4 include stdio h int mainvoid int num
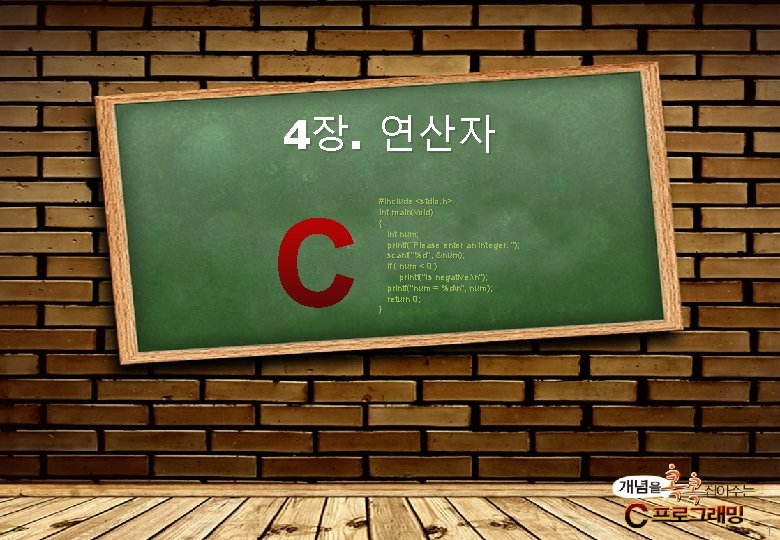
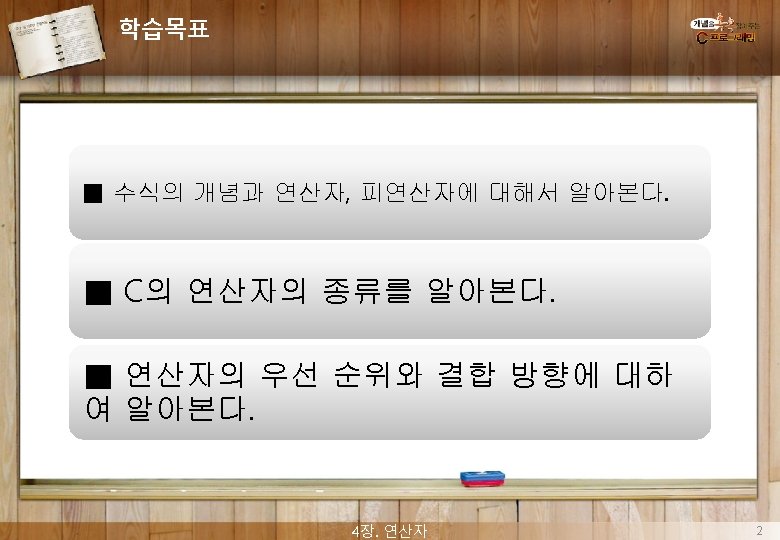
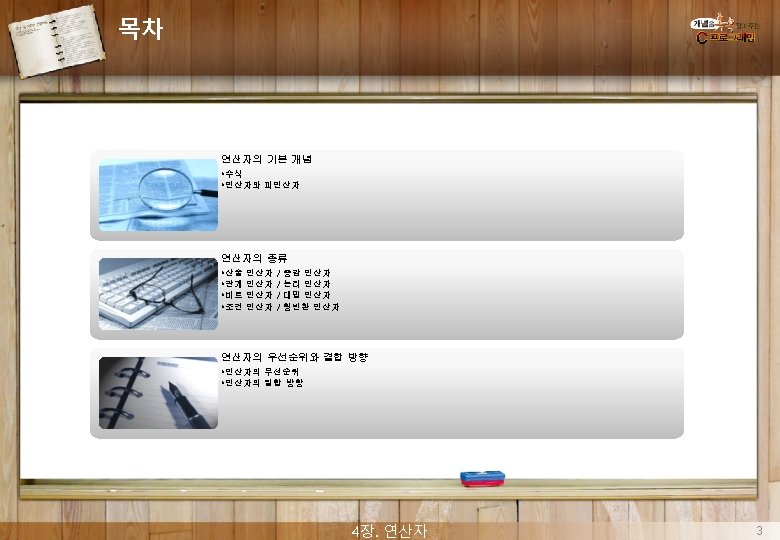
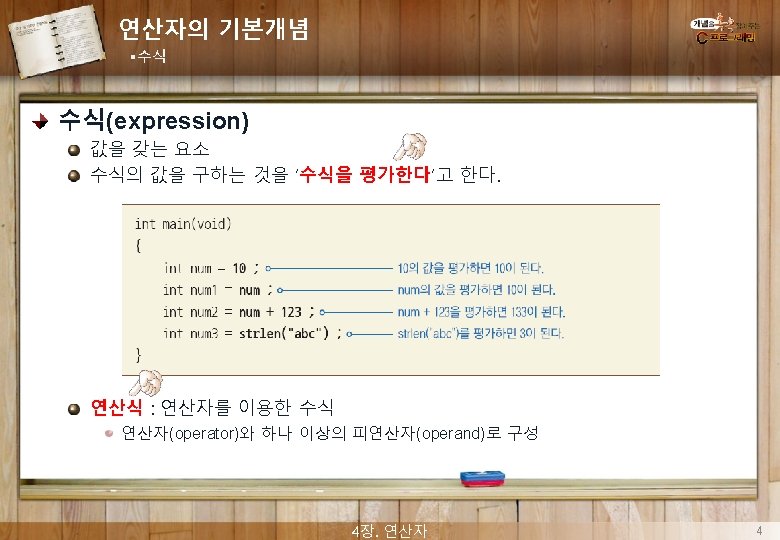
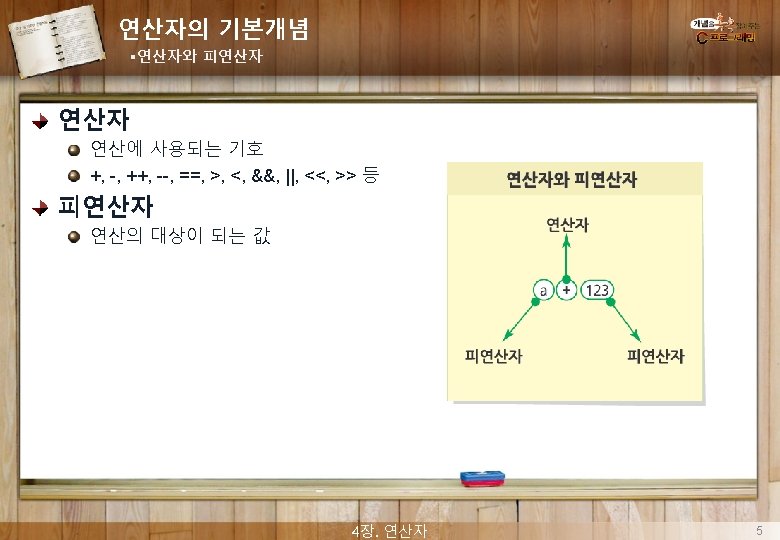
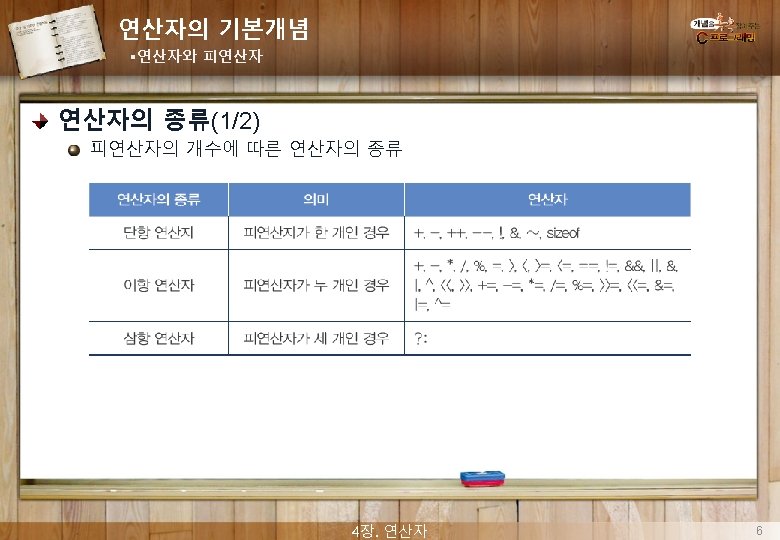
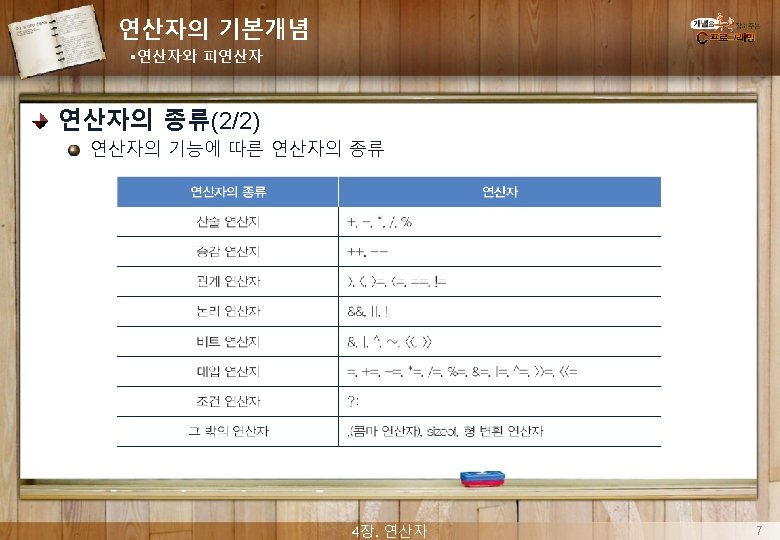
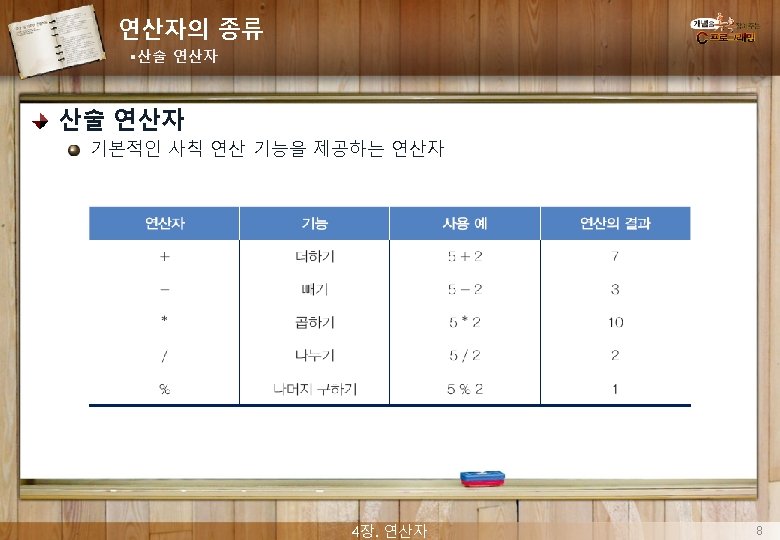
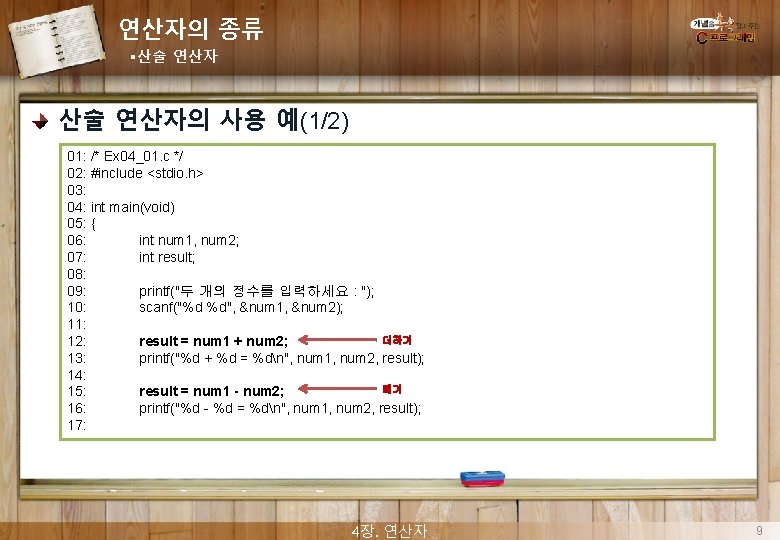
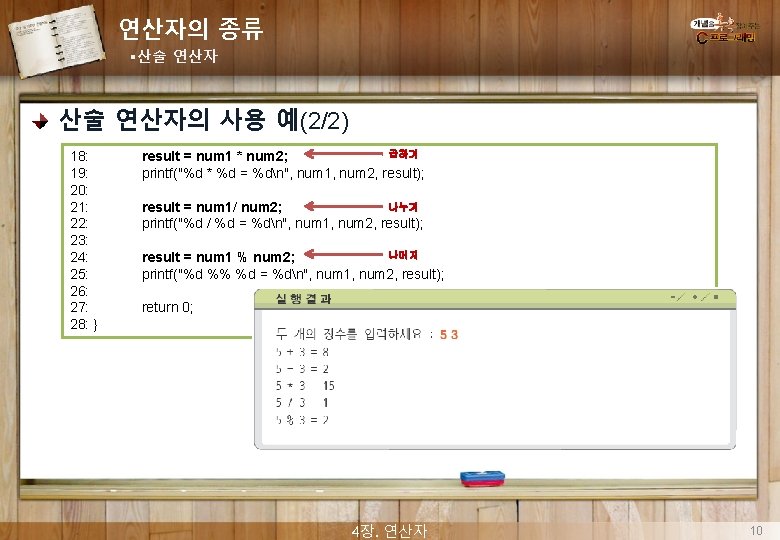
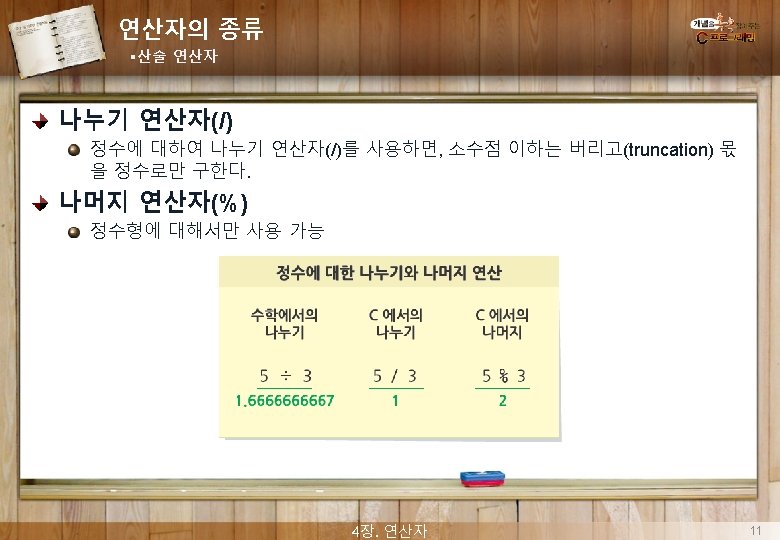
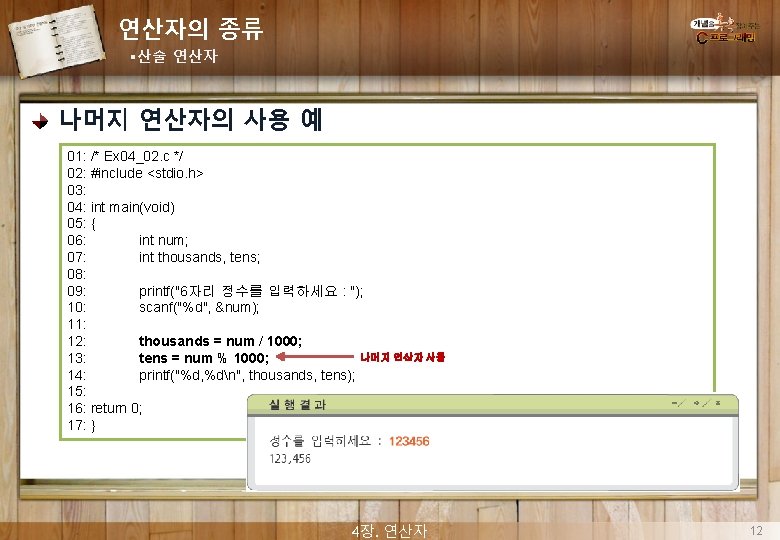
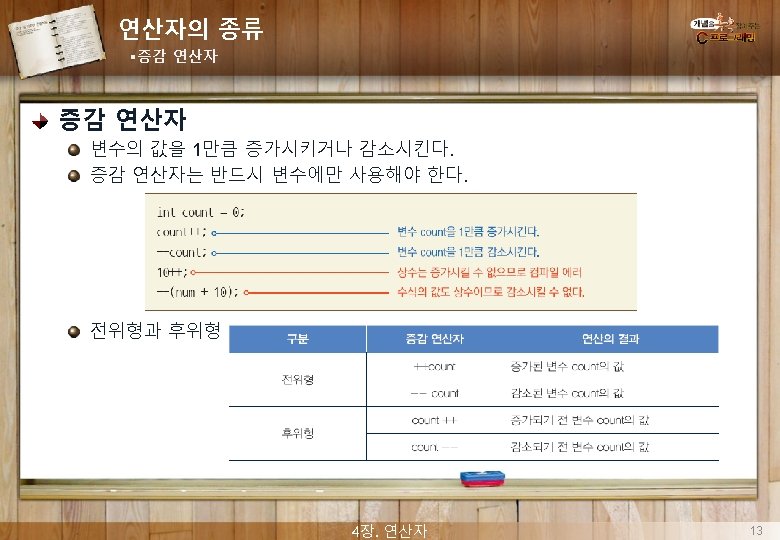
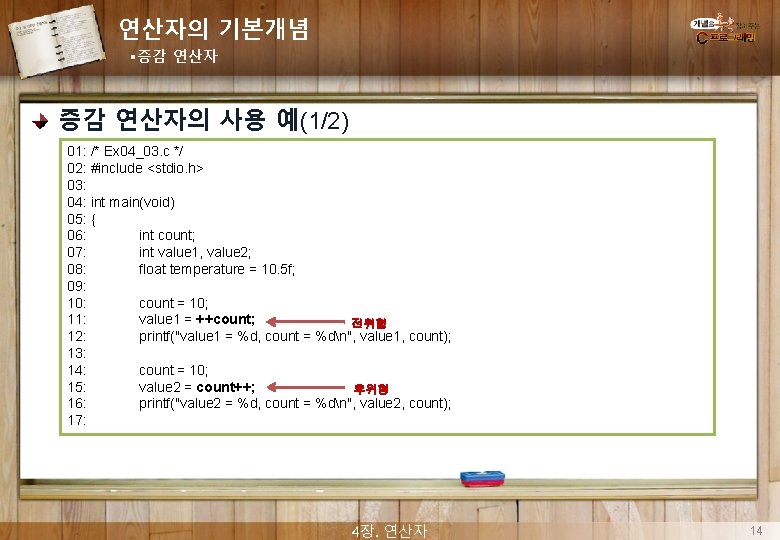
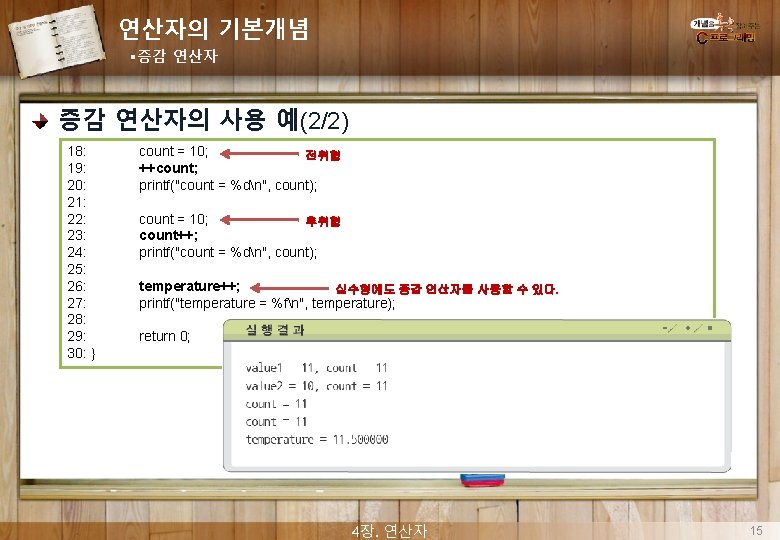
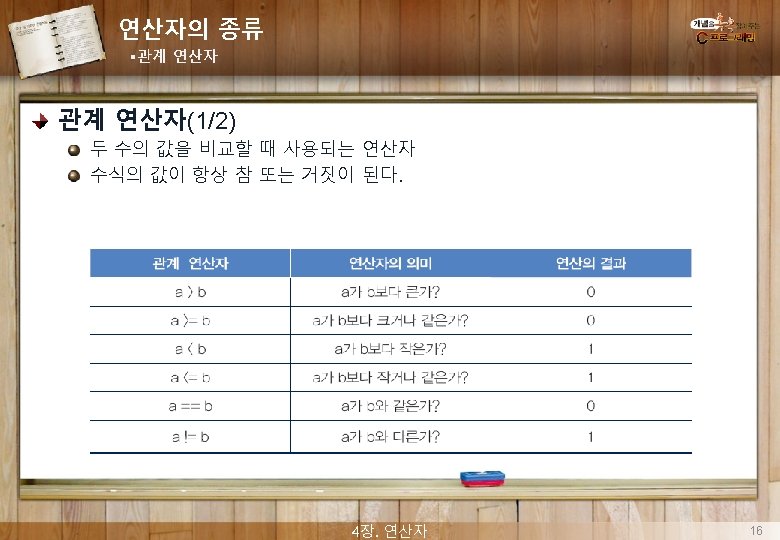
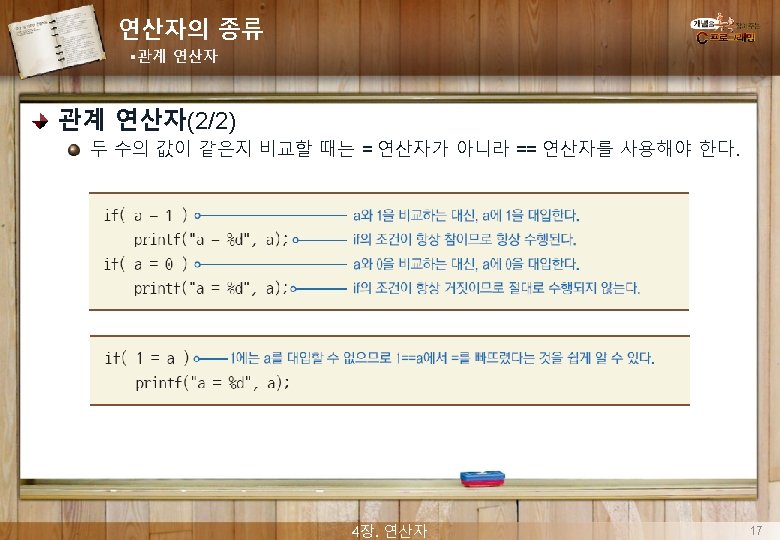
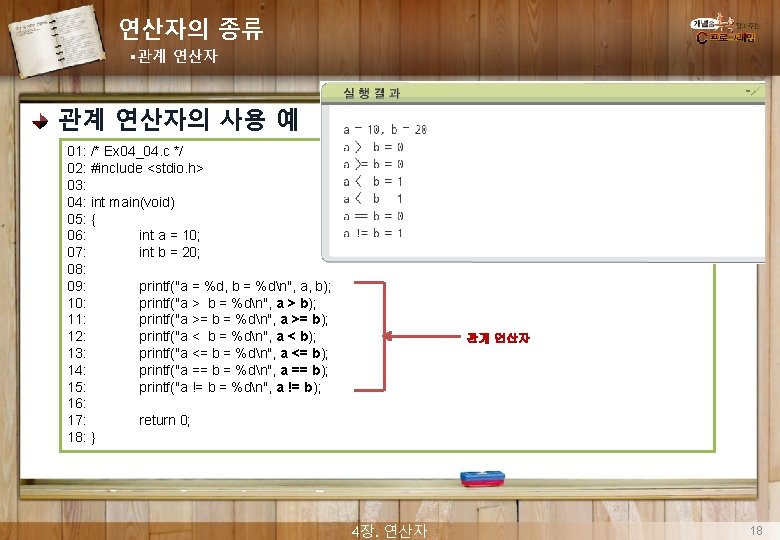
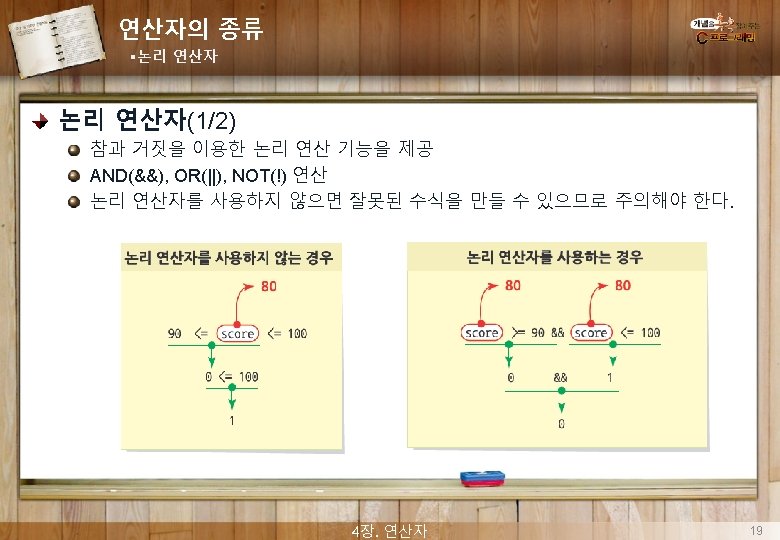
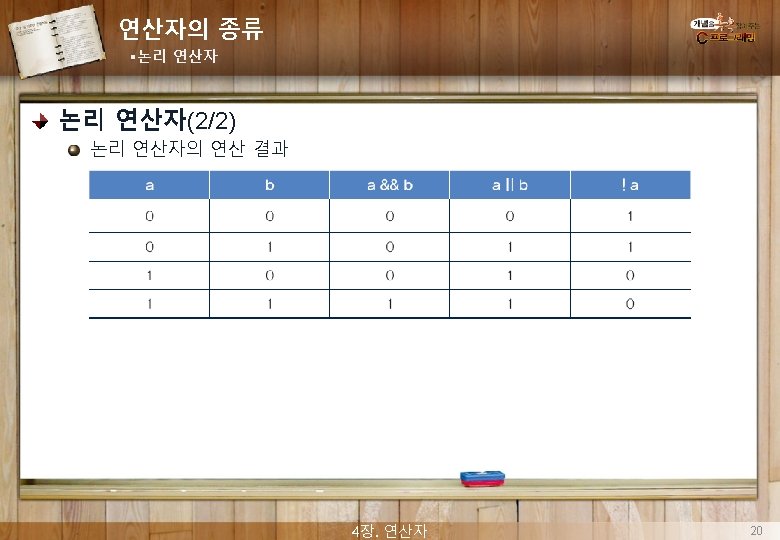
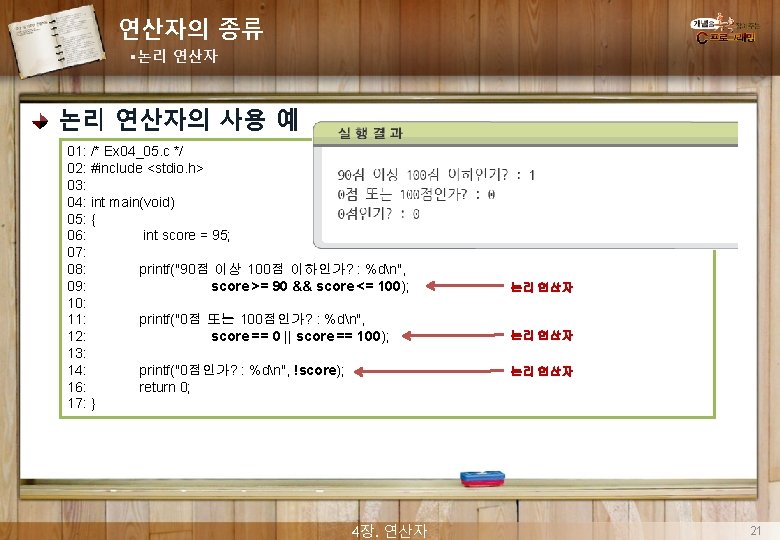
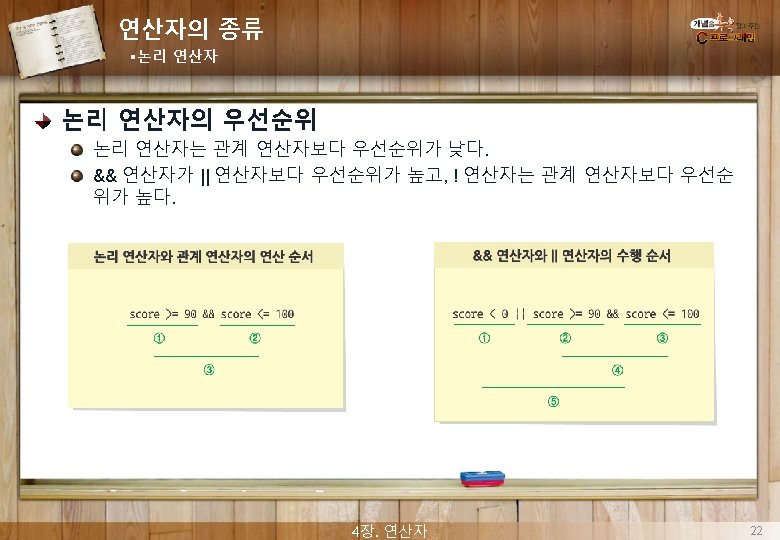
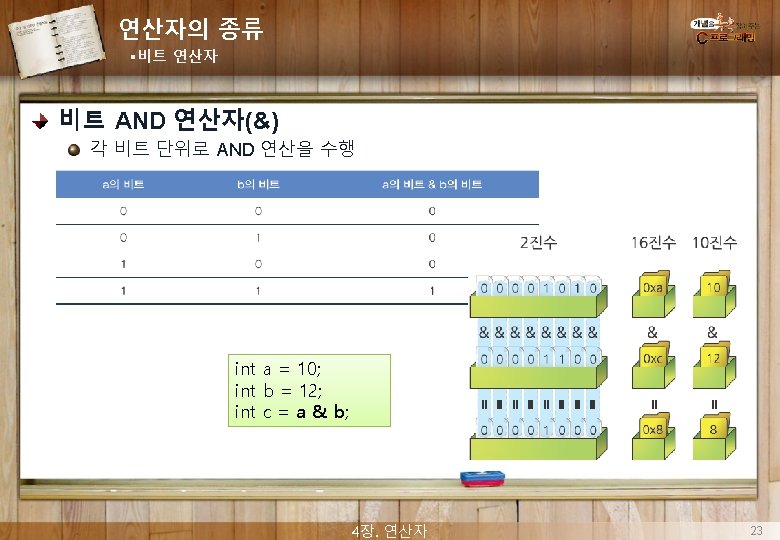
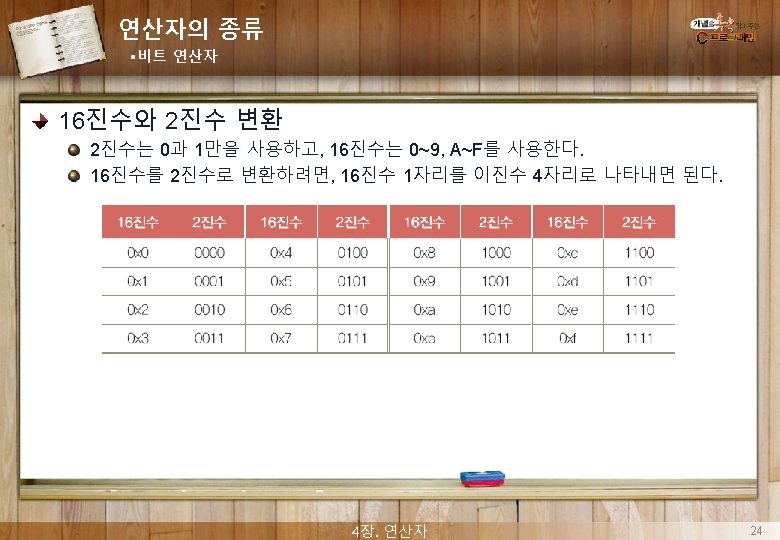
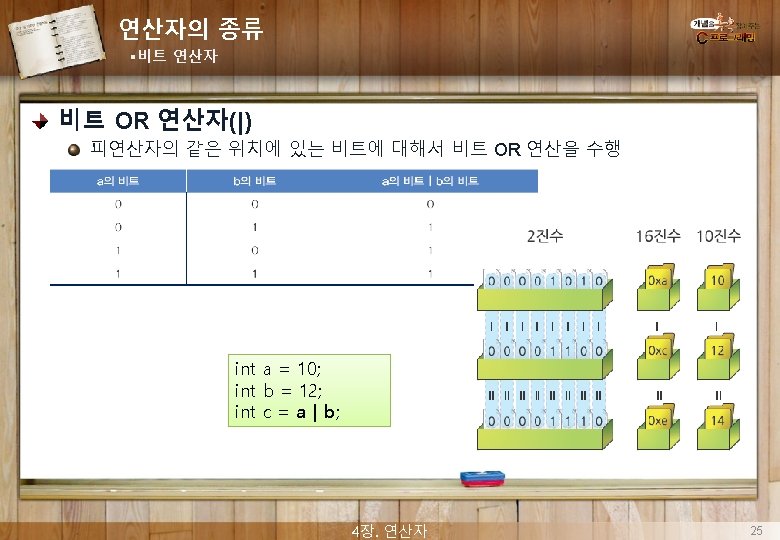
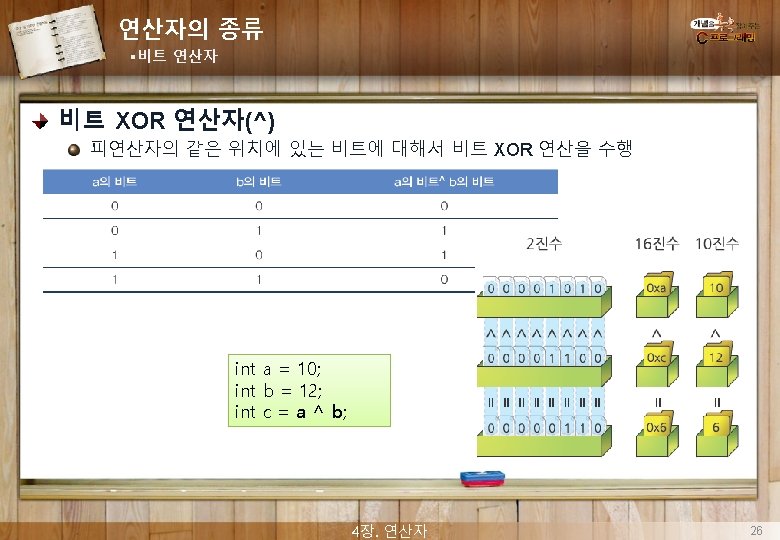
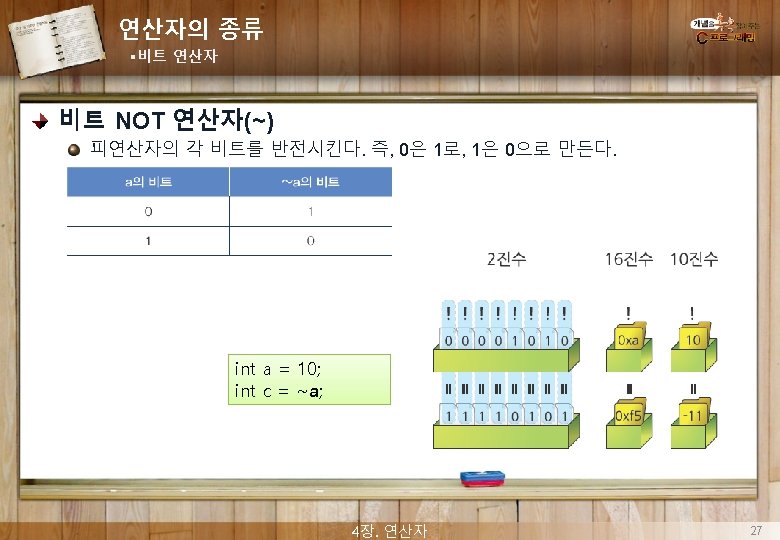
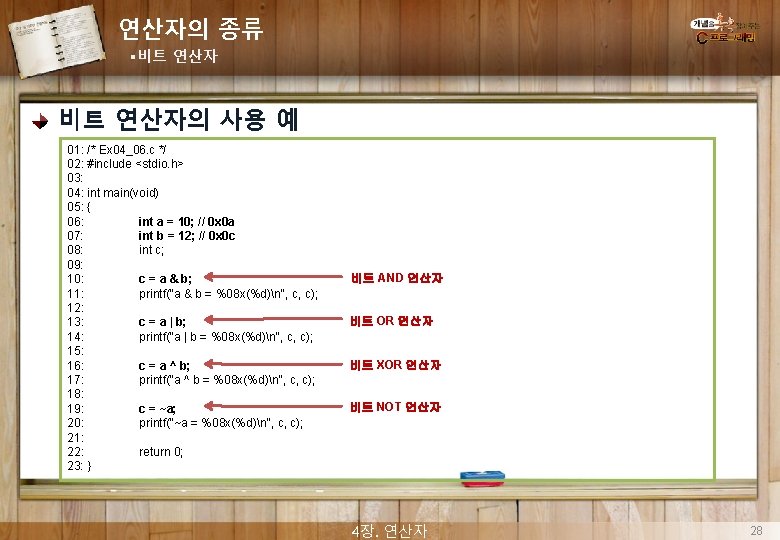
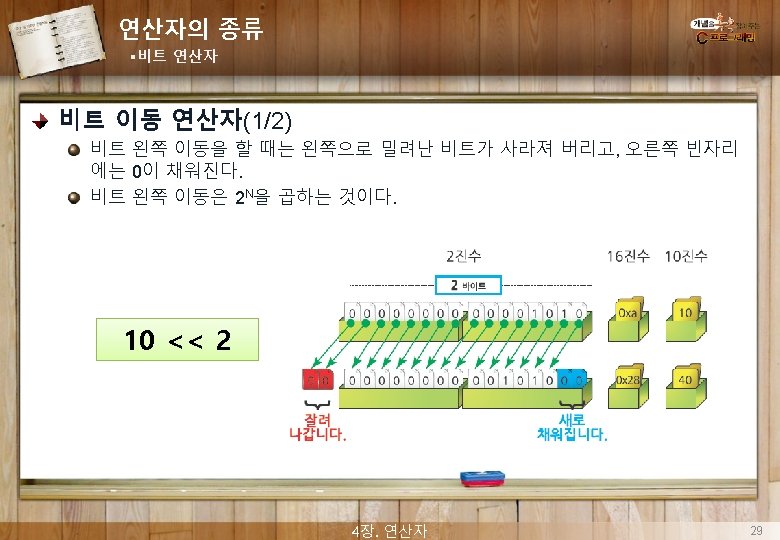
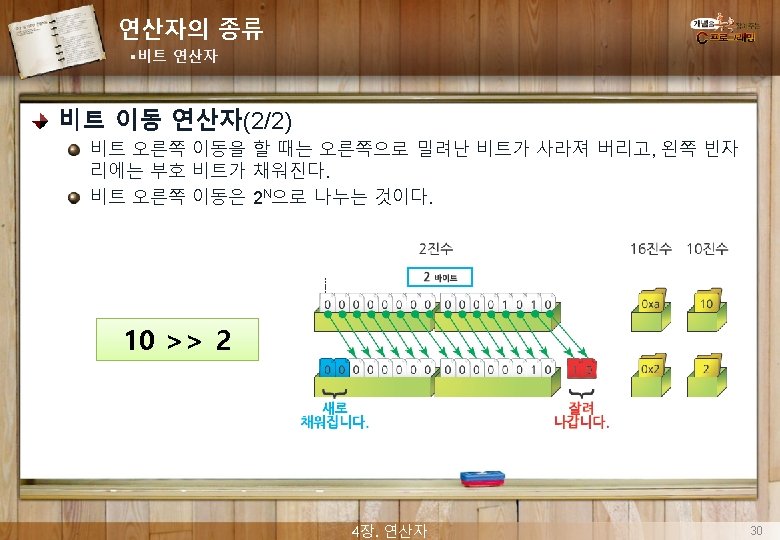
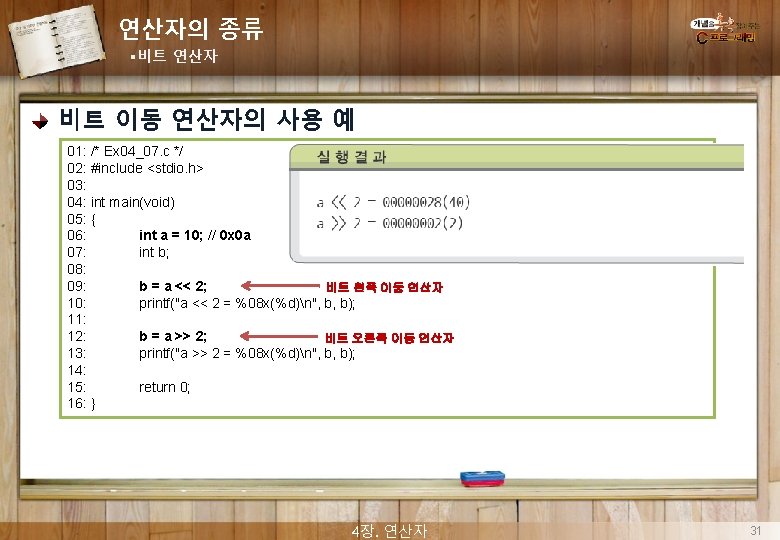
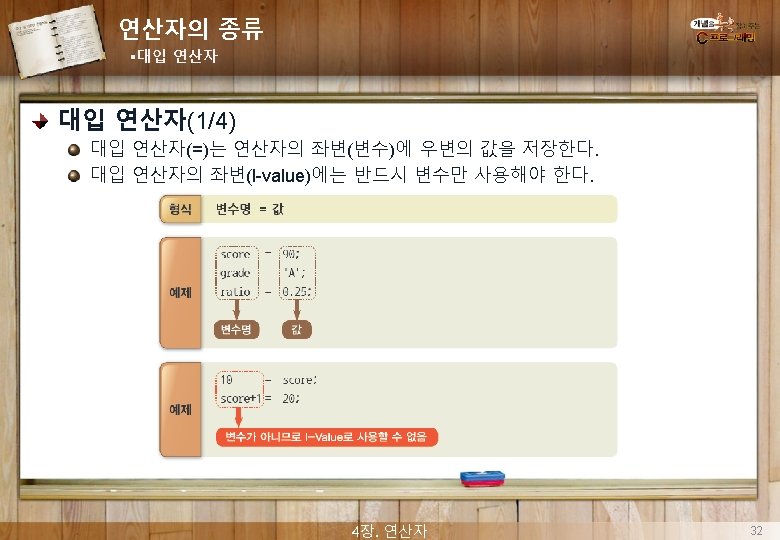
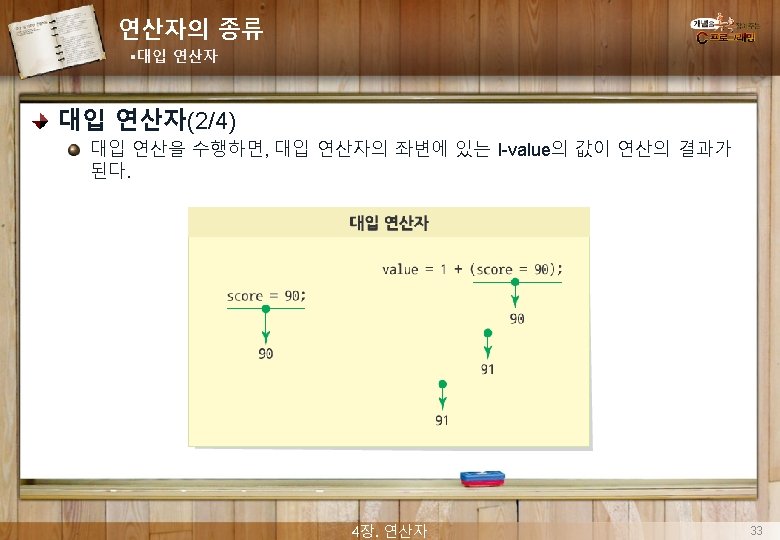
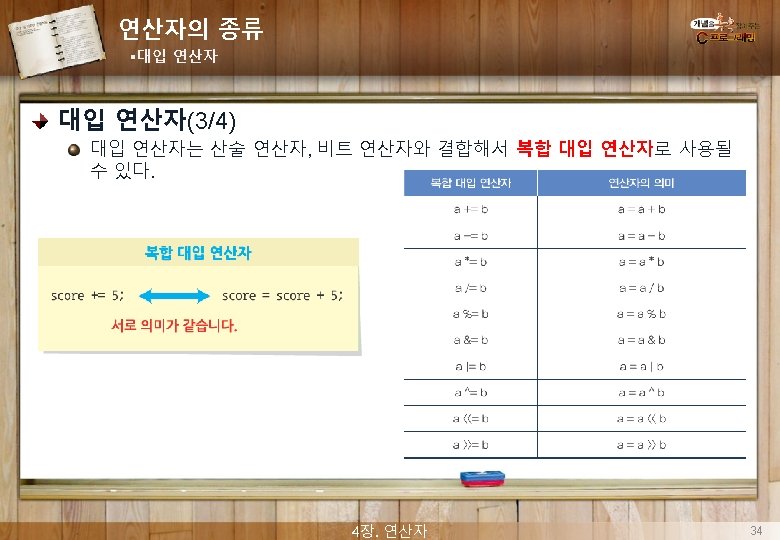
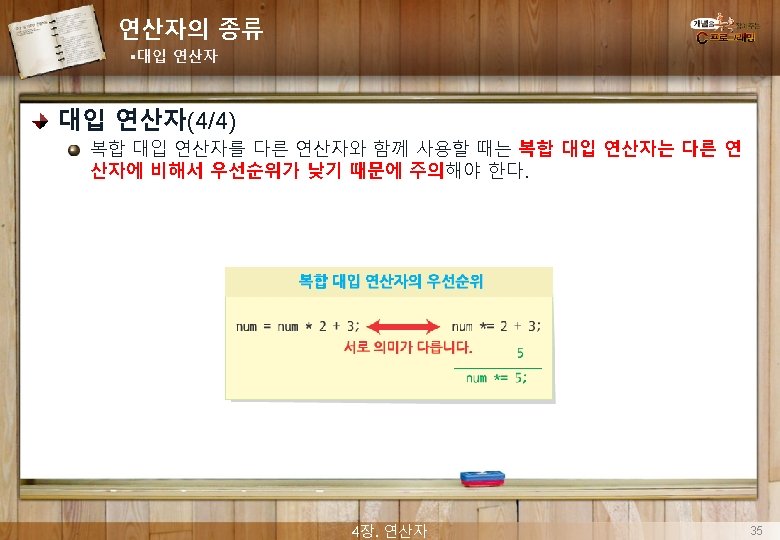
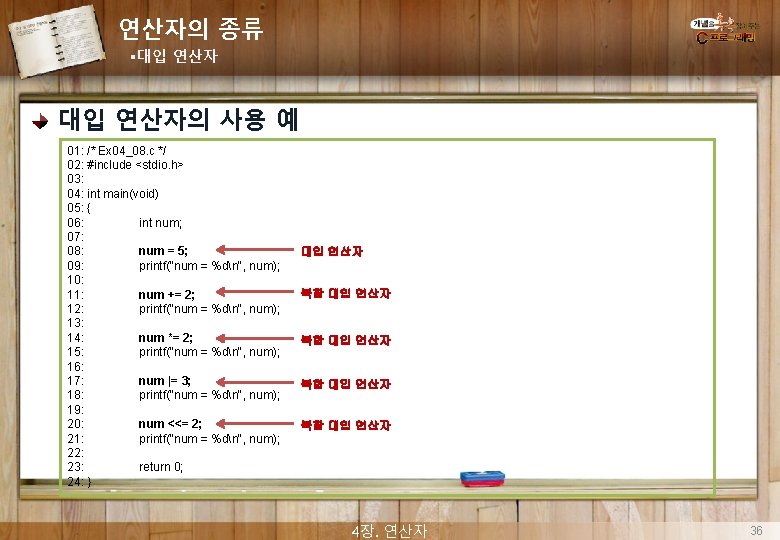
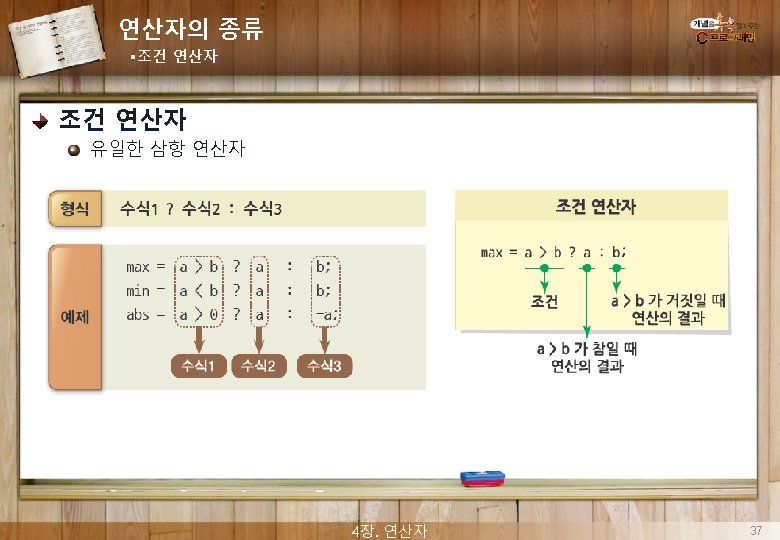
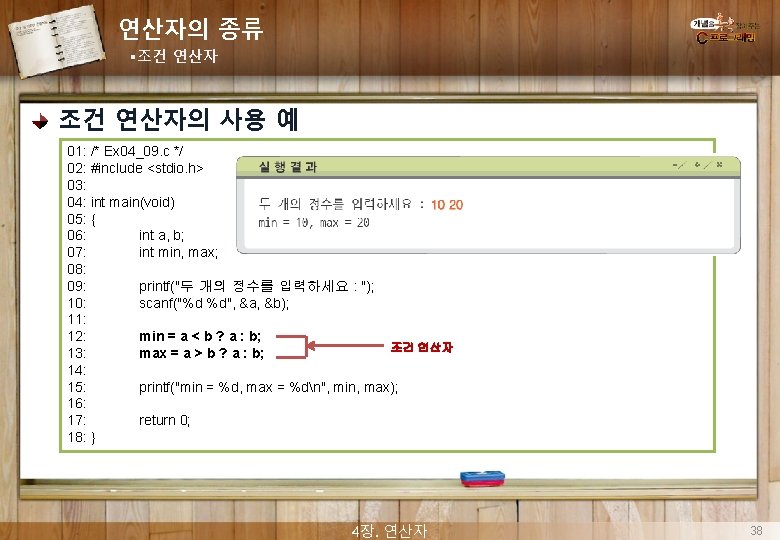
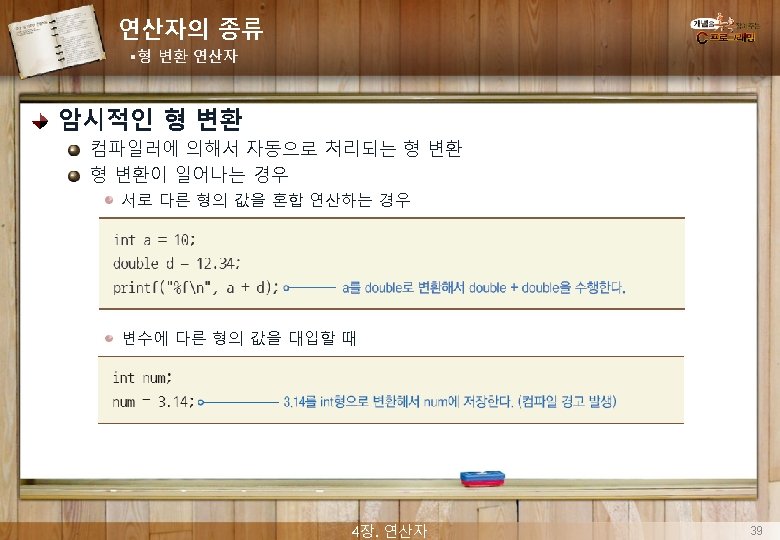
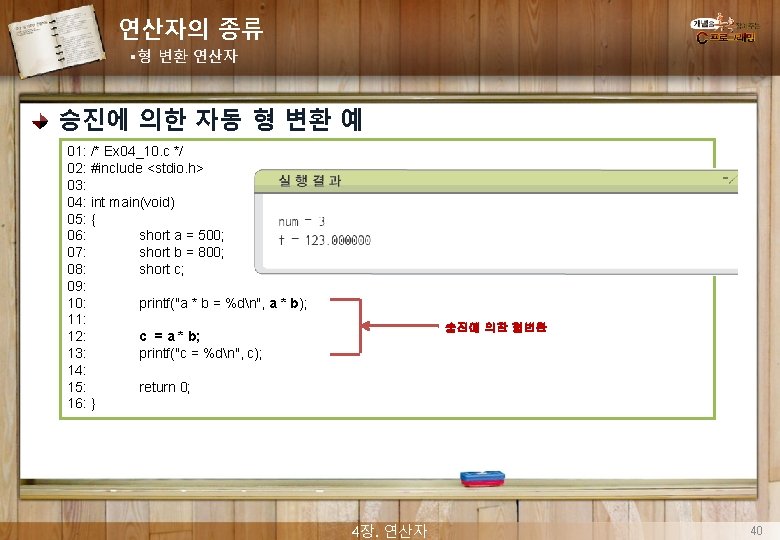
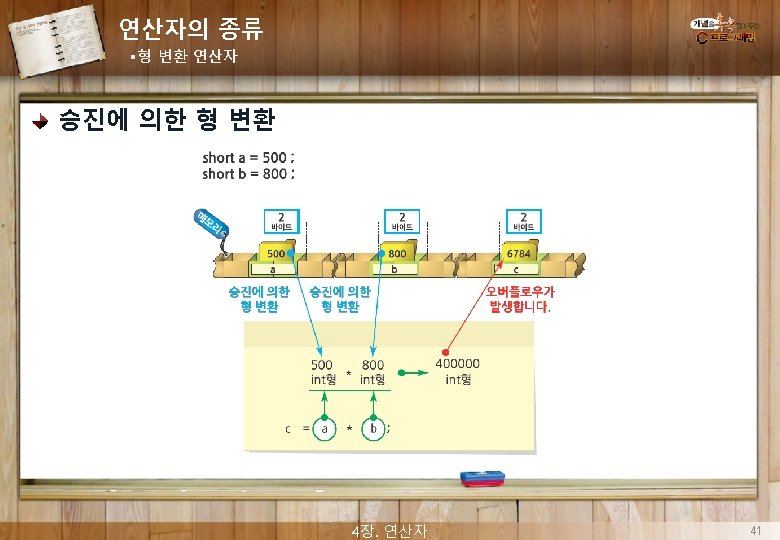
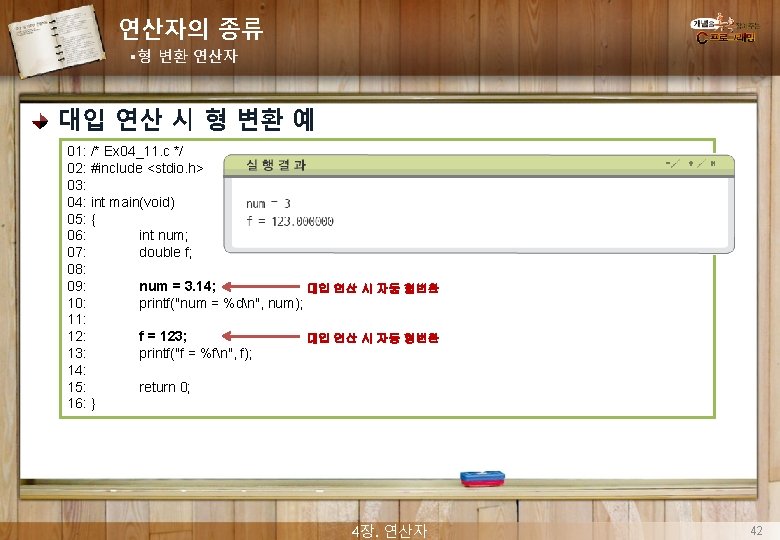
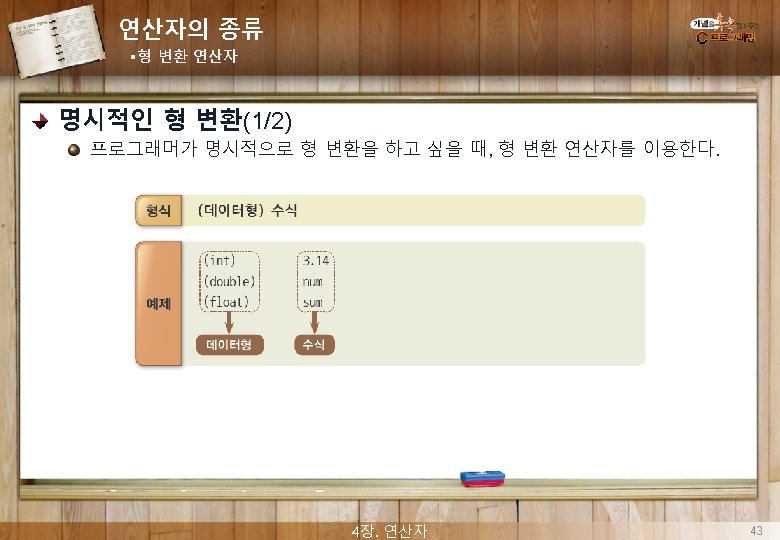
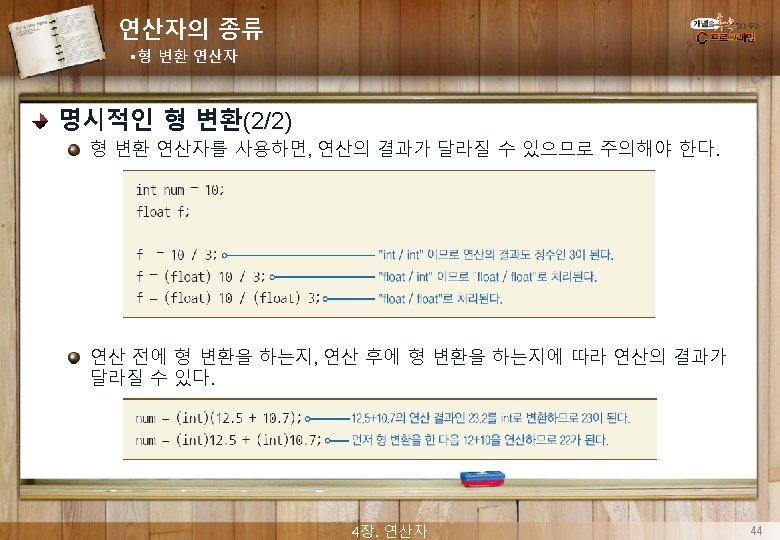
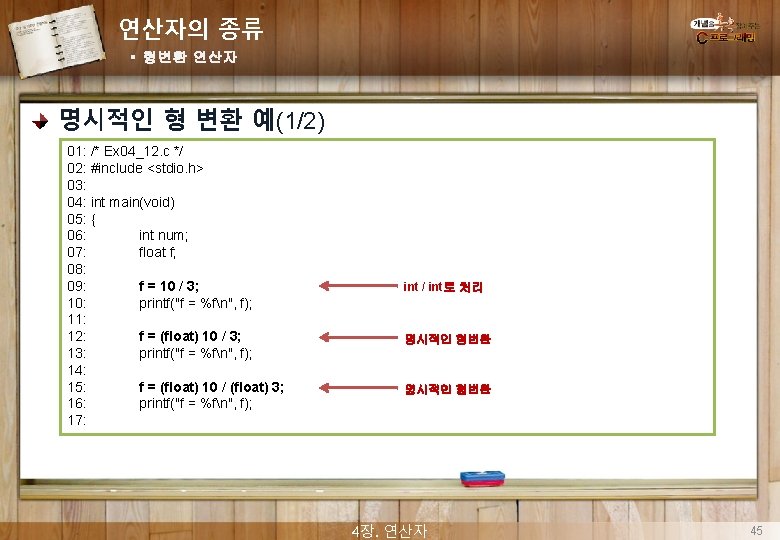
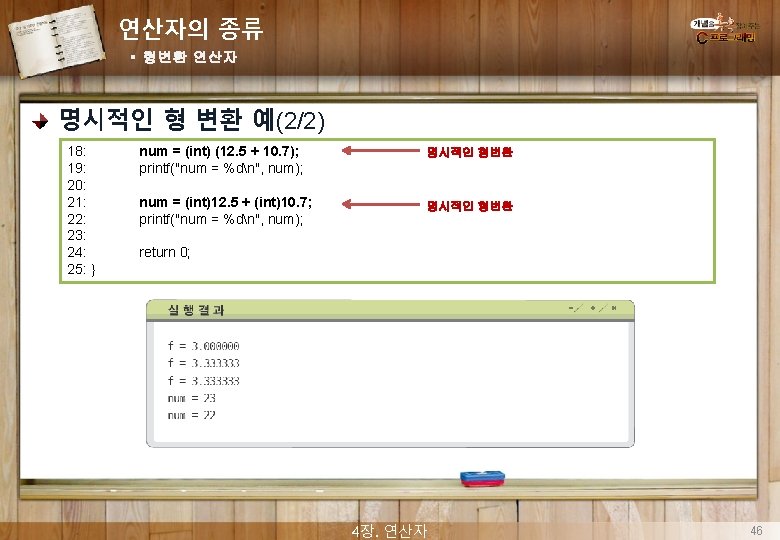
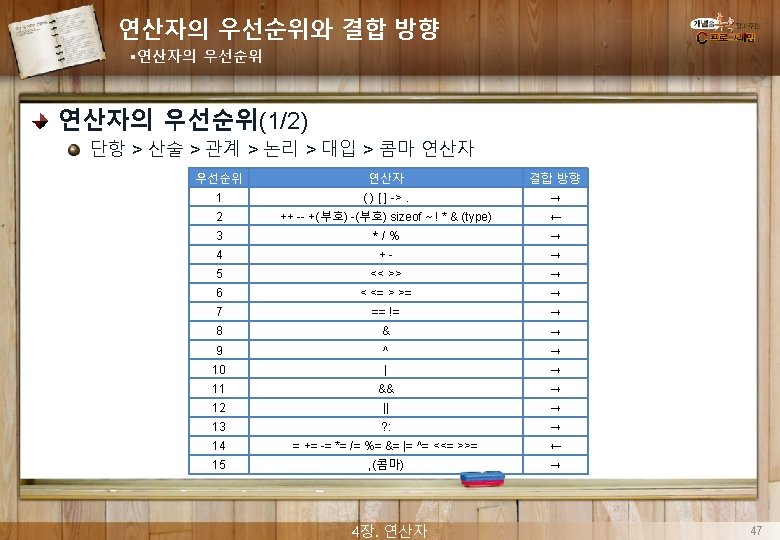
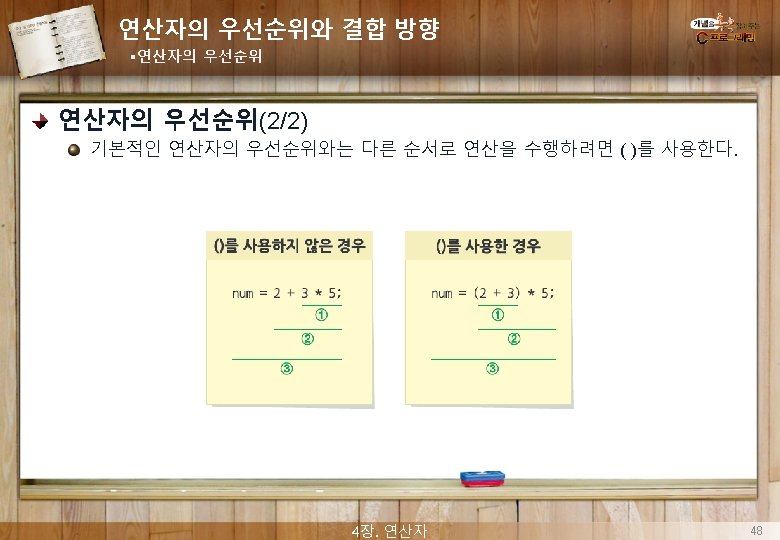
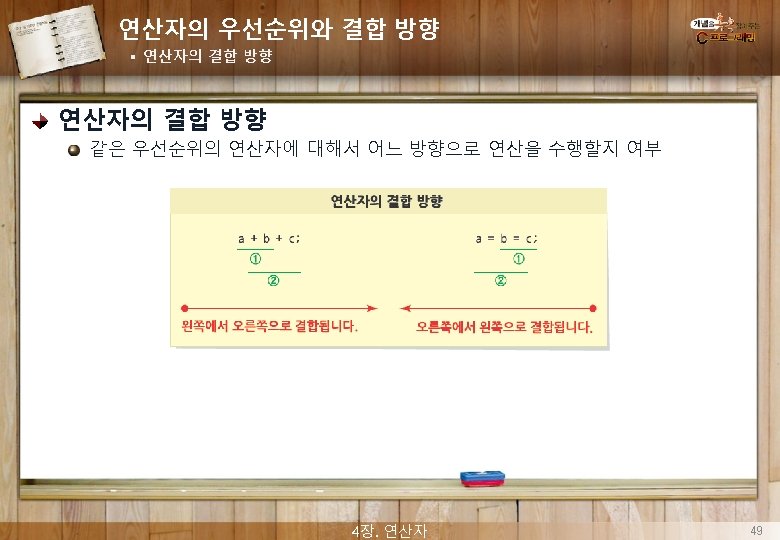
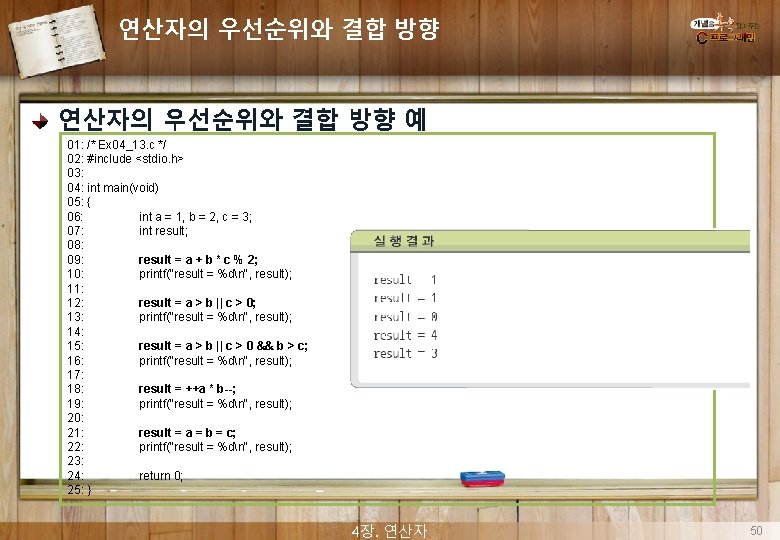
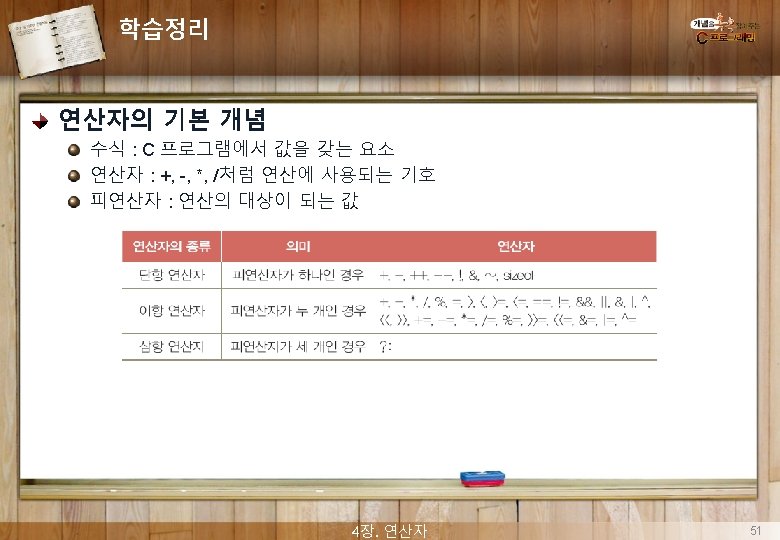
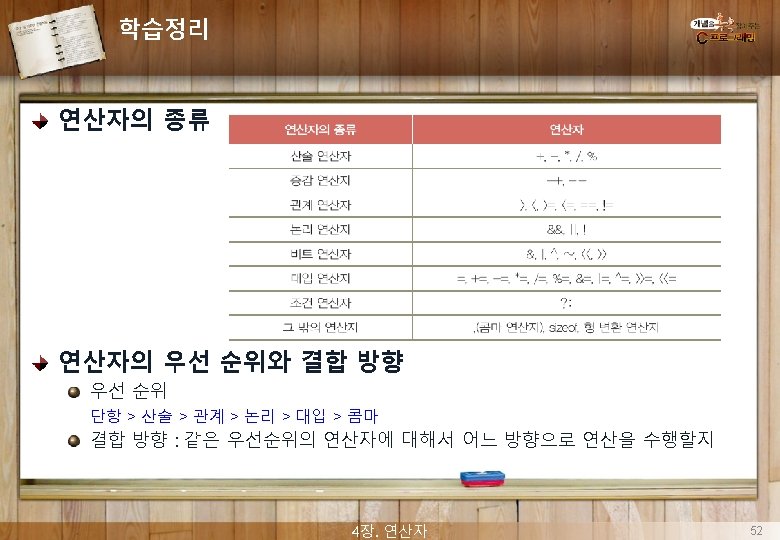
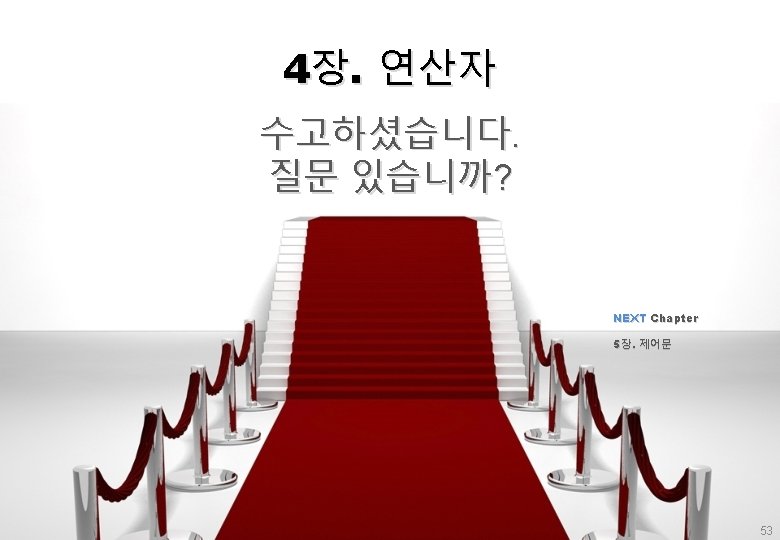
- Slides: 53
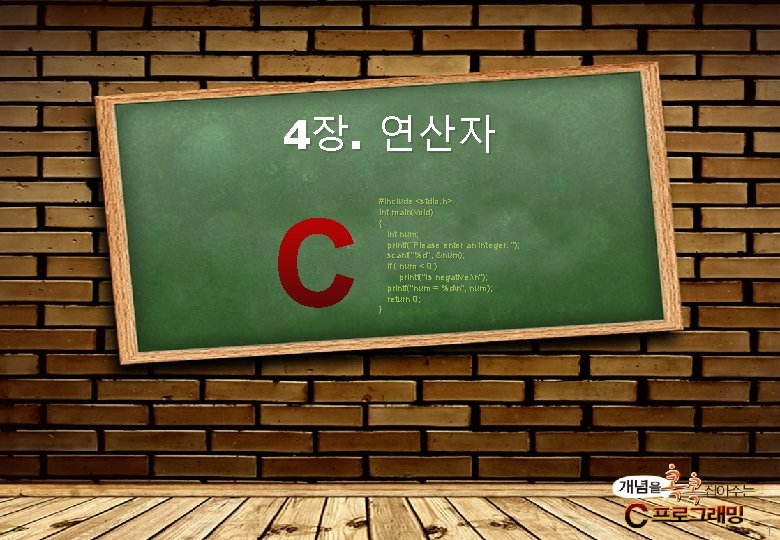
4장. 연산자 #include <stdio. h> int main(void) { int num; printf(“Please enter an integer: "); scanf("%d", &num); if ( num < 0 ) printf("Is negative. n"); printf("num = %dn", num); return 0; } 1
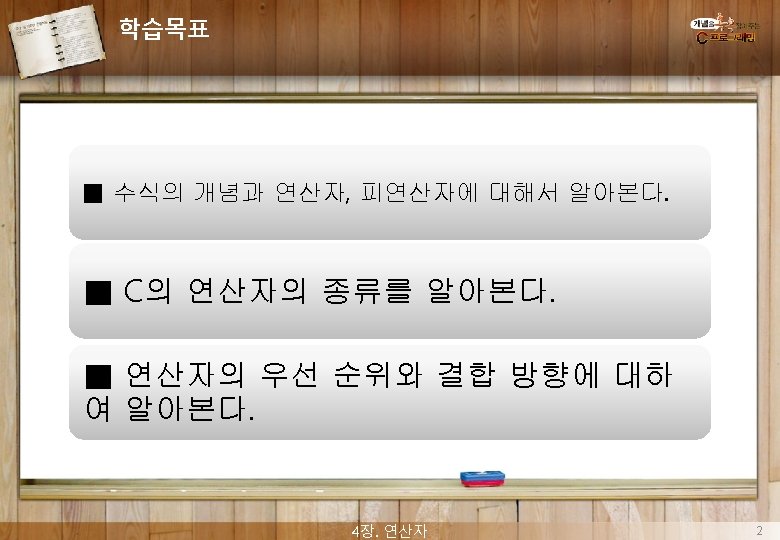
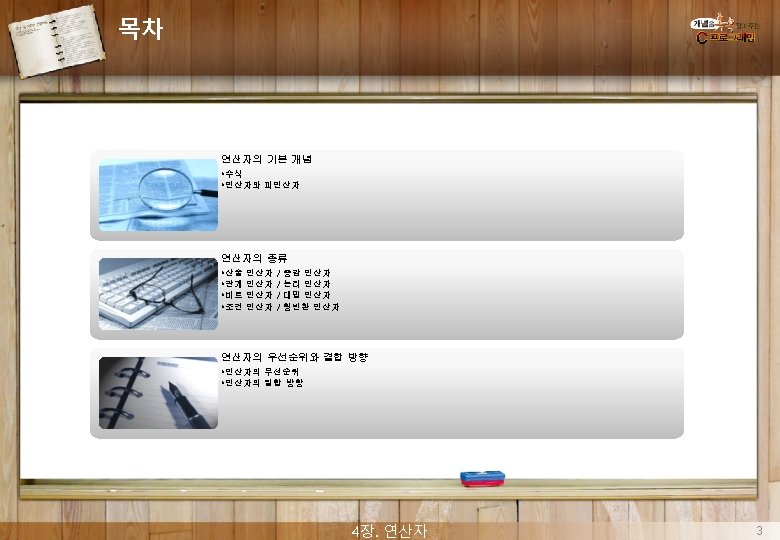
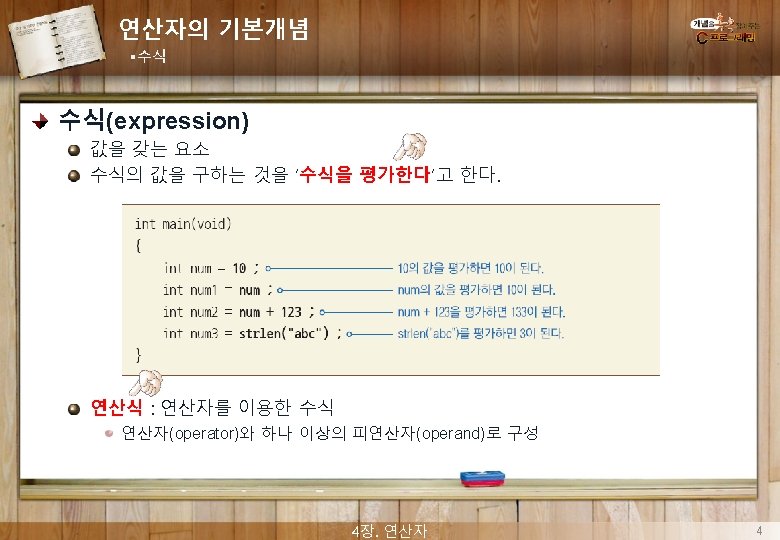
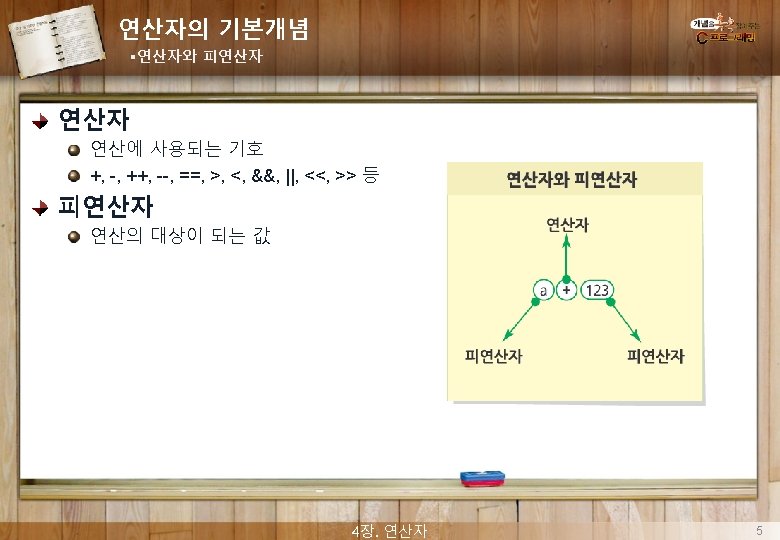
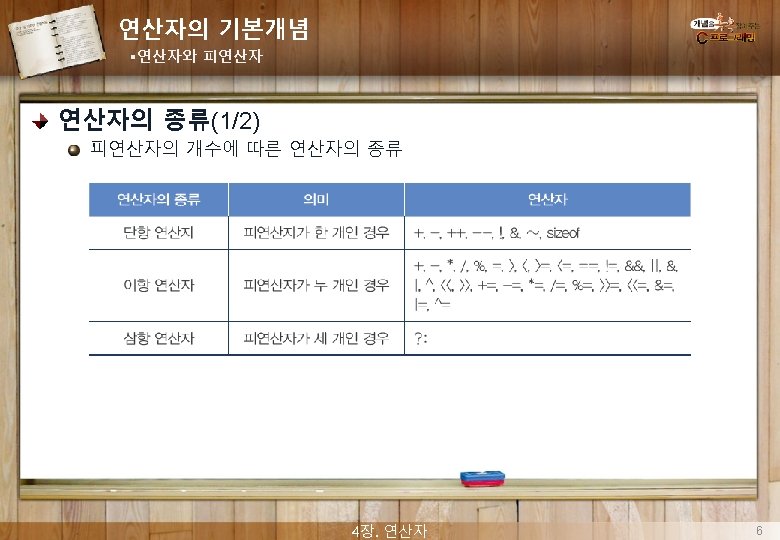
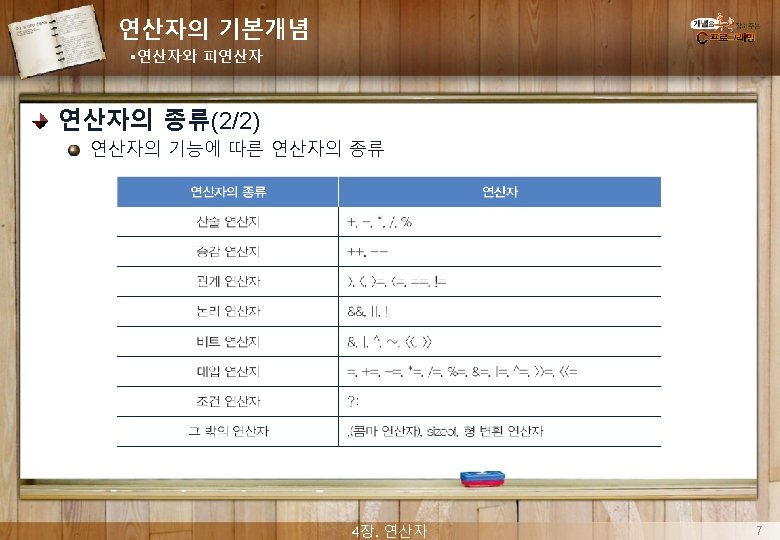
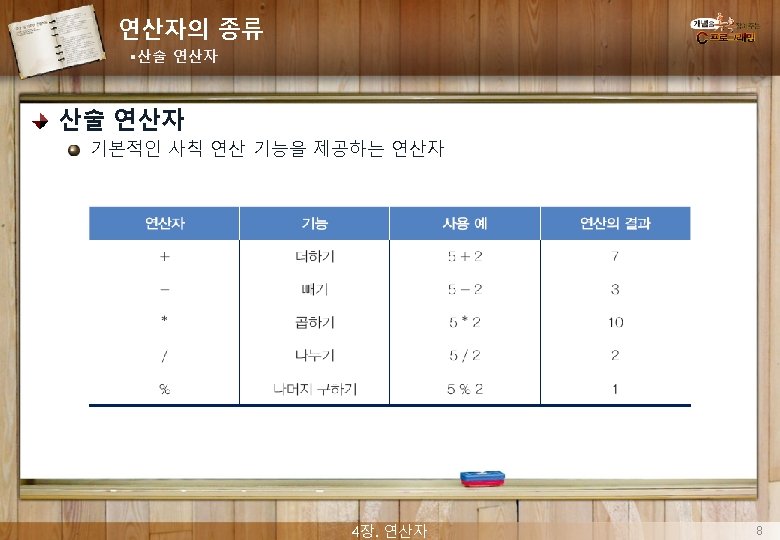
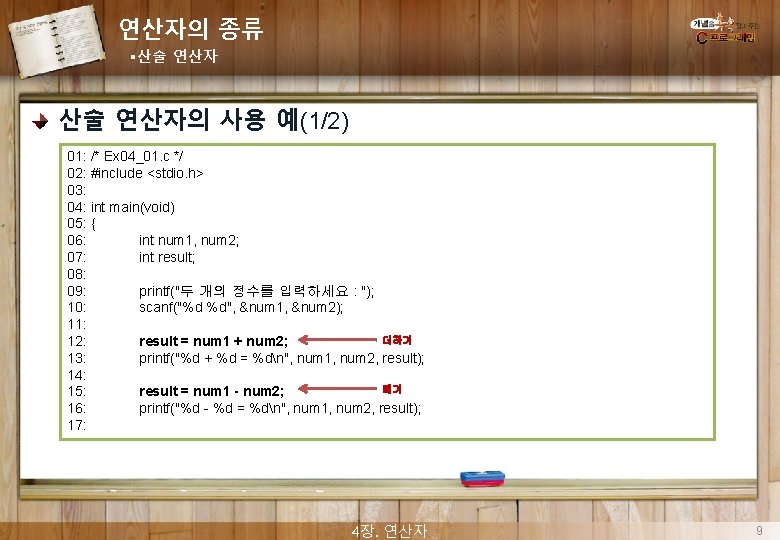
연산자의 종류 §산술 연산자의 사용 예(1/2) 01: /* Ex 04_01. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int num 1, num 2; 07: int result; 08: 09: printf("두 개의 정수를 입력하세요 : "); 10: scanf("%d %d", &num 1, &num 2); 11: 더하기 12: result = num 1 + num 2; 13: printf("%d + %d = %dn", num 1, num 2, result); 14: 빼기 15: result = num 1 - num 2; 16: printf("%d - %d = %dn", num 1, num 2, result); 17: 4장. 연산자 9
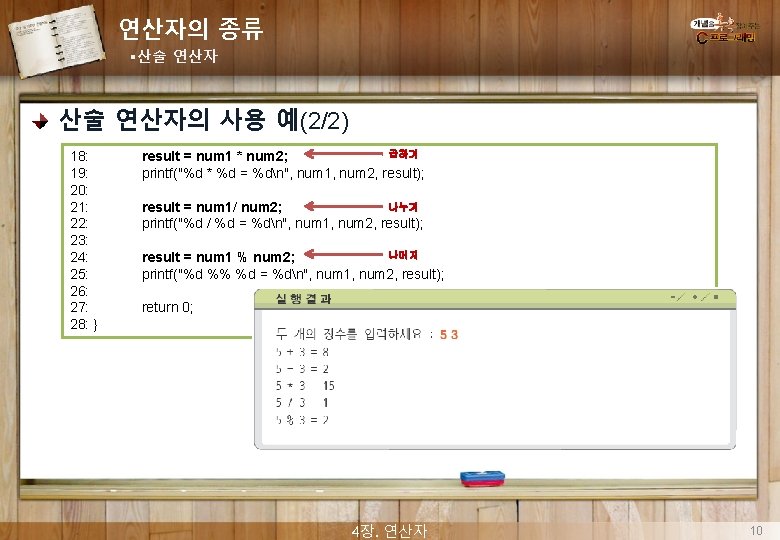
연산자의 종류 §산술 연산자의 사용 예(2/2) 18: 19: 20: 21: 22: 23: 24: 25: 26: 27: 28: } 곱하기 result = num 1 * num 2; printf("%d * %d = %dn", num 1, num 2, result); 나누기 result = num 1/ num 2; printf("%d / %d = %dn", num 1, num 2, result); 나머지 result = num 1 % num 2; printf("%d %% %d = %dn", num 1, num 2, result); return 0; 4장. 연산자 10
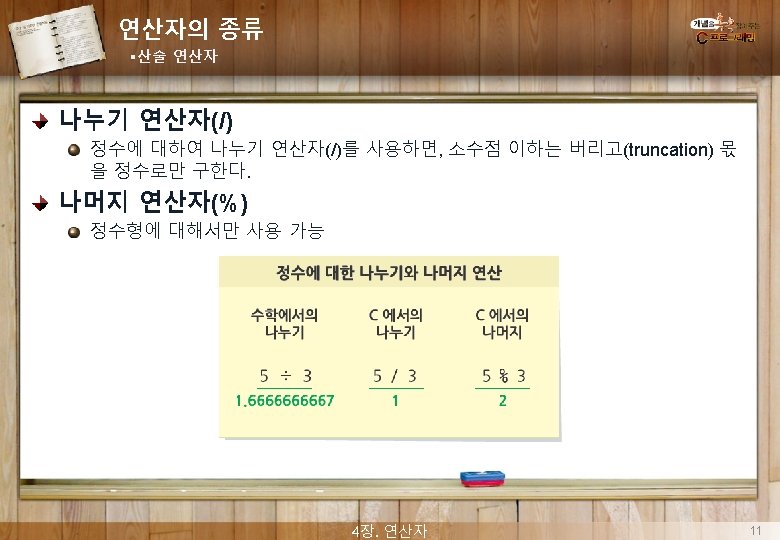
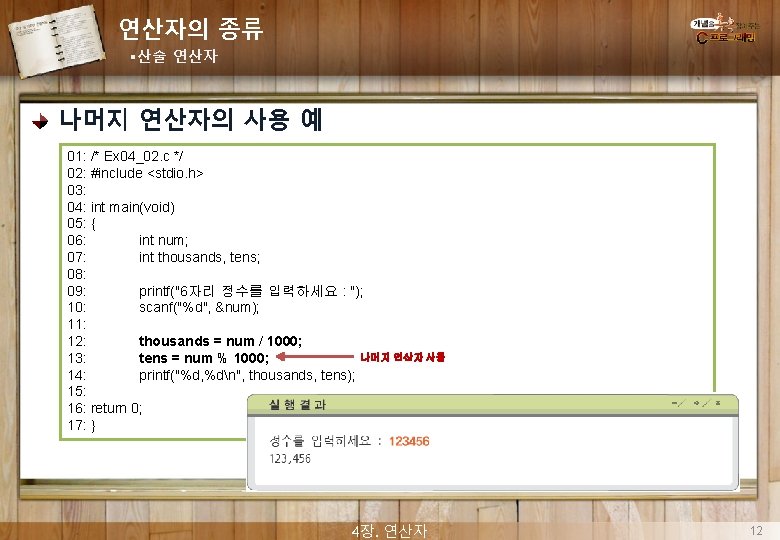
연산자의 종류 §산술 연산자 나머지 연산자의 사용 예 01: /* Ex 04_02. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int num; 07: int thousands, tens; 08: 09: printf("6자리 정수를 입력하세요 : "); 10: scanf("%d", &num); 11: 12: thousands = num / 1000; 나머지 연산자 사용 13: tens = num % 1000; 14: printf("%d, %dn", thousands, tens); 15: 16: return 0; 17: } 4장. 연산자 12
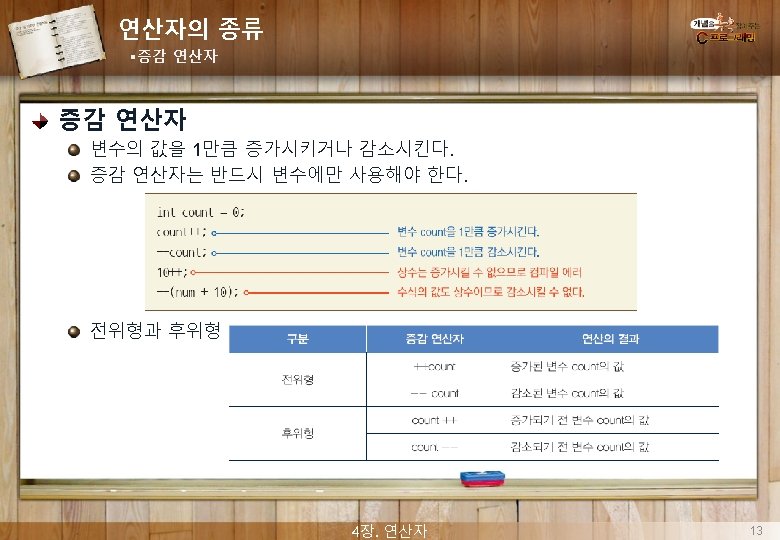
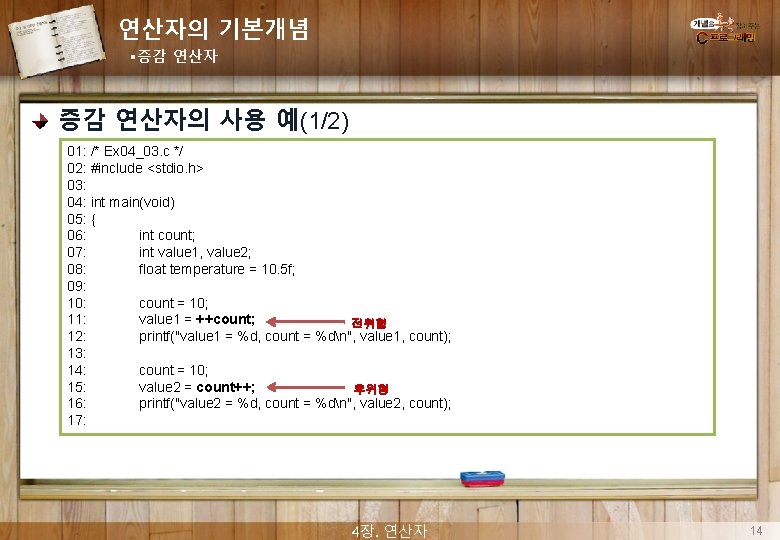
연산자의 기본개념 §증감 연산자의 사용 예(1/2) 01: /* Ex 04_03. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int count; 07: int value 1, value 2; 08: float temperature = 10. 5 f; 09: 10: count = 10; 11: value 1 = ++count; 전위형 12: printf("value 1 = %d, count = %dn", value 1, count); 13: 14: count = 10; 15: value 2 = count++; 후위형 16: printf("value 2 = %d, count = %dn", value 2, count); 17: 4장. 연산자 14
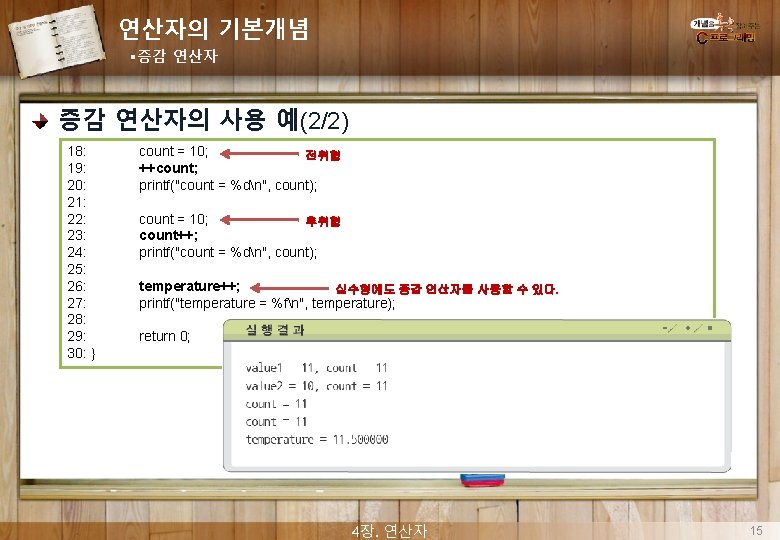
연산자의 기본개념 §증감 연산자의 사용 예(2/2) 18: 19: 20: 21: 22: 23: 24: 25: 26: 27: 28: 29: 30: } count = 10; 전위형 ++count; printf("count = %dn", count); count = 10; 후위형 count++; printf("count = %dn", count); temperature++; 실수형에도 증감 연산자를 사용할 수 있다. printf("temperature = %fn", temperature); return 0; 4장. 연산자 15
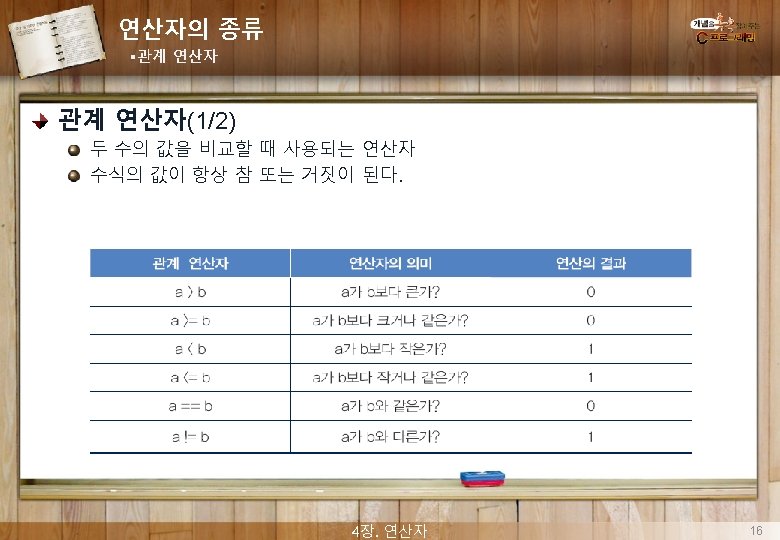
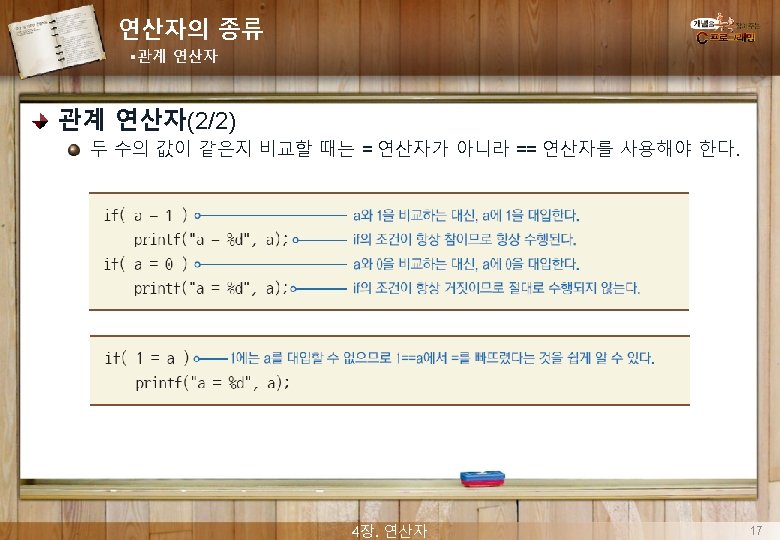
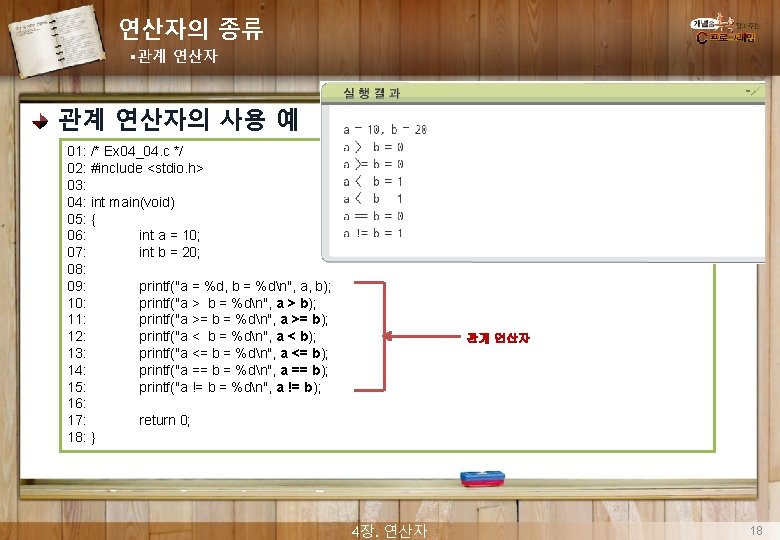
연산자의 종류 §관계 연산자의 사용 예 01: /* Ex 04_04. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int a = 10; 07: int b = 20; 08: 09: printf("a = %d, b = %dn", a, b); 10: printf("a > b = %dn", a > b); 11: printf("a >= b = %dn", a >= b); 12: printf("a < b = %dn", a < b); 13: printf("a <= b = %dn", a <= b); 14: printf("a == b = %dn", a == b); 15: printf("a != b = %dn", a != b); 16: 17: return 0; 18: } 관계 연산자 4장. 연산자 18
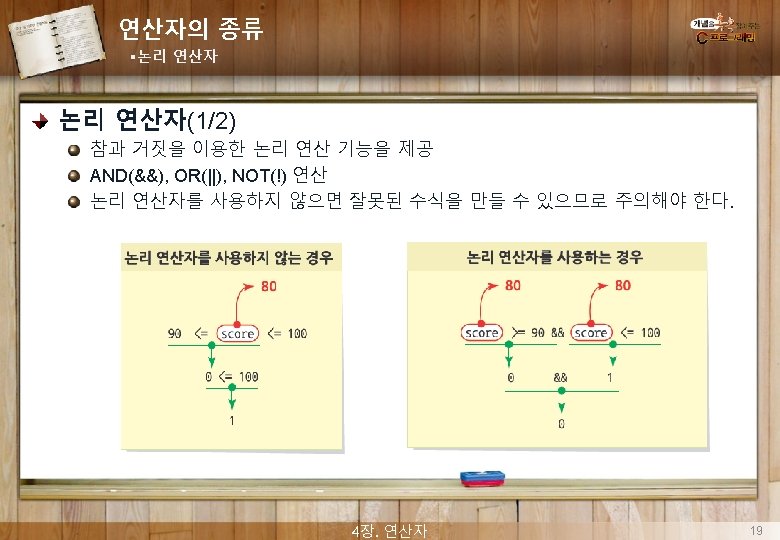
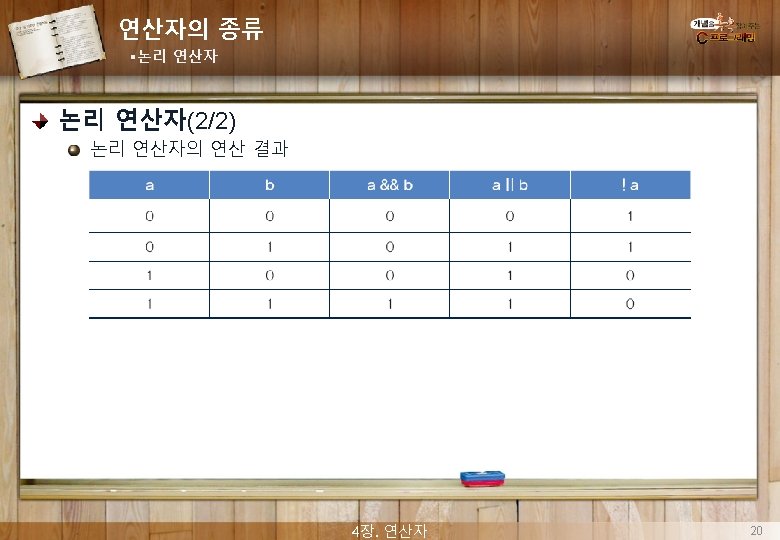
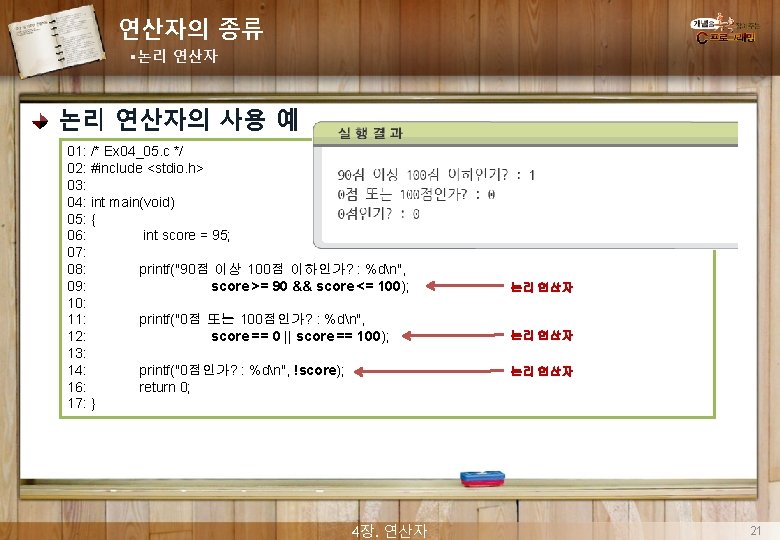
연산자의 종류 §논리 연산자의 사용 예 01: /* Ex 04_05. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int score = 95; 07: 08: printf("90점 이상 100점 이하인가? : %dn", 09: score >= 90 && score <= 100); 10: 11: printf("0점 또는 100점인가? : %dn", 12: score == 0 || score == 100); 13: 14: printf("0점인가? : %dn", !score); 16: return 0; 17: } 4장. 연산자 논리 연산자 21
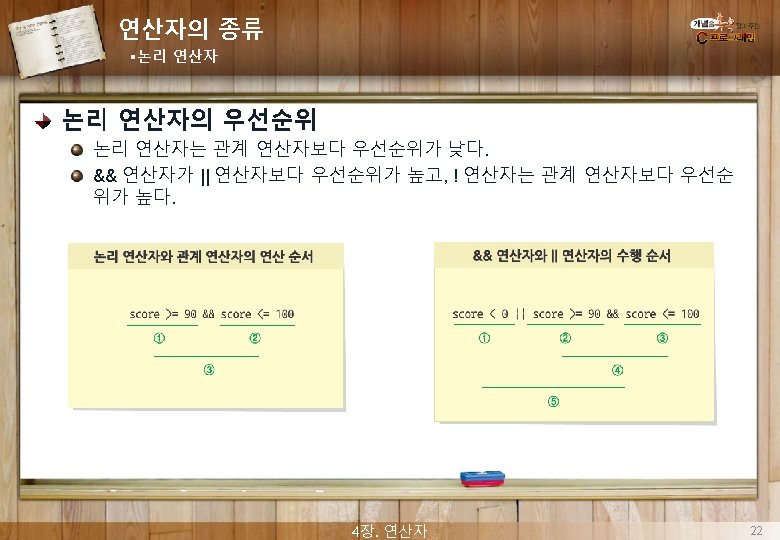
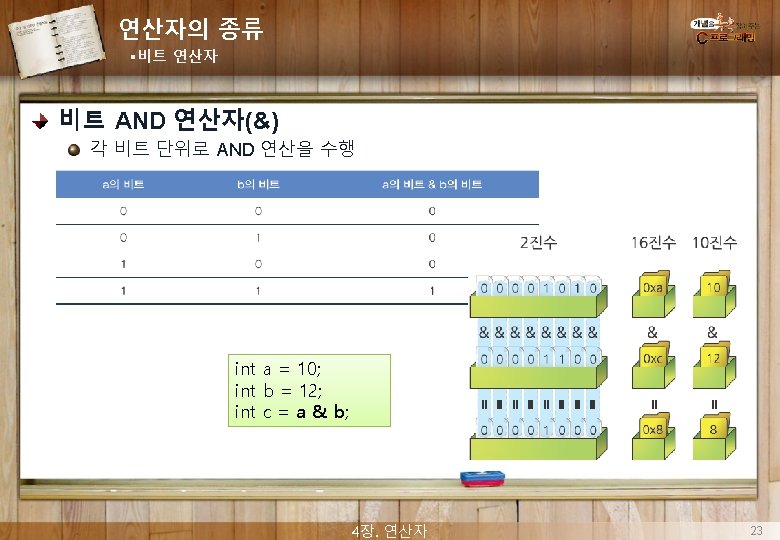
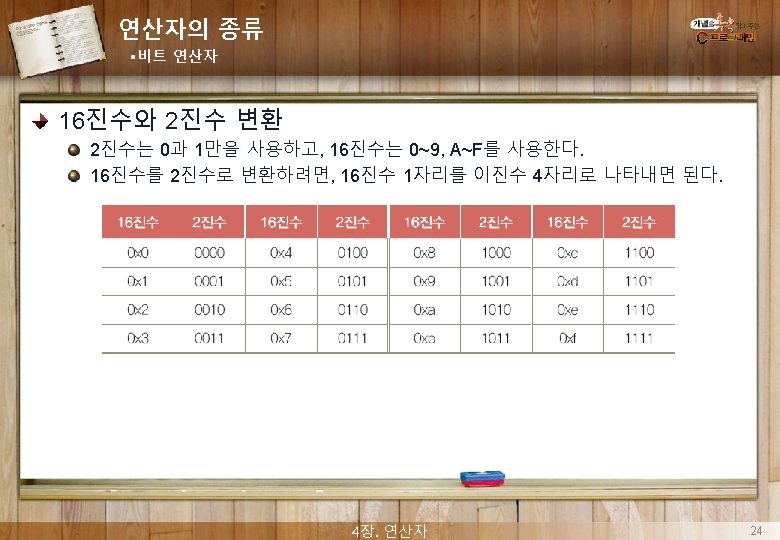
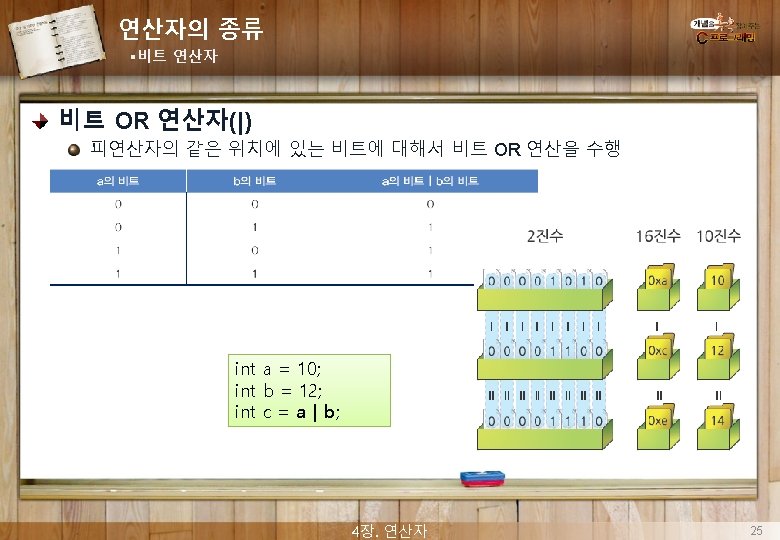
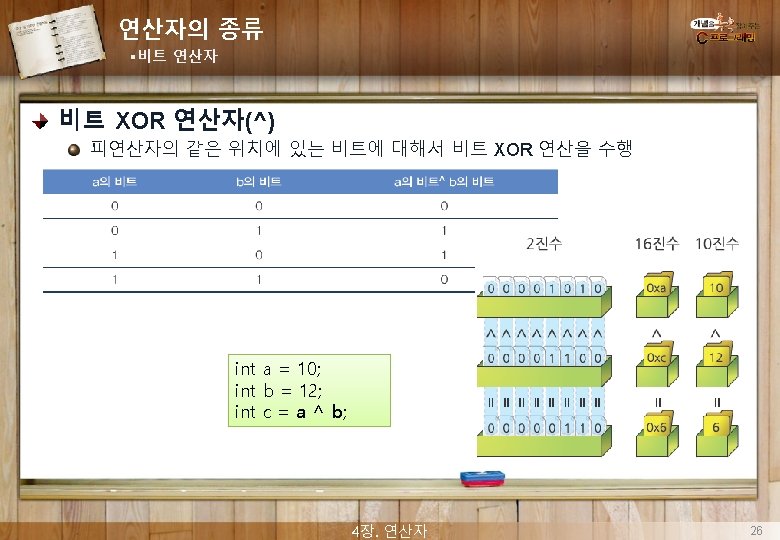
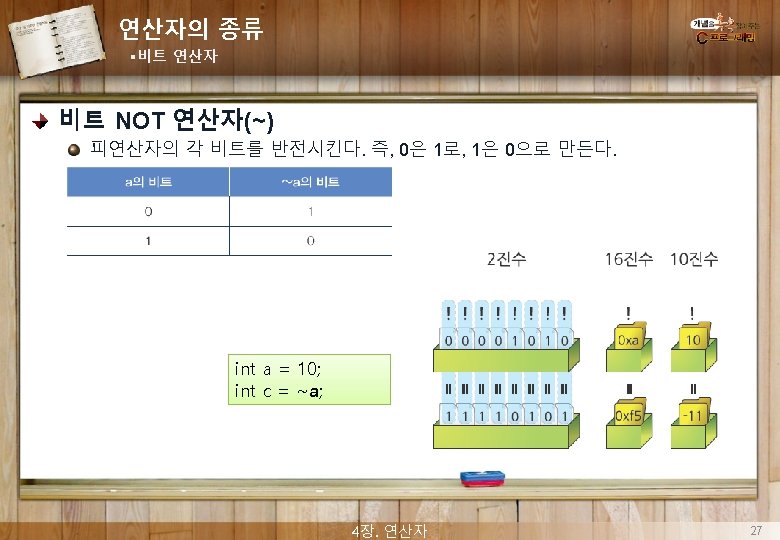
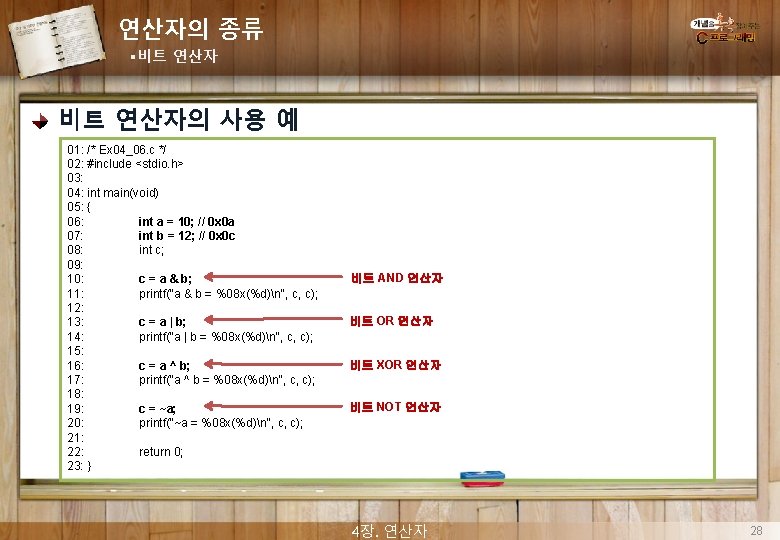
연산자의 종류 §비트 연산자의 사용 예 01: /* Ex 04_06. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int a = 10; // 0 x 0 a 07: int b = 12; // 0 x 0 c 08: int c; 09: 10: c = a & b; 11: printf("a & b = %08 x(%d)n", c, c); 12: 13: c = a | b; 14: printf("a | b = %08 x(%d)n", c, c); 15: 16: c = a ^ b; 17: printf("a ^ b = %08 x(%d)n", c, c); 18: 19: c = ~a; 20: printf("~a = %08 x(%d)n", c, c); 21: 22: return 0; 23: } 비트 AND 연산자 비트 OR 연산자 비트 XOR 연산자 비트 NOT 연산자 4장. 연산자 28
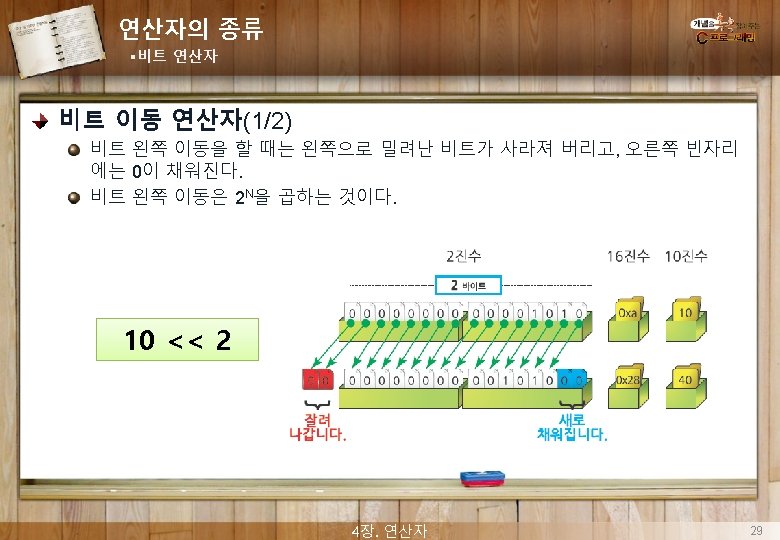
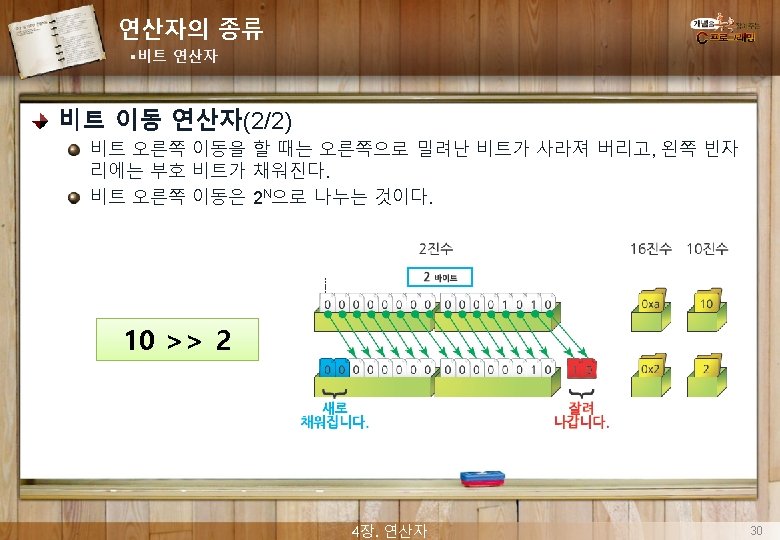
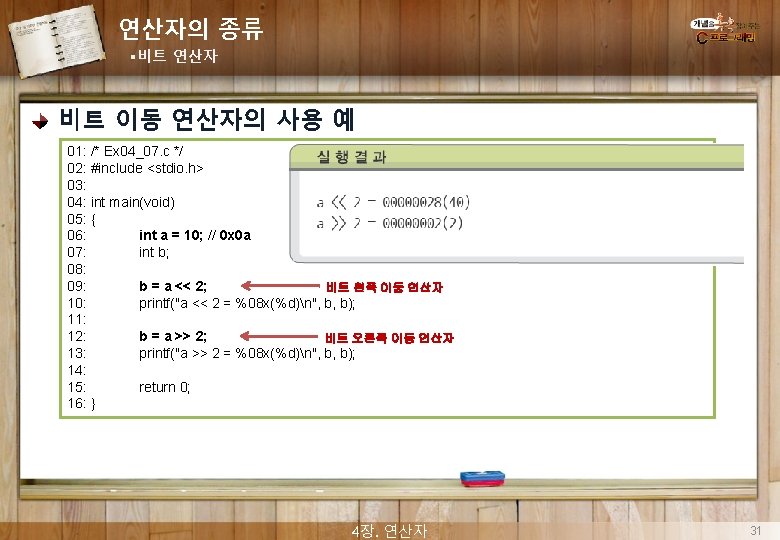
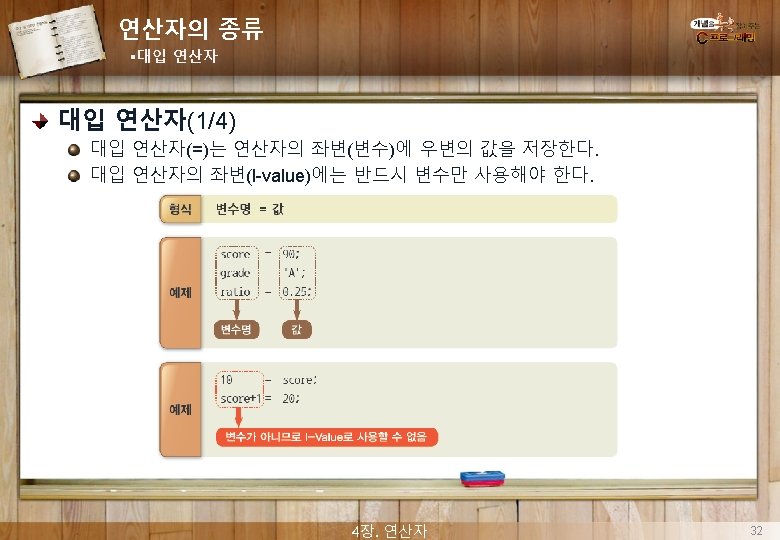
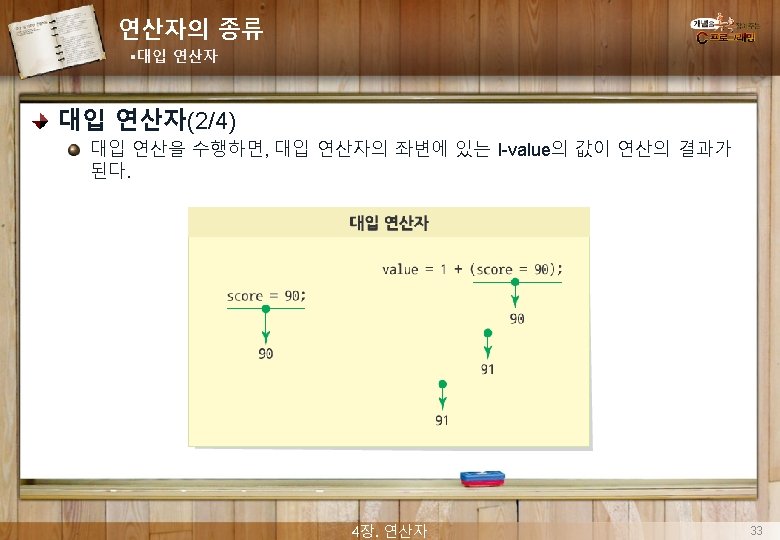
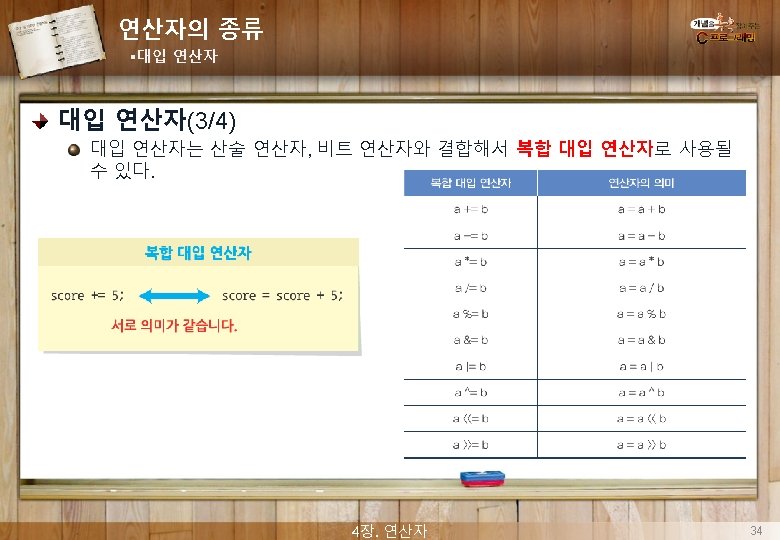
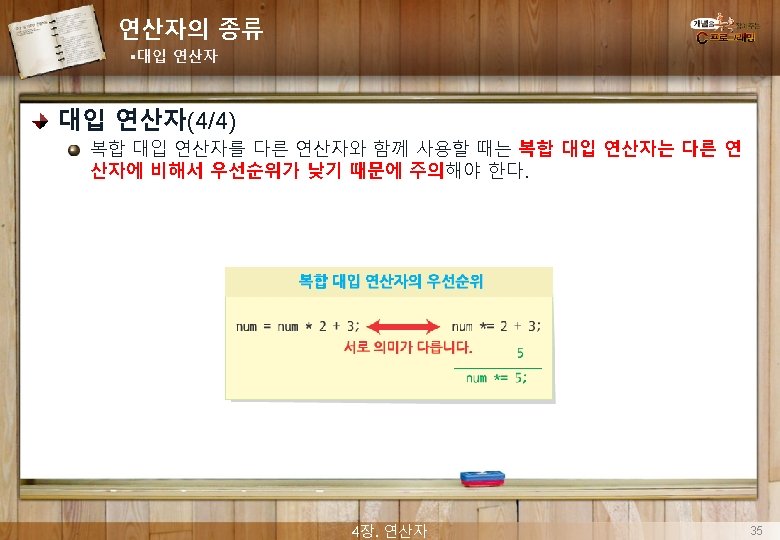
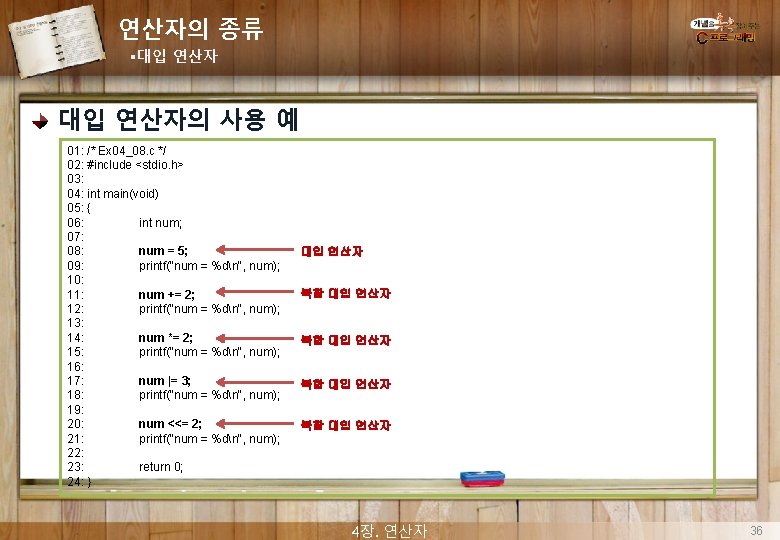
연산자의 종류 §대입 연산자의 사용 예 01: /* Ex 04_08. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int num; 07: 08: num = 5; 09: printf("num = %dn", num); 10: 11: num += 2; 12: printf("num = %dn", num); 13: 14: num *= 2; 15: printf("num = %dn", num); 16: 17: num |= 3; 18: printf("num = %dn", num); 19: 20: num <<= 2; 21: printf("num = %dn", num); 22: 23: return 0; 24: } 대입 연산자 복합 대입 연산자 4장. 연산자 36
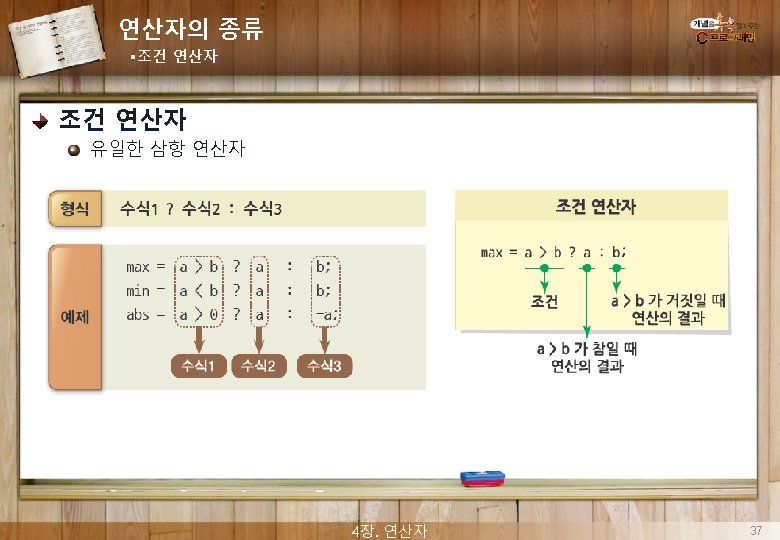
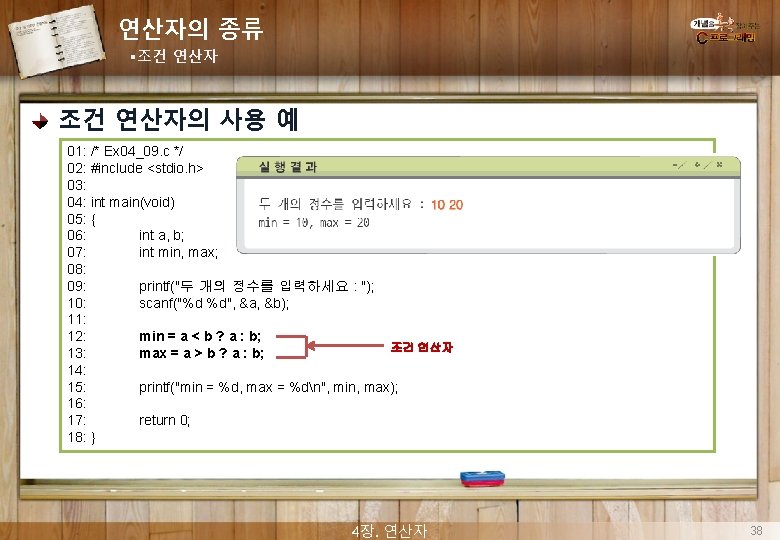
연산자의 종류 §조건 연산자의 사용 예 01: /* Ex 04_09. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int a, b; 07: int min, max; 08: 09: printf("두 개의 정수를 입력하세요 : "); 10: scanf("%d %d", &a, &b); 11: 12: min = a < b ? a : b; 조건 연산자 13: max = a > b ? a : b; 14: 15: printf("min = %d, max = %dn", min, max); 16: 17: return 0; 18: } 4장. 연산자 38
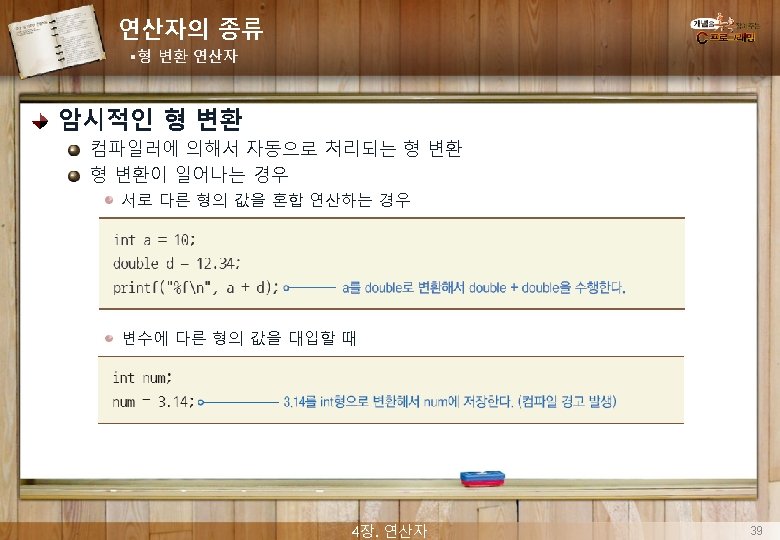
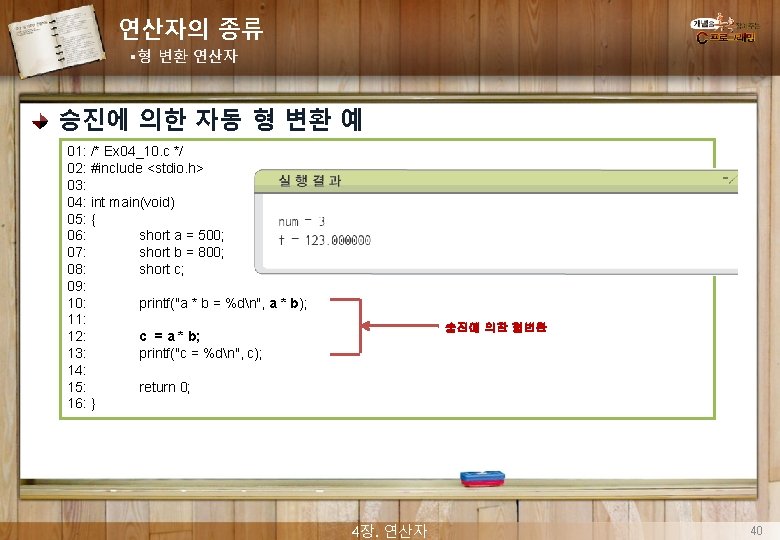
연산자의 종류 §형 변환 연산자 승진에 의한 자동 형 변환 예 01: /* Ex 04_10. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: short a = 500; 07: short b = 800; 08: short c; 09: 10: printf("a * b = %dn", a * b); 11: 12: c = a * b; 13: printf("c = %dn", c); 14: 15: return 0; 16: } 승진에 의한 형변환 4장. 연산자 40
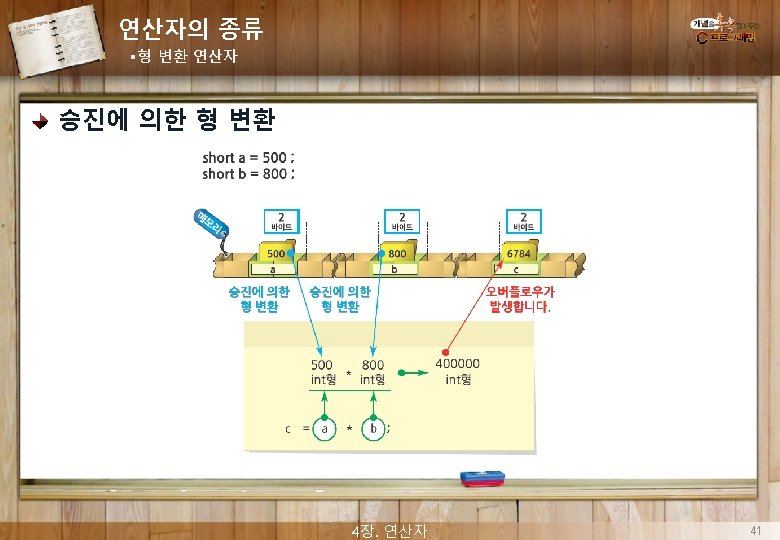
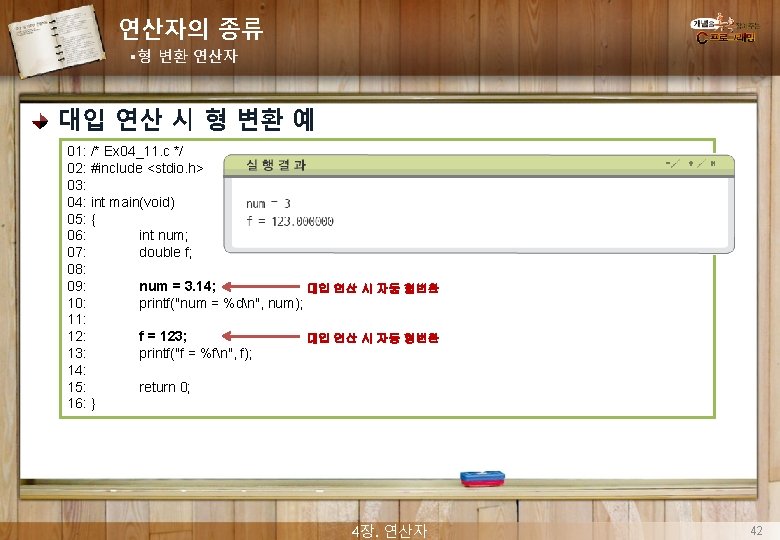
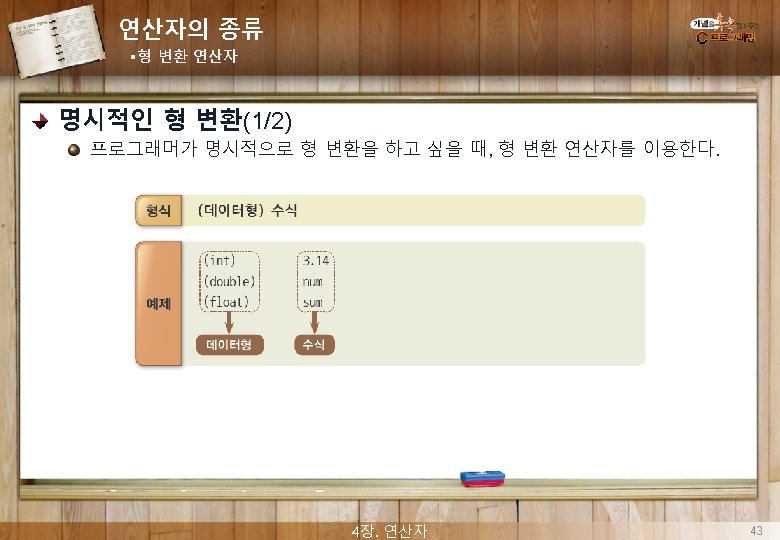
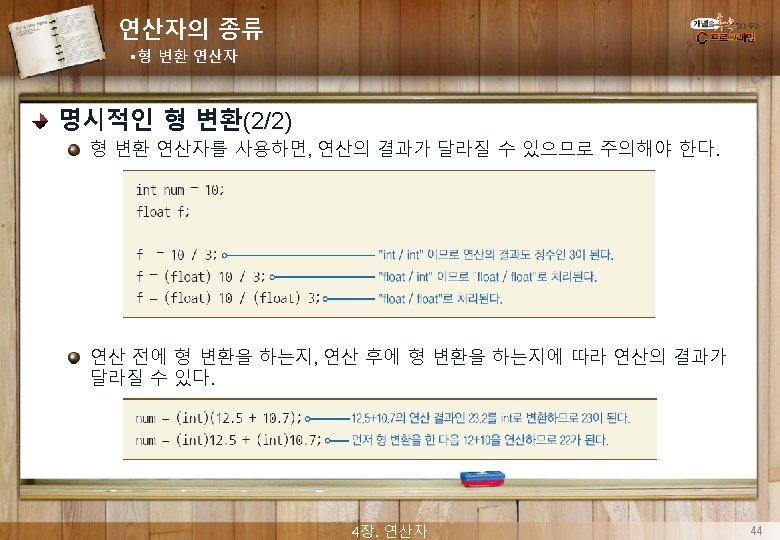
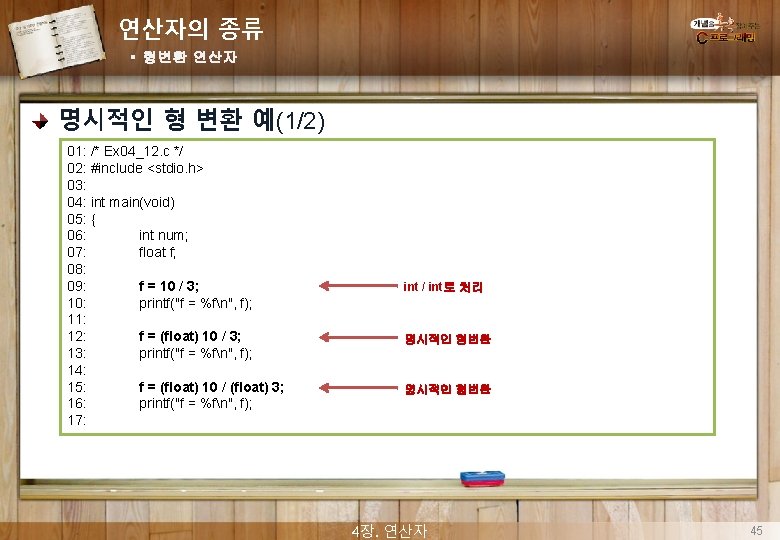
연산자의 종류 § 형변환 연산자 명시적인 형 변환 예(1/2) 01: /* Ex 04_12. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int num; 07: float f; 08: 09: f = 10 / 3; 10: printf("f = %fn", f); 11: 12: f = (float) 10 / 3; 13: printf("f = %fn", f); 14: 15: f = (float) 10 / (float) 3; 16: printf("f = %fn", f); 17: int / int로 처리 명시적인 형변환 4장. 연산자 45
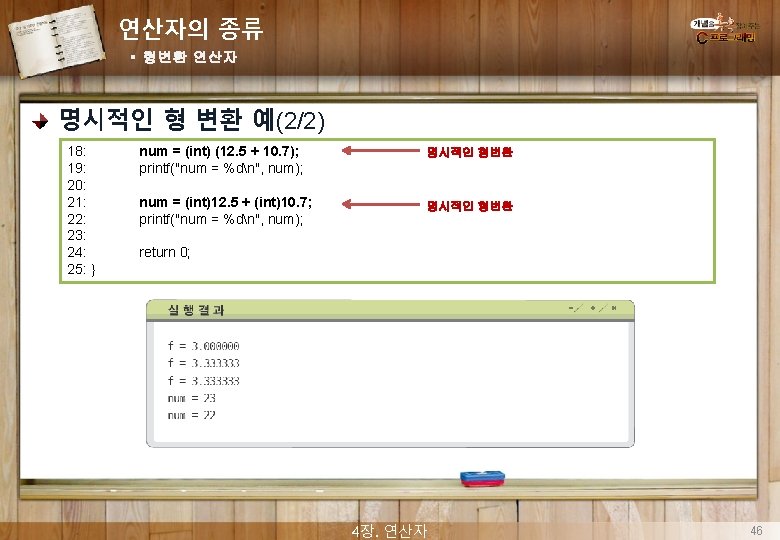
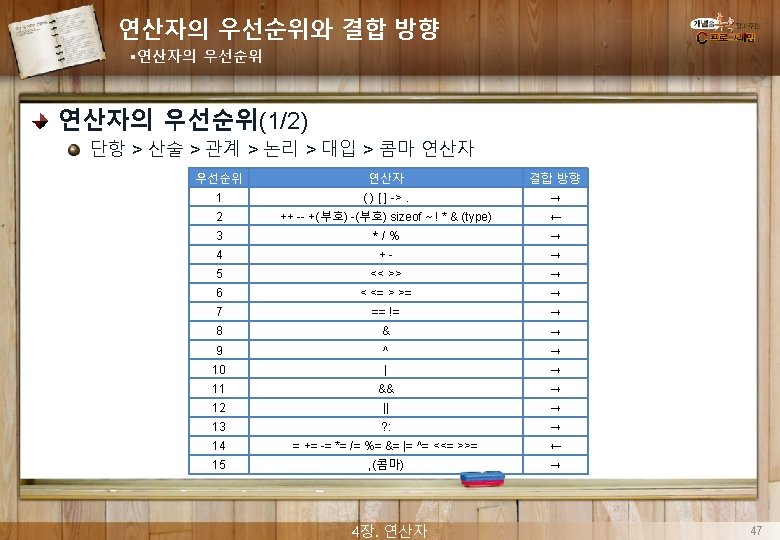
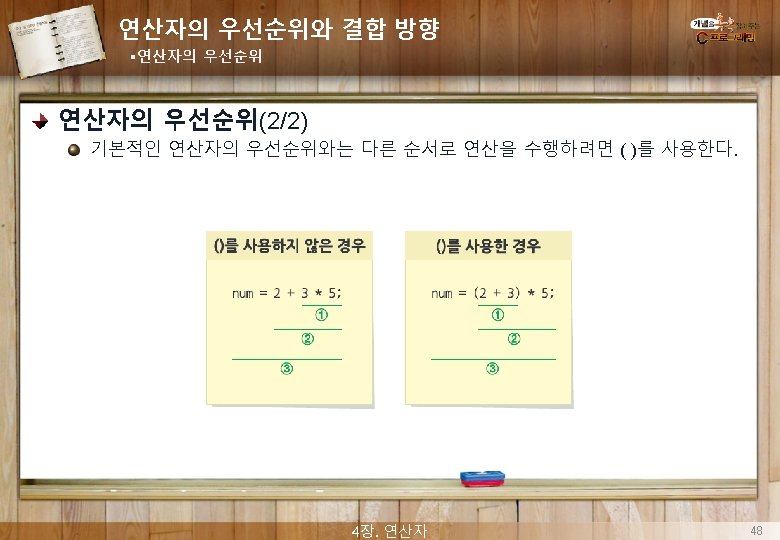
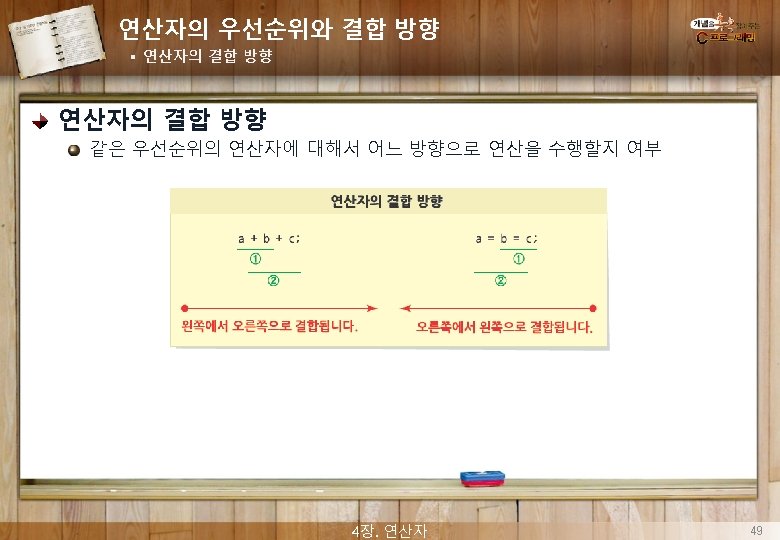
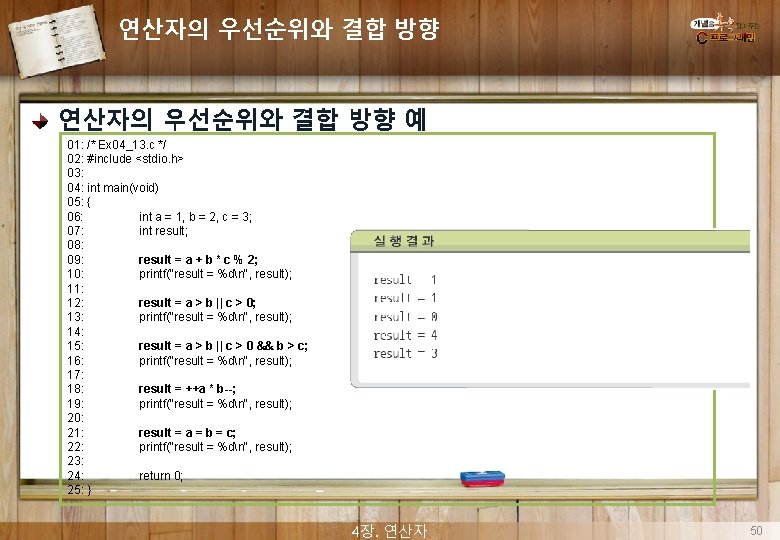
연산자의 우선순위와 결합 방향 예 01: /* Ex 04_13. c */ 02: #include <stdio. h> 03: 04: int main(void) 05: { 06: int a = 1, b = 2, c = 3; 07: int result; 08: 09: result = a + b * c % 2; 10: printf("result = %dn", result); 11: 12: result = a > b || c > 0; 13: printf("result = %dn", result); 14: 15: result = a > b || c > 0 && b > c; 16: printf("result = %dn", result); 17: 18: result = ++a * b--; 19: printf("result = %dn", result); 20: 21: result = a = b = c; 22: printf("result = %dn", result); 23: 24: return 0; 25: } 4장. 연산자 50
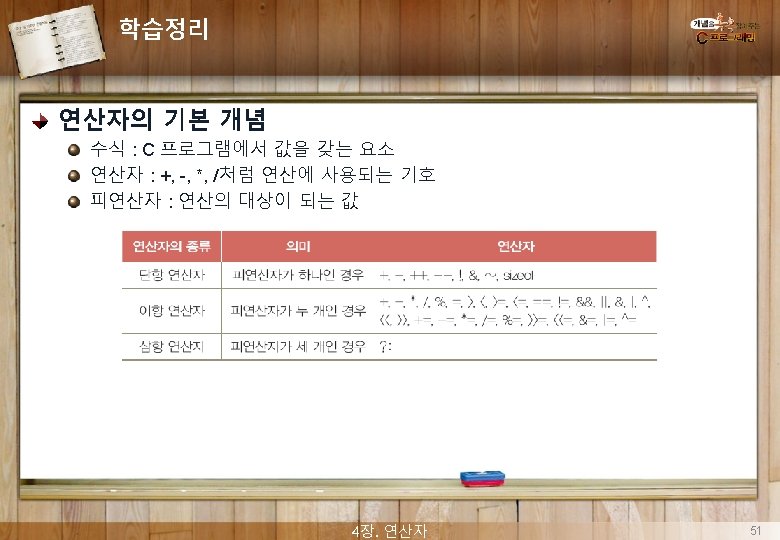
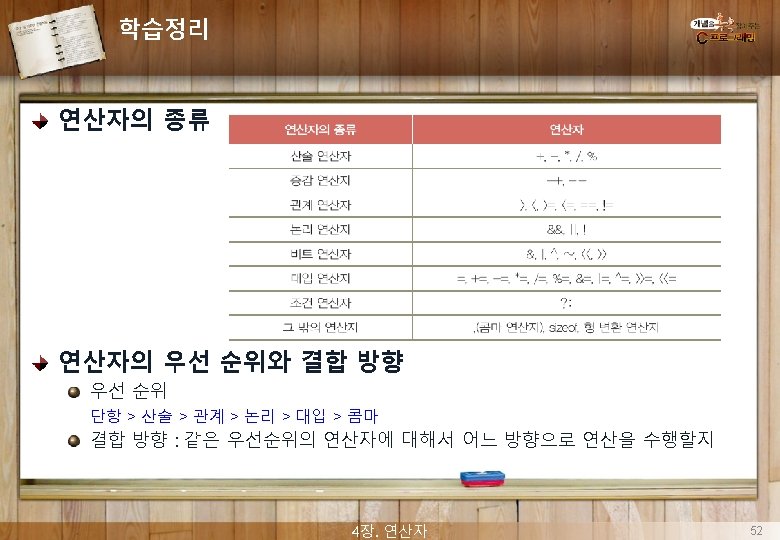
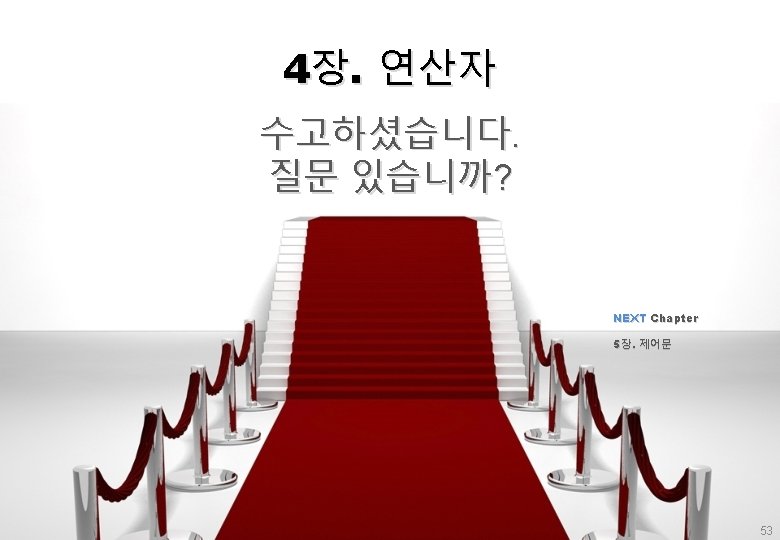