ANDROID VIEWMODEL Peter LarssonGreen Jnkping University Spring 2021
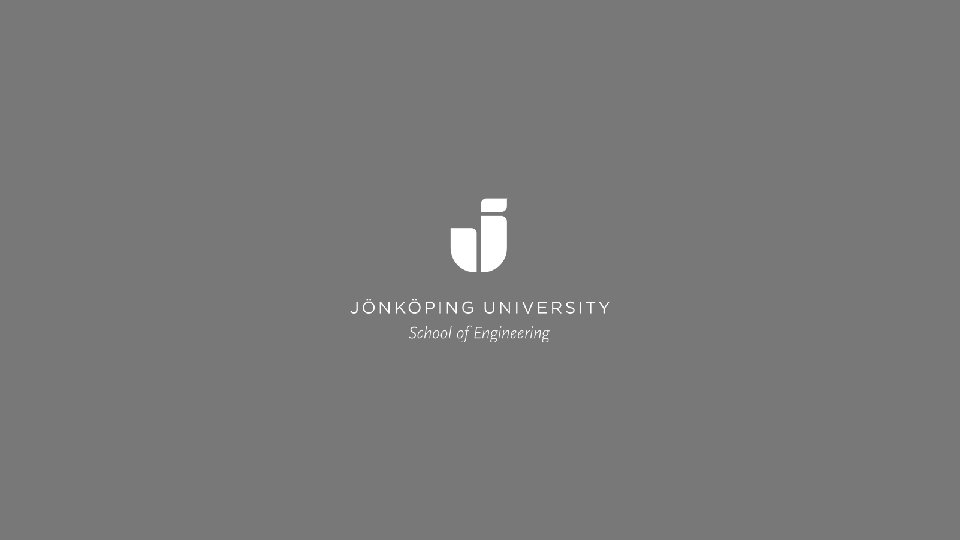
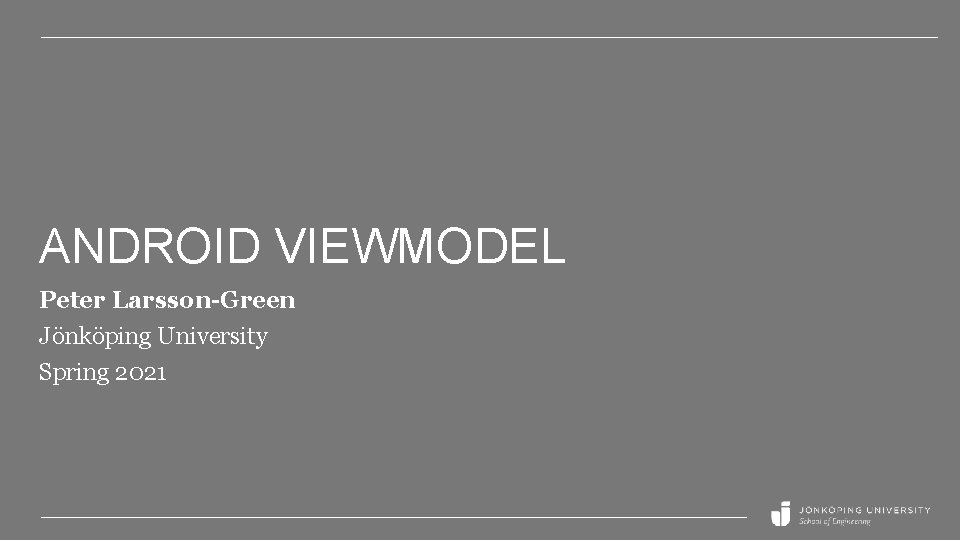
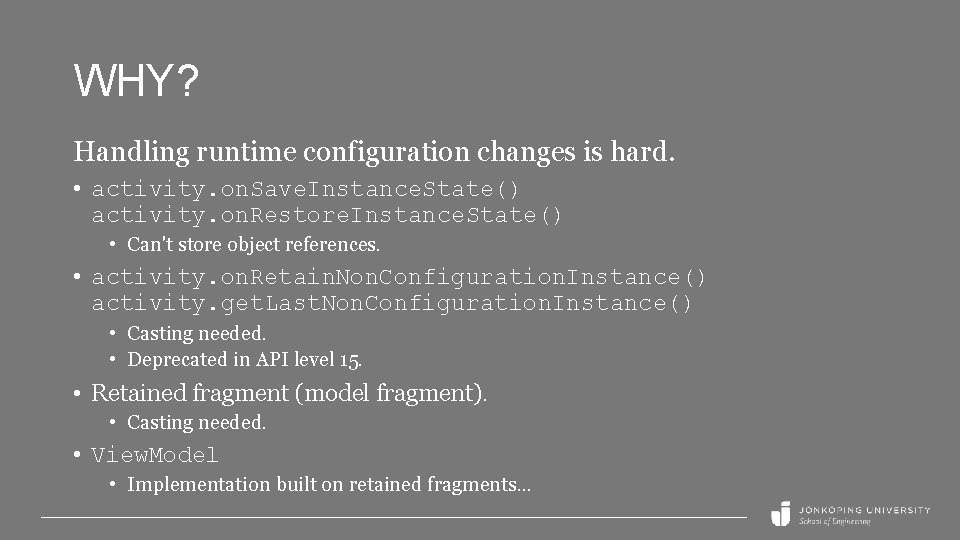
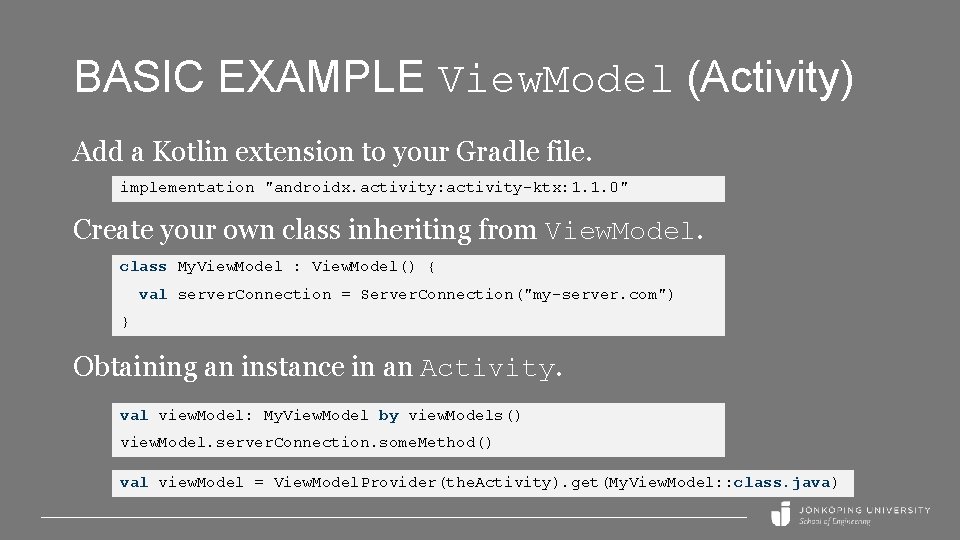
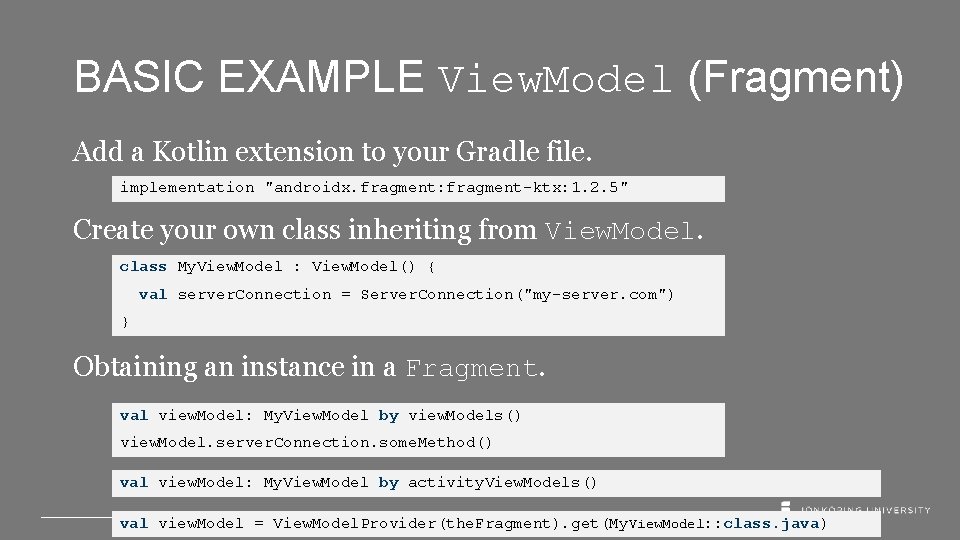
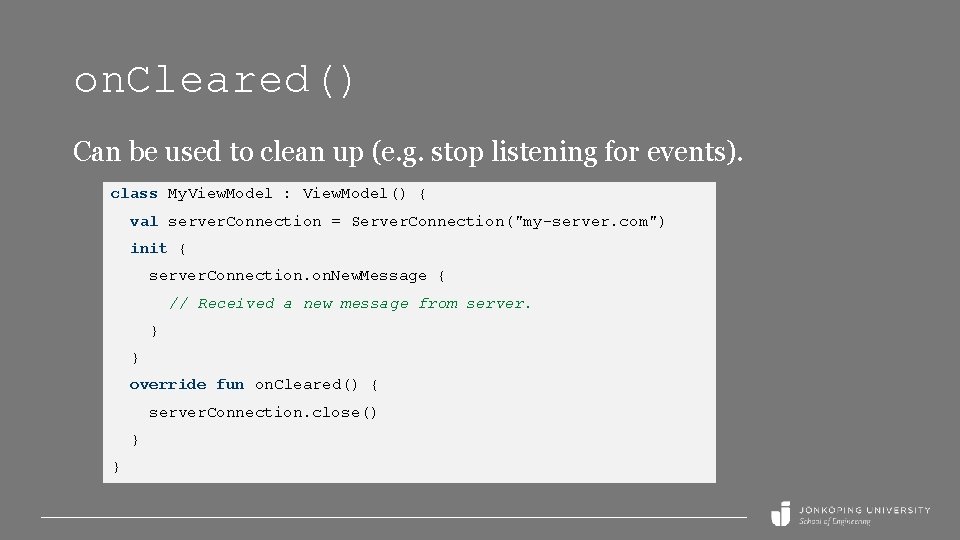
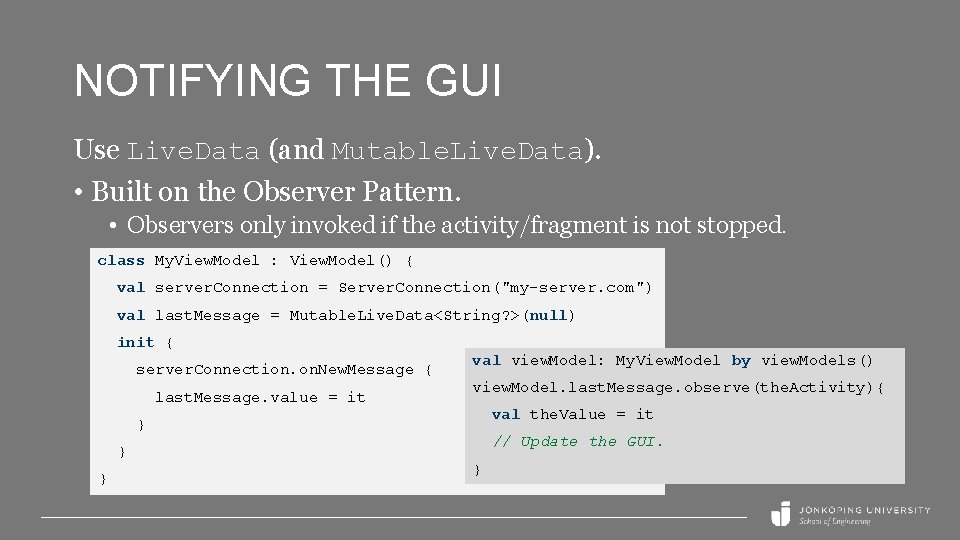
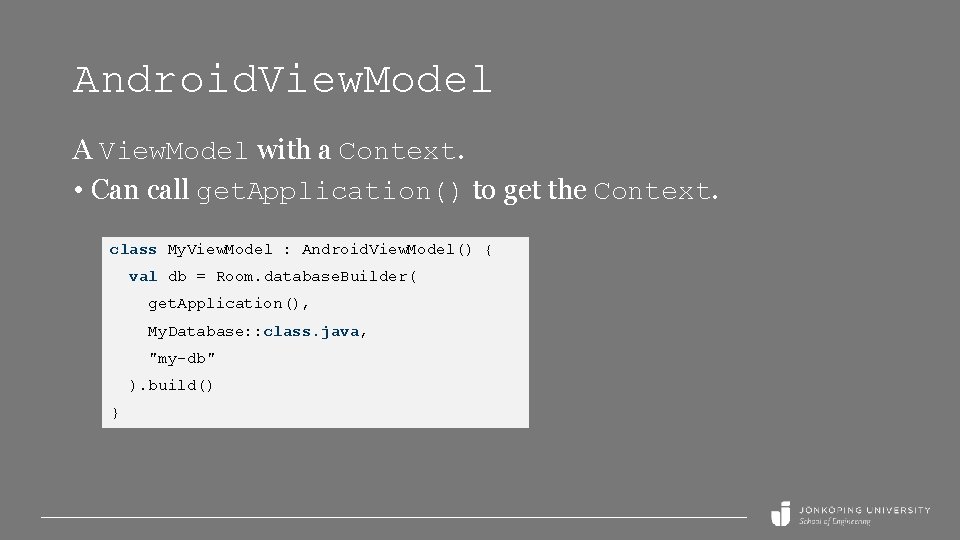
- Slides: 8
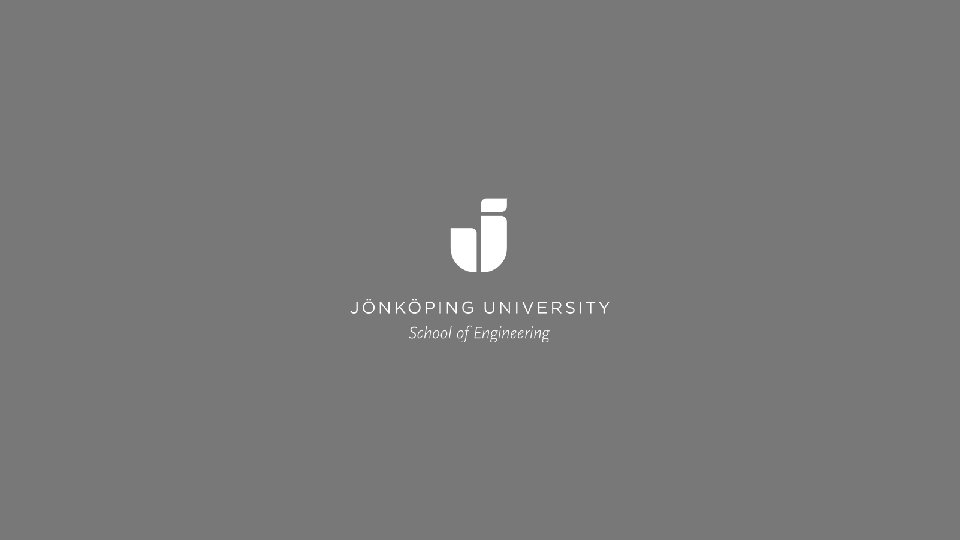
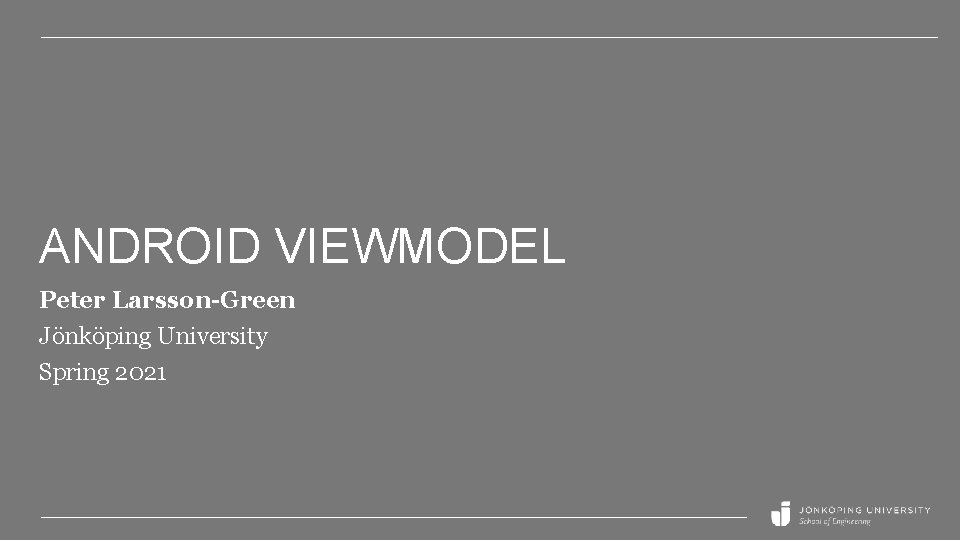
ANDROID VIEWMODEL Peter Larsson-Green Jönköping University Spring 2021
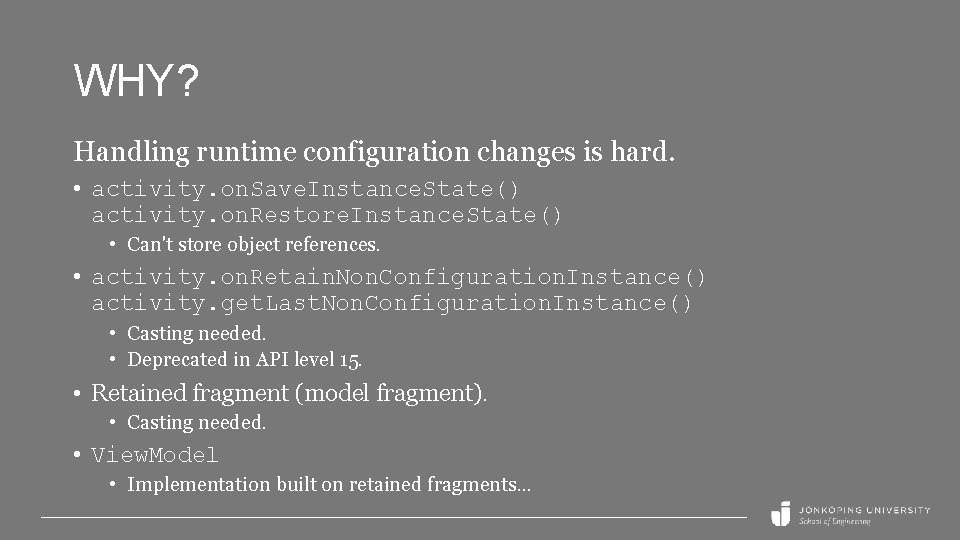
WHY? Handling runtime configuration changes is hard. • activity. on. Save. Instance. State() activity. on. Restore. Instance. State() • Can't store object references. • activity. on. Retain. Non. Configuration. Instance() activity. get. Last. Non. Configuration. Instance() • Casting needed. • Deprecated in API level 15. • Retained fragment (model fragment). • Casting needed. • View. Model • Implementation built on retained fragments…
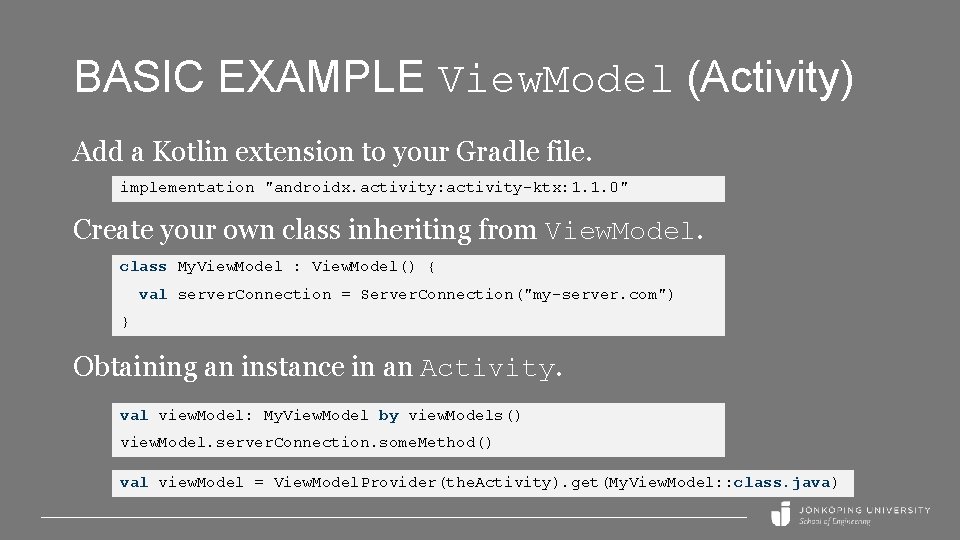
BASIC EXAMPLE View. Model (Activity) Add a Kotlin extension to your Gradle file. implementation "androidx. activity: activity-ktx: 1. 1. 0" Create your own class inheriting from View. Model. class My. View. Model : View. Model() { val server. Connection = Server. Connection("my-server. com") } Obtaining an instance in an Activity. val view. Model: My. View. Model by view. Models() view. Model. server. Connection. some. Method() val view. Model = View. Model. Provider(the. Activity). get(My. View. Model: : class. java)
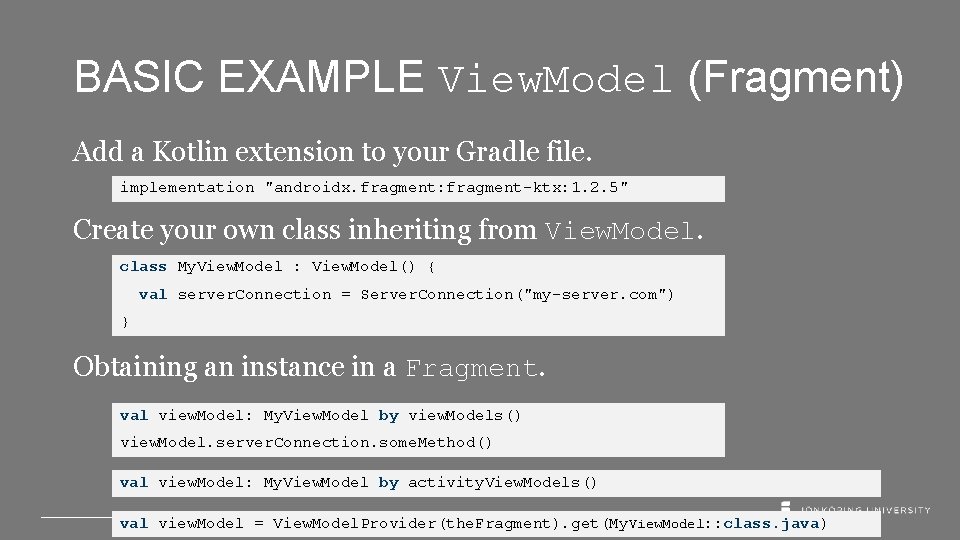
BASIC EXAMPLE View. Model (Fragment) Add a Kotlin extension to your Gradle file. implementation "androidx. fragment: fragment-ktx: 1. 2. 5" Create your own class inheriting from View. Model. class My. View. Model : View. Model() { val server. Connection = Server. Connection("my-server. com") } Obtaining an instance in a Fragment. val view. Model: My. View. Model by view. Models() view. Model. server. Connection. some. Method() val view. Model: My. View. Model by activity. View. Models() val view. Model = View. Model. Provider(the. Fragment). get(My. View. Model: : class. java)
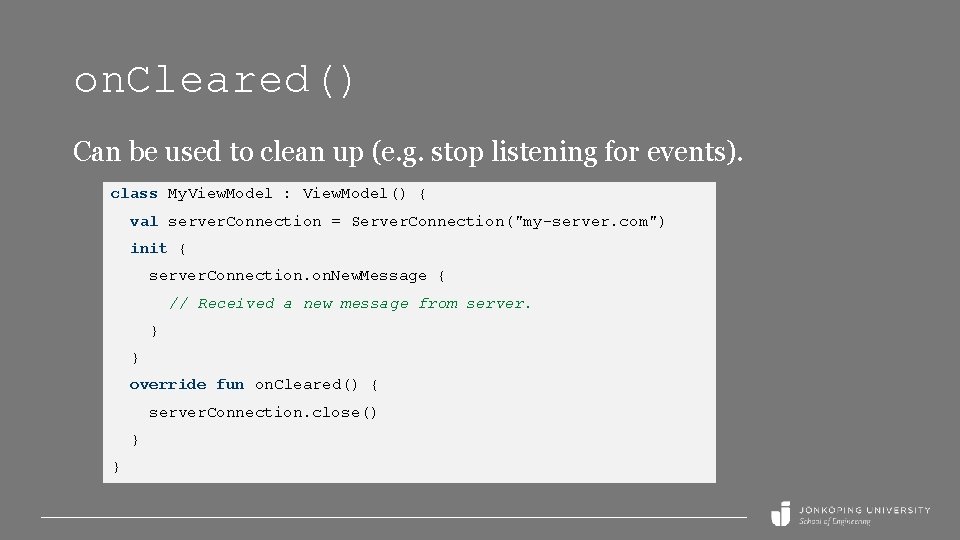
on. Cleared() Can be used to clean up (e. g. stop listening for events). class My. View. Model : View. Model() { val server. Connection = Server. Connection("my-server. com") init { server. Connection. New. Message { // Received a new message from server. } } override fun on. Cleared() { server. Connection. close() } }
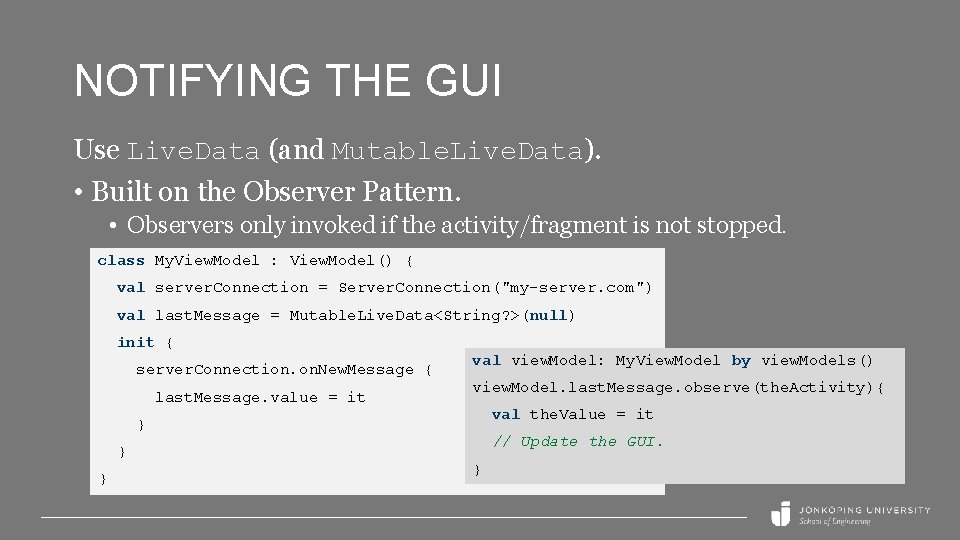
NOTIFYING THE GUI Use Live. Data (and Mutable. Live. Data). • Built on the Observer Pattern. • Observers only invoked if the activity/fragment is not stopped. class My. View. Model : View. Model() { val server. Connection = Server. Connection("my-server. com") val last. Message = Mutable. Live. Data<String? >(null) init { server. Connection. New. Message { last. Message. value = it val view. Model: My. View. Model by view. Models() view. Model. last. Message. observe(the. Activity){ val the. Value = it } } } // Update the GUI. }
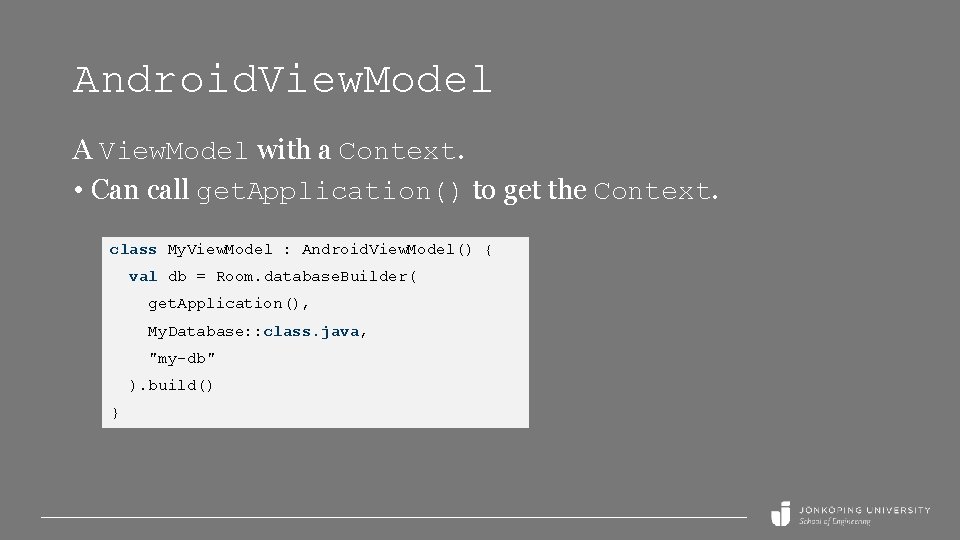
Android. View. Model A View. Model with a Context. • Can call get. Application() to get the Context. class My. View. Model : Android. View. Model() { val db = Room. database. Builder( get. Application(), My. Database: : class. java, "my-db" ). build() }