4 1 Introduction Arrays Structures of related data
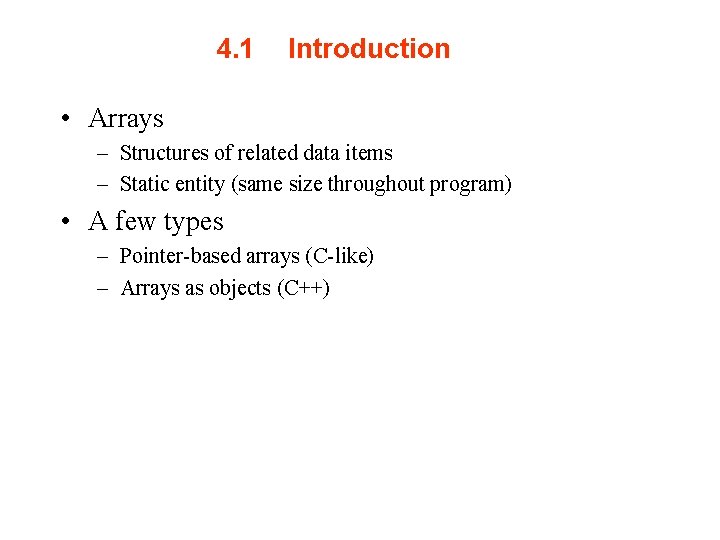
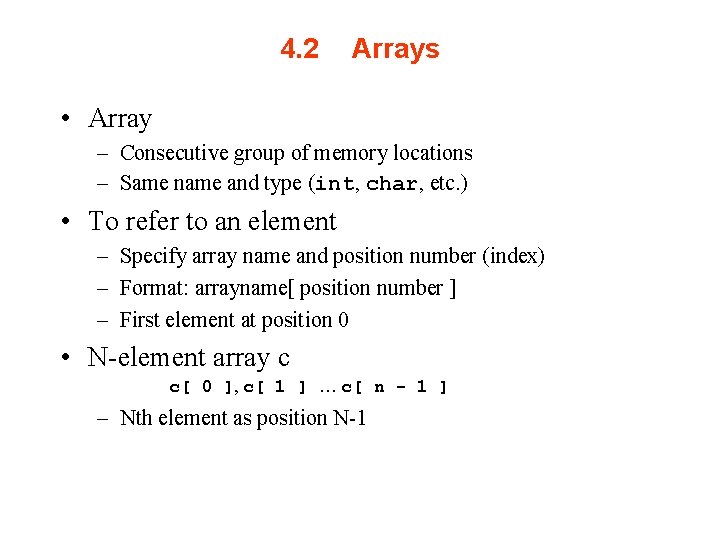
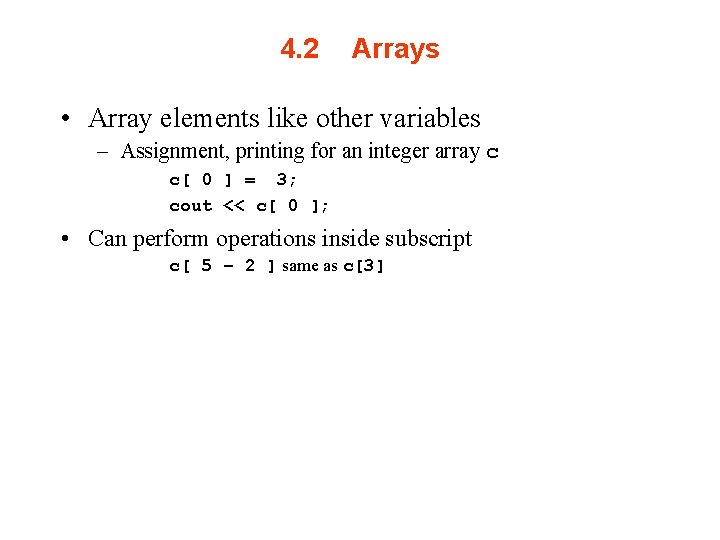
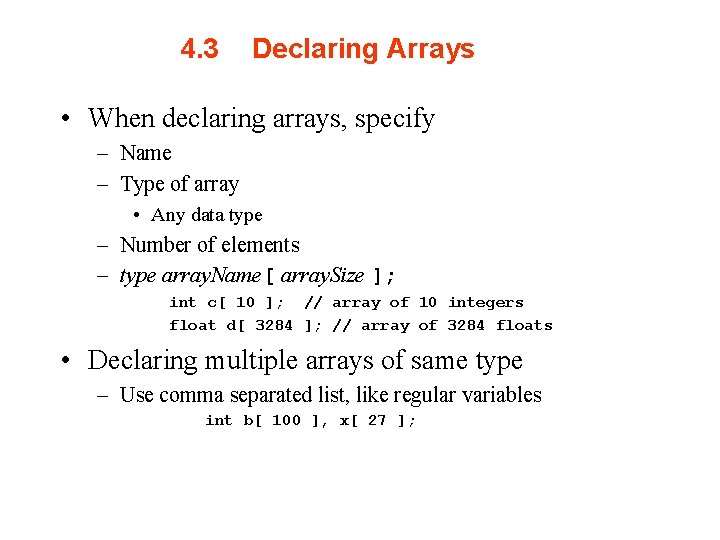
![4. 2 array name c is a (pointer) variable c = address of c[0] 4. 2 array name c is a (pointer) variable c = address of c[0]](https://slidetodoc.com/presentation_image_h2/5a69a87d8e850132eb89c46f1e908c36/image-5.jpg)
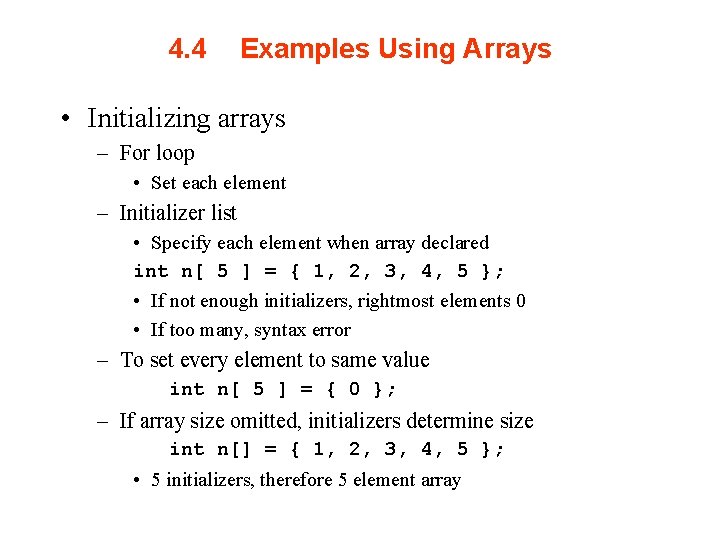
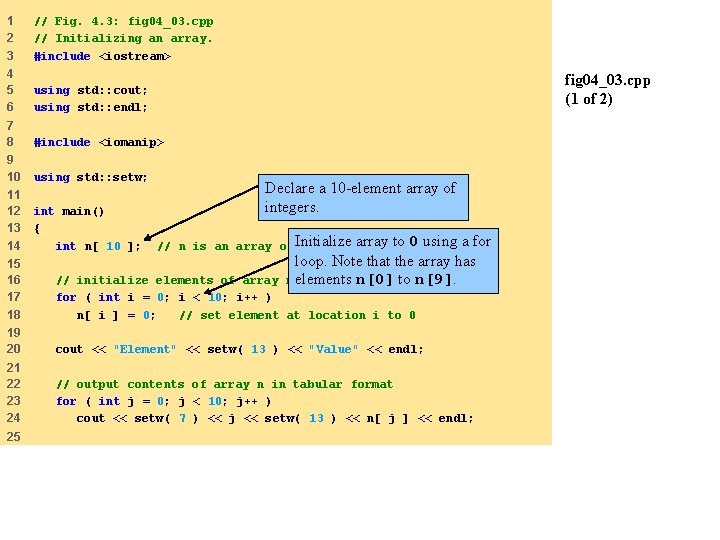
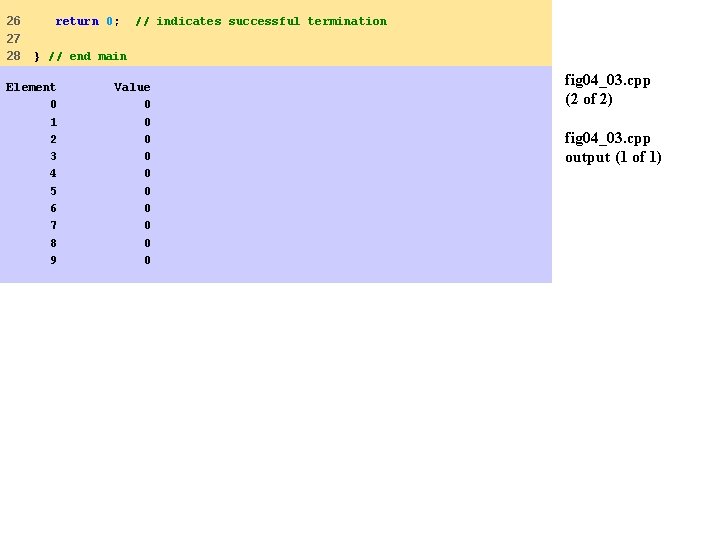
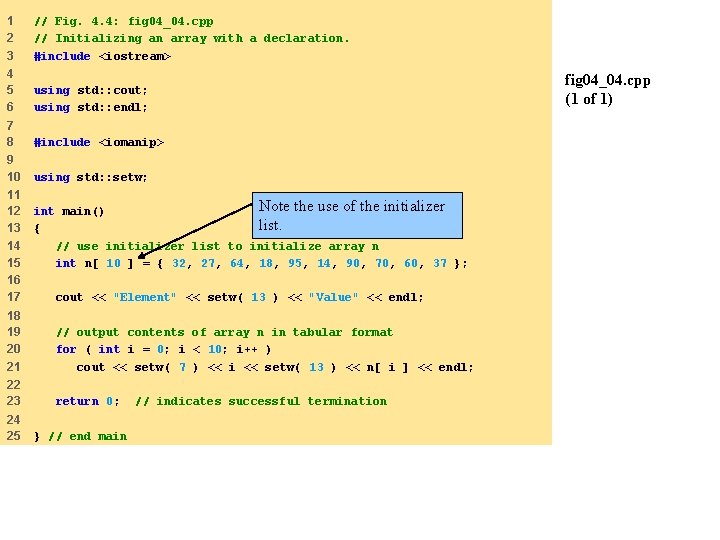
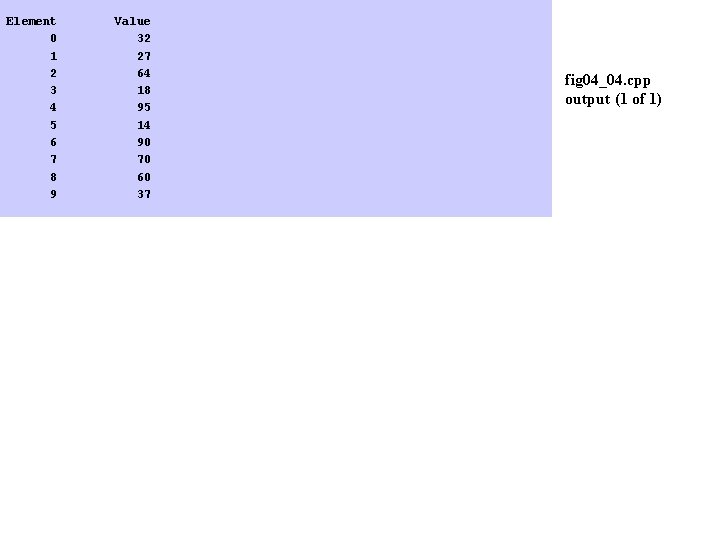
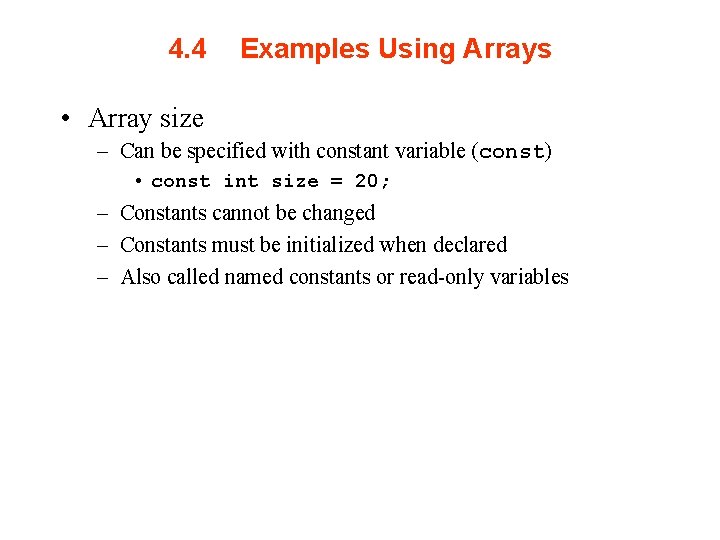
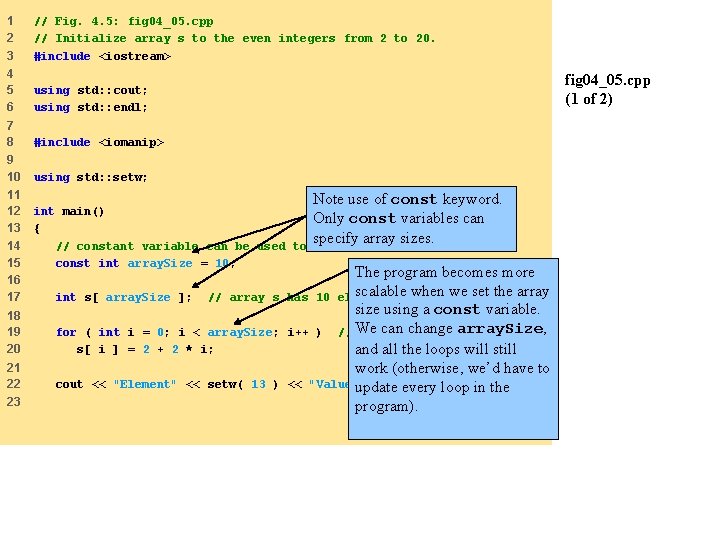
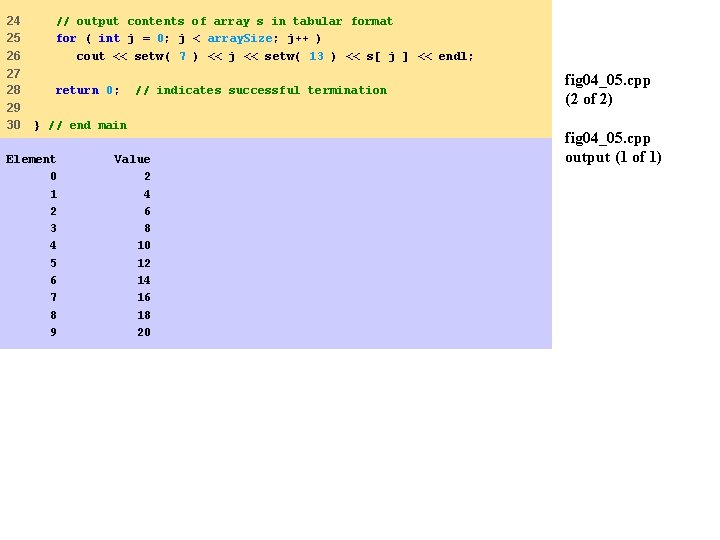
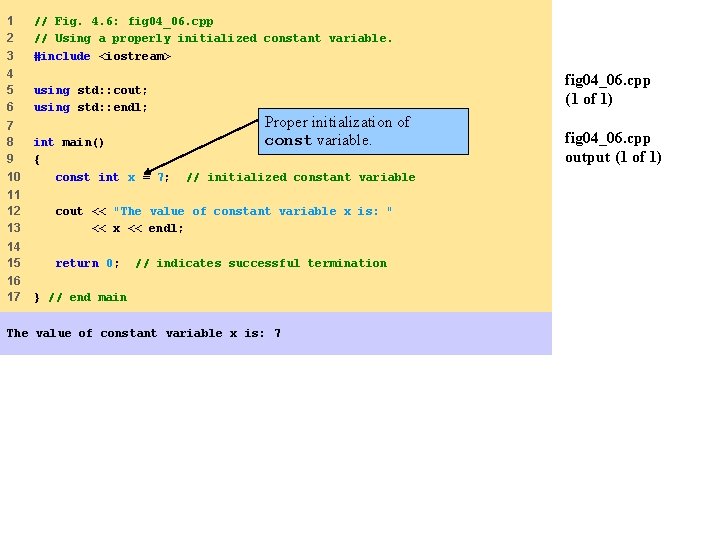
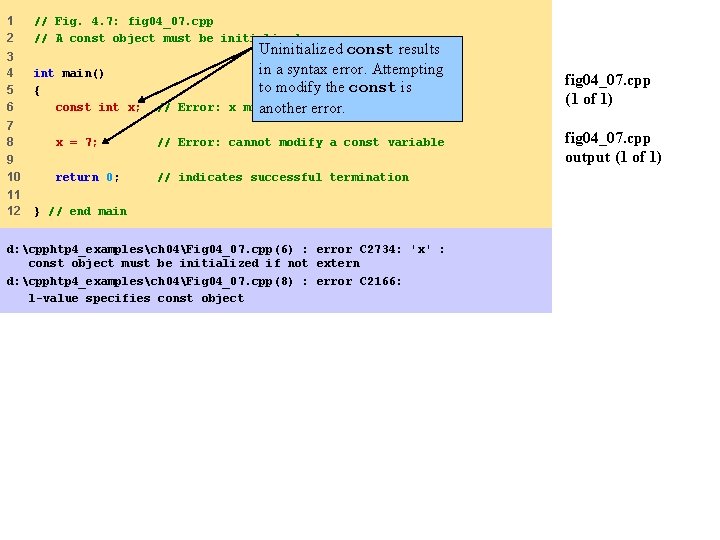
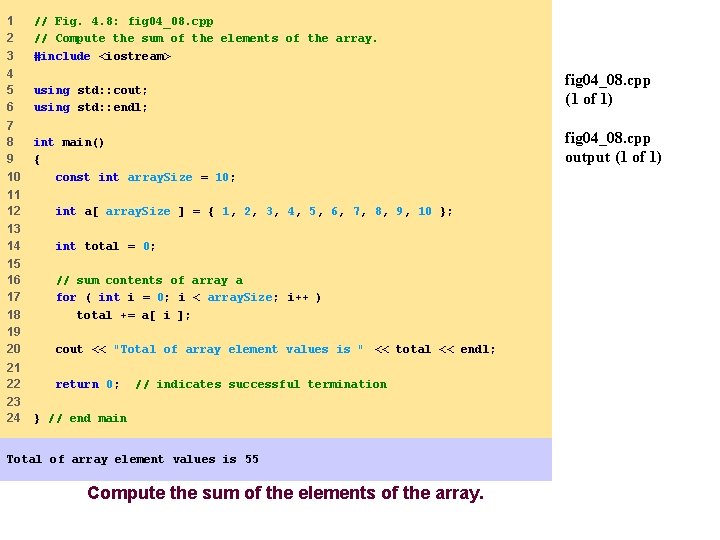
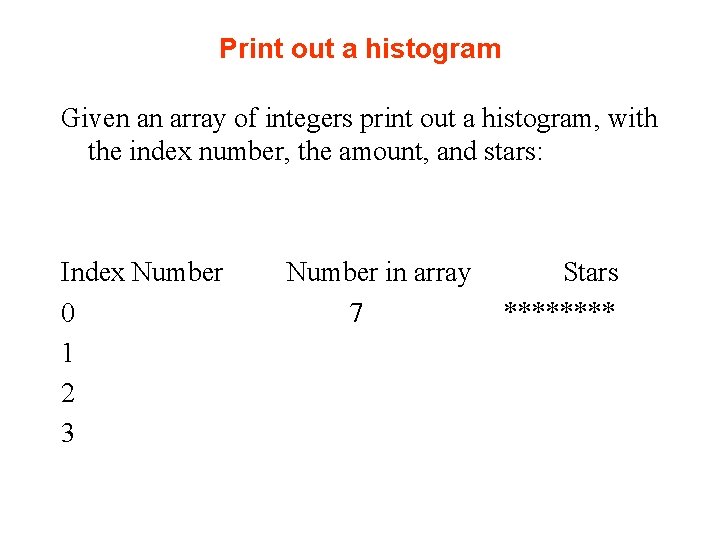
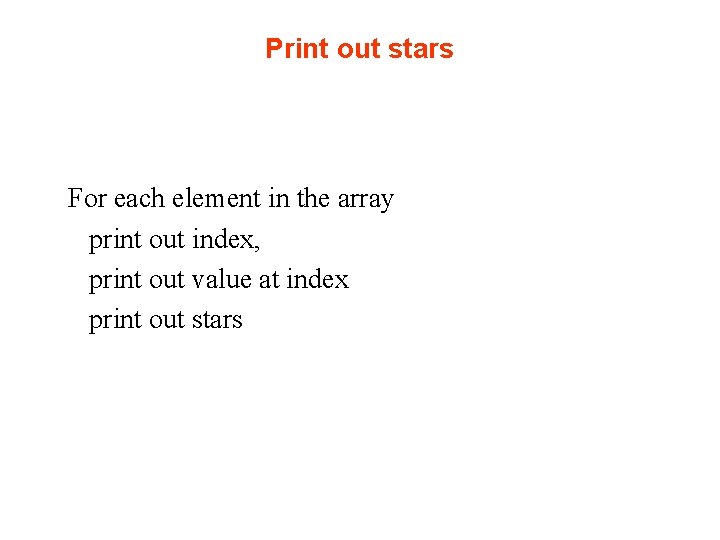
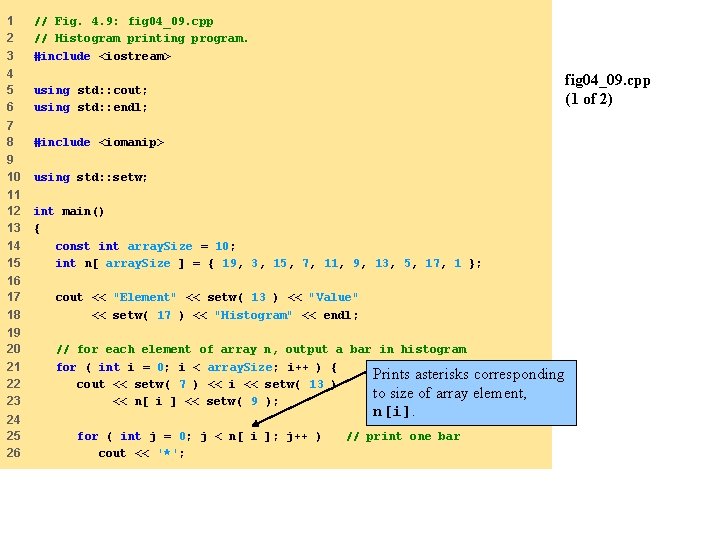
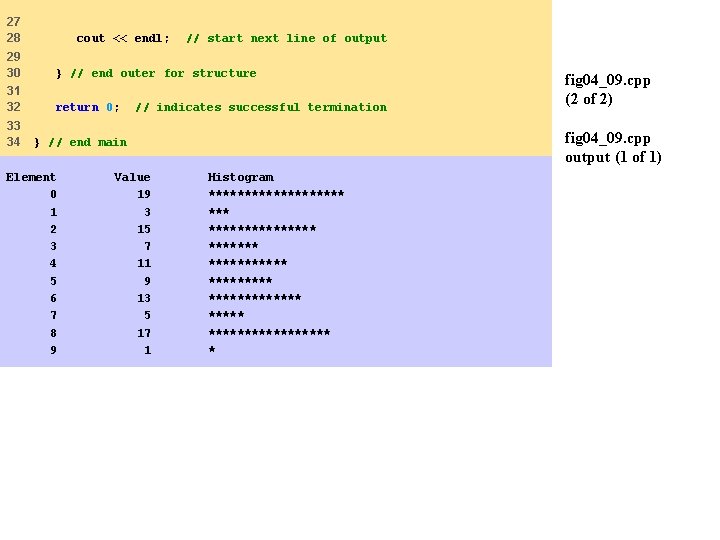
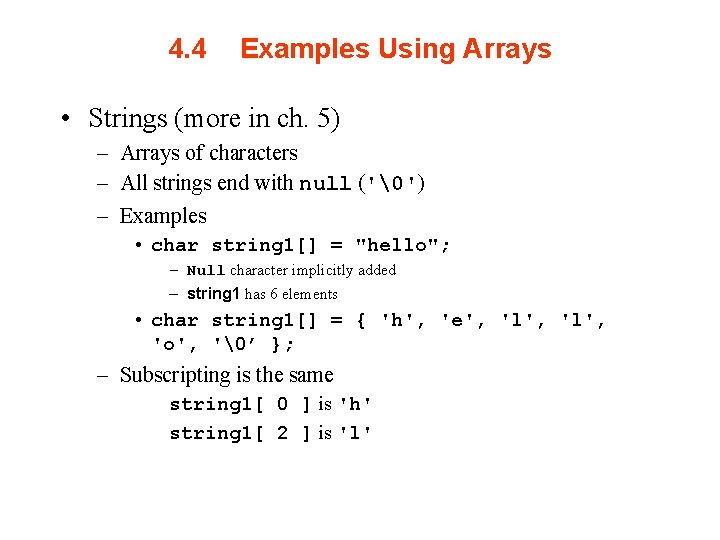
![4. 4 Examples Using Arrays • Input from keyboard char string 2[ 10 ]; 4. 4 Examples Using Arrays • Input from keyboard char string 2[ 10 ];](https://slidetodoc.com/presentation_image_h2/5a69a87d8e850132eb89c46f1e908c36/image-22.jpg)
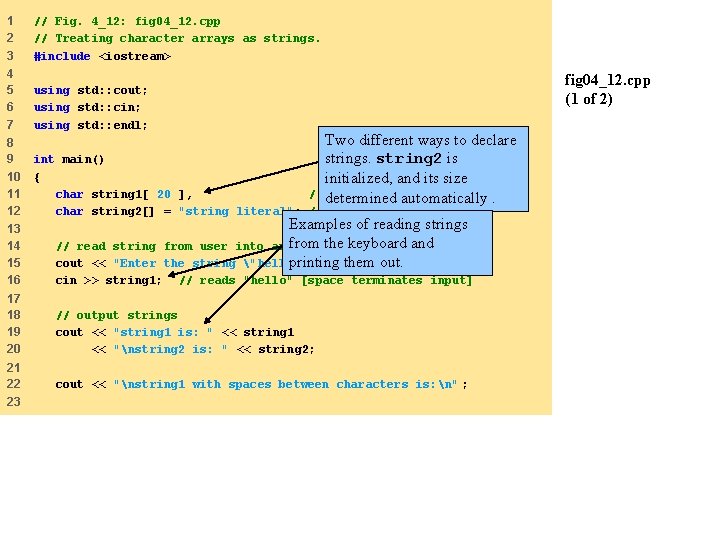
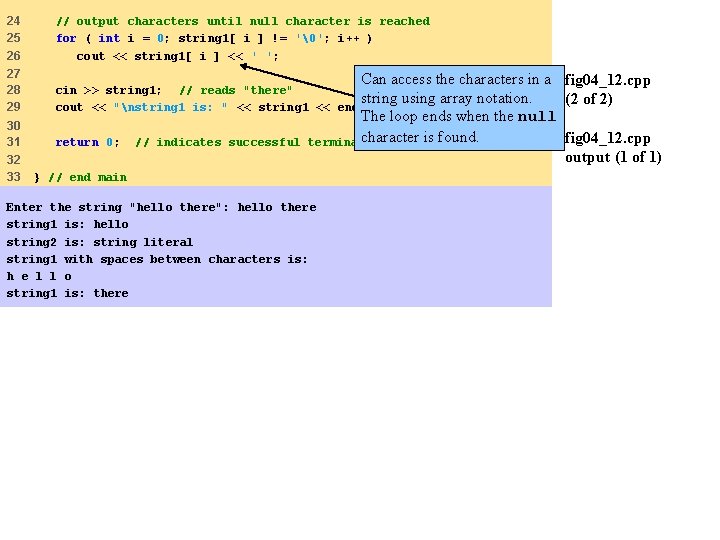
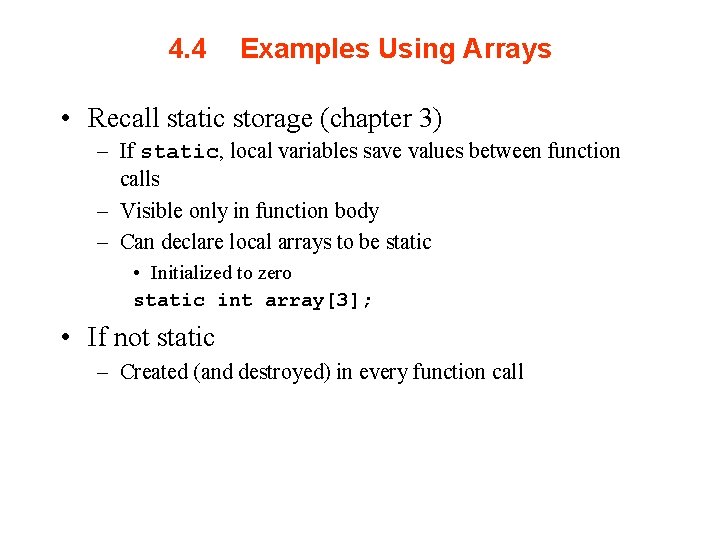
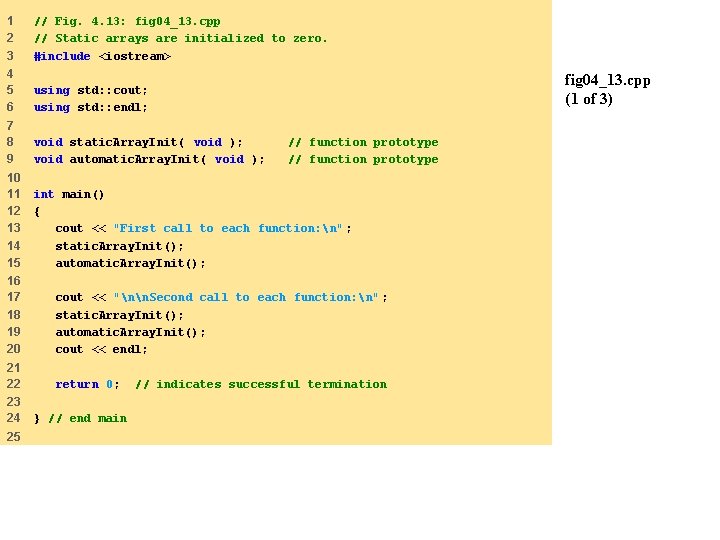
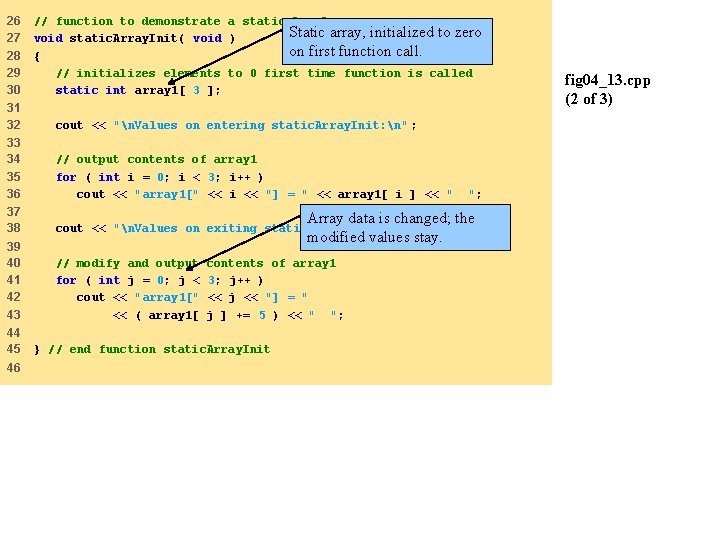
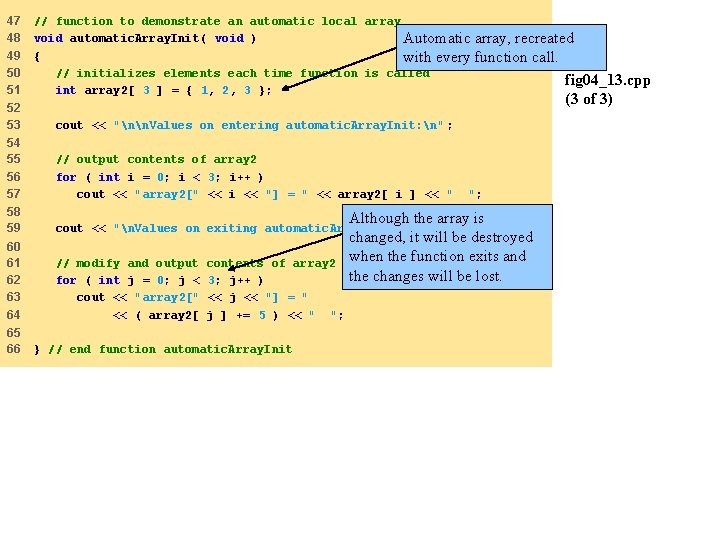
![First call to each function: Values on array 1[0] entering static. Array. Init: = First call to each function: Values on array 1[0] entering static. Array. Init: =](https://slidetodoc.com/presentation_image_h2/5a69a87d8e850132eb89c46f1e908c36/image-29.jpg)
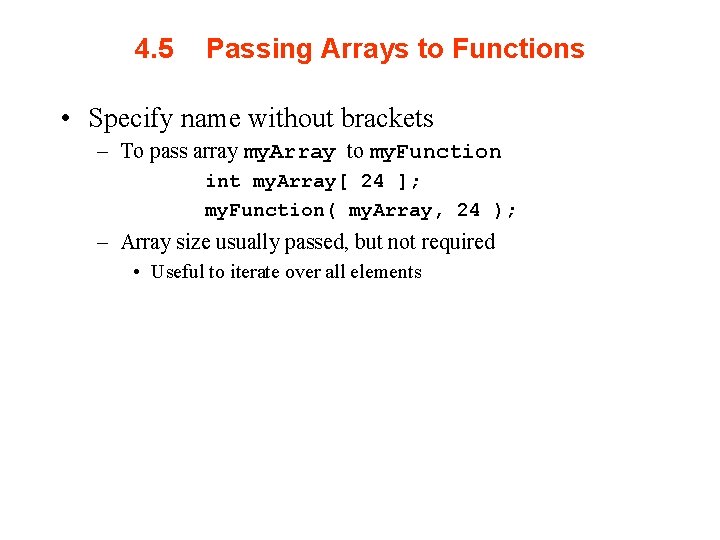
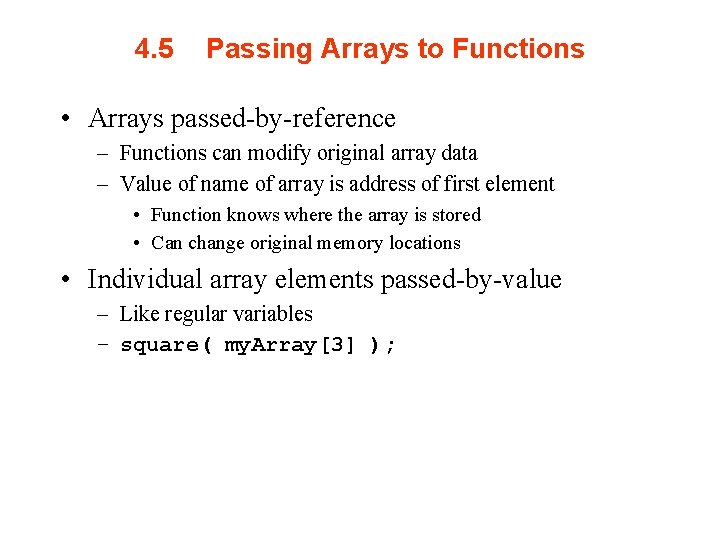
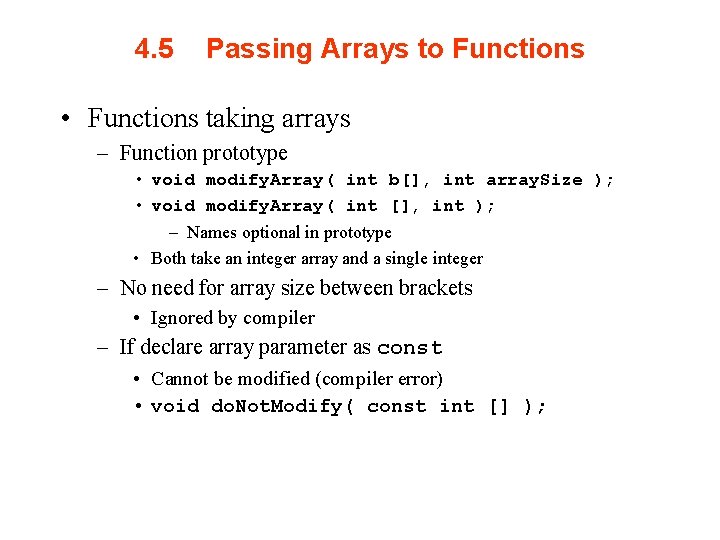
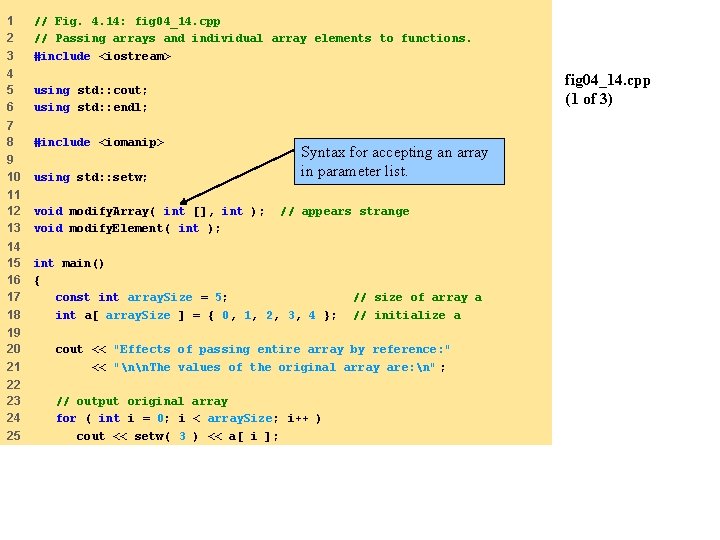
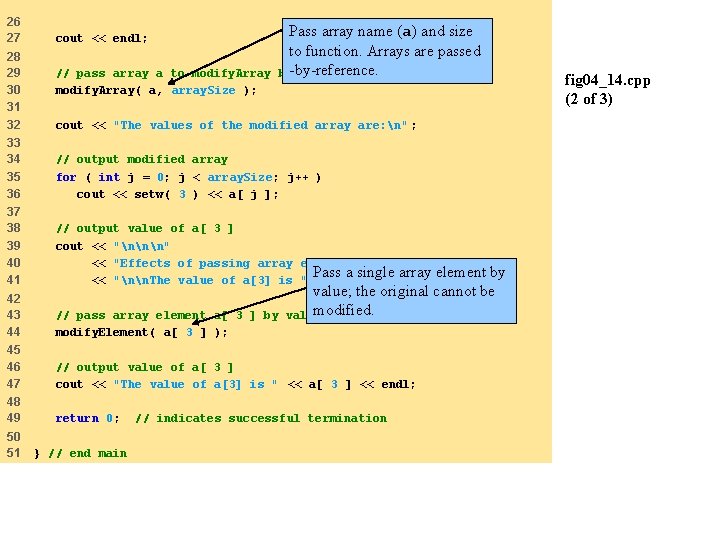
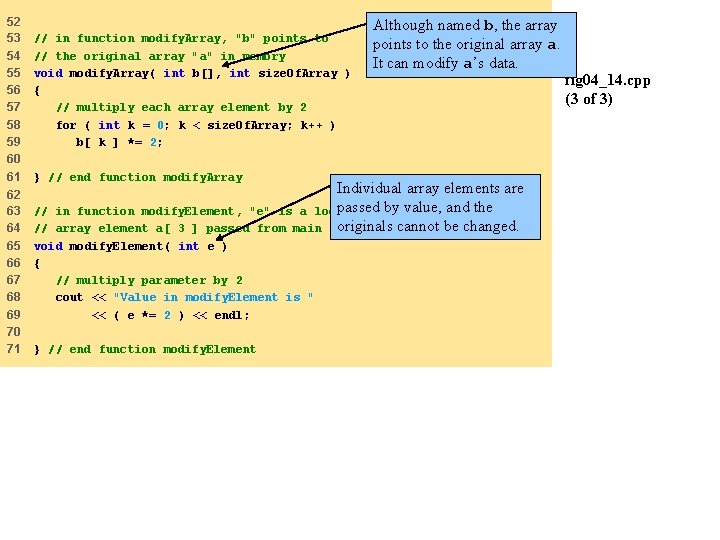
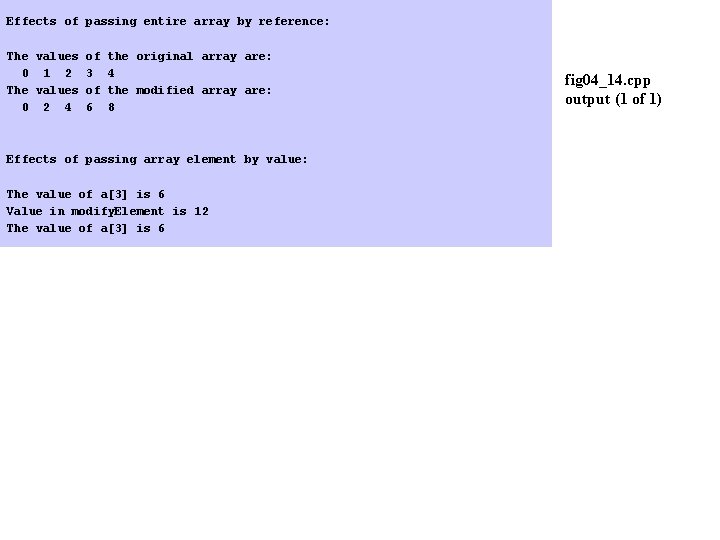
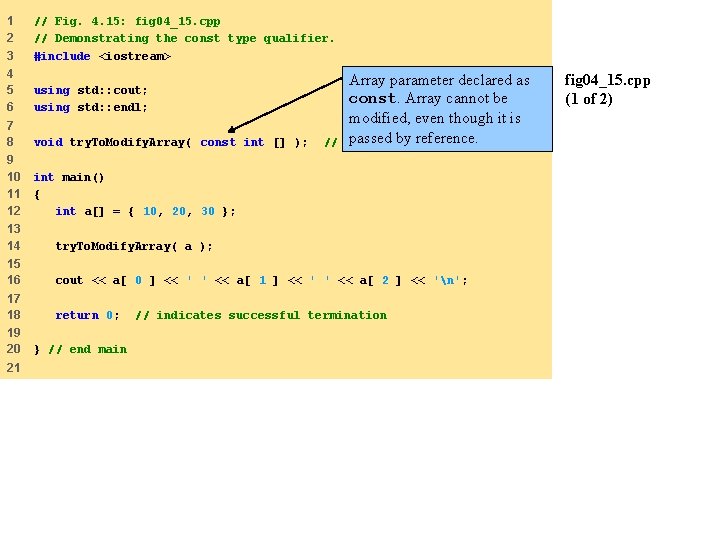
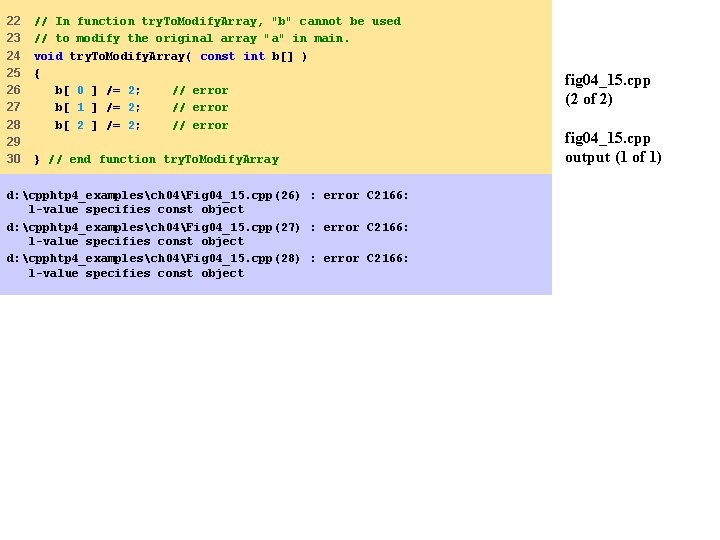
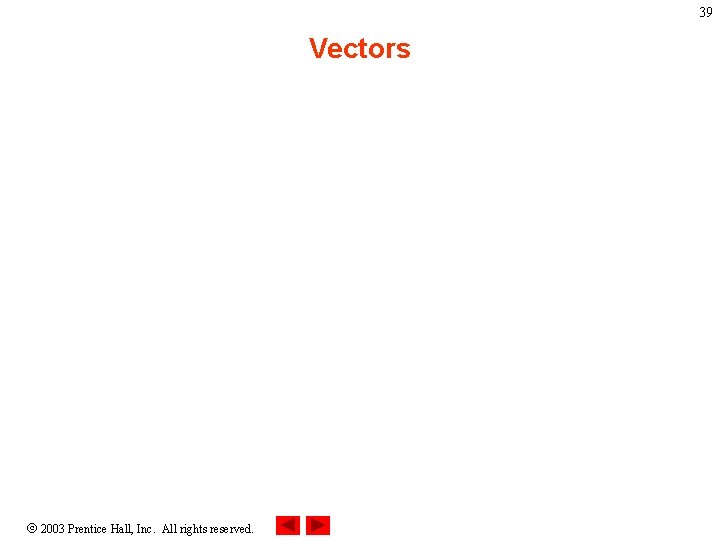
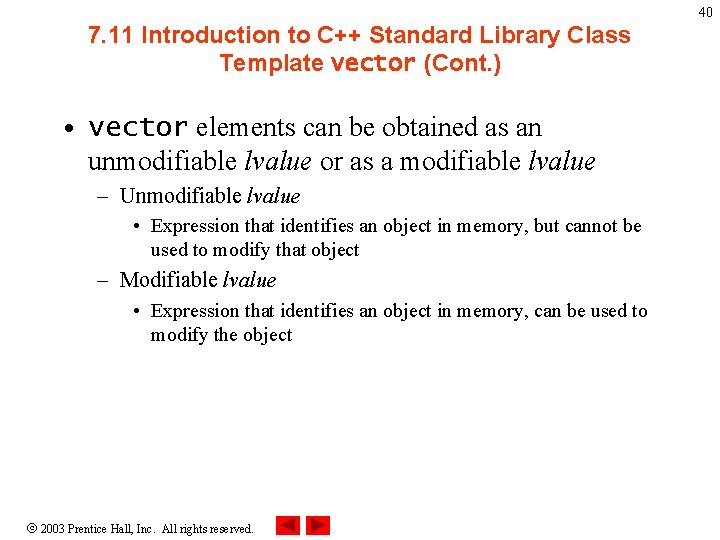
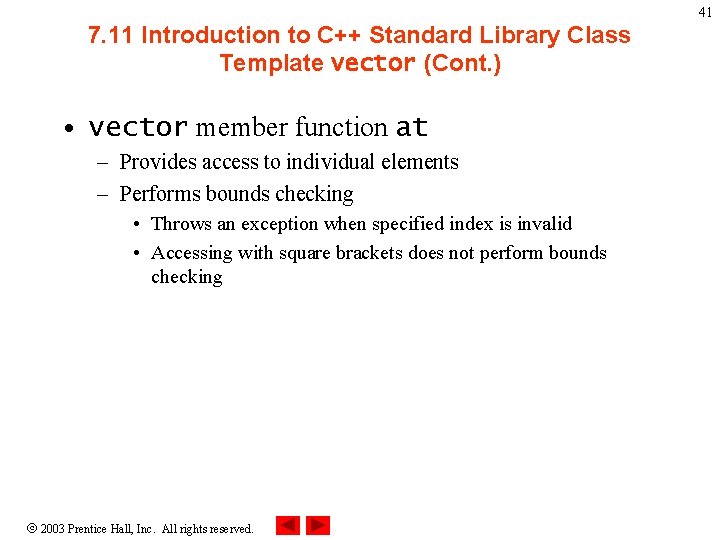
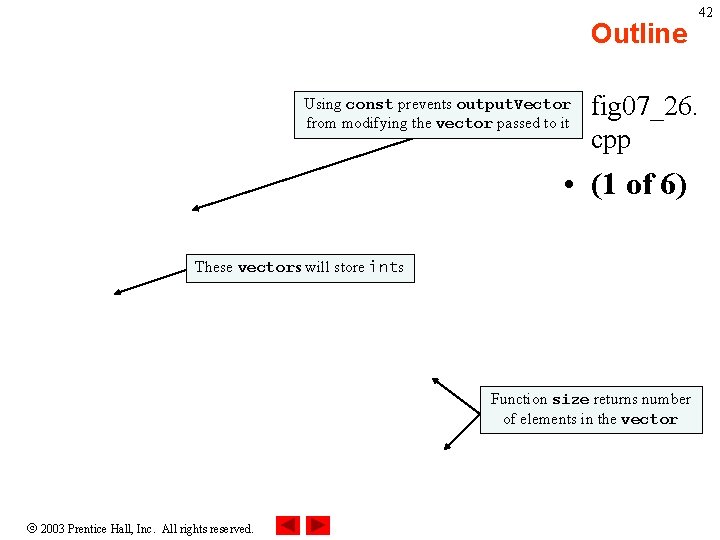
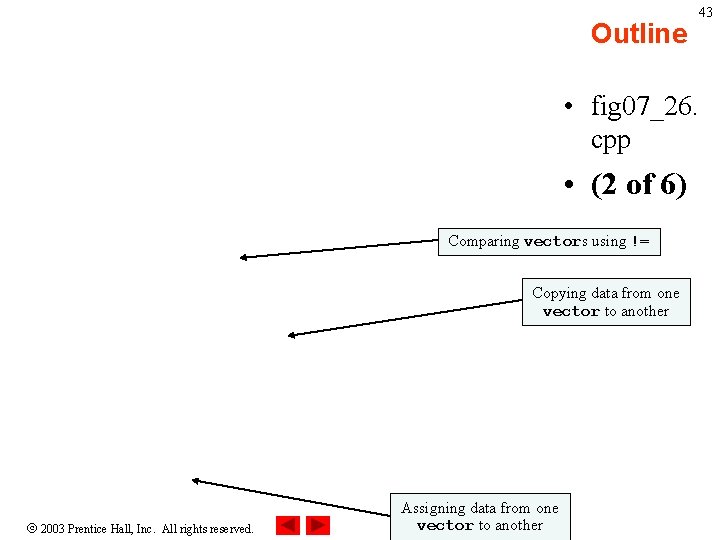
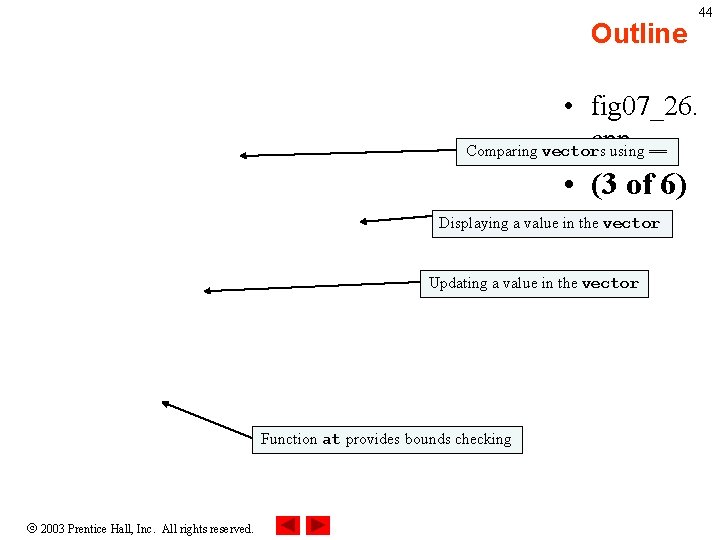
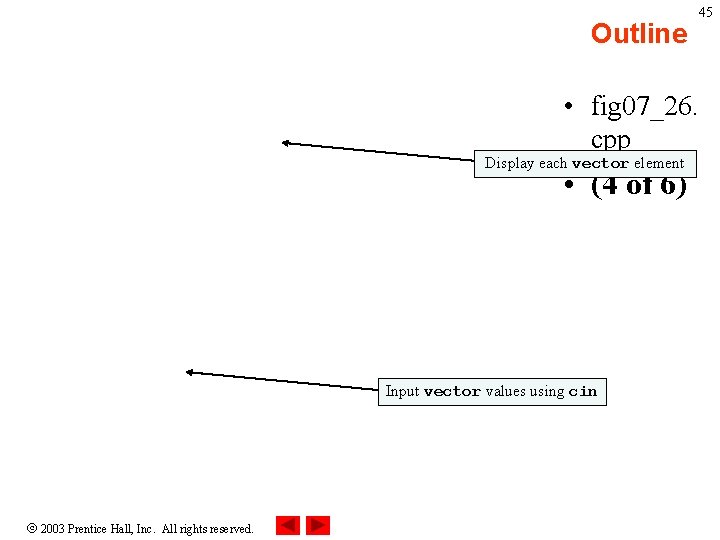
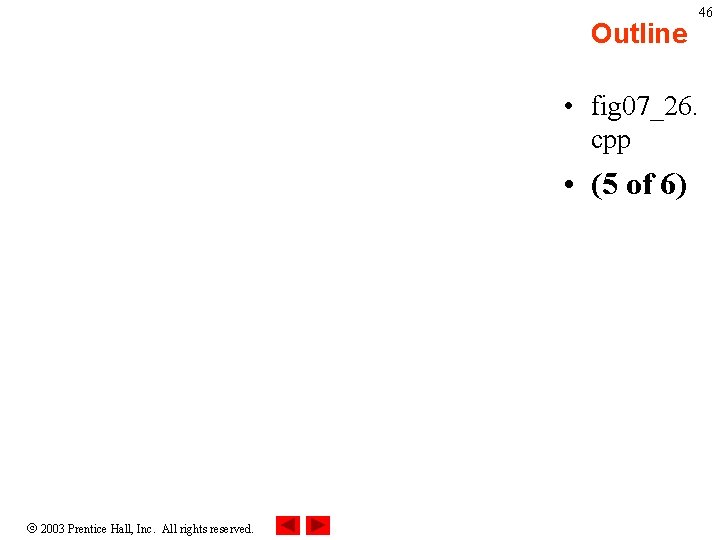
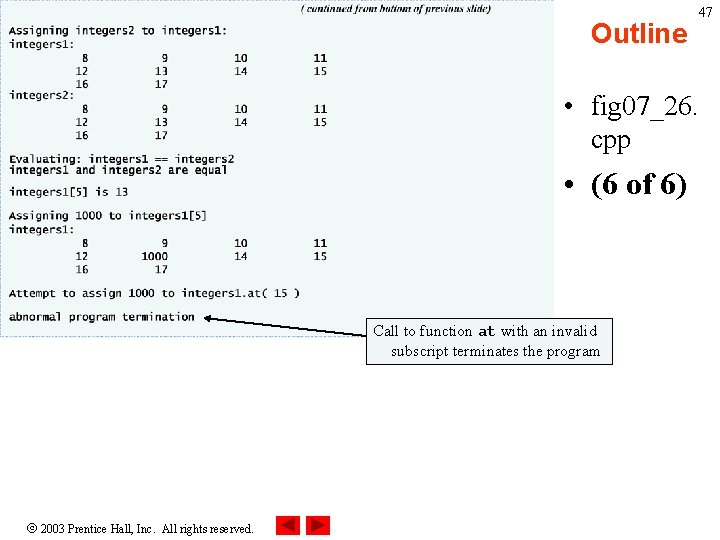
- Slides: 47
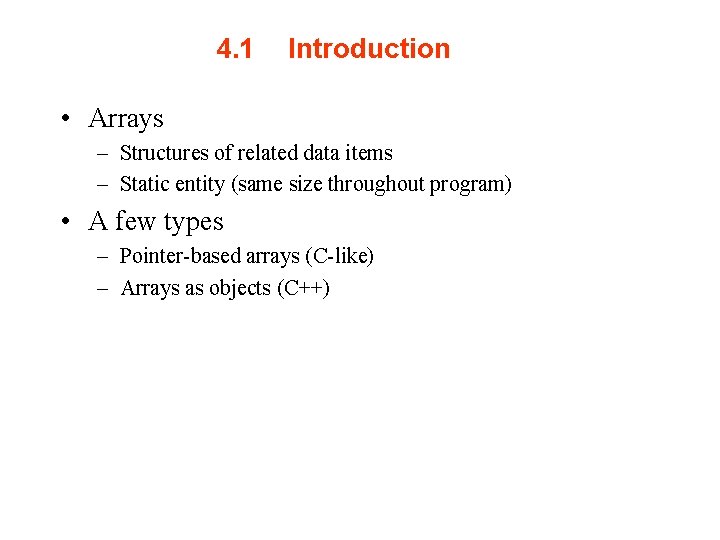
4. 1 Introduction • Arrays – Structures of related data items – Static entity (same size throughout program) • A few types – Pointer-based arrays (C-like) – Arrays as objects (C++)
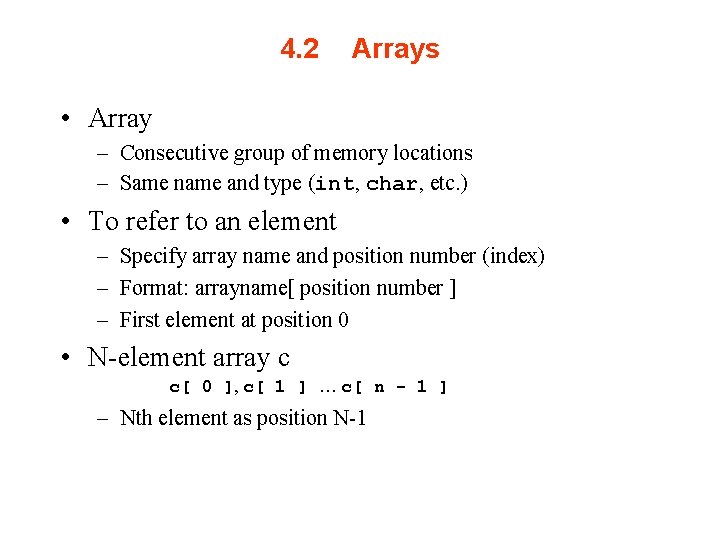
4. 2 Arrays • Array – Consecutive group of memory locations – Same name and type (int, char, etc. ) • To refer to an element – Specify array name and position number (index) – Format: arrayname[ position number ] – First element at position 0 • N-element array c c[ 0 ], c[ 1 ] … c[ n - 1 ] – Nth element as position N-1
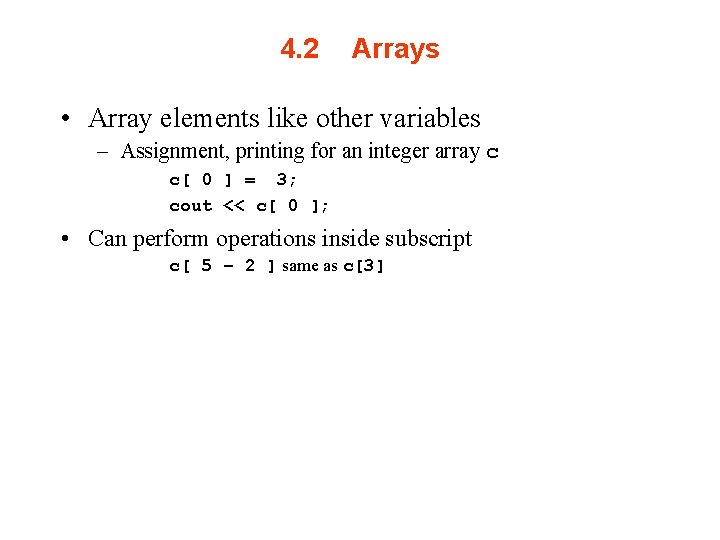
4. 2 Arrays • Array elements like other variables – Assignment, printing for an integer array c c[ 0 ] = 3; cout << c[ 0 ]; • Can perform operations inside subscript c[ 5 – 2 ] same as c[3]
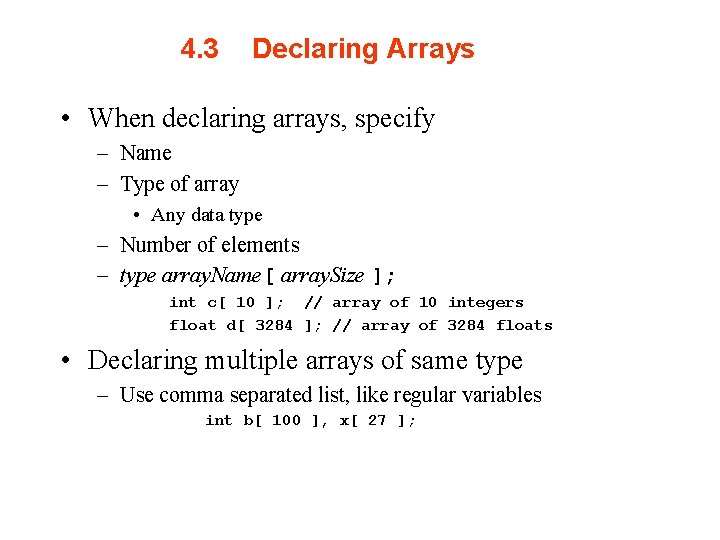
4. 3 Declaring Arrays • When declaring arrays, specify – Name – Type of array • Any data type – Number of elements – type array. Name[ array. Size ]; int c[ 10 ]; // array of 10 integers float d[ 3284 ]; // array of 3284 floats • Declaring multiple arrays of same type – Use comma separated list, like regular variables int b[ 100 ], x[ 27 ];
![4 2 array name c is a pointer variable c address of c0 4. 2 array name c is a (pointer) variable c = address of c[0]](https://slidetodoc.com/presentation_image_h2/5a69a87d8e850132eb89c46f1e908c36/image-5.jpg)
4. 2 array name c is a (pointer) variable c = address of c[0] So, address of c[i] =c+i (in fact, c + i * 4) Name that this same Arrays of array (Note all elements of array have the name, c) c[0] -45 c[1] 6 c[2] 0 c[3] 72 c[4] 1543 c[5] -89 c[6] 0 c[7] 62 c[8] -3 c[9] 1 c[10] 6453 c[11] 78 Position number of the element within array c
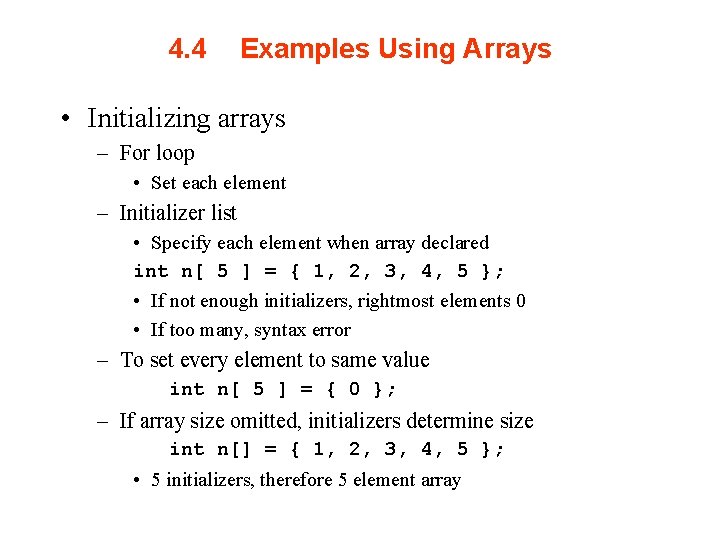
4. 4 Examples Using Arrays • Initializing arrays – For loop • Set each element – Initializer list • Specify each element when array declared int n[ 5 ] = { 1, 2, 3, 4, 5 }; • If not enough initializers, rightmost elements 0 • If too many, syntax error – To set every element to same value int n[ 5 ] = { 0 }; – If array size omitted, initializers determine size int n[] = { 1, 2, 3, 4, 5 }; • 5 initializers, therefore 5 element array
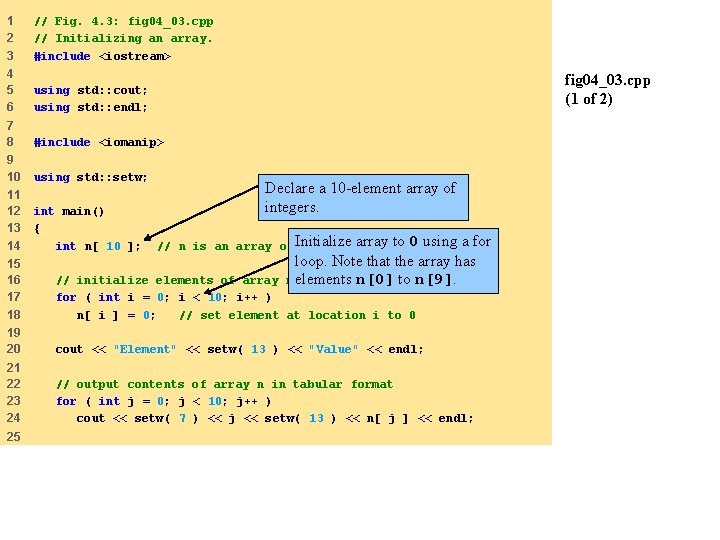
1 2 3 // Fig. 4. 3: fig 04_03. cpp // Initializing an array. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 #include <iomanip> 9 10 using std: : setw; 11 12 13 14 int main() { int n[ 10 ]; fig 04_03. cpp (1 of 2) Declare a 10 -element array of integers. array // n is an array of Initialize 10 integers to 0 using a for loop. Note that the array has n[0] to n[9]. n elements to 0 15 16 17 18 // initialize elements of array for ( int i = 0; i < 10; i++ ) n[ i ] = 0; // set element at location i to 0 19 20 cout << "Element" << setw( 13 ) << "Value" << endl; 21 22 23 24 // output contents of array n in tabular format for ( int j = 0; j < 10; j++ ) cout << setw( 7 ) << j << setw( 13 ) << n[ j ] << endl; 25
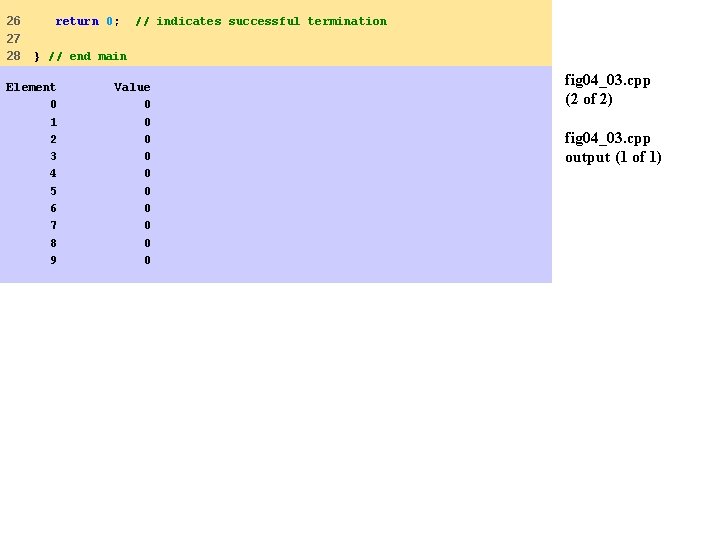
26 27 28 return 0; // indicates successful termination } // end main Element 0 1 2 3 4 5 6 7 8 9 Value 0 0 0 0 0 fig 04_03. cpp (2 of 2) fig 04_03. cpp output (1 of 1)
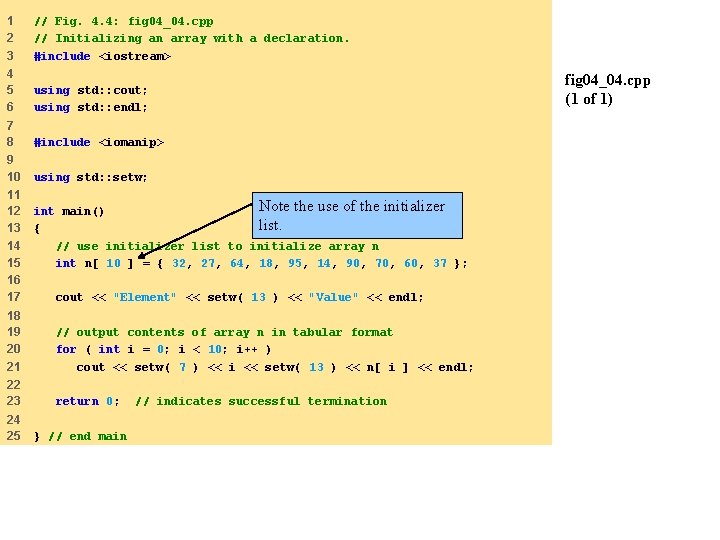
1 2 3 // Fig. 4. 4: fig 04_04. cpp // Initializing an array with a declaration. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 #include <iomanip> 9 10 using std: : setw; 11 12 13 14 15 16 17 Note the use of the initializer int main() list. { // use initializer list to initialize array n int n[ 10 ] = { 32, 27, 64, 18, 95, 14, 90, 70, 60, 37 }; cout << "Element" << setw( 13 ) << "Value" << endl; 18 19 20 21 // output contents of array n in tabular format for ( int i = 0; i < 10; i++ ) cout << setw( 7 ) << i << setw( 13 ) << n[ i ] << endl; 22 23 return 0; 24 25 } // end main // indicates successful termination fig 04_04. cpp (1 of 1)
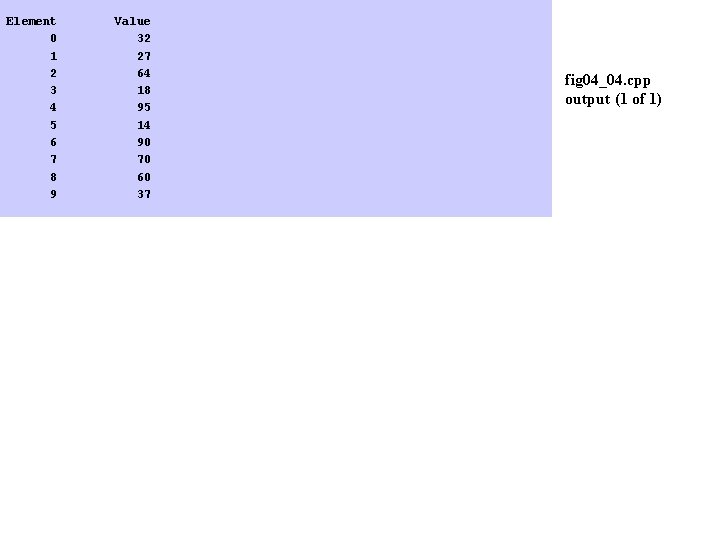
Element 0 1 2 3 4 5 6 7 8 9 Value 32 27 64 18 95 14 90 70 60 37 fig 04_04. cpp output (1 of 1)
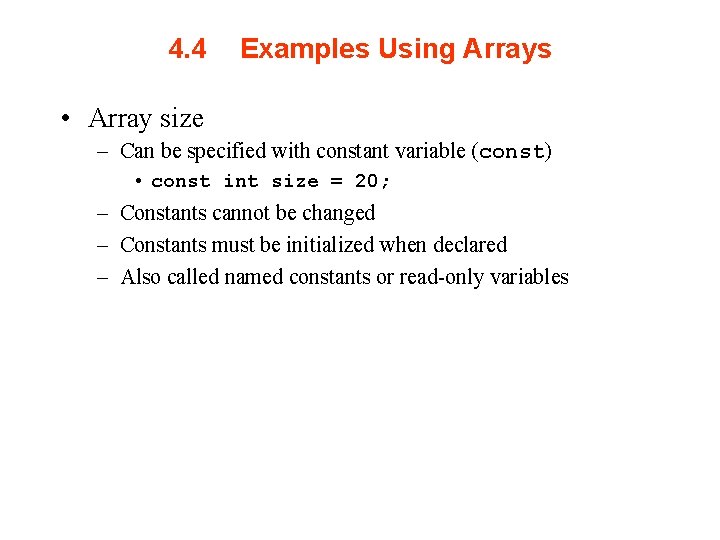
4. 4 Examples Using Arrays • Array size – Can be specified with constant variable (const) • const int size = 20; – Constants cannot be changed – Constants must be initialized when declared – Also called named constants or read-only variables
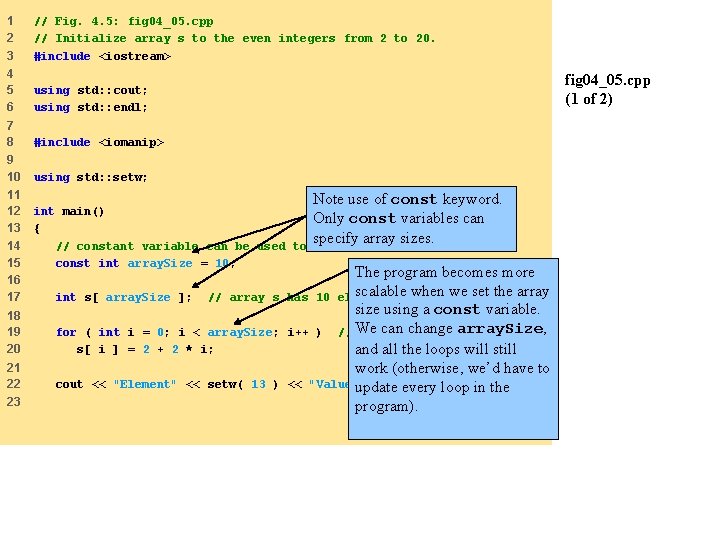
1 2 3 // Fig. 4. 5: fig 04_05. cpp // Initialize array s to the even integers from 2 to 20. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 #include <iomanip> 9 10 using std: : setw; 11 12 13 14 15 16 17 fig 04_05. cpp (1 of 2) Note use of const keyword. can int main() Only const variables { specify array sizes. // constant variable can be used to specify array size const int array. Size = 10; int s[ array. Size ]; // array s 18 19 20 for ( int i = 0; i < array. Size; s[ i ] = 2 + 2 * i; 21 22 cout << "Element" << setw( 13 ) 23 The program becomes more scalable when we set the array has 10 elements size using a const variable. i++ ) // We setcan thechange valuesarray. Size, and all the loops will still work (otherwise, we’d have to << "Value"update << endl; every loop in the program).
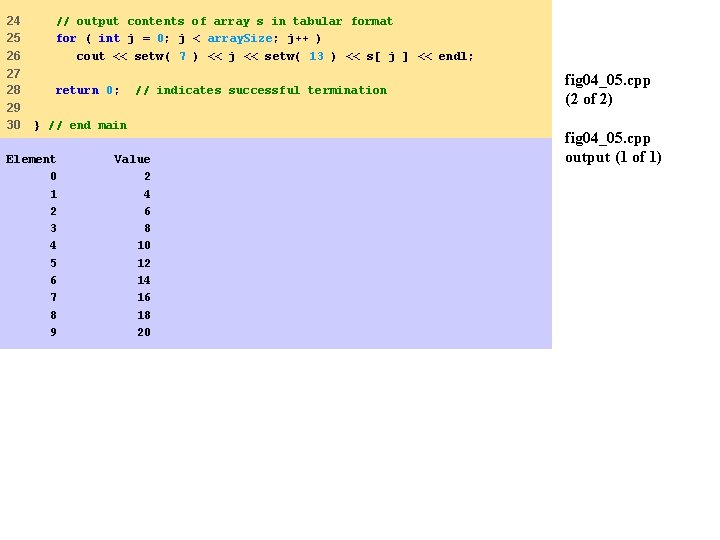
24 25 26 // output contents of array s in tabular format for ( int j = 0; j < array. Size; j++ ) cout << setw( 7 ) << j << setw( 13 ) << s[ j ] << endl; 27 28 return 0; 29 30 // indicates successful termination } // end main Element 0 1 2 3 4 5 6 7 8 9 Value 2 4 6 8 10 12 14 16 18 20 fig 04_05. cpp (2 of 2) fig 04_05. cpp output (1 of 1)
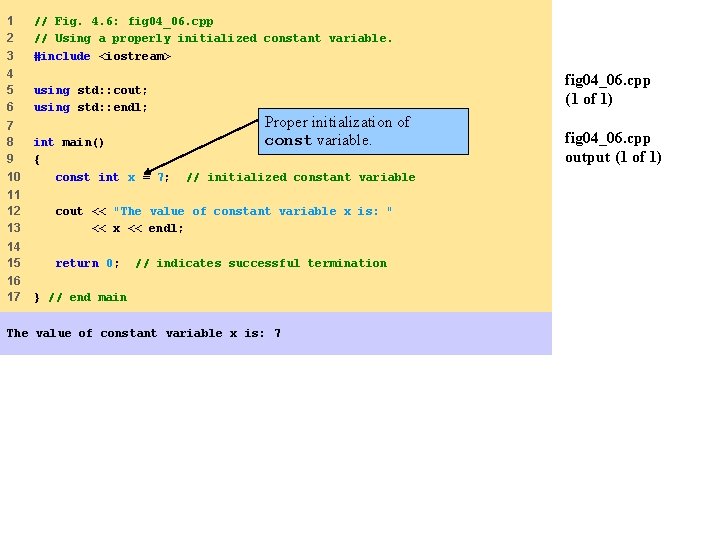
1 2 3 // Fig. 4. 6: fig 04_06. cpp // Using a properly initialized constant variable. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 int main() { const int x = 7; fig 04_06. cpp (1 of 1) Proper initialization of const variable. // initialized constant variable 11 12 13 cout << "The value of constant variable x is: " << x << endl; 14 15 return 0; 16 17 // indicates successful termination } // end main The value of constant variable x is: 7 fig 04_06. cpp output (1 of 1)
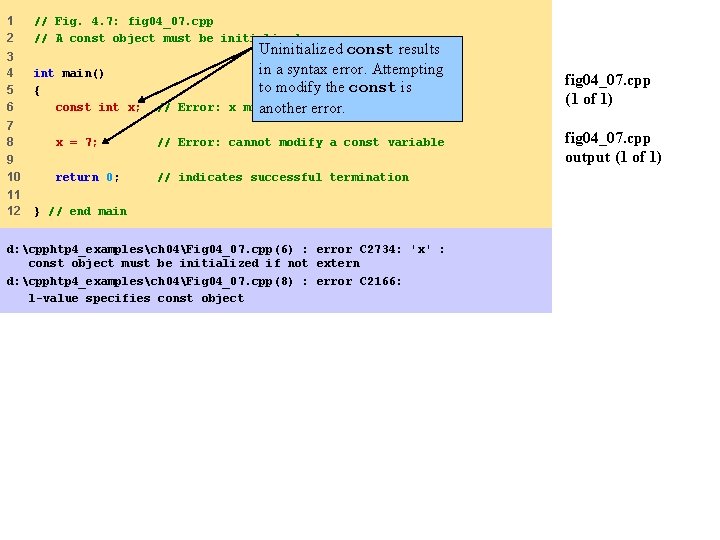
1 2 // Fig. 4. 7: fig 04_07. cpp // A const object must be initialized. 3 4 5 6 int main() { const int x; // Error: x Uninitialized const results in a syntax error. Attempting to modify the const is must be initialized another error. 7 8 x = 7; // Error: cannot modify a const variable 9 10 return 0; // indicates successful termination 11 12 } // end main d: cpphtp 4_examplesch 04Fig 04_07. cpp(6) : error C 2734: 'x' : const object must be initialized if not extern d: cpphtp 4_examplesch 04Fig 04_07. cpp(8) : error C 2166: l-value specifies const object fig 04_07. cpp (1 of 1) fig 04_07. cpp output (1 of 1)
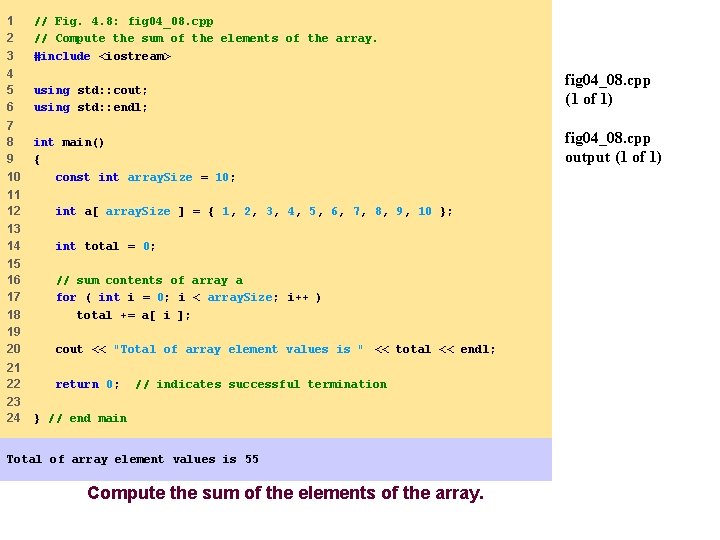
1 2 3 // Fig. 4. 8: fig 04_08. cpp // Compute the sum of the elements of the array. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 int main() { const int array. Size = 10; 11 12 int a[ array. Size ] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; 13 14 int total = 0; 15 16 17 18 19 20 21 22 23 24 // sum contents of array a for ( int i = 0; i < array. Size; i++ ) total += a[ i ]; cout << "Total of array element values is " << total << endl; return 0; // indicates successful termination } // end main Total of array element values is 55 Compute the sum of the elements of the array. fig 04_08. cpp (1 of 1) fig 04_08. cpp output (1 of 1)
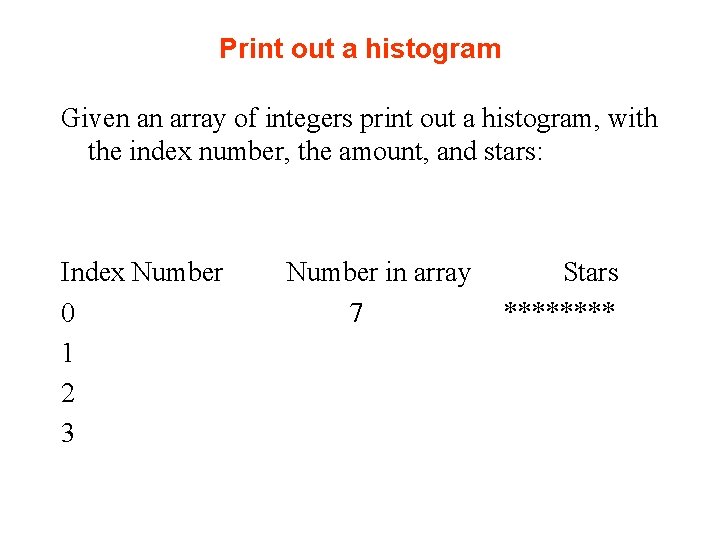
Print out a histogram Given an array of integers print out a histogram, with the index number, the amount, and stars: Index Number 0 1 2 3 Number in array 7 Stars ****
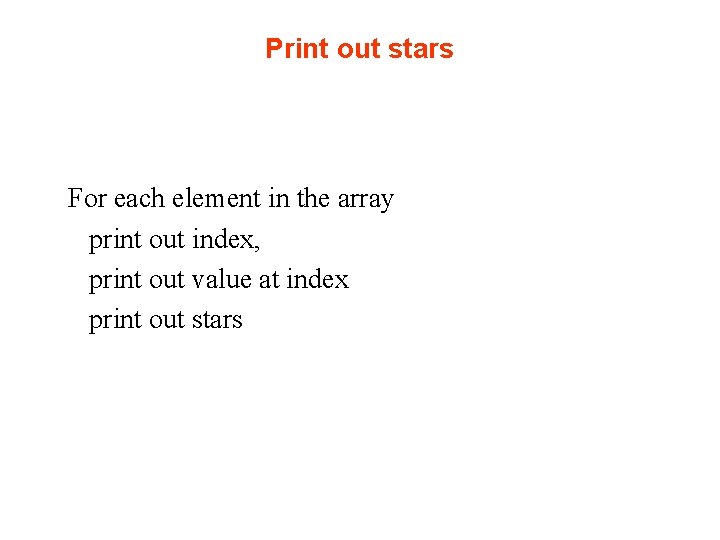
Print out stars For each element in the array print out index, print out value at index print out stars
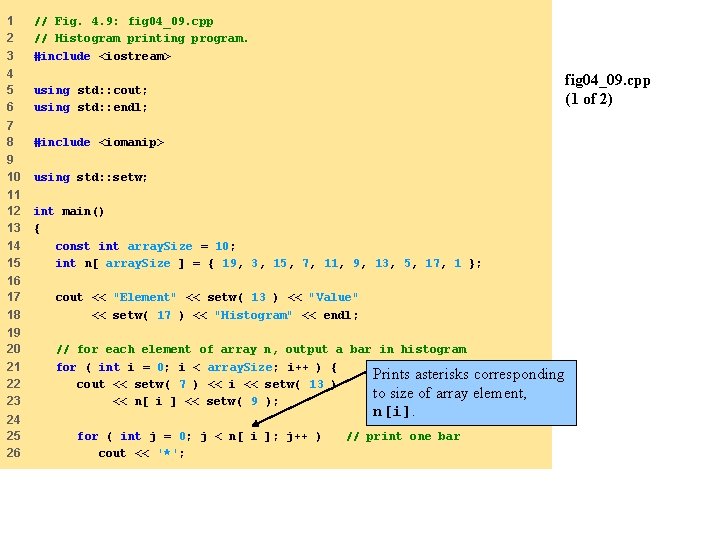
1 2 3 // Fig. 4. 9: fig 04_09. cpp // Histogram printing program. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 #include <iomanip> 9 10 using std: : setw; 11 12 13 14 15 int main() { const int array. Size = 10; int n[ array. Size ] = { 19, 3, 15, 7, 11, 9, 13, 5, 17, 1 }; fig 04_09. cpp (1 of 2) 16 17 18 cout << "Element" << setw( 13 ) << "Value" << setw( 17 ) << "Histogram" << endl; 19 20 21 22 23 // for each element of array n, output a bar in histogram for ( int i = 0; i < array. Size; i++ ) { Prints asterisks corresponding cout << setw( 7 ) << i << setw( 13 ) to size of array element, << n[ i ] << setw( 9 ); 24 25 26 n[i]. for ( int j = 0; j < n[ i ]; j++ ) cout << '*'; // print one bar
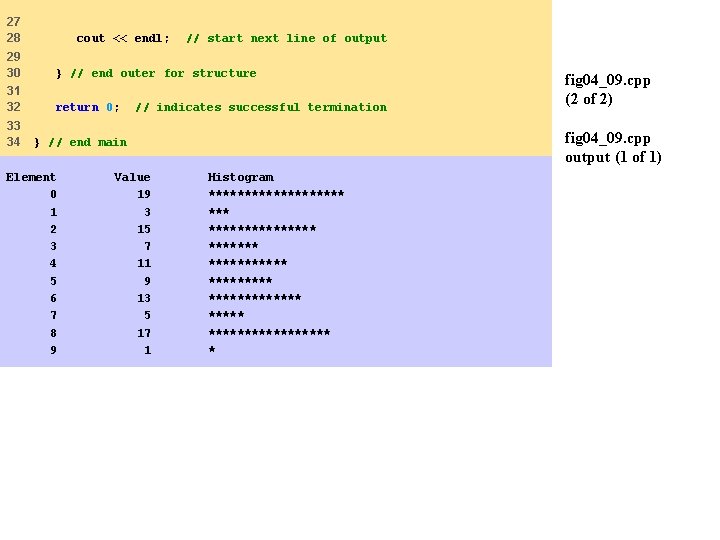
27 28 cout << endl; // start next line of output 29 30 } // end outer for structure 31 32 return 0; 33 34 // indicates successful termination fig 04_09. cpp output (1 of 1) } // end main Element 0 1 2 3 4 5 6 7 8 9 Value 19 3 15 7 11 9 13 5 17 1 fig 04_09. cpp (2 of 2) Histogram ********** *********** *********** *
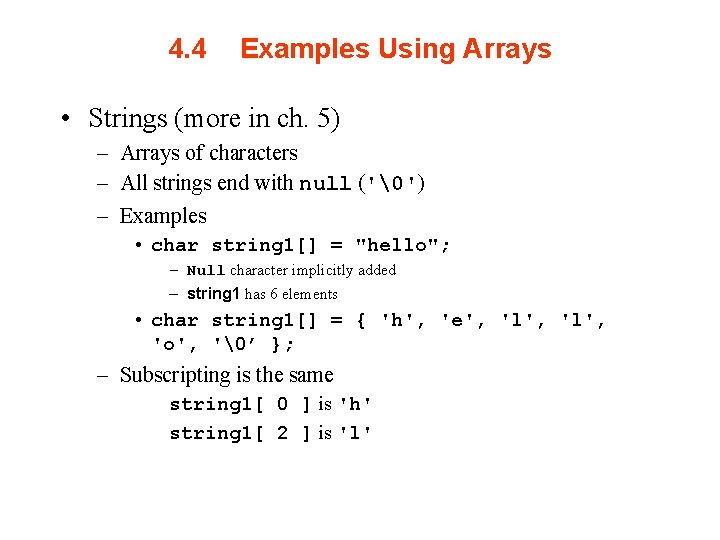
4. 4 Examples Using Arrays • Strings (more in ch. 5) – Arrays of characters – All strings end with null ('