3 3 Iteration Loops Do Loop Until condition
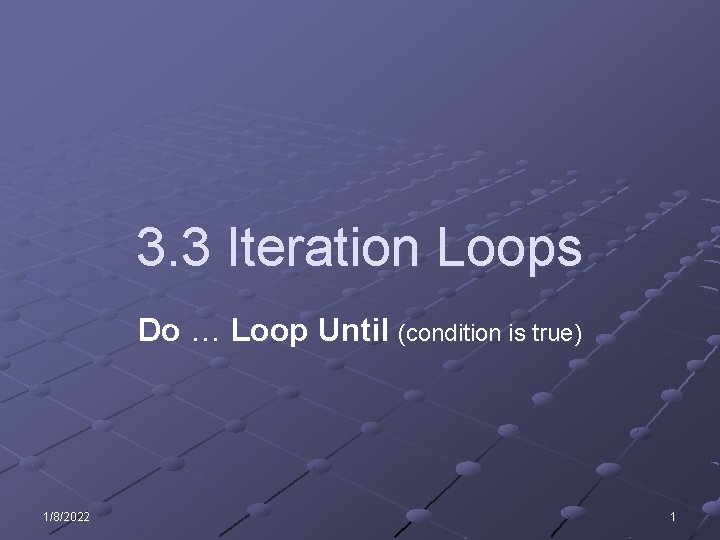
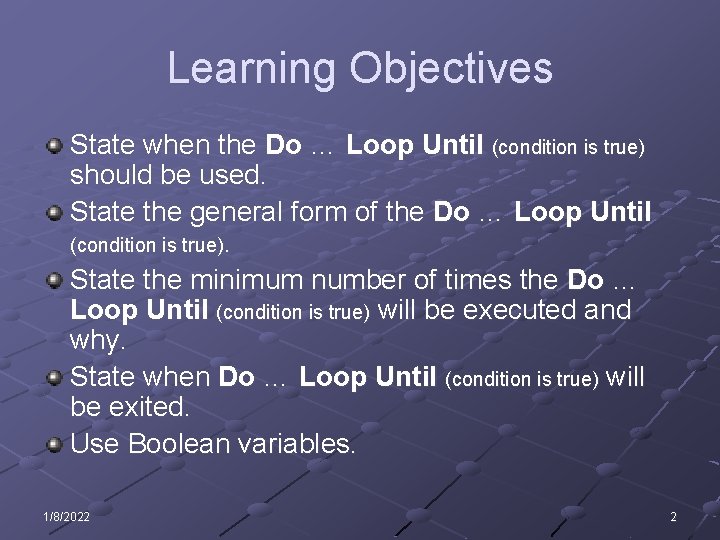
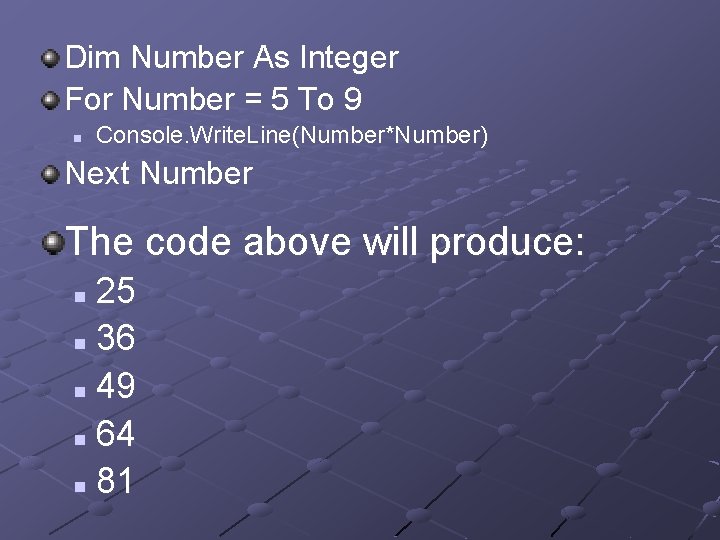
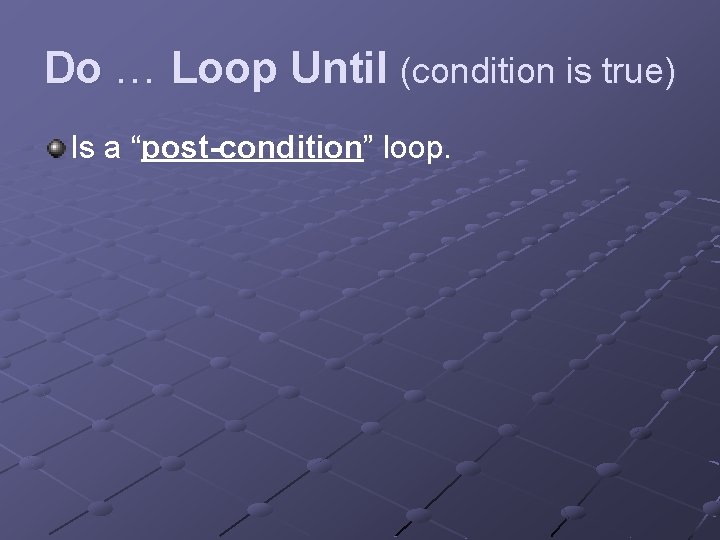
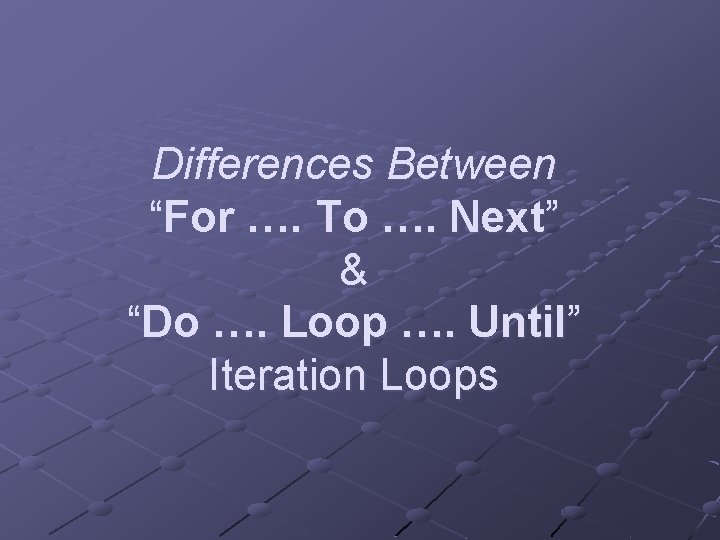
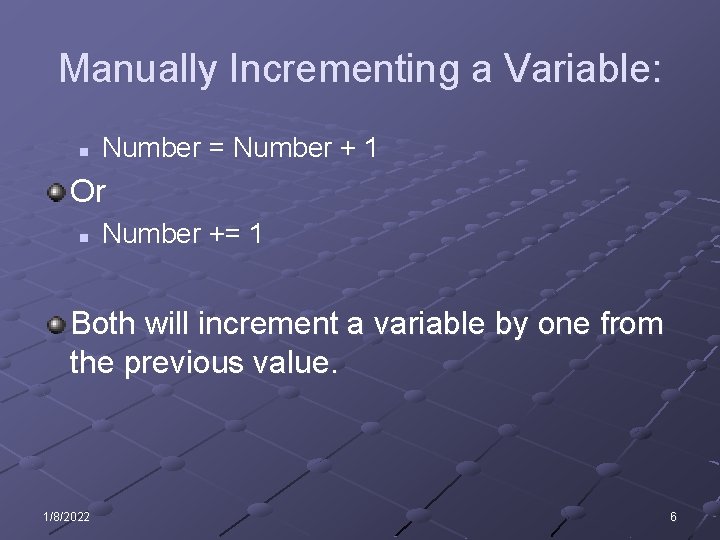
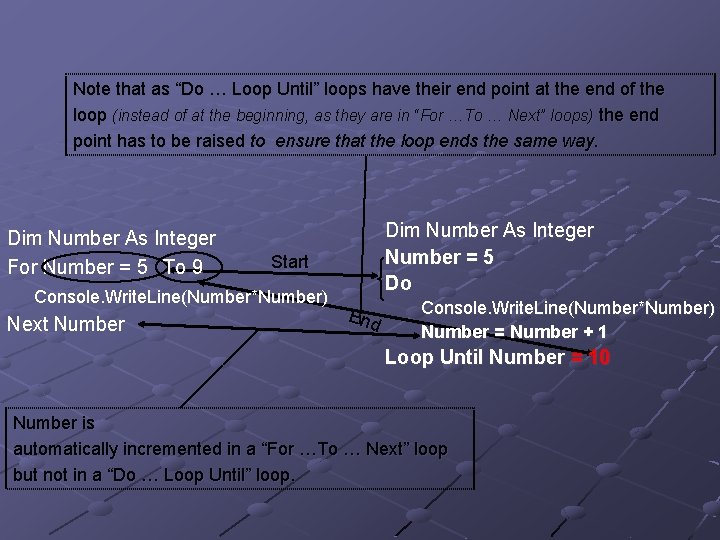
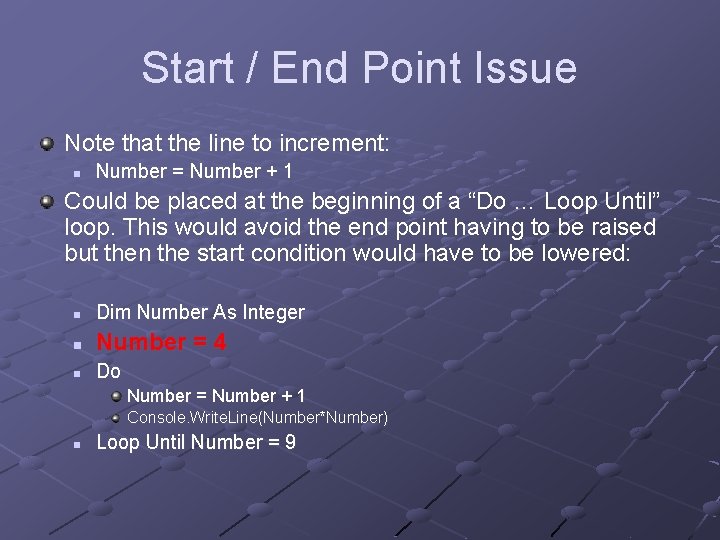
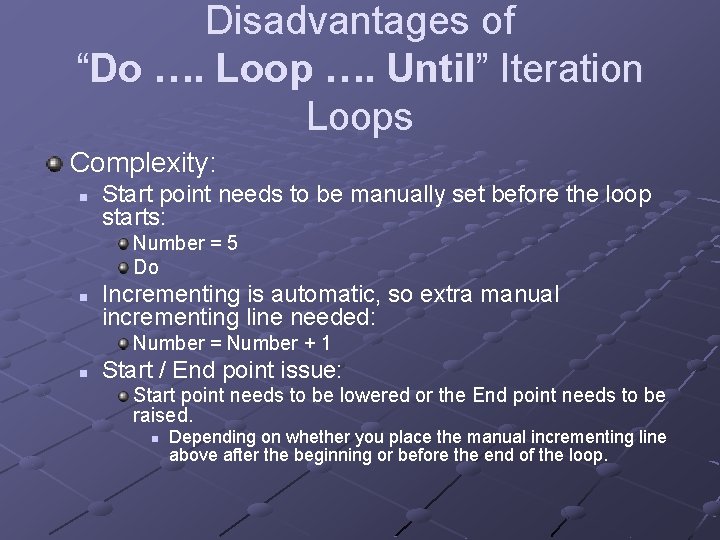
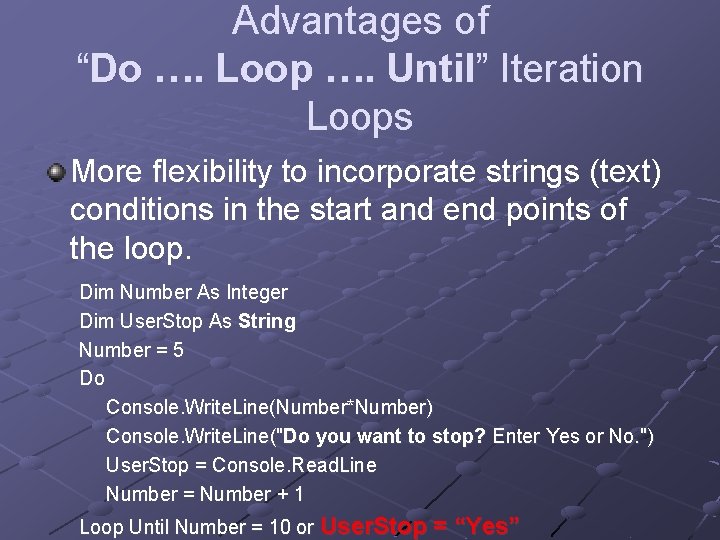
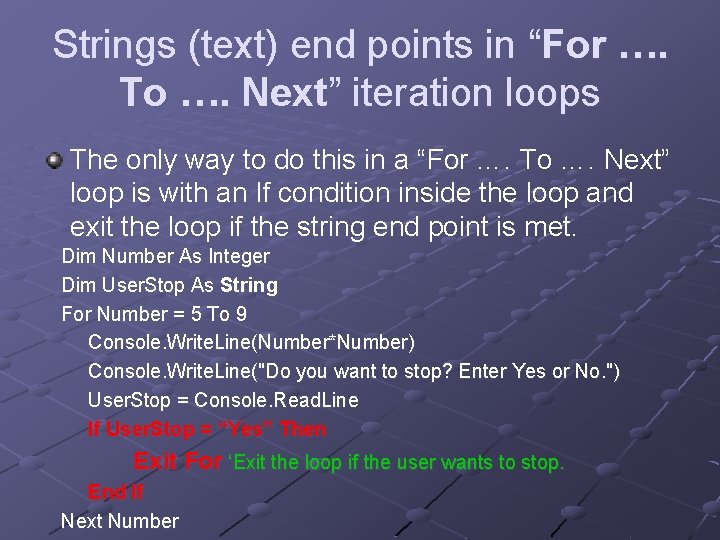
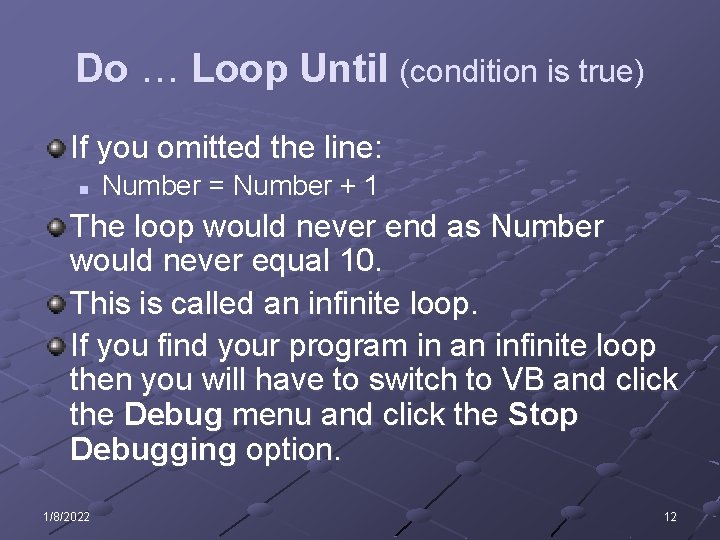
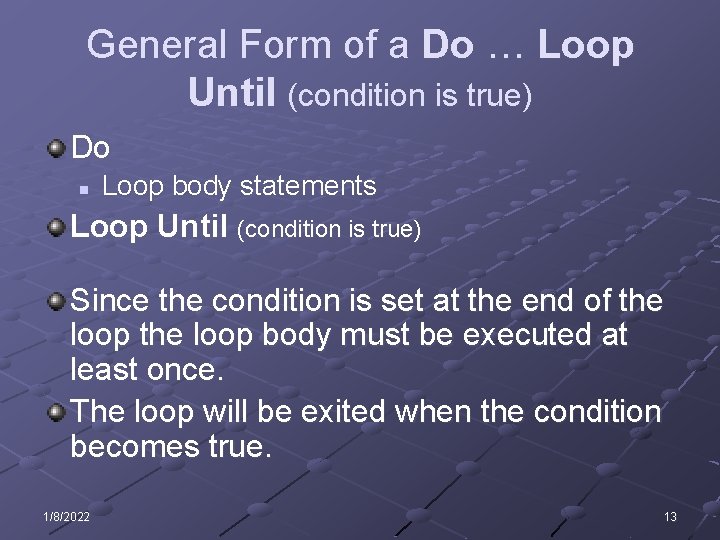
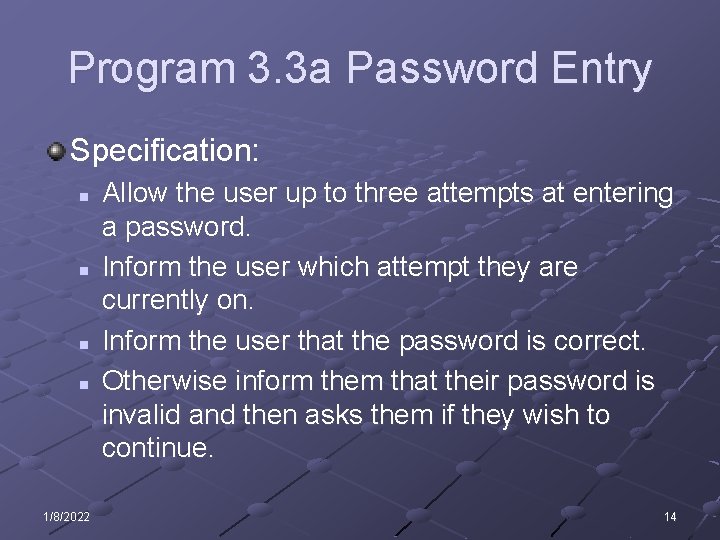
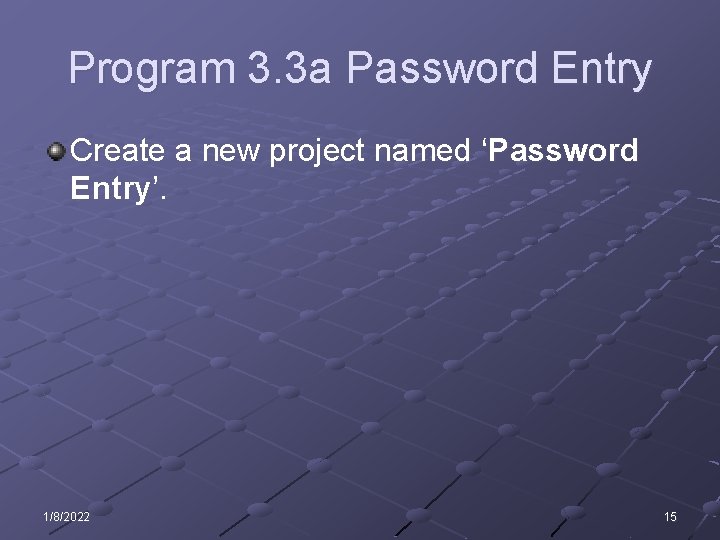
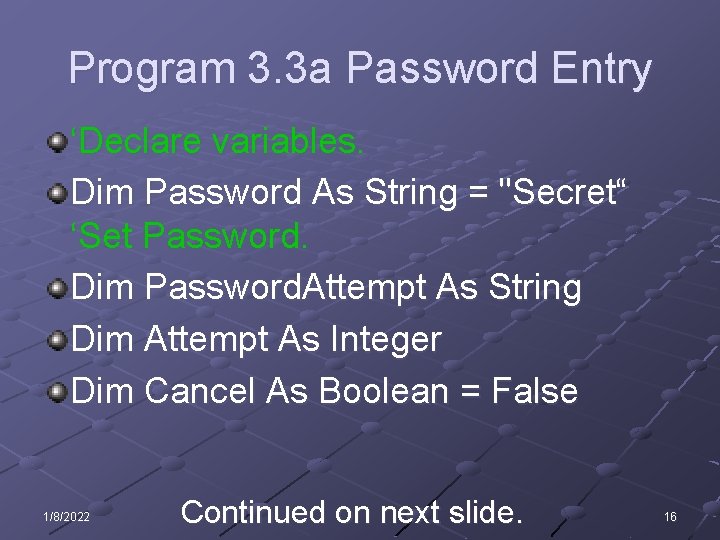
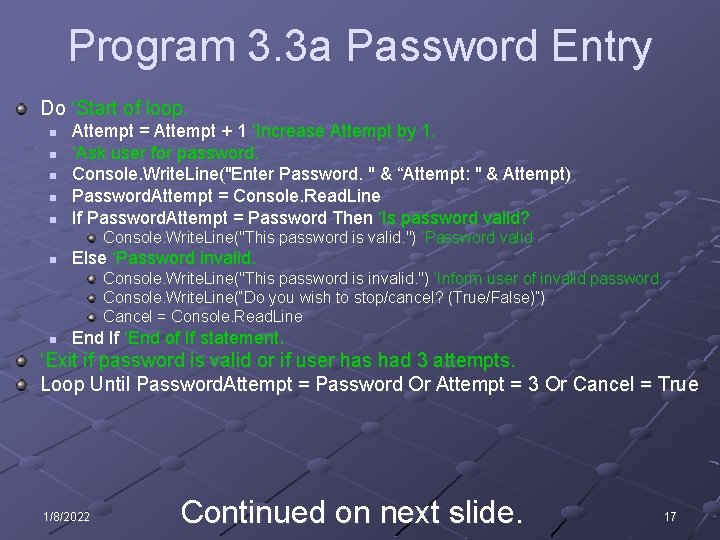
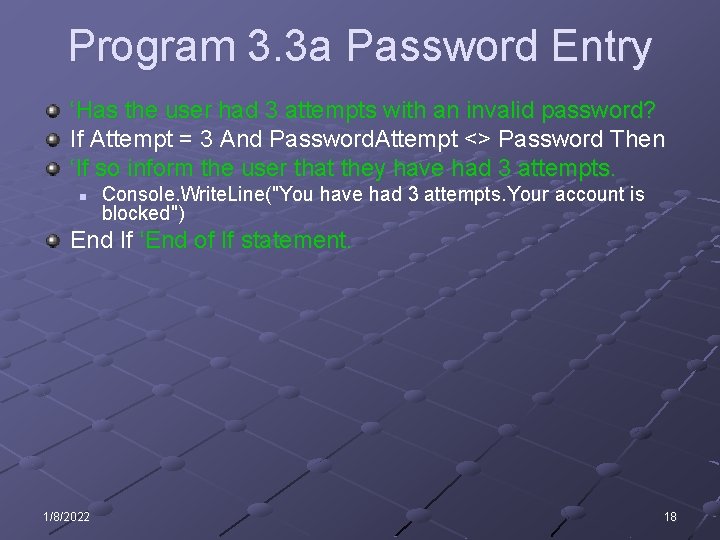
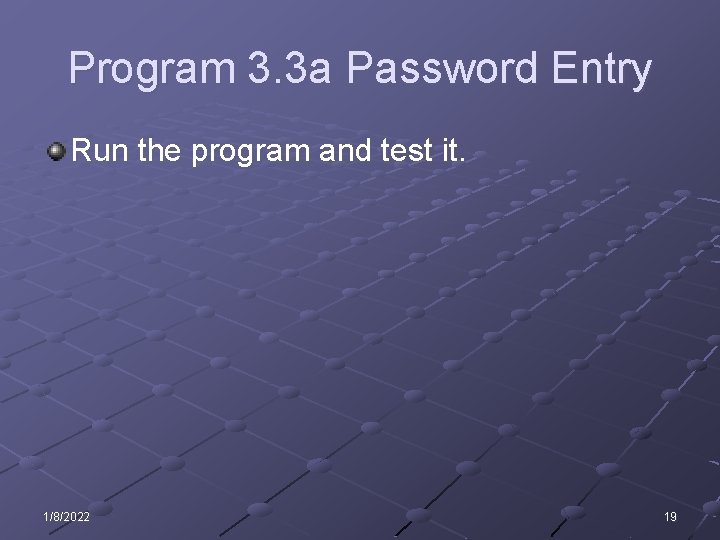
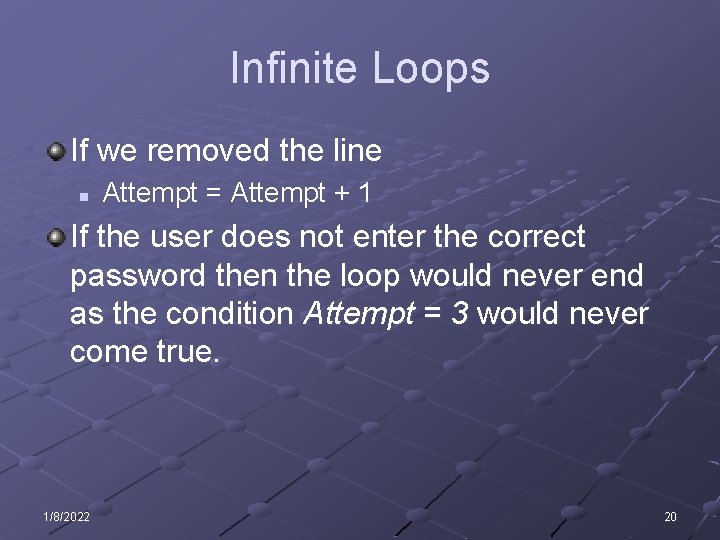
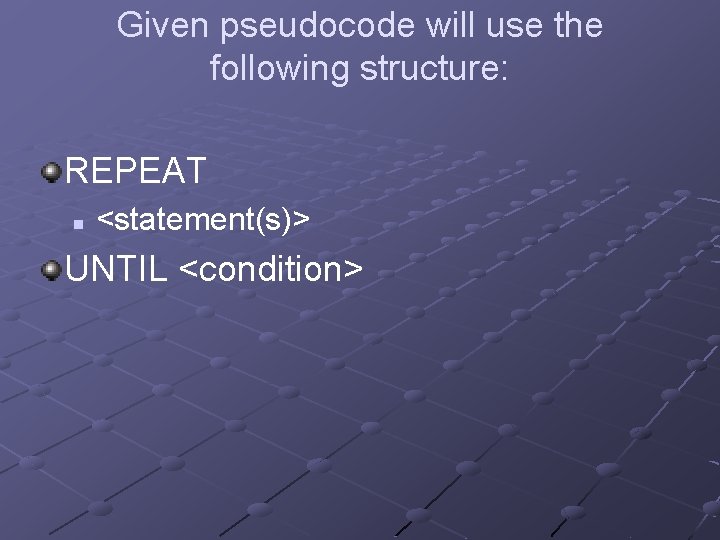
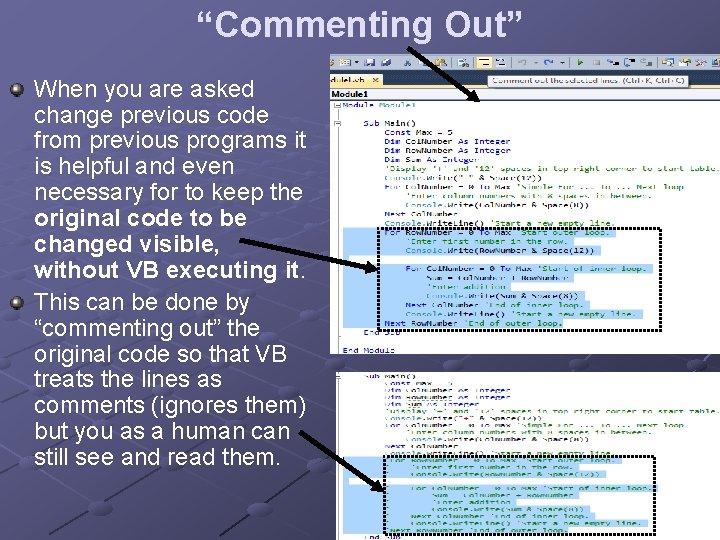
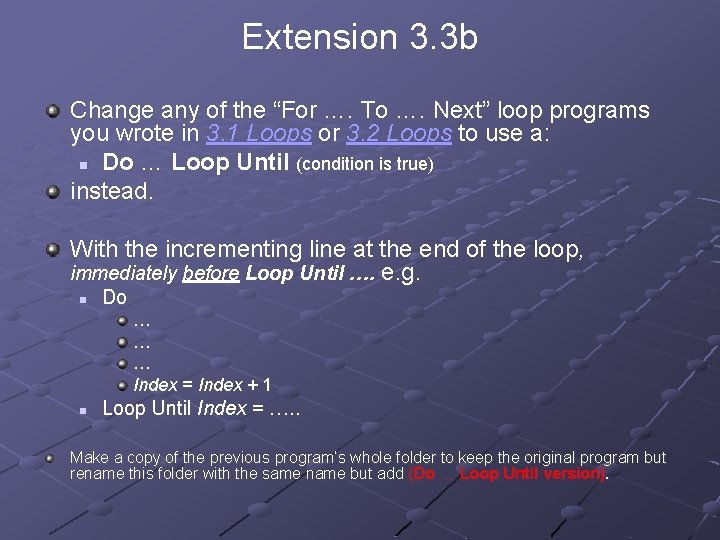
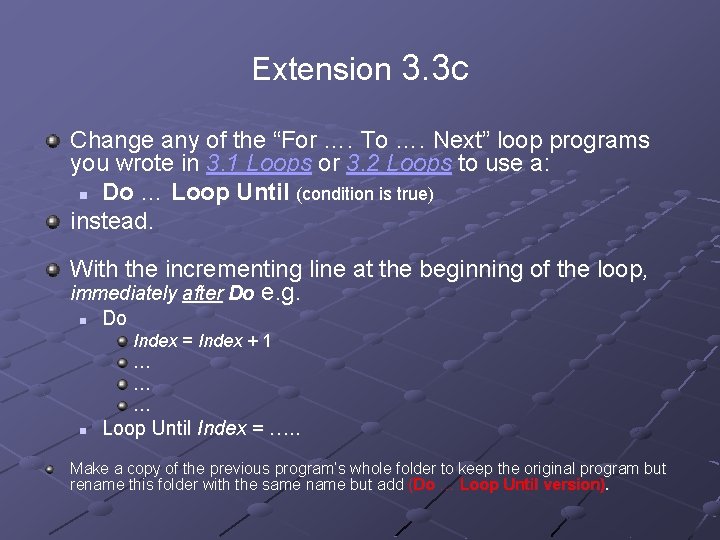
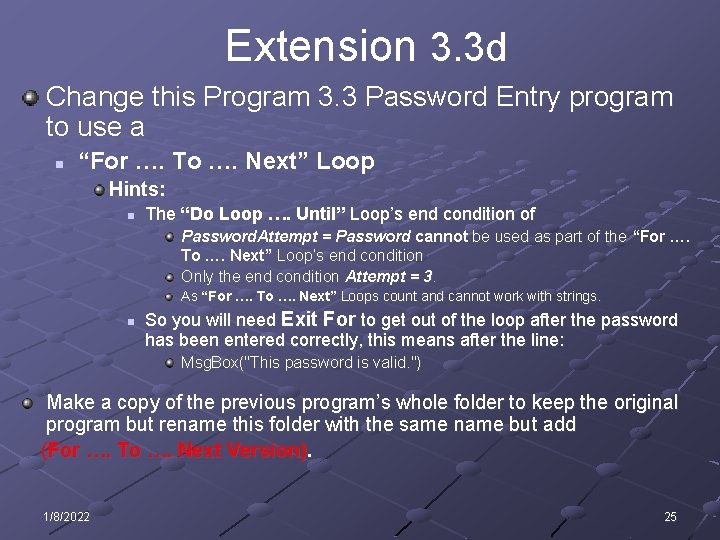
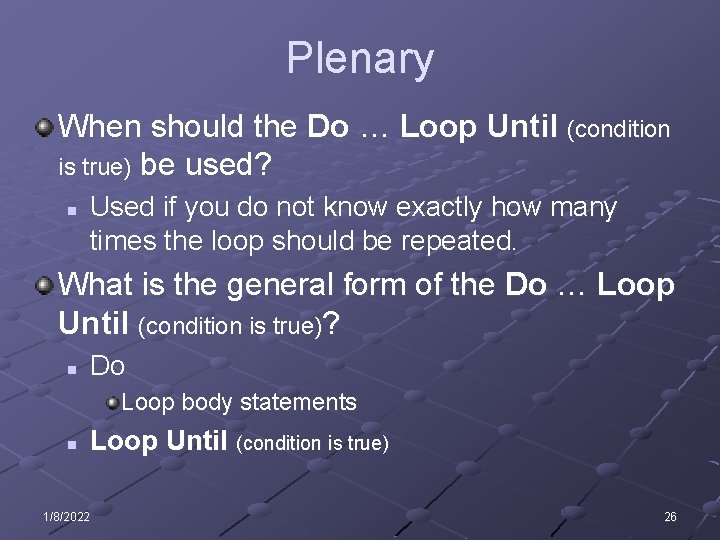
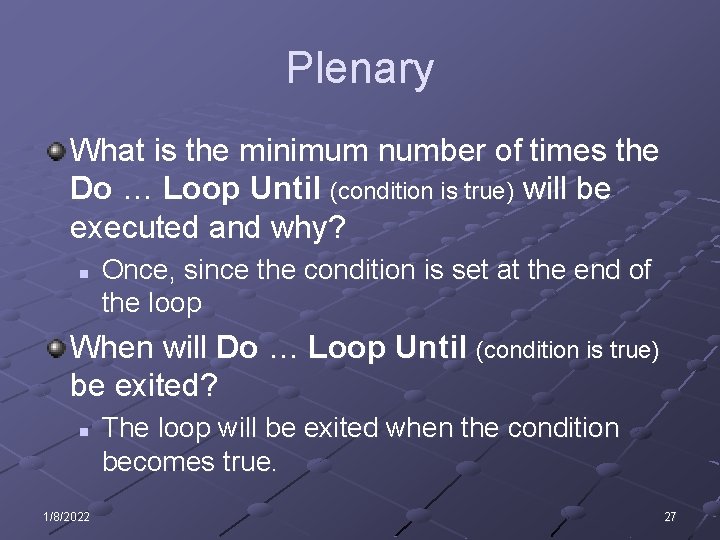
- Slides: 27
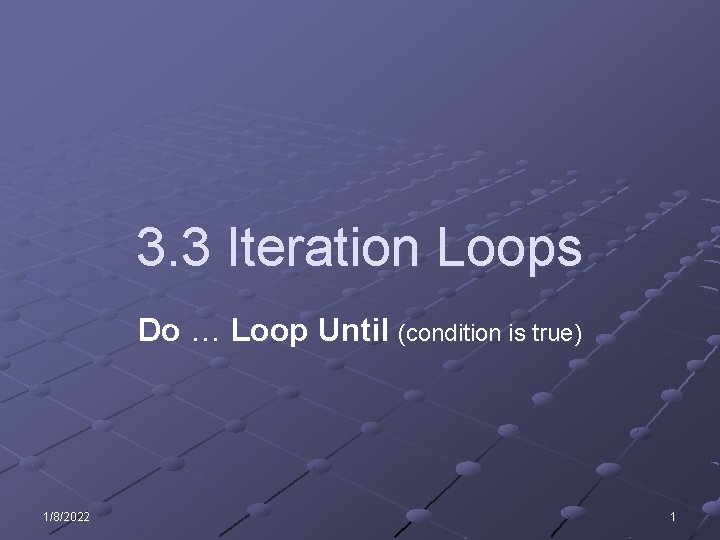
3. 3 Iteration Loops Do … Loop Until (condition is true) 1/8/2022 1
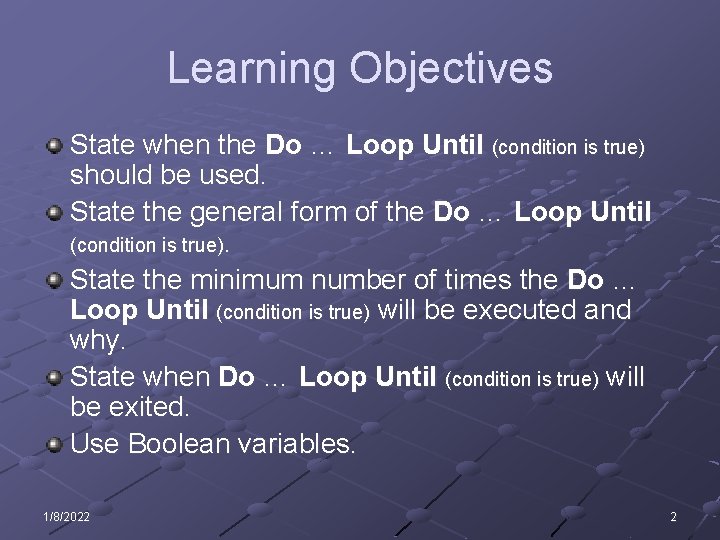
Learning Objectives State when the Do … Loop Until (condition is true) should be used. State the general form of the Do … Loop Until (condition is true). State the minimum number of times the Do … Loop Until (condition is true) will be executed and why. State when Do … Loop Until (condition is true) will be exited. Use Boolean variables. 1/8/2022 2
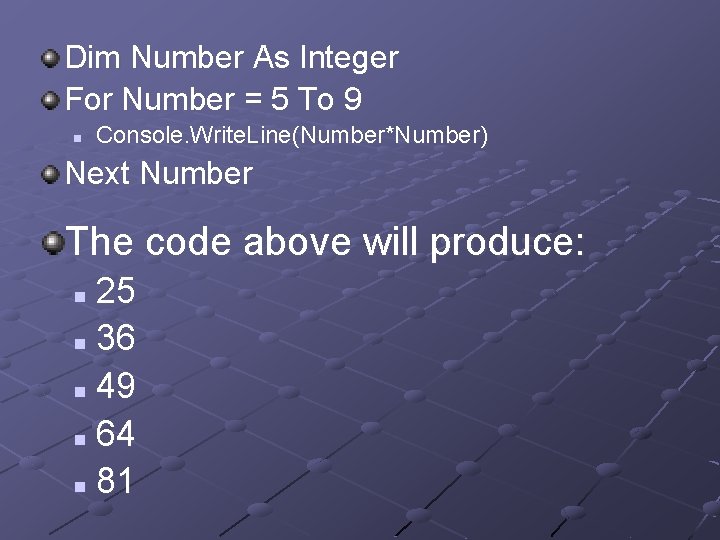
Dim Number As Integer For Number = 5 To 9 n Console. Write. Line(Number*Number) Next Number The code above will produce: 25 n 36 n 49 n 64 n 81 n
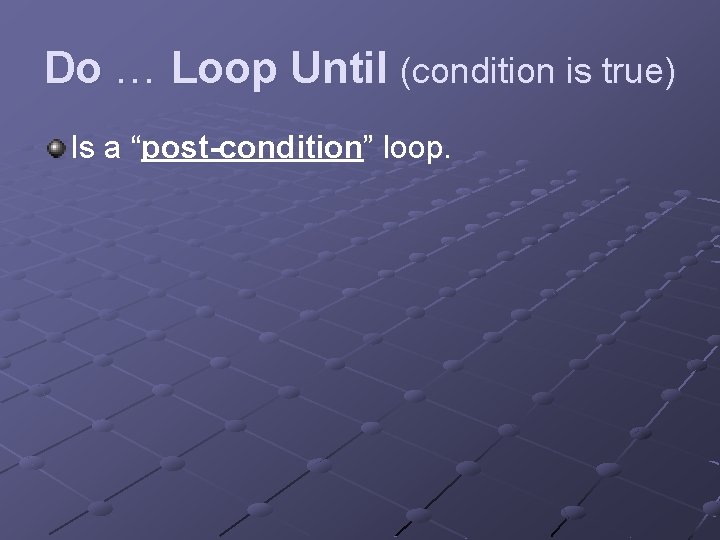
Do … Loop Until (condition is true) Is a “post-condition” loop.
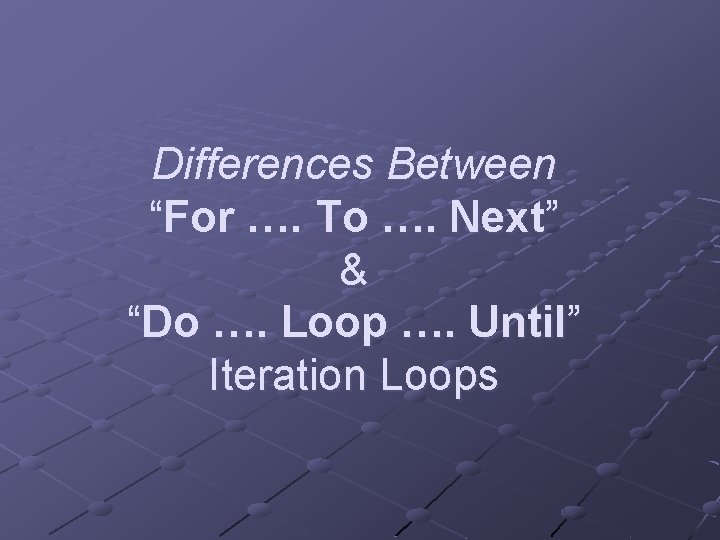
Differences Between “For …. To …. Next” & “Do …. Loop …. Until” Iteration Loops
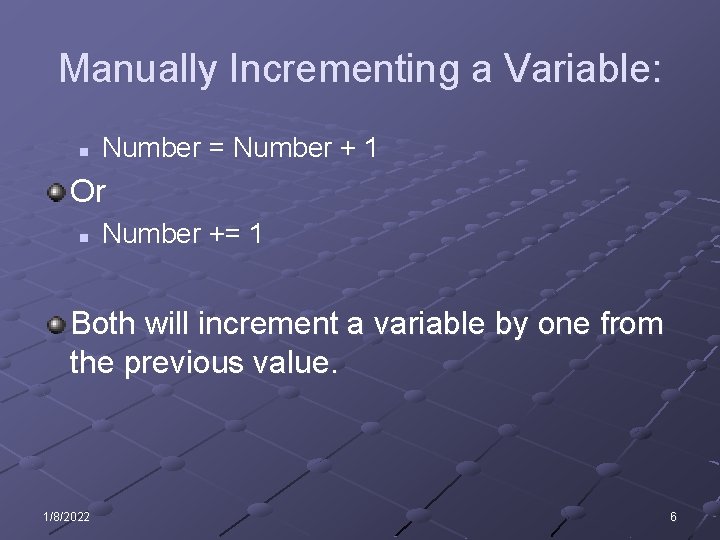
Manually Incrementing a Variable: n Number = Number + 1 Or n Number += 1 Both will increment a variable by one from the previous value. 1/8/2022 6
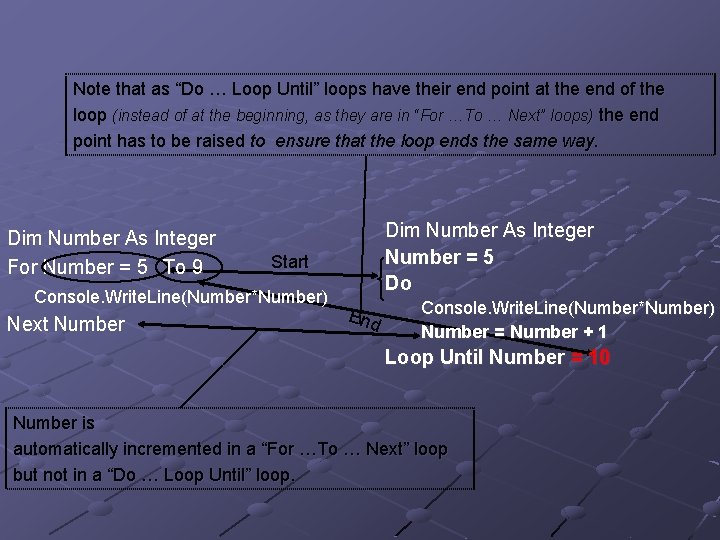
Note that as “Do … Loop Until” loops have their end point at the end of the loop (instead of at the beginning, as they are in “For …To … Next” loops) the end point has to be raised to ensure that the loop ends the same way. Dim Number As Integer For Number = 5 To 9 Start Console. Write. Line(Number*Number) Next Number Dim Number As Integer Number = 5 Do End Console. Write. Line(Number*Number) Number = Number + 1 Loop Until Number = 10 Number is automatically incremented in a “For …To … Next” loop but not in a “Do … Loop Until” loop.
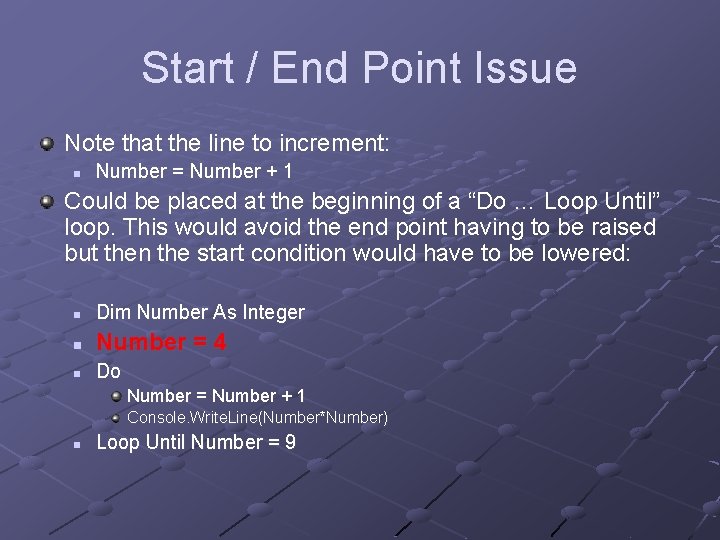
Start / End Point Issue Note that the line to increment: n Number = Number + 1 Could be placed at the beginning of a “Do … Loop Until” loop. This would avoid the end point having to be raised but then the start condition would have to be lowered: n Dim Number As Integer n Number = 4 n Do Number = Number + 1 Console. Write. Line(Number*Number) n Loop Until Number = 9
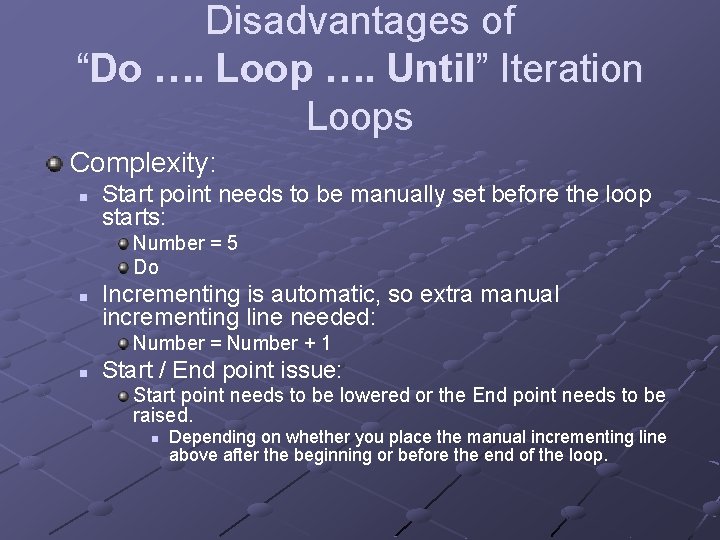
Disadvantages of “Do …. Loop …. Until” Iteration Loops Complexity: n Start point needs to be manually set before the loop starts: Number = 5 Do n Incrementing is automatic, so extra manual incrementing line needed: Number = Number + 1 n Start / End point issue: Start point needs to be lowered or the End point needs to be raised. n Depending on whether you place the manual incrementing line above after the beginning or before the end of the loop.
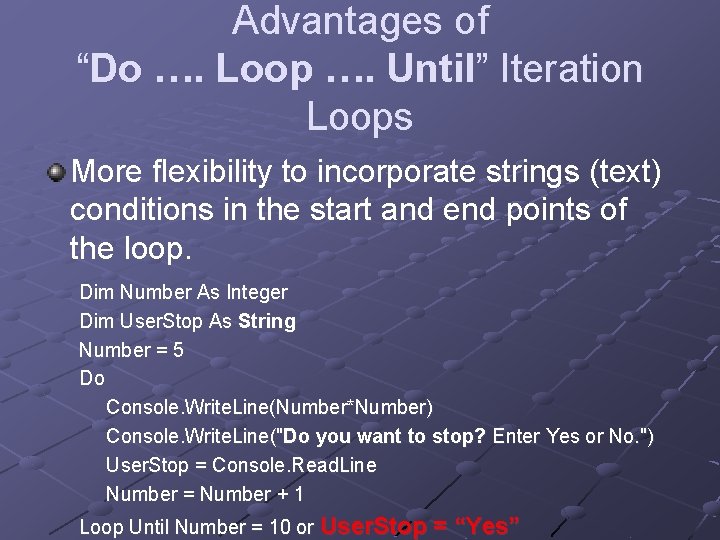
Advantages of “Do …. Loop …. Until” Iteration Loops More flexibility to incorporate strings (text) conditions in the start and end points of the loop. Dim Number As Integer Dim User. Stop As String Number = 5 Do Console. Write. Line(Number*Number) Console. Write. Line("Do you want to stop? Enter Yes or No. ") User. Stop = Console. Read. Line Number = Number + 1 Loop Until Number = 10 or User. Stop = “Yes”
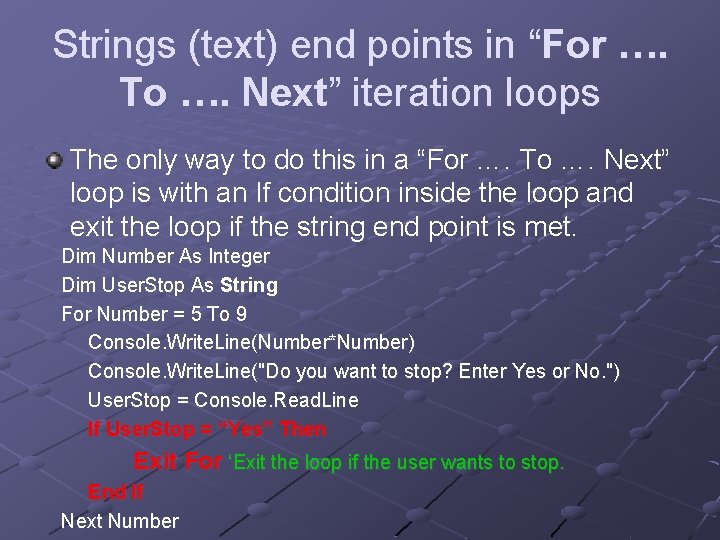
Strings (text) end points in “For …. To …. Next” iteration loops The only way to do this in a “For …. To …. Next” loop is with an If condition inside the loop and exit the loop if the string end point is met. Dim Number As Integer Dim User. Stop As String For Number = 5 To 9 Console. Write. Line(Number*Number) Console. Write. Line("Do you want to stop? Enter Yes or No. ") User. Stop = Console. Read. Line If User. Stop = “Yes” Then Exit For ‘Exit the loop if the user wants to stop. End If Next Number
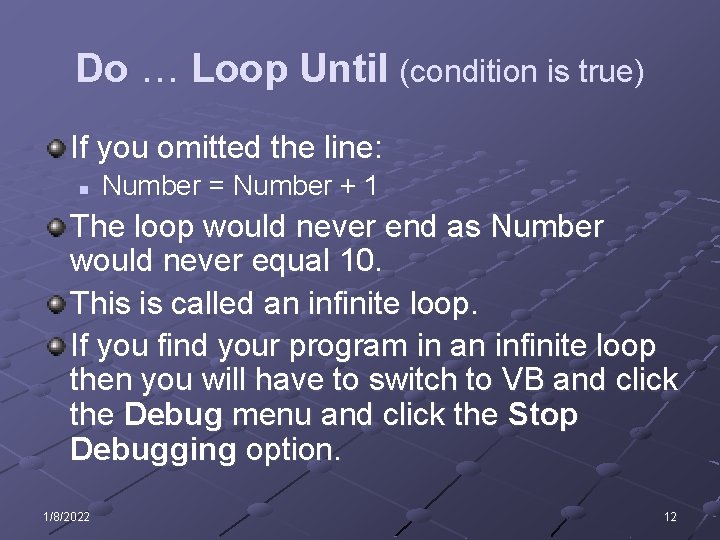
Do … Loop Until (condition is true) If you omitted the line: n Number = Number + 1 The loop would never end as Number would never equal 10. This is called an infinite loop. If you find your program in an infinite loop then you will have to switch to VB and click the Debug menu and click the Stop Debugging option. 1/8/2022 12
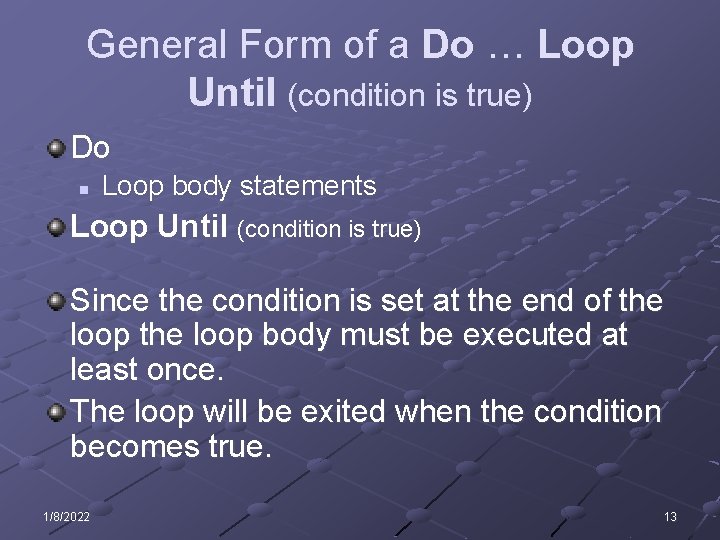
General Form of a Do … Loop Until (condition is true) Do n Loop body statements Loop Until (condition is true) Since the condition is set at the end of the loop body must be executed at least once. The loop will be exited when the condition becomes true. 1/8/2022 13
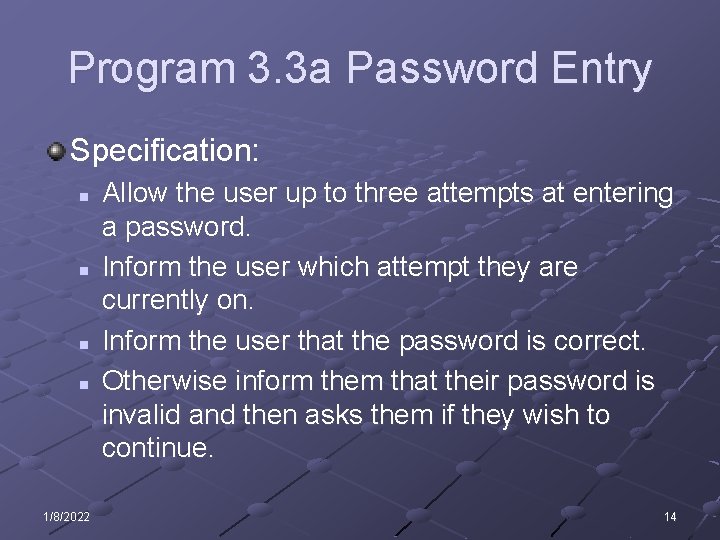
Program 3. 3 a Password Entry Specification: n n 1/8/2022 Allow the user up to three attempts at entering a password. Inform the user which attempt they are currently on. Inform the user that the password is correct. Otherwise inform them that their password is invalid and then asks them if they wish to continue. 14
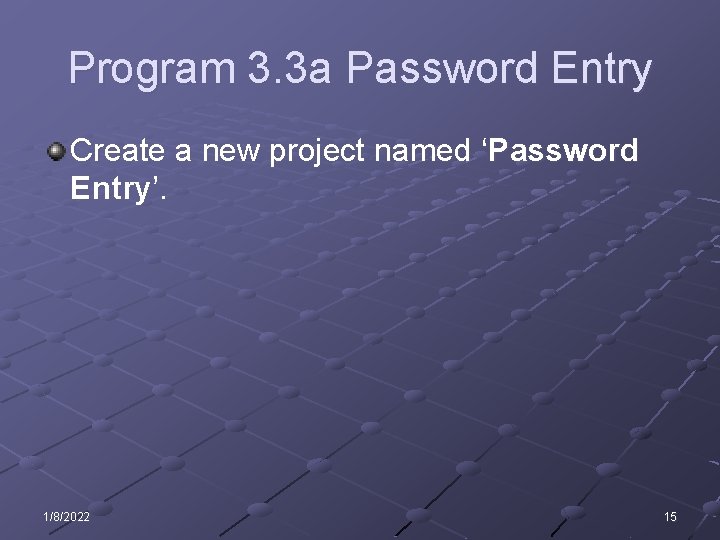
Program 3. 3 a Password Entry Create a new project named ‘Password Entry’. 1/8/2022 15
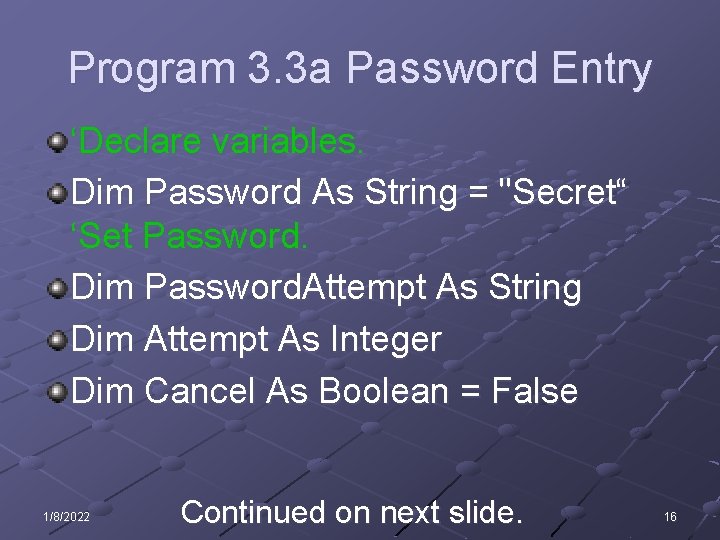
Program 3. 3 a Password Entry ‘Declare variables. Dim Password As String = "Secret“ ‘Set Password. Dim Password. Attempt As String Dim Attempt As Integer Dim Cancel As Boolean = False 1/8/2022 Continued on next slide. 16
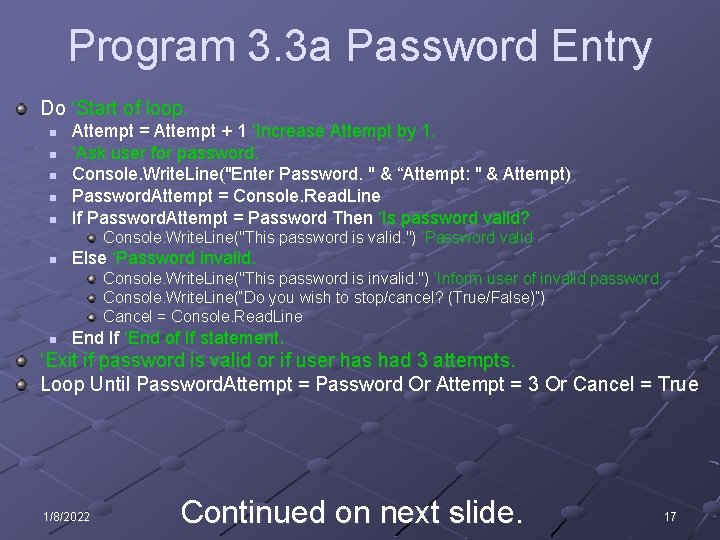
Program 3. 3 a Password Entry Do ‘Start of loop. n n n Attempt = Attempt + 1 ‘Increase Attempt by 1. ‘Ask user for password. Console. Write. Line("Enter Password. " & “Attempt: " & Attempt) Password. Attempt = Console. Read. Line If Password. Attempt = Password Then ‘Is password valid? Console. Write. Line("This password is valid. ") ‘Password valid. n Else ‘Password invalid. Console. Write. Line("This password is invalid. ") ‘Inform user of invalid password. Console. Write. Line(“Do you wish to stop/cancel? (True/False)”) Cancel = Console. Read. Line n End If ‘End of If statement. ‘Exit if password is valid or if user has had 3 attempts. Loop Until Password. Attempt = Password Or Attempt = 3 Or Cancel = True 1/8/2022 Continued on next slide. 17
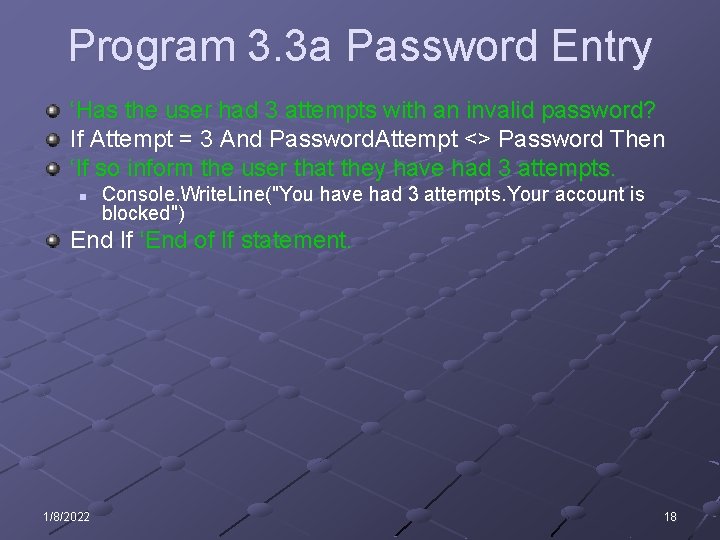
Program 3. 3 a Password Entry ‘Has the user had 3 attempts with an invalid password? If Attempt = 3 And Password. Attempt <> Password Then ‘If so inform the user that they have had 3 attempts. n Console. Write. Line("You have had 3 attempts. Your account is blocked") End If ‘End of If statement. 1/8/2022 18
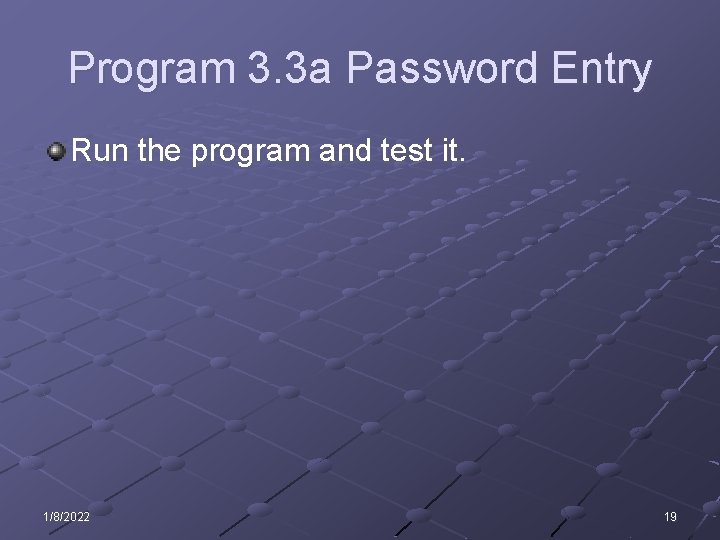
Program 3. 3 a Password Entry Run the program and test it. 1/8/2022 19
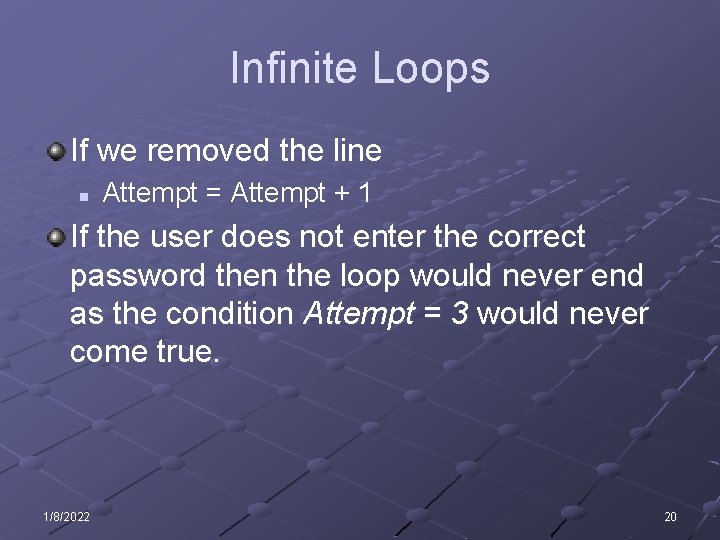
Infinite Loops If we removed the line n Attempt = Attempt + 1 If the user does not enter the correct password then the loop would never end as the condition Attempt = 3 would never come true. 1/8/2022 20
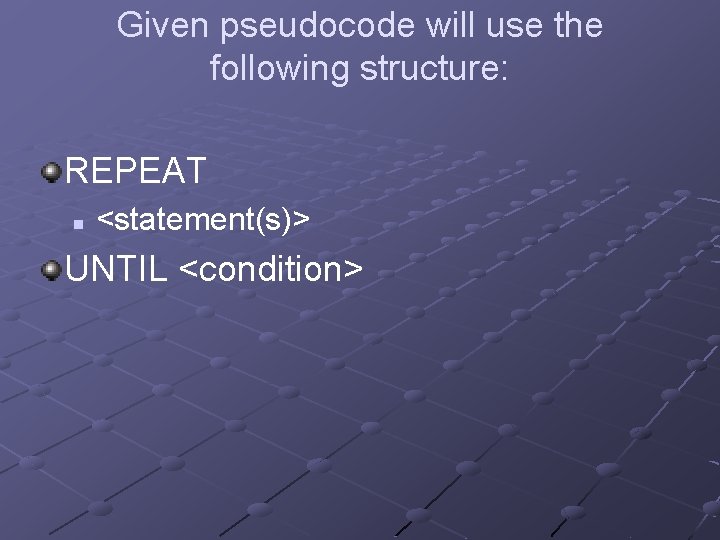
Given pseudocode will use the following structure: REPEAT n <statement(s)> UNTIL <condition>
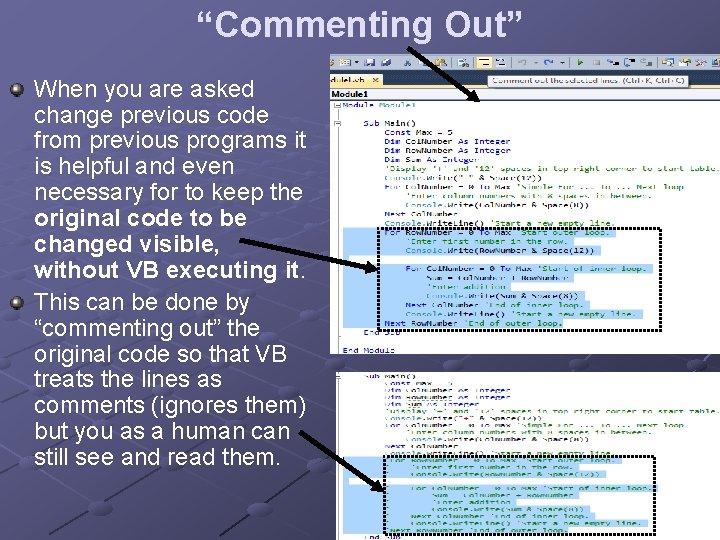
“Commenting Out” When you are asked change previous code from previous programs it is helpful and even necessary for to keep the original code to be changed visible, without VB executing it. This can be done by “commenting out” the original code so that VB treats the lines as comments (ignores them) but you as a human can still see and read them.
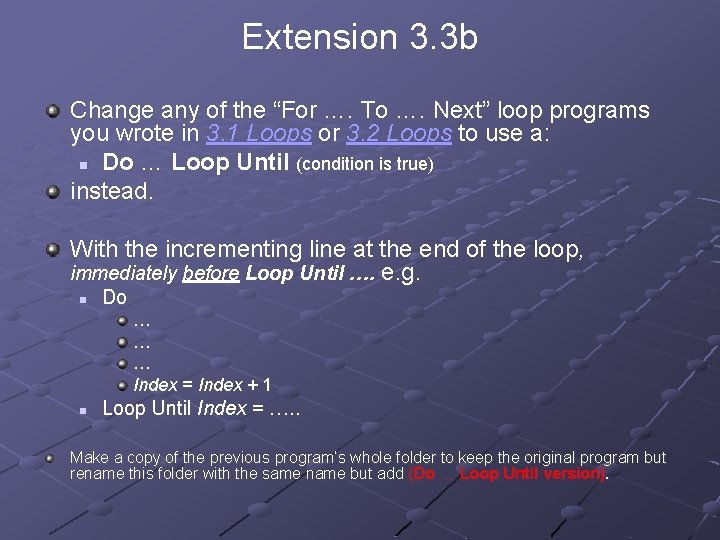
Extension 3. 3 b Change any of the “For …. To …. Next” loop programs you wrote in 3. 1 Loops or 3. 2 Loops to use a: n Do … Loop Until (condition is true) instead. With the incrementing line at the end of the loop, immediately before Loop Until …. e. g. n Do … … … Index = Index + 1 n Loop Until Index = …. . Make a copy of the previous program’s whole folder to keep the original program but rename this folder with the same name but add (Do … Loop Until version).
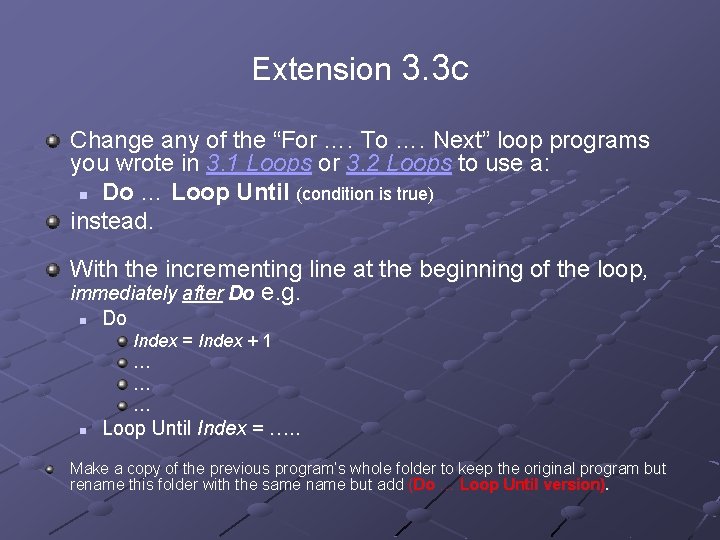
Extension 3. 3 c Change any of the “For …. To …. Next” loop programs you wrote in 3. 1 Loops or 3. 2 Loops to use a: n Do … Loop Until (condition is true) instead. With the incrementing line at the beginning of the loop, immediately after Do e. g. n Do Index = Index + 1 … … … n Loop Until Index = …. . Make a copy of the previous program’s whole folder to keep the original program but rename this folder with the same name but add (Do … Loop Until version).
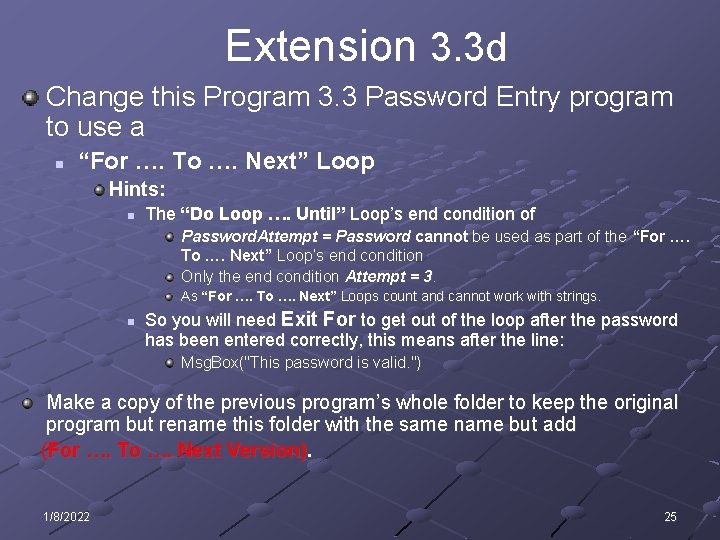
Extension 3. 3 d Change this Program 3. 3 Password Entry program to use a n “For …. To …. Next” Loop Hints: n The “Do Loop …. Until” Loop’s end condition of Password. Attempt = Password cannot be used as part of the “For …. To …. Next” Loop’s end condition Only the end condition Attempt = 3. As “For …. To …. Next” Loops count and cannot work with strings. n So you will need Exit For to get out of the loop after the password has been entered correctly, this means after the line: Msg. Box("This password is valid. ") Make a copy of the previous program’s whole folder to keep the original program but rename this folder with the same name but add (For …. To …. Next Version). 1/8/2022 25
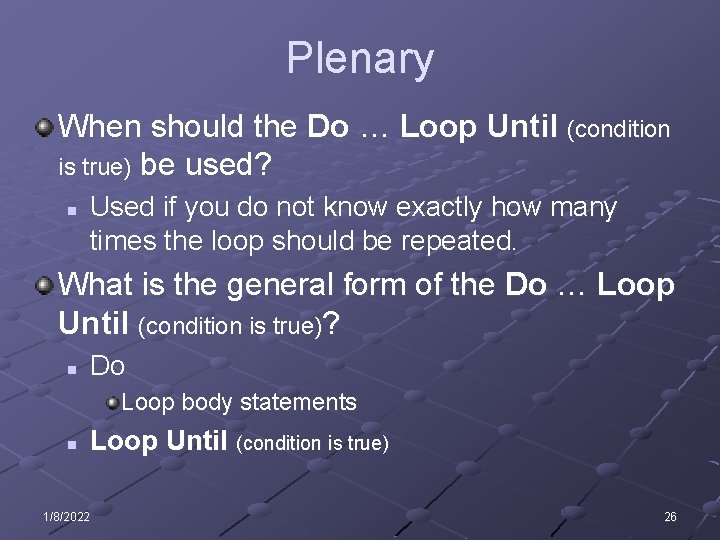
Plenary When should the Do … Loop Until (condition is true) be used? n Used if you do not know exactly how many times the loop should be repeated. What is the general form of the Do … Loop Until (condition is true)? n Do Loop body statements n Loop Until (condition is true) 1/8/2022 26
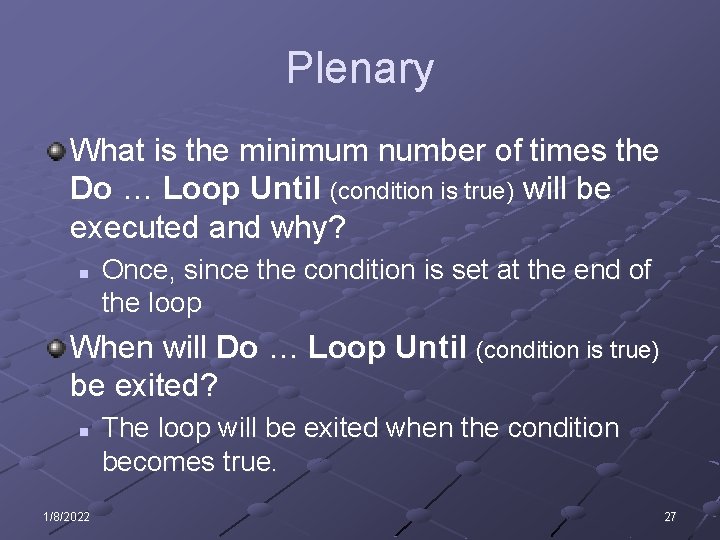
Plenary What is the minimum number of times the Do … Loop Until (condition is true) will be executed and why? n Once, since the condition is set at the end of the loop When will Do … Loop Until (condition is true) be exited? n 1/8/2022 The loop will be exited when the condition becomes true. 27