45 Directory Handling Overview Example Program Fuctions mkdir
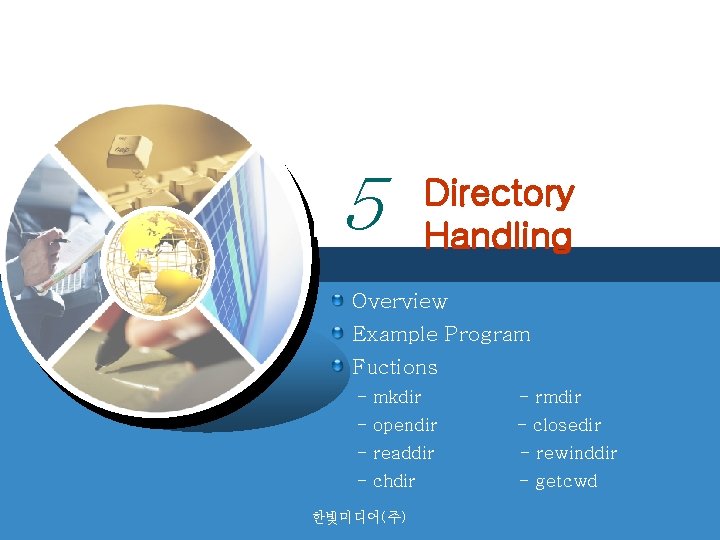
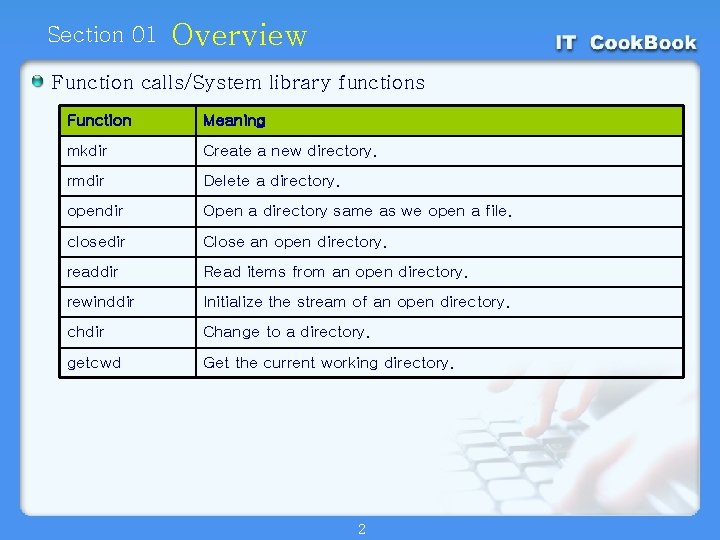
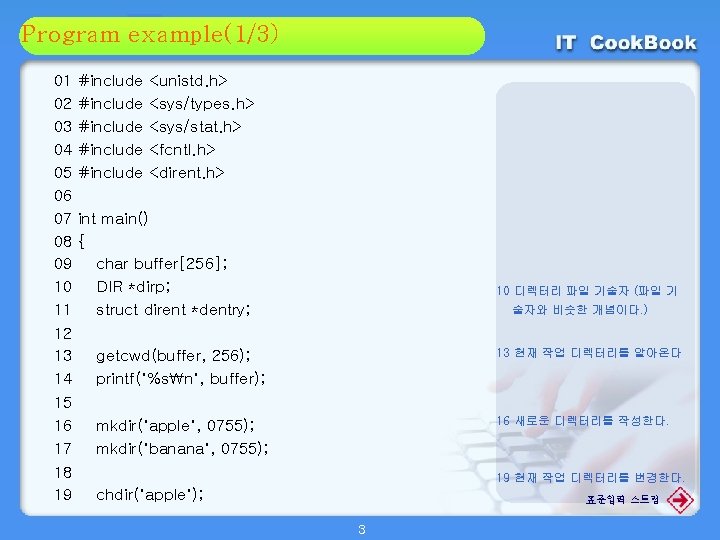
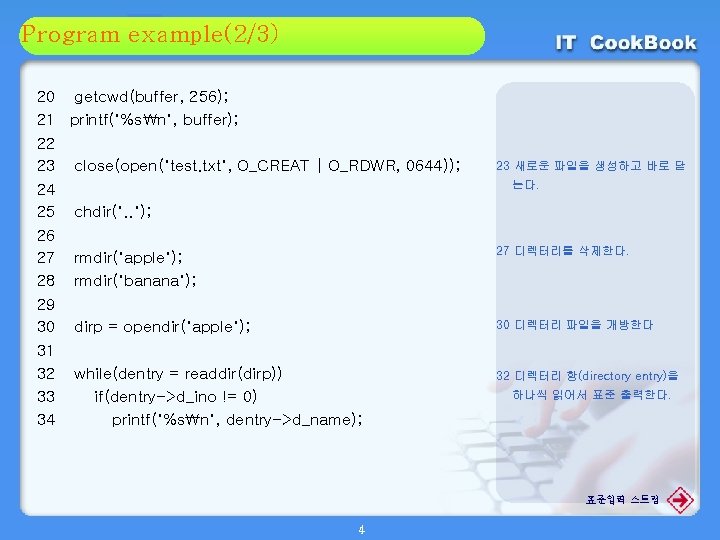
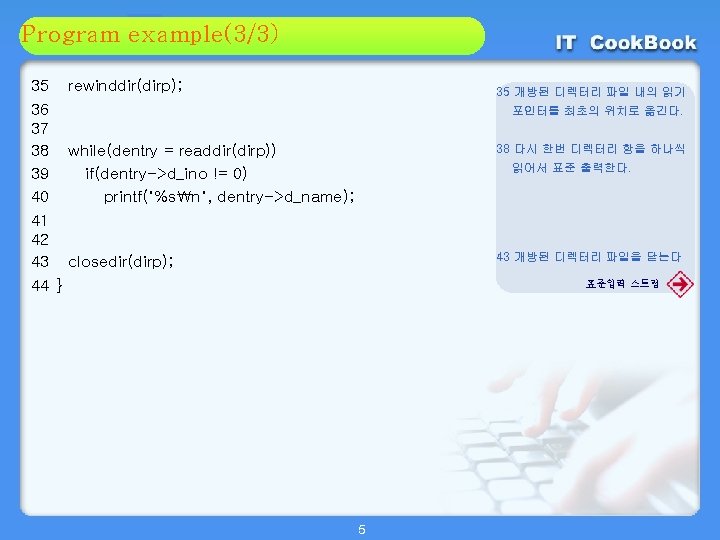
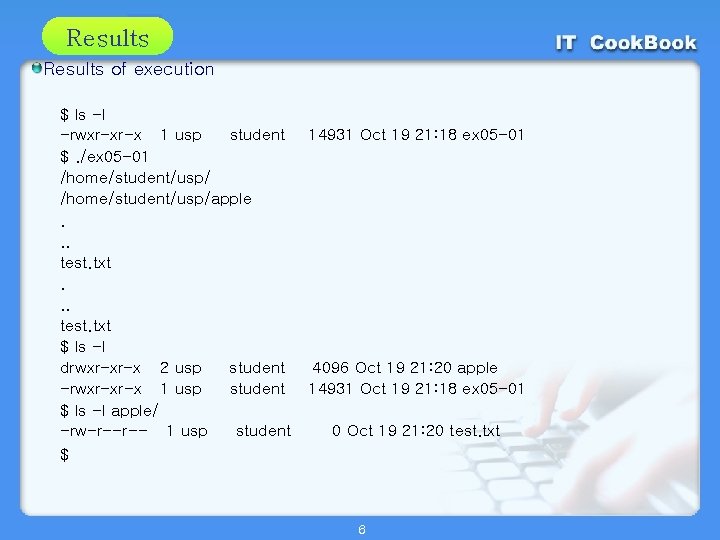
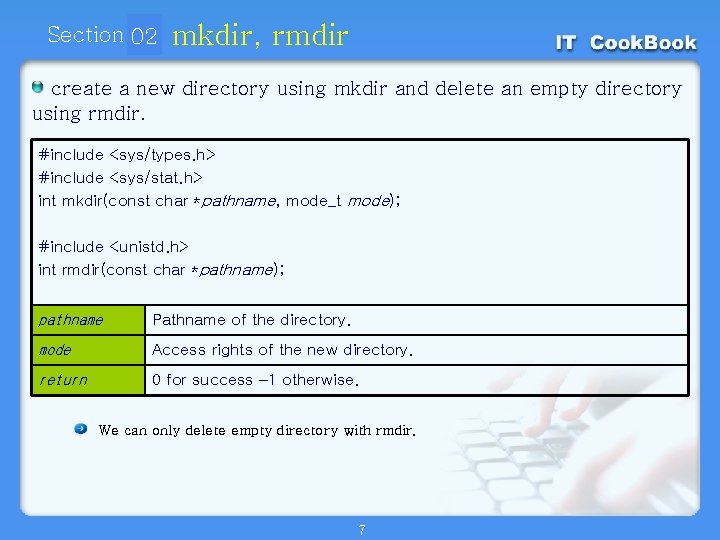
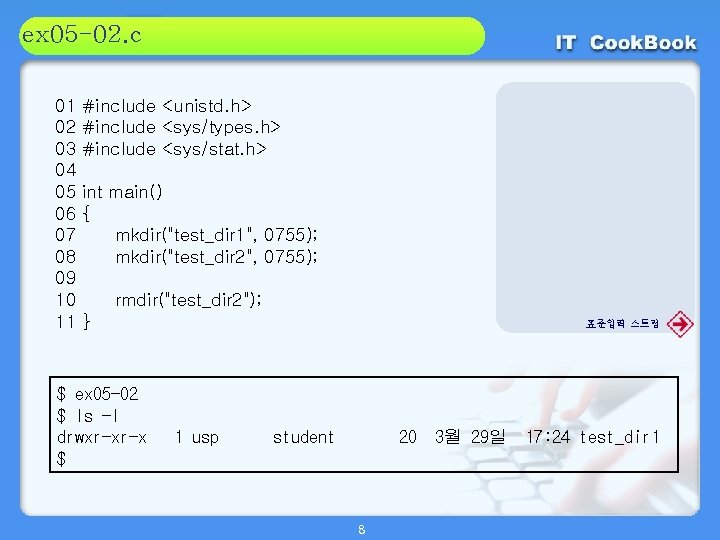
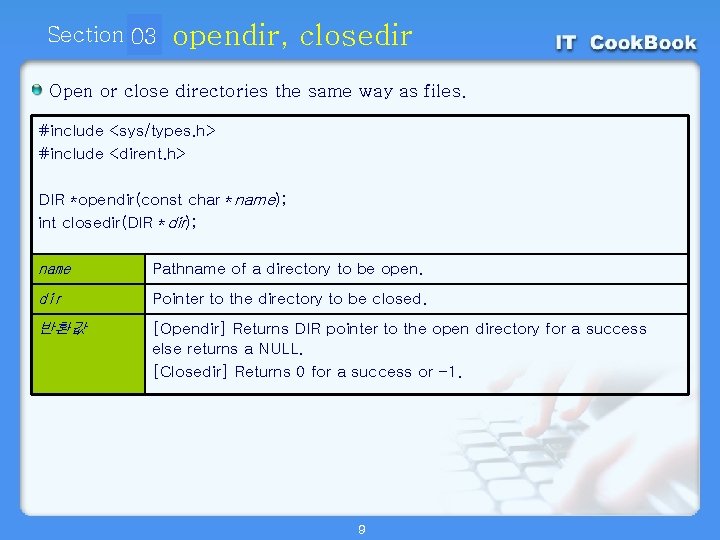
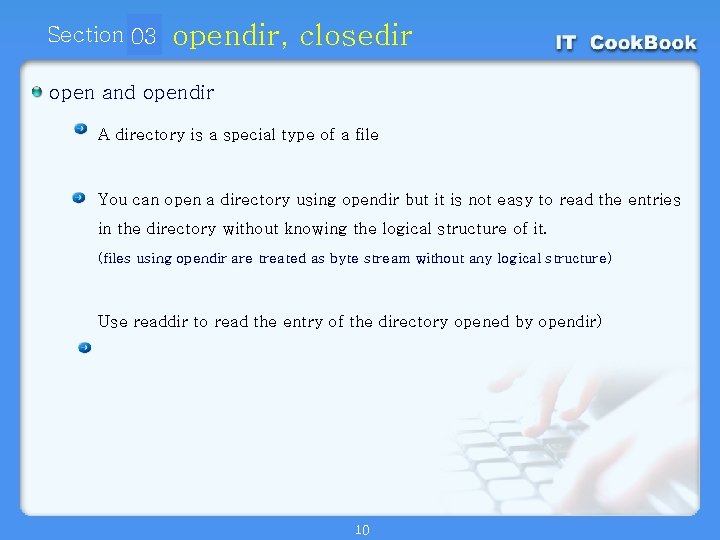
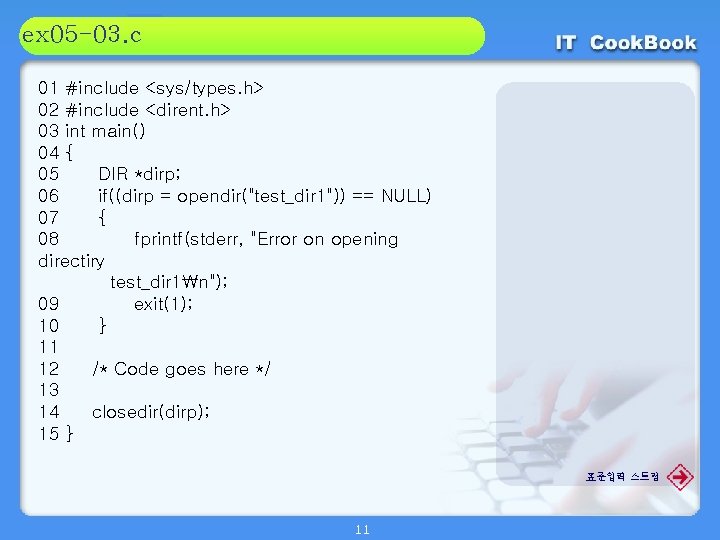
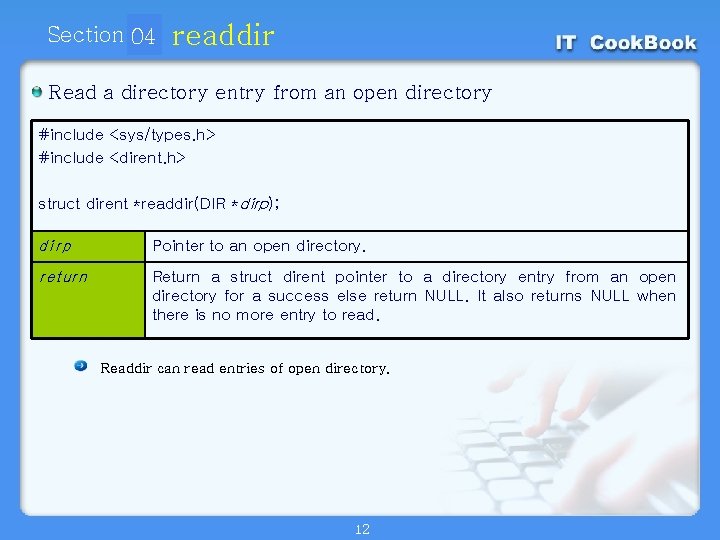
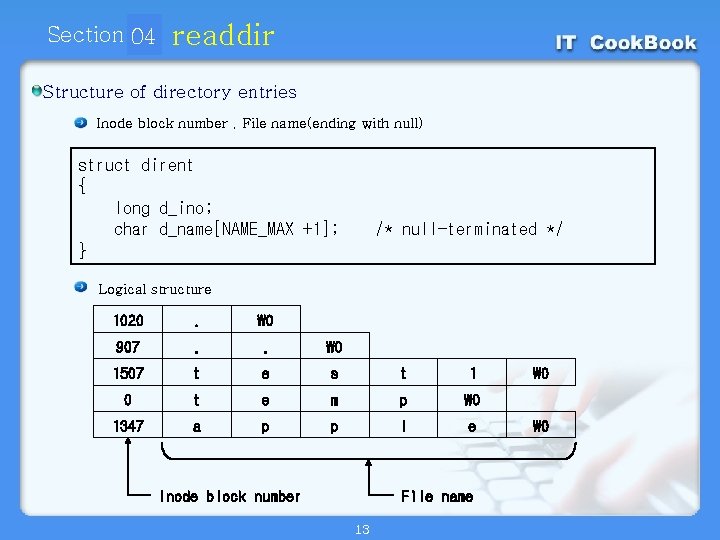
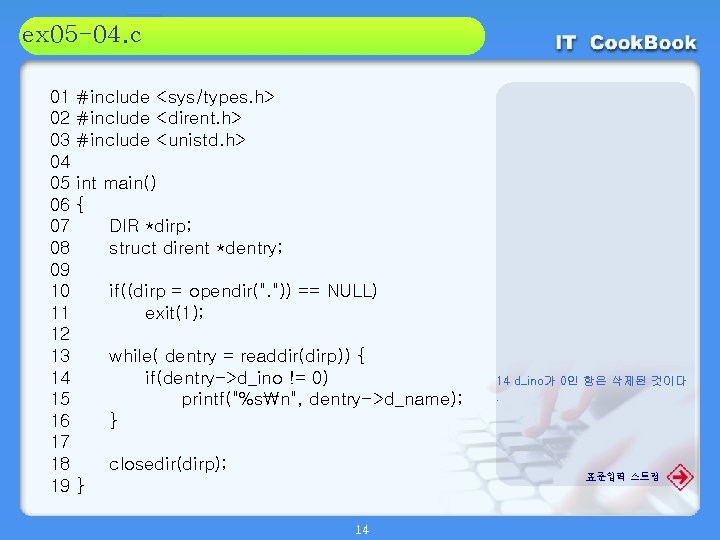
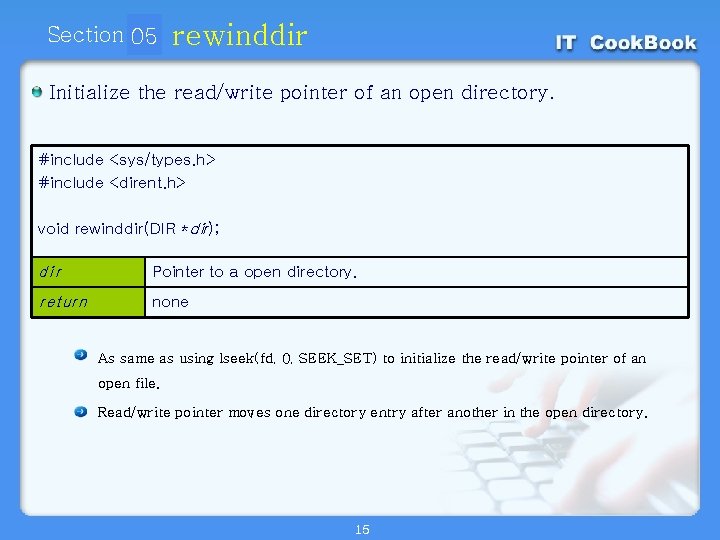
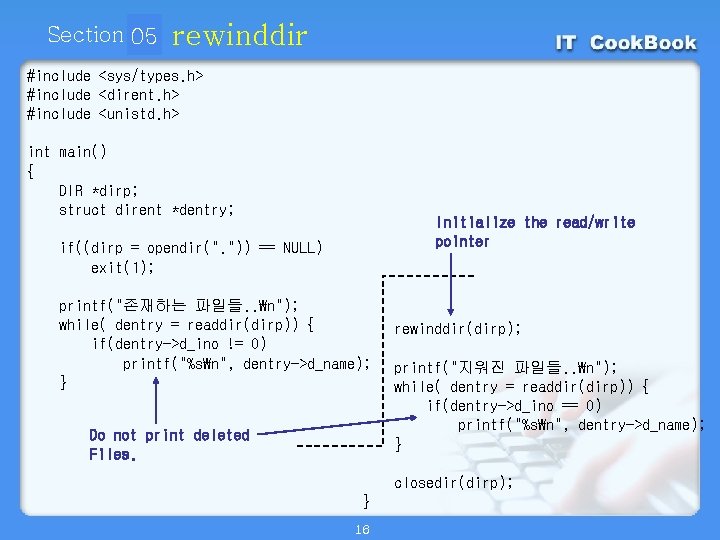
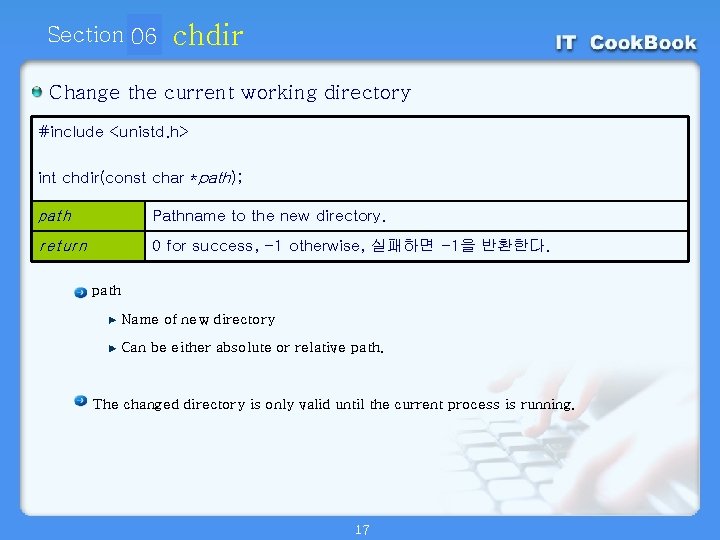
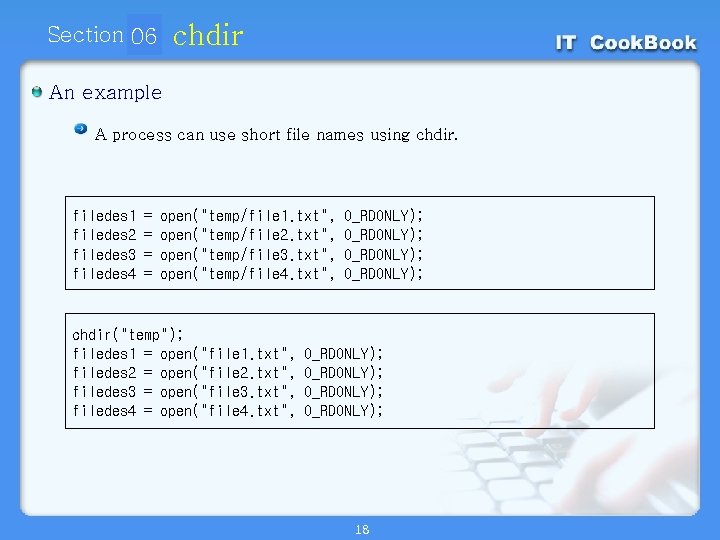
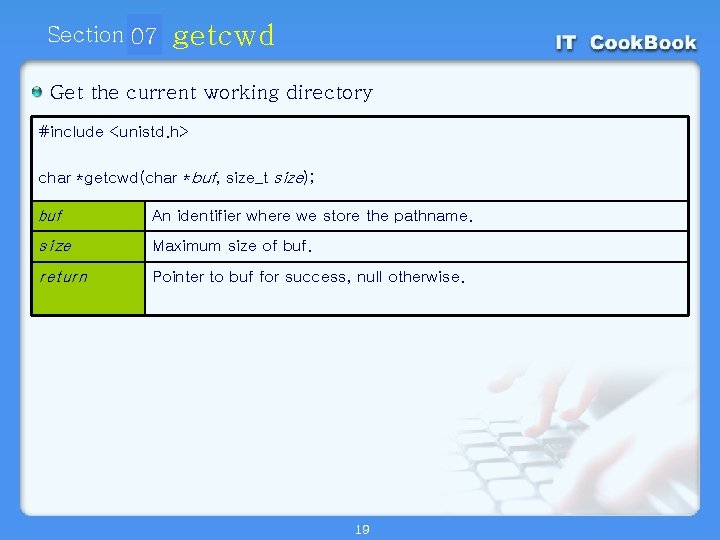
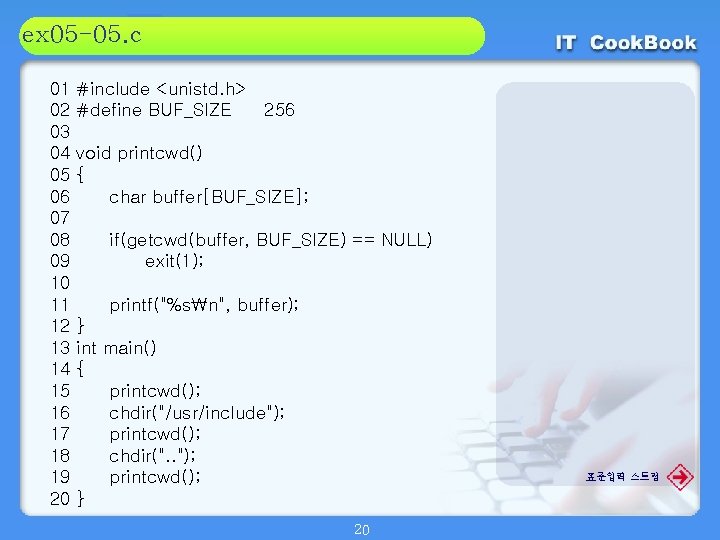
- Slides: 20
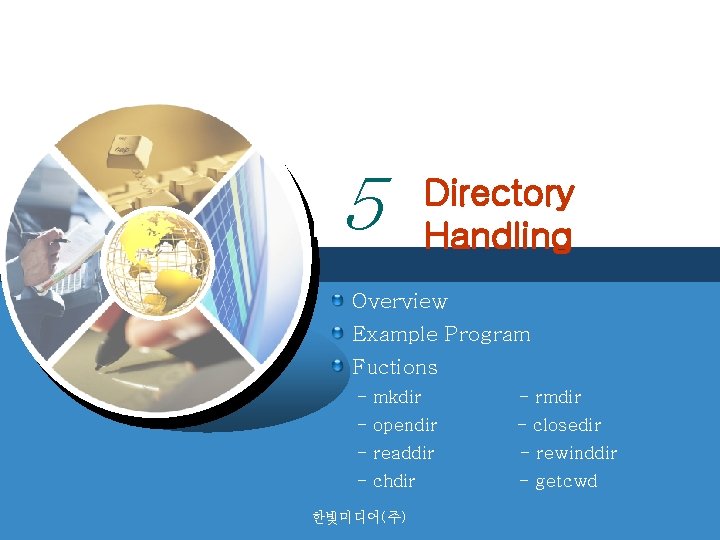
45 Directory 장 Handling Overview Example Program Fuctions - mkdir - rmdir - opendir - closedir - readdir - rewinddir - chdir - getcwd 한빛미디어(주)
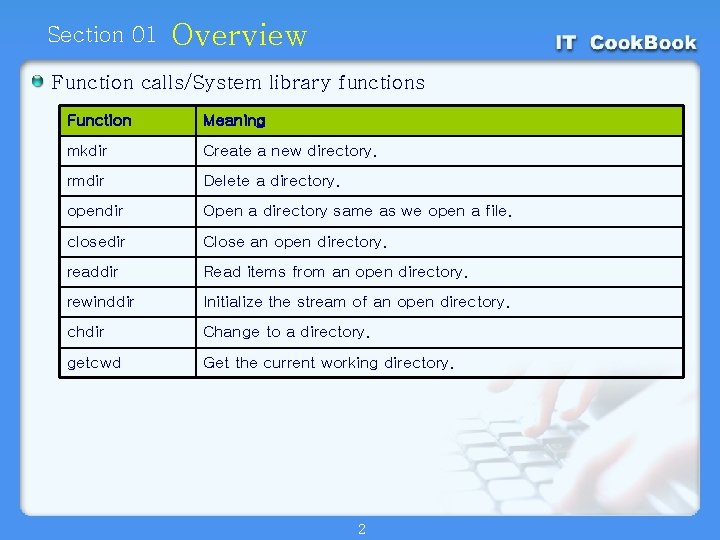
Section 01 Overview Function calls/System library functions Function Meaning mkdir Create a new directory. rmdir Delete a directory. opendir Open a directory same as we open a file. closedir Close an open directory. readdir Read items from an open directory. rewinddir Initialize the stream of an open directory. chdir Change to a directory. getcwd Get the current working directory. 2
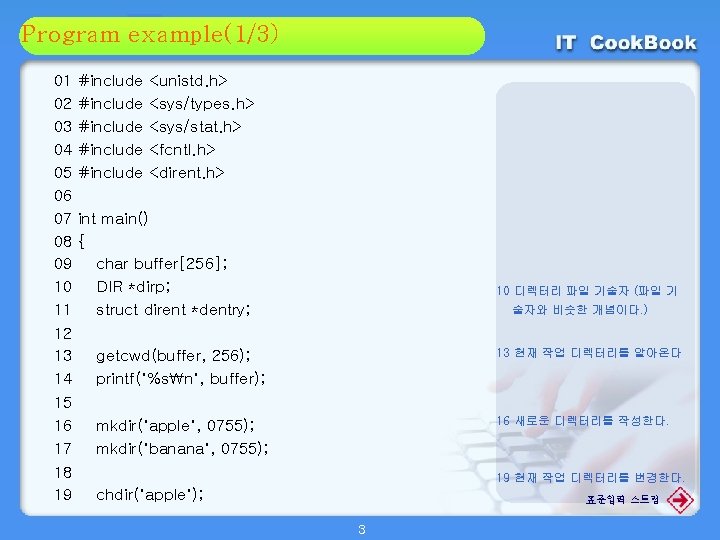
Section example(1/3) 01 Program 02 01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 #include #include <unistd. h> <sys/types. h> <sys/stat. h> <fcntl. h> <dirent. h> int main() { char buffer[256]; DIR *dirp; struct dirent *dentry; 10 디렉터리 파일 기술자 (파일 기 술자와 비슷한 개념이다. ) getcwd(buffer, 256); printf("%sn", buffer); 13 현재 작업 디렉터리를 알아온다 mkdir("apple", 0755); mkdir("banana", 0755); 16 새로운 디렉터리를 작성한다. 19 현재 작업 디렉터리를 변경한다. chdir("apple"); 표준입력 스트림 3
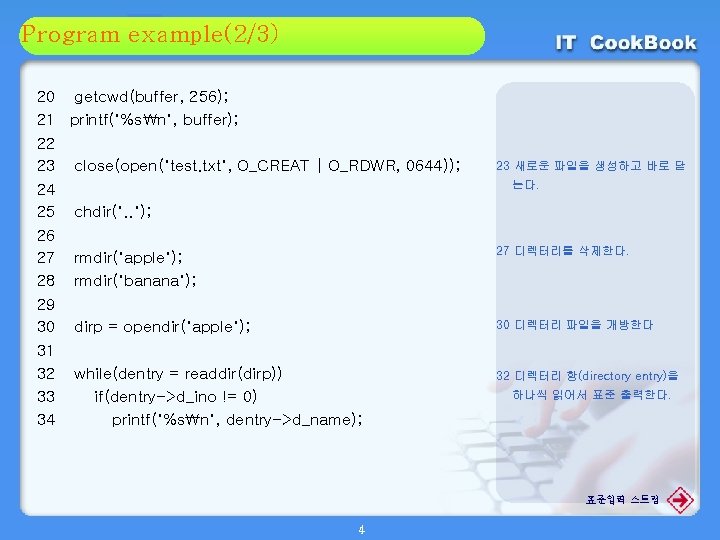
Section example(2/3) 01 Program 02 20 getcwd(buffer, 256); 21 printf("%sn", buffer); 22 23 close(open("test. txt", O_CREAT | O_RDWR, 0644)); 24 25 chdir(". . "); 26 27 rmdir("apple"); 28 rmdir("banana"); 29 30 dirp = opendir("apple"); 31 32 while(dentry = readdir(dirp)) 33 if(dentry->d_ino != 0) 34 printf("%sn", dentry->d_name); 23 새로운 파일을 생성하고 바로 닫 는다. 27 디렉터리를 삭제한다. 30 디렉터리 파일을 개방한다 32 디렉터리 항(directory entry)을 하나씩 읽어서 표준 출력한다. 표준입력 스트림 4
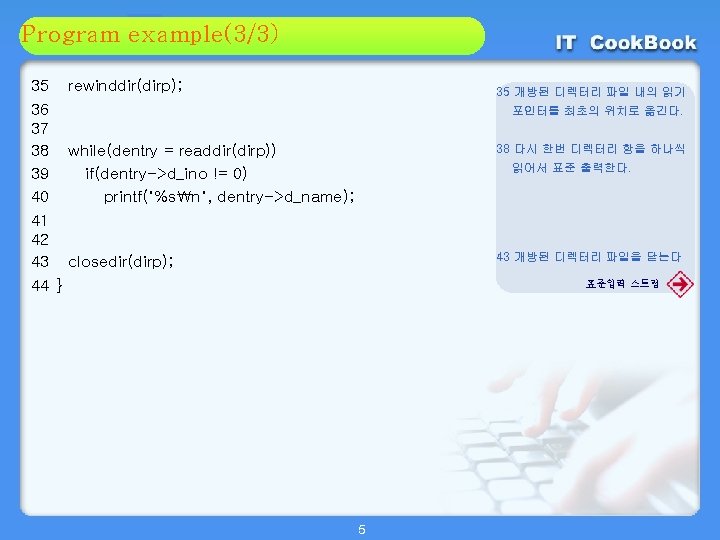
Section example(3/3) 01 Program 02 35 rewinddir(dirp); 36 37 38 while(dentry = readdir(dirp)) 39 if(dentry->d_ino != 0) 40 printf("%sn", dentry->d_name); 41 42 43 closedir(dirp); 44 } 35 개방된 디렉터리 파일 내의 읽기 포인터를 최초의 위치로 옮긴다. 38 다시 한번 디렉터리 항을 하나씩 읽어서 표준 출력한다. 43 개방된 디렉터리 파일을 닫는다 표준입력 스트림 5
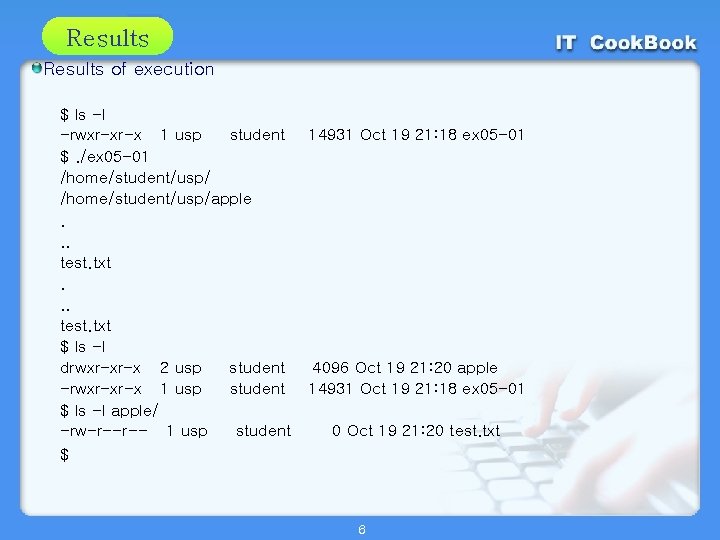
Section 01 08 05 Results of execution $ ls -l -rwxr-xr-x 1 usp student 14931 Oct 19 21: 18 ex 05 -01 $. /ex 05 -01 /home/student/usp/apple. . . test. txt $ ls -l drwxr-xr-x 2 usp student 4096 Oct 19 21: 20 apple -rwxr-xr-x 1 usp student 14931 Oct 19 21: 18 ex 05 -01 $ ls -l apple/ -rw-r--r-- 1 usp student 0 Oct 19 21: 20 test. txt $ 6
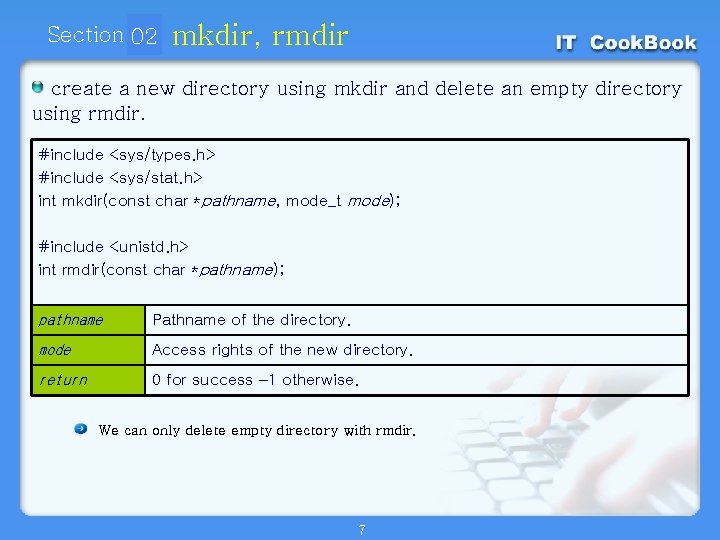
Section 02 01 mkdir, rmdir create a new directory using mkdir and delete an empty directory using rmdir. #include <sys/types. h> #include <sys/stat. h> int mkdir(const char *pathname, mode_t mode); #include <unistd. h> int rmdir(const char *pathname); pathname Pathname of the directory. mode Access rights of the new directory. return 0 for success – 1 otherwise. We can only delete empty directory with rmdir. 7
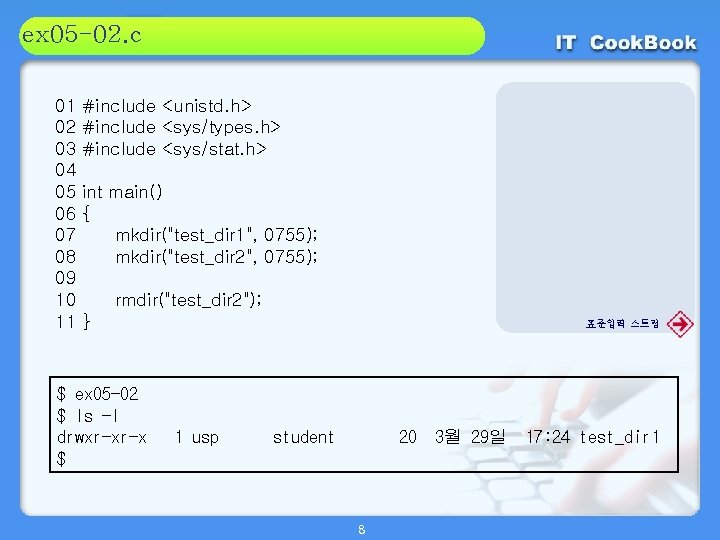
Section 02 01 ex 05 -02. c 01 02 03 04 05 06 07 08 09 10 11 #include <unistd. h> #include <sys/types. h> #include <sys/stat. h> int main() { mkdir("test_dir 1", 0755); mkdir("test_dir 2", 0755); rmdir("test_dir 2"); } 표준입력 스트림 $ ex 05 -02 $ ls -l drwxr-xr-x 1 usp student 20 3월 29일 17: 24 test_dir 1 $ 8
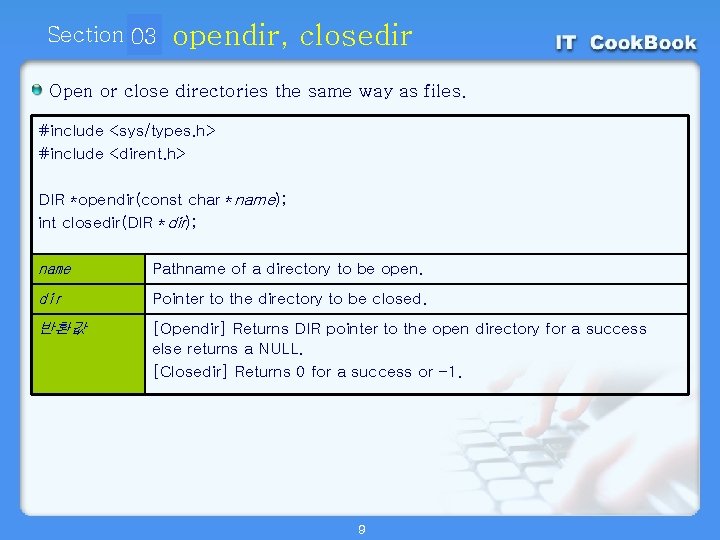
Section 03 01 opendir, closedir Open or close directories the same way as files. #include <sys/types. h> #include <dirent. h> DIR *opendir(const char *name); int closedir(DIR *dir); name Pathname of a directory to be open. dir Pointer to the directory to be closed. 반환값 [Opendir] Returns DIR pointer to the open directory for a success else returns a NULL. [Closedir] Returns 0 for a success or -1. 9
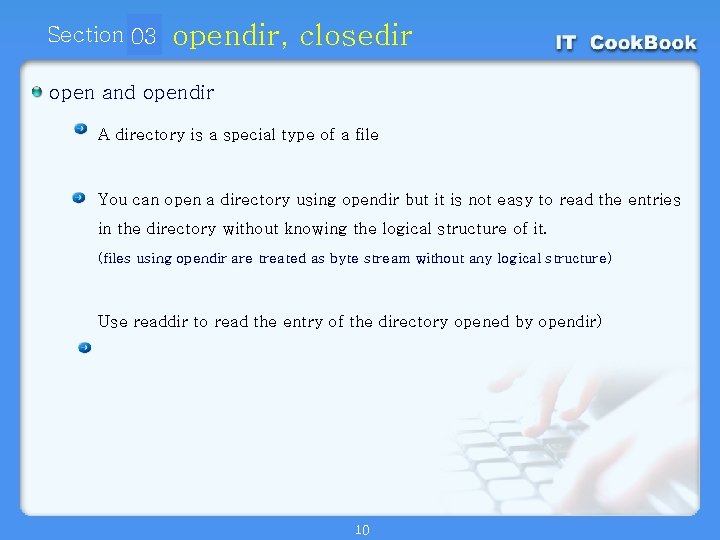
Section 03 01 opendir, closedir open and opendir A directory is a special type of a file You can open a directory using opendir but it is not easy to read the entries in the directory without knowing the logical structure of it. (files using opendir are treated as byte stream without any logical structure) Use readdir to read the entry of the directory opened by opendir) 10
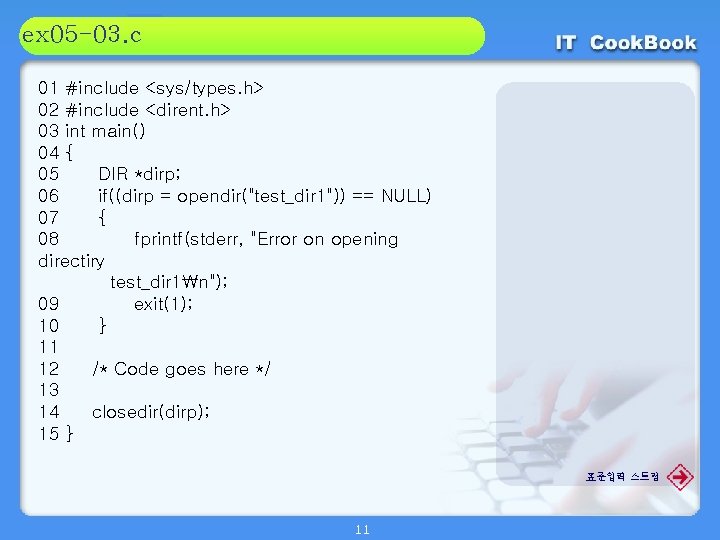
Section 02 01 ex 05 -03. c 01 #include <sys/types. h> 02 #include <dirent. h> 03 int main() 04 { 05 DIR *dirp; 06 if((dirp = opendir("test_dir 1")) == NULL) 07 { 08 fprintf(stderr, "Error on opening directiry test_dir 1n"); 09 exit(1); 10 } 11 12 /* Code goes here */ 13 14 closedir(dirp); 15 } 표준입력 스트림 11
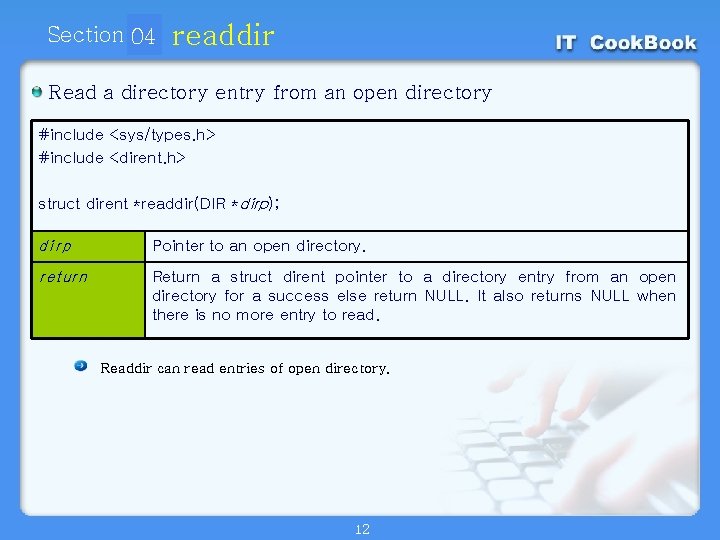
Section 04 01 readdir Read a directory entry from an open directory #include <sys/types. h> #include <dirent. h> struct dirent *readdir(DIR *dirp); dirp Pointer to an open directory. return Return a struct dirent pointer to a directory entry from an open directory for a success else return NULL. It also returns NULL when there is no more entry to read. Readdir can read entries of open directory. 12
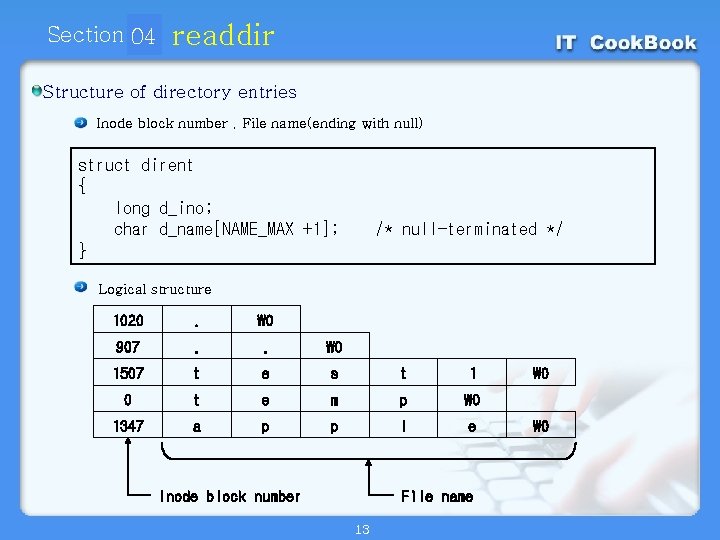
Section 04 01 readdir Structure of directory entries Inode block number , File name(ending with null) struct dirent { long d_ino; char d_name[NAME_MAX +1]; /* null-terminated */ } Logical structure 1020 . ₩ 0 907 . . ₩ 0 1507 t e s t 1 ₩ 0 0 t e m p ₩ 0 1347 a p p l e ₩ 0 Inode block number File name 13
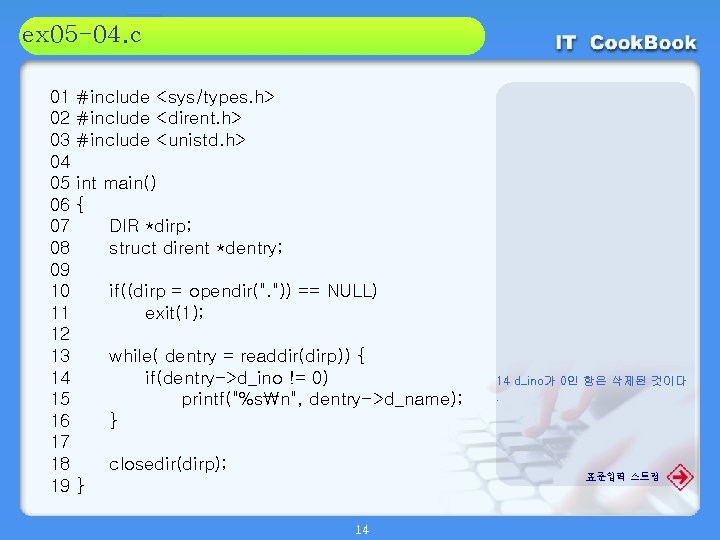
Section 02 01 ex 05 -04. c 01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 #include <sys/types. h> #include <dirent. h> #include <unistd. h> int main() { DIR *dirp; struct dirent *dentry; if((dirp = opendir(". ")) == NULL) exit(1); while( dentry = readdir(dirp)) { if(dentry->d_ino != 0) printf("%sn", dentry->d_name); } closedir(dirp); } 14 d_ino가 0인 항은 삭제된 것이다. 표준입력 스트림 14
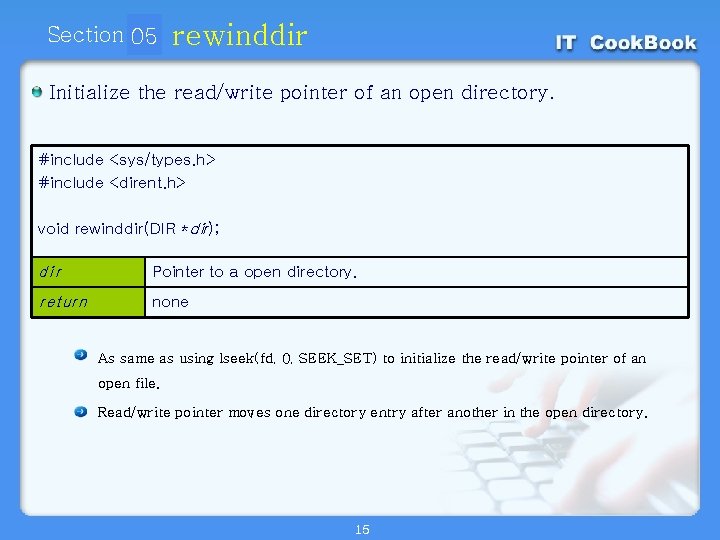
Section 05 01 rewinddir Initialize the read/write pointer of an open directory. #include <sys/types. h> #include <dirent. h> void rewinddir(DIR *dir); dir Pointer to a open directory. return none As same as using lseek(fd, 0, SEEK_SET) to initialize the read/write pointer of an open file. Read/write pointer moves one directory entry after another in the open directory. 15
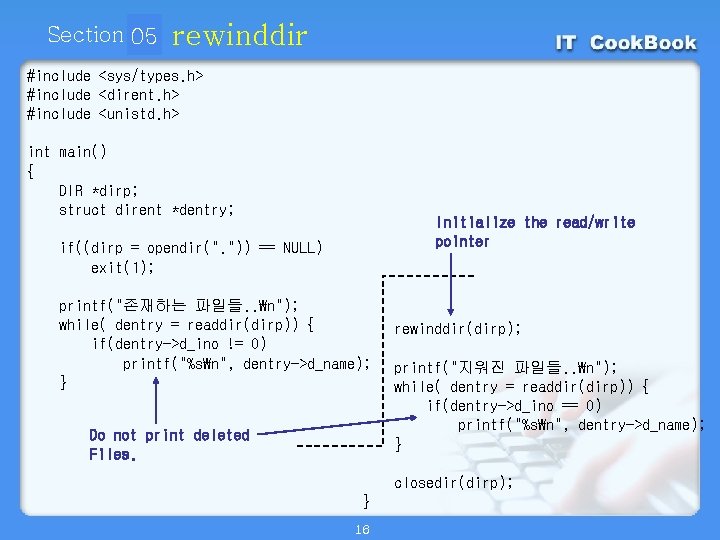
Section 05 01 rewinddir #include <sys/types. h> #include <dirent. h> #include <unistd. h> int main() { DIR *dirp; struct dirent *dentry; Initialize the read/write pointer if((dirp = opendir(". ")) == NULL) exit(1); printf("존재하는 파일들. . ₩n"); while( dentry = readdir(dirp)) { rewinddir(dirp); if(dentry->d_ino != 0) printf("%s₩n", dentry->d_name); printf("지워진 파일들. . ₩n"); } while( dentry = readdir(dirp)) { if(dentry->d_ino == 0) printf("%s₩n", dentry->d_name); Do not print deleted } Files. closedir(dirp); } 16
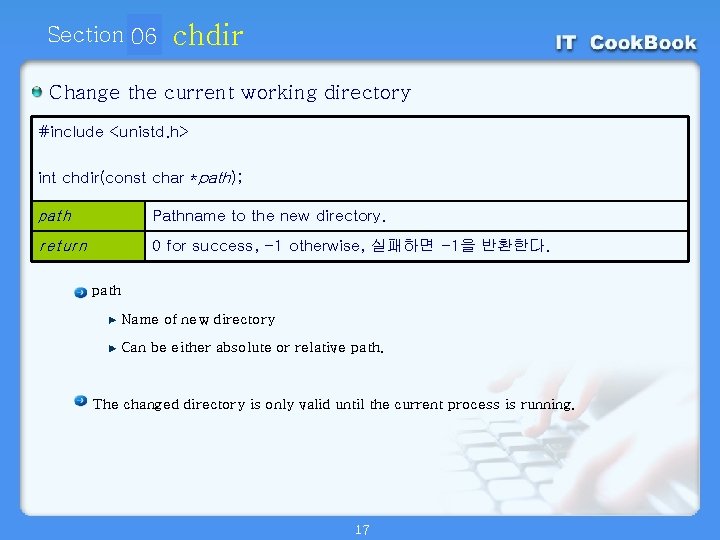
Section 06 01 chdir Change the current working directory #include <unistd. h> int chdir(const char *path); path Pathname to the new directory. return 0 for success, -1 otherwise, 실패하면 -1을 반환한다. path Name of new directory Can be either absolute or relative path. The changed directory is only valid until the current process is running. 17
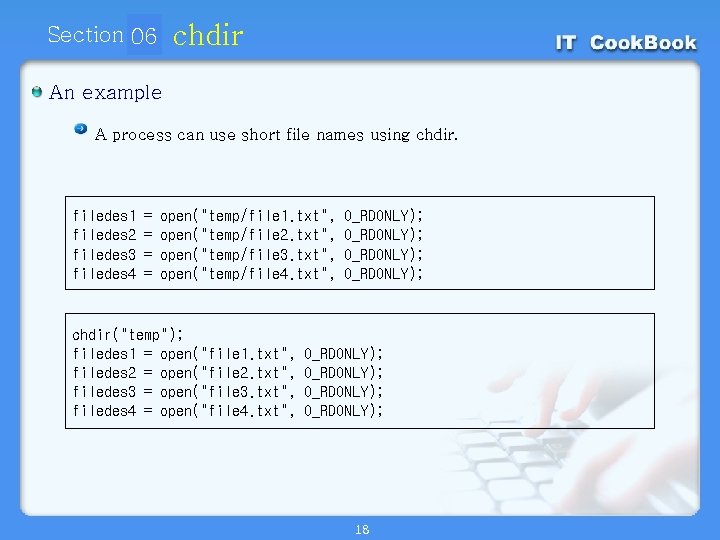
chdir Section 06 01 An example A process can use short file names using chdir. filedes 1 filedes 2 filedes 3 filedes 4 = = open("temp/file 1. txt", open("temp/file 2. txt", open("temp/file 3. txt", open("temp/file 4. txt", chdir("temp"); filedes 1 = open("file 1. txt", filedes 2 = open("file 2. txt", filedes 3 = open("file 3. txt", filedes 4 = open("file 4. txt", O_RDONLY); O_RDONLY); 18
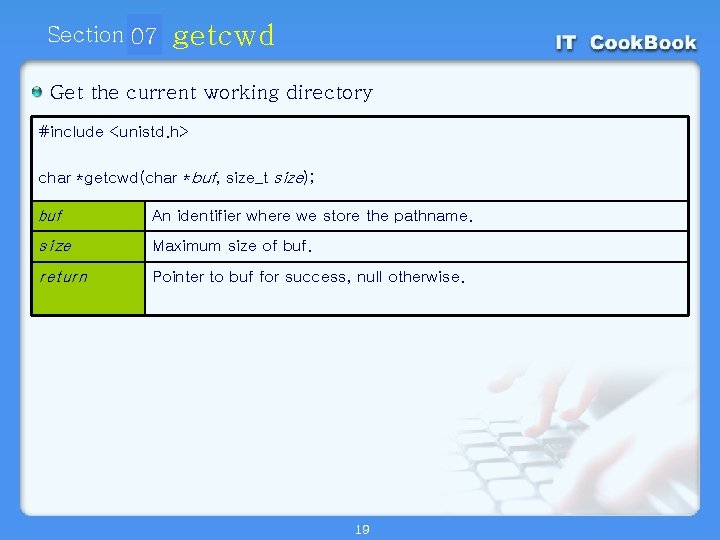
Section 07 01 getcwd Get the current working directory #include <unistd. h> char *getcwd(char *buf, size_t size); buf An identifier where we store the pathname. size Maximum size of buf. return Pointer to buf for success, null otherwise. 19
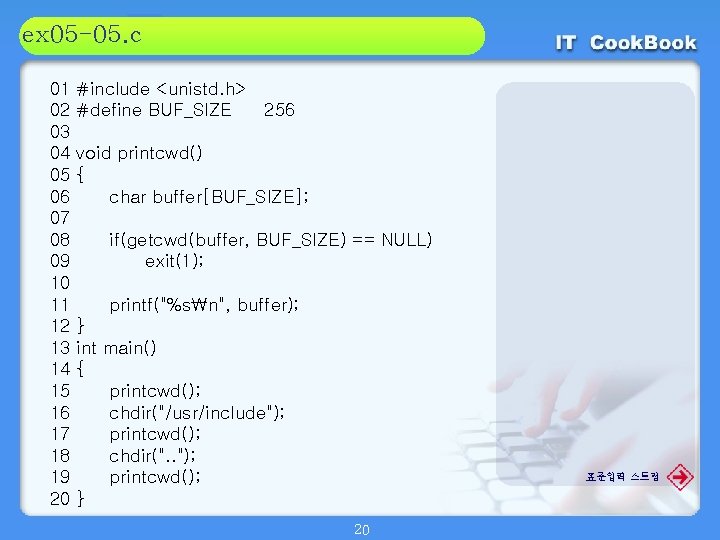
Section 02 01 ex 05 -05. c 01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 #include <unistd. h> #define BUF_SIZE 256 void printcwd() { char buffer[BUF_SIZE]; if(getcwd(buffer, BUF_SIZE) == NULL) exit(1); printf("%sn", buffer); } int main() { printcwd(); chdir("/usr/include"); printcwd(); chdir(". . "); printcwd(); } 20 표준입력 스트림