A function with one argument The stars function
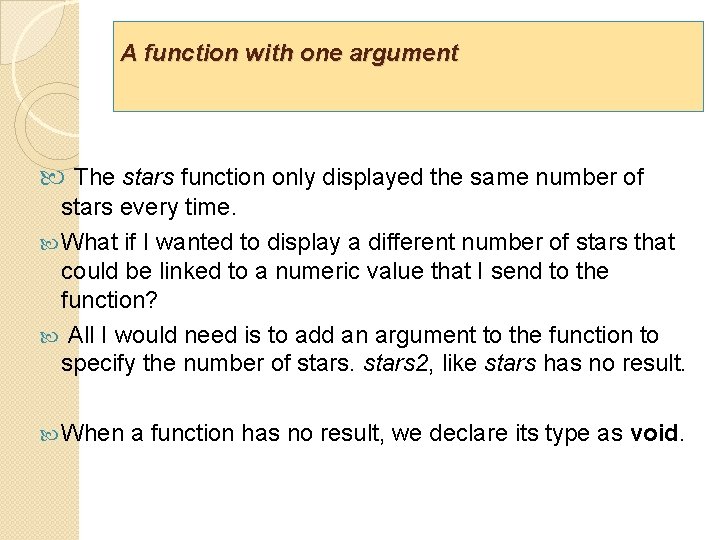
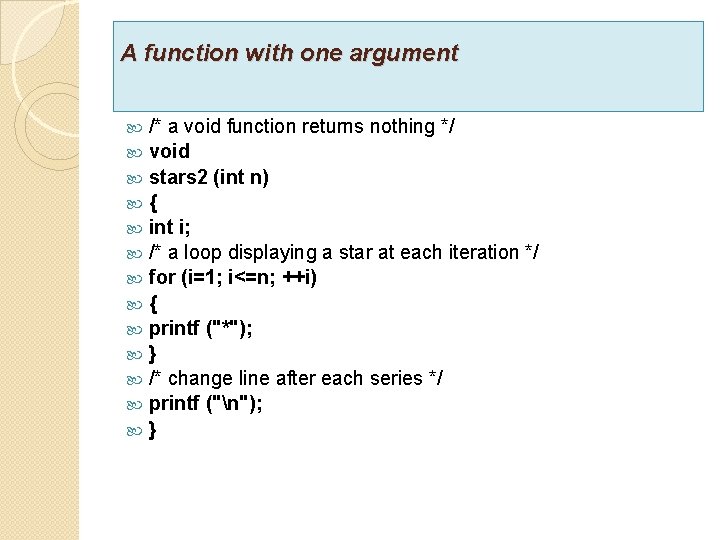
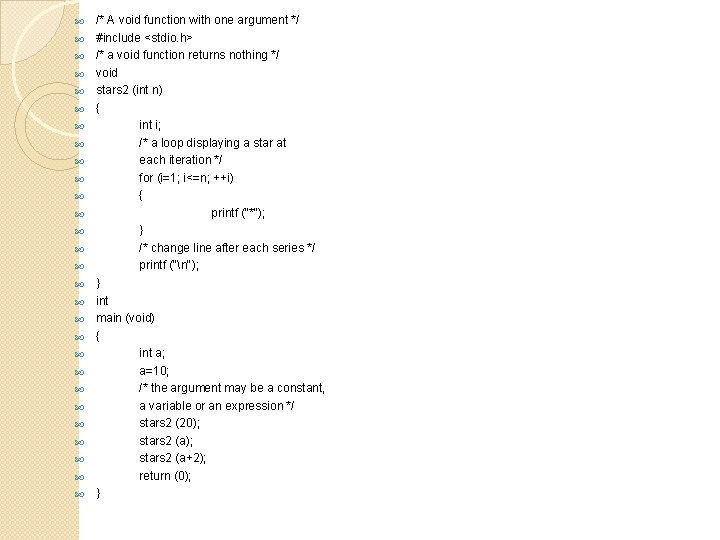
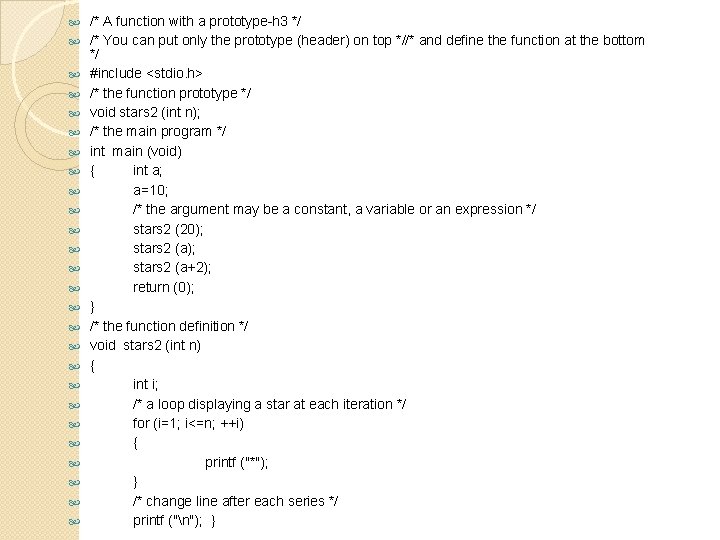
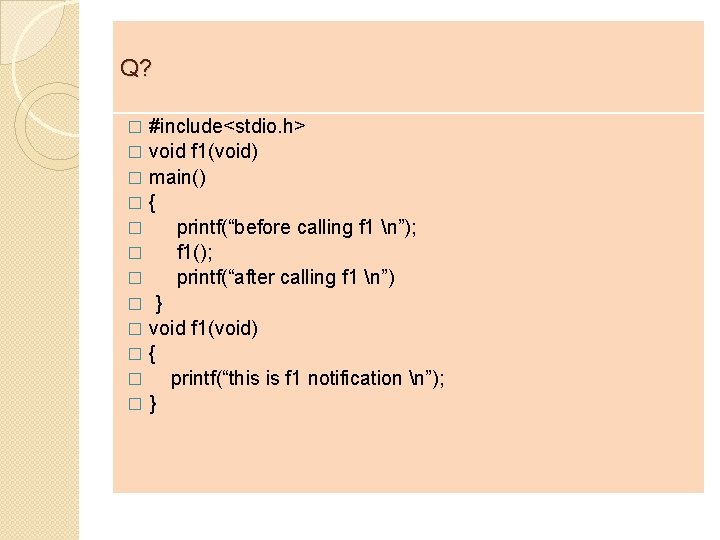
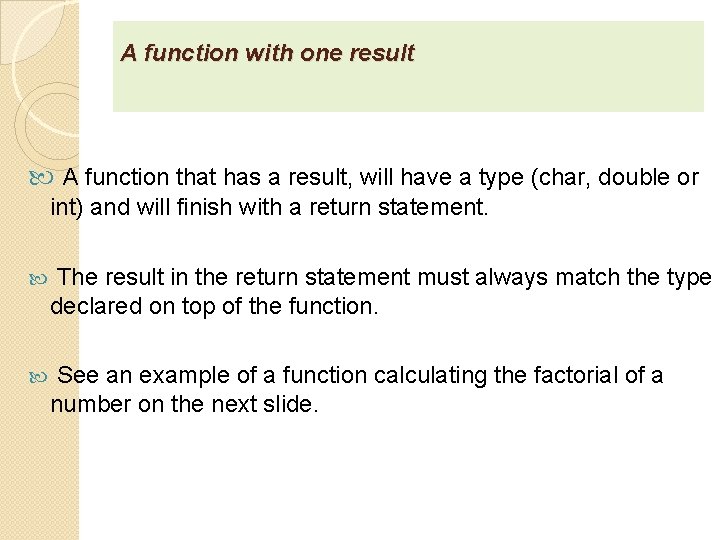
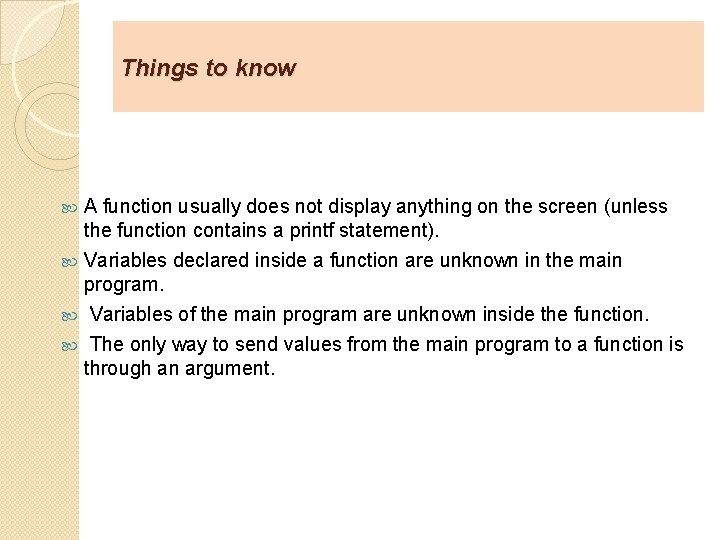
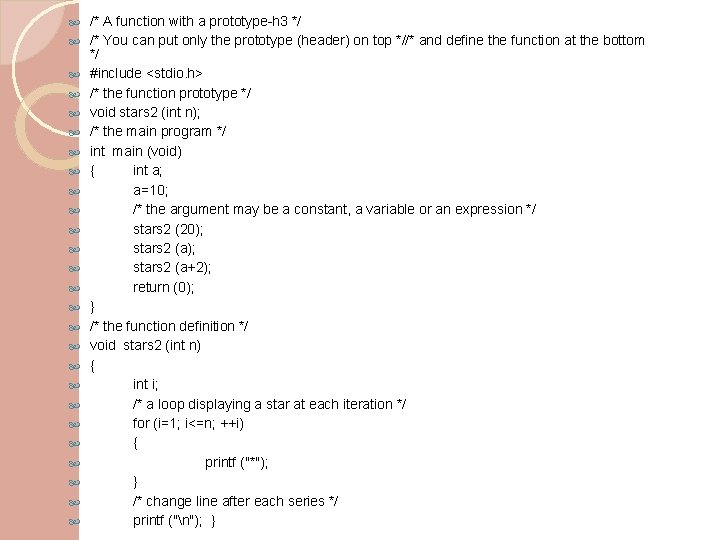
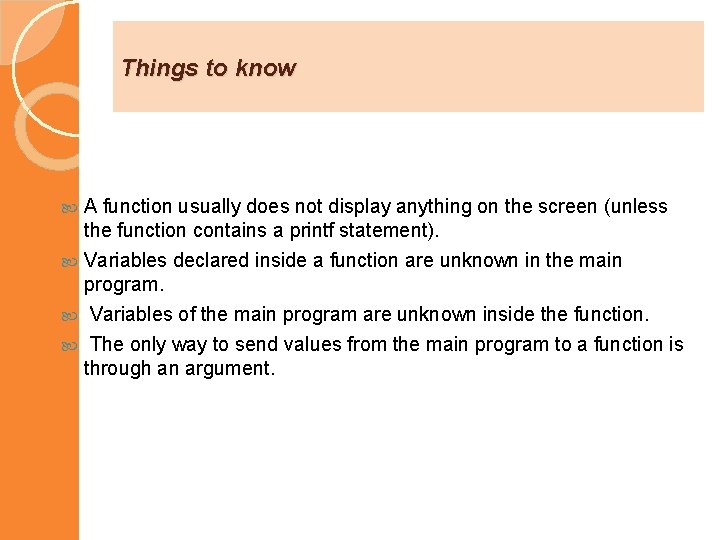
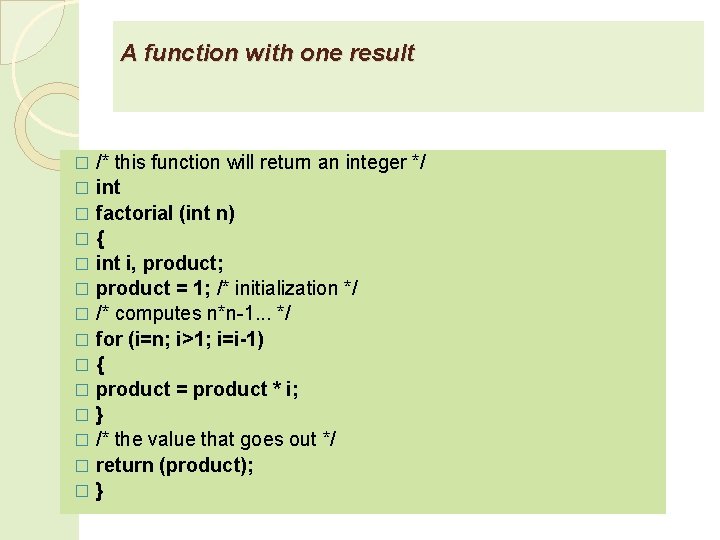
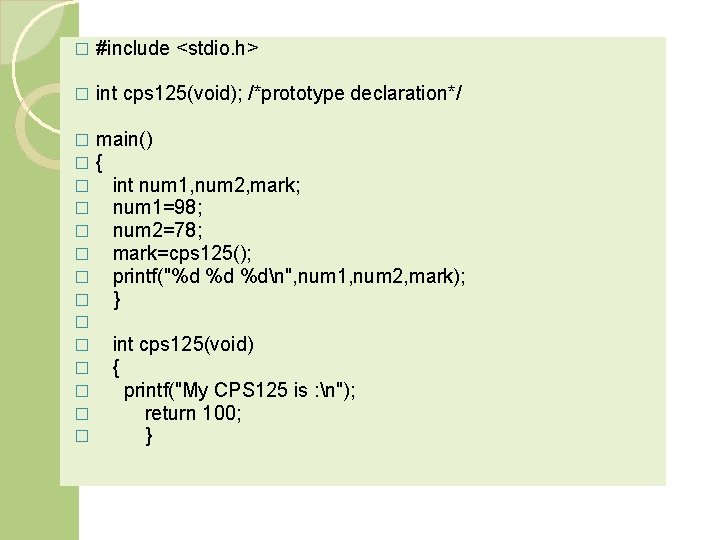
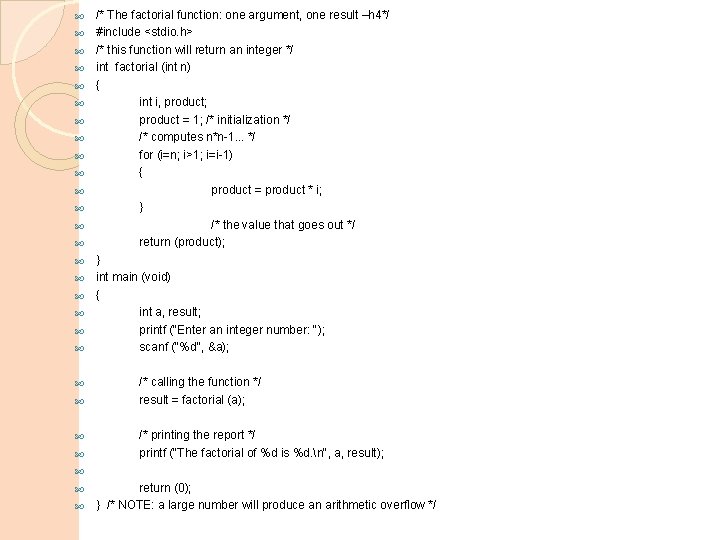
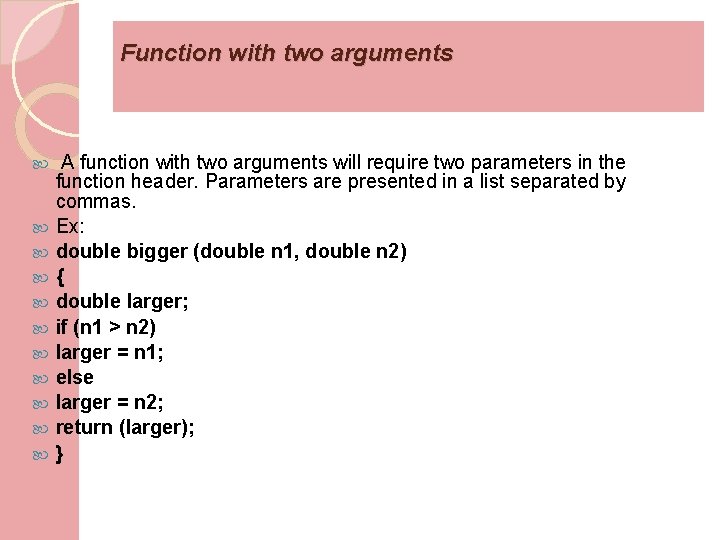
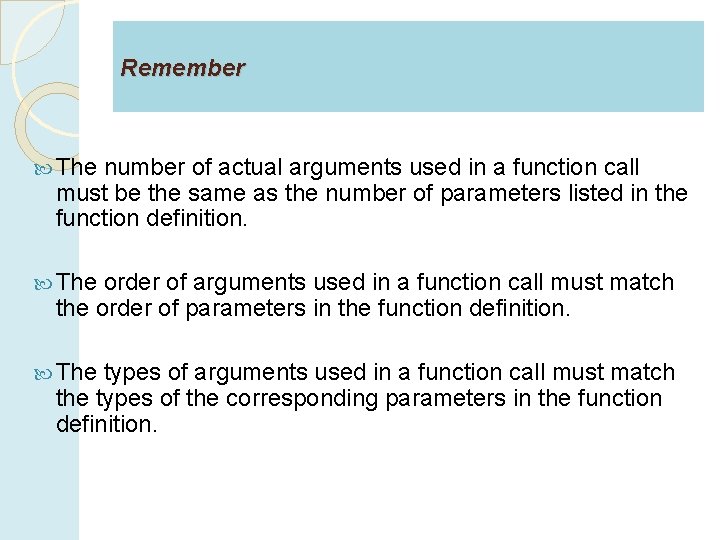
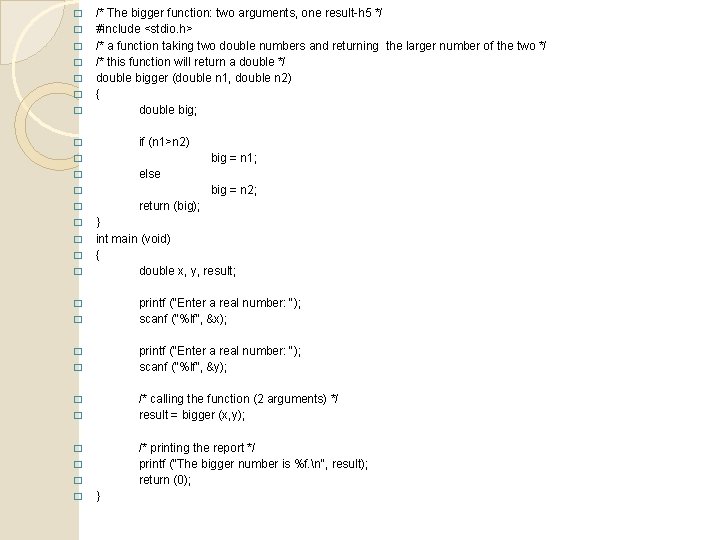
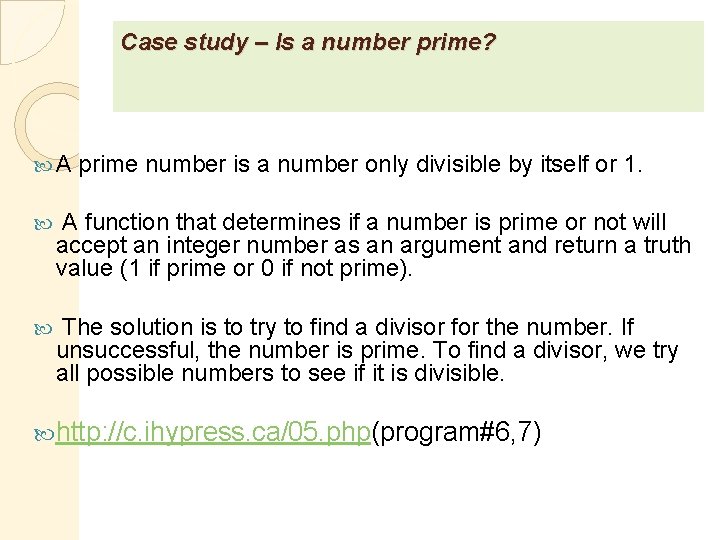
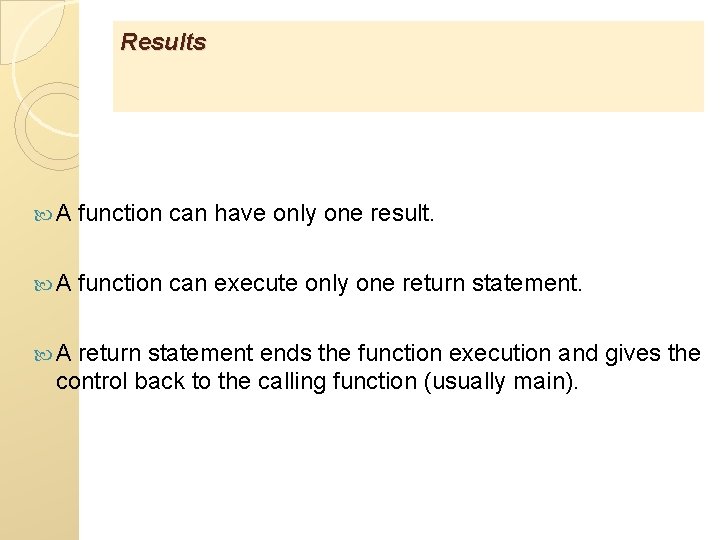
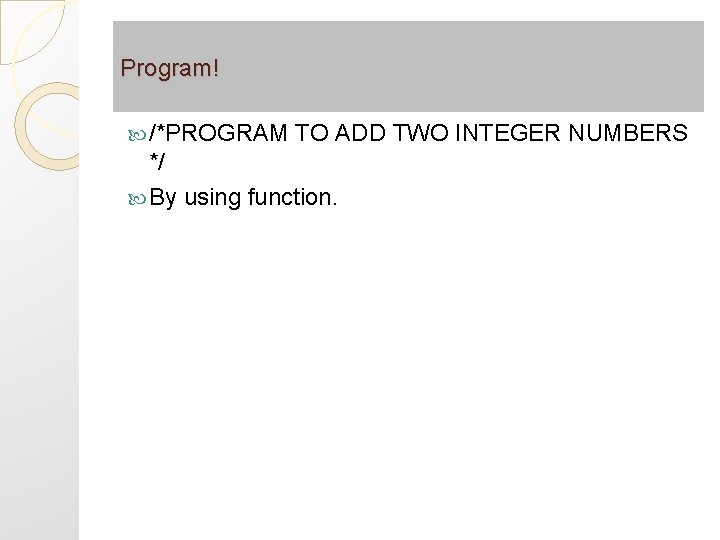
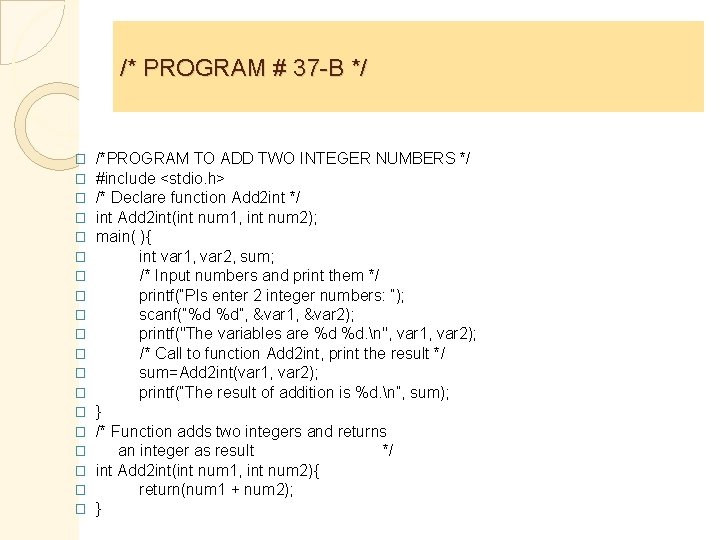
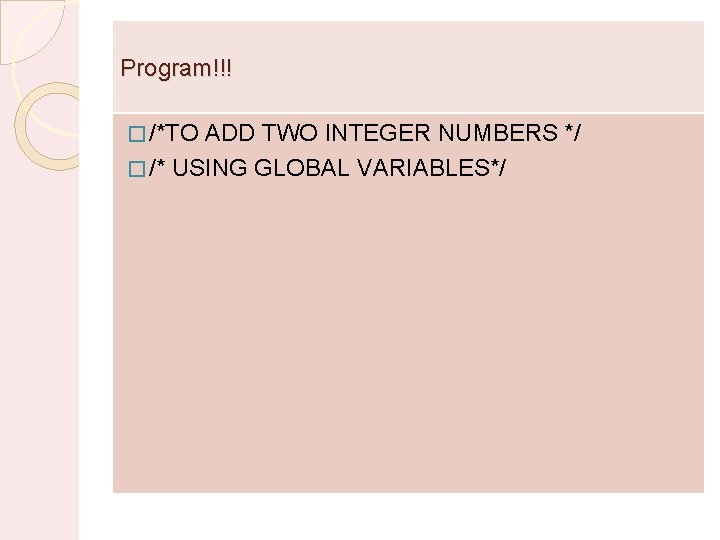
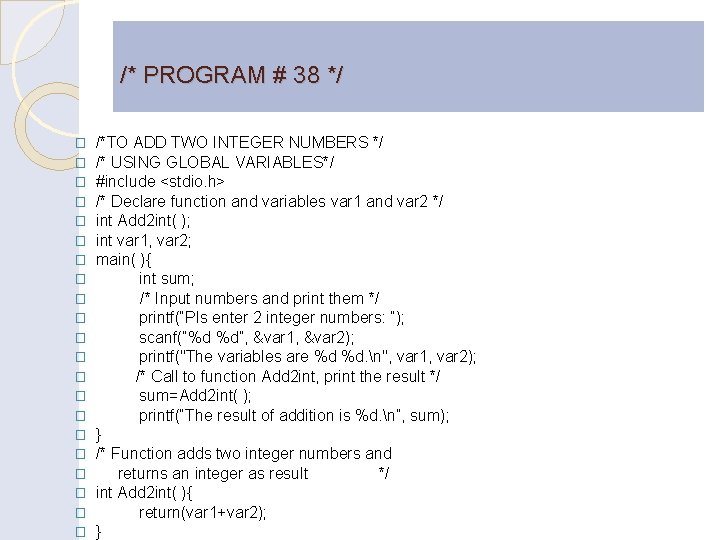
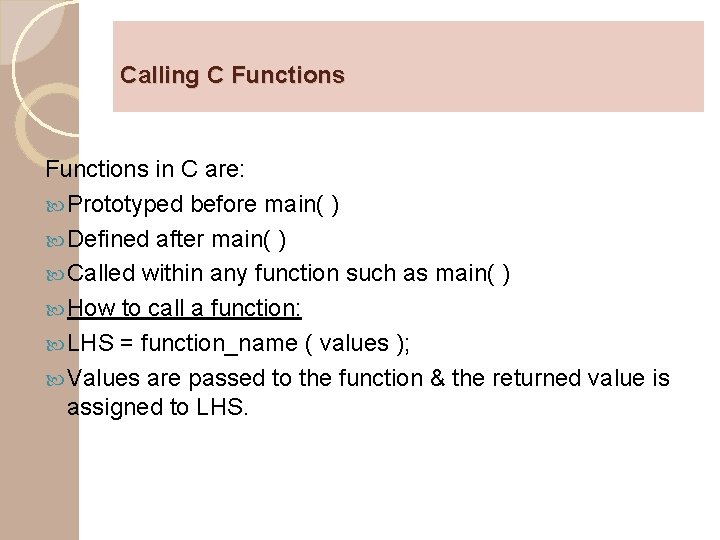
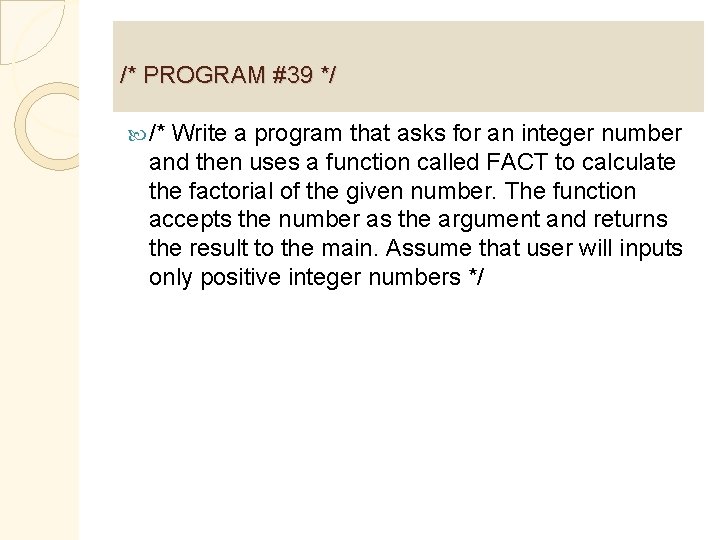
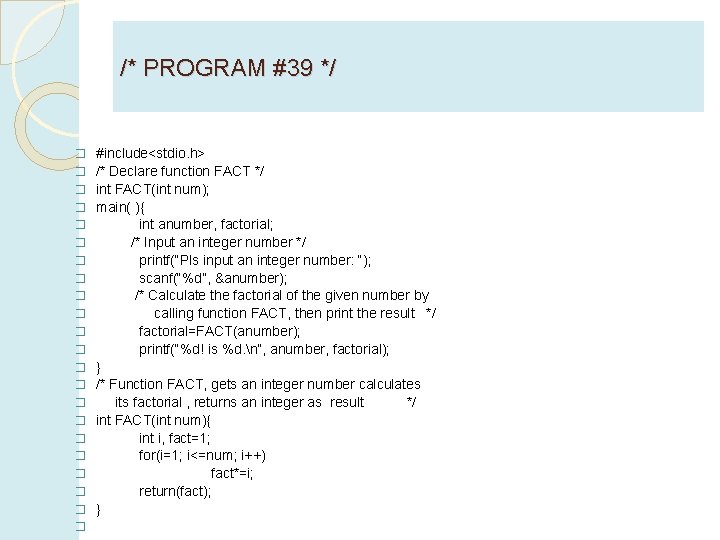
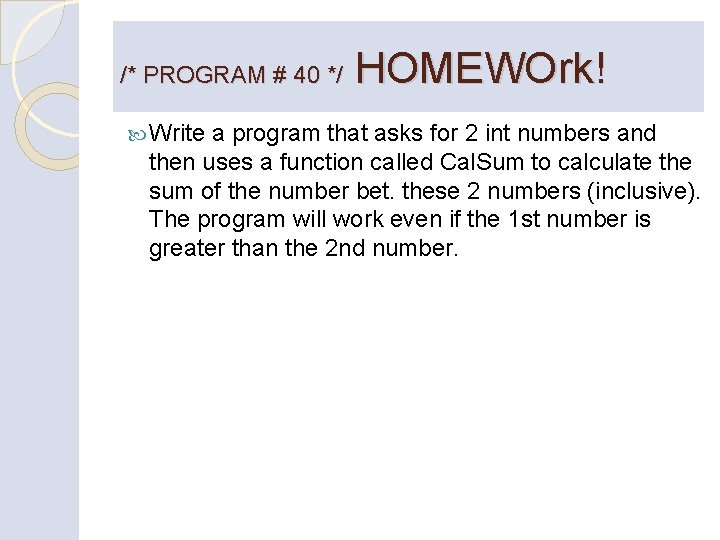
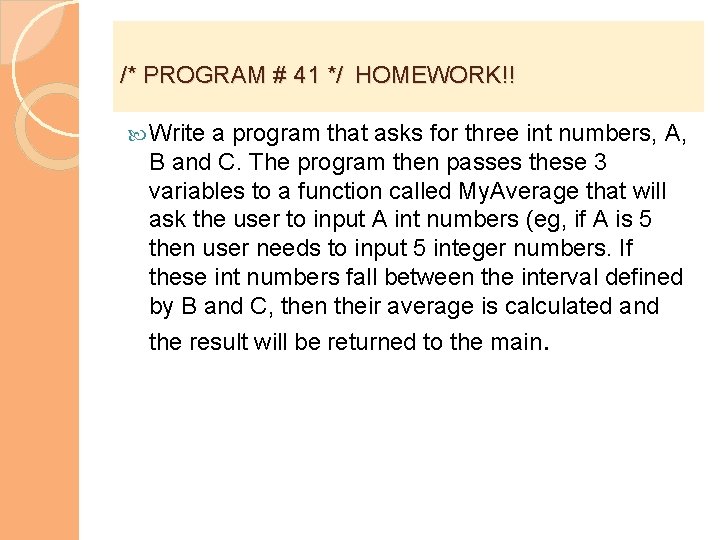
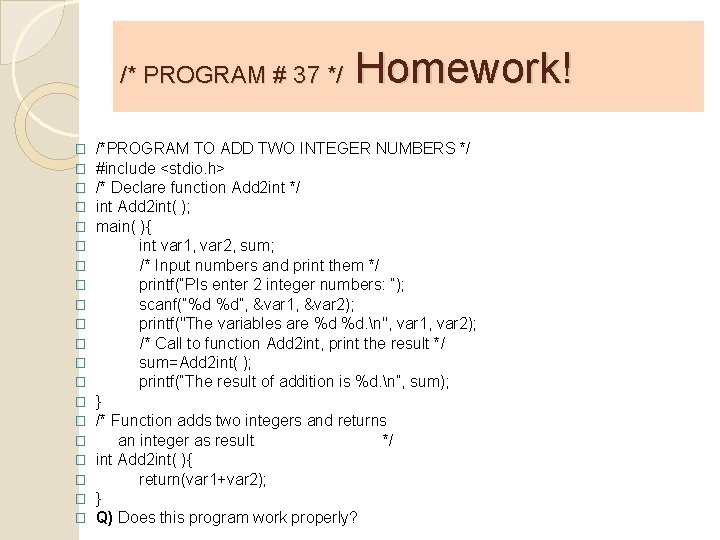
- Slides: 27
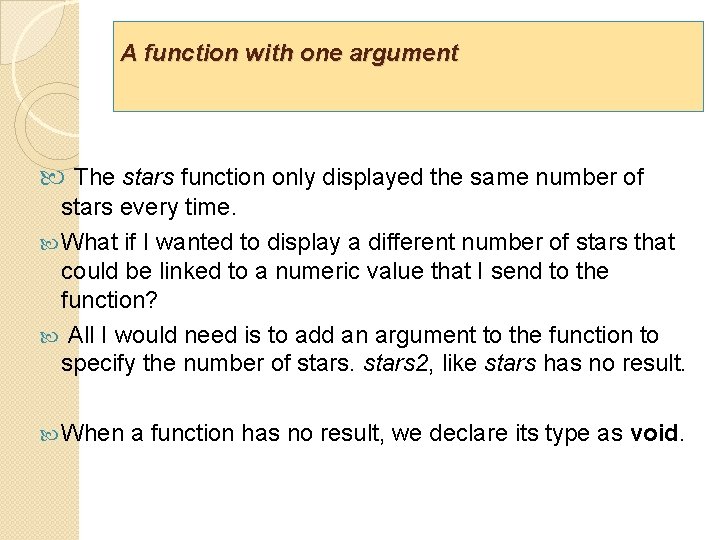
A function with one argument The stars function only displayed the same number of stars every time. What if I wanted to display a different number of stars that could be linked to a numeric value that I send to the function? All I would need is to add an argument to the function to specify the number of stars 2, like stars has no result. When a function has no result, we declare its type as void.
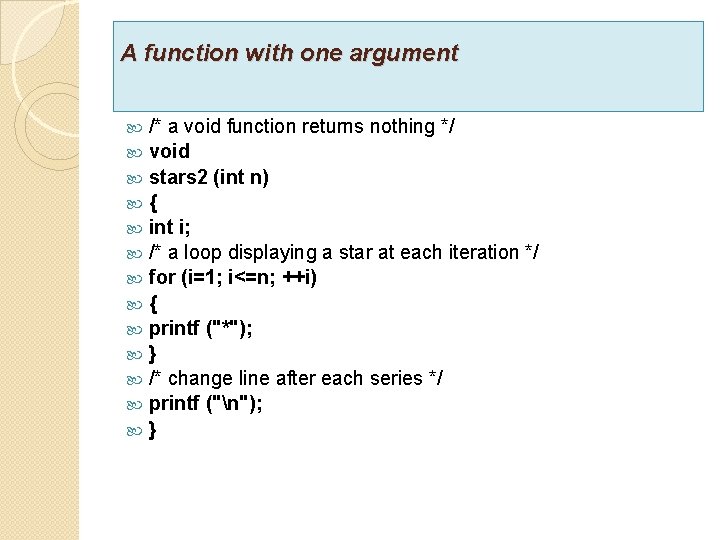
A function with one argument /* a void function returns nothing */ void stars 2 (int n) { int i; /* a loop displaying a star at each iteration */ for (i=1; i<=n; ++i) { printf ("*"); } /* change line after each series */ printf ("n"); }
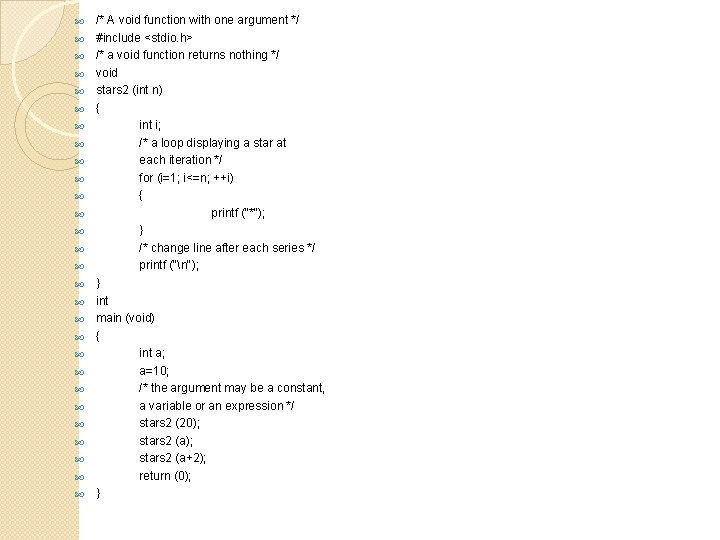
/* A void function with one argument */ #include <stdio. h> /* a void function returns nothing */ void stars 2 (int n) { int i; /* a loop displaying a star at each iteration */ for (i=1; i<=n; ++i) { printf ("*"); } /* change line after each series */ printf ("n"); } int main (void) { int a; a=10; /* the argument may be a constant, a variable or an expression */ stars 2 (20); stars 2 (a+2); return (0); }
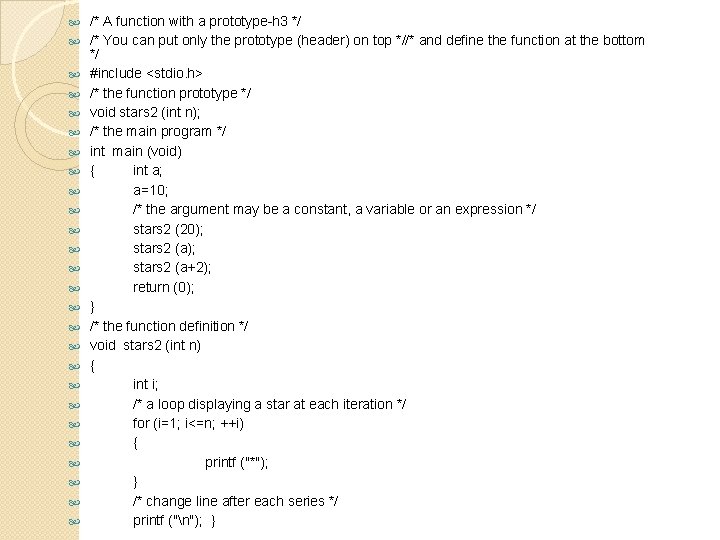
/* A function with a prototype-h 3 */ /* You can put only the prototype (header) on top *//* and define the function at the bottom */ #include <stdio. h> /* the function prototype */ void stars 2 (int n); /* the main program */ int main (void) { int a; a=10; /* the argument may be a constant, a variable or an expression */ stars 2 (20); stars 2 (a+2); return (0); } /* the function definition */ void stars 2 (int n) { int i; /* a loop displaying a star at each iteration */ for (i=1; i<=n; ++i) { printf ("*"); } /* change line after each series */ printf ("n"); }
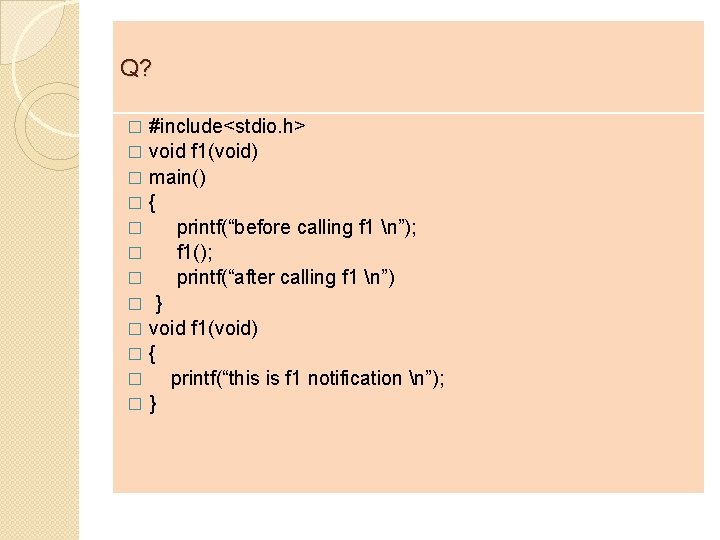
Q? #include<stdio. h> � void f 1(void) � main() �{ � printf(“before calling f 1 n”); � f 1(); � printf(“after calling f 1 n”) � } � void f 1(void) �{ � printf(“this is f 1 notification n”); �} �
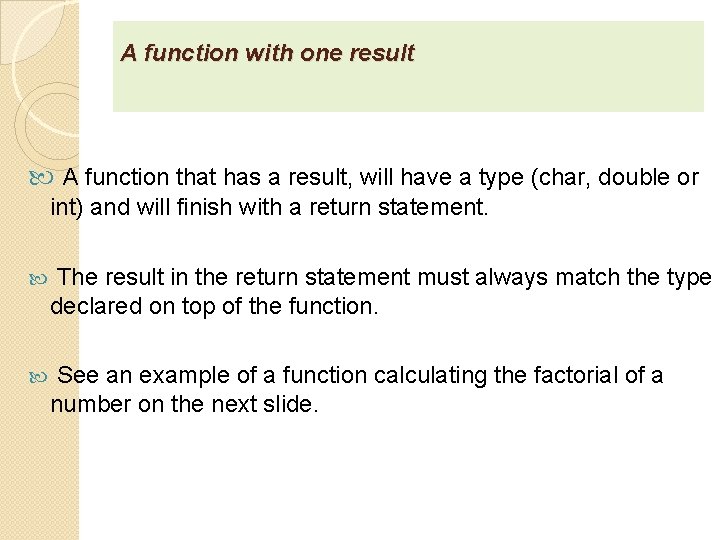
A function with one result A function that has a result, will have a type (char, double or int) and will finish with a return statement. The result in the return statement must always match the type declared on top of the function. See an example of a function calculating the factorial of a number on the next slide.
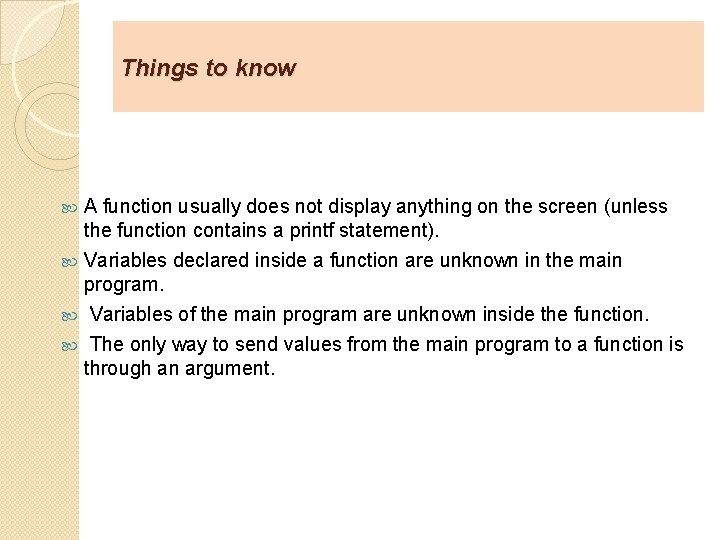
Things to know A function usually does not display anything on the screen (unless the function contains a printf statement). Variables declared inside a function are unknown in the main program. Variables of the main program are unknown inside the function. The only way to send values from the main program to a function is through an argument.
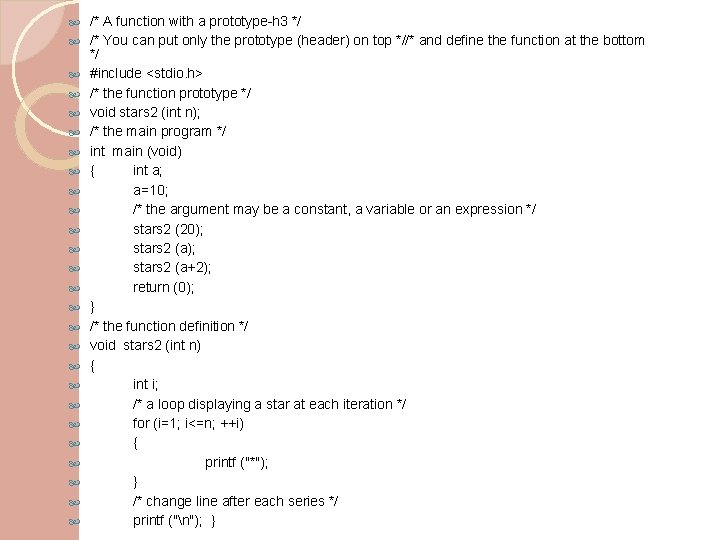
/* A function with a prototype-h 3 */ /* You can put only the prototype (header) on top *//* and define the function at the bottom */ #include <stdio. h> /* the function prototype */ void stars 2 (int n); /* the main program */ int main (void) { int a; a=10; /* the argument may be a constant, a variable or an expression */ stars 2 (20); stars 2 (a+2); return (0); } /* the function definition */ void stars 2 (int n) { int i; /* a loop displaying a star at each iteration */ for (i=1; i<=n; ++i) { printf ("*"); } /* change line after each series */ printf ("n"); }
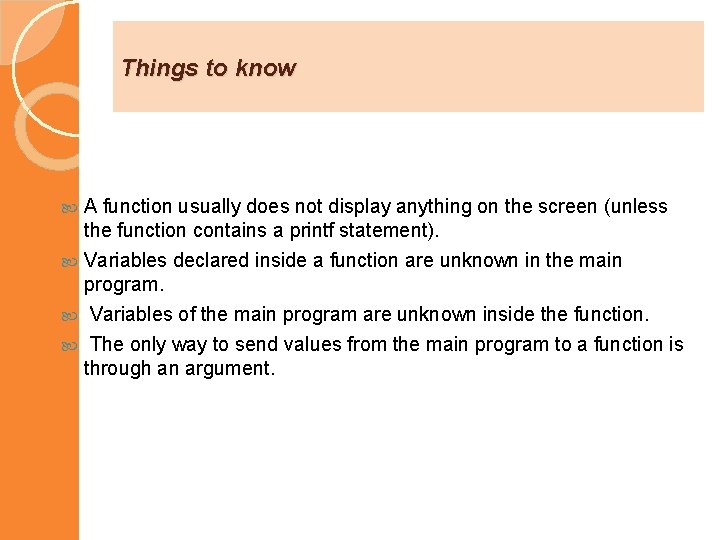
Things to know A function usually does not display anything on the screen (unless the function contains a printf statement). Variables declared inside a function are unknown in the main program. Variables of the main program are unknown inside the function. The only way to send values from the main program to a function is through an argument.
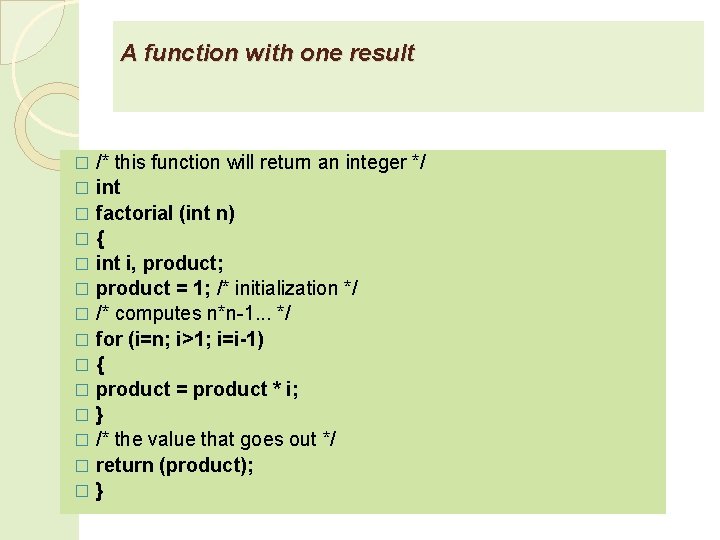
A function with one result /* this function will return an integer */ � int � factorial (int n) �{ � int i, product; � product = 1; /* initialization */ � /* computes n*n-1. . . */ � for (i=n; i>1; i=i-1) �{ � product = product * i; �} � /* the value that goes out */ � return (product); �} �
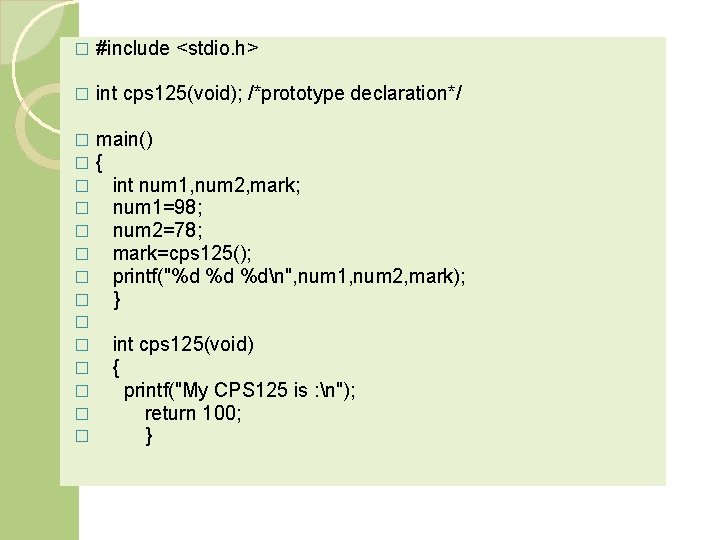
� #include <stdio. h> � int cps 125(void); /*prototype declaration*/ � � � � main() { int num 1, num 2, mark; num 1=98; num 2=78; mark=cps 125(); printf("%d %d %dn", num 1, num 2, mark); } int cps 125(void) { printf("My CPS 125 is : n"); return 100; }
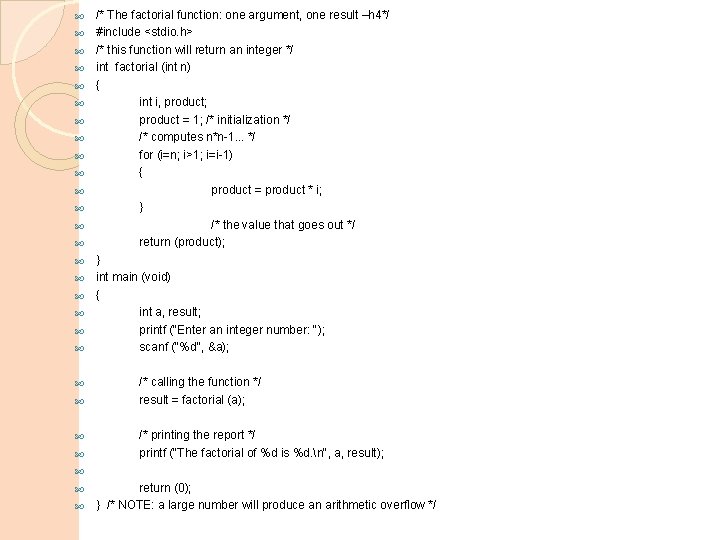
/* The factorial function: one argument, one result –h 4*/ #include <stdio. h> /* this function will return an integer */ int factorial (int n) { int i, product; product = 1; /* initialization */ /* computes n*n-1. . . */ for (i=n; i>1; i=i-1) { product = product * i; } /* the value that goes out */ return (product); } int main (void) { int a, result; printf ("Enter an integer number: "); scanf ("%d", &a); /* calling the function */ result = factorial (a); /* printing the report */ printf ("The factorial of %d is %d. n", a, result); return (0); } /* NOTE: a large number will produce an arithmetic overflow */
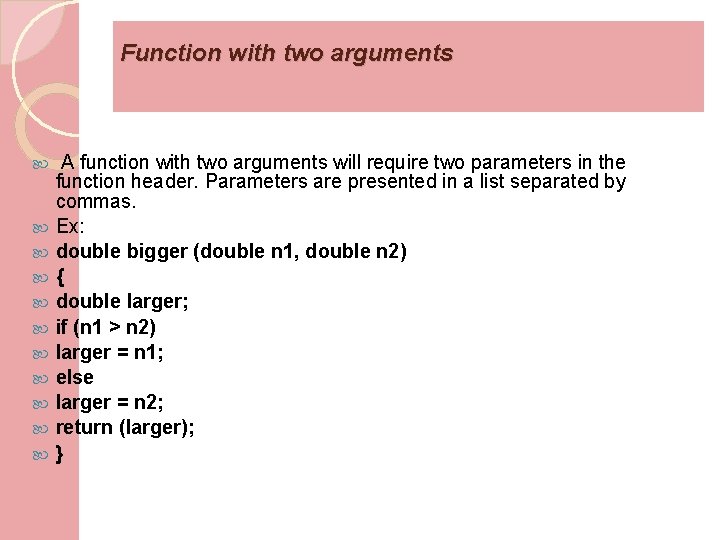
Function with two arguments A function with two arguments will require two parameters in the function header. Parameters are presented in a list separated by commas. Ex: double bigger (double n 1, double n 2) { double larger; if (n 1 > n 2) larger = n 1; else larger = n 2; return (larger); }
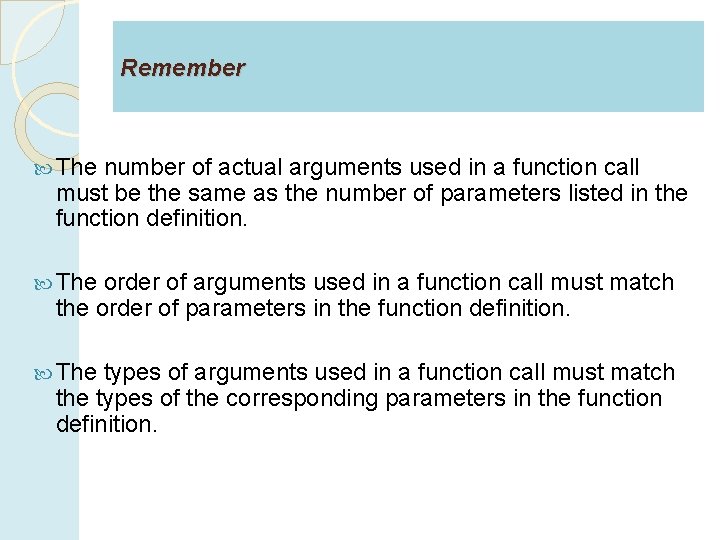
Remember The number of actual arguments used in a function call must be the same as the number of parameters listed in the function definition. The order of arguments used in a function call must match the order of parameters in the function definition. The types of arguments used in a function call must match the types of the corresponding parameters in the function definition.
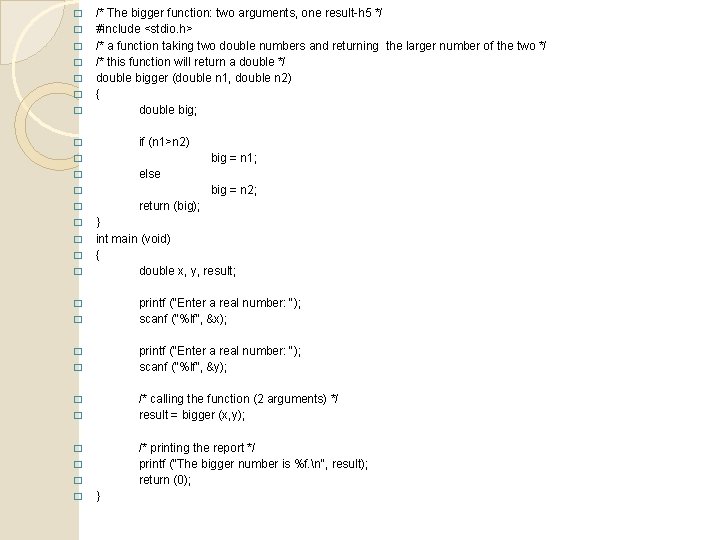
� � � � /* The bigger function: two arguments, one result-h 5 */ #include <stdio. h> /* a function taking two double numbers and returning the larger number of the two */ /* this function will return a double */ double bigger (double n 1, double n 2) { double big; if (n 1>n 2) � big = n 1; � else � big = n 2; � return (big); � � � } int main (void) { double x, y, result; printf ("Enter a real number: "); scanf ("%lf", &x); � � printf ("Enter a real number: "); scanf ("%lf", &y); � � /* calling the function (2 arguments) */ result = bigger (x, y); � � /* printing the report */ printf ("The bigger number is %f. n", result); return (0); � � }
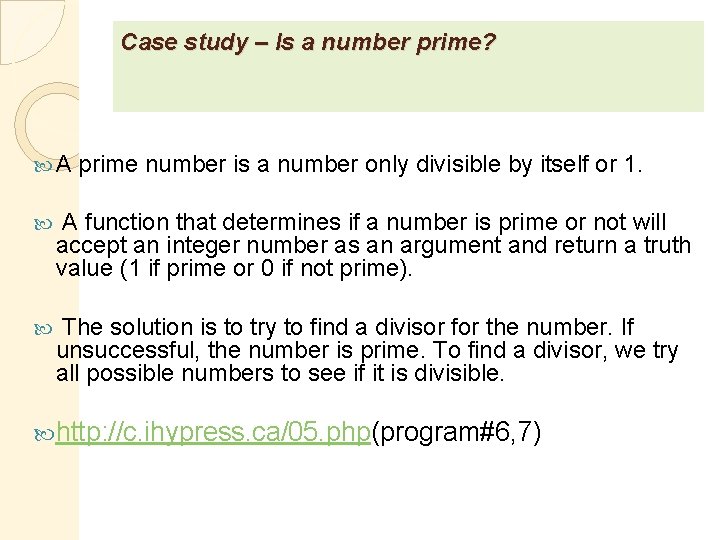
Case study – Is a number prime? A prime number is a number only divisible by itself or 1. A function that determines if a number is prime or not will accept an integer number as an argument and return a truth value (1 if prime or 0 if not prime). The solution is to try to find a divisor for the number. If unsuccessful, the number is prime. To find a divisor, we try all possible numbers to see if it is divisible. http: //c. ihypress. ca/05. php(program#6, 7)
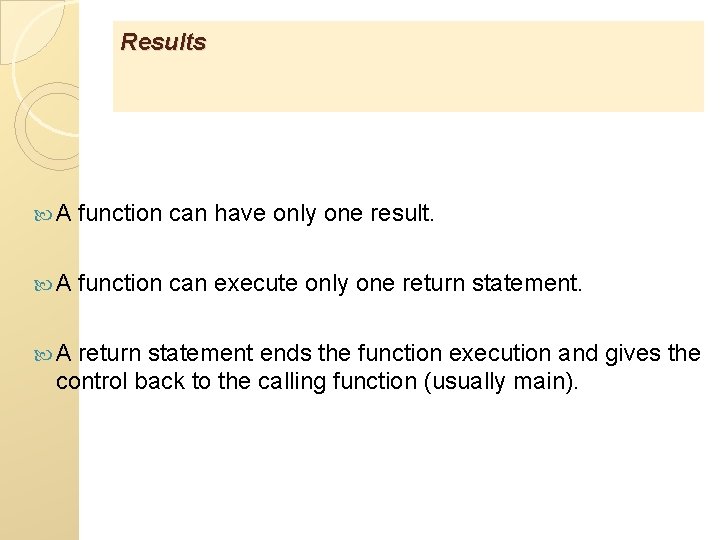
Results A function can have only one result. A function can execute only one return statement. A return statement ends the function execution and gives the control back to the calling function (usually main).
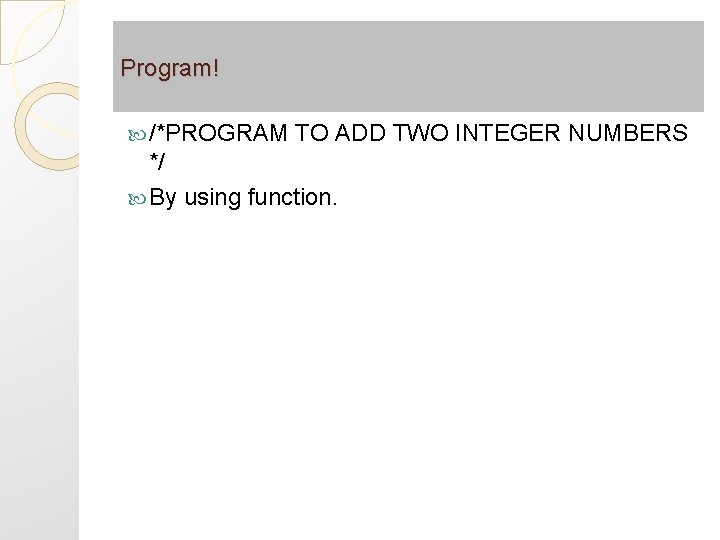
Program! /*PROGRAM TO ADD TWO INTEGER NUMBERS */ By using function.
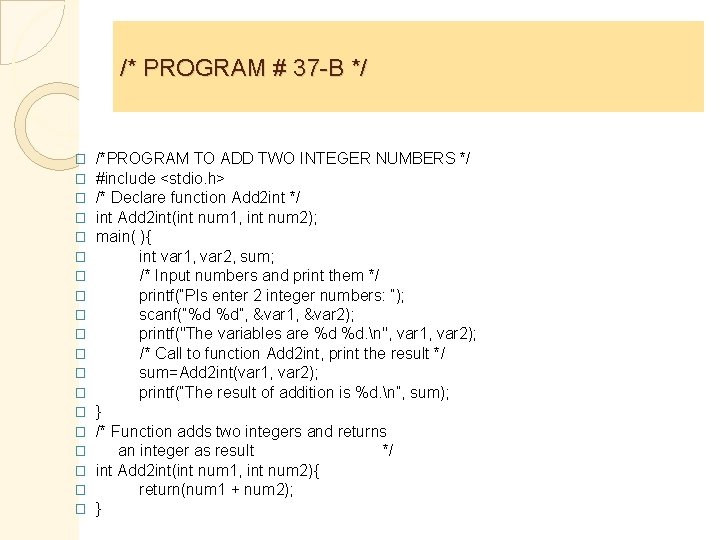
/* PROGRAM # 37 -B */ � � � � � /*PROGRAM TO ADD TWO INTEGER NUMBERS */ #include <stdio. h> /* Declare function Add 2 int */ int Add 2 int(int num 1, int num 2); main( ){ int var 1, var 2, sum; /* Input numbers and print them */ printf(“Pls enter 2 integer numbers: “); scanf(“%d %d”, &var 1, &var 2); printf("The variables are %d %d. n", var 1, var 2); /* Call to function Add 2 int, print the result */ sum=Add 2 int(var 1, var 2); printf(“The result of addition is %d. n”, sum); } /* Function adds two integers and returns an integer as result */ int Add 2 int(int num 1, int num 2){ return(num 1 + num 2); }
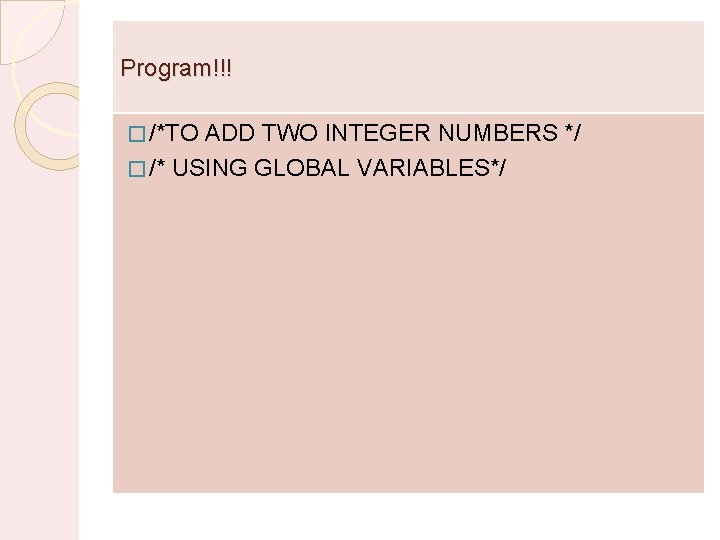
Program!!! � /*TO ADD TWO INTEGER NUMBERS */ � /* USING GLOBAL VARIABLES*/
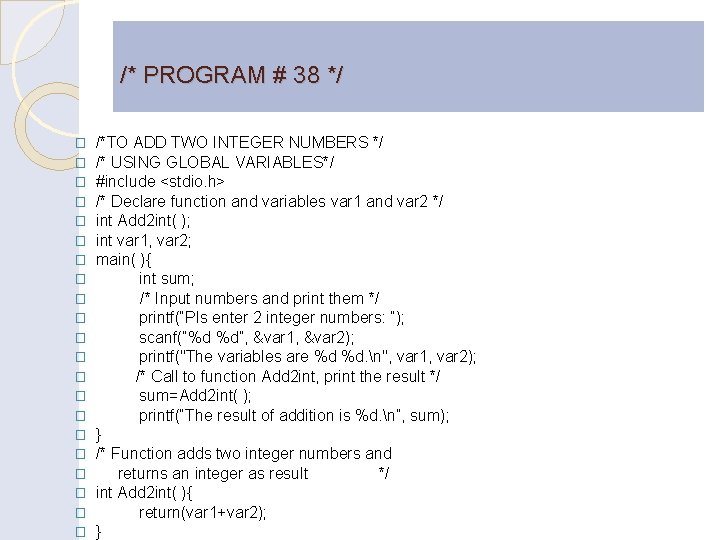
/* PROGRAM # 38 */ � � � � � � /*TO ADD TWO INTEGER NUMBERS */ /* USING GLOBAL VARIABLES*/ #include <stdio. h> /* Declare function and variables var 1 and var 2 */ int Add 2 int( ); int var 1, var 2; main( ){ int sum; /* Input numbers and print them */ printf(“Pls enter 2 integer numbers: “); scanf(“%d %d”, &var 1, &var 2); printf("The variables are %d %d. n", var 1, var 2); /* Call to function Add 2 int, print the result */ sum=Add 2 int( ); printf(“The result of addition is %d. n”, sum); } /* Function adds two integer numbers and returns an integer as result */ int Add 2 int( ){ return(var 1+var 2); }
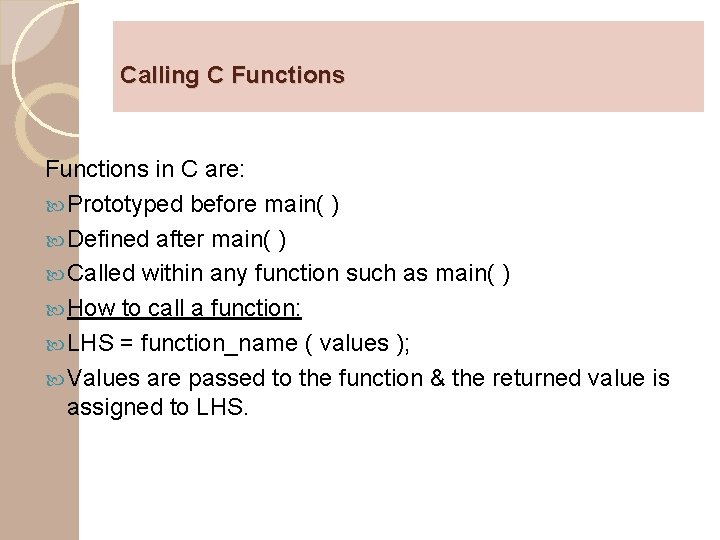
Calling C Functions in C are: Prototyped before main( ) Defined after main( ) Called within any function such as main( ) How to call a function: LHS = function_name ( values ); Values are passed to the function & the returned value is assigned to LHS.
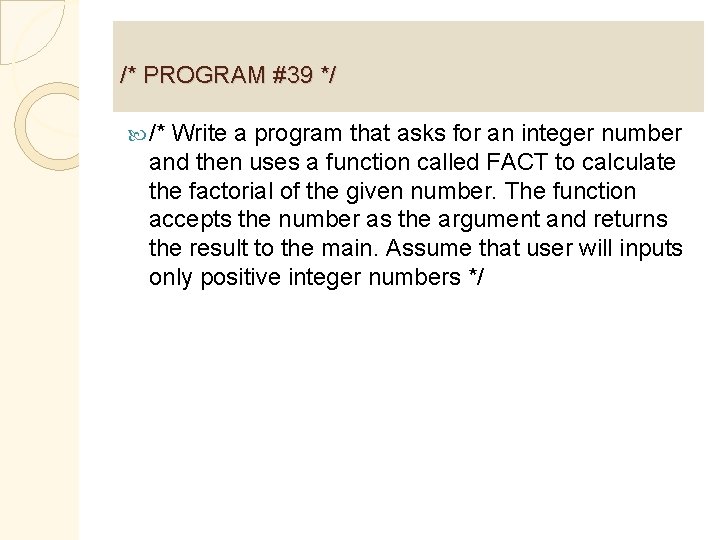
/* PROGRAM #39 */ /* Write a program that asks for an integer number and then uses a function called FACT to calculate the factorial of the given number. The function accepts the number as the argument and returns the result to the main. Assume that user will inputs only positive integer numbers */
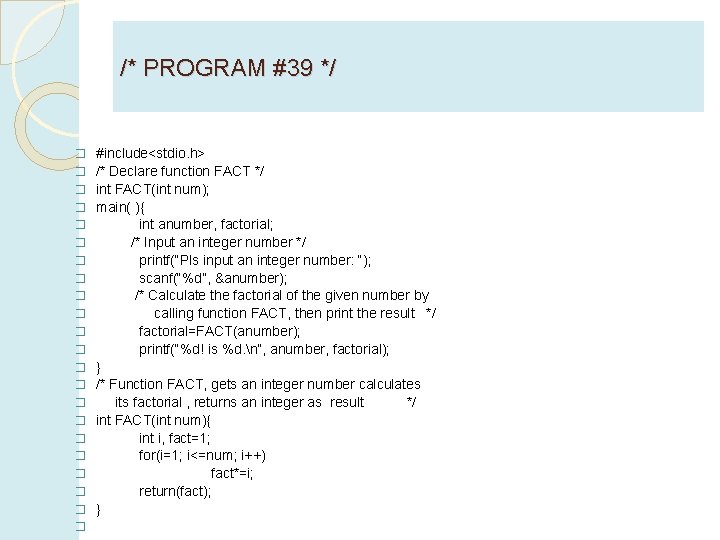
/* PROGRAM #39 */ � #include<stdio. h> � /* Declare function FACT */ � int FACT(int num); � main( ){ int anumber, factorial; /* Input an integer number */ printf(“Pls input an integer number: “); scanf(“%d”, &anumber); /* Calculate the factorial of the given number by calling function FACT, then print the result */ factorial=FACT(anumber); printf(“%d! is %d. n”, anumber, factorial); � � � � � } � /* Function FACT, gets an integer number calculates � � � � its factorial , returns an integer as result int FACT(int num){ int i, fact=1; for(i=1; i<=num; i++) fact*=i; return(fact); } */
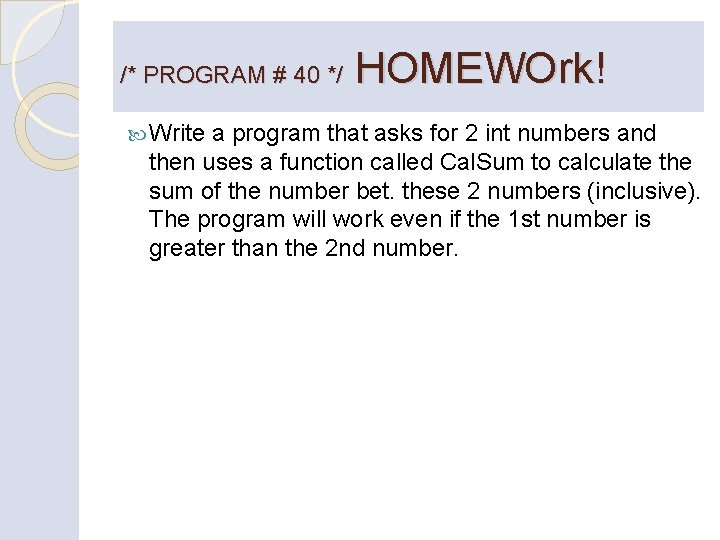
/* PROGRAM # 40 */ Write HOMEWOrk! a program that asks for 2 int numbers and then uses a function called Cal. Sum to calculate the sum of the number bet. these 2 numbers (inclusive). The program will work even if the 1 st number is greater than the 2 nd number.
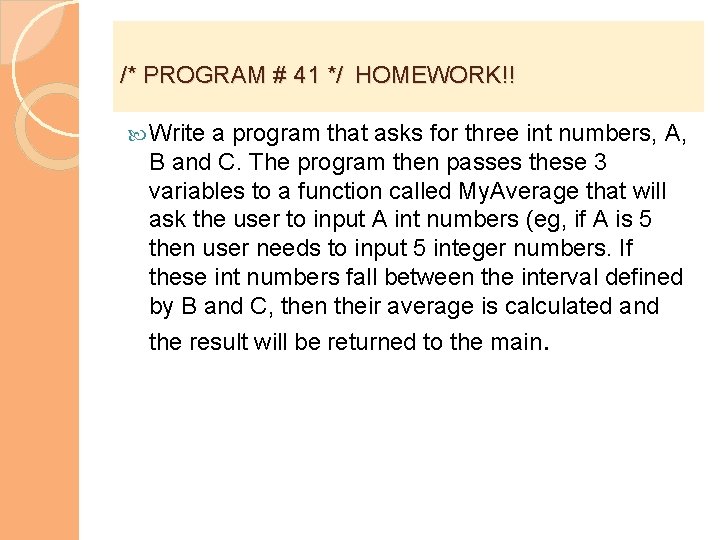
/* PROGRAM # 41 */ HOMEWORK!! Write a program that asks for three int numbers, A, B and C. The program then passes these 3 variables to a function called My. Average that will ask the user to input A int numbers (eg, if A is 5 then user needs to input 5 integer numbers. If these int numbers fall between the interval defined by B and C, then their average is calculated and the result will be returned to the main.
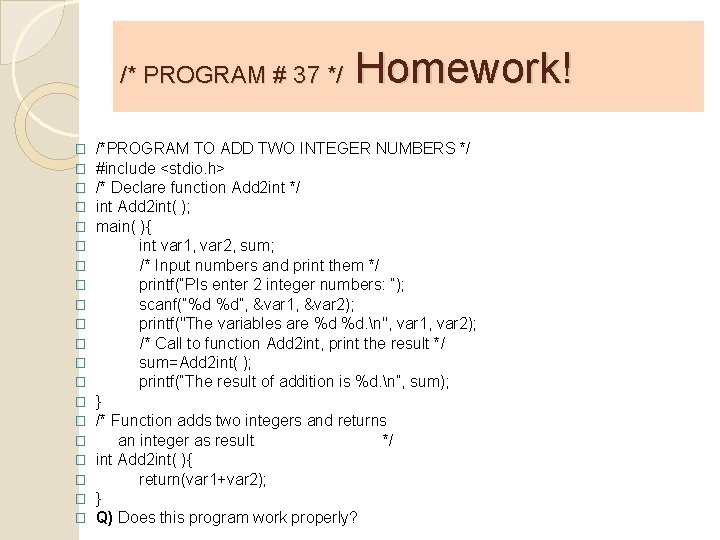
/* PROGRAM # 37 */ � � � � � Homework! /*PROGRAM TO ADD TWO INTEGER NUMBERS */ #include <stdio. h> /* Declare function Add 2 int */ int Add 2 int( ); main( ){ int var 1, var 2, sum; /* Input numbers and print them */ printf(“Pls enter 2 integer numbers: “); scanf(“%d %d”, &var 1, &var 2); printf("The variables are %d %d. n", var 1, var 2); /* Call to function Add 2 int, print the result */ sum=Add 2 int( ); printf(“The result of addition is %d. n”, sum); } /* Function adds two integers and returns an integer as result */ int Add 2 int( ){ return(var 1+var 2); } Q) Does this program work properly?