3 D Drawing Primitives A primitive is a
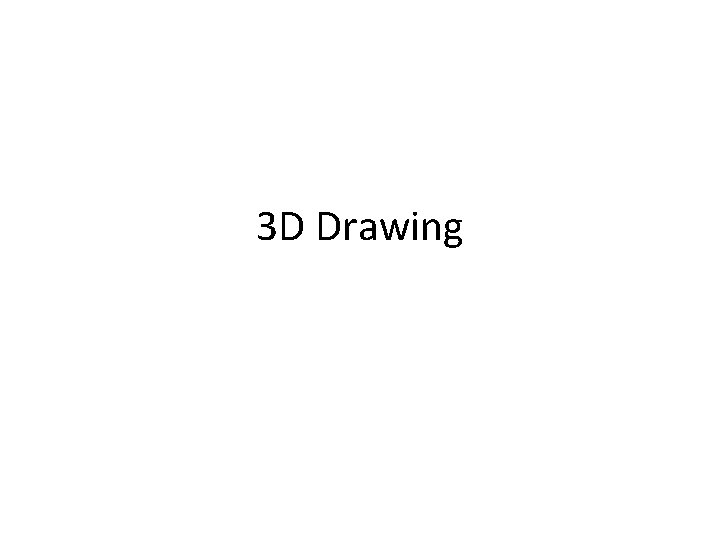
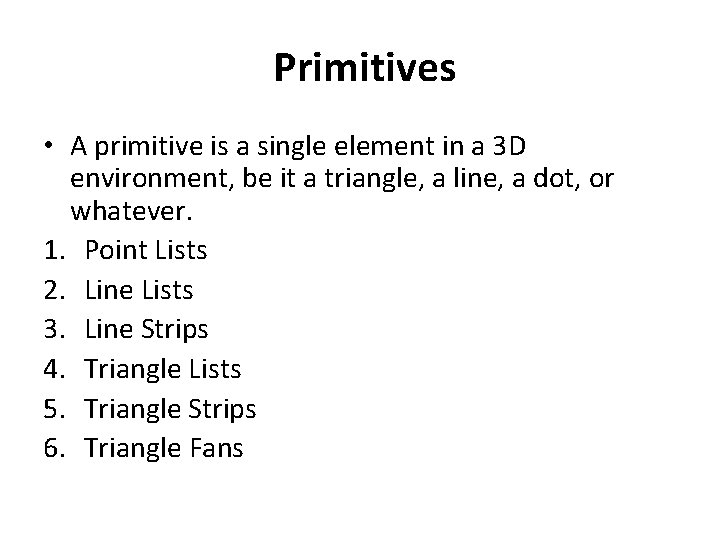
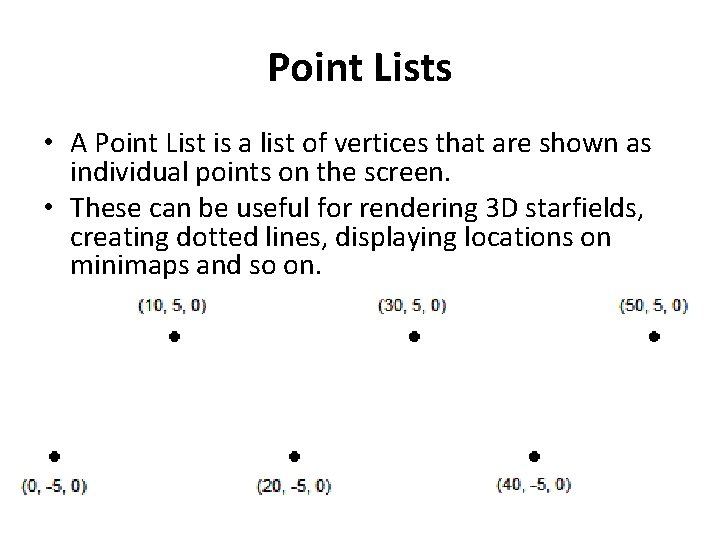
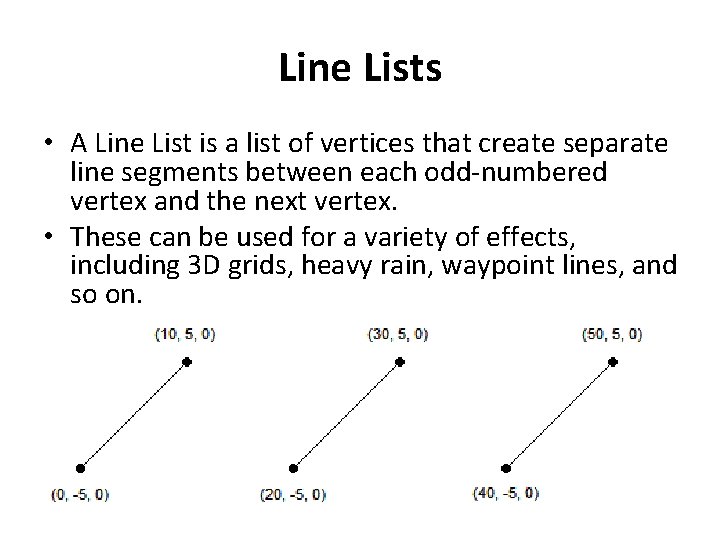
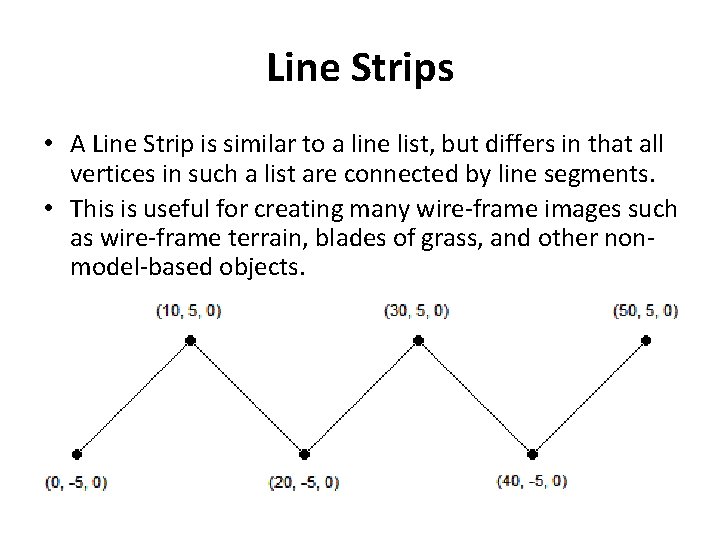
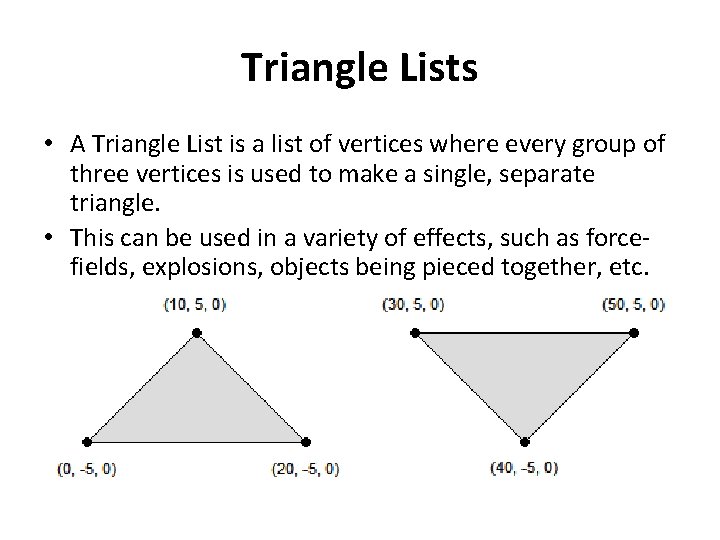
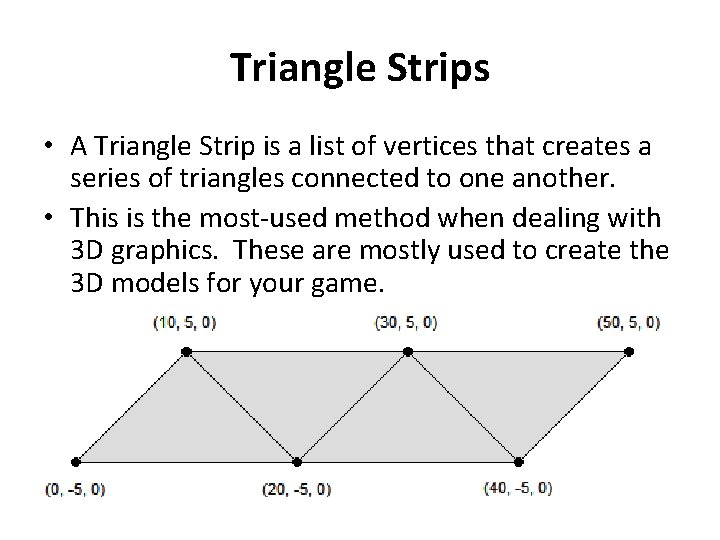
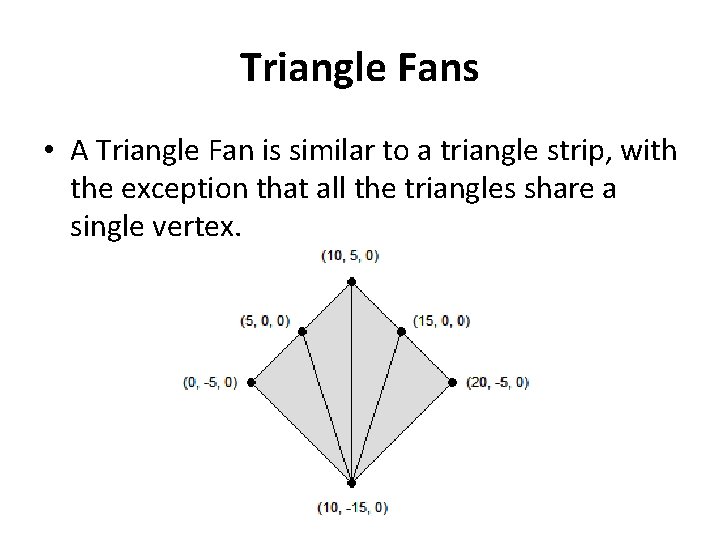
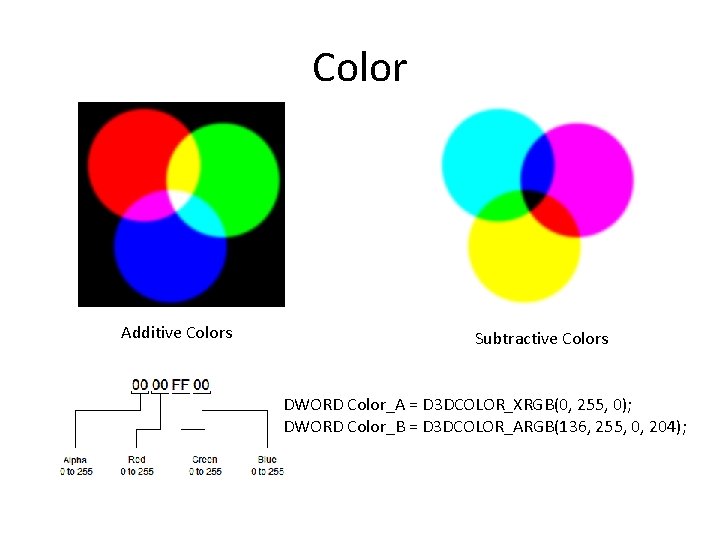
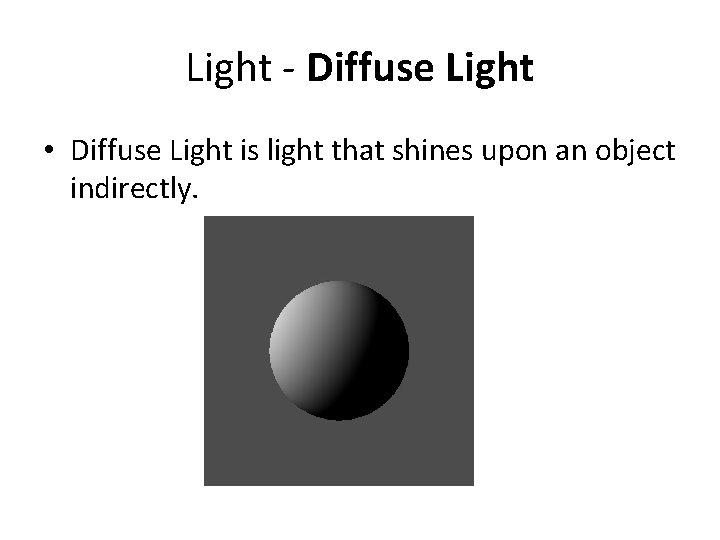
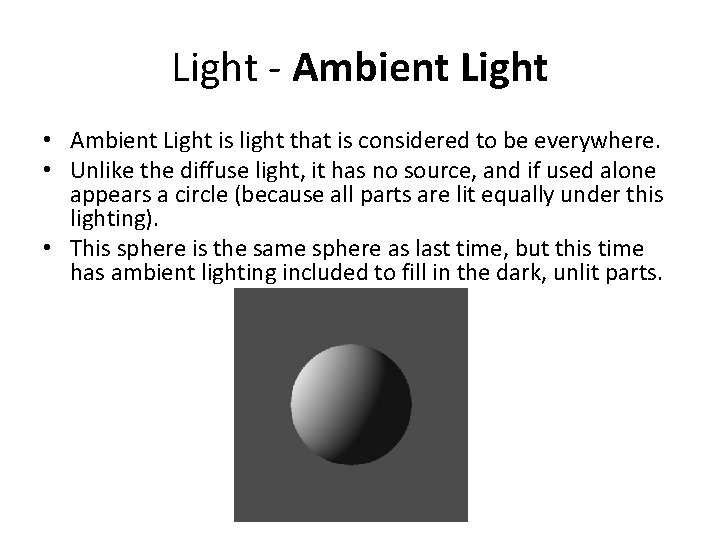
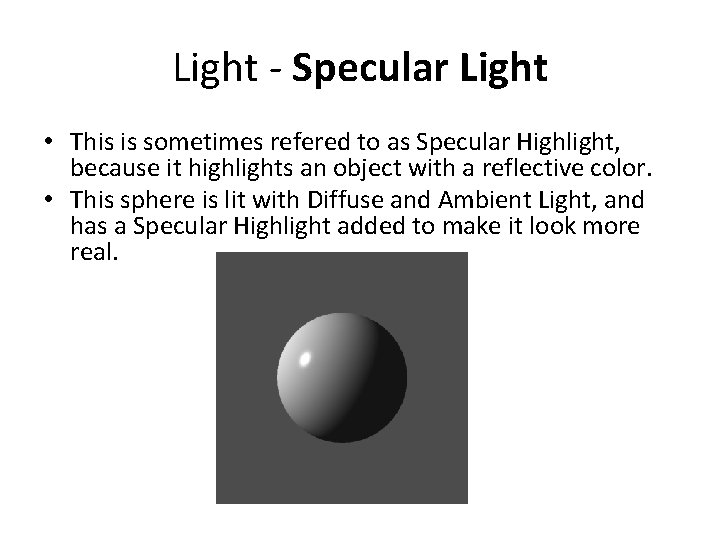
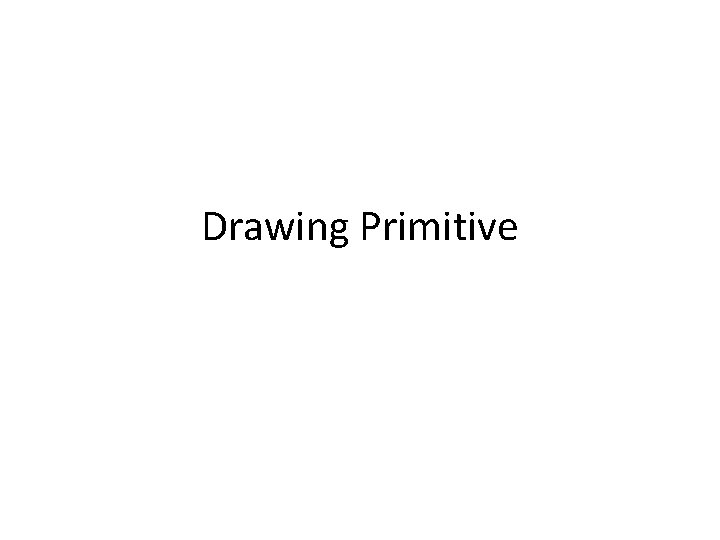
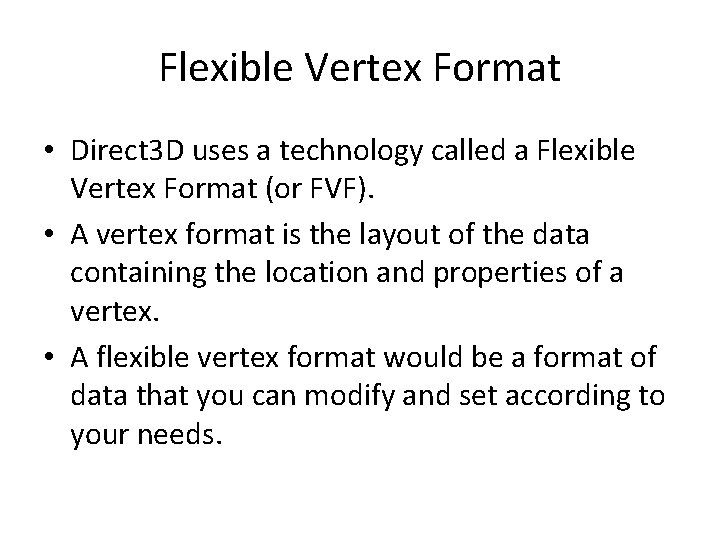
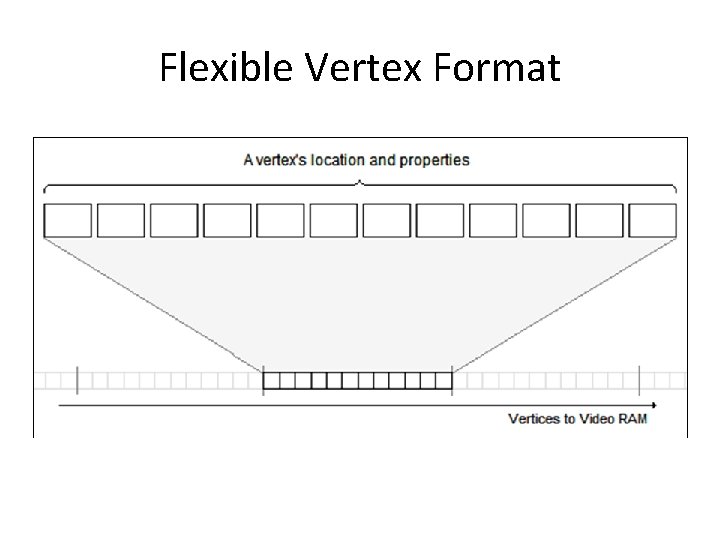
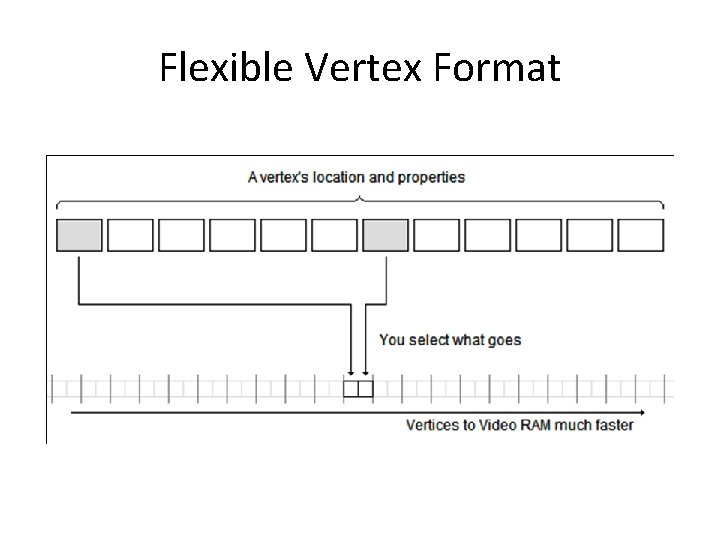
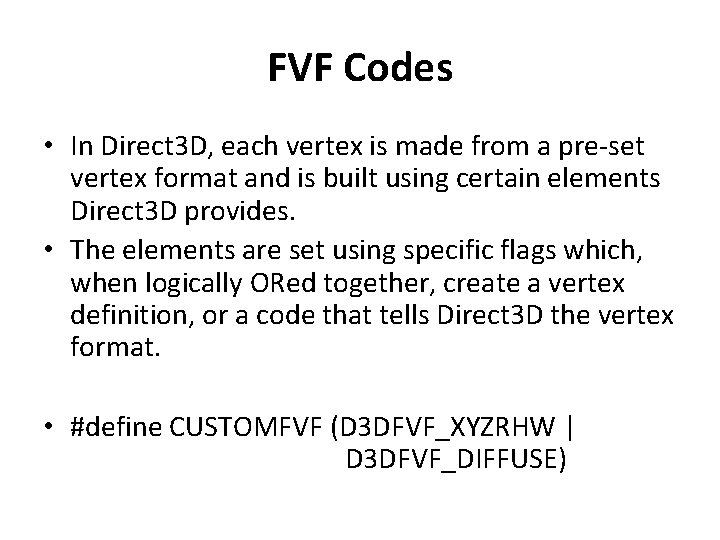
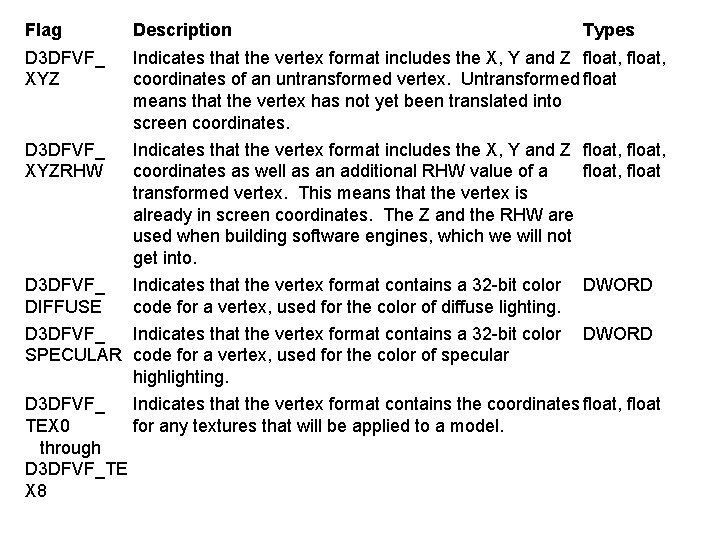
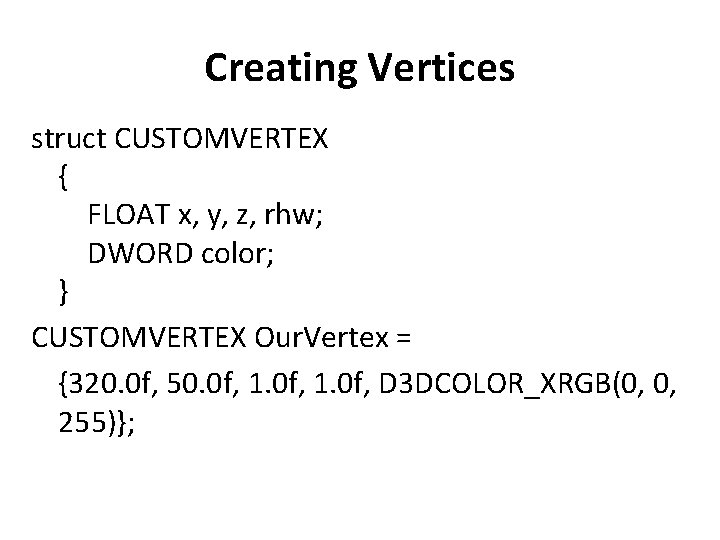
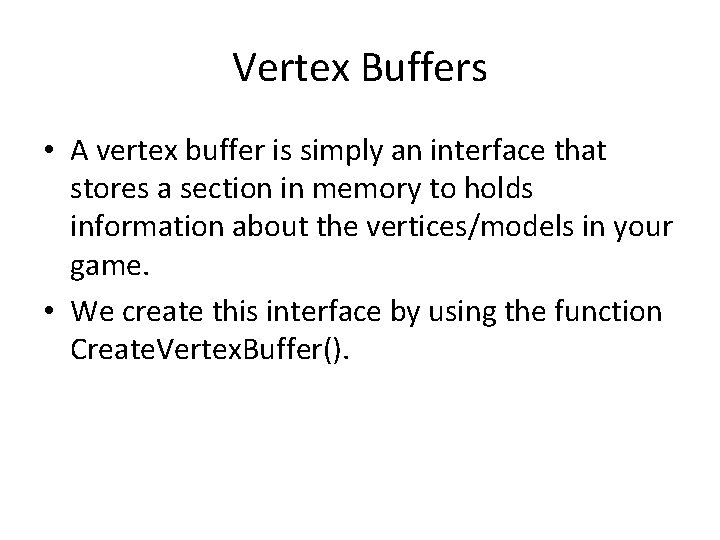
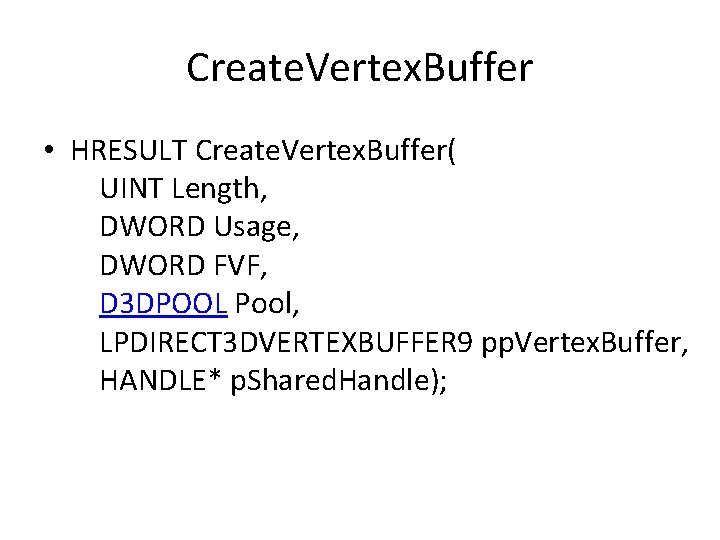
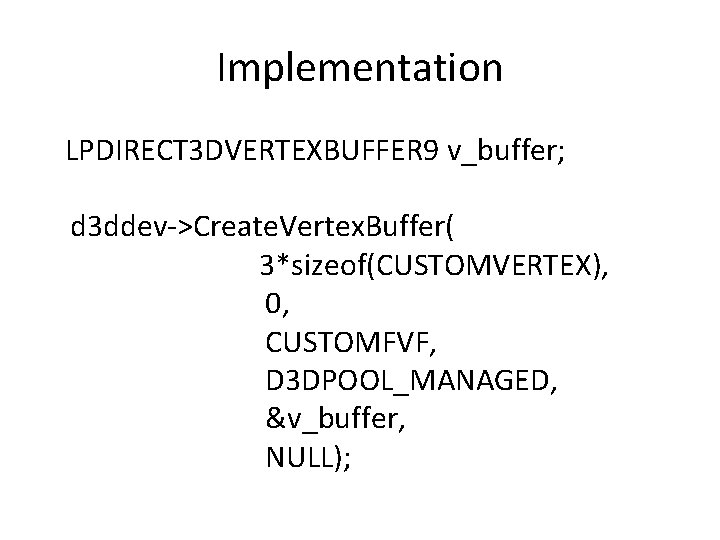
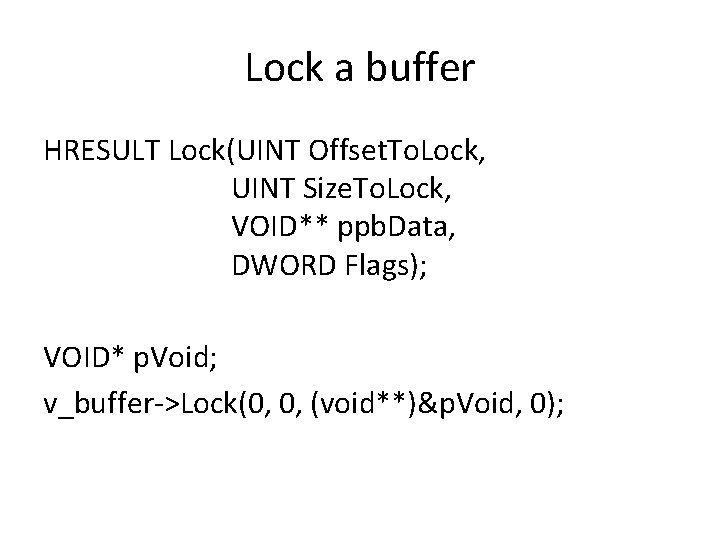
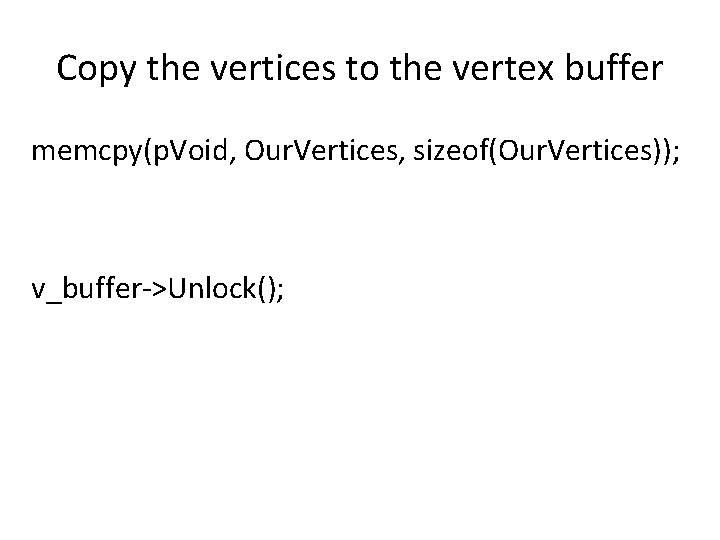
![void init_graphics(void) { CUSTOMVERTEX vertices[] = { { 320. 0 f, 50. 0 f, void init_graphics(void) { CUSTOMVERTEX vertices[] = { { 320. 0 f, 50. 0 f,](https://slidetodoc.com/presentation_image_h2/82c8285179401facc01430ca3270895d/image-25.jpg)
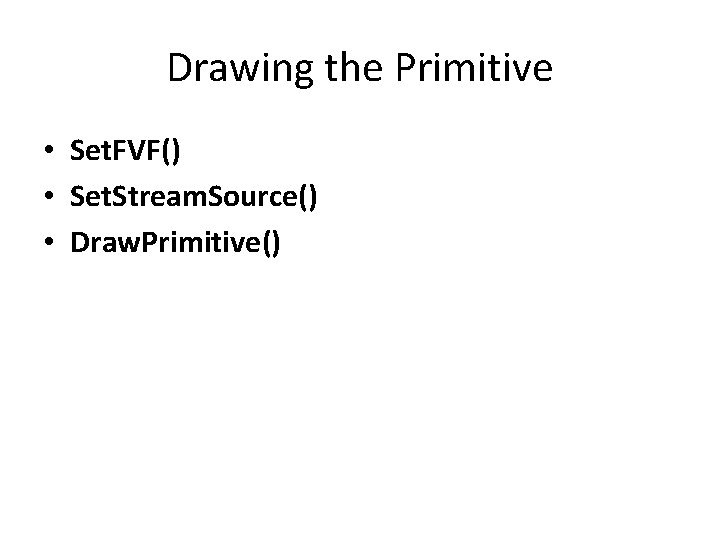
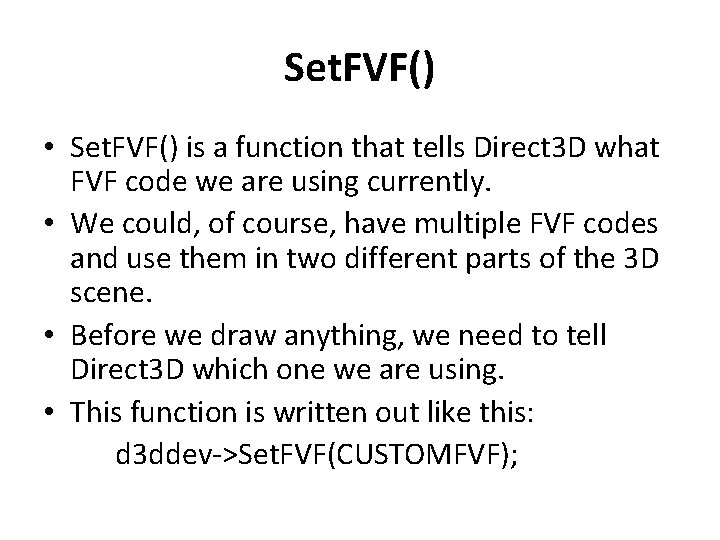
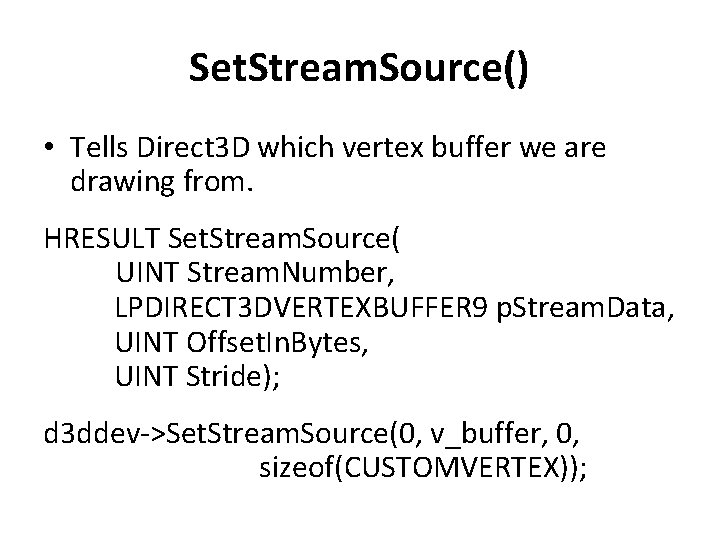
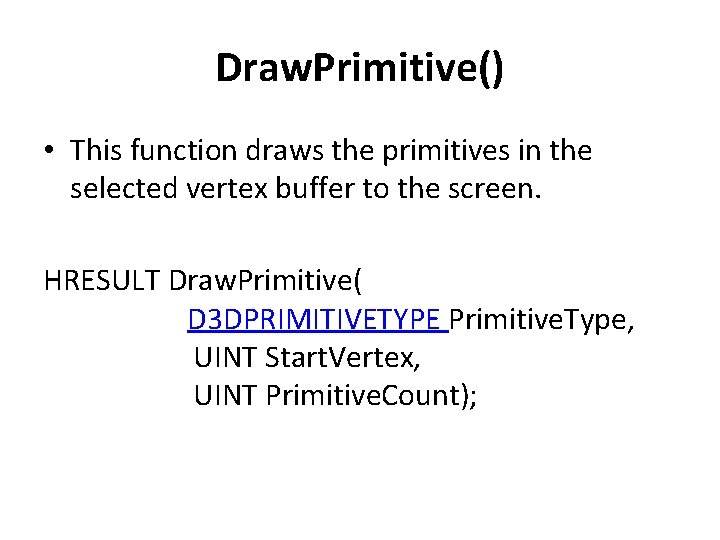
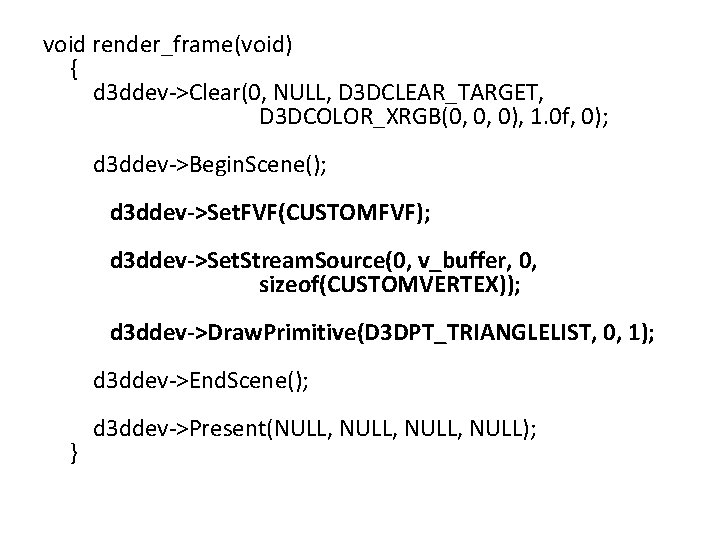
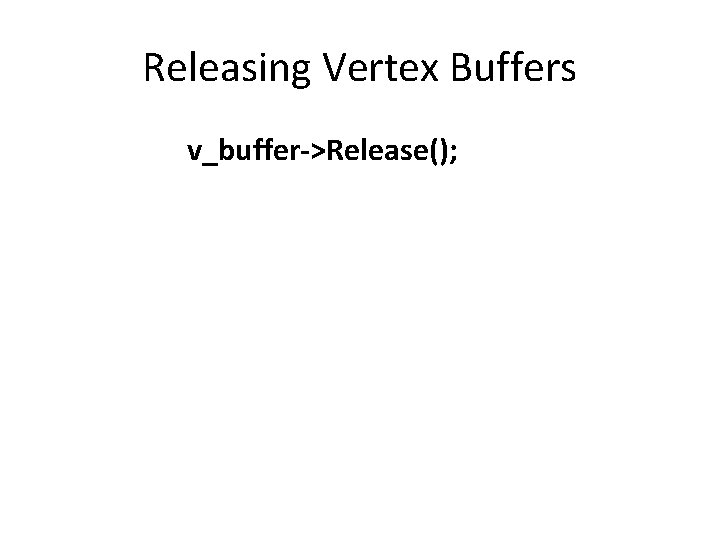
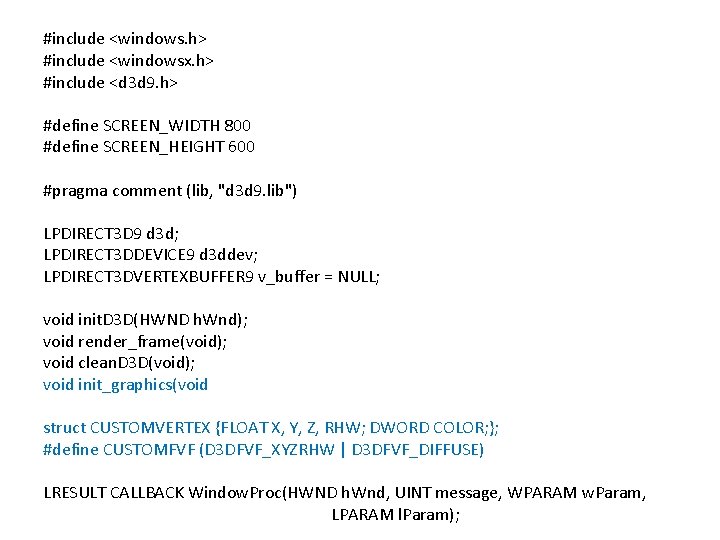
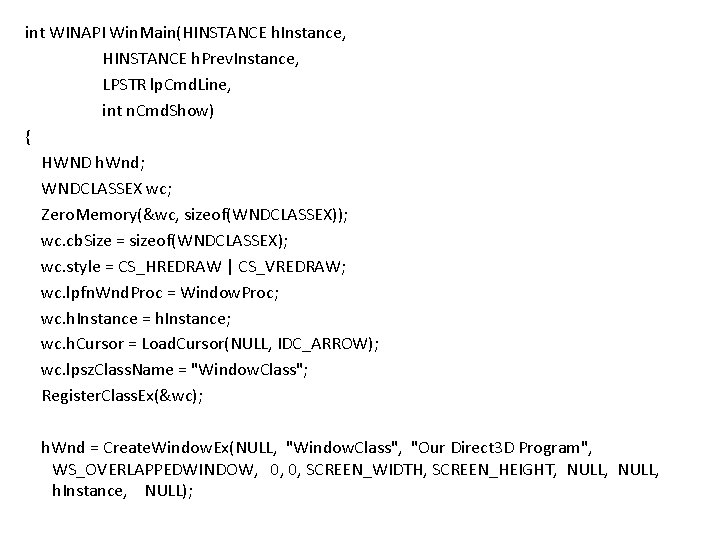
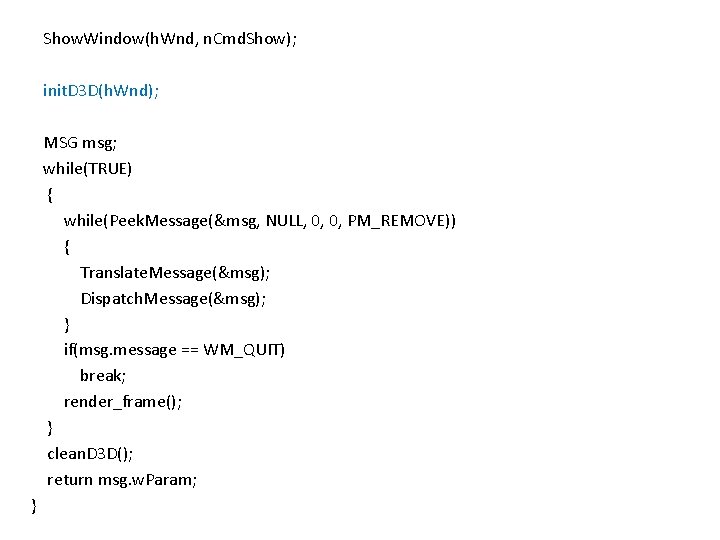
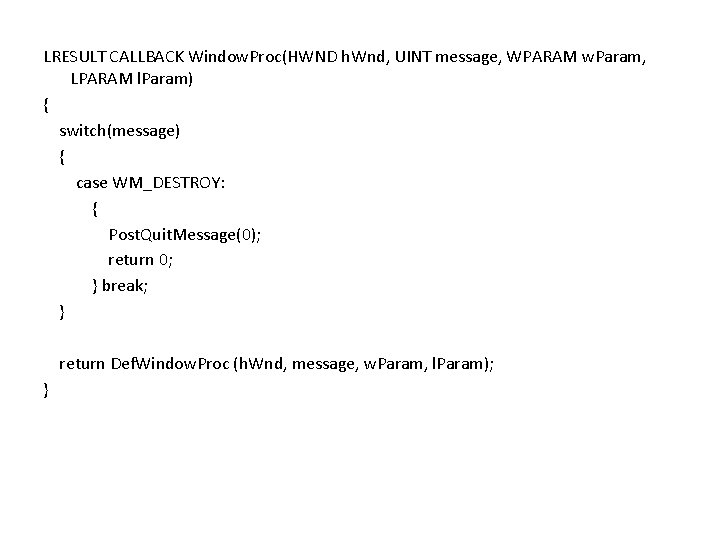
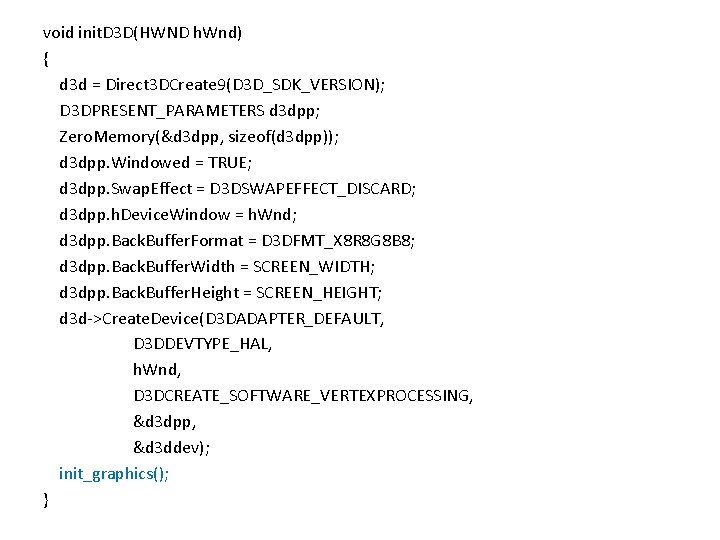
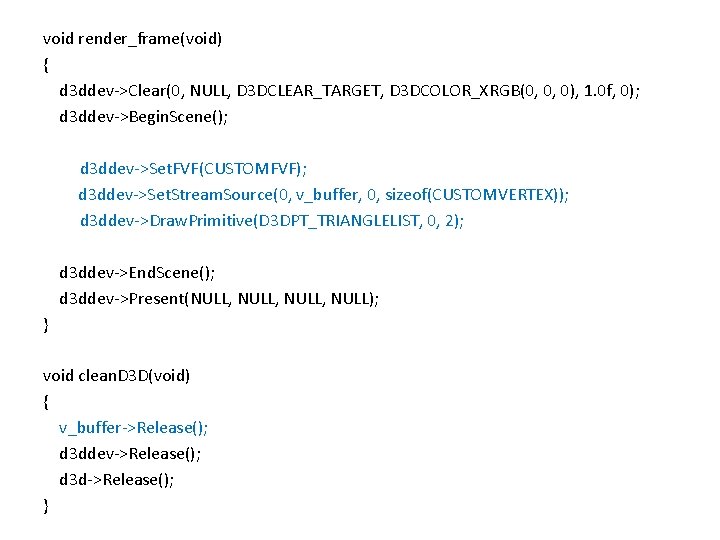
![void init_graphics(void) { CUSTOMVERTEX vertices[] = { { 400. 0 f, 62. 5 f, void init_graphics(void) { CUSTOMVERTEX vertices[] = { { 400. 0 f, 62. 5 f,](https://slidetodoc.com/presentation_image_h2/82c8285179401facc01430ca3270895d/image-38.jpg)
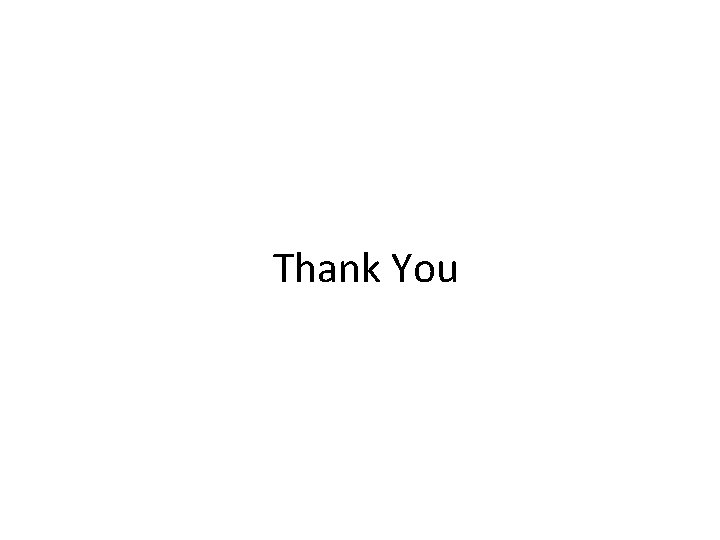
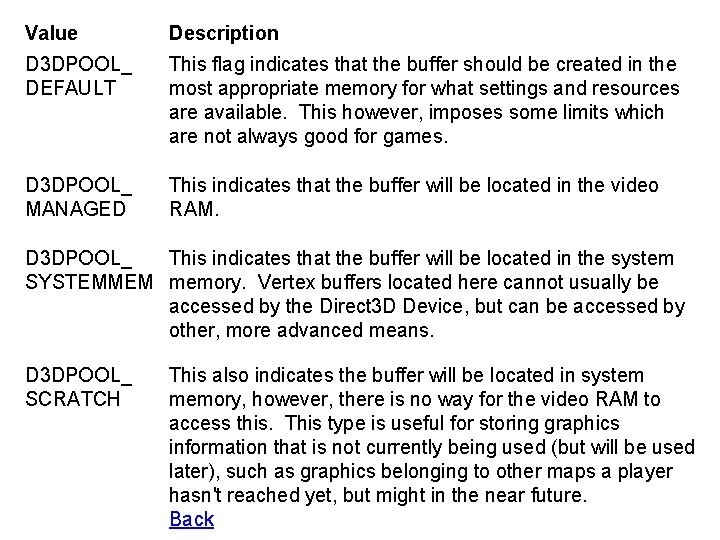
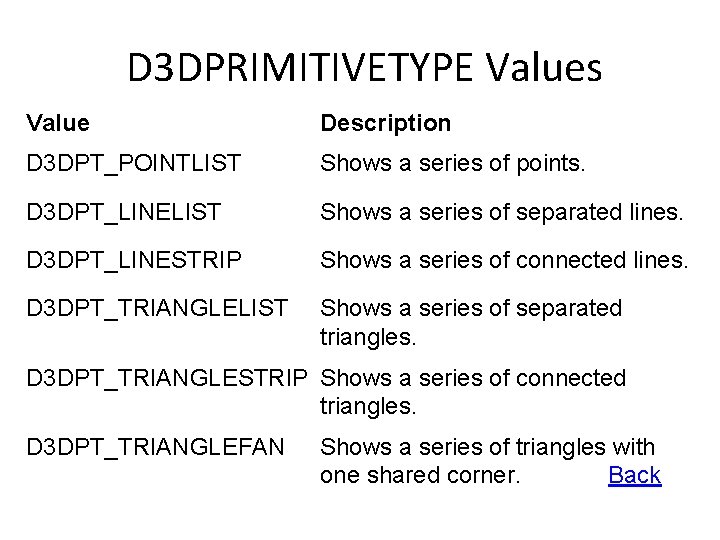
- Slides: 41
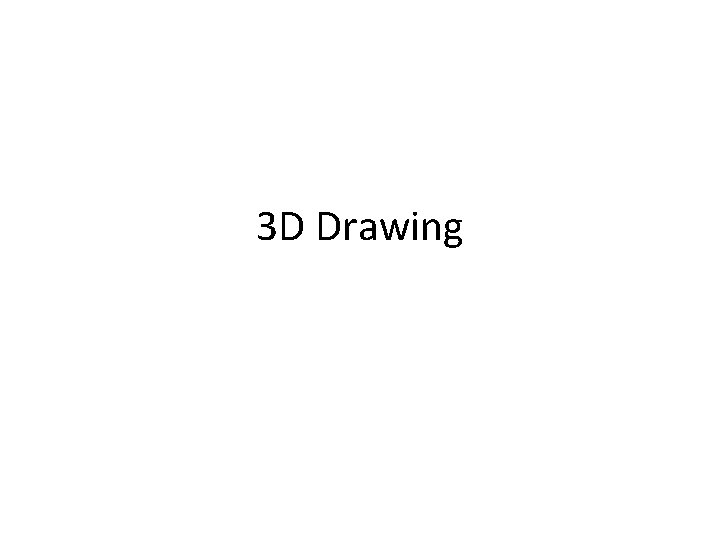
3 D Drawing
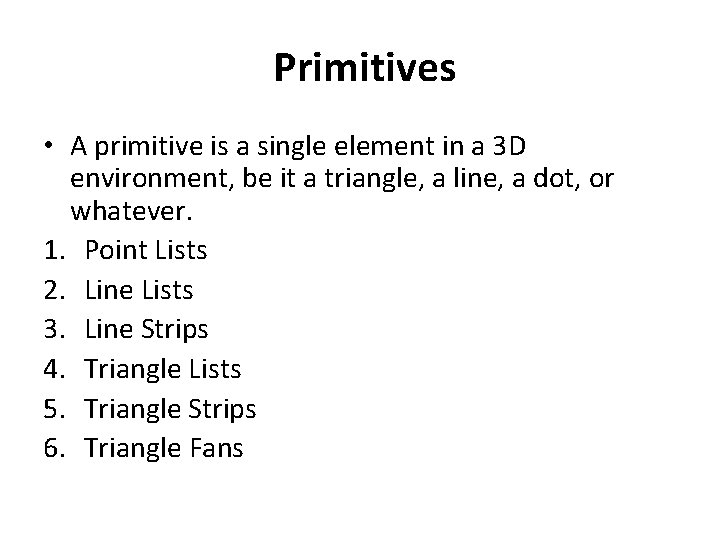
Primitives • A primitive is a single element in a 3 D environment, be it a triangle, a line, a dot, or whatever. 1. Point Lists 2. Line Lists 3. Line Strips 4. Triangle Lists 5. Triangle Strips 6. Triangle Fans
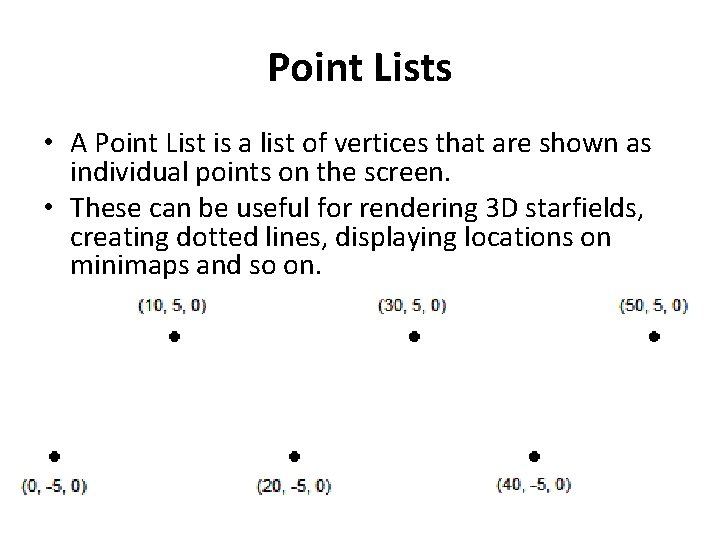
Point Lists • A Point List is a list of vertices that are shown as individual points on the screen. • These can be useful for rendering 3 D starfields, creating dotted lines, displaying locations on minimaps and so on.
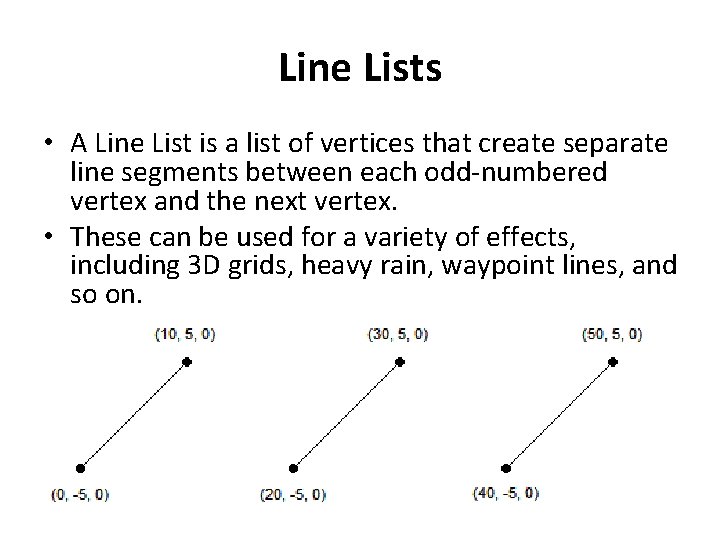
Line Lists • A Line List is a list of vertices that create separate line segments between each odd-numbered vertex and the next vertex. • These can be used for a variety of effects, including 3 D grids, heavy rain, waypoint lines, and so on.
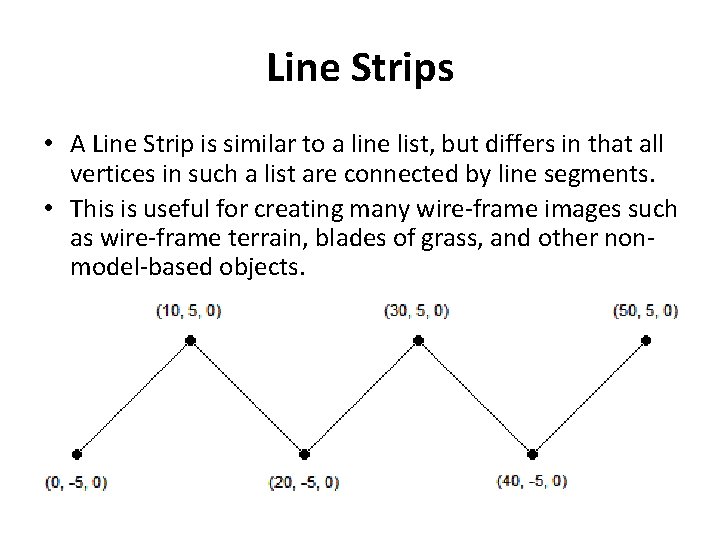
Line Strips • A Line Strip is similar to a line list, but differs in that all vertices in such a list are connected by line segments. • This is useful for creating many wire-frame images such as wire-frame terrain, blades of grass, and other nonmodel-based objects.
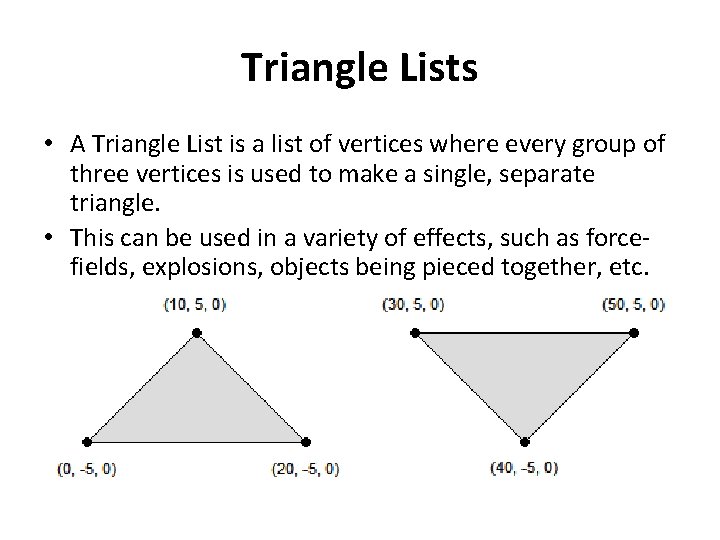
Triangle Lists • A Triangle List is a list of vertices where every group of three vertices is used to make a single, separate triangle. • This can be used in a variety of effects, such as forcefields, explosions, objects being pieced together, etc.
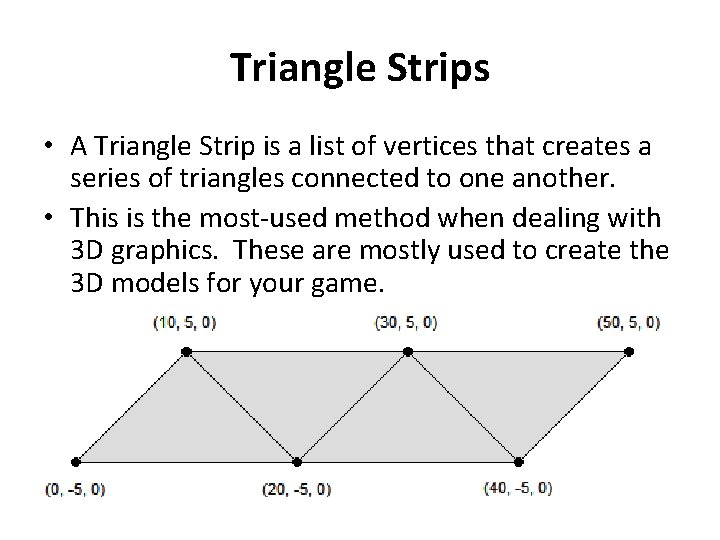
Triangle Strips • A Triangle Strip is a list of vertices that creates a series of triangles connected to one another. • This is the most-used method when dealing with 3 D graphics. These are mostly used to create the 3 D models for your game.
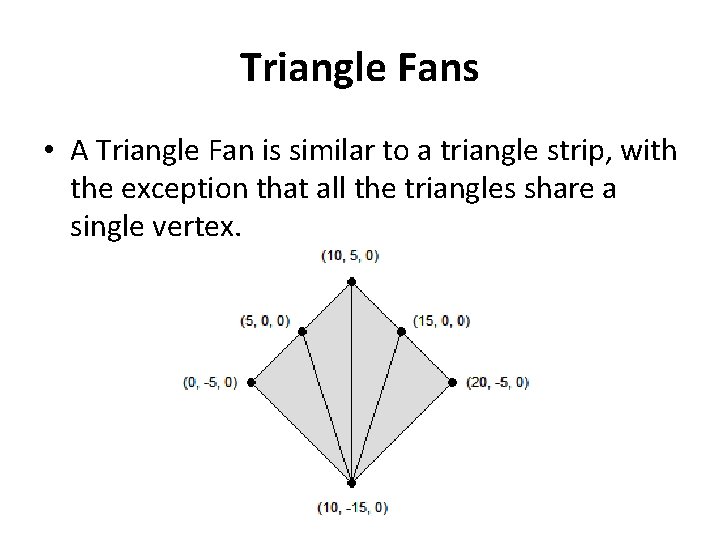
Triangle Fans • A Triangle Fan is similar to a triangle strip, with the exception that all the triangles share a single vertex.
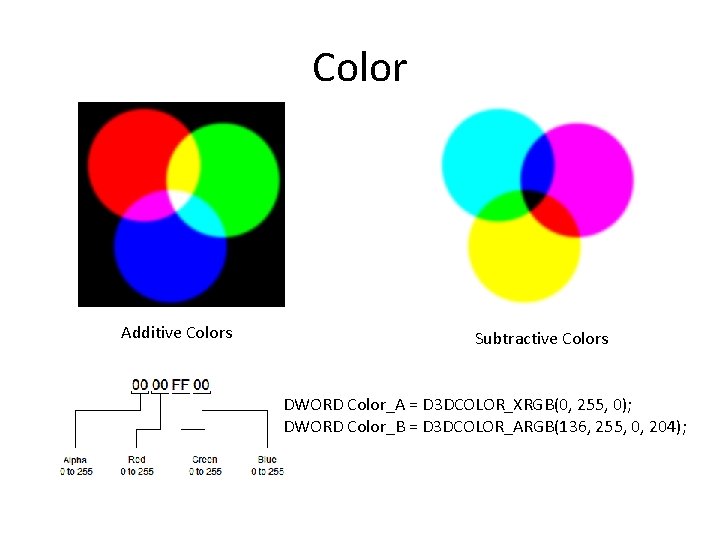
Color Additive Colors Subtractive Colors DWORD Color_A = D 3 DCOLOR_XRGB(0, 255, 0); DWORD Color_B = D 3 DCOLOR_ARGB(136, 255, 0, 204);
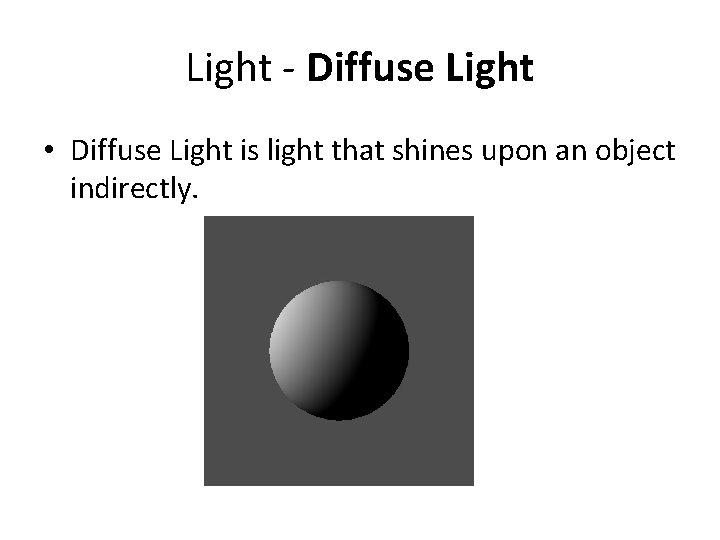
Light - Diffuse Light • Diffuse Light is light that shines upon an object indirectly.
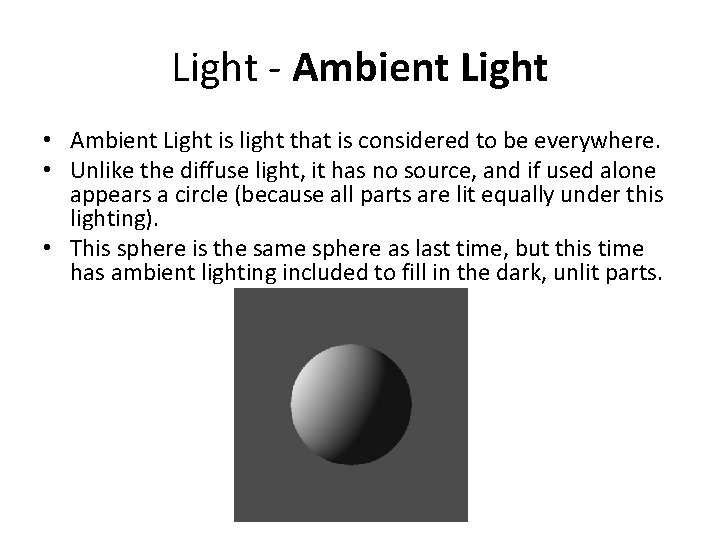
Light - Ambient Light • Ambient Light is light that is considered to be everywhere. • Unlike the diffuse light, it has no source, and if used alone appears a circle (because all parts are lit equally under this lighting). • This sphere is the same sphere as last time, but this time has ambient lighting included to fill in the dark, unlit parts.
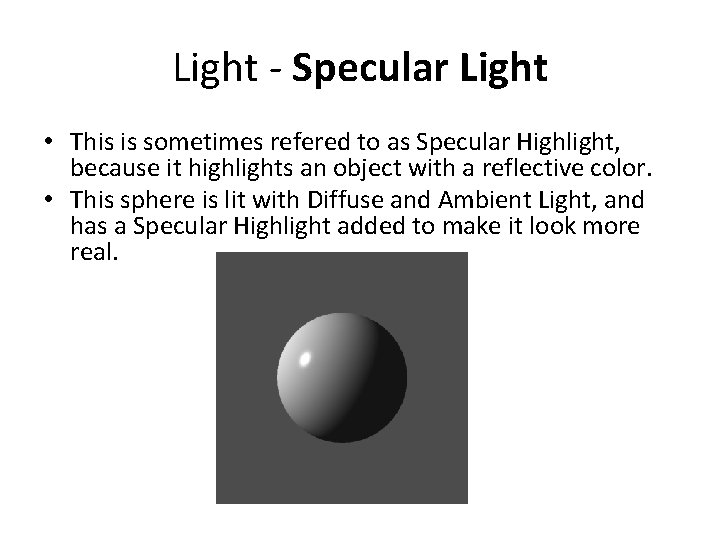
Light - Specular Light • This is sometimes refered to as Specular Highlight, because it highlights an object with a reflective color. • This sphere is lit with Diffuse and Ambient Light, and has a Specular Highlight added to make it look more real.
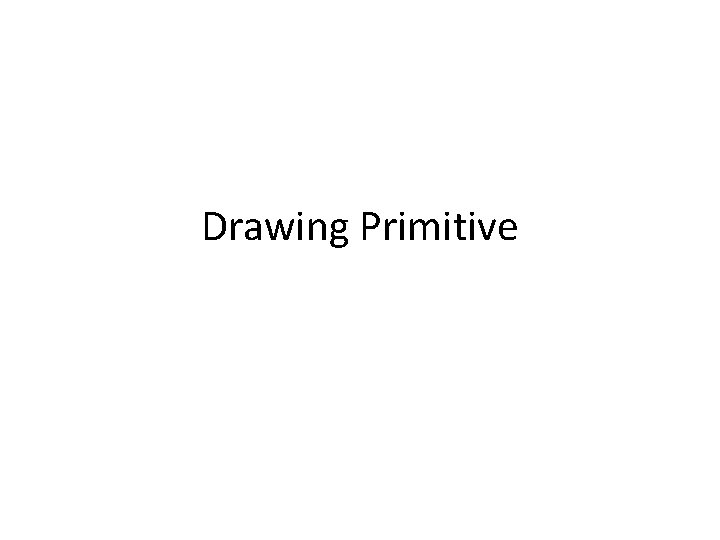
Drawing Primitive
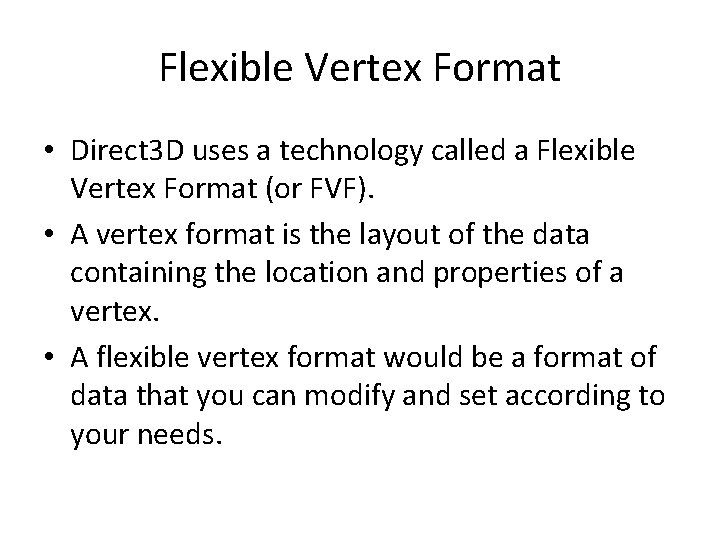
Flexible Vertex Format • Direct 3 D uses a technology called a Flexible Vertex Format (or FVF). • A vertex format is the layout of the data containing the location and properties of a vertex. • A flexible vertex format would be a format of data that you can modify and set according to your needs.
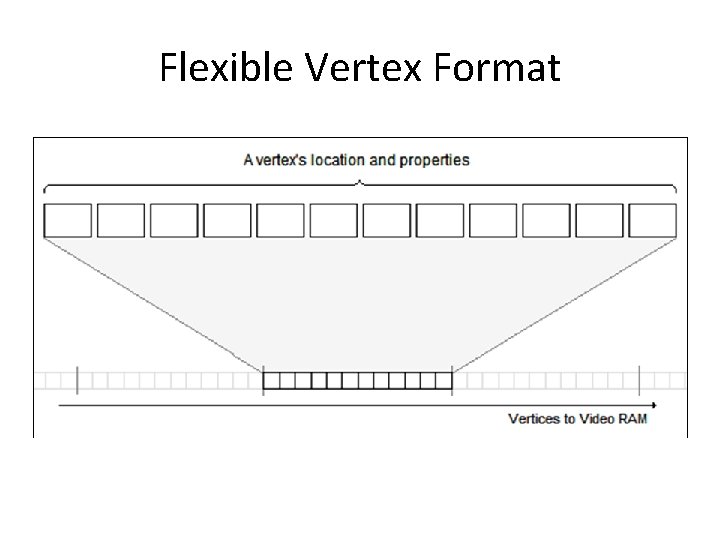
Flexible Vertex Format
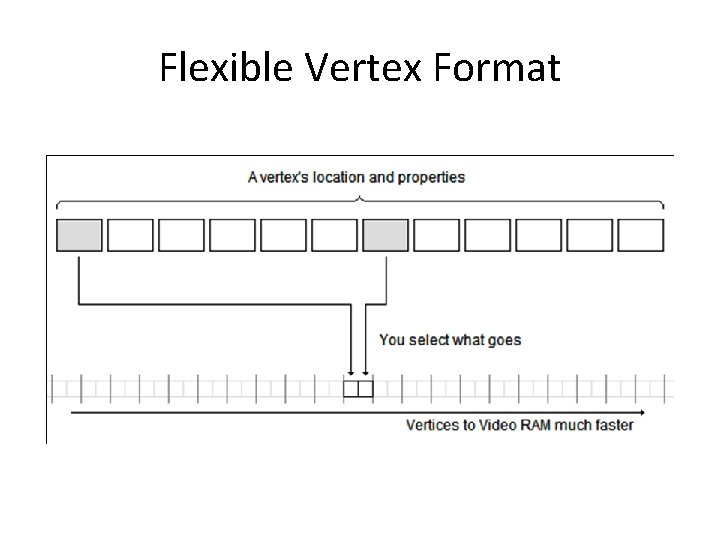
Flexible Vertex Format
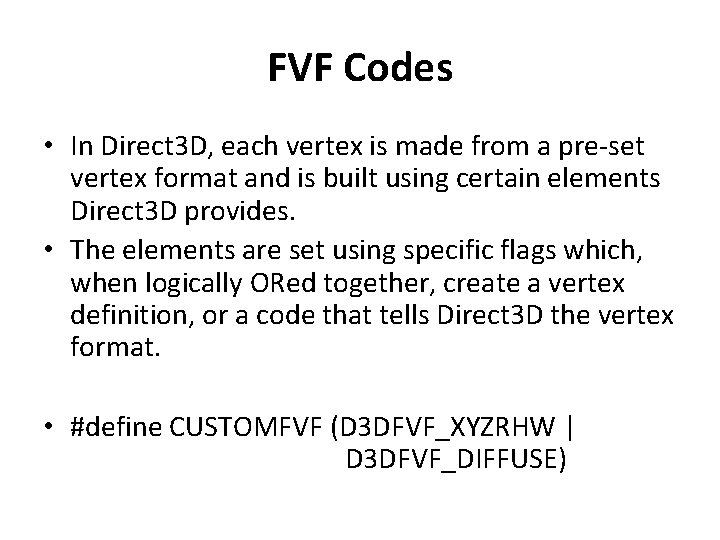
FVF Codes • In Direct 3 D, each vertex is made from a pre-set vertex format and is built using certain elements Direct 3 D provides. • The elements are set using specific flags which, when logically ORed together, create a vertex definition, or a code that tells Direct 3 D the vertex format. • #define CUSTOMFVF (D 3 DFVF_XYZRHW | D 3 DFVF_DIFFUSE)
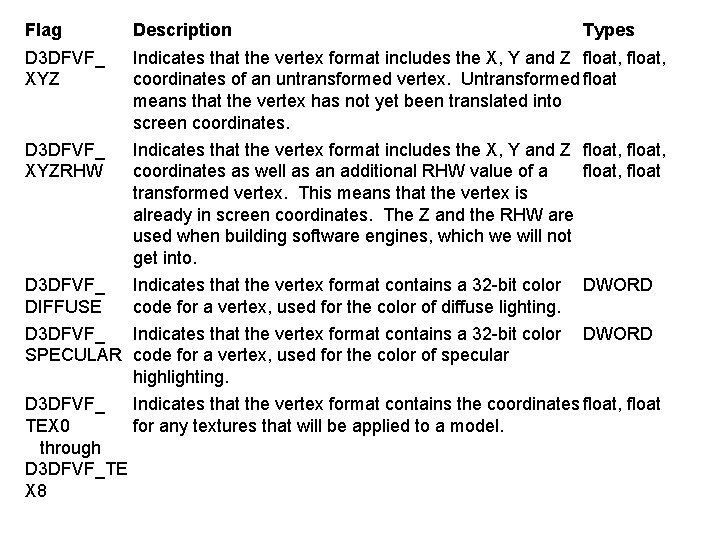
Flag Description Types D 3 DFVF_ XYZ Indicates that the vertex format includes the X, Y and Z float, coordinates of an untransformed vertex. Untransformed float means that the vertex has not yet been translated into screen coordinates. D 3 DFVF_ XYZRHW Indicates that the vertex format includes the X, Y and Z float, coordinates as well as an additional RHW value of a float, float transformed vertex. This means that the vertex is already in screen coordinates. The Z and the RHW are used when building software engines, which we will not get into. D 3 DFVF_ DIFFUSE Indicates that the vertex format contains a 32 -bit color code for a vertex, used for the color of diffuse lighting. DWORD D 3 DFVF_ Indicates that the vertex format contains a 32 -bit color SPECULAR code for a vertex, used for the color of specular highlighting. DWORD D 3 DFVF_ Indicates that the vertex format contains the coordinates float, float TEX 0 for any textures that will be applied to a model. through D 3 DFVF_TE X 8
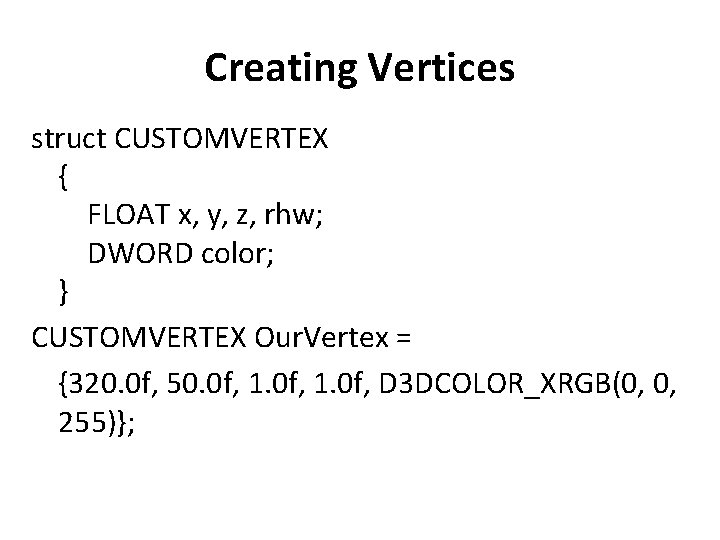
Creating Vertices struct CUSTOMVERTEX { FLOAT x, y, z, rhw; DWORD color; } CUSTOMVERTEX Our. Vertex = {320. 0 f, 50. 0 f, 1. 0 f, D 3 DCOLOR_XRGB(0, 0, 255)};
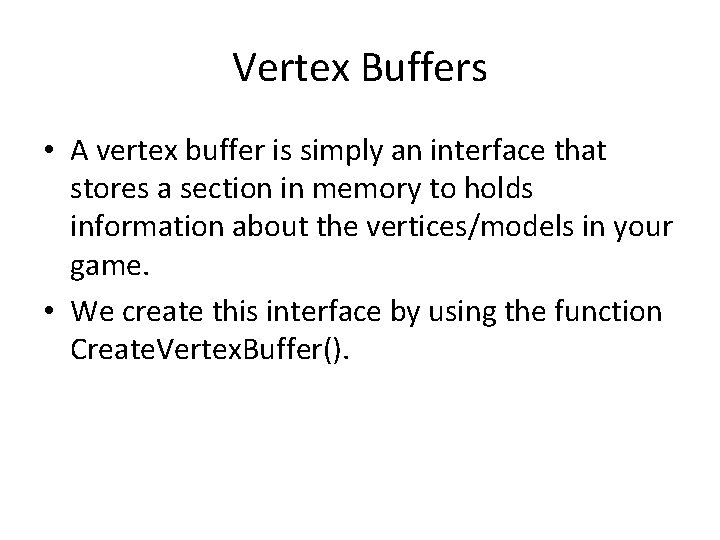
Vertex Buffers • A vertex buffer is simply an interface that stores a section in memory to holds information about the vertices/models in your game. • We create this interface by using the function Create. Vertex. Buffer().
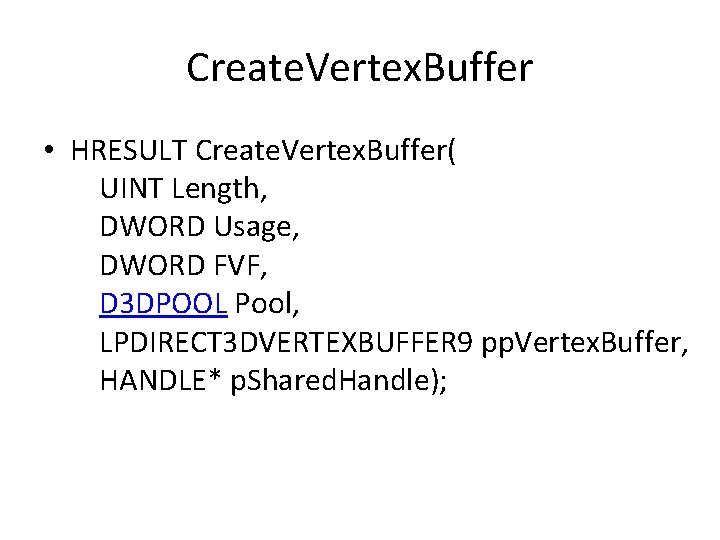
Create. Vertex. Buffer • HRESULT Create. Vertex. Buffer( UINT Length, DWORD Usage, DWORD FVF, D 3 DPOOL Pool, LPDIRECT 3 DVERTEXBUFFER 9 pp. Vertex. Buffer, HANDLE* p. Shared. Handle);
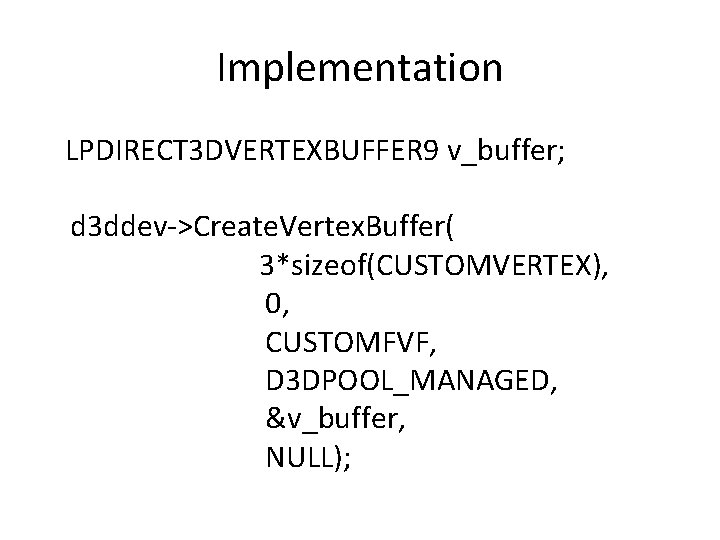
Implementation LPDIRECT 3 DVERTEXBUFFER 9 v_buffer; d 3 ddev->Create. Vertex. Buffer( 3*sizeof(CUSTOMVERTEX), 0, CUSTOMFVF, D 3 DPOOL_MANAGED, &v_buffer, NULL);
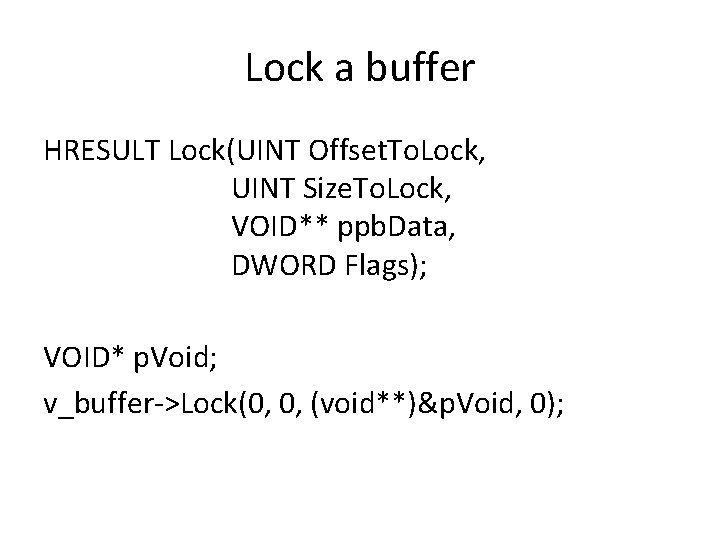
Lock a buffer HRESULT Lock(UINT Offset. To. Lock, UINT Size. To. Lock, VOID** ppb. Data, DWORD Flags); VOID* p. Void; v_buffer->Lock(0, 0, (void**)&p. Void, 0);
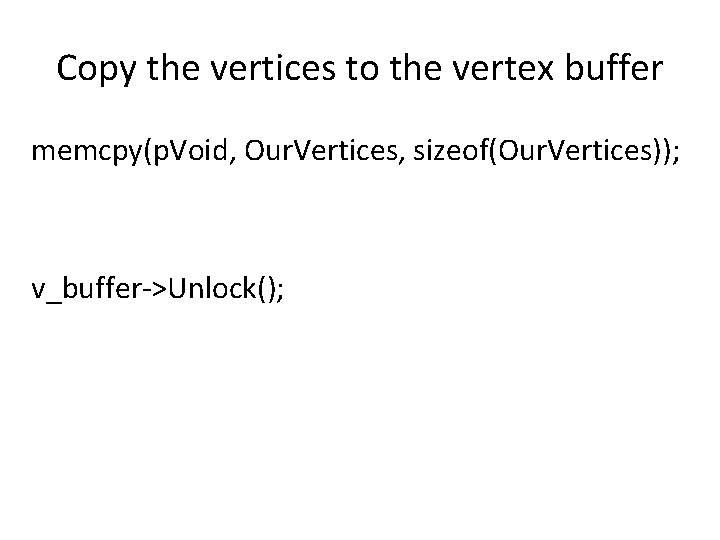
Copy the vertices to the vertex buffer memcpy(p. Void, Our. Vertices, sizeof(Our. Vertices)); v_buffer->Unlock();
![void initgraphicsvoid CUSTOMVERTEX vertices 320 0 f 50 0 f void init_graphics(void) { CUSTOMVERTEX vertices[] = { { 320. 0 f, 50. 0 f,](https://slidetodoc.com/presentation_image_h2/82c8285179401facc01430ca3270895d/image-25.jpg)
void init_graphics(void) { CUSTOMVERTEX vertices[] = { { 320. 0 f, 50. 0 f, 0. 5 f, 1. 0 f, D 3 DCOLOR_XRGB(0, 0, 255), }, { 520. 0 f, 400. 0 f, 0. 5 f, 1. 0 f, D 3 DCOLOR_XRGB(0, 255, 0), }, { 120. 0 f, 400. 0 f, 0. 5 f, 1. 0 f, D 3 DCOLOR_XRGB(255, 0, 0), }, }; d 3 ddev->Create. Vertex. Buffer(3*sizeof(CUSTOMVERTEX), 0, CUSTOMFVF, D 3 DPOOL_MANAGED, &v_buffer, NULL); } VOID* p. Void; v_buffer->Lock(0, 0, (void**)&p. Void, 0); memcpy(p. Void, vertices, sizeof(vertices)); v_buffer->Unlock();
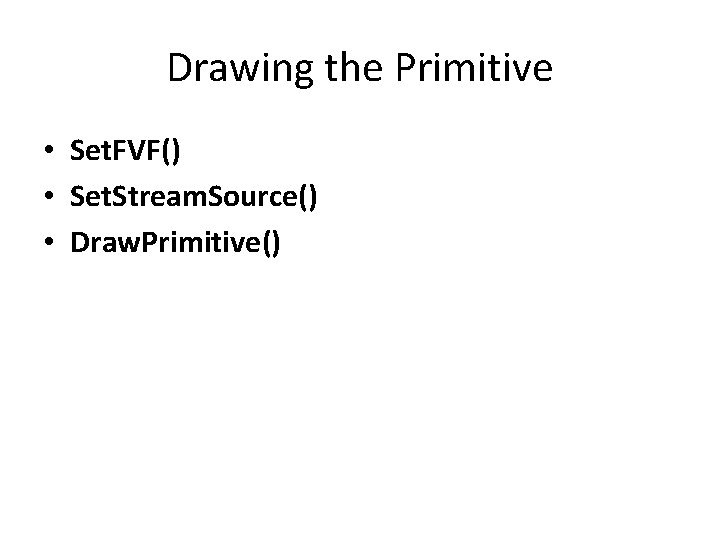
Drawing the Primitive • Set. FVF() • Set. Stream. Source() • Draw. Primitive()
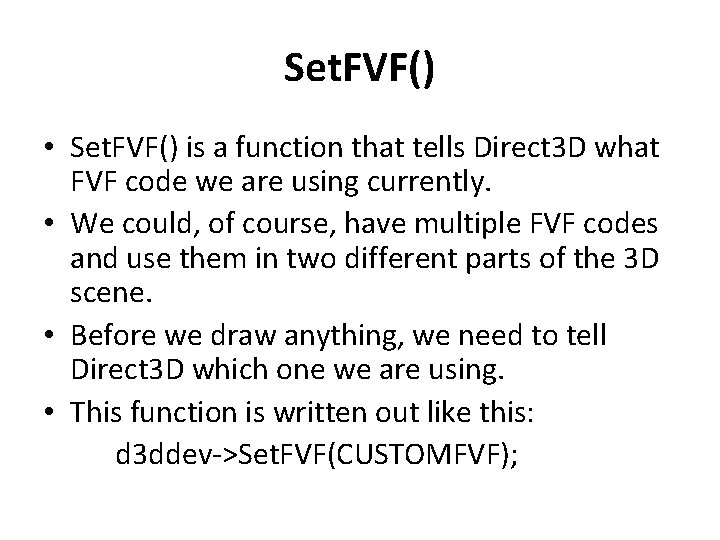
Set. FVF() • Set. FVF() is a function that tells Direct 3 D what FVF code we are using currently. • We could, of course, have multiple FVF codes and use them in two different parts of the 3 D scene. • Before we draw anything, we need to tell Direct 3 D which one we are using. • This function is written out like this: d 3 ddev->Set. FVF(CUSTOMFVF);
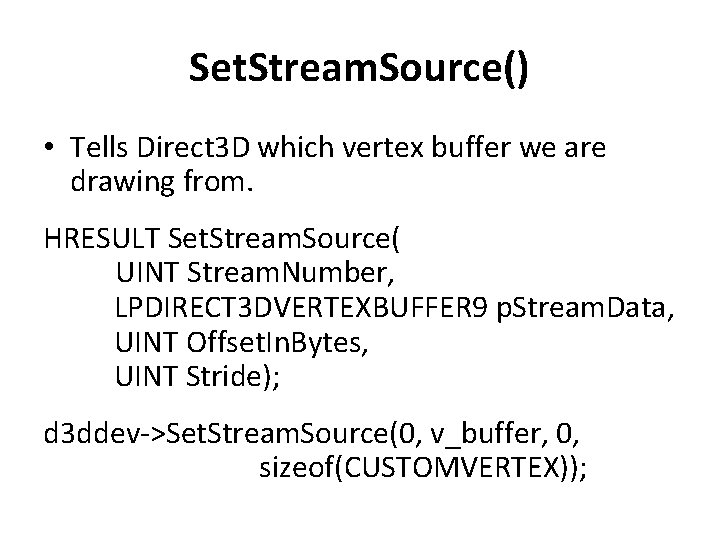
Set. Stream. Source() • Tells Direct 3 D which vertex buffer we are drawing from. HRESULT Set. Stream. Source( UINT Stream. Number, LPDIRECT 3 DVERTEXBUFFER 9 p. Stream. Data, UINT Offset. In. Bytes, UINT Stride); d 3 ddev->Set. Stream. Source(0, v_buffer, 0, sizeof(CUSTOMVERTEX));
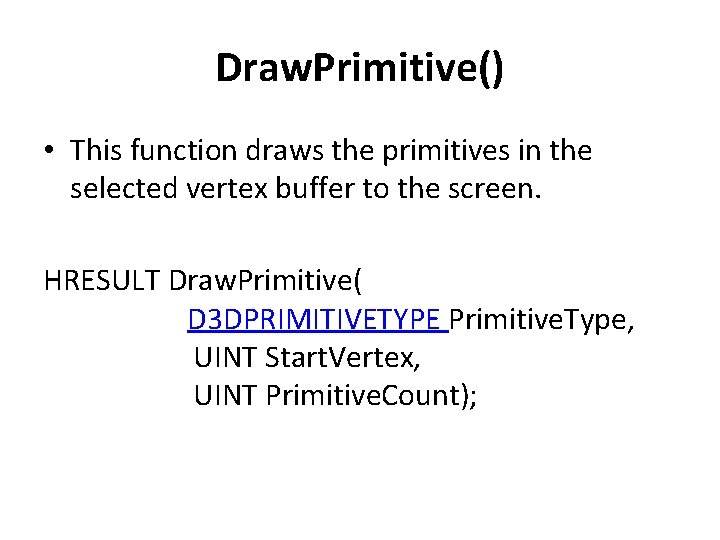
Draw. Primitive() • This function draws the primitives in the selected vertex buffer to the screen. HRESULT Draw. Primitive( D 3 DPRIMITIVETYPE Primitive. Type, UINT Start. Vertex, UINT Primitive. Count);
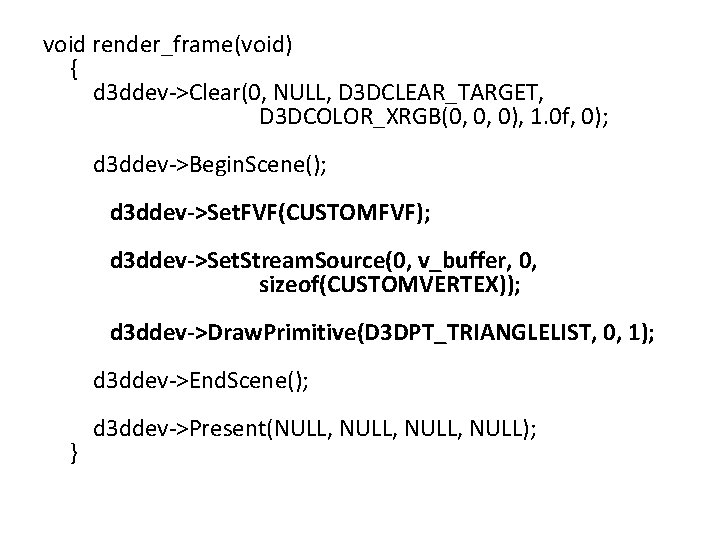
void render_frame(void) { d 3 ddev->Clear(0, NULL, D 3 DCLEAR_TARGET, D 3 DCOLOR_XRGB(0, 0, 0), 1. 0 f, 0); d 3 ddev->Begin. Scene(); d 3 ddev->Set. FVF(CUSTOMFVF); d 3 ddev->Set. Stream. Source(0, v_buffer, 0, sizeof(CUSTOMVERTEX)); d 3 ddev->Draw. Primitive(D 3 DPT_TRIANGLELIST, 0, 1); d 3 ddev->End. Scene(); } d 3 ddev->Present(NULL, NULL);
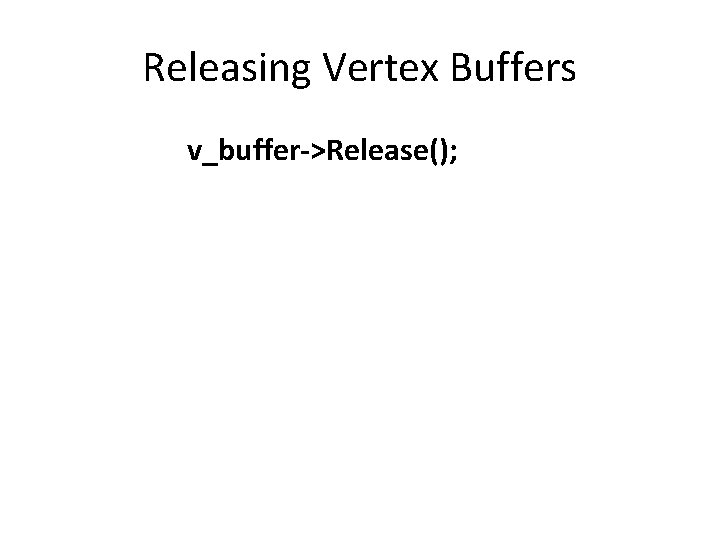
Releasing Vertex Buffers v_buffer->Release();
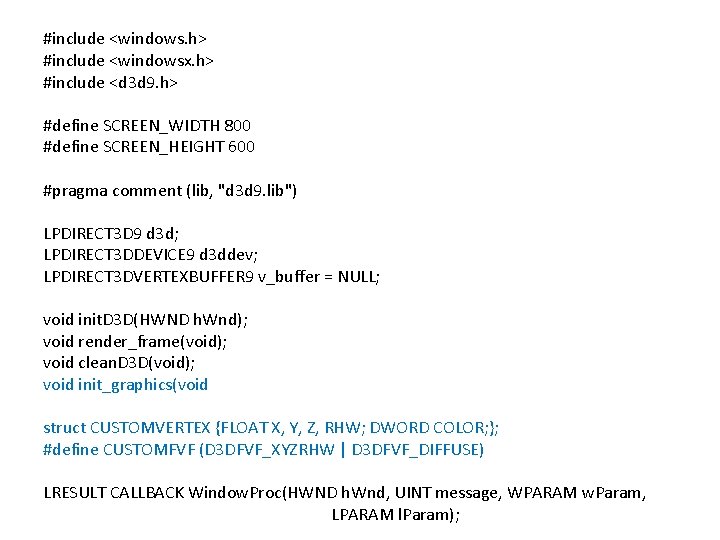
#include <windows. h> #include <windowsx. h> #include <d 3 d 9. h> #define SCREEN_WIDTH 800 #define SCREEN_HEIGHT 600 #pragma comment (lib, "d 3 d 9. lib") LPDIRECT 3 D 9 d 3 d; LPDIRECT 3 DDEVICE 9 d 3 ddev; LPDIRECT 3 DVERTEXBUFFER 9 v_buffer = NULL; void init. D 3 D(HWND h. Wnd); void render_frame(void); void clean. D 3 D(void); void init_graphics(void struct CUSTOMVERTEX {FLOAT X, Y, Z, RHW; DWORD COLOR; }; #define CUSTOMFVF (D 3 DFVF_XYZRHW | D 3 DFVF_DIFFUSE) LRESULT CALLBACK Window. Proc(HWND h. Wnd, UINT message, WPARAM w. Param, LPARAM l. Param);
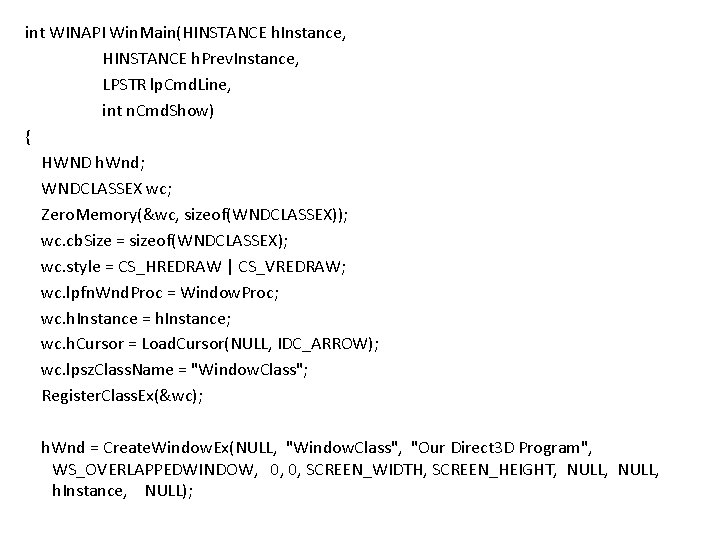
int WINAPI Win. Main(HINSTANCE h. Instance, HINSTANCE h. Prev. Instance, LPSTR lp. Cmd. Line, int n. Cmd. Show) { HWND h. Wnd; WNDCLASSEX wc; Zero. Memory(&wc, sizeof(WNDCLASSEX)); wc. cb. Size = sizeof(WNDCLASSEX); wc. style = CS_HREDRAW | CS_VREDRAW; wc. lpfn. Wnd. Proc = Window. Proc; wc. h. Instance = h. Instance; wc. h. Cursor = Load. Cursor(NULL, IDC_ARROW); wc. lpsz. Class. Name = "Window. Class"; Register. Class. Ex(&wc); h. Wnd = Create. Window. Ex(NULL, "Window. Class", "Our Direct 3 D Program", WS_OVERLAPPEDWINDOW, 0, 0, SCREEN_WIDTH, SCREEN_HEIGHT, NULL, h. Instance, NULL);
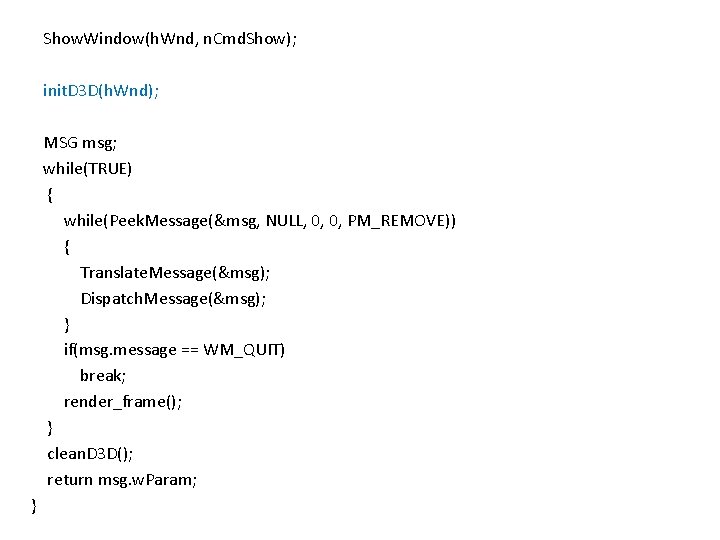
Show. Window(h. Wnd, n. Cmd. Show); init. D 3 D(h. Wnd); MSG msg; while(TRUE) { while(Peek. Message(&msg, NULL, 0, 0, PM_REMOVE)) { Translate. Message(&msg); Dispatch. Message(&msg); } if(msg. message == WM_QUIT) break; render_frame(); } clean. D 3 D(); return msg. w. Param; }
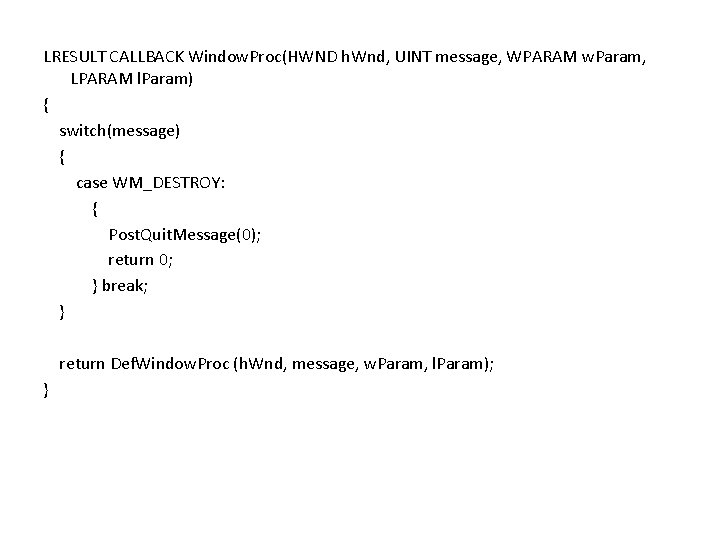
LRESULT CALLBACK Window. Proc(HWND h. Wnd, UINT message, WPARAM w. Param, LPARAM l. Param) { switch(message) { case WM_DESTROY: { Post. Quit. Message(0); return 0; } break; } return Def. Window. Proc (h. Wnd, message, w. Param, l. Param); }
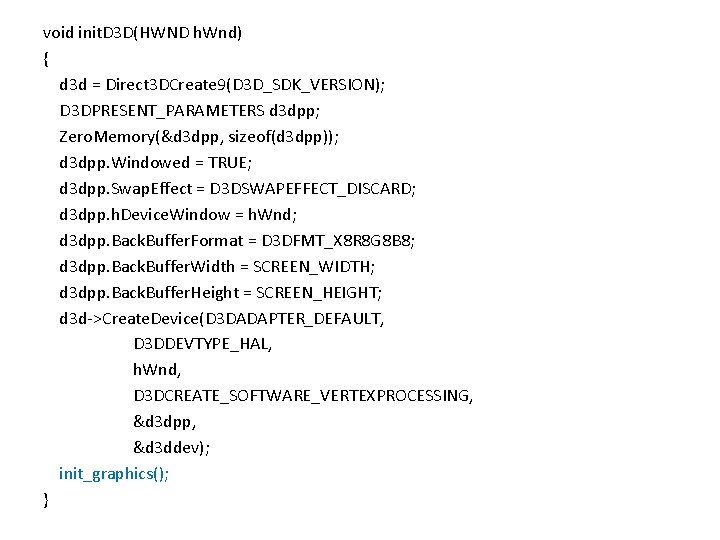
void init. D 3 D(HWND h. Wnd) { d 3 d = Direct 3 DCreate 9(D 3 D_SDK_VERSION); D 3 DPRESENT_PARAMETERS d 3 dpp; Zero. Memory(&d 3 dpp, sizeof(d 3 dpp)); d 3 dpp. Windowed = TRUE; d 3 dpp. Swap. Effect = D 3 DSWAPEFFECT_DISCARD; d 3 dpp. h. Device. Window = h. Wnd; d 3 dpp. Back. Buffer. Format = D 3 DFMT_X 8 R 8 G 8 B 8; d 3 dpp. Back. Buffer. Width = SCREEN_WIDTH; d 3 dpp. Back. Buffer. Height = SCREEN_HEIGHT; d 3 d->Create. Device(D 3 DADAPTER_DEFAULT, D 3 DDEVTYPE_HAL, h. Wnd, D 3 DCREATE_SOFTWARE_VERTEXPROCESSING, &d 3 dpp, &d 3 ddev); init_graphics(); }
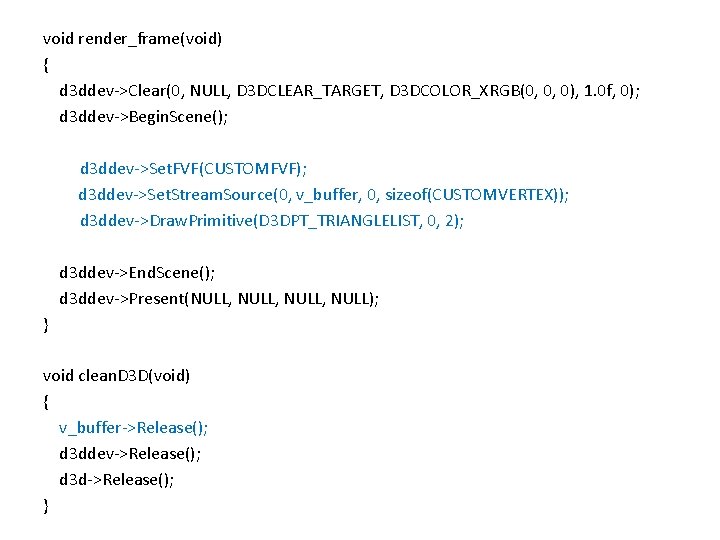
void render_frame(void) { d 3 ddev->Clear(0, NULL, D 3 DCLEAR_TARGET, D 3 DCOLOR_XRGB(0, 0, 0), 1. 0 f, 0); d 3 ddev->Begin. Scene(); d 3 ddev->Set. FVF(CUSTOMFVF); d 3 ddev->Set. Stream. Source(0, v_buffer, 0, sizeof(CUSTOMVERTEX)); d 3 ddev->Draw. Primitive(D 3 DPT_TRIANGLELIST, 0, 2); d 3 ddev->End. Scene(); d 3 ddev->Present(NULL, NULL); } void clean. D 3 D(void) { v_buffer->Release(); d 3 ddev->Release(); d 3 d->Release(); }
![void initgraphicsvoid CUSTOMVERTEX vertices 400 0 f 62 5 f void init_graphics(void) { CUSTOMVERTEX vertices[] = { { 400. 0 f, 62. 5 f,](https://slidetodoc.com/presentation_image_h2/82c8285179401facc01430ca3270895d/image-38.jpg)
void init_graphics(void) { CUSTOMVERTEX vertices[] = { { 400. 0 f, 62. 5 f, 0. 5 f, 1. 0 f, D 3 DCOLOR_XRGB(0, 0, 255), }, { 650. 0 f, 500. 0 f, 0. 5 f, 1. 0 f, D 3 DCOLOR_XRGB(0, 255, 0), }, { 150. 0 f, 500. 0 f, 0. 5 f, 1. 0 f, D 3 DCOLOR_XRGB(255, 0, 0), } }; d 3 ddev->Create. Vertex. Buffer(3*sizeof(CUSTOMVERTEX), 0, CUSTOMFVF, D 3 DPOOL_MANAGED, &v_buffer, NULL); VOID* p. Void; v_buffer->Lock(0, 0, (void**)&p. Void, 0); memcpy(p. Void, vertices, sizeof(vertices)); v_buffer->Unlock(); }
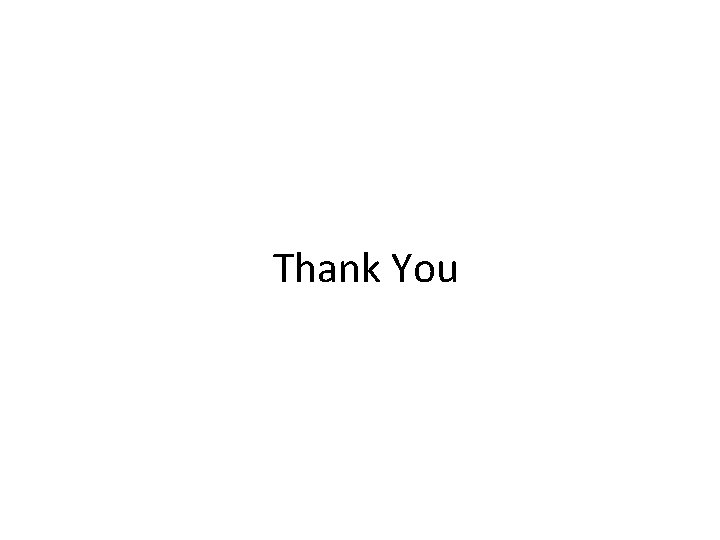
Thank You
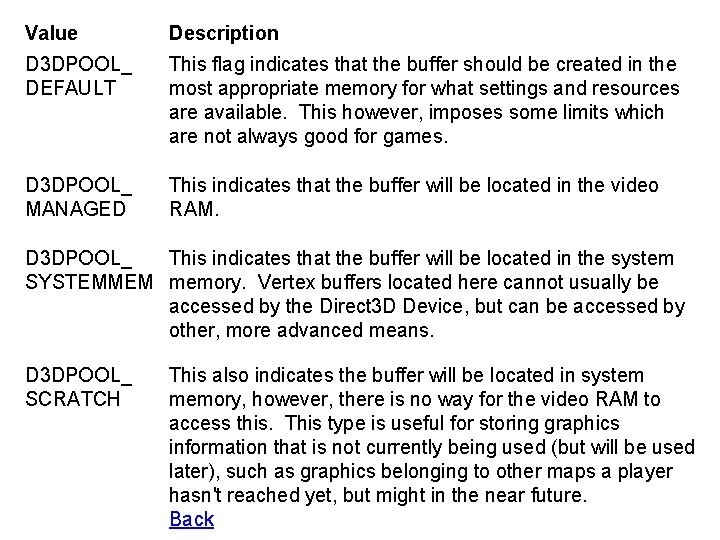
Value Description D 3 DPOOL_ DEFAULT This flag indicates that the buffer should be created in the most appropriate memory for what settings and resources are available. This however, imposes some limits which are not always good for games. D 3 DPOOL_ MANAGED This indicates that the buffer will be located in the video RAM. D 3 DPOOL_ This indicates that the buffer will be located in the system SYSTEMMEM memory. Vertex buffers located here cannot usually be accessed by the Direct 3 D Device, but can be accessed by other, more advanced means. D 3 DPOOL_ SCRATCH This also indicates the buffer will be located in system memory, however, there is no way for the video RAM to access this. This type is useful for storing graphics information that is not currently being used (but will be used later), such as graphics belonging to other maps a player hasn't reached yet, but might in the near future. Back
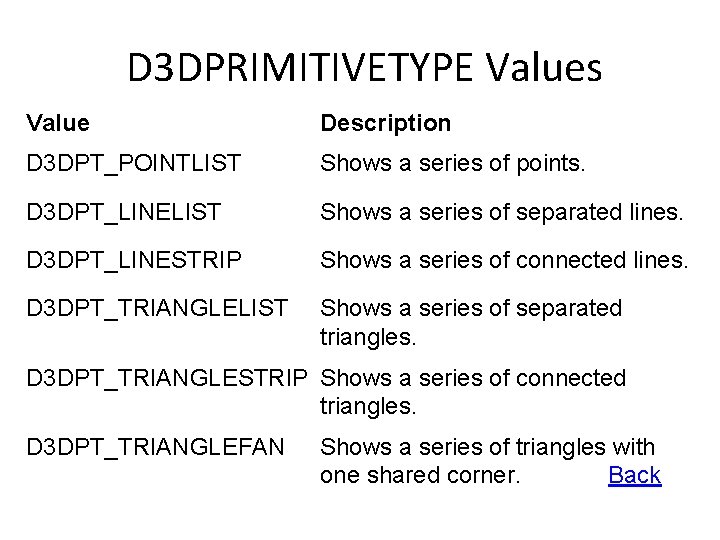
D 3 DPRIMITIVETYPE Values Value Description D 3 DPT_POINTLIST Shows a series of points. D 3 DPT_LINELIST Shows a series of separated lines. D 3 DPT_LINESTRIP Shows a series of connected lines. D 3 DPT_TRIANGLELIST Shows a series of separated triangles. D 3 DPT_TRIANGLESTRIP Shows a series of connected triangles. D 3 DPT_TRIANGLEFAN Shows a series of triangles with one shared corner. Back
Data structure definition
Data structure classification
When was the word technology first used
Primitive drawing
Curve attributes in computer graphics
Convex and concave polygon in computer graphics
Pronombres personales fuertes
Gueorgi khatchatourov
Processing
Buffered vs unbuffered primitives
Thick primitives in computer graphics
Scholarly primitives
Output primitives
Explain about berkeley sockets
Attributes of output primitives in computer graphics
Communication primitives in distributed operating system
Fill area primitives
Cs 4414 cornell
Tableau des dérivées
What is hadoop i/o
Linux synchronization primitives
Raster algorithm
Gl primitives
Berkeley socket primitives
Upward multiplexing
List the primitives that specify a data mining task.
Whats a glut
équations primitives atmosphériques
Tcp connection management finite state machine
Lisp car
Blockinmax
An algorithm is a
Ellipse drawing algorithm in computer graphics ppt
Synchronization primitives c#
Primitive dfd
First organic compound which was formed in primitive ocean
Johann basedow the father of gymnastics
Primitive instructions
Primitive
Appendix embryology
Primitive unit cell
In primitive flow table for gated latch each state has