Agenda Two dimension array Array List class Wrapper
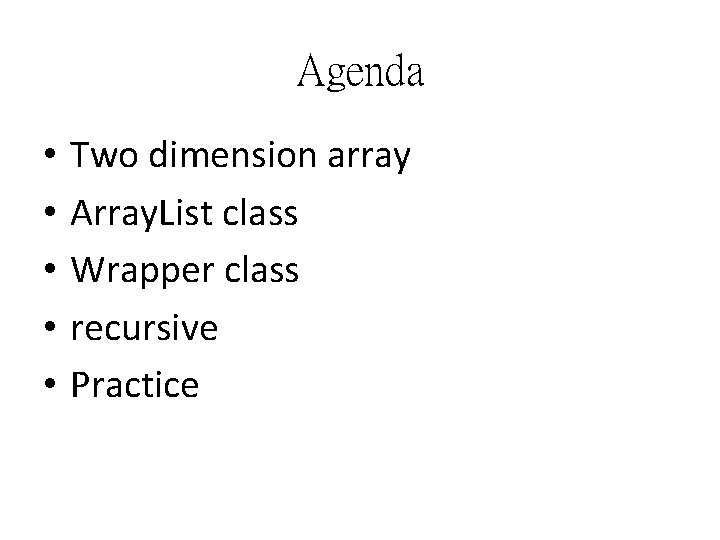
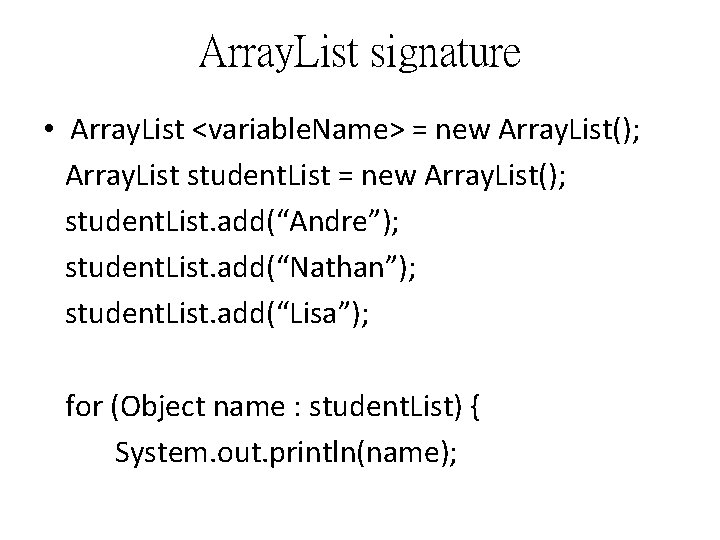
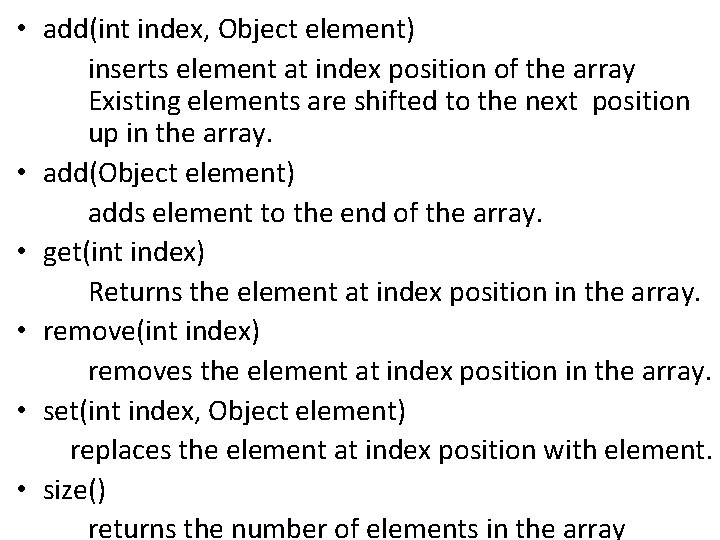
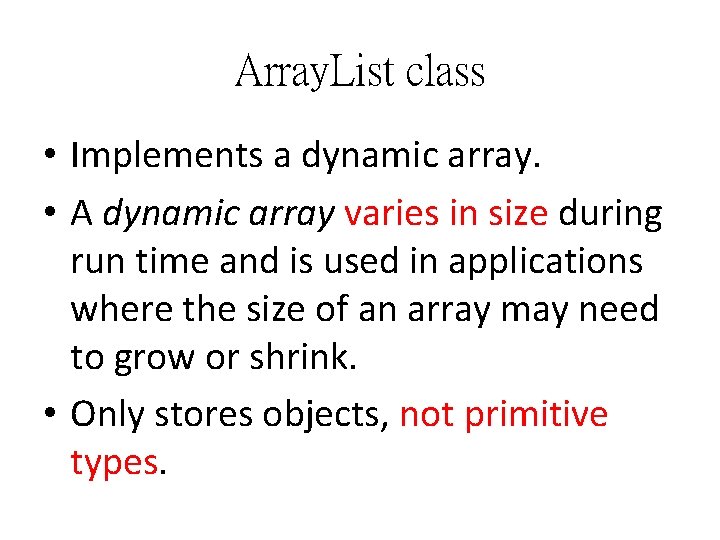
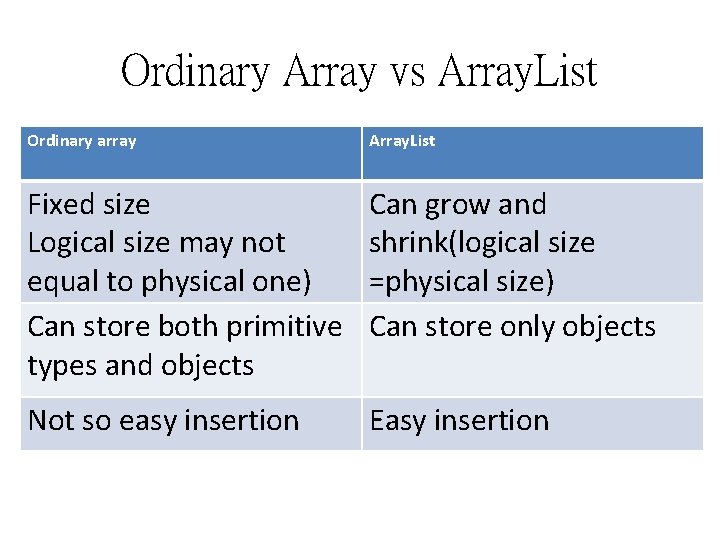
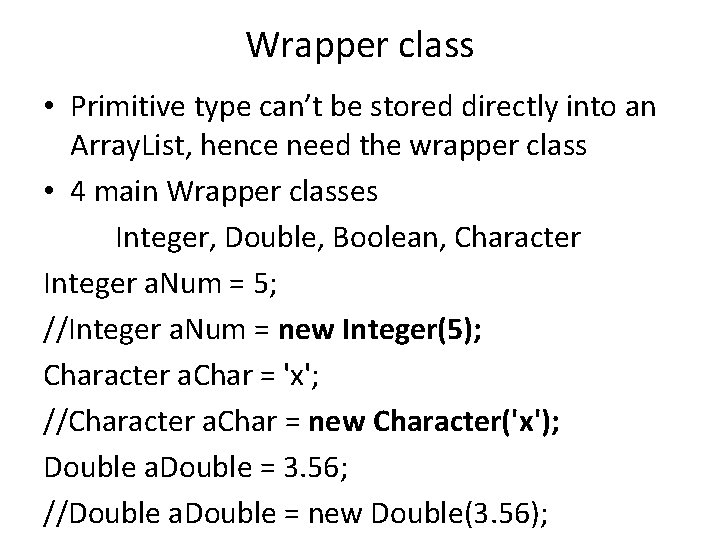
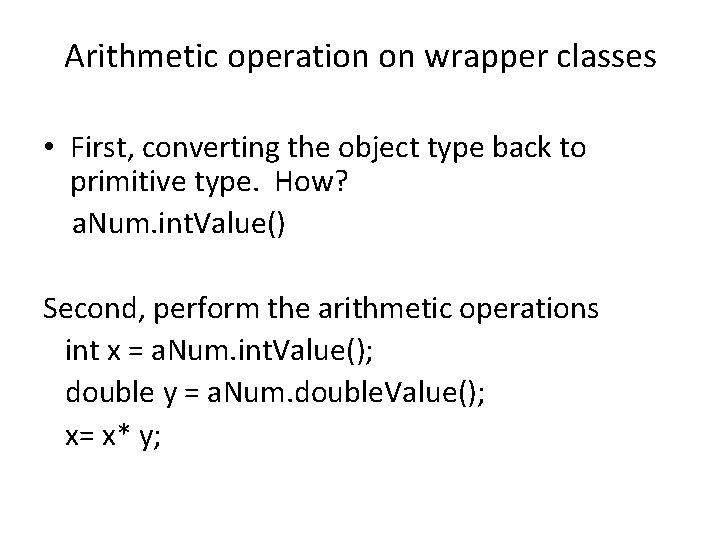
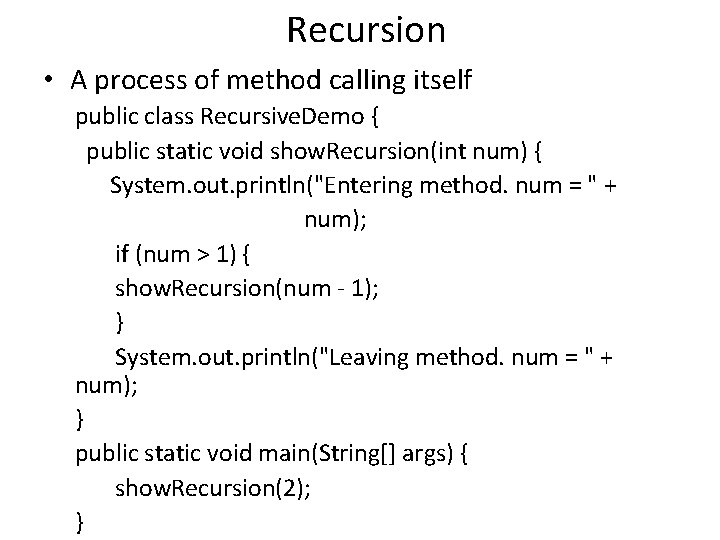
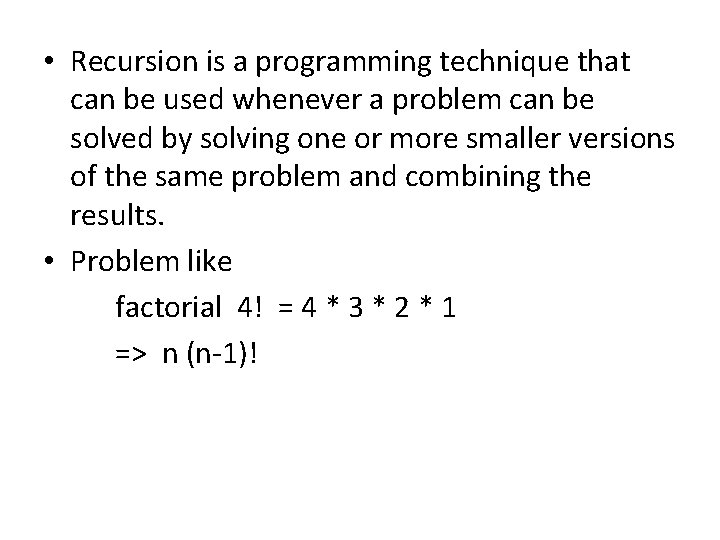
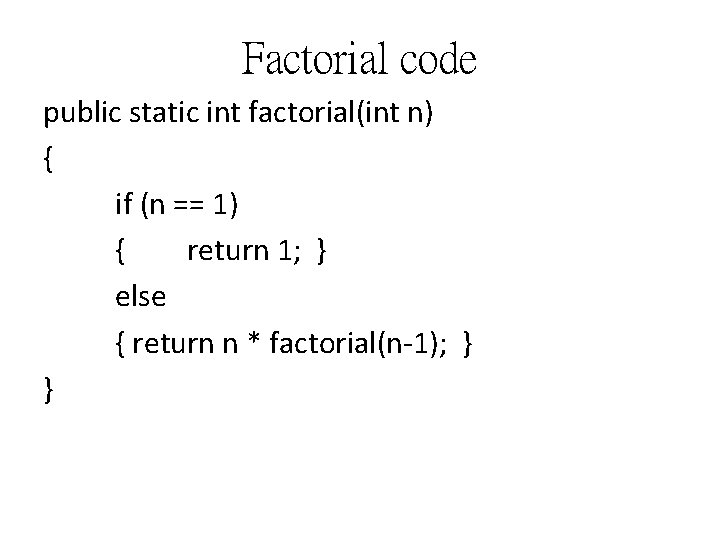
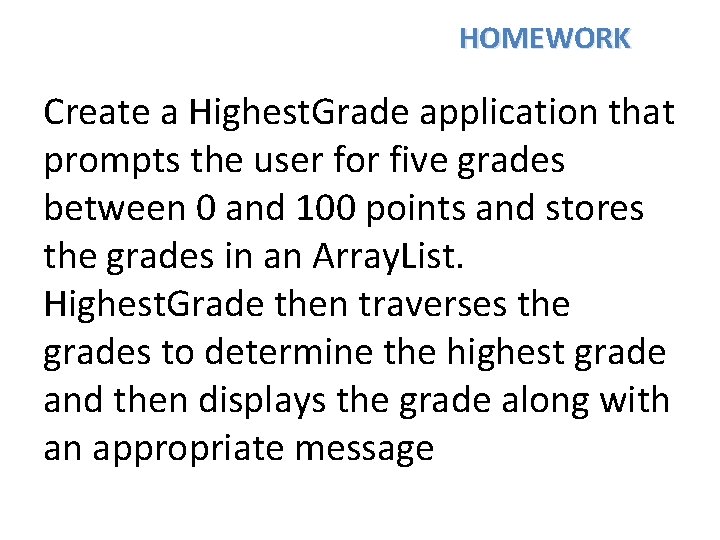
- Slides: 11
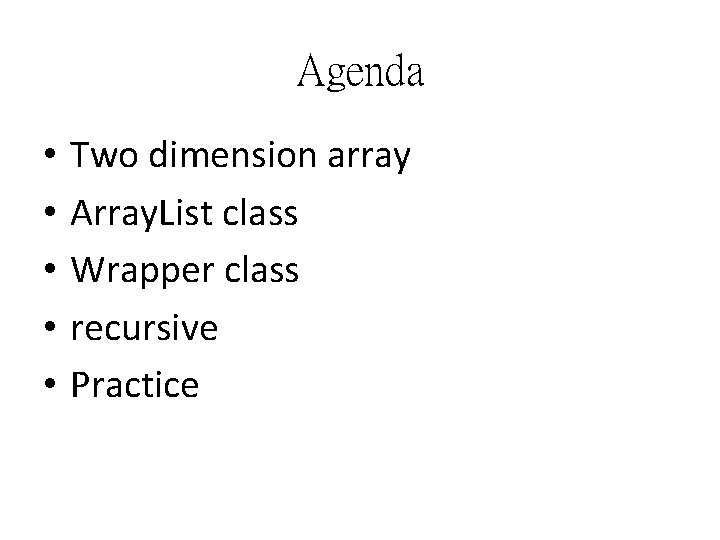
Agenda • • • Two dimension array Array. List class Wrapper class recursive Practice
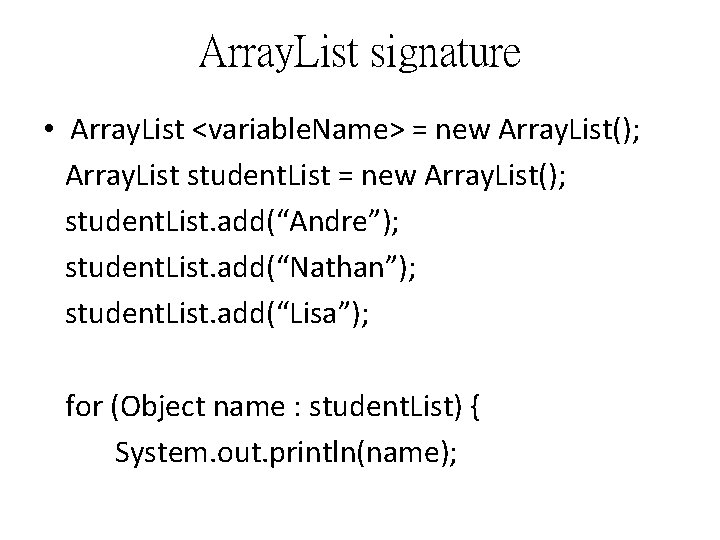
Array. List signature • Array. List <variable. Name> = new Array. List(); Array. List student. List = new Array. List(); student. List. add(“Andre”); student. List. add(“Nathan”); student. List. add(“Lisa”); for (Object name : student. List) { System. out. println(name);
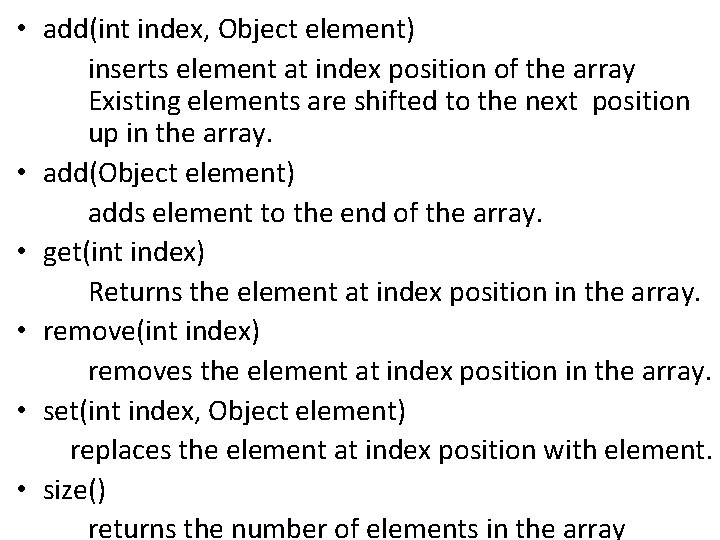
• add(int index, Object element) inserts element at index position of the array Existing elements are shifted to the next position up in the array. • add(Object element) adds element to the end of the array. • get(int index) Returns the element at index position in the array. • remove(int index) removes the element at index position in the array. • set(int index, Object element) replaces the element at index position with element. • size() returns the number of elements in the array
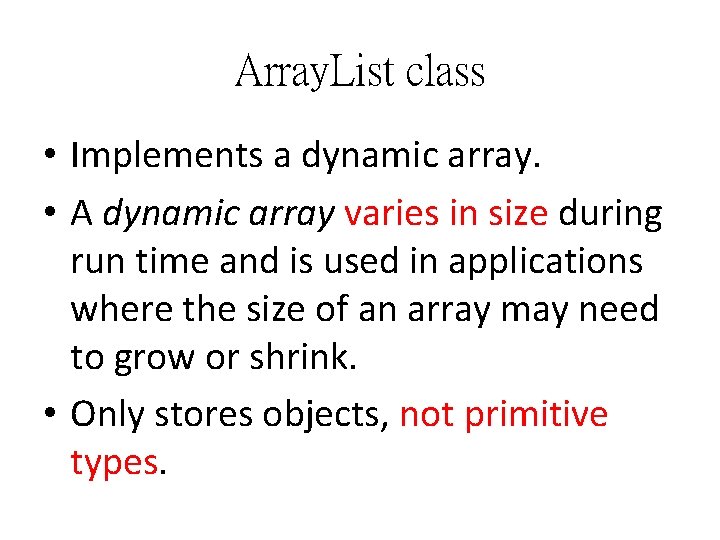
Array. List class • Implements a dynamic array. • A dynamic array varies in size during run time and is used in applications where the size of an array may need to grow or shrink. • Only stores objects, not primitive types.
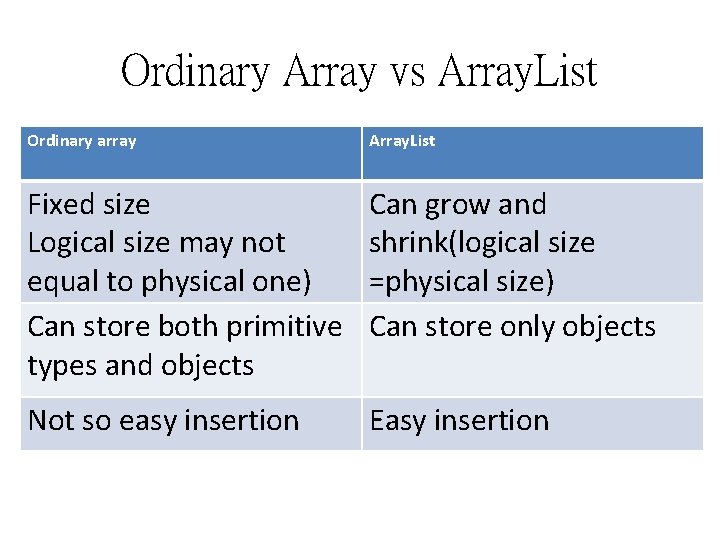
Ordinary Array vs Array. List Ordinary array Array. List Fixed size Logical size may not equal to physical one) Can store both primitive types and objects Can grow and shrink(logical size =physical size) Can store only objects Not so easy insertion Easy insertion
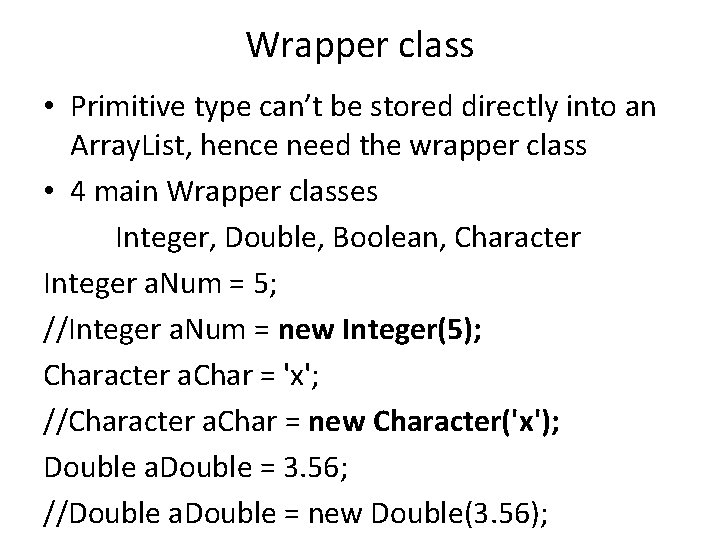
Wrapper class • Primitive type can’t be stored directly into an Array. List, hence need the wrapper class • 4 main Wrapper classes Integer, Double, Boolean, Character Integer a. Num = 5; //Integer a. Num = new Integer(5); Character a. Char = 'x'; //Character a. Char = new Character('x'); Double a. Double = 3. 56; //Double a. Double = new Double(3. 56);
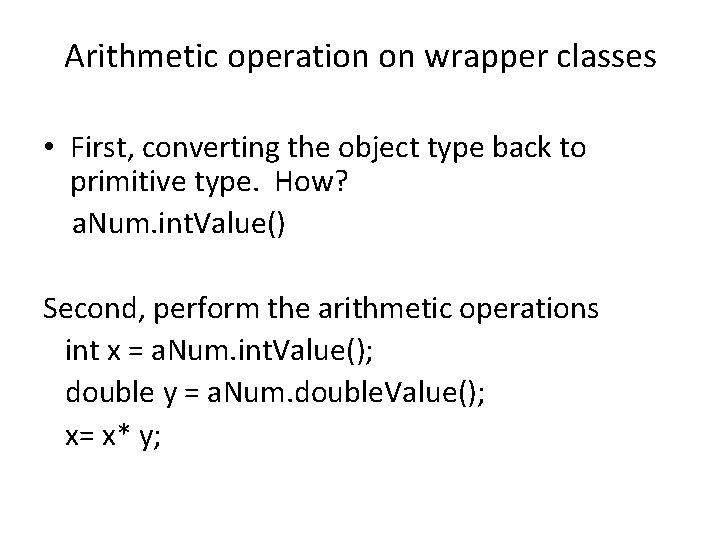
Arithmetic operation on wrapper classes • First, converting the object type back to primitive type. How? a. Num. int. Value() Second, perform the arithmetic operations int x = a. Num. int. Value(); double y = a. Num. double. Value(); x= x* y;
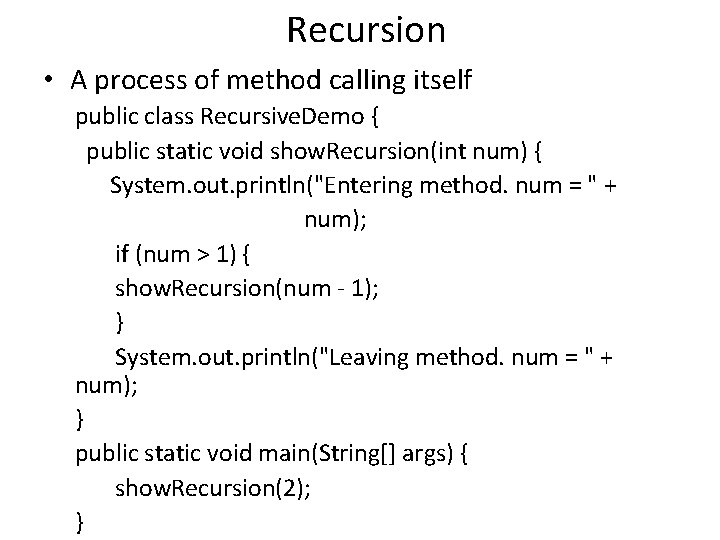
Recursion • A process of method calling itself public class Recursive. Demo { public static void show. Recursion(int num) { System. out. println("Entering method. num = " + num); if (num > 1) { show. Recursion(num - 1); } System. out. println("Leaving method. num = " + num); } public static void main(String[] args) { show. Recursion(2); }
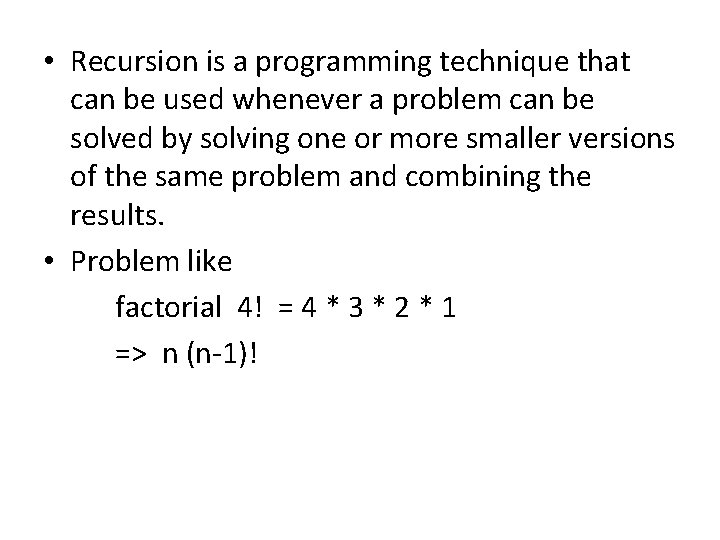
• Recursion is a programming technique that can be used whenever a problem can be solved by solving one or more smaller versions of the same problem and combining the results. • Problem like factorial 4! = 4 * 3 * 2 * 1 => n (n-1)!
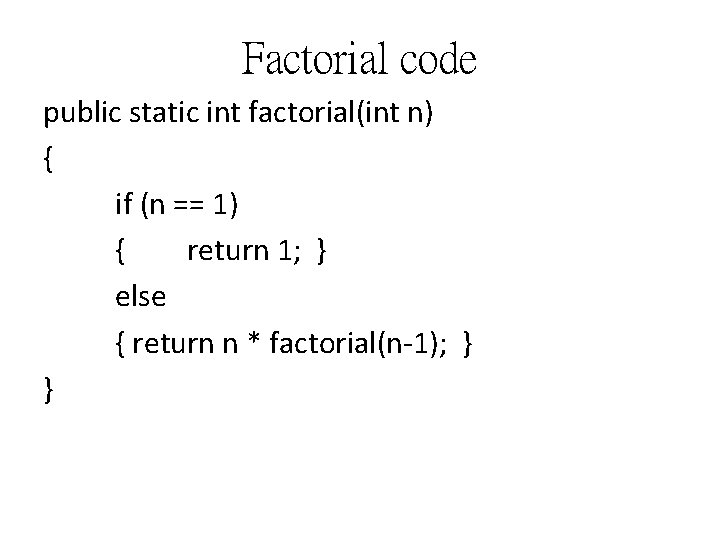
Factorial code public static int factorial(int n) { if (n == 1) { return 1; } else { return n * factorial(n-1); } }
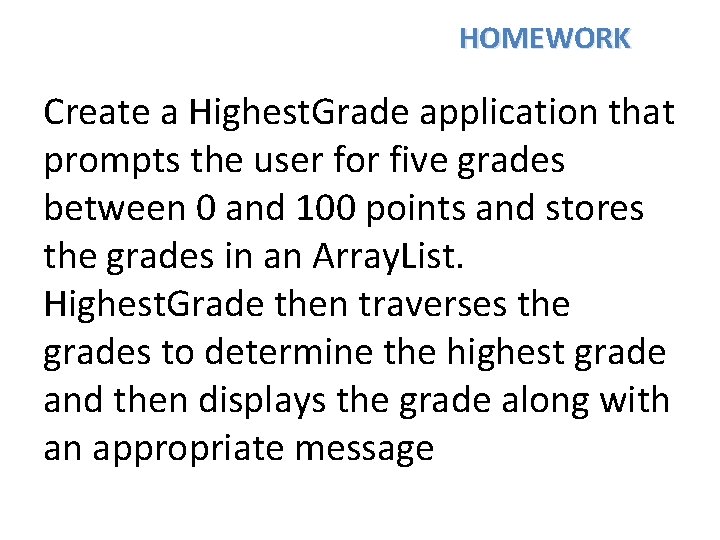
HOMEWORK Create a Highest. Grade application that prompts the user for five grades between 0 and 100 points and stores the grades in an Array. List. Highest. Grade then traverses the grades to determine the highest grade and then displays the grade along with an appropriate message