5 1 1 struct Tree Node 2 int
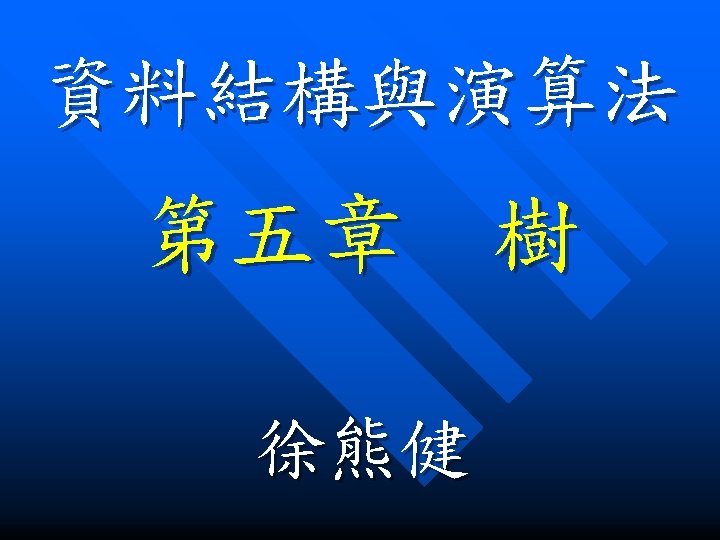
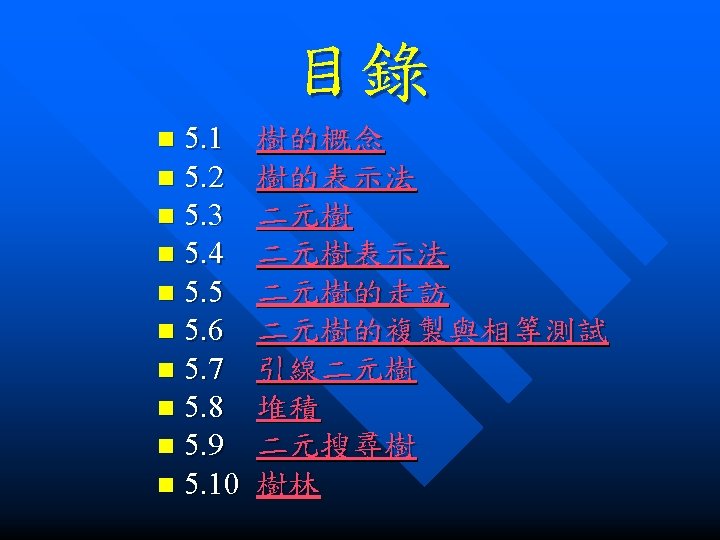
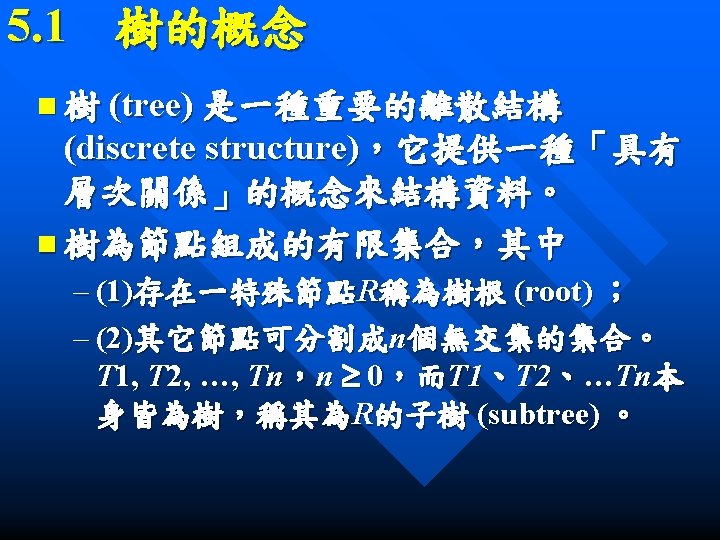
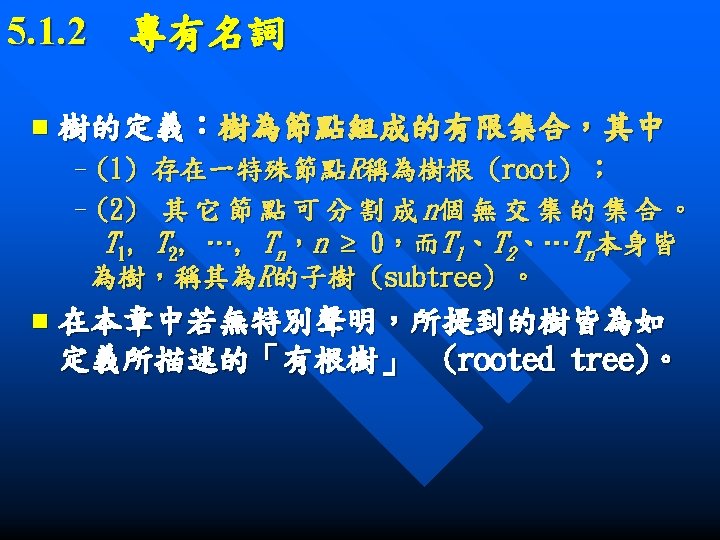
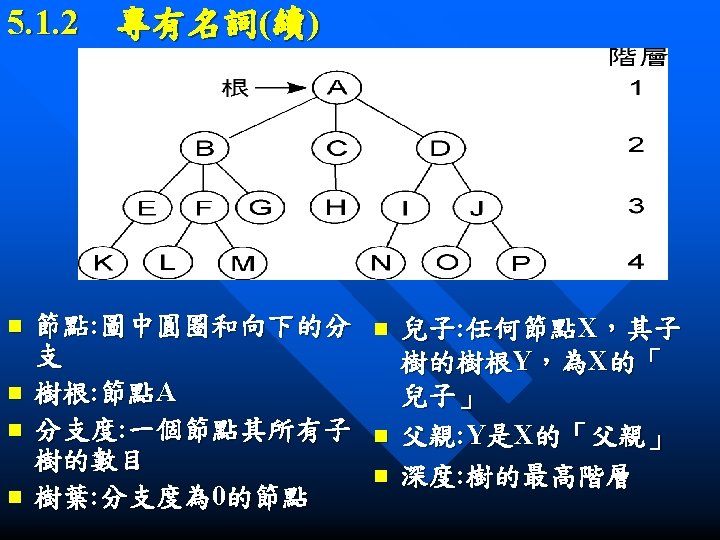
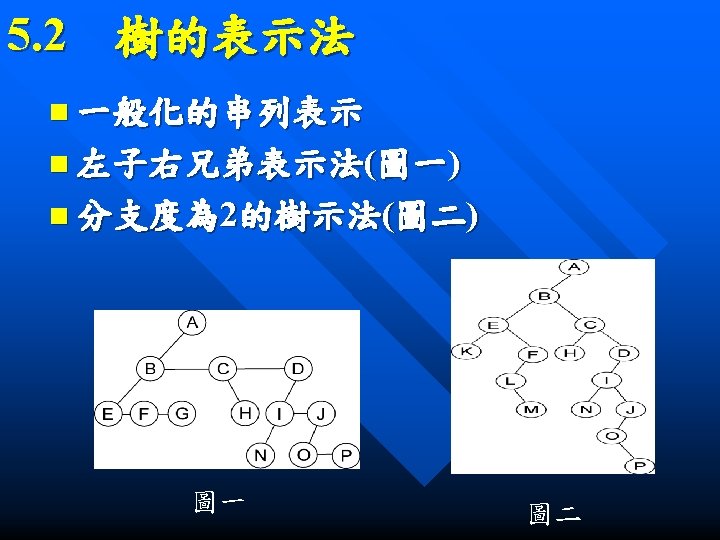
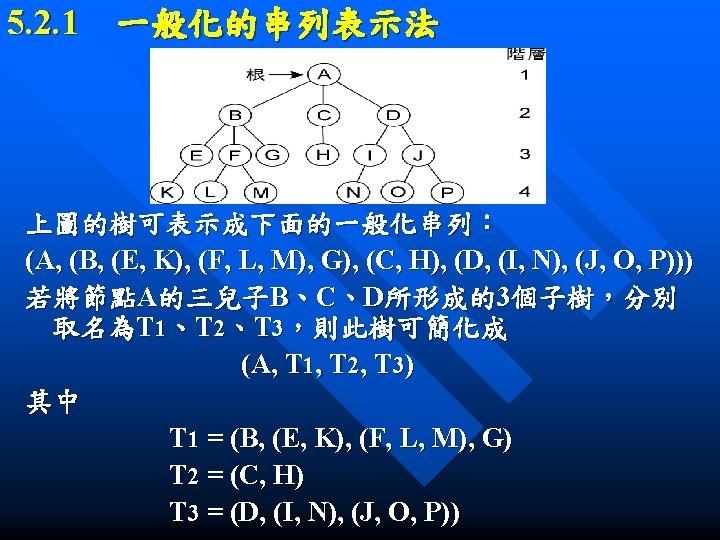
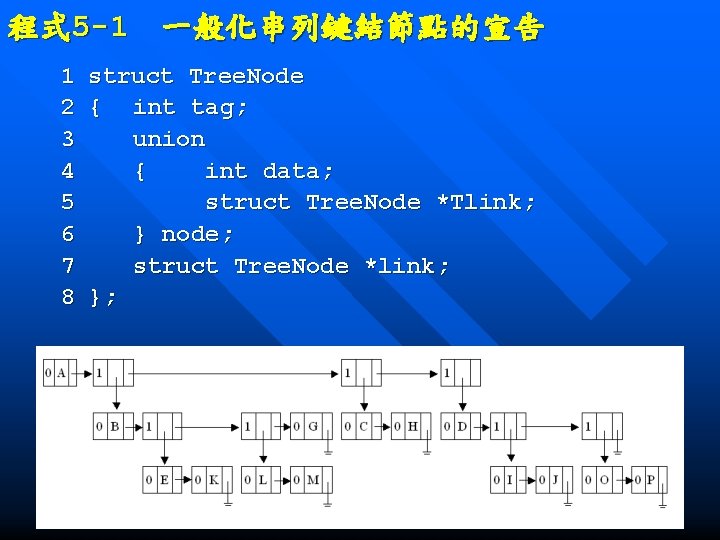
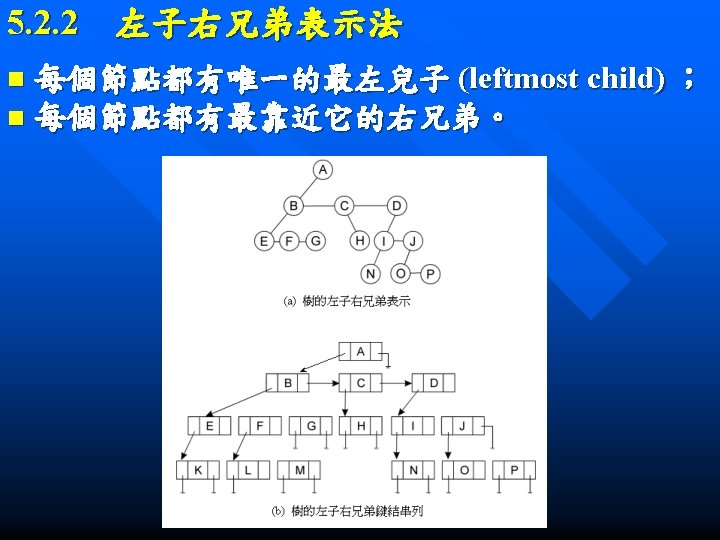
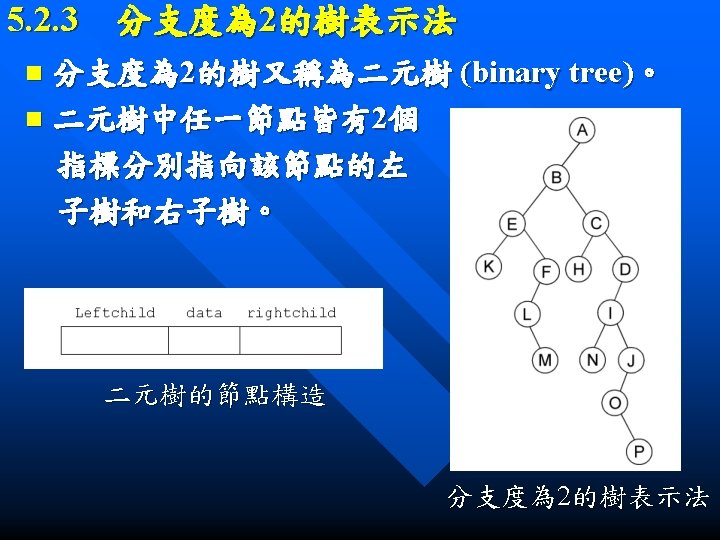
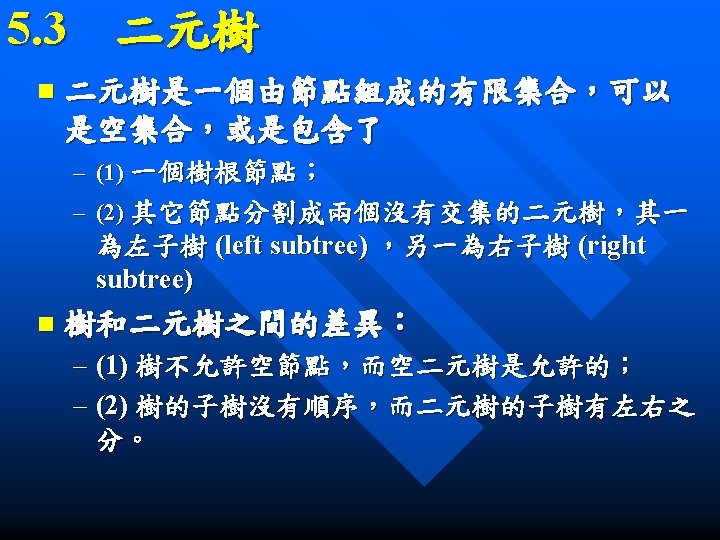
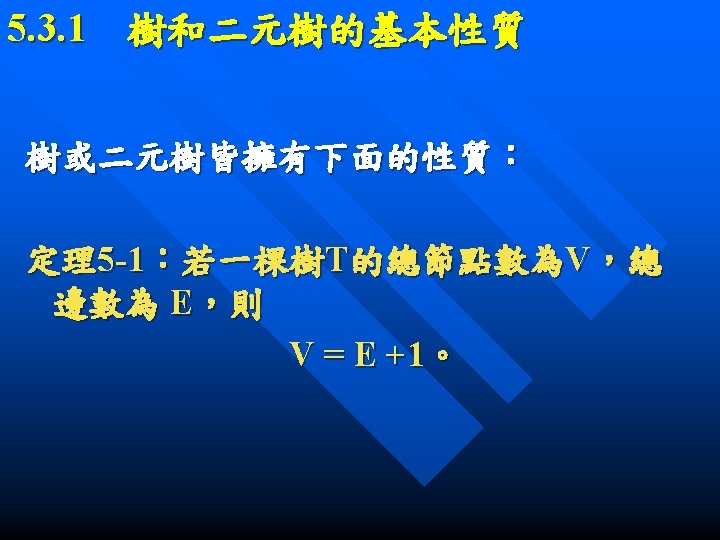
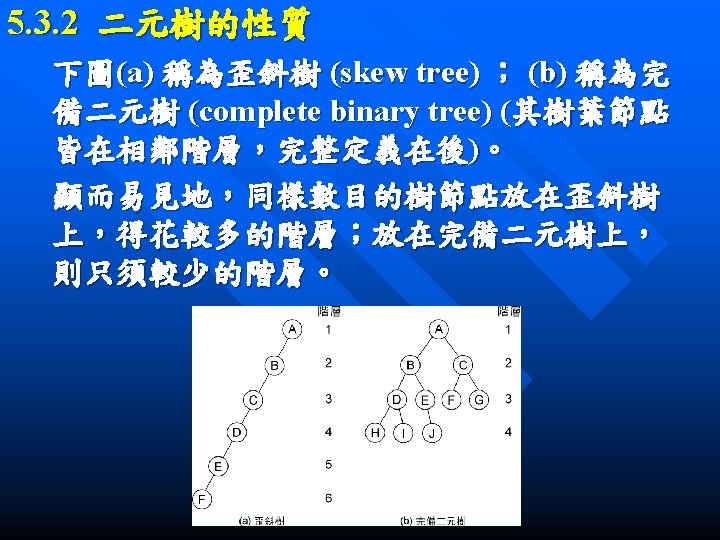
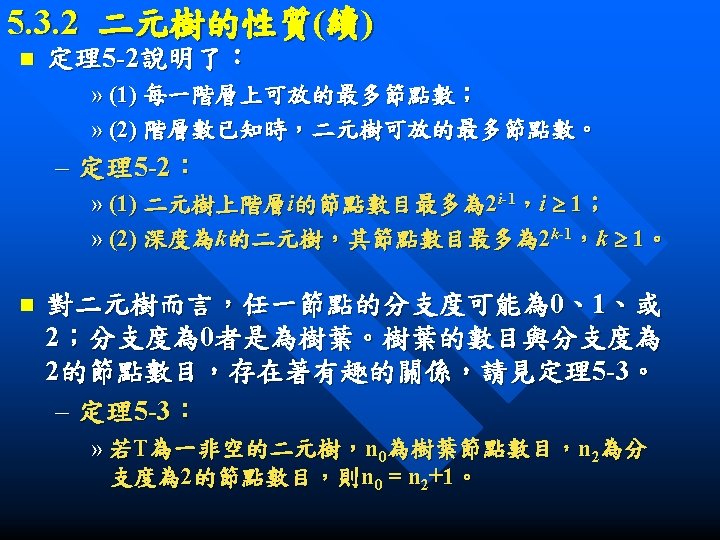
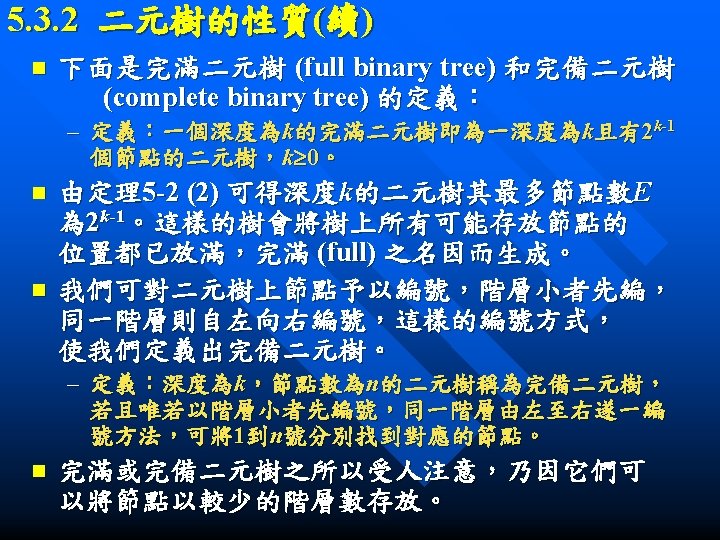
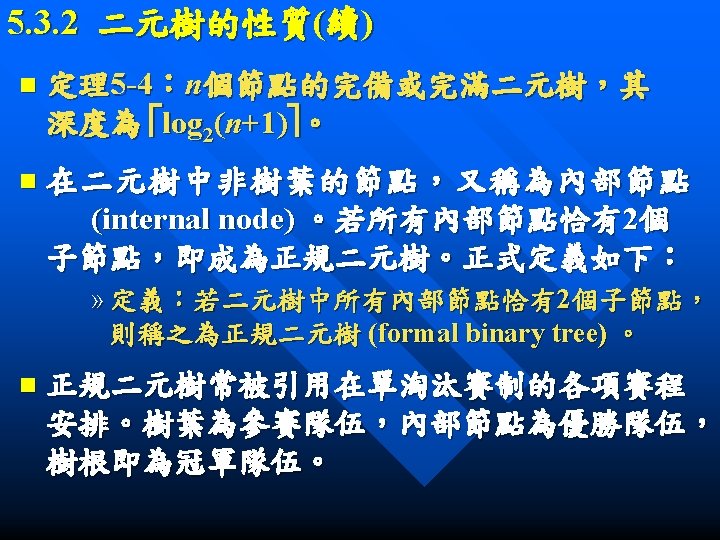
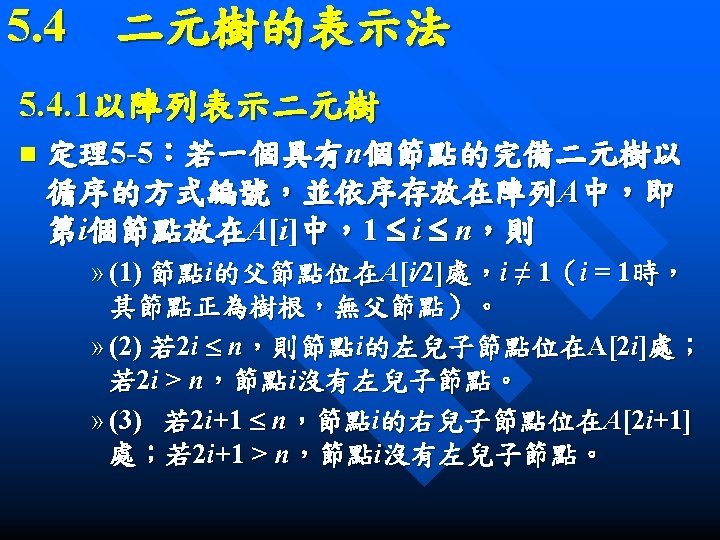
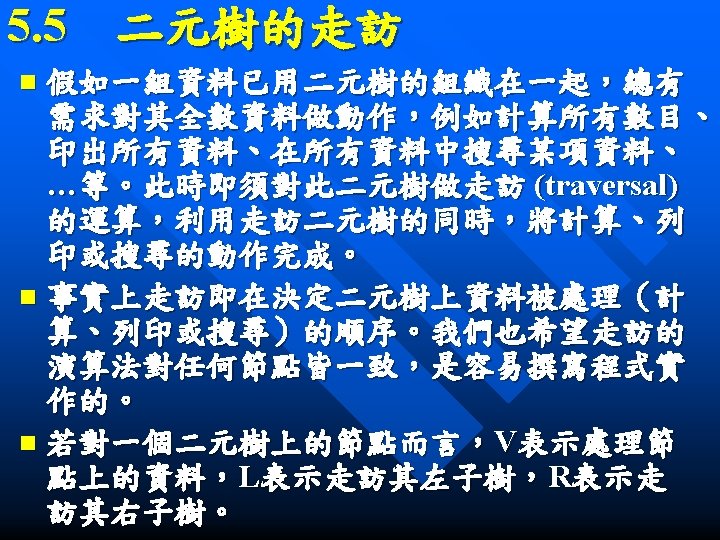
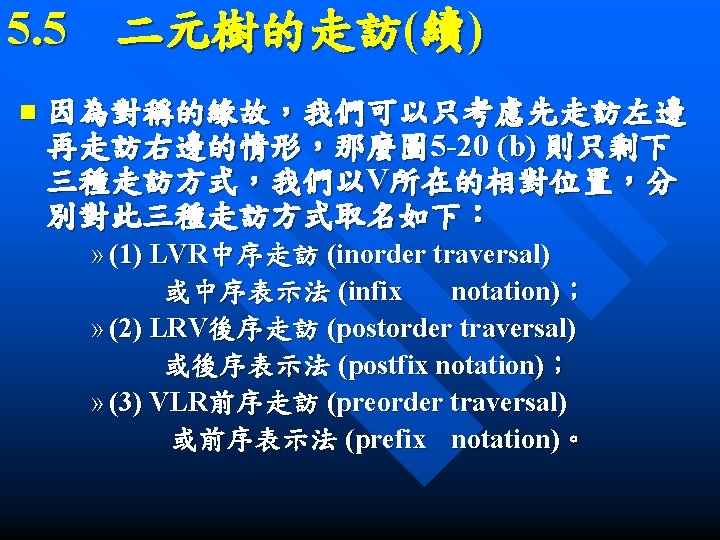
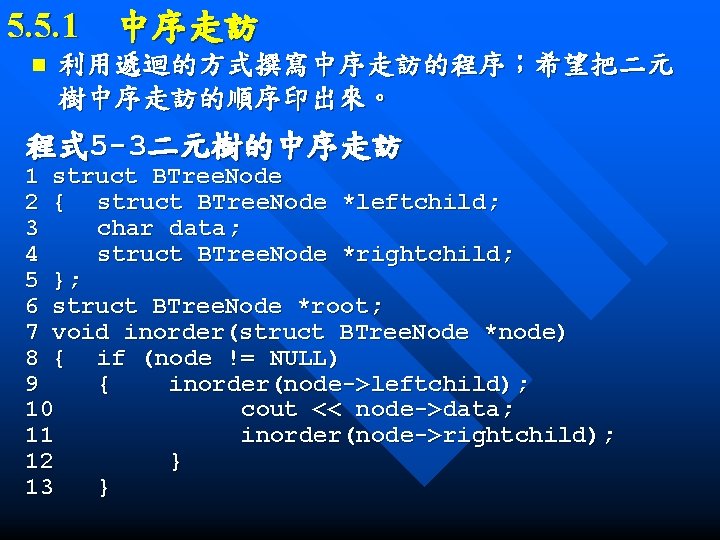
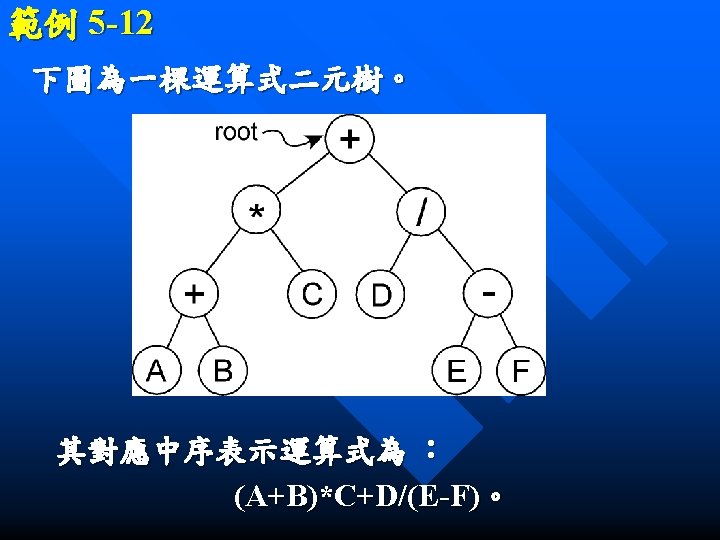
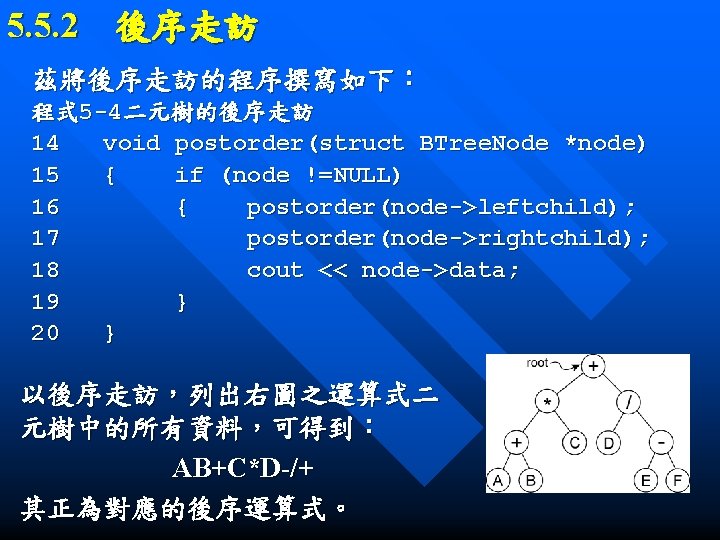
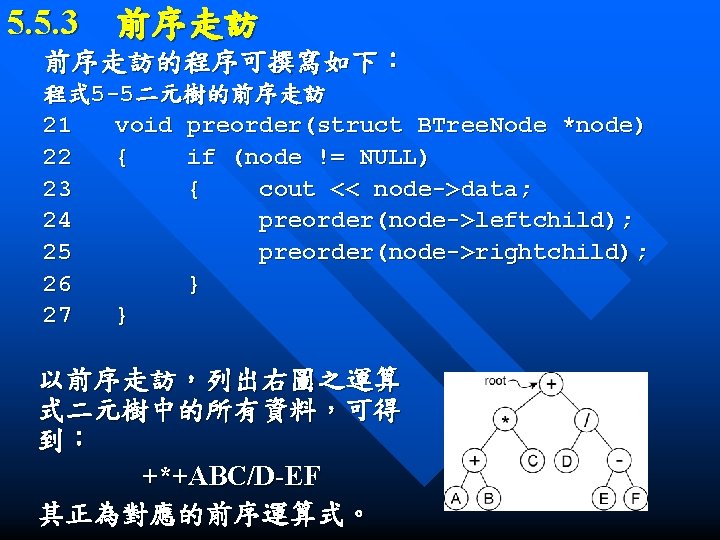
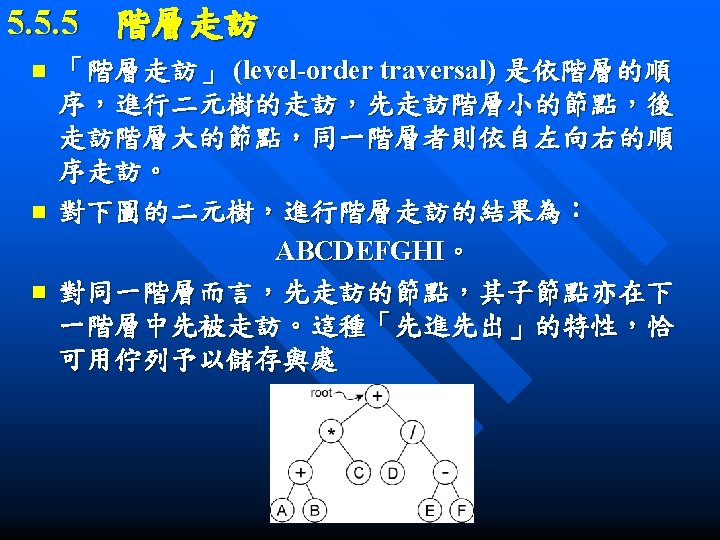
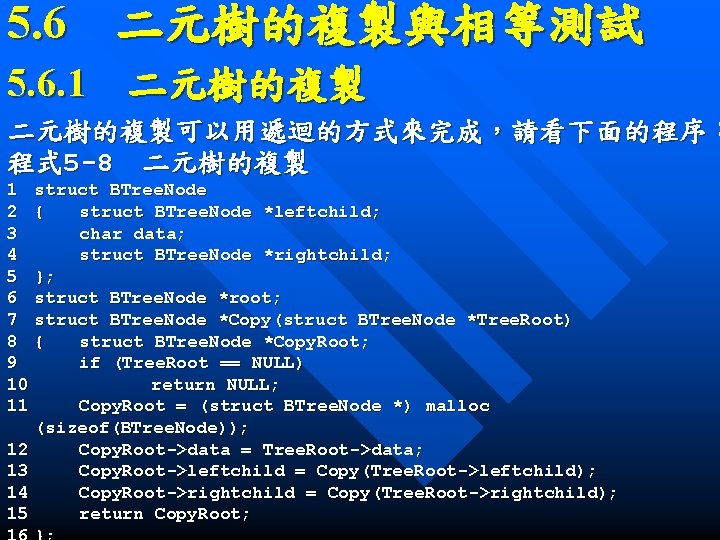
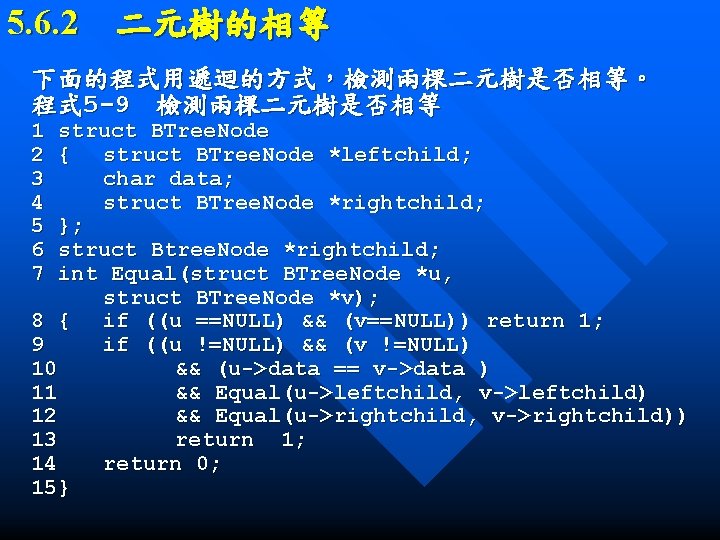
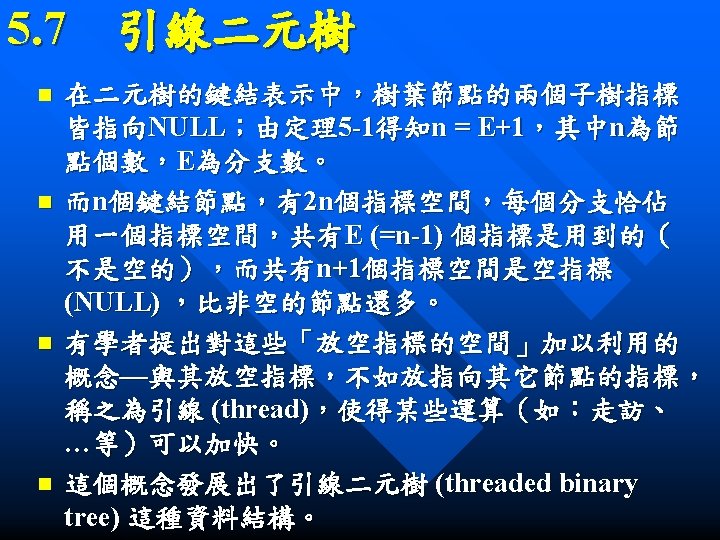
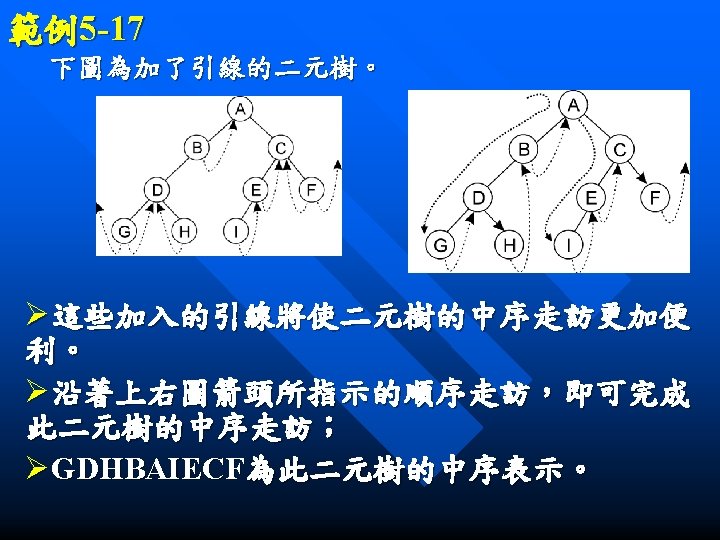
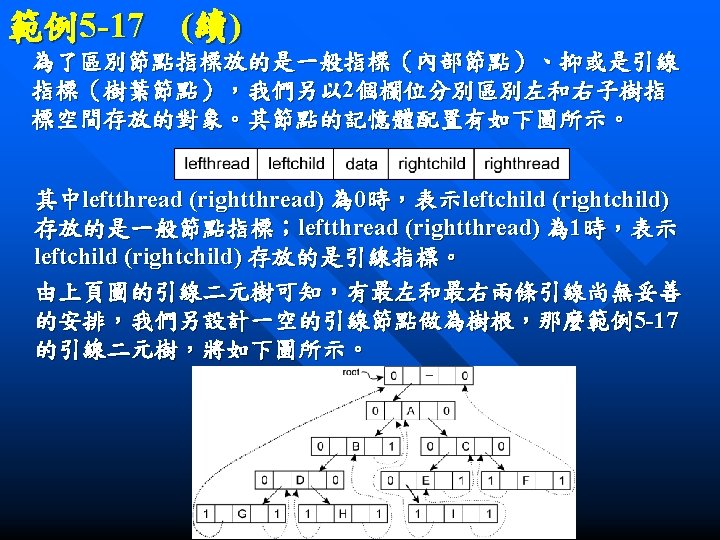
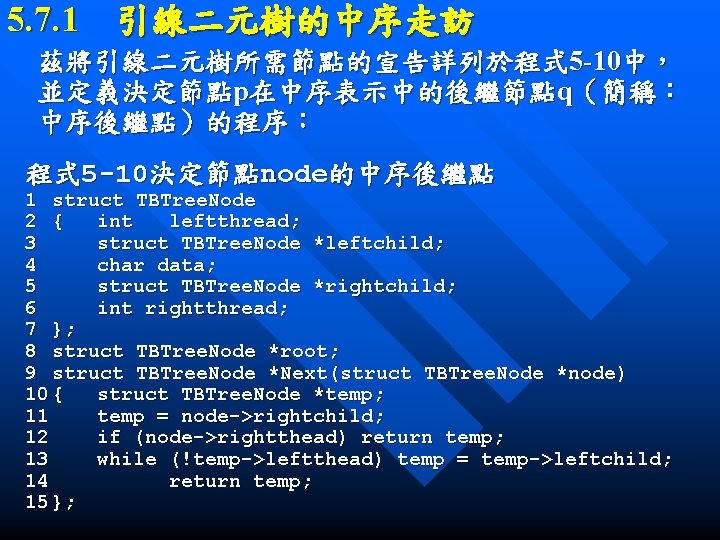
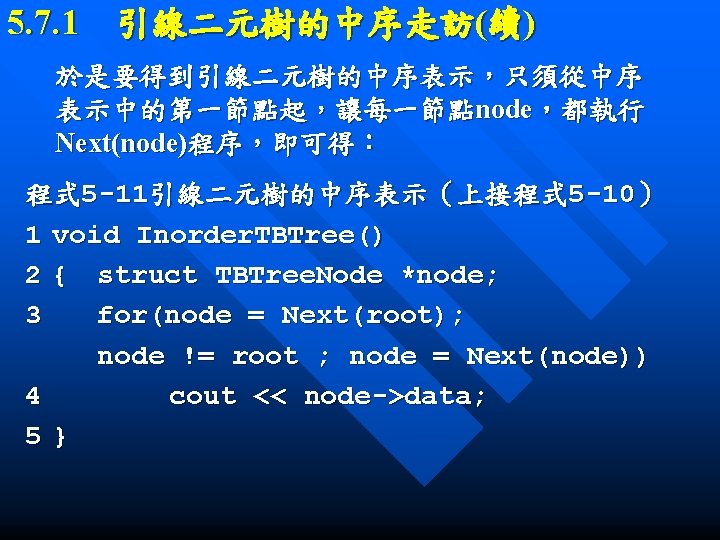
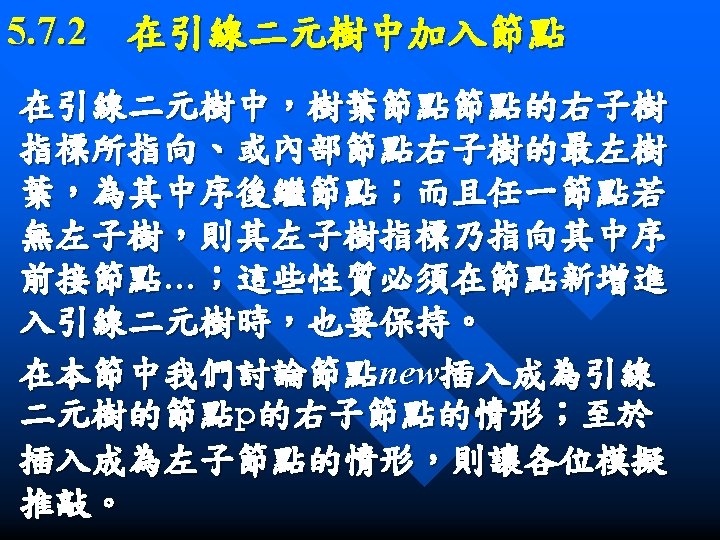
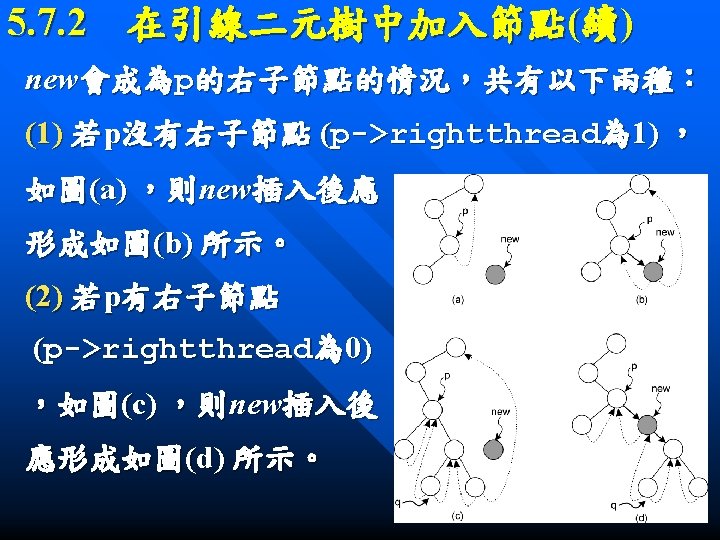
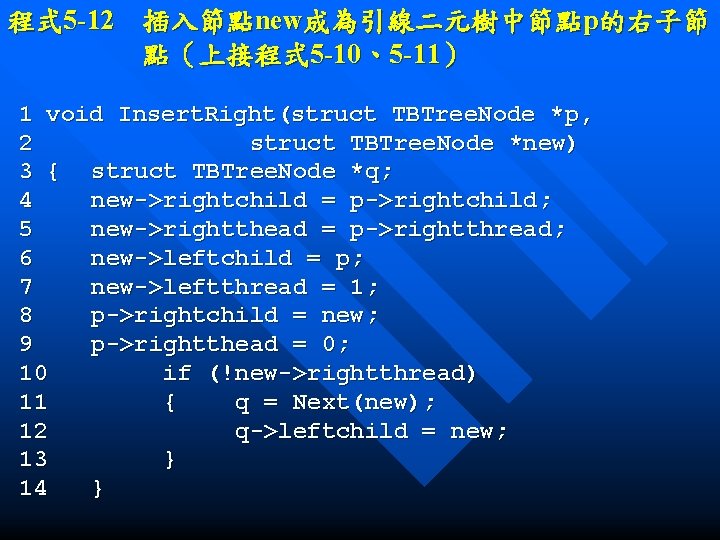
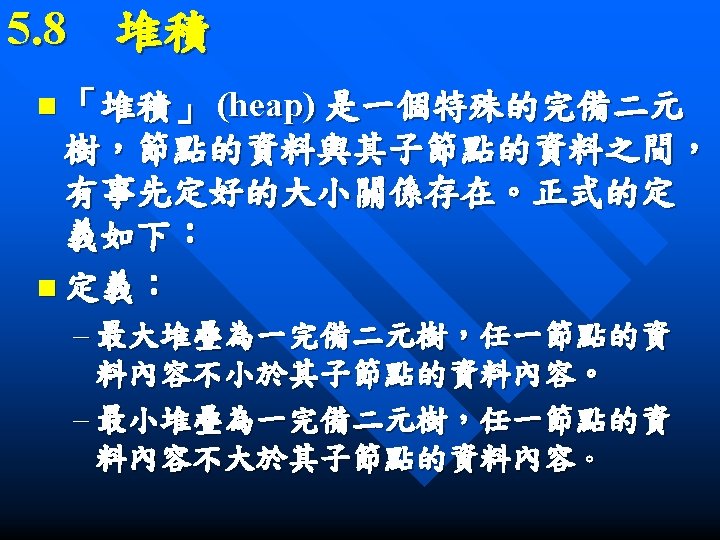
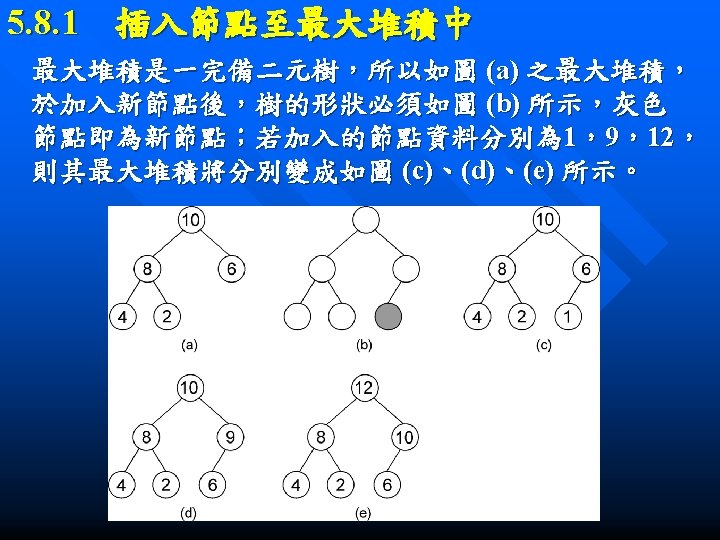
![程式 5 -13新增節點至最大堆積中 最大堆積本身是一棵完備二元樹,可以用陣列表示之 。 下面是新增節點至最大堆積的程序。 1 define maxsize 100 2 int heap[maxsize]; 3 程式 5 -13新增節點至最大堆積中 最大堆積本身是一棵完備二元樹,可以用陣列表示之 。 下面是新增節點至最大堆積的程序。 1 define maxsize 100 2 int heap[maxsize]; 3](https://slidetodoc.com/presentation_image/00ee7c080475d099b06216a957179abe/image-37.jpg)
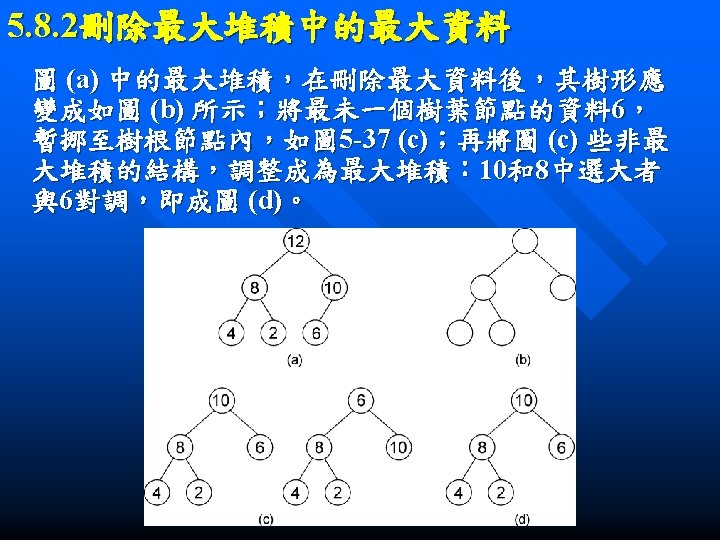
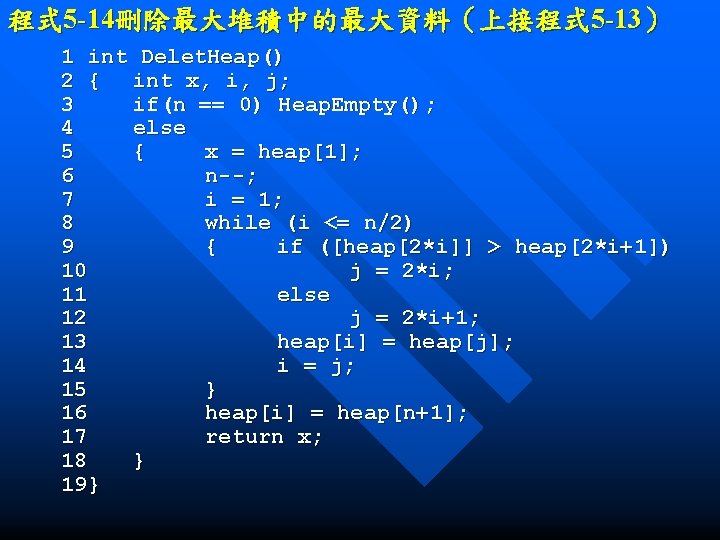
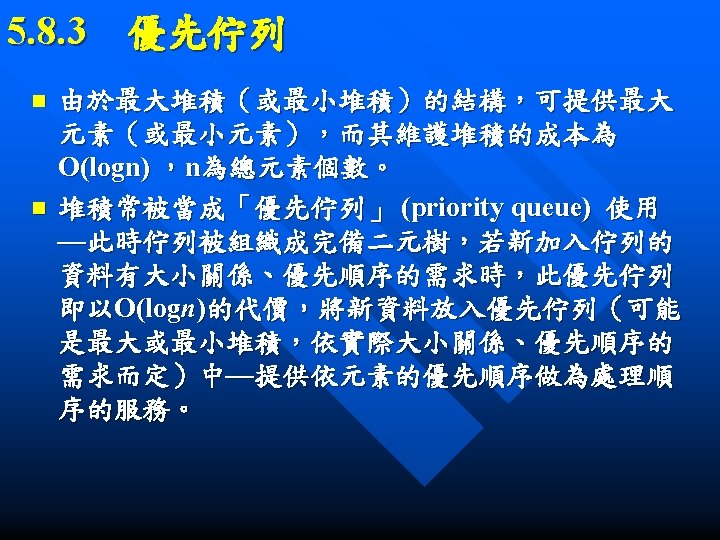
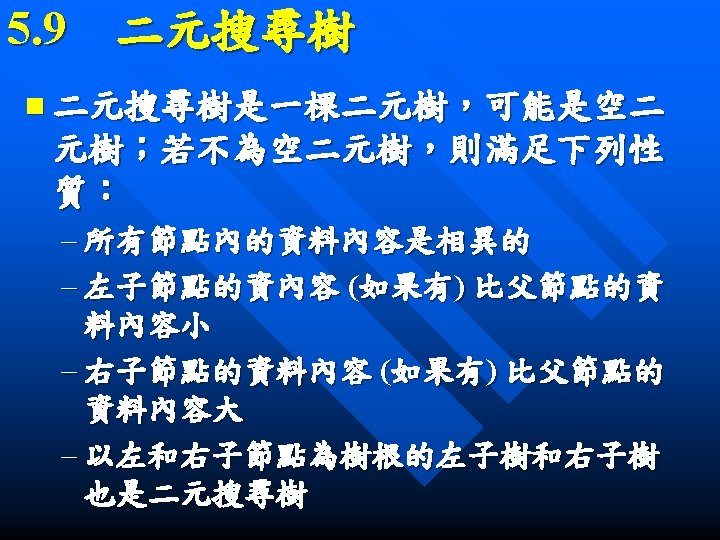
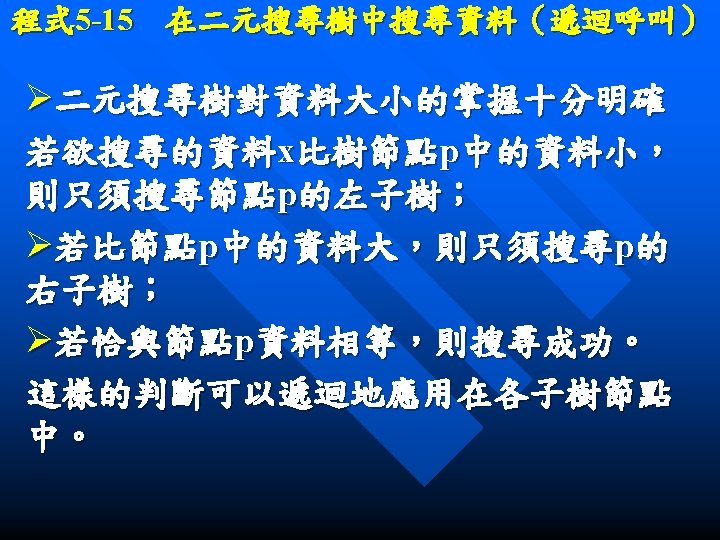
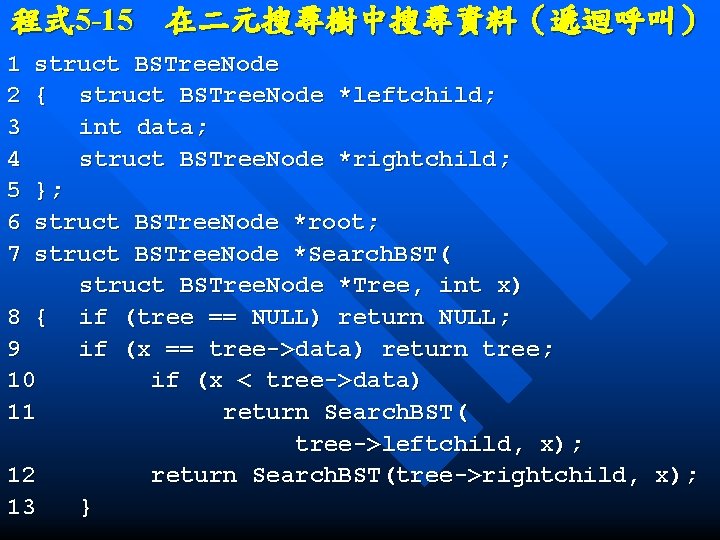
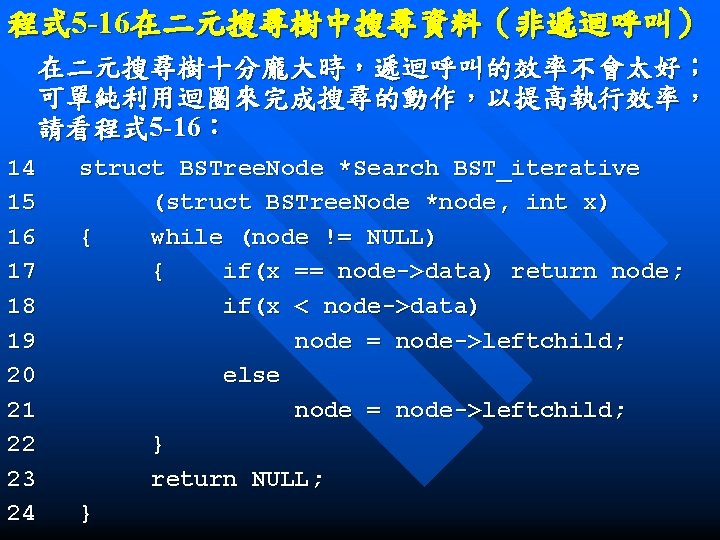
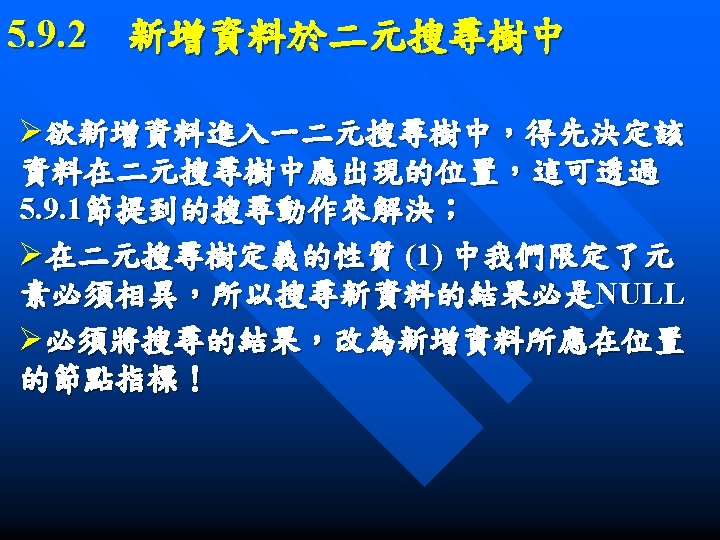
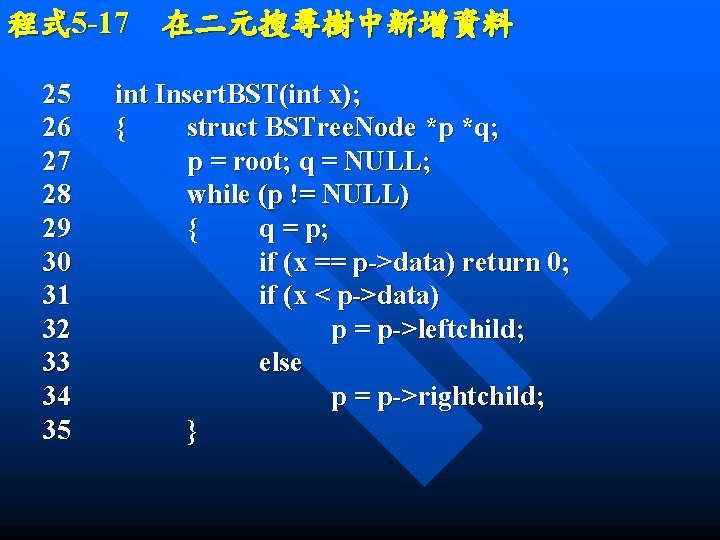
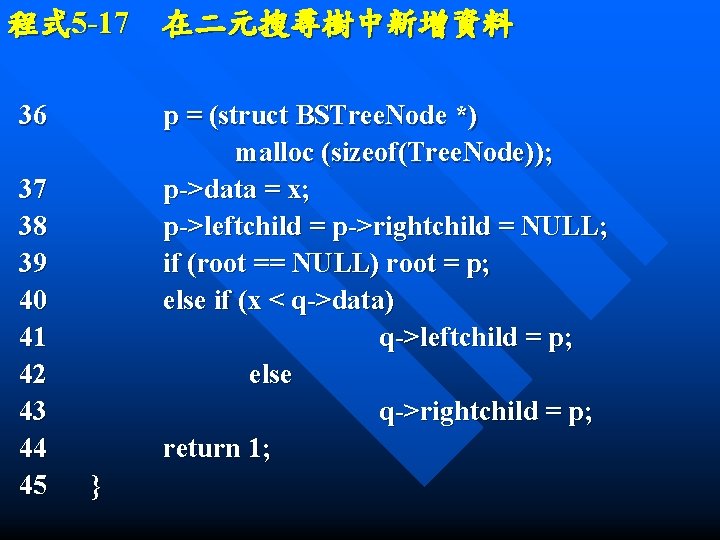
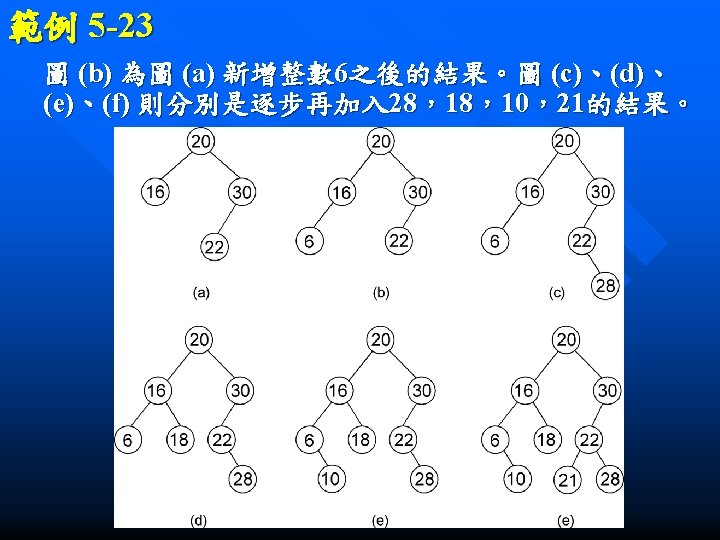
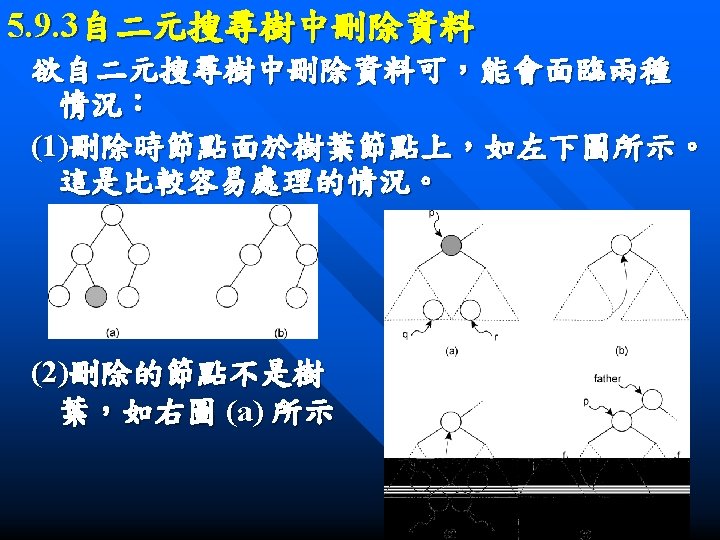
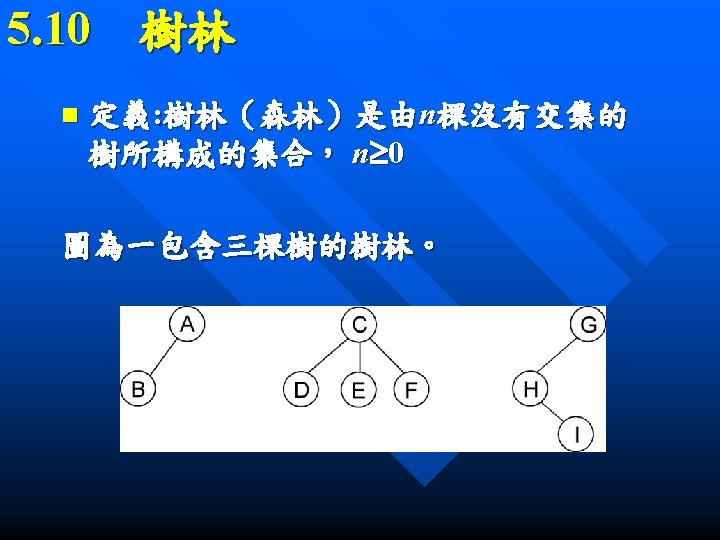
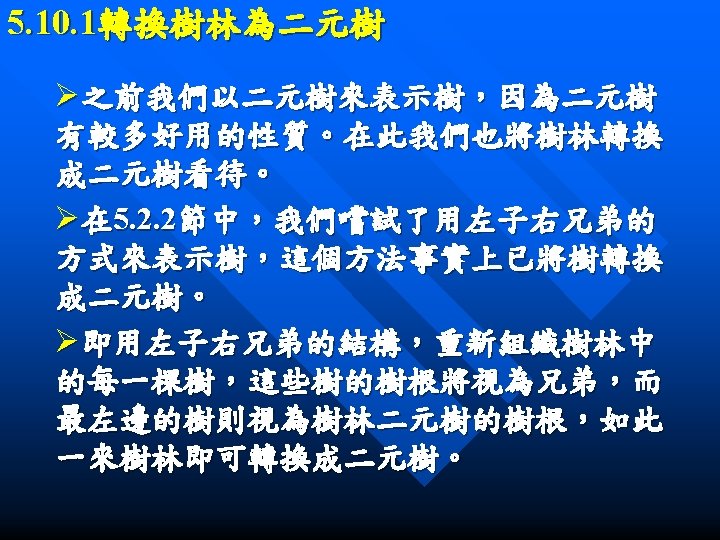
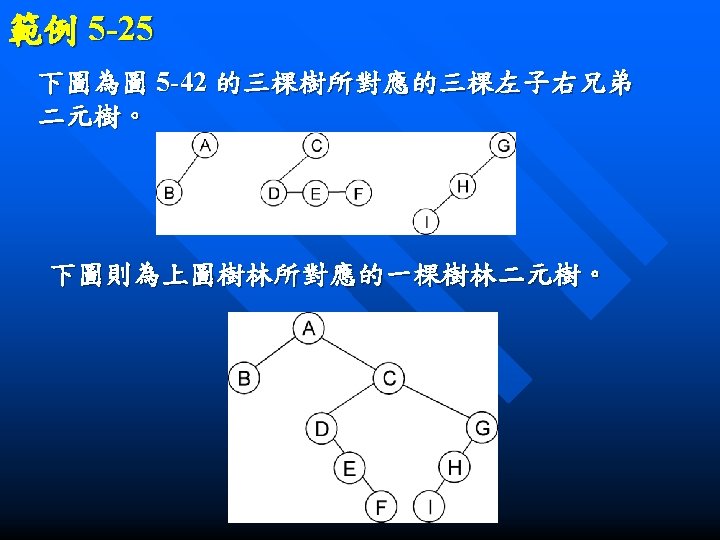
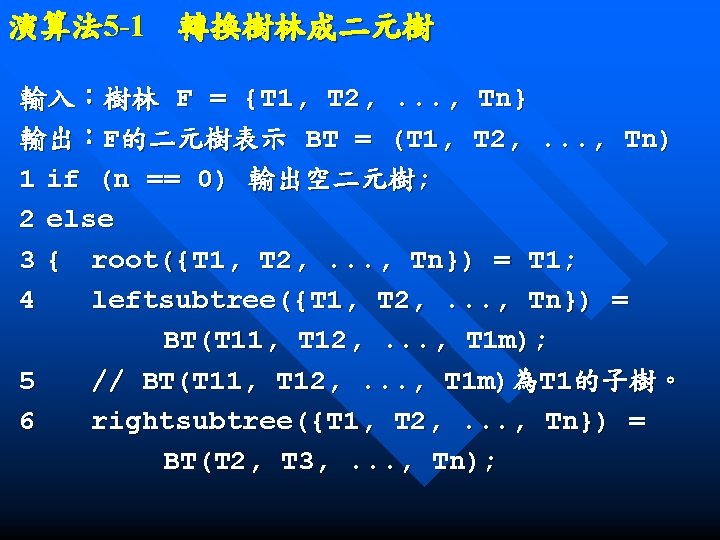
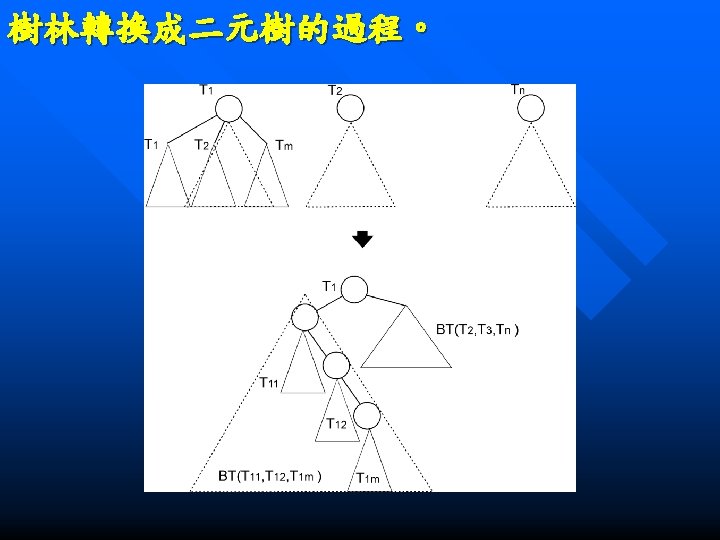
- Slides: 54
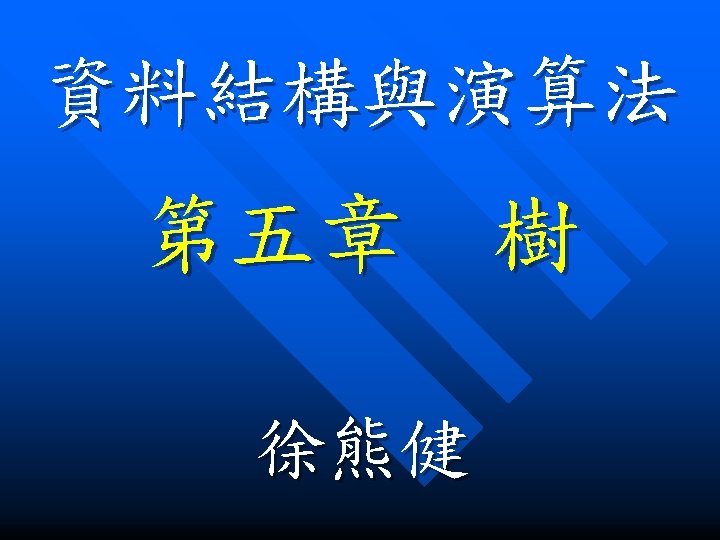
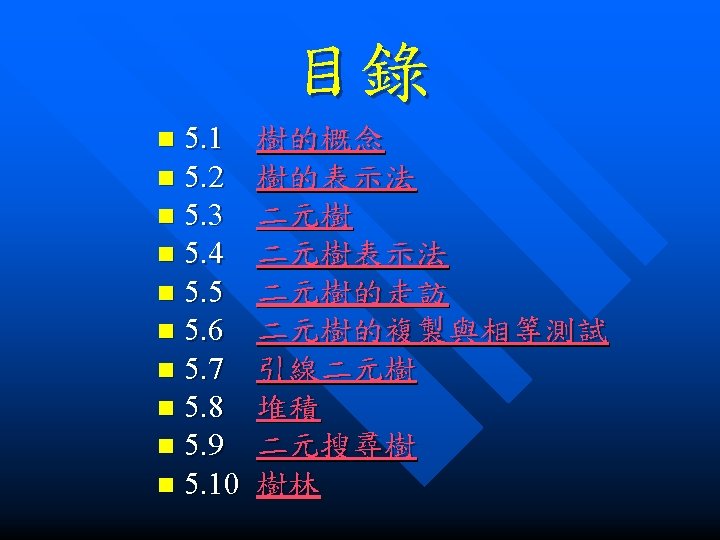
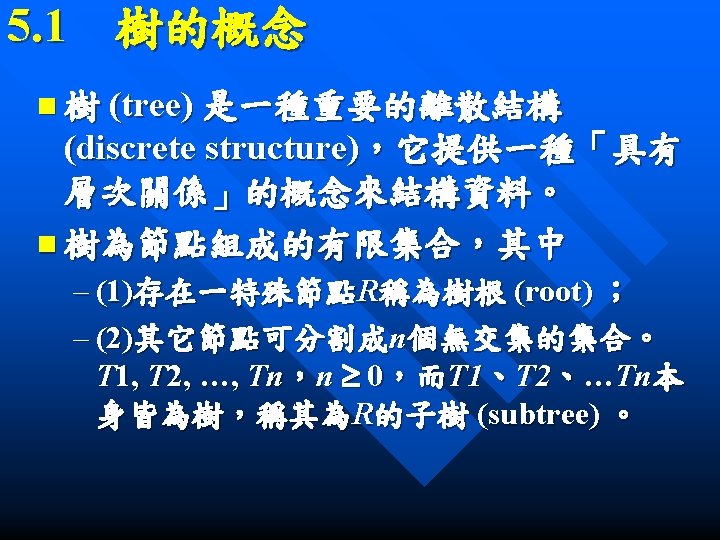
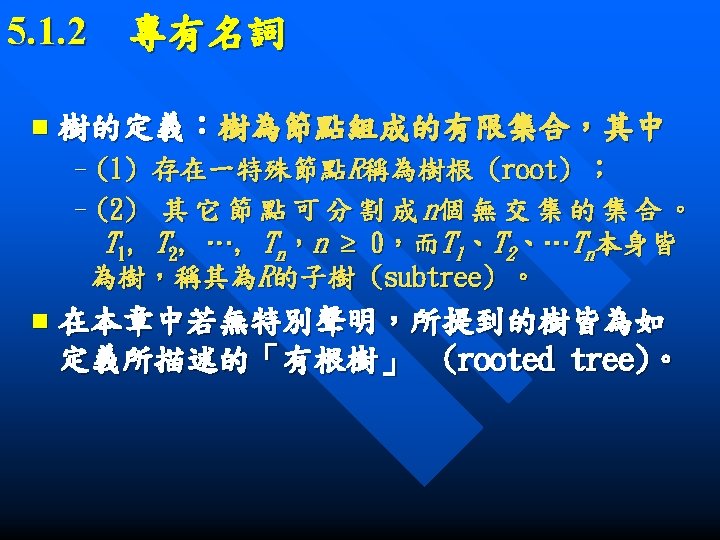
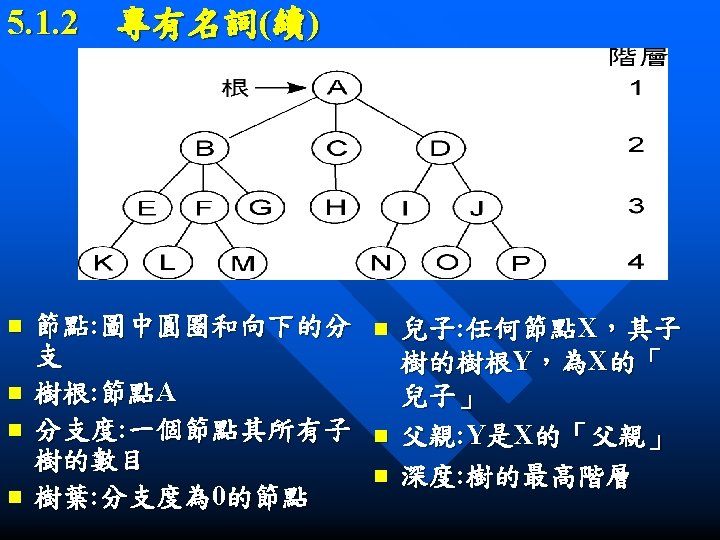
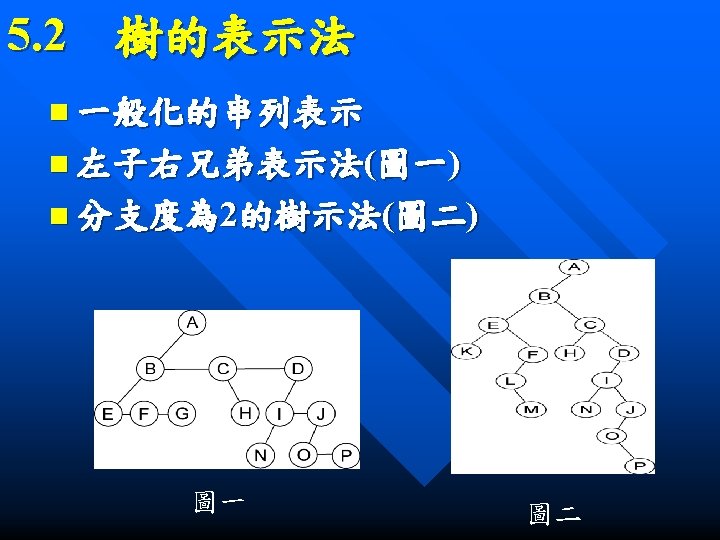
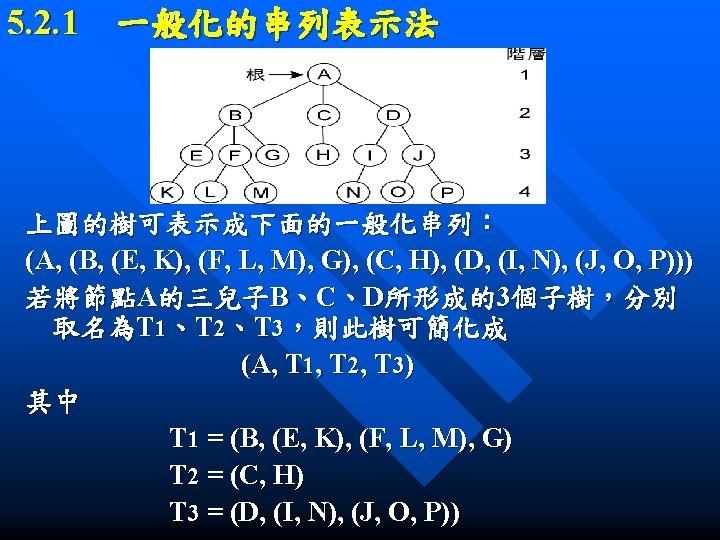
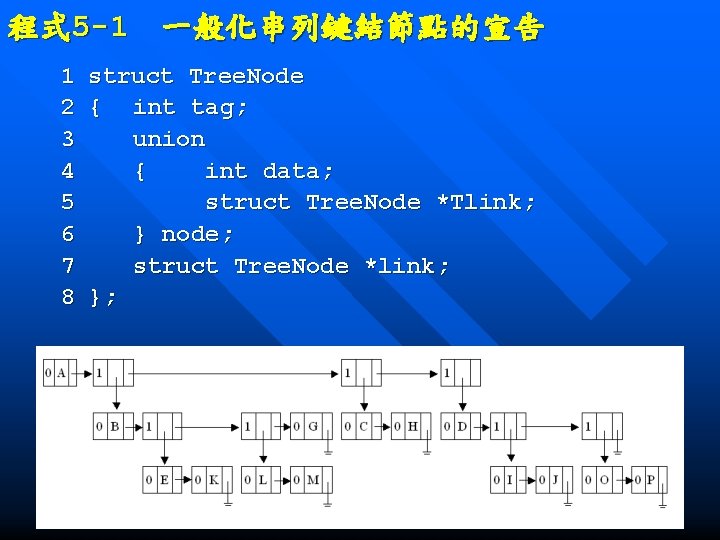
程式 5 -1 一般化串列鍵結節點的宣告 1 struct Tree. Node 2 { int tag; 3 union 4 { int data; 5 struct Tree. Node *Tlink; 6 } node; 7 struct Tree. Node *link; 8 };
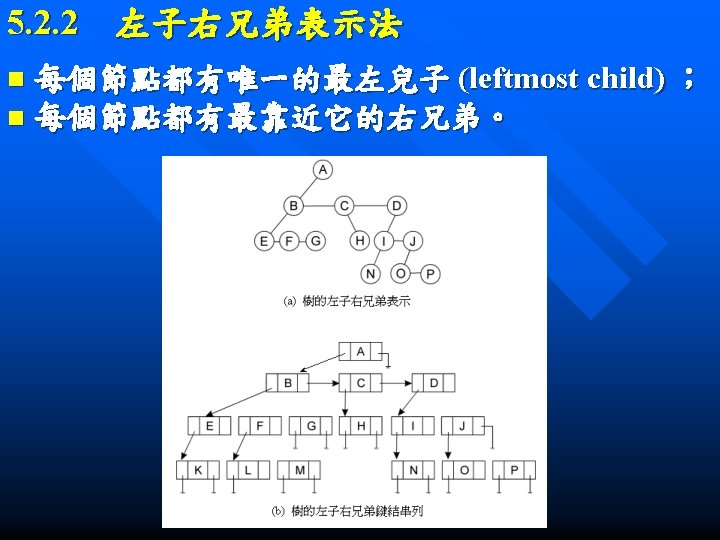
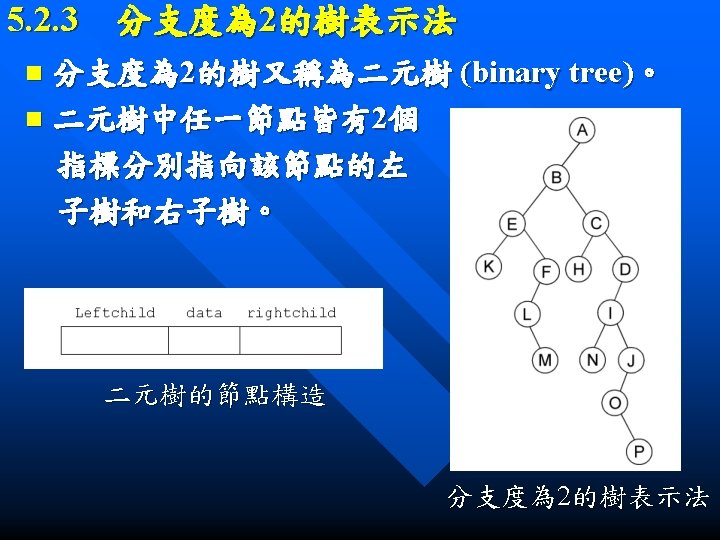
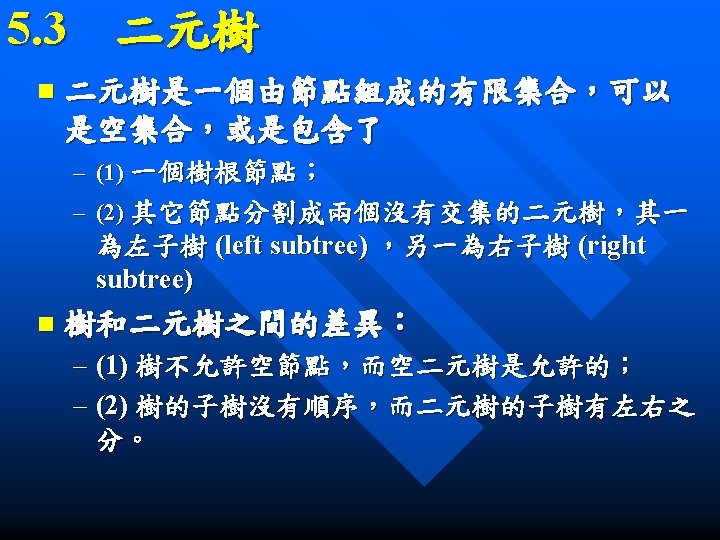
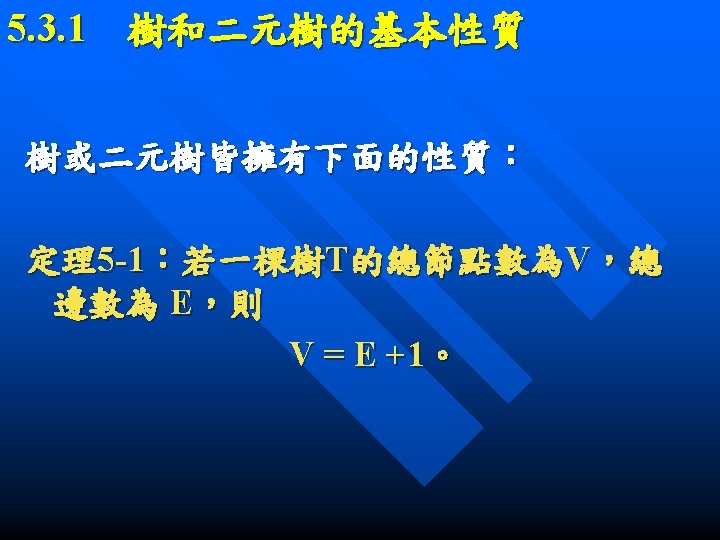
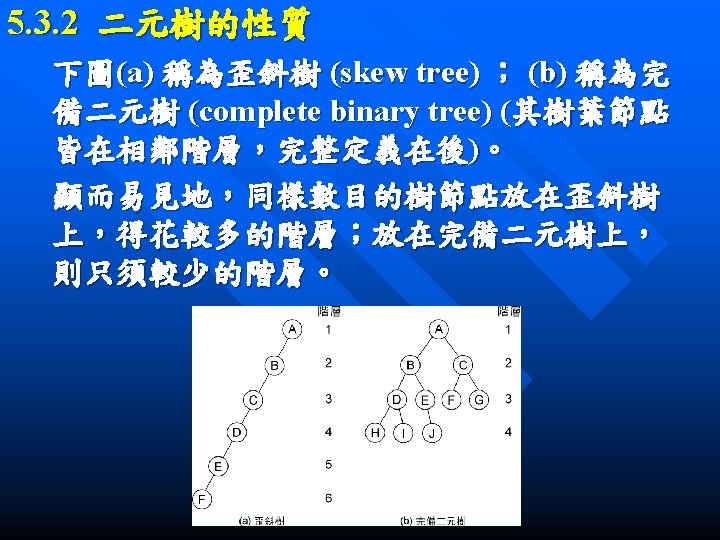
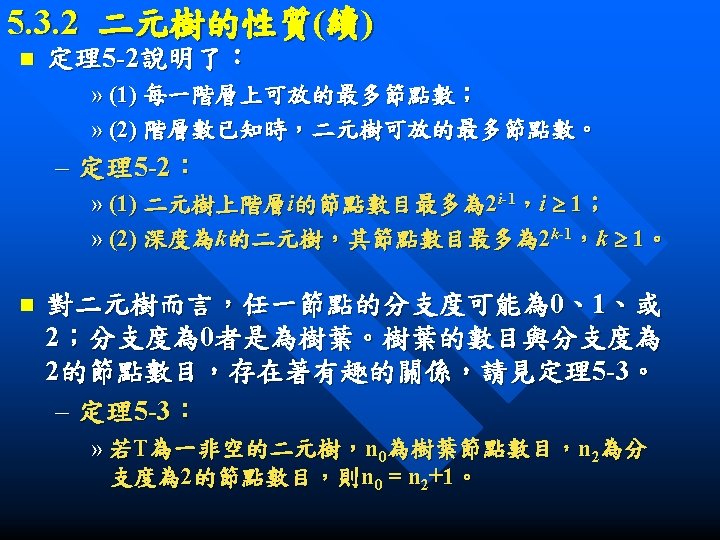
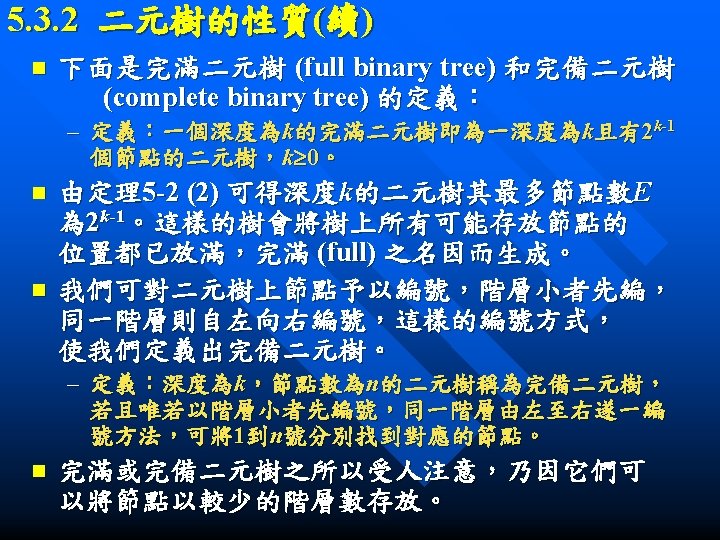
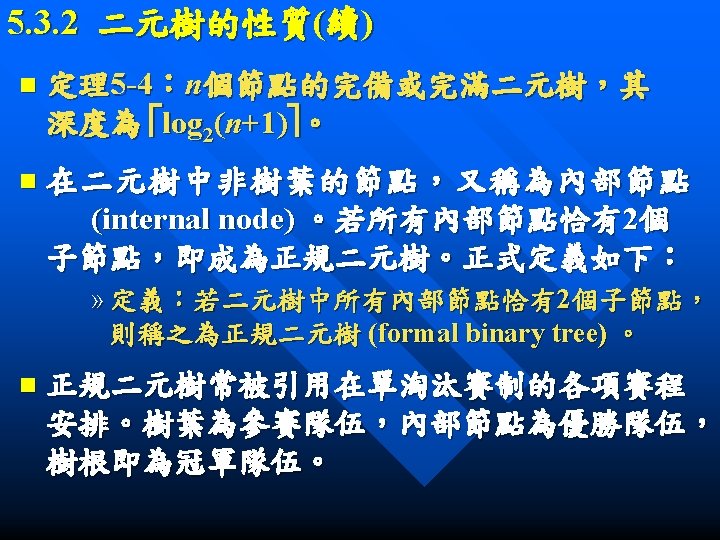
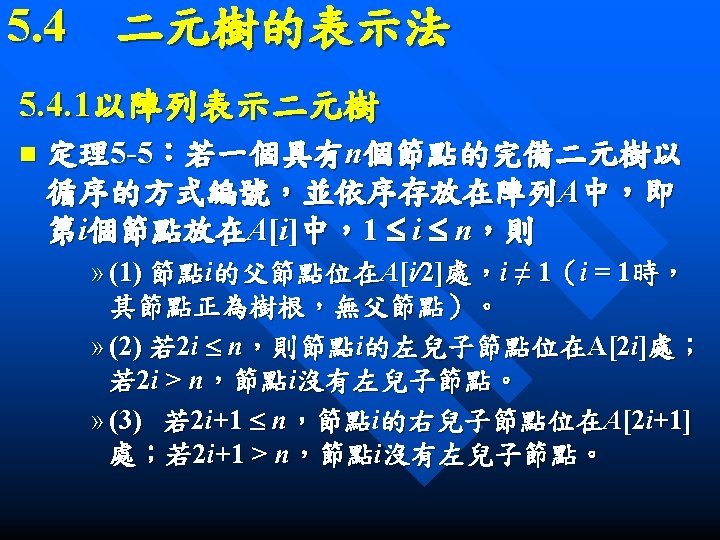
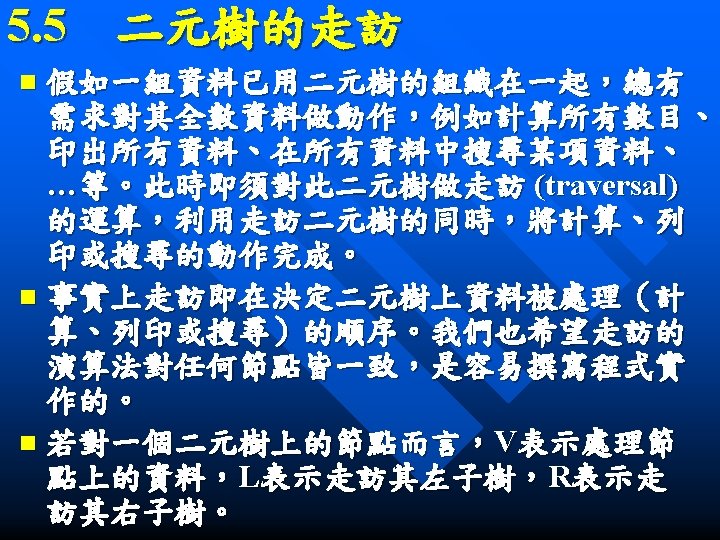
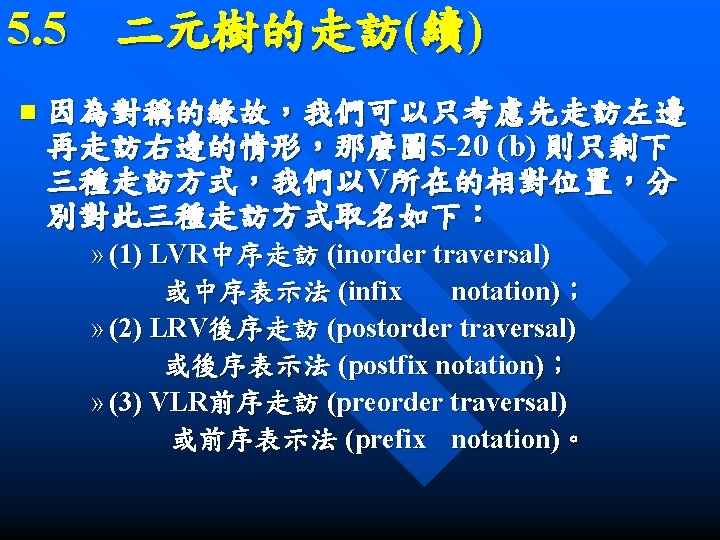
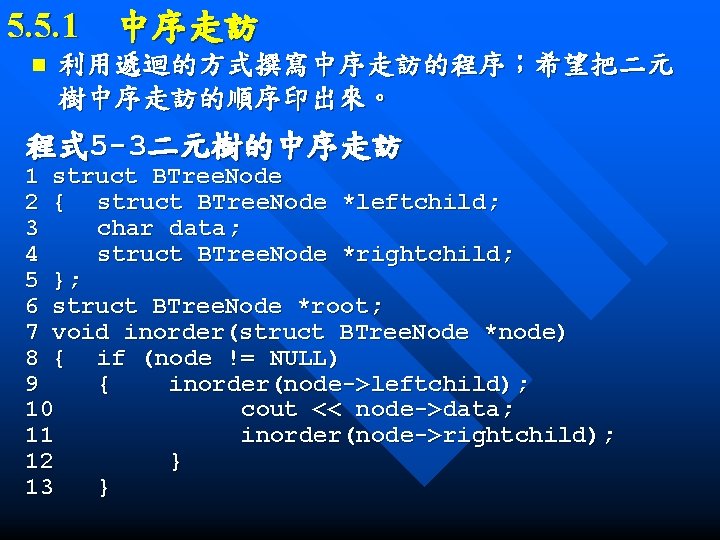
5. 5. 1 中序走訪 n 利用遞迴的方式撰寫中序走訪的程序;希望把二元 樹中序走訪的順序印出來。 程式 5 -3二元樹的中序走訪 1 struct BTree. Node 2 { struct BTree. Node *leftchild; 3 char data; 4 struct BTree. Node *rightchild; 5 }; 6 struct BTree. Node *root; 7 void inorder(struct BTree. Node *node) 8 { if (node != NULL) 9 { inorder(node->leftchild); 10 cout << node->data; 11 inorder(node->rightchild); 12 } 13 }
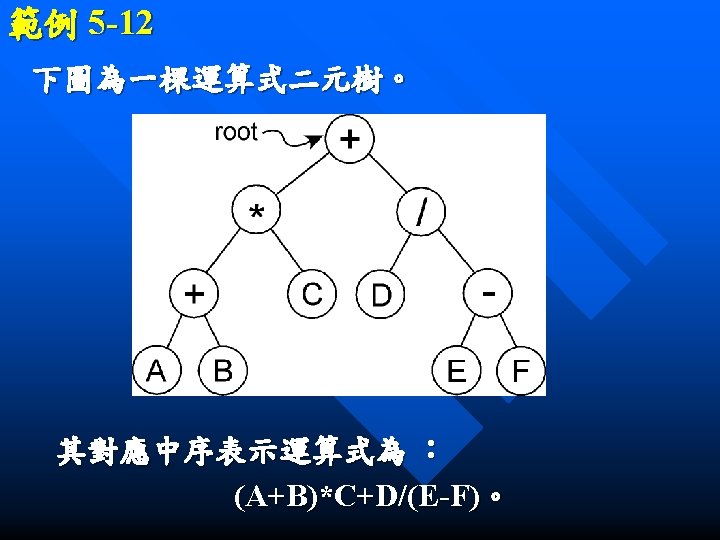
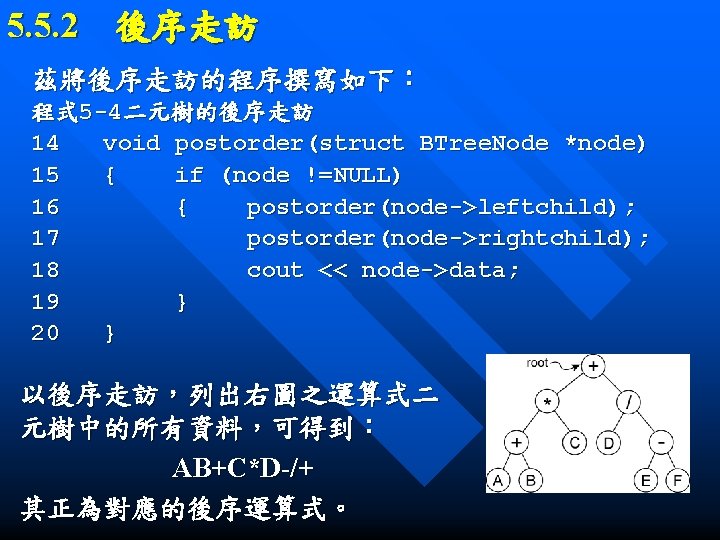
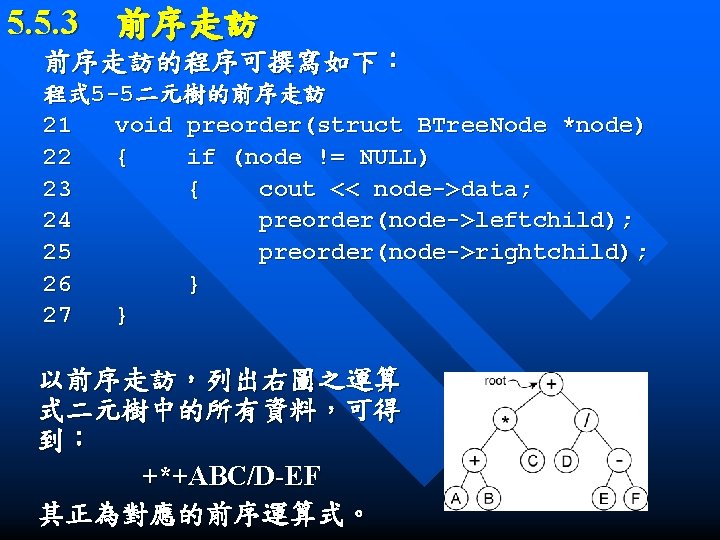
5. 5. 3 前序走訪的程序可撰寫如下: 程式 5 -5二元樹的前序走訪 21 void preorder(struct BTree. Node *node) 22 { if (node != NULL) 23 { cout << node->data; 24 preorder(node->leftchild); 25 preorder(node->rightchild); 26 } 27 } 以前序走訪,列出右圖之運算 式二元樹中的所有資料,可得 到: +*+ABC/D-EF 其正為對應的前序運算式。
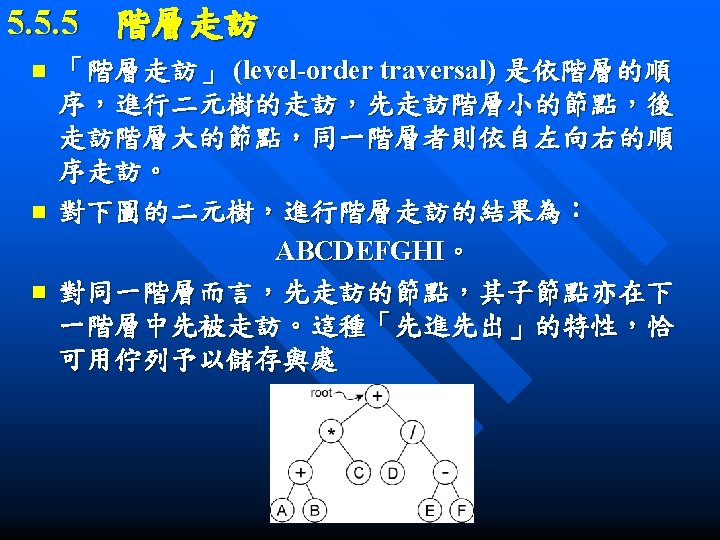
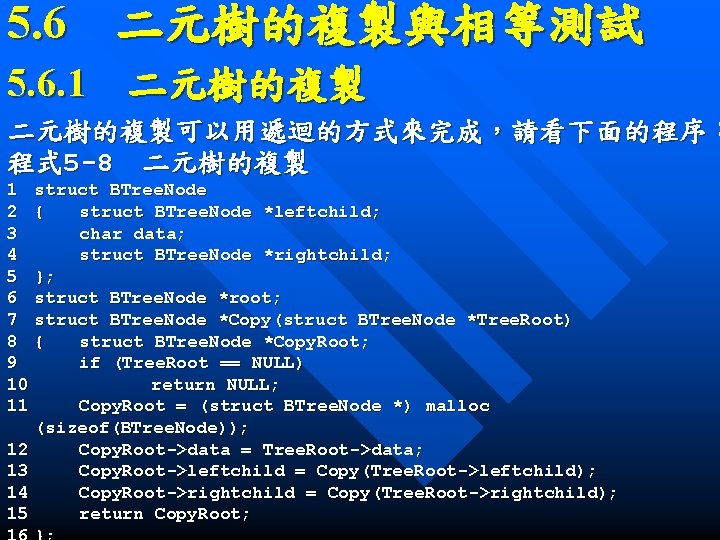
5. 6 二元樹的複製與相等測試 5. 6. 1 二元樹的複製可以用遞迴的方式來完成,請看下面的程序: 程式 5 -8 二元樹的複製 1 struct BTree. Node 2 { struct BTree. Node *leftchild; 3 char data; 4 struct BTree. Node *rightchild; 5 }; 6 struct BTree. Node *root; 7 struct BTree. Node *Copy(struct BTree. Node *Tree. Root) 8 { struct BTree. Node *Copy. Root; 9 if (Tree. Root == NULL) 10 return NULL; 11 Copy. Root = (struct BTree. Node *) malloc (sizeof(BTree. Node)); 12 Copy. Root->data = Tree. Root->data; 13 Copy. Root->leftchild = Copy(Tree. Root->leftchild); 14 Copy. Root->rightchild = Copy(Tree. Root->rightchild); 15 return Copy. Root;
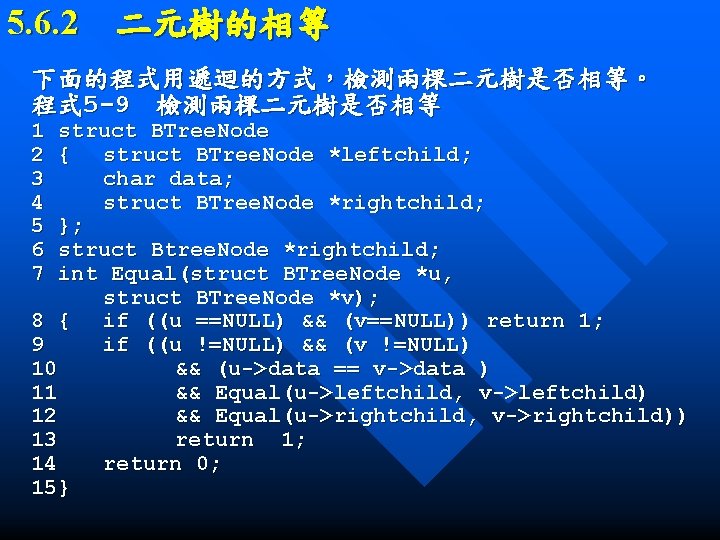
5. 6. 2 二元樹的相等 下面的程式用遞迴的方式,檢測兩棵二元樹是否相等。 程式 5 -9 檢測兩棵二元樹是否相等 1 2 3 4 5 6 7 struct BTree. Node { struct BTree. Node *leftchild; char data; struct BTree. Node *rightchild; }; struct Btree. Node *rightchild; int Equal(struct BTree. Node *u, struct BTree. Node *v); 8 { if ((u ==NULL) && (v==NULL)) return 1; 9 if ((u !=NULL) && (v !=NULL) 10 && (u->data == v->data ) 11 && Equal(u->leftchild, v->leftchild) 12 && Equal(u->rightchild, v->rightchild)) 13 return 1; 14 return 0; 15}
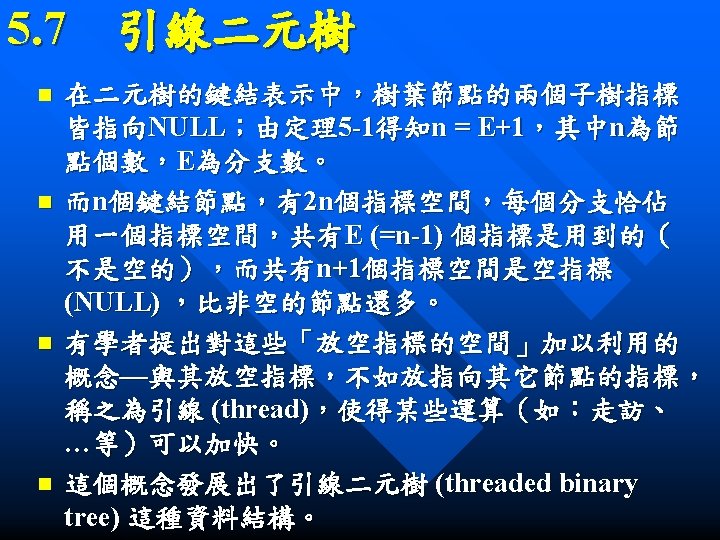
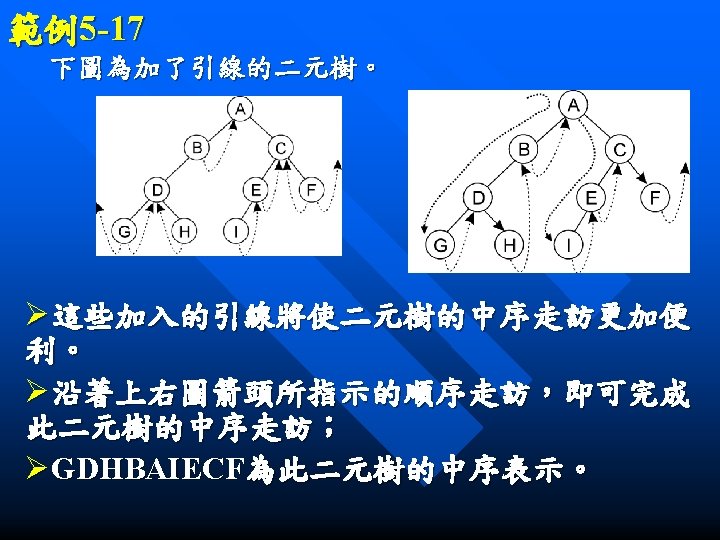
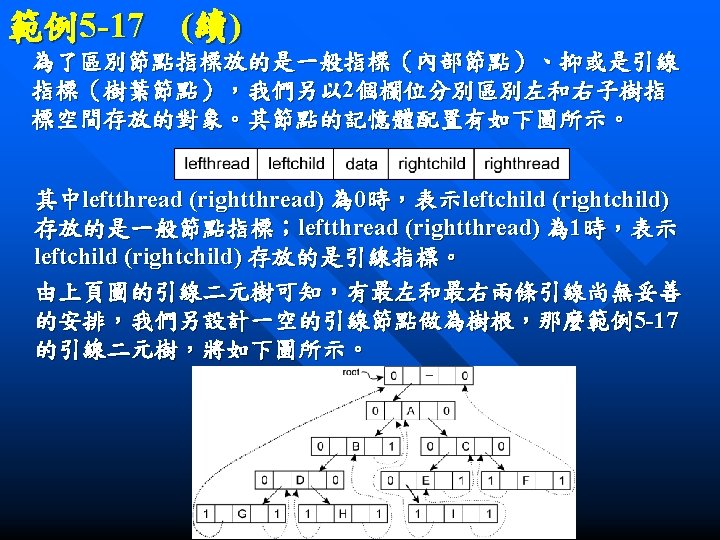
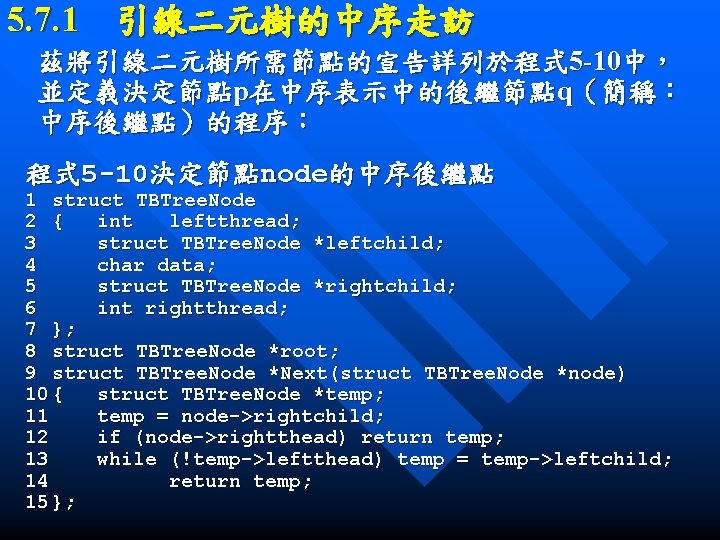
5. 7. 1 引線二元樹的中序走訪 茲將引線二元樹所需節點的宣告詳列於程式 5 -10中, 並定義決定節點p在中序表示中的後繼節點q(簡稱: 中序後繼點)的程序: 程式 5 -10決定節點node的中序後繼點 1 struct TBTree. Node 2 { int leftthread; 3 struct TBTree. Node *leftchild; 4 char data; 5 struct TBTree. Node *rightchild; 6 int rightthread; 7 }; 8 struct TBTree. Node *root; 9 struct TBTree. Node *Next(struct TBTree. Node *node) 10 { struct TBTree. Node *temp; 11 temp = node->rightchild; 12 if (node->rightthead) return temp; 13 while (!temp->leftthead) temp = temp->leftchild; 14 return temp; 15 };
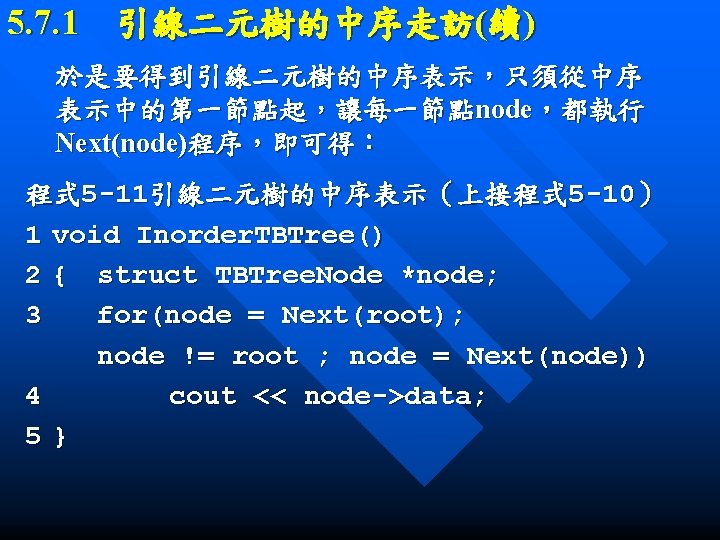
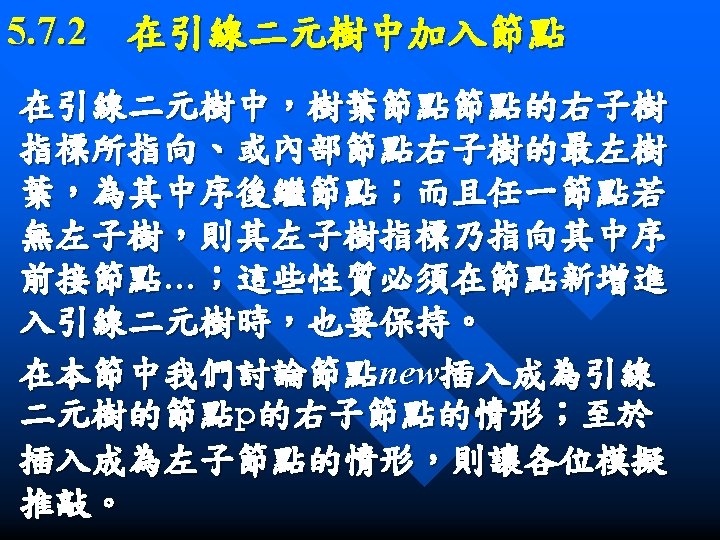
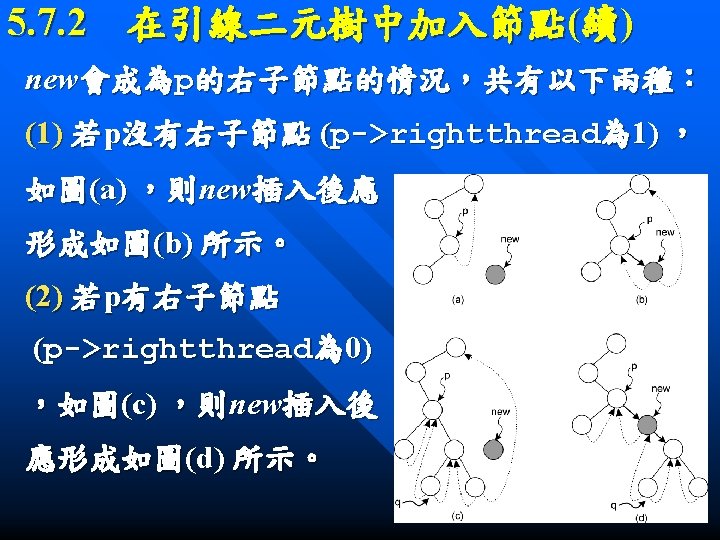
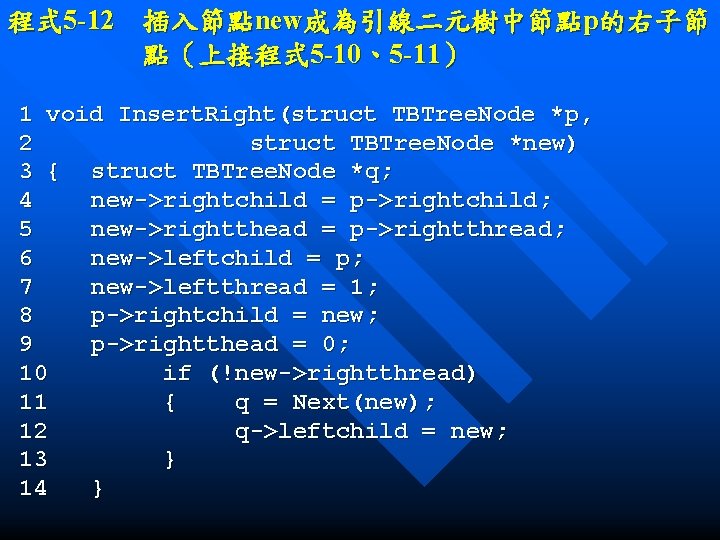
程式 5 -12 插入節點new成為引線二元樹中節點p的右子節 點(上接程式 5 -10、5 -11) 1 void Insert. Right(struct TBTree. Node *p, 2 struct TBTree. Node *new) 3 { struct TBTree. Node *q; 4 new->rightchild = p->rightchild; 5 new->rightthead = p->rightthread; 6 new->leftchild = p; 7 new->leftthread = 1; 8 p->rightchild = new; 9 p->rightthead = 0; 10 if (!new->rightthread) 11 { q = Next(new); 12 q->leftchild = new; 13 } 14 }
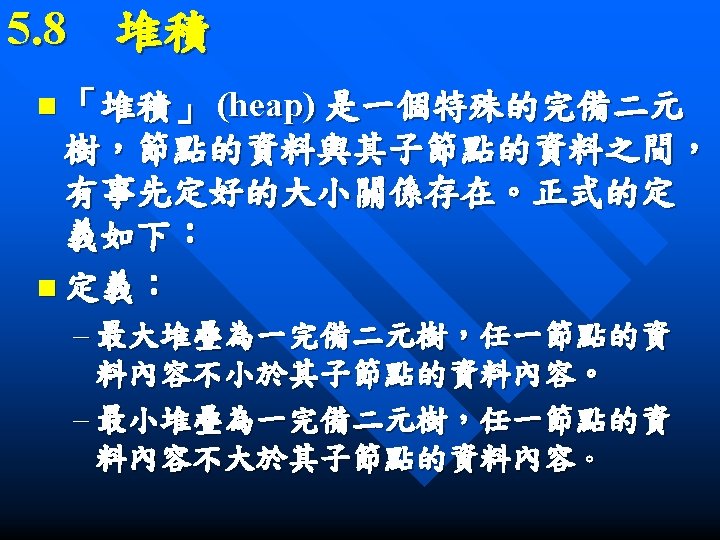
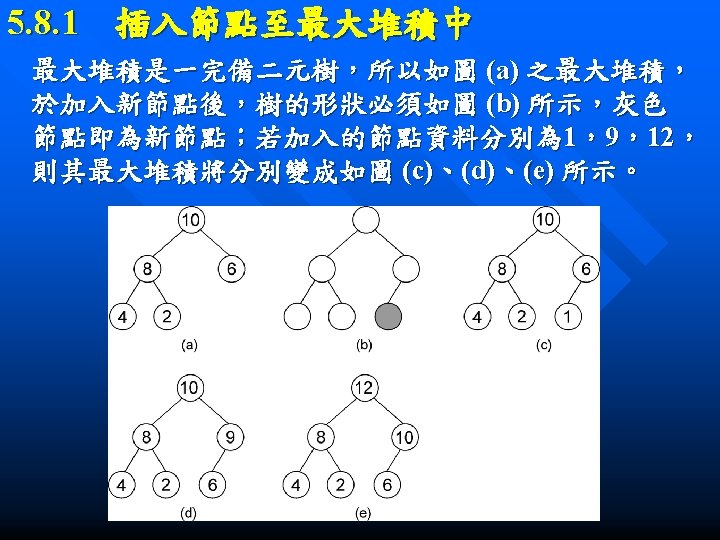
![程式 5 13新增節點至最大堆積中 最大堆積本身是一棵完備二元樹可以用陣列表示之 下面是新增節點至最大堆積的程序 1 define maxsize 100 2 int heapmaxsize 3 程式 5 -13新增節點至最大堆積中 最大堆積本身是一棵完備二元樹,可以用陣列表示之 。 下面是新增節點至最大堆積的程序。 1 define maxsize 100 2 int heap[maxsize]; 3](https://slidetodoc.com/presentation_image/00ee7c080475d099b06216a957179abe/image-37.jpg)
程式 5 -13新增節點至最大堆積中 最大堆積本身是一棵完備二元樹,可以用陣列表示之 。 下面是新增節點至最大堆積的程序。 1 define maxsize 100 2 int heap[maxsize]; 3 int n, i; 4 void Insert. Heap(int x) 5 { if (n == maxsize) Heap. Full(); 6 else 7 { n++; 8 i = n; 9 while ((i >= 1) && (x > heap[i/2])) 10 { heap[i] = heap[i/2]; 11 i /= 2; 12 } 13 heap[i] = x; 14 } 16 }
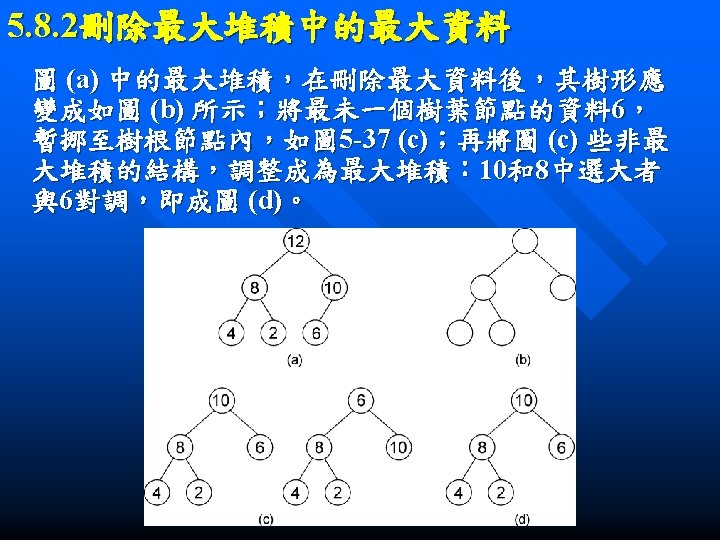
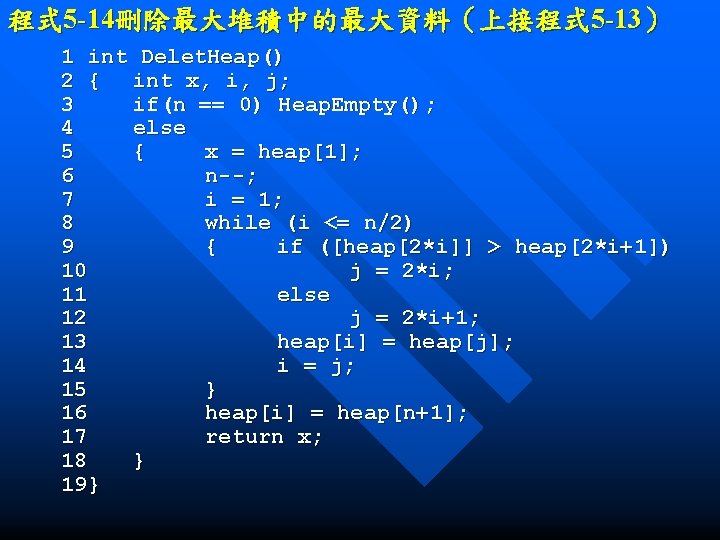
程式 5 -14刪除最大堆積中的最大資料(上接程式 5 -13) 1 int Delet. Heap() 2 { int x, i, j; 3 if(n == 0) Heap. Empty(); 4 else 5 { x = heap[1]; 6 n--; 7 i = 1; 8 while (i <= n/2) 9 { if ([heap[2*i]] > heap[2*i+1]) 10 j = 2*i; 11 else 12 j = 2*i+1; 13 heap[i] = heap[j]; 14 i = j; 15 } 16 heap[i] = heap[n+1]; 17 return x; 18 } 19}
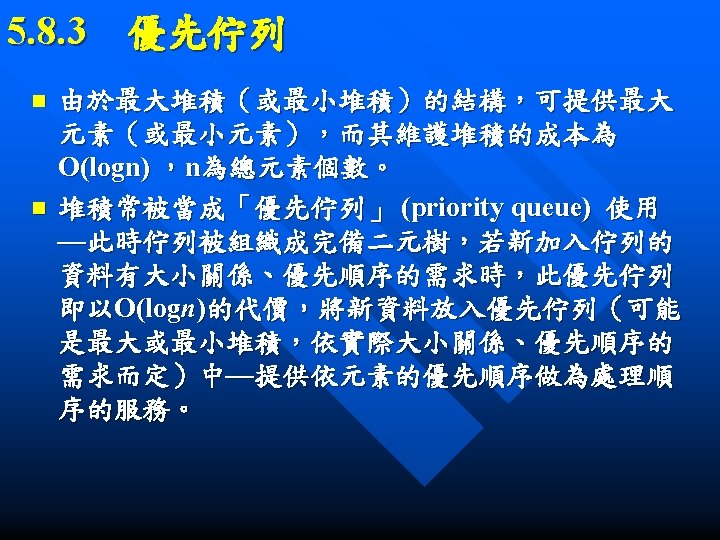
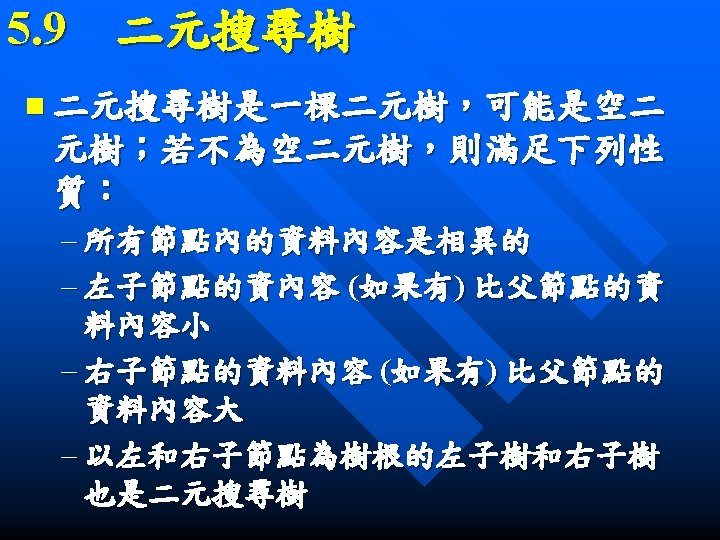
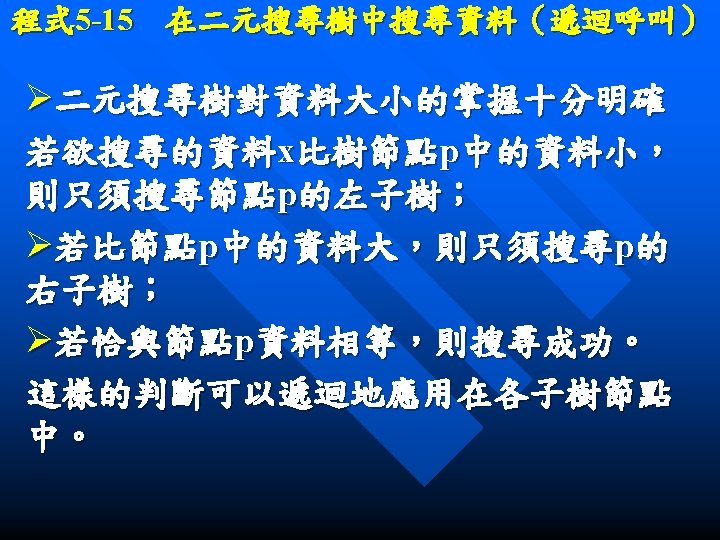
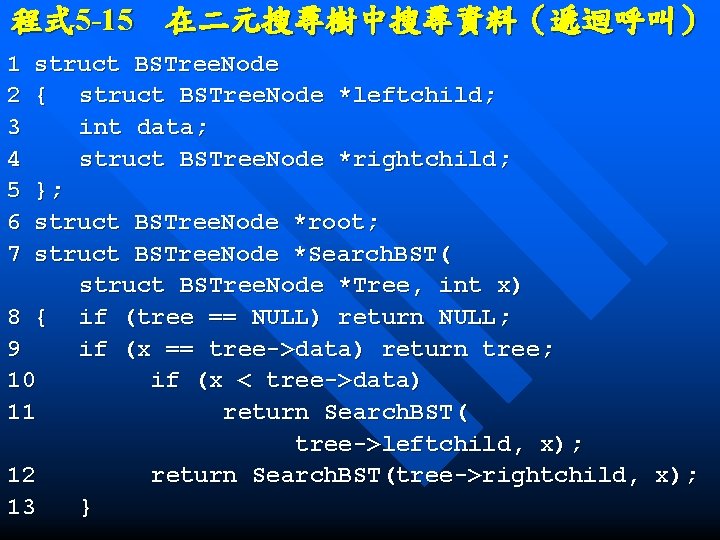
程式 5 -15 在二元搜尋樹中搜尋資料(遞迴呼叫) 1 2 3 4 5 6 7 struct BSTree. Node { struct BSTree. Node *leftchild; int data; struct BSTree. Node *rightchild; }; struct BSTree. Node *root; struct BSTree. Node *Search. BST( struct BSTree. Node *Tree, int x) 8 { if (tree == NULL) return NULL; 9 if (x == tree->data) return tree; 10 if (x < tree->data) 11 return Search. BST( tree->leftchild, x); 12 return Search. BST(tree->rightchild, x); 13 }
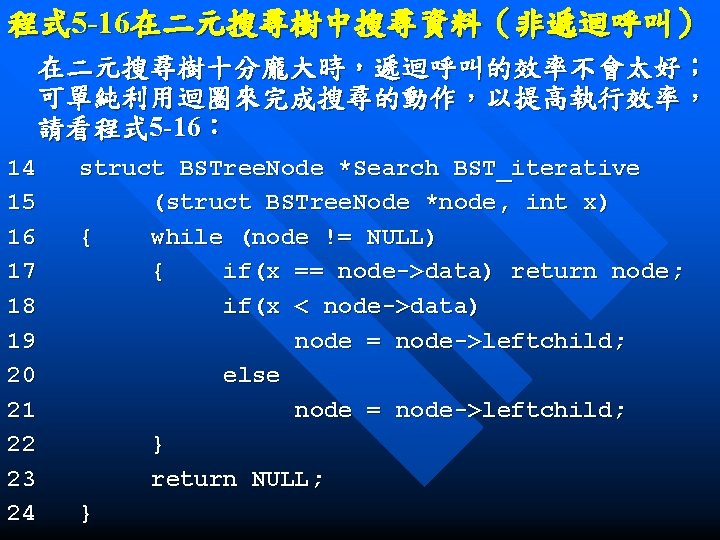
程式 5 -16在二元搜尋樹中搜尋資料(非遞迴呼叫) 在二元搜尋樹十分龐大時,遞迴呼叫的效率不會太好; 可單純利用迴圈來完成搜尋的動作,以提高執行效率, 請看程式 5 -16: 14 15 16 17 18 19 20 21 22 23 24 struct BSTree. Node *Search BST_iterative (struct BSTree. Node *node, int x) { while (node != NULL) { if(x == node->data) return node; if(x < node->data) node = node->leftchild; else node = node->leftchild; } return NULL; }
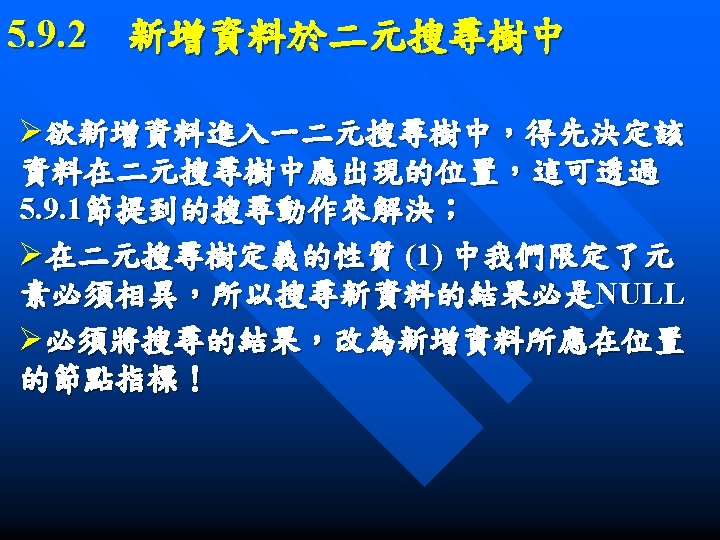
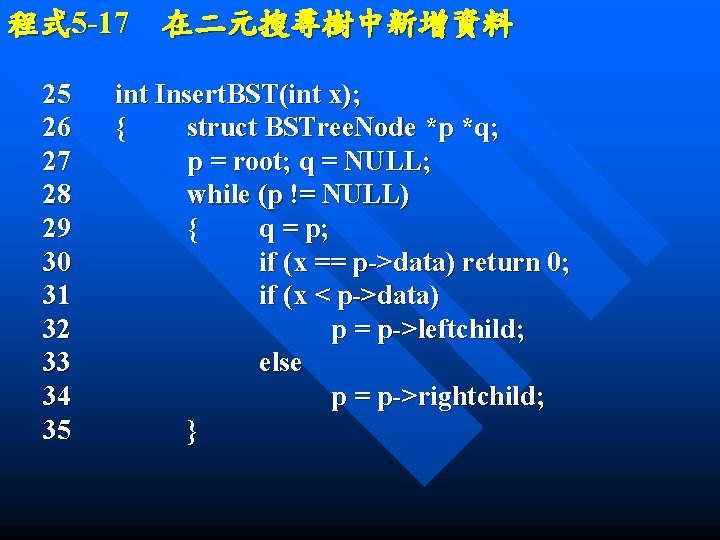
程式 5 -17 在二元搜尋樹中新增資料 25 26 27 28 29 30 31 32 33 34 35 int Insert. BST(int x); { struct BSTree. Node *p *q; p = root; q = NULL; while (p != NULL) { q = p; if (x == p->data) return 0; if (x < p->data) p = p->leftchild; else p = p->rightchild; }
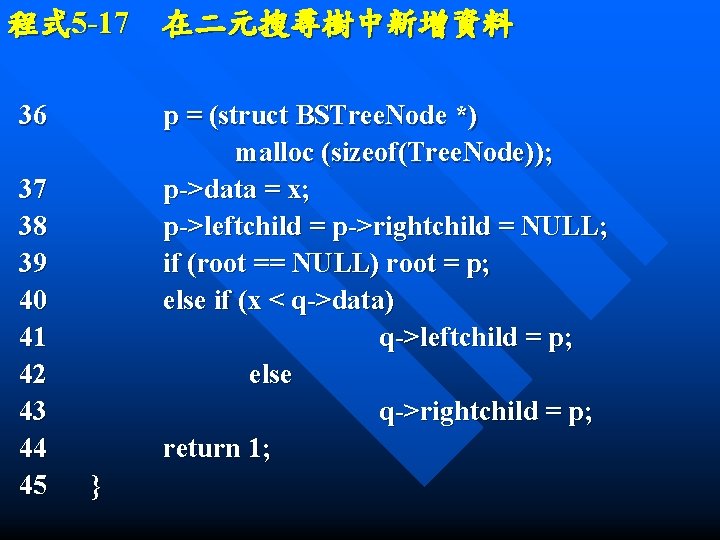
程式 5 -17 在二元搜尋樹中新增資料 36 37 38 39 40 41 42 43 44 45 p = (struct BSTree. Node *) malloc (sizeof(Tree. Node)); p->data = x; p->leftchild = p->rightchild = NULL; if (root == NULL) root = p; else if (x < q->data) q->leftchild = p; else q->rightchild = p; return 1; }
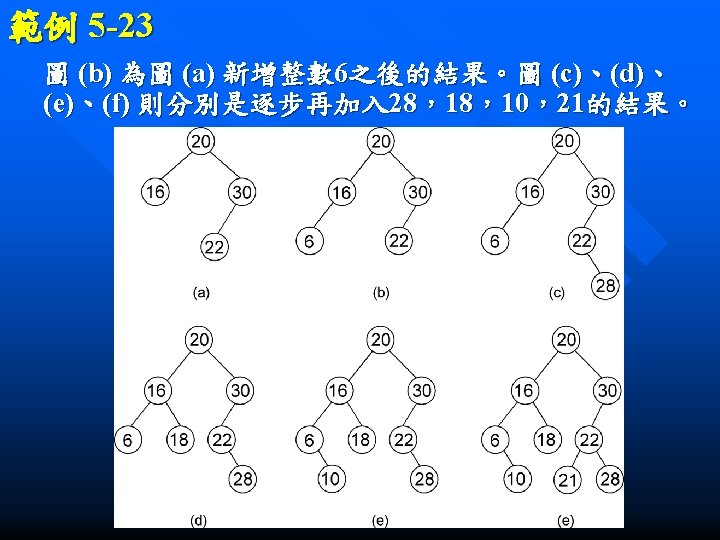
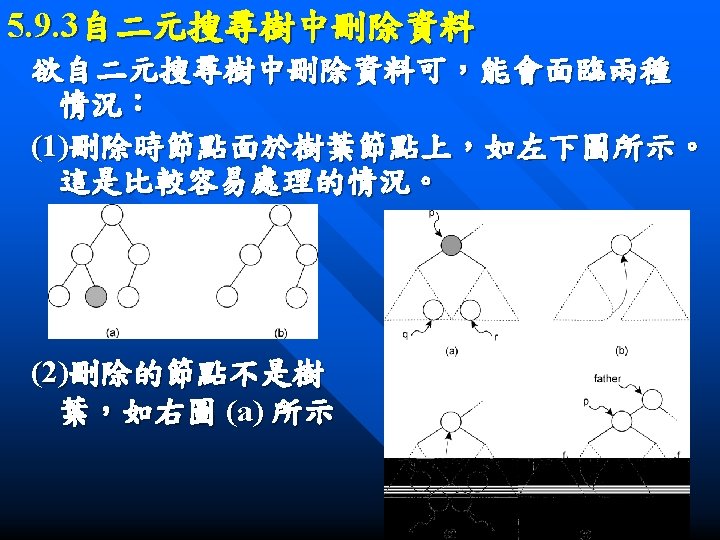
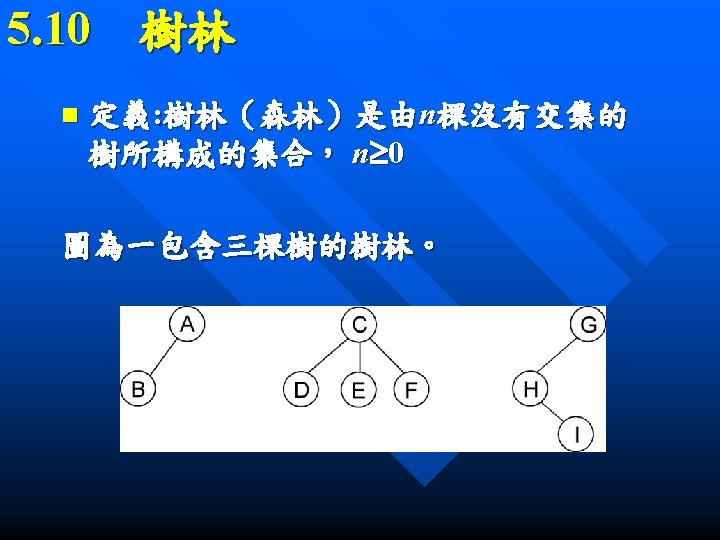
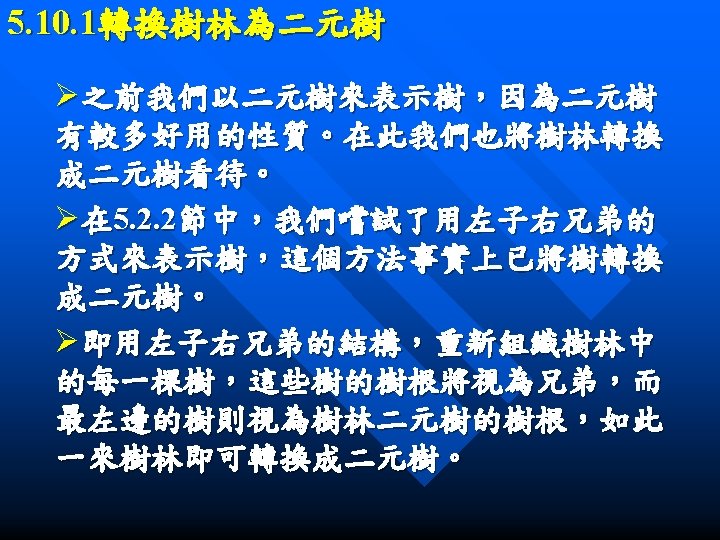
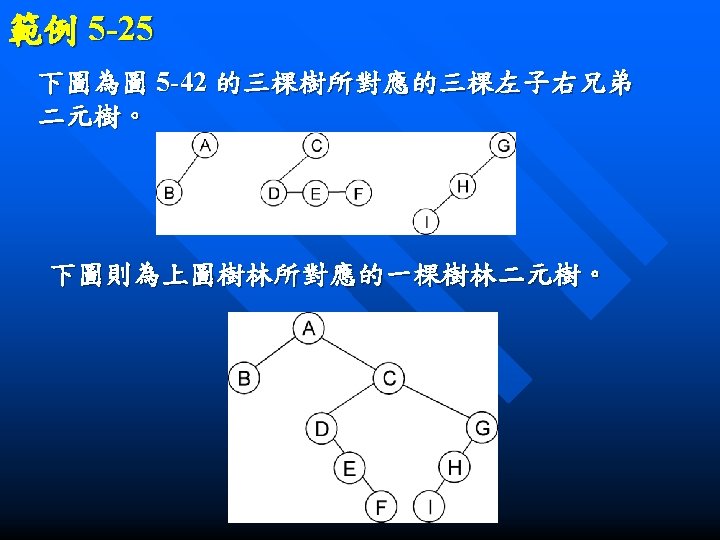
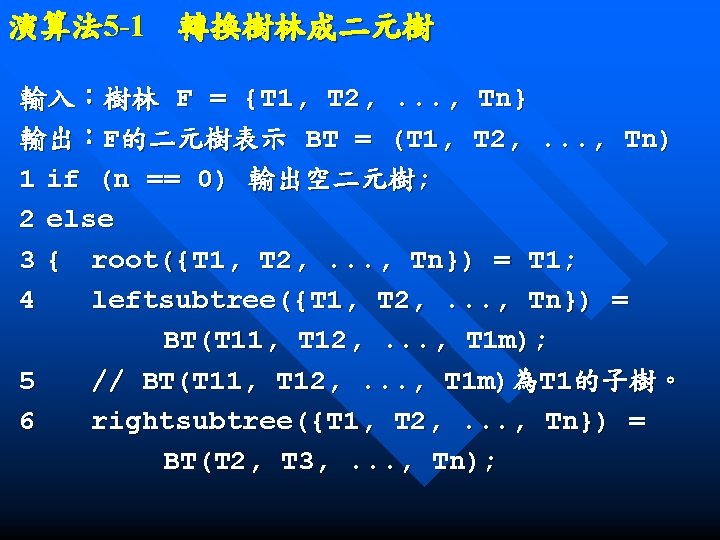
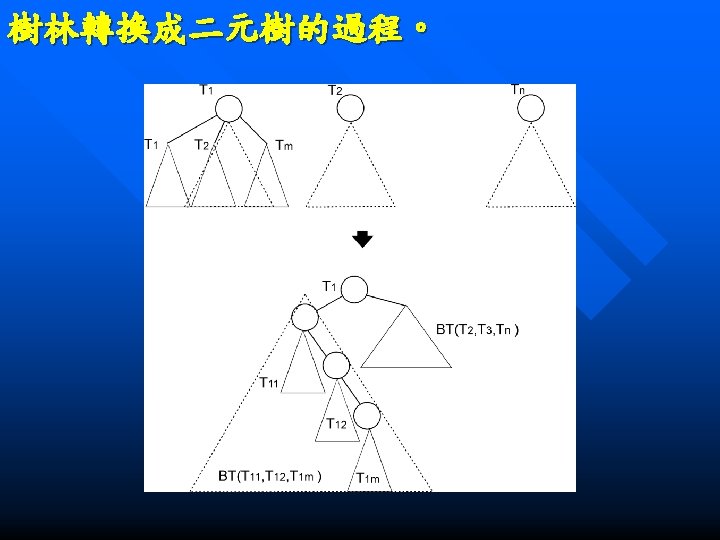