Advanced NET Programming I 3 rd Lecture http
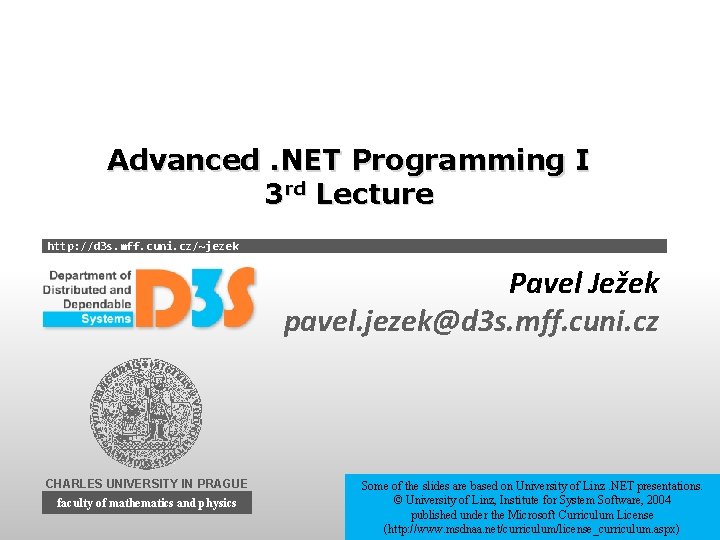
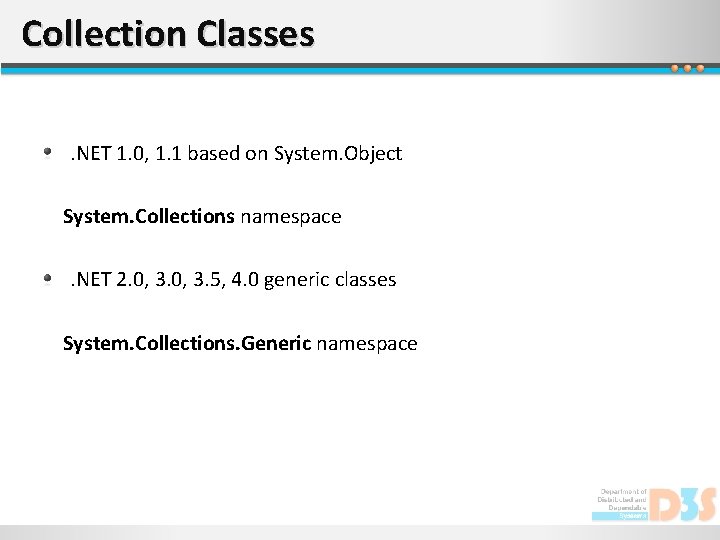
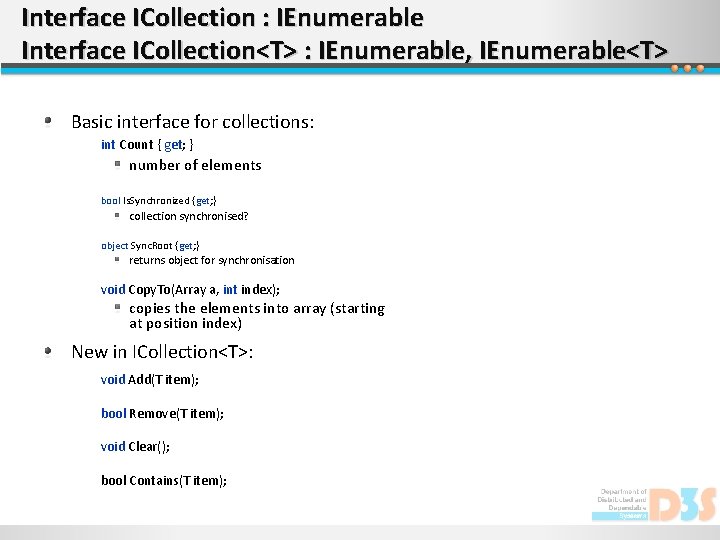
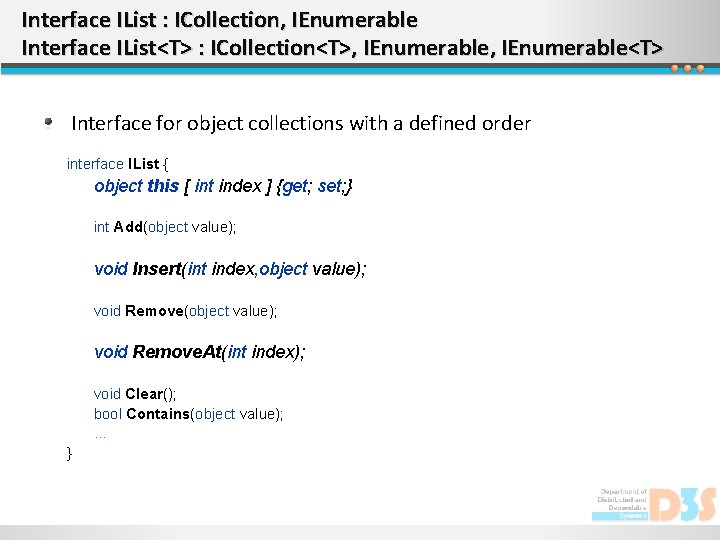
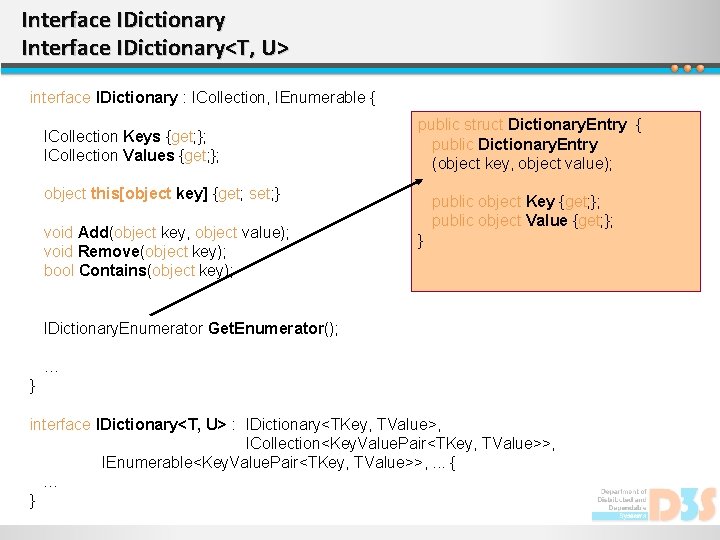
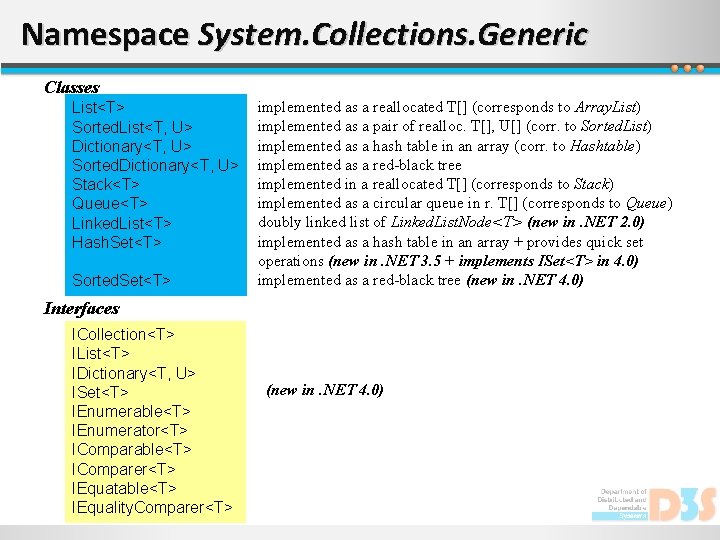
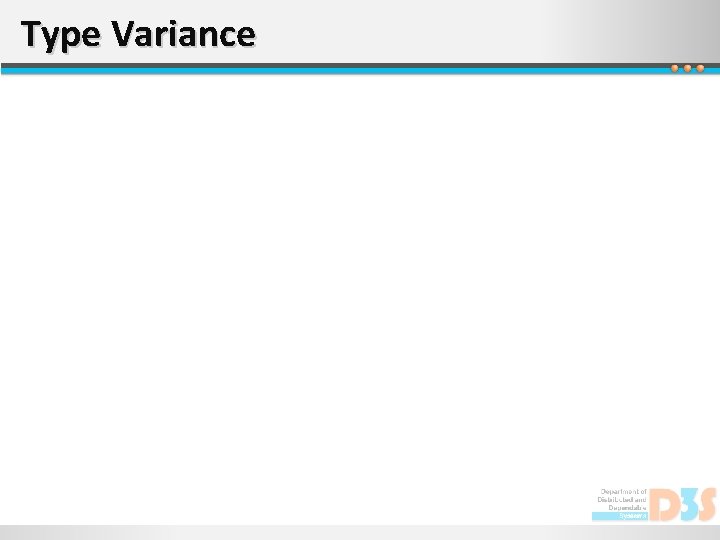
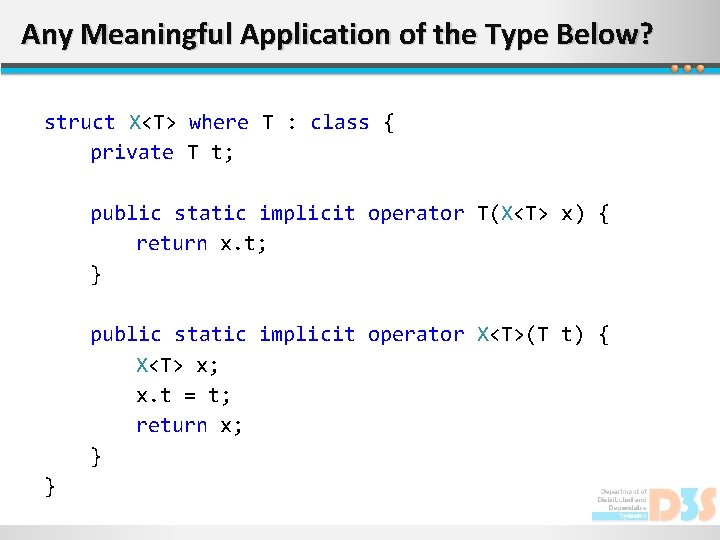
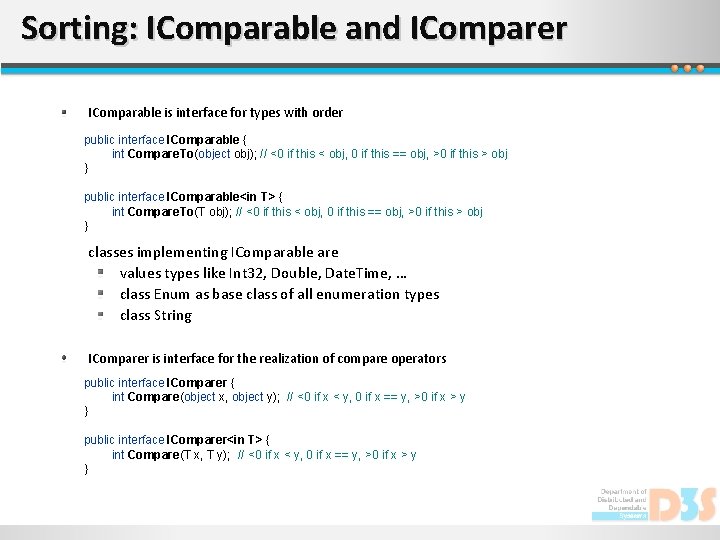
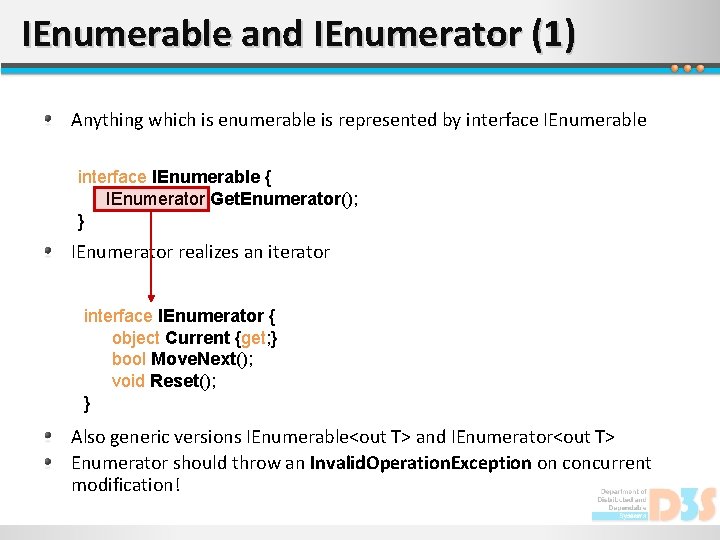
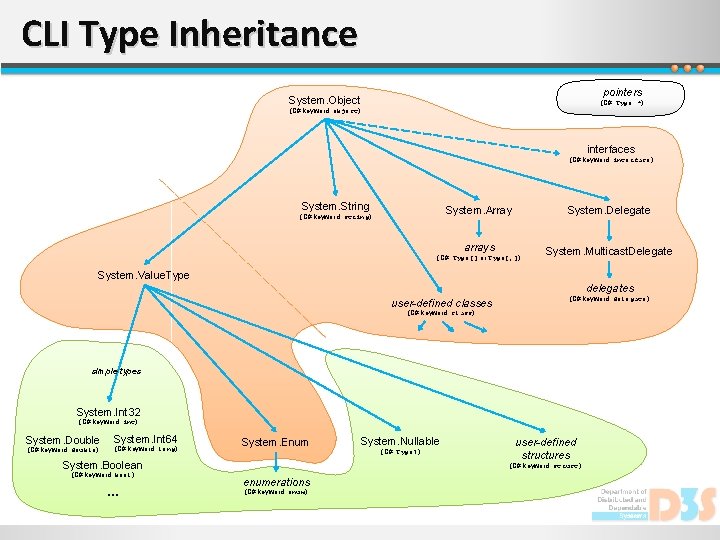
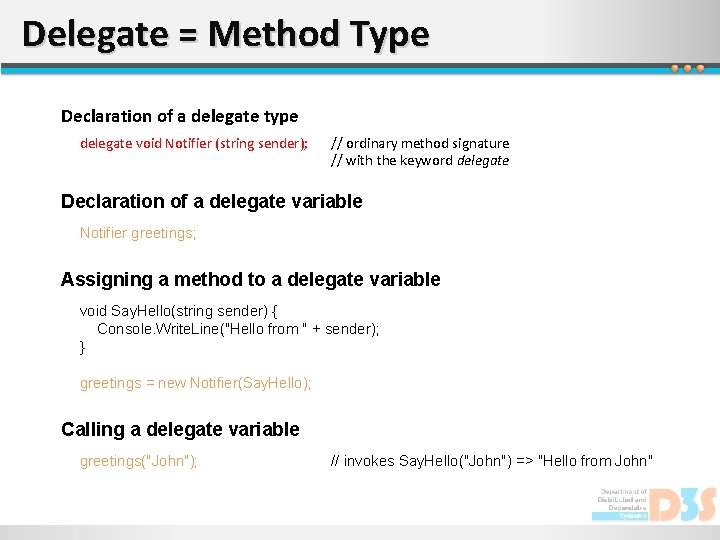
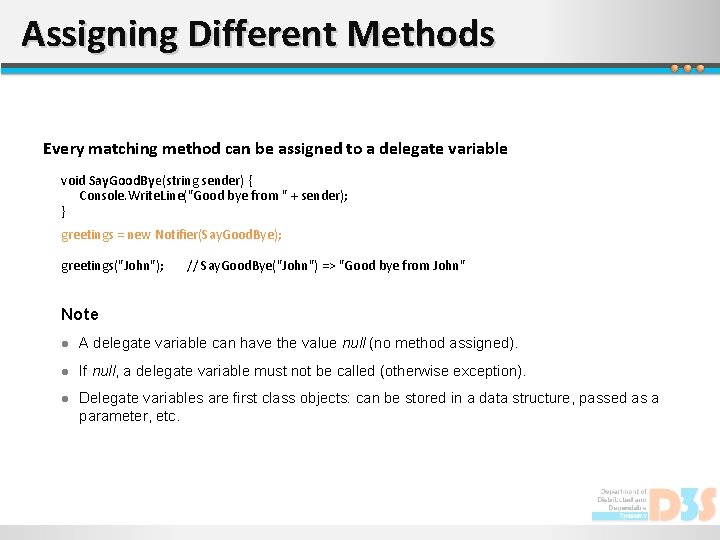
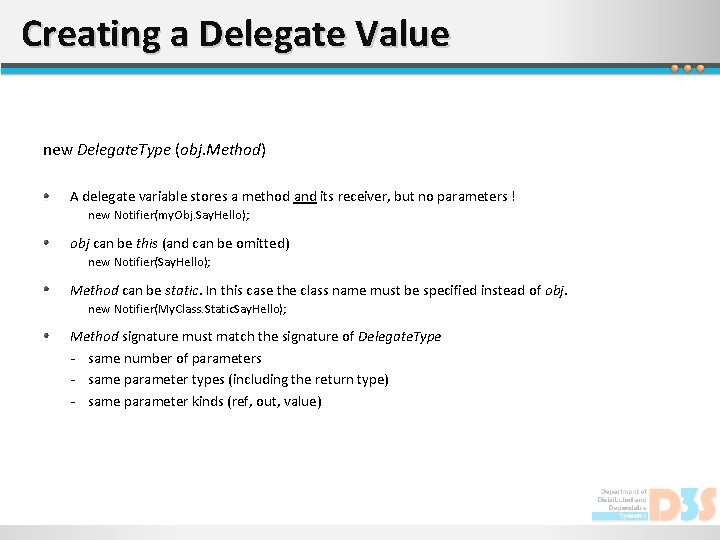
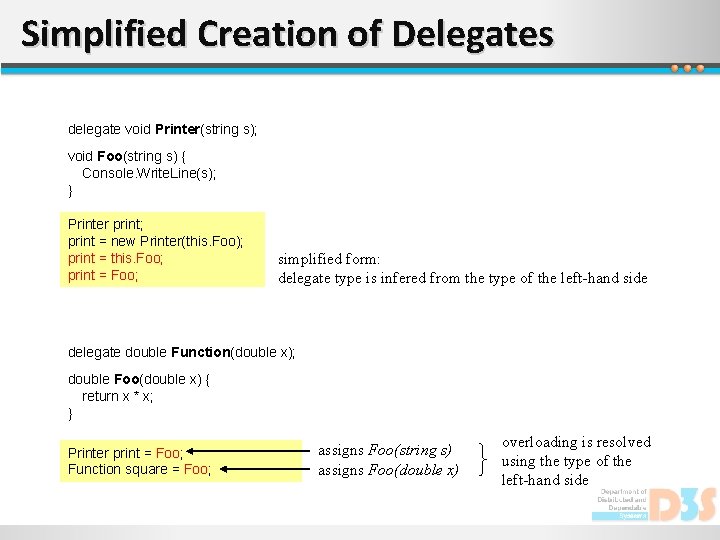
- Slides: 15
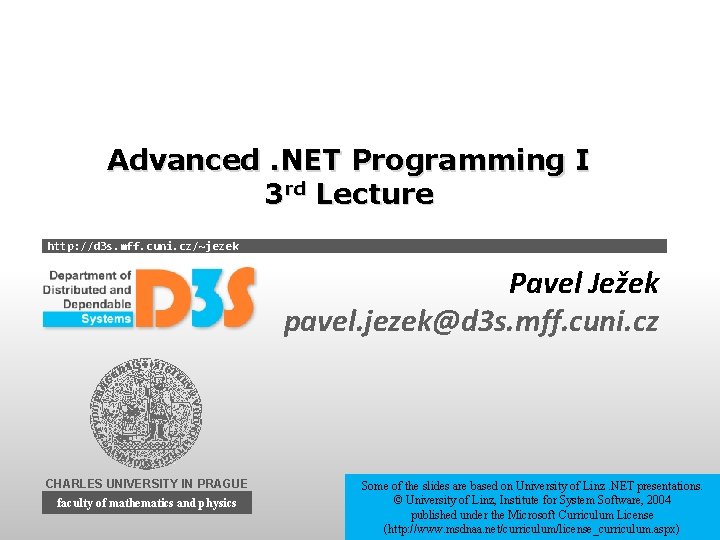
Advanced. NET Programming I 3 rd Lecture http: //d 3 s. mff. cuni. cz/~jezek Pavel Ježek pavel. jezek@d 3 s. mff. cuni. cz CHARLES UNIVERSITY IN PRAGUE faculty of mathematics and physics Some of the slides are based on University of Linz. NET presentations. © University of Linz, Institute for System Software, 2004 published under the Microsoft Curriculum License (http: //www. msdnaa. net/curriculum/license_curriculum. aspx)
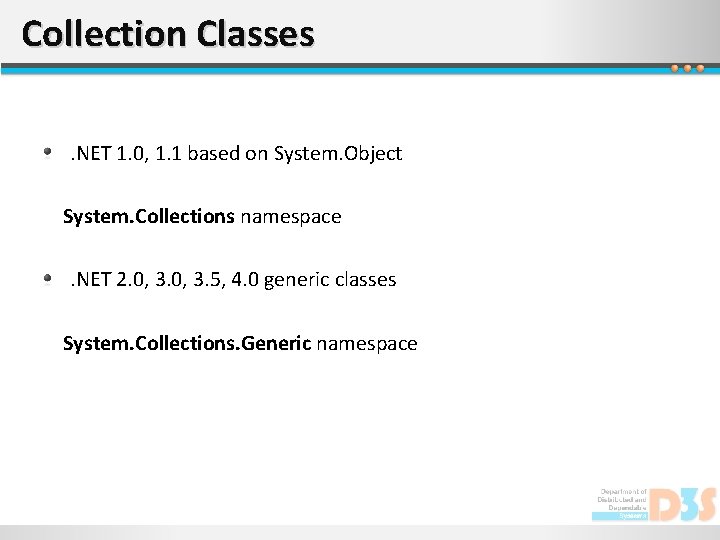
Collection Classes. NET 1. 0, 1. 1 based on System. Object System. Collections namespace. NET 2. 0, 3. 5, 4. 0 generic classes System. Collections. Generic namespace
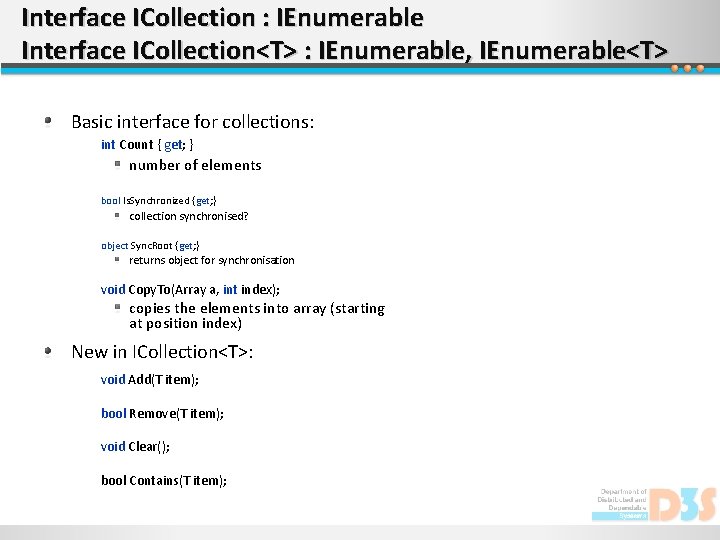
Interface ICollection : IEnumerable Interface ICollection<T> : IEnumerable, IEnumerable<T> Basic interface for collections: int Count { get; } number of elements bool Is. Synchronized {get; } collection synchronised? object Sync. Root {get; } returns object for synchronisation void Copy. To(Array a, int index); copies the elements into array (starting at position index) New in ICollection<T>: void Add(T item); bool Remove(T item); void Clear(); bool Contains(T item);
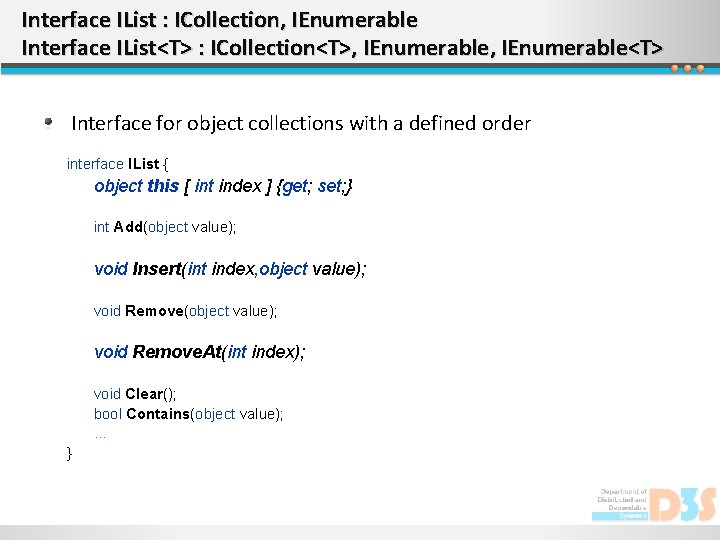
Interface IList : ICollection, IEnumerable Interface IList<T> : ICollection<T>, IEnumerable<T> Interface for object collections with a defined order interface IList { object this [ int index ] {get; set; } int Add(object value); void Insert(int index, object value); void Remove(object value); void Remove. At(int index); void Clear(); bool Contains(object value); … }
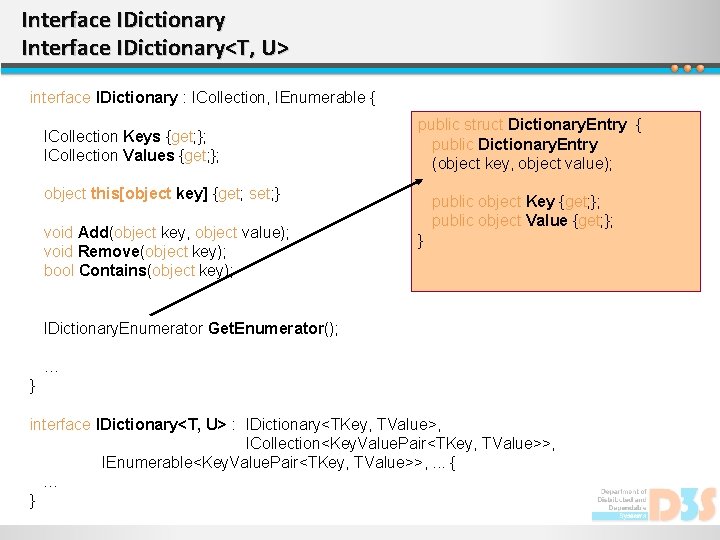
Interface IDictionary<T, U> interface IDictionary : ICollection, IEnumerable { ICollection Keys {get; }; ICollection Values {get; }; public struct Dictionary. Entry { public Dictionary. Entry (object key, object value); object this[object key] {get; set; } void Add(object key, object value); void Remove(object key); bool Contains(object key); public object Key {get; }; public object Value {get; }; } IDictionary. Enumerator Get. Enumerator(); … } interface IDictionary<T, U> : IDictionary<TKey, TValue>, ICollection<Key. Value. Pair<TKey, TValue>>, IEnumerable<Key. Value. Pair<TKey, TValue>>, . . . { … }
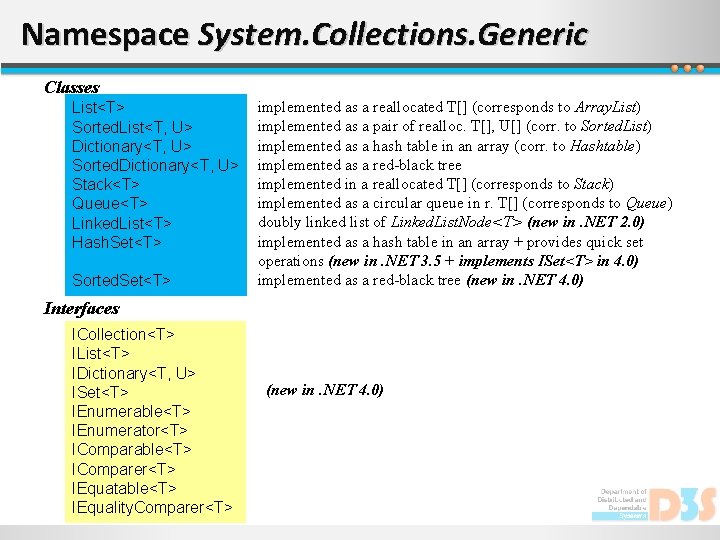
Namespace System. Collections. Generic Classes List<T> Sorted. List<T, U> Dictionary<T, U> Sorted. Dictionary<T, U> Stack<T> Queue<T> Linked. List<T> Hash. Set<T> Sorted. Set<T> implemented as a reallocated T[] (corresponds to Array. List) implemented as a pair of realloc. T[], U[] (corr. to Sorted. List) implemented as a hash table in an array (corr. to Hashtable) implemented as a red-black tree implemented in a reallocated T[] (corresponds to Stack) implemented as a circular queue in r. T[] (corresponds to Queue) doubly linked list of Linked. List. Node<T> (new in. NET 2. 0) implemented as a hash table in an array + provides quick set operations (new in. NET 3. 5 + implements ISet<T> in 4. 0) implemented as a red-black tree (new in. NET 4. 0) Interfaces ICollection<T> IList<T> IDictionary<T, U> ISet<T> IEnumerable<T> IEnumerator<T> IComparable<T> IComparer<T> IEquatable<T> IEquality. Comparer<T> (new in. NET 4. 0)
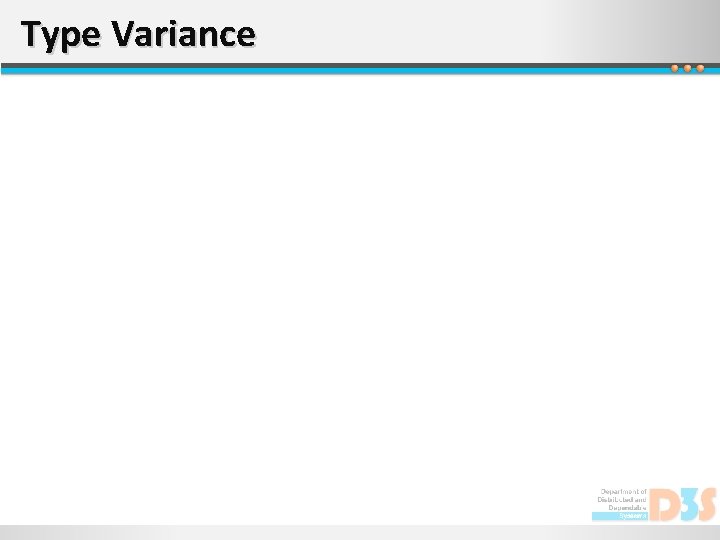
Type Variance
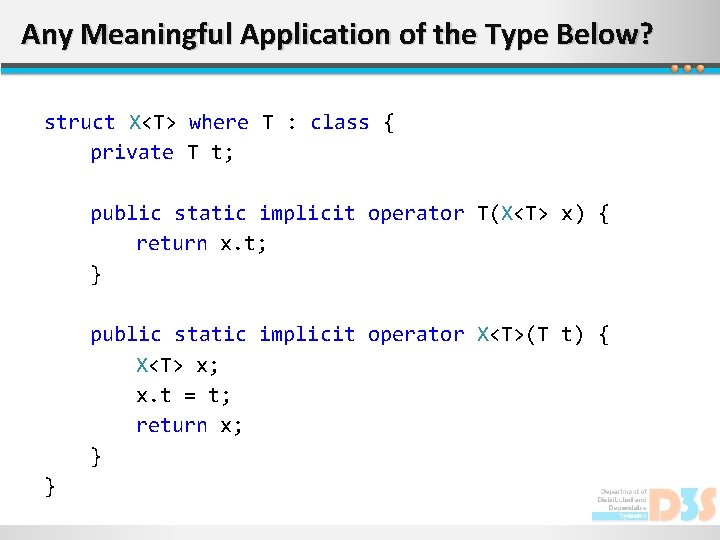
Any Meaningful Application of the Type Below? struct X<T> where T : class { private T t; public static implicit operator T(X<T> x) { return x. t; } public static implicit operator X<T>(T t) { X<T> x; x. t = t; return x; } }
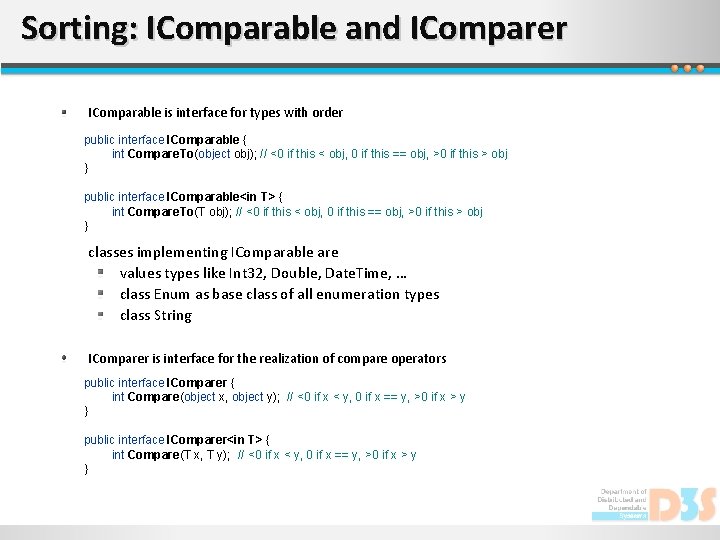
Sorting: IComparable and IComparer IComparable is interface for types with order public interface IComparable { int Compare. To(object obj); // <0 if this < obj, 0 if this == obj, >0 if this > obj } public interface IComparable<in T> { int Compare. To(T obj); // <0 if this < obj, 0 if this == obj, >0 if this > obj } classes implementing IComparable are values types like Int 32, Double, Date. Time, … class Enum as base class of all enumeration types class String IComparer is interface for the realization of compare operators public interface IComparer { int Compare(object x, object y); // <0 if x < y, 0 if x == y, >0 if x > y } public interface IComparer<in T> { int Compare(T x, T y); // <0 if x < y, 0 if x == y, >0 if x > y }
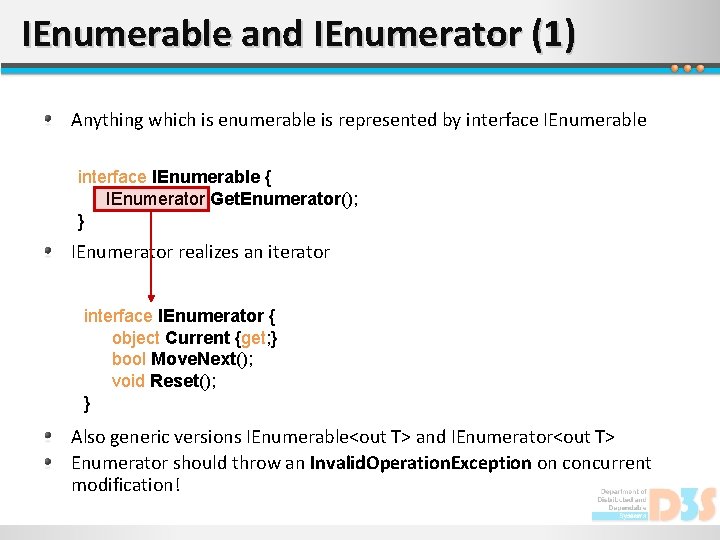
IEnumerable and IEnumerator (1) Anything which is enumerable is represented by interface IEnumerable { IEnumerator Get. Enumerator(); } IEnumerator realizes an iterator interface IEnumerator { object Current {get; } bool Move. Next(); void Reset(); } Also generic versions IEnumerable<out T> and IEnumerator<out T> Enumerator should throw an Invalid. Operation. Exception on concurrent modification!
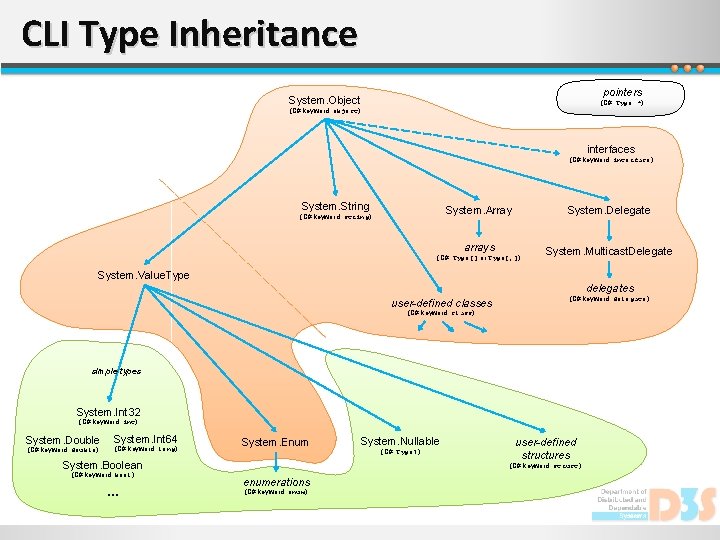
CLI Type Inheritance pointers System. Object (C#: Type *) (C# keyword: object) interfaces (C# keyword: interface) System. String (C# keyword: string) System. Array System. Delegate arrays System. Multicast. Delegate (C#: Type[] or Type[, ]) System. Value. Type delegates user-defined classes (C# keyword: delegate) (C# keyword: class) simple types System. Int 32 (C# keyword: int) System. Double (C# keyword: double) System. Int 64 (C# keyword: long) System. Enum System. Boolean (C# keyword: bool) … System. Nullable (C#: Type? ) user-defined structures (C# keyword: struct) enumerations (C# keyword: enum)
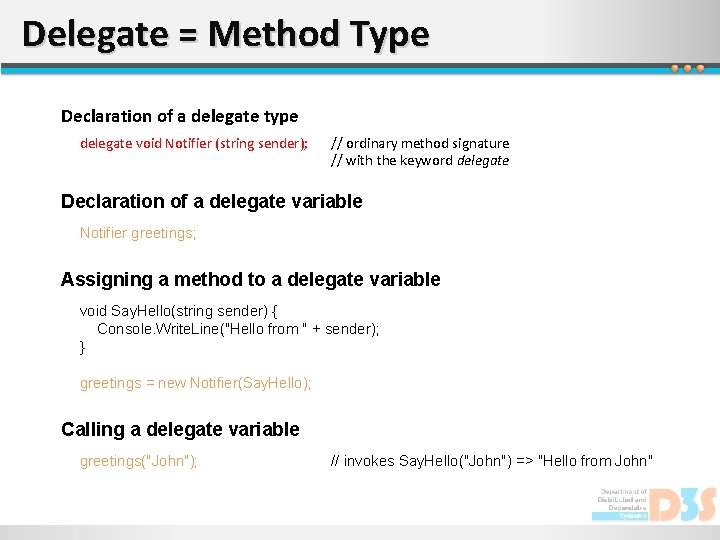
Delegate = Method Type Declaration of a delegate type delegate void Notifier (string sender); // ordinary method signature // with the keyword delegate Declaration of a delegate variable Notifier greetings; Assigning a method to a delegate variable void Say. Hello(string sender) { Console. Write. Line("Hello from " + sender); } greetings = new Notifier(Say. Hello); Calling a delegate variable greetings("John"); // invokes Say. Hello("John") => "Hello from John"
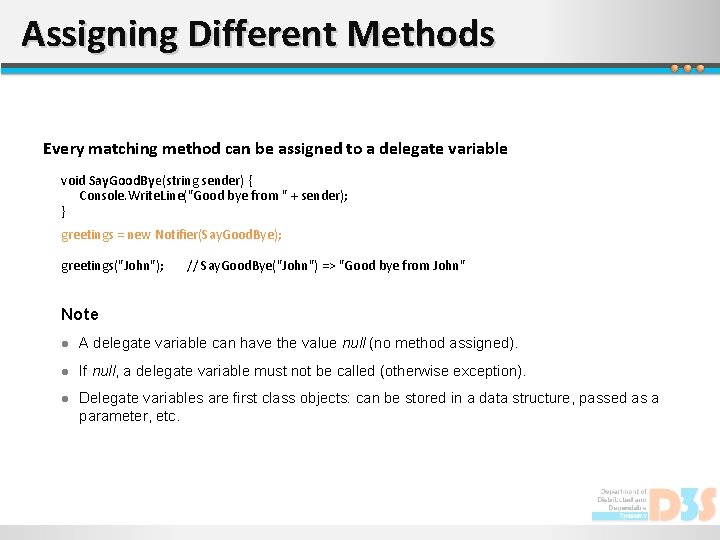
Assigning Different Methods Every matching method can be assigned to a delegate variable void Say. Good. Bye(string sender) { Console. Write. Line("Good bye from " + sender); } greetings = new Notifier(Say. Good. Bye); greetings("John"); // Say. Good. Bye("John") => "Good bye from John" Note l A delegate variable can have the value null (no method assigned). l If null, a delegate variable must not be called (otherwise exception). l Delegate variables are first class objects: can be stored in a data structure, passed as a parameter, etc.
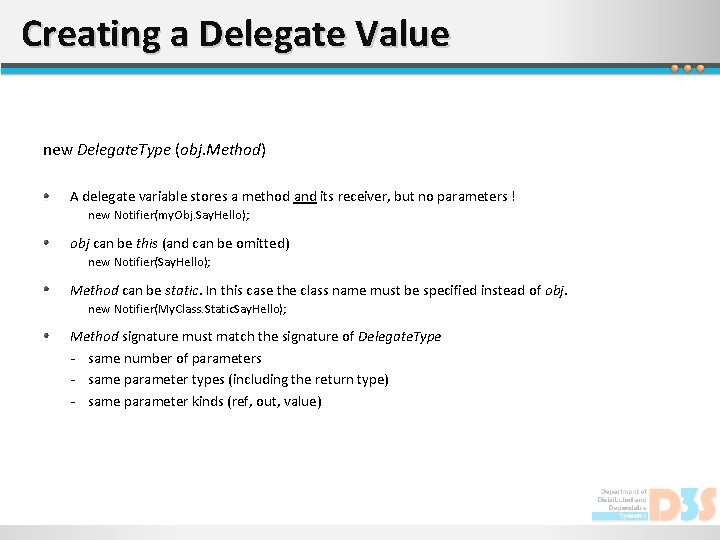
Creating a Delegate Value new Delegate. Type (obj. Method) A delegate variable stores a method and its receiver, but no parameters ! new Notifier(my. Obj. Say. Hello); obj can be this (and can be omitted) new Notifier(Say. Hello); Method can be static. In this case the class name must be specified instead of obj. new Notifier(My. Class. Static. Say. Hello); Method signature must match the signature of Delegate. Type - same number of parameters - same parameter types (including the return type) - same parameter kinds (ref, out, value)
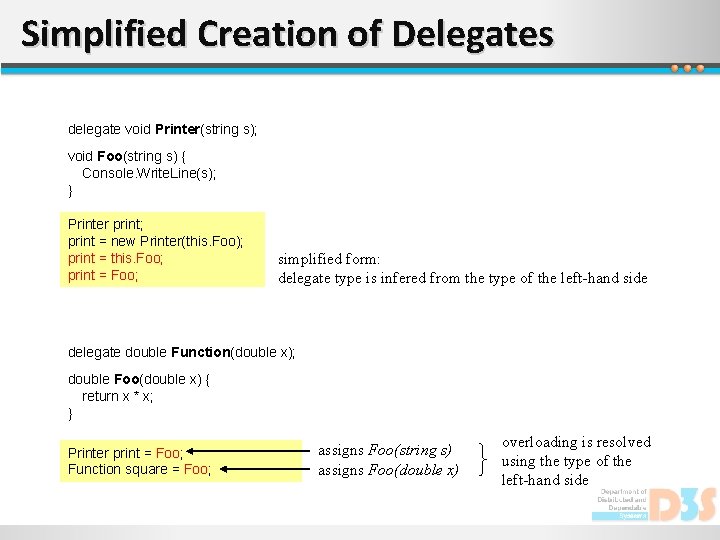
Simplified Creation of Delegates delegate void Printer(string s); void Foo(string s) { Console. Write. Line(s); } Printer print; print = new Printer(this. Foo); print = this. Foo; print = Foo; simplified form: delegate type is infered from the type of the left-hand side delegate double Function(double x); double Foo(double x) { return x * x; } Printer print = Foo; Function square = Foo; assigns Foo(string s) assigns Foo(double x) overloading is resolved using the type of the left-hand side
01:640:244 lecture notes - lecture 15: plat, idah, farad
Advanced inorganic chemistry lecture notes
C programming lecture
Advanced dynamic programming
Advanced internet programming
Assembly process in system programming
For imperative statement 2 pass assembler
Advanced programming in java
Perbedaan linear programming dan integer programming
Greedy vs dynamic
What is system programing
Linear vs integer programming
Definisi linear
Http //mbs.meb.gov.tr/ http //www.alantercihleri.com
Siat.ung.ac.id
Reactive programming for net developers