Abstract Data Types ADT A set of objects
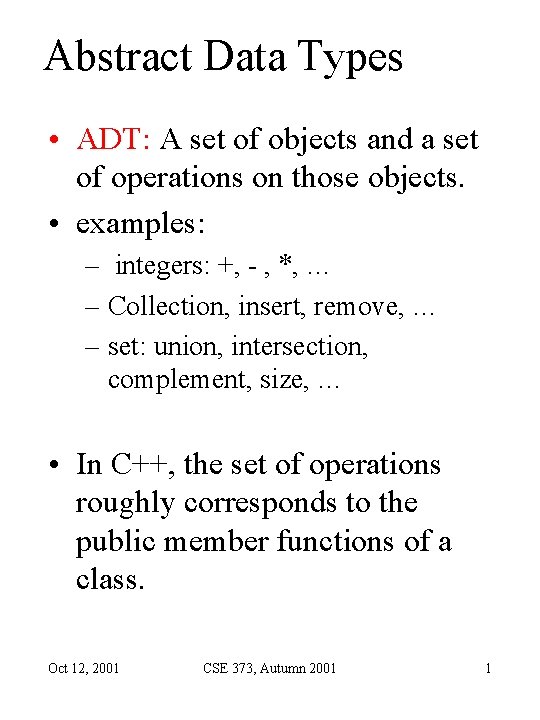
Abstract Data Types • ADT: A set of objects and a set of operations on those objects. • examples: – integers: +, - , *, … – Collection, insert, remove, … – set: union, intersection, complement, size, … • In C++, the set of operations roughly corresponds to the public member functions of a class. Oct 12, 2001 CSE 373, Autumn 2001 1
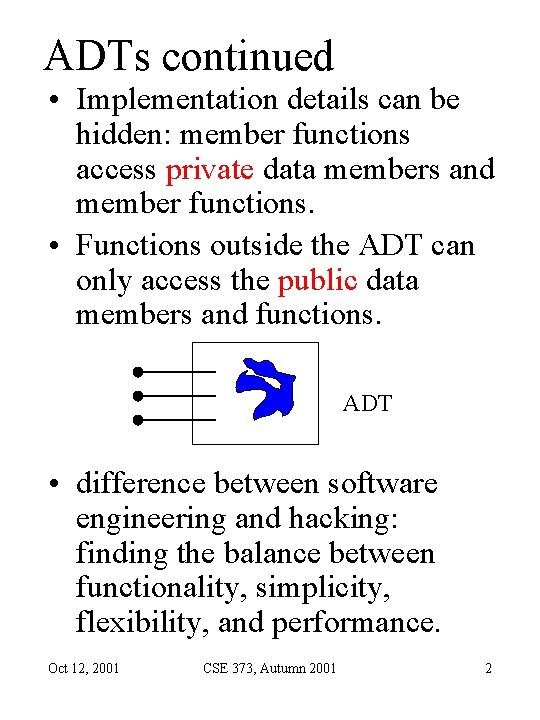
ADTs continued • Implementation details can be hidden: member functions access private data members and member functions. • Functions outside the ADT can only access the public data members and functions. ADT • difference between software engineering and hacking: finding the balance between functionality, simplicity, flexibility, and performance. Oct 12, 2001 CSE 373, Autumn 2001 2
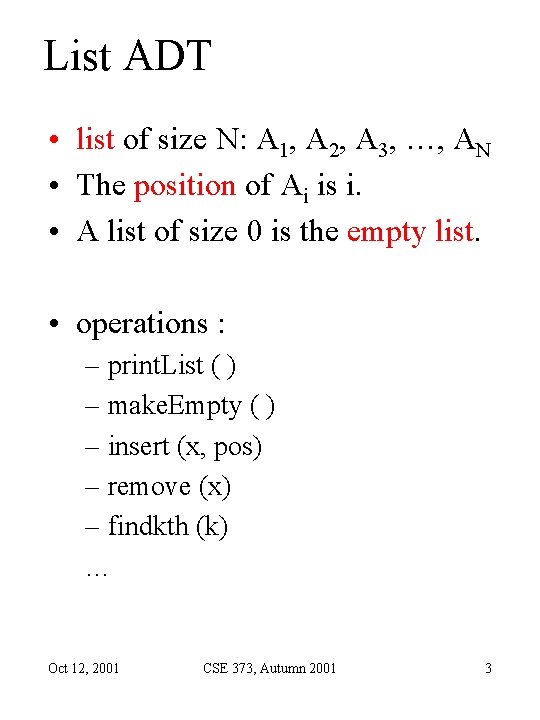
List ADT • list of size N: A 1, A 2, A 3, …, AN • The position of Ai is i. • A list of size 0 is the empty list. • operations : – print. List ( ) – make. Empty ( ) – insert (x, pos) – remove (x) – findkth (k) … Oct 12, 2001 CSE 373, Autumn 2001 3
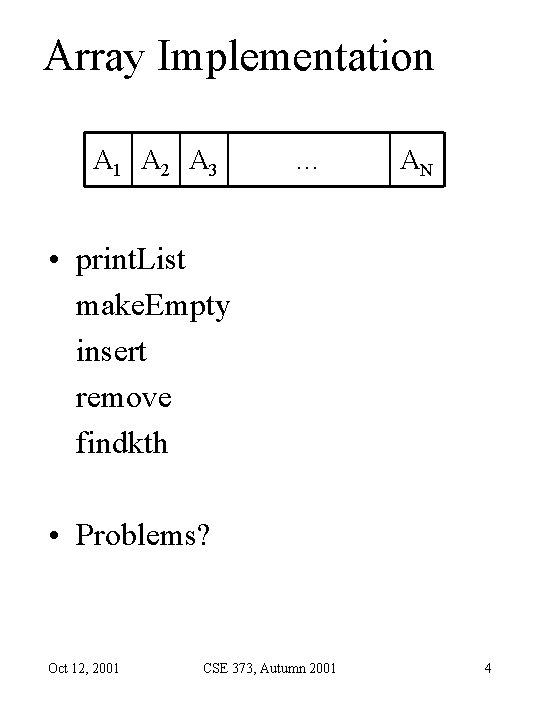
Array Implementation A 1 A 2 A 3 … AN • print. List make. Empty insert remove findkth • Problems? Oct 12, 2001 CSE 373, Autumn 2001 4
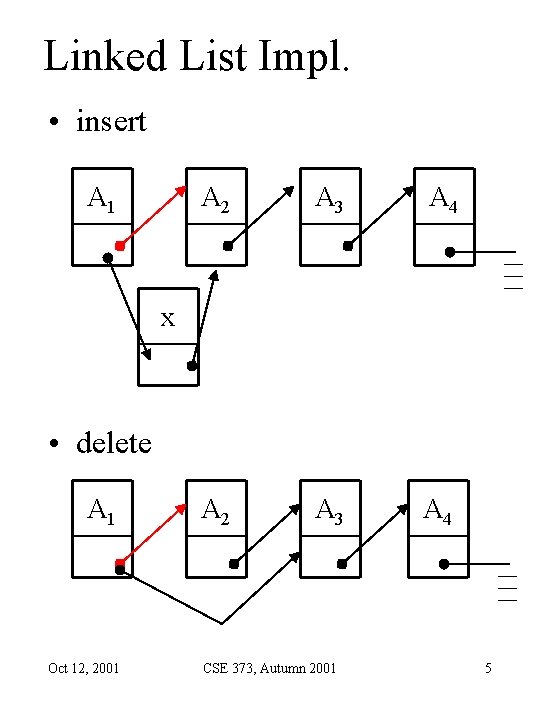
Linked List Impl. • insert A 1 A 2 A 3 A 4 x • delete A 1 Oct 12, 2001 CSE 373, Autumn 2001 5
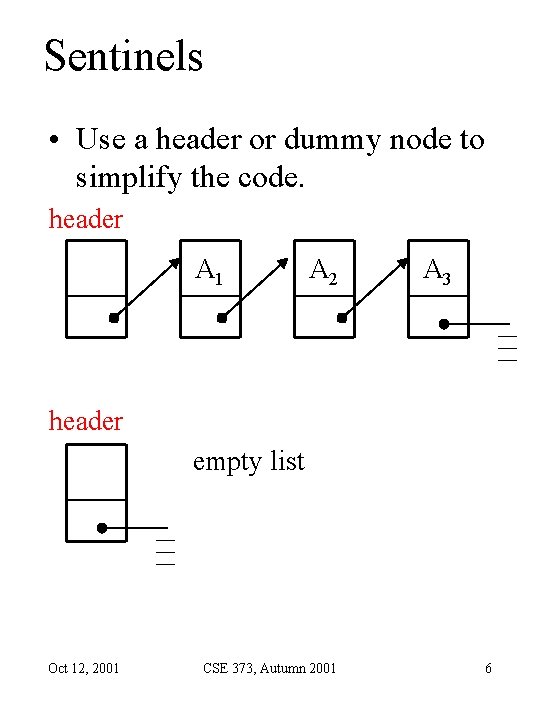
Sentinels • Use a header or dummy node to simplify the code. header A 1 A 2 A 3 header empty list Oct 12, 2001 CSE 373, Autumn 2001 6
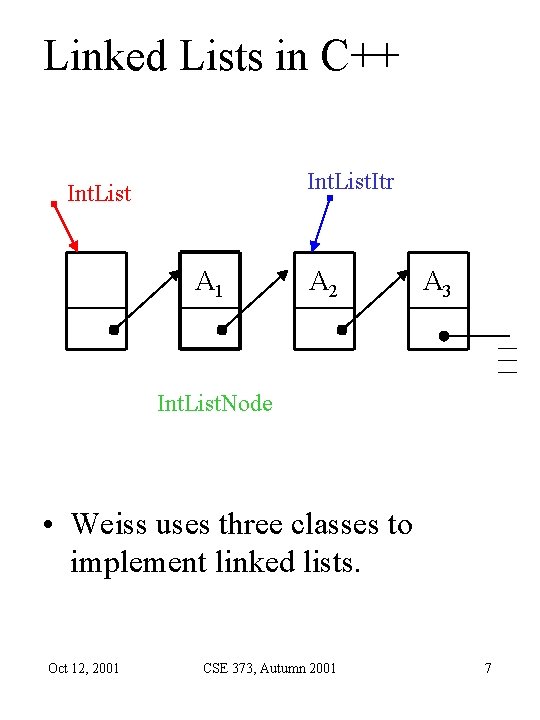
Linked Lists in C++ Int. List. Itr Int. List A 1 A 2 A 3 Int. List. Node • Weiss uses three classes to implement linked lists. Oct 12, 2001 CSE 373, Autumn 2001 7
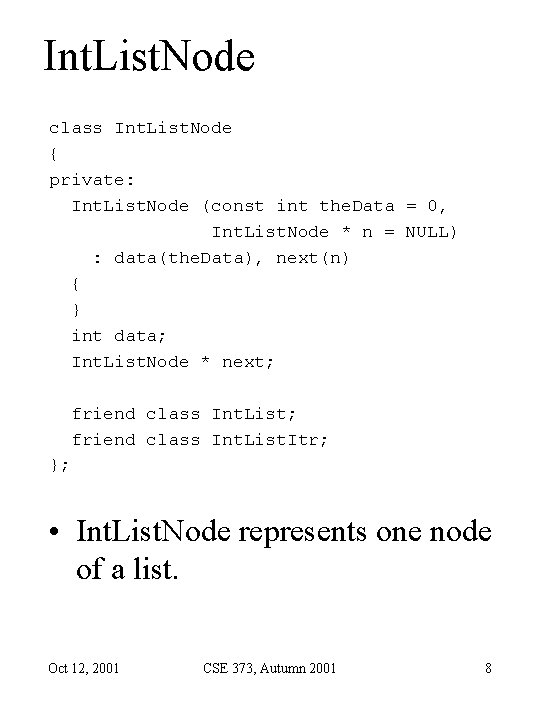
Int. List. Node class Int. List. Node { private: Int. List. Node (const int the. Data = 0, Int. List. Node * n = NULL) : data(the. Data), next(n) { } int data; Int. List. Node * next; friend class Int. List. Itr; }; • Int. List. Node represents one node of a list. Oct 12, 2001 CSE 373, Autumn 2001 8
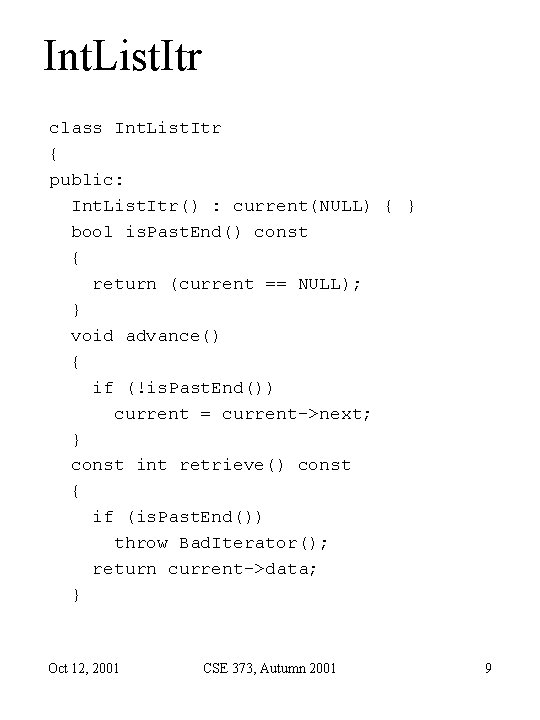
Int. List. Itr class Int. List. Itr { public: Int. List. Itr() : current(NULL) { } bool is. Past. End() const { return (current == NULL); } void advance() { if (!is. Past. End()) current = current->next; } const int retrieve() const { if (is. Past. End()) throw Bad. Iterator(); return current->data; } Oct 12, 2001 CSE 373, Autumn 2001 9
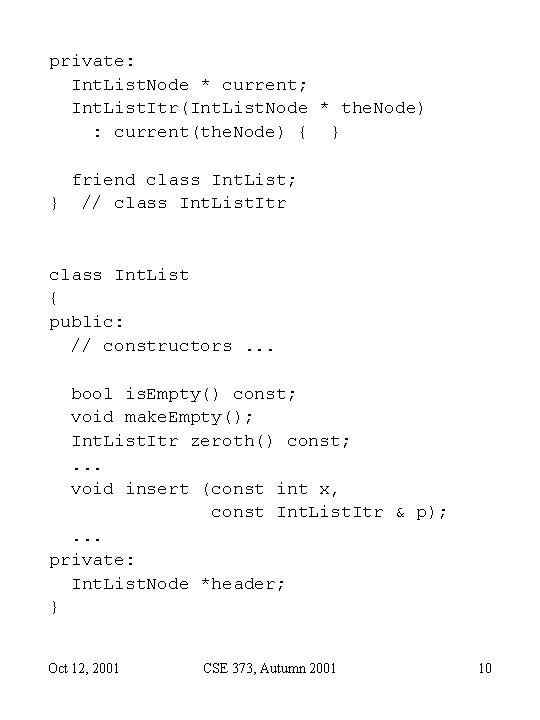
private: Int. List. Node * current; Int. List. Itr(Int. List. Node * the. Node) : current(the. Node) { } friend class Int. List; } // class Int. List. Itr class Int. List { public: // constructors. . . bool is. Empty() const; void make. Empty(); Int. List. Itr zeroth() const; . . . void insert (const int x, const Int. List. Itr & p); . . . private: Int. List. Node *header; } Oct 12, 2001 CSE 373, Autumn 2001 10
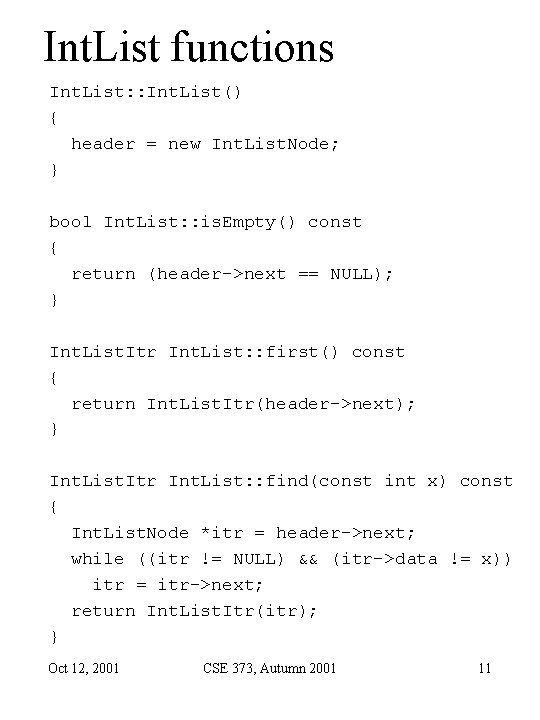
Int. List functions Int. List: : Int. List() { header = new Int. List. Node; } bool Int. List: : is. Empty() const { return (header->next == NULL); } Int. List. Itr Int. List: : first() const { return Int. List. Itr(header->next); } Int. List. Itr Int. List: : find(const int x) const { Int. List. Node *itr = header->next; while ((itr != NULL) && (itr->data != x)) itr = itr->next; return Int. List. Itr(itr); } Oct 12, 2001 CSE 373, Autumn 2001 11
- Slides: 11