3 File Handling Introduction Sample program File Descriptor
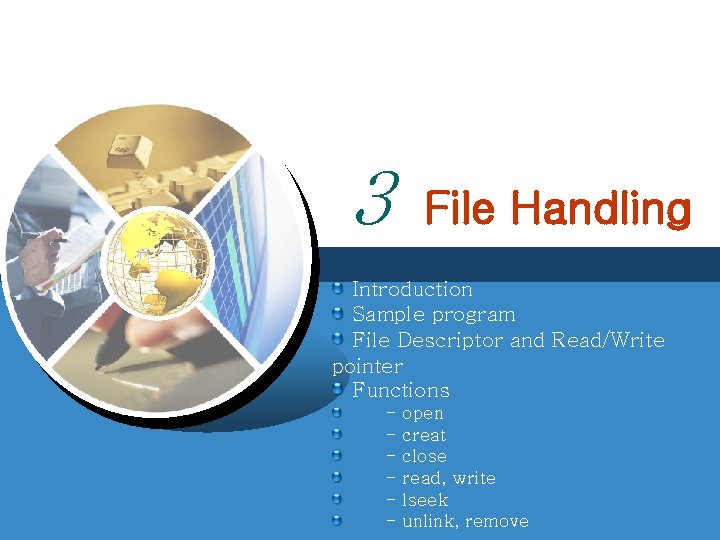
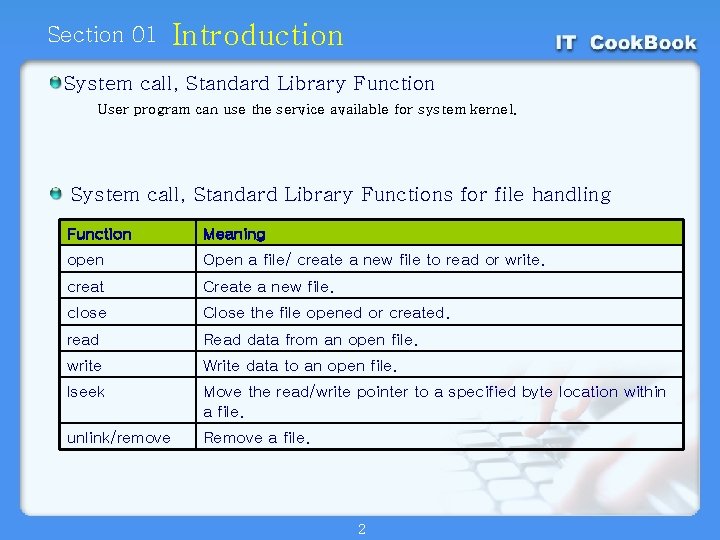
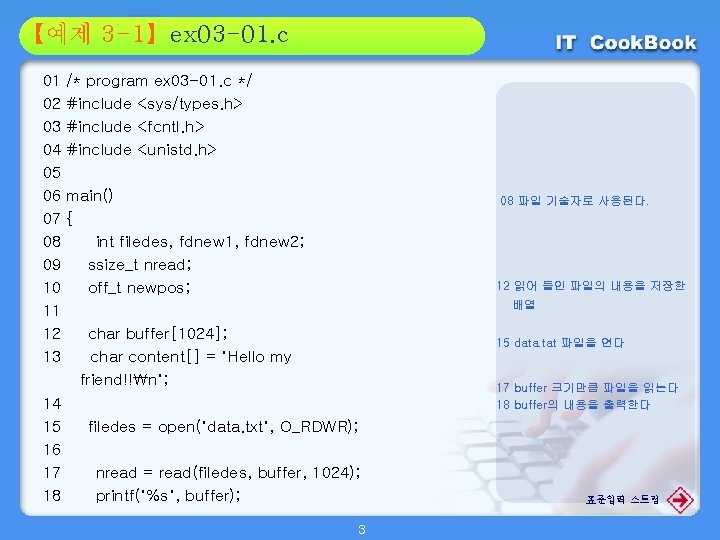
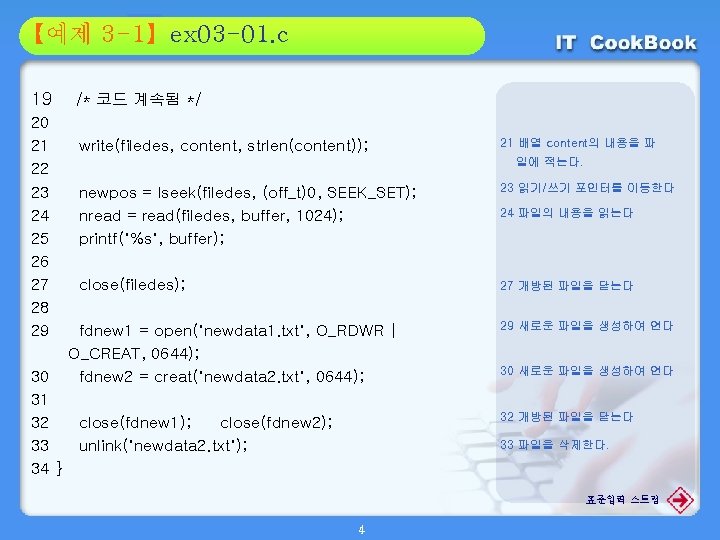
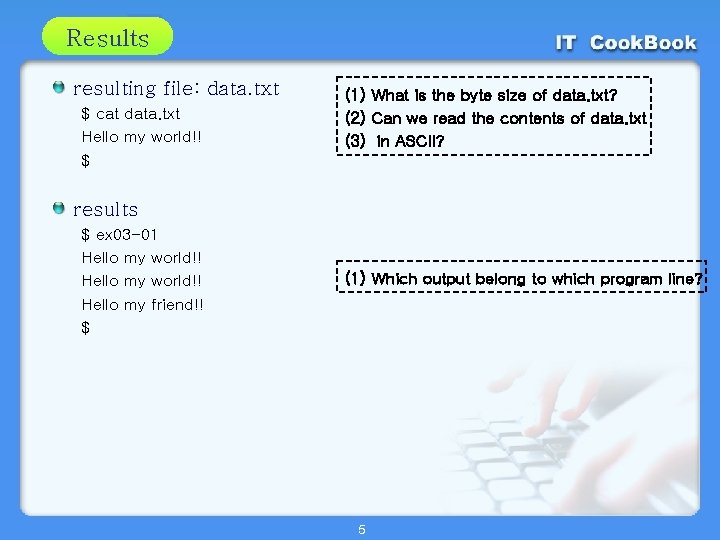
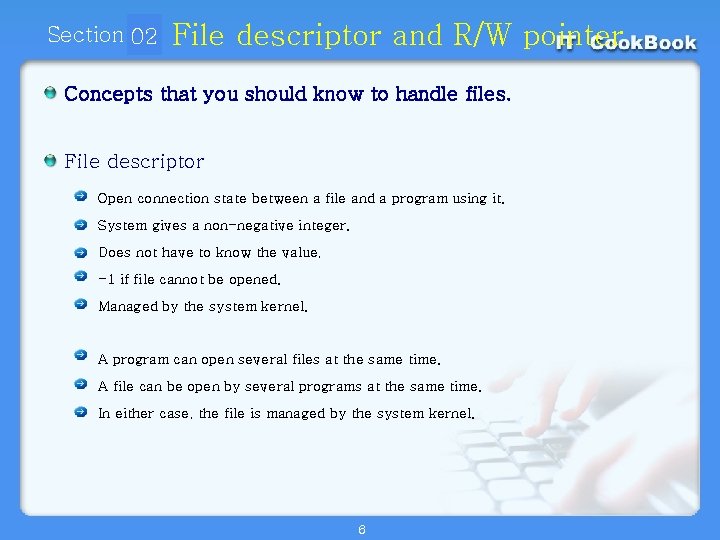
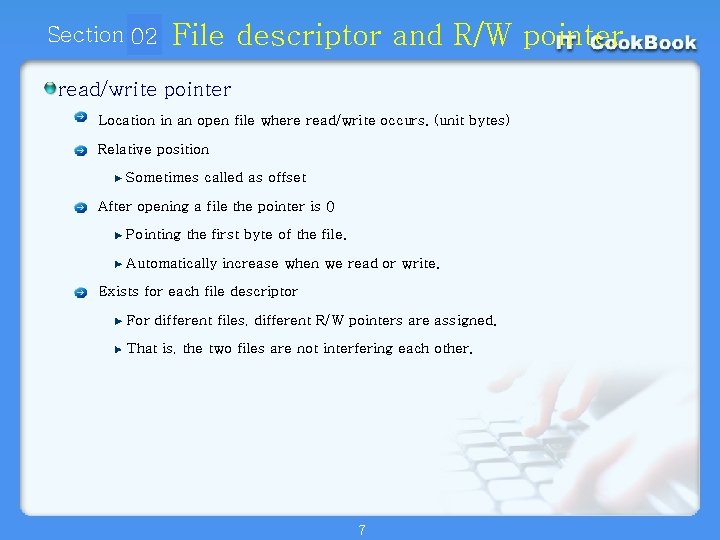
![Section 02 01 File descriptor and R/W pointer [Fig 3 -1] File descriptors and Section 02 01 File descriptor and R/W pointer [Fig 3 -1] File descriptors and](https://slidetodoc.com/presentation_image/4d59ee3ced0ec77e34f4329780c122be/image-8.jpg)
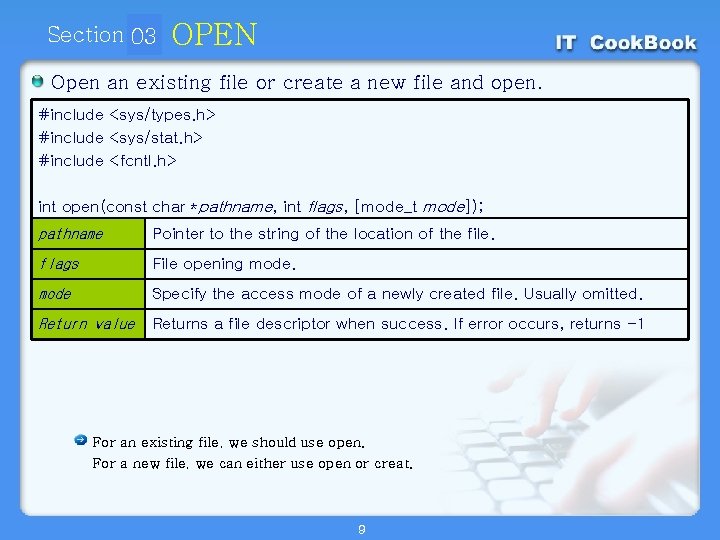
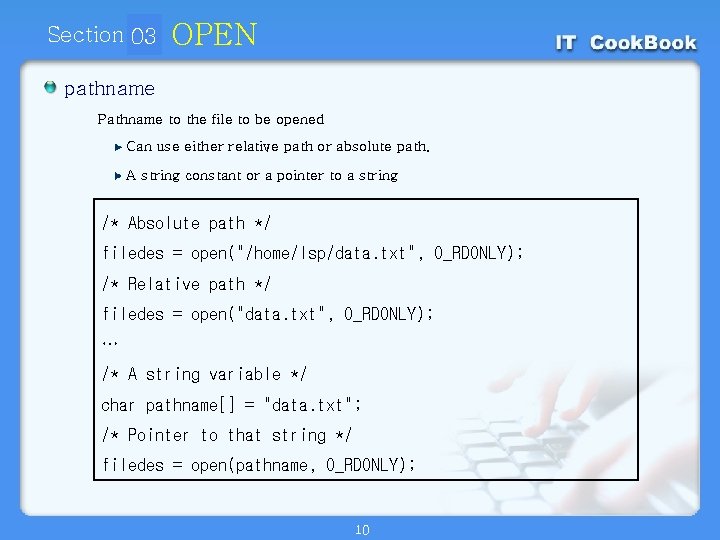
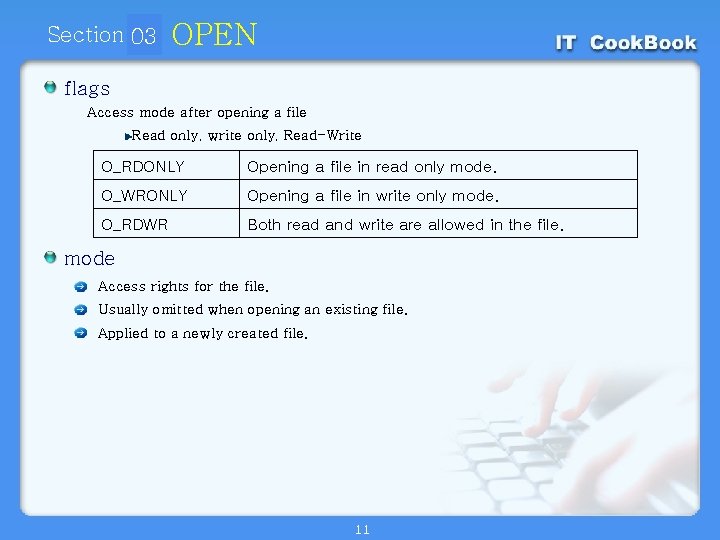
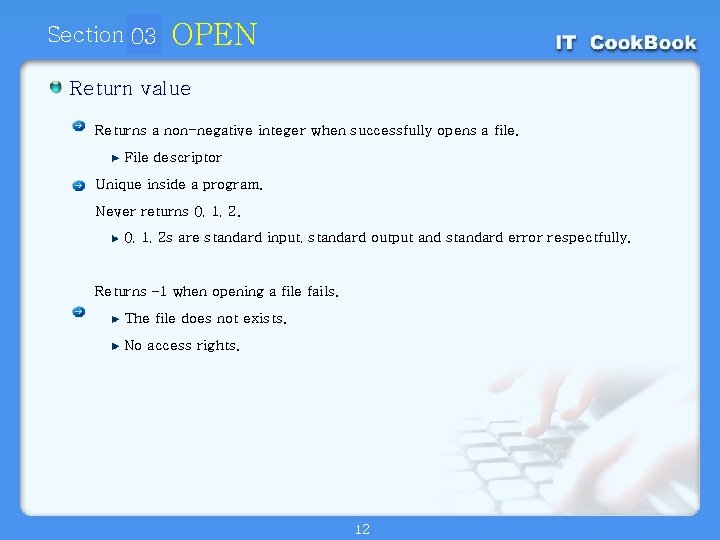
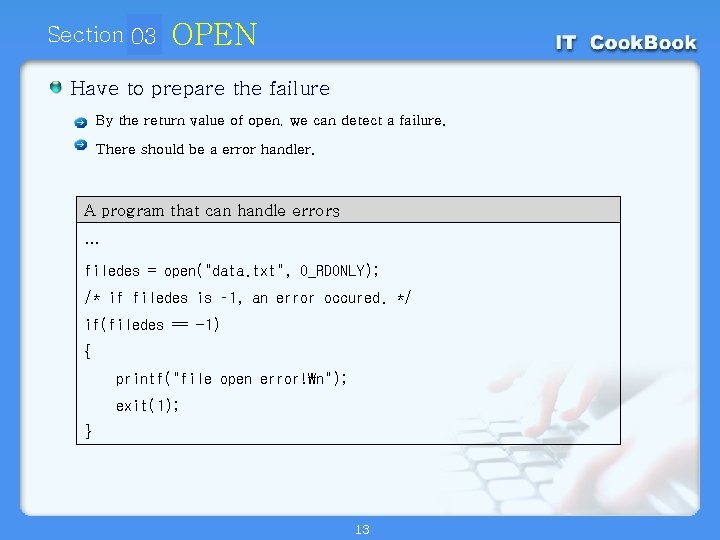
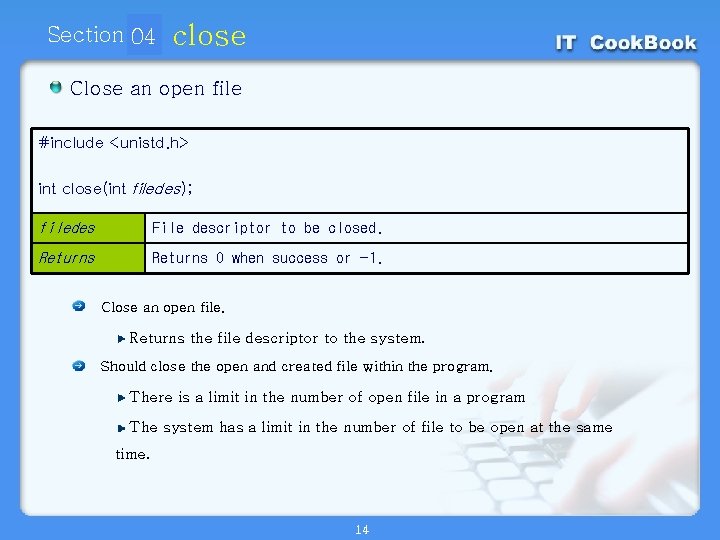
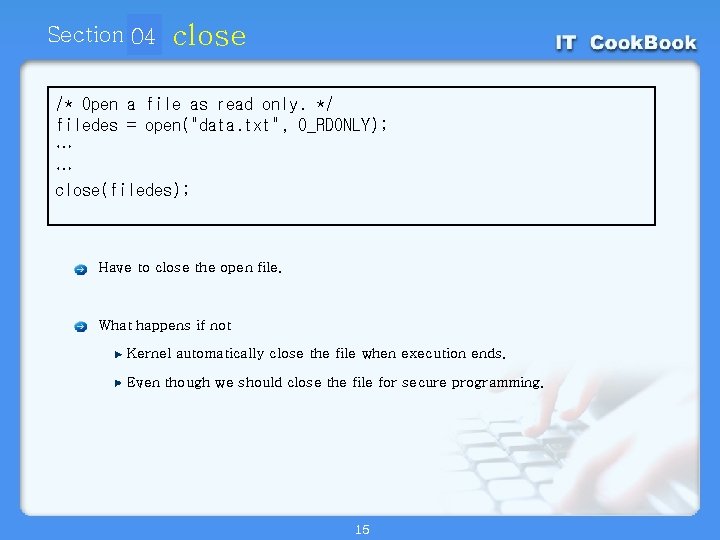
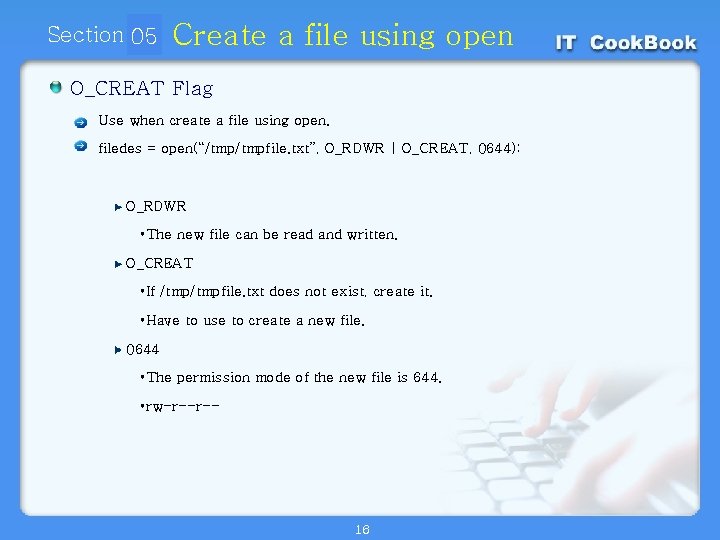
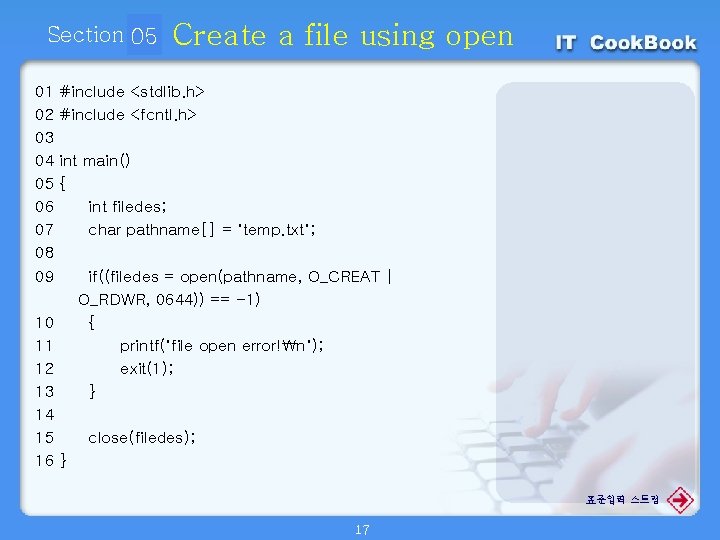
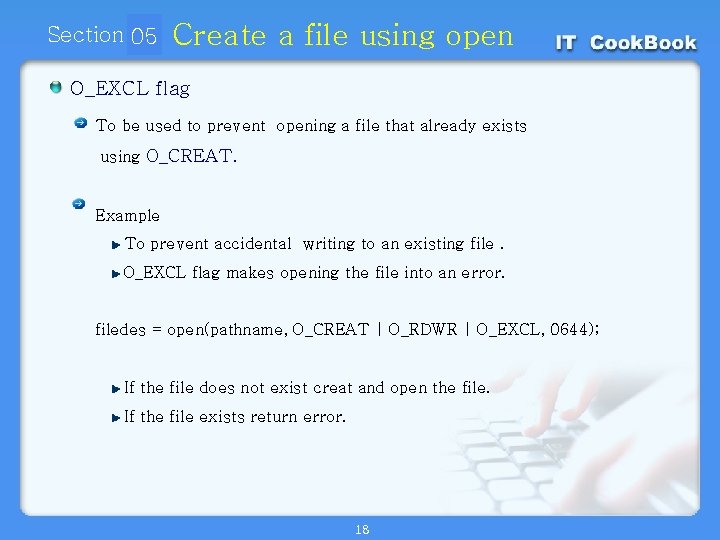
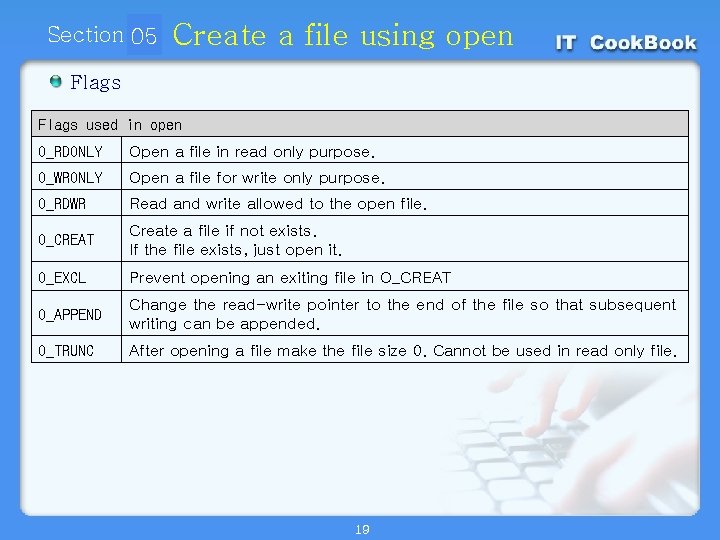
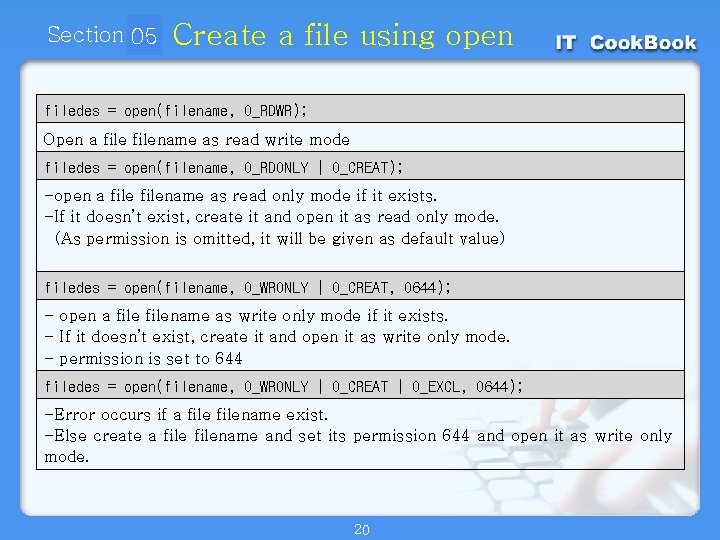
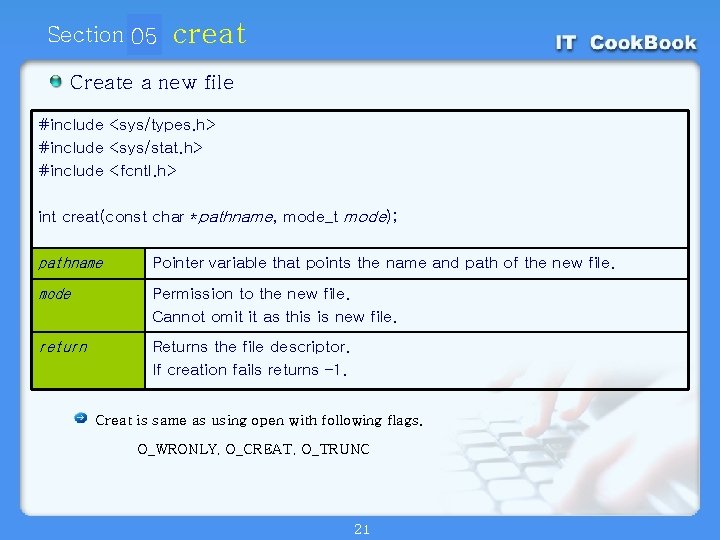
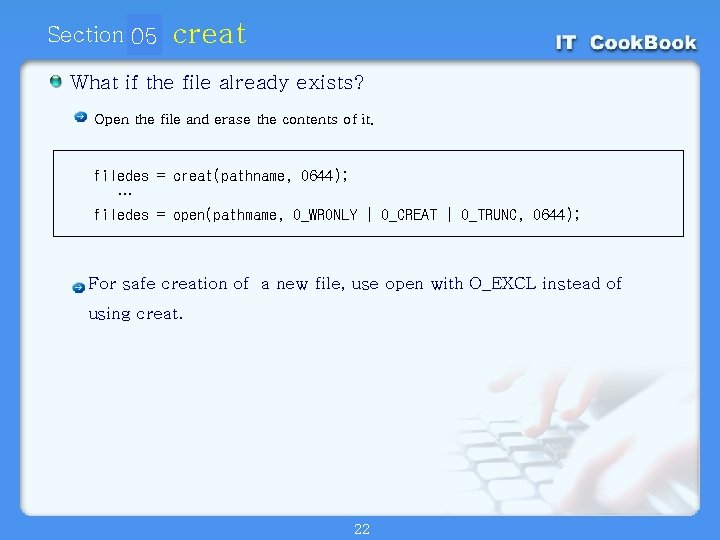
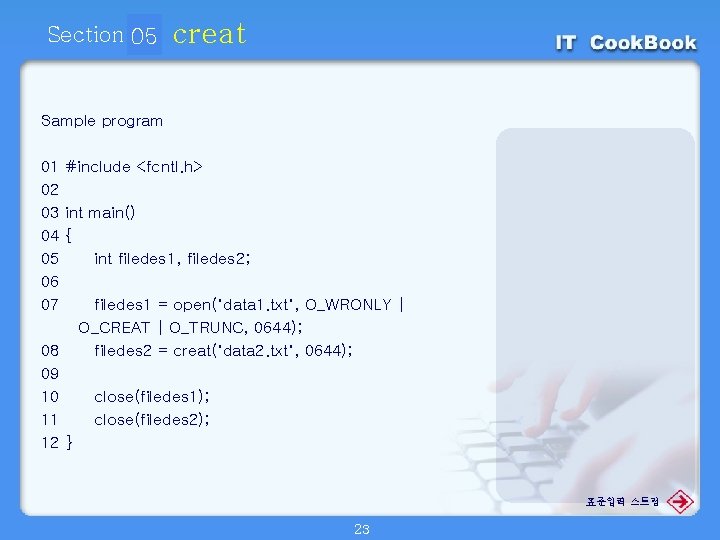
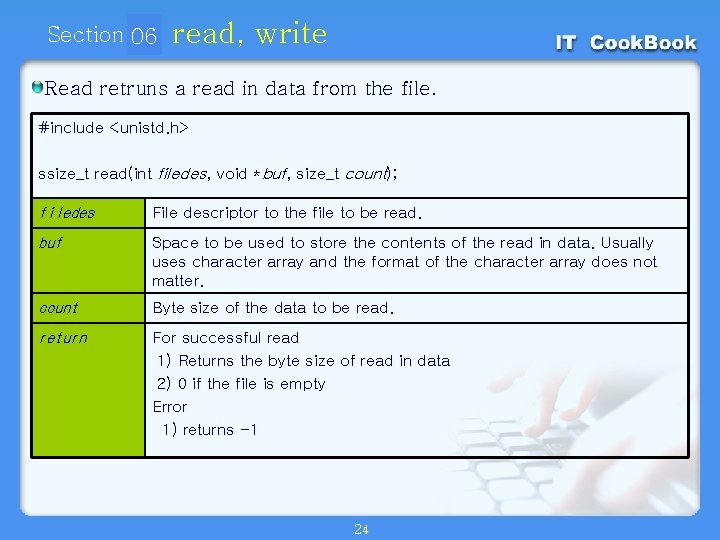
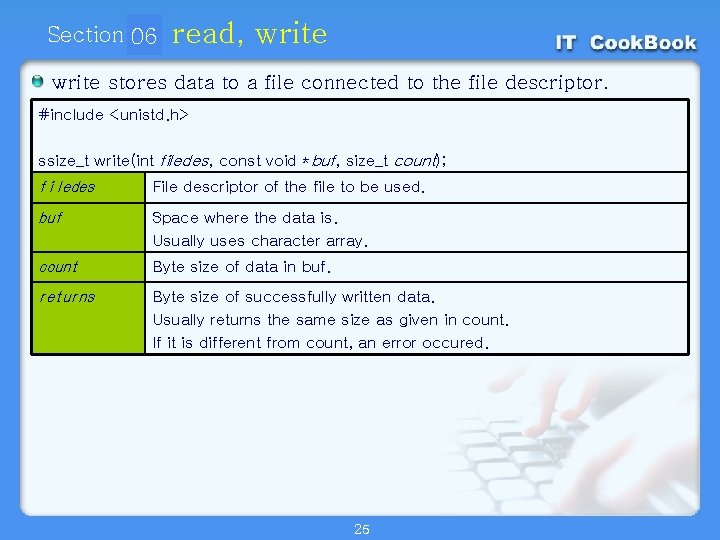
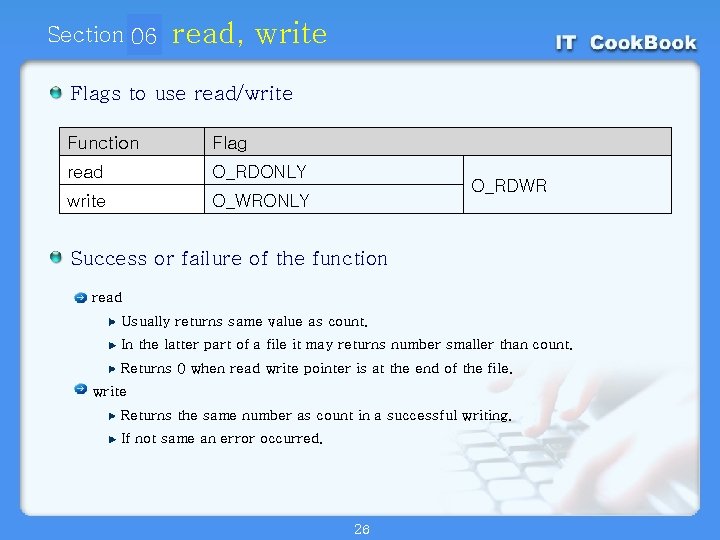
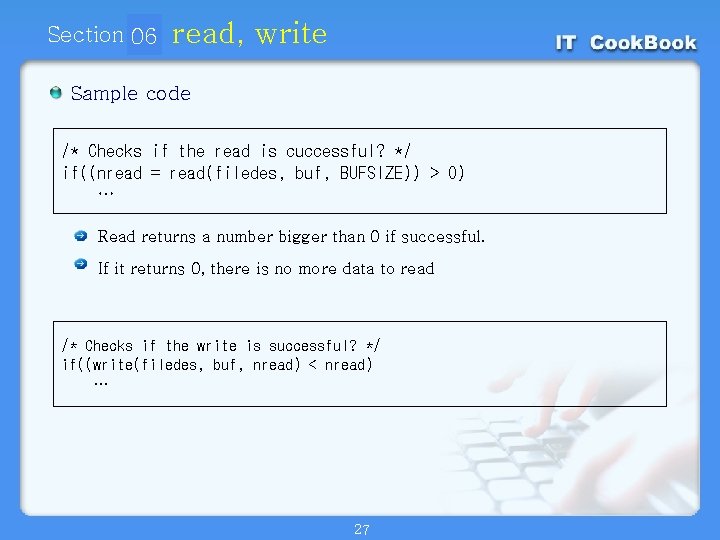
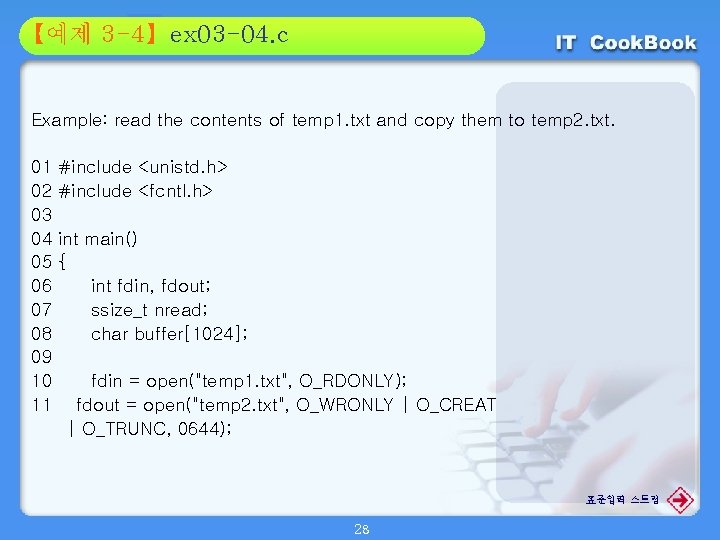
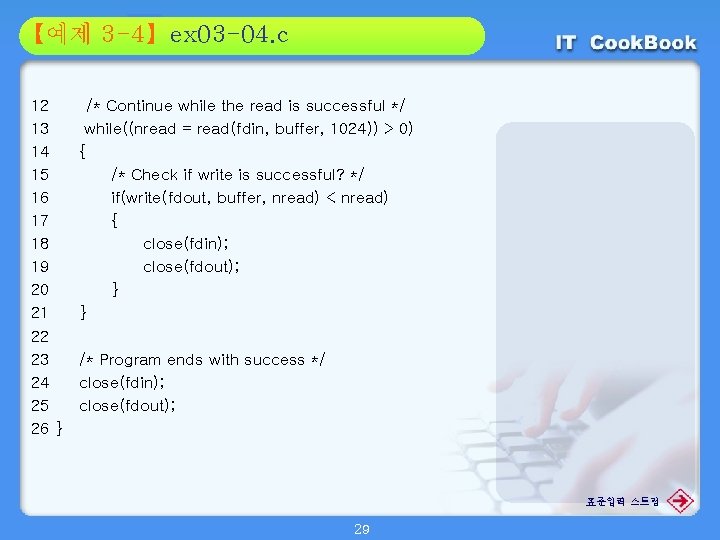
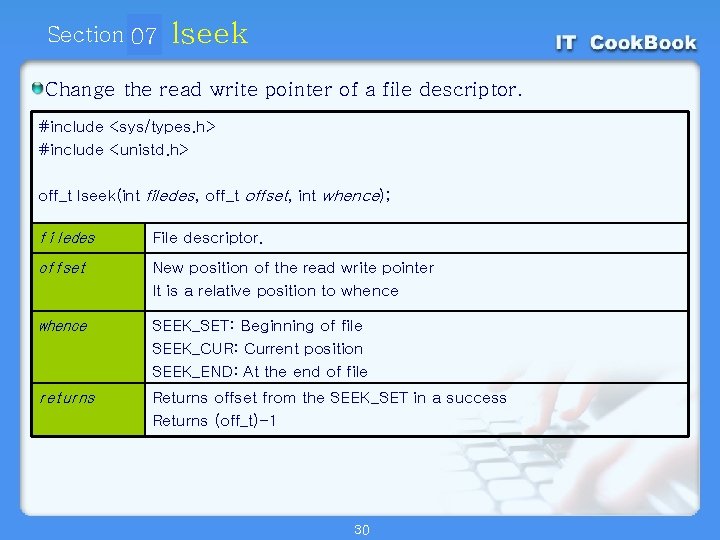
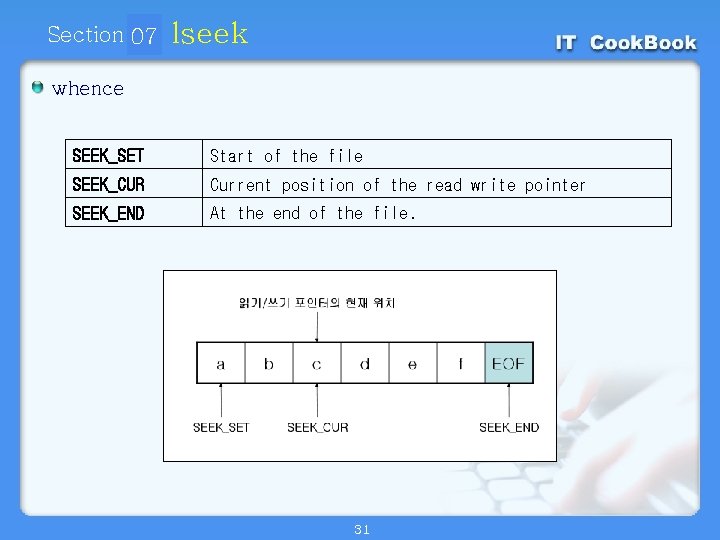
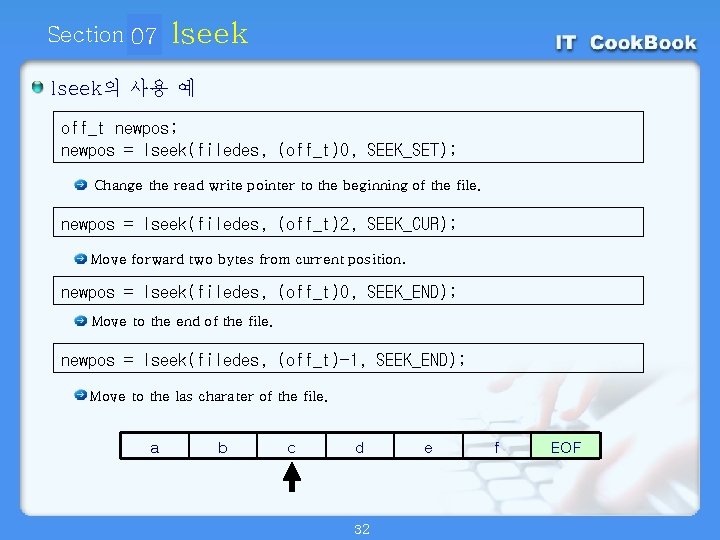
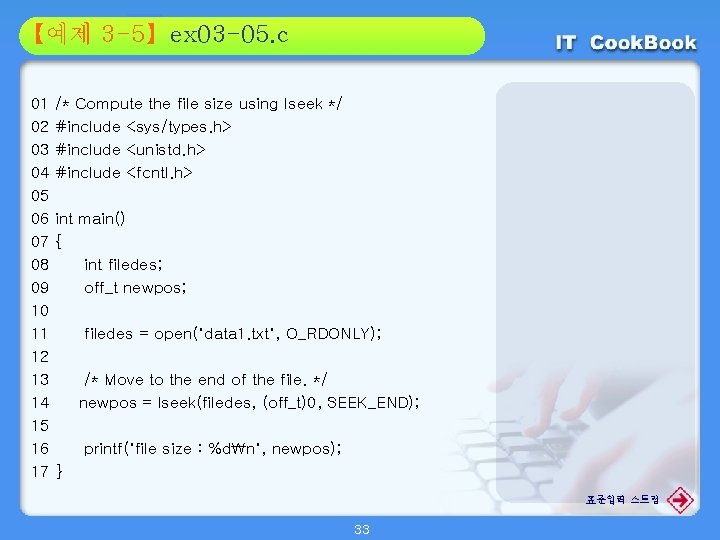
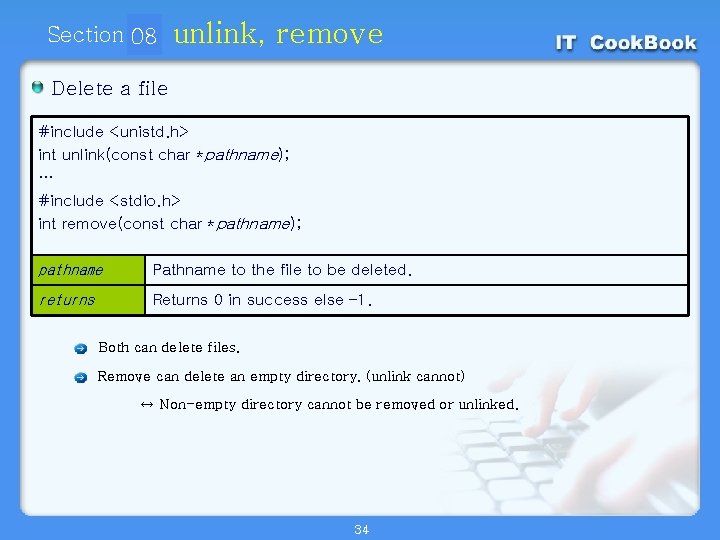
- Slides: 34
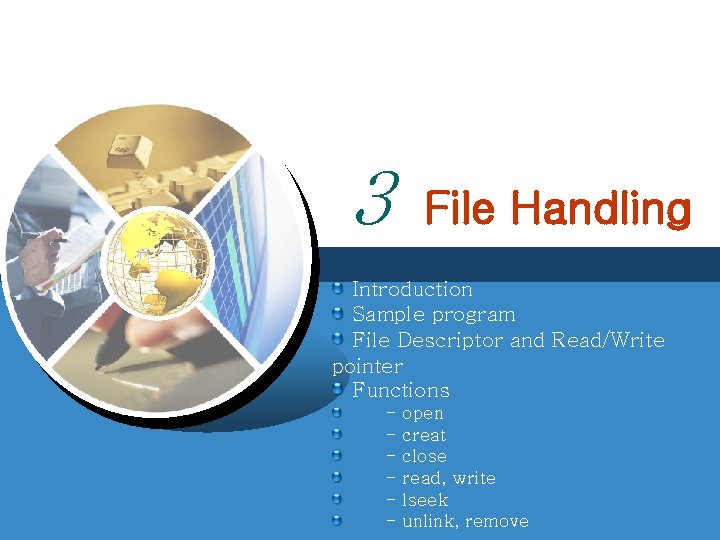
3 File Handling Introduction Sample program File Descriptor and Read/Write pointer Functions - open creat close read, write lseek unlink, remove
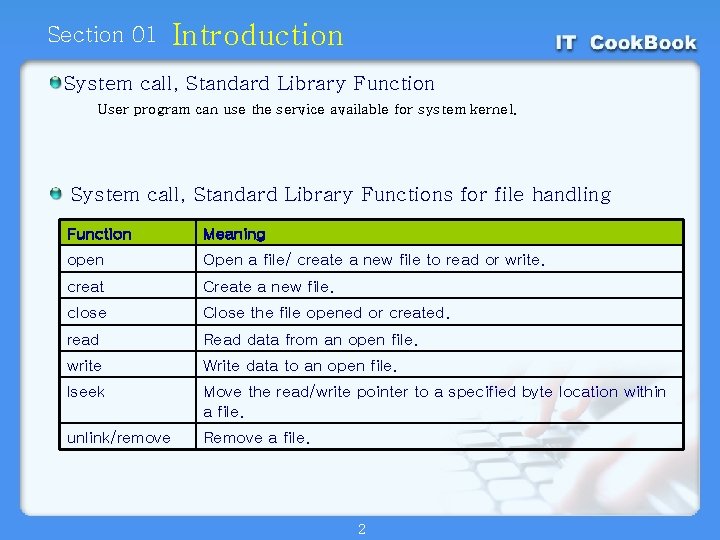
Section 01 Introduction System call, Standard Library Function User program can use the service available for system kernel. System call, Standard Library Functions for file handling Function Meaning open Open a file/ create a new file to read or write. creat Create a new file. close Close the file opened or created. read Read data from an open file. write Write data to an open file. lseek Move the read/write pointer to a specified byte location within a file. unlink/remove Remove a file. 2
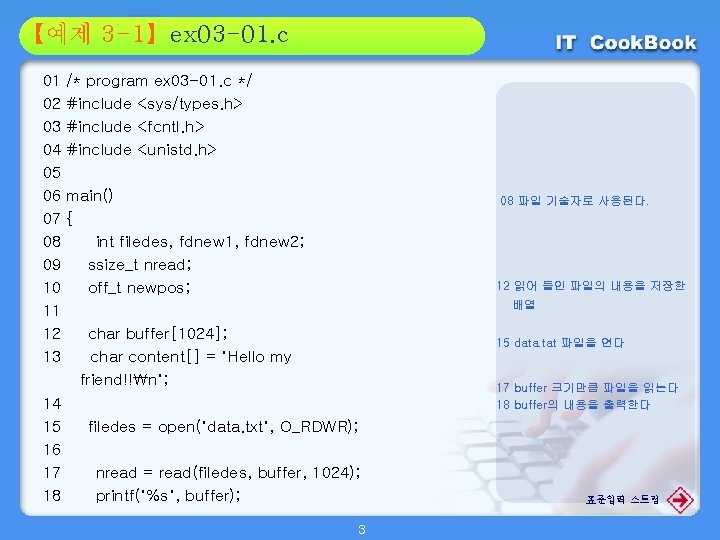
Section 01 【예제 3 -1】ex 03 -01. c 02 01 /* program ex 03 -01. c */ 02 #include <sys/types. h> 03 #include <fcntl. h> 04 #include <unistd. h> 05 06 main() 07 { 08 int filedes, fdnew 1, fdnew 2; 09 ssize_t nread; 10 off_t newpos; 11 12 char buffer[1024]; 13 char content[] = "Hello my friend!!n"; 14 15 filedes = open("data. txt", O_RDWR); 16 17 nread = read(filedes, buffer, 1024); 18 printf("%s", buffer); 3 08 파일 기술자로 사용된다. 12 읽어 들인 파일의 내용을 저장한 배열 15 data. tat 파일을 연다 17 buffer 크기만큼 파일을 읽는다 18 buffer의 내용을 출력한다 표준입력 스트림
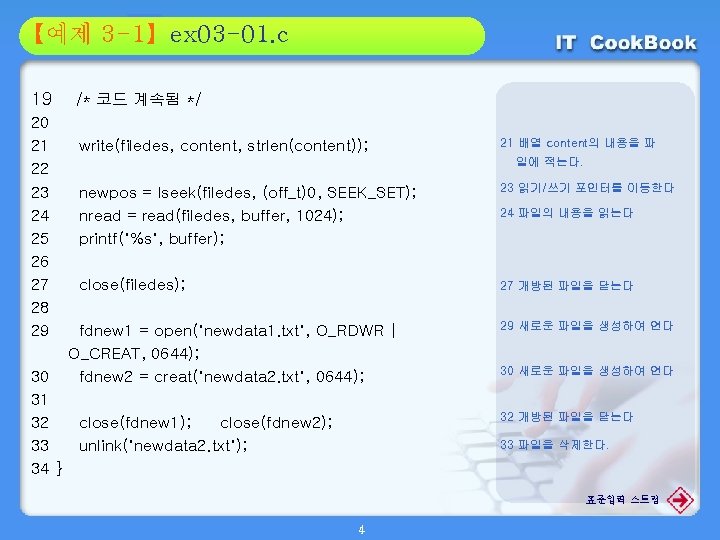
Section 01 【예제 3 -1】ex 03 -01. c 02 19 /* 코드 계속됨 */ 20 21 write(filedes, content, strlen(content)); 22 23 newpos = lseek(filedes, (off_t)0, SEEK_SET); 24 nread = read(filedes, buffer, 1024); 25 printf("%s", buffer); 26 27 close(filedes); 28 29 fdnew 1 = open("newdata 1. txt", O_RDWR | O_CREAT, 0644); 30 fdnew 2 = creat("newdata 2. txt", 0644); 31 32 close(fdnew 1); close(fdnew 2); 33 unlink("newdata 2. txt"); 34 } 21 배열 content의 내용을 파 일에 적는다. 23 읽기/쓰기 포인터를 이동한다 24 파일의 내용을 읽는다 27 개방된 파일을 닫는다 29 새로운 파일을 생성하여 연다 30 새로운 파일을 생성하여 연다 32 개방된 파일을 닫는다 33 파일을 삭제한다. 표준입력 스트림 4
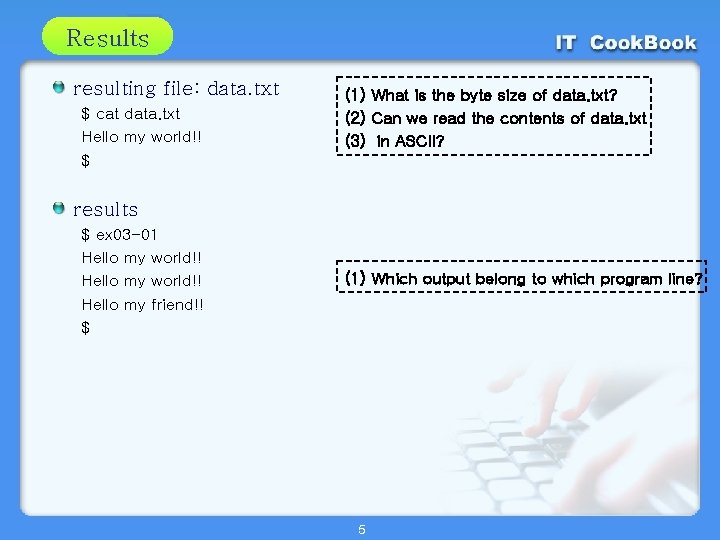
Section 01 08 05 Results resulting file: data. txt $ cat data. txt Hello my world!! $ (1) What is the byte size of data. txt? (2) Can we read the contents of data. txt (3) in ASCII? results $ ex 03 -01 Hello my world!! Hello my friend!! $ (1) Which output belong to which program line? 5
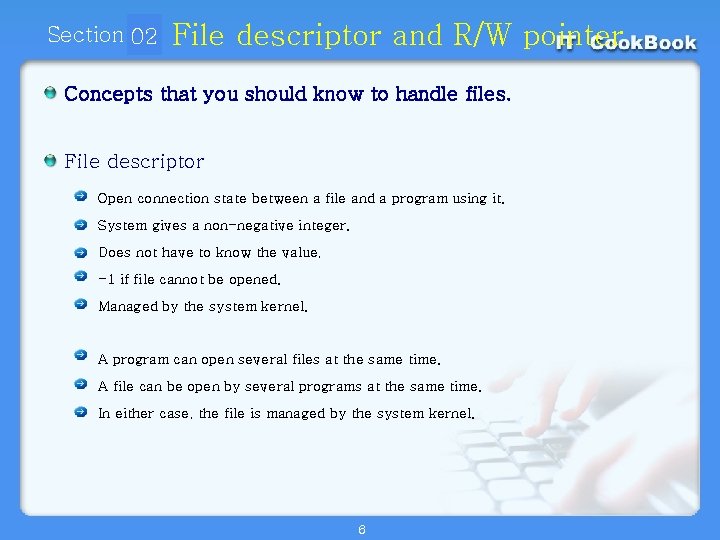
Section 02 01 File descriptor and R/W pointer Concepts that you should know to handle files. File descriptor Open connection state between a file and a program using it. System gives a non-negative integer. Does not have to know the value, -1 if file cannot be opened. Managed by the system kernel. A program can open several files at the same time. A file can be open by several programs at the same time. In either case, the file is managed by the system kernel. 6
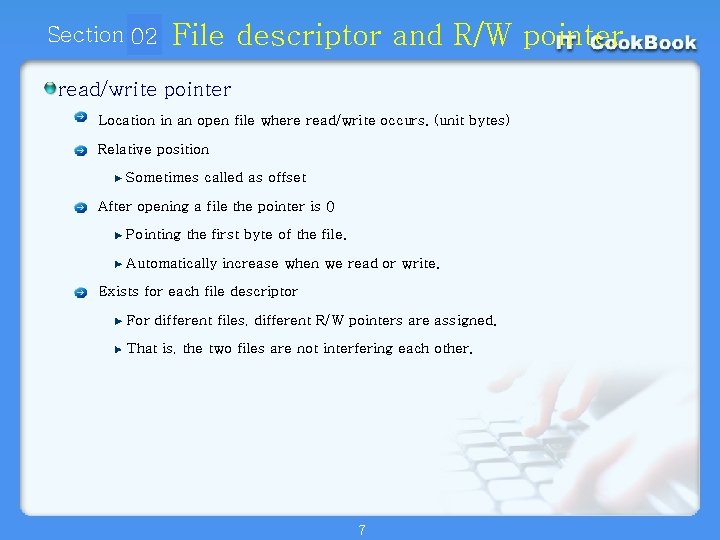
Section 02 01 File descriptor and R/W pointer read/write pointer Location in an open file where read/write occurs. (unit bytes) Relative position Sometimes called as offset After opening a file the pointer is 0 Pointing the first byte of the file. Automatically increase when we read or write. Exists for each file descriptor For different files, different R/W pointers are assigned. That is, the two files are not interfering each other. 7
![Section 02 01 File descriptor and RW pointer Fig 3 1 File descriptors and Section 02 01 File descriptor and R/W pointer [Fig 3 -1] File descriptors and](https://slidetodoc.com/presentation_image/4d59ee3ced0ec77e34f4329780c122be/image-8.jpg)
Section 02 01 File descriptor and R/W pointer [Fig 3 -1] File descriptors and R/W pointers 8
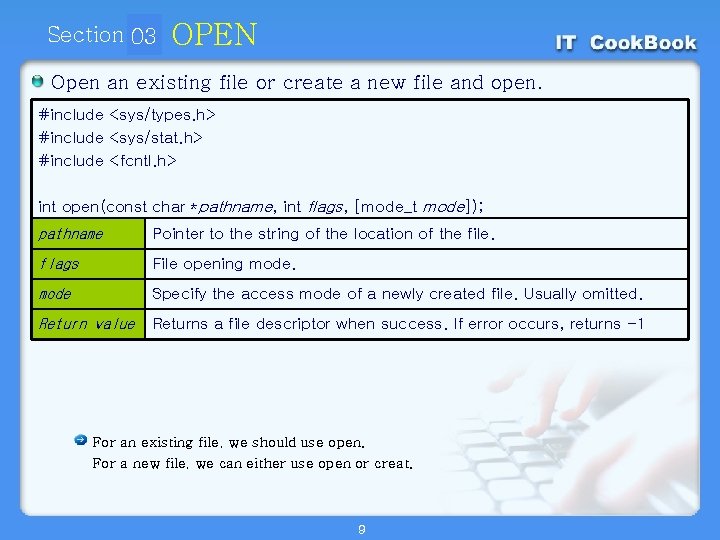
Section 03 01 OPEN Open an existing file or create a new file and open. #include <sys/types. h> #include <sys/stat. h> #include <fcntl. h> int open(const char *pathname, int flags, [mode_t mode]); pathname Pointer to the string of the location of the file. flags File opening mode Specify the access mode of a newly created file. Usually omitted. Return value Returns a file descriptor when success. If error occurs, returns -1 For an existing file, we should use open. For a new file, we can either use open or creat. 9
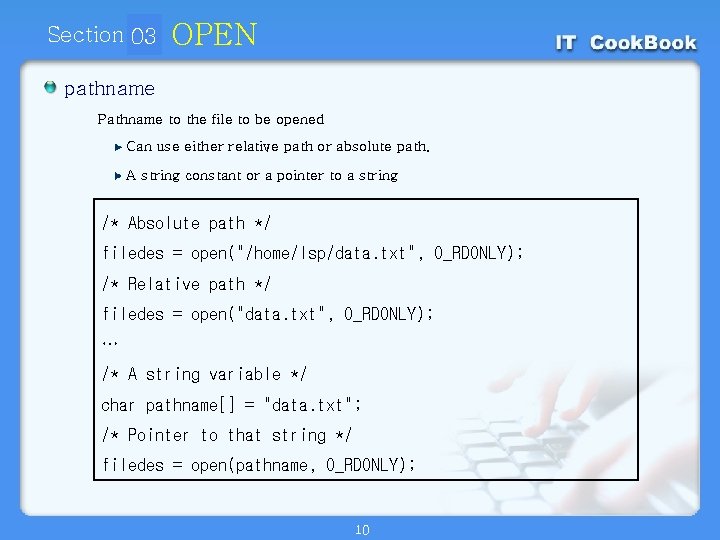
Section 03 01 OPEN pathname Pathname to the file to be opened Can use either relative path or absolute path. A string constant or a pointer to a string /* Absolute path */ filedes = open("/home/lsp/data. txt", O_RDONLY); /* Relative path */ filedes = open("data. txt", O_RDONLY); … /* A string variable */ char pathname[] = "data. txt"; /* Pointer to that string */ filedes = open(pathname, O_RDONLY); 10
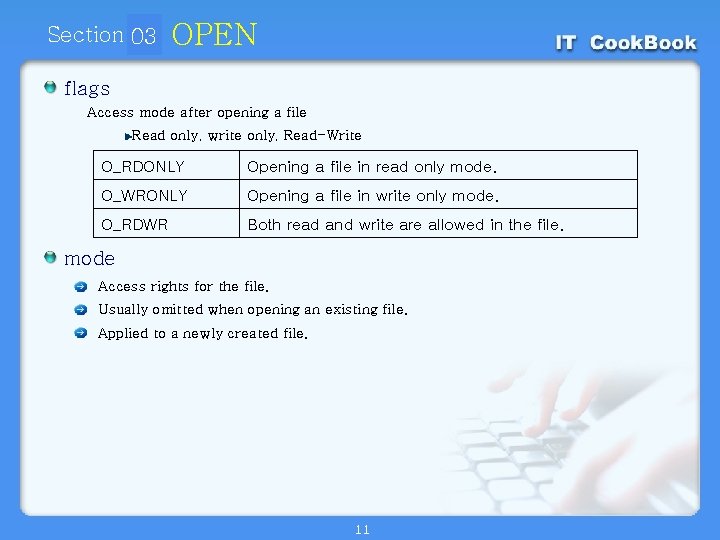
Section 03 01 OPEN flags Access mode after opening a file Read only, write only, Read-Write O_RDONLY Opening a file in read only mode. O_WRONLY Opening a file in write only mode. O_RDWR Both read and write are allowed in the file. mode Access rights for the file. Usually omitted when opening an existing file. Applied to a newly created file. 11
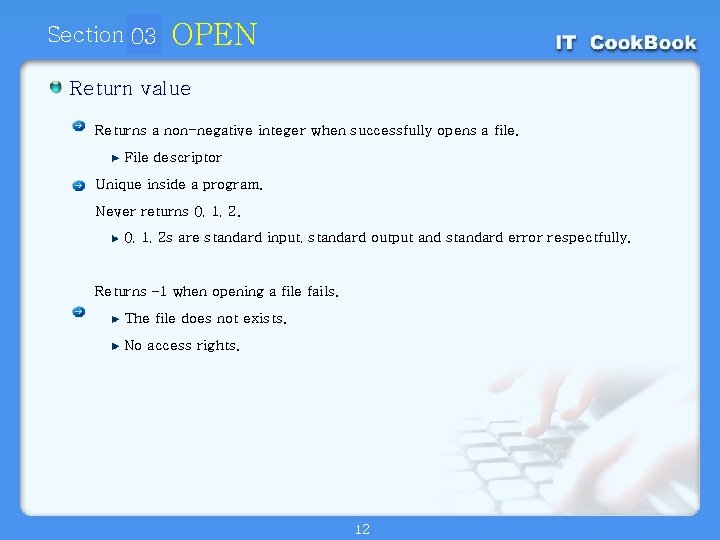
Section 03 01 OPEN Return value Returns a non-negative integer when successfully opens a file. File descriptor Unique inside a program. Never returns 0, 1, 2 s are standard input, standard output and standard error respectfully. Returns – 1 when opening a file fails. The file does not exists. No access rights. 12
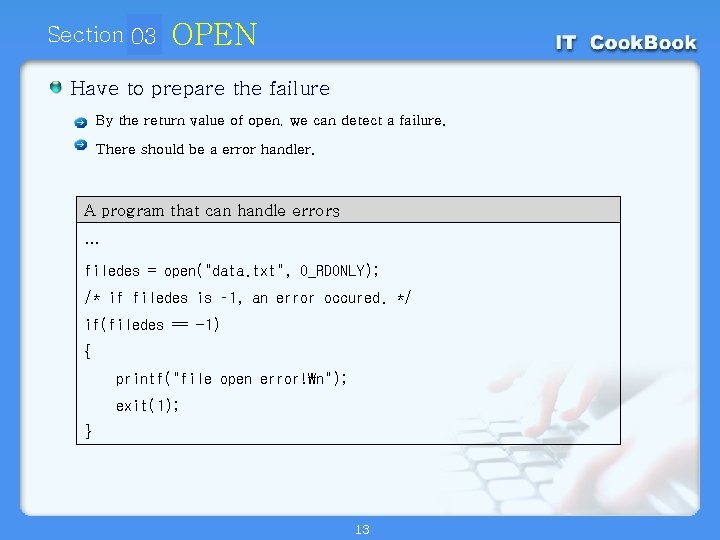
Section 03 01 OPEN Have to prepare the failure By the return value of open, we can detect a failure. There should be a error handler. A program that can handle errors … filedes = open("data. txt", O_RDONLY); /* if filedes is – 1, an error occured. */ if(filedes == -1) { printf("file open error!₩n"); exit(1); } 13
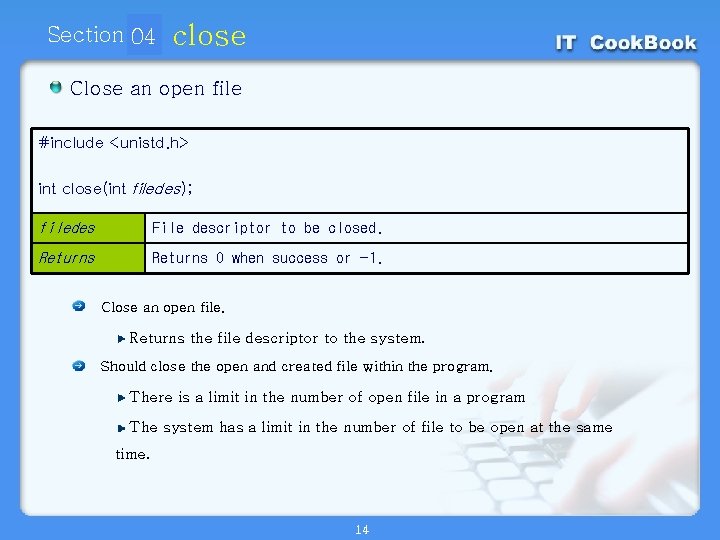
Section 04 01 close Close an open file #include <unistd. h> int close(int filedes); filedes File descriptor to be closed. Returns 0 when success or -1. Close an open file. Returns the file descriptor to the system. Should close the open and created file within the program. There is a limit in the number of open file in a program The system has a limit in the number of file to be open at the same time. 14
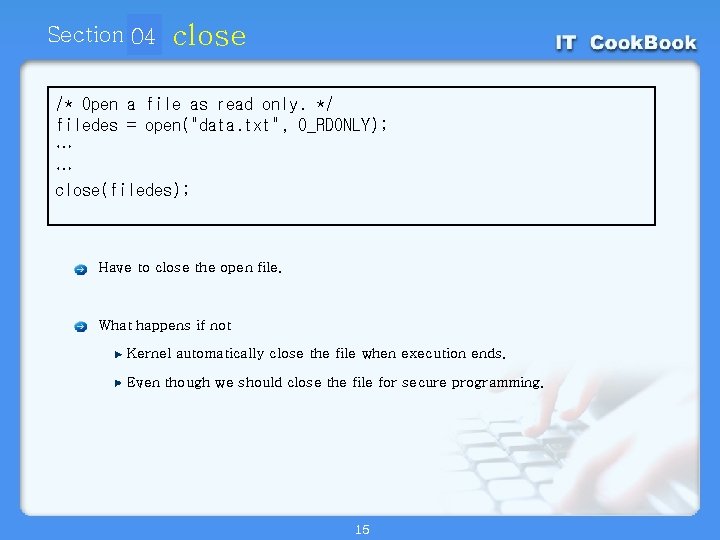
Section 04 01 close /* Open a file as read only. */ filedes = open("data. txt", O_RDONLY); … … close(filedes); Have to close the open file. What happens if not Kernel automatically close the file when execution ends. Even though we should close the file for secure programming. 15
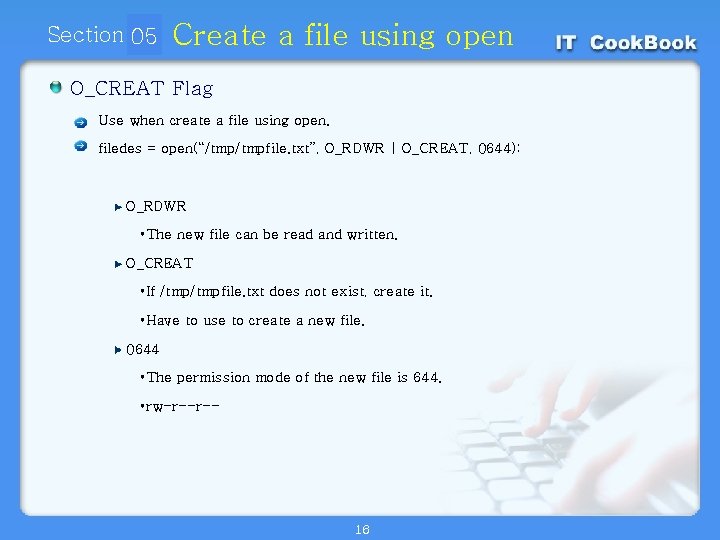
Section 05 01 Create a file using open O_CREAT Flag Use when create a file using open. filedes = open(“/tmpfile. txt”, O_RDWR | O_CREAT, 0644); O_RDWR • The new file can be read and written. O_CREAT • If /tmpfile. txt does not exist, create it. • Have to use to create a new file. 0644 • The permission mode of the new file is 644. • rw-r--r-- 16
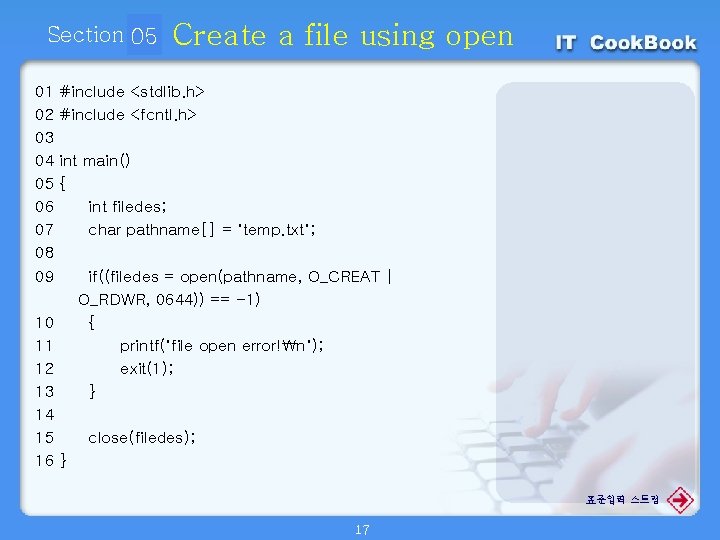
Section 05 01 01 02 03 04 05 06 07 08 09 Create a file using open #include <stdlib. h> #include <fcntl. h> int main() { int filedes; char pathname[] = "temp. txt"; if((filedes = open(pathname, O_CREAT | O_RDWR, 0644)) == -1) { printf("file open error!n"); exit(1); } 10 11 12 13 14 15 close(filedes); 16 } 표준입력 스트림 17
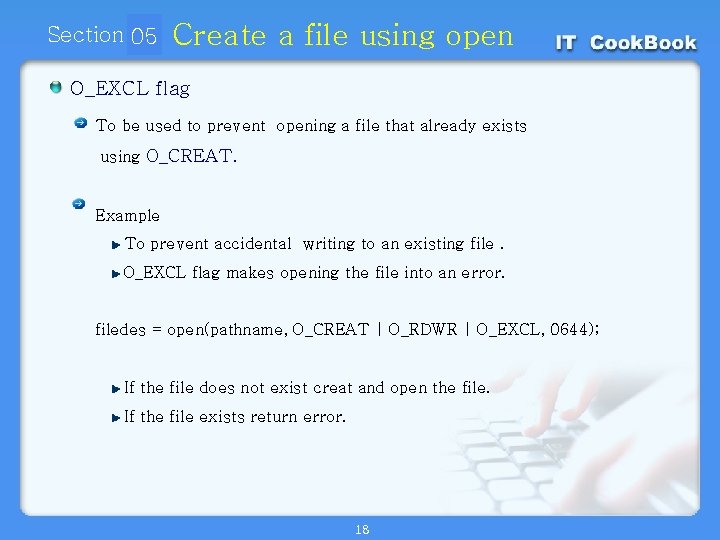
Section 05 01 Create a file using open O_EXCL flag To be used to prevent opening a file that already exists using O_CREAT. Example To prevent accidental writing to an existing file. O_EXCL flag makes opening the file into an error. filedes = open(pathname, O_CREAT | O_RDWR | O_EXCL, 0644); If the file does not exist creat and open the file. If the file exists return error. 18
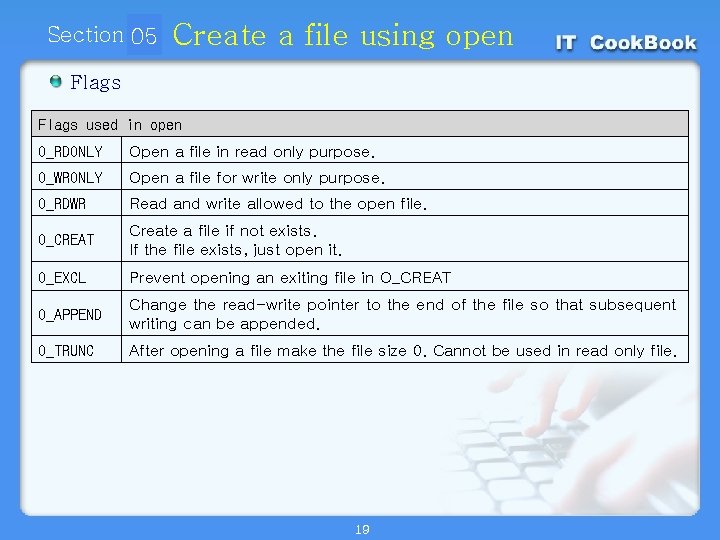
Section 05 01 Create a file using open Flags used in open O_RDONLY Open a file in read only purpose. O_WRONLY Open a file for write only purpose. O_RDWR Read and write allowed to the open file. O_CREAT Create a file if not exists. If the file exists, just open it. O_EXCL Prevent opening an exiting file in O_CREAT O_APPEND Change the read-write pointer to the end of the file so that subsequent writing can be appended. O_TRUNC After opening a file make the file size 0. Cannot be used in read only file. 19
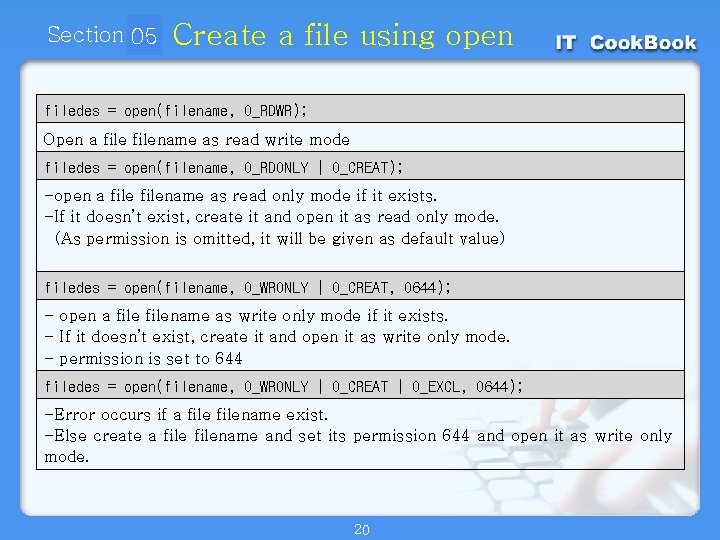
Section 05 01 Create a file using open filedes = open(filename, O_RDWR); Open a filename as read write mode filedes = open(filename, O_RDONLY | O_CREAT); -open a filename as read only mode if it exists. -If it doesn’t exist, create it and open it as read only mode. (As permission is omitted, it will be given as default value) filedes = open(filename, O_WRONLY | O_CREAT, 0644); - open a filename as write only mode if it exists. - If it doesn’t exist, create it and open it as write only mode. - permission is set to 644 filedes = open(filename, O_WRONLY | O_CREAT | O_EXCL, 0644); -Error occurs if a filename exist. -Else create a filename and set its permission 644 and open it as write only mode. 20
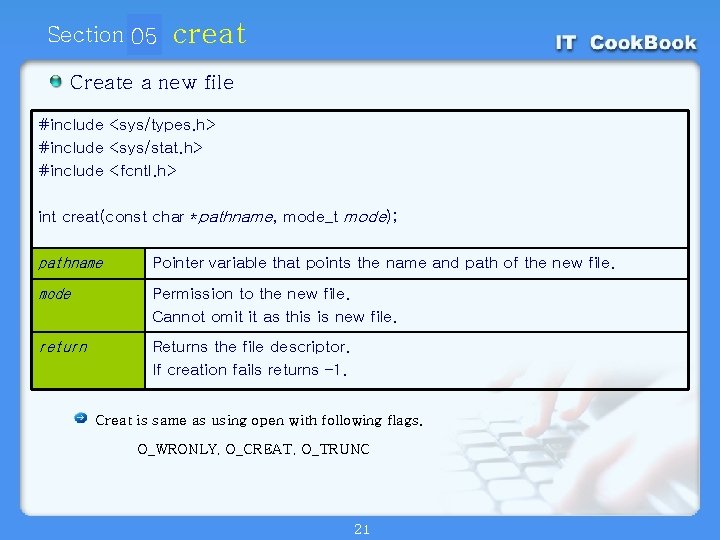
Section 05 01 creat Create a new file #include <sys/types. h> #include <sys/stat. h> #include <fcntl. h> int creat(const char *pathname, mode_t mode); pathname Pointer variable that points the name and path of the new file. mode Permission to the new file. Cannot omit it as this is new file. return Returns the file descriptor. If creation fails returns – 1. Creat is same as using open with following flags. O_WRONLY, O_CREAT, O_TRUNC 21
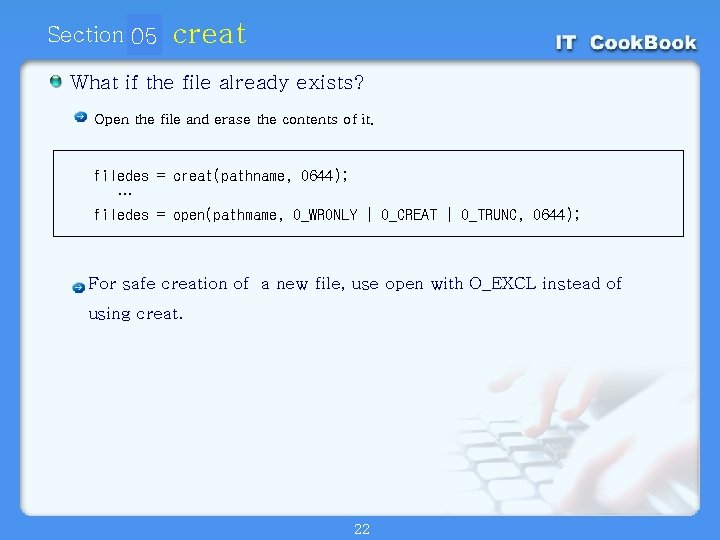
Section 05 01 creat What if the file already exists? Open the file and erase the contents of it. filedes = creat(pathname, 0644); … filedes = open(pathmame, O_WRONLY | O_CREAT | O_TRUNC, 0644); For safe creation of a new file, use open with O_EXCL instead of using creat. 22
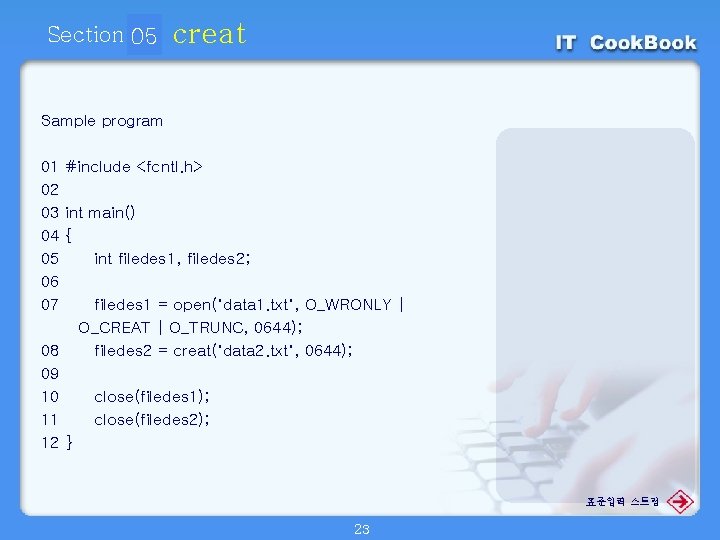
Section 05 01 creat Sample program 01 02 03 04 05 06 07 #include <fcntl. h> int main() { int filedes 1, filedes 2; filedes 1 = open("data 1. txt", O_WRONLY | O_CREAT | O_TRUNC, 0644); 08 filedes 2 = creat("data 2. txt", 0644); 09 10 close(filedes 1); 11 close(filedes 2); 12 } 표준입력 스트림 23
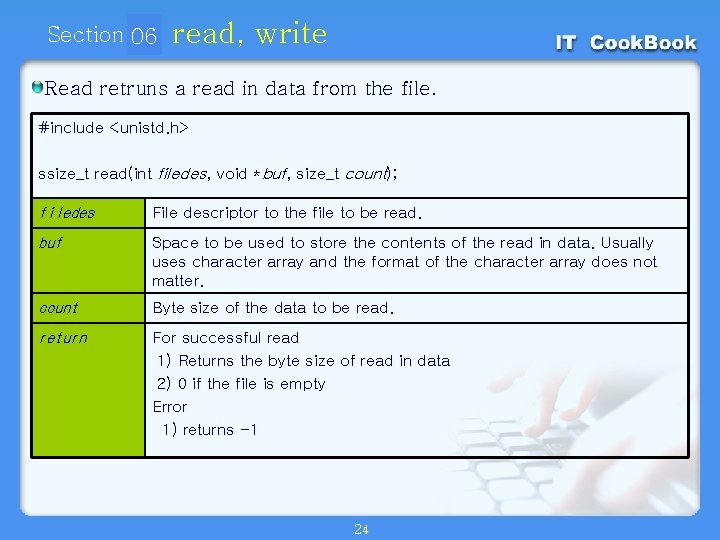
Section 06 01 read, write Read retruns a read in data from the file. #include <unistd. h> ssize_t read(int filedes, void *buf, size_t count); filedes File descriptor to the file to be read. buf Space to be used to store the contents of the read in data. Usually uses character array and the format of the character array does not matter. count Byte size of the data to be read. return For successful read 1) Returns the byte size of read in data 2) 0 if the file is empty Error 1) returns -1 24
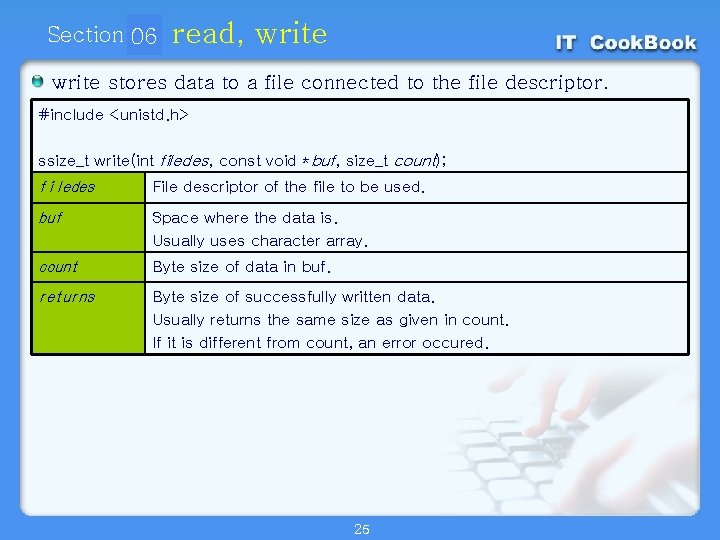
Section 06 01 read, write stores data to a file connected to the file descriptor. #include <unistd. h> ssize_t write(int filedes, const void *buf, size_t count); filedes File descriptor of the file to be used. buf Space where the data is. Usually uses character array. count Byte size of data in buf. returns Byte size of successfully written data. Usually returns the same size as given in count. If it is different from count, an error occured. 25
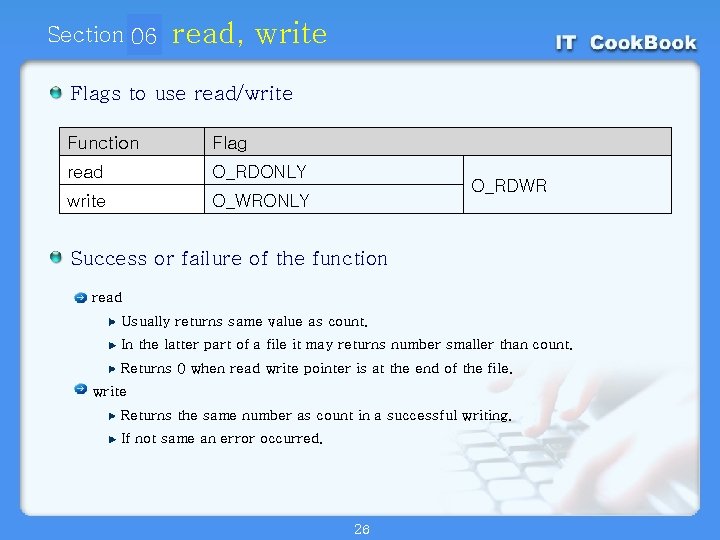
Section 06 01 read, write Flags to use read/write Function Flag read O_RDONLY write O_WRONLY O_RDWR Success or failure of the function read Usually returns same value as count. In the latter part of a file it may returns number smaller than count. Returns 0 when read write pointer is at the end of the file. write Returns the same number as count in a successful writing. If not same an error occurred. 26
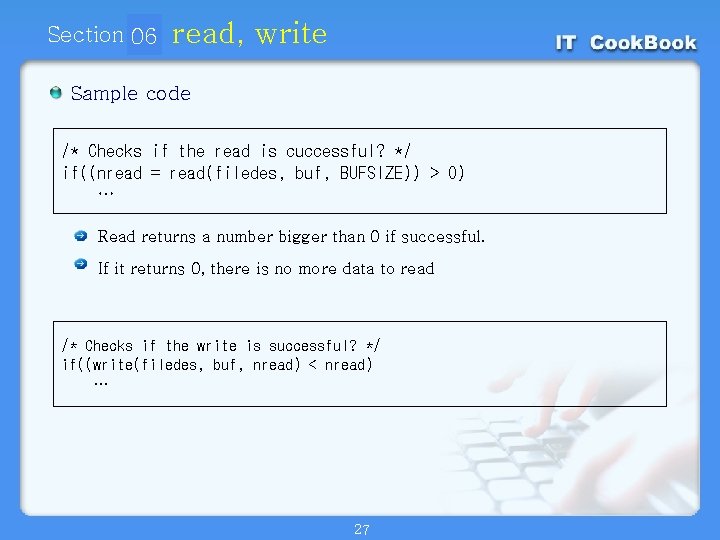
Section 06 01 read, write Sample code /* Checks if the read is cuccessful? */ if((nread = read(filedes, buf, BUFSIZE)) > 0) … Read returns a number bigger than 0 if successful. If it returns 0, there is no more data to read /* Checks if the write is successful? */ if((write(filedes, buf, nread) < nread) … 27
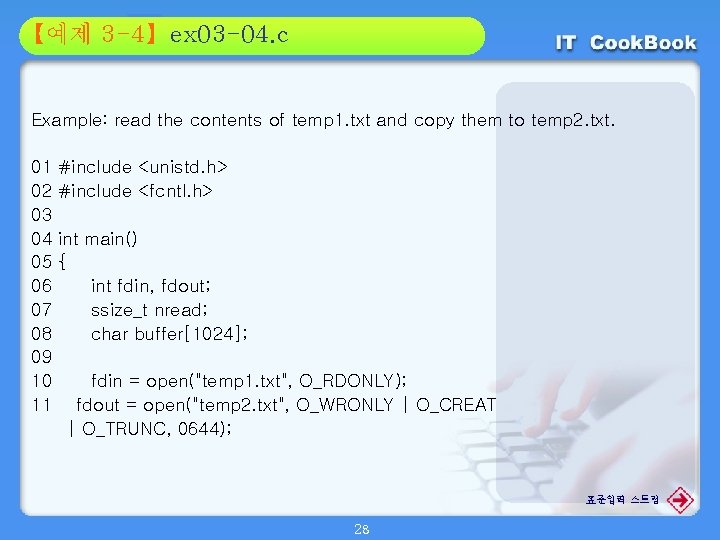
Section 01 【예제 3 -4】ex 03 -04. c 02 Example: read the contents of temp 1. txt and copy them to temp 2. txt. 01 #include <unistd. h> 02 #include <fcntl. h> 03 04 int main() 05 { 06 int fdin, fdout; 07 ssize_t nread; 08 char buffer[1024]; 09 10 fdin = open("temp 1. txt", O_RDONLY); 11 fdout = open("temp 2. txt", O_WRONLY | O_CREAT | O_TRUNC, 0644); 표준입력 스트림 28
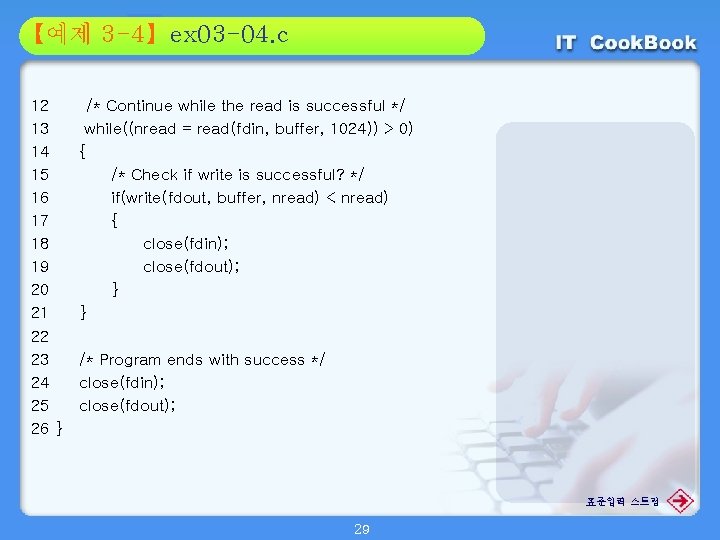
Section 01 【예제 3 -4】ex 03 -04. c 02 12 /* Continue while the read is successful */ 13 while((nread = read(fdin, buffer, 1024)) > 0) 14 { 15 /* Check if write is successful? */ 16 if(write(fdout, buffer, nread) < nread) 17 { 18 close(fdin); 19 close(fdout); 20 } 21 } 22 23 /* Program ends with success */ 24 close(fdin); 25 close(fdout); 26 } 표준입력 스트림 29
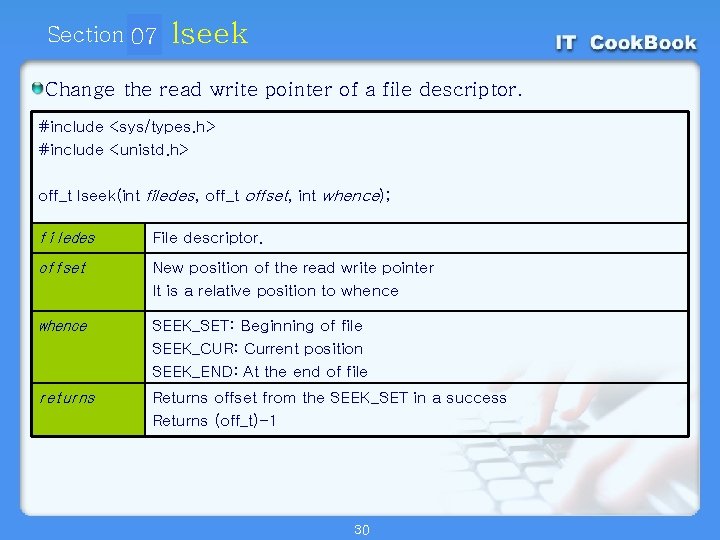
Section 07 01 lseek Change the read write pointer of a file descriptor. #include <sys/types. h> #include <unistd. h> off_t lseek(int filedes, off_t offset, int whence); filedes File descriptor. offset New position of the read write pointer It is a relative position to whence SEEK_SET: Beginning of file SEEK_CUR: Current position SEEK_END: At the end of file returns Returns offset from the SEEK_SET in a success Returns (off_t)-1 30
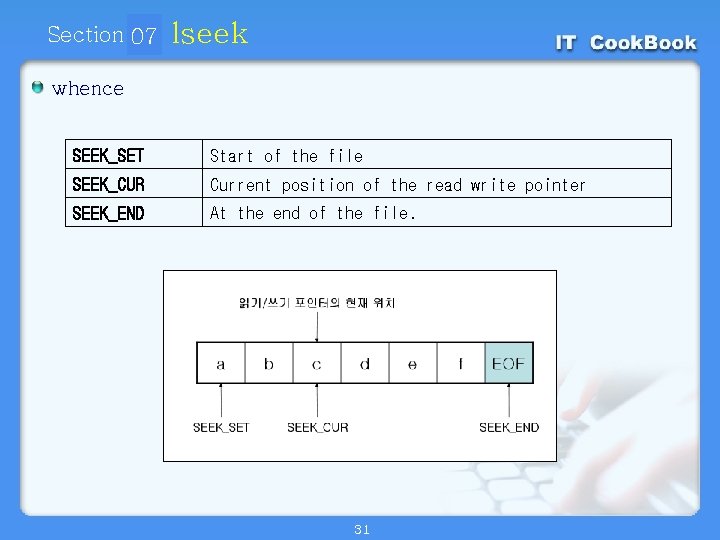
Section 07 01 lseek whence SEEK_SET Start of the file SEEK_CUR Current position of the read write pointer SEEK_END At the end of the file. 31
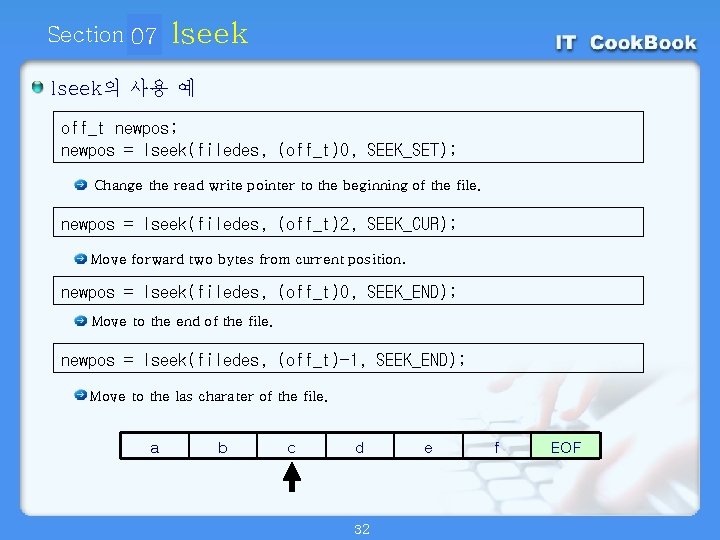
Section 07 01 lseek의 사용 예 off_t newpos; newpos = lseek(filedes, (off_t)0, SEEK_SET); Change the read write pointer to the beginning of the file. newpos = lseek(filedes, (off_t)2, SEEK_CUR); Move forward two bytes from current position. newpos = lseek(filedes, (off_t)0, SEEK_END); Move to the end of the file. newpos = lseek(filedes, (off_t)-1, SEEK_END); Move to the las charater of the file. a b c d 32 e f EOF
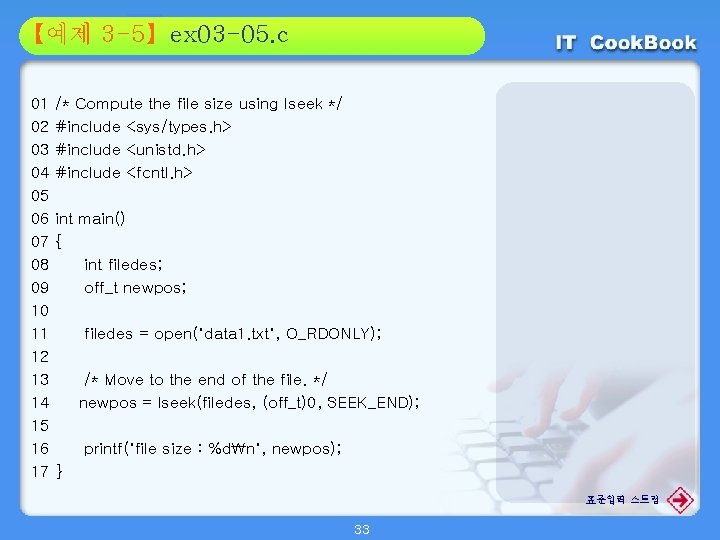
Section 01 【예제 3 -5】ex 03 -05. c 02 01 /* Compute the file size using lseek */ 02 #include <sys/types. h> 03 #include <unistd. h> 04 #include <fcntl. h> 05 06 int main() 07 { 08 int filedes; 09 off_t newpos; 10 11 filedes = open("data 1. txt", O_RDONLY); 12 13 /* Move to the end of the file. */ 14 newpos = lseek(filedes, (off_t)0, SEEK_END); 15 16 printf("file size : %dn", newpos); 17 } 표준입력 스트림 33
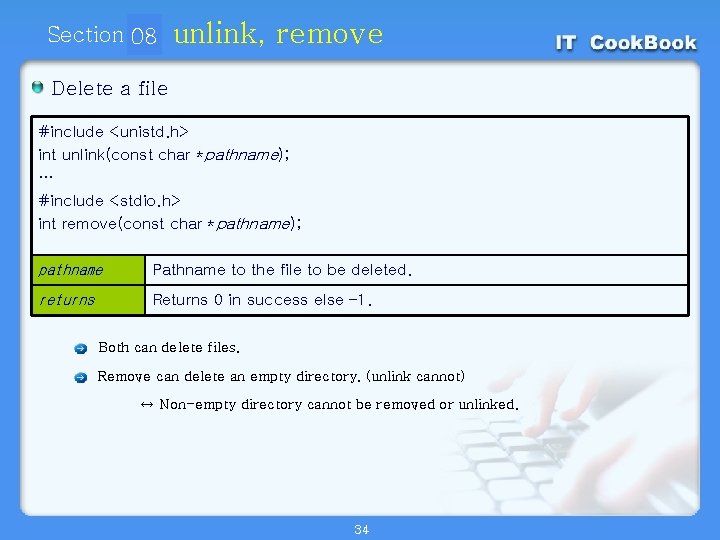
Section 08 01 unlink, remove Delete a file #include <unistd. h> int unlink(const char *pathname); … #include <stdio. h> int remove(const char *pathname); pathname Pathname to the file to be deleted. returns Returns 0 in success else – 1. Both can delete files. Remove can delete an empty directory. (unlink cannot) ↔ Non-empty directory cannot be removed or unlinked. 34