A Scheme procedure is A Scheme procedure is
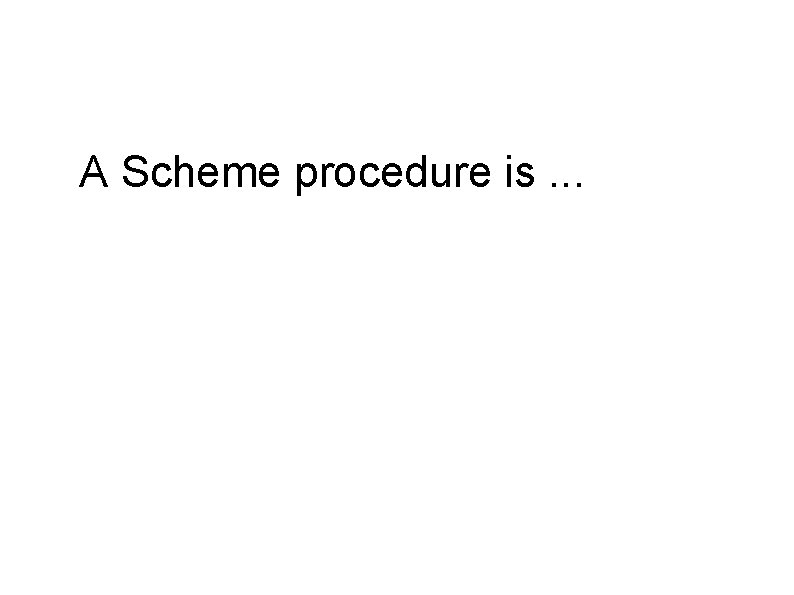
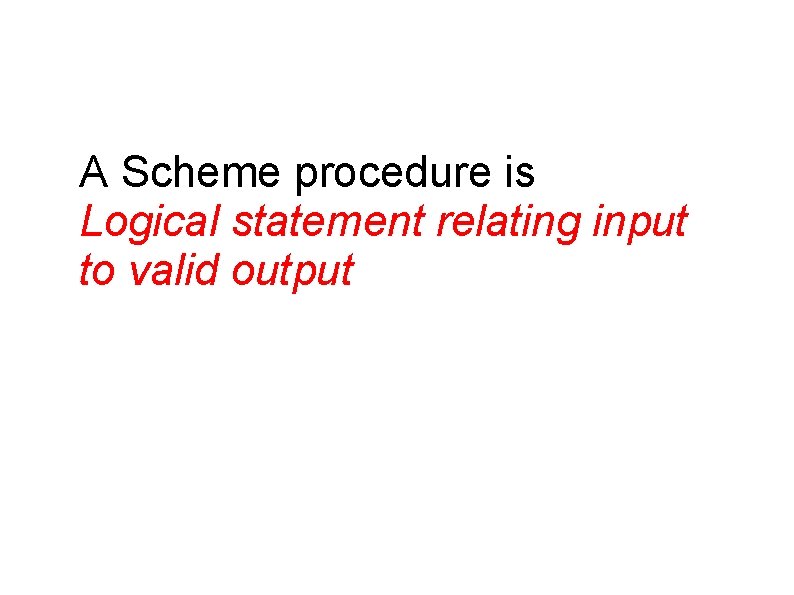
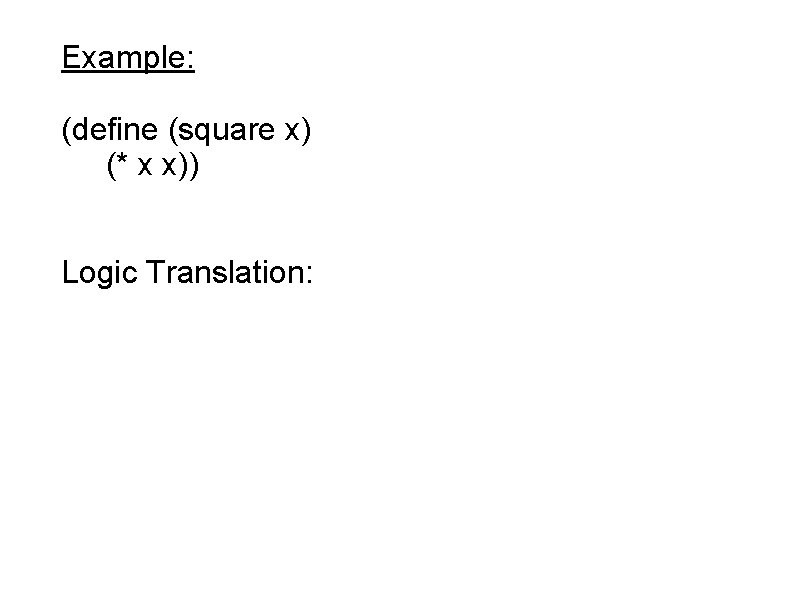
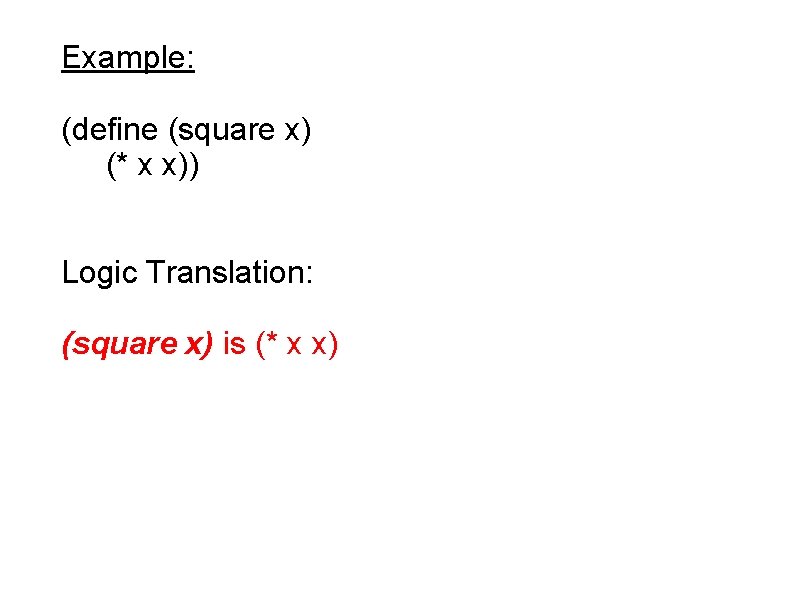
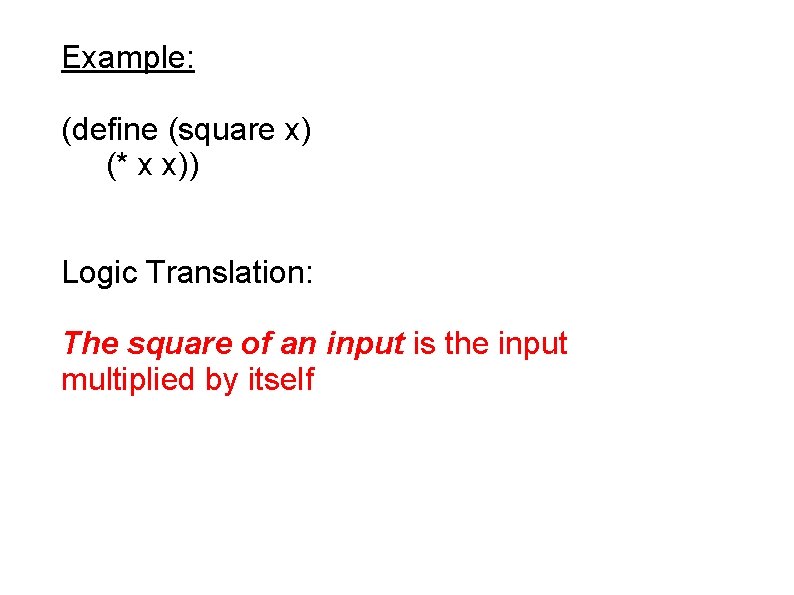
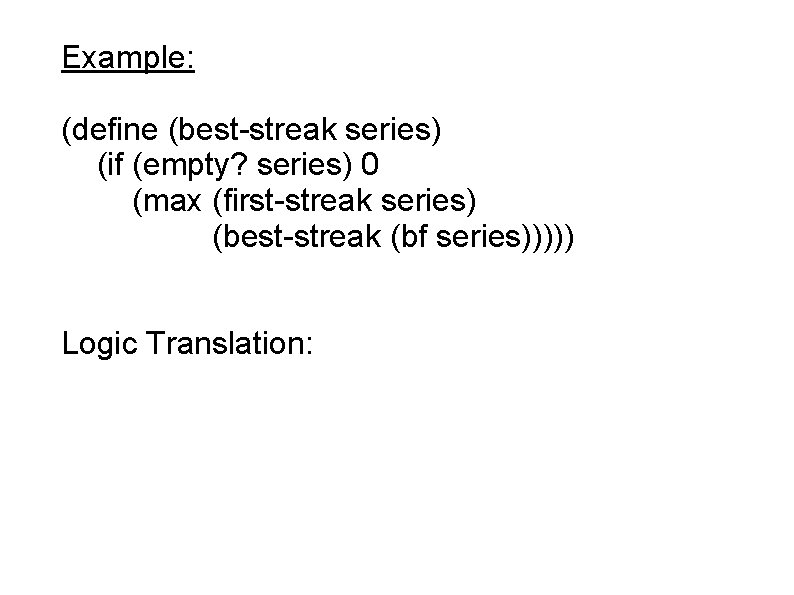
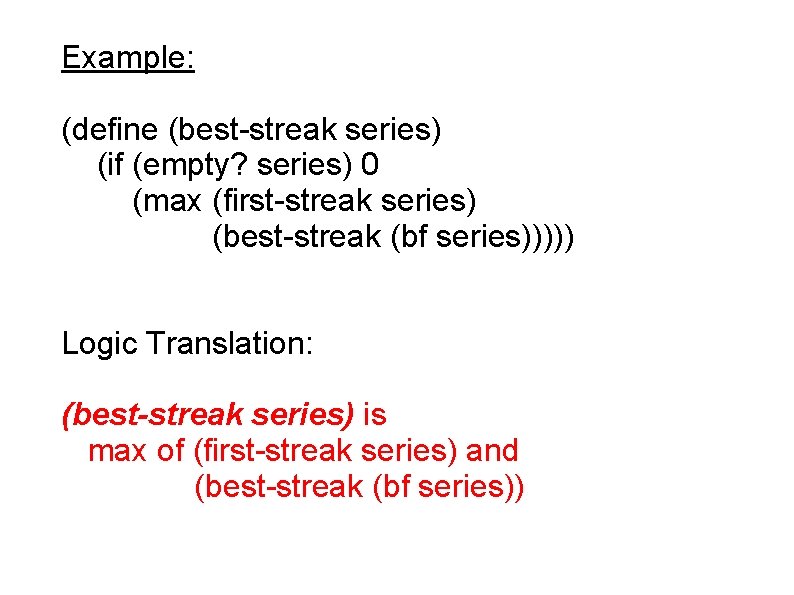
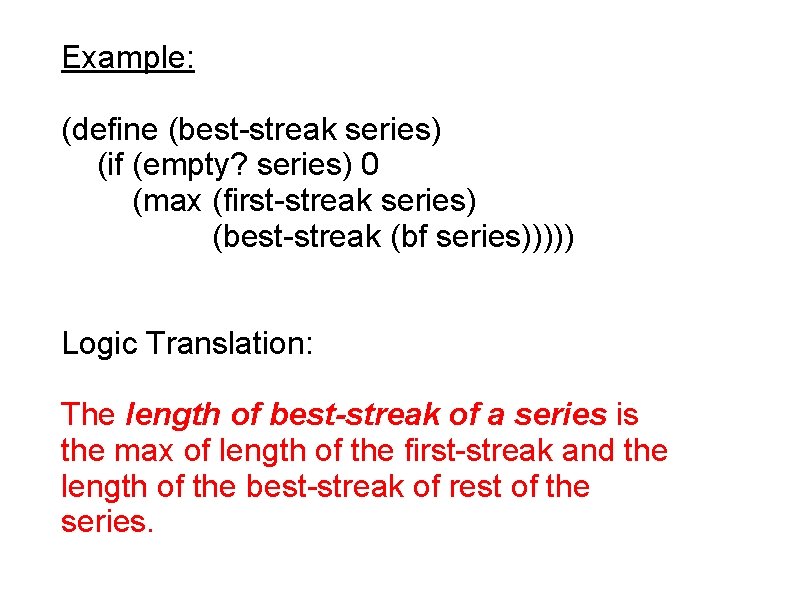
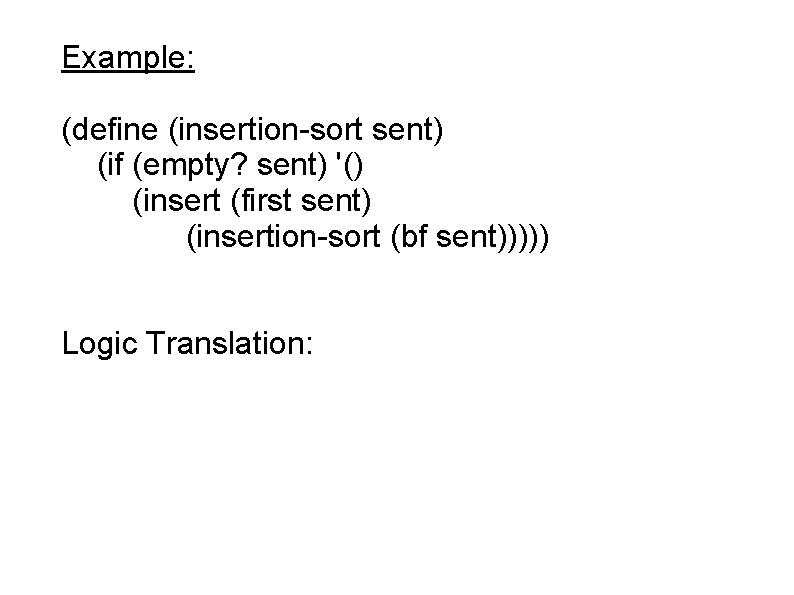
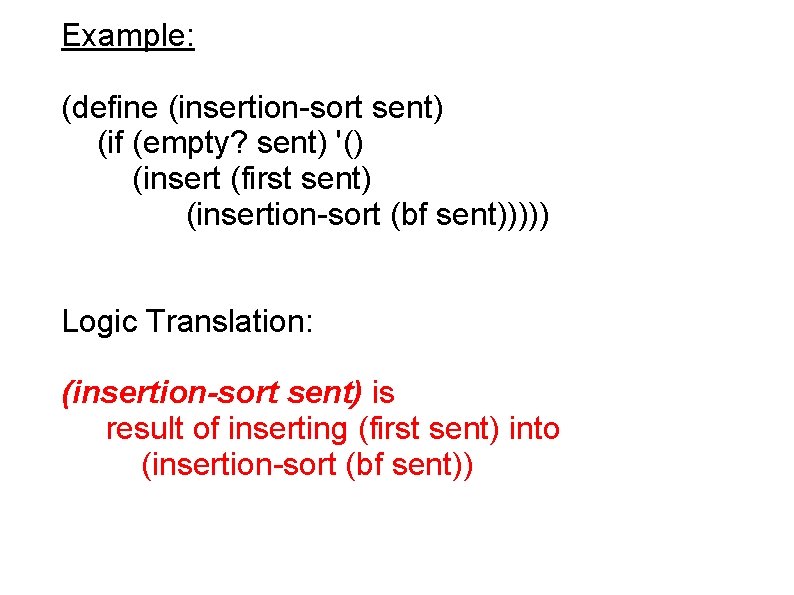
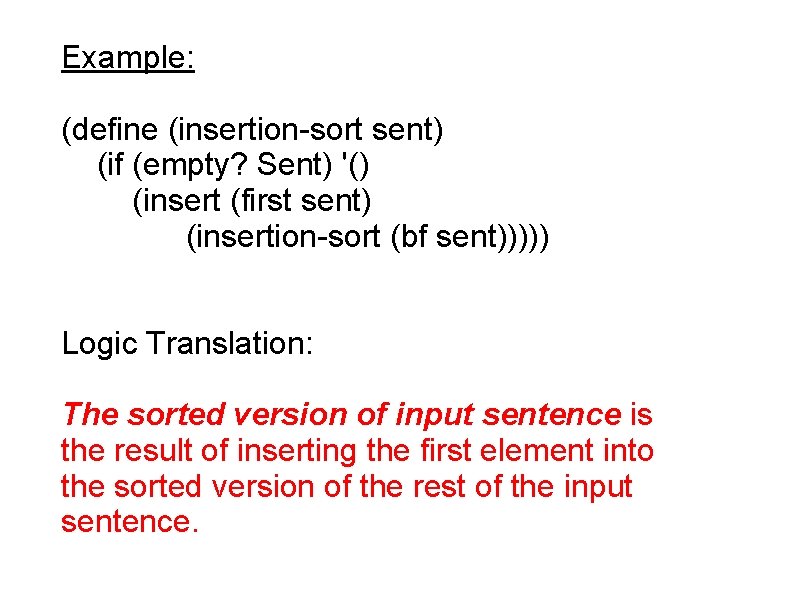
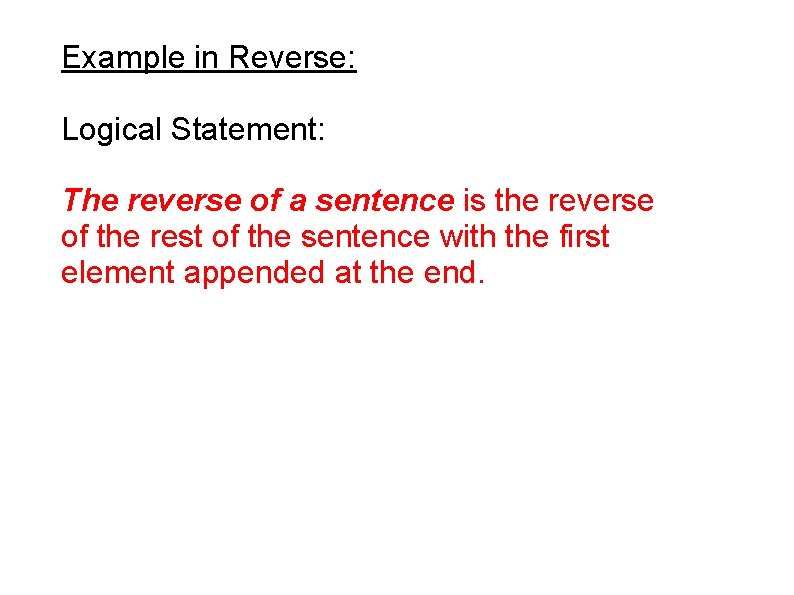
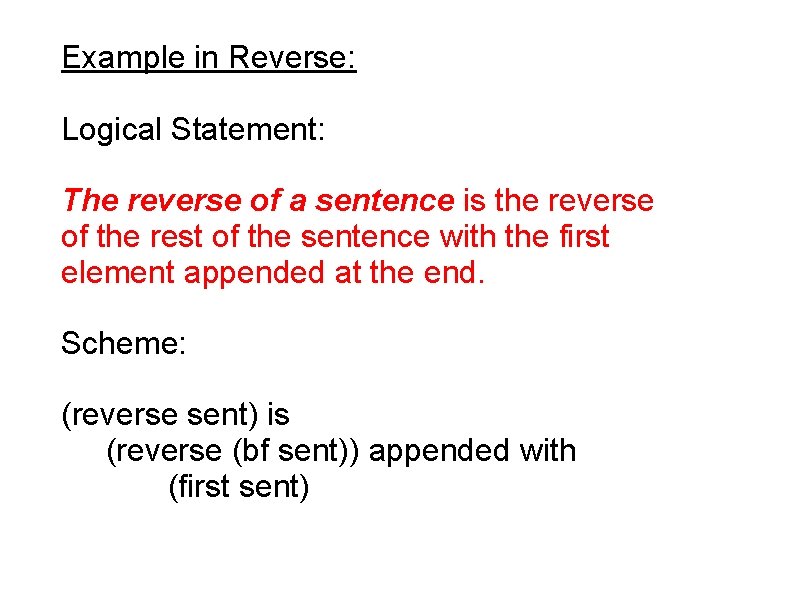
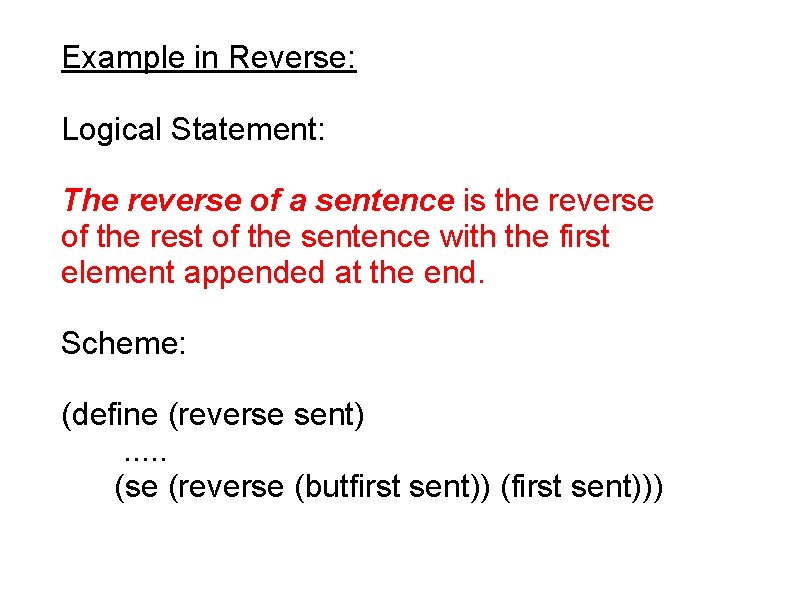
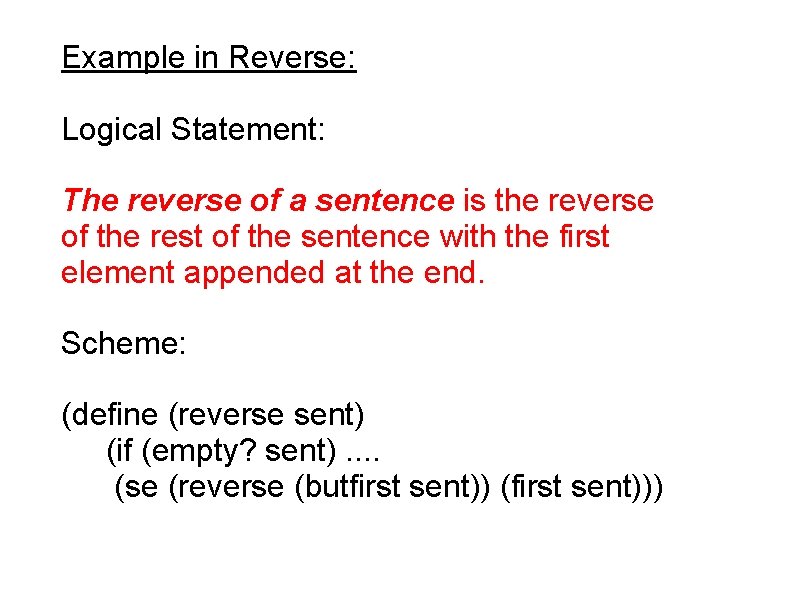
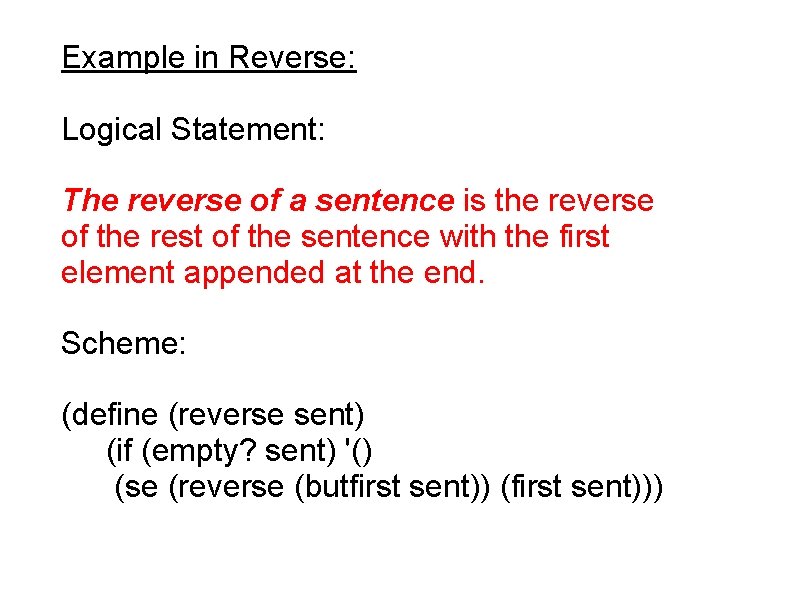
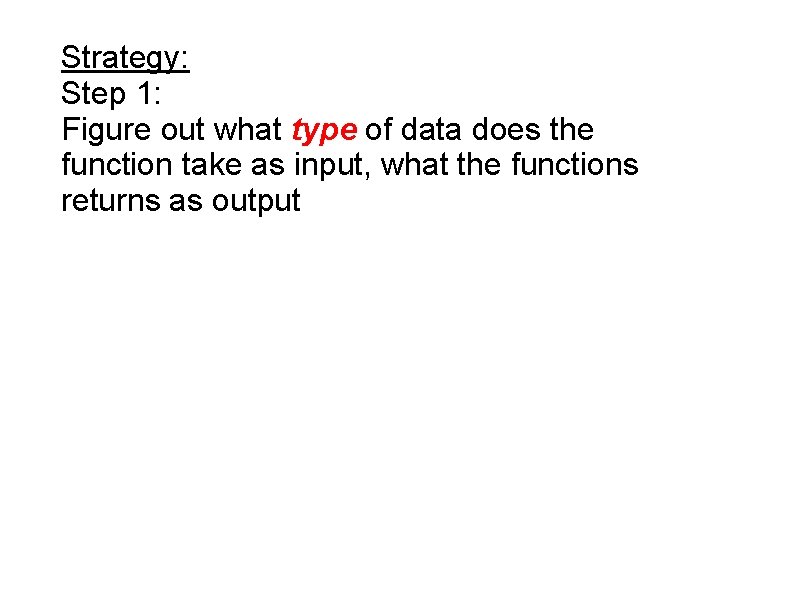
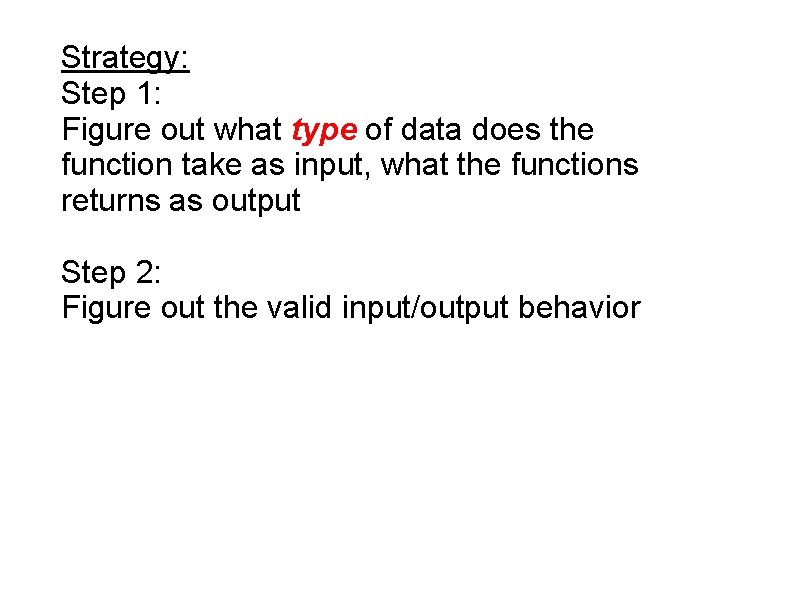
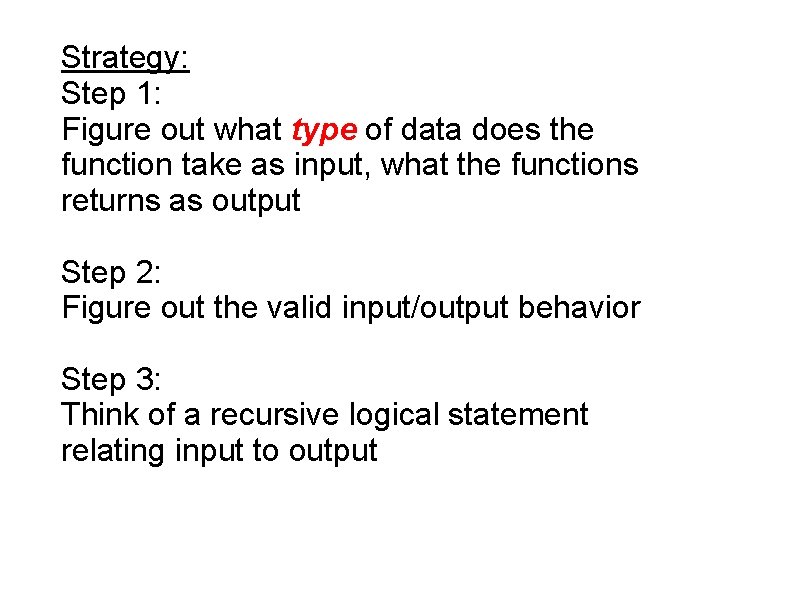
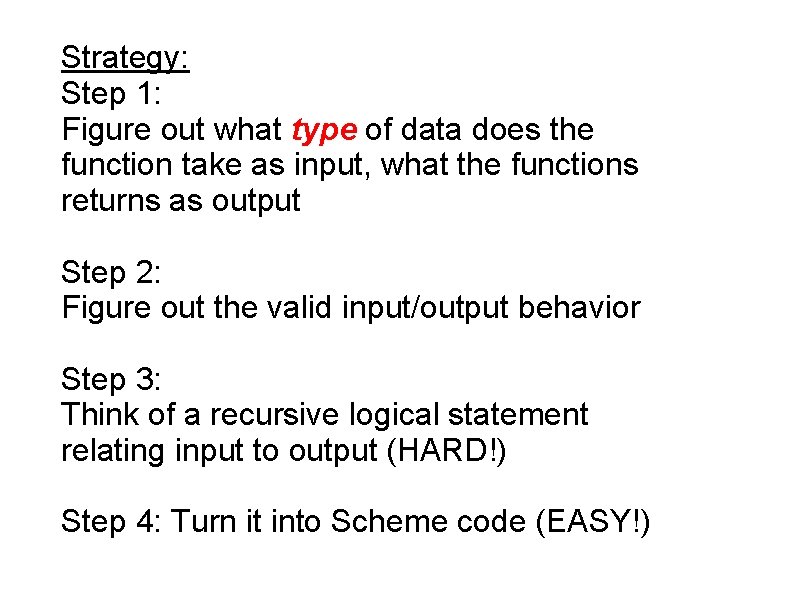
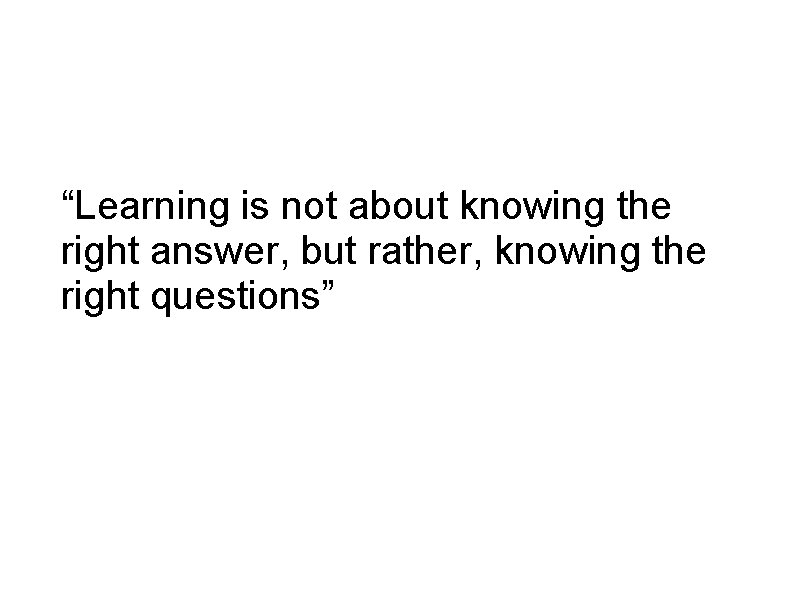
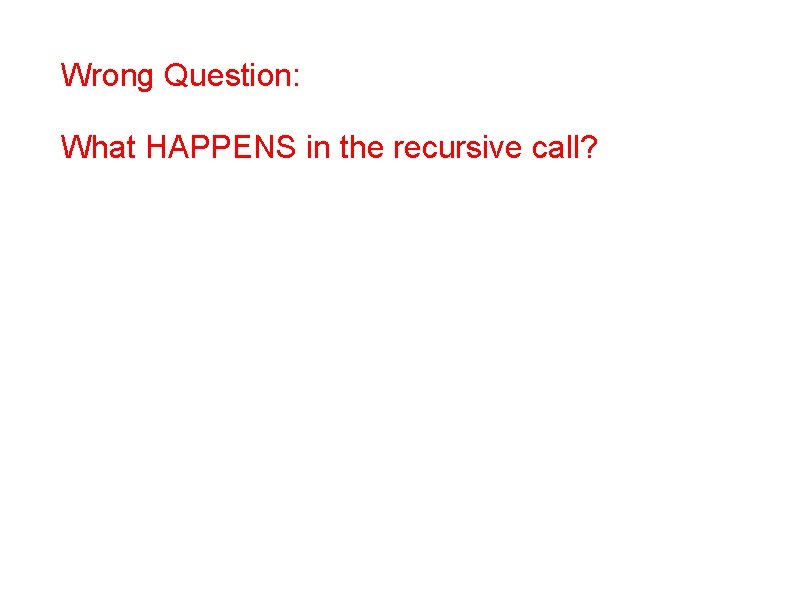
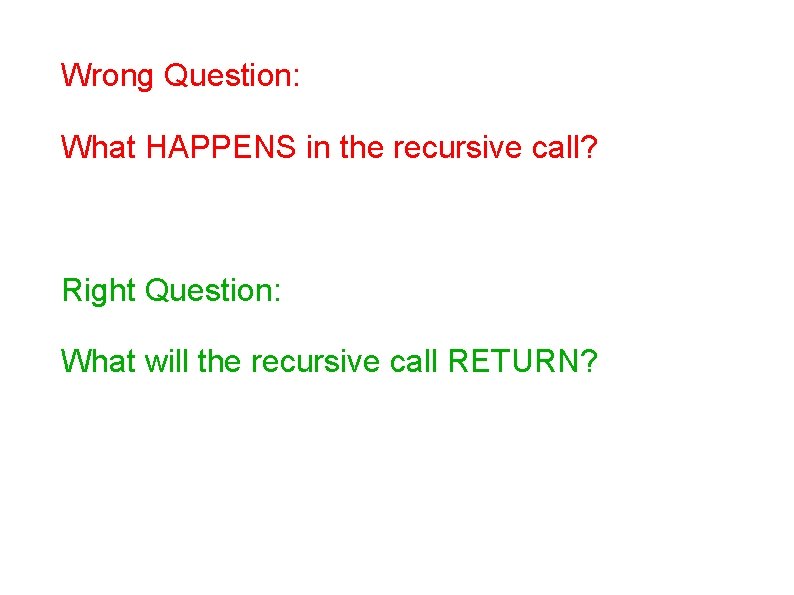
- Slides: 23
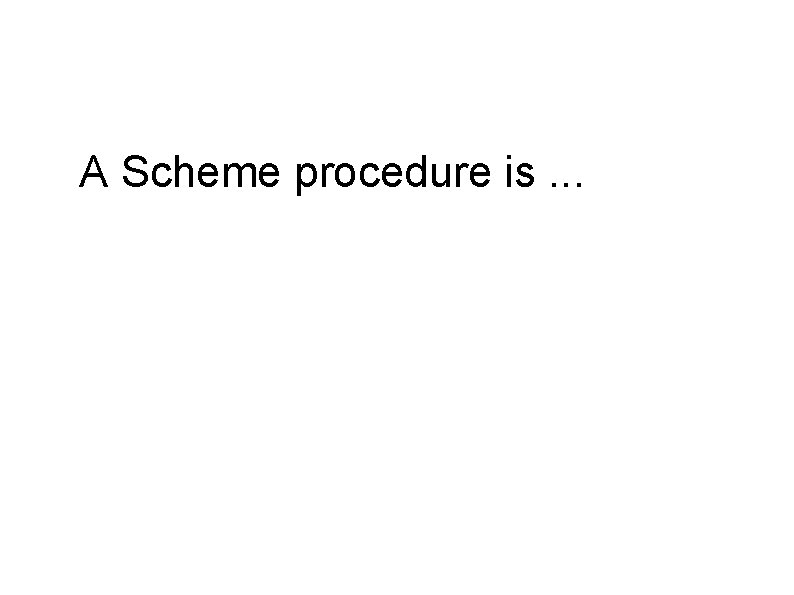
A Scheme procedure is. . .
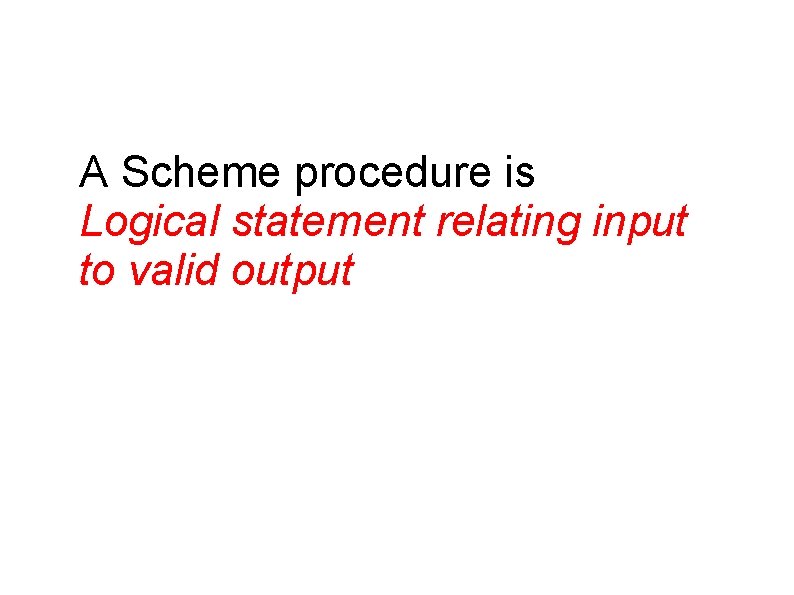
A Scheme procedure is Logical statement relating input to valid output
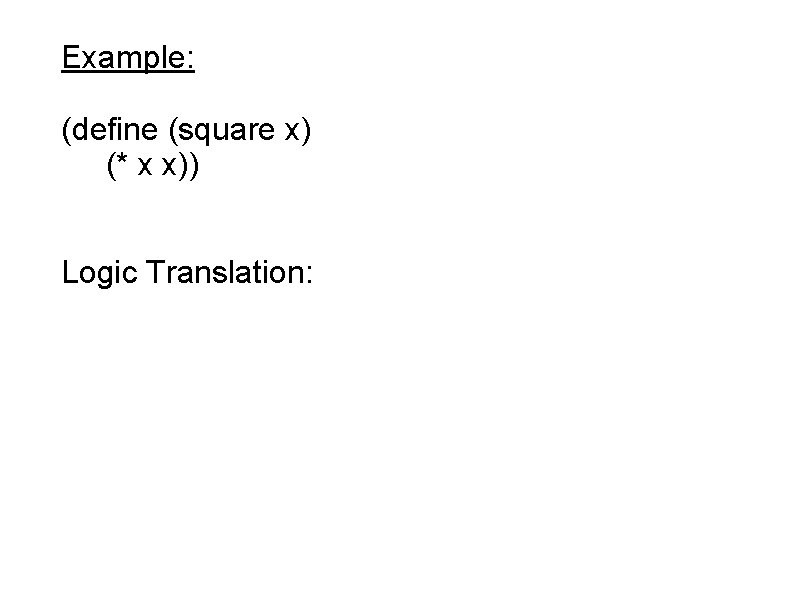
Example: (define (square x) (* x x)) Logic Translation:
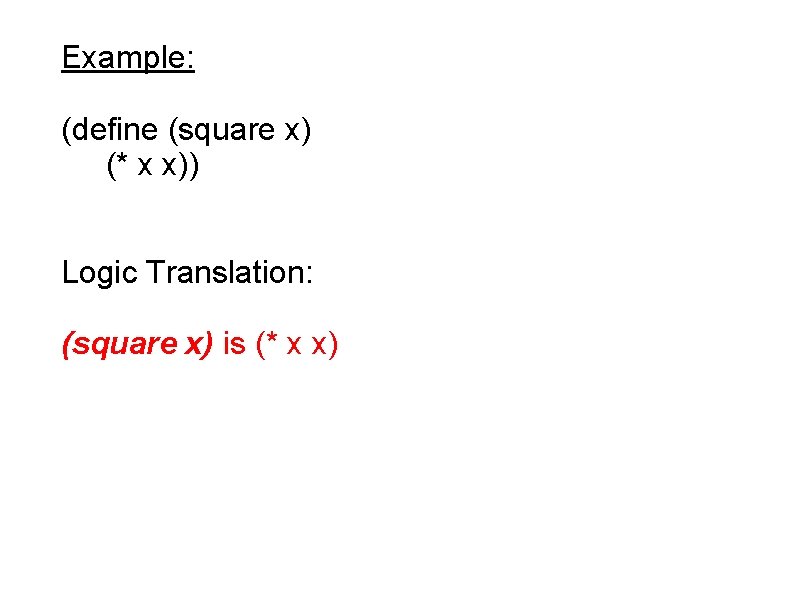
Example: (define (square x) (* x x)) Logic Translation: (square x) is (* x x)
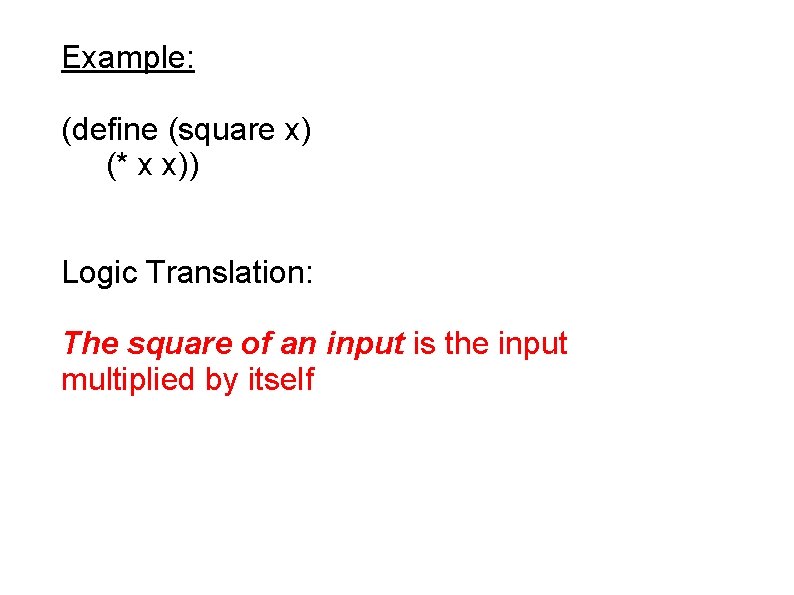
Example: (define (square x) (* x x)) Logic Translation: The square of an input is the input multiplied by itself
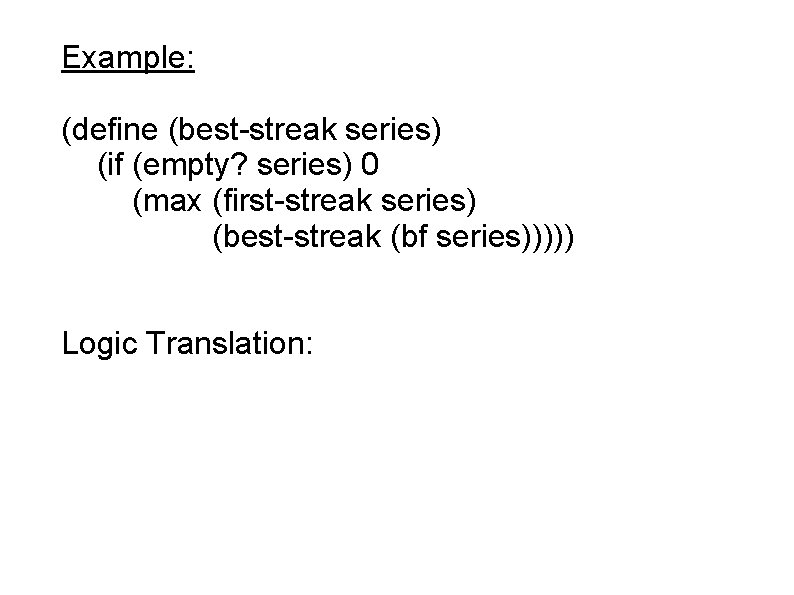
Example: (define (best-streak series) (if (empty? series) 0 (max (first-streak series) (best-streak (bf series))))) Logic Translation:
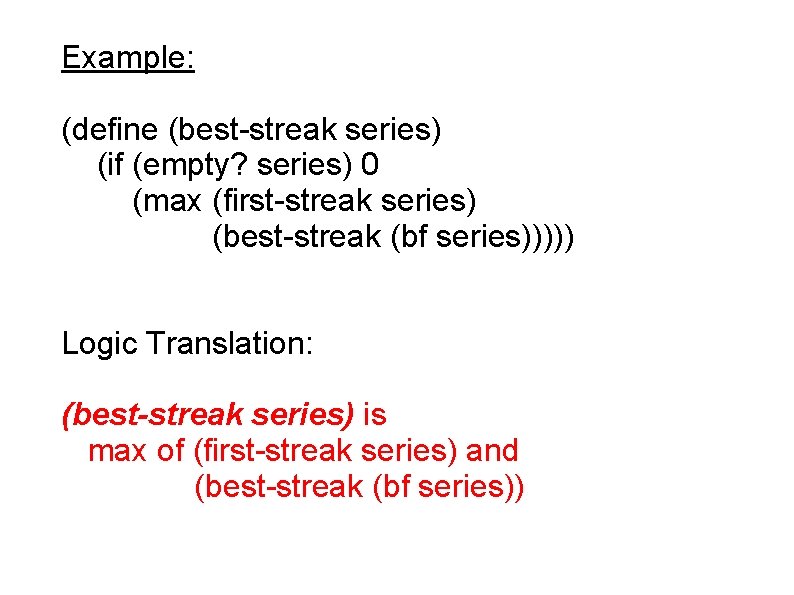
Example: (define (best-streak series) (if (empty? series) 0 (max (first-streak series) (best-streak (bf series))))) Logic Translation: (best-streak series) is max of (first-streak series) and (best-streak (bf series))
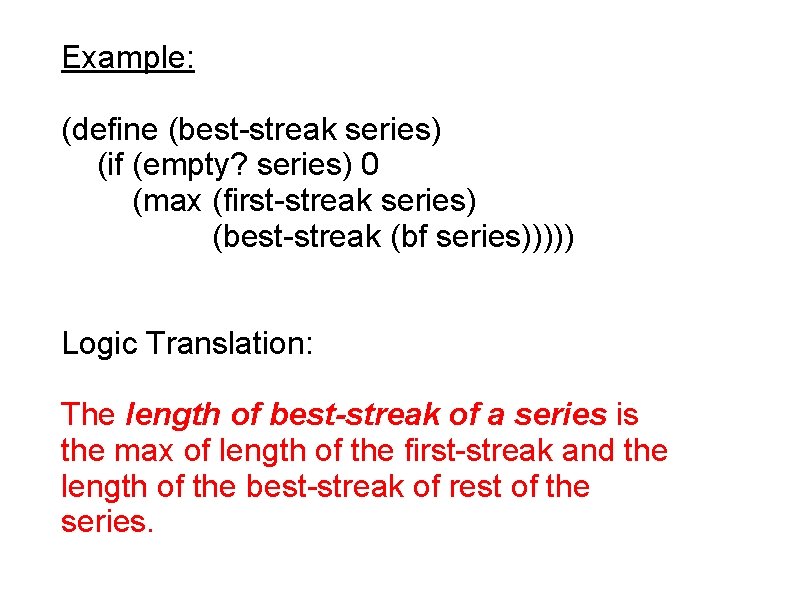
Example: (define (best-streak series) (if (empty? series) 0 (max (first-streak series) (best-streak (bf series))))) Logic Translation: The length of best-streak of a series is the max of length of the first-streak and the length of the best-streak of rest of the series.
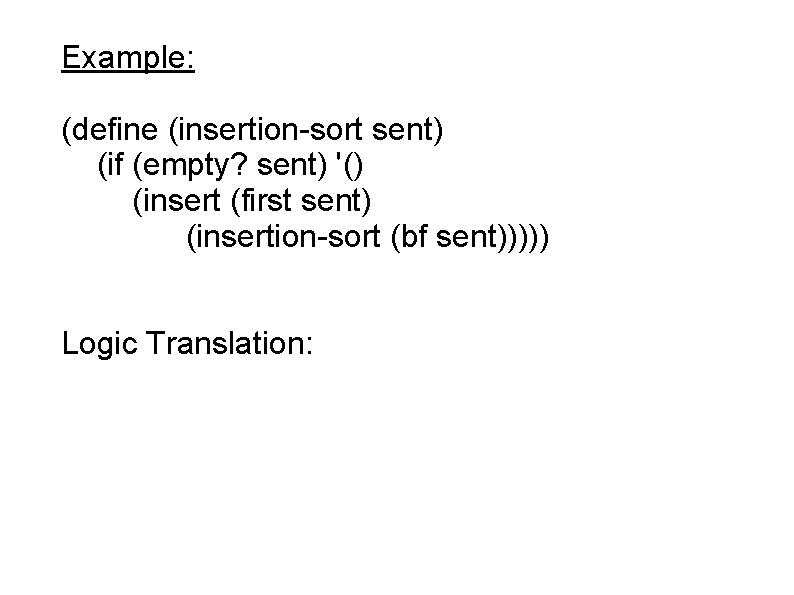
Example: (define (insertion-sort sent) (if (empty? sent) '() (insert (first sent) (insertion-sort (bf sent))))) Logic Translation:
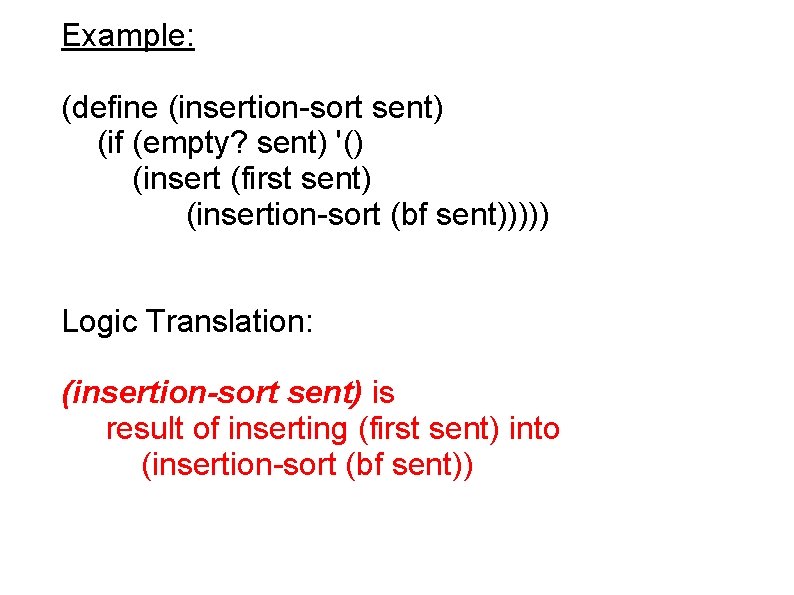
Example: (define (insertion-sort sent) (if (empty? sent) '() (insert (first sent) (insertion-sort (bf sent))))) Logic Translation: (insertion-sort sent) is result of inserting (first sent) into (insertion-sort (bf sent))
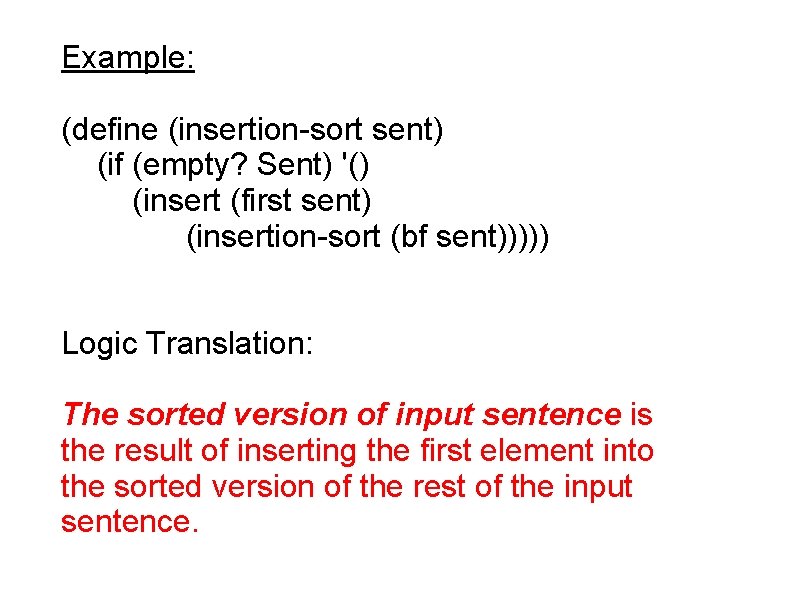
Example: (define (insertion-sort sent) (if (empty? Sent) '() (insert (first sent) (insertion-sort (bf sent))))) Logic Translation: The sorted version of input sentence is the result of inserting the first element into the sorted version of the rest of the input sentence.
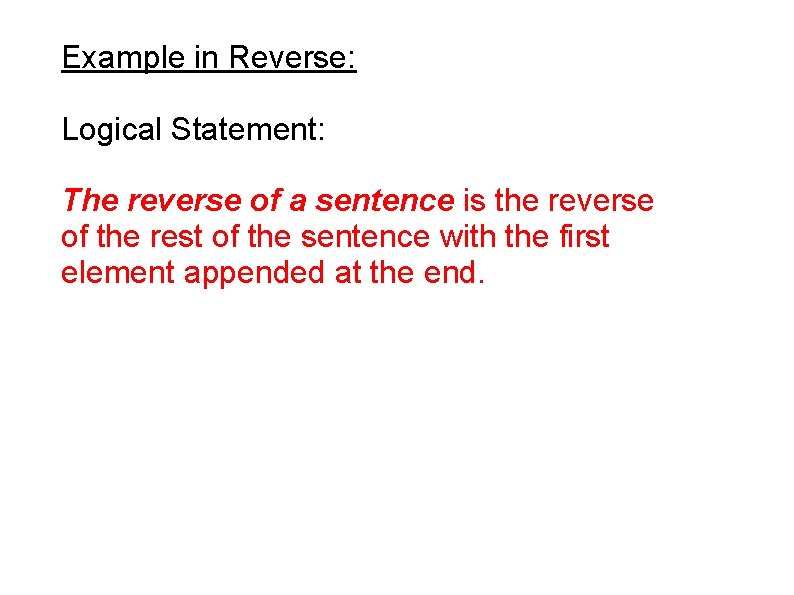
Example in Reverse: Logical Statement: The reverse of a sentence is the reverse of the rest of the sentence with the first element appended at the end.
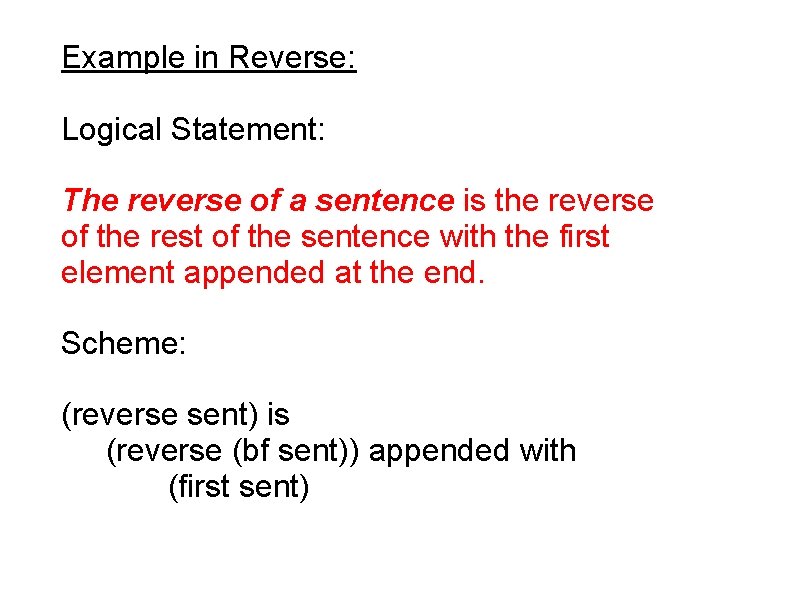
Example in Reverse: Logical Statement: The reverse of a sentence is the reverse of the rest of the sentence with the first element appended at the end. Scheme: (reverse sent) is (reverse (bf sent)) appended with (first sent)
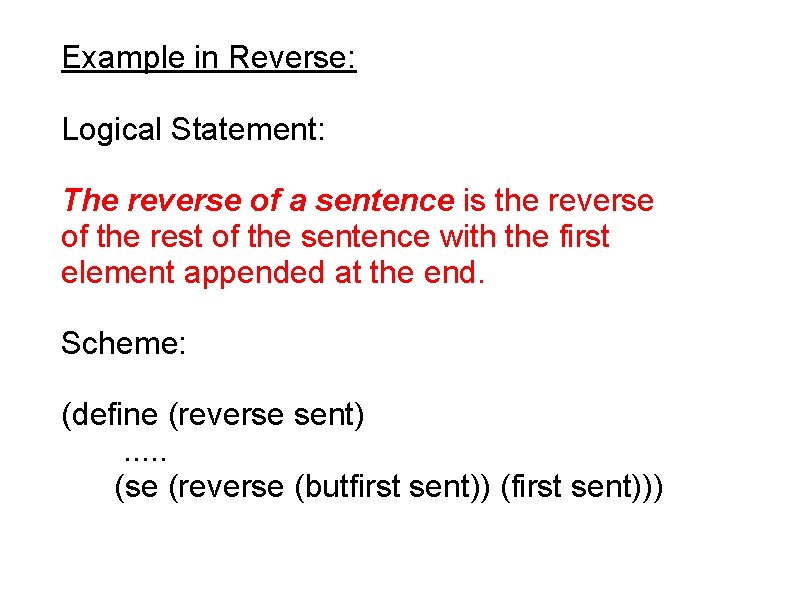
Example in Reverse: Logical Statement: The reverse of a sentence is the reverse of the rest of the sentence with the first element appended at the end. Scheme: (define (reverse sent). . . (se (reverse (butfirst sent)) (first sent)))
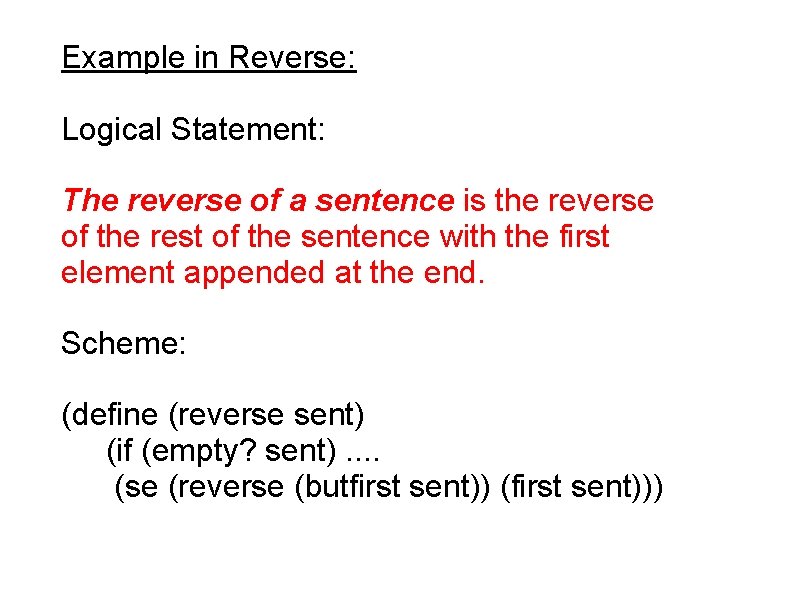
Example in Reverse: Logical Statement: The reverse of a sentence is the reverse of the rest of the sentence with the first element appended at the end. Scheme: (define (reverse sent) (if (empty? sent). . (se (reverse (butfirst sent)) (first sent)))
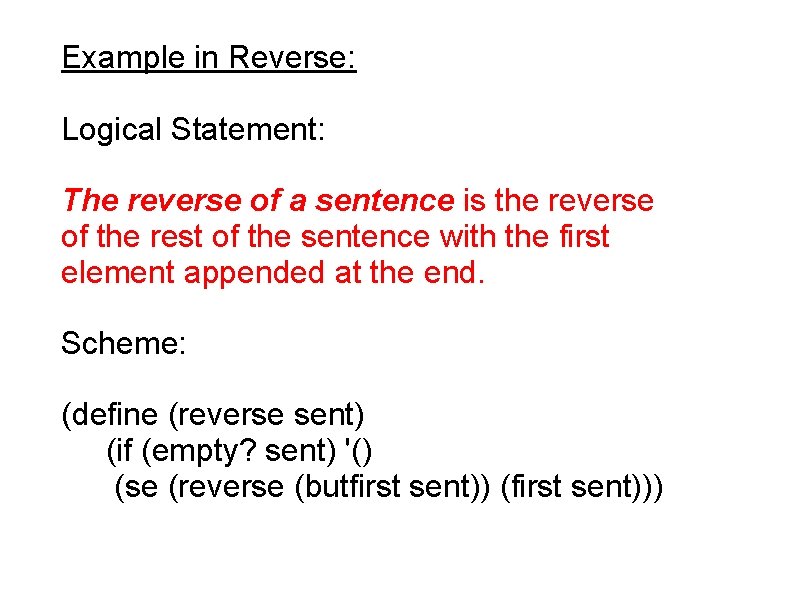
Example in Reverse: Logical Statement: The reverse of a sentence is the reverse of the rest of the sentence with the first element appended at the end. Scheme: (define (reverse sent) (if (empty? sent) '() (se (reverse (butfirst sent)) (first sent)))
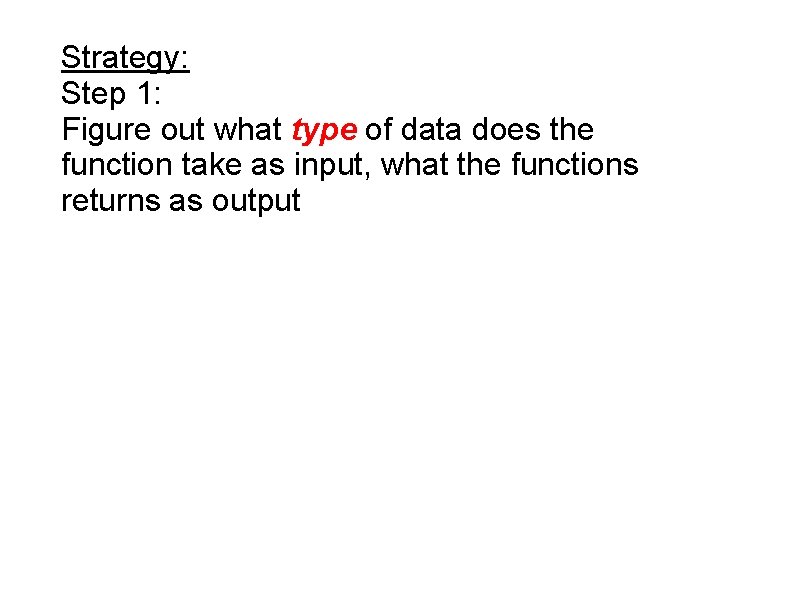
Strategy: Step 1: Figure out what type of data does the function take as input, what the functions returns as output
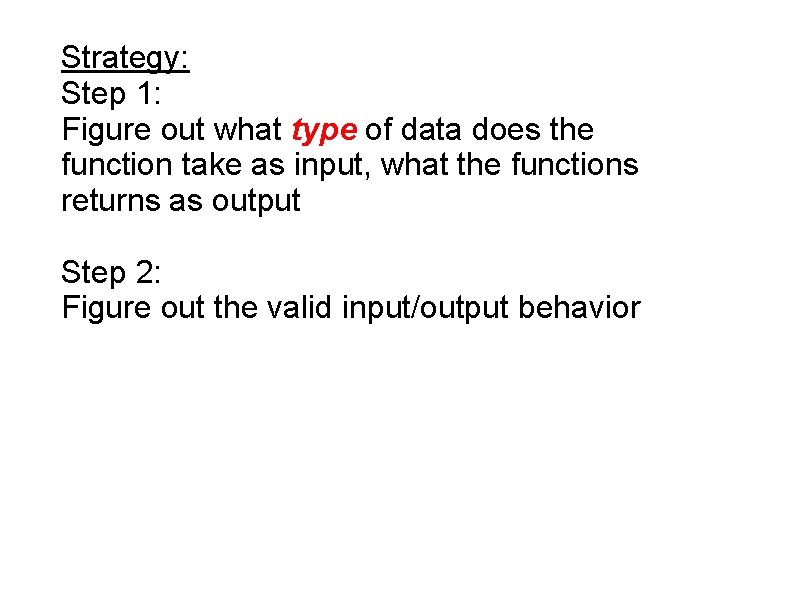
Strategy: Step 1: Figure out what type of data does the function take as input, what the functions returns as output Step 2: Figure out the valid input/output behavior
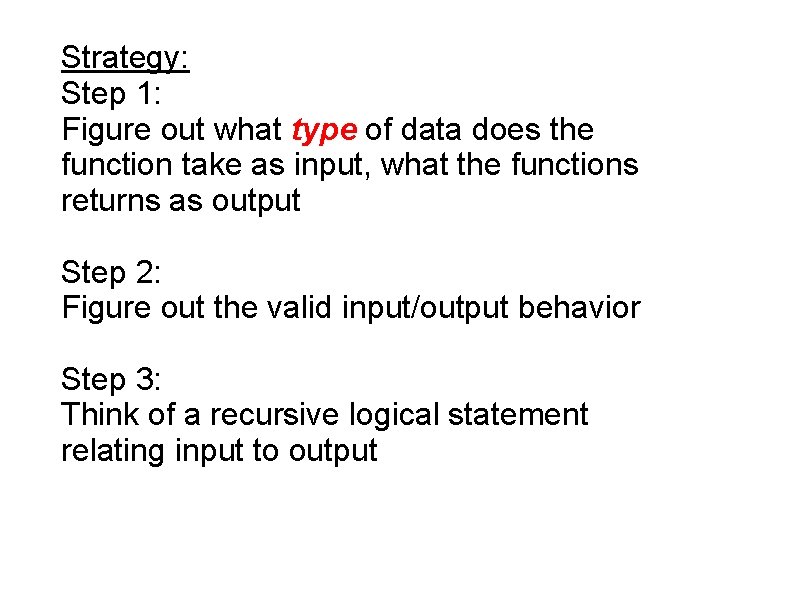
Strategy: Step 1: Figure out what type of data does the function take as input, what the functions returns as output Step 2: Figure out the valid input/output behavior Step 3: Think of a recursive logical statement relating input to output
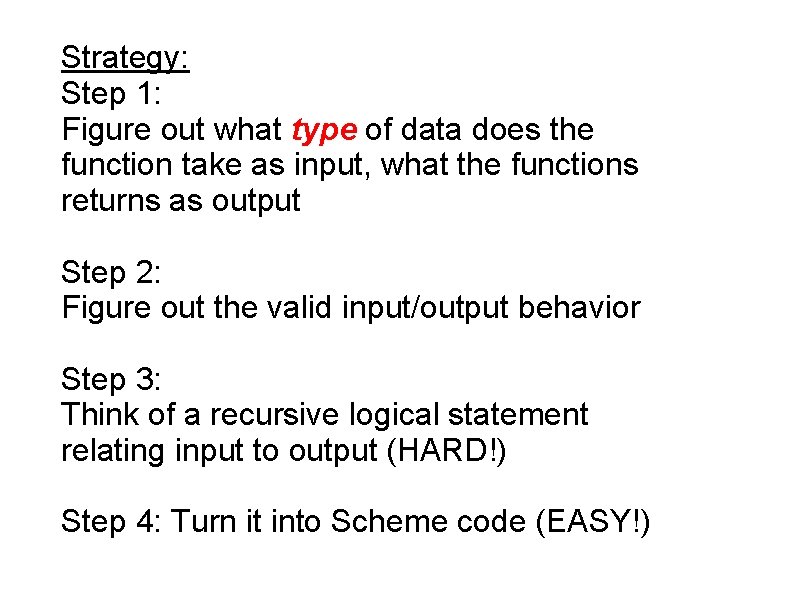
Strategy: Step 1: Figure out what type of data does the function take as input, what the functions returns as output Step 2: Figure out the valid input/output behavior Step 3: Think of a recursive logical statement relating input to output (HARD!) Step 4: Turn it into Scheme code (EASY!)
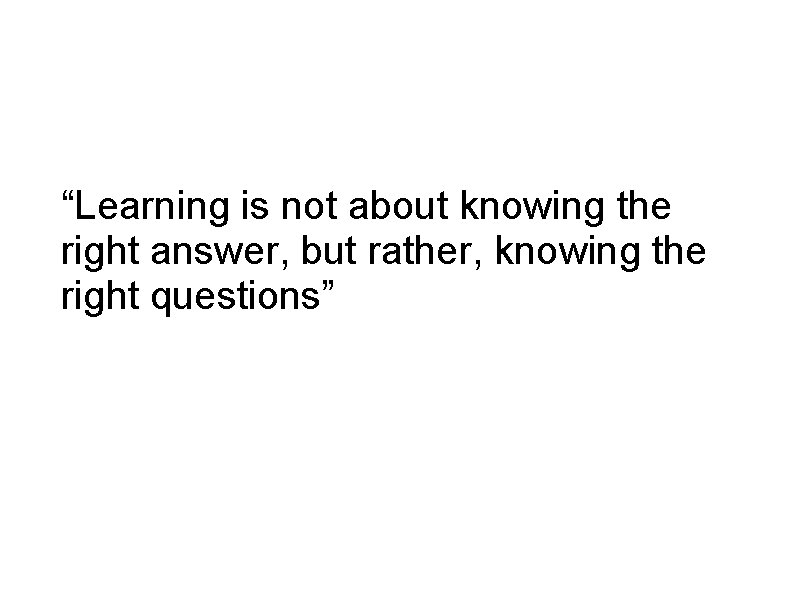
“Learning is not about knowing the right answer, but rather, knowing the right questions”
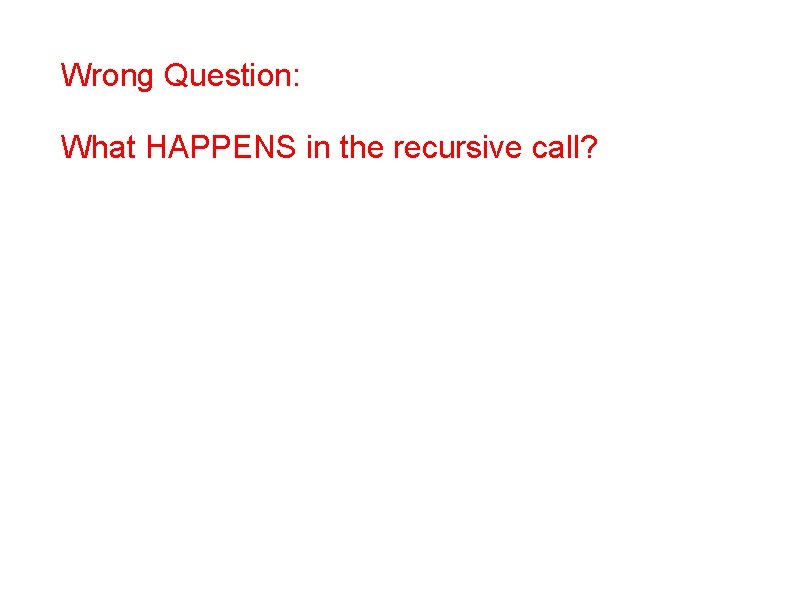
Wrong Question: What HAPPENS in the recursive call?
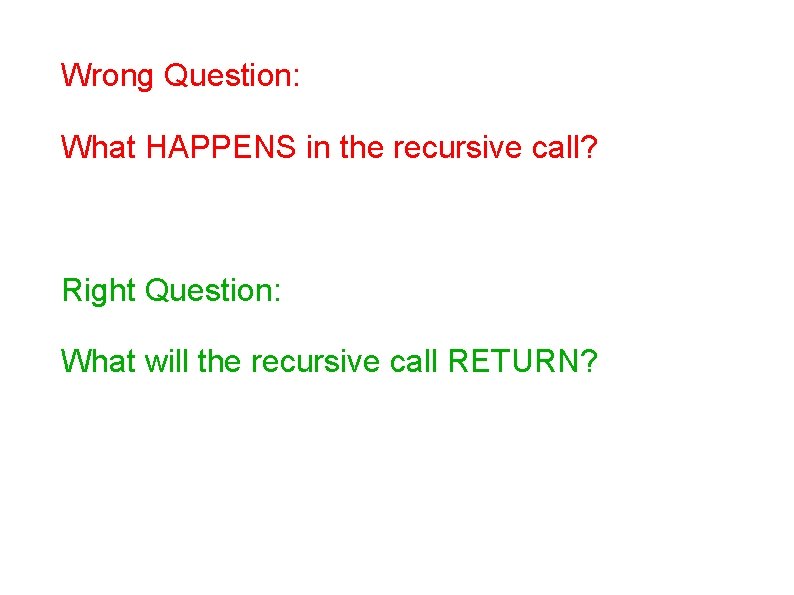
Wrong Question: What HAPPENS in the recursive call? Right Question: What will the recursive call RETURN?