Android Development Tools Android App Lifecycle Context Central
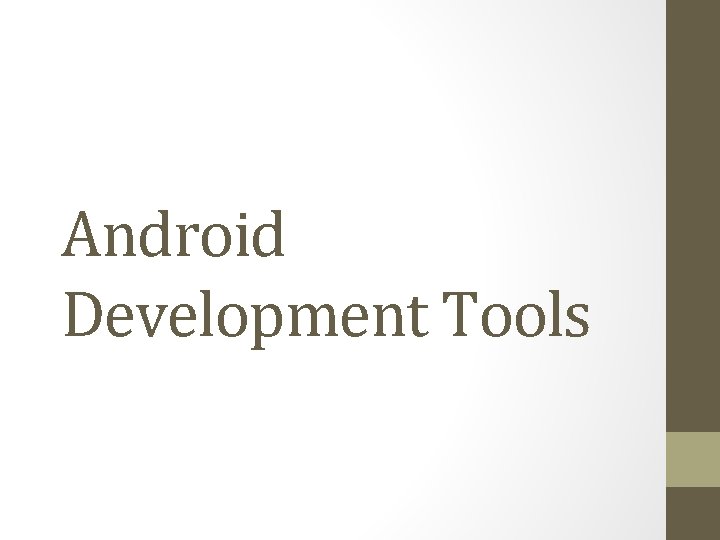
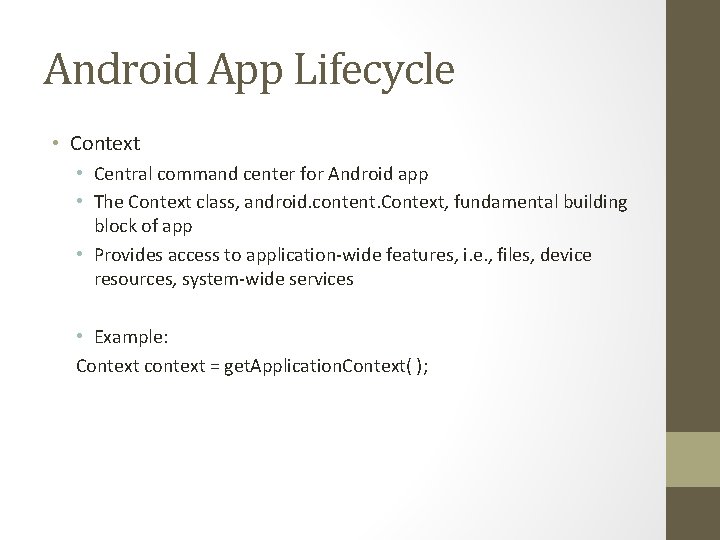
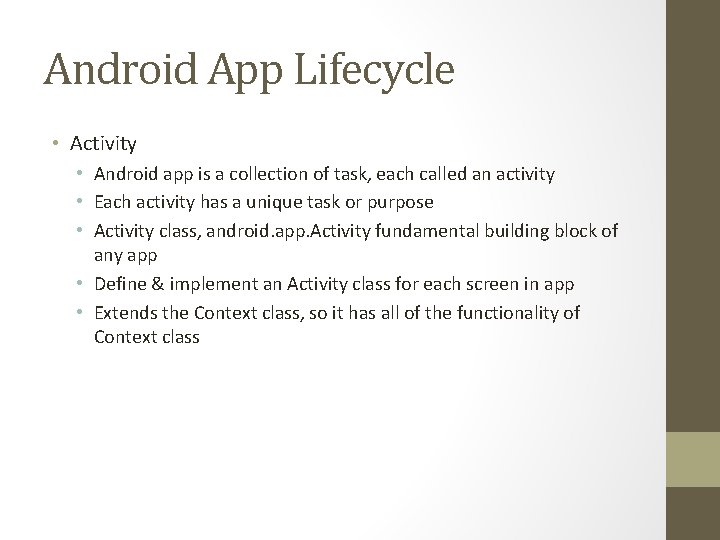
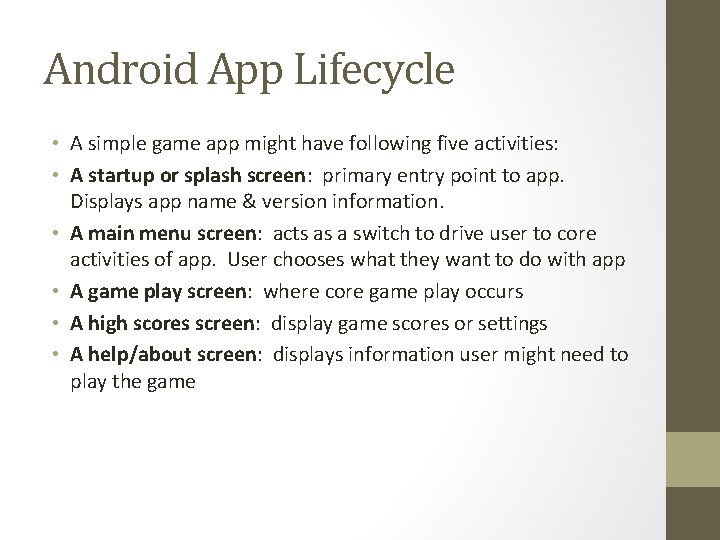
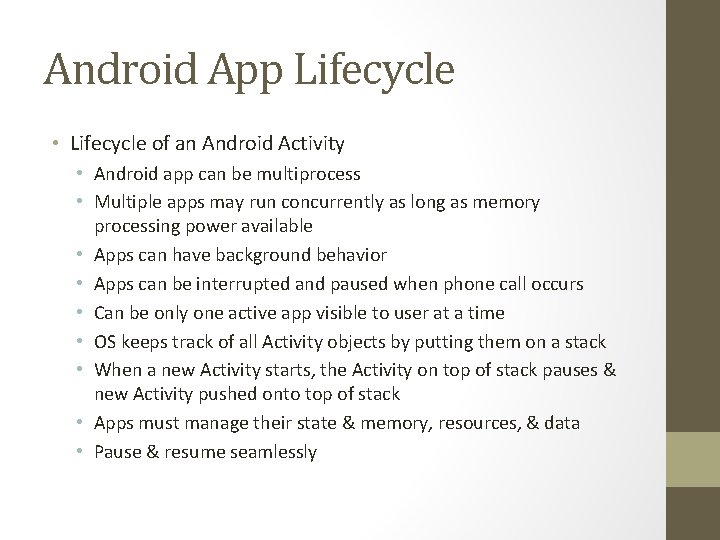
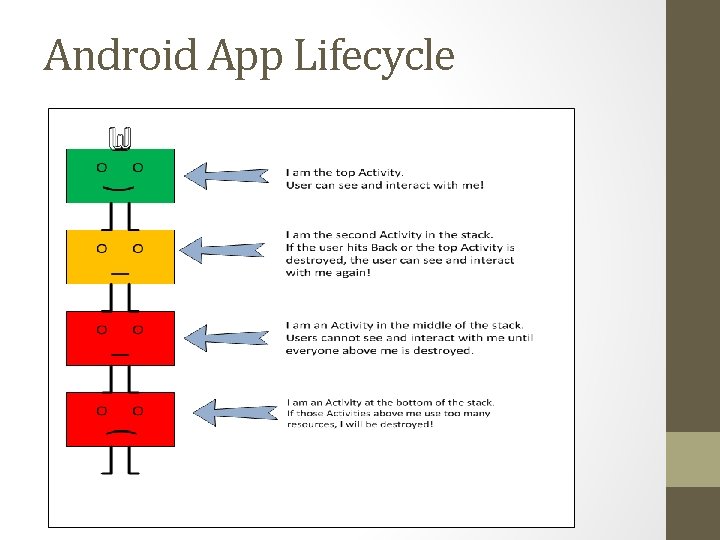
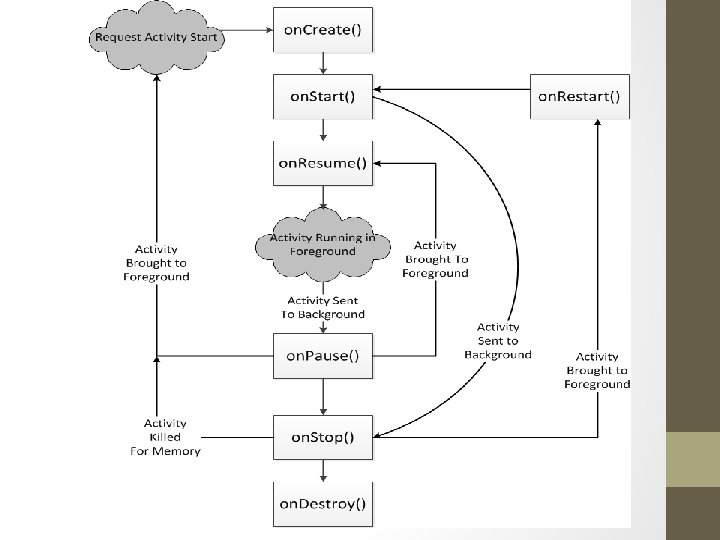
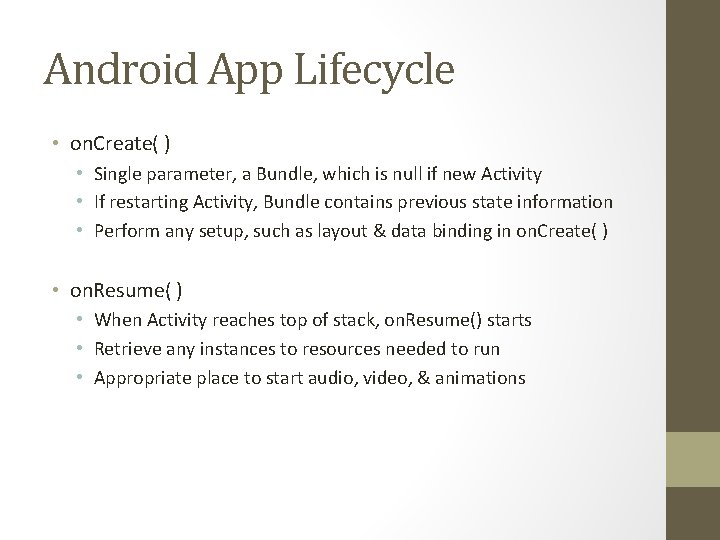
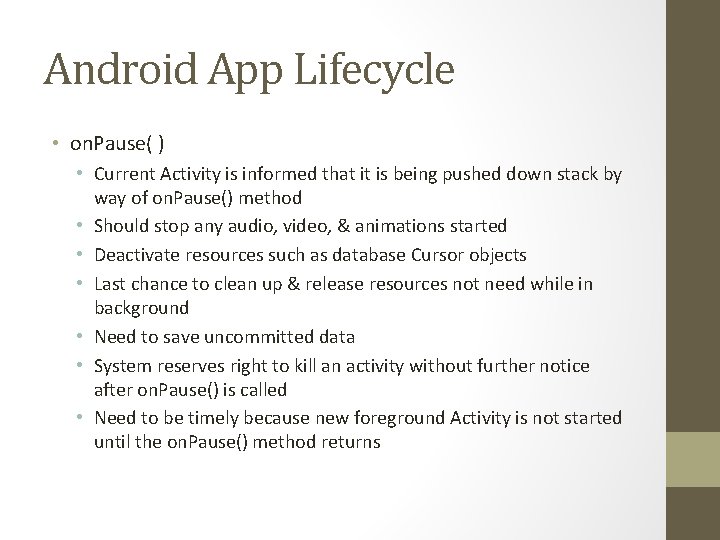
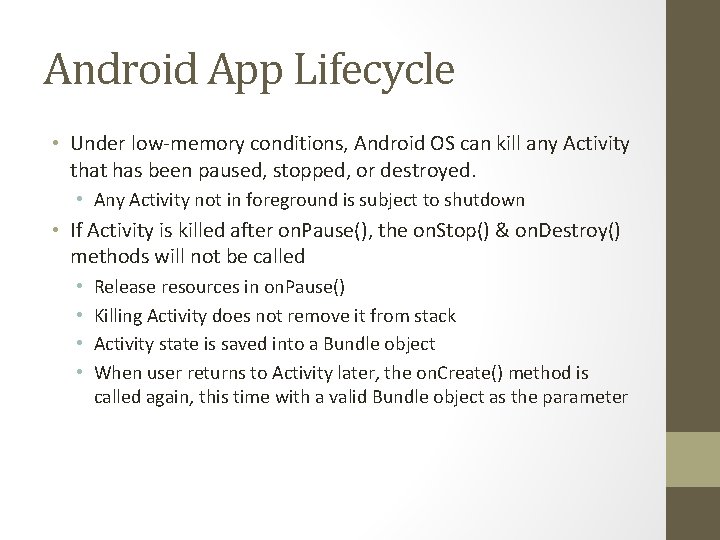
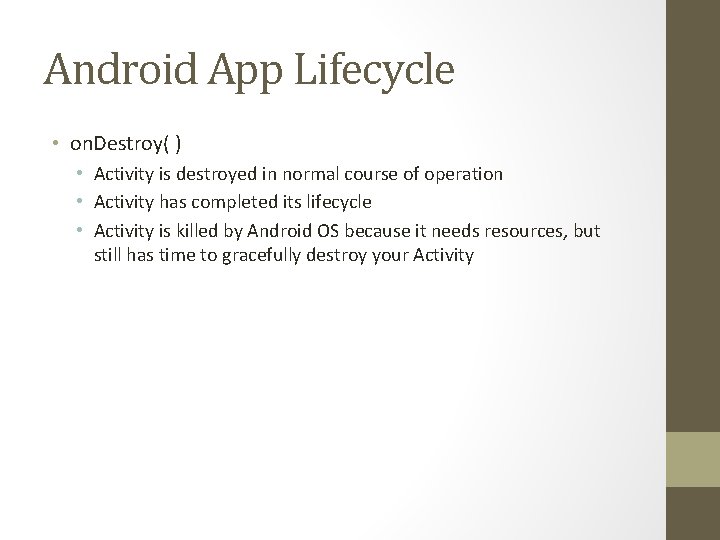
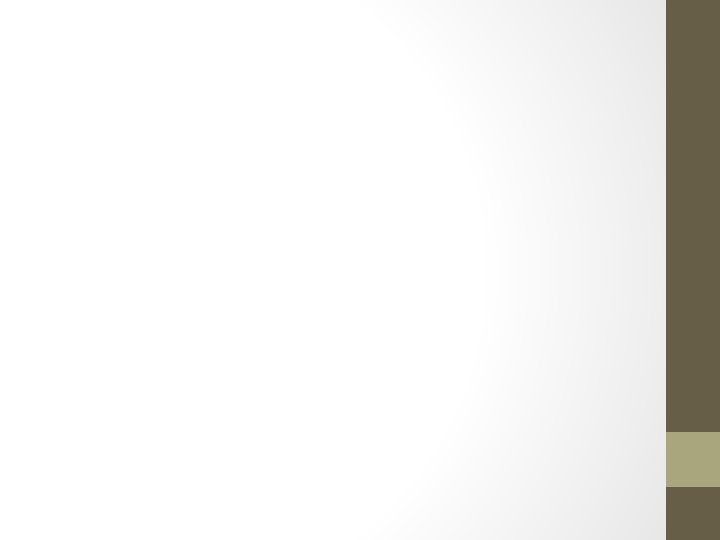
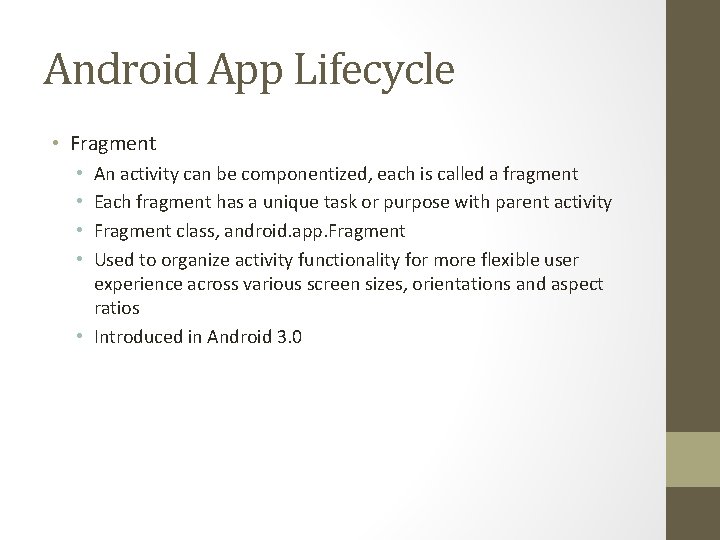
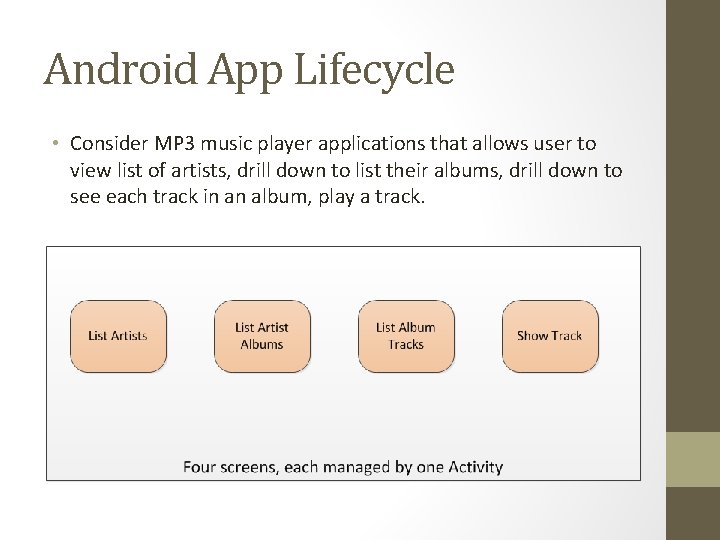
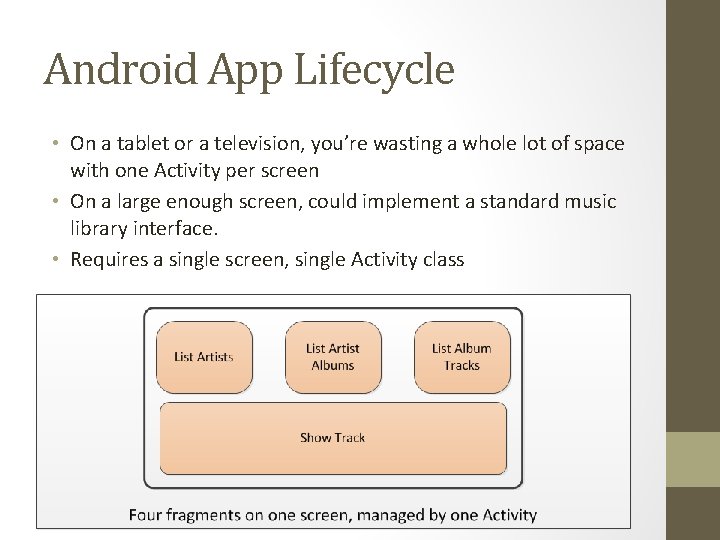
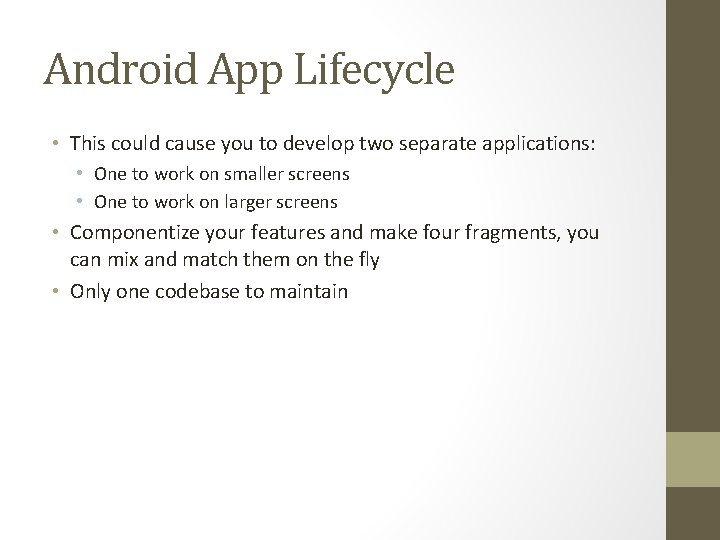
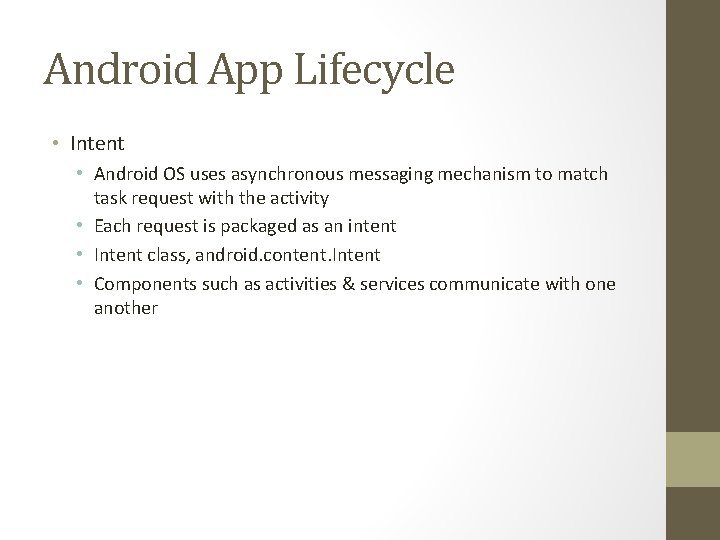
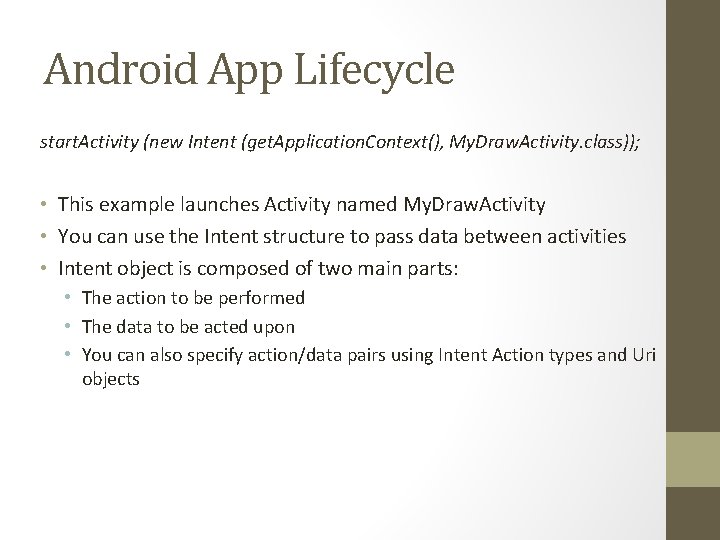
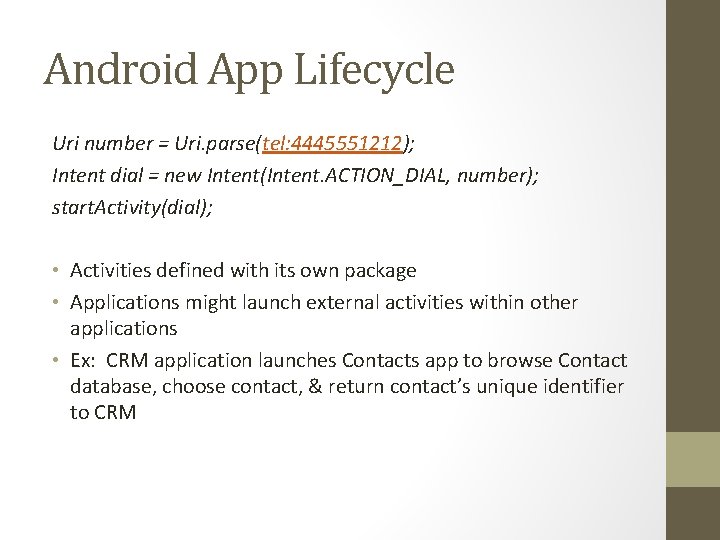
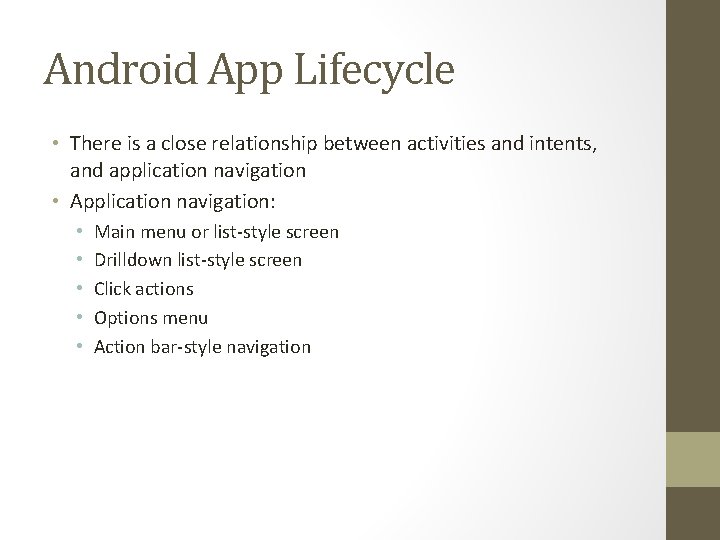
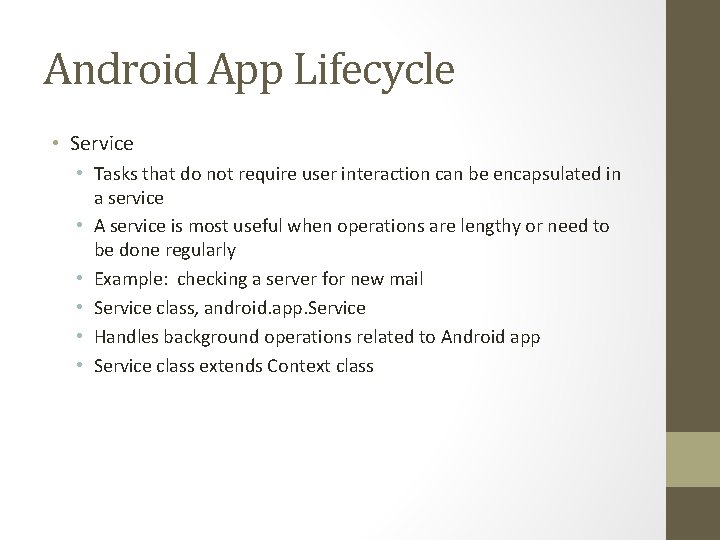
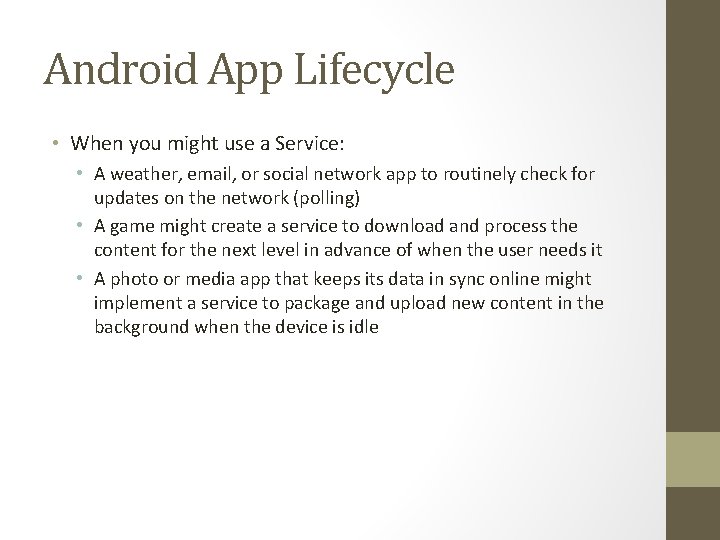
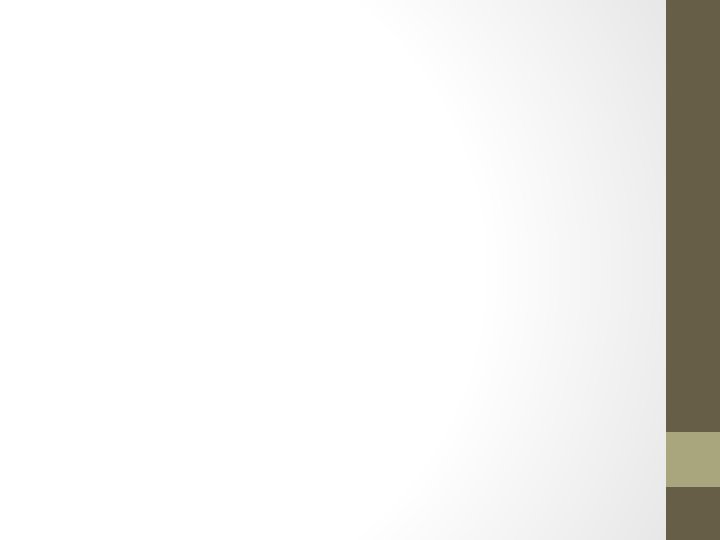
- Slides: 23
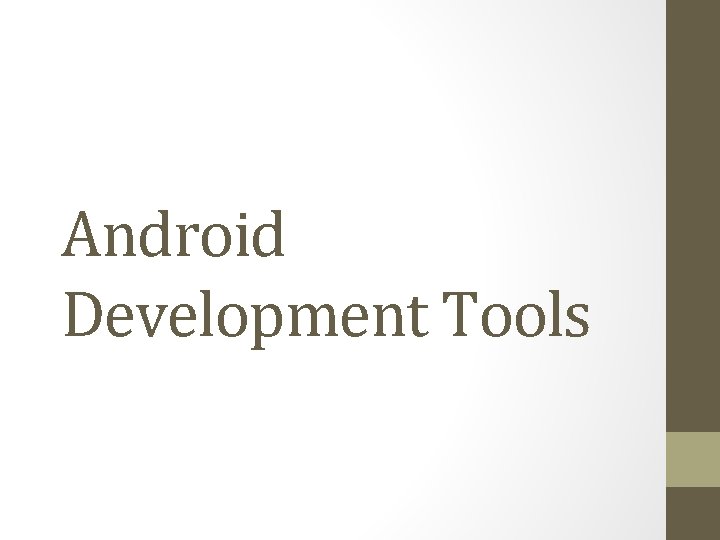
Android Development Tools
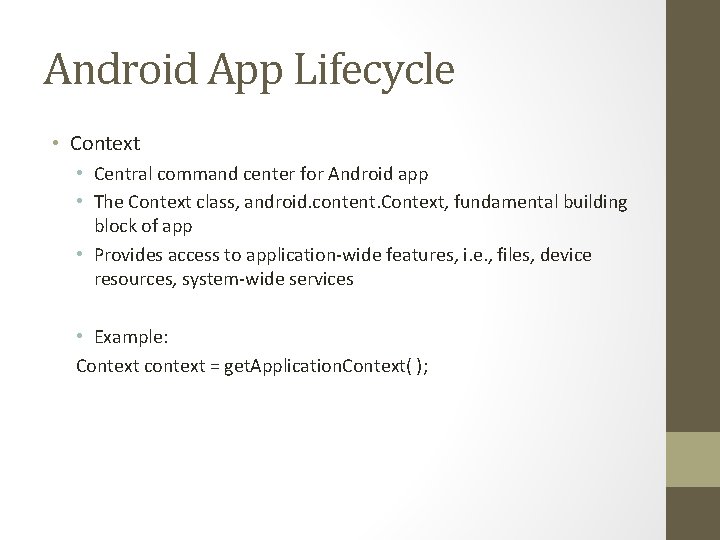
Android App Lifecycle • Context • Central command center for Android app • The Context class, android. content. Context, fundamental building block of app • Provides access to application-wide features, i. e. , files, device resources, system-wide services • Example: Context context = get. Application. Context( );
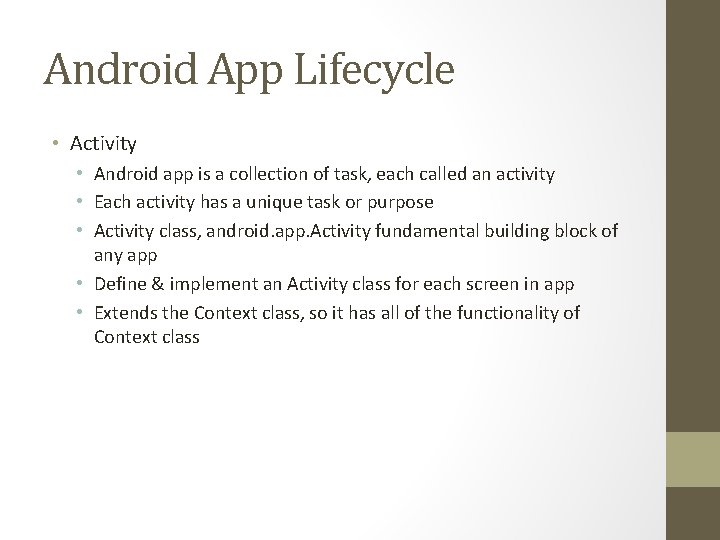
Android App Lifecycle • Activity • Android app is a collection of task, each called an activity • Each activity has a unique task or purpose • Activity class, android. app. Activity fundamental building block of any app • Define & implement an Activity class for each screen in app • Extends the Context class, so it has all of the functionality of Context class
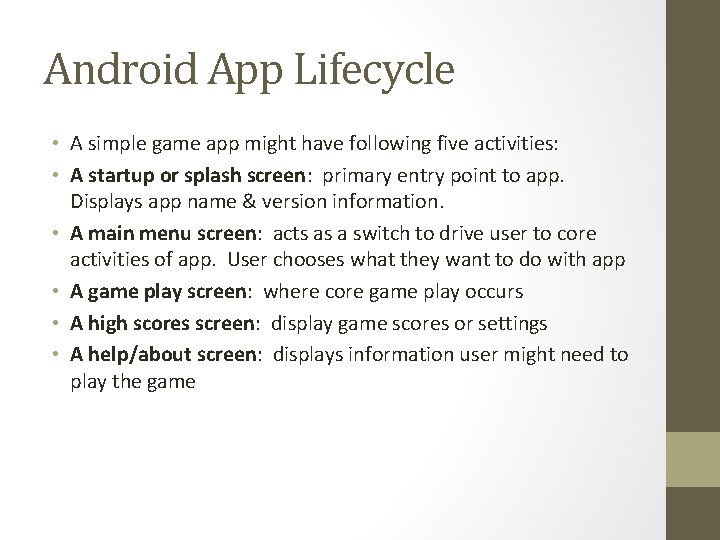
Android App Lifecycle • A simple game app might have following five activities: • A startup or splash screen: primary entry point to app. Displays app name & version information. • A main menu screen: acts as a switch to drive user to core activities of app. User chooses what they want to do with app • A game play screen: where core game play occurs • A high scores screen: display game scores or settings • A help/about screen: displays information user might need to play the game
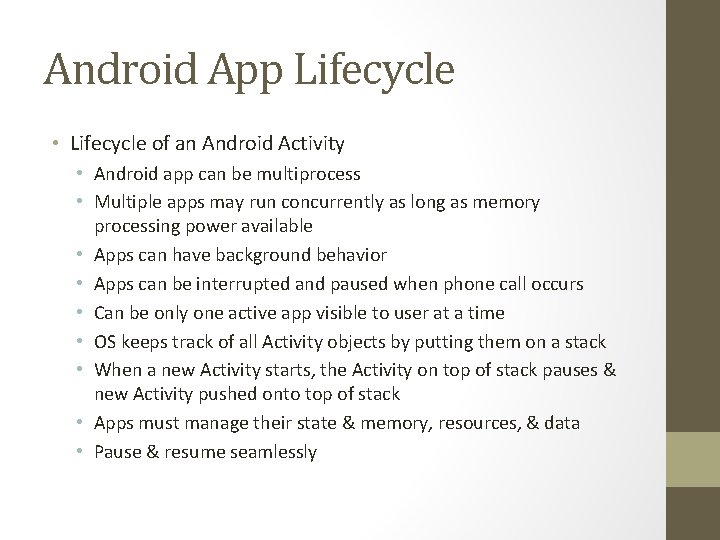
Android App Lifecycle • Lifecycle of an Android Activity • Android app can be multiprocess • Multiple apps may run concurrently as long as memory processing power available • Apps can have background behavior • Apps can be interrupted and paused when phone call occurs • Can be only one active app visible to user at a time • OS keeps track of all Activity objects by putting them on a stack • When a new Activity starts, the Activity on top of stack pauses & new Activity pushed onto top of stack • Apps must manage their state & memory, resources, & data • Pause & resume seamlessly
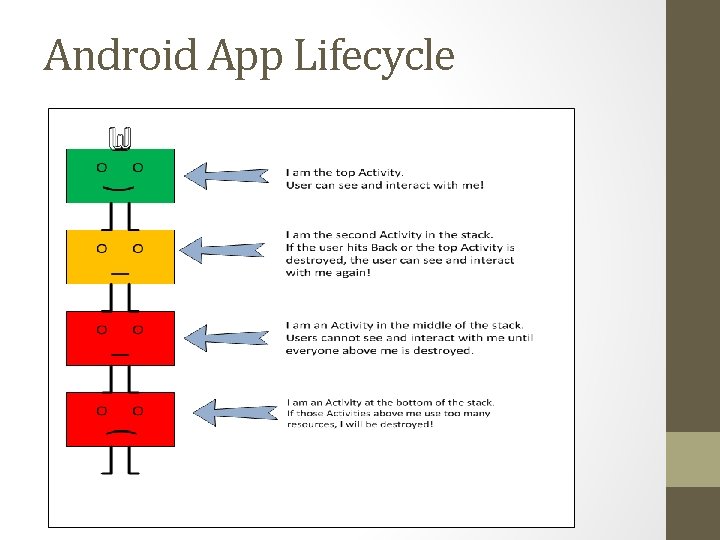
Android App Lifecycle
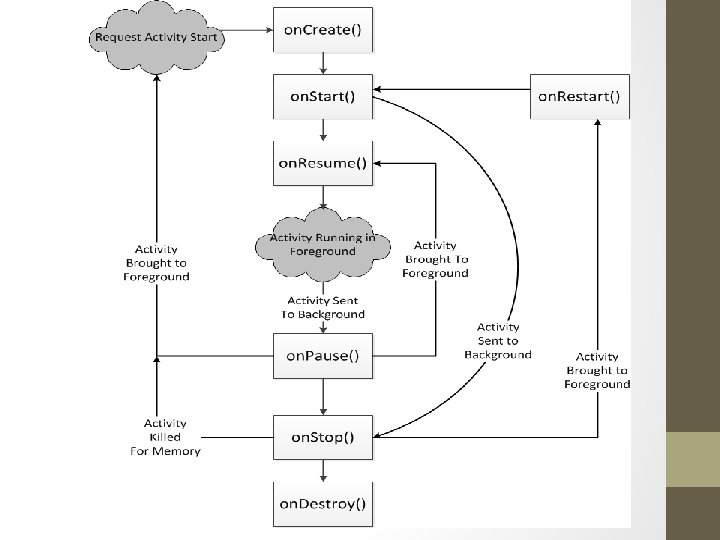
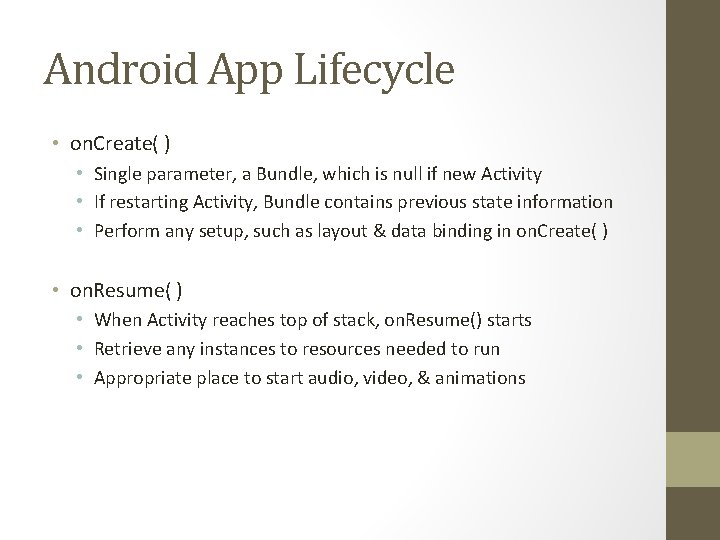
Android App Lifecycle • on. Create( ) • Single parameter, a Bundle, which is null if new Activity • If restarting Activity, Bundle contains previous state information • Perform any setup, such as layout & data binding in on. Create( ) • on. Resume( ) • When Activity reaches top of stack, on. Resume() starts • Retrieve any instances to resources needed to run • Appropriate place to start audio, video, & animations
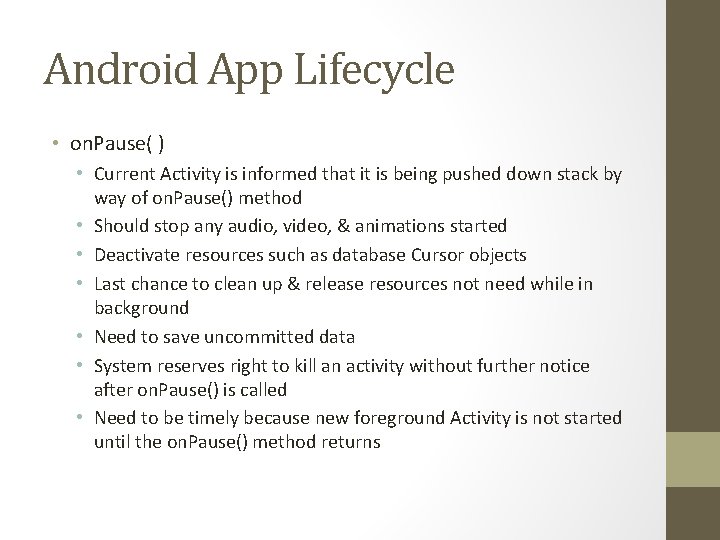
Android App Lifecycle • on. Pause( ) • Current Activity is informed that it is being pushed down stack by way of on. Pause() method • Should stop any audio, video, & animations started • Deactivate resources such as database Cursor objects • Last chance to clean up & release resources not need while in background • Need to save uncommitted data • System reserves right to kill an activity without further notice after on. Pause() is called • Need to be timely because new foreground Activity is not started until the on. Pause() method returns
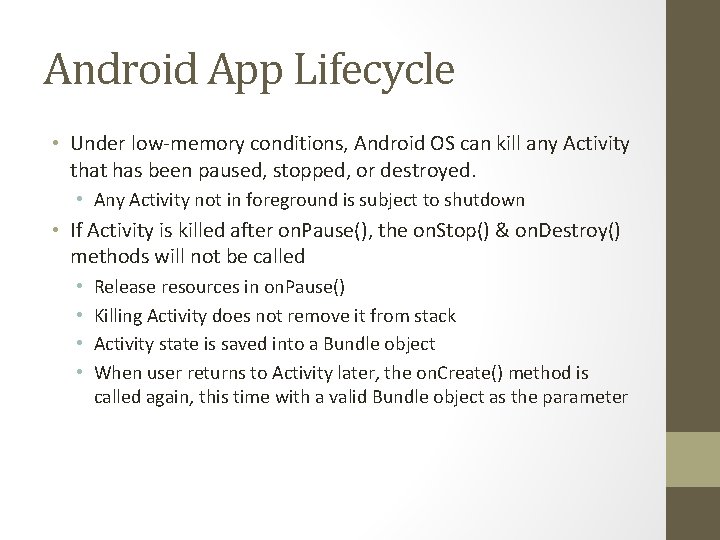
Android App Lifecycle • Under low-memory conditions, Android OS can kill any Activity that has been paused, stopped, or destroyed. • Any Activity not in foreground is subject to shutdown • If Activity is killed after on. Pause(), the on. Stop() & on. Destroy() methods will not be called • • Release resources in on. Pause() Killing Activity does not remove it from stack Activity state is saved into a Bundle object When user returns to Activity later, the on. Create() method is called again, this time with a valid Bundle object as the parameter
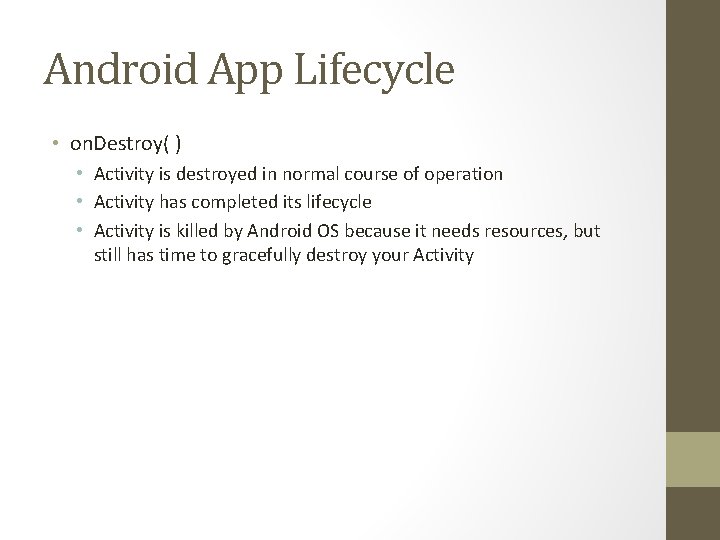
Android App Lifecycle • on. Destroy( ) • Activity is destroyed in normal course of operation • Activity has completed its lifecycle • Activity is killed by Android OS because it needs resources, but still has time to gracefully destroy your Activity
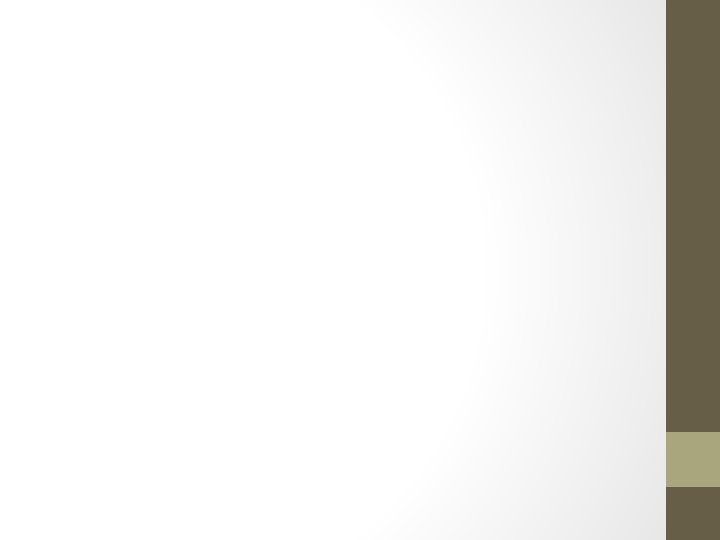
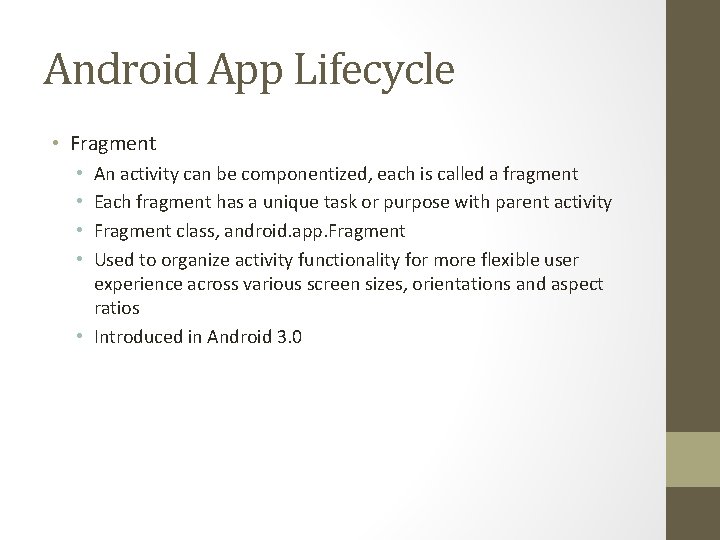
Android App Lifecycle • Fragment An activity can be componentized, each is called a fragment Each fragment has a unique task or purpose with parent activity Fragment class, android. app. Fragment Used to organize activity functionality for more flexible user experience across various screen sizes, orientations and aspect ratios • Introduced in Android 3. 0 • •
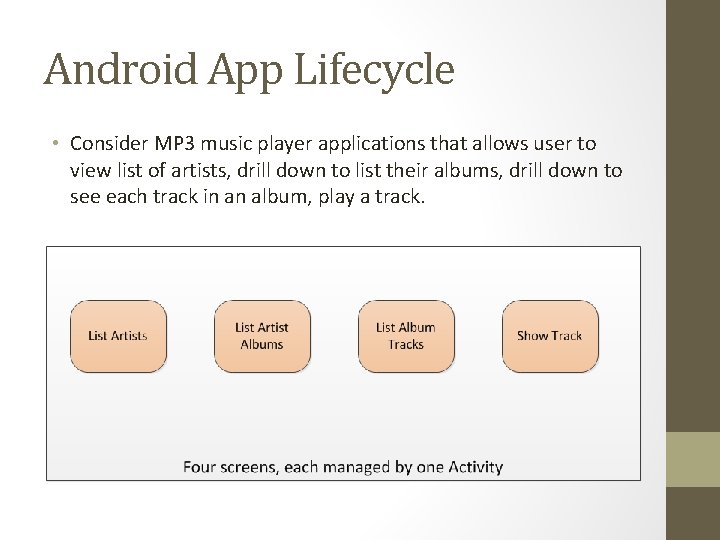
Android App Lifecycle • Consider MP 3 music player applications that allows user to view list of artists, drill down to list their albums, drill down to see each track in an album, play a track.
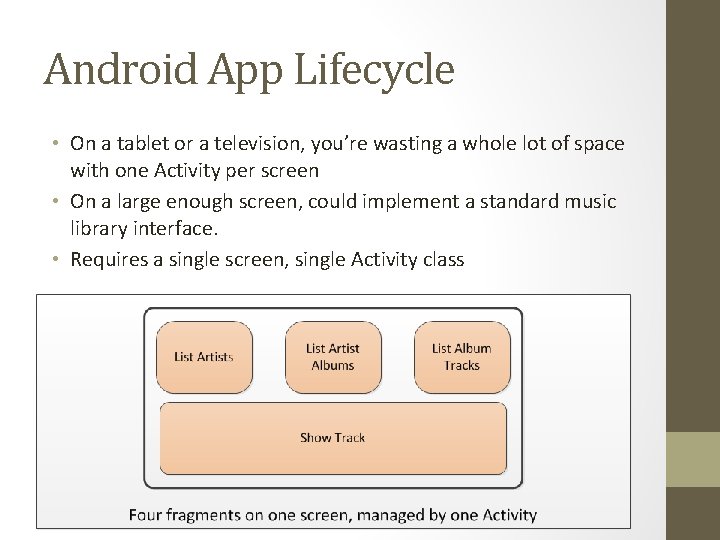
Android App Lifecycle • On a tablet or a television, you’re wasting a whole lot of space with one Activity per screen • On a large enough screen, could implement a standard music library interface. • Requires a single screen, single Activity class
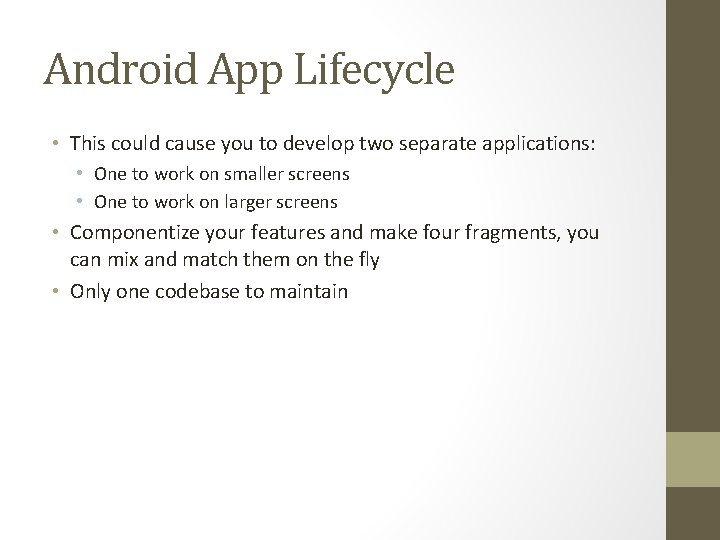
Android App Lifecycle • This could cause you to develop two separate applications: • One to work on smaller screens • One to work on larger screens • Componentize your features and make four fragments, you can mix and match them on the fly • Only one codebase to maintain
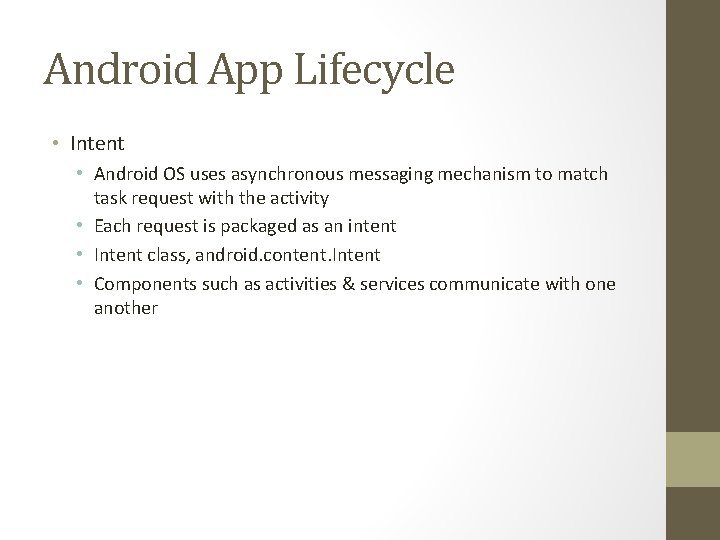
Android App Lifecycle • Intent • Android OS uses asynchronous messaging mechanism to match task request with the activity • Each request is packaged as an intent • Intent class, android. content. Intent • Components such as activities & services communicate with one another
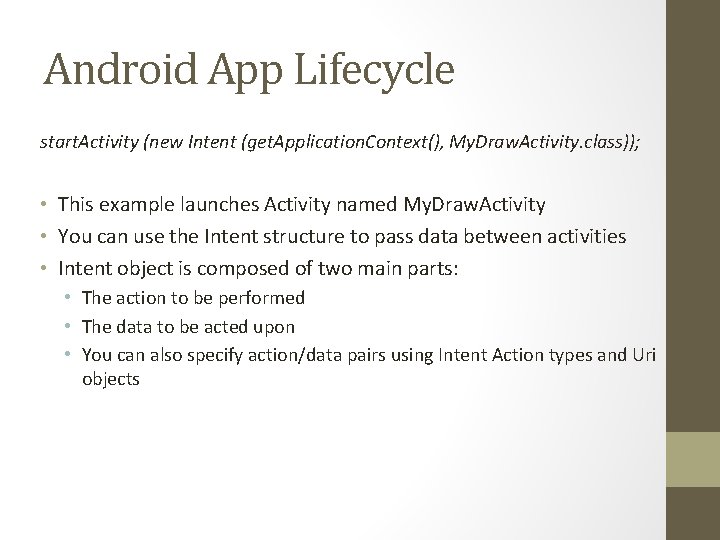
Android App Lifecycle start. Activity (new Intent (get. Application. Context(), My. Draw. Activity. class)); • This example launches Activity named My. Draw. Activity • You can use the Intent structure to pass data between activities • Intent object is composed of two main parts: • The action to be performed • The data to be acted upon • You can also specify action/data pairs using Intent Action types and Uri objects
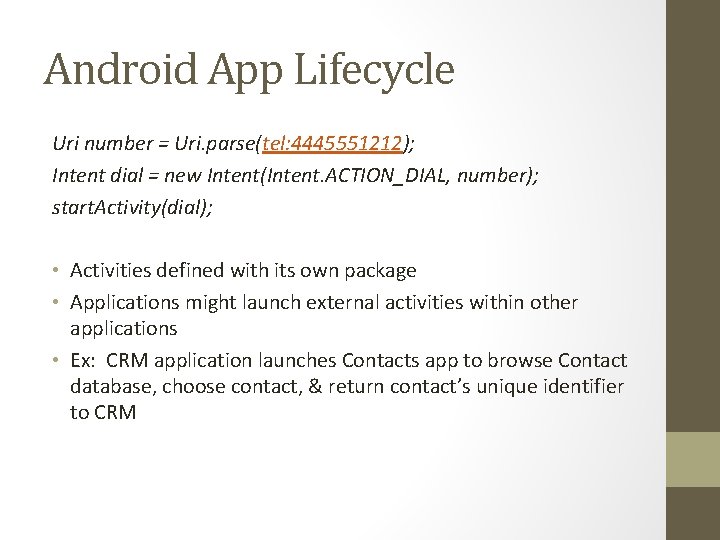
Android App Lifecycle Uri number = Uri. parse(tel: 4445551212); Intent dial = new Intent(Intent. ACTION_DIAL, number); start. Activity(dial); • Activities defined with its own package • Applications might launch external activities within other applications • Ex: CRM application launches Contacts app to browse Contact database, choose contact, & return contact’s unique identifier to CRM
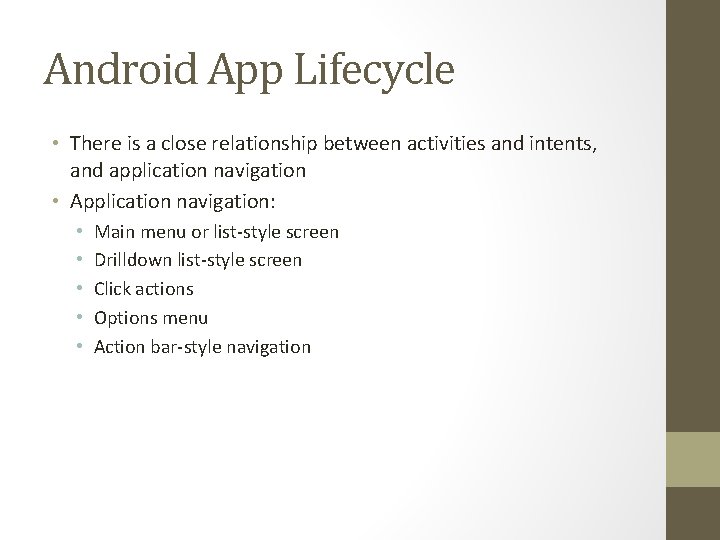
Android App Lifecycle • There is a close relationship between activities and intents, and application navigation • Application navigation: • • • Main menu or list-style screen Drilldown list-style screen Click actions Options menu Action bar-style navigation
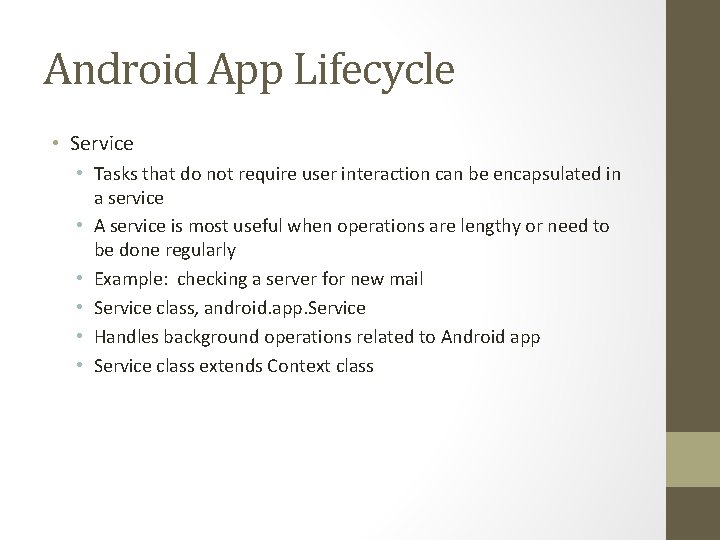
Android App Lifecycle • Service • Tasks that do not require user interaction can be encapsulated in a service • A service is most useful when operations are lengthy or need to be done regularly • Example: checking a server for new mail • Service class, android. app. Service • Handles background operations related to Android app • Service class extends Context class
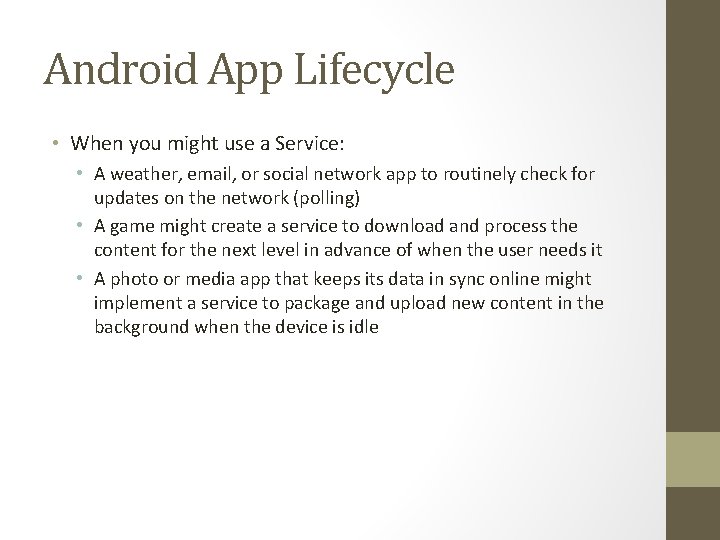
Android App Lifecycle • When you might use a Service: • A weather, email, or social network app to routinely check for updates on the network (polling) • A game might create a service to download and process the content for the next level in advance of when the user needs it • A photo or media app that keeps its data in sync online might implement a service to package and upload new content in the background when the device is idle
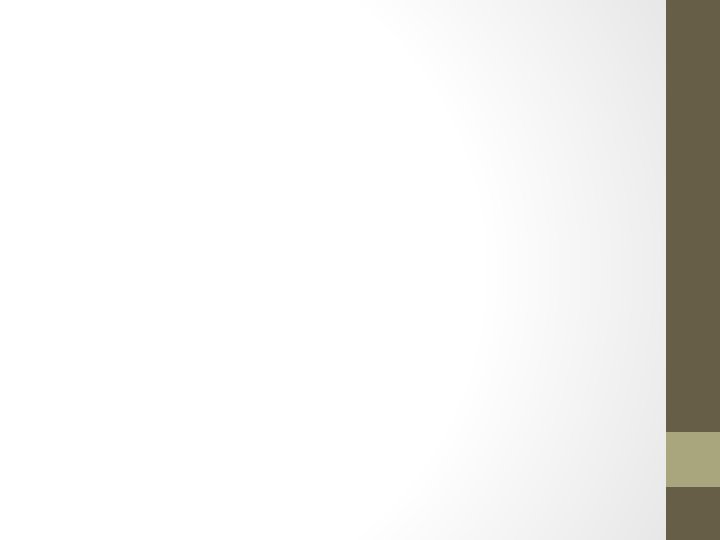