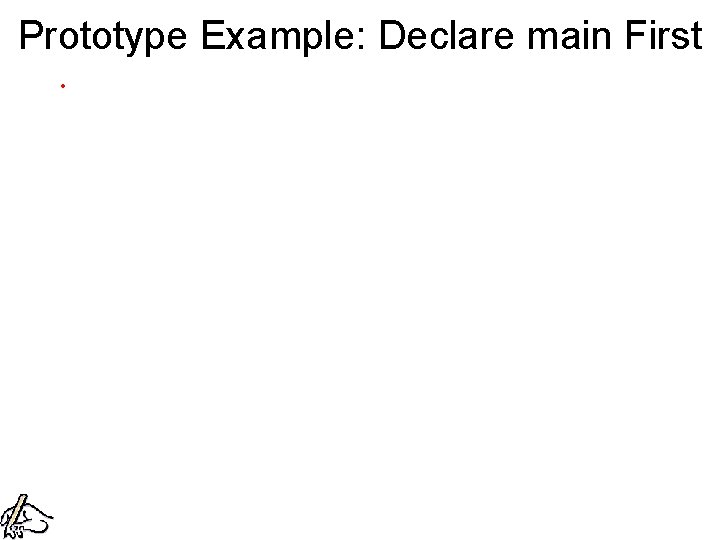
Prototype Example: Declare main First.
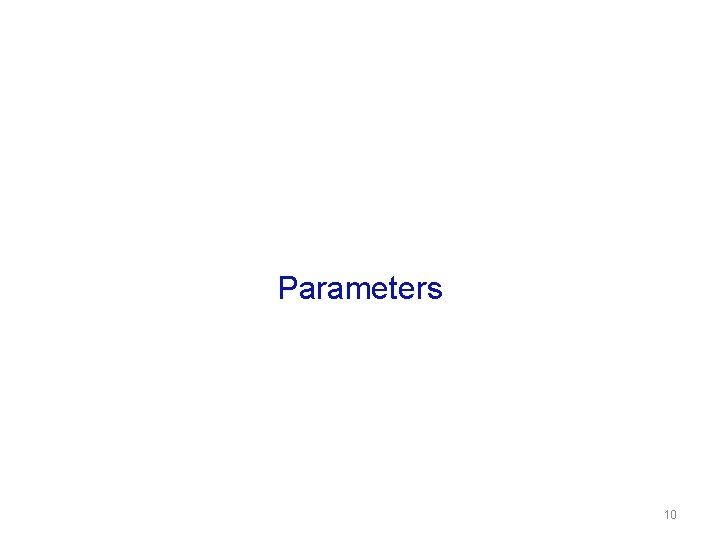
Parameters 10
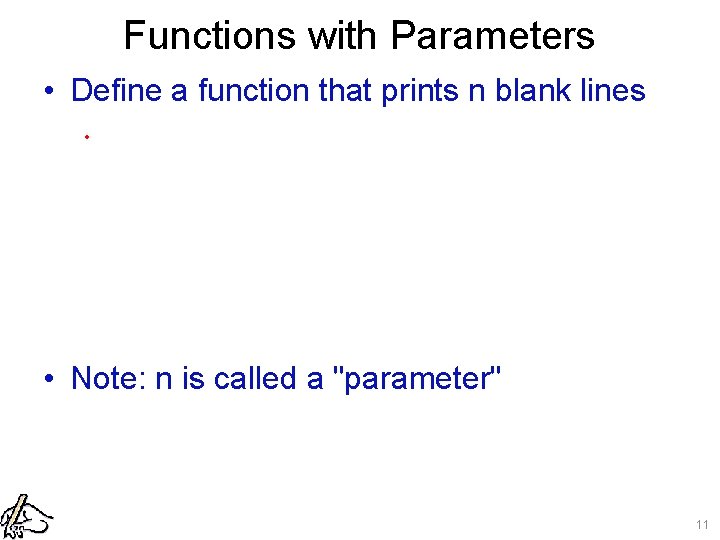
Functions with Parameters • Define a function that prints n blank lines. • Note: n is called a "parameter" 11
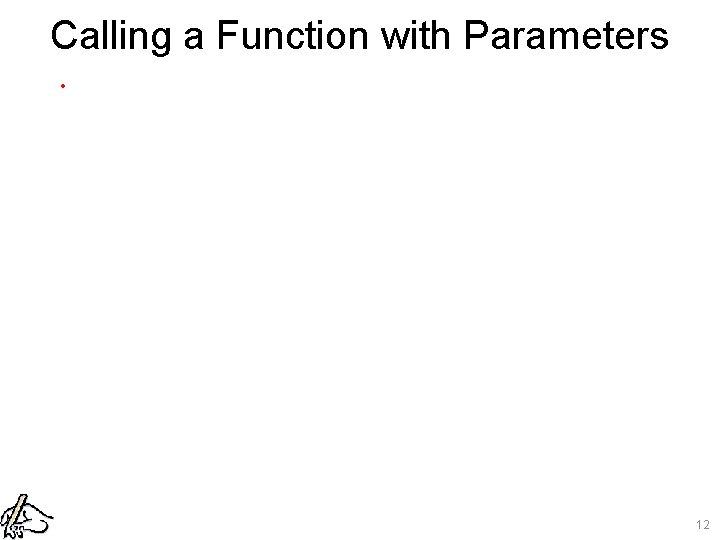
Calling a Function with Parameters. 12
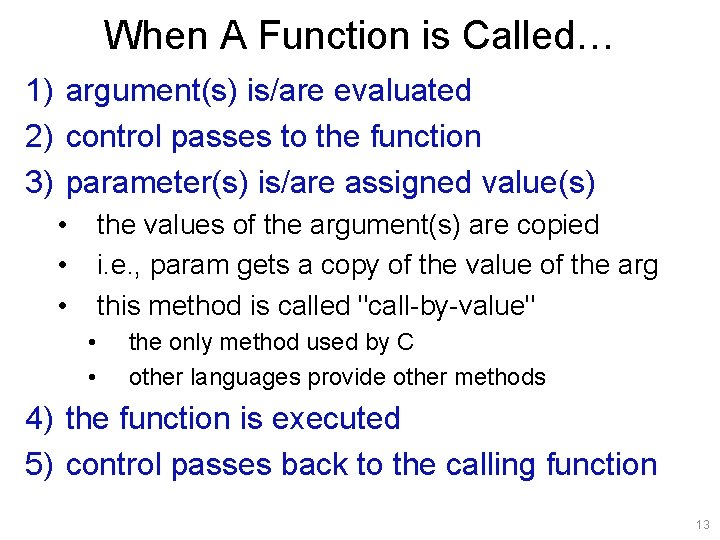
When A Function is Called… 1) argument(s) is/are evaluated 2) control passes to the function 3) parameter(s) is/are assigned value(s) • • • the values of the argument(s) are copied i. e. , param gets a copy of the value of the arg this method is called "call-by-value" • • the only method used by C other languages provide other methods 4) the function is executed 5) control passes back to the calling function 13
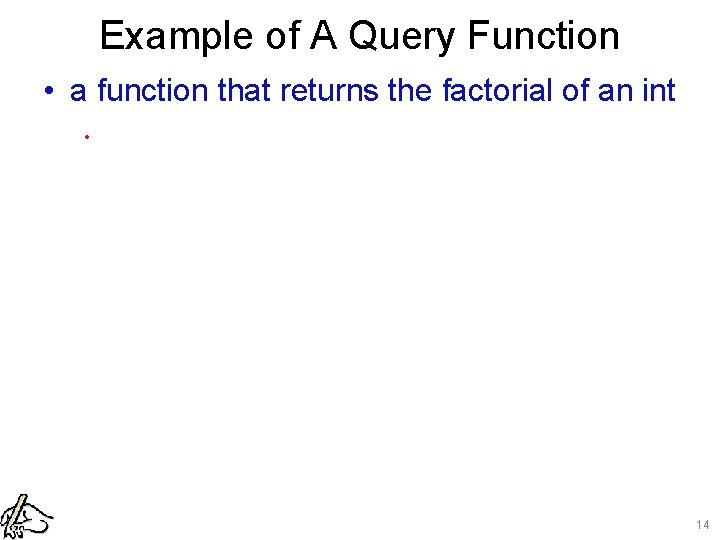
Example of A Query Function • a function that returns the factorial of an int. 14
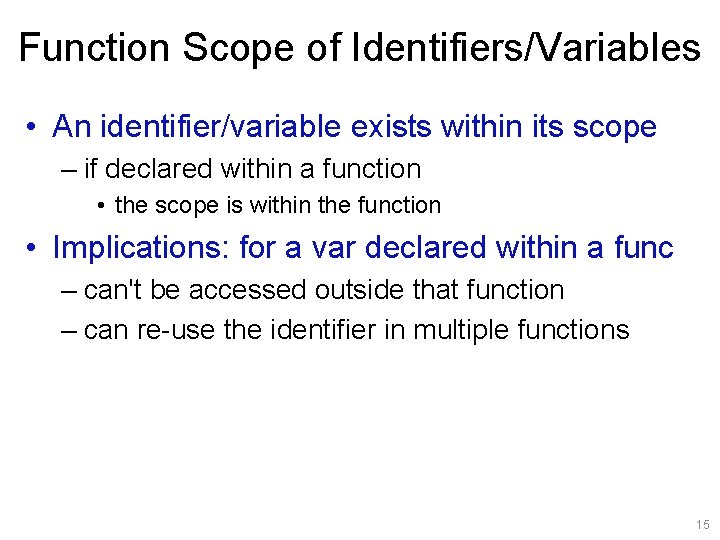
Function Scope of Identifiers/Variables • An identifier/variable exists within its scope – if declared within a function • the scope is within the function • Implications: for a var declared within a func – can't be accessed outside that function – can re-use the identifier in multiple functions 15
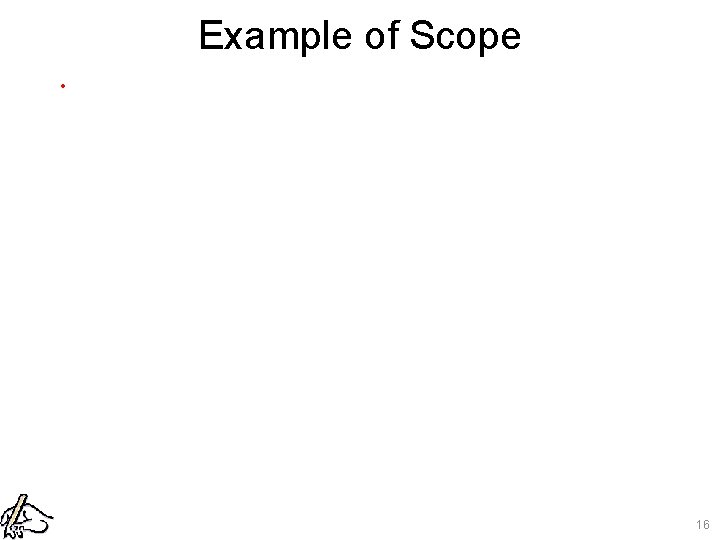
Example of Scope. 16
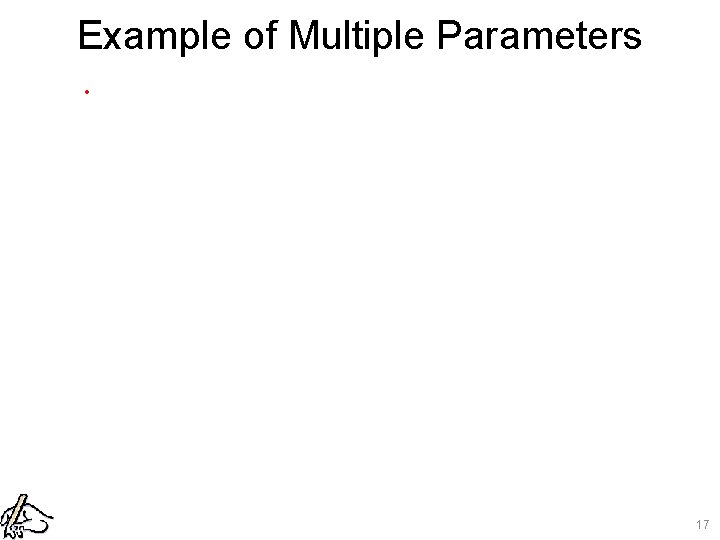
Example of Multiple Parameters. 17
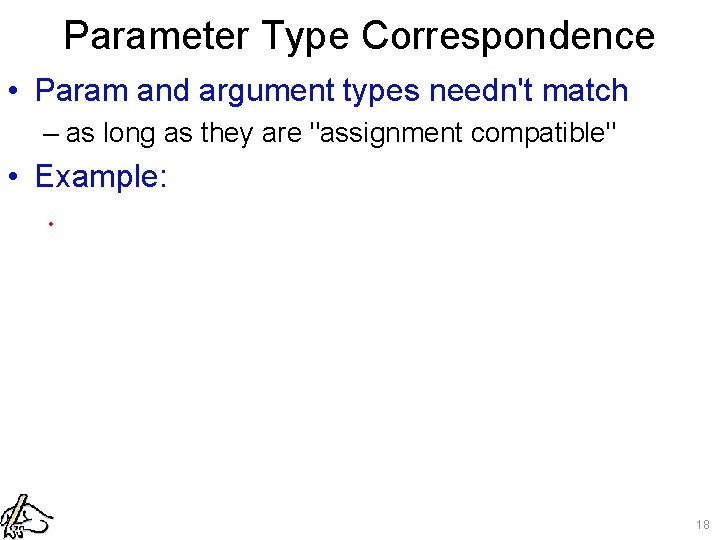
Parameter Type Correspondence • Param and argument types needn't match – as long as they are "assignment compatible" • Example: . 18
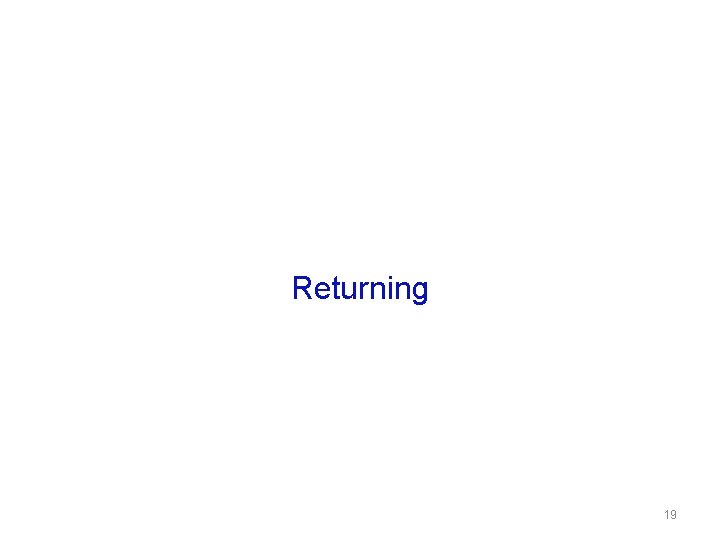
Returning 19
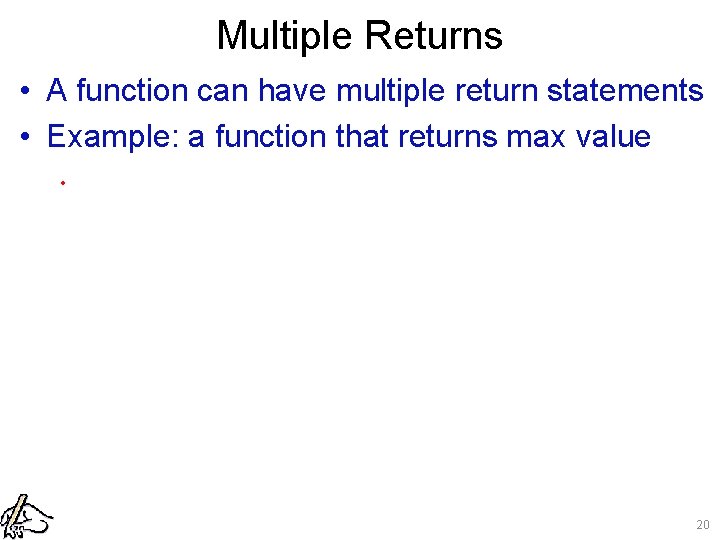
Multiple Returns • A function can have multiple return statements • Example: a function that returns max value. 20
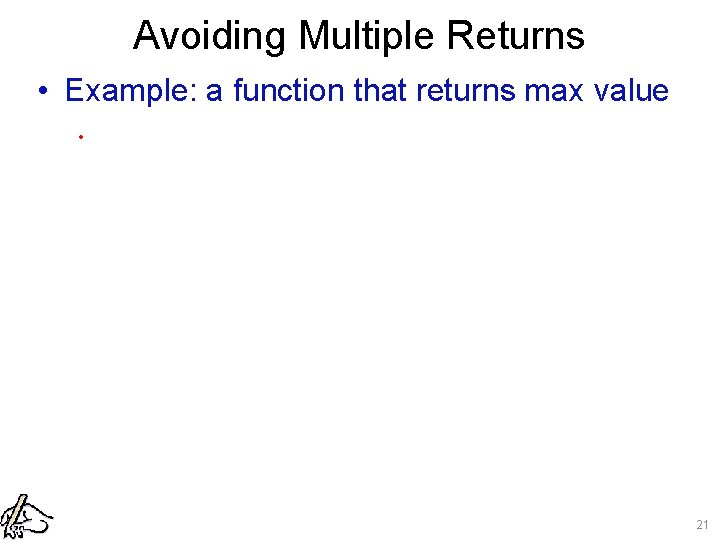
Avoiding Multiple Returns • Example: a function that returns max value. 21
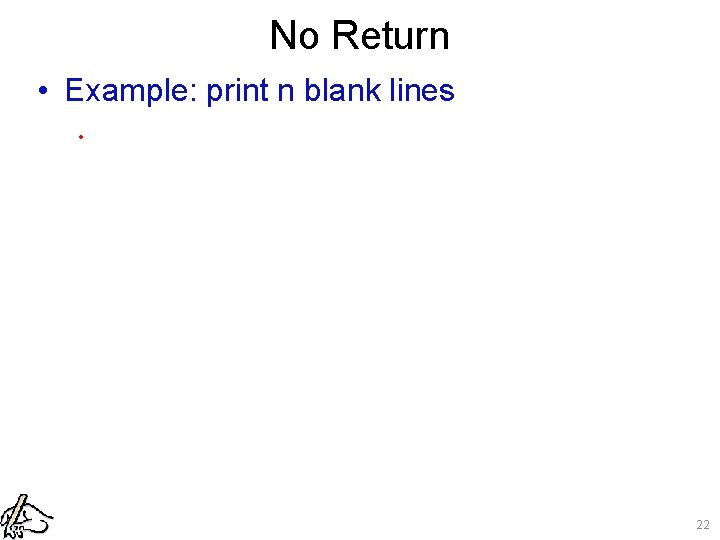
No Return • Example: print n blank lines. 22
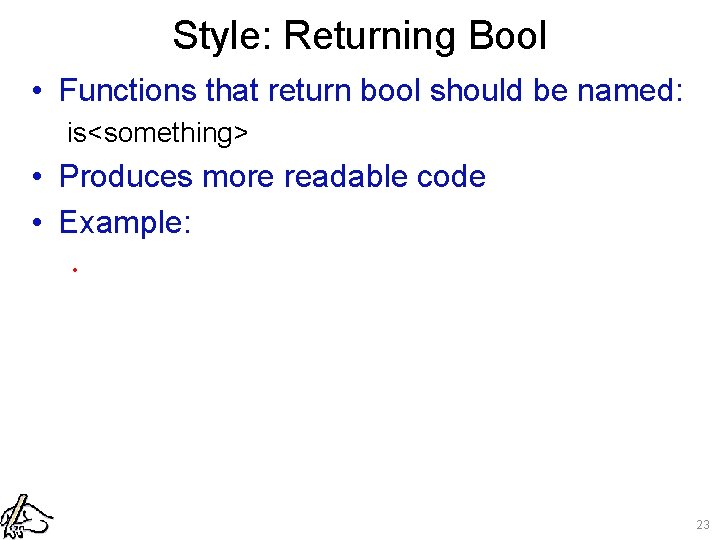
Style: Returning Bool • Functions that return bool should be named: is<something> • Produces more readable code • Example: . 23
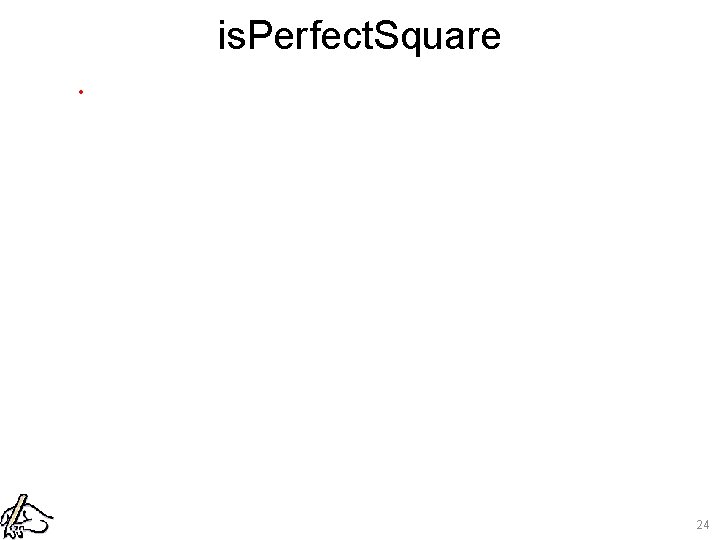
is. Perfect. Square. 24
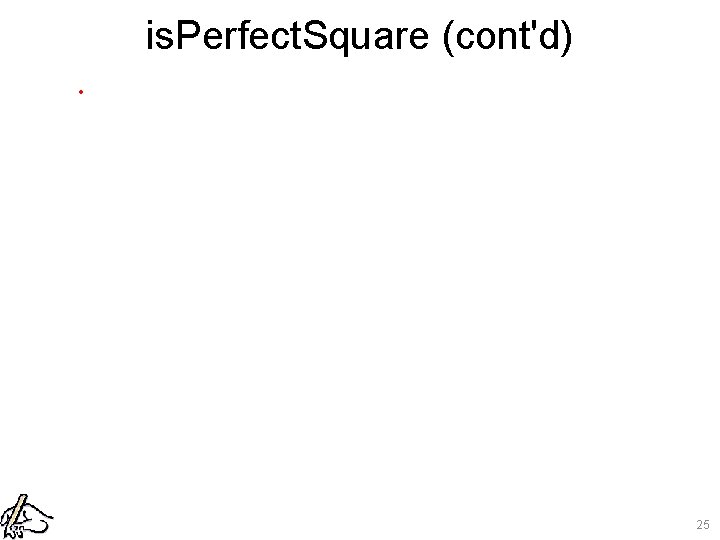
is. Perfect. Square (cont'd). 25
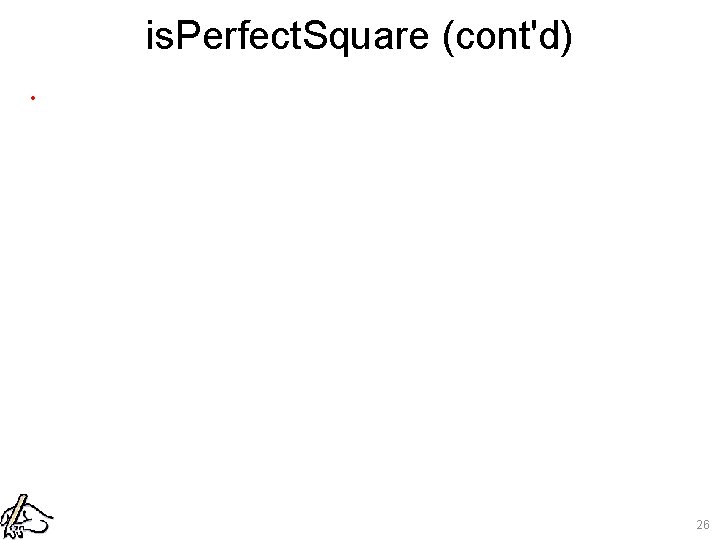
is. Perfect. Square (cont'd). 26
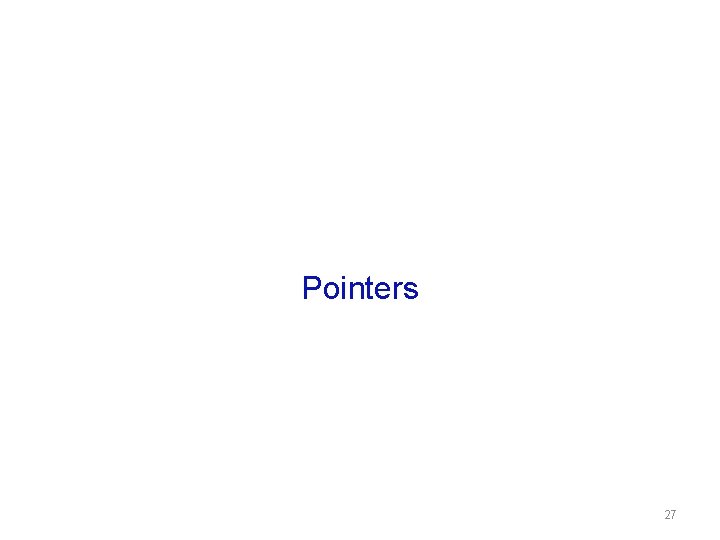
Pointers 27
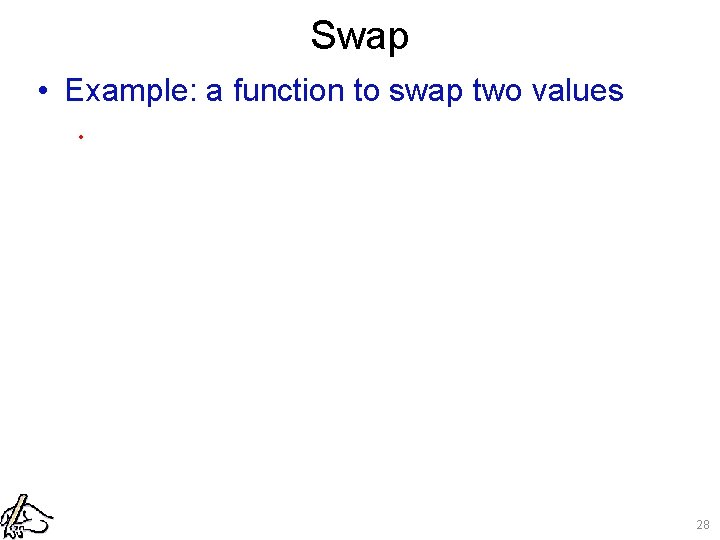
Swap • Example: a function to swap two values. 28
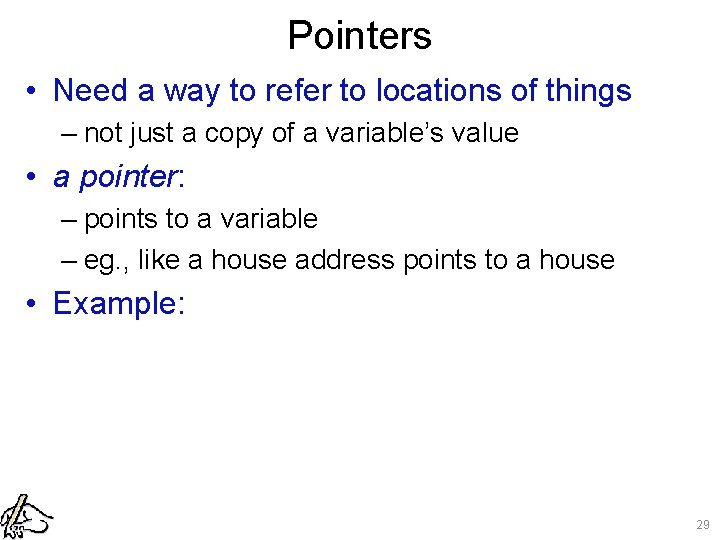
Pointers • Need a way to refer to locations of things – not just a copy of a variable’s value • a pointer: – points to a variable – eg. , like a house address points to a house • Example: 29
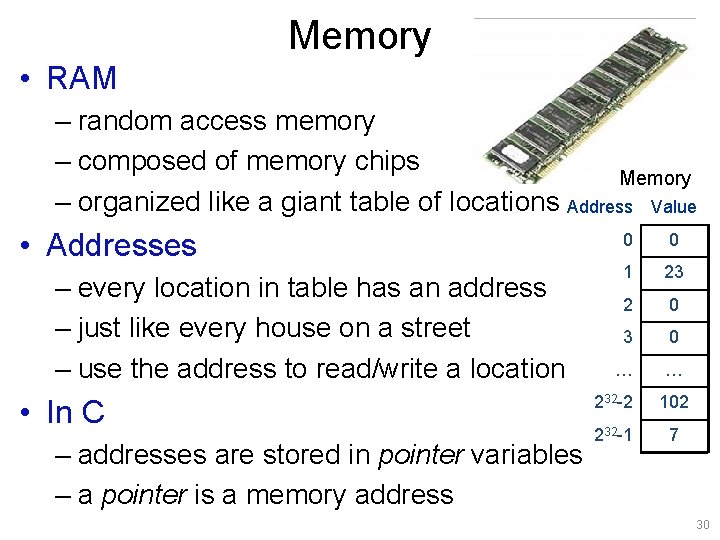
Memory • RAM – random access memory – composed of memory chips Memory – organized like a giant table of locations Address Value • Addresses – every location in table has an address – just like every house on a street – use the address to read/write a location • In C – addresses are stored in pointer variables – a pointer is a memory address 0 0 1 23 2 0 3 0 … … 232 -2 102 232 -1 7 30
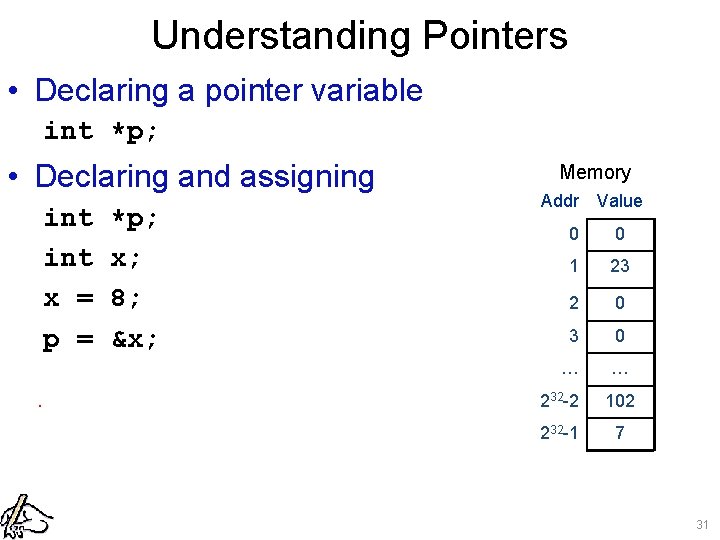
Understanding Pointers • Declaring a pointer variable int *p; • Declaring and assigning int x = p =. *p; x; 8; &x; Memory Addr Value 0 0 1 23 2 0 3 0 … … 232 -2 102 232 -1 7 31
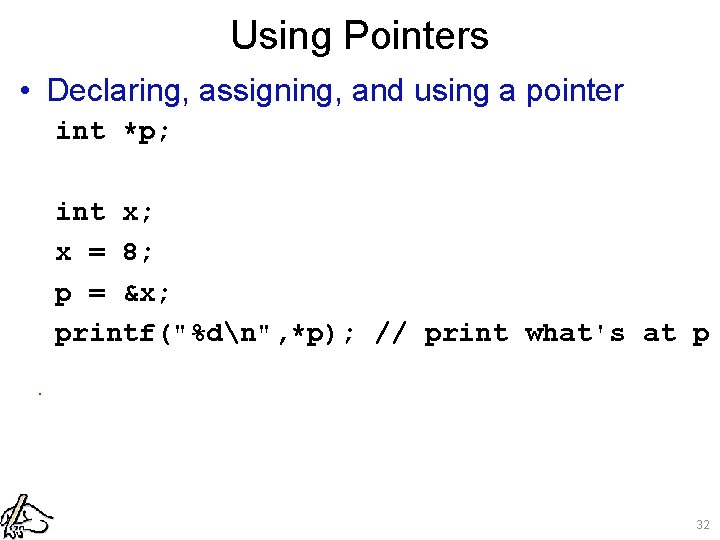
Using Pointers • Declaring, assigning, and using a pointer int *p; int x; x = 8; p = &x; printf("%dn", *p); // print what's at p. 32
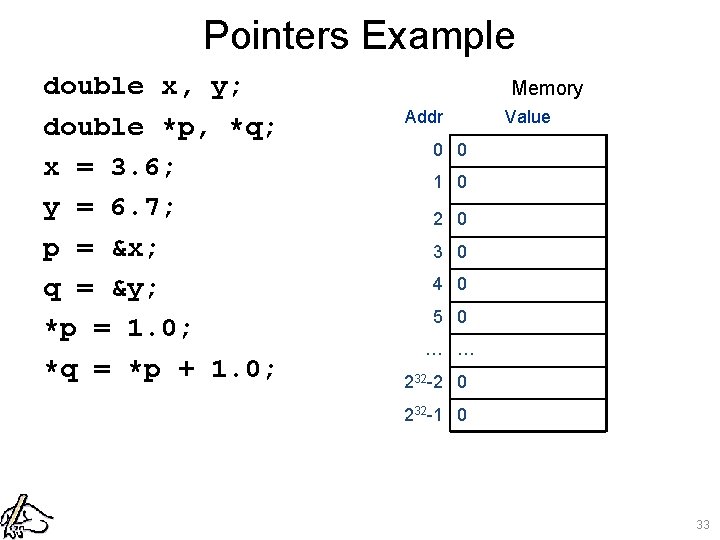
Pointers Example double x, y; double *p, *q; x = 3. 6; y = 6. 7; p = &x; q = &y; *p = 1. 0; *q = *p + 1. 0; Memory Addr Value 0 0 1 0 2 0 3 0 4 0 5 0 … … 232 -2 0 232 -1 0 33
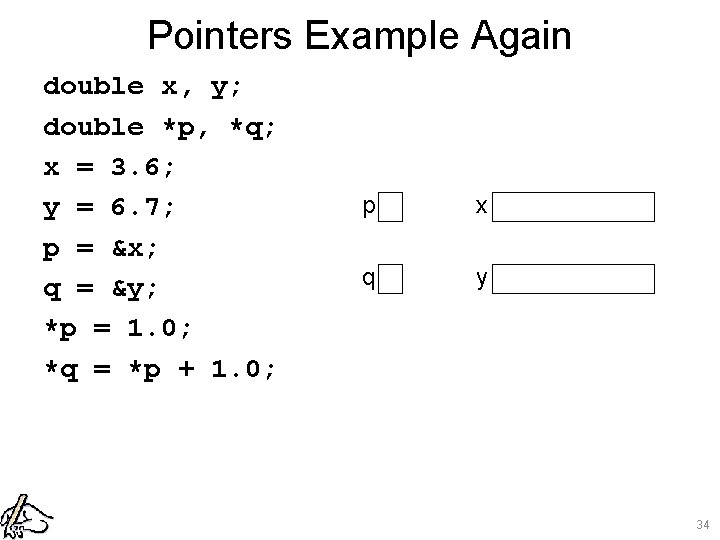
Pointers Example Again double x, y; double *p, *q; x = 3. 6; y = 6. 7; p = &x; q = &y; *p = 1. 0; *q = *p + 1. 0; p x q y 34
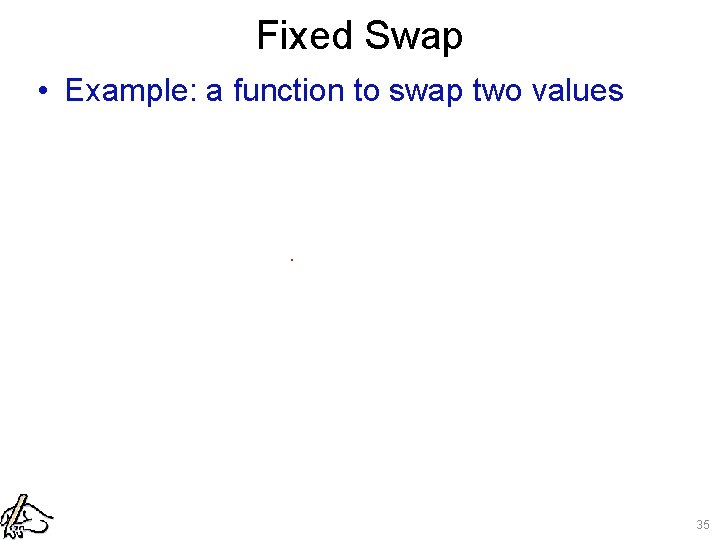
Fixed Swap • Example: a function to swap two values . 35
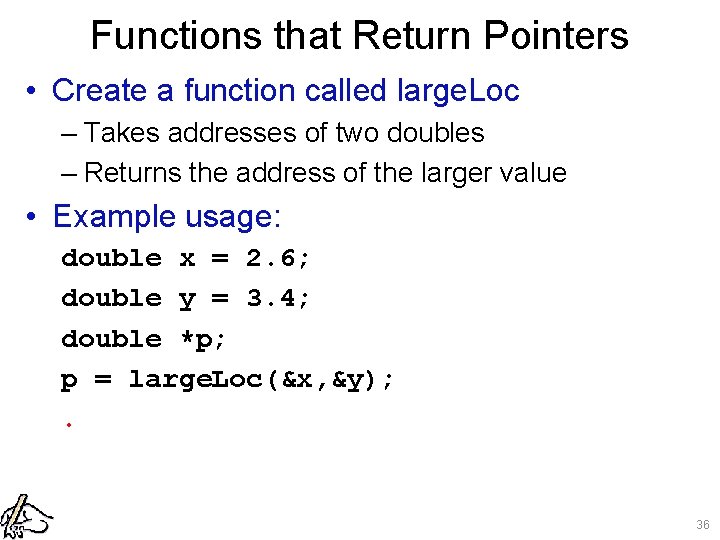
Functions that Return Pointers • Create a function called large. Loc – Takes addresses of two doubles – Returns the address of the larger value • Example usage: double x = 2. 6; double y = 3. 4; double *p; p = large. Loc(&x, &y); . 36
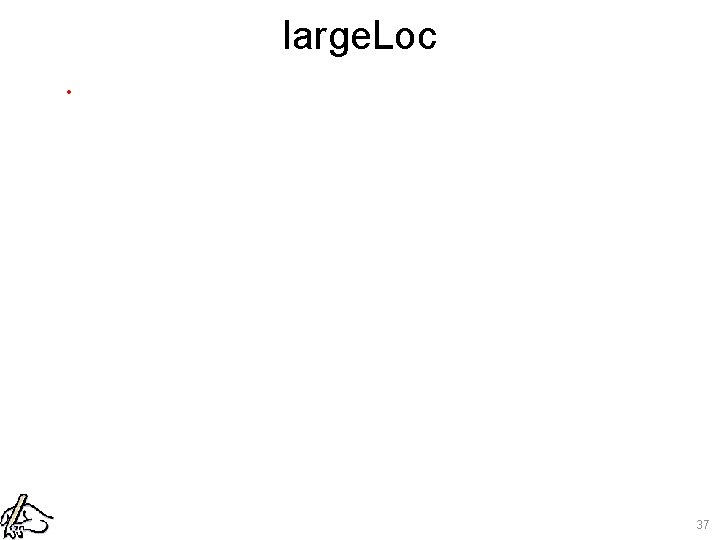
large. Loc. 37
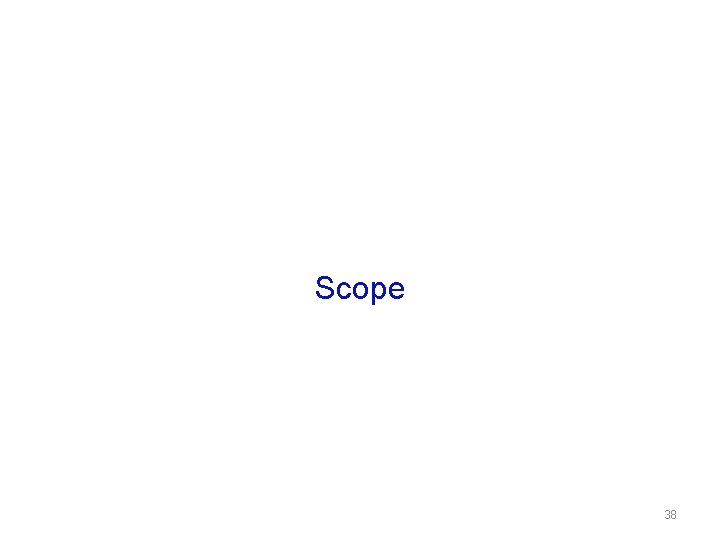
Scope 38
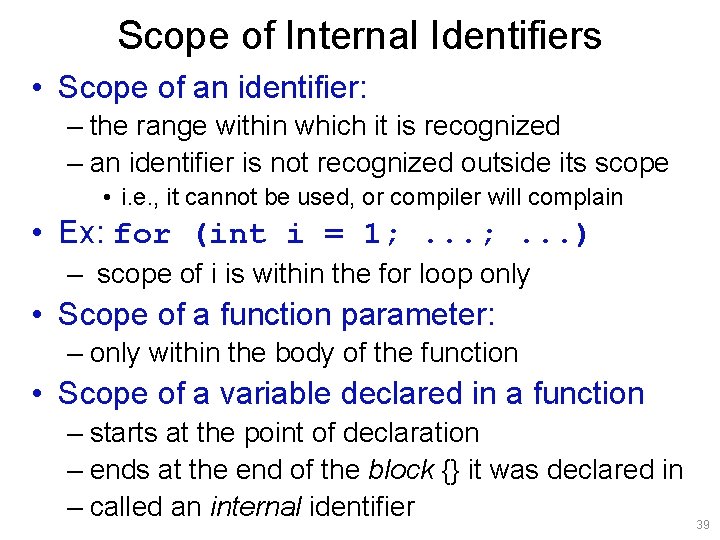
Scope of Internal Identifiers • Scope of an identifier: – the range within which it is recognized – an identifier is not recognized outside its scope • i. e. , it cannot be used, or compiler will complain • Ex: for (int i = 1; . . . ) – scope of i is within the for loop only • Scope of a function parameter: – only within the body of the function • Scope of a variable declared in a function – starts at the point of declaration – ends at the end of the block {} it was declared in – called an internal identifier 39
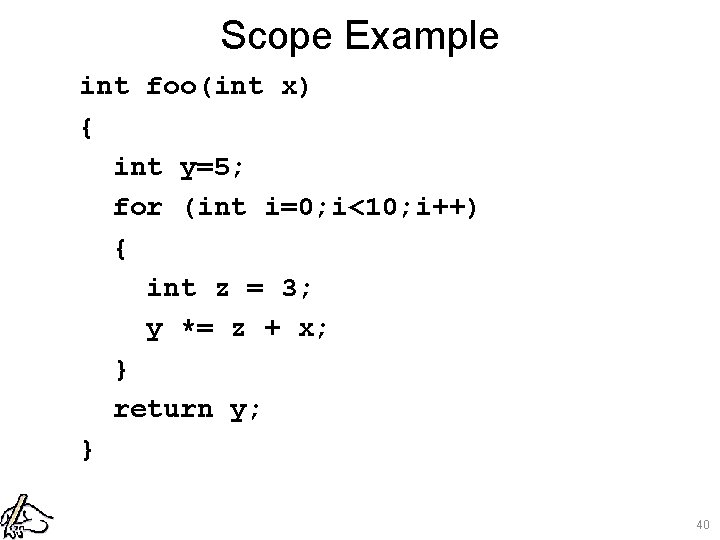
Scope Example int foo(int x) { int y=5; for (int i=0; i<10; i++) { int z = 3; y *= z + x; } return y; } 40
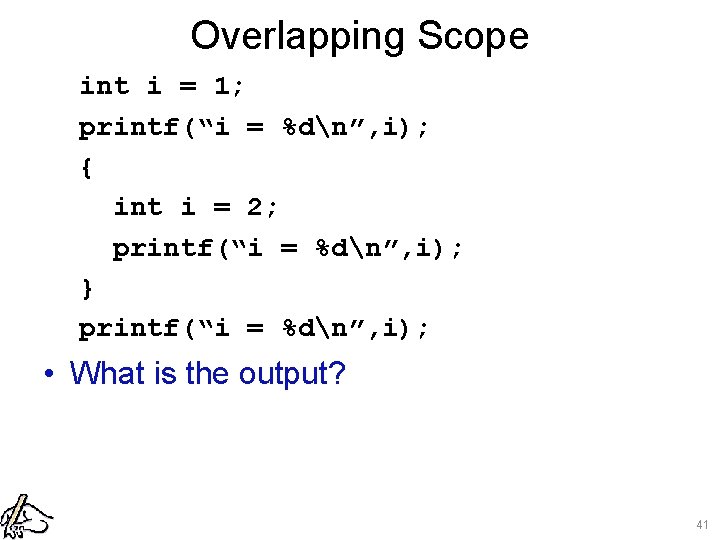
Overlapping Scope int i = 1; printf(“i = %dn”, i); { int i = 2; printf(“i = %dn”, i); } printf(“i = %dn”, i); • What is the output? 41
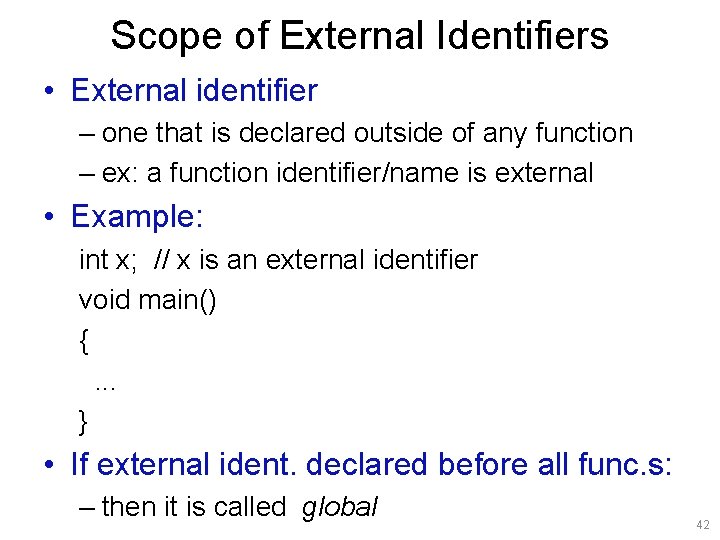
Scope of External Identifiers • External identifier – one that is declared outside of any function – ex: a function identifier/name is external • Example: int x; // x is an external identifier void main() {. . . } • If external ident. declared before all func. s: – then it is called global 42
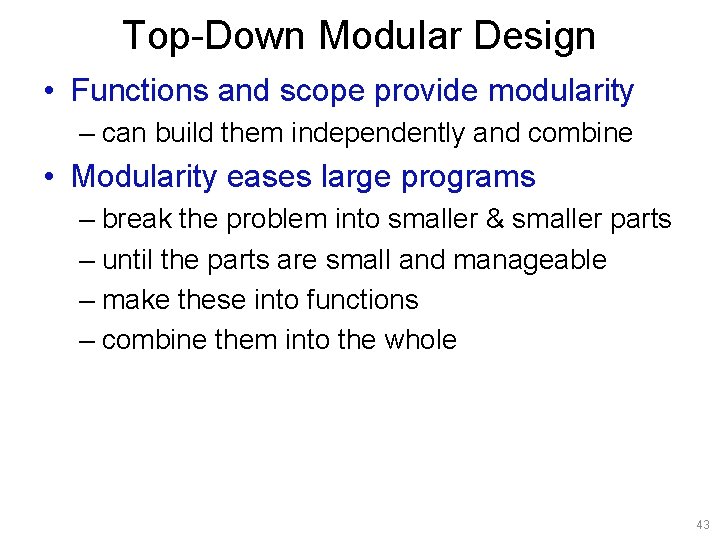
Top-Down Modular Design • Functions and scope provide modularity – can build them independently and combine • Modularity eases large programs – break the problem into smaller & smaller parts – until the parts are small and manageable – make these into functions – combine them into the whole 43
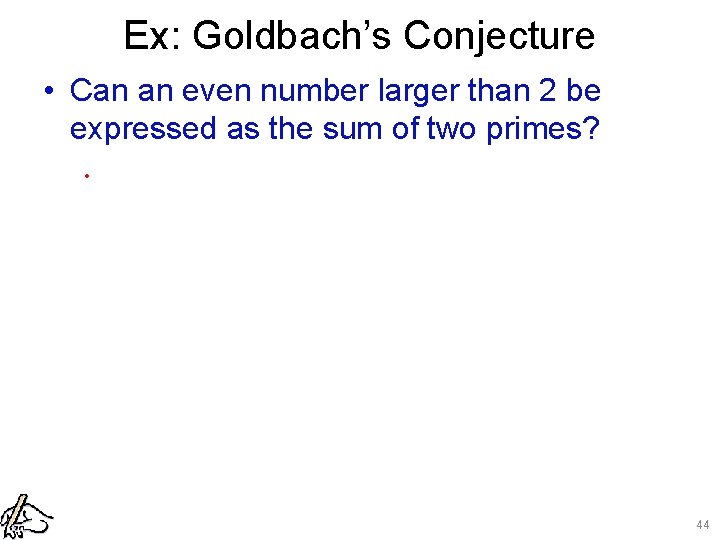
Ex: Goldbach’s Conjecture • Can an even number larger than 2 be expressed as the sum of two primes? . 44
Community detection in networks
Modularity vs efficiency in software testing
Modularity in object oriented programming
Modularity in vlsi design means
Pointers and strings
& vs * in c
Java pointers and references
Aps and gps
Caedb
Significance of pointers in c
C array of pointers to structs
Scale factor of pointer in c
Skip pointers
Skip pointer information retrieval
Which is a good idea for using skip pointers
Explicit pointer
Java pointer to array
Function of inter
Swizzling glsl
Converging pointers algorithm
Hazard pointers
12 pointers
I-need-a-few-pointers-98hpou5
Scientific logbook example
Science logbook example
Pointers are variables that contain
What is the fundamental of pointers?
Pointers are variables that contain as their values
Converging pointers algorithm
Advanced pointers in c
9 pointers
Pointers are variables that contain
Pingo.upb.de
Pointers symbol
Skip pointers
Shuffle left algorithm
Advanced pointers in c
File pointers in c
Pointers in assembly
Tipos de aps
Sacramento county adult protective services
Kipukiila
Aps renovada
Adult protective services san bernardino county
Carrera referencial lineal aps 2022