Advanced Computer Programming Lists Tuples and Dictionaries Sinan
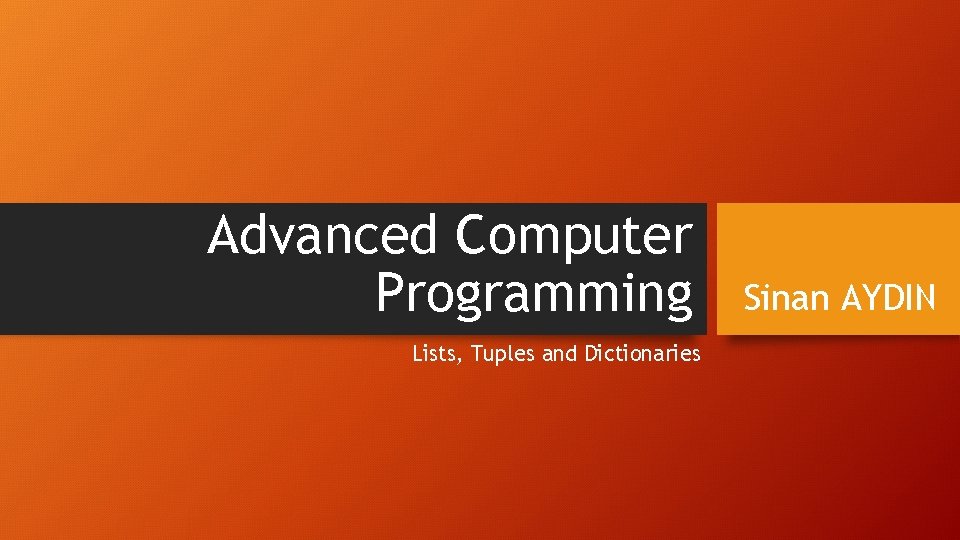
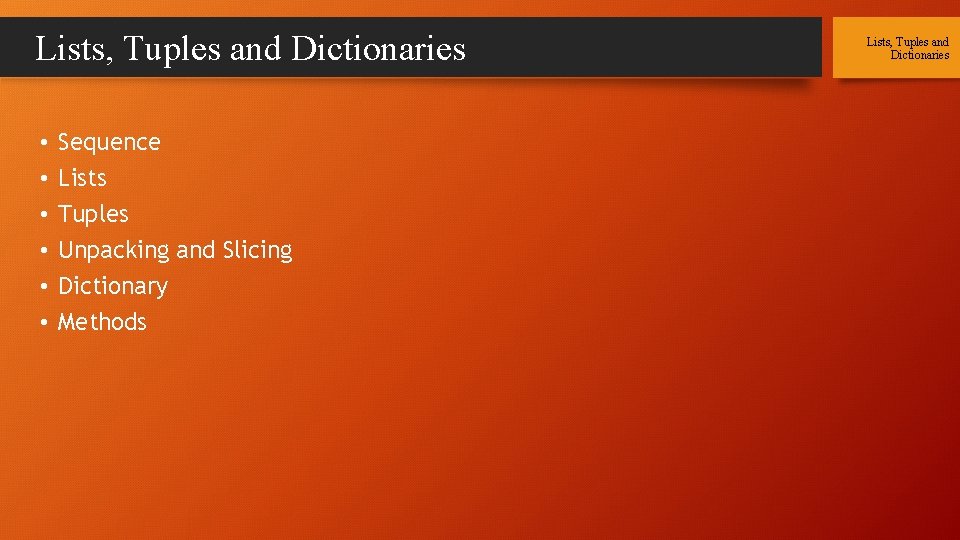
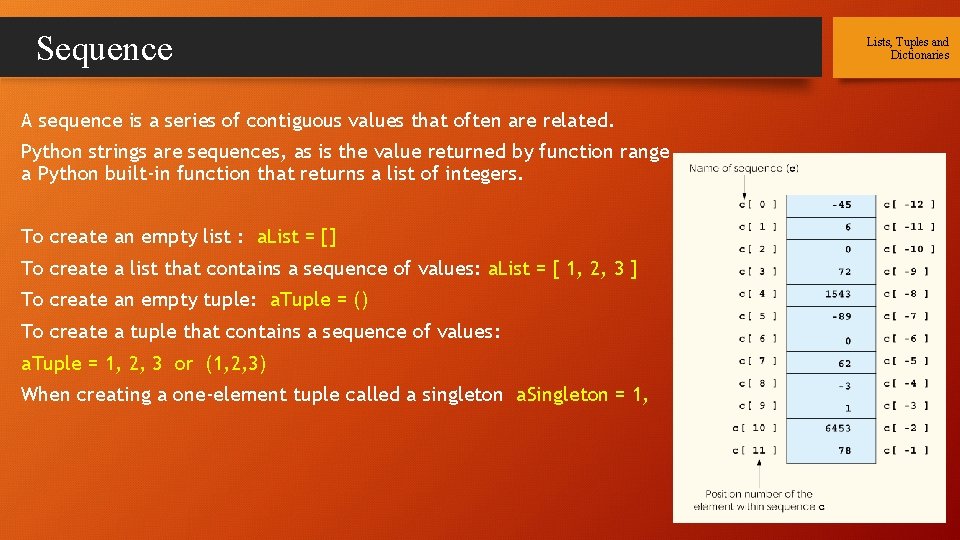
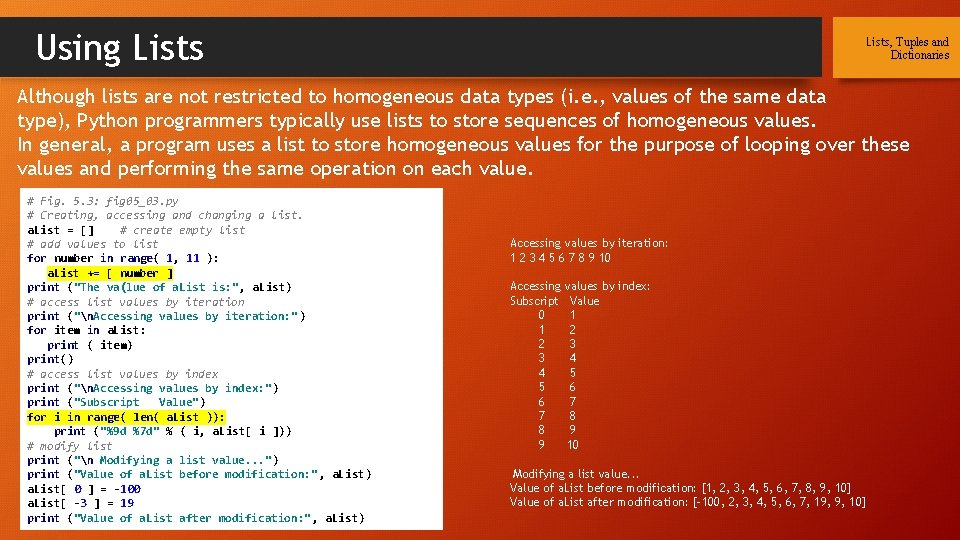
![Using Lists values = [] # a list of values # input 10 values Using Lists values = [] # a list of values # input 10 values](https://slidetodoc.com/presentation_image_h2/b6a1ed01f3181f1908a993cf66a67cb2/image-5.jpg)
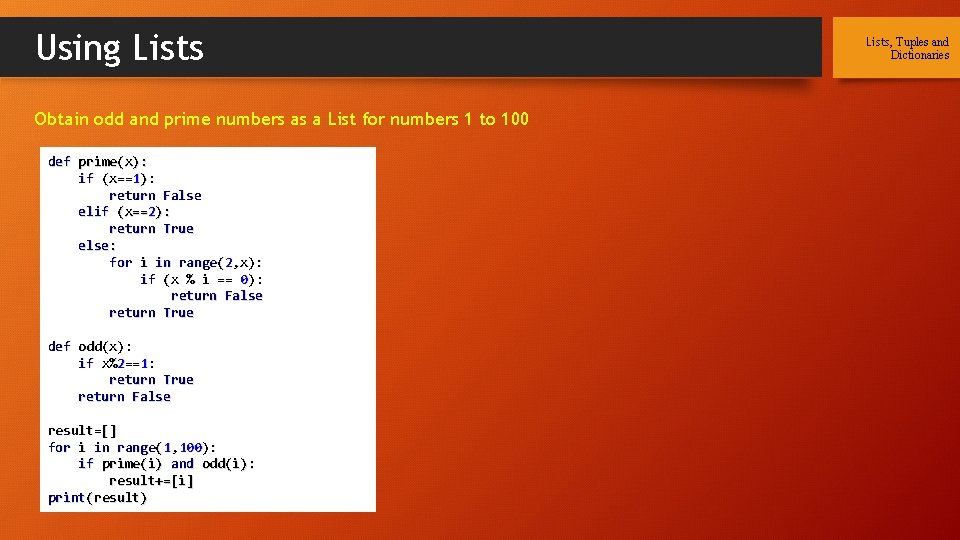
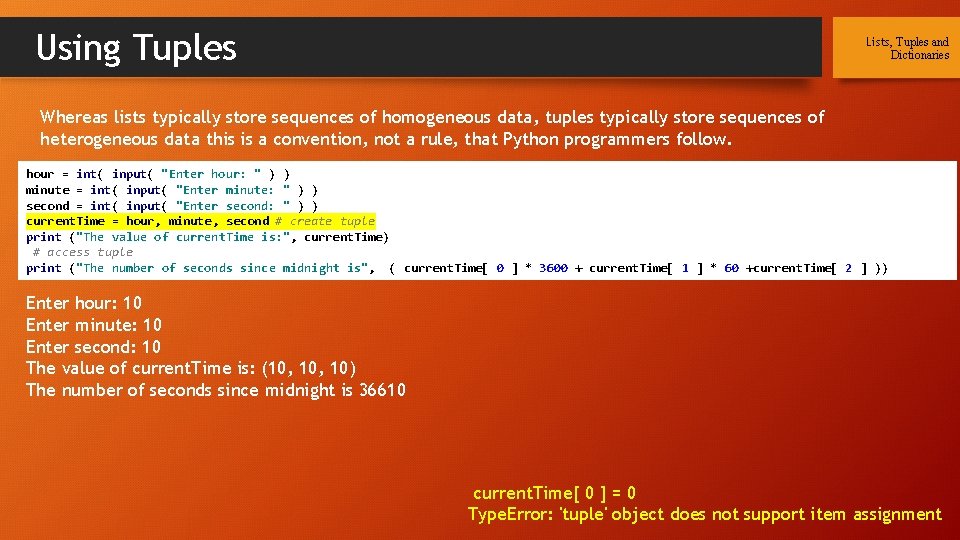
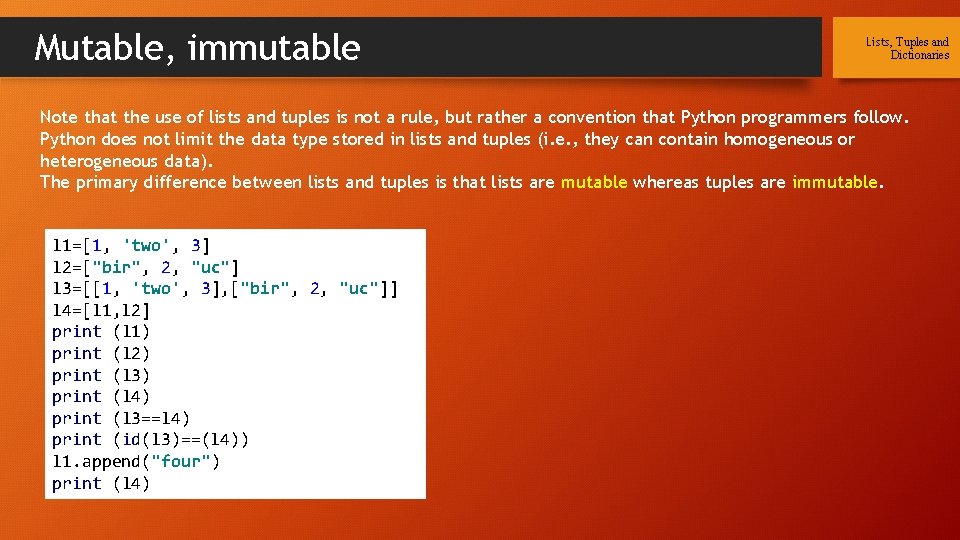
![Sequence Unpacking a. String = "abc" a. List = [ 1, 2, 3 ] Sequence Unpacking a. String = "abc" a. List = [ 1, 2, 3 ]](https://slidetodoc.com/presentation_image_h2/b6a1ed01f3181f1908a993cf66a67cb2/image-9.jpg)
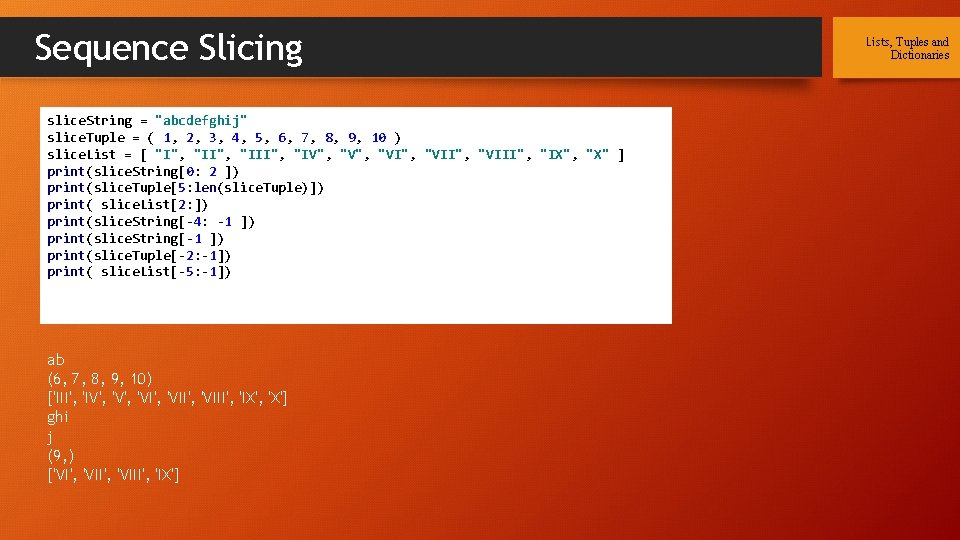
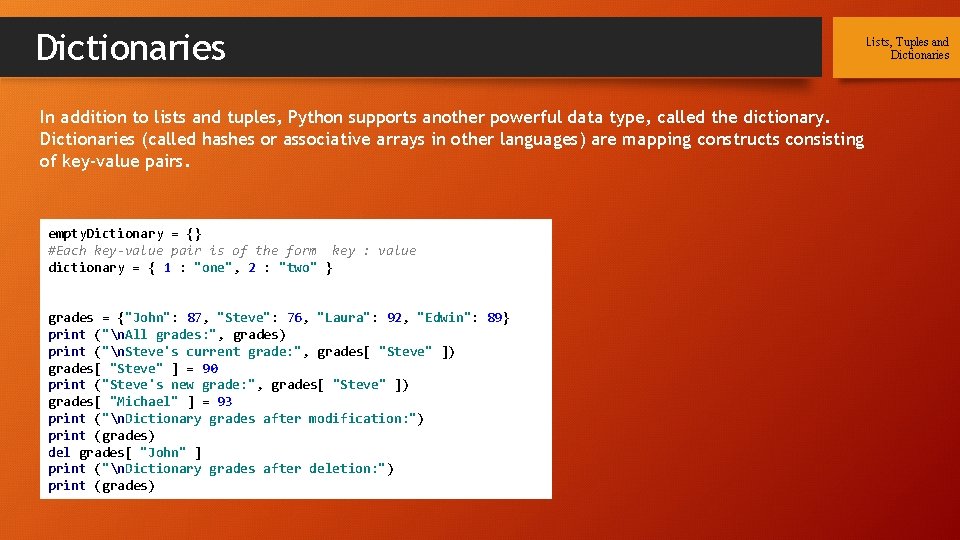
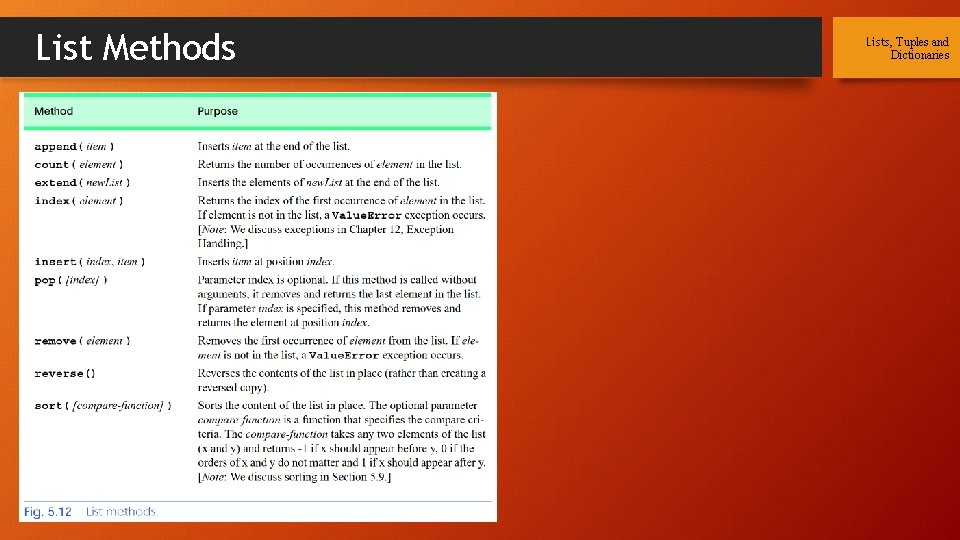
![List and Dictionary Methods --Mylist-[1, 2, 3, 4, 5, 2, 4, 6, 8] --Mylist. List and Dictionary Methods --Mylist-[1, 2, 3, 4, 5, 2, 4, 6, 8] --Mylist.](https://slidetodoc.com/presentation_image_h2/b6a1ed01f3181f1908a993cf66a67cb2/image-13.jpg)
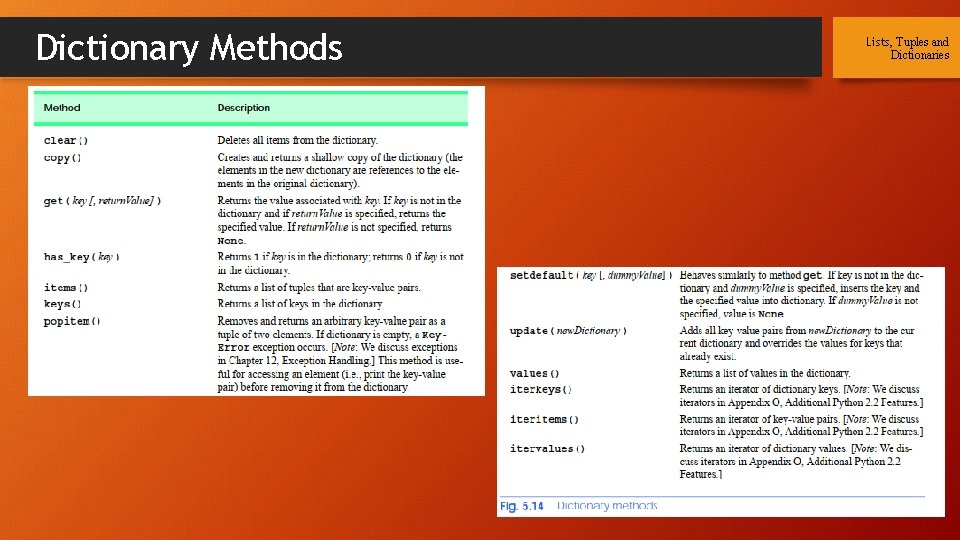
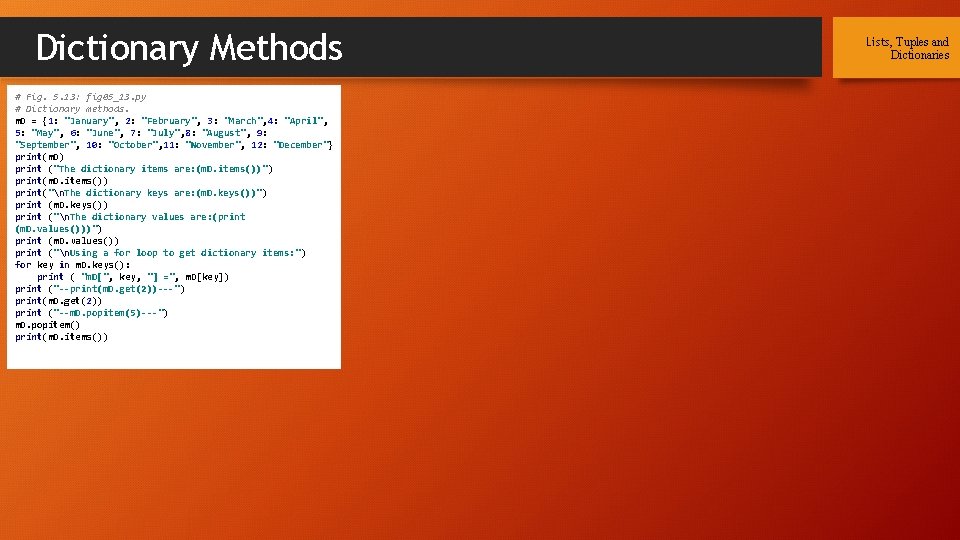
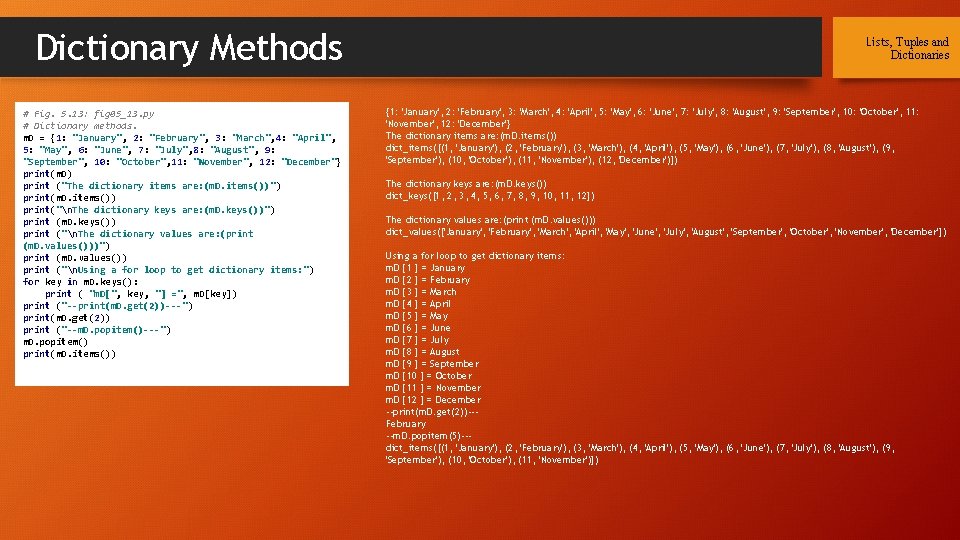
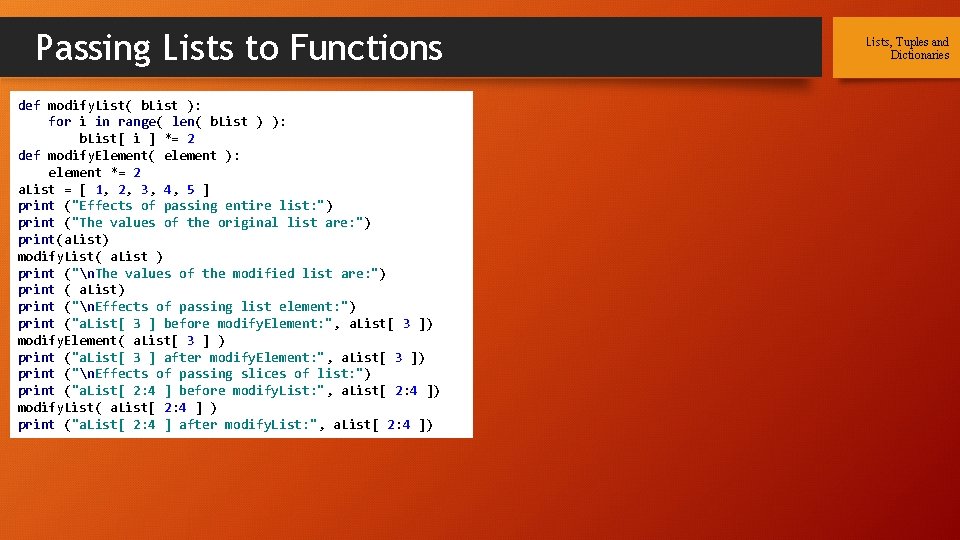
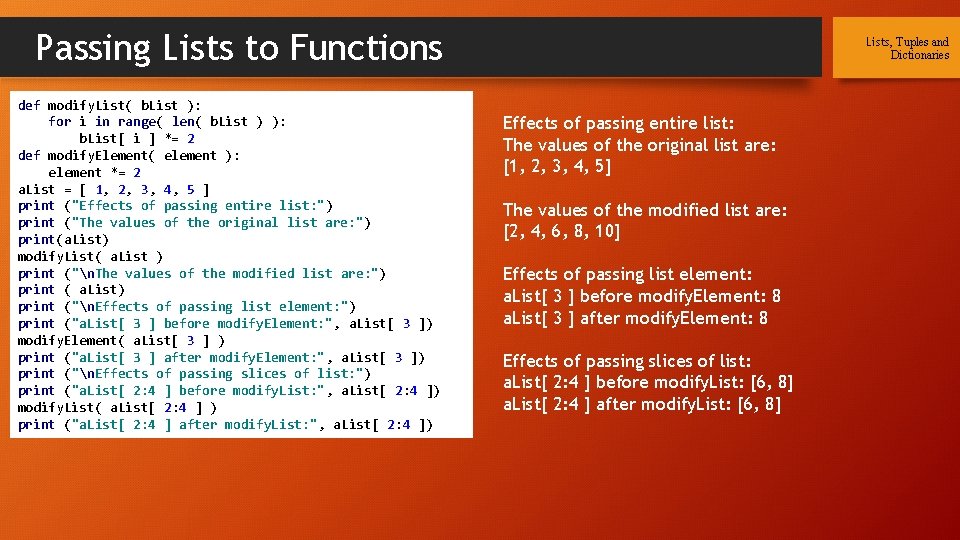
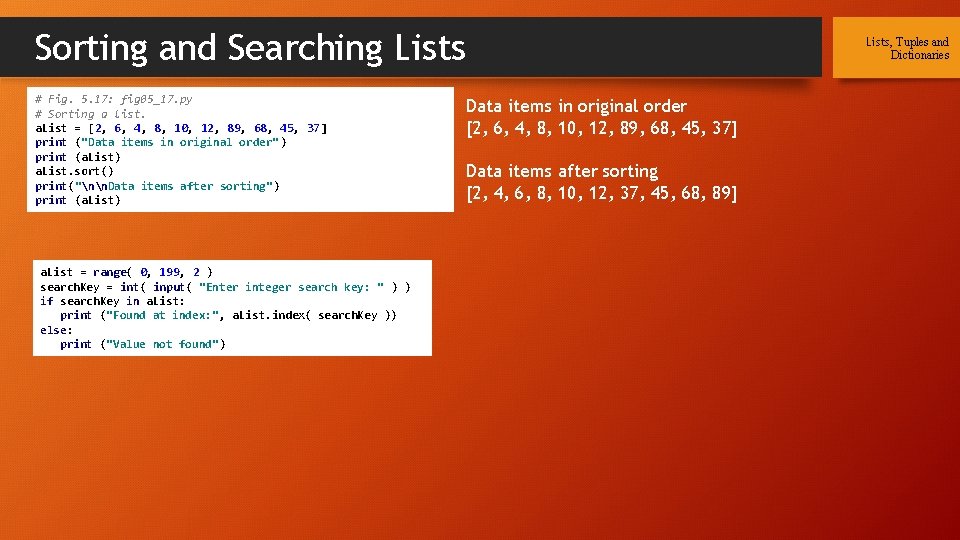
![Multiple-Subscripted Sequences b = [ [ 1, 2 ], [ 3, 4 ] ] Multiple-Subscripted Sequences b = [ [ 1, 2 ], [ 3, 4 ] ]](https://slidetodoc.com/presentation_image_h2/b6a1ed01f3181f1908a993cf66a67cb2/image-20.jpg)
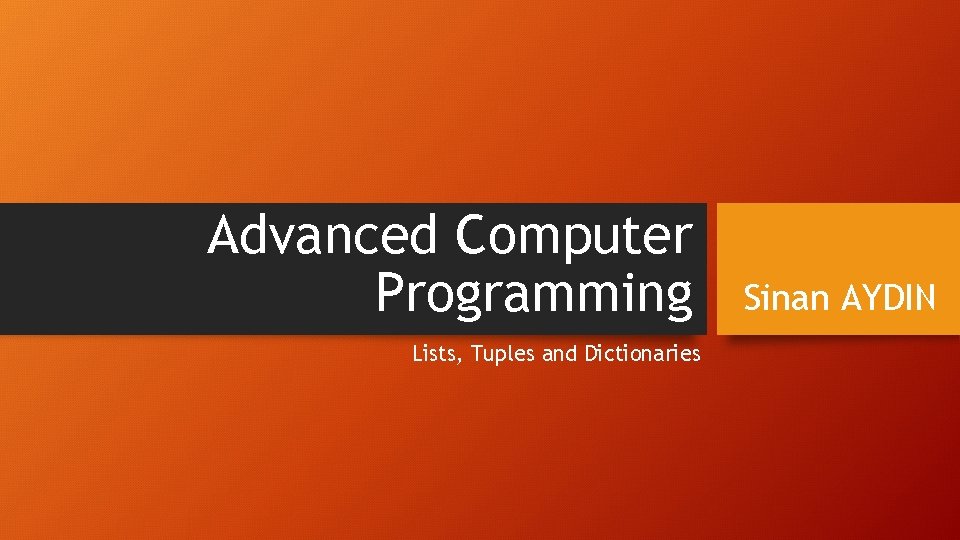
- Slides: 21
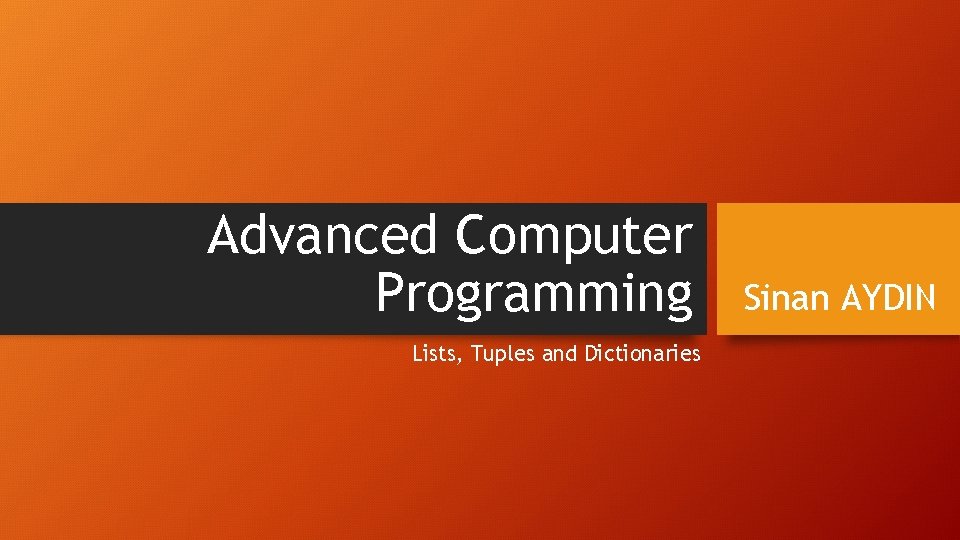
Advanced Computer Programming Lists, Tuples and Dictionaries Sinan AYDIN
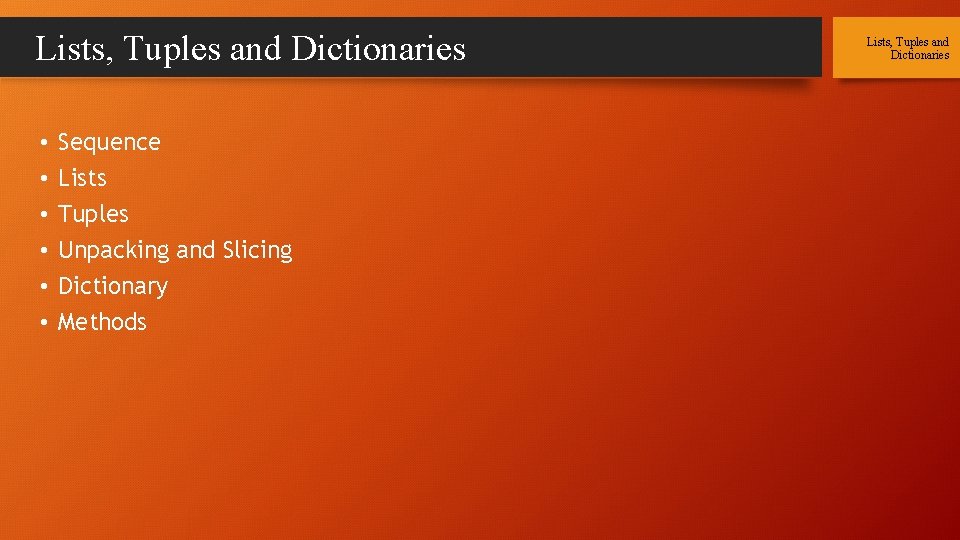
Lists, Tuples and Dictionaries • • • Sequence Lists Tuples Unpacking and Slicing Dictionary Methods Lists, Tuples and Dictionaries
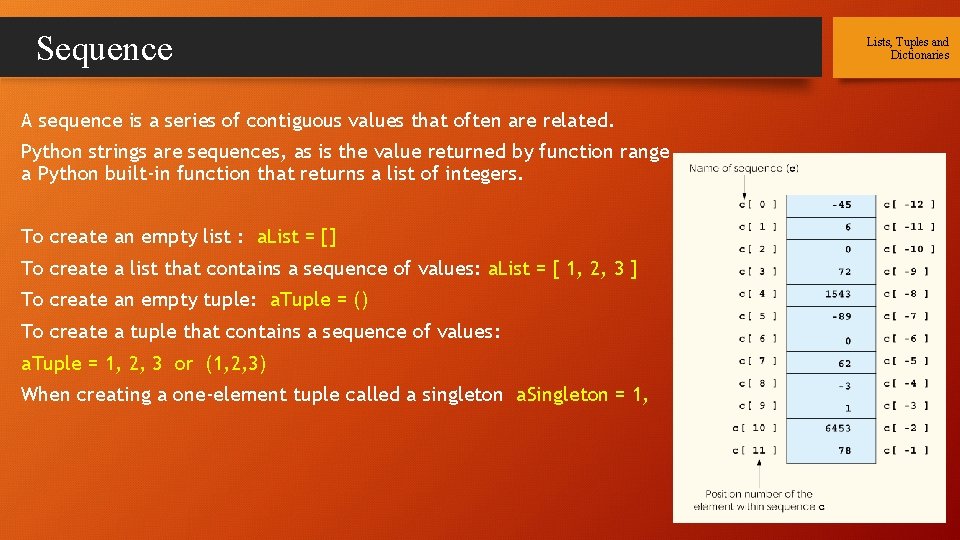
Sequence A sequence is a series of contiguous values that often are related. Python strings are sequences, as is the value returned by function range a Python built-in function that returns a list of integers. To create an empty list : a. List = [] To create a list that contains a sequence of values: a. List = [ 1, 2, 3 ] To create an empty tuple: a. Tuple = () To create a tuple that contains a sequence of values: a. Tuple = 1, 2, 3 or (1, 2, 3) When creating a one-element tuple called a singleton a. Singleton = 1, Lists, Tuples and Dictionaries
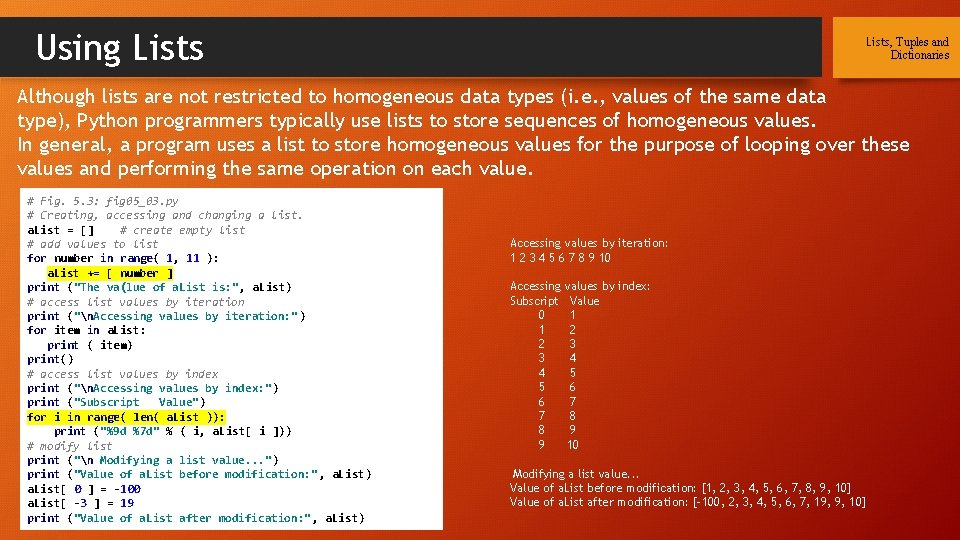
Using Lists, Tuples and Dictionaries Although lists are not restricted to homogeneous data types (i. e. , values of the same data type), Python programmers typically use lists to store sequences of homogeneous values. In general, a program uses a list to store homogeneous values for the purpose of looping over these values and performing the same operation on each value. # Fig. 5. 3: fig 05_03. py # Creating, accessing and changing a list. a. List = [] # create empty list # add values to list for number in range( 1, 11 ): a. List += [ number ] print ("The va(lue of a. List is: ", a. List) # access list values by iteration print ("n. Accessing values by iteration: ") for item in a. List: print ( item) print() # access list values by index print ("n. Accessing values by index: ") print ("Subscript Value") for i in range( len( a. List )): print ("%9 d %7 d" % ( i, a. List[ i ])) # modify list print ("n Modifying a list value. . . ") print ("Value of a. List before modification: " , a. List) a. List[ 0 ] = -100 a. List[ -3 ] = 19 print ("Value of a. List after modification: " , a. List) Accessing values by iteration: 1 2 3 4 5 6 7 8 9 10 Accessing Subscript 0 1 2 3 4 5 6 7 8 9 values by index: Value 1 2 3 4 5 6 7 8 9 10 Modifying a list value. . . Value of a. List before modification: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] Value of a. List after modification: [-100, 2, 3, 4, 5, 6, 7, 19, 9, 10]
![Using Lists values a list of values input 10 values Using Lists values = [] # a list of values # input 10 values](https://slidetodoc.com/presentation_image_h2/b6a1ed01f3181f1908a993cf66a67cb2/image-5.jpg)
Using Lists values = [] # a list of values # input 10 values from user print ("Enter 10 integers: ") for i in range( 10 ): new. Value = int( input( "Enter integer %d: " % ( i + 1 ) ) ) values += [ new. Value ] print ("n. Creating a histogram from values: " ) print ("%s %10 s" % ( "Element", "Value", "Histogram" )) for i in range( len( values ) ): print ("%7 d %10 d %s" % ( i, values[ i ], "*" * values[ i ] )) Obtain odd and prime numbers as a List for numbers 1 to 100 Lists, Tuples and Dictionaries Enter Enter Enter 10 integers: integer 1: 10 integer 2: 8 integer 3: 6 integer 4: 4 integer 5: 2 integer 6: 9 integer 7: 7 integer 8: 5 integer 9: 3 integer 10: 1 Creating a histogram from values: Element Value Histogram 0 10 ***** 1 8 **** 2 6 ****** 3 4 **** 4 2 ** 5 9 ***** 6 7 ******* 7 5 ***** 8 3 *** 9 1 *
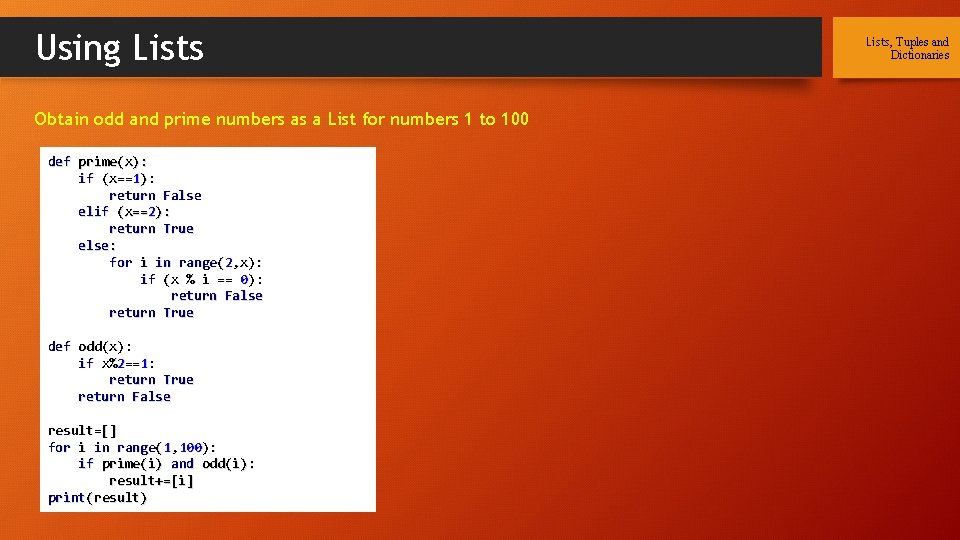
Using Lists Obtain odd and prime numbers as a List for numbers 1 to 100 def prime(x): if (x==1): return False elif (x==2): return True else: for i in range(2, x): if (x % i == 0): return False return True def odd(x): if x%2==1: return True return False result=[] for i in range(1, 100): if prime(i) and odd(i): result+=[i] print(result) Lists, Tuples and Dictionaries
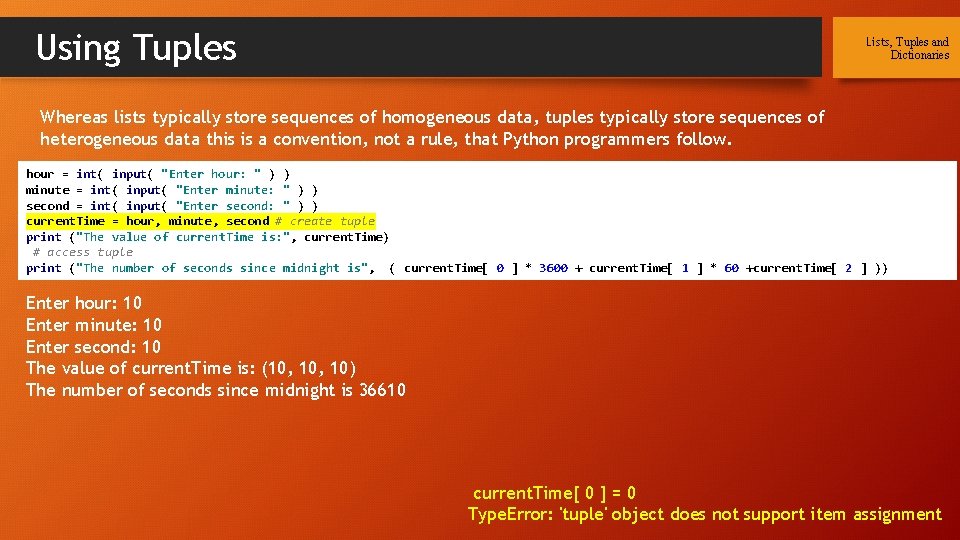
Using Tuples Lists, Tuples and Dictionaries Whereas lists typically store sequences of homogeneous data, tuples typically store sequences of heterogeneous data this is a convention, not a rule, that Python programmers follow. hour = int( input( "Enter hour: " ) ) minute = int( input( "Enter minute: " ) ) second = int( input( "Enter second: " ) ) current. Time = hour, minute, second # create tuple print ("The value of current. Time is: ", current. Time) # access tuple print ("The number of seconds since midnight is", ( current. Time[ 0 ] * 3600 + current. Time[ 1 ] * 60 +current. Time[ 2 ] )) Enter hour: 10 Enter minute: 10 Enter second: 10 The value of current. Time is: (10, 10) The number of seconds since midnight is 36610 current. Time[ 0 ] = 0 Type. Error: 'tuple' object does not support item assignment
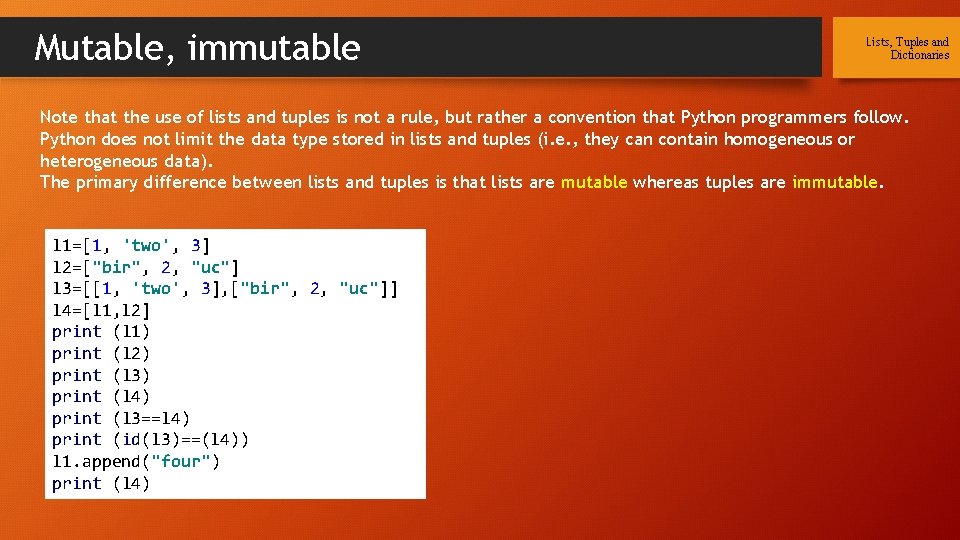
Mutable, immutable Lists, Tuples and Dictionaries Note that the use of lists and tuples is not a rule, but rather a convention that Python programmers follow. Python does not limit the data type stored in lists and tuples (i. e. , they can contain homogeneous or heterogeneous data). The primary difference between lists and tuples is that lists are mutable whereas tuples are immutable. l 1=[1, 'two', 3] l 2=["bir", 2, "uc"] l 3=[[1, 'two', 3], ["bir", 2, "uc"]] l 4=[l 1, l 2] print (l 1) print (l 2) print (l 3) print (l 4) print (l 3==l 4) print (id(l 3)==(l 4)) l 1. append("four") print (l 4)
![Sequence Unpacking a String abc a List 1 2 3 Sequence Unpacking a. String = "abc" a. List = [ 1, 2, 3 ]](https://slidetodoc.com/presentation_image_h2/b6a1ed01f3181f1908a993cf66a67cb2/image-9.jpg)
Sequence Unpacking a. String = "abc" a. List = [ 1, 2, 3 ] a. Tuple = "a", "A", 1 print ("Unpacking string. . . ") first, second, third = a. String print ("String values: ", first, second, third) print ("Unpacking list. . . ") first, second, third = a. List print ("List values: ", first, second, third) print ("Unpacking tuple. . . ") first, second, third = a. Tuple print ("Tuple values: ", first, second, third) # swapping two values x = 3 y = 4 print ("Before swapping: x = %d, y = %d" % ( x, y )) x, y = y, x # swap variables print ("After swapping: x = %d, y = %d" % ( x, y )) Lists, Tuples and Dictionaries Unpacking string. . . String values: a b c Unpacking list. . . List values: 1 2 3 Unpacking tuple. . . Tuple values: a A 1 Before swapping: x = 3, y = 4 After swapping: x = 4, y = 3
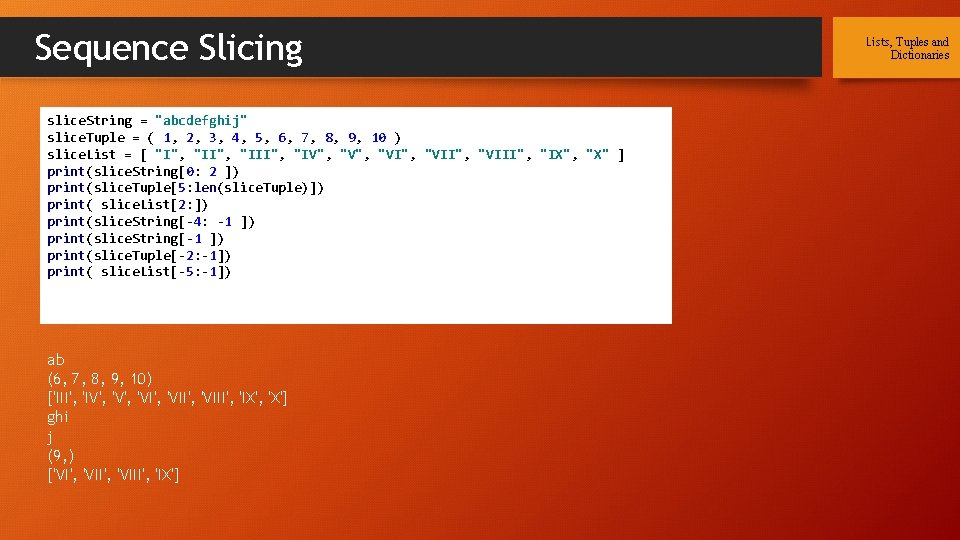
Sequence Slicing slice. String = "abcdefghij" slice. Tuple = ( 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 ) slice. List = [ "I", "III", "IV", "VI", "VIII", "IX", "X" ] print(slice. String[0: 2 ]) print(slice. Tuple[5: len(slice. Tuple)]) print( slice. List[2: ]) print(slice. String[-4: -1 ]) print(slice. String[-1 ]) print(slice. Tuple[-2: -1]) print( slice. List[-5: -1]) ab (6, 7, 8, 9, 10) ['III', 'IV', 'VI', 'VIII', 'IX', 'X'] ghi j (9, ) ['VI', 'VIII', 'IX'] Lists, Tuples and Dictionaries
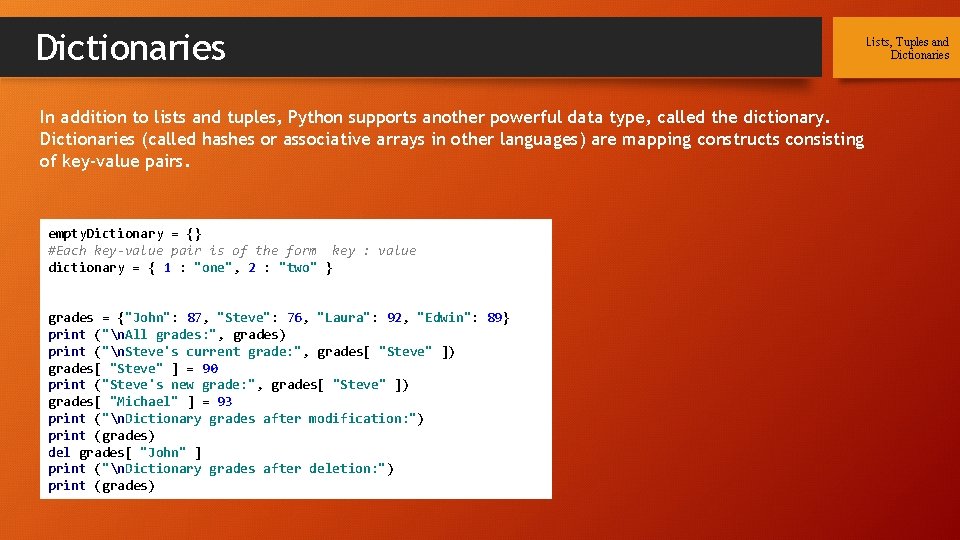
Dictionaries In addition to lists and tuples, Python supports another powerful data type, called the dictionary. Dictionaries (called hashes or associative arrays in other languages) are mapping constructs consisting of key-value pairs. empty. Dictionary = {} #Each key-value pair is of the form key : value dictionary = { 1 : "one", 2 : "two" } grades = {"John": 87, "Steve": 76, "Laura": 92, "Edwin": 89} print ("n. All grades: ", grades) print ("n. Steve's current grade: ", grades[ "Steve" ]) grades[ "Steve" ] = 90 print ("Steve's new grade: ", grades[ "Steve" ]) grades[ "Michael" ] = 93 print ("n. Dictionary grades after modification: ") print (grades) del grades[ "John" ] print ("n. Dictionary grades after deletion: ") print (grades) Lists, Tuples and Dictionaries
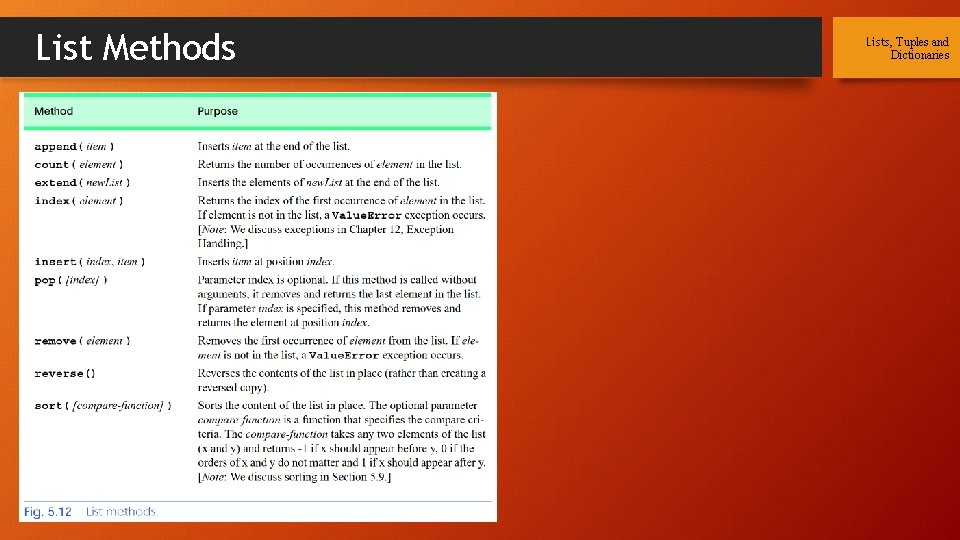
List Methods Lists, Tuples and Dictionaries
![List and Dictionary Methods Mylist1 2 3 4 5 2 4 6 8 Mylist List and Dictionary Methods --Mylist-[1, 2, 3, 4, 5, 2, 4, 6, 8] --Mylist.](https://slidetodoc.com/presentation_image_h2/b6a1ed01f3181f1908a993cf66a67cb2/image-13.jpg)
List and Dictionary Methods --Mylist-[1, 2, 3, 4, 5, 2, 4, 6, 8] --Mylist. append(2)-[1, 2, 3, 4, 5, 2, 4, 6, 8, 2] --Mylist. count(2)-3 --Mylist. extend([10, 11, 12])-[1, 2, 3, 4, 5, 2, 4, 6, 8, 2, 10, 11, 12] --print(Mylist. index(2))-1 --Mylist. insert(3, 99)-[1, 2, 3, 99, 4, 5, 2, 4, 6, 8, 2, 10, 11, 12] --Mylist. pop()-[1, 2, 3, 99, 4, 5, 2, 4, 6, 8, 2, 10, 11] --Mylist. pop(0)-[2, 3, 99, 4, 5, 2, 4, 6, 8, 2, 10, 11] --Mylist. remove(2)-[3, 99, 4, 5, 2, 4, 6, 8, 2, 10, 11] --Mylist. reverse()-[11, 10, 2, 8, 6, 4, 2, 5, 4, 99, 3] --Mylist. sort()-[2, 2, 3, 4, 4, 5, 6, 8, 10, 11, 99] Lists, Tuples and Dictionaries
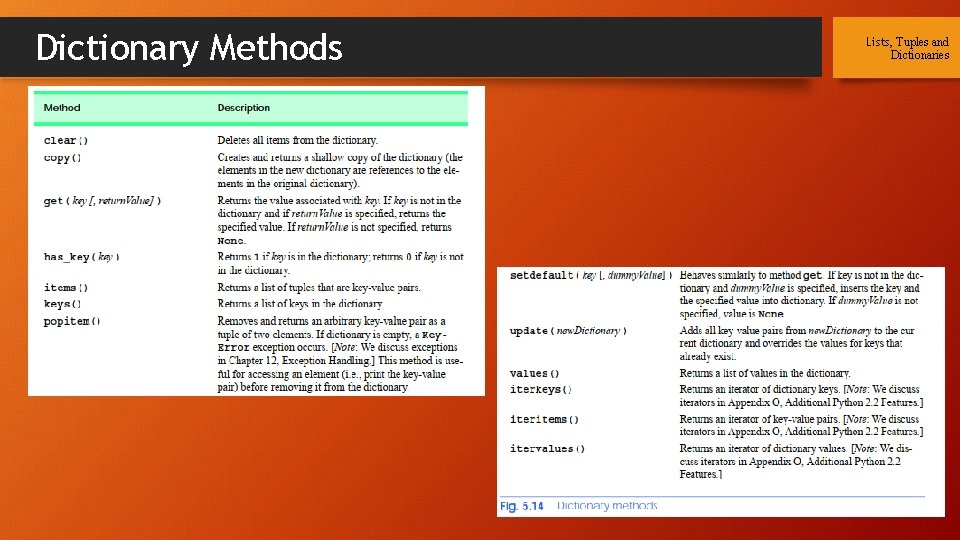
Dictionary Methods Lists, Tuples and Dictionaries
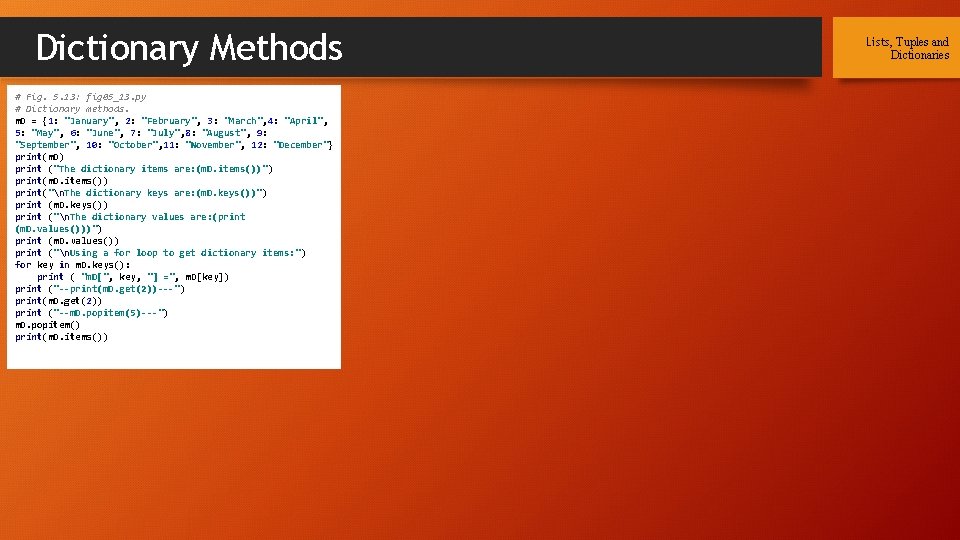
Dictionary Methods # Fig. 5. 13: fig 05_13. py # Dictionary methods. m. D = {1: "January", 2: "February", 3: "March", 4: "April", 5: "May", 6: "June", 7: "July", 8: "August", 9: "September", 10: "October", 11: "November", 12: "December"} print(m. D) print ("The dictionary items are: (m. D. items())") print(m. D. items()) print("n. The dictionary keys are: (m. D. keys())") print (m. D. keys()) print ("n. The dictionary values are: (print (m. D. values()))") print (m. D. values()) print ("n. Using a for loop to get dictionary items: ") for key in m. D. keys(): print ( "m. D[", key, "] =", m. D[key]) print ("--print(m. D. get(2))---") print(m. D. get(2)) print ("--m. D. popitem(5)---") m. D. popitem() print(m. D. items()) Lists, Tuples and Dictionaries
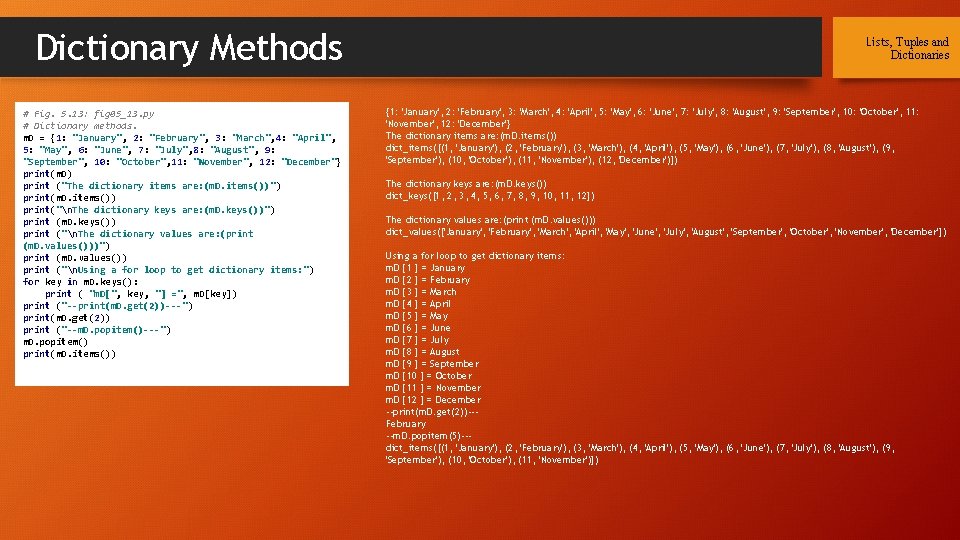
Dictionary Methods # Fig. 5. 13: fig 05_13. py # Dictionary methods. m. D = {1: "January", 2: "February", 3: "March", 4: "April", 5: "May", 6: "June", 7: "July", 8: "August", 9: "September", 10: "October", 11: "November", 12: "December"} print(m. D) print ("The dictionary items are: (m. D. items())") print(m. D. items()) print("n. The dictionary keys are: (m. D. keys())") print (m. D. keys()) print ("n. The dictionary values are: (print (m. D. values()))") print (m. D. values()) print ("n. Using a for loop to get dictionary items: ") for key in m. D. keys(): print ( "m. D[", key, "] =", m. D[key]) print ("--print(m. D. get(2))---") print(m. D. get(2)) print ("--m. D. popitem()---") m. D. popitem() print(m. D. items()) Lists, Tuples and Dictionaries {1: 'January', 2: 'February', 3: 'March', 4: 'April', 5: 'May', 6: 'June', 7: 'July', 8: 'August', 9: 'September', 10: 'October', 11: 'November', 12: 'December'} The dictionary items are: (m. D. items()) dict_items([(1, 'January'), (2, 'February'), (3, 'March'), (4, 'April'), (5, 'May'), (6, 'June'), (7, 'July'), (8, 'August'), (9, 'September'), (10, 'October'), (11, 'November'), (12, 'December')]) The dictionary keys are: (m. D. keys()) dict_keys([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]) The dictionary values are: (print (m. D. values())) dict_values(['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December']) Using a for loop to get dictionary items: m. D[ 1 ] = January m. D[ 2 ] = February m. D[ 3 ] = March m. D[ 4 ] = April m. D[ 5 ] = May m. D[ 6 ] = June m. D[ 7 ] = July m. D[ 8 ] = August m. D[ 9 ] = September m. D[ 10 ] = October m. D[ 11 ] = November m. D[ 12 ] = December --print(m. D. get(2))--February --m. D. popitem(5)--dict_items([(1, 'January'), (2, 'February'), (3, 'March'), (4, 'April'), (5, 'May'), (6, 'June'), (7, 'July'), (8, 'August'), (9, 'September'), (10, 'October'), (11, 'November')])
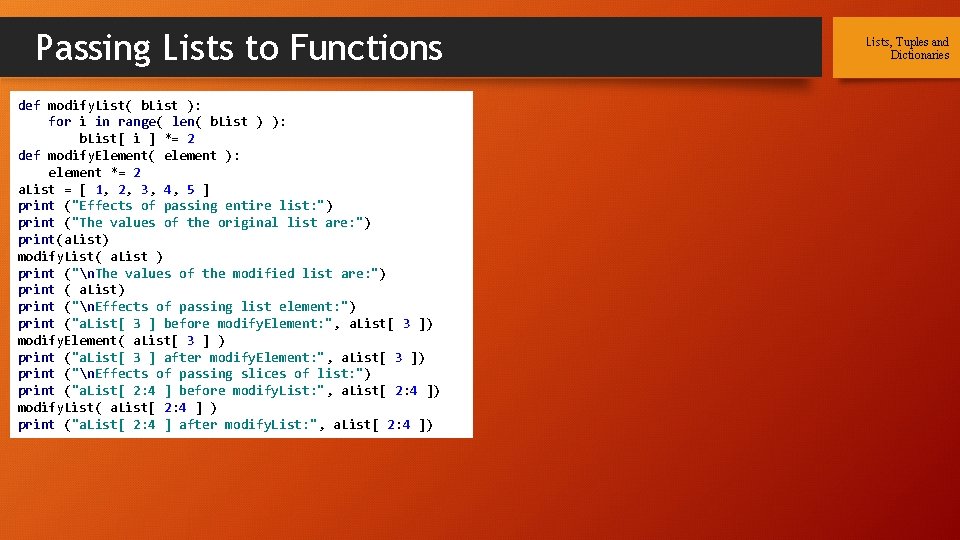
Passing Lists to Functions def modify. List( b. List ): for i in range( len( b. List ) ): b. List[ i ] *= 2 def modify. Element( element ): element *= 2 a. List = [ 1, 2, 3, 4, 5 ] print ("Effects of passing entire list: ") print ("The values of the original list are: ") print(a. List) modify. List( a. List ) print ("n. The values of the modified list are: ") print ( a. List) print ("n. Effects of passing list element: ") print ("a. List[ 3 ] before modify. Element: ", a. List[ 3 ]) modify. Element( a. List[ 3 ] ) print ("a. List[ 3 ] after modify. Element: ", a. List[ 3 ]) print ("n. Effects of passing slices of list: ") print ("a. List[ 2: 4 ] before modify. List: ", a. List[ 2: 4 ]) modify. List( a. List[ 2: 4 ] ) print ("a. List[ 2: 4 ] after modify. List: ", a. List[ 2: 4 ]) Lists, Tuples and Dictionaries
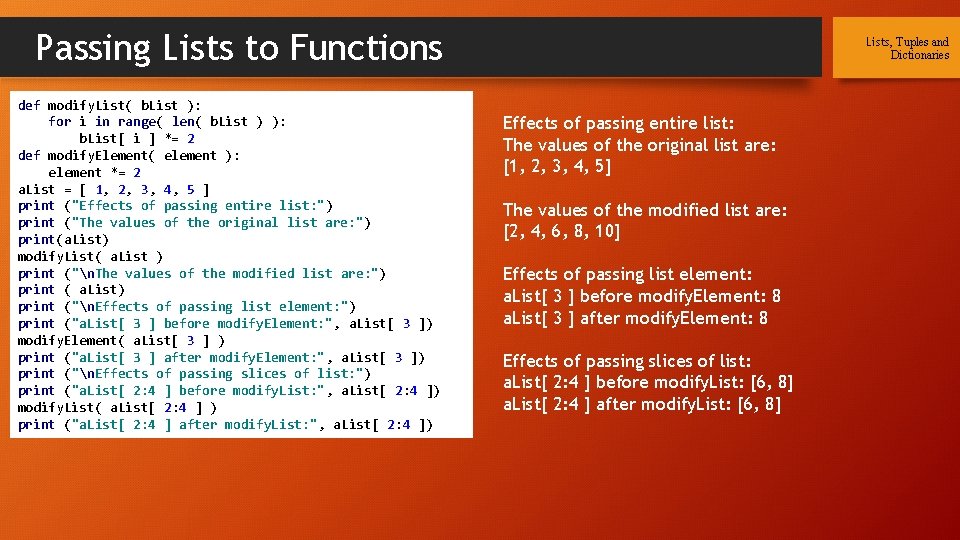
Passing Lists to Functions def modify. List( b. List ): for i in range( len( b. List ) ): b. List[ i ] *= 2 def modify. Element( element ): element *= 2 a. List = [ 1, 2, 3, 4, 5 ] print ("Effects of passing entire list: ") print ("The values of the original list are: ") print(a. List) modify. List( a. List ) print ("n. The values of the modified list are: ") print ( a. List) print ("n. Effects of passing list element: ") print ("a. List[ 3 ] before modify. Element: ", a. List[ 3 ]) modify. Element( a. List[ 3 ] ) print ("a. List[ 3 ] after modify. Element: ", a. List[ 3 ]) print ("n. Effects of passing slices of list: ") print ("a. List[ 2: 4 ] before modify. List: ", a. List[ 2: 4 ]) modify. List( a. List[ 2: 4 ] ) print ("a. List[ 2: 4 ] after modify. List: ", a. List[ 2: 4 ]) Lists, Tuples and Dictionaries Effects of passing entire list: The values of the original list are: [1, 2, 3, 4, 5] The values of the modified list are: [2, 4, 6, 8, 10] Effects of passing list element: a. List[ 3 ] before modify. Element: 8 a. List[ 3 ] after modify. Element: 8 Effects of passing slices of list: a. List[ 2: 4 ] before modify. List: [6, 8] a. List[ 2: 4 ] after modify. List: [6, 8]
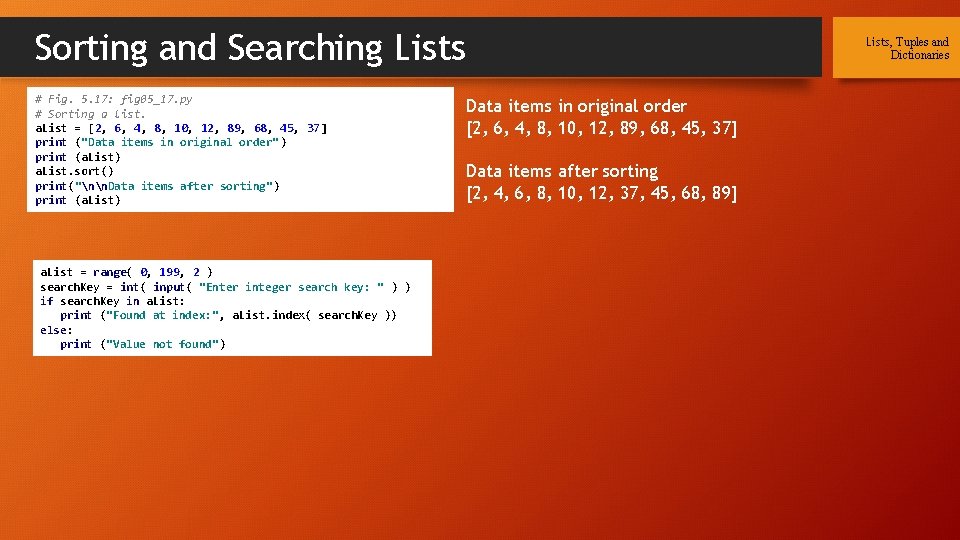
Sorting and Searching Lists # Fig. 5. 17: fig 05_17. py # Sorting a list. a. List = [2, 6, 4, 8, 10, 12, 89, 68, 45, 37] print ("Data items in original order") print (a. List) a. List. sort() print("nn. Data items after sorting") print (a. List) a. List = range( 0, 199, 2 ) search. Key = int( input( "Enter integer search key: " ) ) if search. Key in a. List: print ("Found at index: ", a. List. index( search. Key )) else: print ("Value not found") Data items in original order [2, 6, 4, 8, 10, 12, 89, 68, 45, 37] Data items after sorting [2, 4, 6, 8, 10, 12, 37, 45, 68, 89] Lists, Tuples and Dictionaries
![MultipleSubscripted Sequences b 1 2 3 4 Multiple-Subscripted Sequences b = [ [ 1, 2 ], [ 3, 4 ] ]](https://slidetodoc.com/presentation_image_h2/b6a1ed01f3181f1908a993cf66a67cb2/image-20.jpg)
Multiple-Subscripted Sequences b = [ [ 1, 2 ], [ 3, 4 ] ] c = ( ( 1, 2 ), ( 3, 4, 5 ) ) from random import * Matrix = [[randint(1, 100) for j in range(0, 10)] for i in range(0, 10)] for x in range(len(Matrix)): print(Matrix[x]) Lists, Tuples and Dictionaries
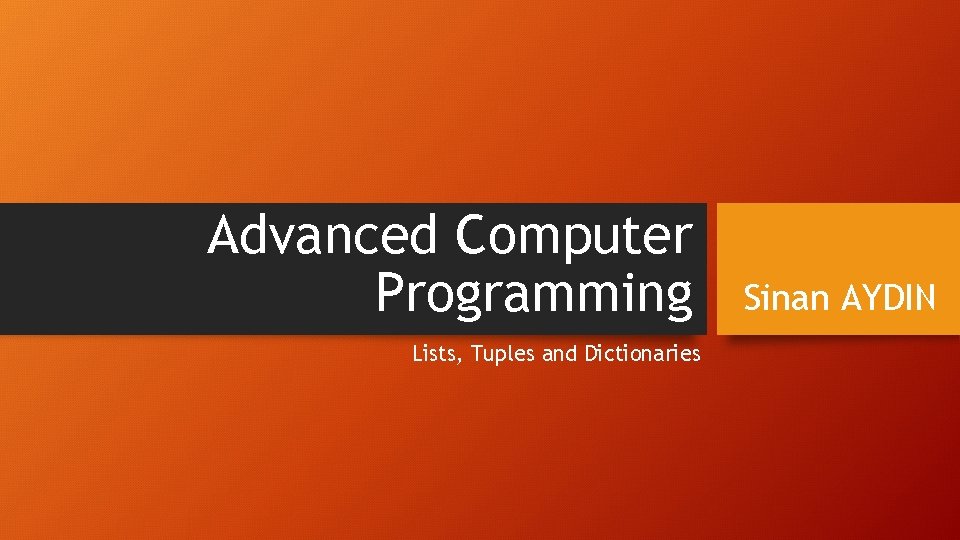
Advanced Computer Programming Lists, Tuples and Dictionaries Sinan AYDIN