ANDROID SERVICES Peter LarssonGreen Jnkping University Spring 2020
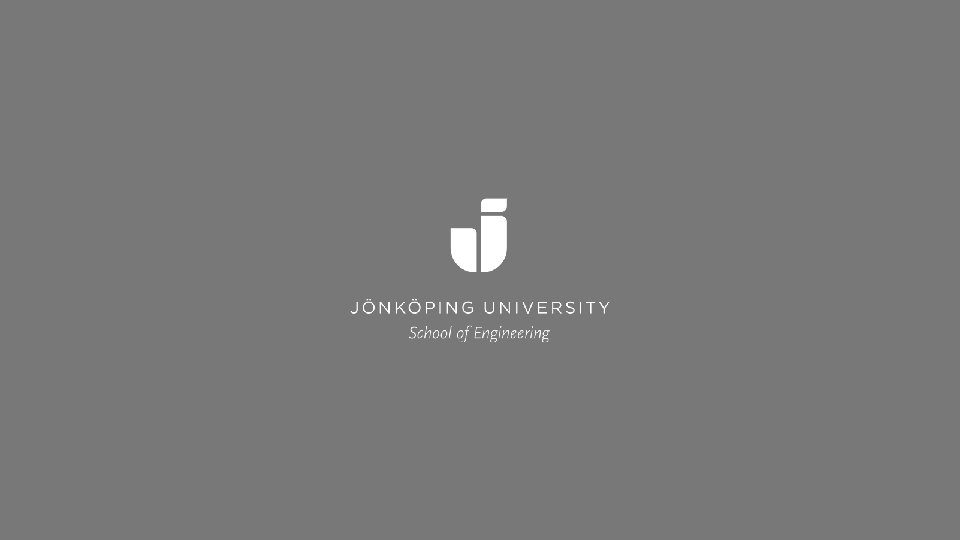
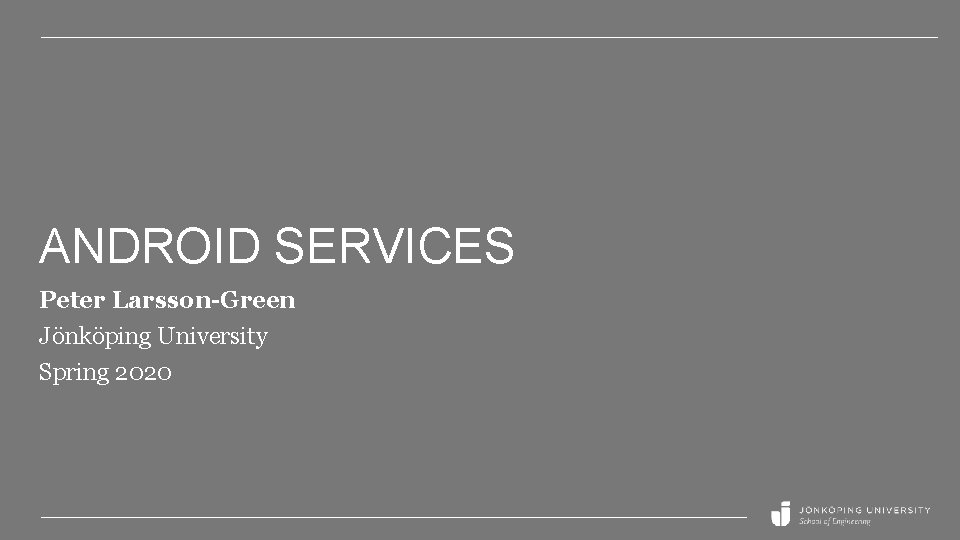
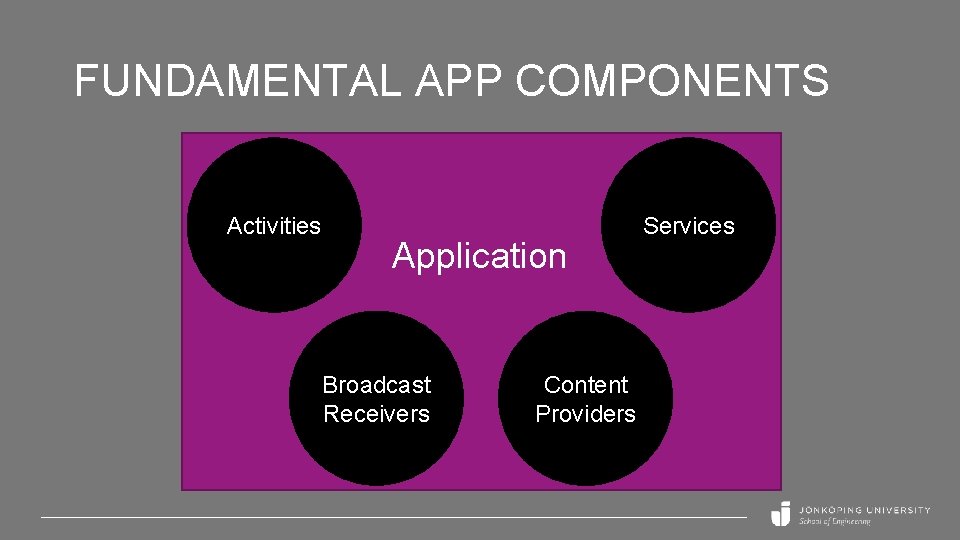
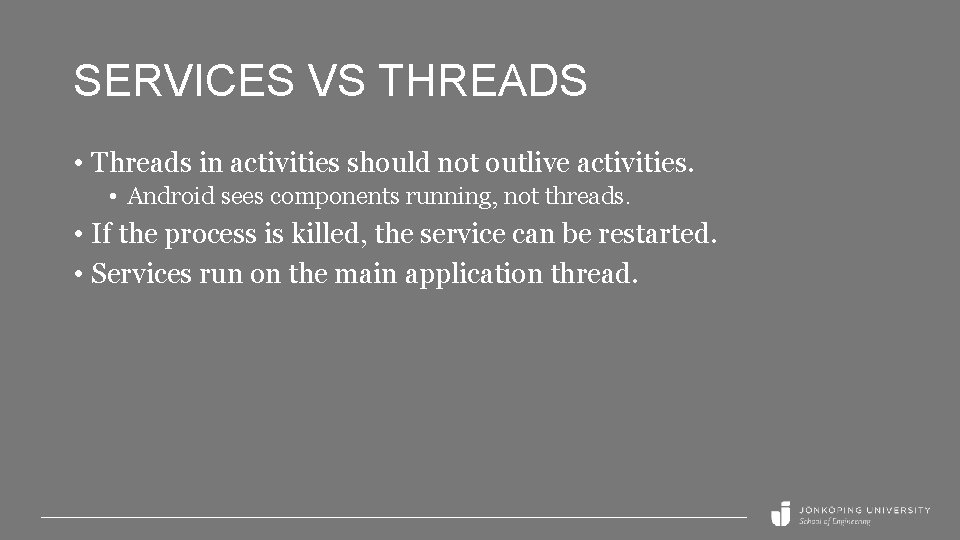
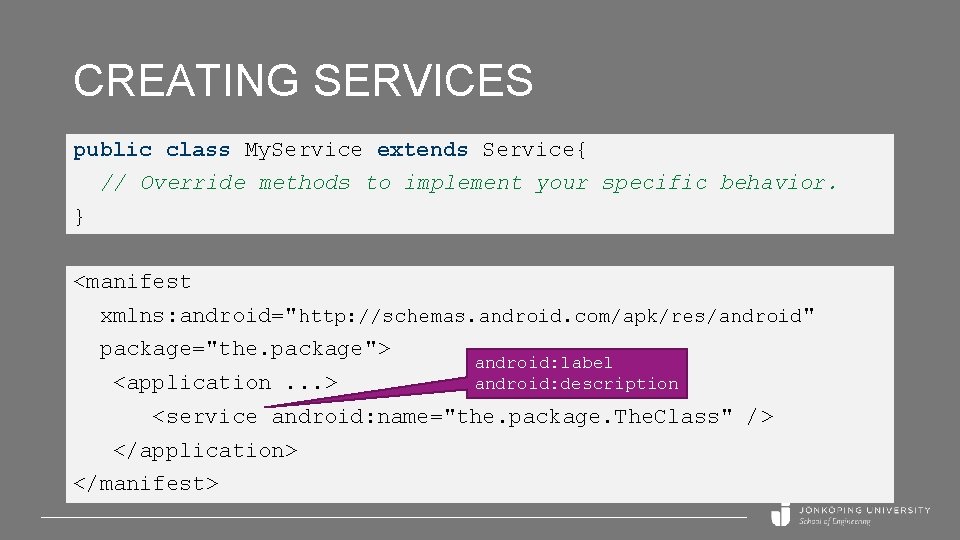
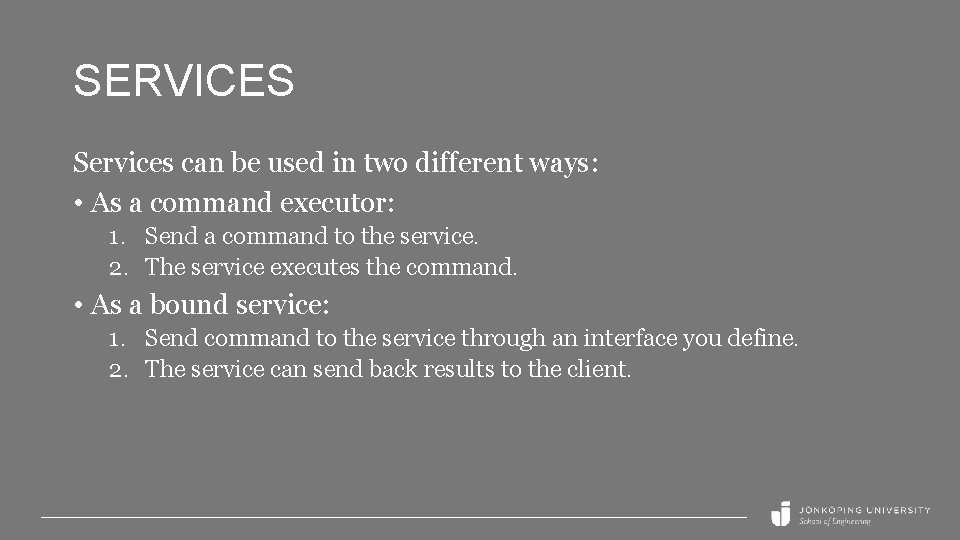
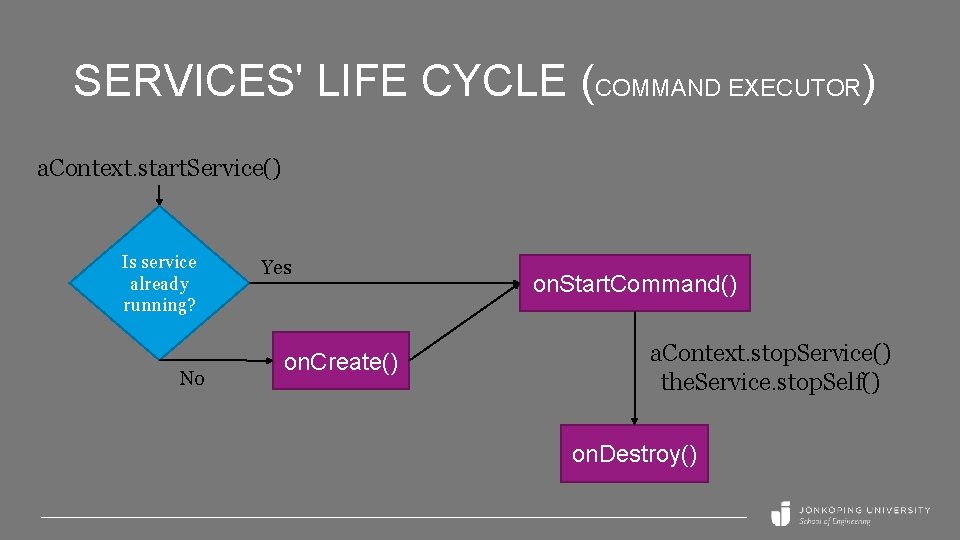
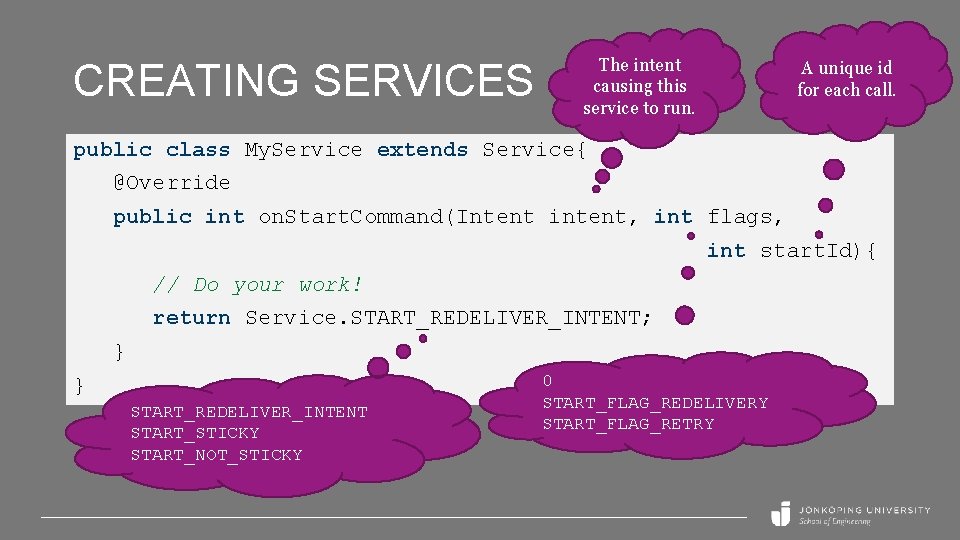
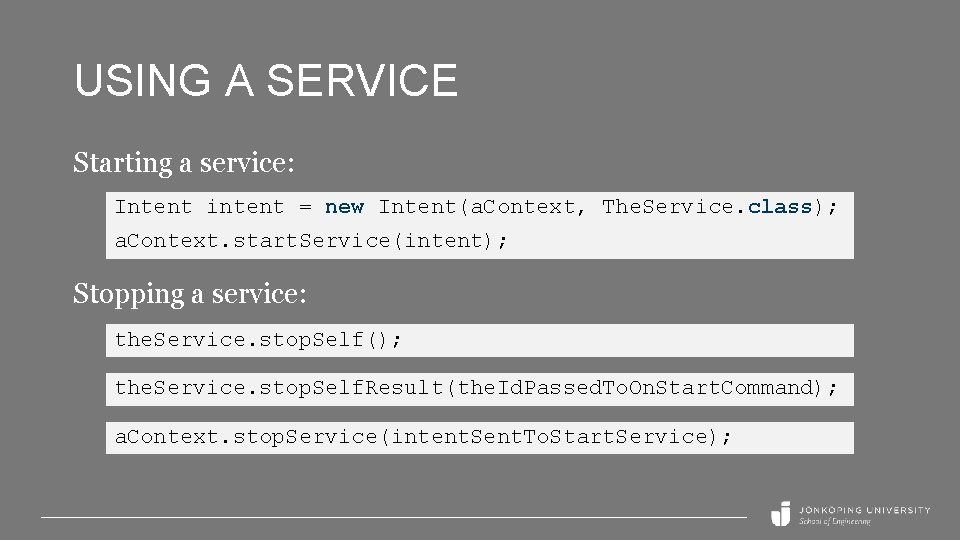
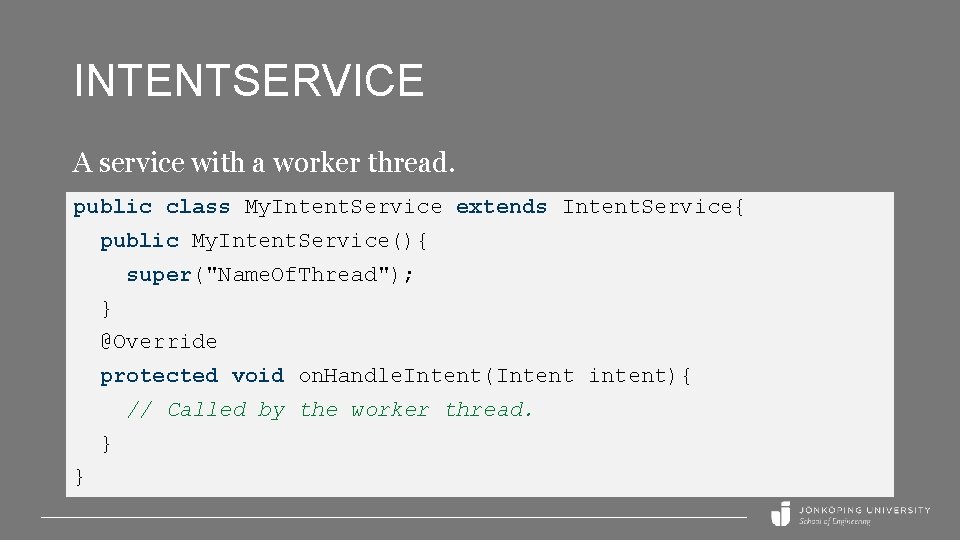
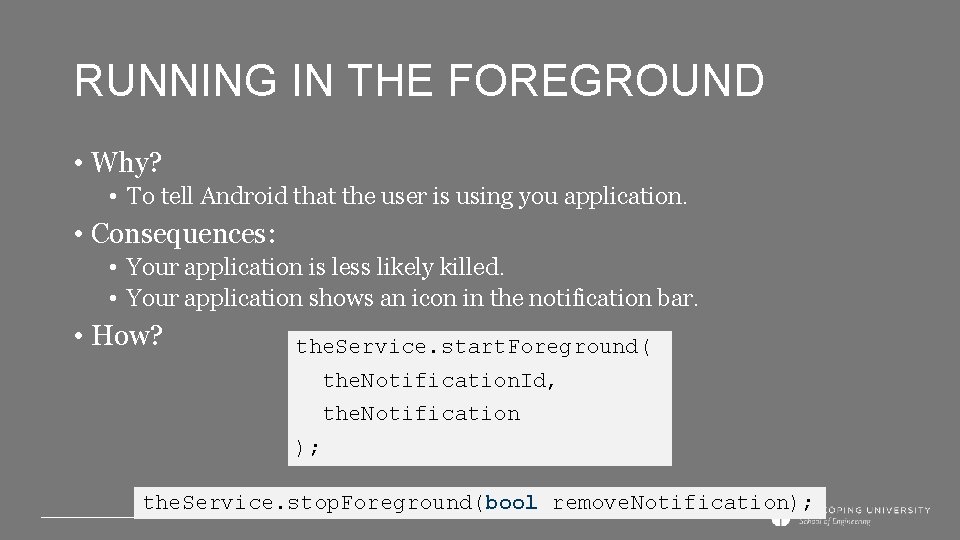
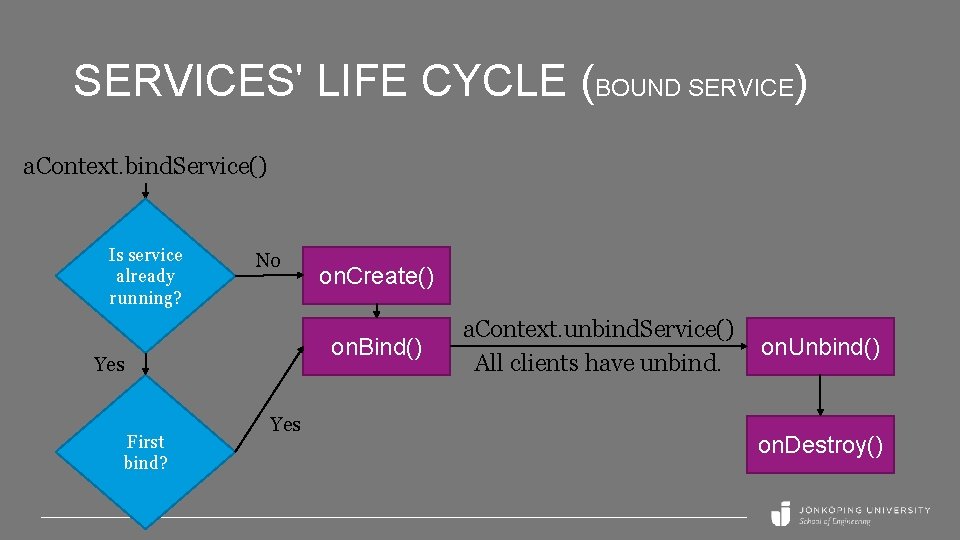
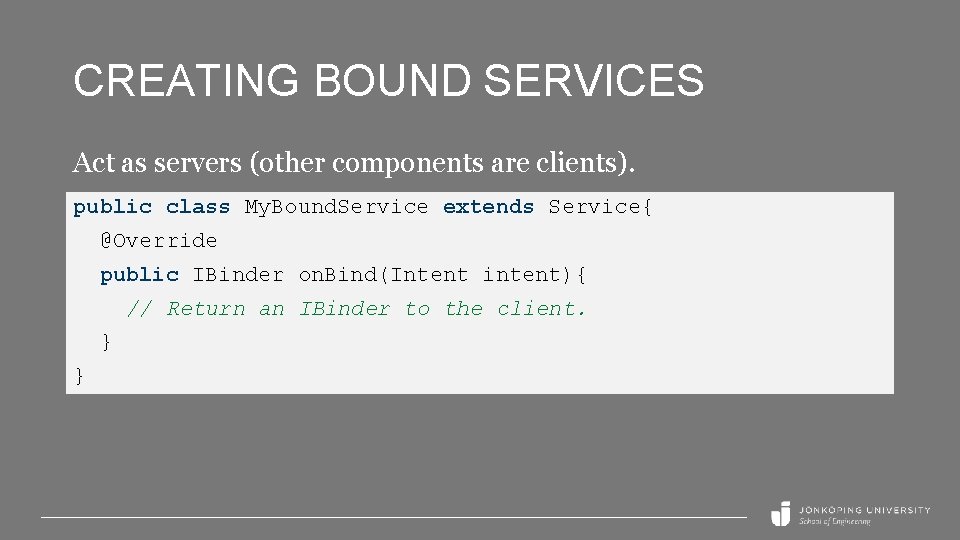
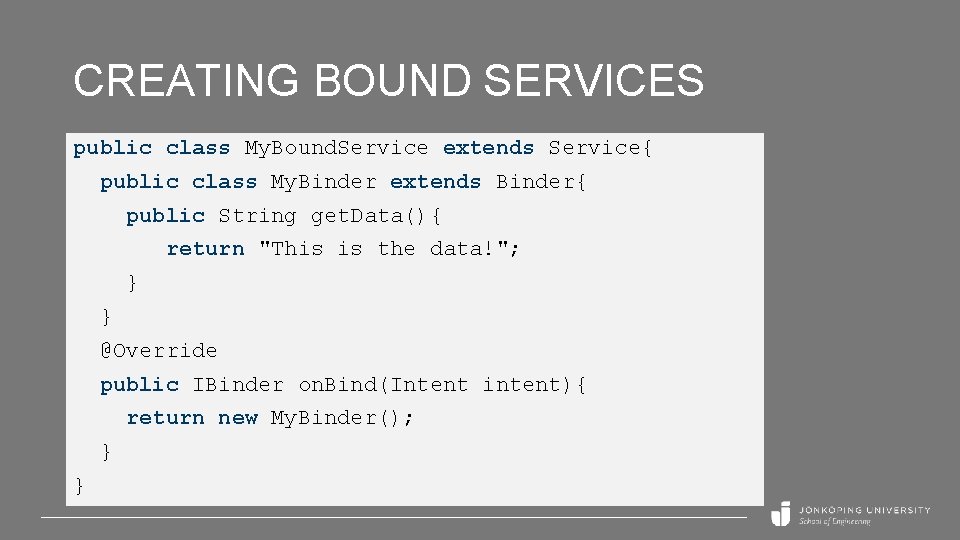
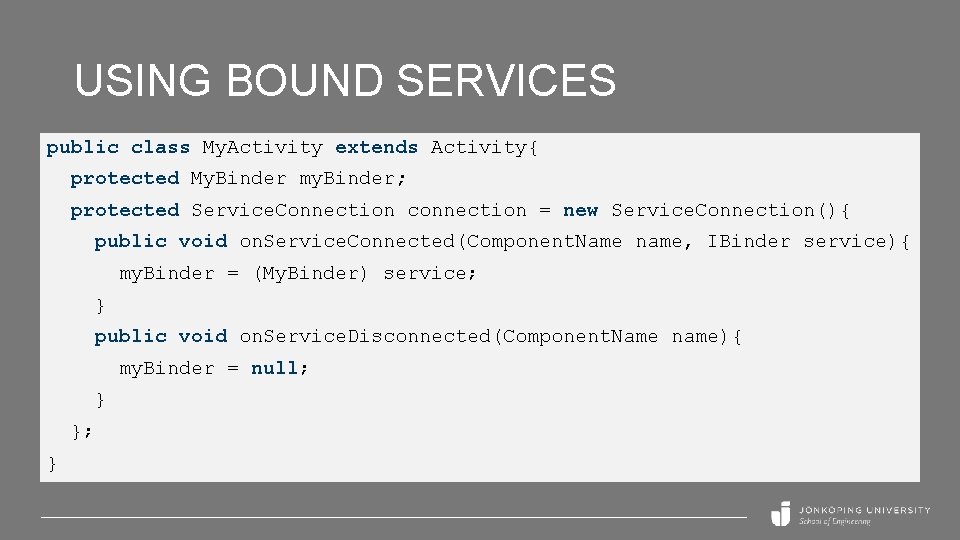
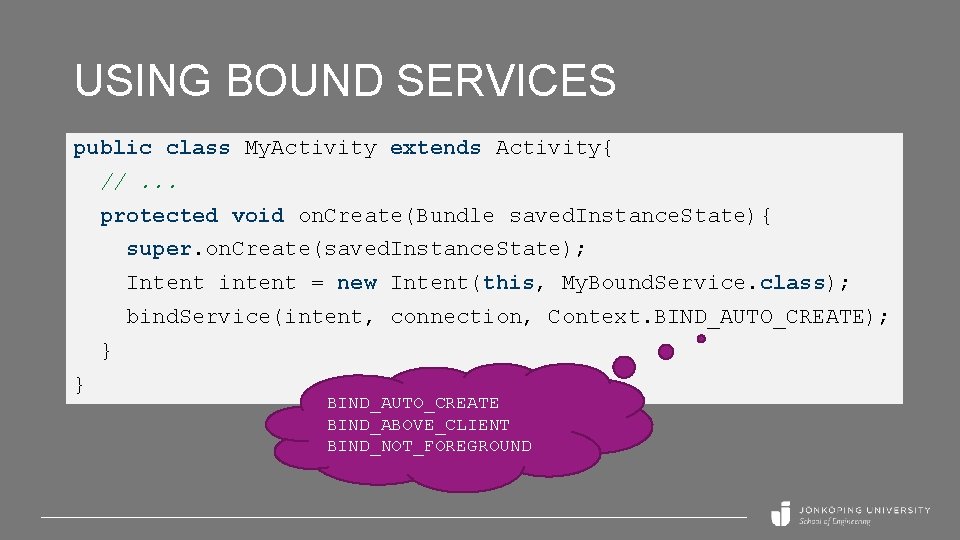
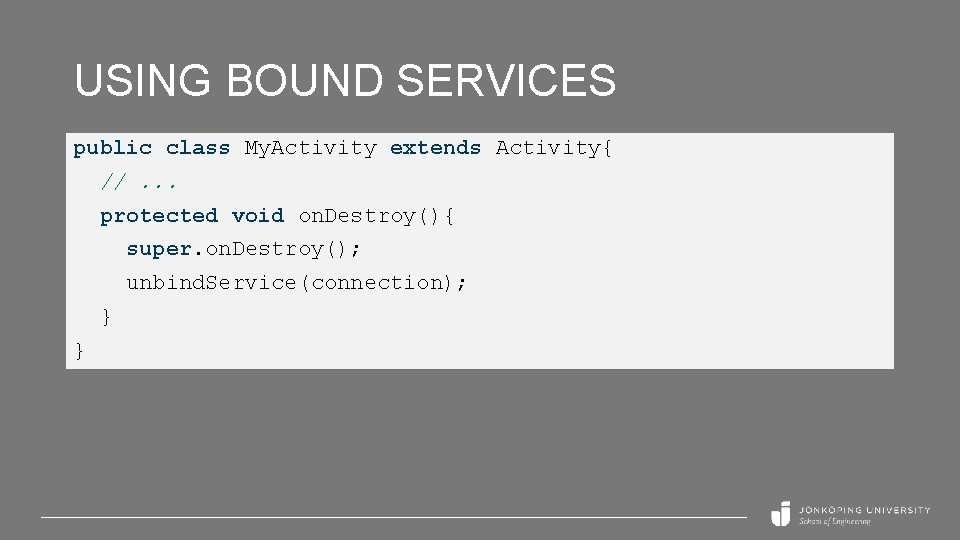
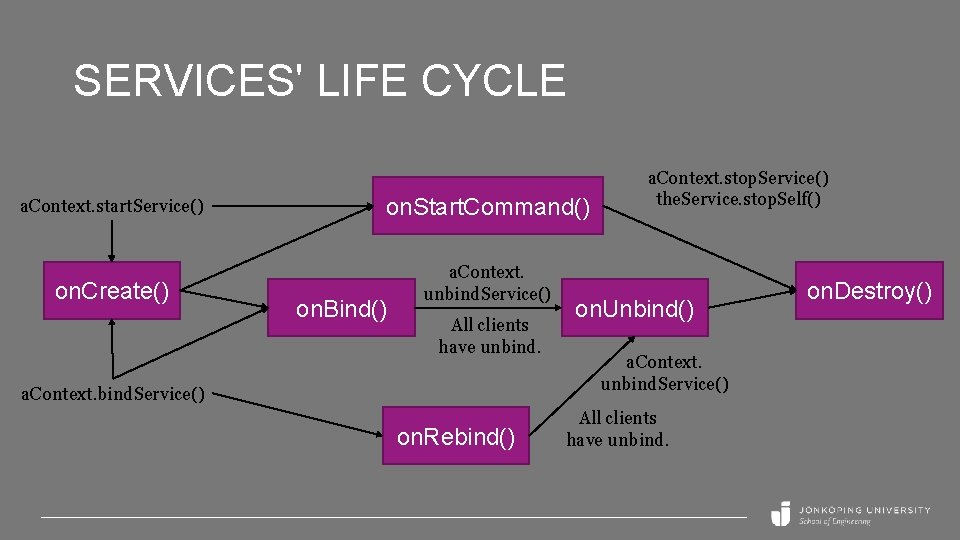
- Slides: 18
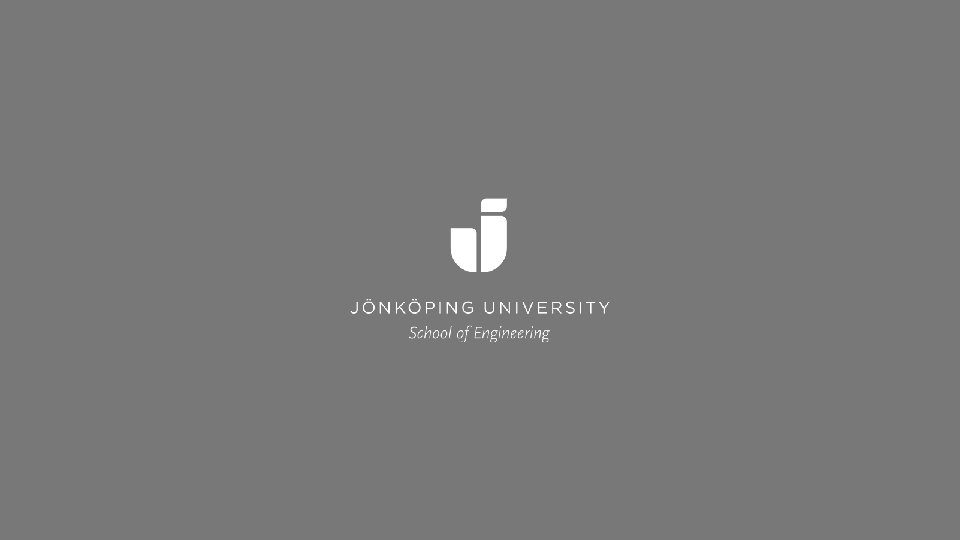
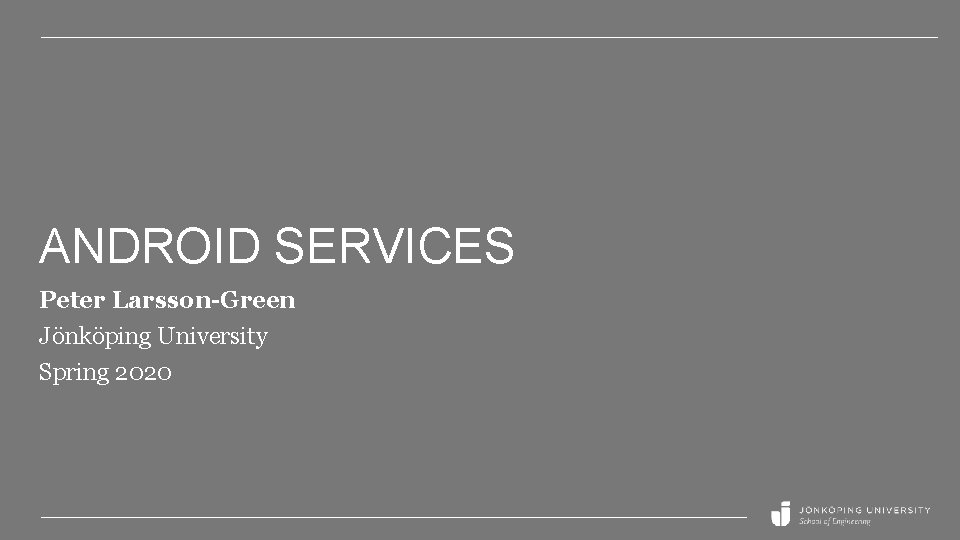
ANDROID SERVICES Peter Larsson-Green Jönköping University Spring 2020
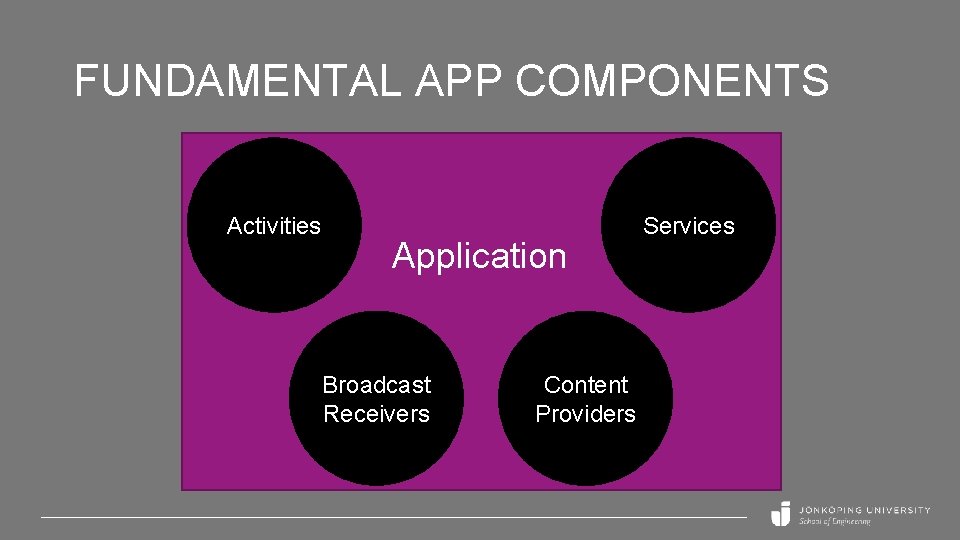
FUNDAMENTAL APP COMPONENTS Activities Application Broadcast Receivers Content Providers Services
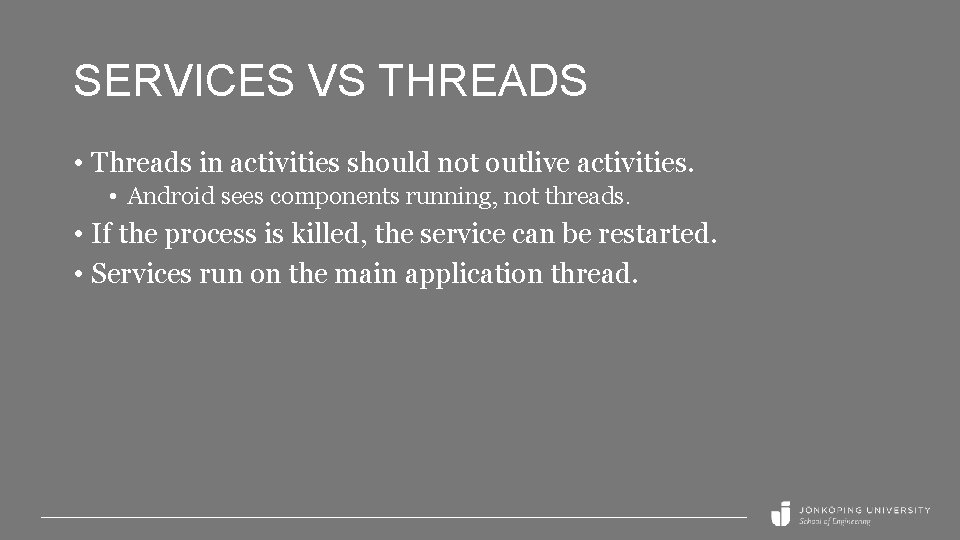
SERVICES VS THREADS • Threads in activities should not outlive activities. • Android sees components running, not threads. • If the process is killed, the service can be restarted. • Services run on the main application thread.
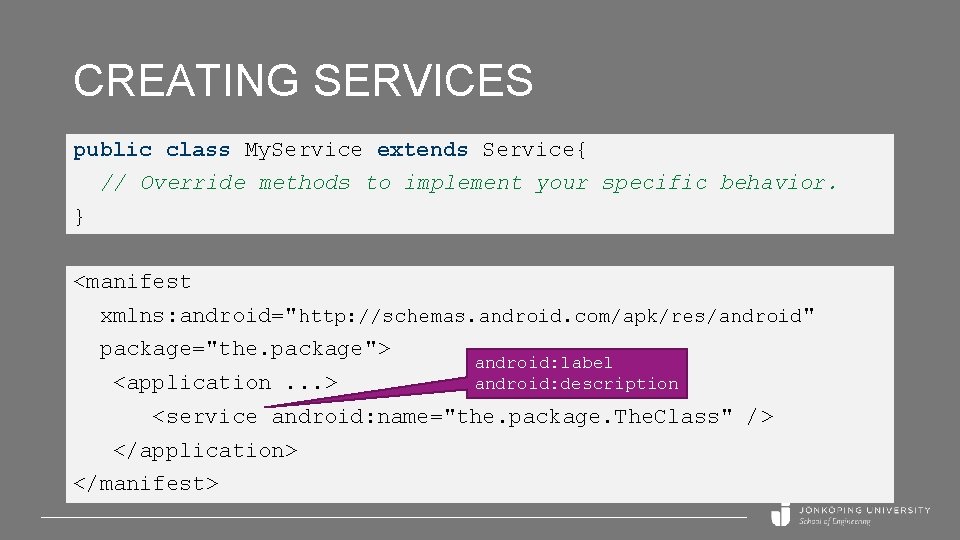
CREATING SERVICES public class My. Service extends Service{ // Override methods to implement your specific behavior. } <manifest xmlns: android="http: //schemas. android. com/apk/res/android" package="the. package"> <application. . . > android: label android: description <service android: name="the. package. The. Class" /> </application> </manifest>
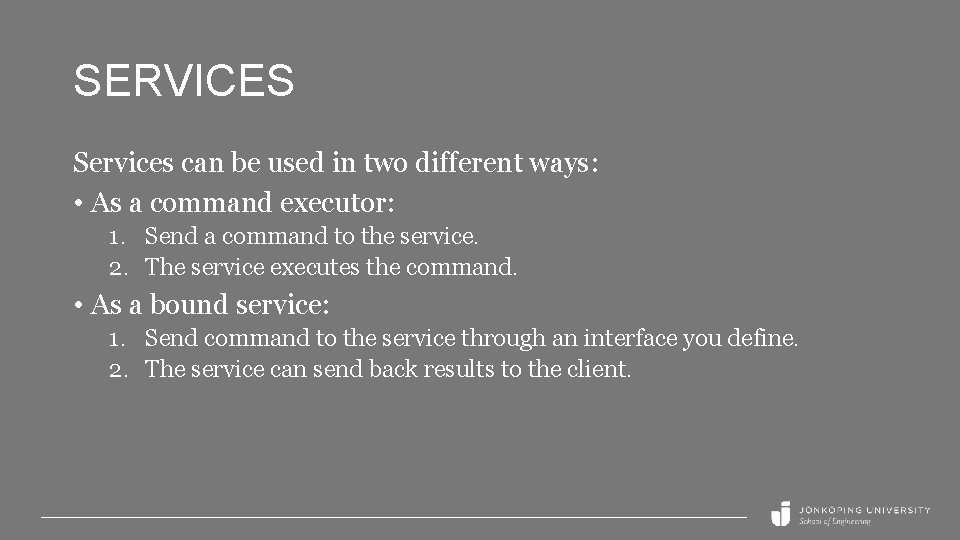
SERVICES Services can be used in two different ways: • As a command executor: 1. Send a command to the service. 2. The service executes the command. • As a bound service: 1. Send command to the service through an interface you define. 2. The service can send back results to the client.
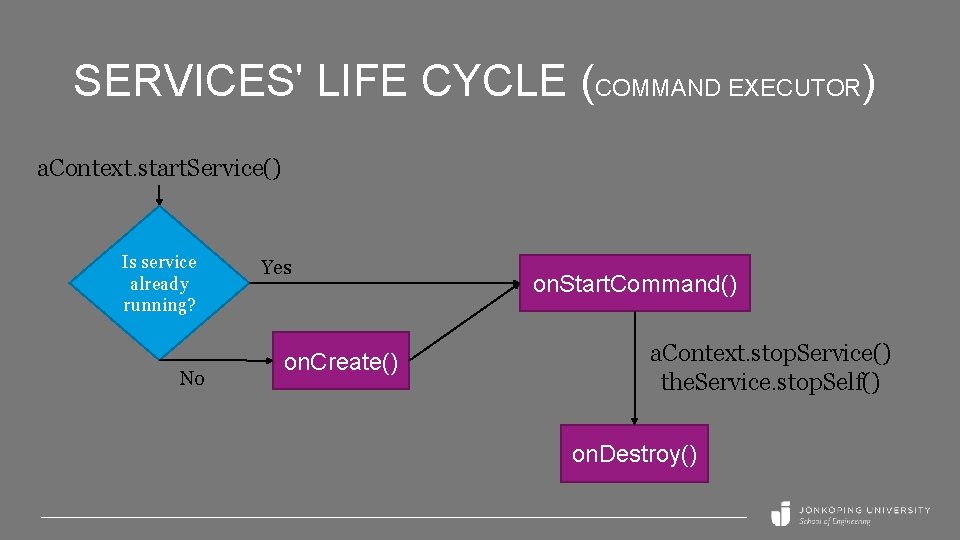
SERVICES' LIFE CYCLE (COMMAND EXECUTOR) a. Context. start. Service() Is service already running? No Yes on. Create() on. Start. Command() a. Context. stop. Service() the. Service. stop. Self() on. Destroy()
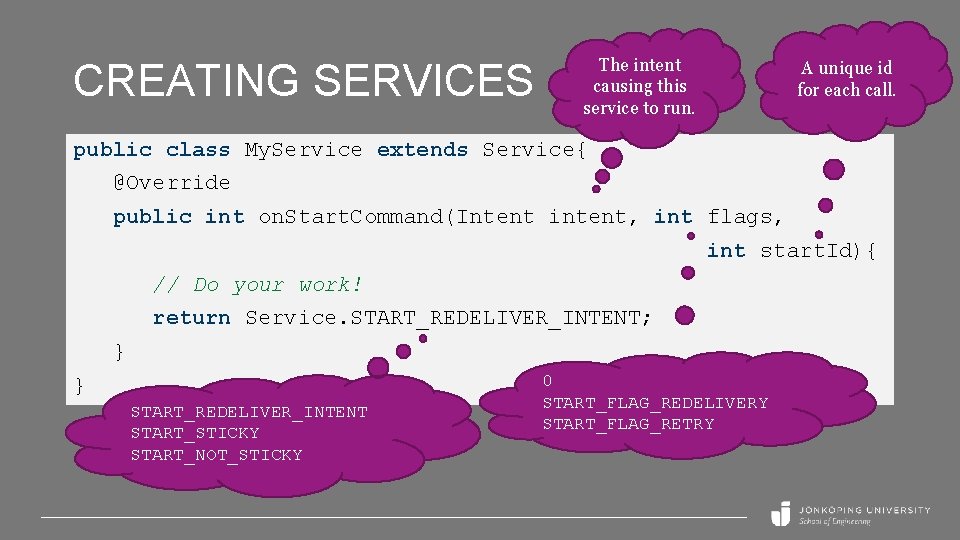
CREATING SERVICES The intent causing this service to run. A unique id for each call. public class My. Service extends Service{ @Override public int on. Start. Command(Intent intent, int flags, int start. Id){ // Do your work! return Service. START_REDELIVER_INTENT; } } START_REDELIVER_INTENT START_STICKY START_NOT_STICKY 0 START_FLAG_REDELIVERY START_FLAG_RETRY
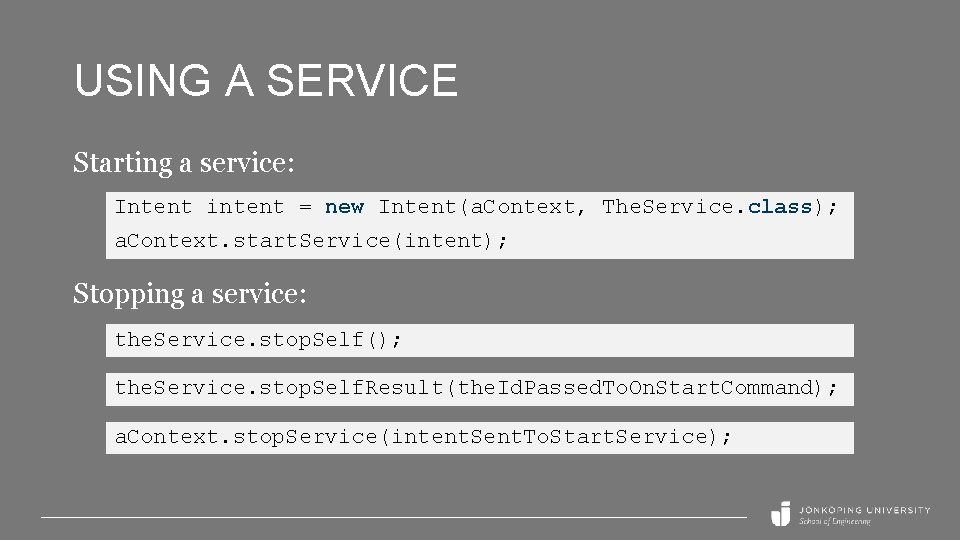
USING A SERVICE Starting a service: Intent intent = new Intent(a. Context, The. Service. class); a. Context. start. Service(intent); Stopping a service: the. Service. stop. Self(); the. Service. stop. Self. Result(the. Id. Passed. To. On. Start. Command); a. Context. stop. Service(intent. Sent. To. Start. Service);
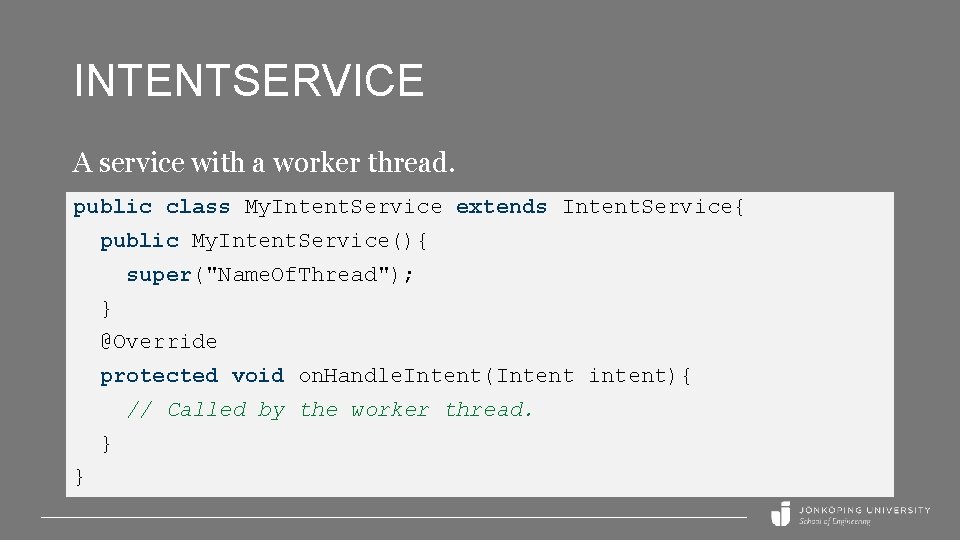
INTENTSERVICE A service with a worker thread. public class My. Intent. Service extends Intent. Service{ public My. Intent. Service(){ super("Name. Of. Thread"); } @Override protected void on. Handle. Intent(Intent intent){ // Called by the worker thread. } }
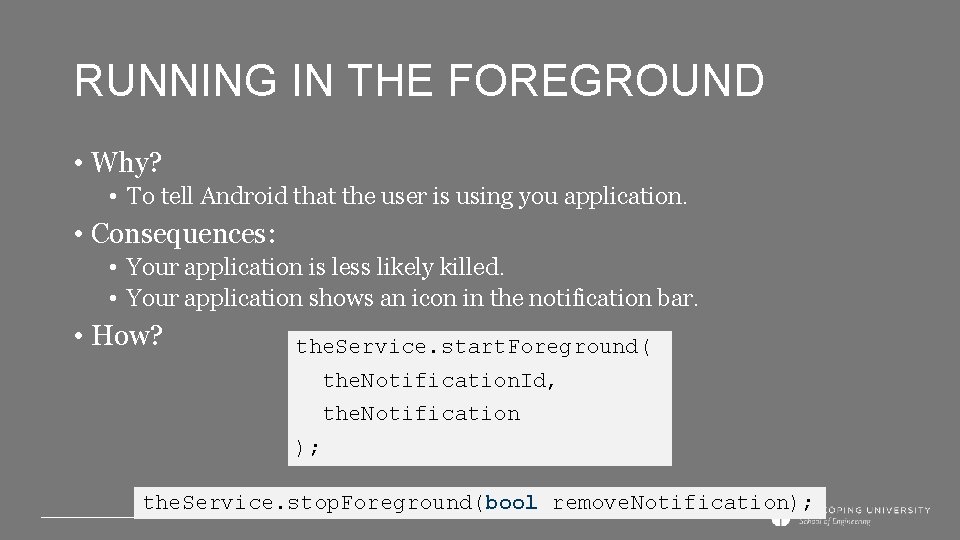
RUNNING IN THE FOREGROUND • Why? • To tell Android that the user is using you application. • Consequences: • Your application is less likely killed. • Your application shows an icon in the notification bar. • How? the. Service. start. Foreground( the. Notification. Id, the. Notification ); the. Service. stop. Foreground(bool remove. Notification);
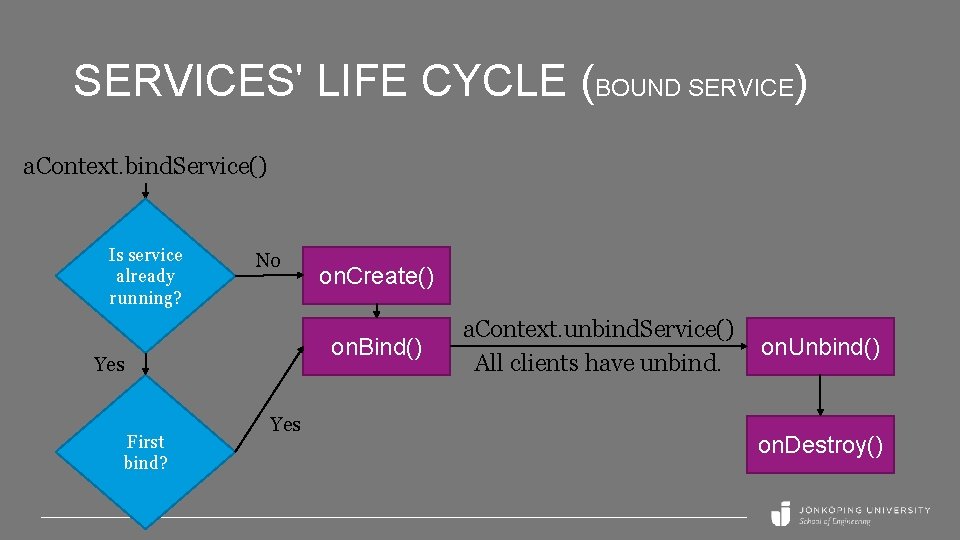
SERVICES' LIFE CYCLE (BOUND SERVICE) a. Context. bind. Service() Is service already running? No on. Bind() Yes First bind? on. Create() Yes a. Context. unbind. Service() All clients have unbind. on. Unbind() on. Destroy()
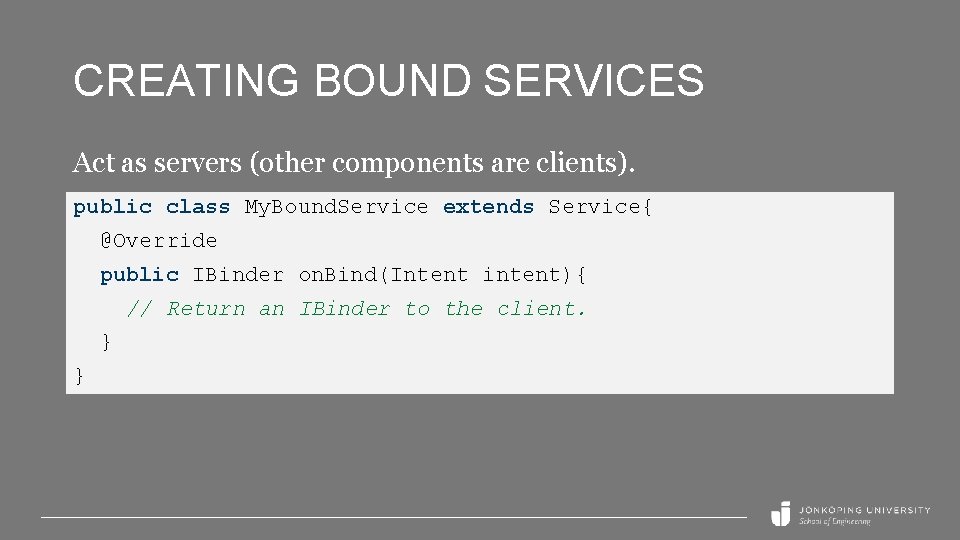
CREATING BOUND SERVICES Act as servers (other components are clients). public class My. Bound. Service extends Service{ @Override public IBinder on. Bind(Intent intent){ // Return an IBinder to the client. } }
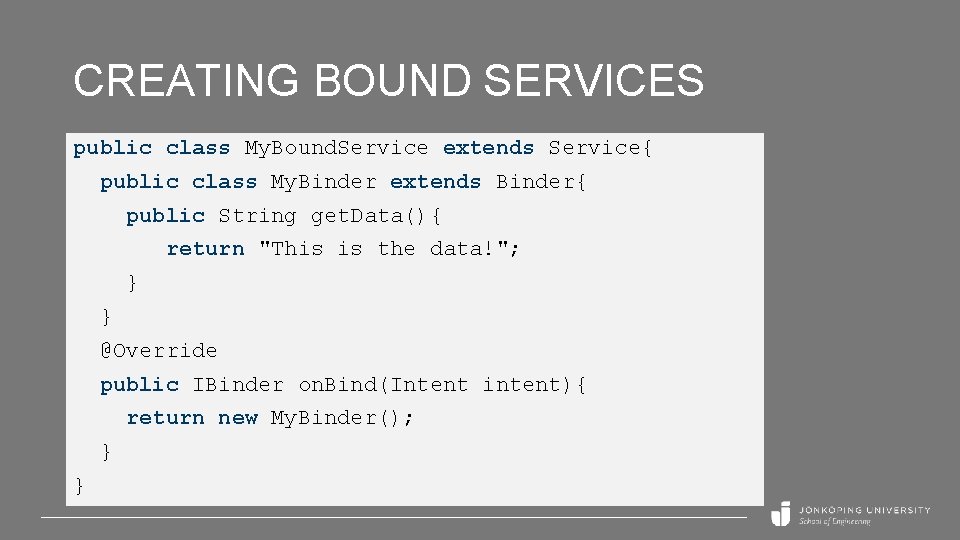
CREATING BOUND SERVICES public class My. Bound. Service extends Service{ public class My. Binder extends Binder{ public String get. Data(){ return "This is the data!"; } } @Override public IBinder on. Bind(Intent intent){ return new My. Binder(); } }
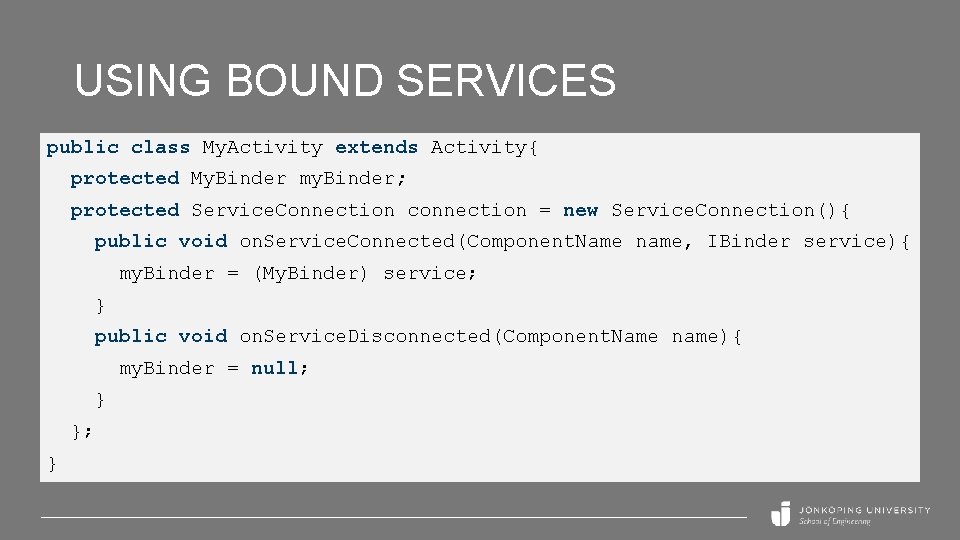
USING BOUND SERVICES public class My. Activity extends Activity{ protected My. Binder my. Binder; protected Service. Connection connection = new Service. Connection(){ public void on. Service. Connected(Component. Name name, IBinder service){ my. Binder = (My. Binder) service; } public void on. Service. Disconnected(Component. Name name){ my. Binder = null; } }; }
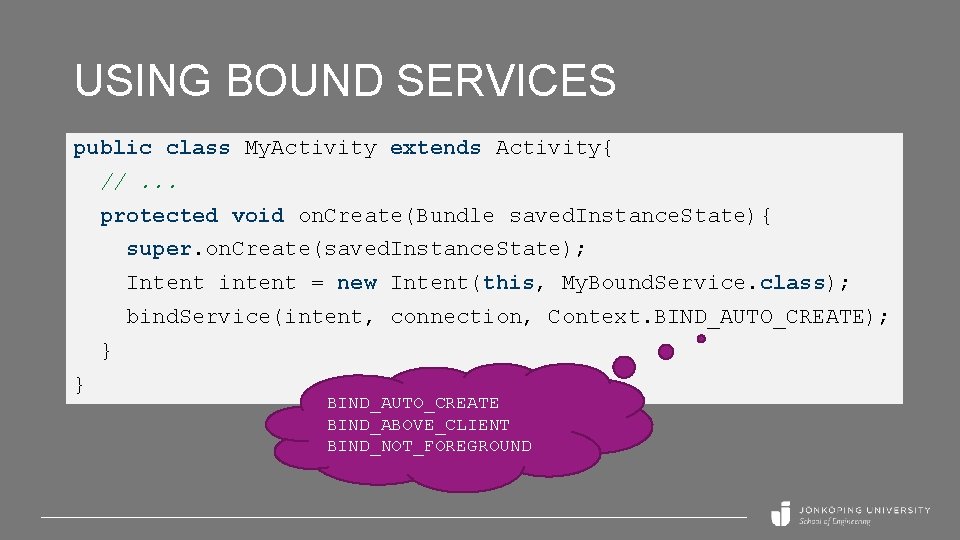
USING BOUND SERVICES public class My. Activity extends Activity{ //. . . protected void on. Create(Bundle saved. Instance. State){ super. on. Create(saved. Instance. State); Intent intent = new Intent(this, My. Bound. Service. class); bind. Service(intent, connection, Context. BIND_AUTO_CREATE); } } BIND_AUTO_CREATE BIND_ABOVE_CLIENT BIND_NOT_FOREGROUND
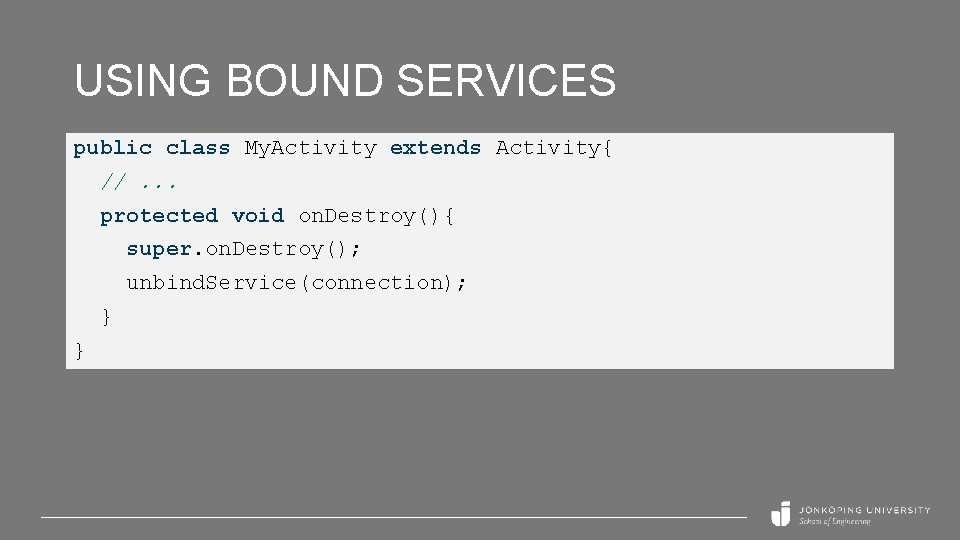
USING BOUND SERVICES public class My. Activity extends Activity{ //. . . protected void on. Destroy(){ super. on. Destroy(); unbind. Service(connection); } }
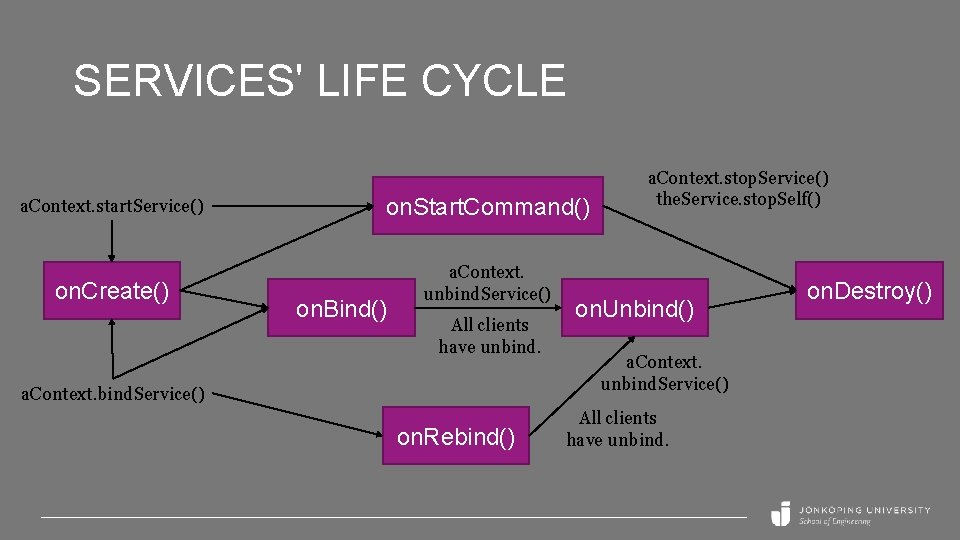
SERVICES' LIFE CYCLE a. Context. start. Service() on. Start. Command() on. Create() a. Context. unbind. Service() on. Bind() All clients have unbind. a. Context. bind. Service() on. Rebind() a. Context. stop. Service() the. Service. stop. Self() on. Unbind() a. Context. unbind. Service() All clients have unbind. on. Destroy()
Cast of spring, summer, fall, winter... and spring
Spring seasons months
Spring io conference 2020
Math enrichment spring 2020
Cs162 spring 2020
Android system services
Lifecycle services android
Capgemini world wealth report 2020
Intserv vs diffserv
Wake county human services community services center
Western washington university human services
Chapman business office
K state counseling services
203 mary martin hall
University of utah financial and business services
Duke visa services cpt
Drs temple university
Bhathal student services center
Student business services texas state