AJAX Asynchronous Java Script and XML AJAX An
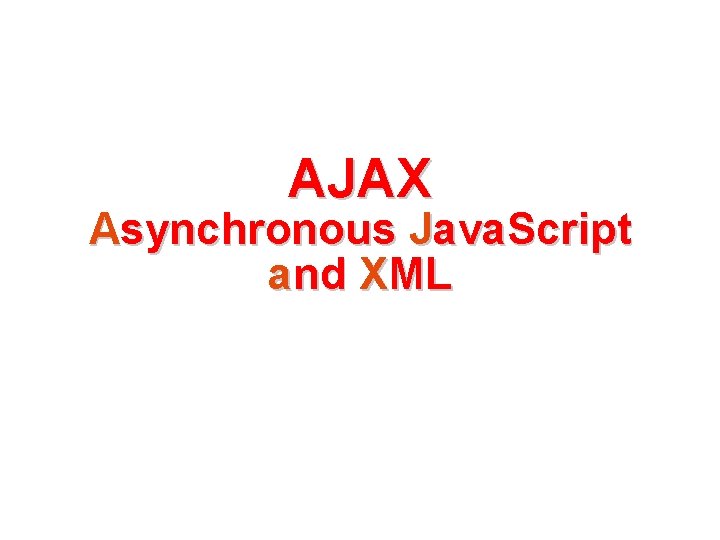
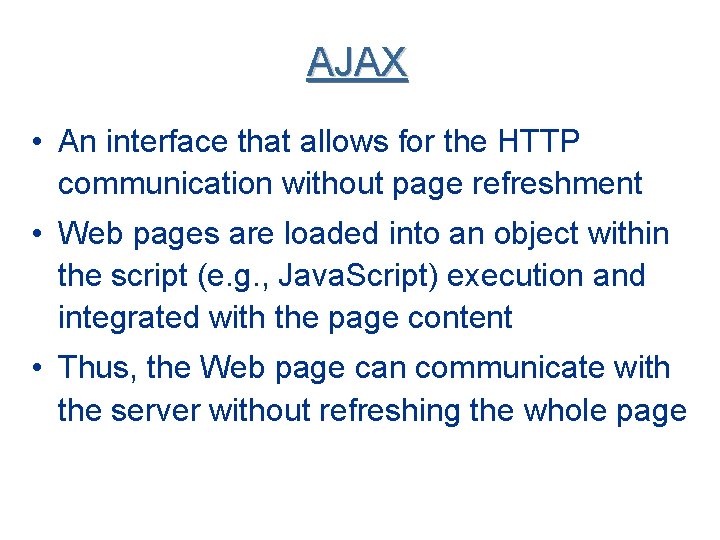
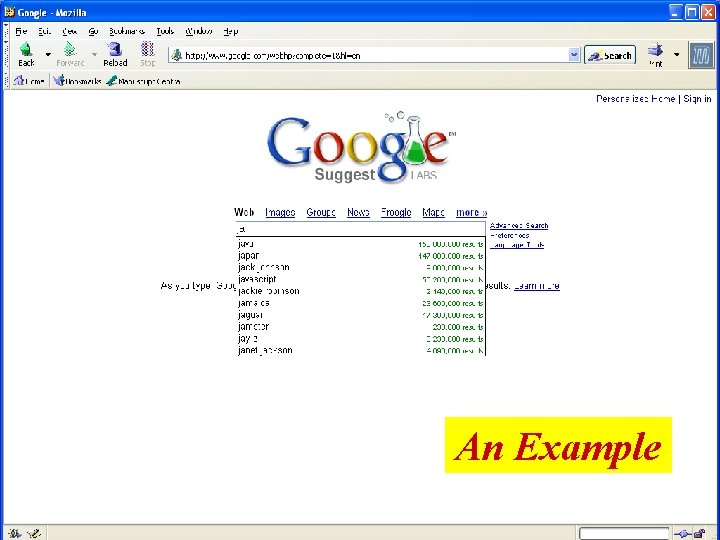
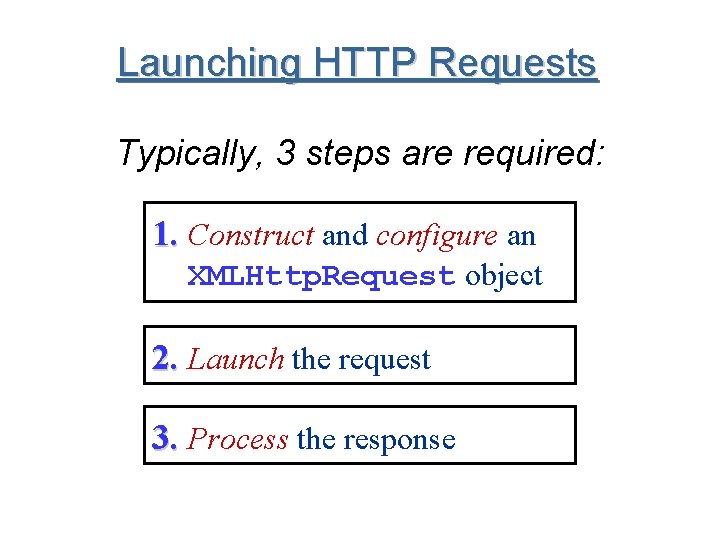
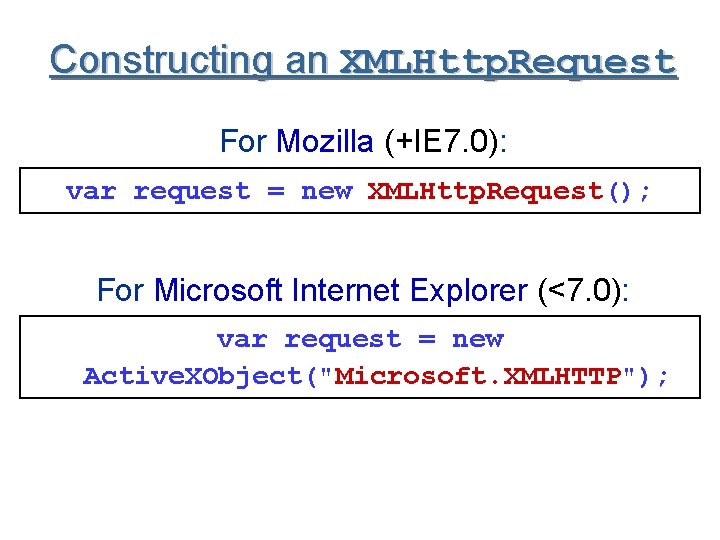
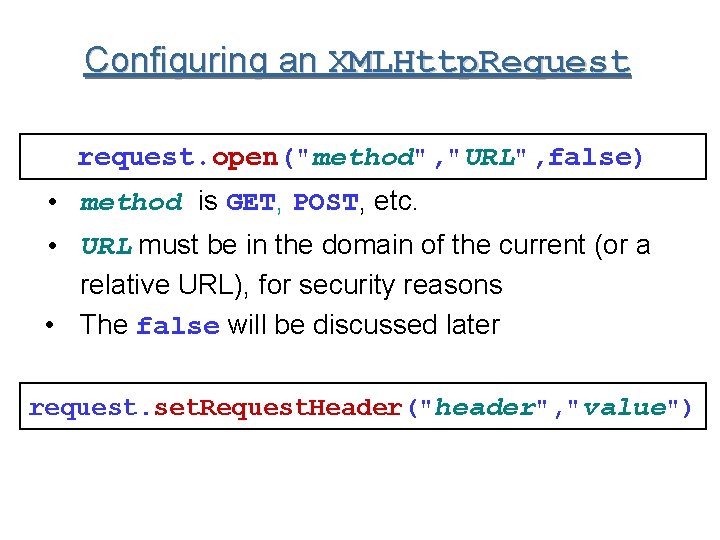
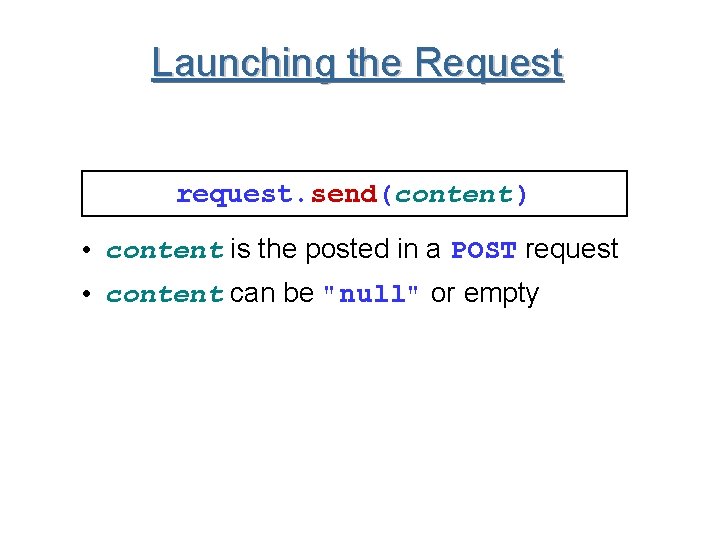
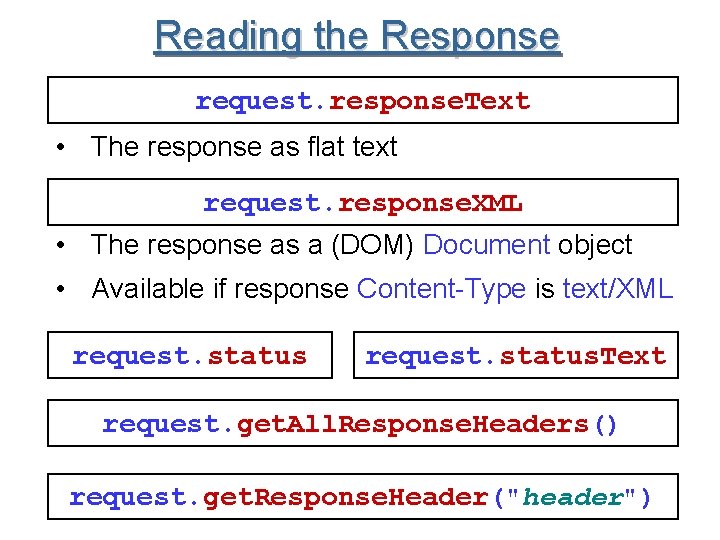
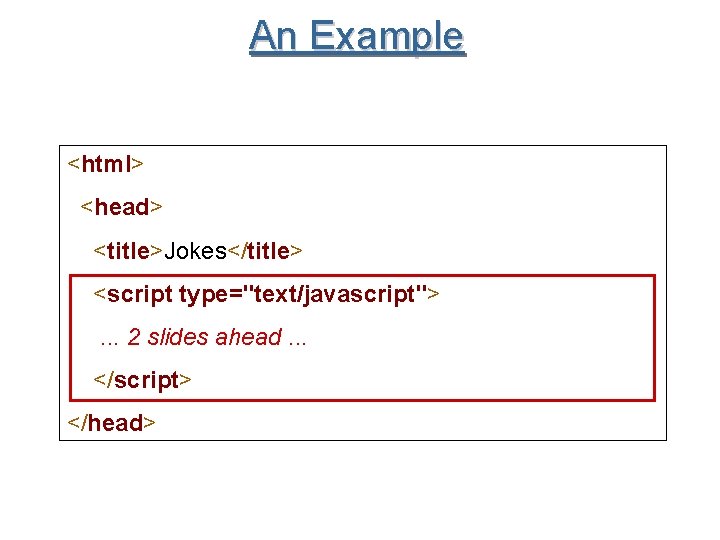
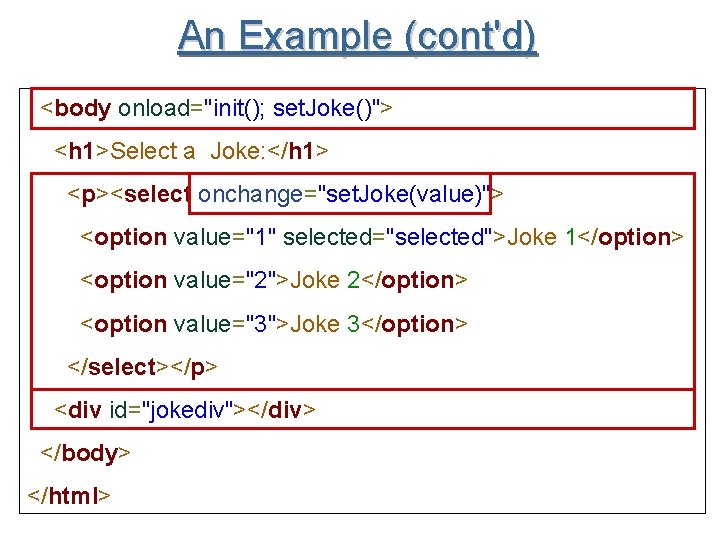
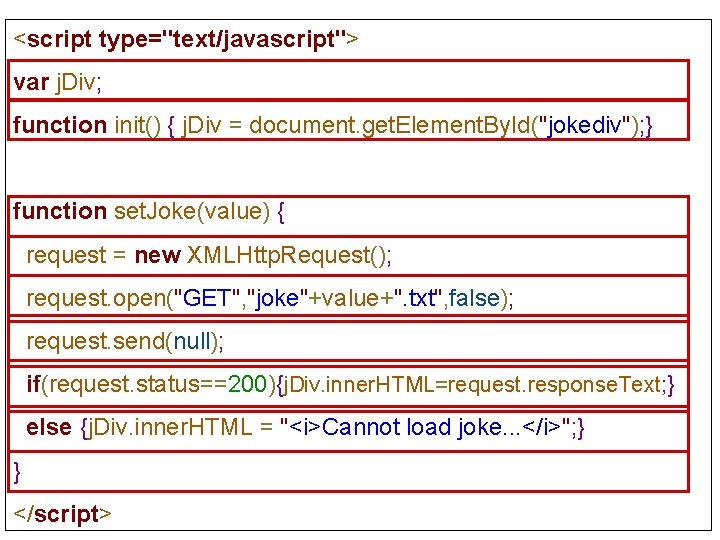
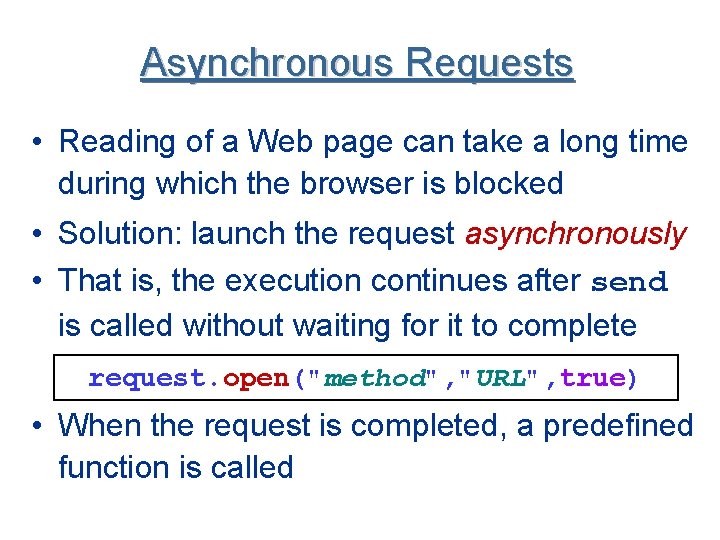
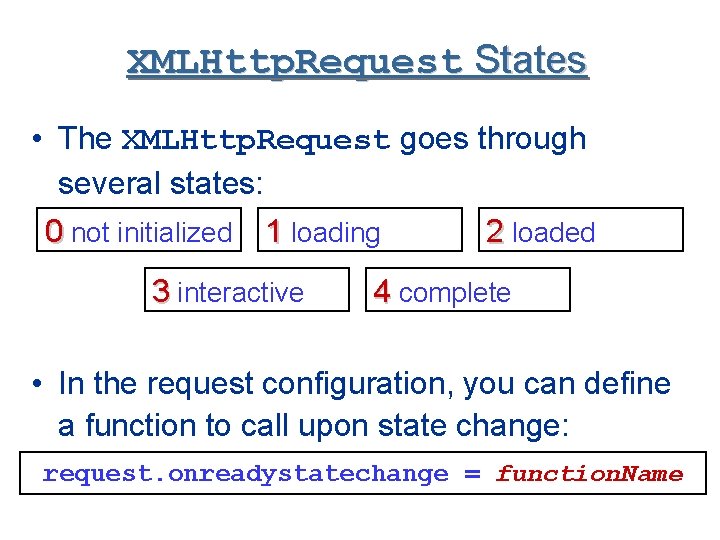
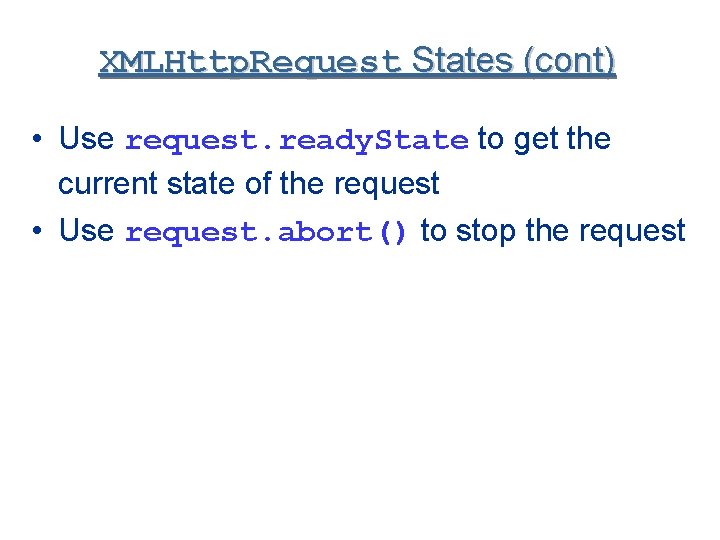
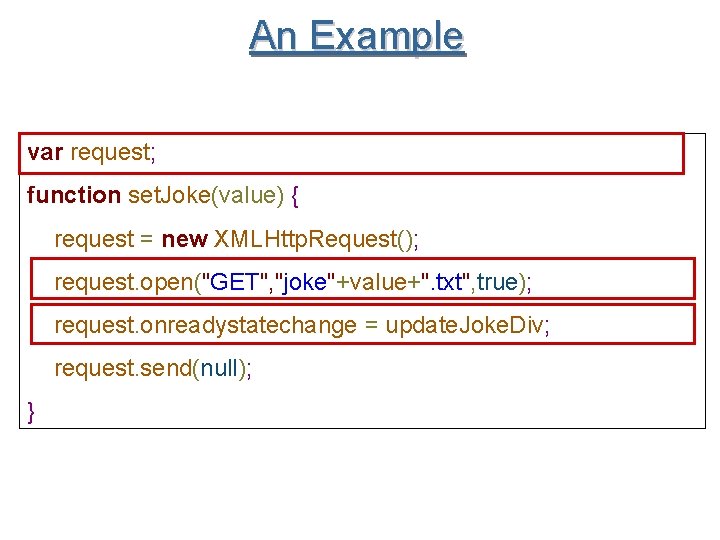
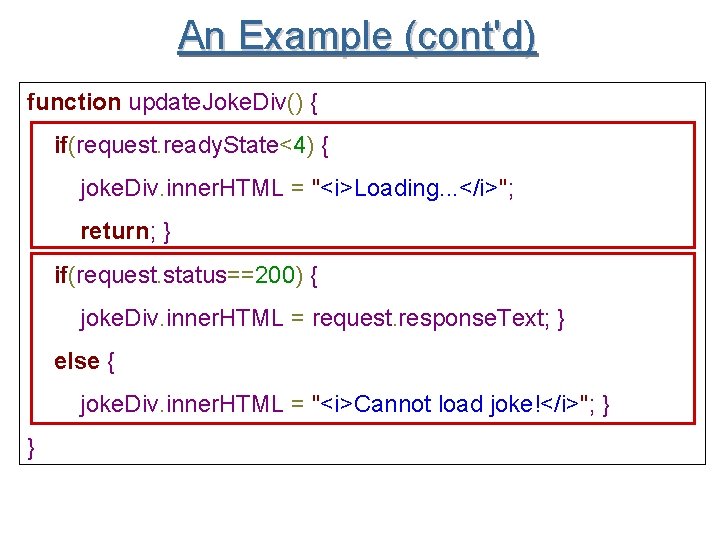
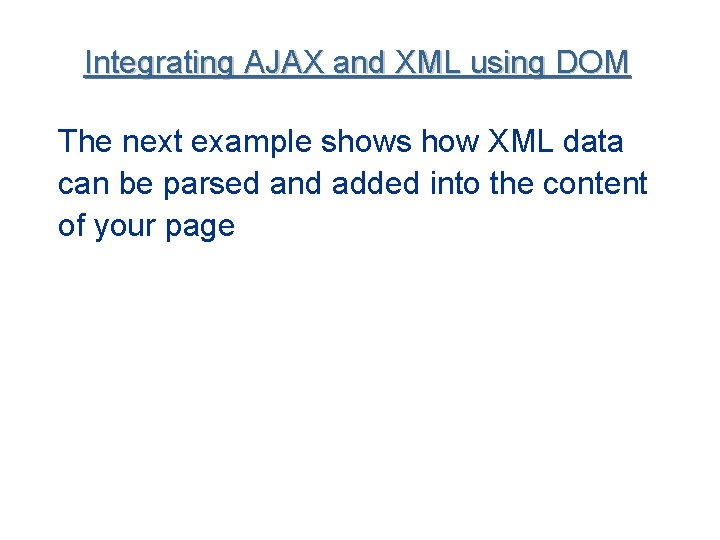
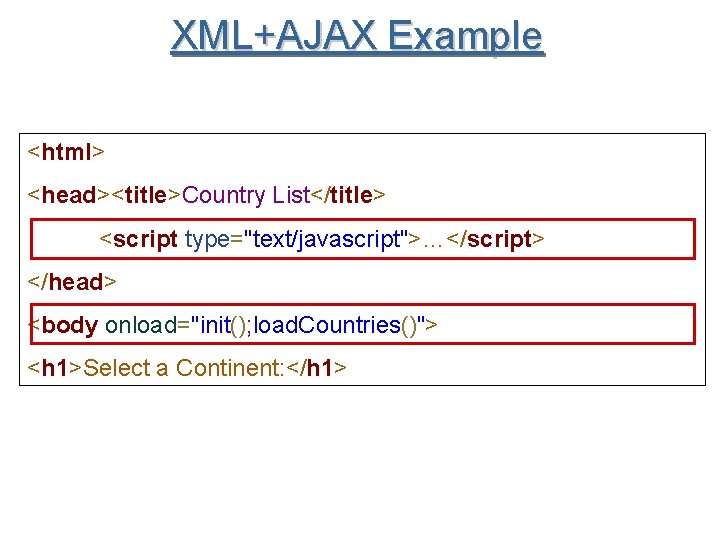
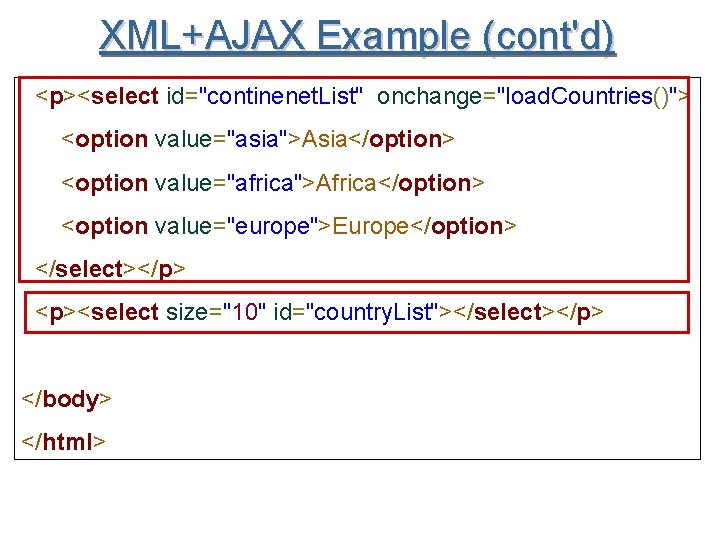
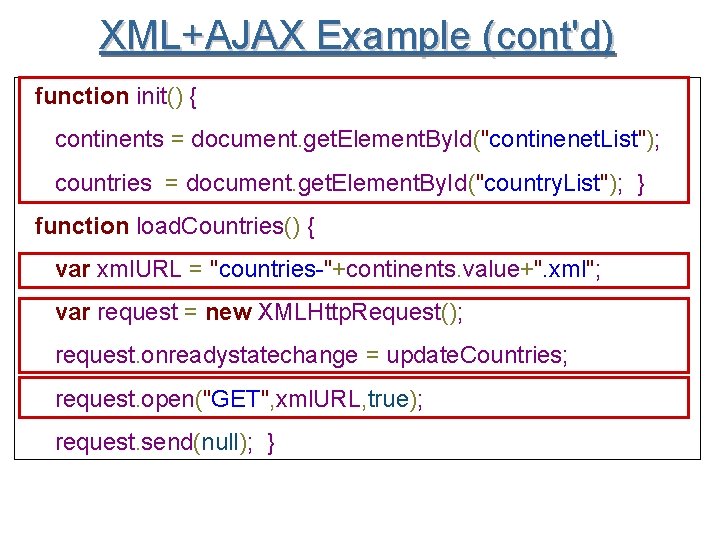
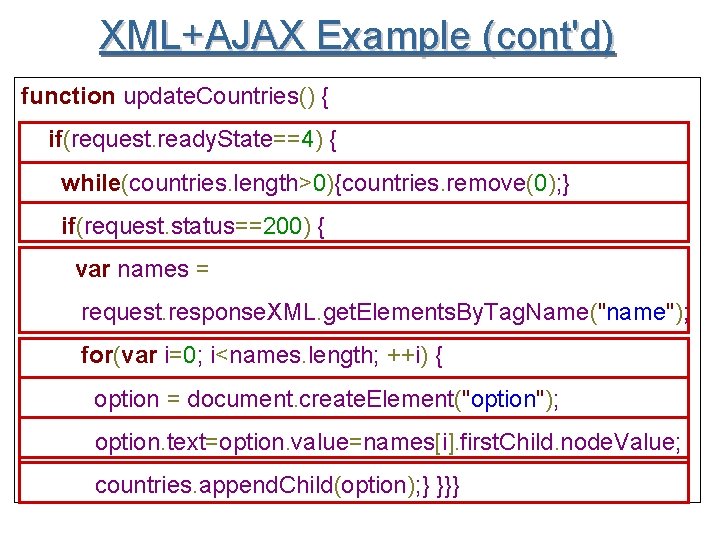
- Slides: 21
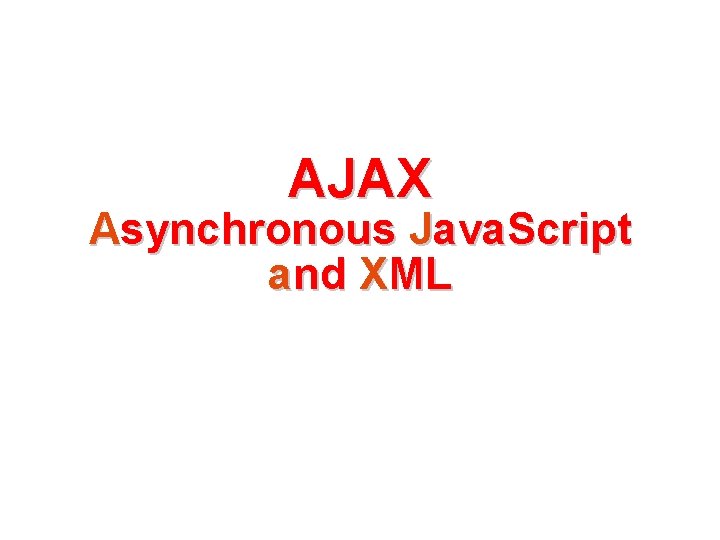
AJAX Asynchronous Java. Script and XML
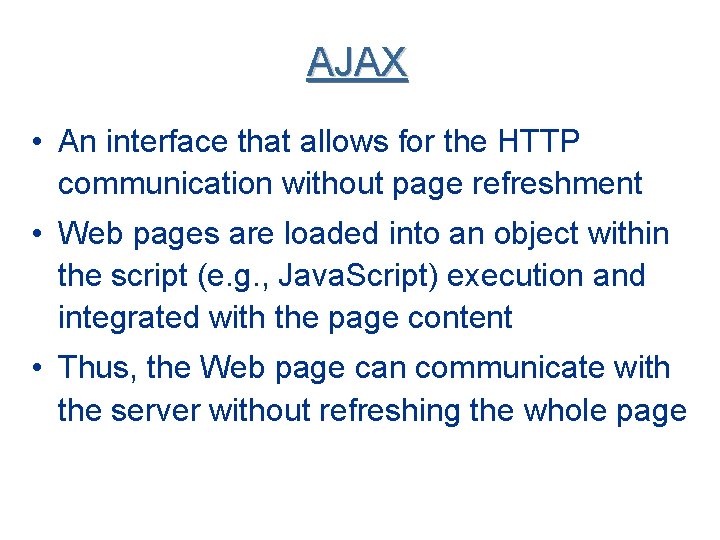
AJAX • An interface that allows for the HTTP communication without page refreshment • Web pages are loaded into an object within the script (e. g. , Java. Script) execution and integrated with the page content • Thus, the Web page can communicate with the server without refreshing the whole page
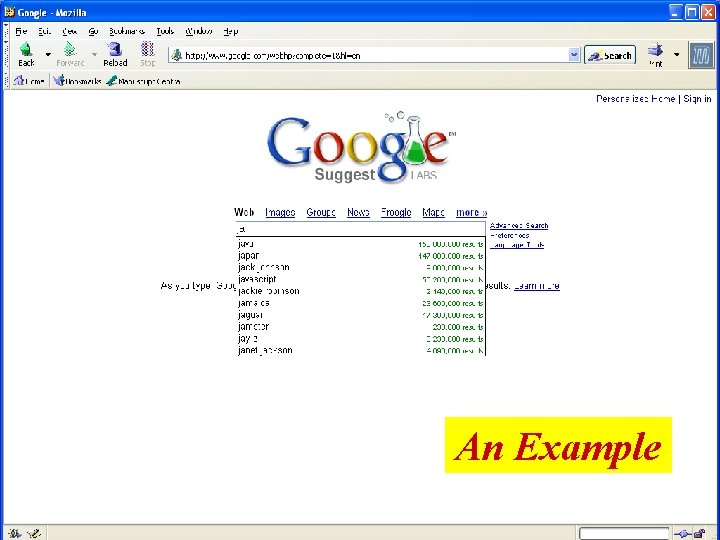
An Example
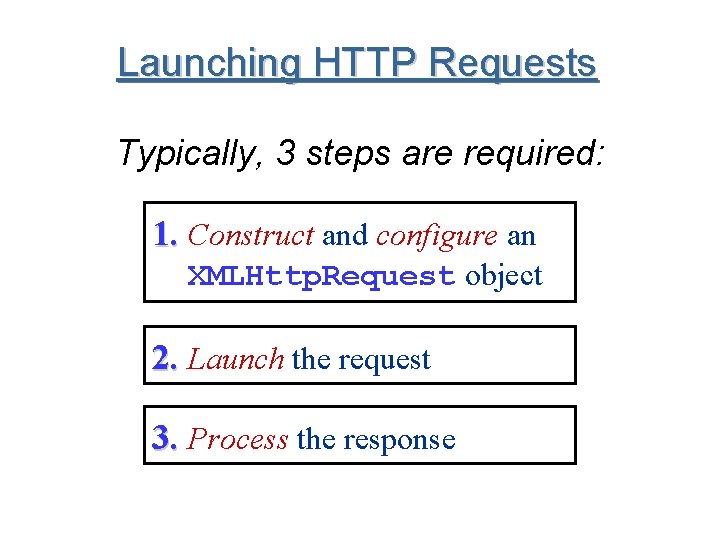
Launching HTTP Requests Typically, 3 steps are required: 1. Construct and configure an XMLHttp. Request object 2. Launch the request 3. Process the response
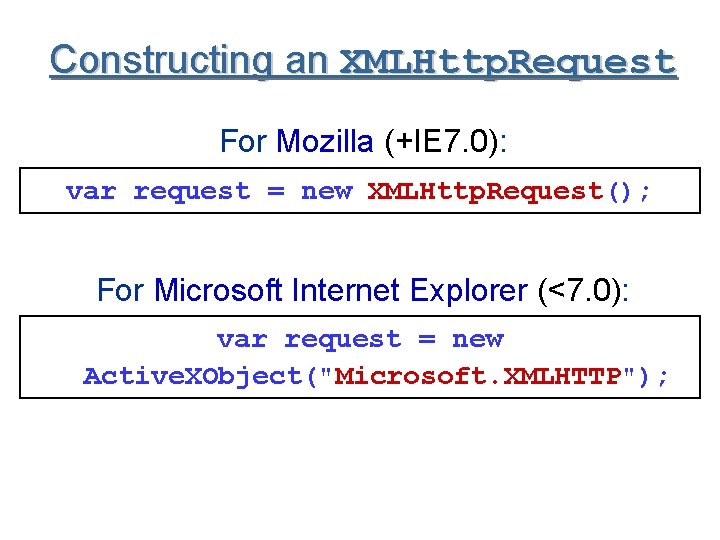
Constructing an XMLHttp. Request For Mozilla (+IE 7. 0): var request = new XMLHttp. Request(); For Microsoft Internet Explorer (<7. 0): var request = new Active. XObject("Microsoft. XMLHTTP");
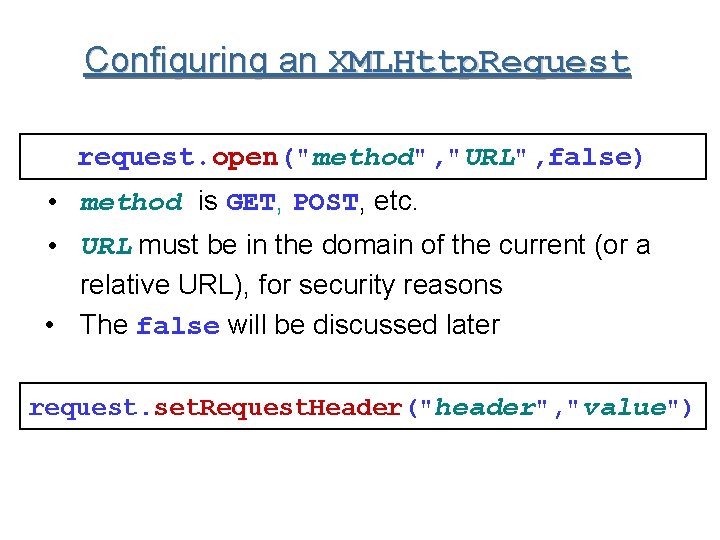
Configuring an XMLHttp. Request request. open("method", "URL", false) • method is GET, POST, etc. • URL must be in the domain of the current (or a relative URL), for security reasons • The false will be discussed later request. set. Request. Header("header", "value")
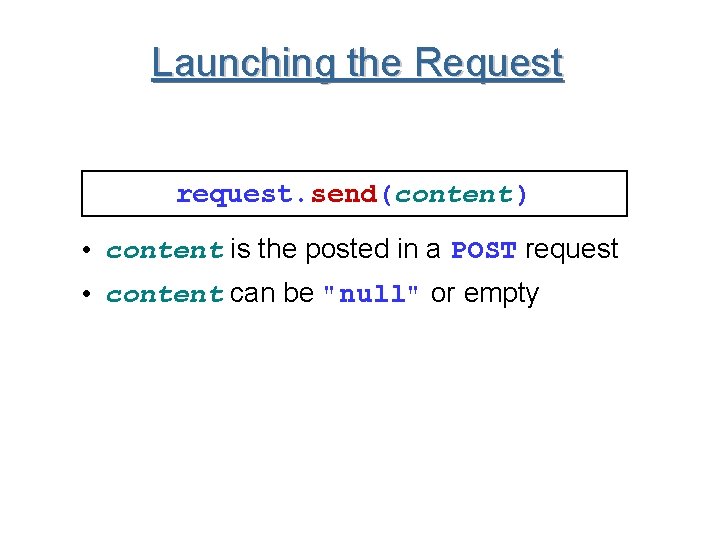
Launching the Request request. send(content ) • content is the posted in a POST request • content can be "null" or empty
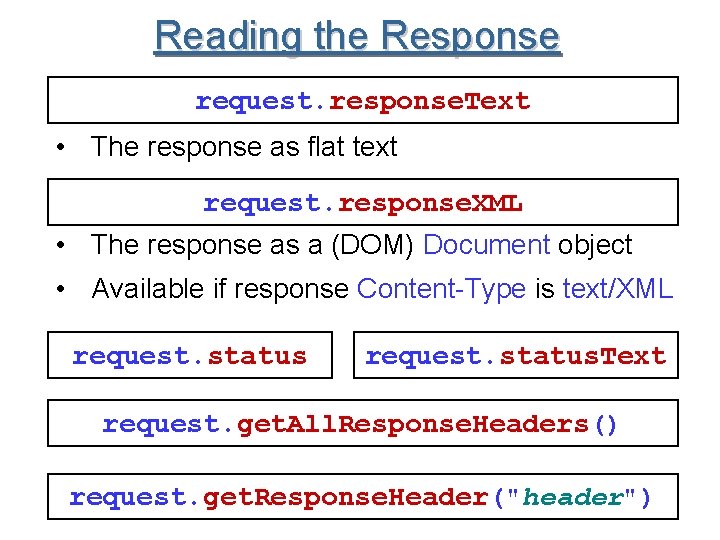
Reading the Response request. response. Text • The response as flat text request. response. XML • The response as a (DOM) Document object • Available if response Content-Type is text/XML request. status. Text request. get. All. Response. Headers() request. get. Response. Header("header")
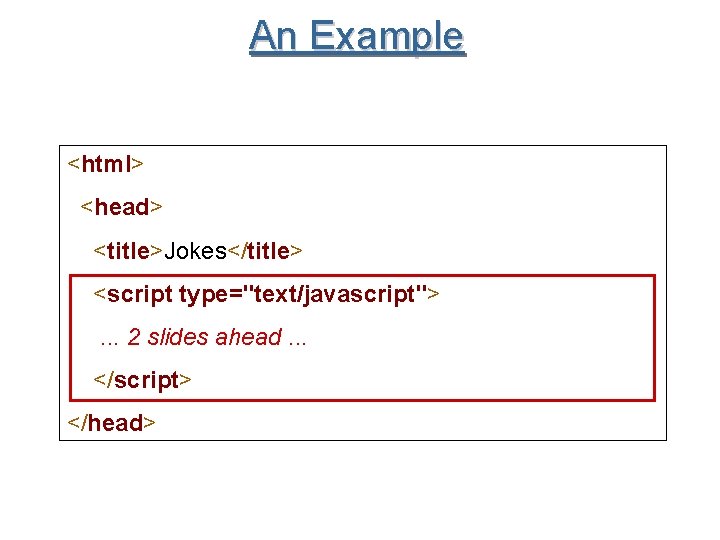
An Example <html> <head> <title>Jokes</title> <script type="text/javascript">. . . 2 slides ahead. . . </script> </head>
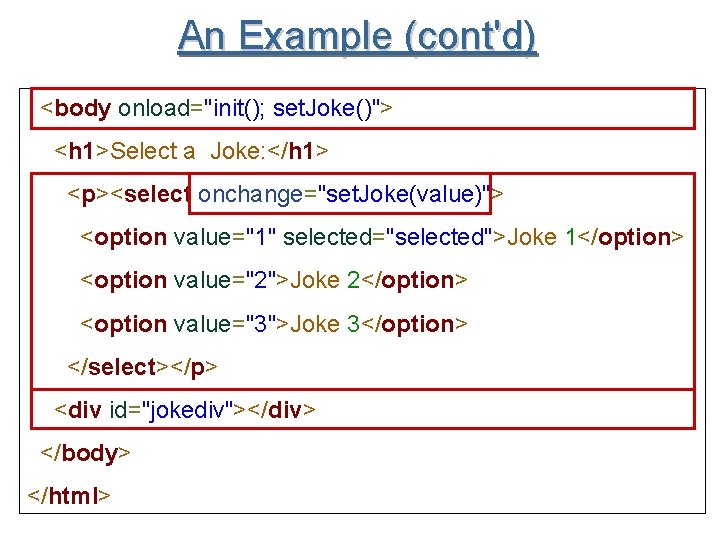
An Example (cont'd) <body onload="init(); set. Joke()"> <h 1>Select a Joke: </h 1> <p><select onchange="set. Joke(value)"> <option value="1" selected="selected">Joke 1</option> <option value="2">Joke 2</option> <option value="3">Joke 3</option> </select></p> <div id="jokediv"></div> </body> </html>
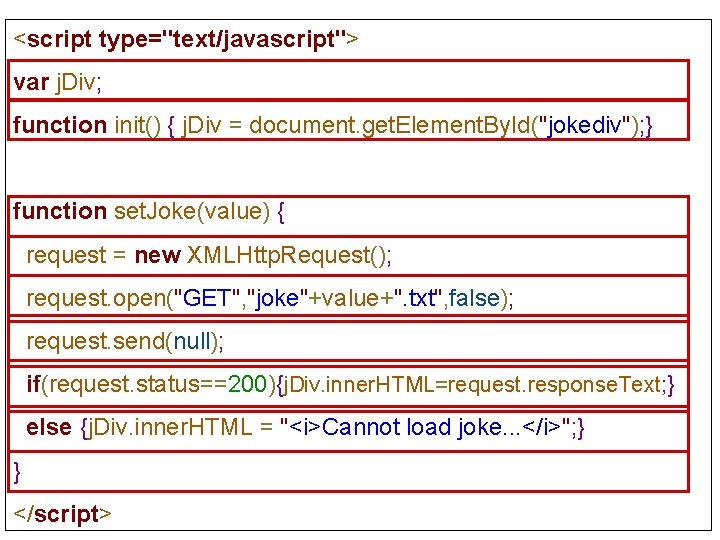
<script type="text/javascript"> var j. Div; function init() { j. Div = document. get. Element. By. Id("jokediv"); } function set. Joke(value) { request = new XMLHttp. Request(); request. open("GET", "joke"+value+". txt", false); request. send(null); if(request. status==200){j. Div. inner. HTML=request. response. Text; } else {j. Div. inner. HTML = "<i>Cannot load joke. . . </i>"; } } </script>
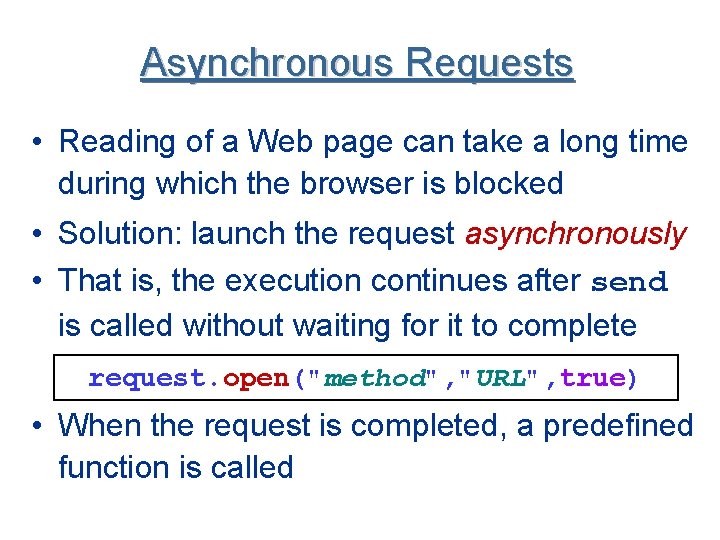
Asynchronous Requests • Reading of a Web page can take a long time during which the browser is blocked • Solution: launch the request asynchronously • That is, the execution continues after send is called without waiting for it to complete request. open("method", "URL", true) • When the request is completed, a predefined function is called
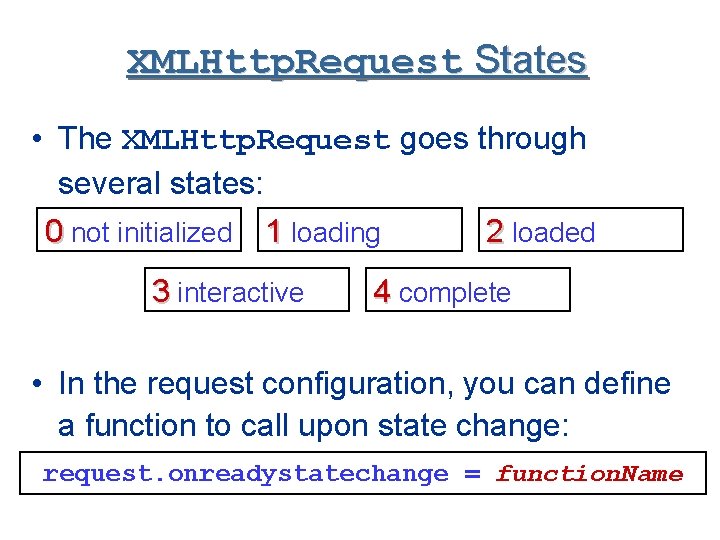
XMLHttp. Request States • The XMLHttp. Request goes through several states: 0 not initialized 1 loading 2 loaded 3 interactive 4 complete • In the request configuration, you can define a function to call upon state change: request. onreadystatechange = function. Name
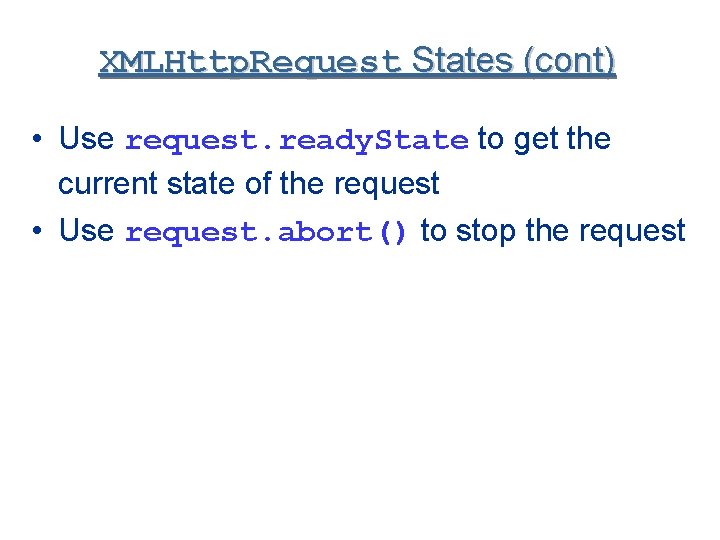
XMLHttp. Request States (cont) • Use request. ready. State to get the current state of the request • Use request. abort() to stop the request
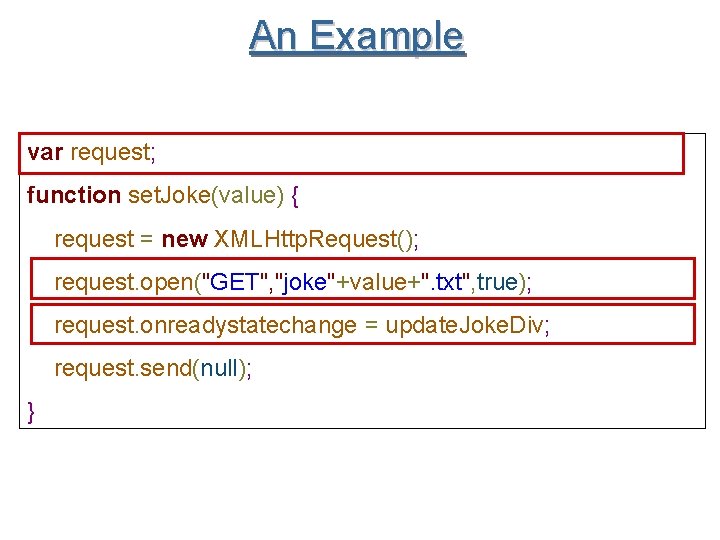
An Example var request; function set. Joke(value) { request = new XMLHttp. Request(); request. open("GET", "joke"+value+". txt", true); request. onreadystatechange = update. Joke. Div; request. send(null); }
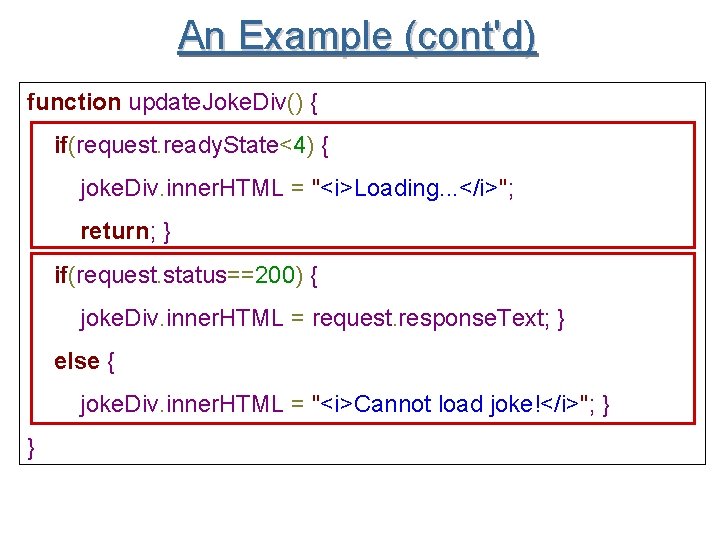
An Example (cont'd) function update. Joke. Div() { if(request. ready. State<4) { joke. Div. inner. HTML = "<i>Loading. . . </i>"; return; } if(request. status==200) { joke. Div. inner. HTML = request. response. Text; } else { joke. Div. inner. HTML = "<i>Cannot load joke!</i>"; } }
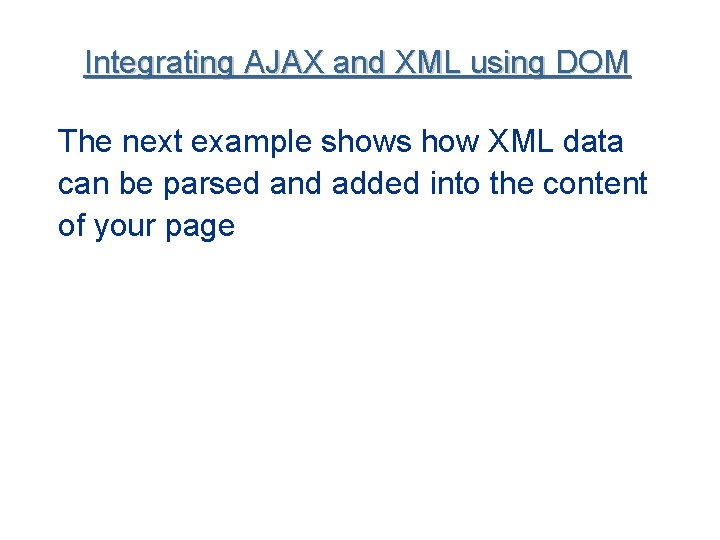
Integrating AJAX and XML using DOM The next example shows how XML data can be parsed and added into the content of your page
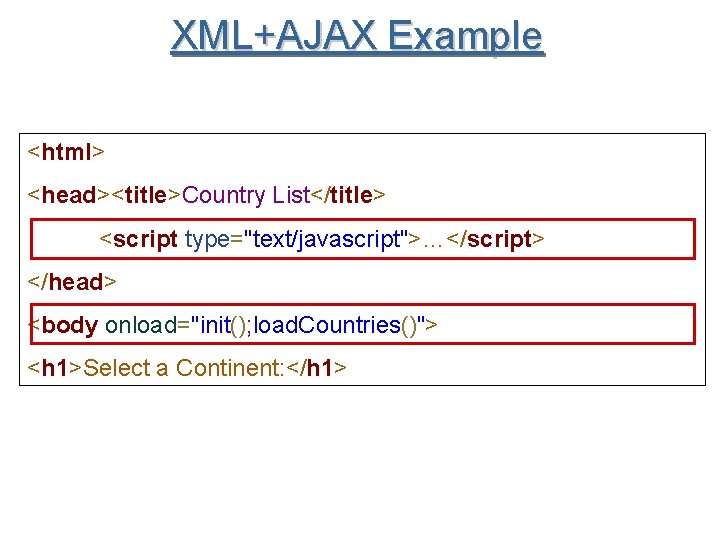
XML+AJAX Example <html> <head><title>Country List</title> <script type="text/javascript">…</script> </head> <body onload="init(); load. Countries()"> <h 1>Select a Continent: </h 1>
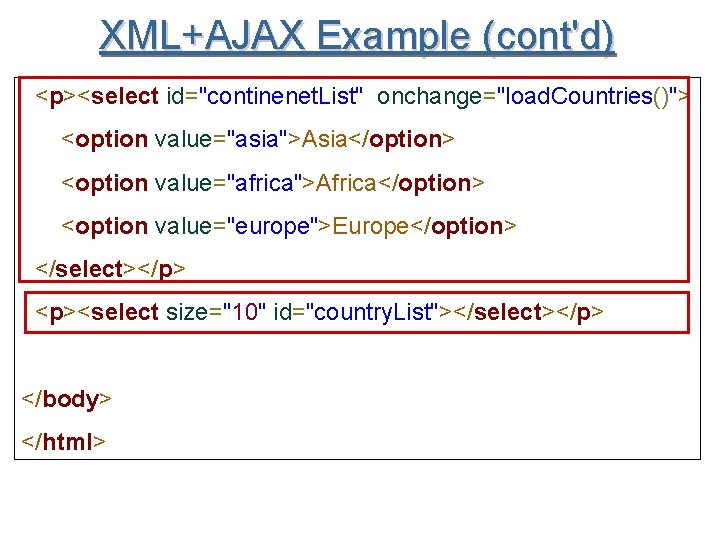
XML+AJAX Example (cont'd) <p><select id="continenet. List" onchange="load. Countries()"> <option value="asia">Asia</option> <option value="africa">Africa</option> <option value="europe">Europe</option> </select></p> <p><select size="10" id="country. List"></select></p> </body> </html>
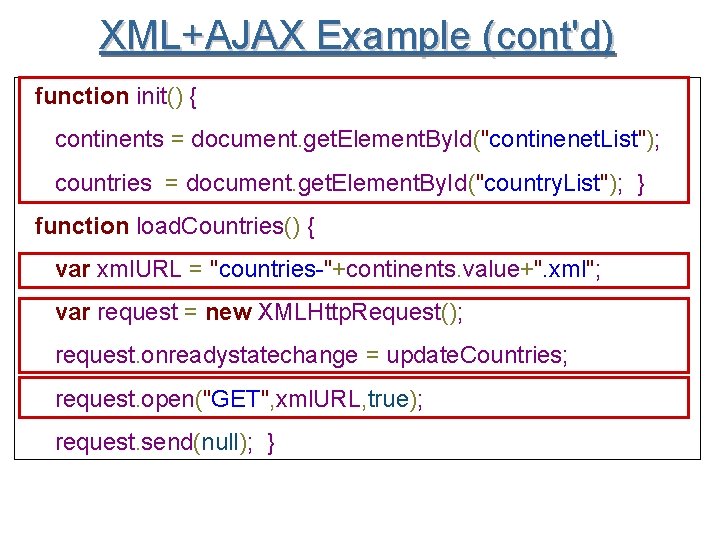
XML+AJAX Example (cont'd) function init() { continents = document. get. Element. By. Id("continenet. List"); countries = document. get. Element. By. Id("country. List"); } function load. Countries() { var xml. URL = "countries-"+continents. value+". xml"; var request = new XMLHttp. Request(); request. onreadystatechange = update. Countries; request. open("GET", xml. URL, true); request. send(null); }
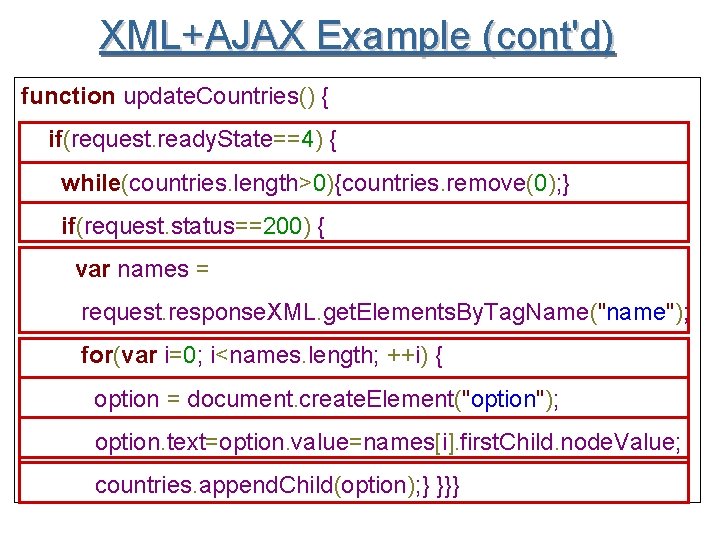
XML+AJAX Example (cont'd) function update. Countries() { if(request. ready. State==4) { while(countries. length>0){countries. remove(0); } if(request. status==200) { var names = request. response. XML. get. Elements. By. Tag. Name("name"); for(var i=0; i<names. length; ++i) { option = document. create. Element("option"); option. text=option. value=names[i]. first. Child. node. Value; countries. append. Child(option); } }}}