Allowing File Uploads The File Upload Control 2
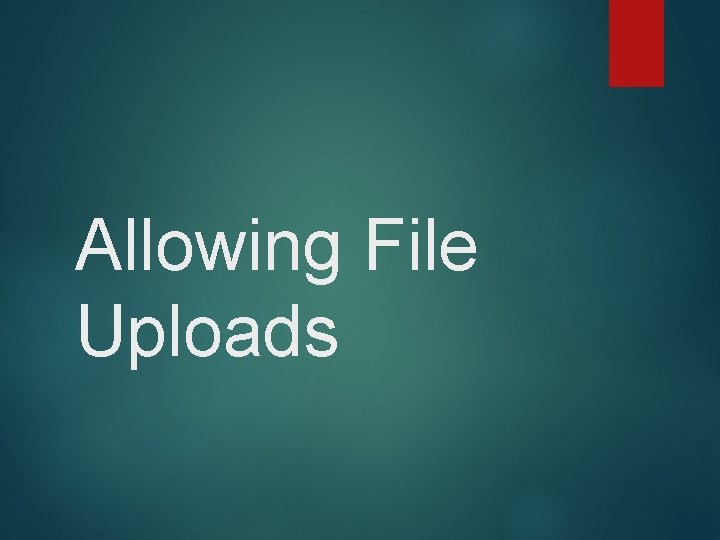
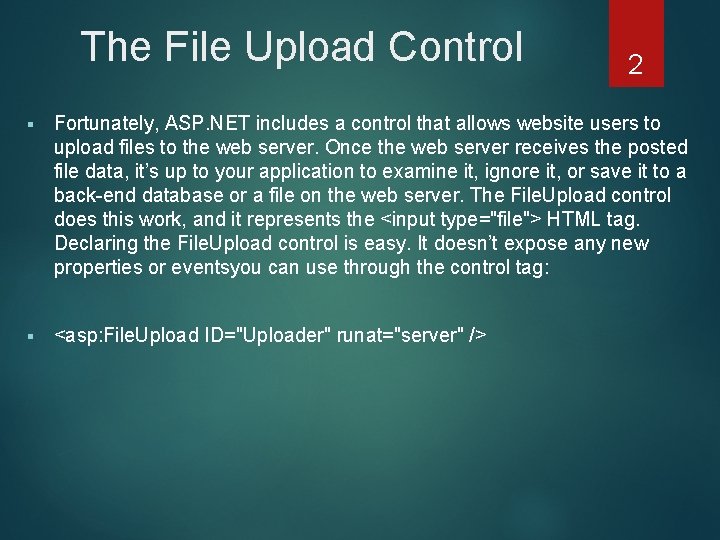
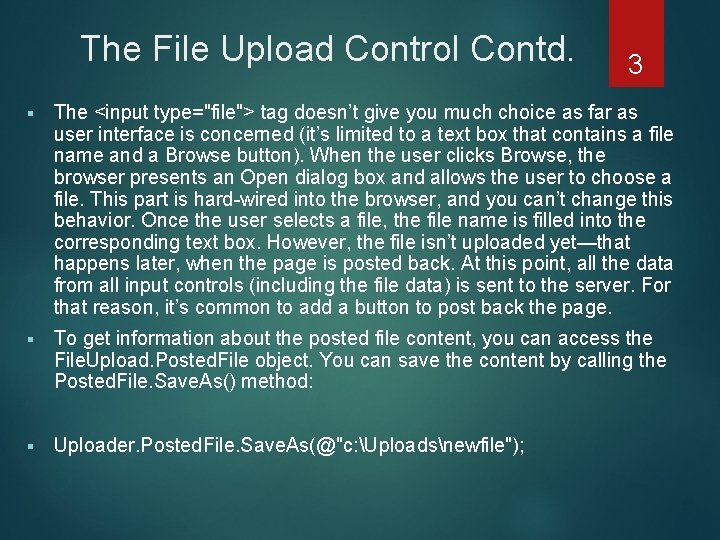
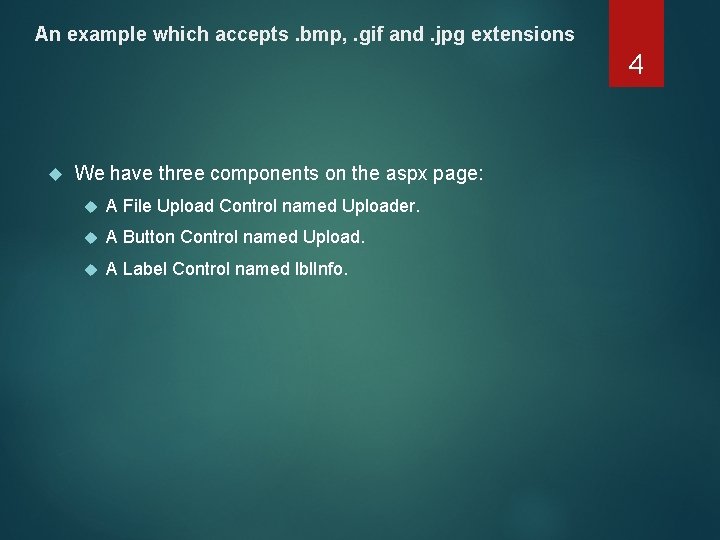
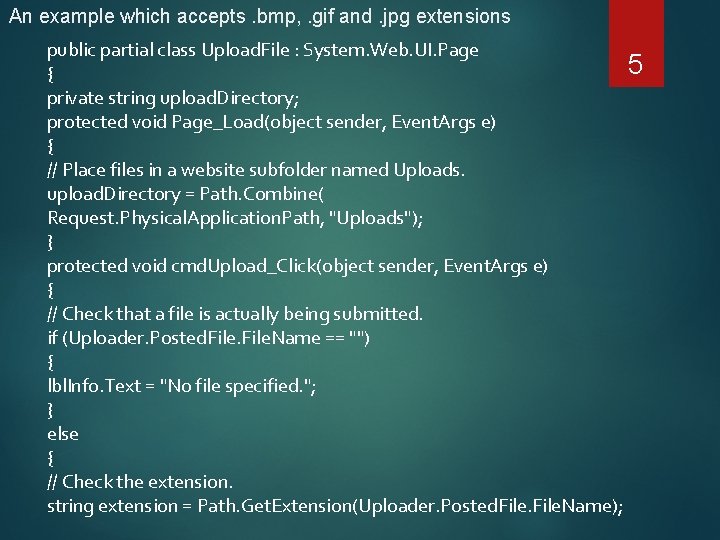
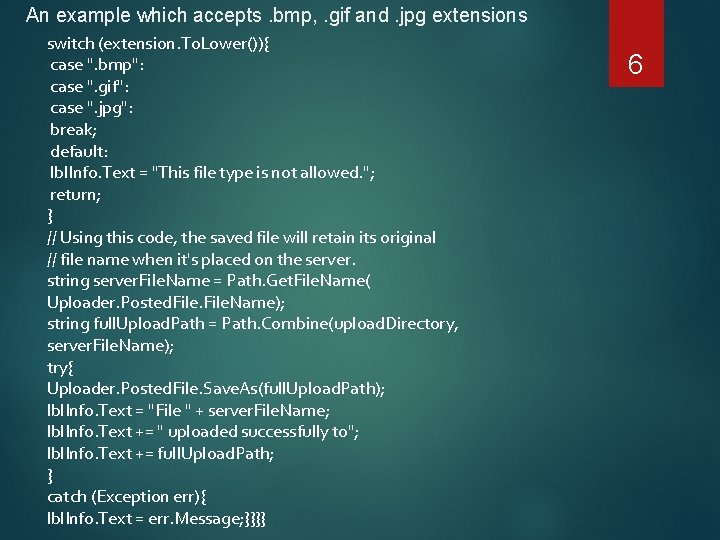
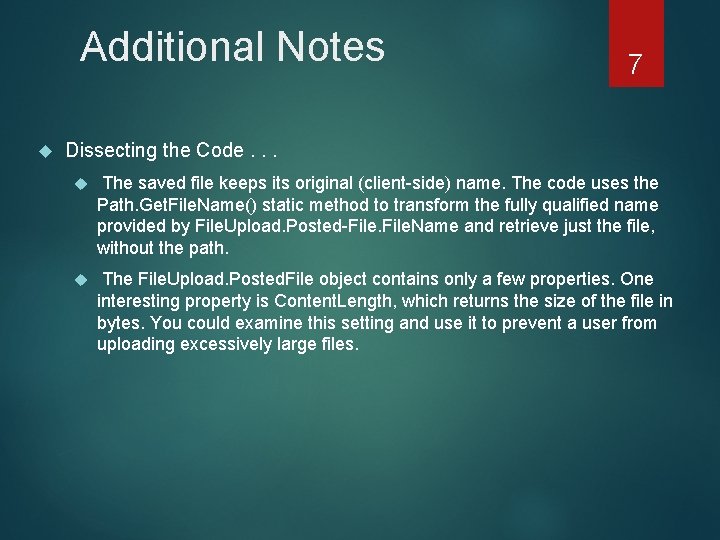
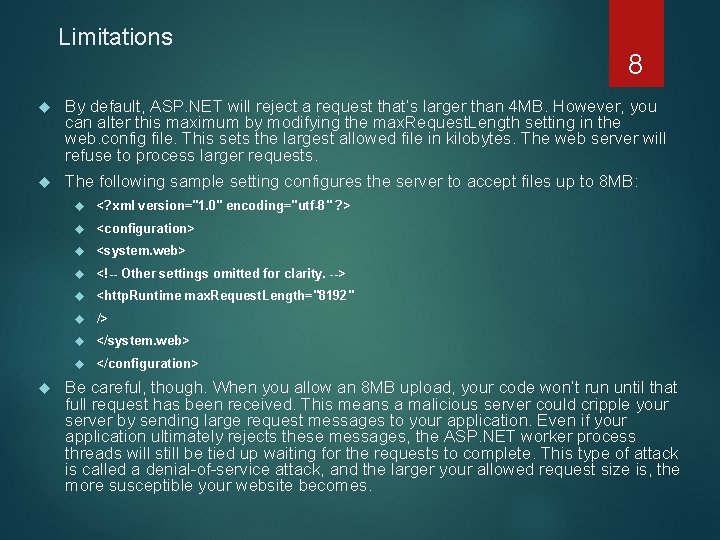
- Slides: 8
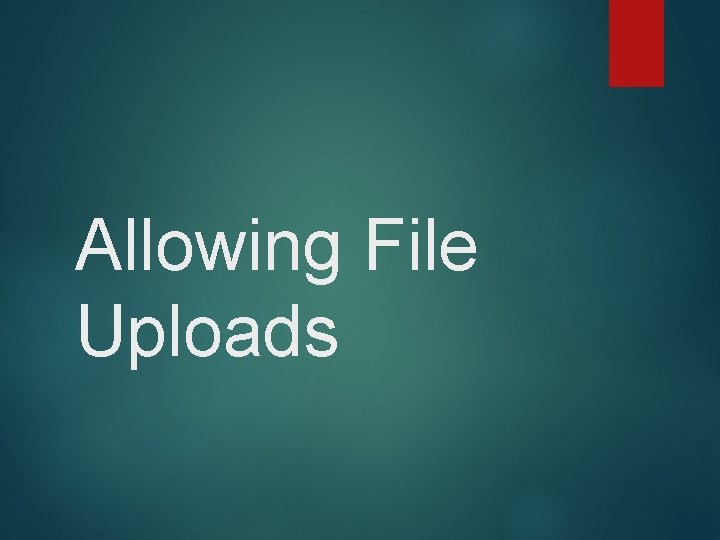
Allowing File Uploads
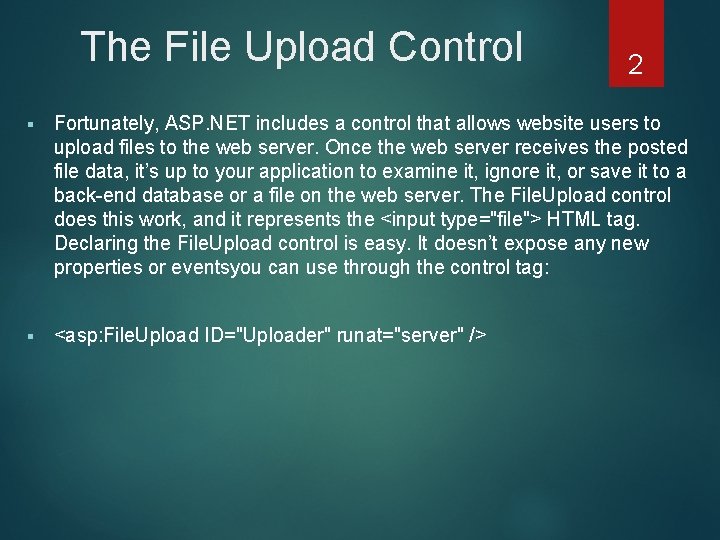
The File Upload Control 2 Fortunately, ASP. NET includes a control that allows website users to upload files to the web server. Once the web server receives the posted file data, it’s up to your application to examine it, ignore it, or save it to a back-end database or a file on the web server. The File. Upload control does this work, and it represents the <input type="file"> HTML tag. Declaring the File. Upload control is easy. It doesn’t expose any new properties or eventsyou can use through the control tag: <asp: File. Upload ID="Uploader" runat="server" />
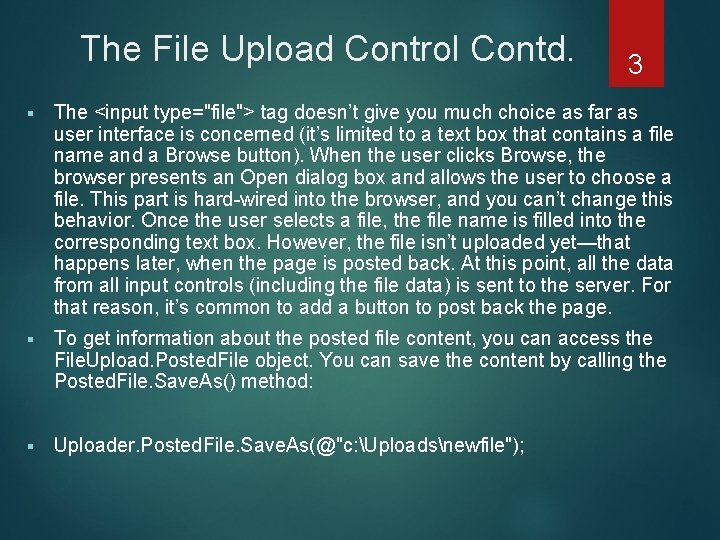
The File Upload Control Contd. 3 The <input type="file"> tag doesn’t give you much choice as far as user interface is concerned (it’s limited to a text box that contains a file name and a Browse button). When the user clicks Browse, the browser presents an Open dialog box and allows the user to choose a file. This part is hard-wired into the browser, and you can’t change this behavior. Once the user selects a file, the file name is filled into the corresponding text box. However, the file isn’t uploaded yet—that happens later, when the page is posted back. At this point, all the data from all input controls (including the file data) is sent to the server. For that reason, it’s common to add a button to post back the page. To get information about the posted file content, you can access the File. Upload. Posted. File object. You can save the content by calling the Posted. File. Save. As() method: Uploader. Posted. File. Save. As(@"c: Uploadsnewfile");
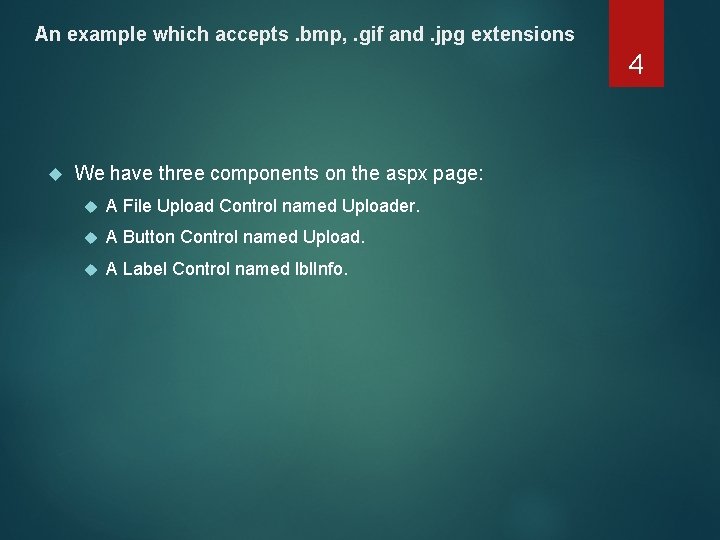
An example which accepts. bmp, . gif and. jpg extensions 4 We have three components on the aspx page: A File Upload Control named Uploader. A Button Control named Upload. A Label Control named lbl. Info.
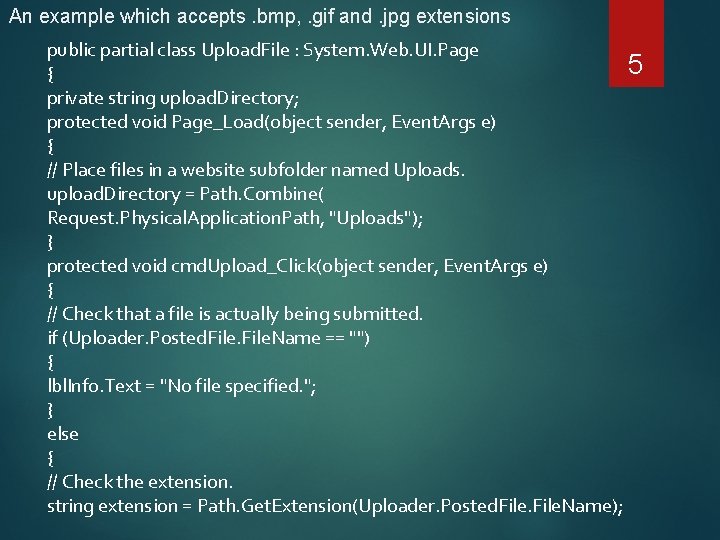
An example which accepts. bmp, . gif and. jpg extensions public partial class Upload. File : System. Web. UI. Page 5 { private string upload. Directory; protected void Page_Load(object sender, Event. Args e) { // Place files in a website subfolder named Uploads. upload. Directory = Path. Combine( Request. Physical. Application. Path, "Uploads"); } protected void cmd. Upload_Click(object sender, Event. Args e) { // Check that a file is actually being submitted. if (Uploader. Posted. File. Name == "") { lbl. Info. Text = "No file specified. "; } else { // Check the extension. string extension = Path. Get. Extension(Uploader. Posted. File. Name);
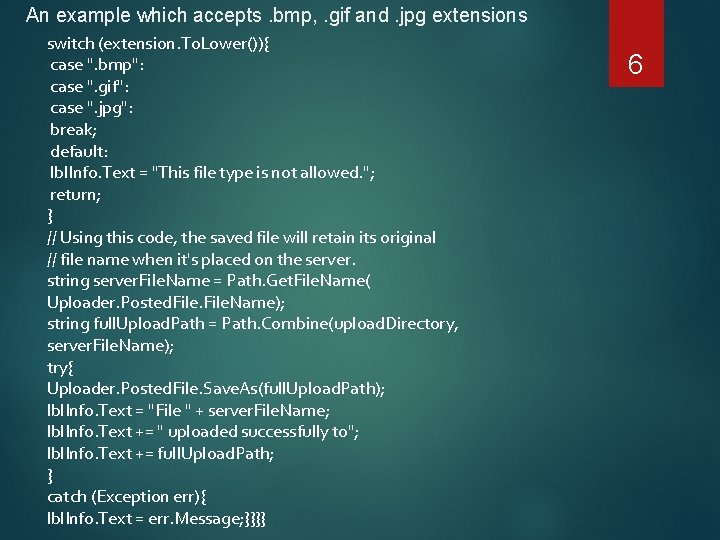
An example which accepts. bmp, . gif and. jpg extensions switch (extension. To. Lower()){ case ". bmp": case ". gif": case ". jpg": break; default: lbl. Info. Text = "This file type is not allowed. "; return; } // Using this code, the saved file will retain its original // file name when it's placed on the server. string server. File. Name = Path. Get. File. Name( Uploader. Posted. File. Name); string full. Upload. Path = Path. Combine(upload. Directory, server. File. Name); try{ Uploader. Posted. File. Save. As(full. Upload. Path); lbl. Info. Text = "File " + server. File. Name; lbl. Info. Text += " uploaded successfully to"; lbl. Info. Text += full. Upload. Path; } catch (Exception err){ lbl. Info. Text = err. Message; }}}} 6
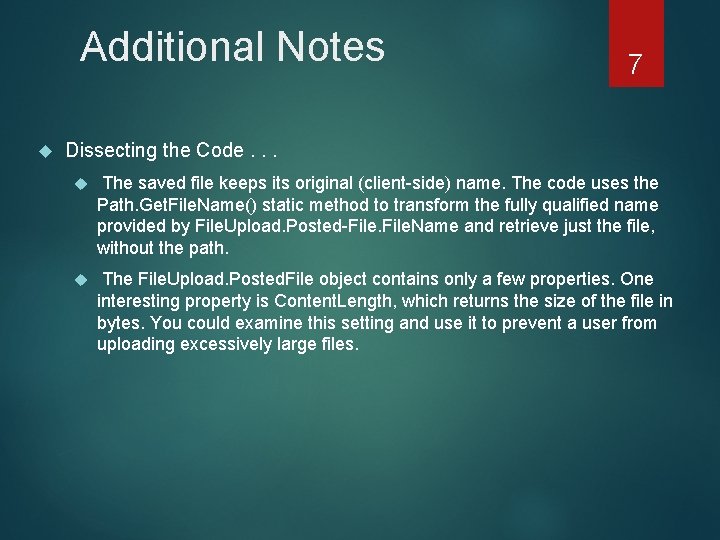
Additional Notes 7 Dissecting the Code. . . The saved file keeps its original (client-side) name. The code uses the Path. Get. File. Name() static method to transform the fully qualified name provided by File. Upload. Posted-File. Name and retrieve just the file, without the path. The File. Upload. Posted. File object contains only a few properties. One interesting property is Content. Length, which returns the size of the file in bytes. You could examine this setting and use it to prevent a user from uploading excessively large files.
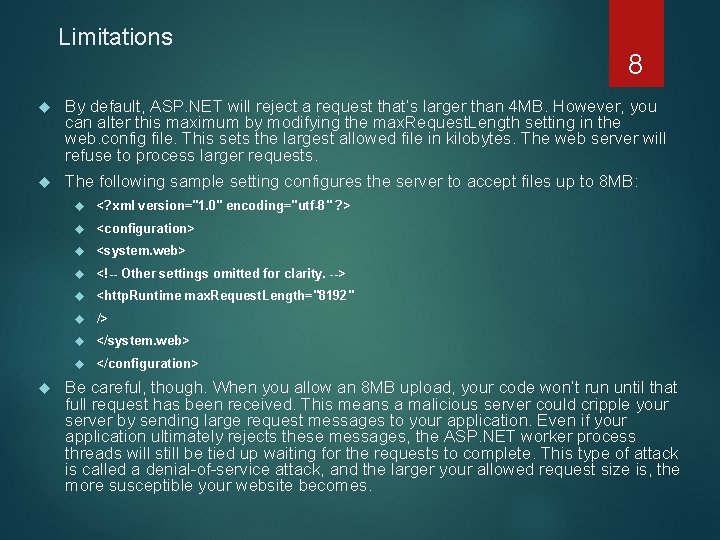
Limitations 8 By default, ASP. NET will reject a request that’s larger than 4 MB. However, you can alter this maximum by modifying the max. Request. Length setting in the web. config file. This sets the largest allowed file in kilobytes. The web server will refuse to process larger requests. The following sample setting configures the server to accept files up to 8 MB: <? xml version="1. 0" encoding="utf-8" ? > <configuration> <system. web> <!-- Other settings omitted for clarity. --> <http. Runtime max. Request. Length="8192" /> </system. web> </configuration> Be careful, though. When you allow an 8 MB upload, your code won’t run until that full request has been received. This means a malicious server could cripple your server by sending large request messages to your application. Even if your application ultimately rejects these messages, the ASP. NET worker process threads will still be tied up waiting for the requests to complete. This type of attack is called a denial-of-service attack, and the larger your allowed request size is, the more susceptible your website becomes.