An Introduction to C Programming Software are programs
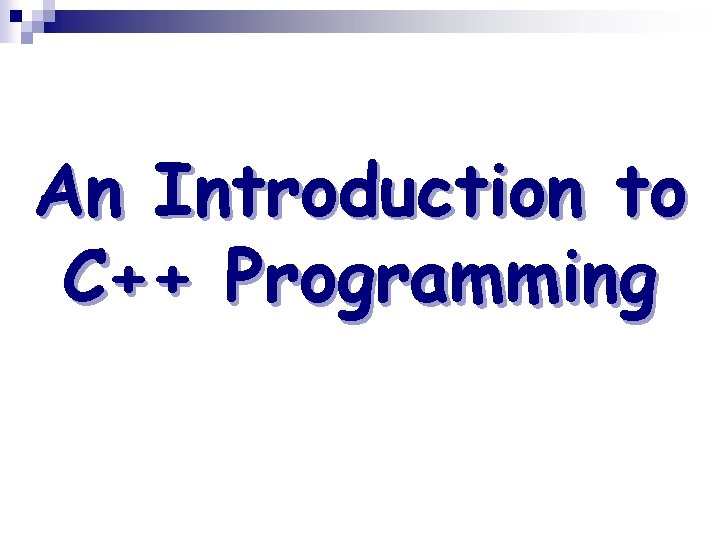
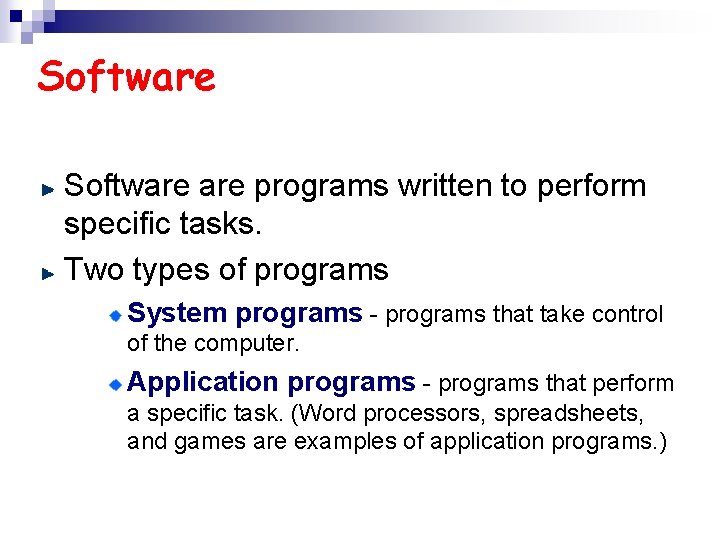
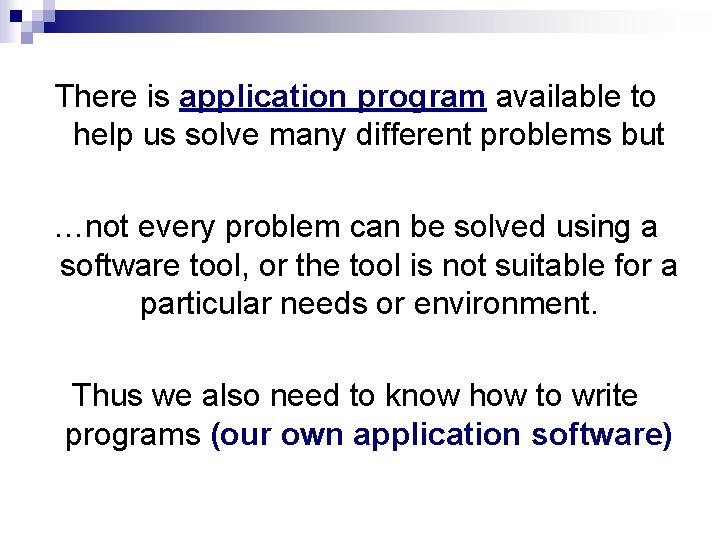
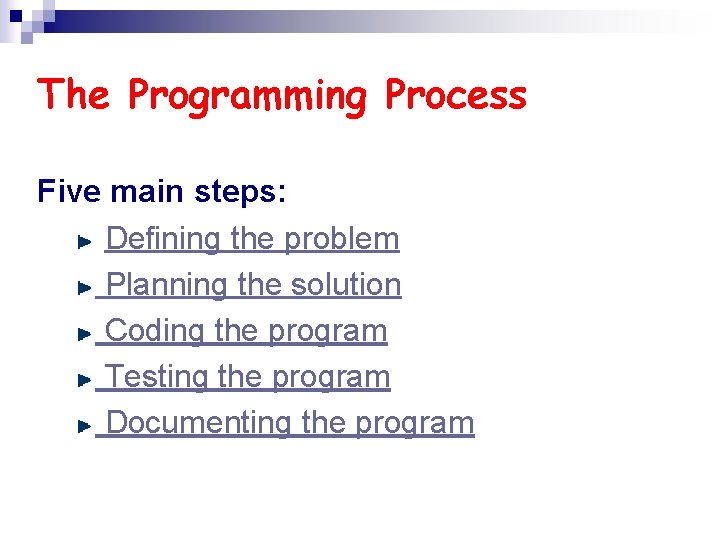
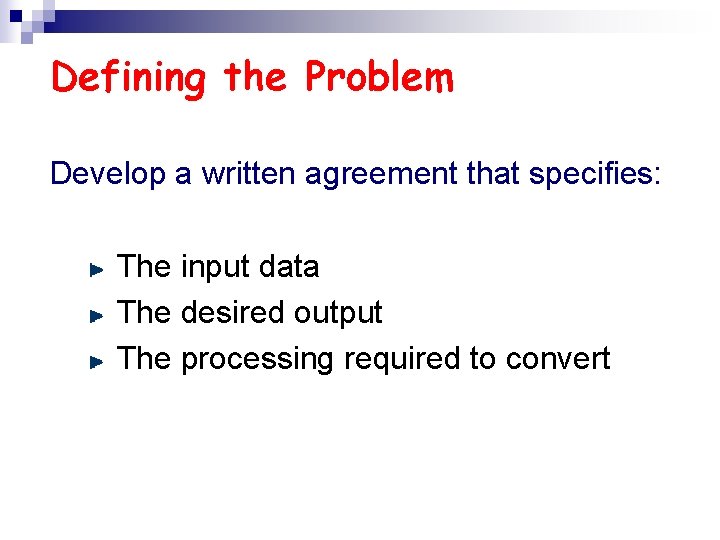
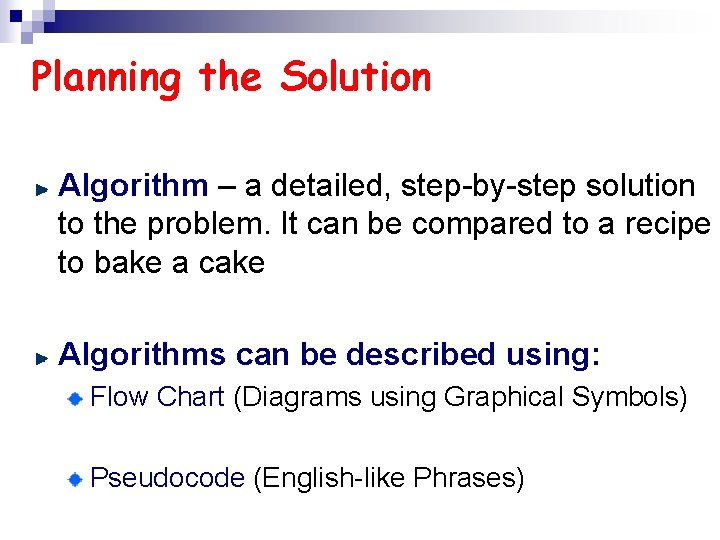
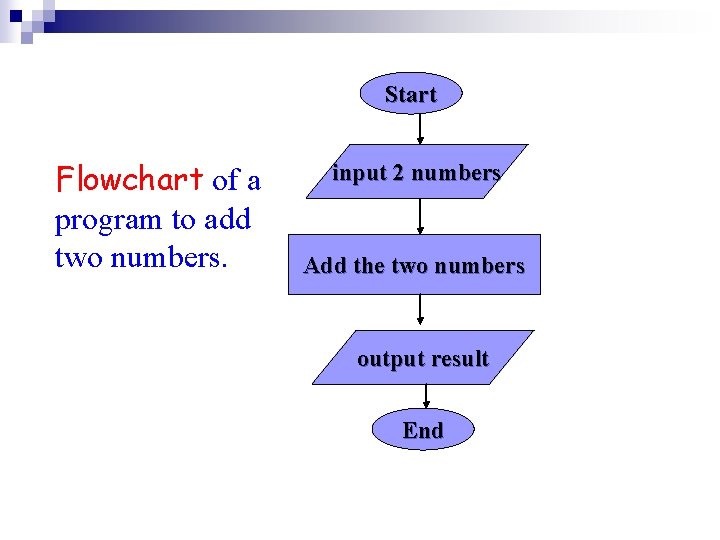
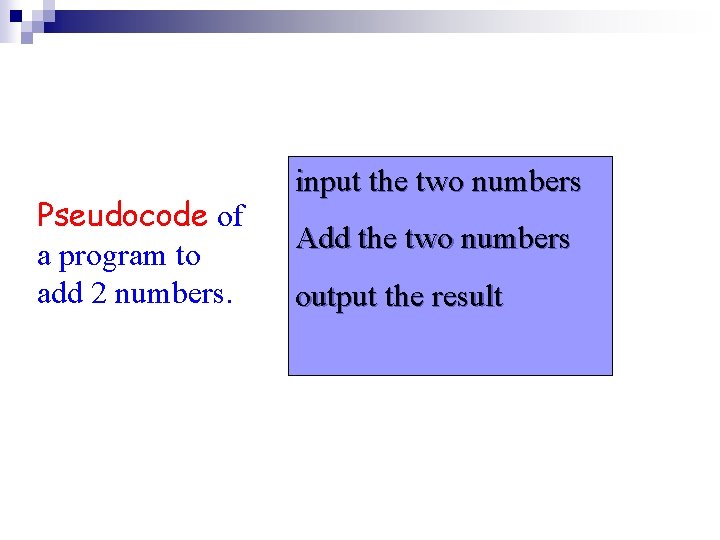
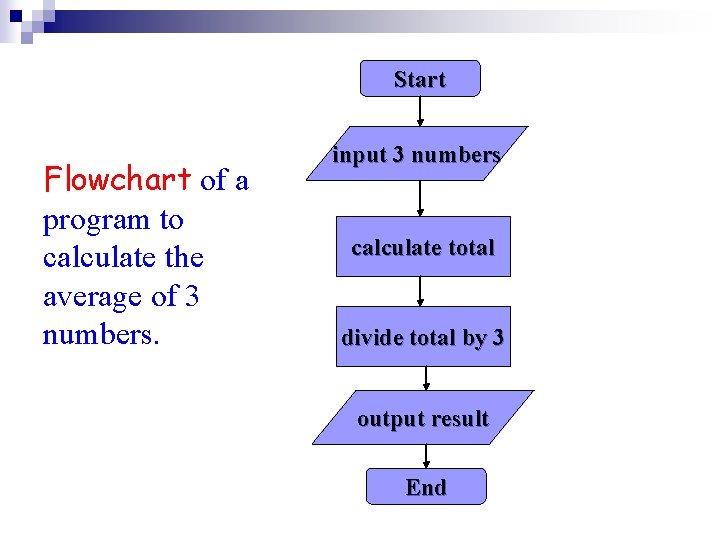
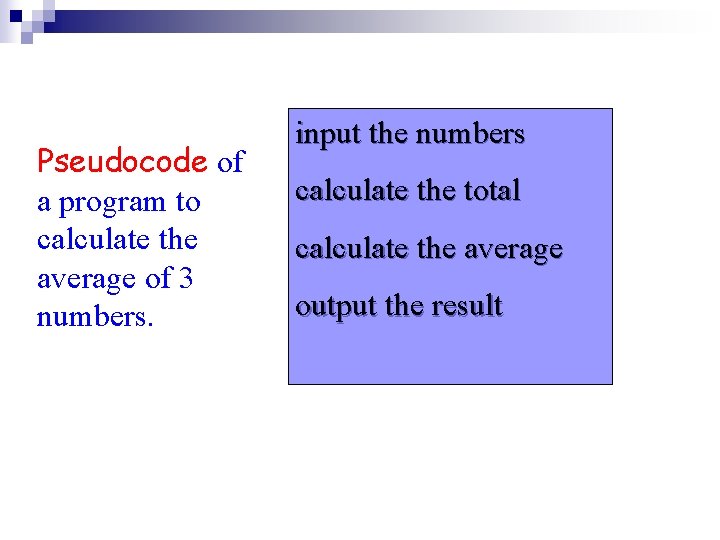
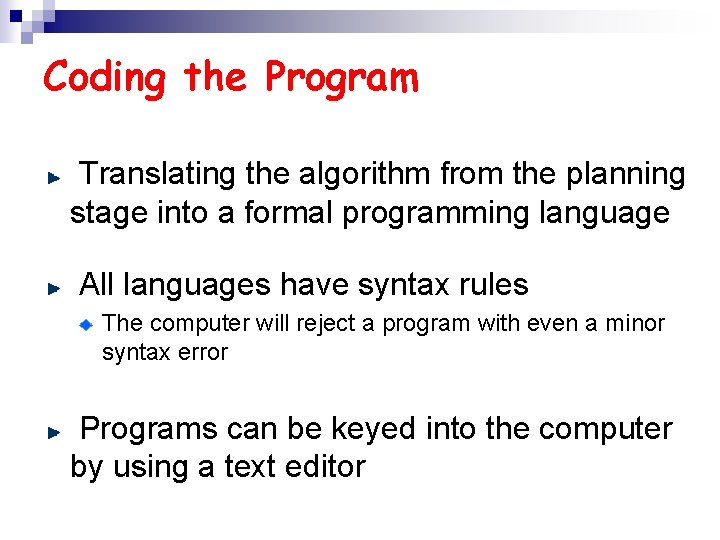
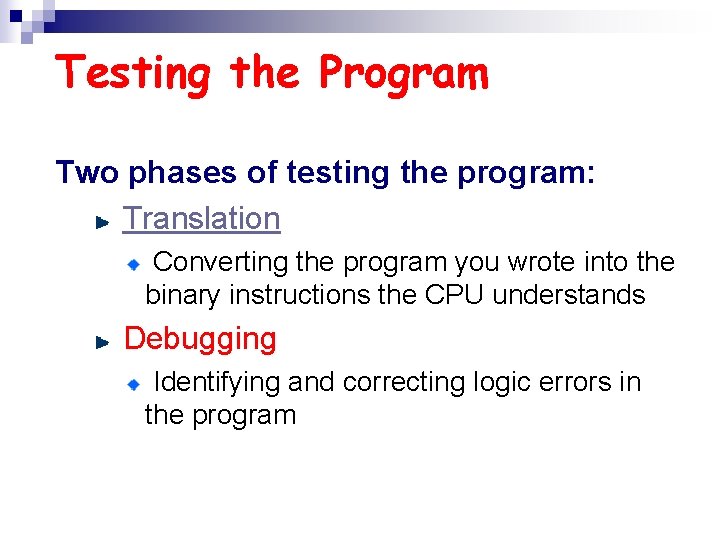
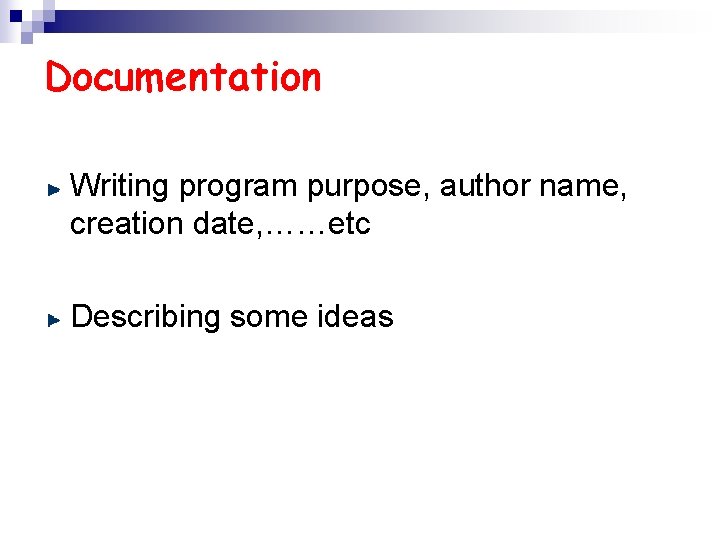
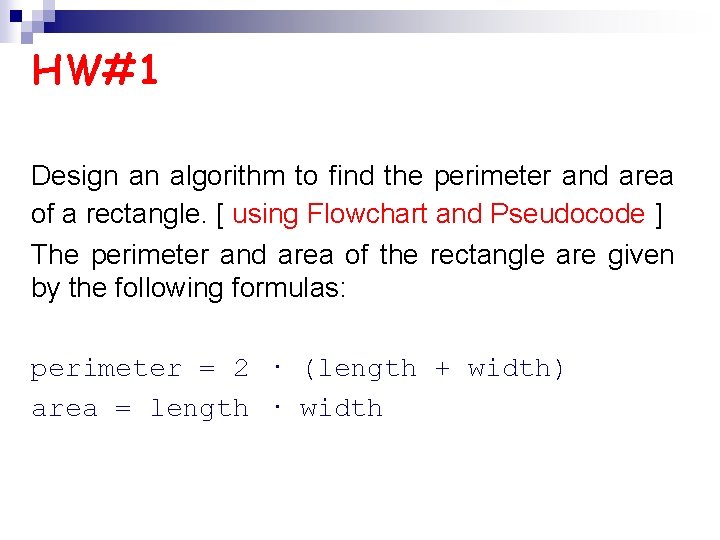
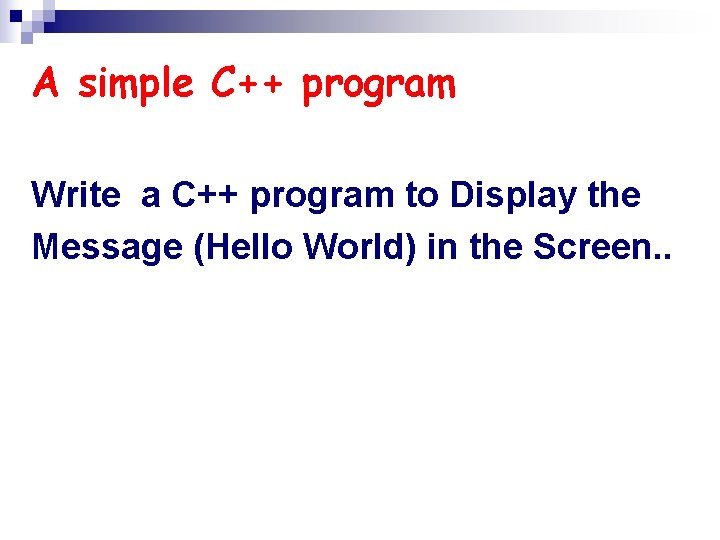
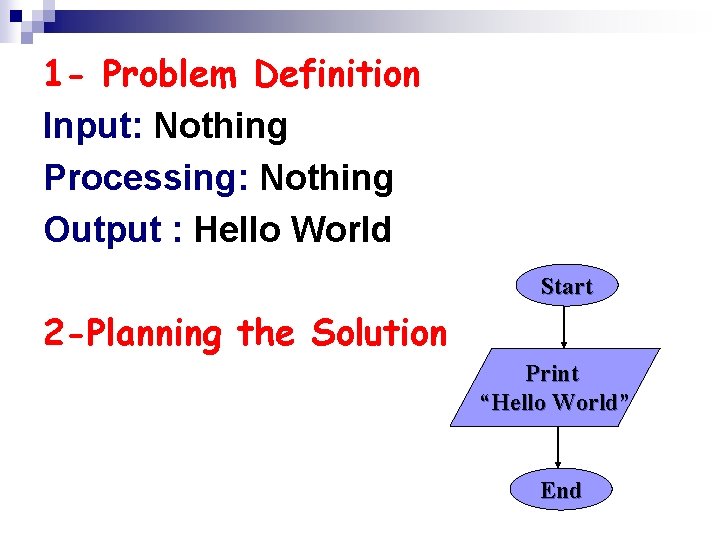
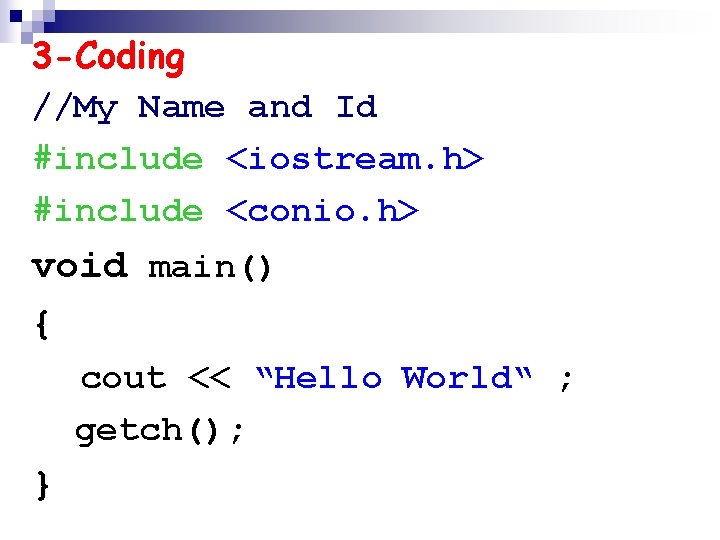
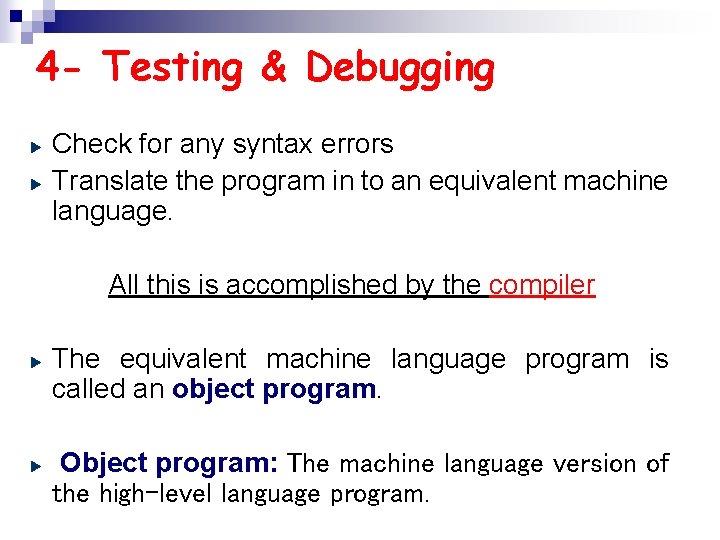
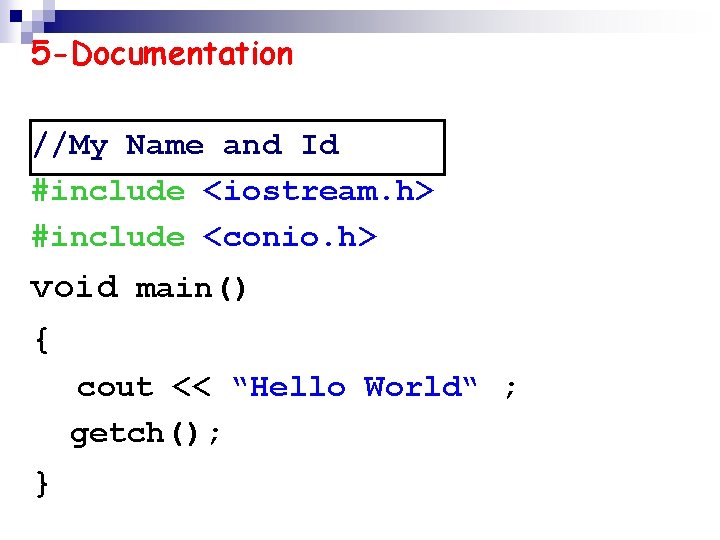
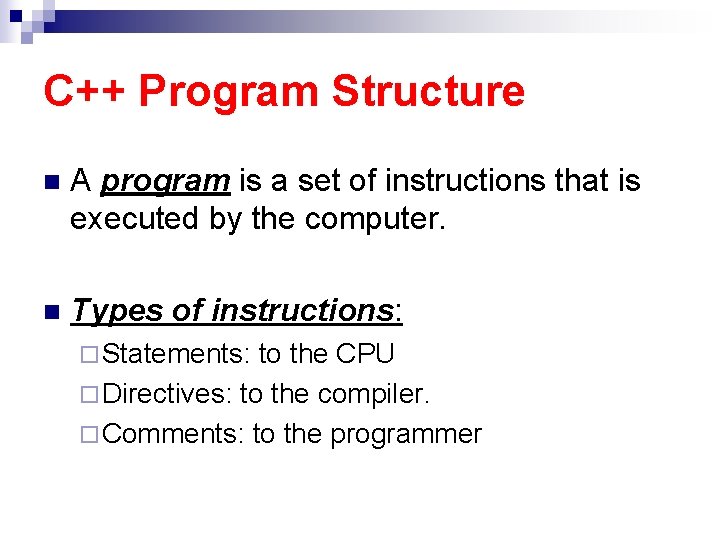
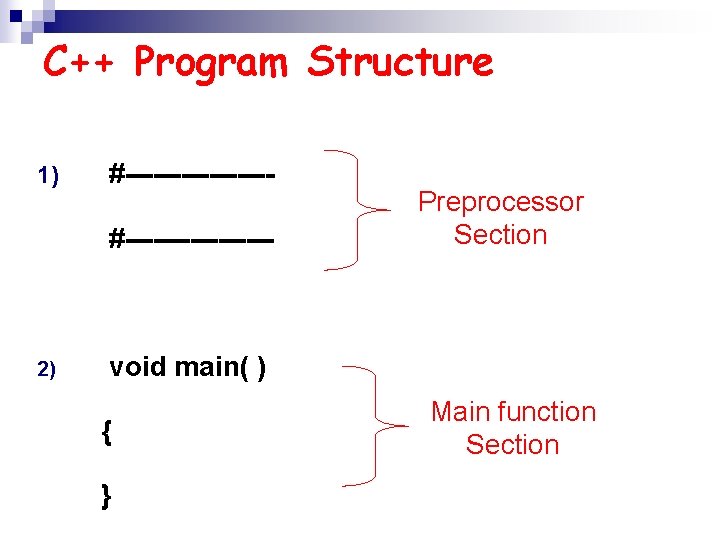
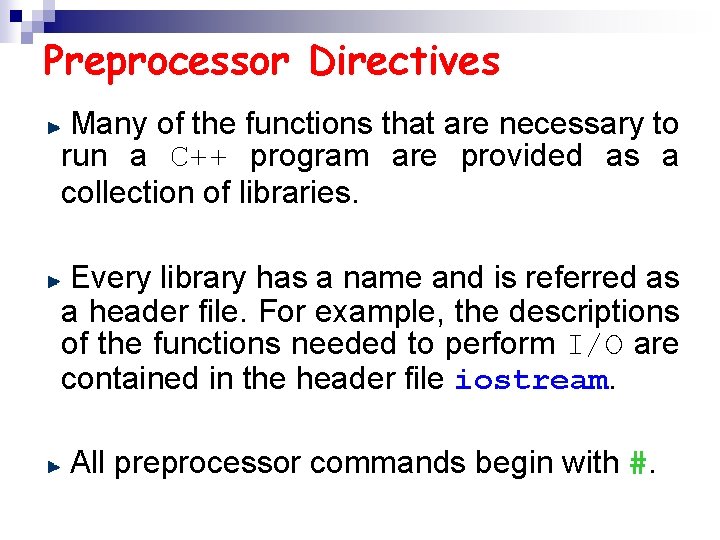
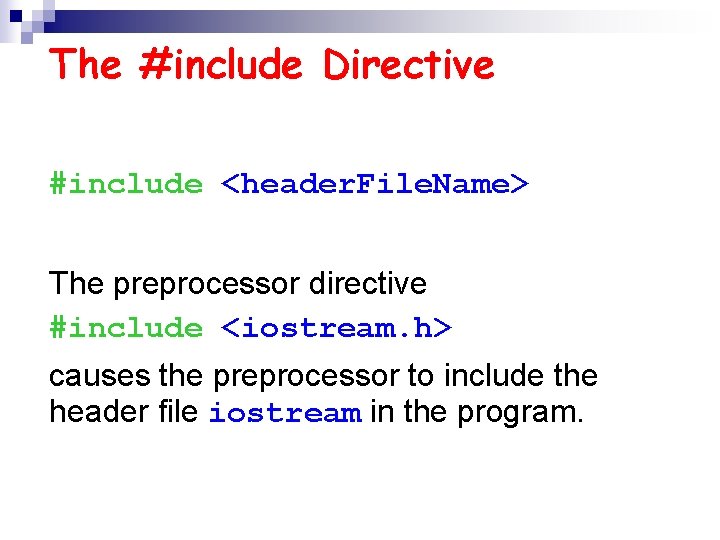
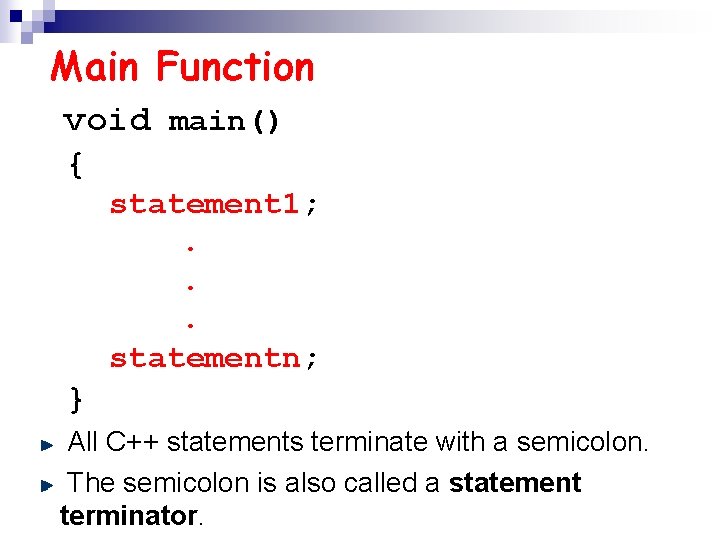
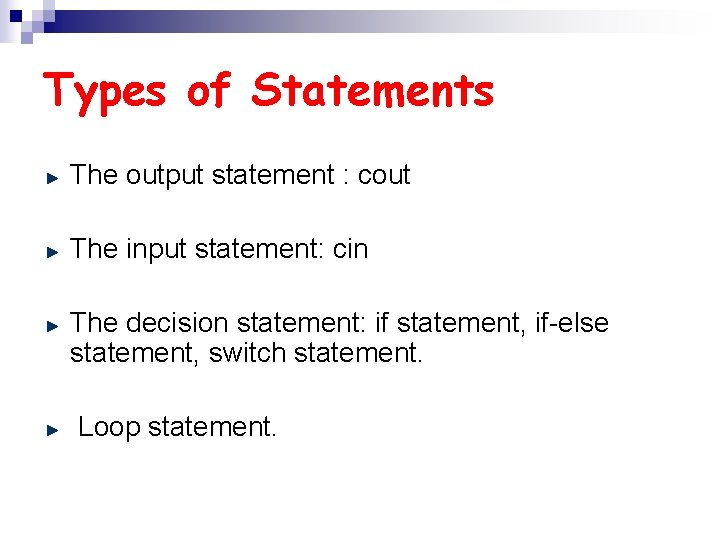
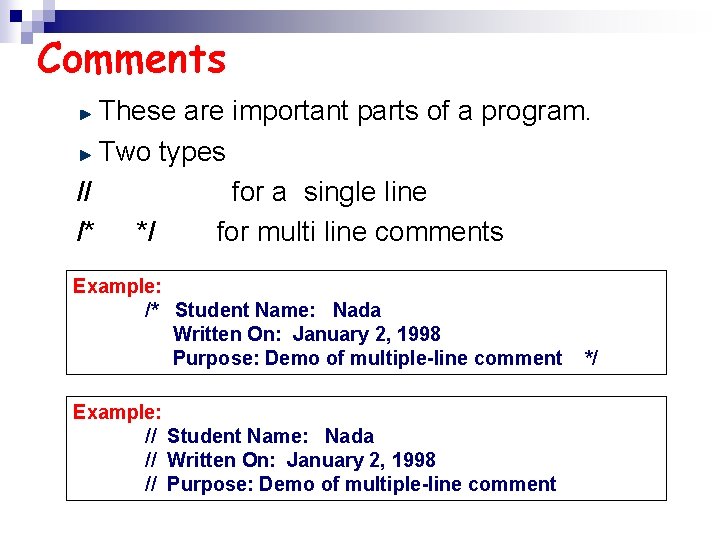
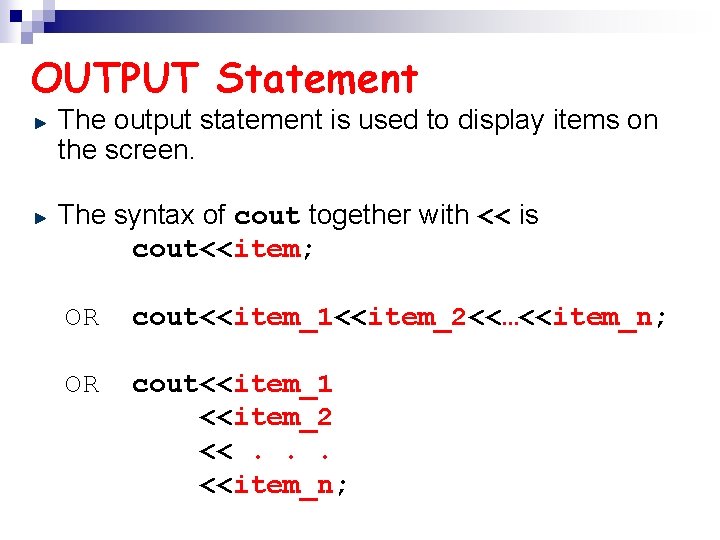
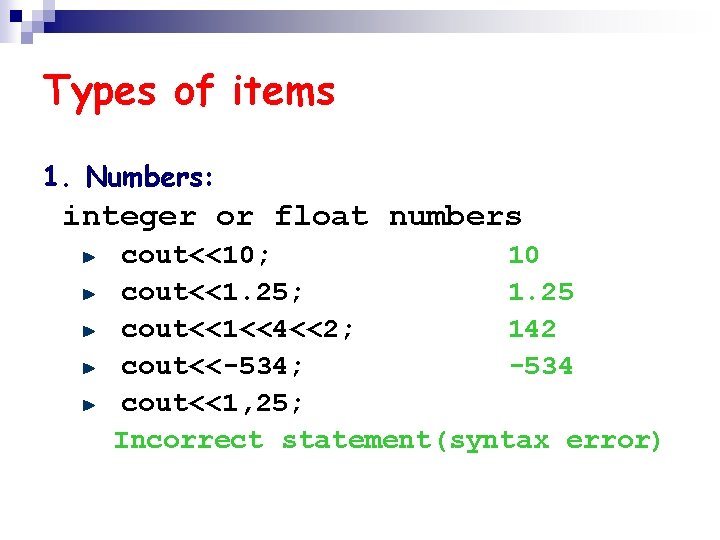
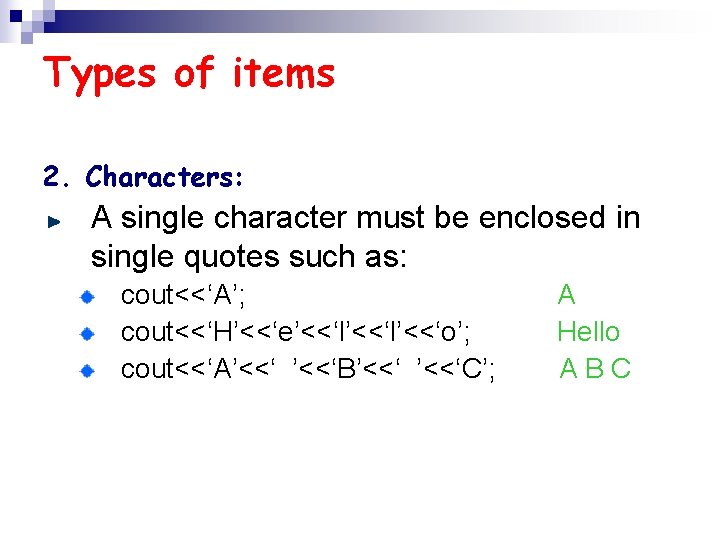
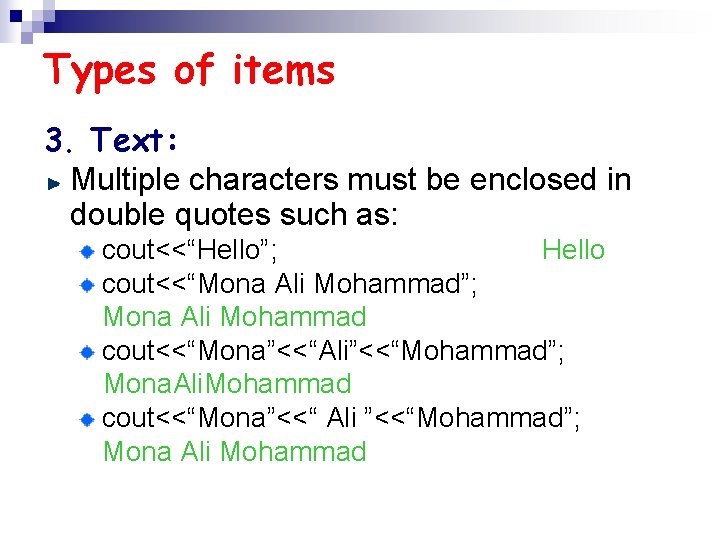
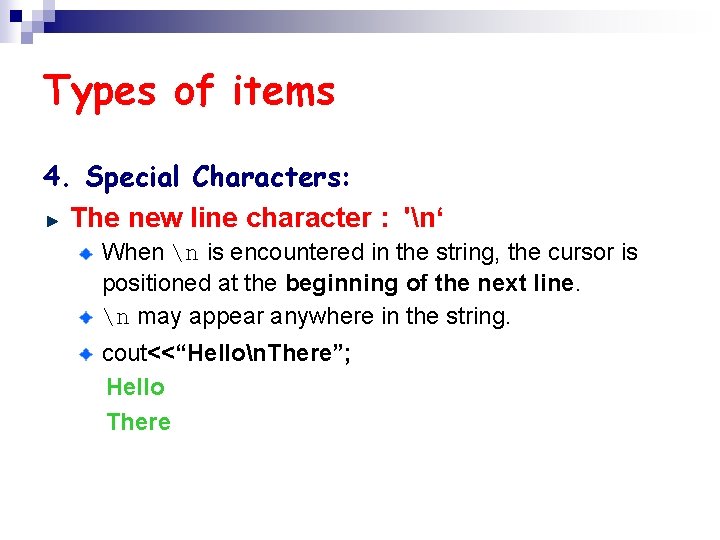
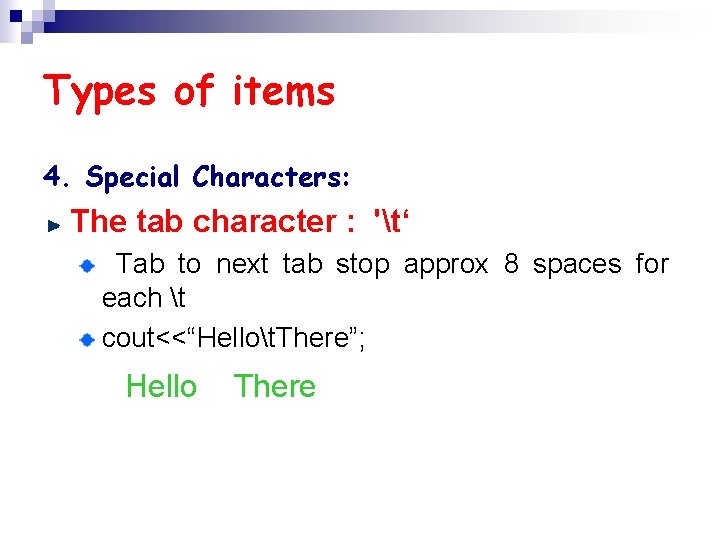
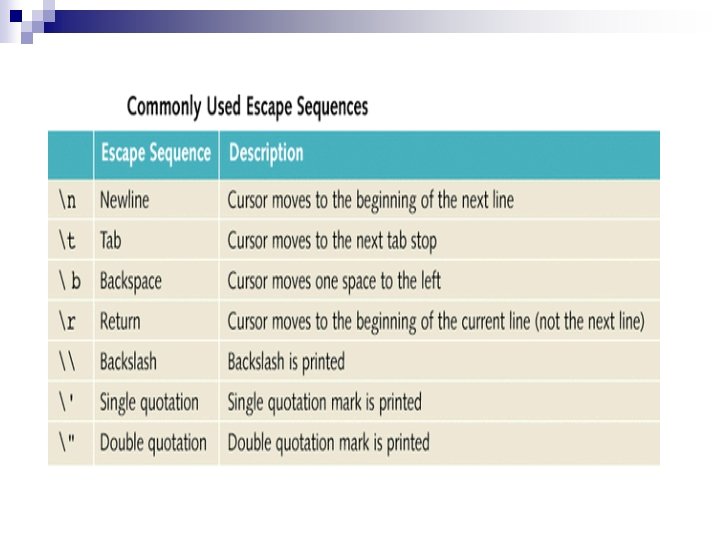
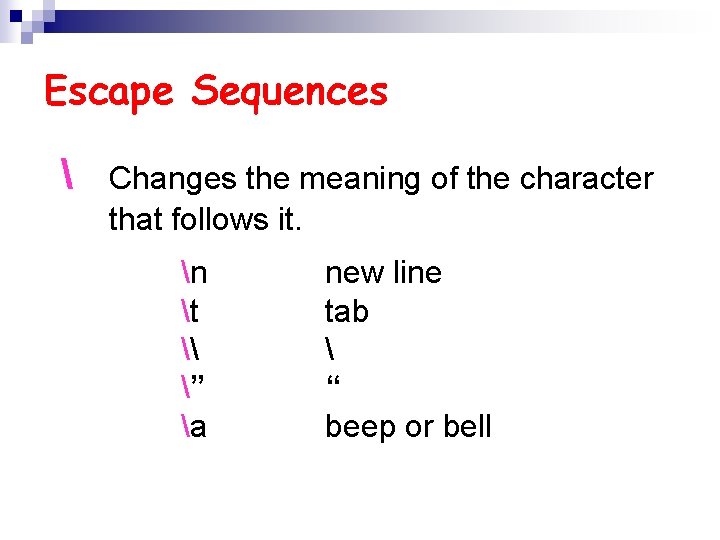
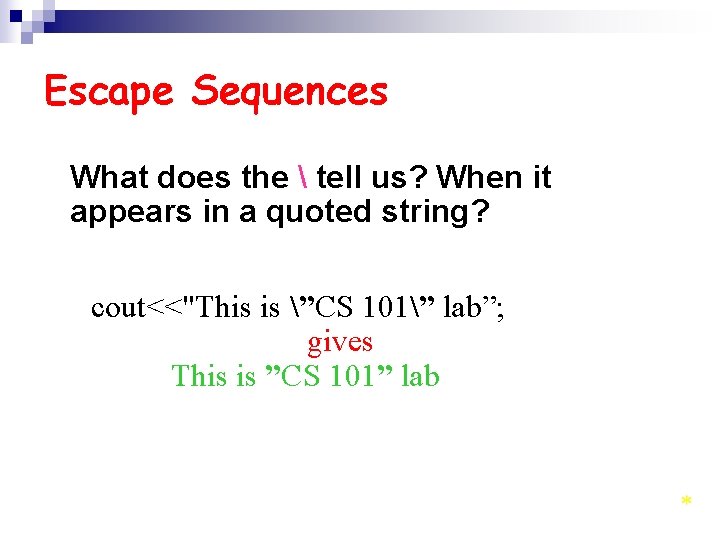
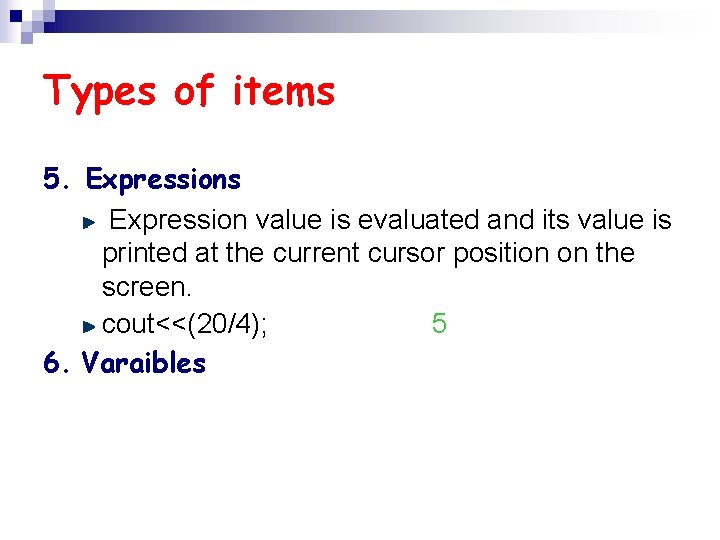
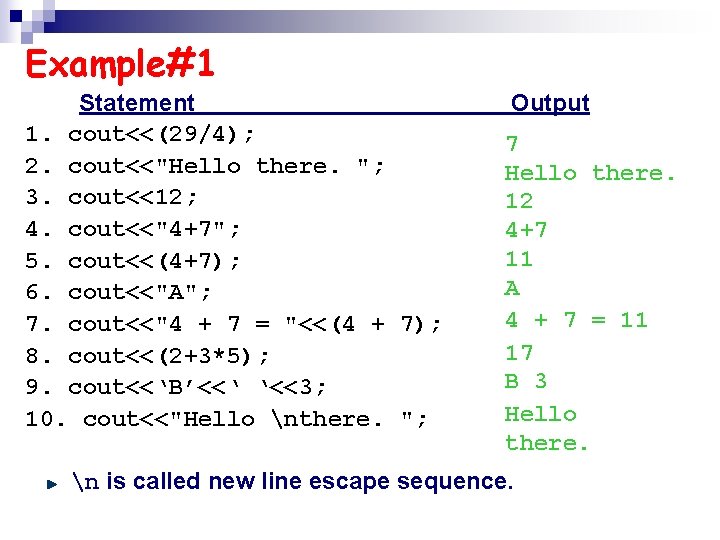
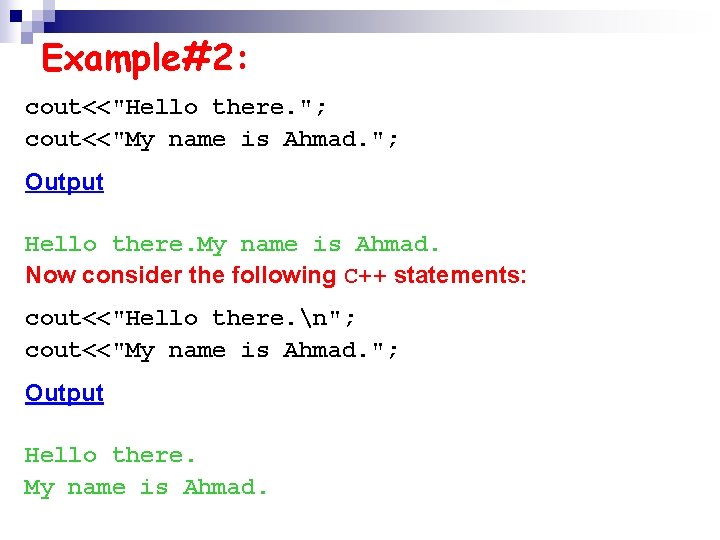
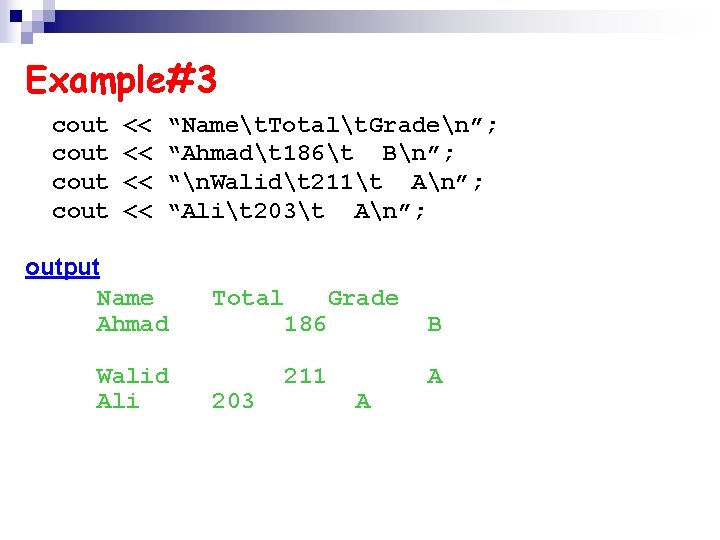
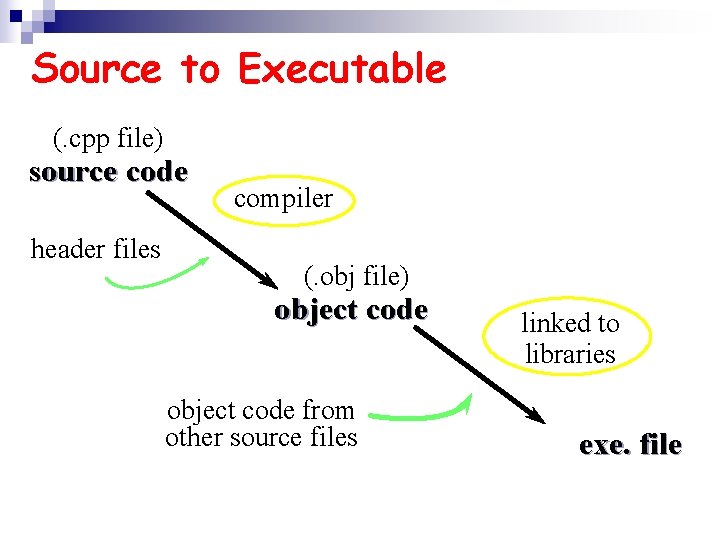
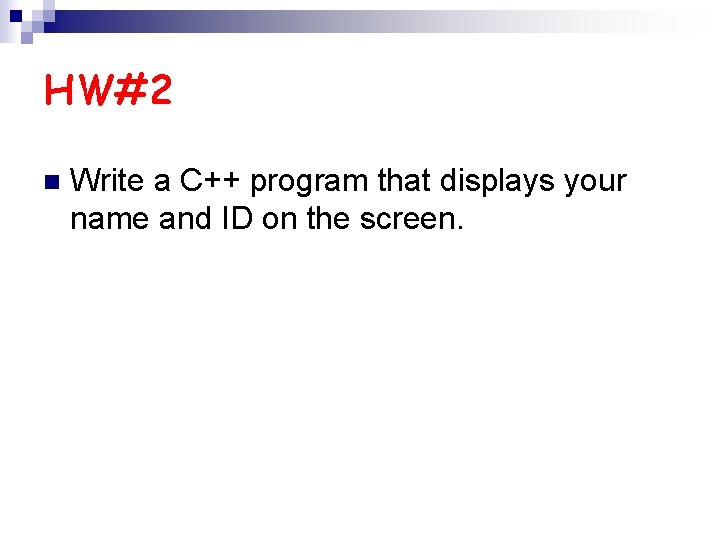
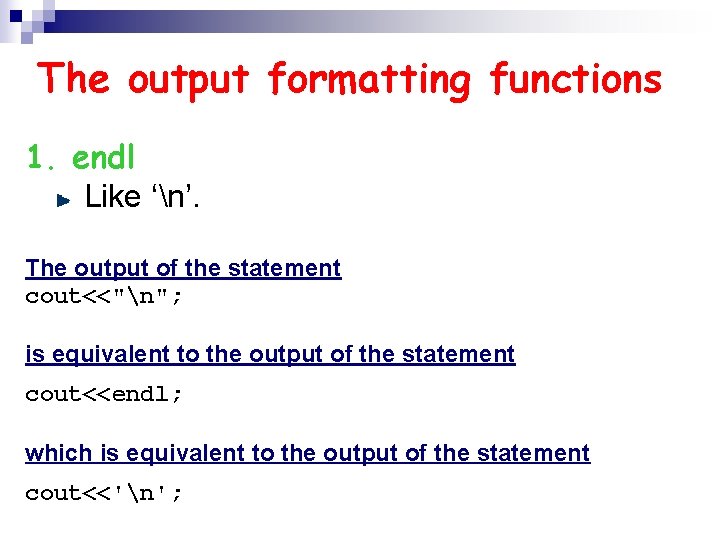
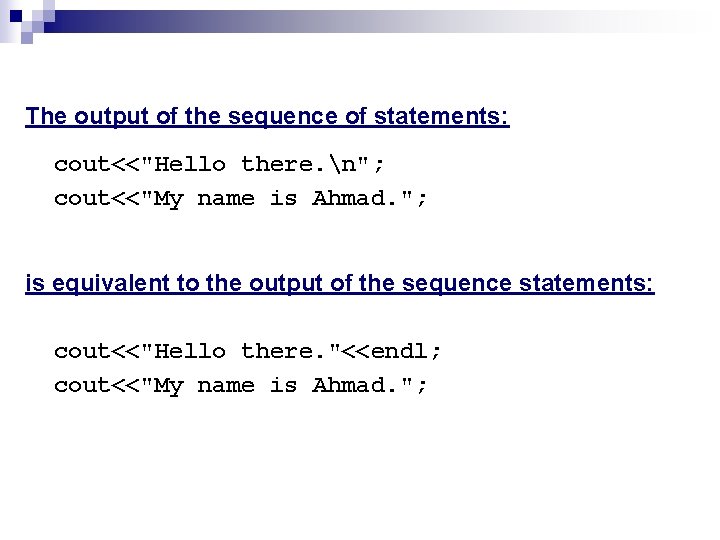
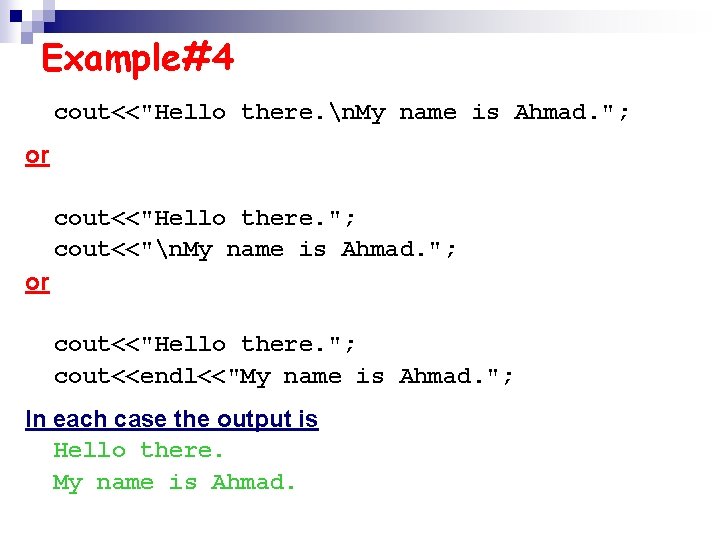
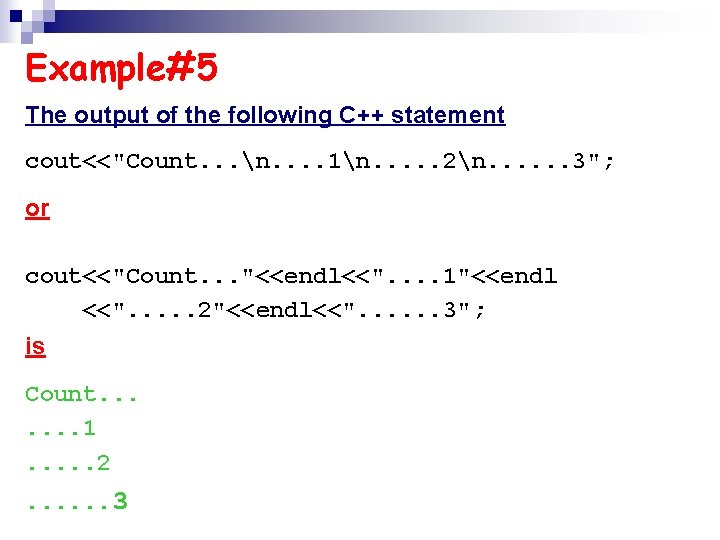
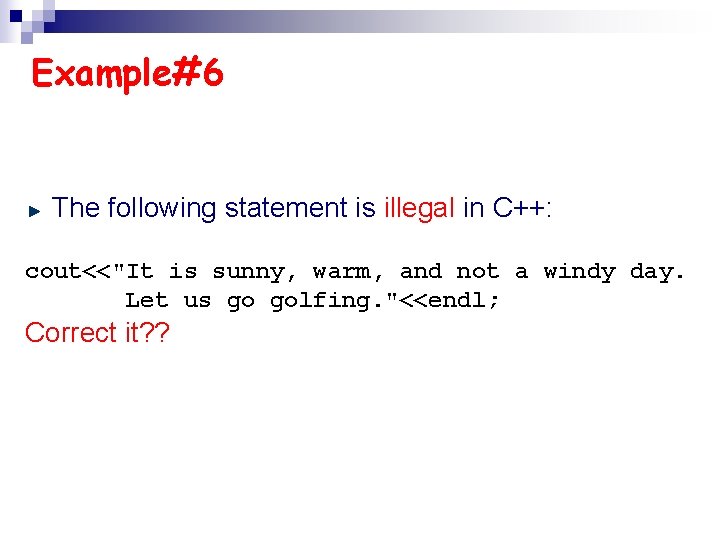
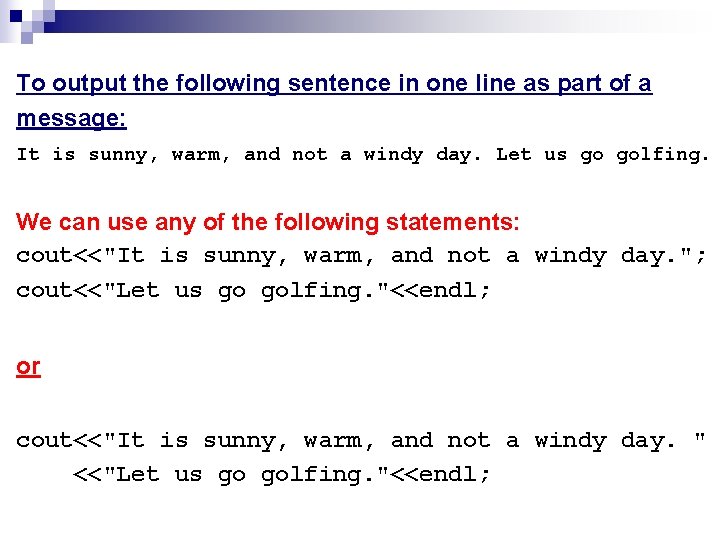
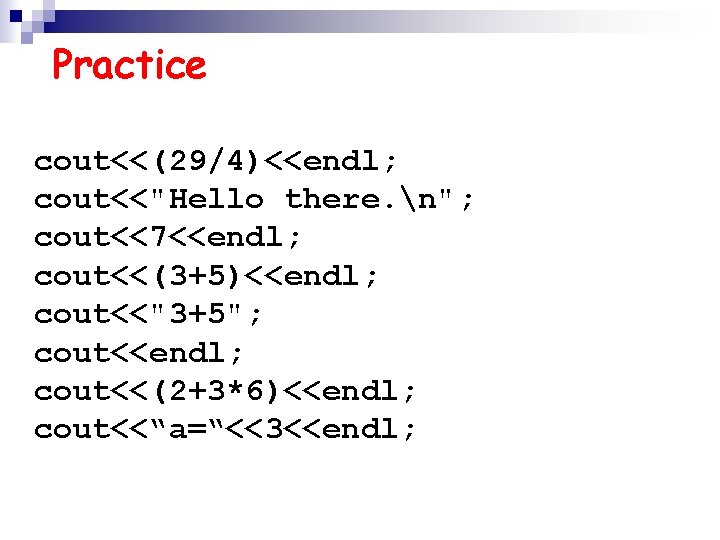
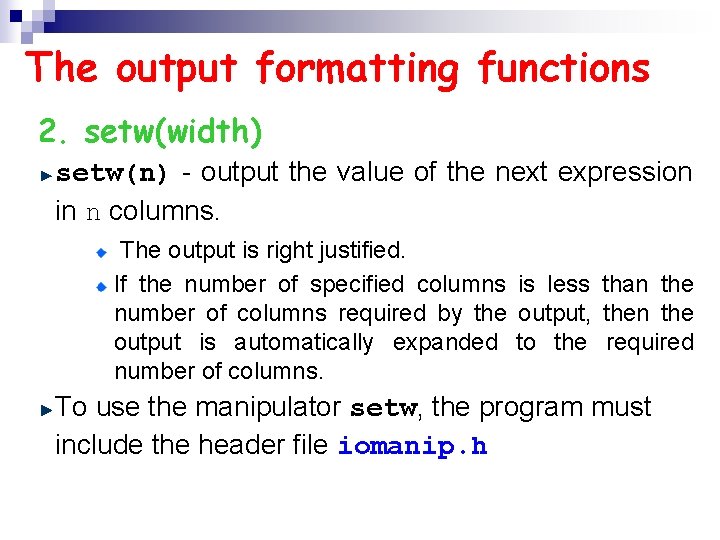
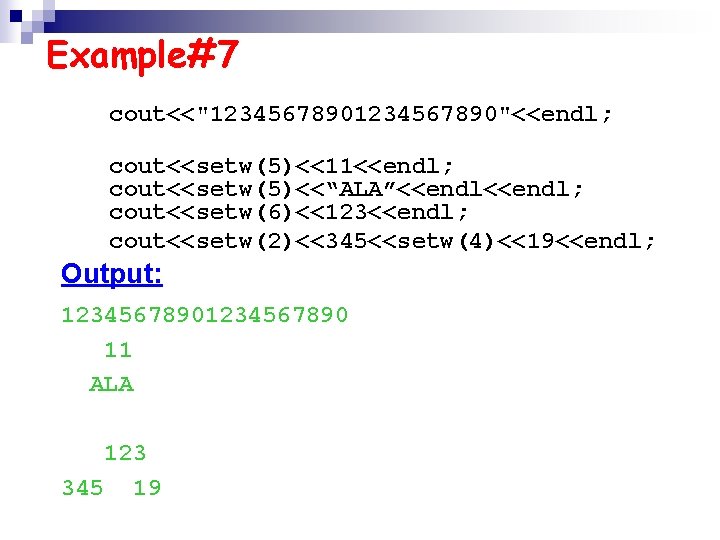
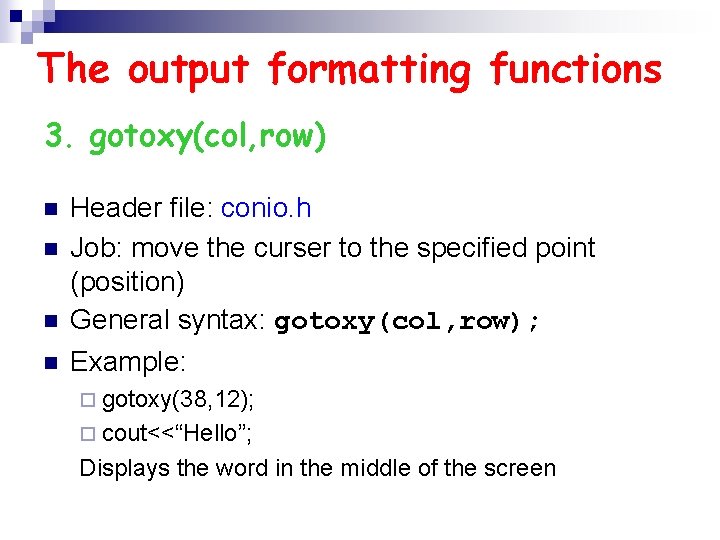
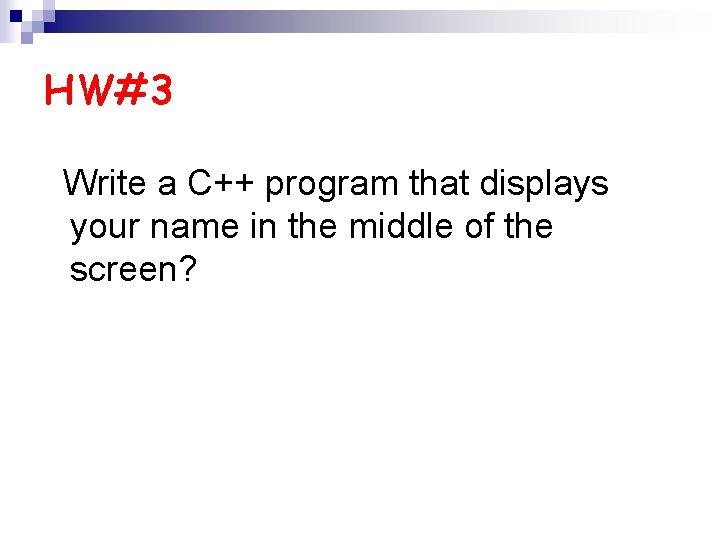
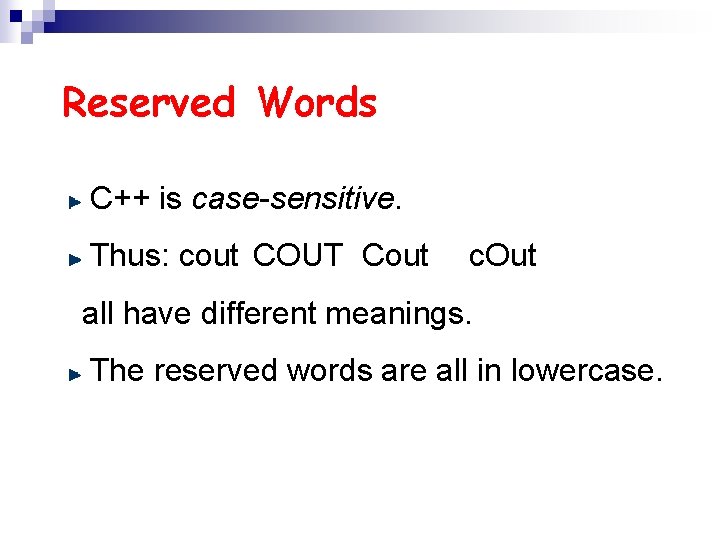
- Slides: 53
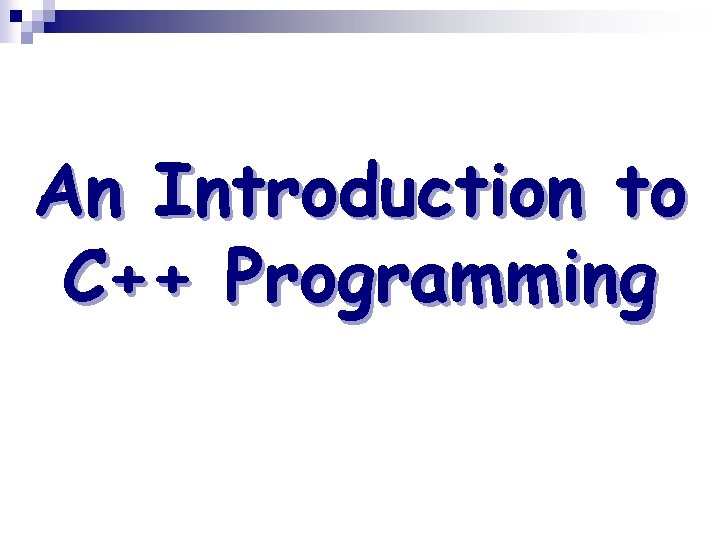
An Introduction to C++ Programming
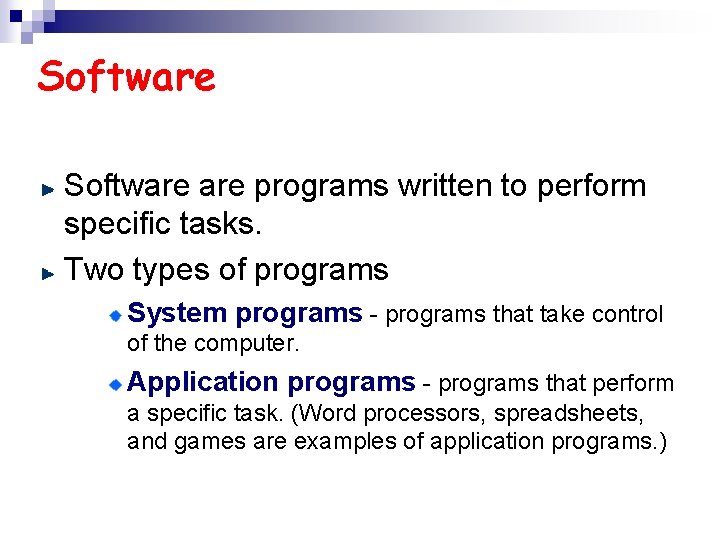
Software are programs written to perform specific tasks. Two types of programs System programs - programs that take control of the computer. Application programs - programs that perform a specific task. (Word processors, spreadsheets, and games are examples of application programs. )
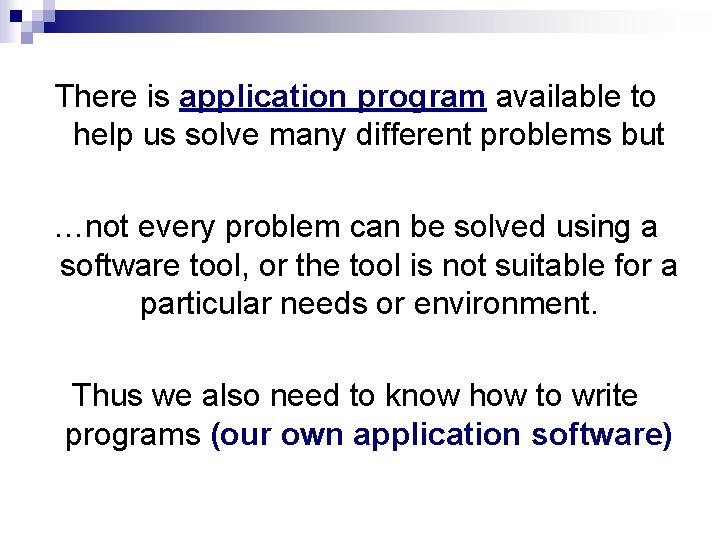
There is application program available to help us solve many different problems but …not every problem can be solved using a software tool, or the tool is not suitable for a particular needs or environment. Thus we also need to know how to write programs (our own application software)
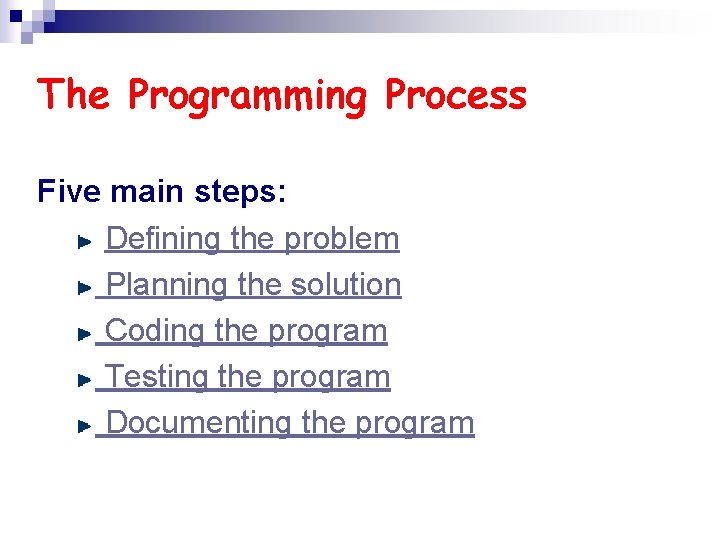
The Programming Process Five main steps: Defining the problem Planning the solution Coding the program Testing the program Documenting the program
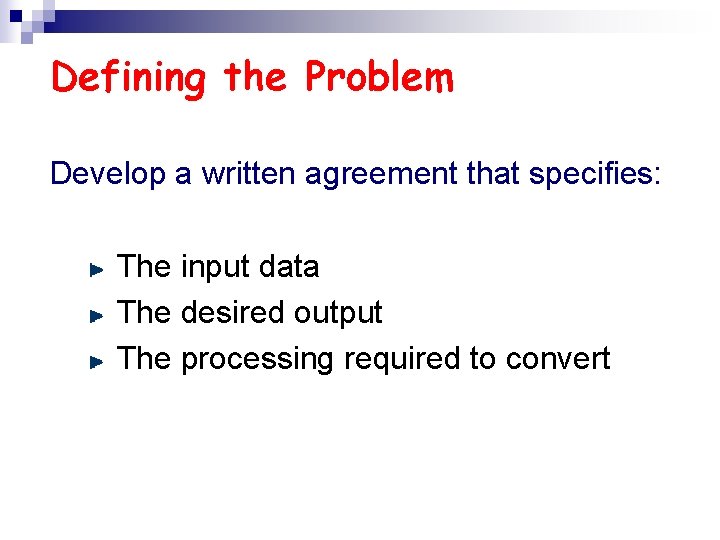
Defining the Problem Develop a written agreement that specifies: The input data The desired output The processing required to convert
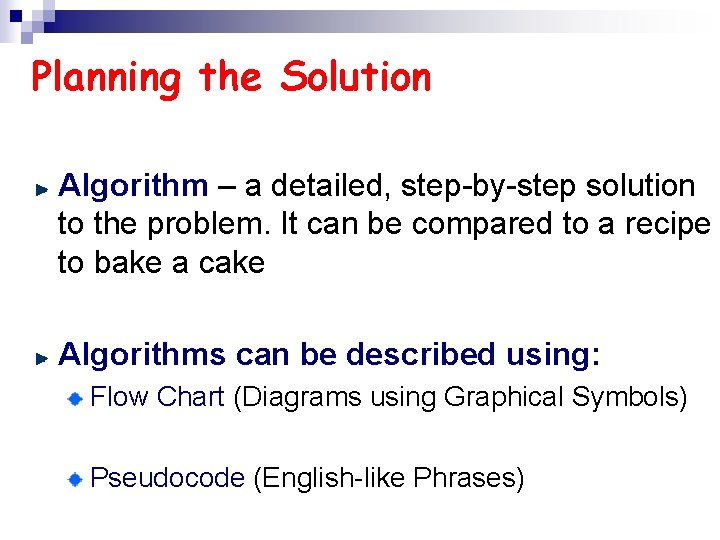
Planning the Solution Algorithm – a detailed, step-by-step solution to the problem. It can be compared to a recipe to bake a cake Algorithms can be described using: Flow Chart (Diagrams using Graphical Symbols) Pseudocode (English-like Phrases)
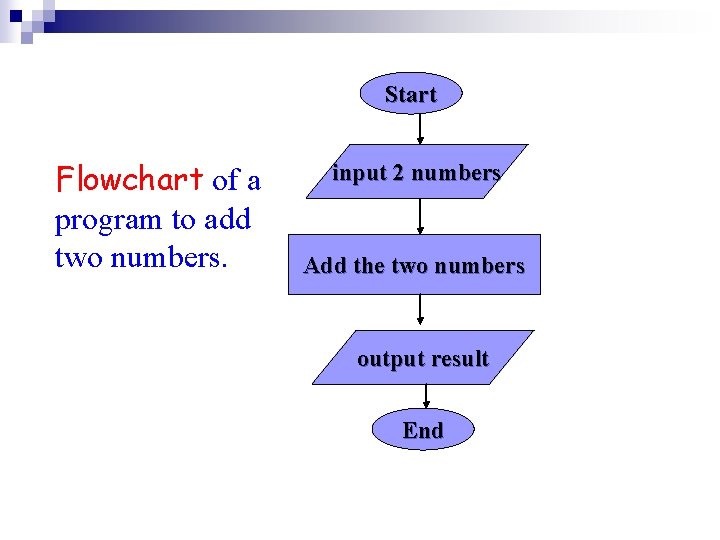
Start Flowchart of a program to add two numbers. input 2 numbers Add the two numbers output result End
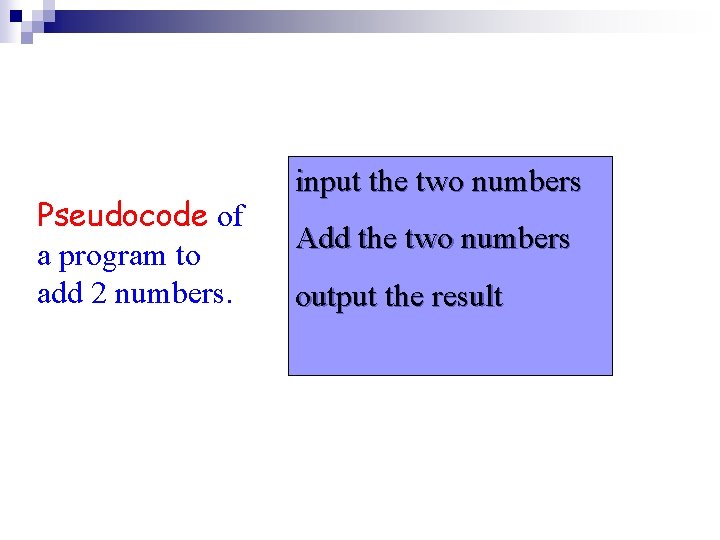
Pseudocode of a program to add 2 numbers. input the two numbers Add the two numbers output the result
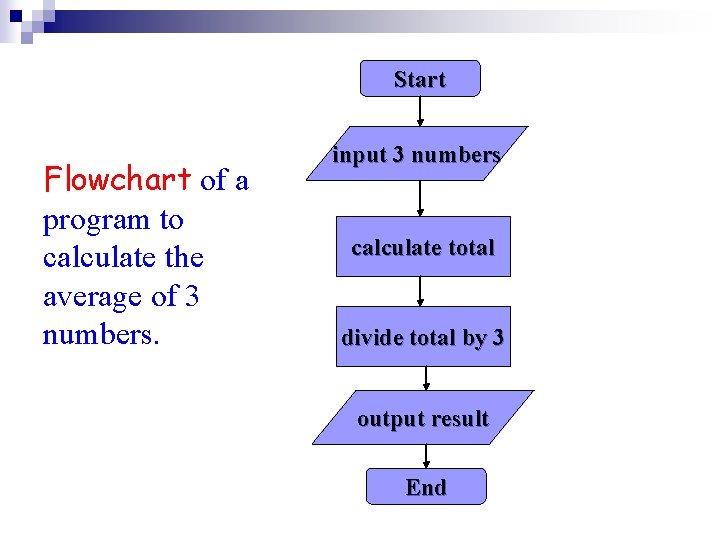
Start Flowchart of a program to calculate the average of 3 numbers. input 3 numbers calculate total divide total by 3 output result End
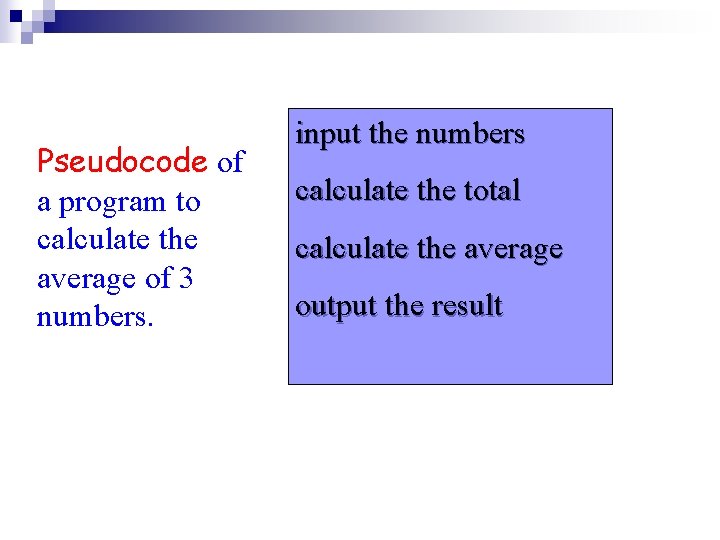
Pseudocode of a program to calculate the average of 3 numbers. input the numbers calculate the total calculate the average output the result
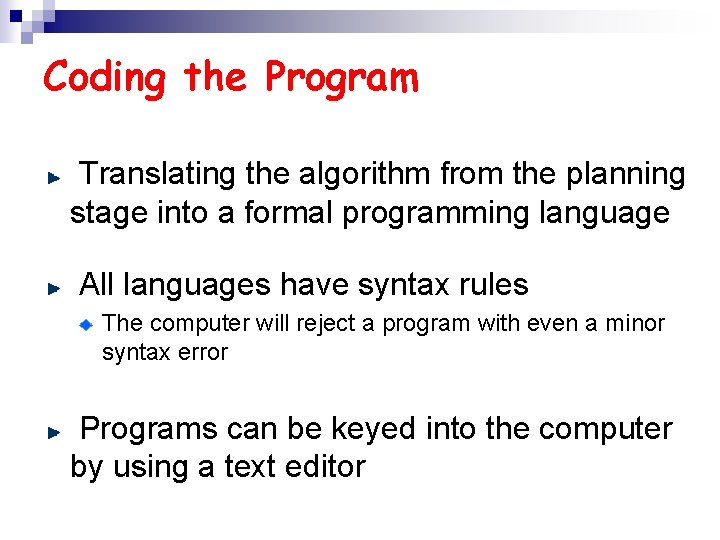
Coding the Program Translating the algorithm from the planning stage into a formal programming language All languages have syntax rules The computer will reject a program with even a minor syntax error Programs can be keyed into the computer by using a text editor
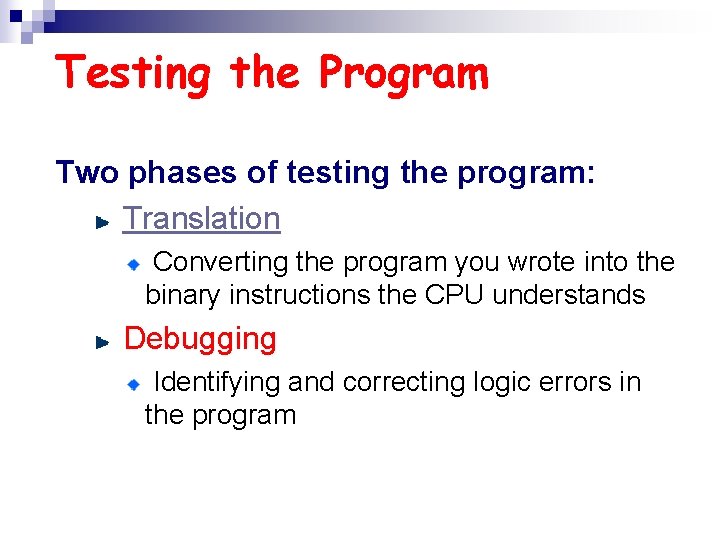
Testing the Program Two phases of testing the program: Translation Converting the program you wrote into the binary instructions the CPU understands Debugging Identifying and correcting logic errors in the program
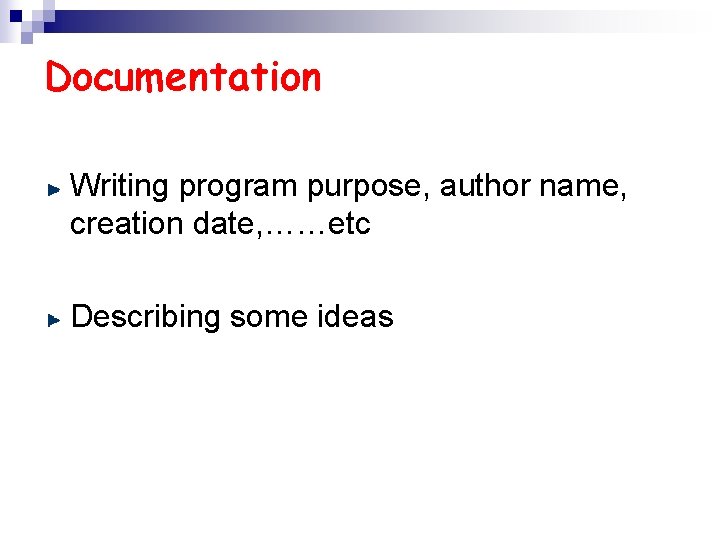
Documentation Writing program purpose, author name, creation date, ……etc Describing some ideas
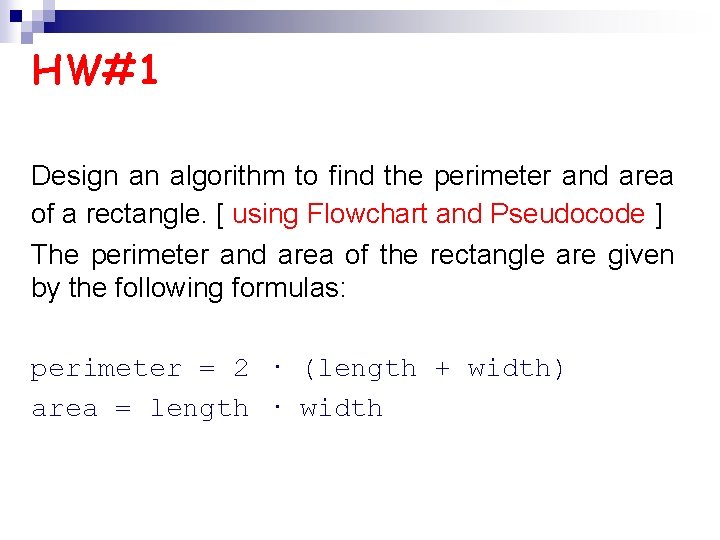
HW#1 Design an algorithm to find the perimeter and area of a rectangle. [ using Flowchart and Pseudocode ] The perimeter and area of the rectangle are given by the following formulas: perimeter = 2 · (length + width) area = length · width
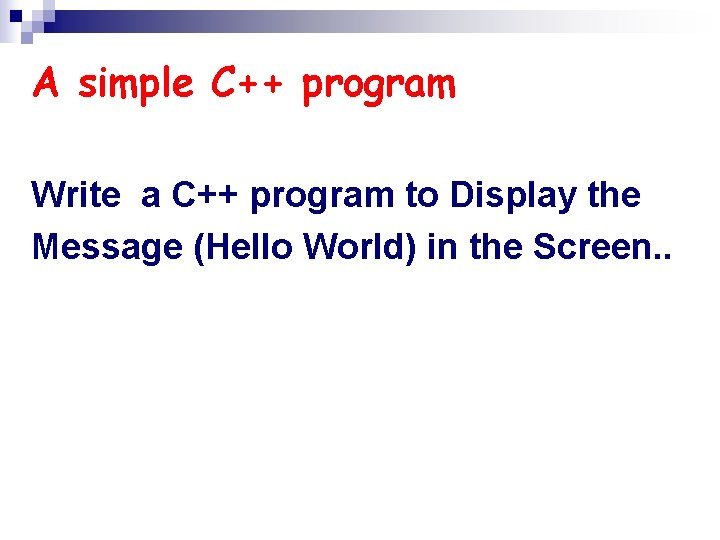
A simple C++ program Write a C++ program to Display the Message (Hello World) in the Screen. .
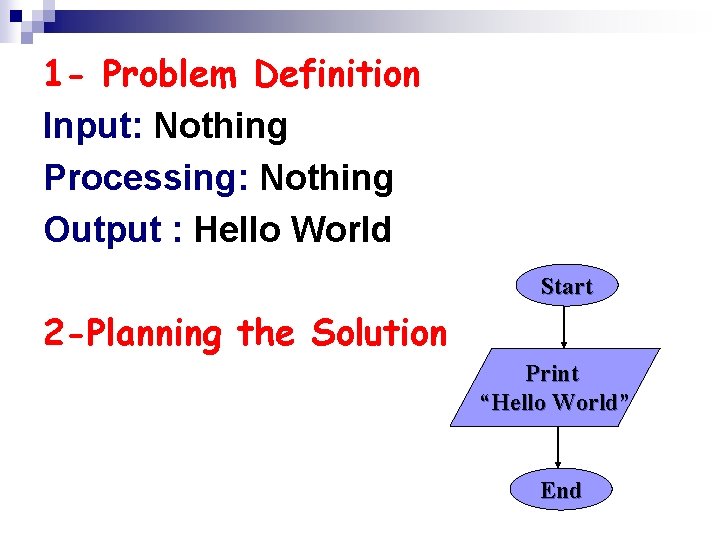
1 - Problem Definition Input: Nothing Processing: Nothing Output : Hello World Start 2 -Planning the Solution Print “Hello World” End
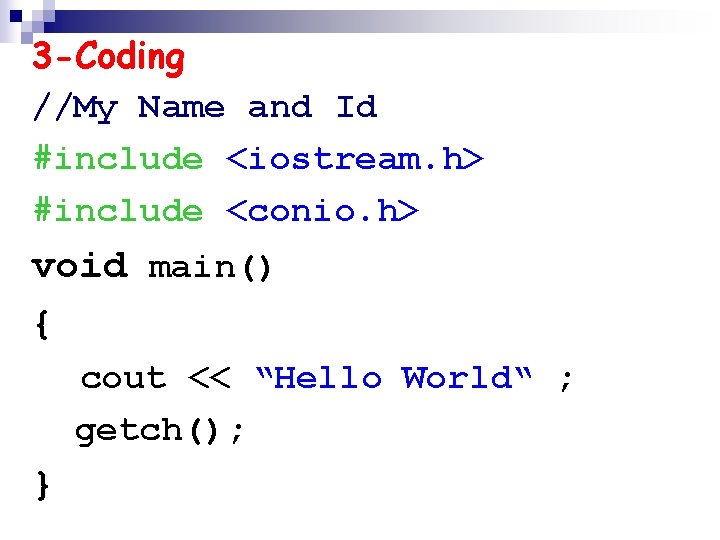
3 -Coding //My Name and Id #include <iostream. h> #include <conio. h> void main() { cout << “Hello World“ ; getch(); }
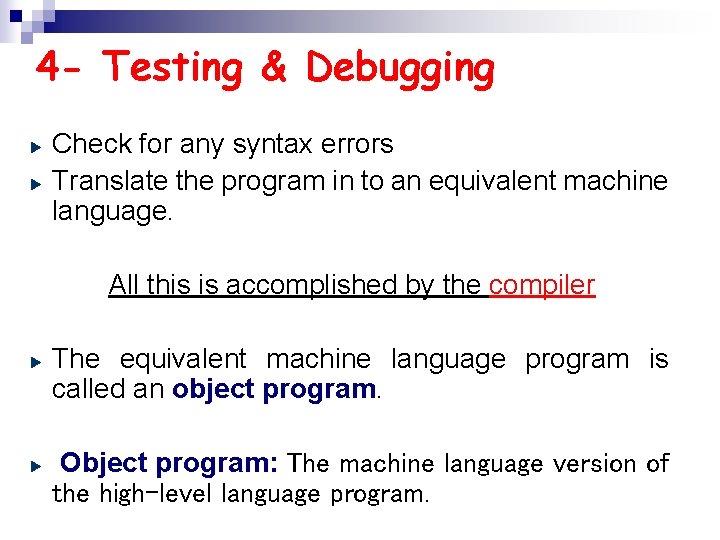
4 - Testing & Debugging Check for any syntax errors Translate the program in to an equivalent machine language. All this is accomplished by the compiler The equivalent machine language program is called an object program. Object program: The machine language version of the high-level language program.
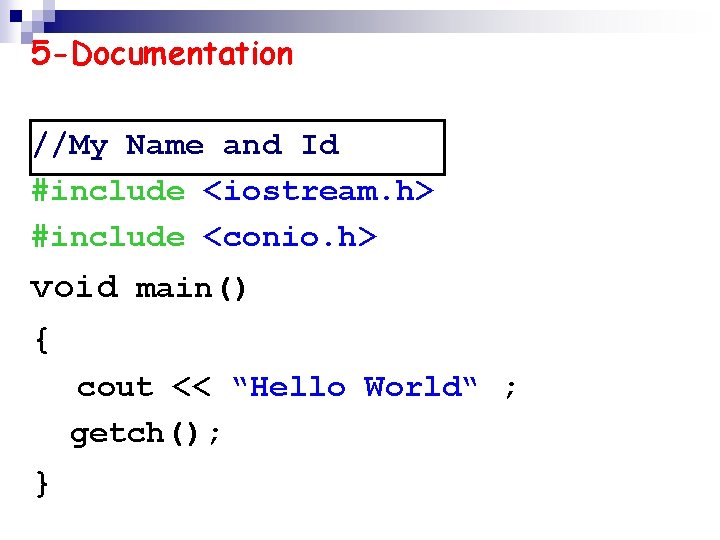
5 -Documentation //My Name and Id #include <iostream. h> #include <conio. h> void main() { cout << “Hello World“ ; getch(); }
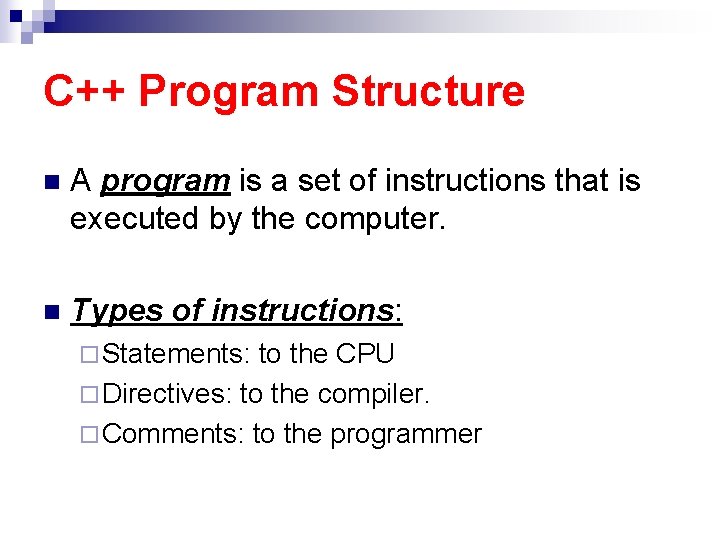
C++ Program Structure n A program is a set of instructions that is executed by the computer. n Types of instructions: ¨ Statements: to the CPU ¨ Directives: to the compiler. ¨ Comments: to the programmer
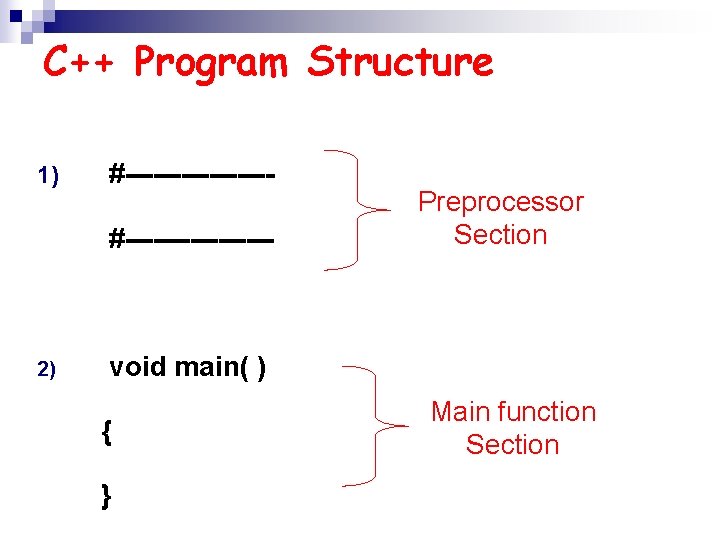
C++ Program Structure 1) #---------------- 2) Preprocessor Section void main( ) { } Main function Section
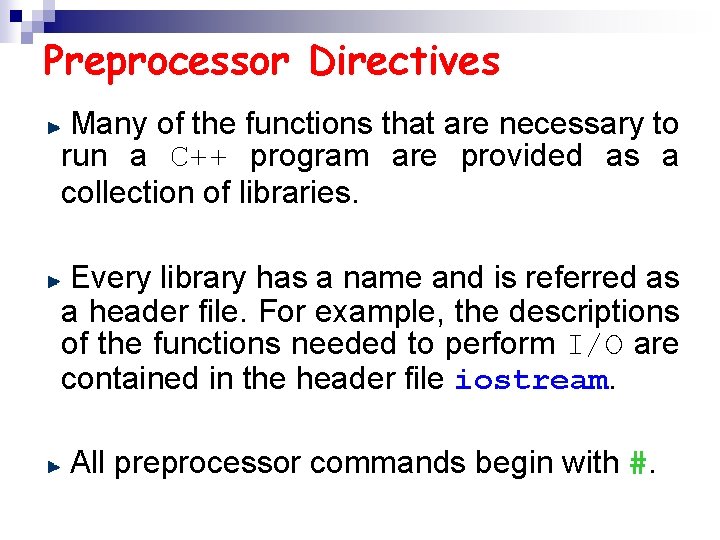
Preprocessor Directives Many of the functions that are necessary to run a C++ program are provided as a collection of libraries. Every library has a name and is referred as a header file. For example, the descriptions of the functions needed to perform I/O are contained in the header file iostream. All preprocessor commands begin with #.
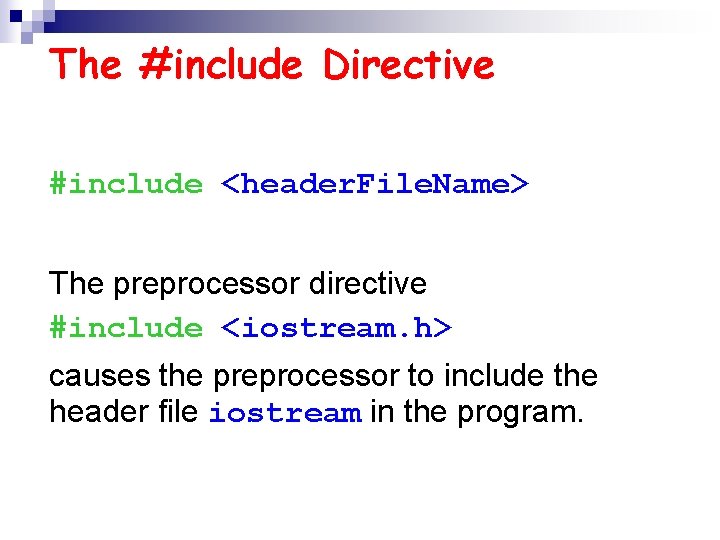
The #include Directive #include <header. File. Name> The preprocessor directive #include <iostream. h> causes the preprocessor to include the header file iostream in the program.
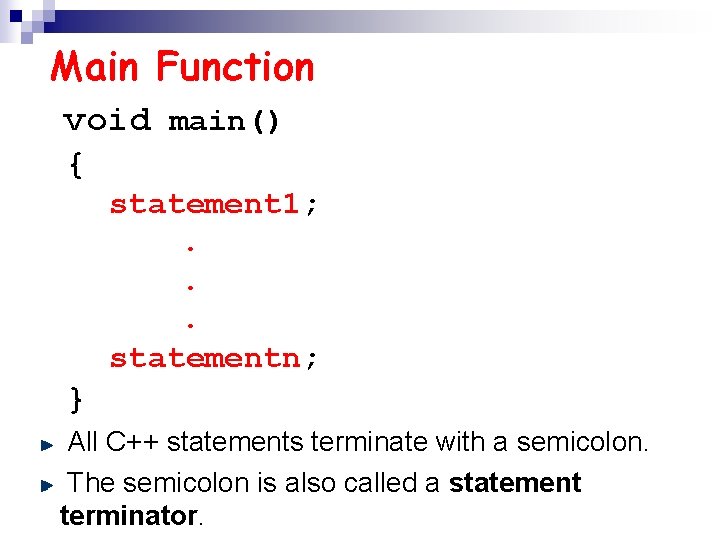
Main Function void main() { statement 1; . . . statementn; } All C++ statements terminate with a semicolon. The semicolon is also called a statement terminator.
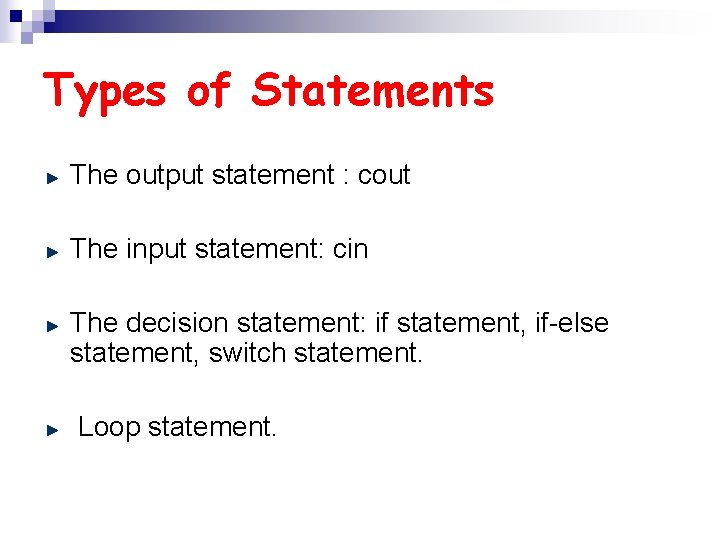
Types of Statements The output statement : cout The input statement: cin The decision statement: if statement, if-else statement, switch statement. Loop statement.
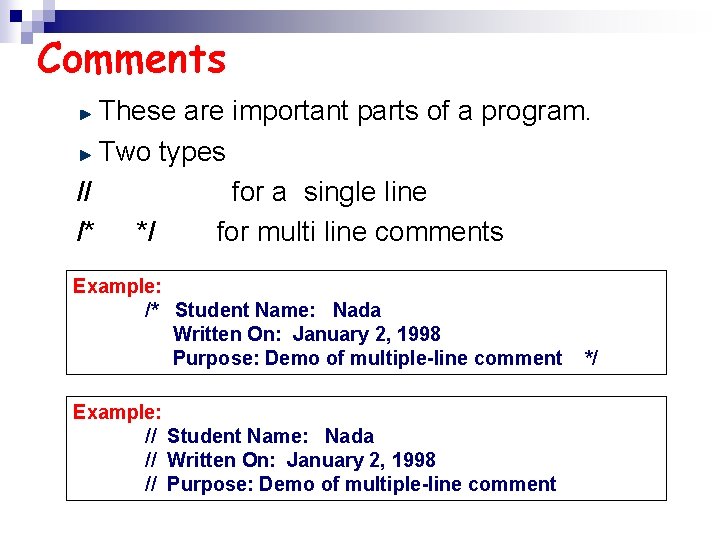
Comments These are important parts of a program. Two types // for a single line /* */ for multi line comments Example: /* Student Name: Nada Written On: January 2, 1998 Purpose: Demo of multiple-line comment Example: // Student Name: Nada // Written On: January 2, 1998 // Purpose: Demo of multiple-line comment */
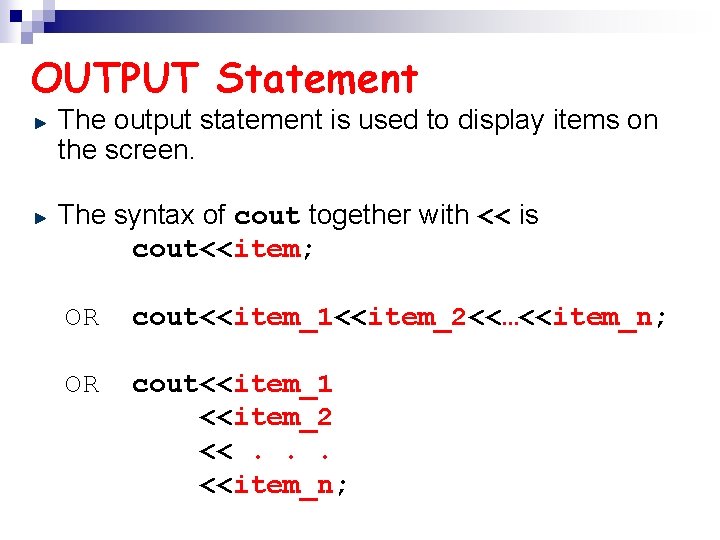
OUTPUT Statement The output statement is used to display items on the screen. The syntax of cout together with << is cout<<item; OR cout<<item_1<<item_2<<…<<item_n; OR cout<<item_1 <<item_2 <<. . . <<item_n;
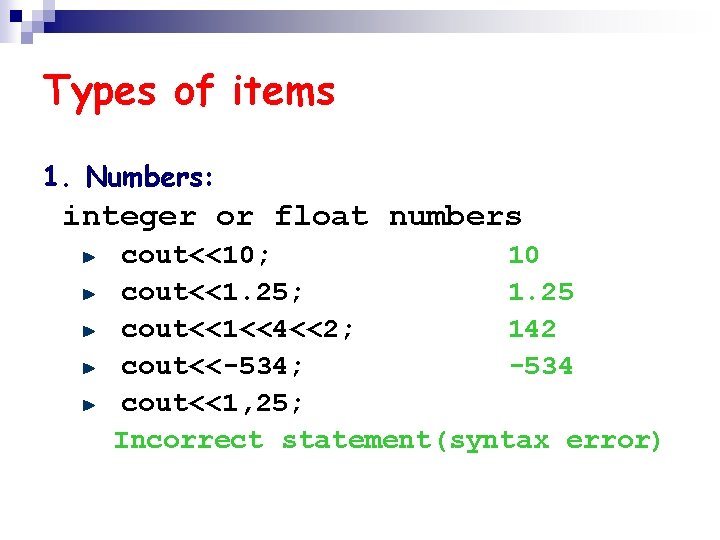
Types of items 1. Numbers: integer or float numbers cout<<10; 10 cout<<1. 25; 1. 25 cout<<1<<4<<2; 142 cout<<-534; -534 cout<<1, 25; Incorrect statement(syntax error)
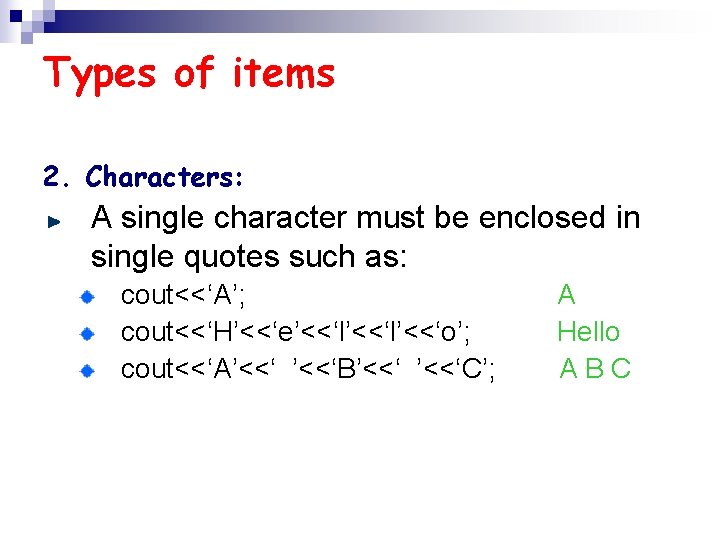
Types of items 2. Characters: A single character must be enclosed in single quotes such as: cout<<‘A’; cout<<‘H’<<‘e’<<‘l’<<‘o’; cout<<‘A’<<‘B’<<‘C’; A Hello ABC
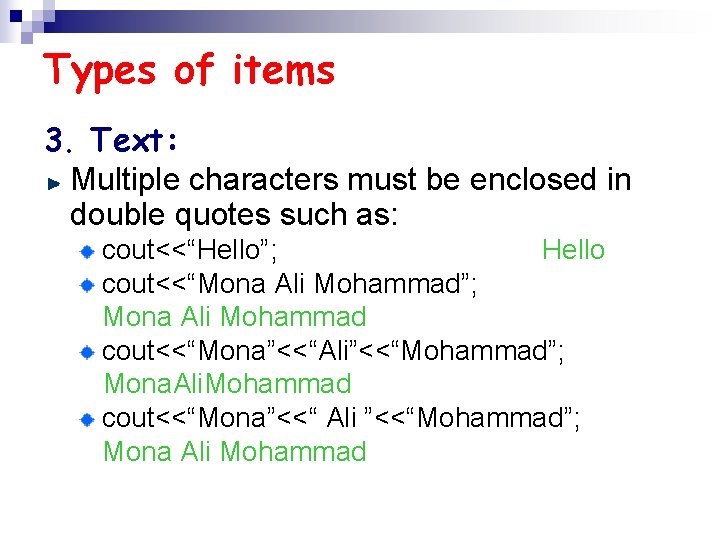
Types of items 3. Text: Multiple characters must be enclosed in double quotes such as: cout<<“Hello”; Hello cout<<“Mona Ali Mohammad”; Mona Ali Mohammad cout<<“Mona”<<“Ali”<<“Mohammad”; Mona. Ali. Mohammad cout<<“Mona”<<“ Ali ”<<“Mohammad”; Mona Ali Mohammad
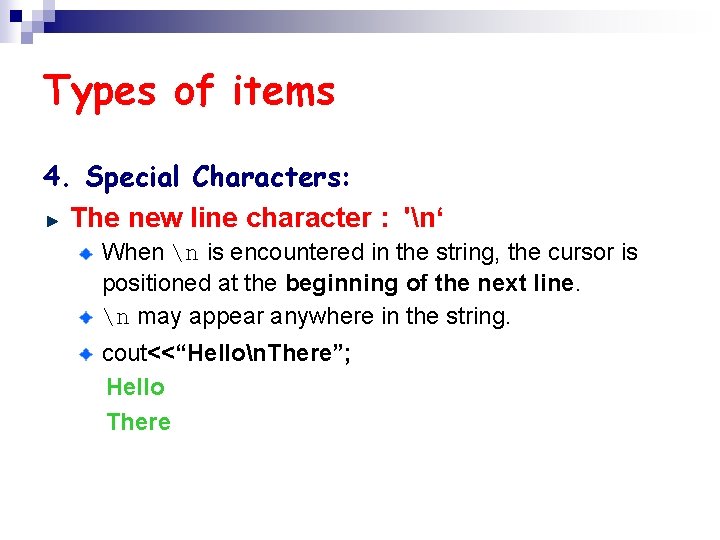
Types of items 4. Special Characters: The new line character : 'n‘ When n is encountered in the string, the cursor is positioned at the beginning of the next line. n may appear anywhere in the string. cout<<“Hellon. There”; Hello There
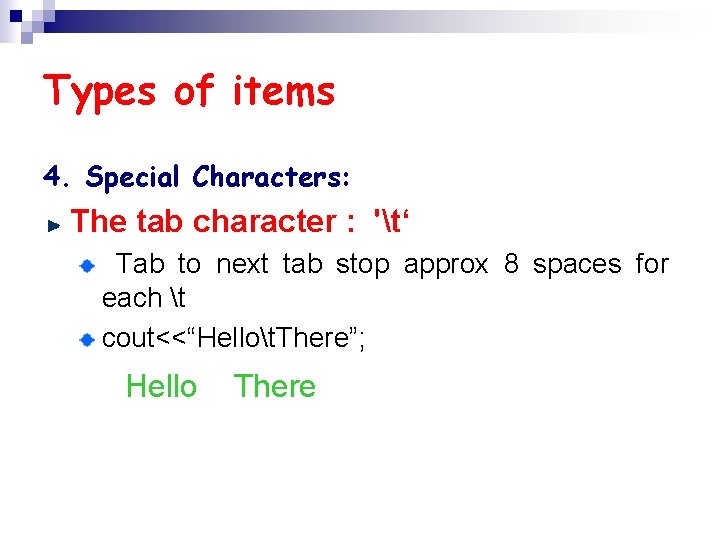
Types of items 4. Special Characters: The tab character : 't‘ Tab to next tab stop approx 8 spaces for each t cout<<“Hellot. There”; Hello There
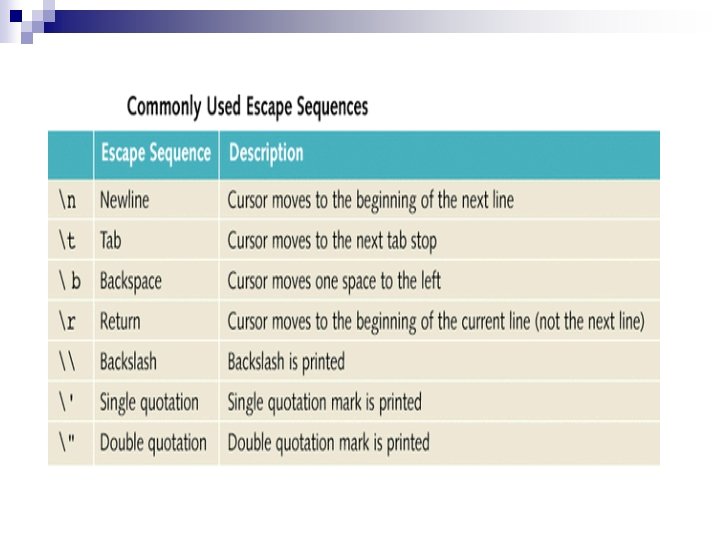
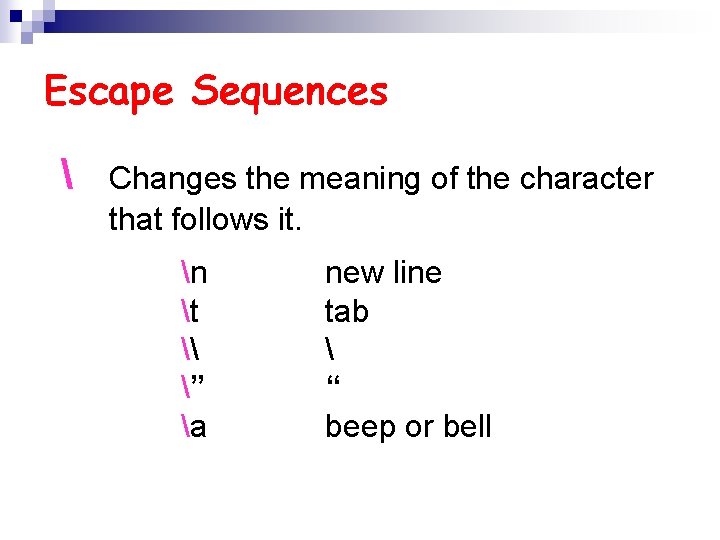
Escape Sequences Changes the meaning of the character that follows it. n t \ ” a new line tab “ beep or bell
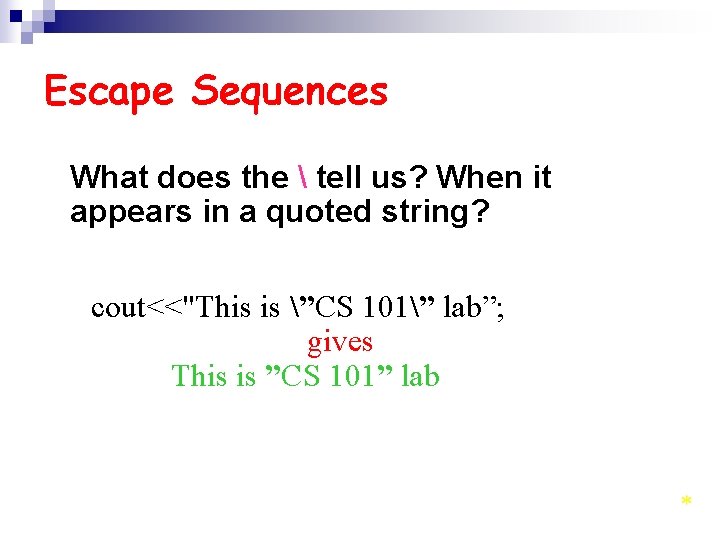
Escape Sequences What does the tell us? When it appears in a quoted string? cout<<"This is ”CS 101” lab”; gives This is ”CS 101” lab *
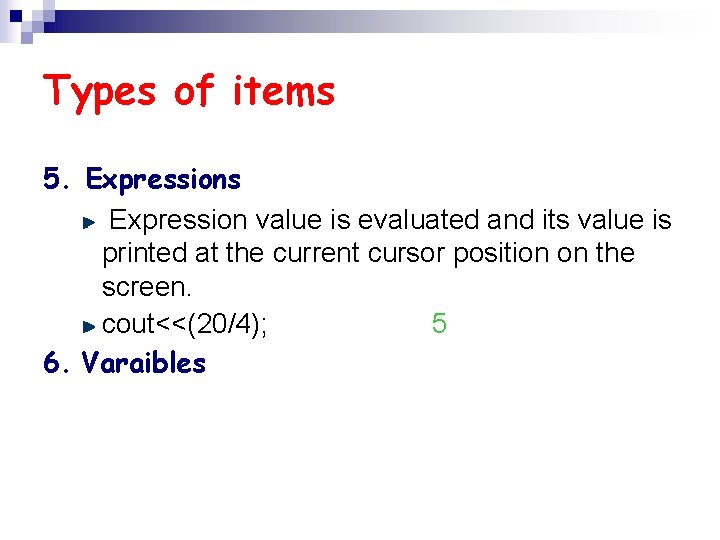
Types of items 5. Expressions Expression value is evaluated and its value is printed at the current cursor position on the screen. cout<<(20/4); 5 6. Varaibles
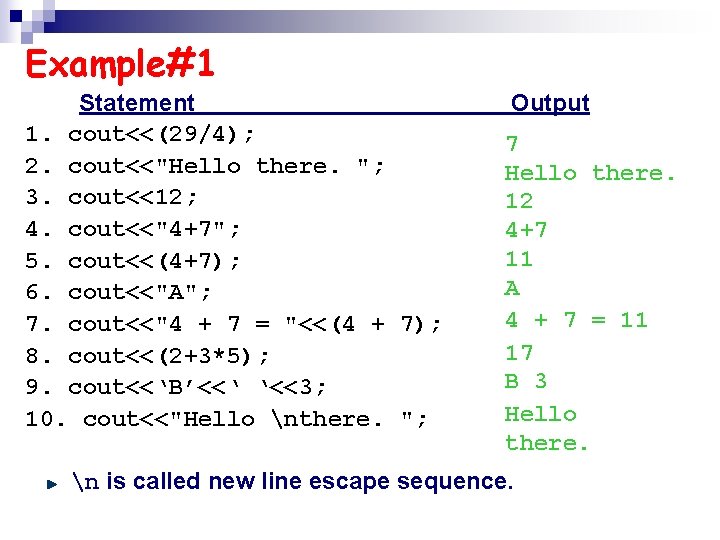
Example#1 Statement 1. cout<<(29/4); 2. cout<<"Hello there. "; 3. cout<<12; 4. cout<<"4+7"; 5. cout<<(4+7); 6. cout<<"A"; 7. cout<<"4 + 7 = "<<(4 + 7); 8. cout<<(2+3*5); 9. cout<<‘B’<<‘ ‘<<3; 10. cout<<"Hello nthere. "; Output 77 Hello there. Hello 12 12 4+7 11 11 AA 4 + 7 4= +117 = 1 17 17 BB 33 Hello there n is called new line escape sequence.
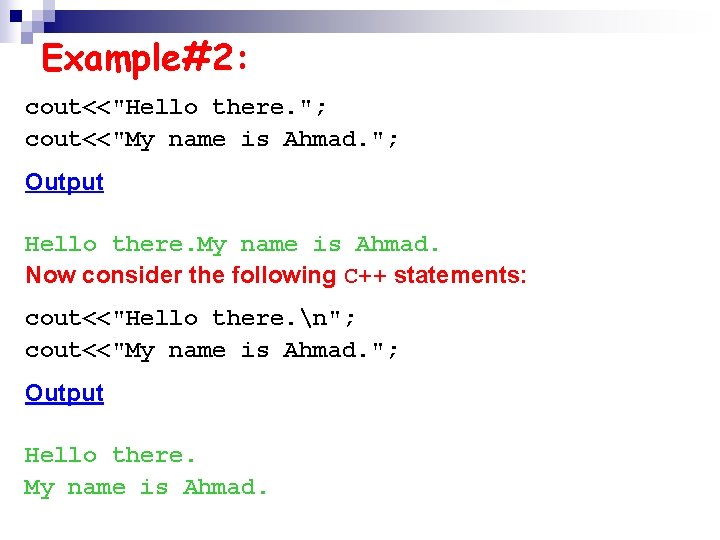
Example#2: cout<<"Hello there. "; cout<<"My name is Ahmad. "; Output Hello there. My name is Ahmad. Now consider the following C++ statements: cout<<"Hello there. n"; cout<<"My name is Ahmad. "; Output Hello there. My name is Ahmad.
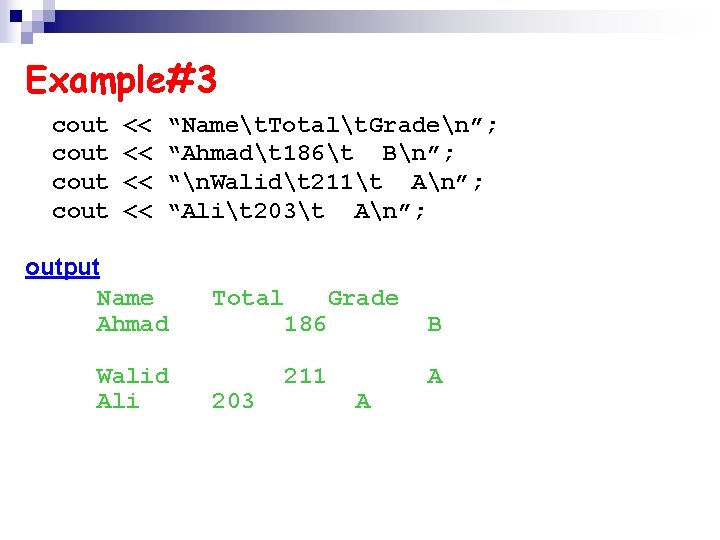
Example#3 cout << << “Namet. Totalt. Graden”; “Ahmadt 186t Bn”; “n. Walidt 211t An”; “Alit 203t An”; output Name Ahmad Walid Ali Total Grade 186 B 211 A 203 A
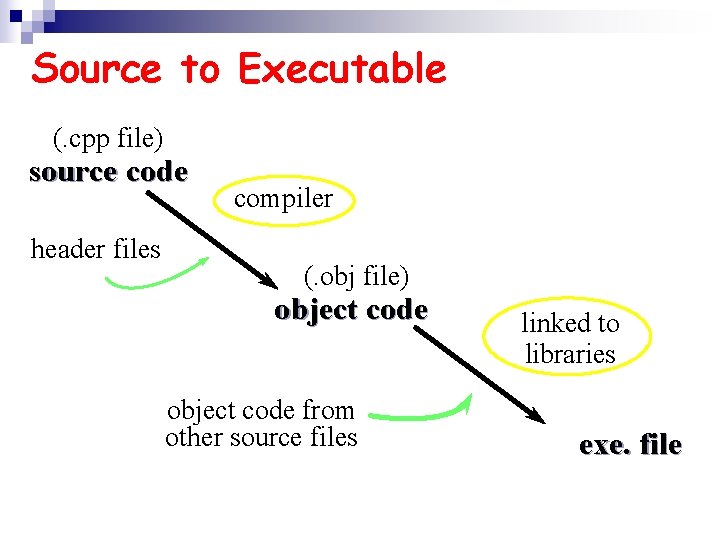
Source to Executable (. cpp file) source code header files compiler (. obj file) object code from other source files linked to libraries exe. file
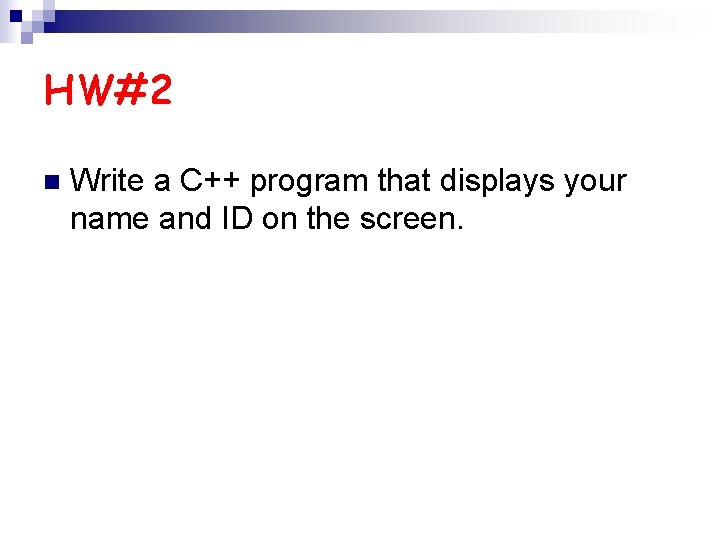
HW#2 n Write a C++ program that displays your name and ID on the screen.
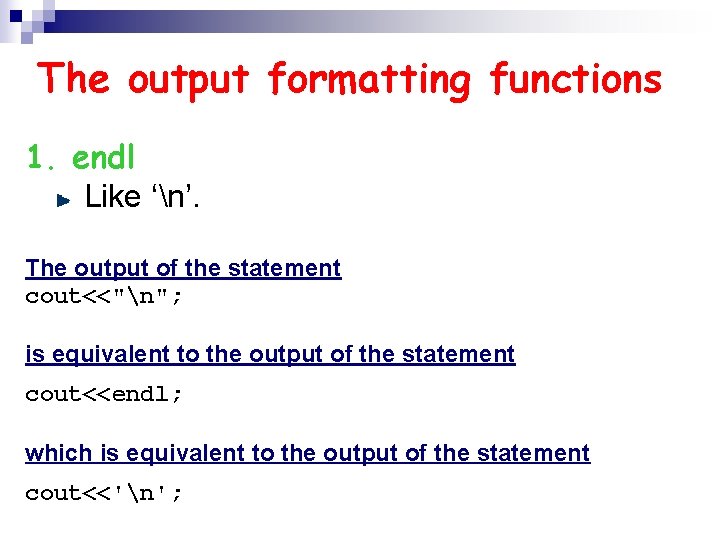
The output formatting functions 1. endl Like ‘n’. The output of the statement cout<<"n"; is equivalent to the output of the statement cout<<endl; which is equivalent to the output of the statement cout<<'n';
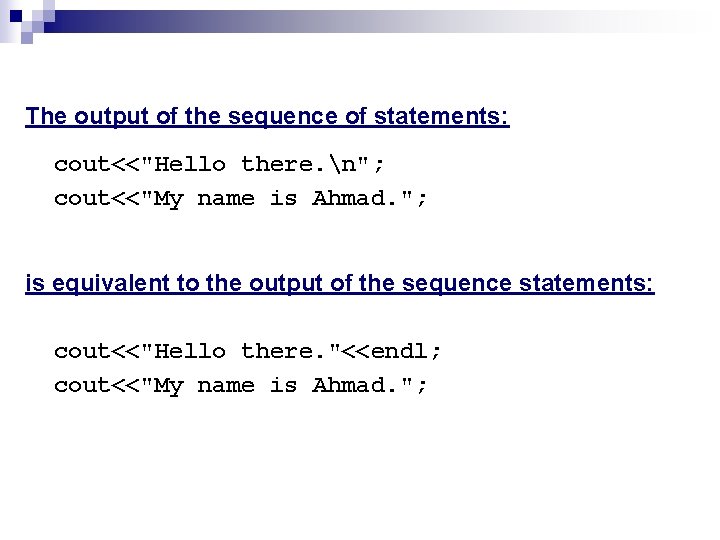
The output of the sequence of statements: cout<<"Hello there. n"; cout<<"My name is Ahmad. "; is equivalent to the output of the sequence statements: cout<<"Hello there. "<<endl; cout<<"My name is Ahmad. ";
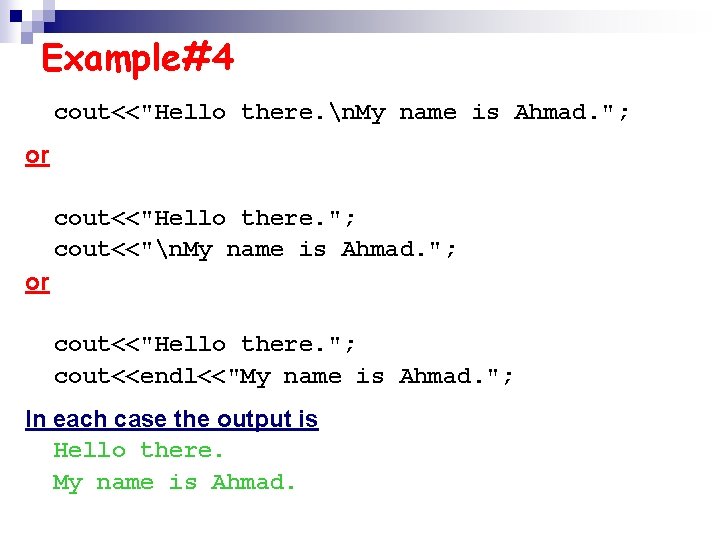
Example#4 cout<<"Hello there. n. My name is Ahmad. "; or cout<<"Hello there. "; cout<<"n. My name is Ahmad. "; or cout<<"Hello there. "; cout<<endl<<"My name is Ahmad. "; In each case the output is Hello there. My name is Ahmad.
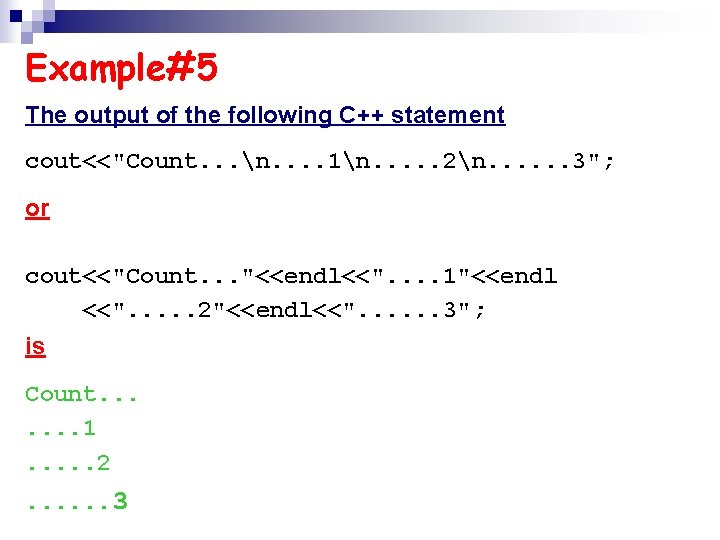
Example#5 The output of the following C++ statement cout<<"Count. . . n. . 1n. . . 2n. . . 3"; or cout<<"Count. . . "<<endl<<". . 1"<<endl <<". . . 2"<<endl<<". . . 3"; is Count. . . . 1. . . 2. . . 3
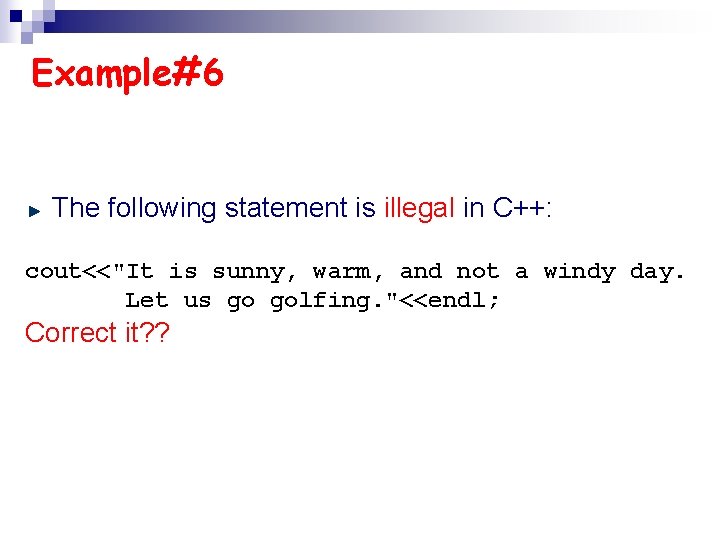
Example#6 The following statement is illegal in C++: cout<<"It is sunny, warm, and not a windy day. Let us go golfing. "<<endl; Correct it? ?
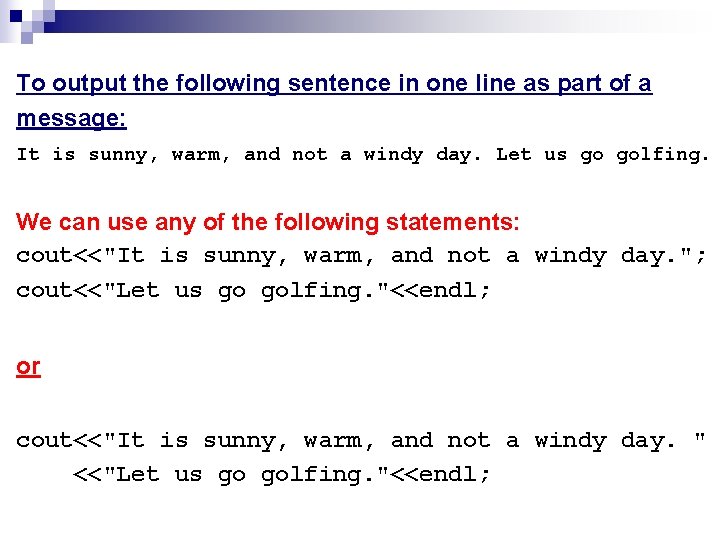
To output the following sentence in one line as part of a message: It is sunny, warm, and not a windy day. Let us go golfing. We can use any of the following statements: cout<<"It is sunny, warm, and not a windy day. "; cout<<"Let us go golfing. "<<endl; or cout<<"It is sunny, warm, and not a windy day. " <<"Let us go golfing. "<<endl;
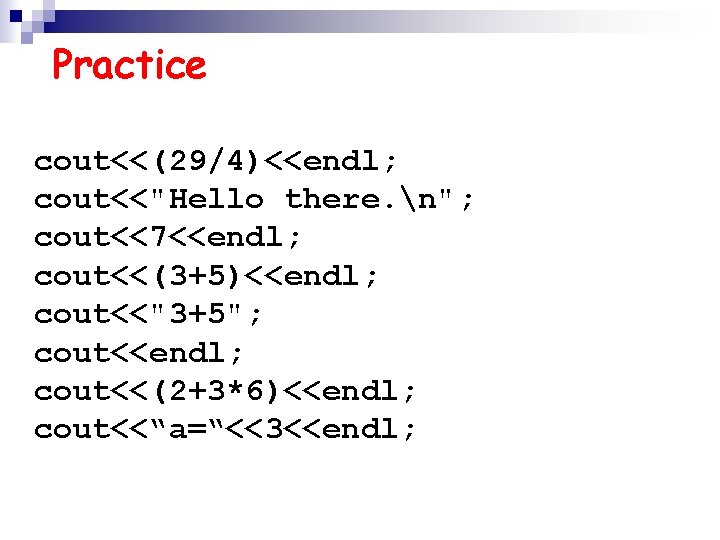
Practice cout<<(29/4)<<endl; cout<<"Hello there. n"; cout<<7<<endl; cout<<(3+5)<<endl; cout<<"3+5"; cout<<endl; cout<<(2+3*6)<<endl; cout<<“a=“<<3<<endl;
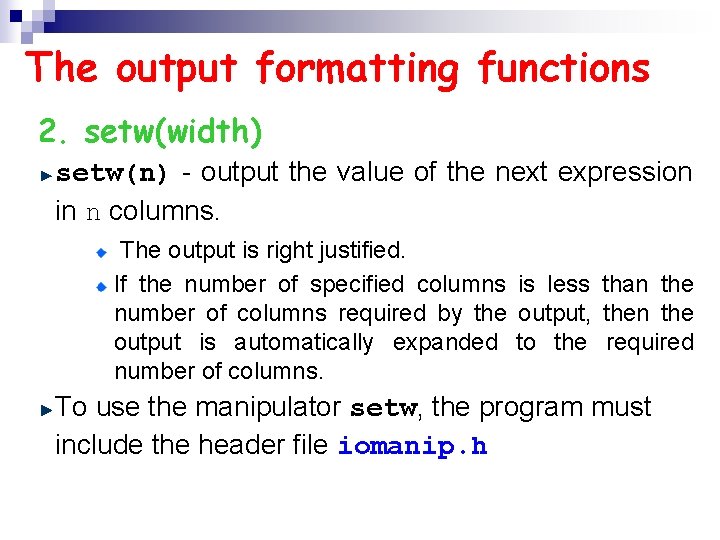
The output formatting functions 2. setw(width) setw(n) - output the value of the next expression in n columns. The output is right justified. If the number of specified columns is less than the number of columns required by the output, then the output is automatically expanded to the required number of columns. To use the manipulator setw, the program must include the header file iomanip. h
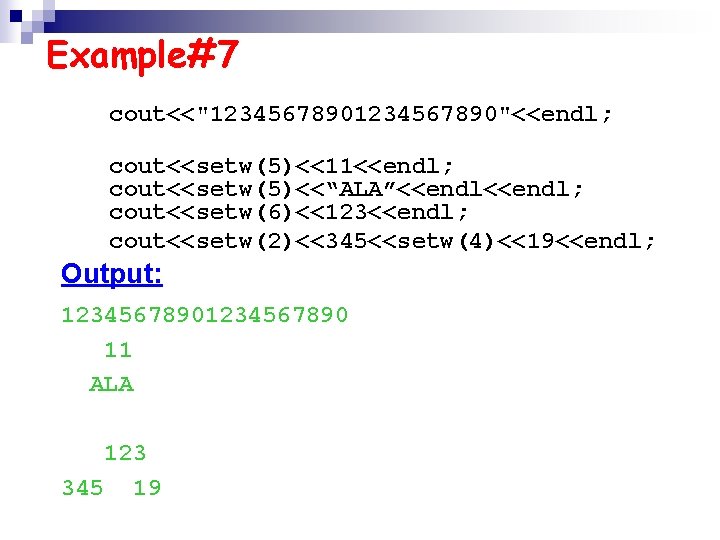
Example#7 cout<<"1234567890"<<endl; cout<<setw(5)<<11<<endl; cout<<setw(5)<<“ALA”<<endl; cout<<setw(6)<<123<<endl; cout<<setw(2)<<345<<setw(4)<<19<<endl; Output: 1234567890 11 ALA 123 345 19
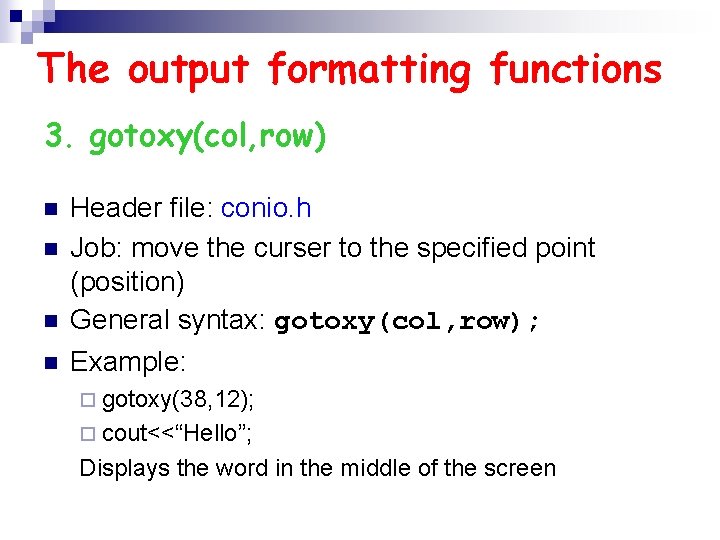
The output formatting functions 3. gotoxy(col, row) n Header file: conio. h Job: move the curser to the specified point (position) General syntax: gotoxy(col, row); n Example: n n ¨ gotoxy(38, 12); ¨ cout<<“Hello”; Displays the word in the middle of the screen
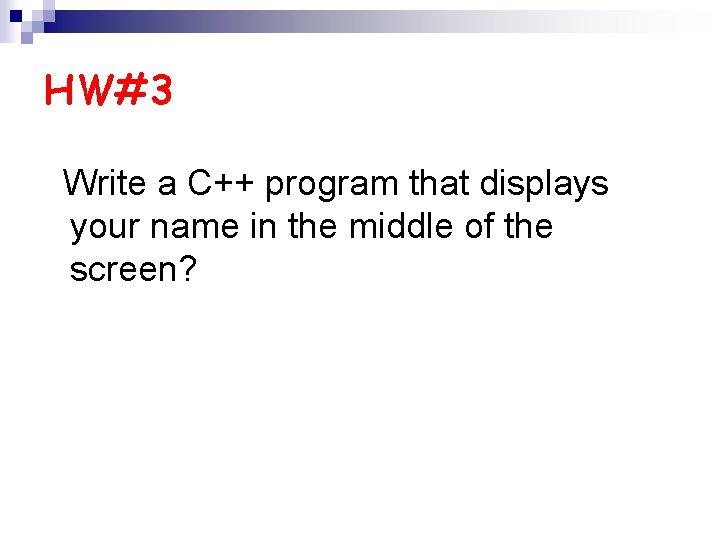
HW#3 Write a C++ program that displays your name in the middle of the screen?
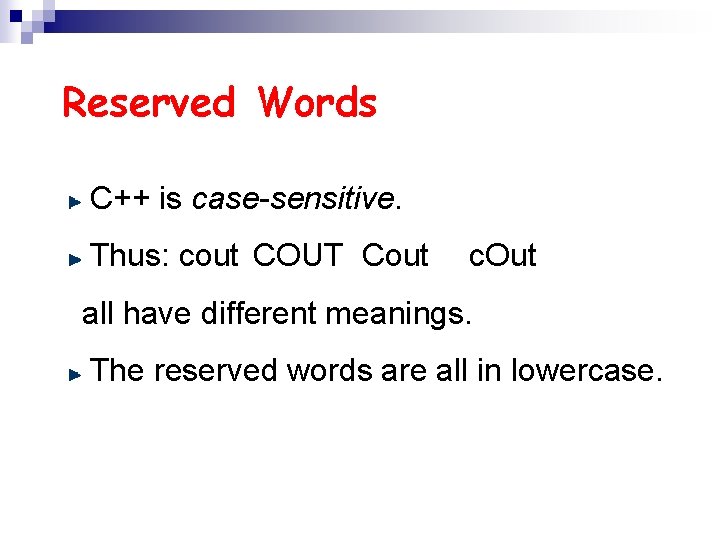
Reserved Words C++ is case-sensitive. Thus: cout COUT Cout c. Out all have different meanings. The reserved words are all in lowercase.
Insidan region jh
Cpmcd in software engineering
Sic programming examples
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
System programming definition
Linear vs integer programming
Programing adalah
Catering sales and marketing
Unlike application software programs
Csc
Categories of software
Vending software programs
Web page authoring software examples
Extreme programming in software engineering
Haas macros
Parts of msw logo screen
Be next frontier software development
Introduction to server side programming
Java introduction to problem solving and programming
Introduction to programming languages
Elementary programming in java
An introduction to parallel programming peter pacheco
Introduction to visual basic
Plc stands for
Java an introduction to problem solving and programming
Console programming
Programming language
Cpe102/csc102 introduction to programming
A web based introduction to programming
Computer programming chapter 1
C programming lectures
Introduction to visual basic
Introduction to programming concepts with scratch
Python programming an introduction to computer science
Java introduction to problem solving and programming
Computer programming chapter 1
Introduction to java programming 10th edition quizzes
Introduction to sql programming techniques
Introduction to sql programming techniques
Chapter 1 introduction to computers and programming
Chapter 1 introduction to computers and programming
Software maintenance process models ppt
What is software implementation in software engineering
Modern software economics
Software engineering
Metrics computer science
Skills and applications chapter 3
Generic and customized software
Difference between student software and industrial software
Software engineering crisis
Software metrics example
Is an os system software or application software
Eic software reviews