3 Data Types and Expressions C Programming From
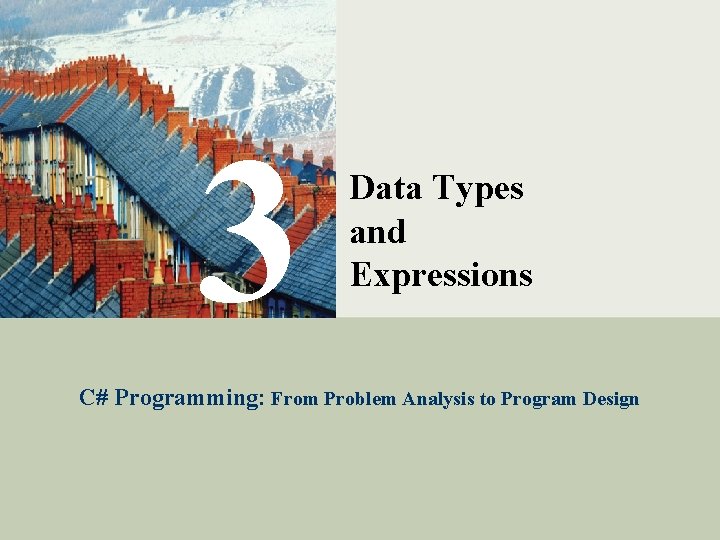
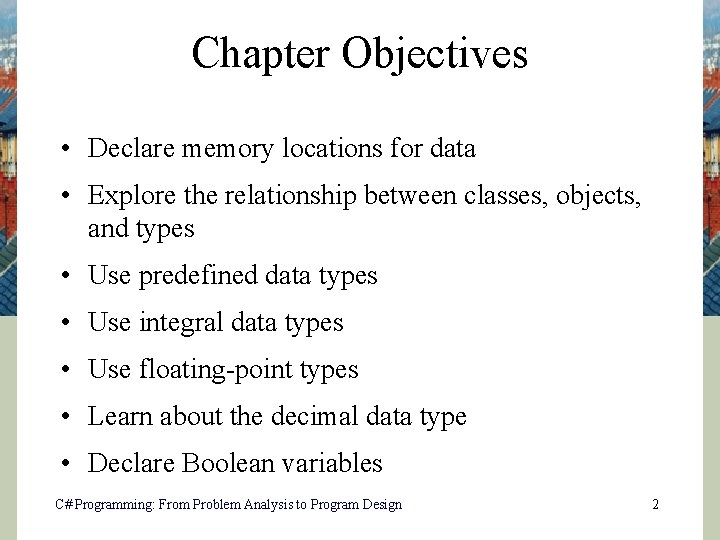
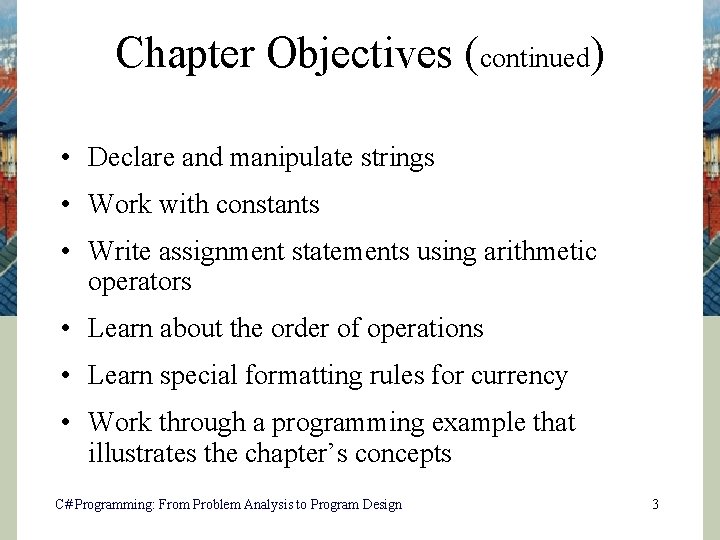
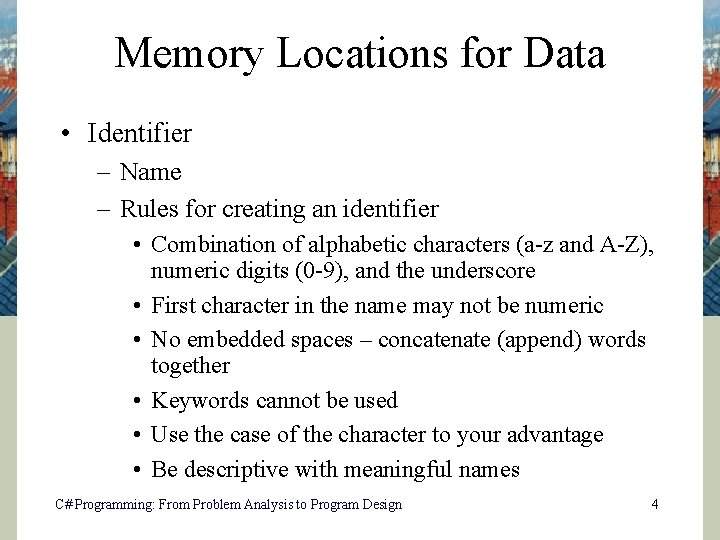
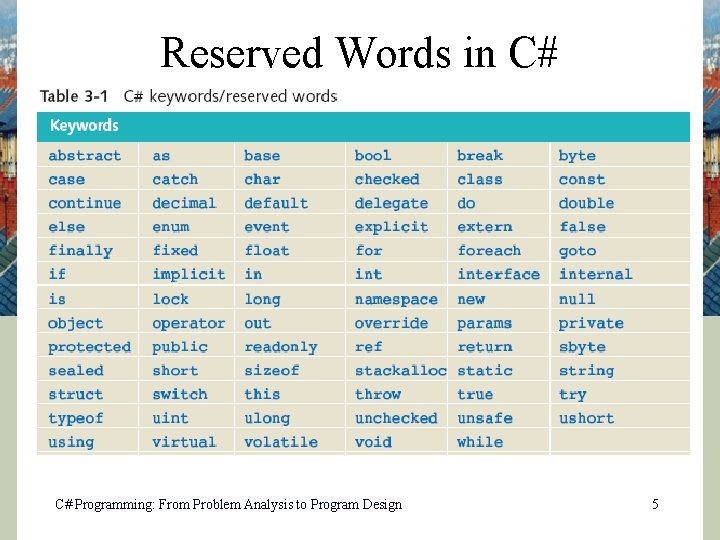
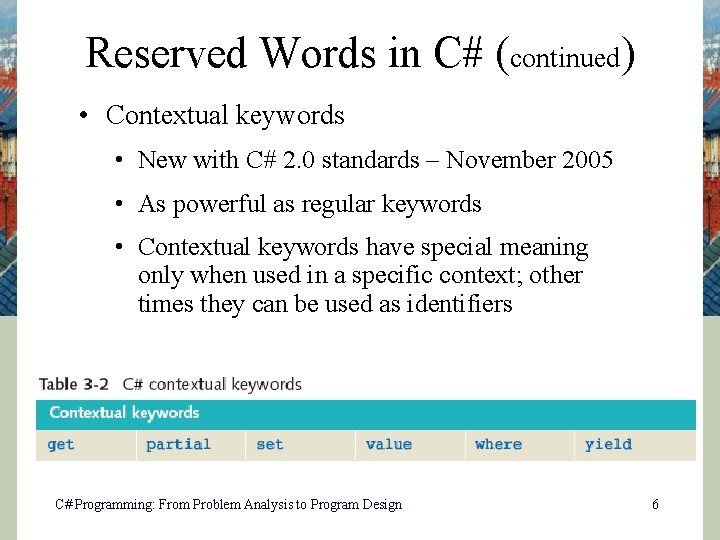
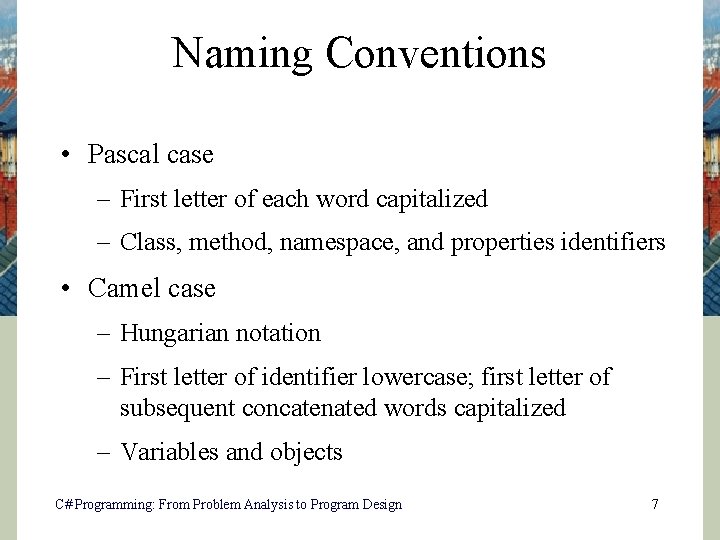
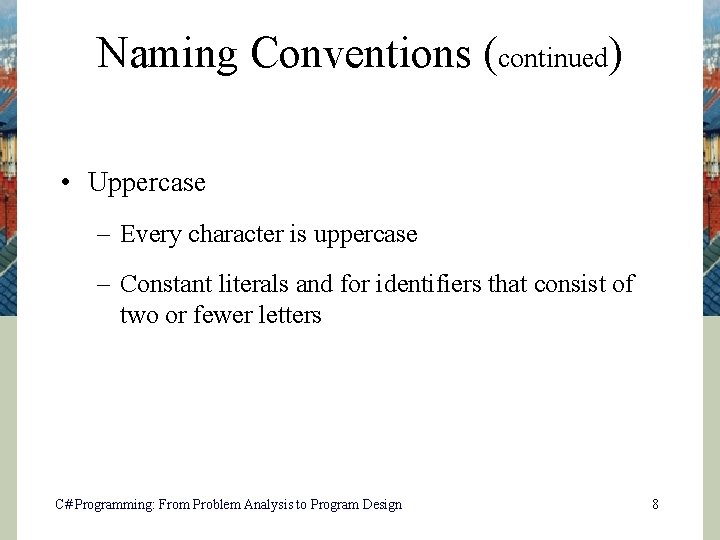
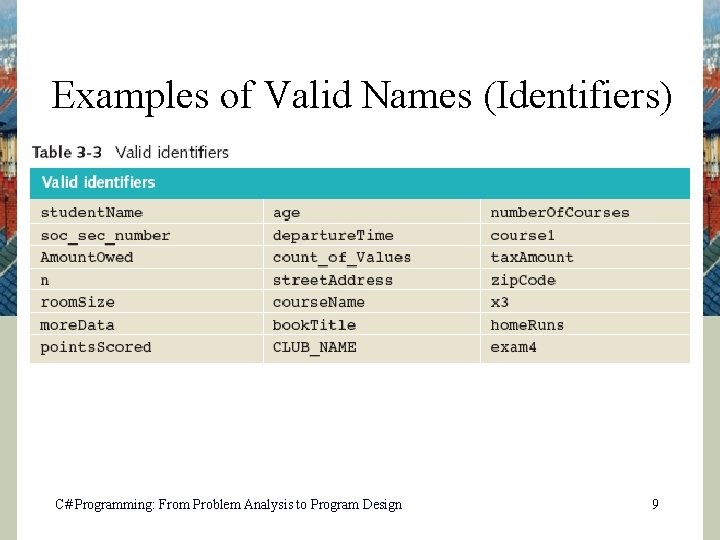
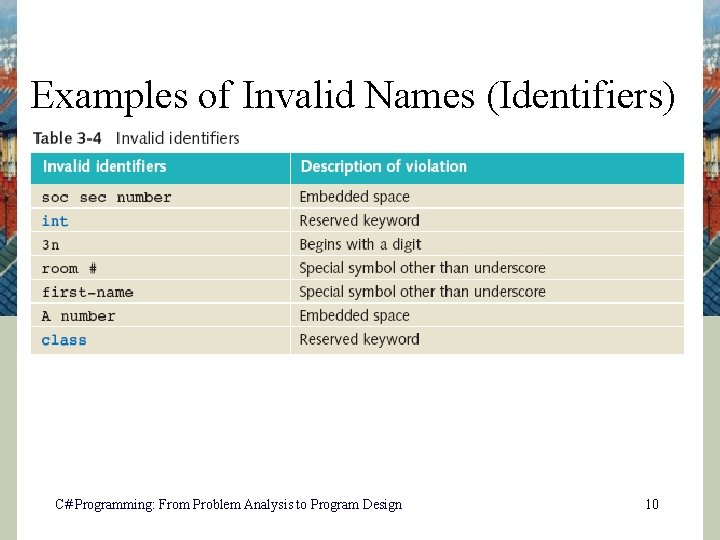
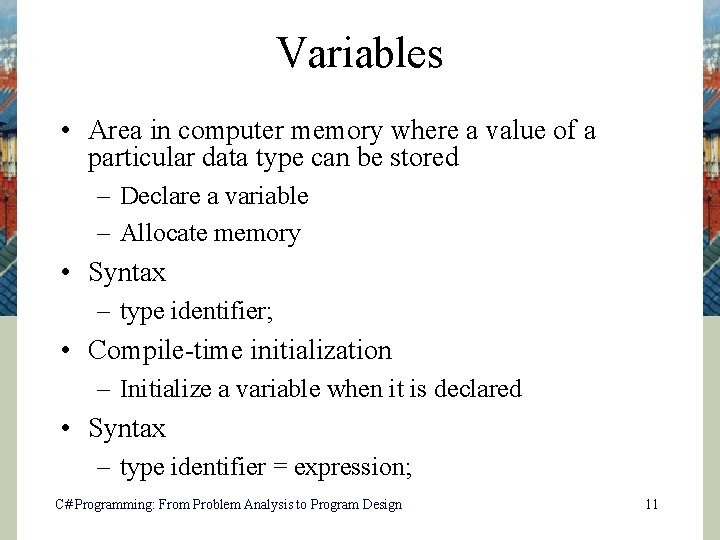
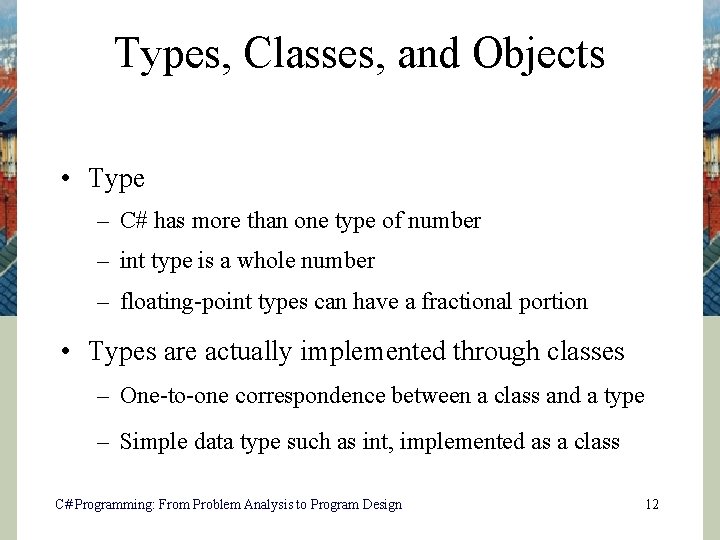
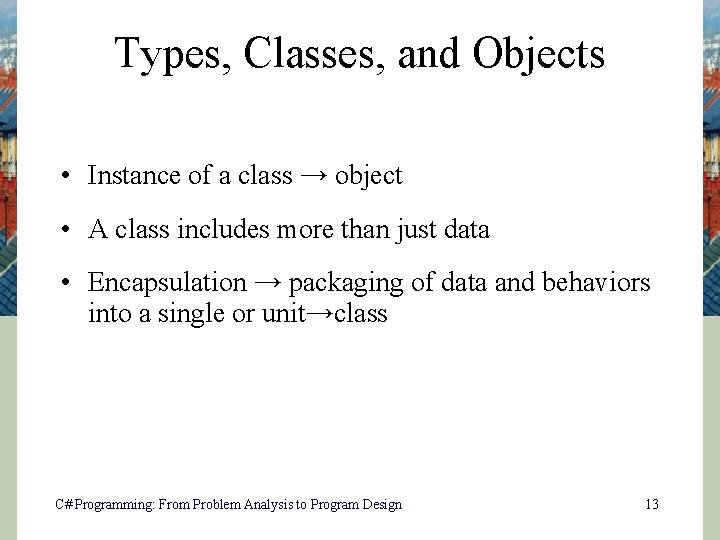
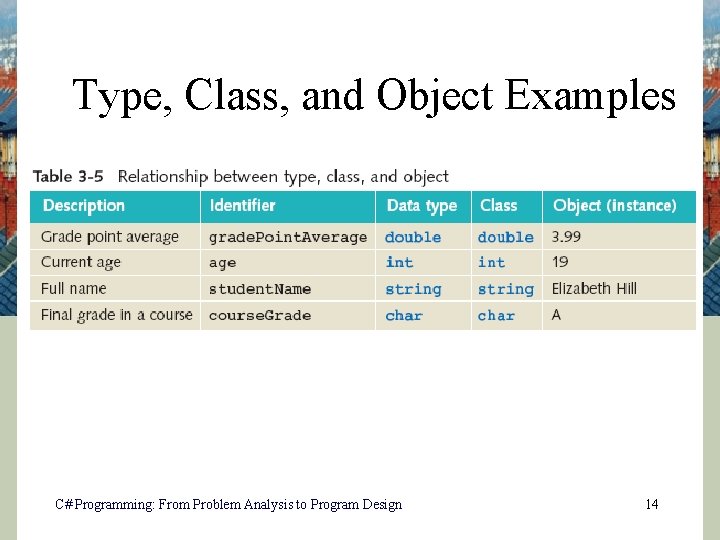
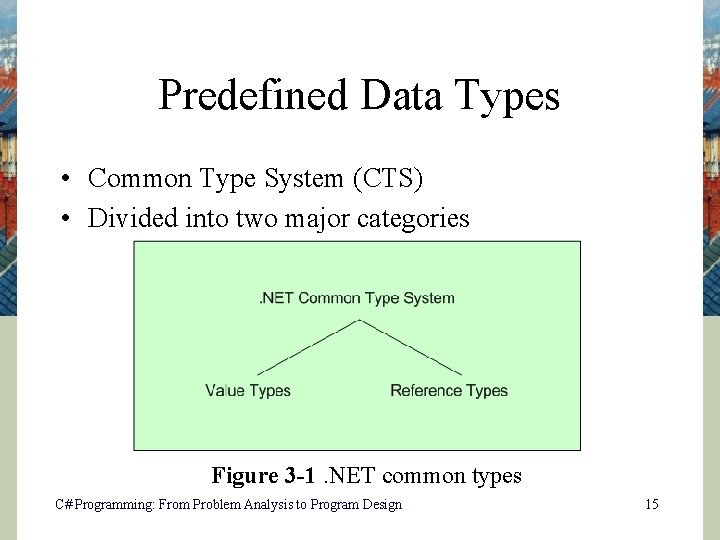
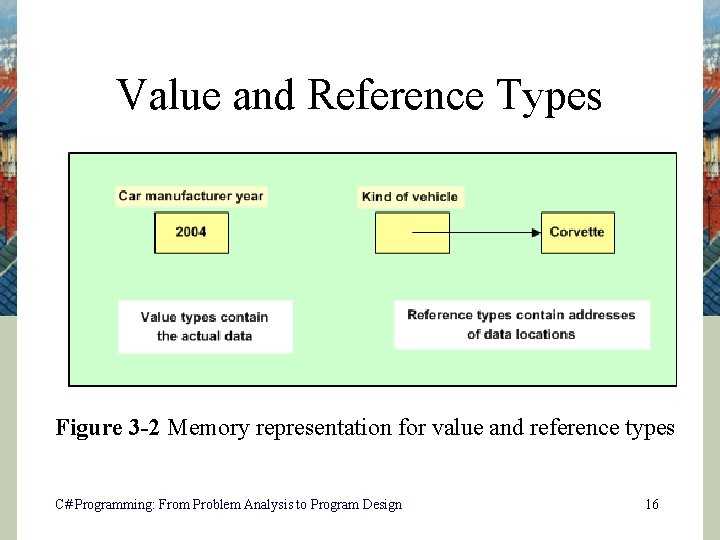
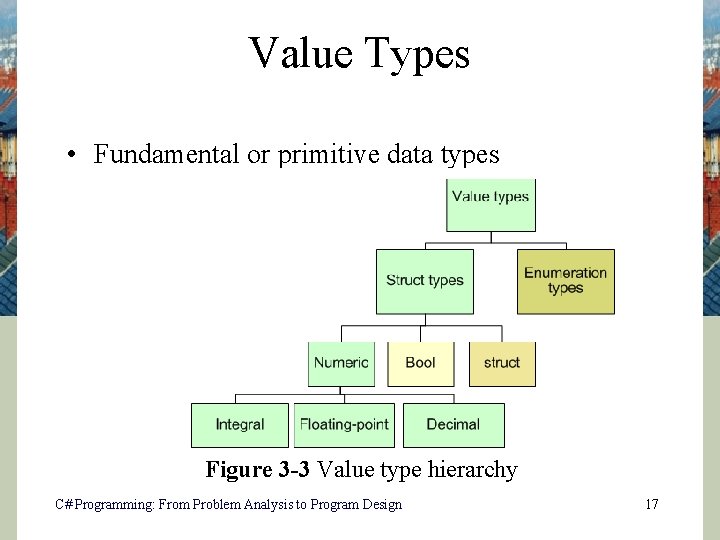
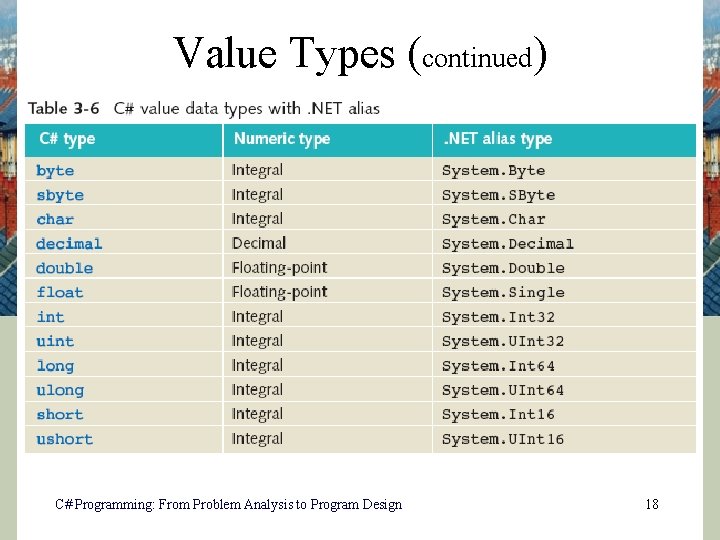
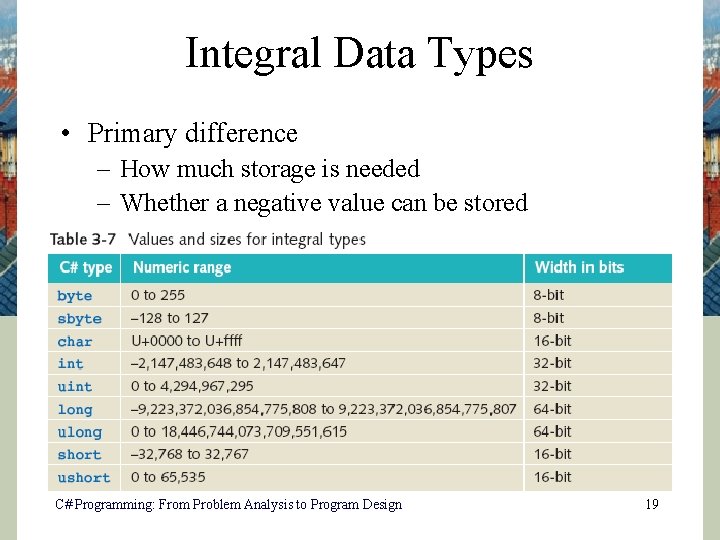
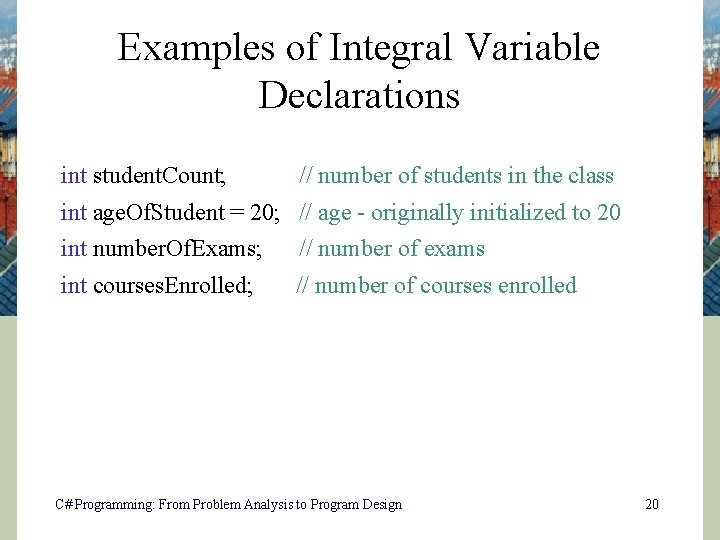
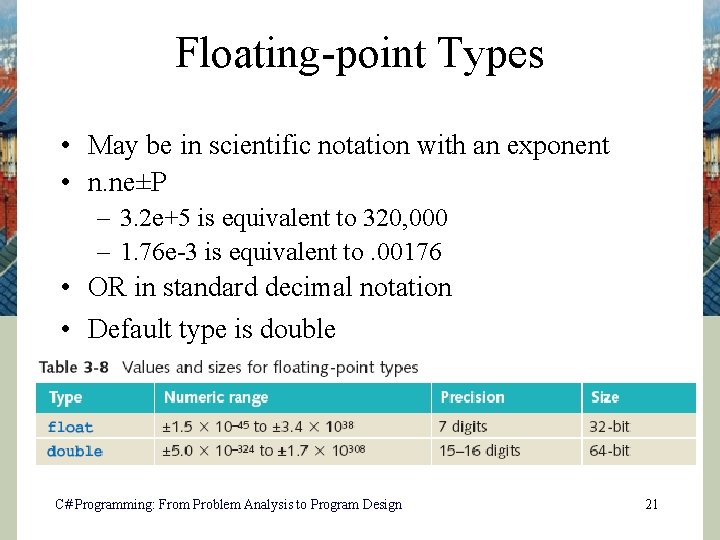
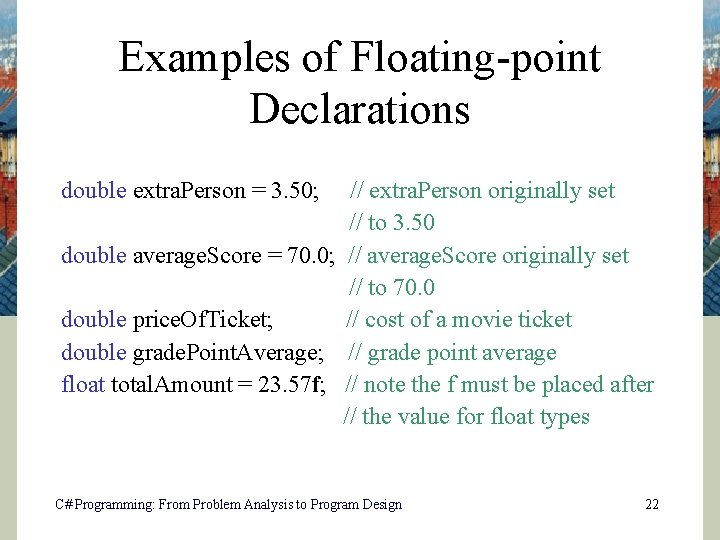
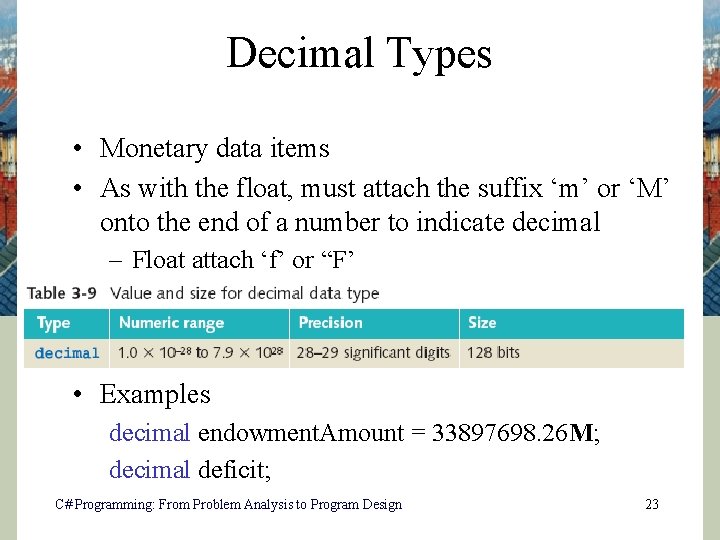
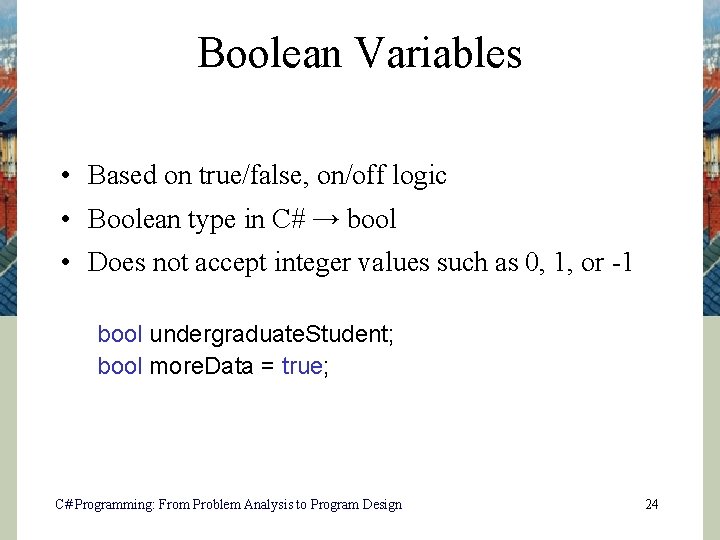
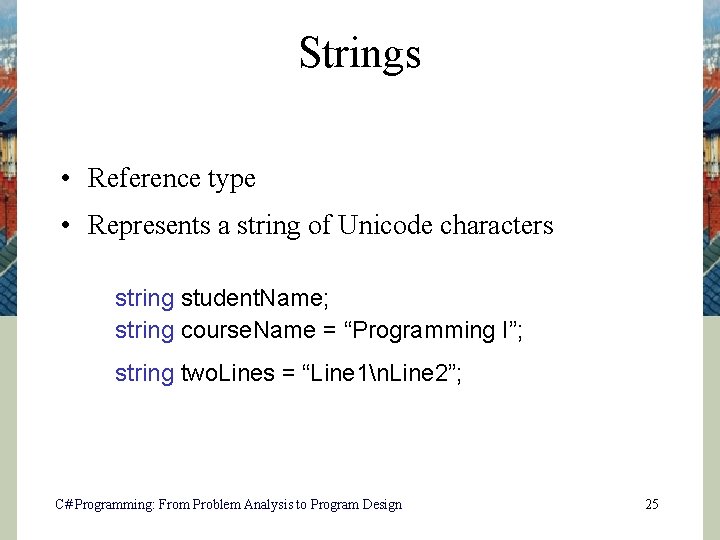
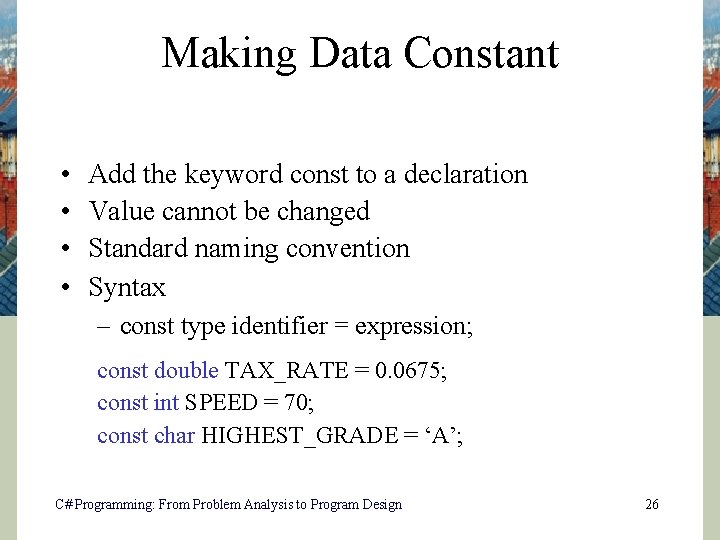
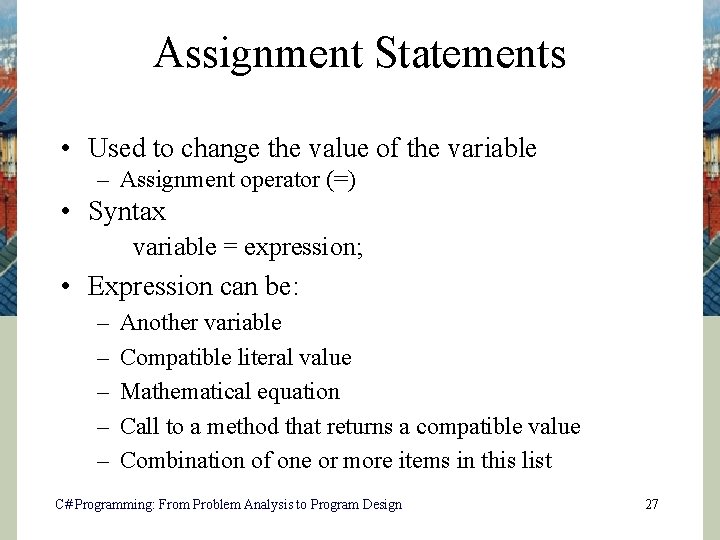
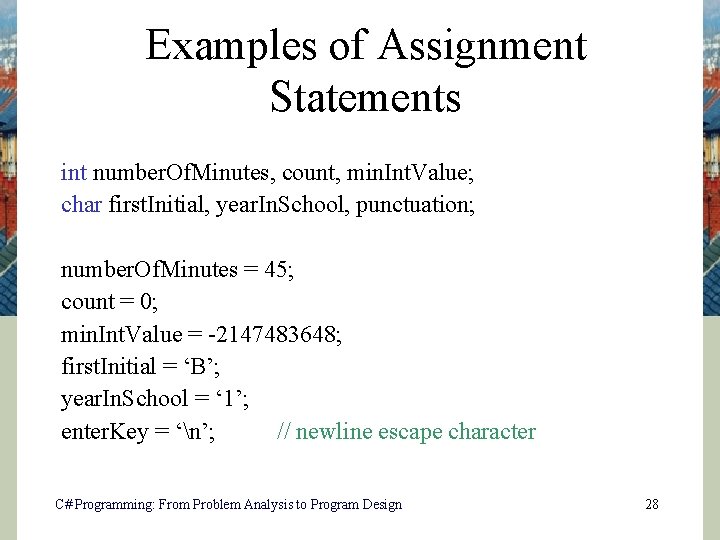
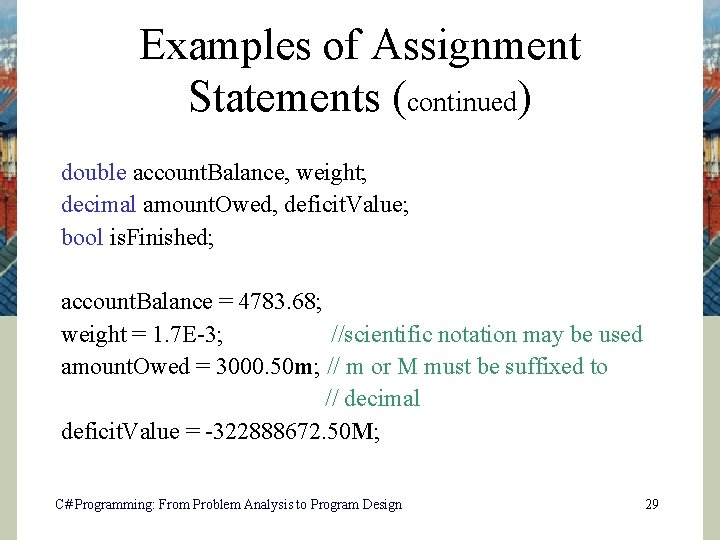
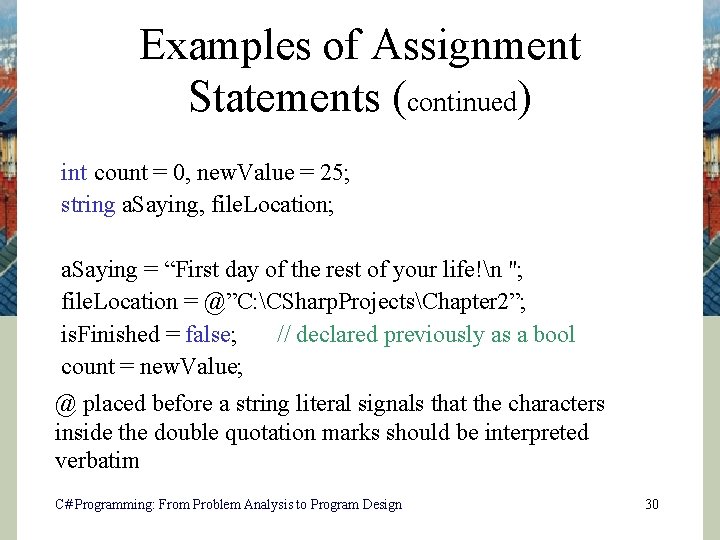
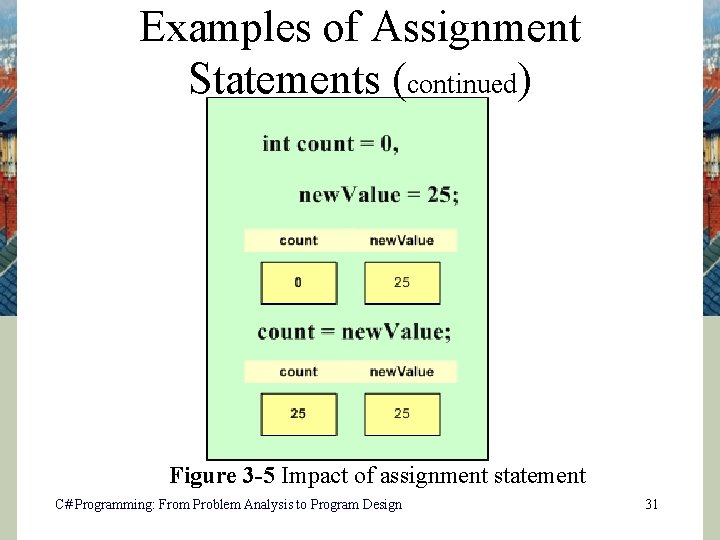
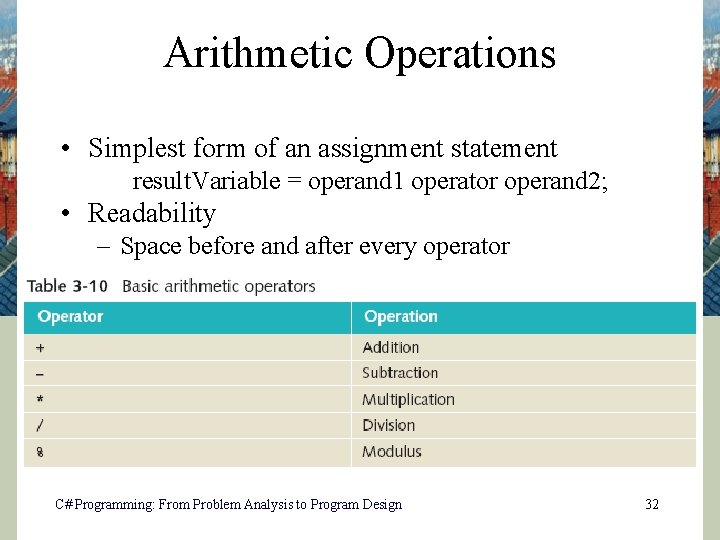
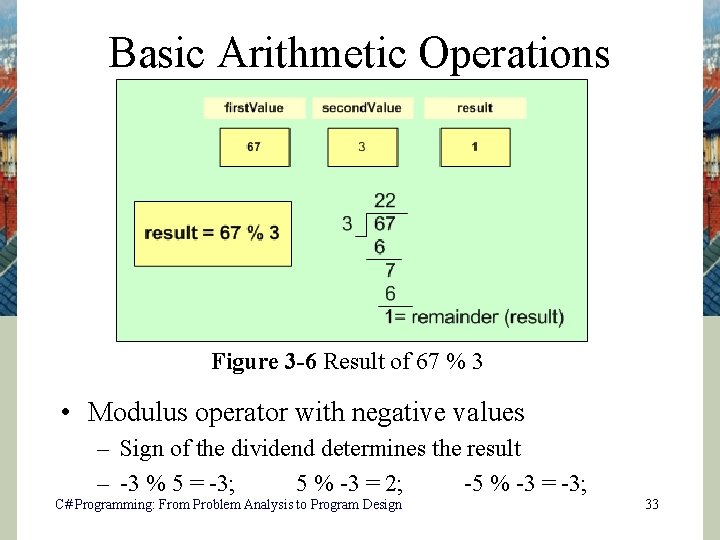
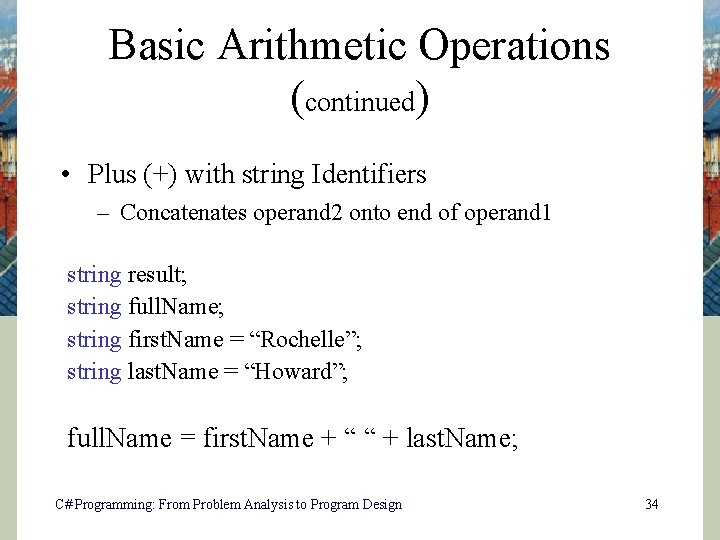
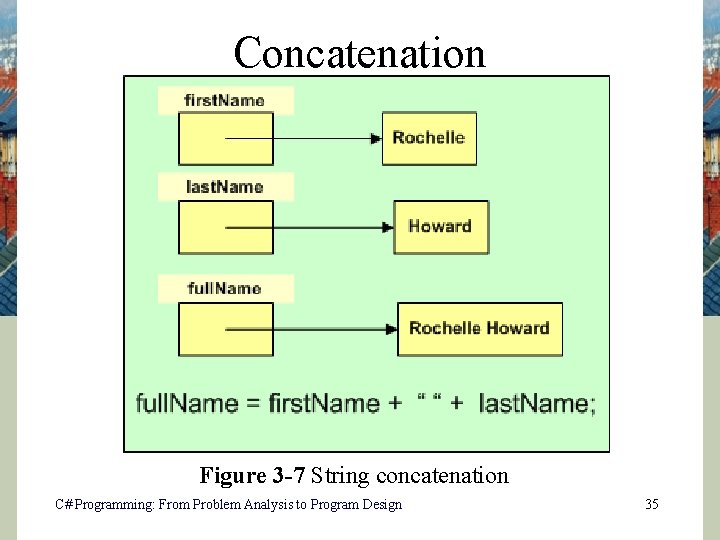
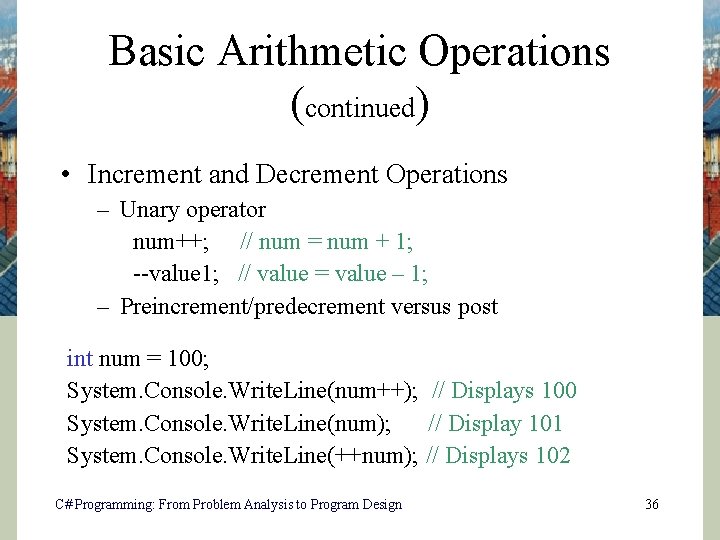
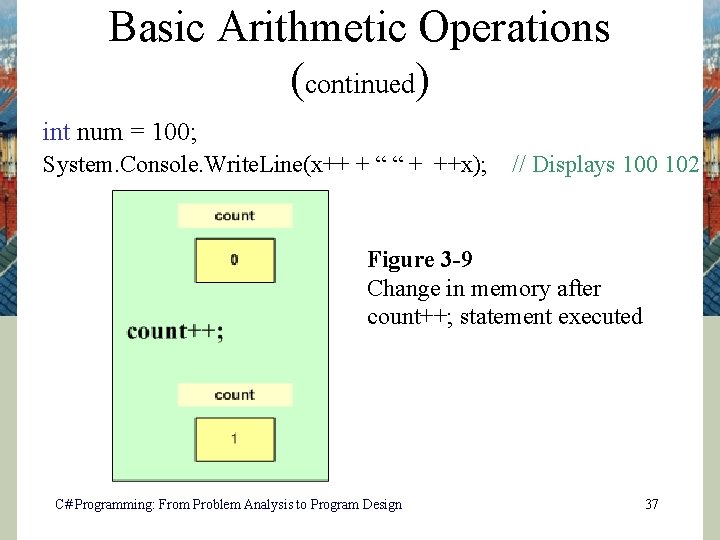
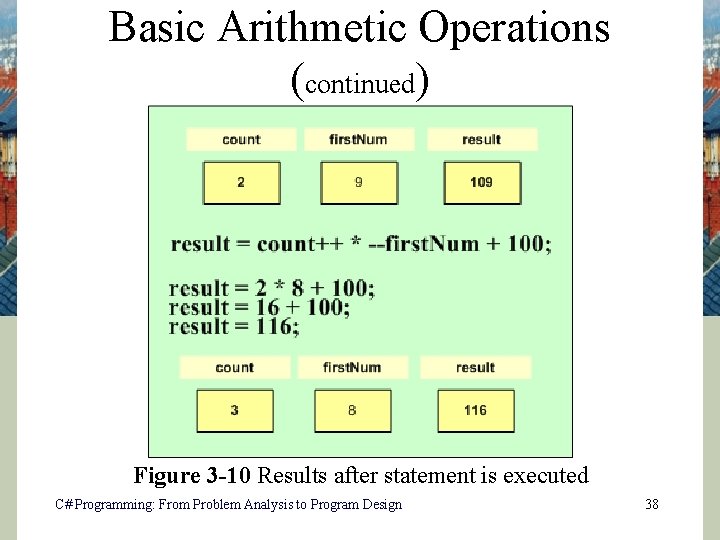
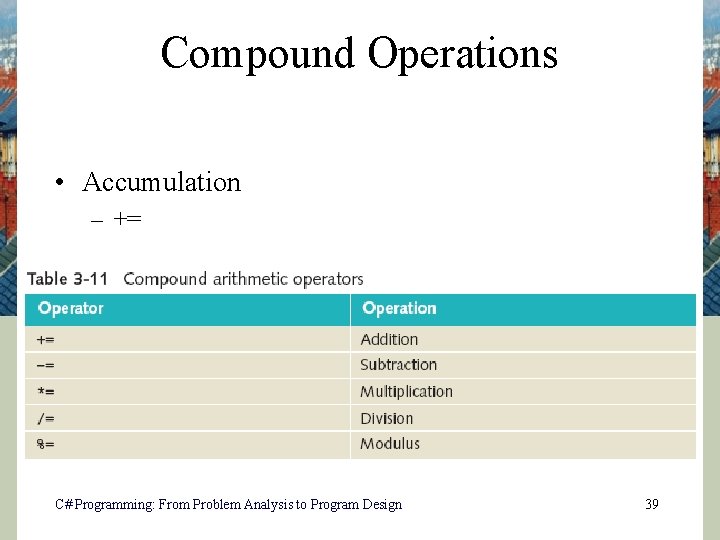
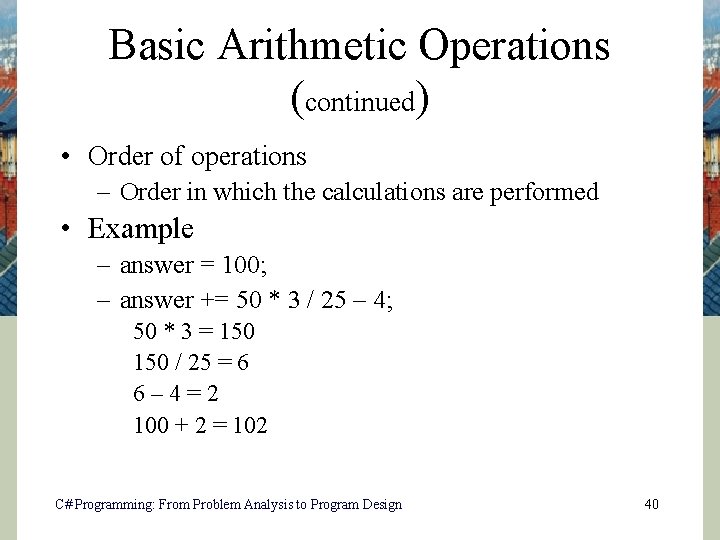
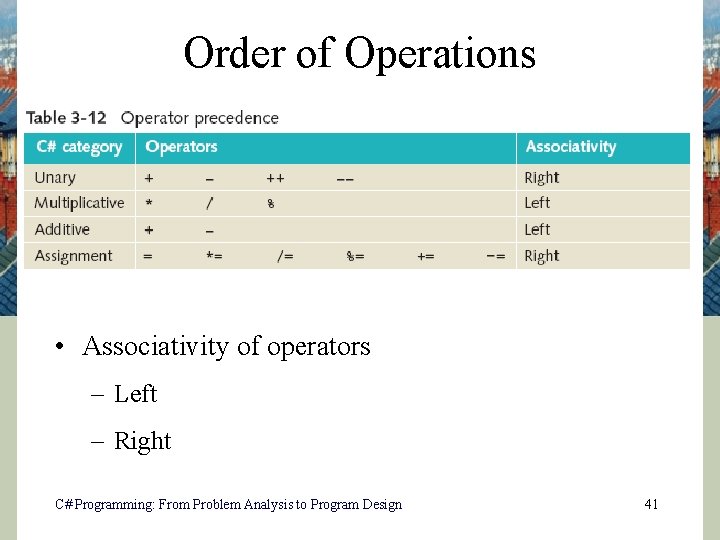
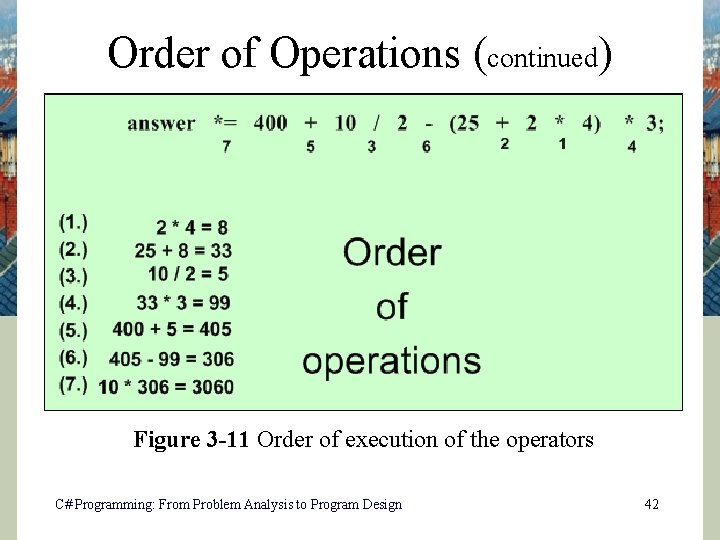
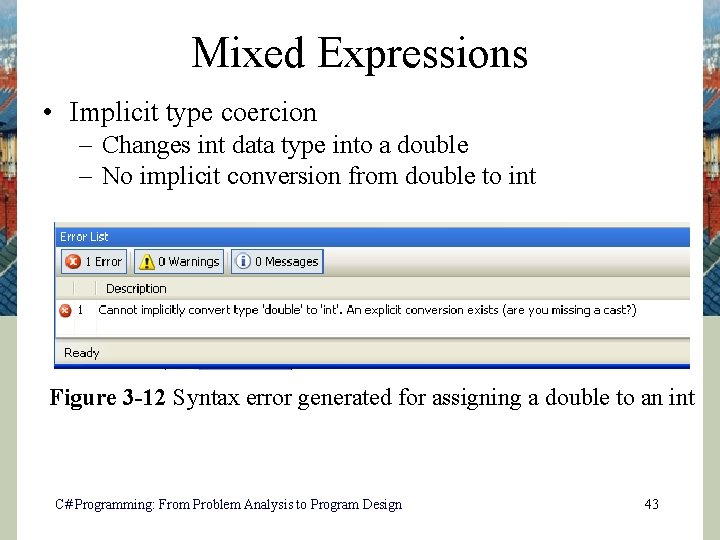
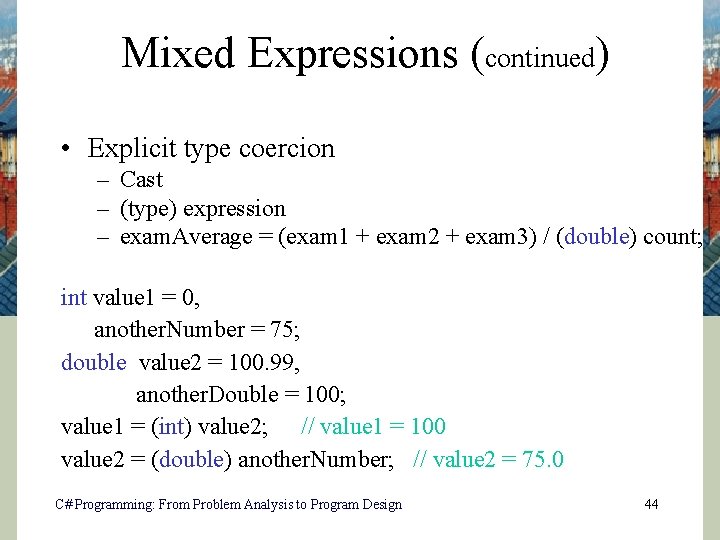
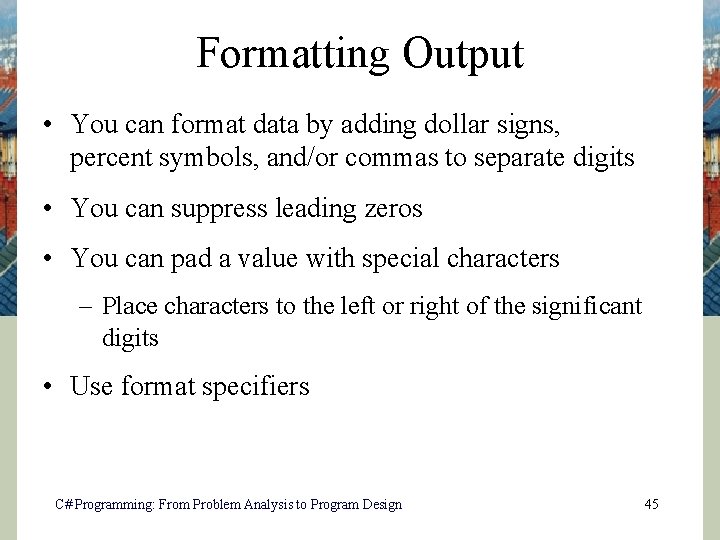
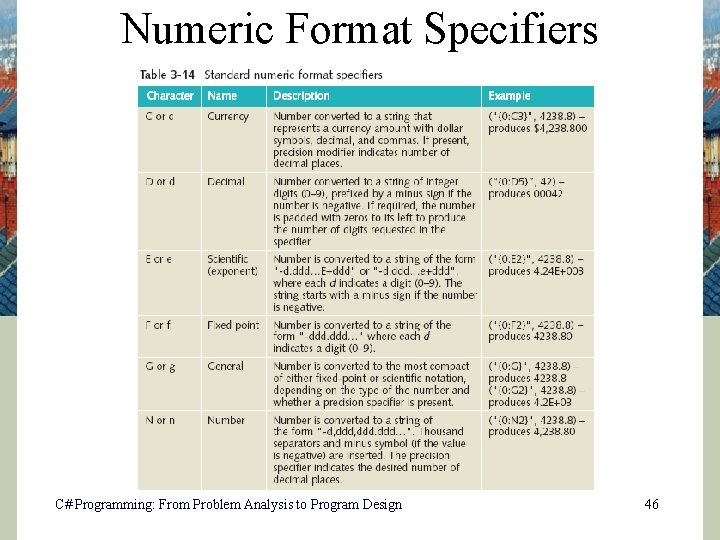
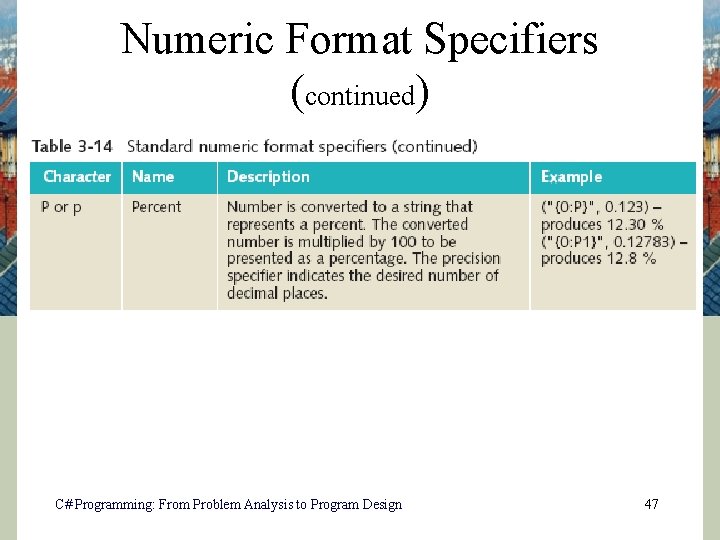
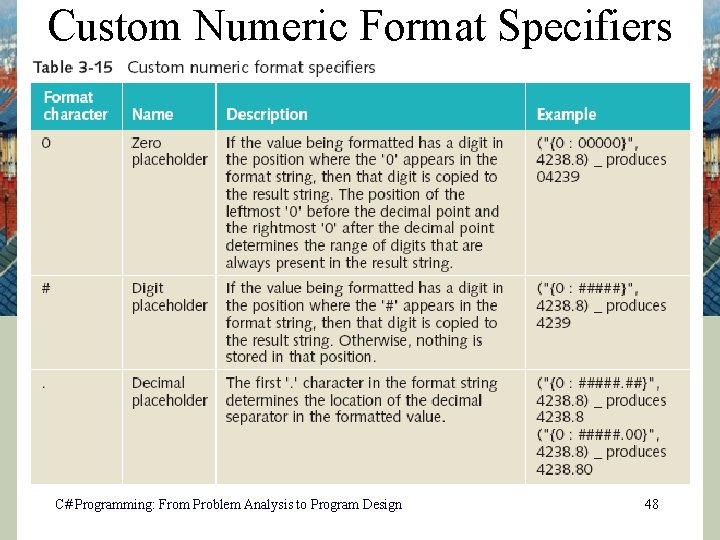
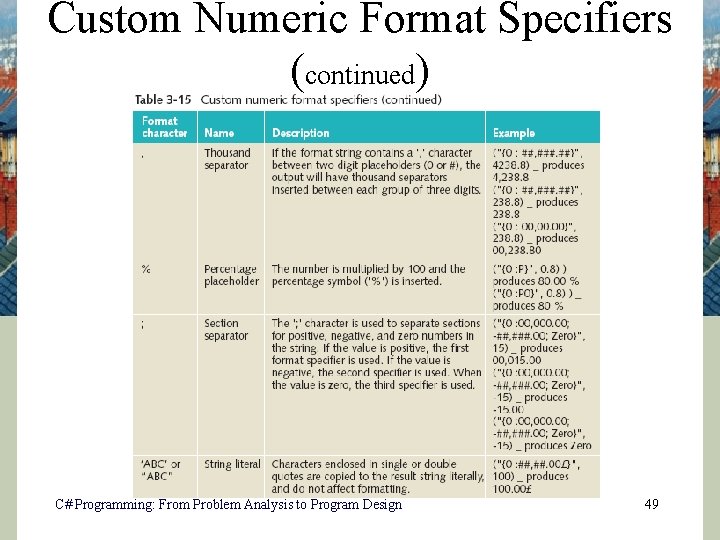
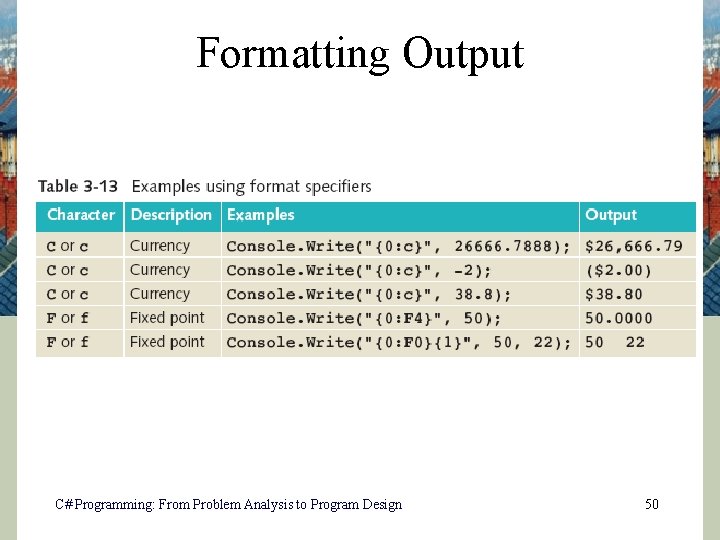
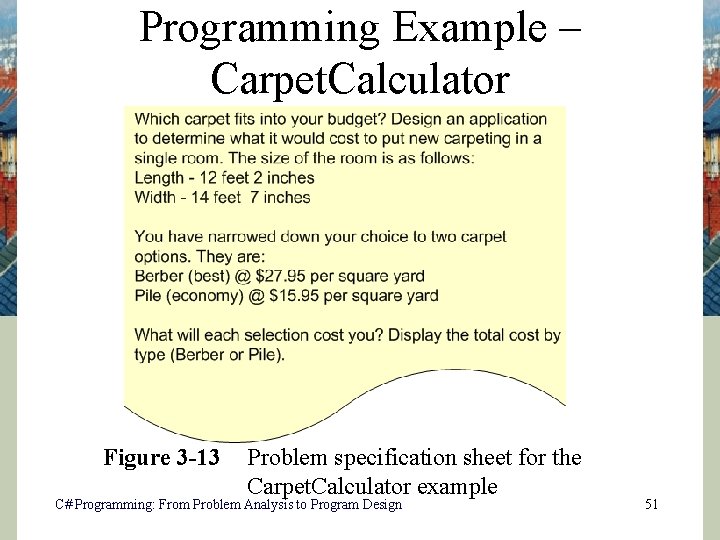
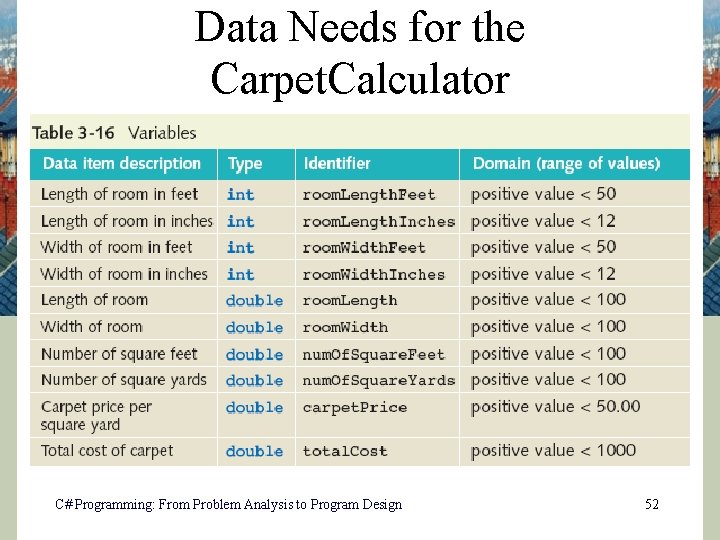
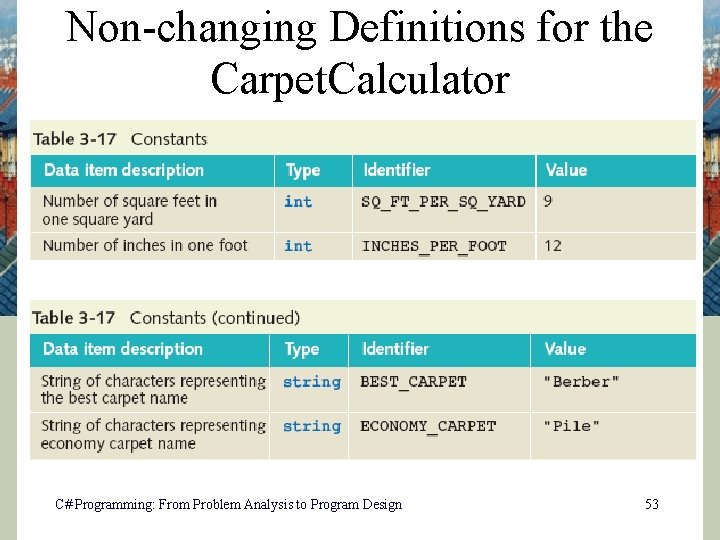
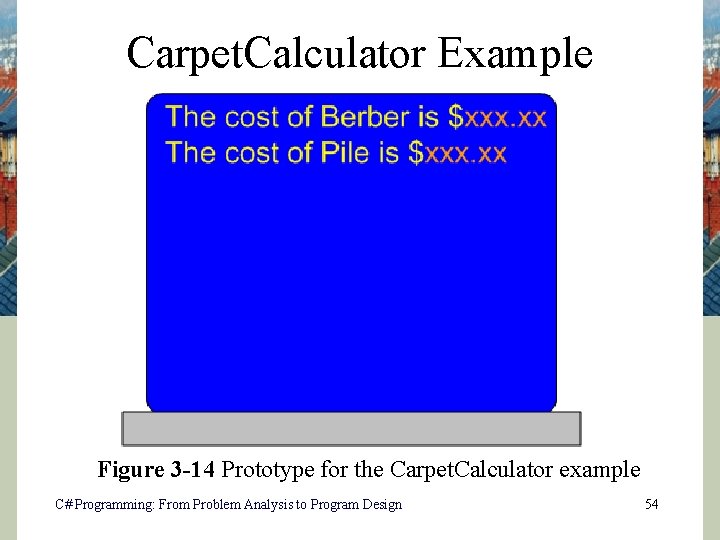
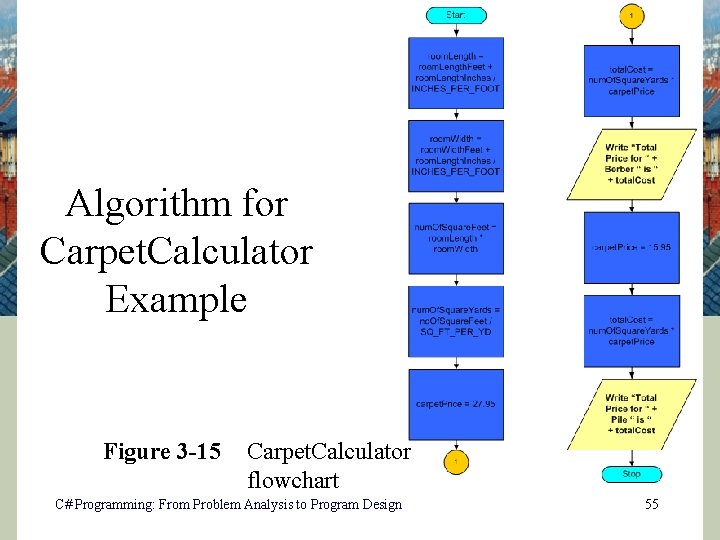
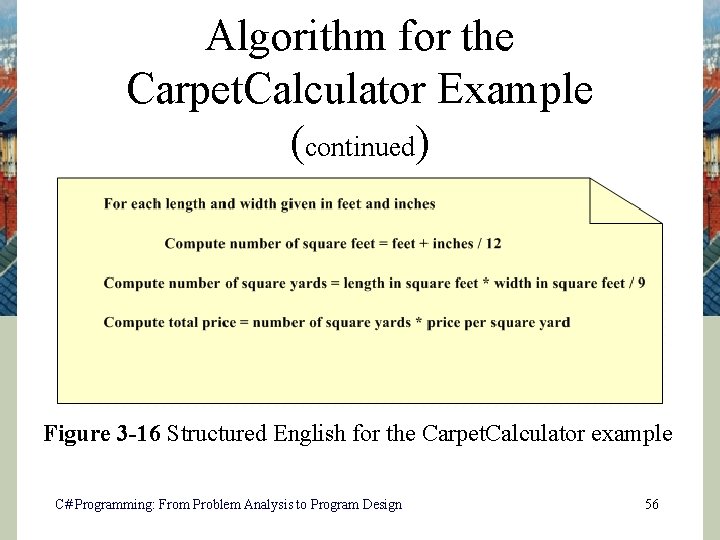
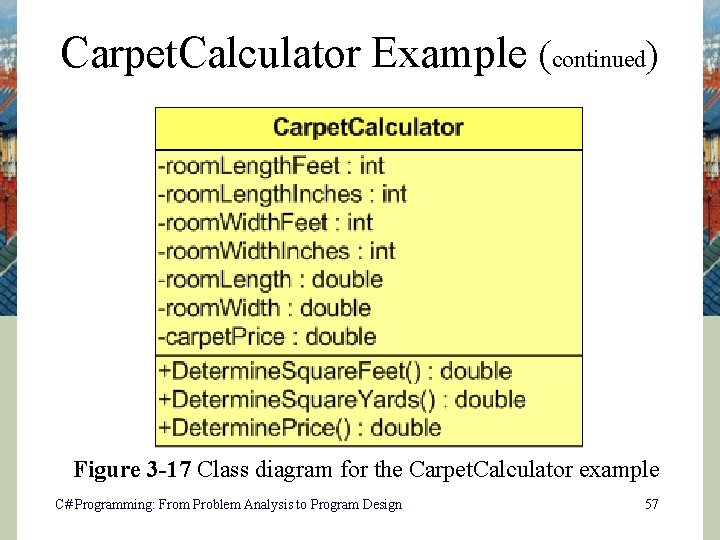
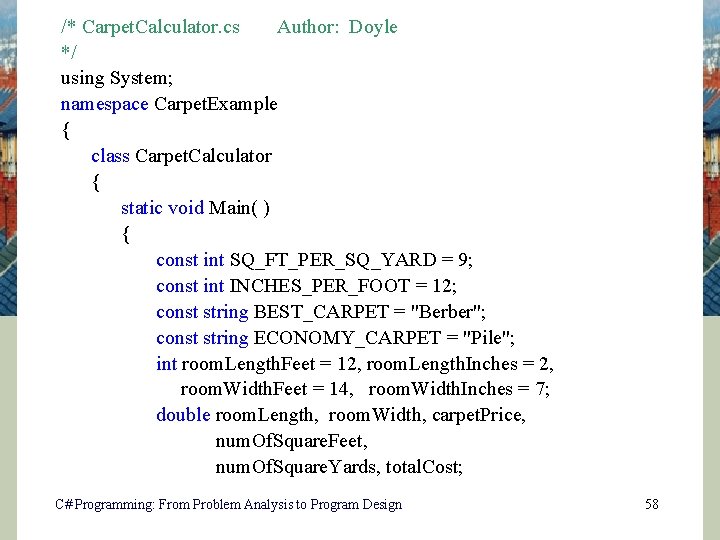
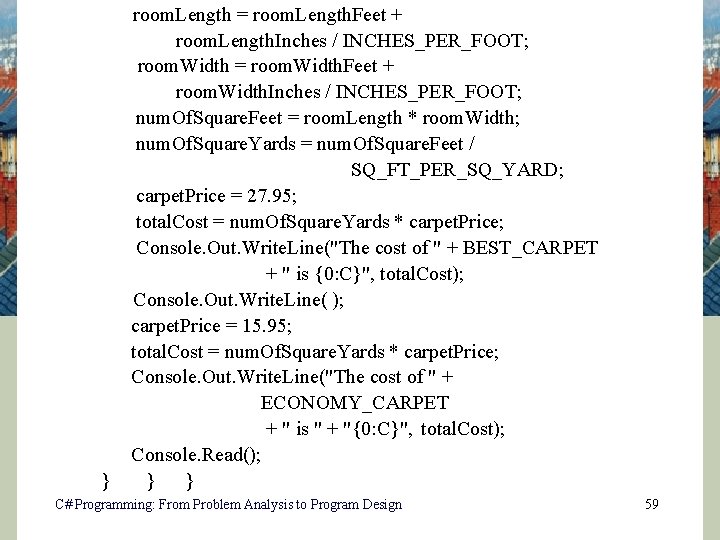
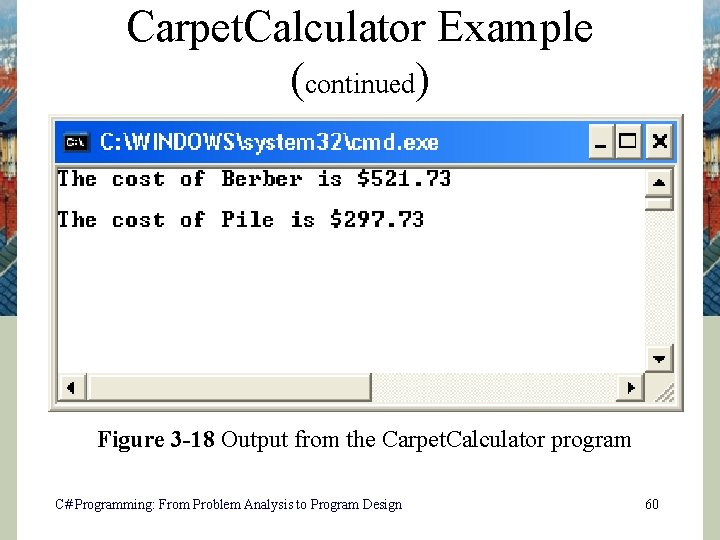
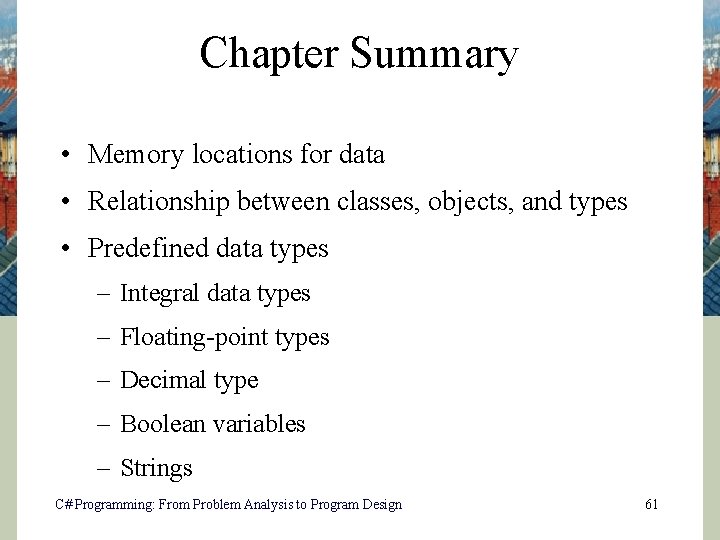
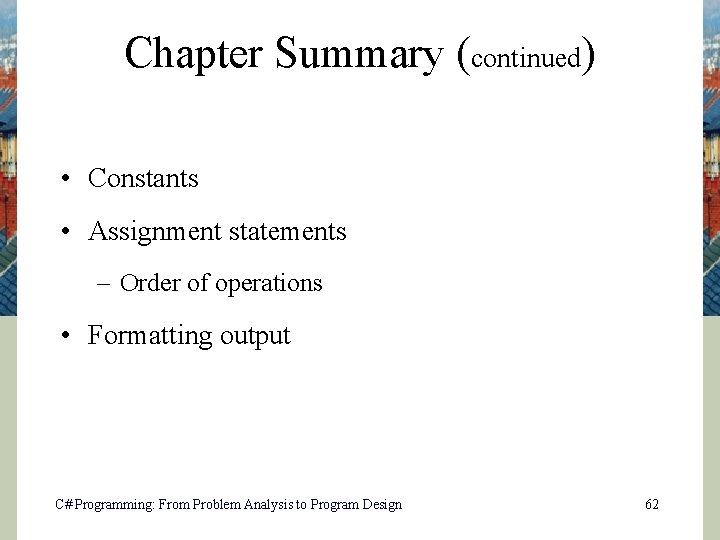
- Slides: 62
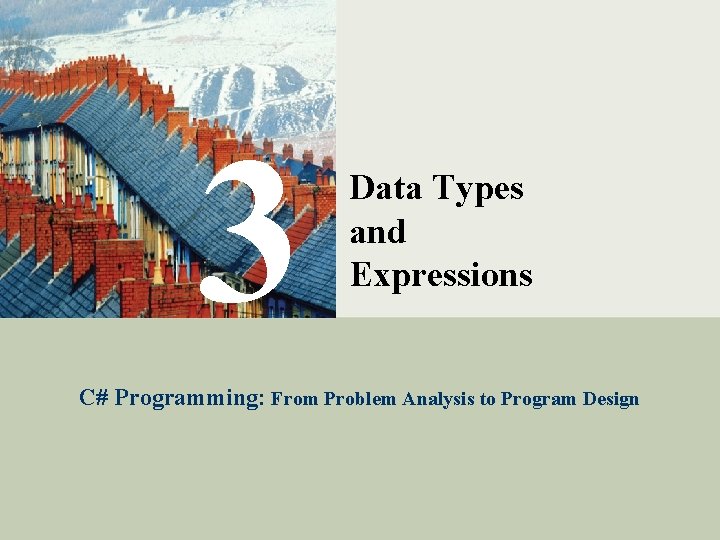
3 Data Types and Expressions C# Programming: From Problem Analysis to Program Design 1
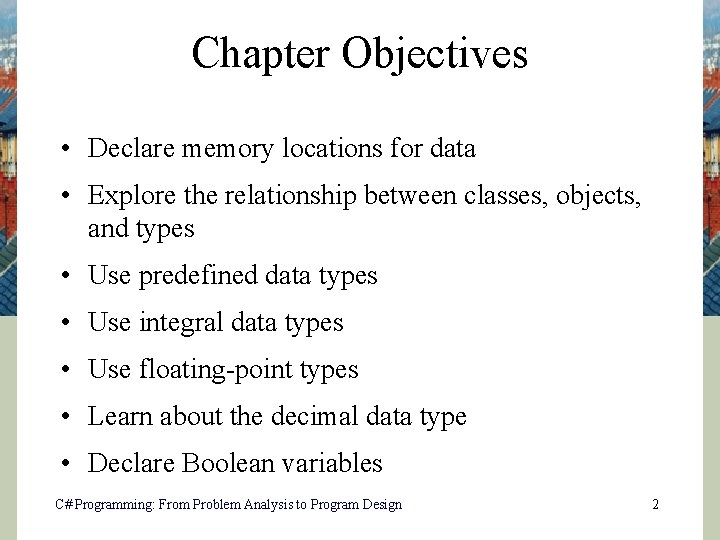
Chapter Objectives • Declare memory locations for data • Explore the relationship between classes, objects, and types • Use predefined data types • Use integral data types • Use floating-point types • Learn about the decimal data type • Declare Boolean variables C# Programming: From Problem Analysis to Program Design 2
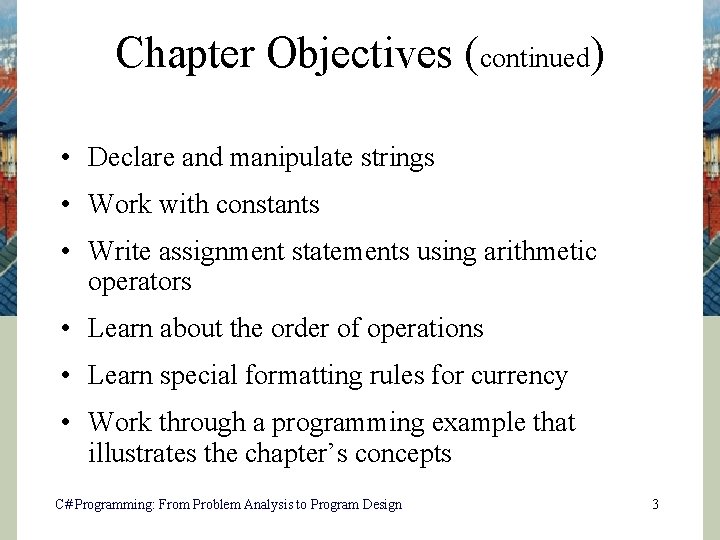
Chapter Objectives (continued) • Declare and manipulate strings • Work with constants • Write assignment statements using arithmetic operators • Learn about the order of operations • Learn special formatting rules for currency • Work through a programming example that illustrates the chapter’s concepts C# Programming: From Problem Analysis to Program Design 3
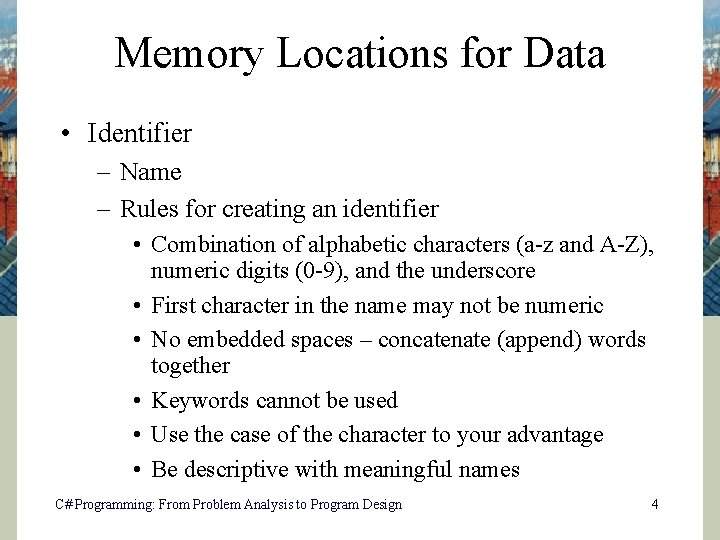
Memory Locations for Data • Identifier – Name – Rules for creating an identifier • Combination of alphabetic characters (a-z and A-Z), numeric digits (0 -9), and the underscore • First character in the name may not be numeric • No embedded spaces – concatenate (append) words together • Keywords cannot be used • Use the case of the character to your advantage • Be descriptive with meaningful names C# Programming: From Problem Analysis to Program Design 4
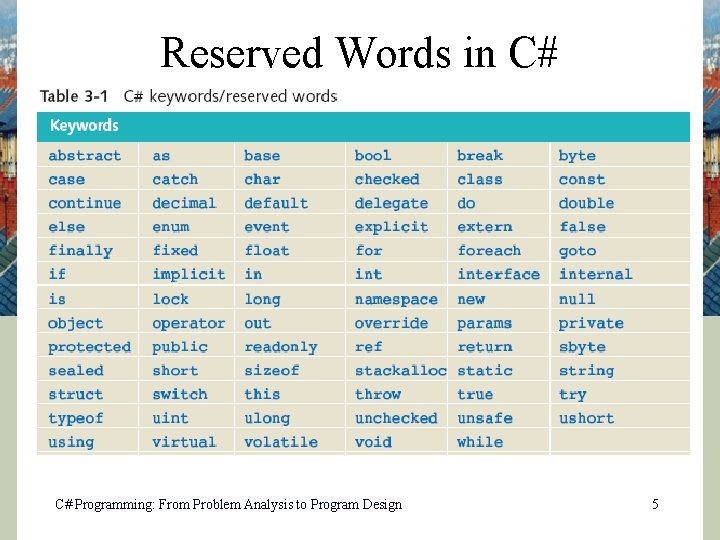
Reserved Words in C# C# Programming: From Problem Analysis to Program Design 5
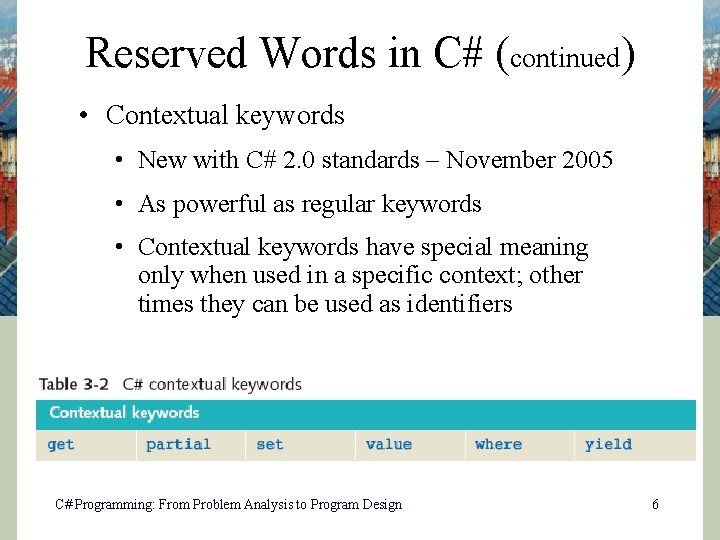
Reserved Words in C# (continued) • Contextual keywords • New with C# 2. 0 standards – November 2005 • As powerful as regular keywords • Contextual keywords have special meaning only when used in a specific context; other times they can be used as identifiers C# Programming: From Problem Analysis to Program Design 6
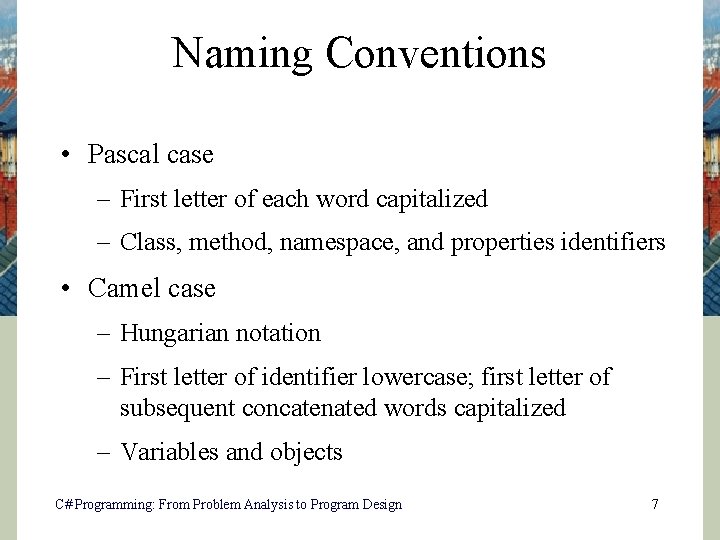
Naming Conventions • Pascal case – First letter of each word capitalized – Class, method, namespace, and properties identifiers • Camel case – Hungarian notation – First letter of identifier lowercase; first letter of subsequent concatenated words capitalized – Variables and objects C# Programming: From Problem Analysis to Program Design 7
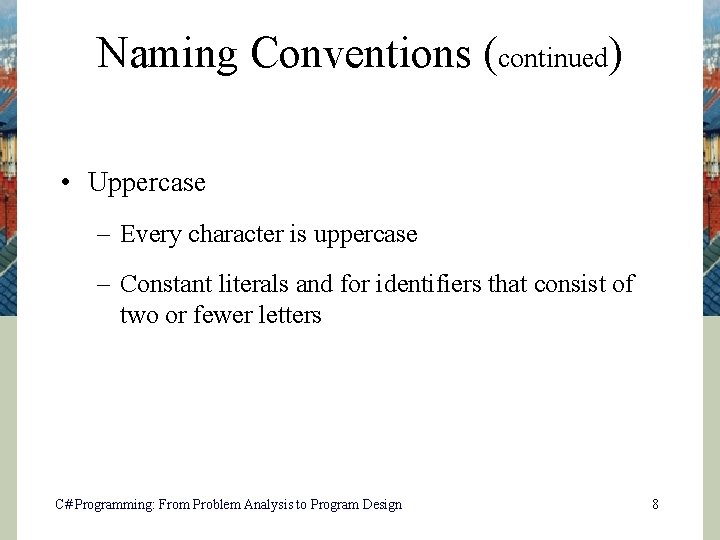
Naming Conventions (continued) • Uppercase – Every character is uppercase – Constant literals and for identifiers that consist of two or fewer letters C# Programming: From Problem Analysis to Program Design 8
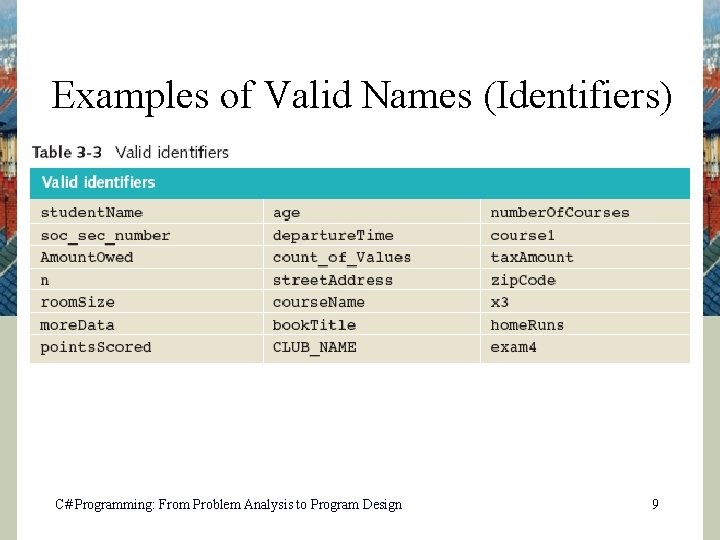
Examples of Valid Names (Identifiers) C# Programming: From Problem Analysis to Program Design 9
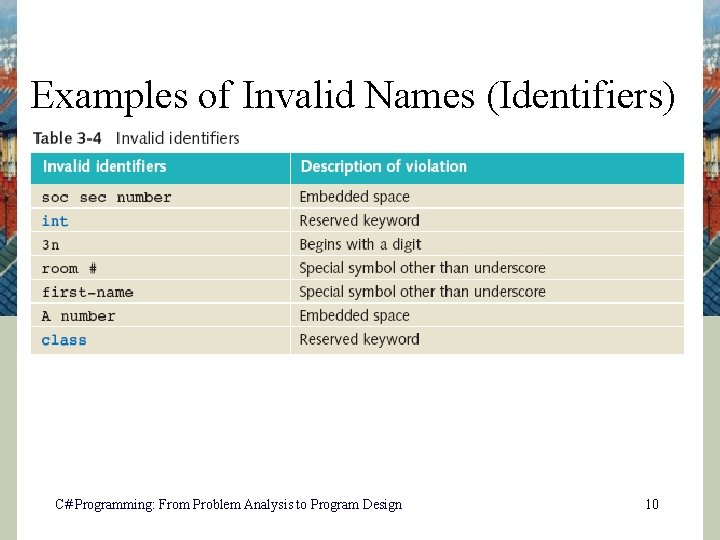
Examples of Invalid Names (Identifiers) C# Programming: From Problem Analysis to Program Design 10
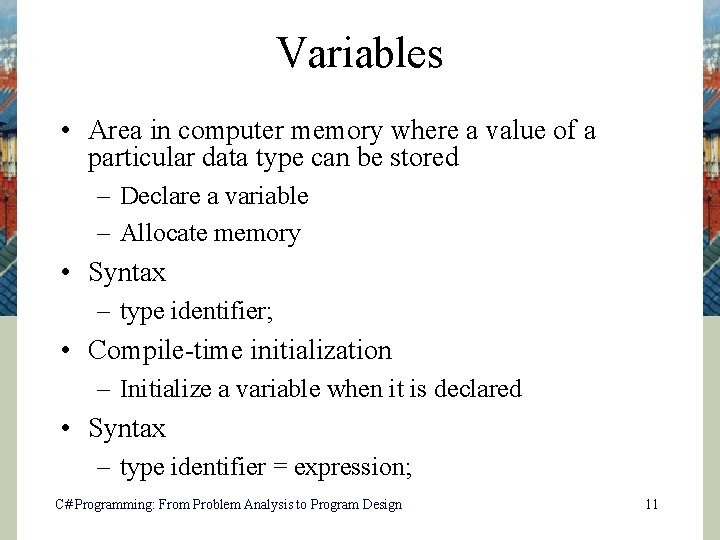
Variables • Area in computer memory where a value of a particular data type can be stored – Declare a variable – Allocate memory • Syntax – type identifier; • Compile-time initialization – Initialize a variable when it is declared • Syntax – type identifier = expression; C# Programming: From Problem Analysis to Program Design 11
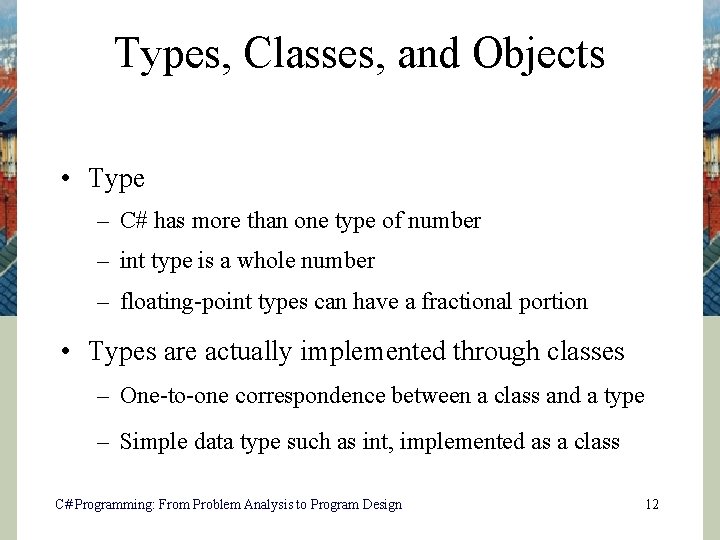
Types, Classes, and Objects • Type – C# has more than one type of number – int type is a whole number – floating-point types can have a fractional portion • Types are actually implemented through classes – One-to-one correspondence between a class and a type – Simple data type such as int, implemented as a class C# Programming: From Problem Analysis to Program Design 12
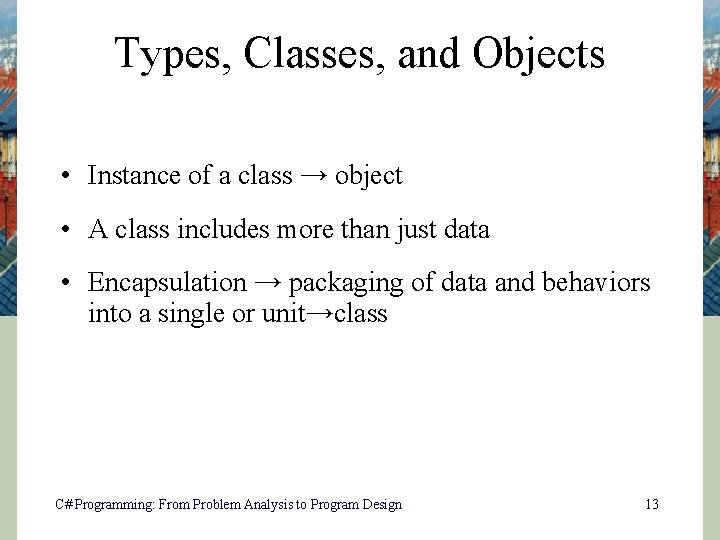
Types, Classes, and Objects • Instance of a class → object • A class includes more than just data • Encapsulation → packaging of data and behaviors into a single or unit→class C# Programming: From Problem Analysis to Program Design 13
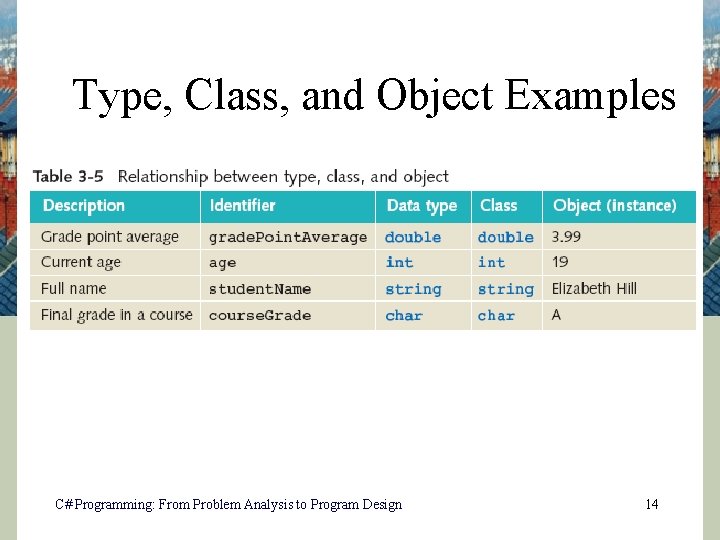
Type, Class, and Object Examples C# Programming: From Problem Analysis to Program Design 14
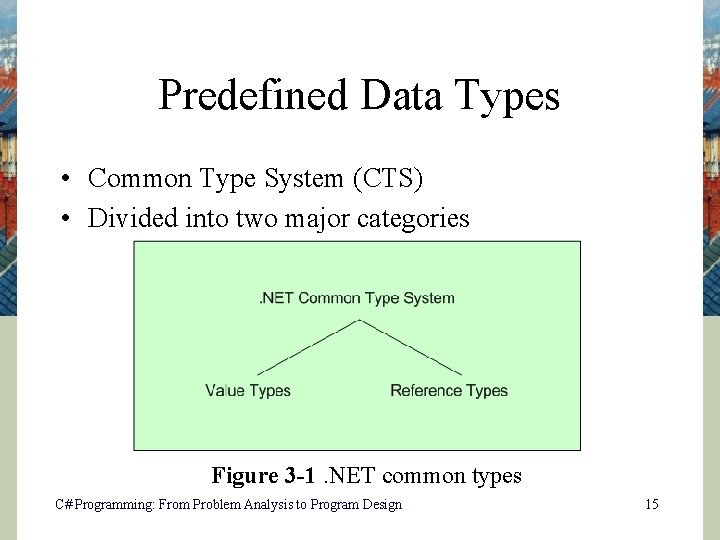
Predefined Data Types • Common Type System (CTS) • Divided into two major categories Figure 3 -1. NET common types C# Programming: From Problem Analysis to Program Design 15
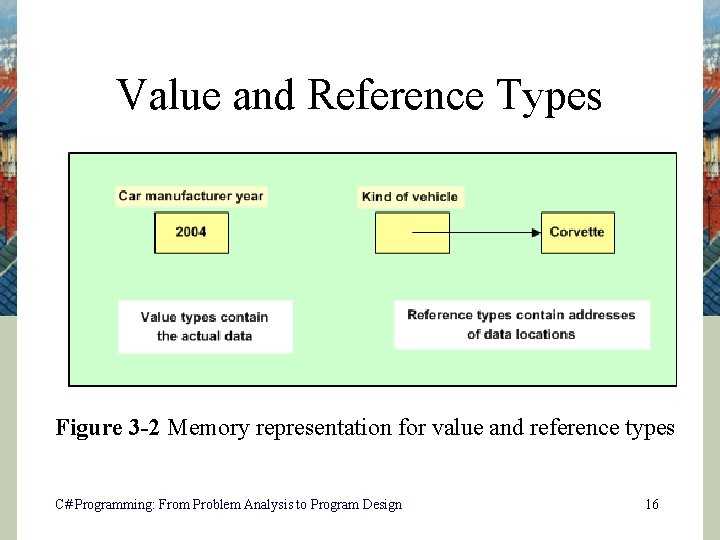
Value and Reference Types Figure 3 -2 Memory representation for value and reference types C# Programming: From Problem Analysis to Program Design 16
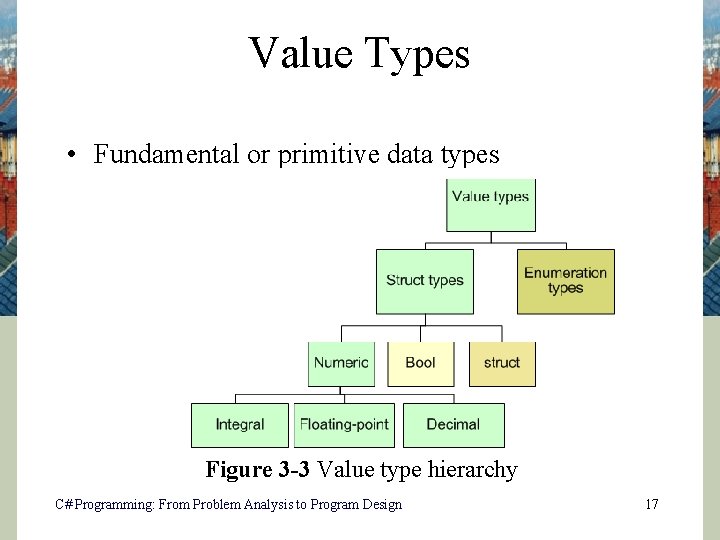
Value Types • Fundamental or primitive data types Figure 3 -3 Value type hierarchy C# Programming: From Problem Analysis to Program Design 17
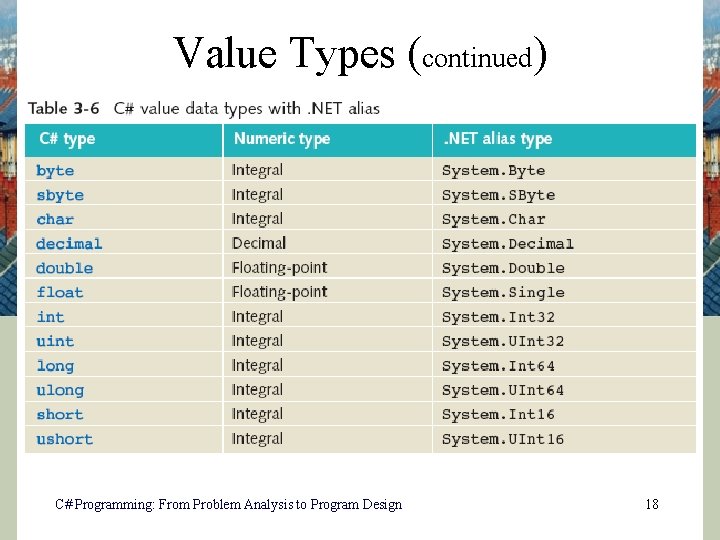
Value Types (continued) C# Programming: From Problem Analysis to Program Design 18
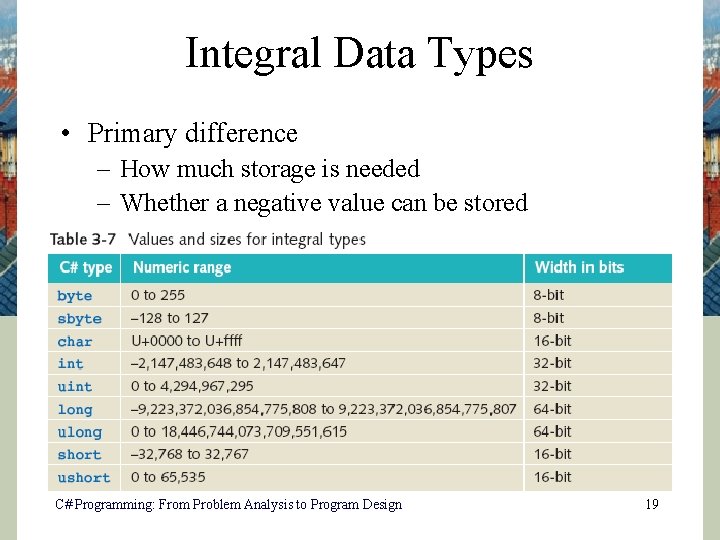
Integral Data Types • Primary difference – How much storage is needed – Whether a negative value can be stored C# Programming: From Problem Analysis to Program Design 19
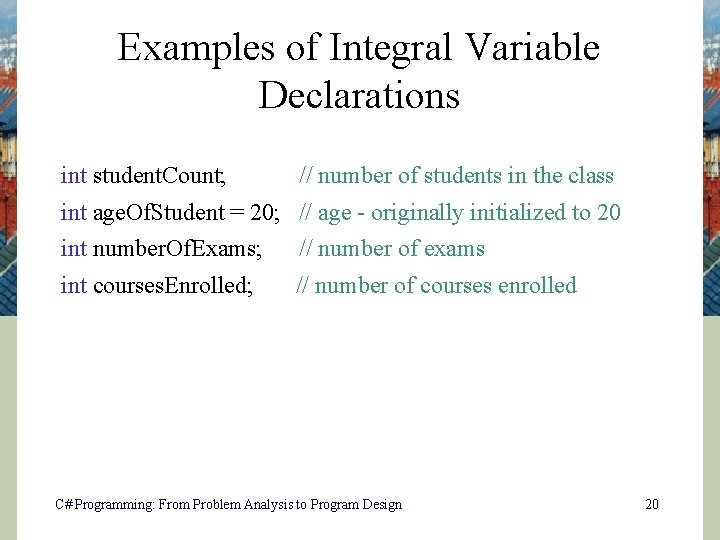
Examples of Integral Variable Declarations int student. Count; // number of students in the class int age. Of. Student = 20; // age - originally initialized to 20 int number. Of. Exams; // number of exams int courses. Enrolled; // number of courses enrolled C# Programming: From Problem Analysis to Program Design 20
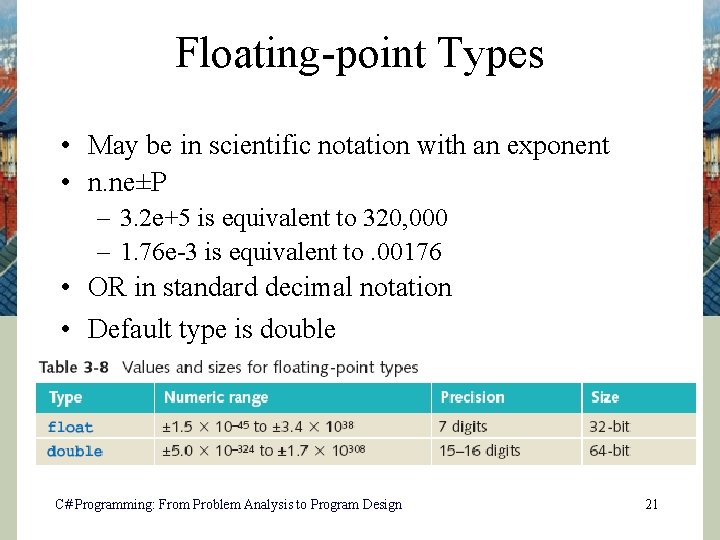
Floating-point Types • May be in scientific notation with an exponent • n. ne±P – 3. 2 e+5 is equivalent to 320, 000 – 1. 76 e-3 is equivalent to. 00176 • OR in standard decimal notation • Default type is double C# Programming: From Problem Analysis to Program Design 21
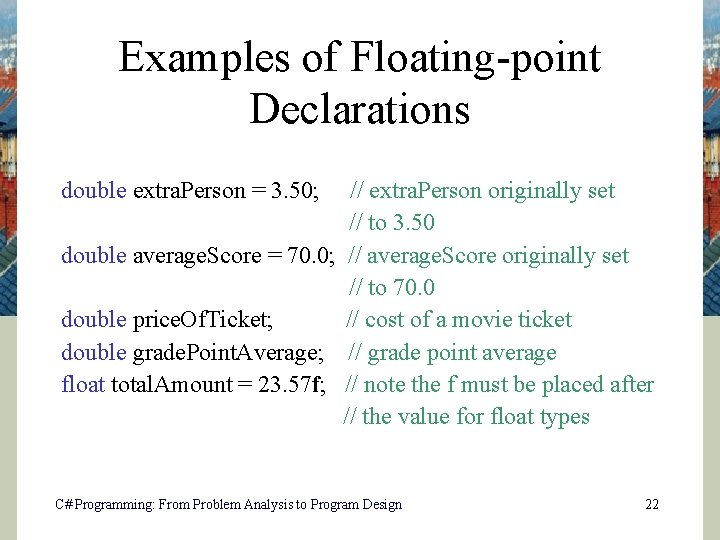
Examples of Floating-point Declarations double extra. Person = 3. 50; // extra. Person originally set // to 3. 50 double average. Score = 70. 0; // average. Score originally set // to 70. 0 double price. Of. Ticket; // cost of a movie ticket double grade. Point. Average; // grade point average float total. Amount = 23. 57 f; // note the f must be placed after // the value for float types C# Programming: From Problem Analysis to Program Design 22
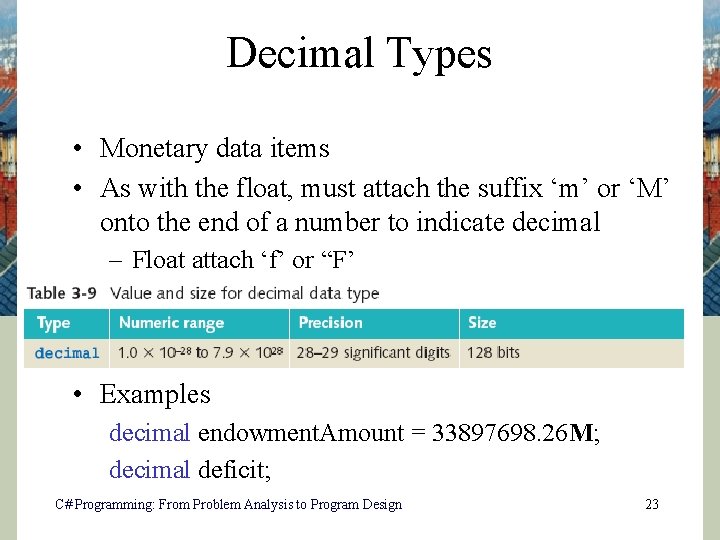
Decimal Types • Monetary data items • As with the float, must attach the suffix ‘m’ or ‘M’ onto the end of a number to indicate decimal – Float attach ‘f’ or “F’ • Examples decimal endowment. Amount = 33897698. 26 M; decimal deficit; C# Programming: From Problem Analysis to Program Design 23
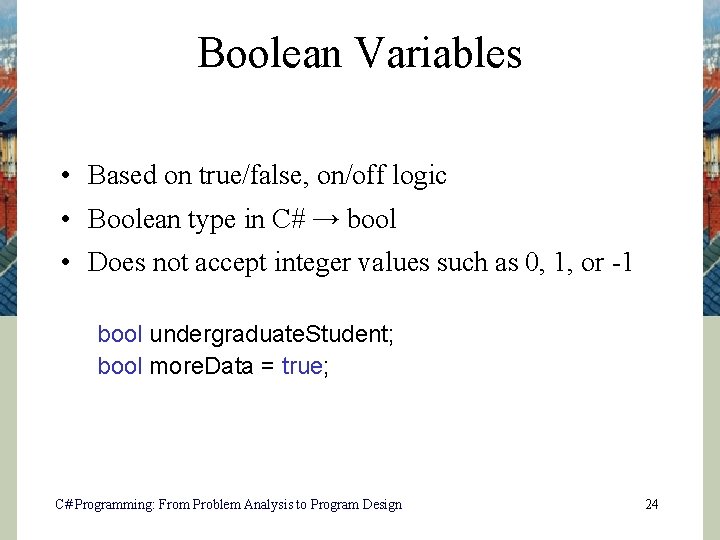
Boolean Variables • Based on true/false, on/off logic • Boolean type in C# → bool • Does not accept integer values such as 0, 1, or -1 bool undergraduate. Student; bool more. Data = true; C# Programming: From Problem Analysis to Program Design 24
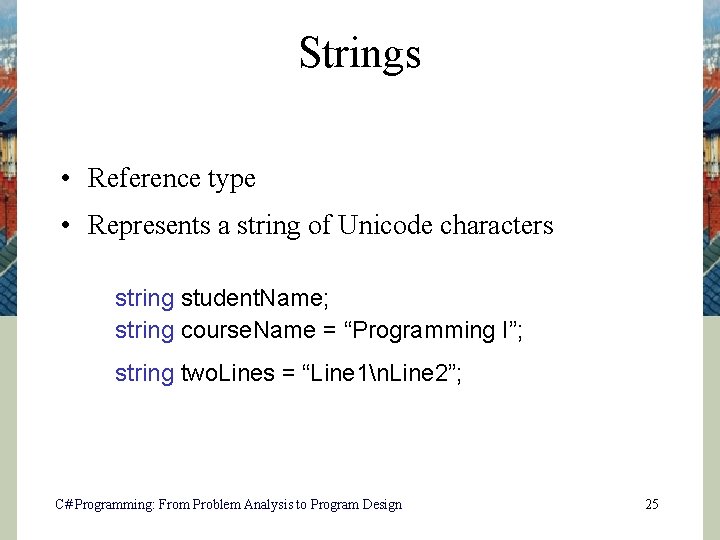
Strings • Reference type • Represents a string of Unicode characters string student. Name; string course. Name = “Programming I”; string two. Lines = “Line 1n. Line 2”; C# Programming: From Problem Analysis to Program Design 25
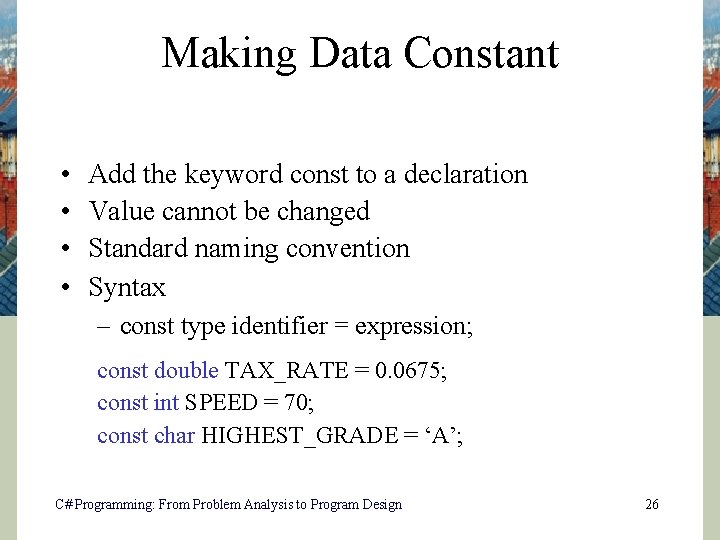
Making Data Constant • • Add the keyword const to a declaration Value cannot be changed Standard naming convention Syntax – const type identifier = expression; const double TAX_RATE = 0. 0675; const int SPEED = 70; const char HIGHEST_GRADE = ‘A’; C# Programming: From Problem Analysis to Program Design 26
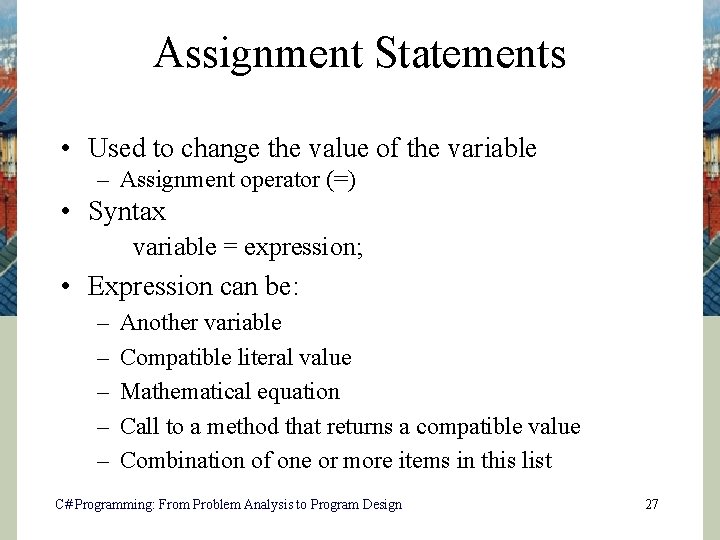
Assignment Statements • Used to change the value of the variable – Assignment operator (=) • Syntax variable = expression; • Expression can be: – – – Another variable Compatible literal value Mathematical equation Call to a method that returns a compatible value Combination of one or more items in this list C# Programming: From Problem Analysis to Program Design 27
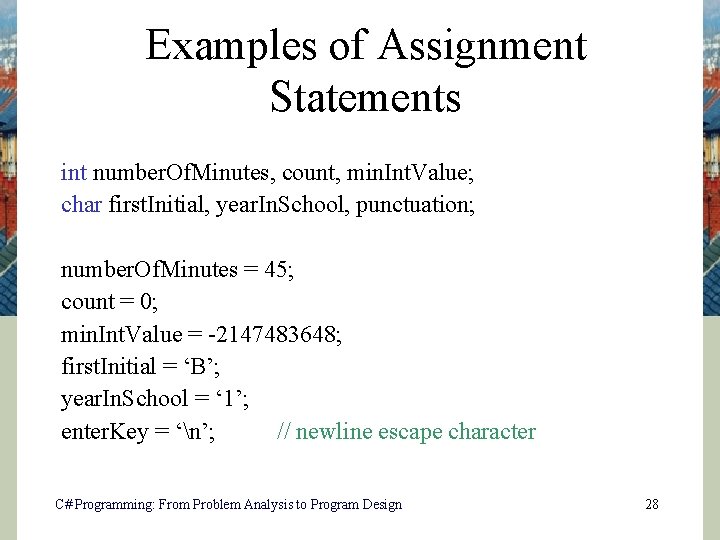
Examples of Assignment Statements int number. Of. Minutes, count, min. Int. Value; char first. Initial, year. In. School, punctuation; number. Of. Minutes = 45; count = 0; min. Int. Value = -2147483648; first. Initial = ‘B’; year. In. School = ‘ 1’; enter. Key = ‘n’; // newline escape character C# Programming: From Problem Analysis to Program Design 28
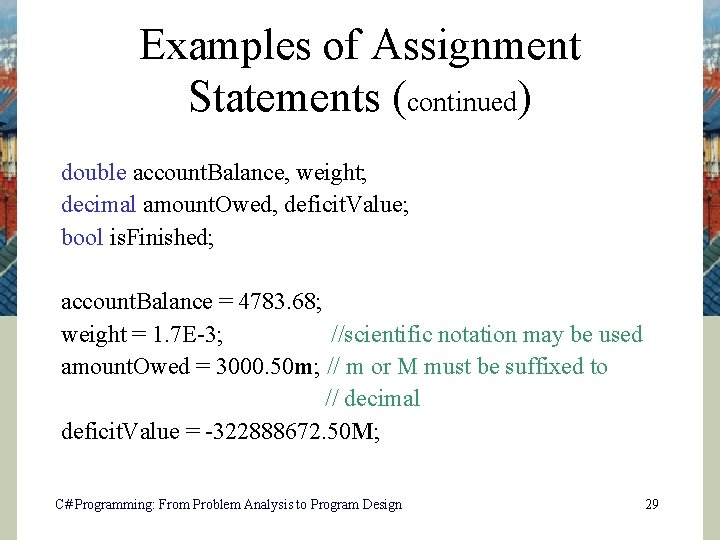
Examples of Assignment Statements (continued) double account. Balance, weight; decimal amount. Owed, deficit. Value; bool is. Finished; account. Balance = 4783. 68; weight = 1. 7 E-3; //scientific notation may be used amount. Owed = 3000. 50 m; // m or M must be suffixed to // decimal deficit. Value = -322888672. 50 M; C# Programming: From Problem Analysis to Program Design 29
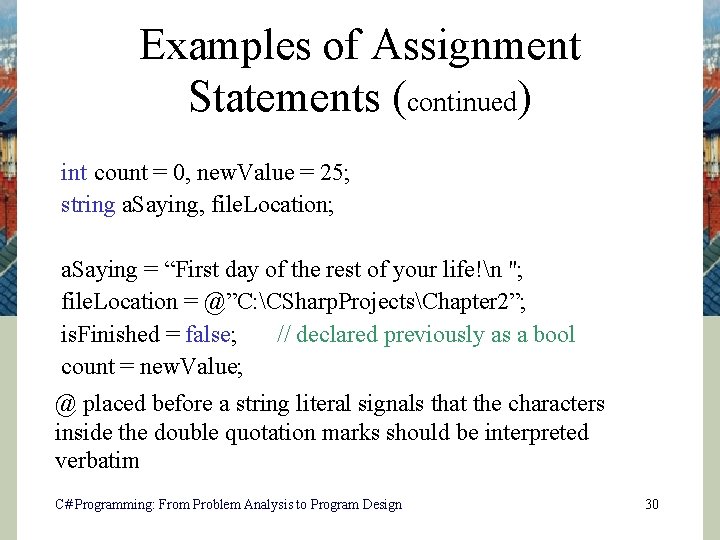
Examples of Assignment Statements (continued) int count = 0, new. Value = 25; string a. Saying, file. Location; a. Saying = “First day of the rest of your life!n "; file. Location = @”C: CSharp. ProjectsChapter 2”; is. Finished = false; // declared previously as a bool count = new. Value; @ placed before a string literal signals that the characters inside the double quotation marks should be interpreted verbatim C# Programming: From Problem Analysis to Program Design 30
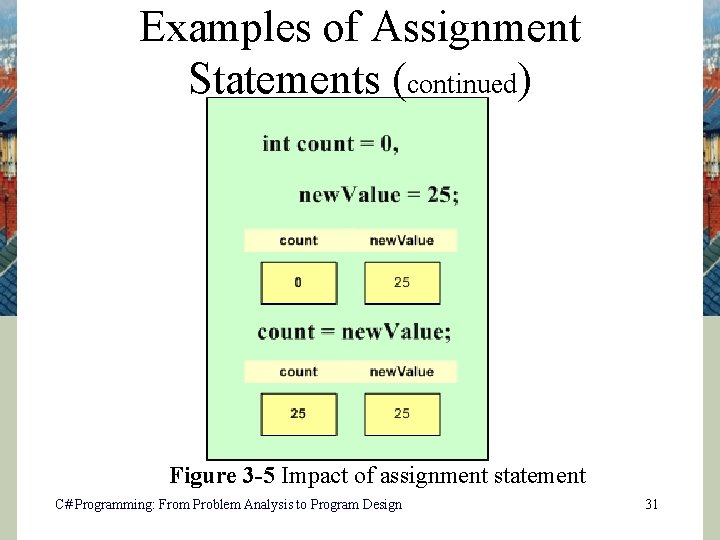
Examples of Assignment Statements (continued) Figure 3 -5 Impact of assignment statement C# Programming: From Problem Analysis to Program Design 31
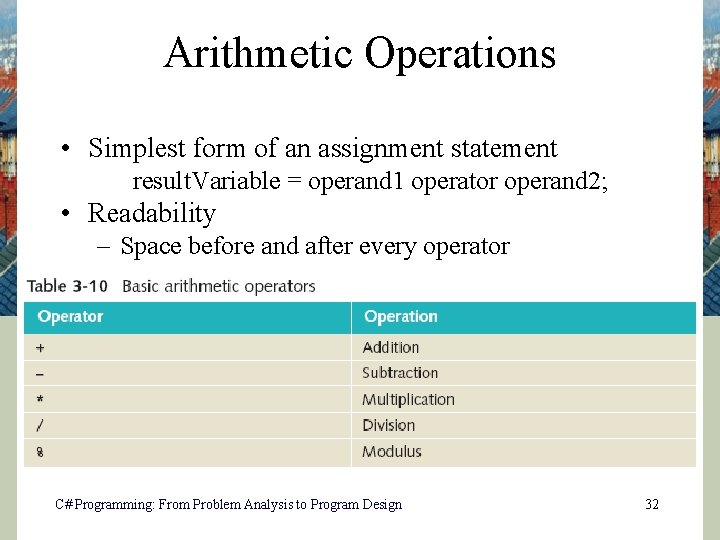
Arithmetic Operations • Simplest form of an assignment statement result. Variable = operand 1 operator operand 2; • Readability – Space before and after every operator C# Programming: From Problem Analysis to Program Design 32
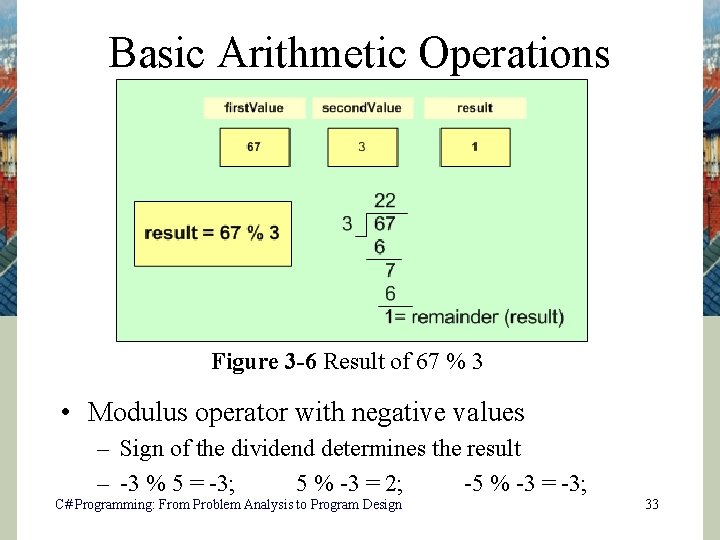
Basic Arithmetic Operations Figure 3 -6 Result of 67 % 3 • Modulus operator with negative values – Sign of the dividend determines the result – -3 % 5 = -3; 5 % -3 = 2; -5 % -3 = -3; C# Programming: From Problem Analysis to Program Design 33
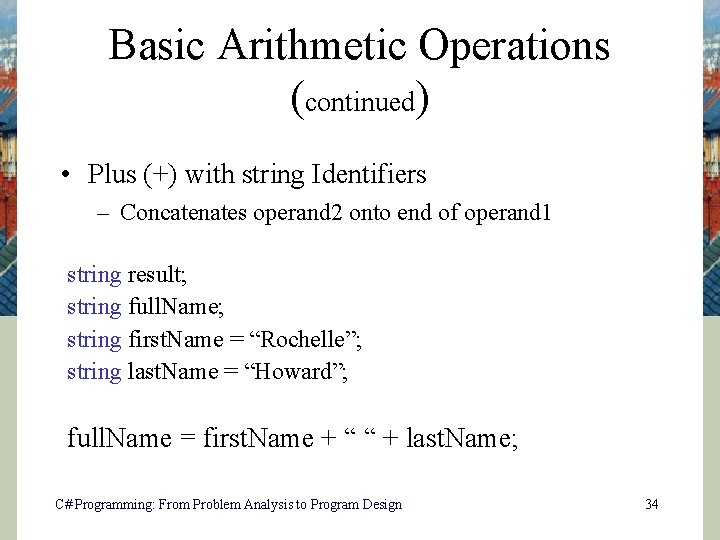
Basic Arithmetic Operations (continued) • Plus (+) with string Identifiers – Concatenates operand 2 onto end of operand 1 string result; string full. Name; string first. Name = “Rochelle”; string last. Name = “Howard”; full. Name = first. Name + “ “ + last. Name; C# Programming: From Problem Analysis to Program Design 34
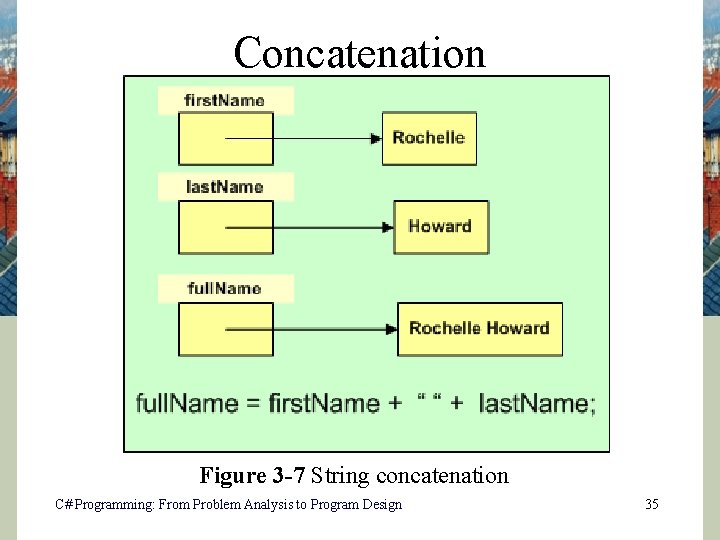
Concatenation Figure 3 -7 String concatenation C# Programming: From Problem Analysis to Program Design 35
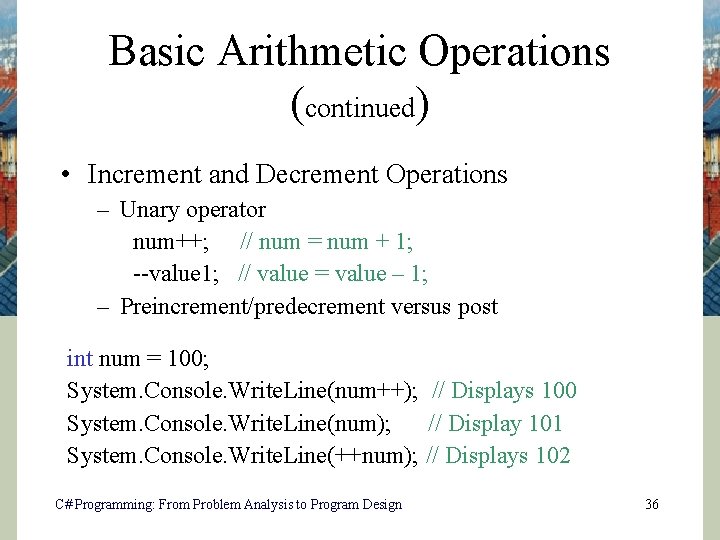
Basic Arithmetic Operations (continued) • Increment and Decrement Operations – Unary operator num++; // num = num + 1; --value 1; // value = value – 1; – Preincrement/predecrement versus post int num = 100; System. Console. Write. Line(num++); // Displays 100 System. Console. Write. Line(num); // Display 101 System. Console. Write. Line(++num); // Displays 102 C# Programming: From Problem Analysis to Program Design 36
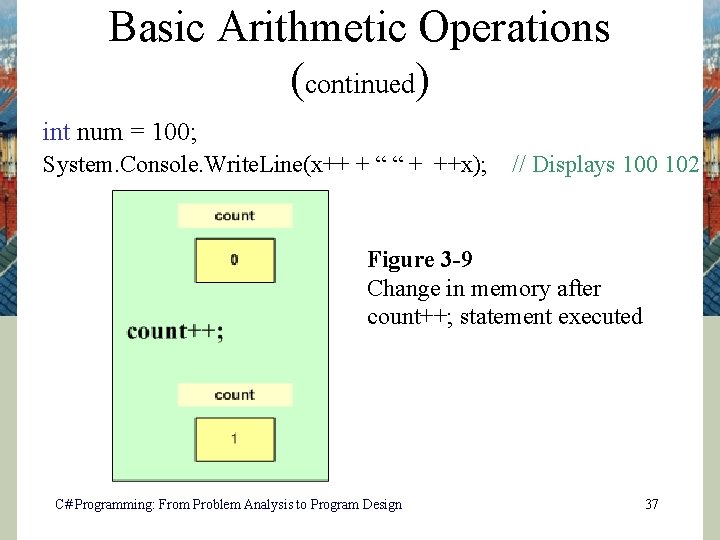
Basic Arithmetic Operations (continued) int num = 100; System. Console. Write. Line(x++ + “ “ + ++x); // Displays 100 102 Figure 3 -9 Change in memory after count++; statement executed C# Programming: From Problem Analysis to Program Design 37
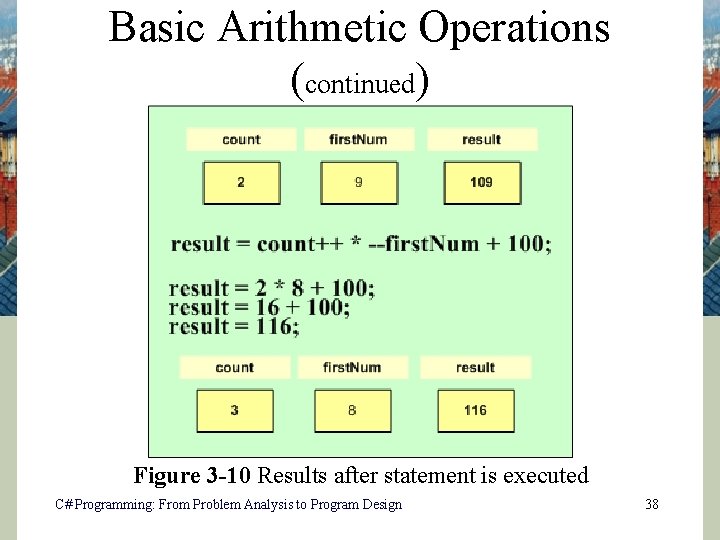
Basic Arithmetic Operations (continued) Figure 3 -10 Results after statement is executed C# Programming: From Problem Analysis to Program Design 38
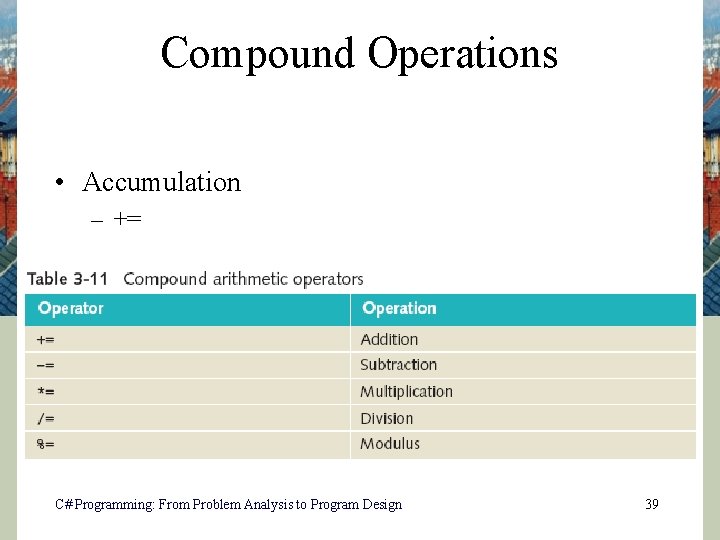
Compound Operations • Accumulation – += C# Programming: From Problem Analysis to Program Design 39
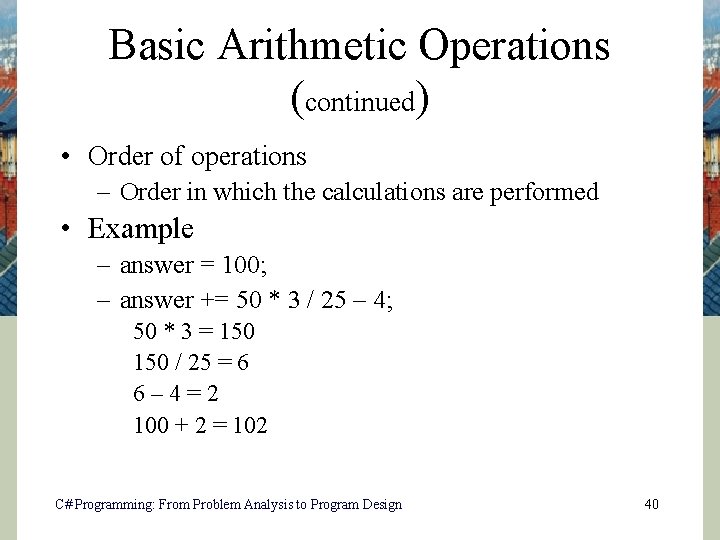
Basic Arithmetic Operations (continued) • Order of operations – Order in which the calculations are performed • Example – answer = 100; – answer += 50 * 3 / 25 – 4; 50 * 3 = 150 / 25 = 6 6– 4=2 100 + 2 = 102 C# Programming: From Problem Analysis to Program Design 40
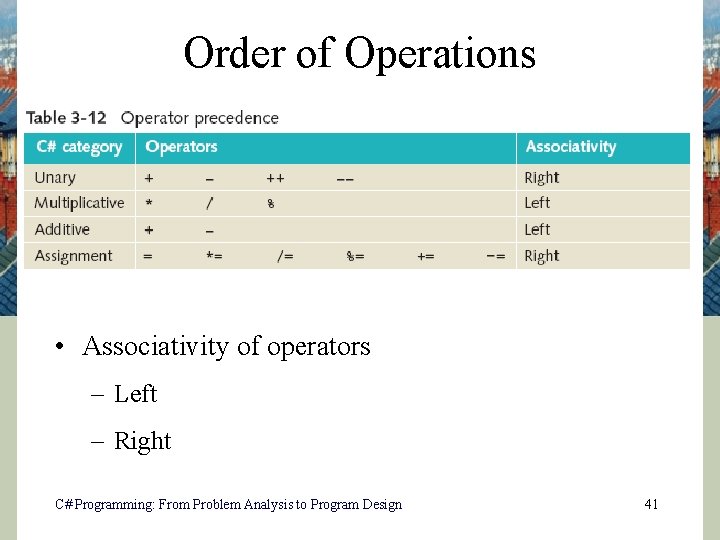
Order of Operations • Associativity of operators – Left – Right C# Programming: From Problem Analysis to Program Design 41
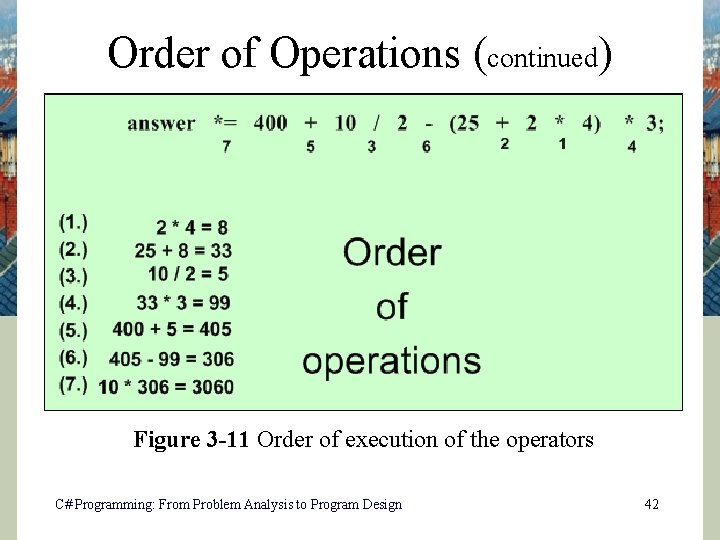
Order of Operations (continued) Figure 3 -11 Order of execution of the operators C# Programming: From Problem Analysis to Program Design 42
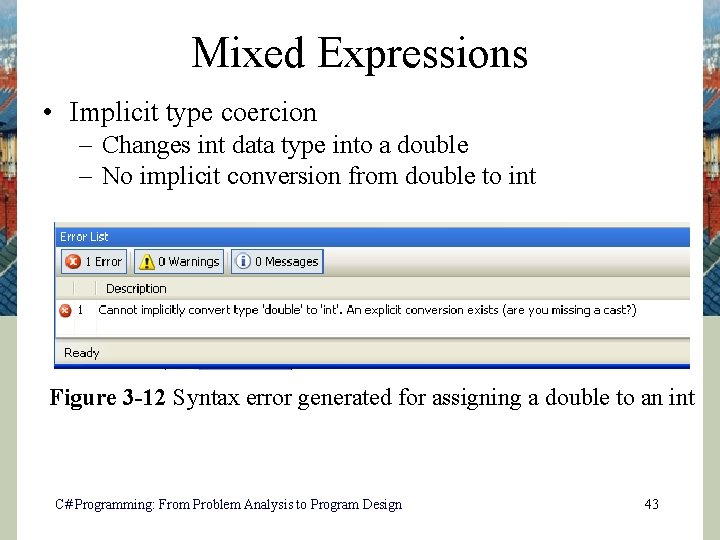
Mixed Expressions • Implicit type coercion – Changes int data type into a double – No implicit conversion from double to int Figure 3 -12 Syntax error generated for assigning a double to an int C# Programming: From Problem Analysis to Program Design 43
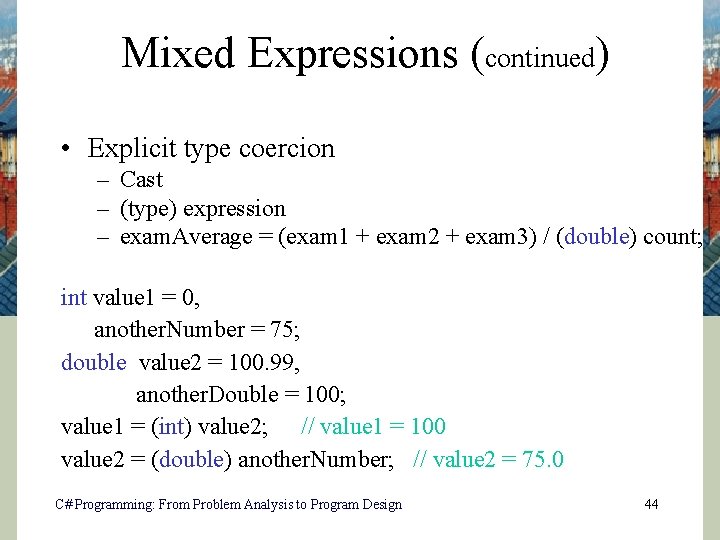
Mixed Expressions (continued) • Explicit type coercion – Cast – (type) expression – exam. Average = (exam 1 + exam 2 + exam 3) / (double) count; int value 1 = 0, another. Number = 75; double value 2 = 100. 99, another. Double = 100; value 1 = (int) value 2; // value 1 = 100 value 2 = (double) another. Number; // value 2 = 75. 0 C# Programming: From Problem Analysis to Program Design 44
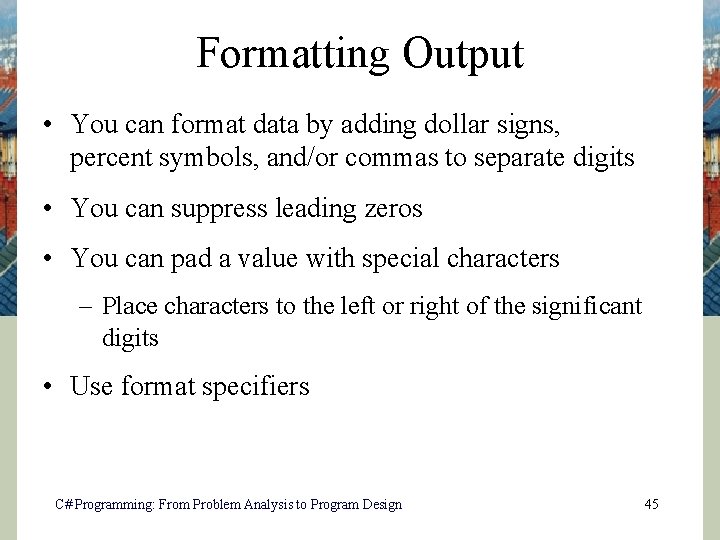
Formatting Output • You can format data by adding dollar signs, percent symbols, and/or commas to separate digits • You can suppress leading zeros • You can pad a value with special characters – Place characters to the left or right of the significant digits • Use format specifiers C# Programming: From Problem Analysis to Program Design 45
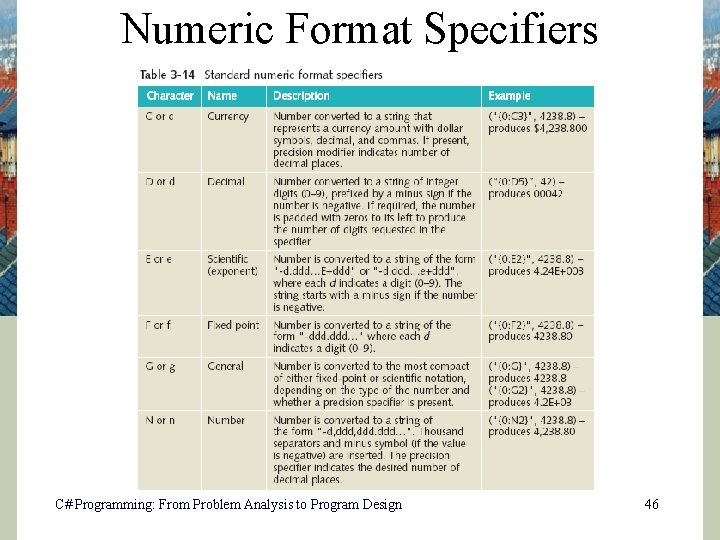
Numeric Format Specifiers C# Programming: From Problem Analysis to Program Design 46
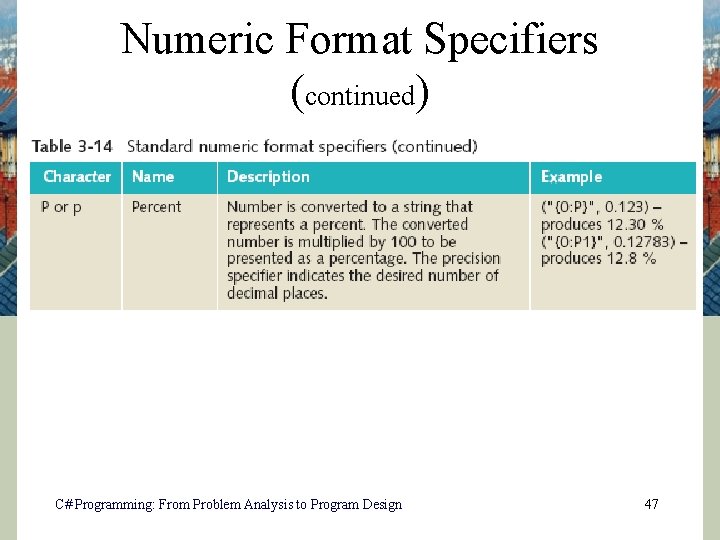
Numeric Format Specifiers (continued) C# Programming: From Problem Analysis to Program Design 47
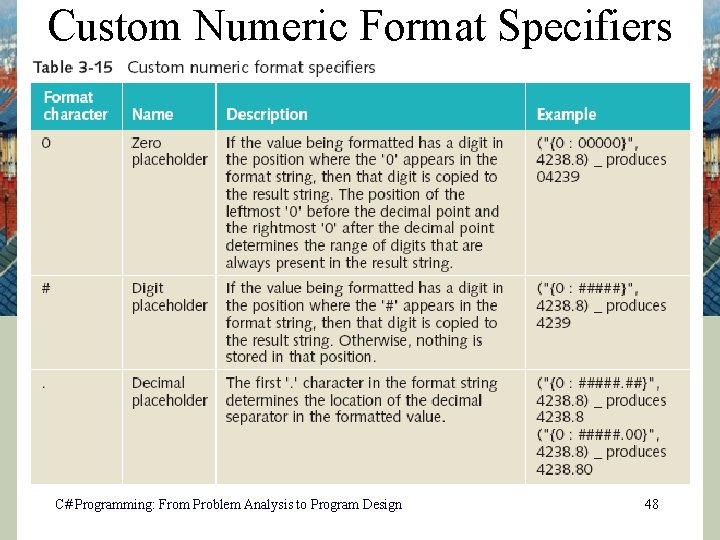
Custom Numeric Format Specifiers C# Programming: From Problem Analysis to Program Design 48
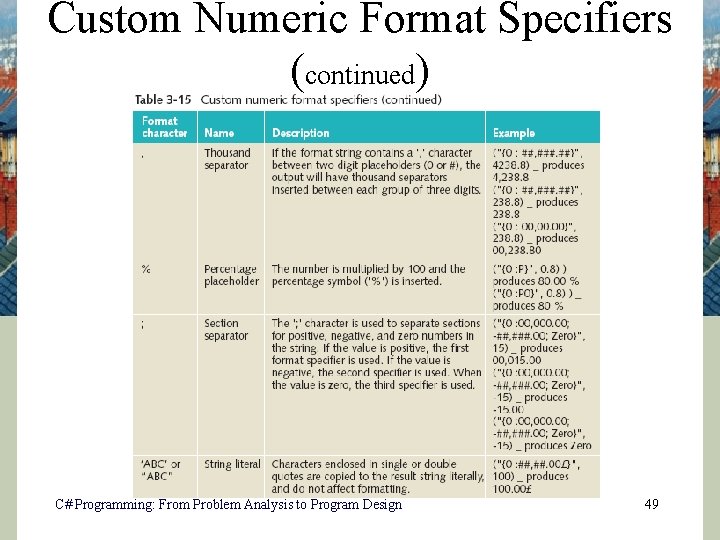
Custom Numeric Format Specifiers (continued) C# Programming: From Problem Analysis to Program Design 49
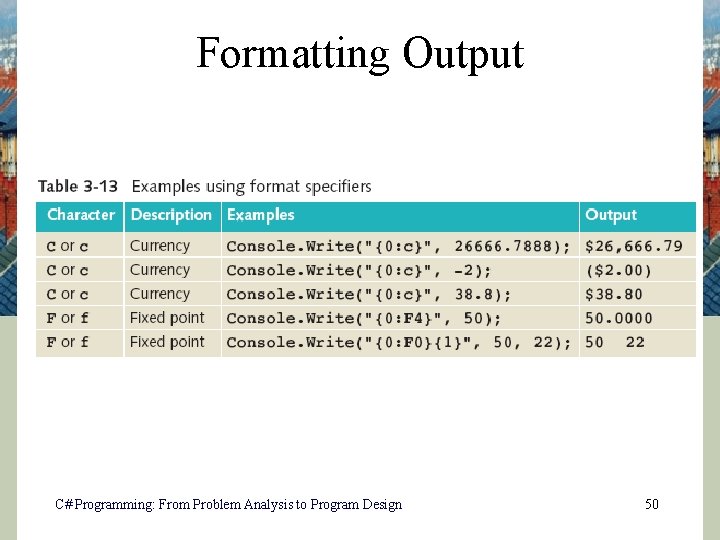
Formatting Output C# Programming: From Problem Analysis to Program Design 50
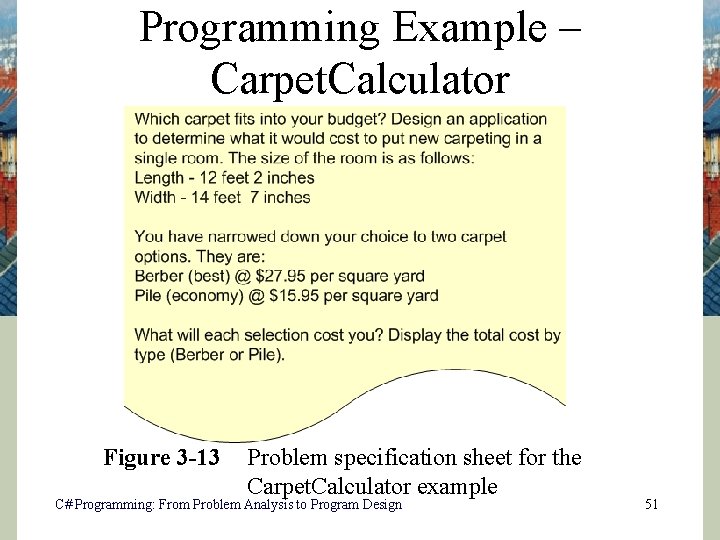
Programming Example – Carpet. Calculator Figure 3 -13 Problem specification sheet for the Carpet. Calculator example C# Programming: From Problem Analysis to Program Design 51
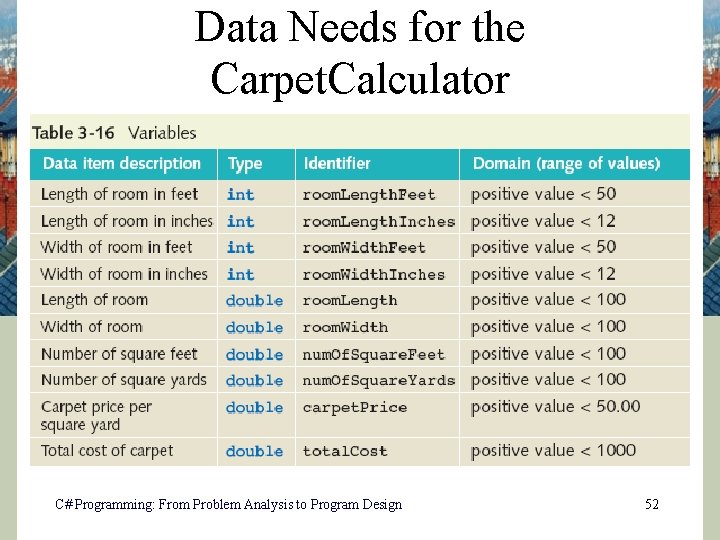
Data Needs for the Carpet. Calculator C# Programming: From Problem Analysis to Program Design 52
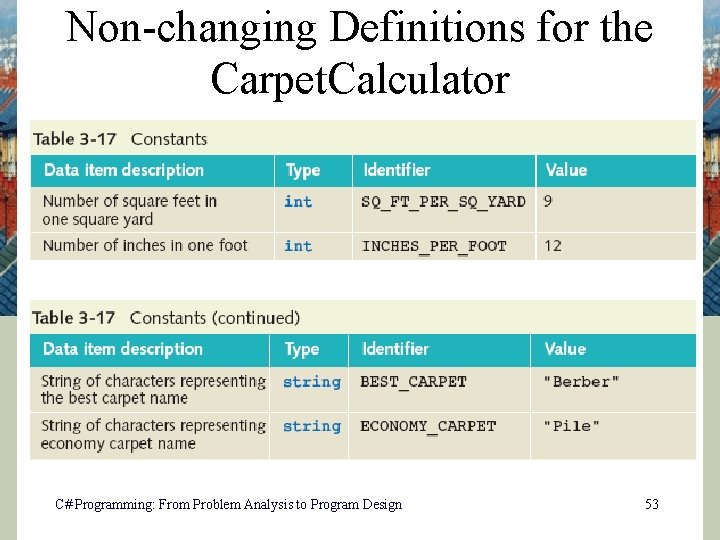
Non-changing Definitions for the Carpet. Calculator C# Programming: From Problem Analysis to Program Design 53
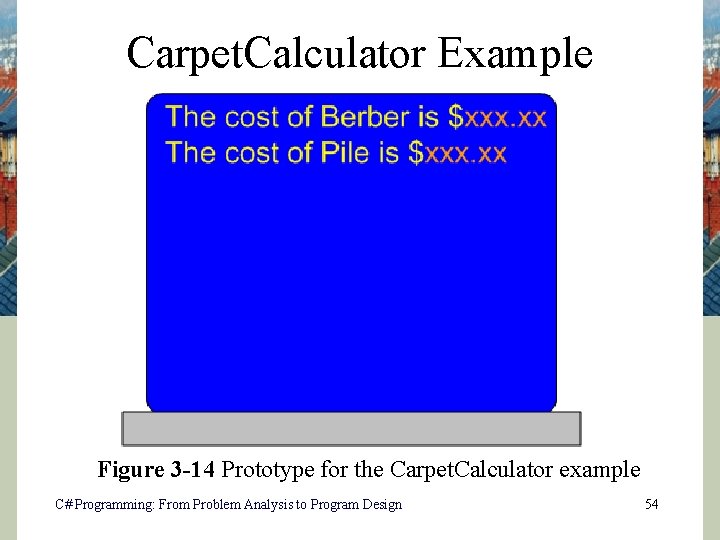
Carpet. Calculator Example Figure 3 -14 Prototype for the Carpet. Calculator example C# Programming: From Problem Analysis to Program Design 54
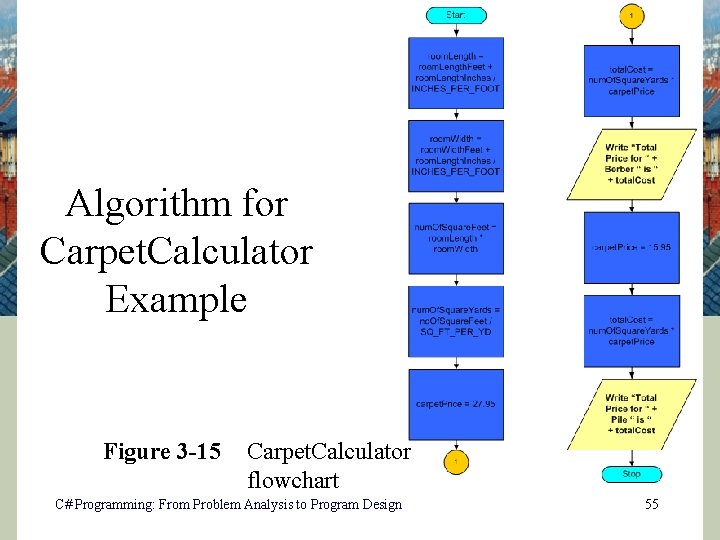
Algorithm for Carpet. Calculator Example Figure 3 -15 Carpet. Calculator flowchart C# Programming: From Problem Analysis to Program Design 55
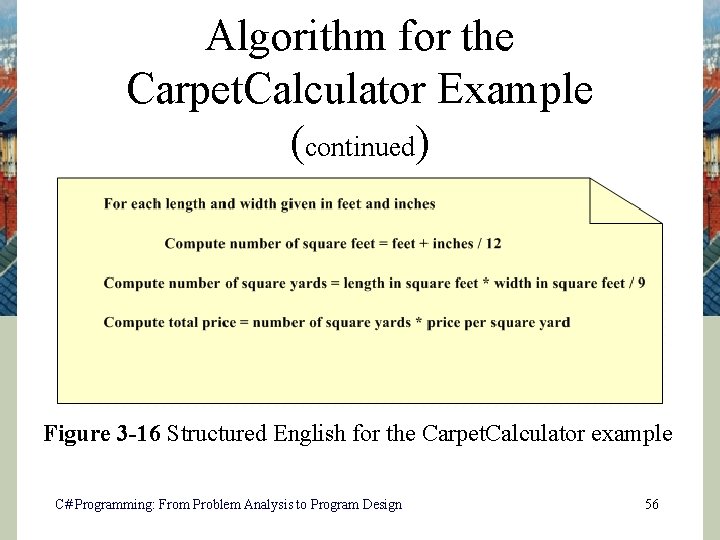
Algorithm for the Carpet. Calculator Example (continued) Figure 3 -16 Structured English for the Carpet. Calculator example C# Programming: From Problem Analysis to Program Design 56
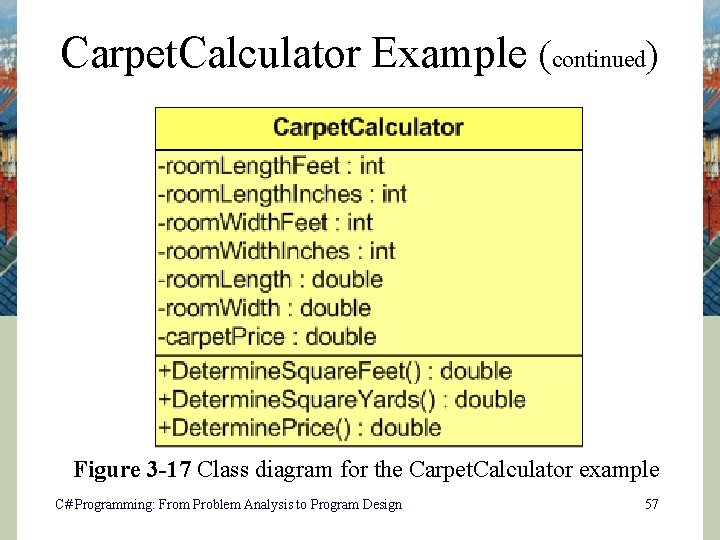
Carpet. Calculator Example (continued) Figure 3 -17 Class diagram for the Carpet. Calculator example C# Programming: From Problem Analysis to Program Design 57
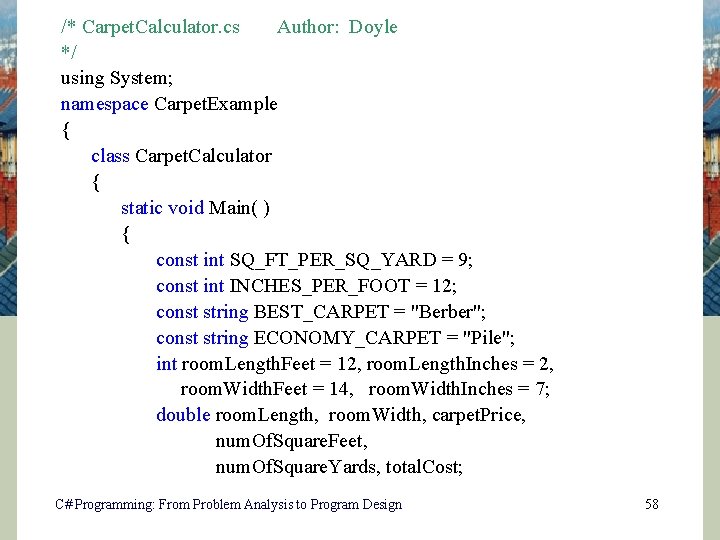
/* Carpet. Calculator. cs Author: Doyle */ using System; namespace Carpet. Example { class Carpet. Calculator { static void Main( ) { const int SQ_FT_PER_SQ_YARD = 9; const int INCHES_PER_FOOT = 12; const string BEST_CARPET = "Berber"; const string ECONOMY_CARPET = "Pile"; int room. Length. Feet = 12, room. Length. Inches = 2, room. Width. Feet = 14, room. Width. Inches = 7; double room. Length, room. Width, carpet. Price, num. Of. Square. Feet, num. Of. Square. Yards, total. Cost; C# Programming: From Problem Analysis to Program Design 58
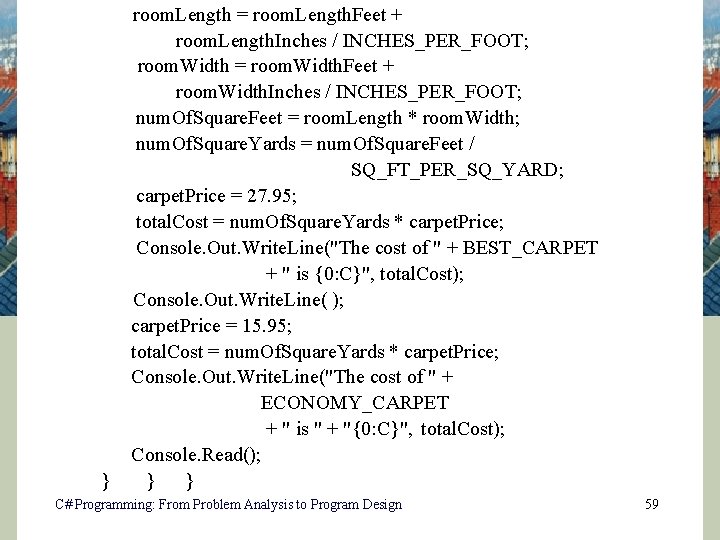
} room. Length = room. Length. Feet + room. Length. Inches / INCHES_PER_FOOT; room. Width = room. Width. Feet + room. Width. Inches / INCHES_PER_FOOT; num. Of. Square. Feet = room. Length * room. Width; num. Of. Square. Yards = num. Of. Square. Feet / SQ_FT_PER_SQ_YARD; carpet. Price = 27. 95; total. Cost = num. Of. Square. Yards * carpet. Price; Console. Out. Write. Line("The cost of " + BEST_CARPET + " is {0: C}", total. Cost); Console. Out. Write. Line( ); carpet. Price = 15. 95; total. Cost = num. Of. Square. Yards * carpet. Price; Console. Out. Write. Line("The cost of " + ECONOMY_CARPET + " is " + "{0: C}", total. Cost); Console. Read(); } } C# Programming: From Problem Analysis to Program Design 59
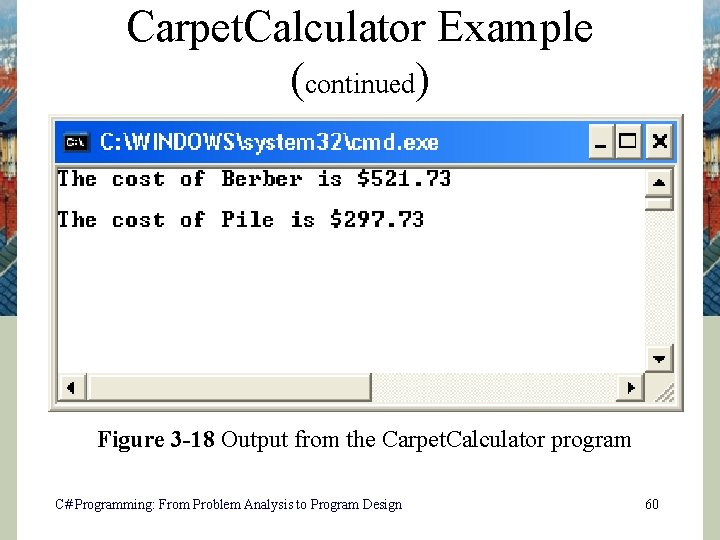
Carpet. Calculator Example (continued) Figure 3 -18 Output from the Carpet. Calculator program C# Programming: From Problem Analysis to Program Design 60
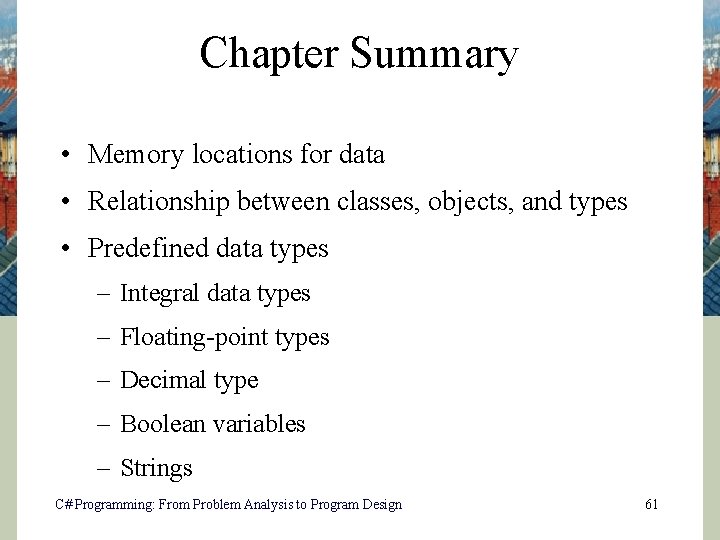
Chapter Summary • Memory locations for data • Relationship between classes, objects, and types • Predefined data types – Integral data types – Floating-point types – Decimal type – Boolean variables – Strings C# Programming: From Problem Analysis to Program Design 61
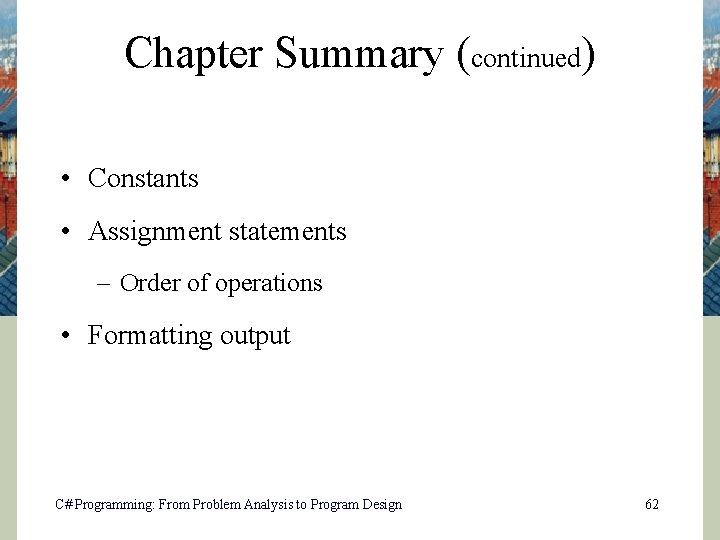
Chapter Summary (continued) • Constants • Assignment statements – Order of operations • Formatting output C# Programming: From Problem Analysis to Program Design 62
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
Definition of system programming
Linear vs integer programming
Perbedaan linear programming dan integer programming
Descriptive mining of complex data objects
Different types of data representation
Mining complex types of data in data mining
Complex data types in data mining
Types of functions in programming
Types of programming error
Which cards are generated by absolute loader?
Strongly typed vs weakly typed
Types of programming errors
Types of variables in computer programming
Int vs short
Ibm 360 machine structure
Writing and evaluating expressions
Rational expressions and functions
1-1 variables and expressions answer key
Symbols and expressions.
11-4 practice multiplying and dividing rational expressions
6-2 practice multiplying and dividing radical expressions
Present simple always
Present continuous time expressions
Expression of quantity
Multiplying and dividing rational expressions quizlet
Expressions and equations jeopardy
Expressions equations and inequalities unit test
Expressions, equations, and inequalities unit test part 1
Chapter 9 rational expressions and equations answers
Practice 9-5 adding and subtracting rational expressions
Affirmative negative words spanish
8-3 adding and subtracting rational expressions
How to subtract expressions
8-1 multiplying and dividing rational expressions
7-3 lesson quiz
5-6 radical expressions and rational exponents
How to subtract rational expressions
11-4 multiplying and dividing rational expressions
11-4 practice adding and subtracting rational expressions
Addition of rational expressions
Numerical expression
Translating expressions and equations
Adding subtracting and multiplying radical expressions
Positive and negative expressions
What are the two vocal styles of singing in pakistan?
Leave takings examples
Statements expressing congratulation
Invert the subject and verb with place expressions
Materi expressions of giving and asking plans
Pointer expressions and pointer arithmetic
5-6 radical expressions and rational exponents
Order of operations and evaluating expressions
Parts of expressions
Process column algebra 2
Algebra 2 patterns
Radical and rational expressions
Translating algebraic expressions
Likes and opinions
Legal moves algebra tiles
Greetings and farewells in english and spanish
Greetings farewells and courtesy expressions en español