Announcements l Homework 5 l Due this Wednesday
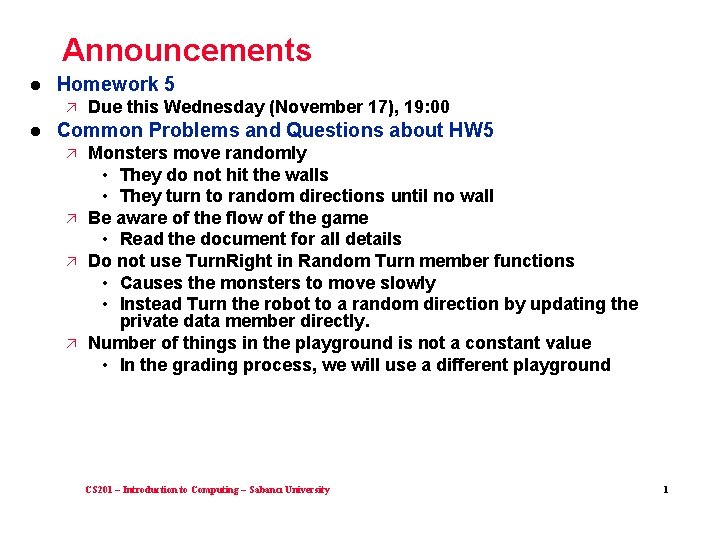
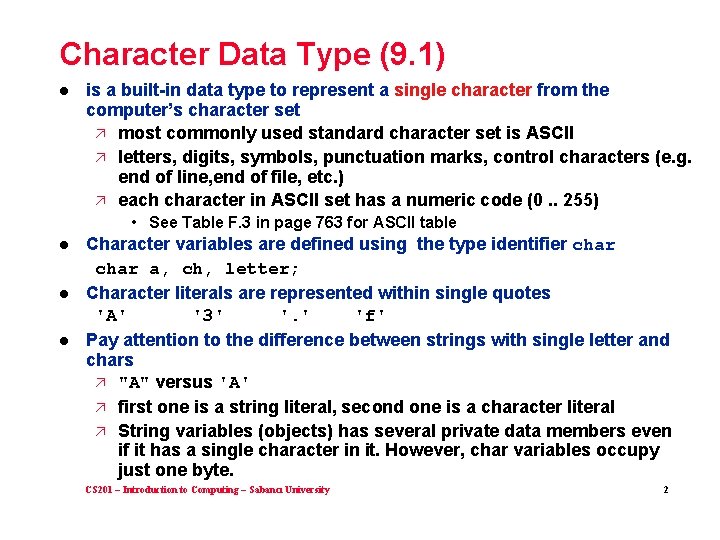
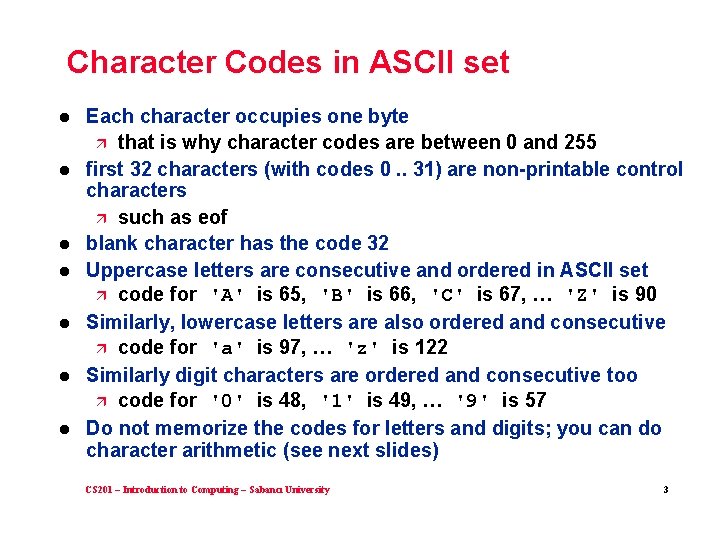
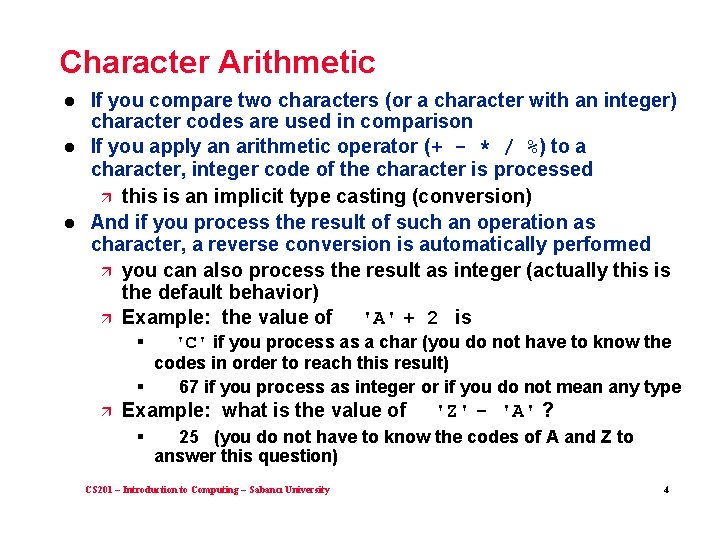
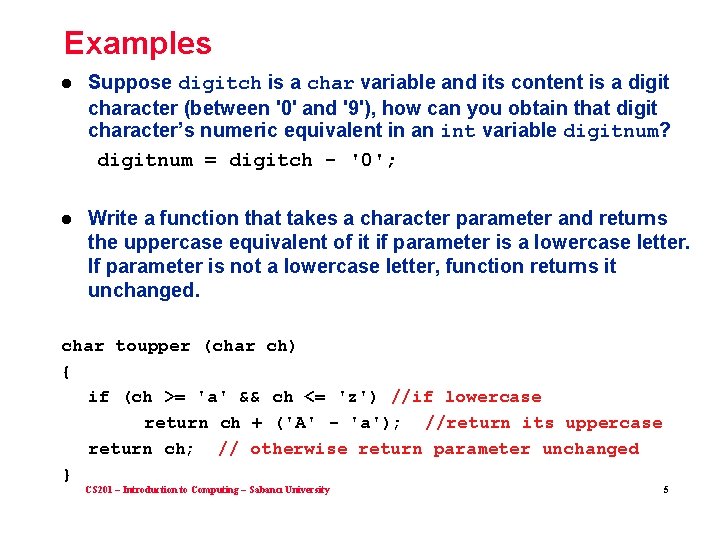
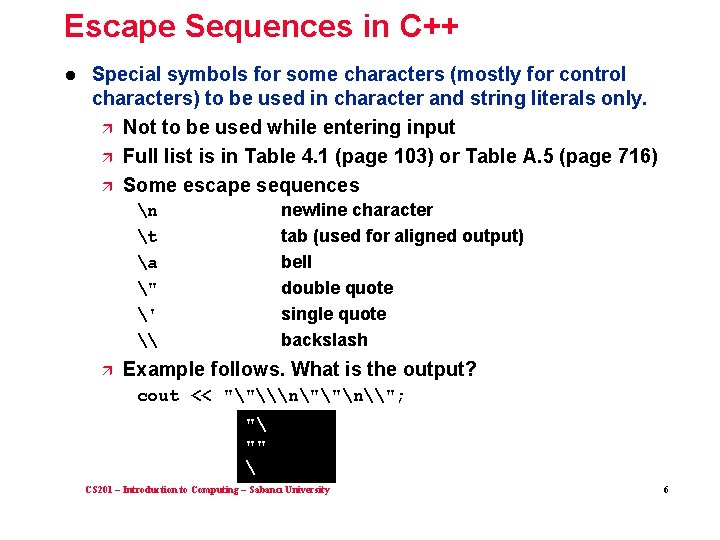
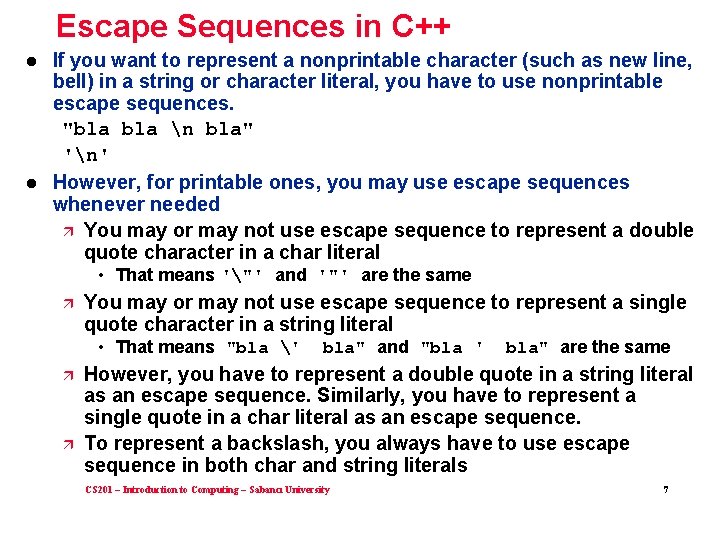
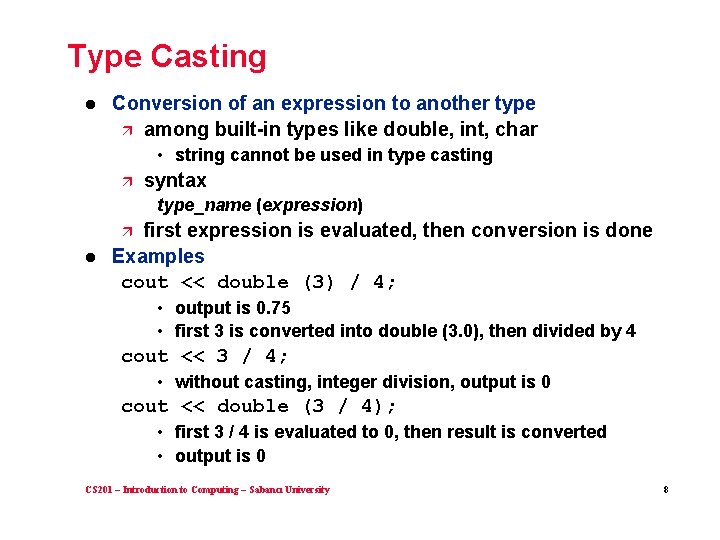
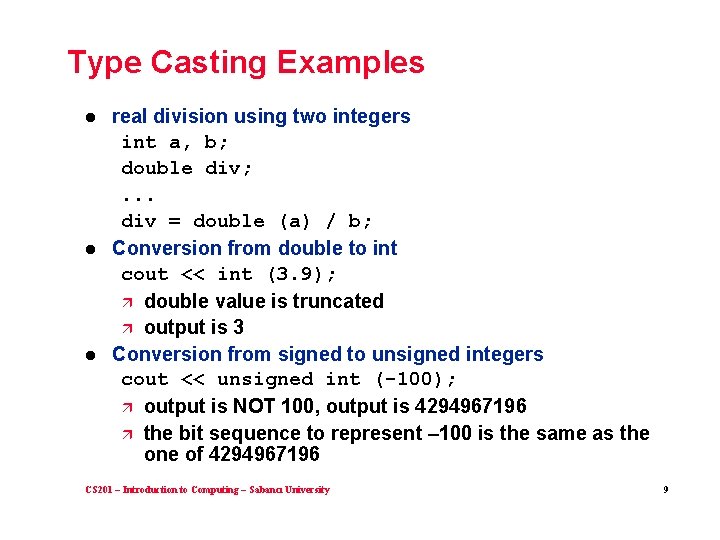
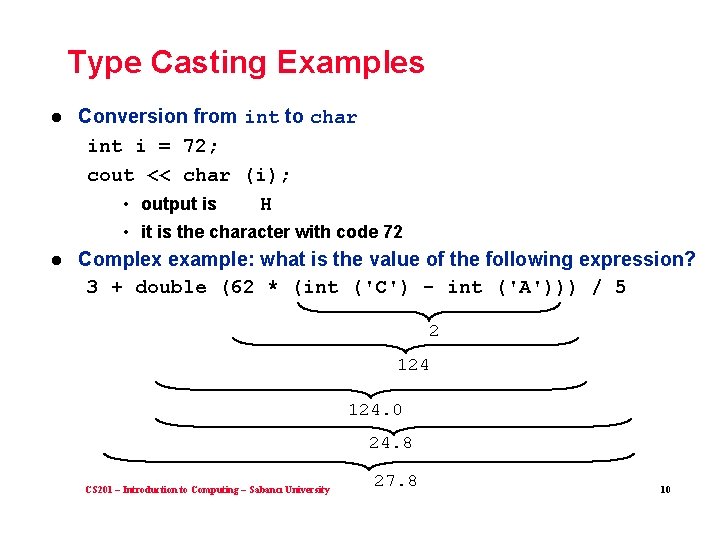
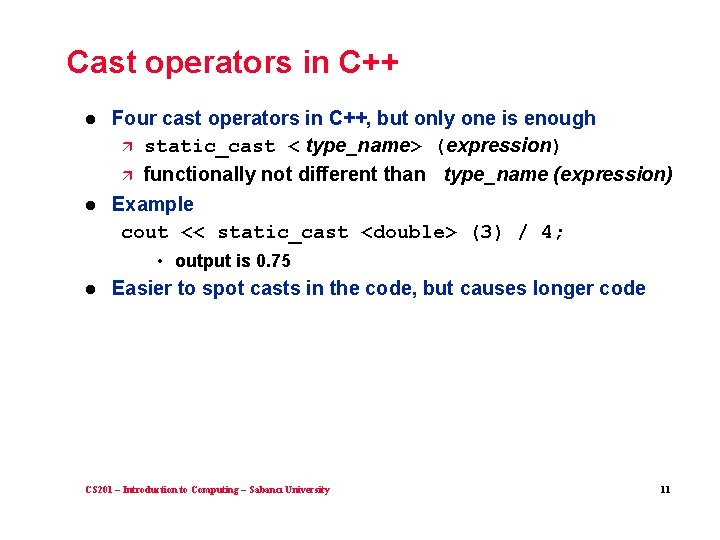
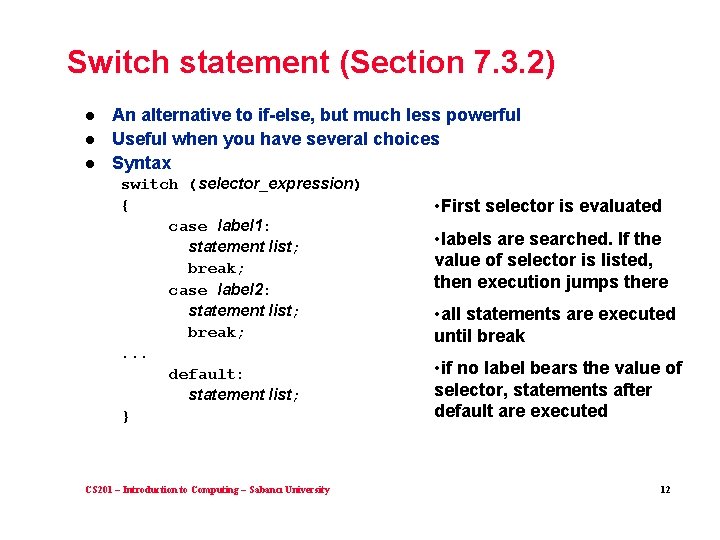
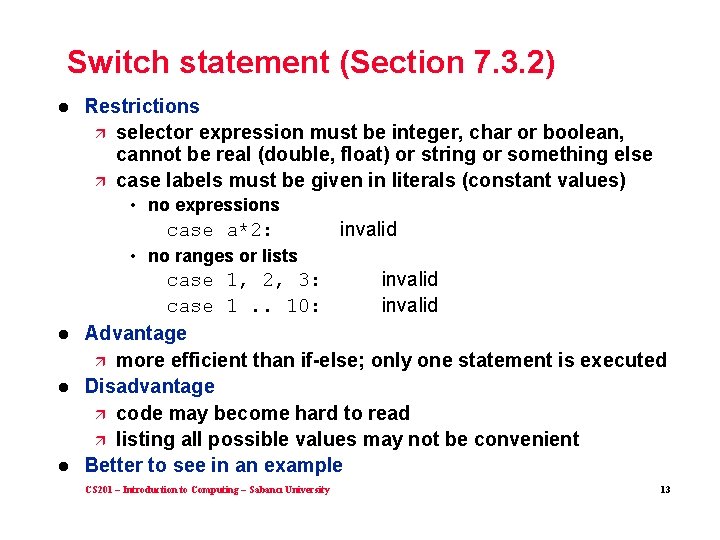
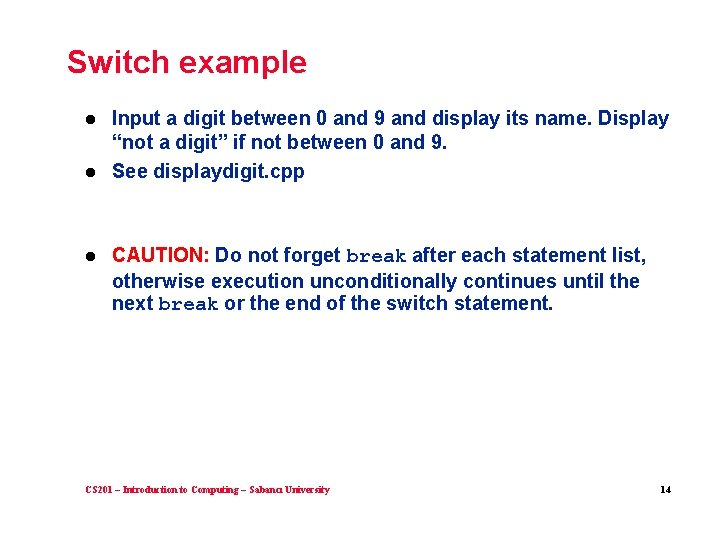
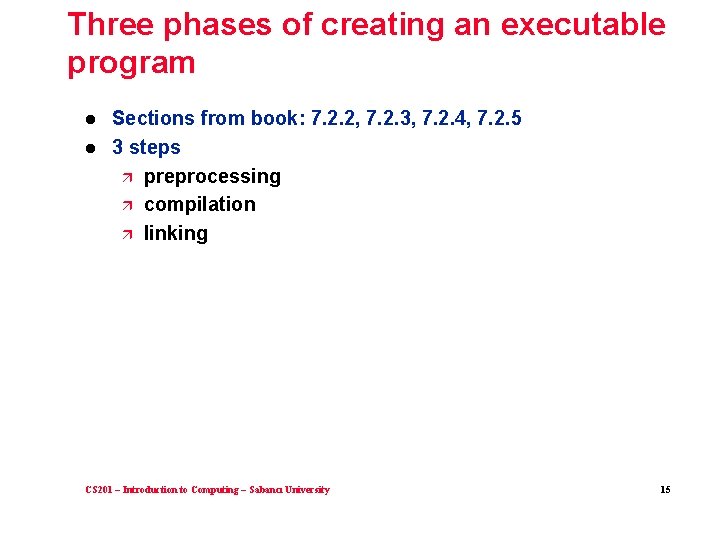
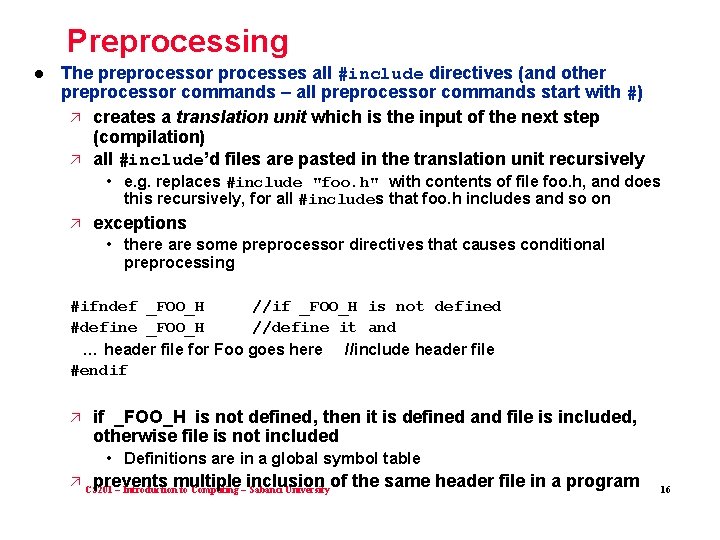
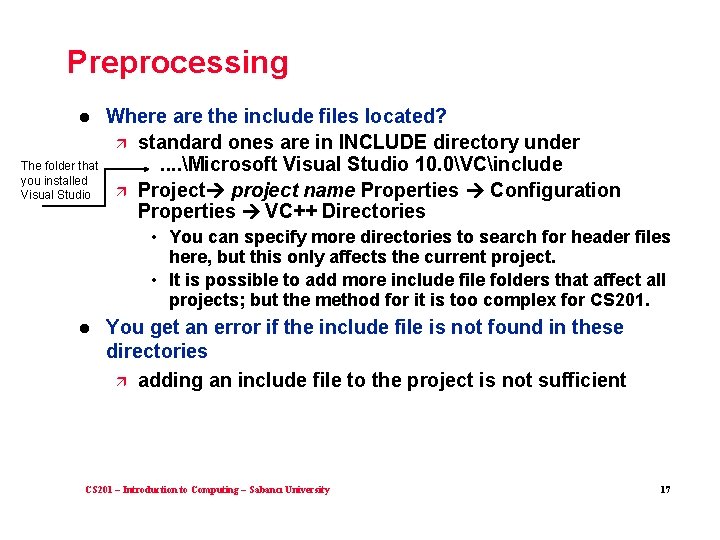
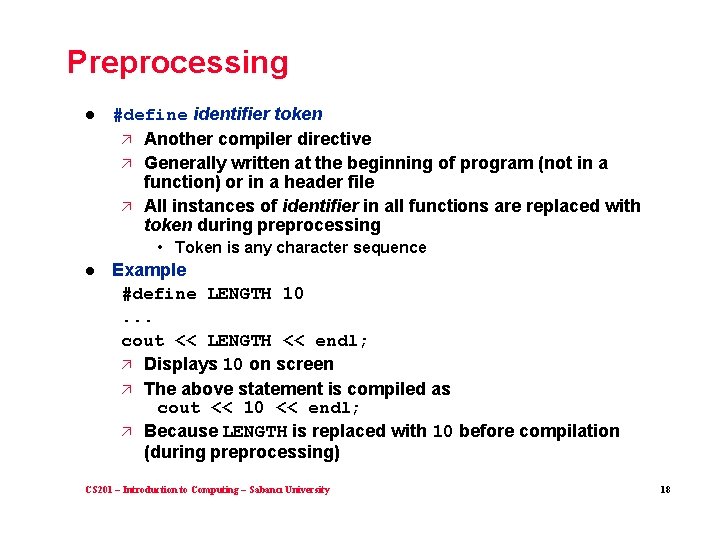
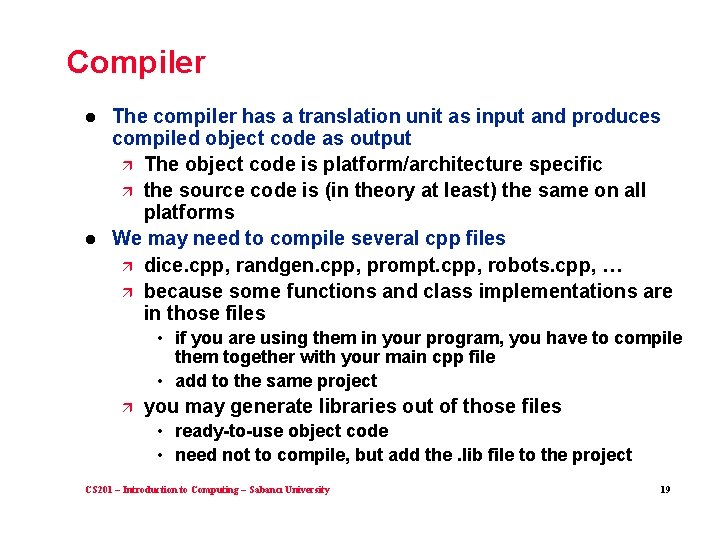
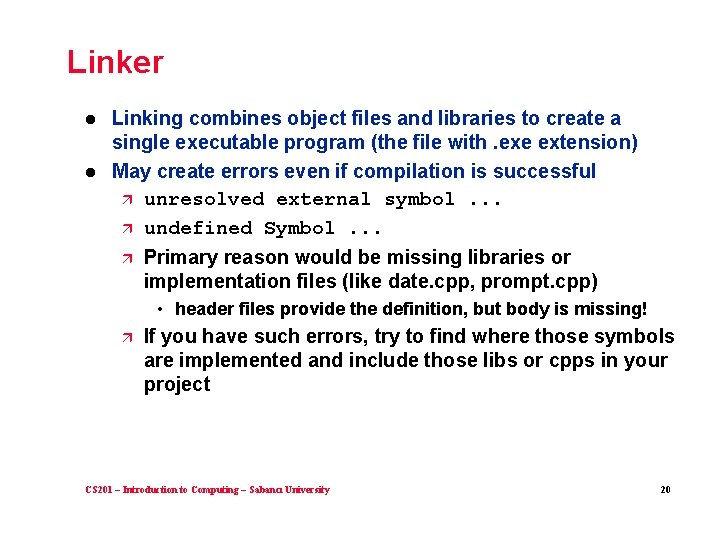
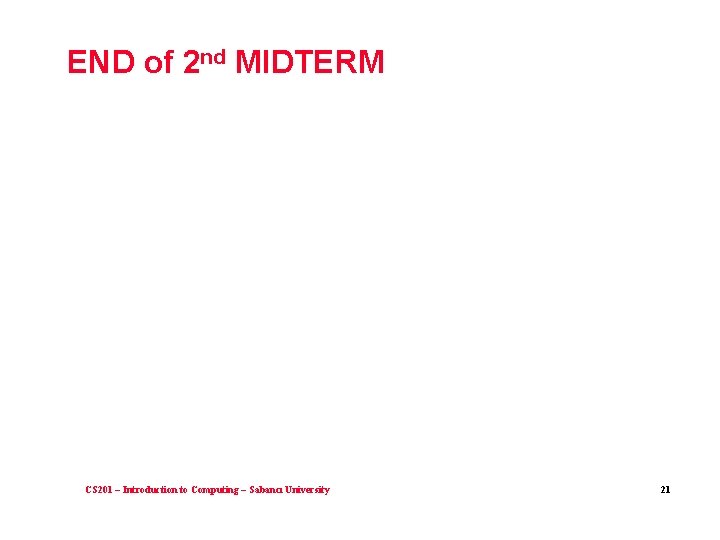
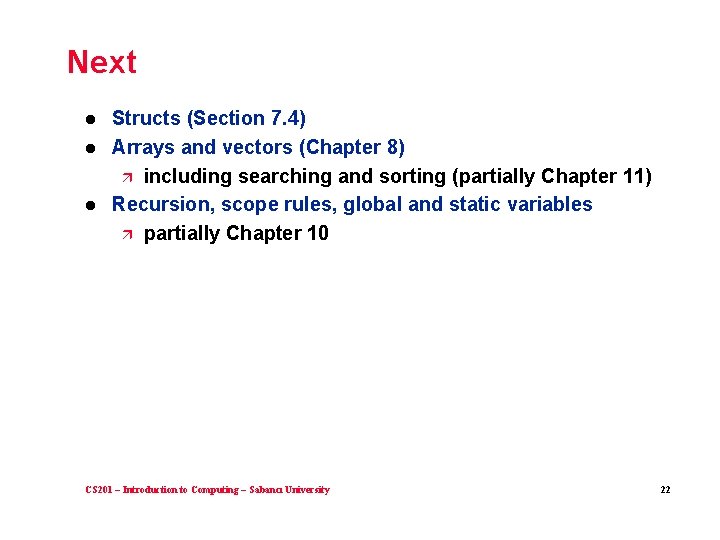
- Slides: 22
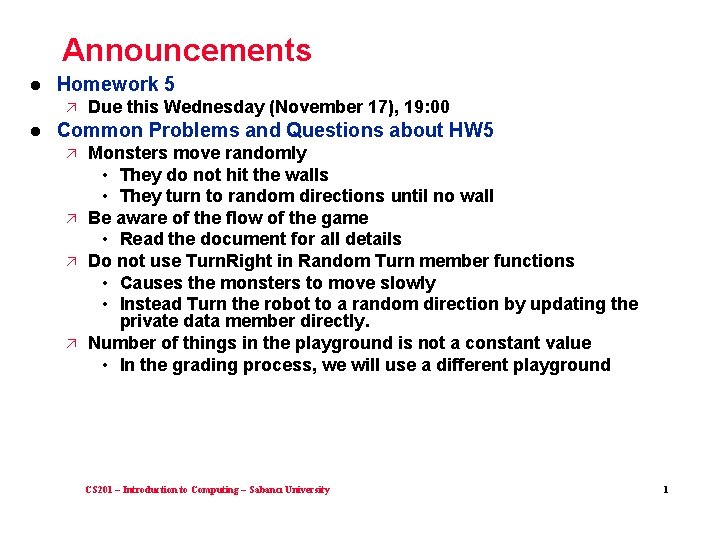
Announcements l Homework 5 ä l Due this Wednesday (November 17), 19: 00 Common Problems and Questions about HW 5 ä ä Monsters move randomly • They do not hit the walls • They turn to random directions until no wall Be aware of the flow of the game • Read the document for all details Do not use Turn. Right in Random Turn member functions • Causes the monsters to move slowly • Instead Turn the robot to a random direction by updating the private data member directly. Number of things in the playground is not a constant value • In the grading process, we will use a different playground CS 201 – Introduction to Computing – Sabancı University 1
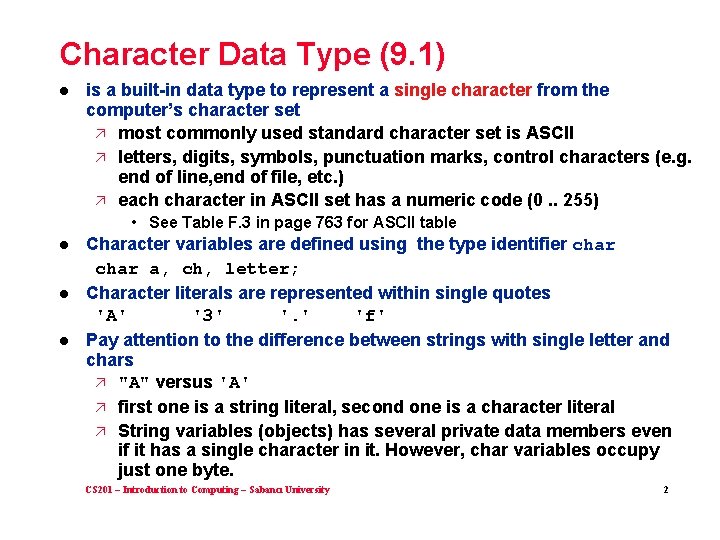
Character Data Type (9. 1) l is a built-in data type to represent a single character from the computer’s character set ä most commonly used standard character set is ASCII ä letters, digits, symbols, punctuation marks, control characters (e. g. end of line, end of file, etc. ) ä each character in ASCII set has a numeric code (0. . 255) • See Table F. 3 in page 763 for ASCII table l l l Character variables are defined using the type identifier char a, ch, letter; Character literals are represented within single quotes 'A' '3' '. ' 'f' Pay attention to the difference between strings with single letter and chars ä "A" versus 'A' ä first one is a string literal, second one is a character literal ä String variables (objects) has several private data members even if it has a single character in it. However, char variables occupy just one byte. CS 201 – Introduction to Computing – Sabancı University 2
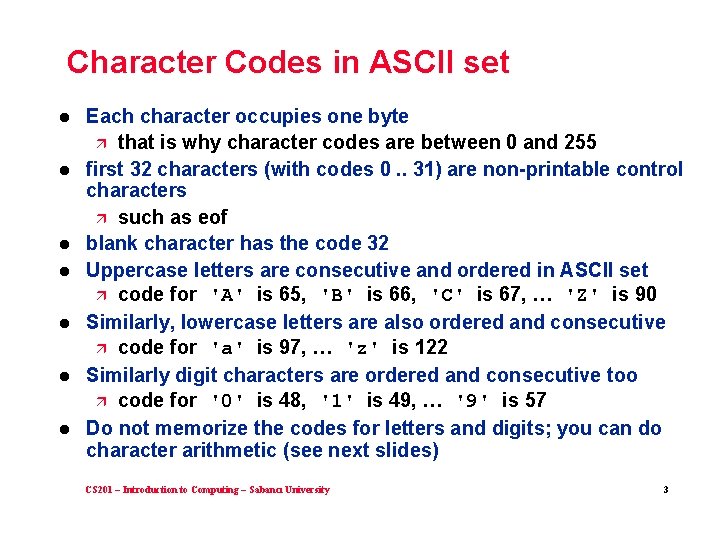
Character Codes in ASCII set l l l l Each character occupies one byte ä that is why character codes are between 0 and 255 first 32 characters (with codes 0. . 31) are non-printable control characters ä such as eof blank character has the code 32 Uppercase letters are consecutive and ordered in ASCII set ä code for 'A' is 65, 'B' is 66, 'C' is 67, … 'Z' is 90 Similarly, lowercase letters are also ordered and consecutive ä code for 'a' is 97, … 'z' is 122 Similarly digit characters are ordered and consecutive too ä code for '0' is 48, '1' is 49, … '9' is 57 Do not memorize the codes for letters and digits; you can do character arithmetic (see next slides) CS 201 – Introduction to Computing – Sabancı University 3
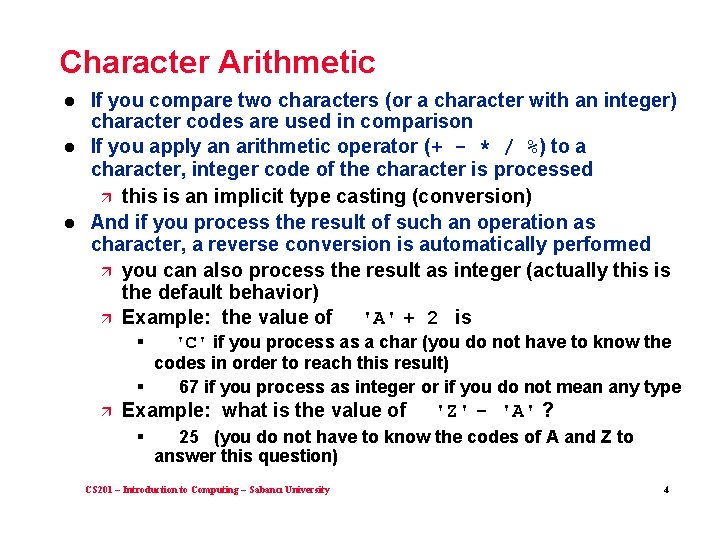
Character Arithmetic l l l If you compare two characters (or a character with an integer) character codes are used in comparison If you apply an arithmetic operator (+ - * / %) to a character, integer code of the character is processed ä this is an implicit type casting (conversion) And if you process the result of such an operation as character, a reverse conversion is automatically performed ä you can also process the result as integer (actually this is the default behavior) ä Example: the value of 'A' + 2 is § 'C' if you process as a char (you do not have to know the codes in order to reach this result) § 67 if you process as integer or if you do not mean any type ä Example: what is the value of § 'Z' - 'A' ? 25 (you do not have to know the codes of A and Z to answer this question) CS 201 – Introduction to Computing – Sabancı University 4
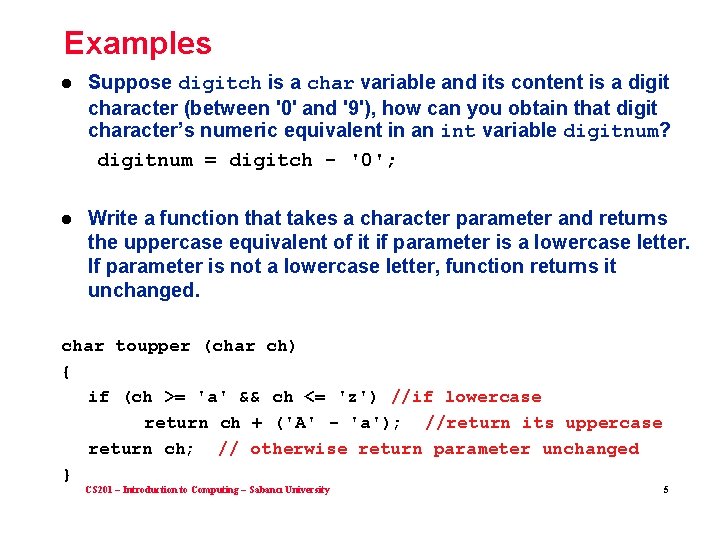
Examples l Suppose digitch is a char variable and its content is a digit character (between '0' and '9'), how can you obtain that digit character’s numeric equivalent in an int variable digitnum? digitnum = digitch - '0'; l Write a function that takes a character parameter and returns the uppercase equivalent of it if parameter is a lowercase letter. If parameter is not a lowercase letter, function returns it unchanged. char toupper (char ch) { if (ch >= 'a' && ch <= 'z') //if lowercase return ch + ('A' - 'a'); //return its uppercase return ch; // otherwise return parameter unchanged } CS 201 – Introduction to Computing – Sabancı University 5
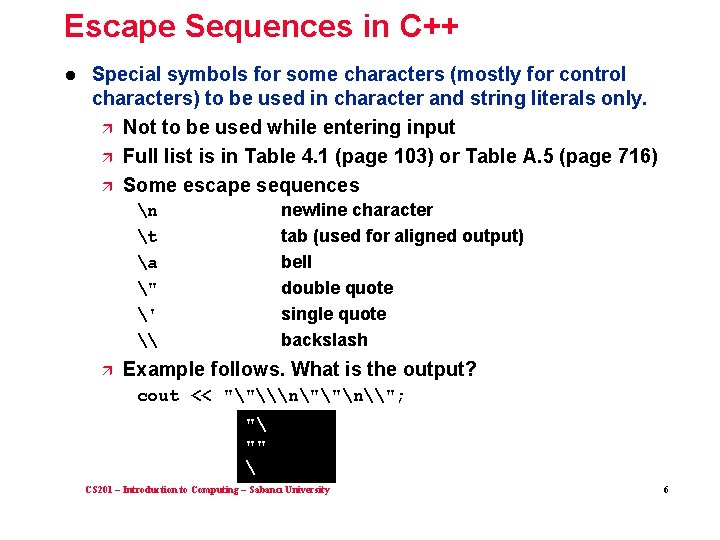
Escape Sequences in C++ l Special symbols for some characters (mostly for control characters) to be used in character and string literals only. ä Not to be used while entering input ä Full list is in Table 4. 1 (page 103) or Table A. 5 (page 716) ä Some escape sequences newline character tab (used for aligned output) bell double quote single quote backslash n t a " ' \ ä Example follows. What is the output? cout << ""\n""n\"; " "" CS 201 – Introduction to Computing – Sabancı University 6
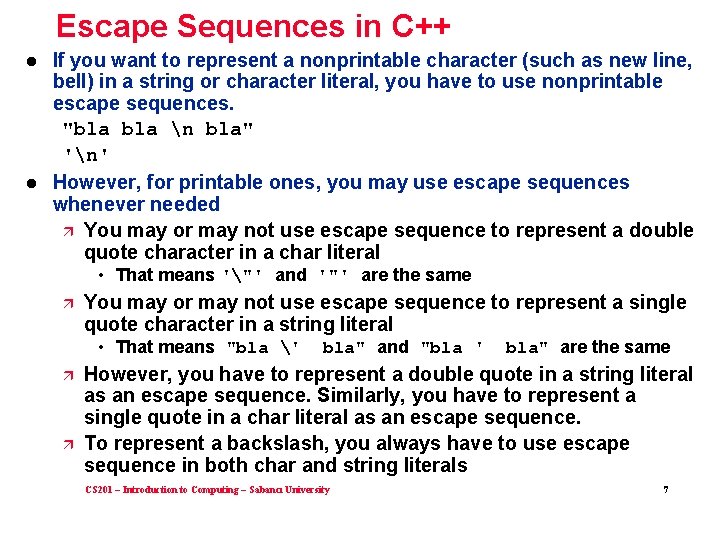
Escape Sequences in C++ l l If you want to represent a nonprintable character (such as new line, bell) in a string or character literal, you have to use nonprintable escape sequences. "bla n bla" 'n' However, for printable ones, you may use escape sequences whenever needed ä You may or may not use escape sequence to represent a double quote character in a char literal • That means '"' and '"' are the same ä You may or may not use escape sequence to represent a single quote character in a string literal • That means "bla ' ä ä bla" and "bla ' bla" are the same However, you have to represent a double quote in a string literal as an escape sequence. Similarly, you have to represent a single quote in a char literal as an escape sequence. To represent a backslash, you always have to use escape sequence in both char and string literals CS 201 – Introduction to Computing – Sabancı University 7
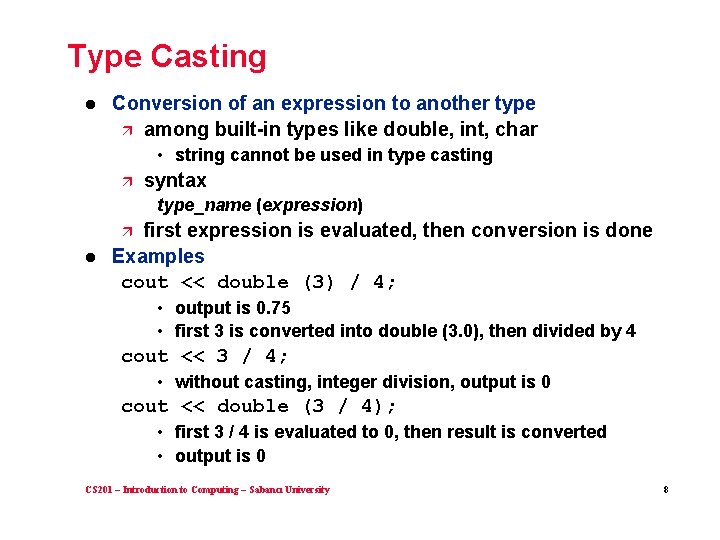
Type Casting l Conversion of an expression to another type ä among built-in types like double, int, char • string cannot be used in type casting ä syntax type_name (expression) first expression is evaluated, then conversion is done Examples cout << double (3) / 4; ä l • output is 0. 75 • first 3 is converted into double (3. 0), then divided by 4 cout << 3 / 4; • without casting, integer division, output is 0 cout << double (3 / 4); • first 3 / 4 is evaluated to 0, then result is converted • output is 0 CS 201 – Introduction to Computing – Sabancı University 8
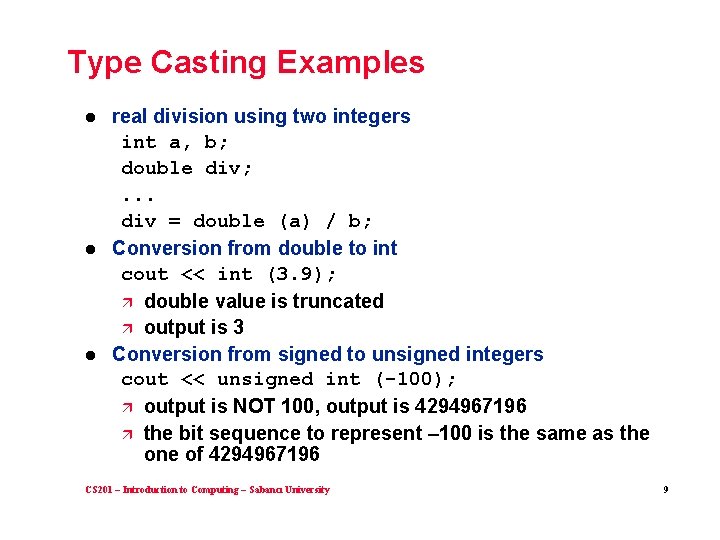
Type Casting Examples l l l real division using two integers int a, b; double div; . . . div = double (a) / b; Conversion from double to int cout << int (3. 9); ä double value is truncated ä output is 3 Conversion from signed to unsigned integers cout << unsigned int (-100); ä output is NOT 100, output is 4294967196 ä the bit sequence to represent – 100 is the same as the one of 4294967196 CS 201 – Introduction to Computing – Sabancı University 9
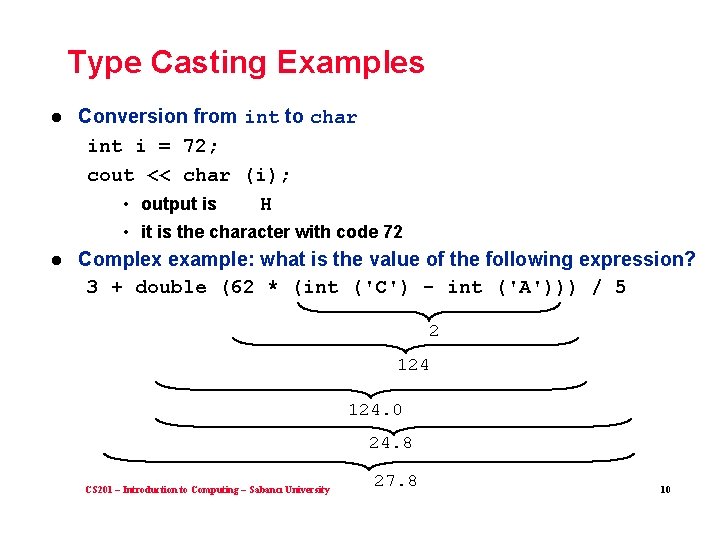
Type Casting Examples l Conversion from int to char int i = 72; cout << char (i); • output is H • it is the character with code 72 l Complex example: what is the value of the following expression? 3 + double (62 * (int ('C') - int ('A'))) / 5 2 124. 0 24. 8 CS 201 – Introduction to Computing – Sabancı University 27. 8 10
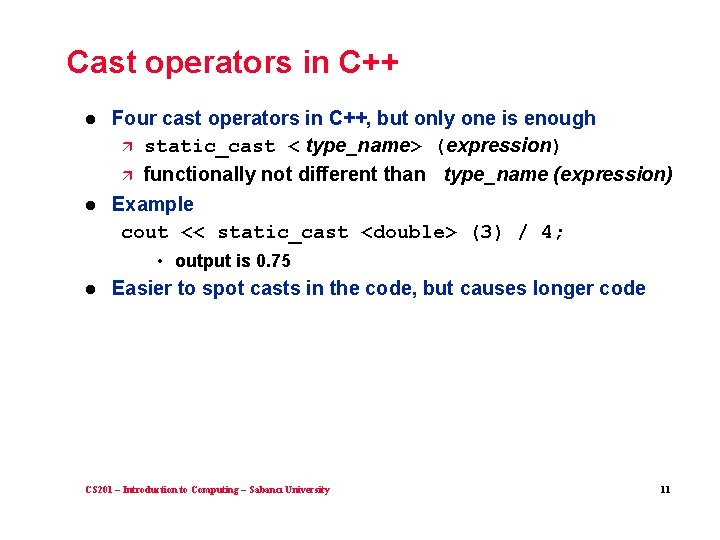
Cast operators in C++ l Four cast operators in C++, but only one is enough ä static_cast < type_name> (expression) ä functionally not different than type_name (expression) l Example cout << static_cast <double> (3) / 4; • output is 0. 75 l Easier to spot casts in the code, but causes longer code CS 201 – Introduction to Computing – Sabancı University 11
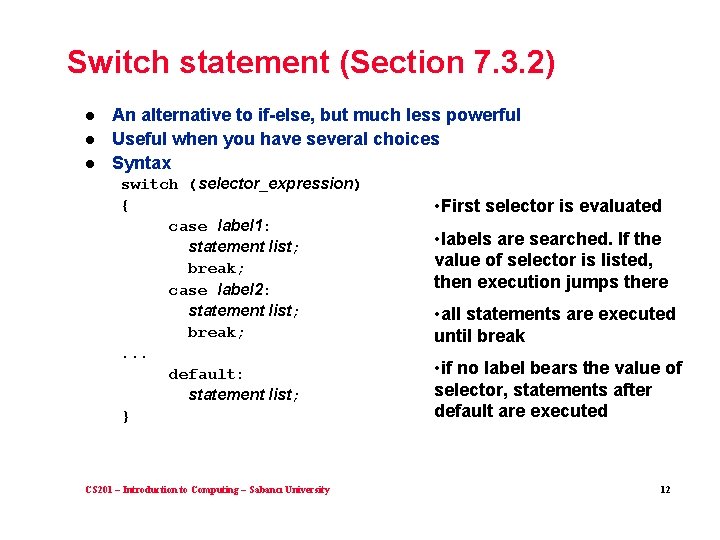
Switch statement (Section 7. 3. 2) l l l An alternative to if-else, but much less powerful Useful when you have several choices Syntax switch (selector_expression) { case label 1: statement list; break; case label 2: statement list; break; . . . default: statement list; } CS 201 – Introduction to Computing – Sabancı University • First selector is evaluated • labels are searched. If the value of selector is listed, then execution jumps there • all statements are executed until break • if no label bears the value of selector, statements after default are executed 12
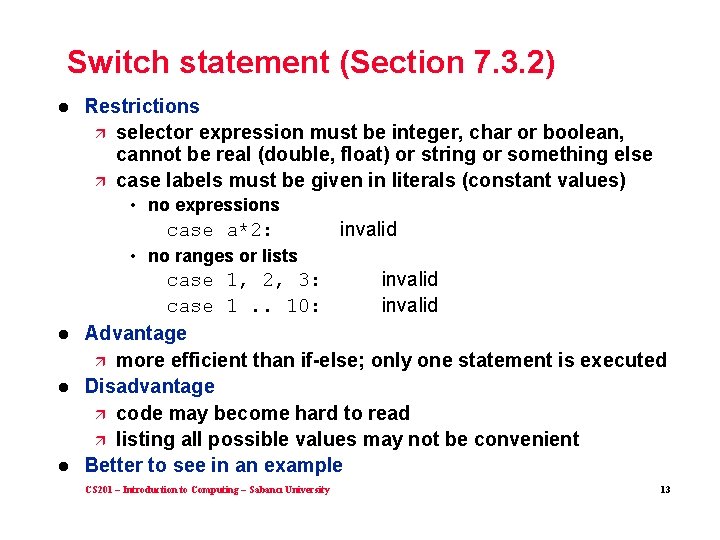
Switch statement (Section 7. 3. 2) l Restrictions ä selector expression must be integer, char or boolean, cannot be real (double, float) or string or something else ä case labels must be given in literals (constant values) • no expressions case a*2: invalid • no ranges or lists l l l case 1, 2, 3: invalid case 1. . 10: invalid Advantage ä more efficient than if-else; only one statement is executed Disadvantage ä code may become hard to read ä listing all possible values may not be convenient Better to see in an example CS 201 – Introduction to Computing – Sabancı University 13
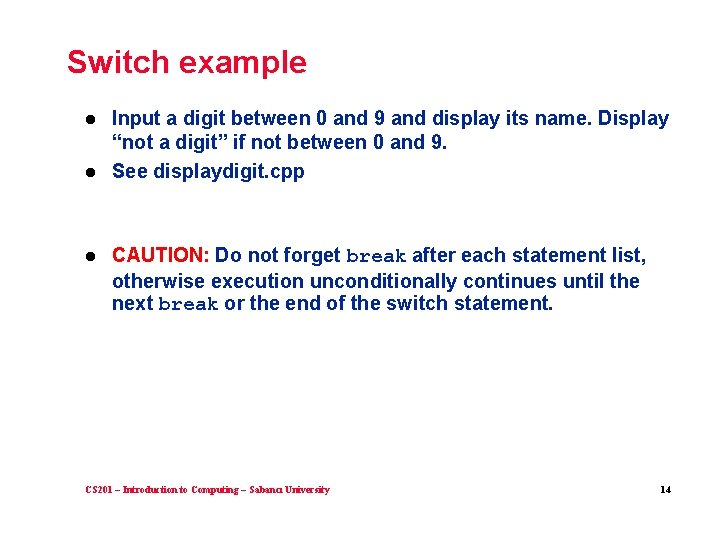
Switch example l l l Input a digit between 0 and 9 and display its name. Display “not a digit” if not between 0 and 9. See displaydigit. cpp CAUTION: Do not forget break after each statement list, otherwise execution unconditionally continues until the next break or the end of the switch statement. CS 201 – Introduction to Computing – Sabancı University 14
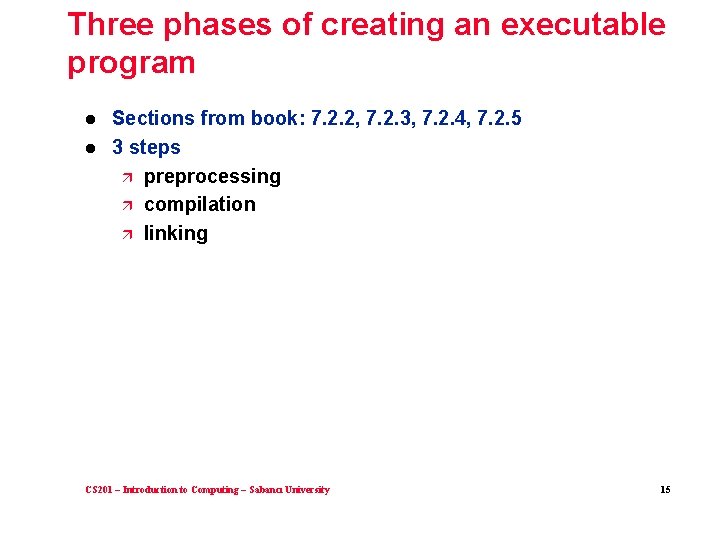
Three phases of creating an executable program l l Sections from book: 7. 2. 2, 7. 2. 3, 7. 2. 4, 7. 2. 5 3 steps ä preprocessing ä compilation ä linking CS 201 – Introduction to Computing – Sabancı University 15
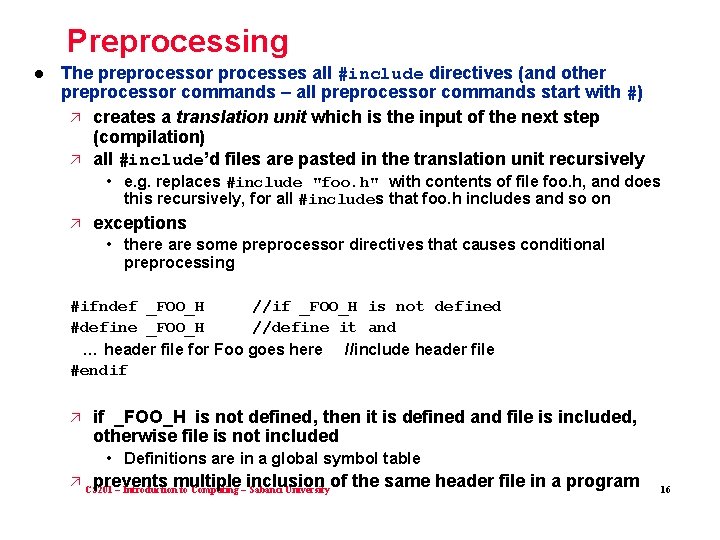
Preprocessing l The preprocessor processes all #include directives (and other preprocessor commands – all preprocessor commands start with #) ä creates a translation unit which is the input of the next step (compilation) ä all #include’d files are pasted in the translation unit recursively • e. g. replaces #include "foo. h" with contents of file foo. h, and does this recursively, for all #includes that foo. h includes and so on ä exceptions • there are some preprocessor directives that causes conditional preprocessing #ifndef _FOO_H //if _FOO_H is not defined #define _FOO_H //define it and … header file for Foo goes here //include header file #endif ä if _FOO_H is not defined, then it is defined and file is included, otherwise file is not included • Definitions are in a global symbol table ä CS 201 prevents multiple inclusion of – Introduction to Computing – Sabancı University the same header file in a program 16
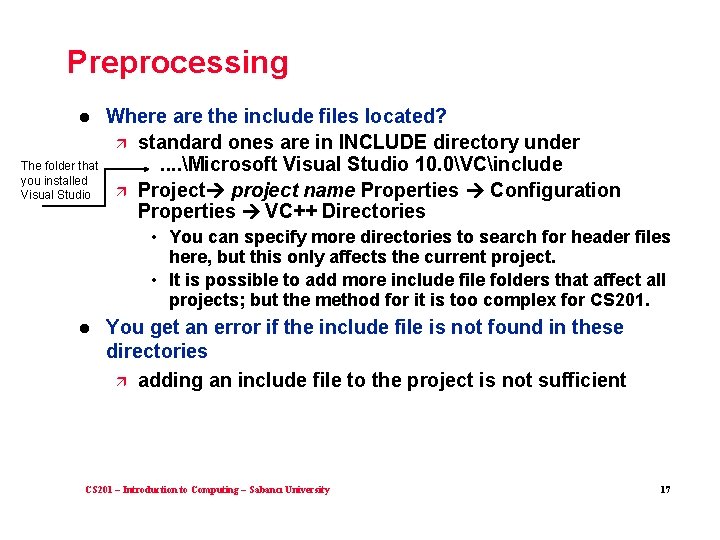
Preprocessing l The folder that you installed Visual Studio Where are the include files located? ä standard ones are in INCLUDE directory under. . Microsoft Visual Studio 10. 0VCinclude ä Project project name Properties Configuration Properties VC++ Directories • You can specify more directories to search for header files here, but this only affects the current project. • It is possible to add more include file folders that affect all projects; but the method for it is too complex for CS 201. l You get an error if the include file is not found in these directories ä adding an include file to the project is not sufficient CS 201 – Introduction to Computing – Sabancı University 17
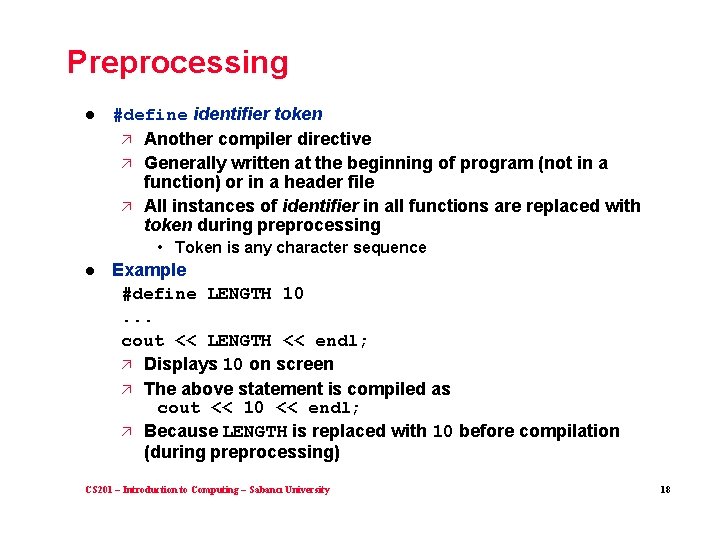
Preprocessing l #define identifier token ä Another compiler directive ä Generally written at the beginning of program (not in a function) or in a header file ä All instances of identifier in all functions are replaced with token during preprocessing • Token is any character sequence l Example #define LENGTH 10. . . cout << LENGTH << endl; ä Displays 10 on screen ä The above statement is compiled as cout << 10 << endl; ä Because LENGTH is replaced with 10 before compilation (during preprocessing) CS 201 – Introduction to Computing – Sabancı University 18
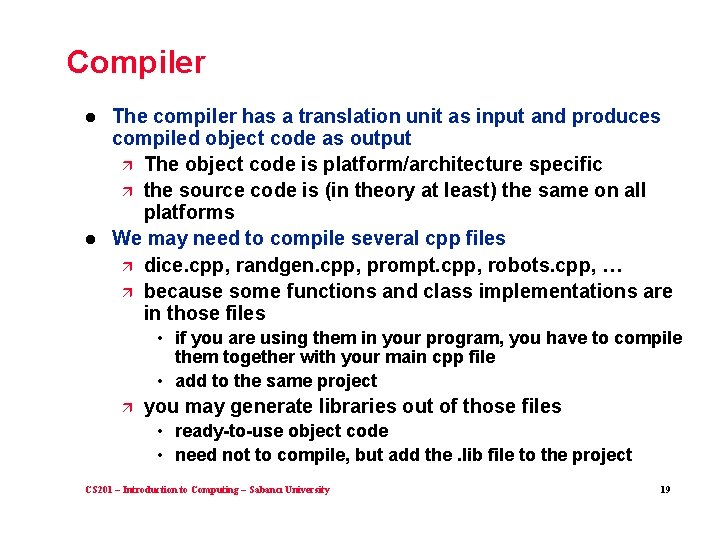
Compiler l l The compiler has a translation unit as input and produces compiled object code as output ä The object code is platform/architecture specific ä the source code is (in theory at least) the same on all platforms We may need to compile several cpp files ä dice. cpp, randgen. cpp, prompt. cpp, robots. cpp, … ä because some functions and class implementations are in those files • if you are using them in your program, you have to compile them together with your main cpp file • add to the same project ä you may generate libraries out of those files • ready-to-use object code • need not to compile, but add the. lib file to the project CS 201 – Introduction to Computing – Sabancı University 19
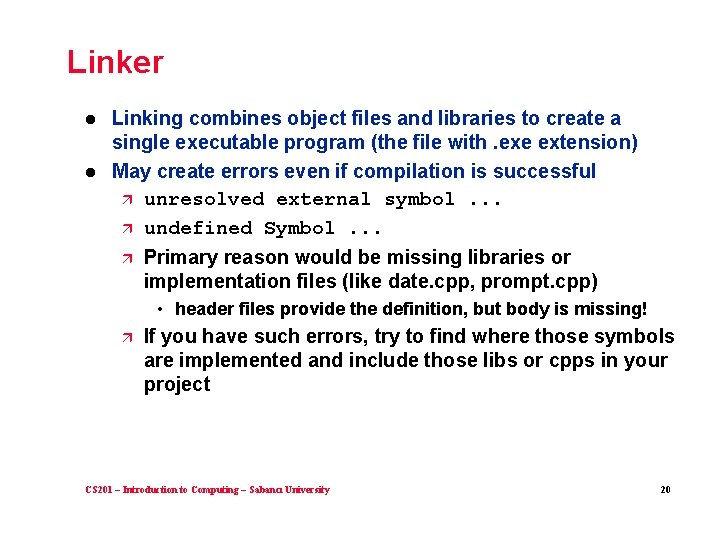
Linker l l Linking combines object files and libraries to create a single executable program (the file with. exe extension) May create errors even if compilation is successful ä unresolved external symbol. . . ä undefined Symbol. . . ä Primary reason would be missing libraries or implementation files (like date. cpp, prompt. cpp) • header files provide the definition, but body is missing! ä If you have such errors, try to find where those symbols are implemented and include those libs or cpps in your project CS 201 – Introduction to Computing – Sabancı University 20
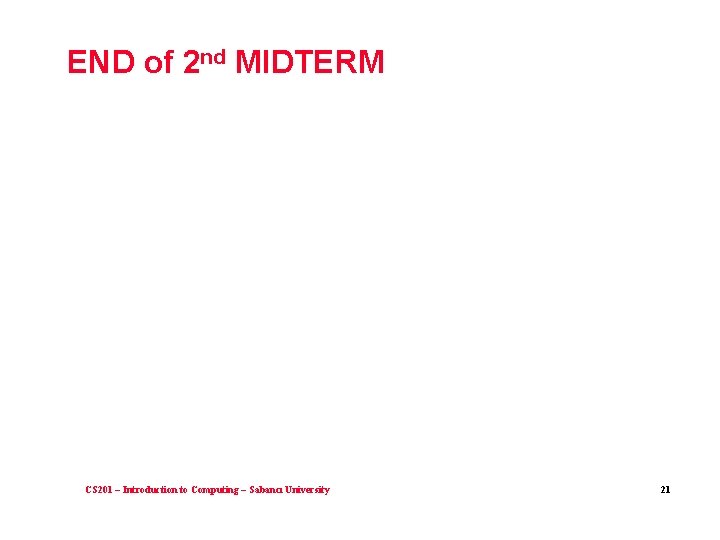
END of 2 nd MIDTERM CS 201 – Introduction to Computing – Sabancı University 21
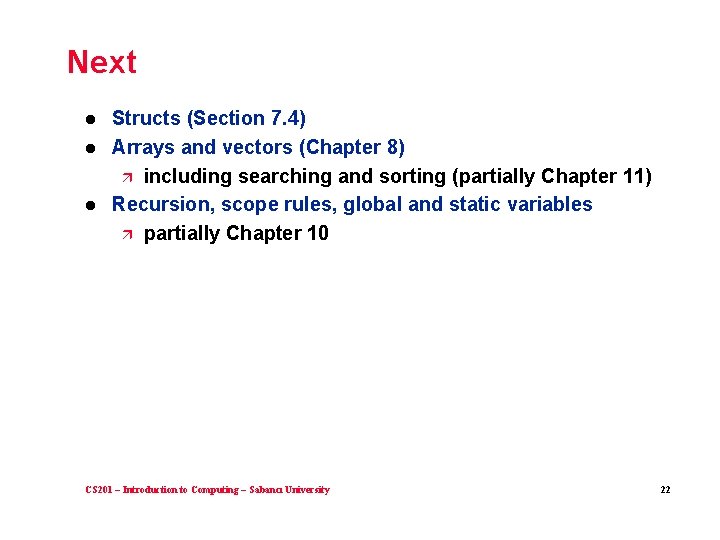
Next l l l Structs (Section 7. 4) Arrays and vectors (Chapter 8) ä including searching and sorting (partially Chapter 11) Recursion, scope rules, global and static variables ä partially Chapter 10 CS 201 – Introduction to Computing – Sabancı University 22